Home » Perl if Statement
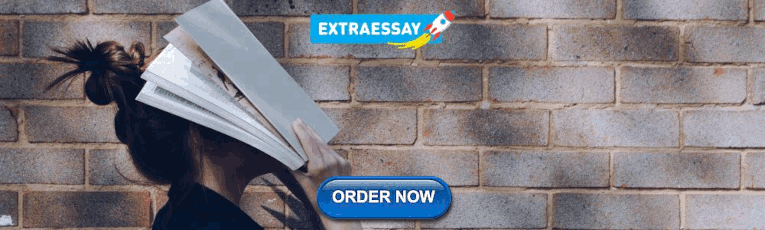
Perl if Statement
Summary : in this tutorial, you are going to learn about Perl if statement that allows you to control the execution of code conditionally.
Simple Perl if statement
Perl if statement allows you to control the execution of your code based on conditions. The simplest form of the if statement is as follows:
In this form, you can put the if statement after another statement. Let’s take a look at the following example:
The message is only displayed if the expression $a == 1 evaluates to true . How Perl defines true and false ? The following rules are applied when Perl evaluates an expression:
- Both number 0 and string “0” are false .
- The undefined value is false .
- The empty list () is false .
- The empty string "" is false .
- Everything else is true .
If you do not remember the rules or you doubt whether a condition is true or false , you can always write a simple if statement to test the expression.
The limitation of the Perl if statement is that it allows you to execute a single statement only. If you want to execute multiple statements based on a condition, you use the following form:
Noticed that the curly braces {} are required even if you have a single statement to execute. Watch out C/C++ and C# programmers!
The following flowchart illustrates the Perl if statement:
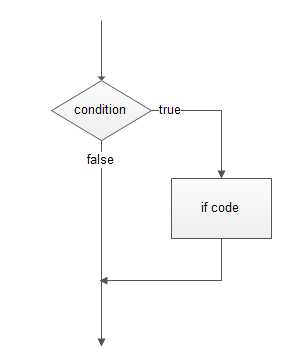
And the following is an example of using the if statement:
Perl if else statement
In some cases, you want to also execute another code block if the expression does not evaluate to true . Perl provides the if else statement that allows you to execute a code block if the expression evaluates to true , otherwise, the code block inside the else branch will execute.
The following flowchart illustrates the Perl if else statement:
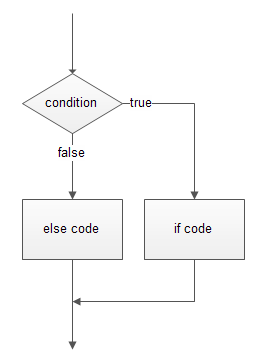
See the following example, the code block in the else branch will execute because $a and $b are not equal.
Perl if elsif statement
In some cases, you want to test more than one condition, for example:
- If $a and $b are equal, then do this.
- If $a is greater than $b then do that.
- Otherwise, do something else.
Perl provides the if elsif statement for checking multiple conditions and executing the corresponding code block as follows:
The following flowchart illustrates the if elseif else statement:
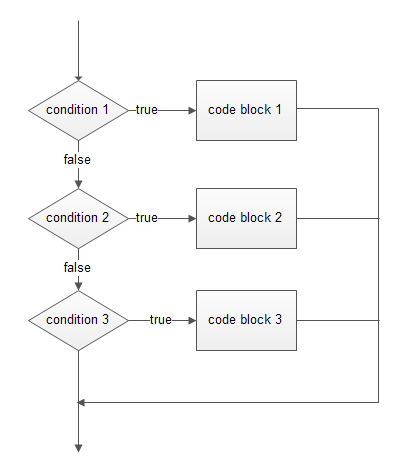
Technically speaking, you can have many elsif branches in an if elseif statement. However, it is not a good practice to use a lengthy if elsif statement.
Perl if statement example
We are going to apply what we have learned so far to create a simple program called currency converter.
- We will use a hash to store the exchange rates.
- To get the inputs from users via the command line, we will use <STDIN>. We use the chomp() function to remove the newline character (\n) from the user’s inputs.
- We convert the amount from local currency to foreign currency if the currencies are supported.
The following source code illustrates the simple currency converter program:
In this tutorial, you’ve learned various forms of the Perl if statement including simple if , if else , and if esif else statements to control the execution of the code.
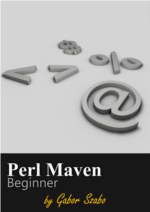
- Installing and getting started with Perl
- The Hash-bang line, or how to make a Perl scripts executable on Linux
- Perl Editor
- How to get Help for Perl?
- Perl on the command line
- Core Perl documentation and CPAN module documentation
- POD - Plain Old Documentation
- Debugging Perl scripts
- Common Warnings and Error messages in Perl
- Prompt, read from STDIN, read from the keyboard in Perl
- Automatic string to number conversion or casting in Perl
Conditional statements, using if, else, elsif in Perl
- Boolean values in Perl
- Numerical operators
- String operators: concatenation (.), repetition (x)
- undef, the initial value and the defined function of Perl
- Strings in Perl: quoted, interpolated and escaped
- Here documents, or how to create multi-line strings in Perl
- Scalar variables
- Comparing scalars in Perl
- String functions: length, lc, uc, index, substr
- Number Guessing game
- Scope of variables in Perl
- Short-circuit in boolean expressions
- How to exit from a Perl script?
- Standard output, standard error and command line redirection
- Warning when something goes wrong
- What does die do?
- Writing to files with Perl
- Appending to files
- Open and read from text files
- Don't Open Files in the old way
- Reading and writing binary files in Perl
- EOF - End of file in Perl
- tell how far have we read a file
- seek - move the position in the filehandle in Perl
- slurp mode - reading a file in one step
- Perl for loop explained with examples
- Perl Arrays
- Processing command line arguments - @ARGV in Perl
- How to process command line arguments in Perl using Getopt::Long
- Advanced usage of Getopt::Long for accepting command line arguments
- Perl split - to cut up a string into pieces
- How to read a CSV file using Perl?
- The year of 19100
- Scalar and List context in Perl, the size of an array
- Reading from a file in scalar and list context
- STDIN in scalar and list context
- Sorting arrays in Perl
- Sorting mixed strings
- Unique values in an array in Perl
- Manipulating Perl arrays: shift, unshift, push, pop
- Reverse Polish Calculator in Perl using a stack
- Using a queue in Perl
- Reverse an array, a string or a number
- The ternary operator in Perl
- Loop controls: next, last, continue, break
- min, max, sum in Perl using List::Util
- qw - quote word
- Subroutines and functions in Perl
- Passing multiple parameters to a function in Perl
- Variable number of parameters in Perl subroutines
- Returning multiple values or a list from a subroutine in Perl
- Understanding recursive subroutines - traversing a directory tree
- Hashes in Perl
- Creating a hash from an array in Perl
- Perl hash in scalar and list context
- exists - check if a key exists in a hash
- delete an element from a hash
- How to sort a hash in Perl?
- Count the frequency of words in text using Perl
- Introduction to Regexes in Perl 5
- Regex character classes
- Regex: special character classes
- Perl 5 Regex Quantifiers
- trim - removing leading and trailing white spaces with Perl
- Perl 5 Regex Cheat sheet
- What are -e, -z, -s, -M, -A, -C, -r, -w, -x, -o, -f, -d , -l in Perl?
- Current working directory in Perl (cwd, pwd)
- Running external programs from Perl with system
- qx or backticks - running external command and capturing the output
- How to remove, copy or rename a file with Perl
- Reading the content of a directory
- Traversing the filesystem - using a queue
- Download and install Perl
- Installing a Perl Module from CPAN on Windows, Linux and Mac OSX
- How to change @INC to find Perl modules in non-standard locations
- How to add a relative directory to @INC
- How to replace a string in a file with Perl
- How to read an Excel file in Perl
- How to create an Excel file with Perl?
- Sending HTML e-mail using Email::Stuffer
- Perl/CGI script with Apache2
- JSON in Perl
- Simple Database access using Perl DBI and SQL
- Reading from LDAP in Perl using Net::LDAP
- Global symbol requires explicit package name
- Variable declaration in Perl
- Use of uninitialized value
- Barewords in Perl
- Name "main::x" used only once: possible typo at ...
- Unknown warnings category
- Can't use string (...) as an HASH ref while "strict refs" in use at ...
- Symbolic references in Perl
- Can't locate ... in @INC
- Scalar found where operator expected
- "my" variable masks earlier declaration in same scope
- Can't call method ... on unblessed reference
- Argument ... isn't numeric in numeric ...
- Can't locate object method "..." via package "1" (perhaps you forgot to load "1"?)
- Useless use of hash element in void context
- Useless use of private variable in void context
- readline() on closed filehandle in Perl
- Possible precedence issue with control flow operator
- Scalar value ... better written as ...
- substr outside of string at ...
- Have exceeded the maximum number of attempts (1000) to open temp file/dir
- Use of implicit split to @_ is deprecated ...
- Multi dimensional arrays in Perl
- Multi dimensional hashes in Perl
- Minimal requirement to build a sane CPAN package
- Statement modifiers: reversed if statements
- What is autovivification?
- Formatted printing in Perl using printf and sprintf
- indentation
Layout: Indentation
Nested if statements, else if in perl, empty block.
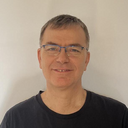
Published on 2014-04-22
Author: Gabor Szabo
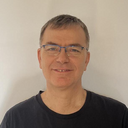
- about the translations
- Perl tutorial

- AUTHORS
- CATEGORIES
- # TAGS
The conditional (ternary) operator
Apr 16, 2013 by David Farrell
One way to reduce the verbosity of Perl code is to replace if-else statements with a conditional operator expression. The conditional operator (aka ternary operator) takes the form: logical test ? value if true : value if false .
Let’s convert a standard Perl if-else into its conditional operator equivalent, using a fictitious subroutine. First here is the if-else:
And here is the same statement using the conditional operator:
Hopefully this example shows how using the conditional operator can shorten and simplify Perl code. For further detail, check out the official documentation .
This article was originally posted on PerlTricks.com .
David Farrell
David is a professional programmer who regularly tweets and blogs about code and the art of programming.
Browse their articles
Something wrong with this article? Help us out by opening an issue or pull request on GitHub
To get in touch, send an email to [email protected] , or submit an issue to tpf/perldotcom on GitHub.
This work is licensed under a Creative Commons Attribution-NonCommercial 3.0 Unported License .

Perl.com and the authors make no representations with respect to the accuracy or completeness of the contents of all work on this website and specifically disclaim all warranties, including without limitation warranties of fitness for a particular purpose. The information published on this website may not be suitable for every situation. All work on this website is provided with the understanding that Perl.com and the authors are not engaged in rendering professional services. Neither Perl.com nor the authors shall be liable for damages arising herefrom.
Using if, elsif, else and unless
Perl if statements can consist of an 'if' evaluation or an 'unless' evaluation, an 'elsif' evaluation (yes the 'e' is missing), and an 'else' evaluation.
The syntax for an if statement can be arranged a few ways:
The unless statement is just like an 'if-not'. For example, the following two lines of code are equivalent:
Most commonly, you will see the following use of unless:
The else statement gets run when the preceding 'if' or 'unless' is not true:
If you want to check for more than one condition, you can build a larger statement using 'elsif':
The expression inside the 'if' condition, is evaluated. This means it is checked to see whether it is true or false. The following condition evaluates to true:
And the following evaluates to false:
The above example could also be written as:
You can also use if to check if a variable has a defined value. The following evaluates to false because $value has no defined value.
This works quite nicely with boolean values and objects, however you must be careful with strings. While the string in the following code has a defined value, it still evaluates to false:
This is because perl is not a strongly typed language. If you wanted to check if $value had a defined value, it is better to use the 'defined' function:
The c-style ? operator
Another way of writing an if statement is using the c-style shorthand conditional operator ?. This is very useful when assigning values. An example is:
If $flag evaluates to true, then $c will equal 4, otherwise $c will equal 5.
The form of 'if-else' statement can be easily embedded in other statements, for example, a print statement:
To find out more, run the command:

How to use the Perl ternary operator
In most languages there is an operator named the "ternary" operator that lets you write concise if/then statements. This makes for less verbose, which is generally a good thing. Perl also has a ternary operator, and I'll demonstrate it here.
General syntax of the ternary operator
The general syntax for Perl's ternary operator looks like this:
Let's take a look at a brief example to demonstrate this.
A brief example
Here's a brief example that demonstrates the Perl ternary operator. As you might guess, the first set of print statements will print true , and the second set prints false .
(I use $TRUE and $FALSE variables because I think they're easier to remember than 1 and 0 , especially if you're new to Perl.)
A more complex example
Here's a slightly more complicated example to demonstrate the use of a comparison/equality operator in the "test expression" portion of the ternary operator:
As you might guess, this segment of code will print:
(Which summarizes my driving habits.)
The ternary operator is cool -- I hope you like it!
Help keep this website running!
- Perl ‘equals’ FAQ: What is true and false in Perl?
- How to exclude certain elements in an array with pattern matching
- A Perl module template (template code for a Perl module)
- Perl if, else, elsif ("else if") syntax
- Perl delete - how to delete a file in a Perl script
books by alvin
- And when he died, all he left us was a loan
- A great Halloween pumpkin squirrel card
- Gonna need a new t-shirt
- Functional Programming, Simplified (a best-selling FP book)
- The purposes of mindfulness (or, why bother being mindful, and motivation)

Popular Articles
- Perl Empty Array (Jan 05, 2024)
- Perl Change Directory (Jan 05, 2024)
- Perl Backticks (Jan 05, 2024)
- Perl Vs Bash (Jan 05, 2024)
- Perl Or Operator (Jan 05, 2024)
Perl Else If
Switch to English
Table of Contents
Introduction
Understanding the perl 'if' statement, introducing the perl 'else' statement, exploring the perl 'elsif' statement, common pitfalls and tips.
- The 'if' statement in Perl is used to execute a block of code if a certain condition is true. The syntax of the 'if' statement in Perl is as follows:
- The 'else' statement in Perl can be used in conjunction with the 'if' statement to execute a block of code if the condition in the 'if' statement is not true. Here is the syntax:
- Perl provides an 'elsif' keyword to allow for multiple, distinct conditions to be tested in sequence. The 'elsif' statement is only executed if the preceding 'if' or 'elsif' condition is not true and its own condition is true. The syntax is as follows:
- One of the most common pitfalls when using 'if-elsif-else' in Perl is the incorrect use of conditions. Always remember that the condition should return a boolean value (true or false).
- Another common mistake is forgetting to use the curly braces '{}' to define the scope of the 'if', 'elsif', and 'else' blocks. Without these, Perl will not know where the block begins and ends.
- It's crucial to remember that the conditions are evaluated from top to bottom. If a condition is true, Perl executes that block of code and skips the rest, even if they may also be true. Therefore, the order in which conditions are written is important.
- Lastly, 'elsif' in Perl is written as 'elsif', not 'elseif' or 'else if'. This is a common typo that can lead to syntax errors.
- COPYRIGHT AND LICENCE
if - use a Perl module if a condition holds
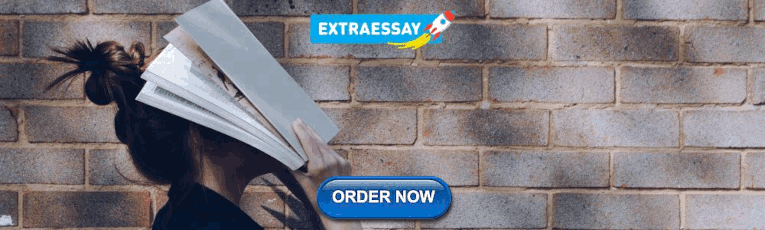
# DESCRIPTION
The if module is used to conditionally load another module. The construct:
... will load MODULE only if CONDITION evaluates to true; it has no effect if CONDITION evaluates to false. (The module name, assuming it contains at least one :: , must be quoted when 'use strict "subs";' is in effect.) If the CONDITION does evaluate to true, then the above line has the same effect as:
For example, the Unicode::UCD module's charinfo function will use two functions from Unicode::Normalize only if a certain condition is met:
Suppose you wanted ARGUMENTS to be an empty list, i.e. , to have the effect of:
You can't do this with the if pragma; however, you can achieve exactly this effect, at compile time, with:
The no if construct is mainly used to deactivate categories of warnings when those categories would produce superfluous output under specified versions of perl .
For example, the redundant category of warnings was introduced in Perl-5.22. This warning flags certain instances of superfluous arguments to printf and sprintf . But if your code was running warnings-free on earlier versions of perl and you don't care about redundant warnings in more recent versions, you can call:
The no if construct assumes that a module or pragma has correctly implemented an unimport() method -- but most modules and pragmata have not. That explains why the no if construct is of limited applicability.
The current implementation does not allow specification of the required version of the module.
Module::Requires can be used to conditionally load one or more modules, with constraints based on the version of the module. Unlike if though, Module::Requires is not a core module.
Module::Load::Conditional provides a number of functions you can use to query what modules are available, and then load one or more of them at runtime.
The provide module from CPAN can be used to select one of several possible modules to load based on the version of Perl that is running.
Ilya Zakharevich mailto:[email protected] .
# COPYRIGHT AND LICENCE
This software is copyright (c) 2002 by Ilya Zakharevich.
This is free software; you can redistribute it and/or modify it under the same terms as the Perl 5 programming language system itself.
Perldoc Browser is maintained by Dan Book ( DBOOK ). Please contact him via the GitHub issue tracker or email regarding any issues with the site itself, search, or rendering of documentation.
The Perl documentation is maintained by the Perl 5 Porters in the development of Perl. Please contact them via the Perl issue tracker , the mailing list , or IRC to report any issues with the contents or format of the documentation.
Perl - If else
Learn Perl If else conditional statement using string, one line simple logical and or operator tutorial for beginner examples..
This tutorial explains how to use if-else conditional statements in Perl with examples.
if conditional statements are used to execute code blocks based on a conditional expression. Perl provides the following conditional statements
It provides the following features
- simple if statements
- if else statements
- if else if else
- nested if else
Simple Perl if conditional statement
if the conditional statement is used to execute code block based on conditional expression statements.
-The condition_expression contains either single or multiple conditions. Multiple conditions are used with logical operators.
condition_expression is always evaluated as true or false . if it is true , It executes a code block.
Here is an example
- if is a keyword in Perl
- conditional expression are always enclosed () and must require for simple condition
- code statements are enclosed in {} and it is compulsory to include for single statement also
conditional_expression is a Boolean expression that returns true or false .
- if the condition is true, the code block is executed.
- if the condition is false, the code block is not executed. Here is an example of Perl if statements example for checking even numbers.
Here is a simple if conditional statement example for Odd numbers
Perl if else conditional statements
if else in Perl is used to execute code statements based on true and false conditional expressions.
condition is a Boolean expression that returns true or false
- if the condition is true , code block inside if block is executed.
- if the condition is false , the code block inside the else block is executed. Here is an example of Perl if conditional true example
Here is a Perl if else conditional expression example
Perl if else if statement example
This is a combination of if-else and if statements.
This is used to execute code blocks based on multiple conditions.
perl if condition with and operator
The conditional expression contains logical Operator Logic And operator( and or &&) Logic OR operator( or or || ) Logic Not operator( not )
In the above example, the Logical And operator are used in multiple conditions. It returns true if both conditions are true, else returns false.
Perl If condition string example
We can check whether given two strings are equal or not using Perl if the conditional statement
Strings are equal or not using == and eq operator in If statements.
- == is numeric comparision operator, that evaluates both operands to numeric value(0) and compare its value.
- The eq operator is a string comparison operator that compares two string characters that are equal with the exact case.
Perl one line if statement
Perl supports if-else statements in a single line using the ternary operator
For example, here is an if-else statement in Perl.
The same above can be rewritten using short hand ternary conditional operator
if-elsif-else Statement
Let's discuss if-elsif-else statements using different examples in this lesson.
- Explanation
- Logical operators in if condition
The elsif statement
When there are different possible actions against a set of different conditions, then elsif is used to extend the if statement. The conditional block starts with an if-statement followed by multiple elsif statements, each with a different condition, and may end with an optional else-statement.
The syntax and usage of the if-statement with the elsif statement are presented in the following example.
Create a free account to access the full course.
By signing up, you agree to Educative's Terms of Service and Privacy Policy
- Perl - Home
- Perl - Introduction
- Perl - Comments
Perl - Operators
- Perl - If Else
- Perl - Unless Else
- Perl - Given Statement
- Perl - While Loop
- Perl - Until Loop
- Perl - For Loop
- Perl - Foreach Loop
- Perl - goto Statement
- Perl - Next Statement
- Perl - Last Statement
- Perl - Continue Statement
- Perl - Redo Statement
- Perl - Math Functions
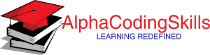
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- C Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
Operators are used to perform operation on a single operand or two operands. Operators in Perl can be categorized as follows:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- Bitwise operators
Quote-Like operators
- Miscellaneous operators
Perl Arithmetic operators
Arithmetic operators are used to perform arithmetic operations on two operands.
Perl Assignment operators
Assignment operators are used to assign values of right hand side expression to left hand side operand.
Perl Comparison operators
Comparison operators are used to compare values of two operands. It returns true when values matches and returns false when values does not match. Perl has two sets of comparison operators. One is used for comparing numerical values while other is used for comparing string values.
Numeric Comparison operators
String comparison operators, perl increment/decrement operators.
Increment and decrement operators are used to increase and decrease the value of variable.
Perl Logical operators
Logical operators are used to combine two or more conditions.
Perl Bitwise operators
Bitwise operators are used to perform bitwise operations on two operands.
Perl supports the following Quote-like operators:
Perl Miscellaneous operators
The table below describes other operators supported by Perl:
Perl Operators Precedence
Operator precedence ( order of operations ) is a collection of rules that reflect conventions about which procedures to perform first in order to evaluate a given expression.
For example, multiplication has higher precedence than addition. Thus, the expression 1 + 2 × 3 is interpreted to have the value 1 + (2 × 3) = 7, and not (1 + 2) × 3 = 9. When exponent is used in the expression, it has precedence over both addition and multiplication. Thus 3 + 5 2 = 28 and 3 × 5 2 = 75.
The following table lists the precedence and associativity of Perl operators. Operators are listed top to bottom, in descending precedence . Operators with higher precedence are evaluated before operators with relatively lower precedence. When operators have the same precedence, associativity of the operators determines the order in which the operations are performed.
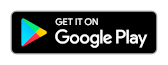
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
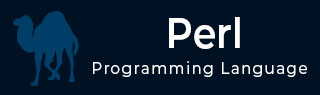
- Perl Basics
- Perl - Home
- Perl - Introduction
- Perl - Environment
- Perl - Syntax Overview
- Perl - Data Types
- Perl - Variables
- Perl - Scalars
- Perl - Arrays
- Perl - Hashes
- Perl - IF...ELSE
- Perl - Loops
- Perl - Operators
- Perl - Date & Time
- Perl - Subroutines
- Perl - References
- Perl - Formats
- Perl - File I/O
- Perl - Directories
- Perl - Error Handling
- Perl - Special Variables
- Perl - Coding Standard
- Perl - Regular Expressions
- Perl - Sending Email
- Perl Advanced
- Perl - Socket Programming
- Perl - Object Oriented
- Perl - Database Access
- Perl - CGI Programming
- Perl - Packages & Modules
- Perl - Process Management
- Perl - Embedded Documentation
- Perl - Functions References
- Perl Useful Resources
- Perl - Questions and Answers
- Perl - Quick Guide
- Perl - Useful Resources
- Perl - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Perl IF...ELSIF statement
An if statement can be followed by an optional elsif...else statement, which is very useful to test the various conditions using single if...elsif statement.
When using if , elsif , else statements there are few points to keep in mind.
An if can have zero or one else 's and it must come after any elsif 's.
An if can have zero to many elsif 's and they must come before the else .
Once an elsif succeeds, none of the remaining elsif 's or else 's will be tested.
The syntax of an if...elsif...else statement in Perl programming language is −
Here we are using the equality operator == which is used to check if two operands are equal or not. If both the operands are same, then it returns true otherwise it returns false. When the above code is executed, it produces the following result −
To Continue Learning Please Login
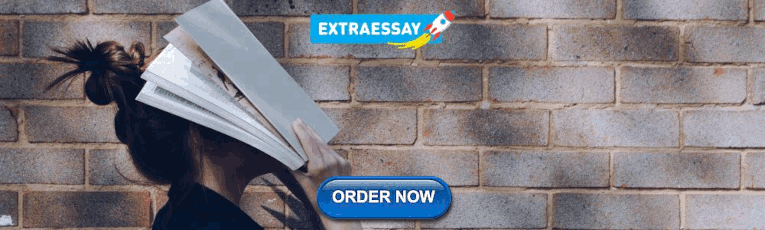
IMAGES
VIDEO
COMMENTS
In general, you should really get out of the habit of using conditionals to do assignment, as in the original example -- it's the sort of thing that leads to Perl getting a reputation for being write-only. A good rule of thumb is that conditionals are only for simple values, never expressions with side effects.
The assignment operator returns the assigned value, 0, which equates to false, thus the logical and '&&' always fails. Share. ... I don't see what your confusion has to do with the fact that perl uses "elsif" rather than "else if" or "elseif". In every language I've ever used, from pre-1970s-style FORTRAN to Java and including along the way ...
In this tutorial, you've learned various forms of the Perl if statement including simple if, if else, and if esif else statements to control the execution of the code. Was this tutorial helpful ? Yes No
else if in Perl. Please also note that the spelling of the "else if" construct has the most diversity among programming languages. In Perl it is written in one word with a single 'e': elsif. Empty block. Though usually it is not necessary, in Perl we can also have empty blocks: examples/empty_block.pl
One way to reduce the verbosity of Perl code is to replace if-else statements with a conditional operator expression. The conditional operator (aka ternary operator) takes the form: logical test? value if true: value if false. Let's convert a standard Perl if-else into its conditional operator equivalent, using a fictitious subroutine.
That is also false, so Perl executes the code in the `else` block, printing "The number is less than or equal to 5". Tips, Tricks and Common Errors; Remember that Perl considers zero, the empty string "", and the special undefined value `undef` to be false. All other values are considered true. This can be a common source of errors for new Perl ...
Perl if statements can consist of an 'if' evaluation or an 'unless' evaluation, an 'elsif' evaluation (yes the 'e' is missing), and an 'else' evaluation. if The syntax for an if statement can be arranged a few ways:
Perl also has a ternary operator, and I'll demonstrate it here. General syntax of the ternary operator. The general syntax for Perl's ternary operator looks like this: test-expression ? if-true-expression : if-false-expression Let's take a look at a brief example to demonstrate this. A brief example
One of the most common pitfalls when using 'if-elsif-else' in Perl is the incorrect use of conditions. Always remember that the condition should return a boolean value (true or false). Another common mistake is forgetting to use the curly braces '{}' to define the scope of the 'if', 'elsif', and 'else' blocks. Without these, Perl will not know ...
In perl, we have following conditional statements. Click on the links below to read a statement in detail with example. if statement - If statement consists a condition, if the condition evaluates to true then the statements inside "if" execute else they do not execute. if-else statement - if statement has an optional else statement, if ...
Conditional Operator (Programming Perl) 3.16. Conditional Operator. As in C, ?: is the only trinary operator. It's often called the conditional operator because it works much like an if-then-else, except that, since it's an expression and not a statement, it can be safely embedded within other expressions and functions calls.
The if module is used to conditionally load another module. The construct: use if CONDITION, "MODULE", ARGUMENTS; ... will load MODULE only if CONDITION evaluates to true; it has no effect if CONDITION evaluates to false. (The module name, assuming it contains at least one ::, must be quoted when 'use strict "subs";' is in effect.)
Nested if statement in perl When there is an if statement inside another if statement then it is called the nested if statement. The structure of nested if looks like this:
Learn Perl If else conditional statement using string, one line simple logical and or operator tutorial for beginner examples. w3schools is a free tutorial to learn web development. It's short (just as long as a 50 page book), simple (for everyone: beginners, designers, developers), and free (as in 'free beer' and 'free speech').
Perl programming language provides the following types of conditional statements. Sr.No. Statement & Description. 1. if statement. An if statement consists of a boolean expression followed by one or more statements. 2. if...else statement. An if statement can be followed by an optional else statement.
The conditional block starts with an if-statement followed by multiple elsif statements, each with a different condition, and may end with an optional else-statement. Syntax. The syntax and usage of the if-statement with the elsif statement are presented in the following example.
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
else { #if none of the condition is true #then these statements gets executed statement(s); } Note: The most important point to note here is that in if-elsif-else statement as soon as the condition is met, the corresponding set of statements get executed, rest gets ignored.
Perl Assignment operators. Assignment operators are used to assign values of right hand side expression to left hand side operand. Operator Expression Equivalent to Description = ... else returns 0. More Info ^ XOR: Returns 1 if only one of two bits at the same position in both operands is 1, else returns 0. More Info ~ NOT: Reverse all the bits.
OverflowAI is here! AI power for your Stack Overflow for Teams knowledge community. Learn more
An if statement can be followed by an optional elsif...else statement, which is very useful to test the various conditions using single if...elsif statement.. When using if , elsif , else statements there are few points to keep in mind.. An if can have zero or one else's and it must come after any elsif's.. An if can have zero to many elsif's and they must come before the else.