Nullish coalescing operator '??'
The nullish coalescing operator is written as two question marks ?? .
As it treats null and undefined similarly, we’ll use a special term here, in this article. For brevity, we’ll say that a value is “defined” when it’s neither null nor undefined .
The result of a ?? b is:
- if a is defined, then a ,
- if a isn’t defined, then b .
In other words, ?? returns the first argument if it’s not null/undefined . Otherwise, the second one.
The nullish coalescing operator isn’t anything completely new. It’s just a nice syntax to get the first “defined” value of the two.
We can rewrite result = a ?? b using the operators that we already know, like this:
Now it should be absolutely clear what ?? does. Let’s see where it helps.
The common use case for ?? is to provide a default value.
For example, here we show user if its value isn’t null/undefined , otherwise Anonymous :
Here’s the example with user assigned to a name:
We can also use a sequence of ?? to select the first value from a list that isn’t null/undefined .
Let’s say we have a user’s data in variables firstName , lastName or nickName . All of them may be not defined, if the user decided not to fill in the corresponding values.
We’d like to display the user name using one of these variables, or show “Anonymous” if all of them are null/undefined .
Let’s use the ?? operator for that:
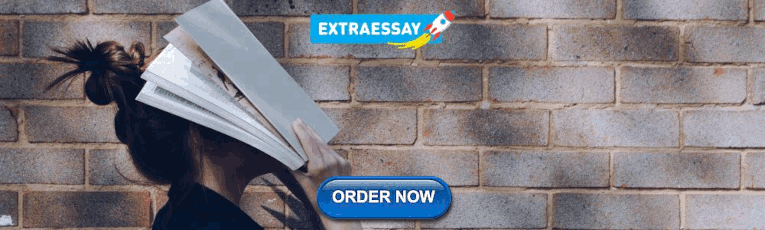
Comparison with ||
The OR || operator can be used in the same way as ?? , as it was described in the previous chapter .
For example, in the code above we could replace ?? with || and still get the same result:
Historically, the OR || operator was there first. It’s been there since the beginning of JavaScript, so developers were using it for such purposes for a long time.
On the other hand, the nullish coalescing operator ?? was added to JavaScript only recently, and the reason for that was that people weren’t quite happy with || .
The important difference between them is that:
- || returns the first truthy value.
- ?? returns the first defined value.
In other words, || doesn’t distinguish between false , 0 , an empty string "" and null/undefined . They are all the same – falsy values. If any of these is the first argument of || , then we’ll get the second argument as the result.
In practice though, we may want to use default value only when the variable is null/undefined . That is, when the value is really unknown/not set.
For example, consider this:
- so the result of || is the second argument, 100 .
- so the result is height “as is”, that is 0 .
In practice, the zero height is often a valid value, that shouldn’t be replaced with the default. So ?? does just the right thing.
The precedence of the ?? operator is the same as || . They both equal 3 in the MDN table .
That means that, just like || , the nullish coalescing operator ?? is evaluated before = and ? , but after most other operations, such as + , * .
So we may need to add parentheses in expressions like this:
Otherwise, if we omit parentheses, then as * has the higher precedence than ?? , it would execute first, leading to incorrect results.
Using ?? with && or ||
Due to safety reasons, JavaScript forbids using ?? together with && and || operators, unless the precedence is explicitly specified with parentheses.
The code below triggers a syntax error:
The limitation is surely debatable, it was added to the language specification with the purpose to avoid programming mistakes, when people start to switch from || to ?? .
Use explicit parentheses to work around it:
The nullish coalescing operator ?? provides a short way to choose the first “defined” value from a list.
It’s used to assign default values to variables:
The operator ?? has a very low precedence, only a bit higher than ? and = , so consider adding parentheses when using it in an expression.
It’s forbidden to use it with || or && without explicit parentheses.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
The Nullish Coalescing Operator ?? in JavaScript
The nullish coalescing operator provides a concise syntax for setting a default value if the given value is nullish . null and undefined are the only nullish values in JavaScript.
Nullish coalescing is typically used for assigning default values. Older JavaScript code often used || for assigning default values, but || is error prone because it captures all falsy values , like 0 , empty string, and false .
Nullish Coalescing Assignment
The nullish coalescing assignment operator is similar to the nullish coalescing operator, just for assignments. Nullish coalescing assignment only assigns the value if the left hand side is nullish. x ??= y is equivalent to x = x ?? y .
Pre-ES2020 Alternative
The nullish coalescing operator was introduced in ES2020 , which makes it a relatively new addition to the JavaScript language. For example, the nullish coalescing operator isn't supported in Node.js 12. However, there is a concise alternative with the ternary operator that works in any version of JavaScript.
More Fundamentals Tutorials
- JavaScript Array flatMap()
- How to Get Distinct Values in a JavaScript Array
- Check if a Date is Valid in JavaScript
- Encode base64 in JavaScript
- Check if URL Contains a String
- JavaScript Add Month to Date
- JavaScript Add Days to Date
- Web Development
What Is the JavaScript Logical Nullish Assignment Operator?
- Daniyal Hamid
- 24 Dec, 2021
Introduced in ES12, you can use the logical nullish assignment operator ( ??= ) to assign a value to a variable if it hasn't been set already. Consider the following examples, which are all equivalent:
The null coalescing assignment operator only assigns the default value if x is a nullish value (i.e. undefined or null ). For example:
In the following example, x already has a value, therefore, the logical nullish assignment operator would not set a default (i.e. it won't return the value on the right side of the assignment operator):
In case, you use the nullish assignment operator on an undeclared variable, the following ReferenceError is thrown:
This post was published 24 Dec, 2021 by Daniyal Hamid . Daniyal currently works as the Head of Engineering in Germany and has 20+ years of experience in software engineering, design and marketing. Please show your love and support by sharing this post .
Home » An Essential Guide to JavaScript null
An Essential Guide to JavaScript null
Summary : in this tutorial, you’ll learn about the JavaScript null and how to handle it effectively.
What is null in JavaScript
JavaScript null is a primitive type that contains a special value null .
JavaScript uses the null value to represent the intentional absence of any object value .
If you find a variable or a function that returns null , it means that the expected object couldn’t be created.
The following example defines the Circle class whose constructor accepts an argument radius . The Circle class has a static method called create() that returns a new Circle object with a specified radius.
This creates a new Circle object with the radius 10 :
However, the following example returns null because the radius argument isn’t passed into the create() method:
Check if a value is null
To check if a value is null , you use the strict equality operator === like this:
For example:
The rect === null evaluates to true because the rect variable is assigned to a null value. On the other hand, the square === null evaluates to false because it’s assigned to an object.
To check if a value is not null , you use the strict inequality operator ( !== ):
JavaScript null features
JavaScript null has the following features.
1) null is falsy
Besides false , 0 , an empty string ( '' ), undefined , NaN , null is a falsy value. It means that JavaScript will coerce null to false in conditionals. For example:
In this example, the square variable is null therefore the if statement evaluates it to false and executes the statement in the else clause.
2) typeof null is object
The typeof value returns the type of the value. For example:
Surprisingly, the typeof null returns 'object' :
In JavaScript, null is a primitive value, not an object. It turns out that this is a historical bug from the first version of JavaScript that may never be fixed.
A common JavaScript null mistake
In JavaScript, you often call a function to get an object. And then you access the property of that object.
However, if the function returns null instead of an object, you’ll get a TypeError . For example:
In this example, we select an element with id save and access its classList property.
If the page doesn’t have any element with the id save , the document.querySelector('#save') returns null . Hence, accessing the className property of the null value results in an error:
To avoid this, you can use the optional chaining operator ( ?. )
The optional chaining operator returns undefined instead of throwing an error when you attempt to access a property of a null (or undefined ) value.
The following example uses the optional chaining operator that returns undefined instead of an error:
JavaScript null vs. undefined
Both null and undefined are primitive values.
The undefined is the value of an uninitialized vairable or object property.
For example, when you declare a variable without initializing a value, the variable evaluates to undefined :
The null represents an intentional absence of an object while the undefined represents an unintentional absence of a value .
In other words, the null represents a missing object while the undefined represents an uninitialized variable.
The null and undefined are equal when you use the loose equality operator ( == ):
The strict equality operator === differentiates the null and undefined . For example:
- Javascript null is a primitive type that has one value null.
- JavaScript uses the null value to represent a missing object.
- Use the strict equality operator ( === ) to check if a value is null .
- The typeof null returns 'object' , which is historical bug in JavaScript that may never be fixed.
- Use the optional chaining operator ( ?. ) to access the property of an object that may be null .
JS Check for Null – Null Checking in JavaScript Explained

Null is a primitive type in JavaScript. This means you are supposed to be able to check if a variable is null with the typeof() method. But unfortunately, this returns “object” because of an historical bug that cannot be fixed.
So how can you now check for null? This article will teach you how to check for null, along with the difference between the JavaScript type null and undefined.
Null vs Undefined in JavaScript
Null and undefined are very similar in JavaScript and are both primitive types.
A variable has the type of null if it intentionally contains the value of null . In contrast, a variable has the type of undefined when you declare it without initiating a value.
Undefined works well because when you check the type using the typeof() method, it will return undefined :
Let’s now see the two major ways you can check for null and how it relates to undefined .
How to Check for Null in JavaScript with Equality Operators
The equality operators provide the best way to check for null . You can either use the loose/double equality operator ( == ) or the strict/triple equality operator ( === ).
How to use the loose equality operator to check for null
You can use the loose equality operator to check for null values:
But, this can be tricky because if the variable is undefined, it will also return true because both null and undefined are loosely equal.
Note: This can be useful when you want to check if a variable has no value because when a variable has no value, it can either be null or undefined .
But suppose you only want to check for null – then you can use the strict equality operator.
How to use the strict equality operator to check for null
The strict equality operator, compared to the loose equality operator, will only return true when you have exactly a null value. Otherwise, it will return false (this includes undefined ).
As you can see, it only returns true when a null variable is compared with null , and an undefined variable is compared with undefined .
How to Check for Null in JavaScript with the Object.is() Method
Object.is() is an ES6 method that determines whether two values are the same. This works like the strict equality operator.
Let’s make use of the previous example to see if it works like the strict equality operator:
This happens because it only returns true when both values are the same. This means that it will only return true when a variable set to null is compared with null , and an undefined variable is compared with undefined .
Now you know how to check for null with confidence. You can also check whether a variable is set to null or undefined , and you know the difference between the loose and strict equality operators.
I hope this was helpful. Have fun coding!
Frontend Developer & Technical Writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JS Arithmetic Operators
- Addition(+) Arithmetic Operator in JavaScript
- Subtraction(-) Arithmetic Operator in JavaScript
- Multiplication(*) Arithmetic Operator in JavaScript
- Division(/) Arithmetic Operator in JavaScript
- Modulus(%) Arithmetic Operator in JavaScript
- Exponentiation(**) Arithmetic Operator in JavaScript
- Increment(+ +) Arithmetic Operator in JavaScript
- Decrement(--) Arithmetic Operator in JavaScript
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Arithmetic Unary Negation(-) Operator
JS Assignment Operators
- Addition Assignment (+=) Operator in Javascript
- Subtraction Assignment( -=) Operator in Javascript
- Multiplication Assignment(*=) Operator in JavaScript
- Division Assignment(/=) Operator in JavaScript
- JavaScript Remainder Assignment(%=) Operator
- Exponentiation Assignment(**=) Operator in JavaScript
- Left Shift Assignment (<<=) Operator in JavaScript
- Right Shift Assignment(>>=) Operator in JavaScript
- Bitwise AND Assignment (&=) Operator in JavaScript
- Bitwise OR Assignment (|=) Operator in JavaScript
- Bitwise XOR Assignment (^=) Operator in JavaScript
- JavaScript Logical AND assignment (&&=) Operator
- JavaScript Logical OR assignment (||=) Operator
Nullish Coalescing Assignment (??=) Operator in JavaScript
Js comparison operators.
- Equality(==) Comparison Operator in JavaScript
- Inequality(!=) Comparison Operator in JavaScript
- Strict Equality(===) Comparison Operator in JavaScript
- Strict Inequality(!==) Comparison Operator in JavaScript
- Greater than(>) Comparison Operator in JavaScript
- Greater Than or Equal(>=) Comparison Operator in JavaScript
- Less Than or Equal(
JS Logical Operators
- NOT(!) Logical Operator inJavaScript
- AND(&&) Logical Operator in JavaScript
- OR(||) Logical Operator in JavaScript
JS Bitwise Operators
- AND(&) Bitwise Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- XOR(^) Bitwise Operator in JavaScript
- NOT(~) Bitwise Operator in JavaScript
- Left Shift (
- Right Shift (>>) Bitwise Operator in JavaScript
- Zero Fill Right Shift (>>>) Bitwise Operator in JavaScript
JS Unary Operators
- JavaScript typeof Operator
- JavaScript delete Operator
JS Relational Operators
- JavaScript in Operator
- JavaScript Instanceof Operator
JS Other Operators
- JavaScript String Operators
- JavaScript yield Operator
- JavaScript Pipeline Operator
- JavaScript Grouping Operator
This is a new operator introduced by javascript. This operator is represented by x ??= y and it is called Logical nullish assignment operator. Only if the value of x is nullish then the value of y will be assigned to x that means if the value of x is null or undefined then the value of y will be assigned to x.
Let’s discuss how this logical nullish assignment operator works. Firstly we all know that logical nullish assignment is represented as x ??= y , this is derived by two operators nullish coalescing operator and assignment operator we can also write it as x ?? (x = y) . Now javascript checks the x first, if it is nullish then the value of y will be assigned to x .
Example 1 :
Example 2 :
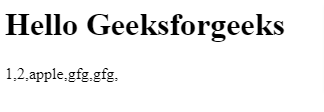
Supported browsers
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript typeof Operator
JavaScript Booleans
JavaScript Data Types
- JavaScript isFinite()
JavaScript Type Conversion
- JavaScript Object.is()
JavaScript null and undefined
There are 8 types of data types in JavaScript. They are:
undefined and null are the two data types that we will discuss in this tutorial.
- JavaScript undefined
If a variable is declared but the value is not assigned, then the value of that variable will be undefined . For example,
It is also possible to explicitly assign undefined to a variable. For example,
Note: Usually, null is used to assign 'unknown' or 'empty' value to a variable. Hence, you can assign null to a variable.
- JavaScript null
In JavaScript, null is a special value that represents an empty or unknown value . For example,
The code above suggests that the number variable is empty at the moment and may have a value later.
Note : null is not the same as NULL or Null.
False Values
In JavaScript, undefined and null are treated as false values. For example,
An undefined or null gets converted to false when used with the Boolean() function. For example,
JavaScript typeof: null and undefined
In JavaScript, null is treated as an object. You can check this using the typeof operator . The typeof operator determines the type of variables and values. For example,
When the typeof operator is used to determine the undefined value, it returns undefined . For example,
JavaScript Default Values: null and undefined
Before you visit this section, be sure to check the JavaScript default parameter tutorial.
In JavaScript, when you pass undefined to a function parameter that takes a default value, the undefined is ignored and the default value is used. For example,
However, when you pass null to a default parameter function, the function takes the null as a value. For example,
Comparing null and undefined
When comparing null and undefined with equal to operator == , they are considered equal. For example,
In JavaScript, == compares values by performing type conversion . Both null and undefined return false. Hence, null and undefined are considered equal.
However, when comparing null and undefined with strict equal to operator === , the result is false. For example,
Table of Contents
- Falsy Values
- JavaScript typeof
- JavaScript Default Values
- null vs undefined
Sorry about that.
Related Tutorials
JavaScript Tutorial
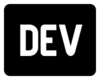
DEV Community

Posted on Feb 9, 2020
JavaScript OR Assignment Operator
In JavaScript, there may be times when you want to assign one variable to a non-null value. The JavaScript OR assignment operator can be used to quickly assign the value of a variable to one of two options based on one value and if it is null or undefined.
The below code shows a function called 'getNotNull' which takes two parameters 'a' and 'b'. If the value of 'a' is defined and isn't null, it is returned, otherwise, the variable 'b' is returned. This doesn't prevent a null value being returned though, as if both 'a' and 'b' are null then the value of 'b' will be returned and therefore a null value will be returned.
A ternary operator can also be used to the same effect. In the below code a ternary operator is used to set the value of the variable 'result' to either the value of 'a' if it defined and not null otherwise it will be set to the value 'b'. Again, this does not prevent a null value if both variables are null or undefined.
The JavaScript OR assignment operator is represented with two pipe '|' symbols. This can be used to achieve the same effect as the above two code snippets. The value of the 'result' variable will be assigned to the value of 'a' if it is defined or not null otherwise it will be assigned to the value of 'b'.
The OR assignment operator does not need to be used with variables, it can be used with raw values too. The below code snippet shows using the OR operator to set the value of the 'result' variable uses raw values, 'null' or the number '2'. The value of the 'result' variable will be 2 as the left side of the OR assignment operator is null.
The OR assignment operator can be used to assign the value of one variable to either one or another value based on if the first value is null or undefined. Using the OR assignment operator does not prevent the variable from being assigned a null or undefined value, if both sides of the OR assignment operator are null then the resulting value will also be null.
This post was originally published on https://acroynon.com
Top comments (14)
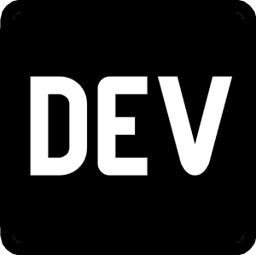
Templates let you quickly answer FAQs or store snippets for re-use.
- Joined Feb 5, 2018
You should replace "null" / "null or undefined" by "falsy".
And also have a look at the "??" operator.
Keep writing it is a good way to improve!

- Location United Kingdom
- Education Computer Science Bsc
- Work Senior Software Engineer
- Joined Nov 7, 2019
Yeah, you're correct, it is a "falsely" value that is evaluated, I didn't want to make this post too complicated, but great to mention this for the curious readers. Thanks
I am not sure what you mean by "??" operator, I have written a post on the ternary operator and I plan to write a post about the optional chaining operator ('?.') in the future.
Here is the related documentation:
developer.mozilla.org/en-US/docs/W...
Thanks, it seems like a more strict version of the OR assignment operator. Perhaps I shall create a post comparing the two
I think that you should point out the differences between falsy values (cf. developer.mozilla.org/en-US/docs/G... ) and null/undefined checks (with ??). I think that will add value to your post.
I think I shall leave this post as it is for now and cover the falsy values and the coalescing operator in separate posts, thank you for the feedback and ideas
Up to you, I just recommend you to be careful with the terms that you use in your post and in my opinion it is a good to support your posts with official references.
For example you function:
Doesn't work if you pass to it a falsy value like 0 or an empty string.
By being not precise you will assume wrong things (I have been there so many times, and it is still happening).
I would recommend the "you don't know js" by Kyle Simpson ( @getify ), to expand your knowledge.
Thanks for all the feedback. My plan isn't to fill every post with all the detail needed, at least not right now (I don't have the time to put that much time into each post). I mainly want to encourage people to begin to learn how to code, or experiment with something they haven't used before. I don't admit to knowing everything, but I don't want to overload the reader with too much information that reduces their interest in code. Again, thank you for all the feedback, I super appreciate it and I guarantee some readers will benefit from some of the links you've posted.

- Joined May 7, 2020
You’re talking about the regular OR-operator; the OR assignment -operator doesn’t exist in Javascript (unfortunately)
I consider the standard or operator the one used in if statement, for boolean logic. Then the or assignment operator being used to assign values to variables (assign this value, if its falsey assign this value instead). I could be completely wrong in my phrasing, but I think it makes a nice distinction between the two use cases
You can consider a melon a nail when you hit it with a hammer, but it’s not going to make sense to anybody.
I’m afraid the long and short of it is just that the OR-assignment operator ( ||= ) is not the OR-operator ( || ), sorry man.
Okay, thanks for the clarification
Okay thank you
Great stuff, do you think my explanation in this post is good?
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Web3: The Intro
thesonicstar - May 15

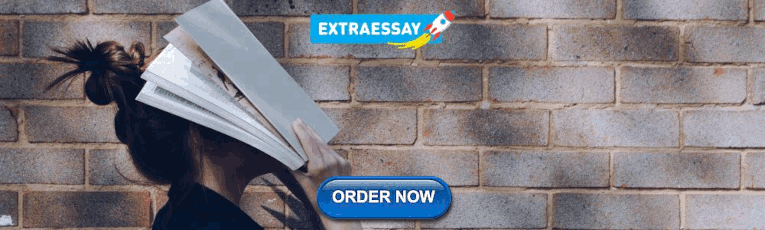
Understanding Callbacks in Rails: Enhancing Model Interactions and Lifecycle Management
Afaq Shahid Khan - May 28
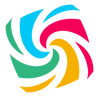
What is DevOps…from business point of view?
DevOps Pass AI - May 15
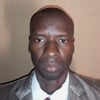
How to create an Artificial Intelligence - powered antivirus for mobile applications
Olatunji Ayodele Abidemi - May 15
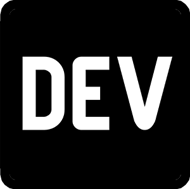
We're a place where coders share, stay up-to-date and grow their careers.
JavaScript: Check if Variable is undefined or null
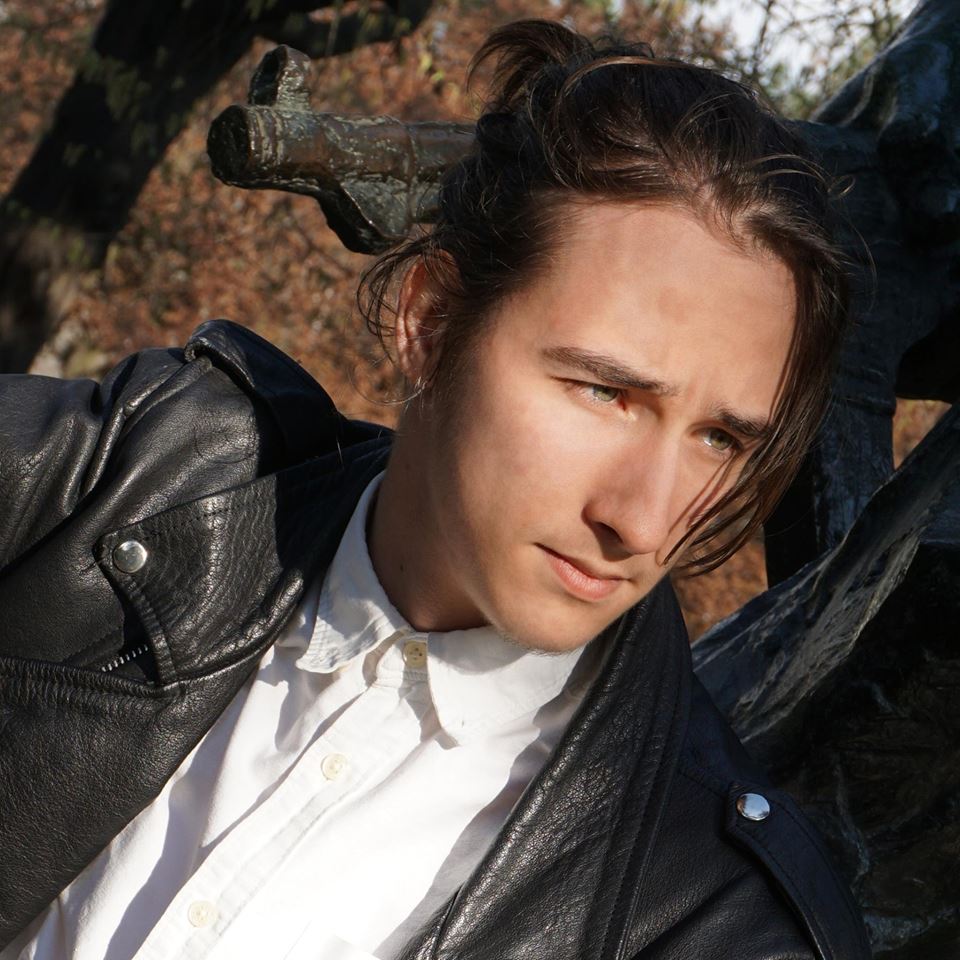
- Introduction
undefined and null values sneak their way into code flow all the time. Whether we lose a reference through side-effects, forget to assign a reference variable to an object in memory, or we get an empty response from another resource, database or API - we have to deal with undefined and null values all the time.
In this short guide, we'll take a look at how to check if a variable is undefined or null in JavaScript.
- Difference Between undefined and null
undefined and null variables often go hand-in-hand, and some use the terms interchangeably. Though, there is a difference between them:
- undefined is a variable that refers to something that doesn't exist, and the variable isn't defined to be anything.
- null is a variable that is defined but is missing a value.
The difference between the two is perhaps a bit more clear through code:
a is undefined - it's not assigned to anything, and there's no clear definition as to what it really is. b is defined as a null-value .
Whether b was straight up defined as null or defined as the returned value of a function (that just turns out to return a null value) doesn't matter - it's defined as something .
On the other hand, a is quite literally nothing . No assignment was done and it's fully unclear what it should or could be.
In practice, most of the null and undefined values arise from human error during programming, and these two go together in most cases. When checking for one - we typically check for the other as well.
- Check if Variable is undefined or null
There are two approaches you can opt for when checking whether a variable is undefined or null in vanilla JavaScript.
- == and === Operators
There's a difference between the Loose Equality Operator ( == ) and Strict Equality Operator ( === ) in JavaScript. Loose equality may lead to unexpected results, and behaves differently in this context from the strict equality operator:
Note: This shouldn't be taken as proof that null and undefined are the same. The loose equality operator uses "colloquial" definitions of truthy/falsy values. 0 , "" and [] are evaluated as false as they denote the lack of data, even though they're not actually equal to a boolean.
That being said - since the loose equality operator treats null and undefined as the same - you can use it as the shorthand version of checking for both:
This would check whether a is either null or undefined . Since a is undefined , this results in:
Though, we don't really know which one of these is it. If we were to use the strict operator, which checks if a is null , we'd be unpleasantly surprised to run into an undefined value in the console.log() statement:
a really isn't null , but it is undefined . In this case, we'd want to check them separately, but have the flexibility of knowing the real reason for the flow:
Here, we've combined them together with an exclusive OR - though you can separate them for different recovery operations if you'd like to as well:
Note: It's worth noting that if the reference doesn't exist, a ReferenceError will be thrown. This can be avoided through the use of the typeof operator, though it might not be the best choice from the perspective of code design. If you're using a non-existent reference variable - silently ignoring that issue by using typeof might lead to silent failure down the line.
- typeof Operator
The typeof operator can additionally be used alongside the === operator to check if the type of a variable is equal to 'undefined' or 'null' :
This results in:
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
However, it's worth noting that if you chuck in a non-existing reference variable - typeof is happy to work with it, treating it as undefined :
This code also results in:
Technically speaking, some_var is an undefined variable, as it has no assignment. On the other hand, this can make typeof silently fail and signal that the incoming value might have an issue, instead of raising an error that makes it clear you're dealing with a non-existing variable.
For instance - imagine you made a typo, and accidentally input somevariable instead of someVariable in the if clause:
Here, we're trying to check whether someVariable is null or undefined , and it isn't. However, due to the typo, somevariable is checked instead, and the result is:
In a more complex scenario - it might be harder to spot this typo than in this one. We've had a silent failure and might spend time on a false trail. On the other hand, using just the == and === operators here would have alerted us about the non-existing reference variable:
This code results in:
Note: This isn't to say that typeof is inherently a bad choice - but it does entail this implication as well.
Using Lodash to Check if Variable is null , undefined or nil
Finally - you may opt to choose external libraries besides the built-in operators. While importing an external library isn't justified just for performing this check - in which case, you'll be better off just using the operators.
However, Lodash is already present in many projects - it's a widely used library, and when it's already present, there's no efficiency loss with using a couple of the methods it provides. Most notably, Lodash offers several useful methods that check whether a variable is null , undefined or nil .
- Install and Import Lodash
You can import Lodash through a CDN:
Import it locally from a .js file:
Or install it via NPM:
Furthermore, it can be imported as an ES6 module or imported via the require() syntax:
Note: It's convention to name the Lodash instance _ , implied by the name, though, you don't have to.
Now, we can use the library to check whether a variable is null , undefined or nil - where nil refers to both of the previous two afflictions. It can be used as the shorthand version for checking both:
In this short guide, we've taken a look at how to check if a variable is null, undefined or nil in JavaScript, using the == , === and typeof operators, noting the pros and cons of each approach. Finally, we've taken a quick look at using Lodash - a popular convenience utility library to perform the same checks.
You might also like...
- JavaScript: Check if First Letter of a String is Upper Case
- Using Mocks for Testing in JavaScript with Sinon.js
- For-each Over an Array in JavaScript
- Commenting Code in JavaScript - Types and Best Practices
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
Entrepreneur, Software and Machine Learning Engineer, with a deep fascination towards the application of Computation and Deep Learning in Life Sciences (Bioinformatics, Drug Discovery, Genomics), Neuroscience (Computational Neuroscience), robotics and BCIs.
Great passion for accessible education and promotion of reason, science, humanism, and progress.
In this article
- Using Lodash to Check if Variable is null, undefined or nil
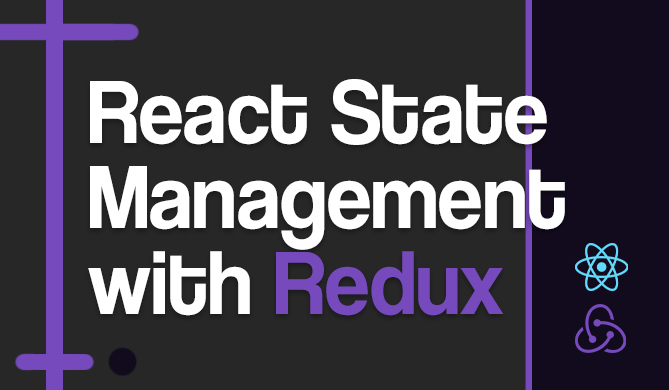
React State Management with Redux and Redux-Toolkit
Coordinating state and keeping components in sync can be tricky. If components rely on the same data but do not communicate with each other when...
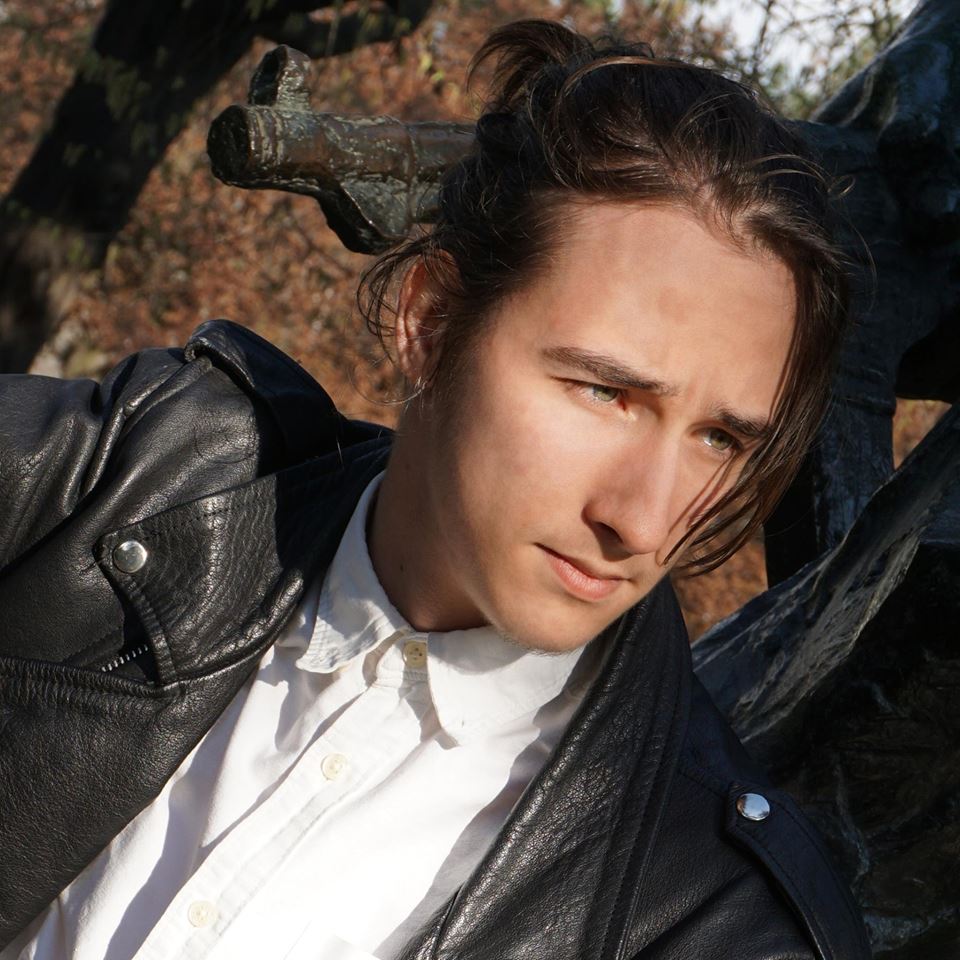
Getting Started with AWS in Node.js
Build the foundation you'll need to provision, deploy, and run Node.js applications in the AWS cloud. Learn Lambda, EC2, S3, SQS, and more!
© 2013- 2024 Stack Abuse. All rights reserved.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Logical OR assignment (||=)
The logical OR assignment ( ||= ) operator only evaluates the right operand and assigns to the left if the left operand is falsy .
Description
Logical OR assignment short-circuits , meaning that x ||= y is equivalent to x || (x = y) , except that the expression x is only evaluated once.
No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const :
Neither would the following trigger the setter:
In fact, if x is not falsy, y is not evaluated at all.
Setting default content
If the "lyrics" element is empty, display a default value:
Here the short-circuit is especially beneficial, since the element will not be updated unnecessarily and won't cause unwanted side-effects such as additional parsing or rendering work, or loss of focus, etc.
Note: Pay attention to the value returned by the API you're checking against. If an empty string is returned (a falsy value), ||= must be used, so that "No lyrics." is displayed instead of a blank space. However, if the API returns null or undefined in case of blank content, ??= should be used instead.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Logical OR ( || )
- Nullish coalescing operator ( ?? )
- Bitwise OR assignment ( |= )
Announcing UNISTR and || operator in Azure SQL Database – preview

Abhiman Tiwari
June 4th, 2024 0 0
We are excited to announce that the UNISTR intrinsic function and ANSI SQL concatenation ope ra tor ( || ) are now available in public preview in Azure SQL Database. The UNISTR function allows you to escape Unicode characters, making it easier to work with international text. The ANSI SQL concatenation ope ra tor ( || ) provides a simple and intuitive way to combine characters or binary strings. These new features will enhance your ability to manipulate and work with text data.
What is UNISTR function?
The UNISTR function takes a text literal or an expression of characters and Unicode values, that resolves to character data and returns it as a UTF-8 or UTF-16 encoded string . This function allows you to use Unicode codepoint escape sequences with other characters in the string. The escape sequence for a Unicode character can be specified in the form of \ xxxx or \+ xxxxxx , where xxxx is a valid UTF-16 codepoint value, and xxxxxx is a valid Unicode codepoint value. This is especially useful for inserting data into NCHAR columns.
The syntax of the UNISTR function is as follows:
- The data type of character_expression could be char , nchar , varchar , or nvarchar . For char and varchar data types, the collation should be a valid UTF-8 collation only.
- A single character representing a user-defined Unicode escape sequence. If not supplied, the default value is \.
Example #1:
For example, the following query returns the Unicode character for the specified value:
——————————-
Example #2:
In this example, the UNISTR function is used with a user-defined escape character ( $ ) and a VARCHAR data type with UTF-8 collation.
I ♥ Azure SQL.
The legacy collations with code page can be identified using the query below:
What is ANSI SQL concatenation operator (||)?
The ANSI SQL concatenation ope ra tor ( || ) concatenates two or more characters or binary strings, columns, or a combination of strings and column names into one expression . The || ope ra tor does not honor the SET CONCAT_NULL_YIELDS_NULL option and always behaves as if the ANSI SQL behavior is enabled . This ope ra tor will work with character strings or binary data of any supported SQL Server collation . The || ope ra tor supports compound assignment || = similar to += . If the ope ra nds are of incompatible collation, then an error will be thrown. The collation behavior is identical to the CONCAT function of character string data.
The syntax of the string concatenation operator is as follows:
- The expression is a character or binary expression. Both expressions must be of the same data type, or one expression must be able to be implicitly converted to the data type of the other expression. If one ope ra nd is of binary type, then an unsupported ope ra nd type error will be thrown.
Example #1:
For example, the following query concatenates two strings and returns the result:
Hello World!
Example #2:
In this example, multiple character strings are concatenated. If at least one input is a character string, non-character strings will be implicitly converted to character strings.
full_name order_details item_desc
Josè Doe Order-1001~TS~Jun 1 2024 6:25AM~442A4706-0002-48EC-84FC-8AF27XXXX NULL
Example #3:
In the example below, concatenating two or more binary strings and also compounding with T-SQL assignment operator.
V1 B1 B2
0x1A2B 0x4E 0xAE8C602E951AC245ADE767A23C834704A5
Example #4:
As shown in the example below, using the || operator with only non-character types or combining binary data with other types is not supported.
Above queries will fail with error messages as below –
In this blog post, we have introduced the UNISTR function and ANSI SQL concatenation operator (||) in Azure SQL Database. The UNISTR function allows you to escape Unicode characters, making it easier to work with international text. ANSI SQL concatenation operator (||) provides a simple and intuitive way to combine characters or binary data. These new features will enhance your ability to manipulate and work with text data efficiently.
We hope you will explore these enhancements, apply them in your projects, and share your feedback with us to help us continue improving. Thank you!

Abhiman Tiwari Senior Product Manager, Azure SQL

Leave a comment Cancel reply
Log in to start the discussion.

Insert/edit link
Enter the destination URL
Or link to existing content
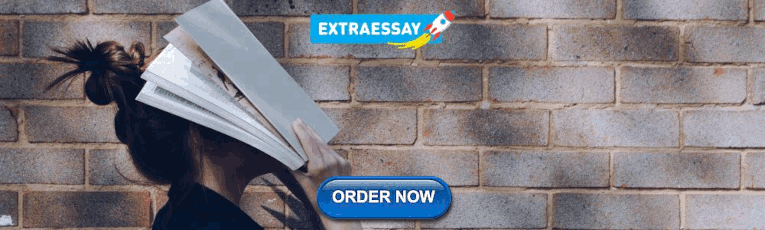
IMAGES
VIDEO
COMMENTS
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
Here's the JavaScript equivalent: var i = null; var j = i || 10; //j is now 10. Note that the logical operator || does not return a boolean value but the first value that can be converted to true. Additionally use an array of objects instead of one single object: var options = {. filters: [.
The nullish coalescing operator is written as two question marks ??.. As it treats null and undefined similarly, we'll use a special term here, in this article. For brevity, we'll say that a value is "defined" when it's neither null nor undefined.. The result of a ?? b is:. if a is defined, then a,; if a isn't defined, then b.; In other words, ?? returns the first argument if it ...
The nullish coalescing operator was introduced in ES2020, which makes it a relatively new addition to the JavaScript language. For example, the nullish coalescing operator isn't supported in Node.js 12. However, there is a concise alternative with the ternary operator that works in any version of JavaScript.
Summary: in this tutorial, you'll learn about the JavaScript nullish coalescing operator (??) that accepts two values and returns the second value if the first one is null or undefined.. Introduction to the JavaScript nullish coalescing operator. ES2020 introduced the nullish coalescing operator denoted by the double question marks (??
This post was published 24 Dec, 2021 by Daniyal Hamid. Daniyal currently works as the Head of Engineering in Germany and has 20+ years of experience in software engineering, design and marketing.
Try this: this.approved_by = IsNullOrEmpty(planRec.approved_by) ? string.Empty : planRec.approved_by.toString(); You can also use the null-coalescing operator as other have said - since no one has given an example that works with your code here is one:
The nullish coalescing operator can be seen as a special case of the logical OR ( ||) operator. The latter returns the right-hand side operand if the left operand is any falsy value, not only null or undefined. In other words, if you use || to provide some default value to another variable foo, you may encounter unexpected behaviors if you ...
JavaScript null is a primitive type that contains a special value null. JavaScript uses the null value to represent the intentional absence of any object value. If you find a variable or a function that returns null, it means that the expected object couldn't be created. The following example defines the Circle class whose constructor accepts ...
How to use the loose equality operator to check for null. You can use the loose equality operator to check for null values: let firstName = null; console.log(firstName == null); // true. But, this can be tricky because if the variable is undefined, it will also return true because both null and undefined are loosely equal.
If not null or undefined then it will return left-hand value. There are values in JavaScript like 0 and an empty s. 2 min read. ... JavaScript remainder assignment operator (%=) assigns the remainder to the variable after dividing a variable by the value of the right operand. Syntax: Operator: x %= y Meaning: x = x % y Below example illustrate ...
In JavaScript, == compares values by performing type conversion. Both null and undefined return false. Hence, null and undefined are considered equal. However, when comparing null and undefined with strict equal to operator ===, the result is false. For example, console.log(null === undefined); // false.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
The JavaScript OR assignment operator can be used to quickly assign the value of a variable to one of two options based on one value and if it is null or undefined. The below code shows a function called 'getNotNull' which takes two parameters 'a' and 'b'. If the value of 'a' is defined and isn't null, it is returned, otherwise, the variable 'b ...
In newer JavaScript standards like ES5 and ES6 you can just say. > Boolean (0) //false > Boolean (null) //false > Boolean (undefined) //false. all return false, which is similar to Python's check of empty variables. So if you want to write conditional logic around a variable, just say.
undefined is a variable that refers to something that doesn't exist, and the variable isn't defined to be anything. null is a variable that is defined but is missing a value. The difference between the two is perhaps a bit more clear through code: let a; console .log(a); // undefined let b = null ;
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
null is an empty or non-existent value. null must be assigned. You can assign null to a variable to denote that currently that variable does not have any value but it will have later on. A null means absence of a value. example:-. let a = null; console.log(a); //null. answered Feb 19, 2019 at 13:23.
This is an idiomatic pattern in JavaScript, but it gets verbose when the chain is long, and it's not safe. For example, if obj.first is a Falsy value that's not null or undefined, such as 0, it would still short-circuit and make nestedProp become 0, which may not be desirable. With the optional chaining operator (?.
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.
The || ope ra tor does not honor the SET CONCAT_NULL_YIELDS_NULL option and always behaves as if the ANSI SQL behavior is enabled. This ope ra tor will work with character strings or binary data of any supported SQL Server collation. The || ope ra tor supports compound assignment || = similar to +=.
@HunanRostomyan Good question, and honestly, no, I do not think that there is. You are most likely safe enough using variable === null which I just tested here in this JSFiddle.The reason I also used ` && typeof variable === 'object'` was not only to illustrate the interesting fact that a null value is a typeof object, but also to keep with the flow of the other checks.
Then your question is no fit for StackOverflow. The primary goal of StackOverflow is to build a library of high-quality questions and answers that will not only help you but also others having the same problem.