- Function Reference
- Variable and Type Related Extensions
- Array Functions
(PHP 4, PHP 5, PHP 7, PHP 8)
list — Assign variables as if they were an array
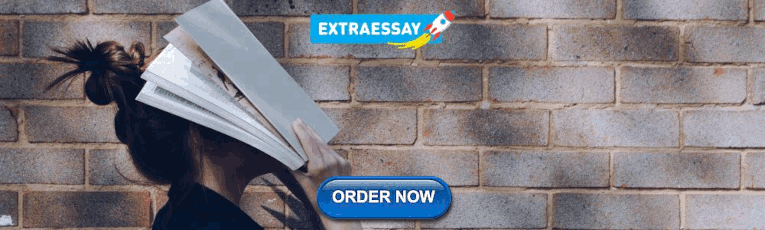
Description
Like array() , this is not really a function, but a language construct. list() is used to assign a list of variables in one operation. Strings can not be unpacked and list() expressions can not be completely empty.
Note : Before PHP 7.1.0, list() only worked on numerical arrays and assumes the numerical indices start at 0.
A variable.
Further variables.
Return Values
Returns the assigned array.
Example #1 list() examples
Example #2 An example use of list()
Example #3 Using nested list()
Example #4 list() and order of index definitions
The order in which the indices of the array to be consumed by list() are defined is irrelevant.
Gives the following output (note the order of the elements compared in which order they were written in the list() syntax):
Example #5 list() with keys
As of PHP 7.1.0 list() can now also contain explicit keys, which can be given as arbitrary expressions. Mixing of integer and string keys is allowed; however, elements with and without keys cannot be mixed.
The above example will output:
- each() - Return the current key and value pair from an array and advance the array cursor
- array() - Create an array
- extract() - Import variables into the current symbol table from an array
Improve This Page
User contributed notes 25 notes.

PHP Tutorial
Php advanced, mysql database, php examples, php reference, php list() function.
❮ PHP Array Reference
Assign variables as if they were an array:
Definition and Usage
The list() function is used to assign values to a list of variables in one operation.
Note: Prior to PHP 7.1, this function only worked on numerical arrays.
Parameter Values
Technical details.
Advertisement
More Examples
Using the first and third variables:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Function Reference
- Variable and Type Related Extensions
- Array Functions
(PHP 4, PHP 5, PHP 7, PHP 8)
list — Assign variables as if they were an array
Description
Like array() , this is not really a function, but a language construct. list() is used to assign a list of variables in one operation. Strings can not be unpacked and list() expressions can not be completely empty.
Note : Before PHP 7.1.0, list() only worked on numerical arrays and assumes the numerical indices start at 0.
A variable.
Further variables.
Return Values
Returns the assigned array.
Example #1 list() examples
Example #2 An example use of list()
Example #3 Using nested list()
Example #4 list() and order of index definitions
The order in which the indices of the array to be consumed by list() are defined is irrelevant.
Gives the following output (note the order of the elements compared in which order they were written in the list() syntax):
Example #5 list() with keys
As of PHP 7.1.0 list() can now also contain explicit keys, which can be given as arbitrary expressions. Mixing of integer and string keys is allowed; however, elements with and without keys cannot be mixed.
The above example will output:
- each() - Return the current key and value pair from an array and advance the array cursor
- array() - Create an array
- extract() - Import variables into the current symbol table from an array
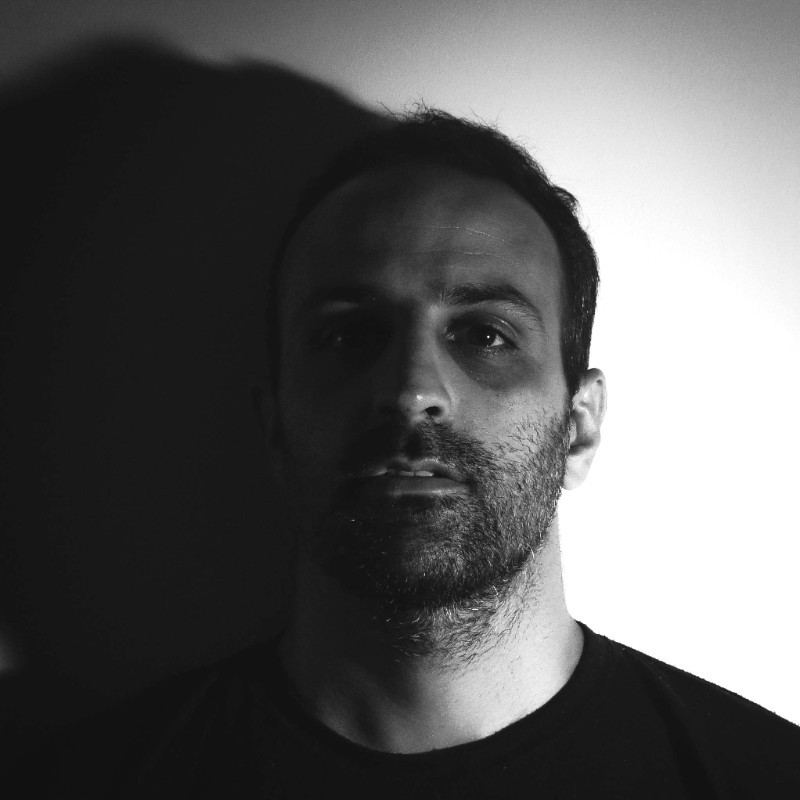
Working with PHP’s list() Function: A Comprehensive Guide
PHP is a versatile and widely-used programming language, known for its web development capabilities. It offers a plethora of functions and features that simplify tasks for developers. Among these is the list() function, which provides a convenient way to assign variables from arrays and has numerous applications in various programming scenarios. In this comprehensive guide, we will dive deep into the list() function, exploring its syntax, applications, and providing practical examples to help you master its usage.
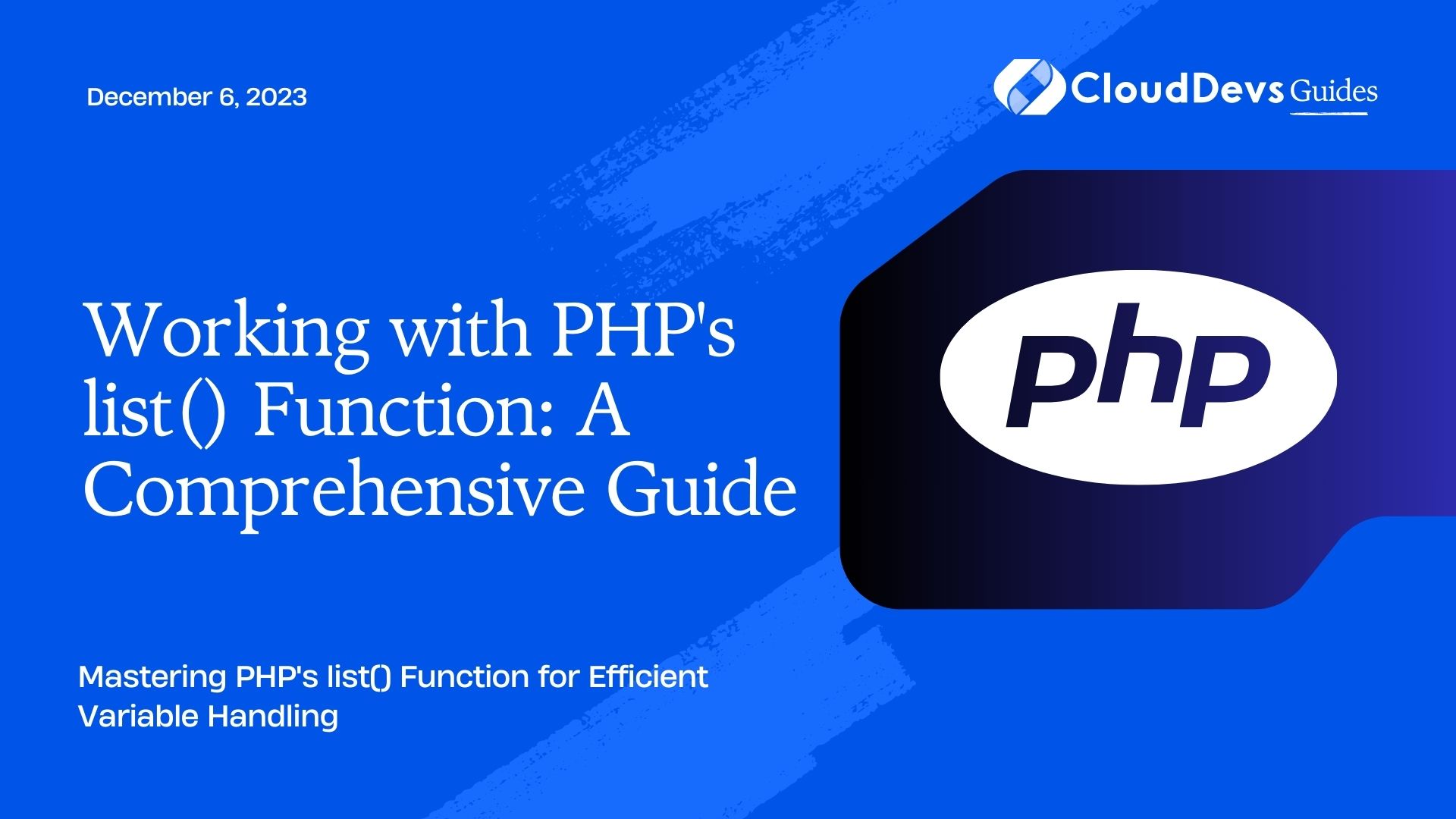
Table of Contents
1. Understanding the Basics
1.1. syntax of list().
The list() function is a unique construct in PHP that allows you to assign variables to the elements of an array in a single line of code. It follows this basic syntax:
Here’s how it works:
- The list of variables on the left side of the assignment operator (=) will be assigned values from the array on the right side.
- The order of variables on the left must match the order of elements in the array.
2. Assigning Variables from an Indexed Array
Let’s start with a simple example. Consider an indexed array:
You can use the list() function to assign values from this array to individual variables:
Now, $firstFruit will hold ‘apple’, $secondFruit will hold ‘banana’, and $thirdFruit will hold ‘cherry’.
3. Assigning Variables from an Associative Array
The list() function also works with associative arrays. Consider the following associative array:
You can assign values from this array to variables by specifying the array keys:
Now, $name will hold ‘John’, $age will hold 30, and $city will hold ‘New York’.
4. Practical Applications
4.1. swapping variables.
One of the most common use cases for the list() function is swapping the values of two variables without needing a temporary variable. Here’s an example:
This code efficiently swaps the values of $a and $b by using list() with an array on the right side of the assignment.
4.2. Exploding Strings
The list() function is extremely useful when working with data from delimited strings. Suppose you have a comma-separated string of values like this:
You can split this string into variables using the explode() function and list():
Now, $firstName holds ‘John’, $lastName holds ‘Doe’, and $age holds 30.
4.3. Fetching Data from a Database
When working with databases, you often fetch rows as associative arrays. The list() function can help you easily extract values from these arrays for further processing. Consider a database query that retrieves a user’s information:
Now, you have individual variables holding each piece of user data, making it more convenient to work with.
4.4. Dealing with Multidimensional Arrays
You can also use the list() function to extract values from multidimensional arrays. Suppose you have an array representing a student’s information:
You can extract specific grades using list():
Now, you have individual variables ($mathGrade, $scienceGrade, and $historyGrade) containing the grades for the student.
5. Advanced Usage
5.1. skipping values.
In some cases, you might not need to assign values to all variables. You can skip variables by leaving placeholders in the list. For example:
Here, the second value is skipped, and $first will be 1, while $third will be 3.
5.2. Using the list() Function with Function Returns
You can also use the list() function to directly assign values from a function return. For instance, if you have a function that returns an array of data:
This way, you can easily extract and work with the returned data.
5.3. Combining list() with foreach
list() can be combined with foreach loops to iterate through arrays and extract values conveniently. Consider an array of users’ data:
This code snippet iterates through the array of users, assigning each user’s data to variables for processing within the loop.
6. Handling Errors and Caveats
While the list() function is a powerful tool, there are some caveats and error-handling considerations to keep in mind:
6.1. Mismatched Variables and Array Elements
If the number of variables on the left side of the list() function doesn’t match the number of elements in the array, PHP will generate an error. For example:
In this case, you’ll get a “Notice: Undefined offset” error because there are more variables than elements in the array.
6.2. Indexed Arrays vs. Associative Arrays
When working with indexed arrays, the order of variables in the list() function should match the order of elements in the array. For associative arrays, the keys must match. Failing to do so will result in incorrect variable assignment.
6.3. Undefined Variables
If you try to access a variable that hasn’t been assigned a value through list(), PHP will raise an “Undefined variable” notice. Always ensure that you have assigned values to variables before using them.
The list() function in PHP is a valuable tool for simplifying variable assignment and manipulation, especially when dealing with arrays. By understanding its syntax and applications, you can enhance your coding efficiency and readability. Whether you’re swapping values, processing data from databases, or working with complex arrays, the list() function offers a concise and elegant solution.
Incorporate the knowledge gained from this comprehensive guide into your PHP projects, and you’ll find yourself writing more efficient and concise code. Experiment with various scenarios to master the versatility of the list() function and unlock its full potential in your development endeavors.
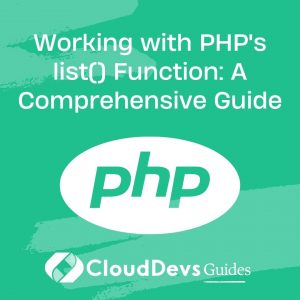
Top 15 Sites to Hire PHP Developers
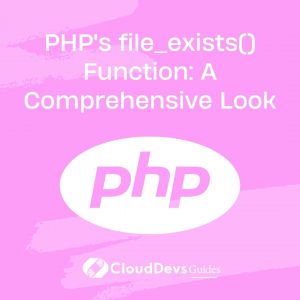
PHP’s file_exists() Function: A Comprehensive Look
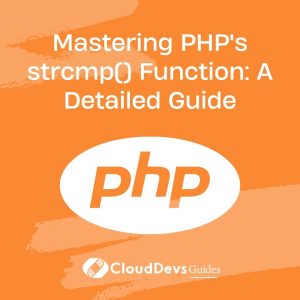
Mastering PHP’s strcmp() Function: A Detailed Guide
- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
- PHP array_chunk() Function
- PHP array_combine() Function
- PHP array_count_values() Function
- PHP array_diff_assoc() Function
- PHP array_diff_key() Function
- PHP array_diff_uassoc() Function
- PHP array_diff_ukey() Function
- PHP array_diff() function
- PHP array_fill_keys() Function
- PHP array_fill() function
- PHP array_filter() Function
- PHP array_flip() Function
- PHP array_intersect_assoc() Function
- PHP array_intersect_key() Function
- PHP array_intersect_uassoc() Function
- PHP array_intersect() Function
- PHP array_key_exists() Function
- PHP array_keys() Function
- PHP array_merge_recursive() Function
- PHP array_multisort() Function
- PHP array_pad() Function
- PHP array_pop() Function
- PHP array_product() Function
- PHP array_push() Function
- PHP array_rand() Function
- PHP array_reduce() Function
- PHP array_replace_recursive() Function
- PHP array_replace() Function
- PHP array_reverse() Function
- PHP array_search() Function
- PHP array_shift() Function
- PHP array_slice() Function
- PHP array_splice() Function
- PHP array_sum() Function
- PHP array_udiff_assoc() Function
- PHP array_udiff() Function
- PHP array_uintersect_assoc() Function
- PHP array_uintersect_uassoc() Function
- PHP array_uintersect() Function
- PHP array_unique() Function
- PHP array_unshift() Function
- PHP array_values() Function
- PHP array_walk_recursive() Function
- PHP array_walk() Function
- PHP array() Function
- PHP arsort() Function
- PHP compact() Function
- PHP count() Function
- PHP current() Function
- PHP end() Function
- PHP extract() Function
- PHP in_array() Function
- PHP key() Function
- PHP krsort() Function
- PHP ksort() Function
PHP list() Function
- PHP natcasesort() Function
- PHP natsort() Function
- PHP next() Function
- PHP pos() Function
- PHP prev() Function
- PHP range() Function
- PHP reset() Function
- PHP rsort() Function
- PHP shuffle() Function
- PHP sizeof() Function
- PHP sort() Function
- PHP uasort() Function
- PHP uksort() Function
- PHP usort() Function
- PHP each() Function
The list() function is an inbuilt function in PHP which is used to assign array values to multiple variables at a time. This function will only work on numerical arrays. When the array is assigned to multiple values, then the first element in the array is assigned to the first variable, second to the second variable, and so on, till the number of variables. The number of variables cannot exceed the length of the numerical array.
Parameter: It accepts a list of variables separated by spaces. These variables are assigned values. At least one variable must be passed to the function.
Return Value: The function returns the assigned array to the multiple variables passed. If the number of variables passed is greater than the number of elements in the array, an error is thrown.
Below programs illustrate the list() function in PHP:
Program 1: Program to demonstrate the use of list() function.
Program 2: Program to demonstrate the runtime error of list() function.
Program 3: Program to demonstrate assignment of particular index values in the array to variables.
Reference : http://php.net/manual/en/function.list.php
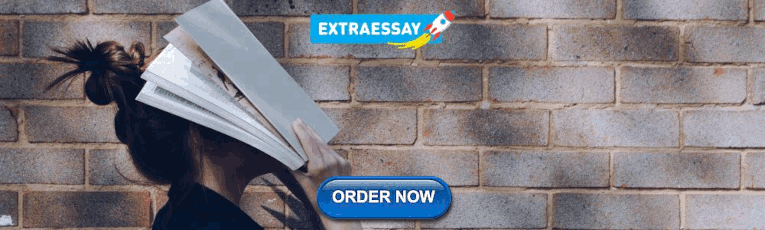
Please Login to comment...
Similar reads.
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Popular Articles
- Php Remove Last Character From String (Nov 08, 2023)
- Php Reset (Nov 08, 2023)
- Php Optional Parameters (Nov 08, 2023)
- Php Null (Nov 08, 2023)
- Php Join Array (Nov 08, 2023)
Switch to English
Table of Contents
Introduction
Understanding php list, working with multi-dimensional arrays, tips and common error-prone cases.
- The PHP List() function is used to assign values to a list of variables in a single operation. It works only on numerical arrays and assumes that the numerical indices start at 0.
- PHP List can also be used with multi-dimensional arrays. Here's an example:
- Using list() with associative arrays: The list() function does not work with associative arrays. It only works with indexed arrays and assumes that the indices start at 0.
- Not providing enough variables: If you do not provide enough variables for the values in your array, PHP will not throw an error; instead, it will just ignore any extra values.

Buckle up, fellow PHP enthusiast! We're loading up the rocket fuel for your coding adventures...
- Best Practices ,
PHP list() function (with example)
Hi everyone, I'm currently working on a PHP project and I came across the `list()` function. I'm trying to understand how it works and how I can use it effectively in my code. I've read the official PHP documentation, but I'm still a bit confused. Could someone please explain the `list()` function to me in simpler terms? I would really appreciate it if someone could provide me with an example of how the `list()` function is used in practical scenarios. This will help me grasp the concept better and apply it correctly in my own project. Thank you in advance for your help! Best regards, [Your Name]
All Replies
Hey there! I totally understand your confusion with the `list()` function. When I first came across it, I had a bit of trouble grasping its concept too. However, I've found it to be quite handy in certain situations. To give you a different perspective, let me share an example from a recent project where I used the `list()` function. I was working on a web application that displayed a list of products. Each product had a name, price, and quantity. The data was retrieved from a database in the form of an associative array. Using the `list()` function, I was able to easily assign the product details to separate variables. Here's an example code snippet: php // Assuming $productData is an associative array containing product details // Extracting the product details using the list() function list('name' => $productName, 'price' => $productPrice, 'quantity' => $productQuantity) = $productData; // Now I can use the variables individually echo "Product: " . $productName . "<br>"; echo "Price: $" . $productPrice . "<br>"; echo "Quantity: " . $productQuantity . "<br>"; In this scenario, I used the associative array's keys as reference points for assigning values to variables. By doing so, I could conveniently access and display the product details in my application. I found that the `list()` function saves me from writing additional lines of code to assign variables manually. It really simplifies the process of extracting data from arrays and makes my code more concise. I hope sharing this example helps you understand how the `list()` function can be utilized in real-world projects. If you have any further questions or need more clarification, feel free to ask. Best regards, [Your Name]
Hey there, Sure, I can help you understand the `list()` function. I've used it quite a bit in my PHP projects, so I have some practical examples to share. Simply put, the `list()` function is used to assign values from an array to a list of variables in a single operation. It allows you to easily extract specific elements from an array and assign them to variables. Here's an example to clear things up a bit: Suppose you have an array called `$userInfo` which contains the name, age, and email of a user. You can use the `list()` function to extract those values and assign them to individual variables. Check out the code snippet below: php $userInfo = array('John Doe', 25, '[email protected]'); // Using the list() function to assign values to variables list($name, $age, $email) = $userInfo; // Now you can use the variables individually echo "Name: " . $name . "<br>"; echo "Age: " . $age . "<br>"; echo "Email: " . $email . "<br>"; In this example, the `list()` function helps us assign the array values to `$name`, `$age`, and `$email` in a single line. This way, we can access and use these variables independently for further operations. It's important to note that the number of variables in the list must match the number of elements in the array, or else you may encounter unexpected behavior. I hope this example clarifies how the `list()` function works! If you have any more questions or need further clarification, feel free to ask. Best regards, [Your Name]
More Topics Related to PHP
- What are the recommended PHP versions for different operating systems?
- Can I install PHP without root/administrator access?
- How can I verify the integrity of the PHP installation files?
- Are there any specific considerations when installing PHP on a shared hosting environment?
- Is it possible to install PHP alongside other programming languages like Python or Ruby?
More Topics Related to Application
- Can I install PHP on a Unix system that already has another programming language installed, such as Node.js or Go?
- Are there any specific configurations or optimizations for PHP-FPM to improve performance?
- apache - How to set global environment variables for PHP
- get file content of google docs using google drive API v3 php in a variable
- CakePHP 3 : Define global contant variable
More Topics Related to PHP Project
- Can I use Homebrew to install specific PHP extensions or libraries on macOS?
- How can I enable or disable specific PHP extensions on Windows?
- How does PHP-FPM handle process management and resource allocation compared to other PHP execution methods?
- How can I manage and update installed PECL extensions?
- Can I create my own custom PECL extension to add functionality or integrate with specific APIs?
Popular Tags
- Best Practices
- Web Development
- Documentation
- Implementation

New to LearnPHP.org Community?

- PHP Tutorial
- Static/Dynamic Web Pages
- What's New Of PHP7?
- PHP Development Environment Setup
- PHP Commonly Used Editors
- The First PHP Program
- PHP Data Types
- PHP Variables
- PHP Variable Scope
- PHP Variable Assignment
- PHP Variable Variables
- PHP Predefined Variables
- PHP Static Variables
- PHP global and $GLOBALS
- PHP Comments
- PHP Constants
- PHP Magic Constants and Predefined Constants
- PHP Delimiter
- PHP Operators
- PHP Logical Operators
- PHP Comparison Operators
- PHP Ternary Operator
- PHP Operator Precedence
PHP Flow Control
- PHP if else
- PHP while And do while
- PHP switch case
- PHP foreach
- PHP break keyword
- PHP continue keyword
- PHP goto Operator
- PHP die() And exit() Functions
- PHP include And require
PHP Functions
- PHP Define Function
- PHP Function Parameters
- PHP Declare Parameter Types
- PHP Pass Parameters to Function
- PHP Function Return Value
- PHP Anonymous Function
- PHP Variable Function
- PHP Callback Function
- PHP Recursive Function
- PHP Single/Double Quotes
- PHP String Concatenation
- PHP String Case Conversion
- PHP String Query
- PHP String Replacement
- PHP String Interception
- PHP Remove Spaces On Both Sides Of A String
- PHP Get String Length
- PHP String Escaping And Restoration
- PHP Repeat A String
- PHP Shuffle A String
- PHP Convert String To Array
- PHP Define An Array
- PHP 2D And Multidimensional Arrays
- PHP Get Array Length
- PHP Sort Arrays
- PHP Returns The Current Element Of The Array
- PHP Move Array Pointer Up/down
- PHP Point Array Internal Pointer To Last Element
- PHP Point Array Internal Pointer To First Element
- PHP Get The Key Of Array Current Element
- PHP Get The Key-value Pair Of Array Current Element
- PHP Check Array Key Or Index Exists
- PHP foreach Iterates Over Array
- PHP Check If A Value Exists In An Array
- PHP Convert Array To String
- PHP Assign Values from An Array To Variables
PHP Date Time
- PHP Set Timezone
- PHP Get Current Time
- PHP Date Time Formatting
- PHP Calculate Time Difference
- PHP Extract Date Time From String
- PHP Check If Date And Time Are Valid
- PHP Get The Current Timestamp
- PHP Convert Date To Timestamp
- PHP Implements Countdown Function
PHP Object Oriented
- PHP Define A Class
- PHP Instantiate Object
- PHP Constructor
- PHP Destructor
- PHP Inheritance
- PHP Current Object
- PHP Namespace
- PHP Design Patterns
- PHP Magic Methods
- PHP Abstract Classes And Abstract Methods
- PHP Interface
- PHP Final Classes And Final Methods
- PHP clone Object
- PHP Check If An Object Belongs To A Class
- PHP Autoloading
Regular Expression
- PHP Regular Expression
- PHP preg_match()
- PHP preg_match_all()
- PHP preg_grep()
- PHP preg_replace()
- PHP preg_filter()
- PHP preg_split()
- PHP preg_quote()
PHP Cookie & Session
- How Cookies Stored In The Browser
- PHP Set Cookie
- PHP Get Cookie Value
- PHP Clear Cookies
- PHP Automatic Login Using Cookie
- PHP Cookies Advantages/Disadvantages
- What Is PHP Session
- PHP Start Session
- PHP Set And Get Session
- PHP Delete Session
- PHP Session VS Cookie
PHP Error & Exception handling
- PHP Exception Handling
- PHP Error Types
- PHP Error Level
- PHP Error Logs Configuration and Usage
- PHP Custom Error Handler
- PHP Mask Error
- PHP7 Error Handling
MySQL in PHP
- PHP Connect to Database
- PHP Select Database
- PHP Execute SQL Statements
- PHP Get SQL Query Results
- PHP Get Query Result Row Number
- PHP Execute Multiple SQL Statements At Once
- What Is PHP PDO
- PHP Connect To Database By PDO
- PHP Execute SQL Statements By PDO
- PHP Get Query Results By PDO
- PHP Error Handling In PDO
- PHP Set And Get PDO Attributes
PHP File Directory
- PHP Open And Close Files
- PHP Read File Content
- PHP Read A Character From A File
- PHP Read File Line By Line
- PHP Read File (Any Length)
- PHP Read Entire File Content
- PHP Read The Entire File Into An Array
- PHP Read File Into A String
- PHP Write Data To A File
- PHP Check If The File Exists
- PHP Get File Attributes
- PHP Delete, Copy, Rename Files
- PHP File Upload
- PHP Open And Close Directory
- PHP Read Files And Directories
- PHP Create Directory
- PHP Delete Directory
- PHP Iterates Over All Files And Directories Under A Directory
PHP Image Processing
- PHP GD Library
- PHP Create Canvas
- PHP Output Image
- PHP Release Image Resources
- PHP Define Colors For Images
- PHP Draw Image
- PHP Draw Text On Image
- PHP Generates Graphic Verification Code
- PHP Image Compression
- PHP Add A Watermark To An Image
- PHP JpGraph
- PHP Uses JpGraph To Create Images
PHP list(): Assign Values from An Array To A Set Of Variables
The list() function in PHP is used to assign values to a list of variables in a single operation. It works only on numerical arrays and assumes that the numerical indices start at 0.
Here's a tutorial on how to use the list() function:
Step 1: Create an Indexed Array
First, you need to create an array. An array is a special variable that allows you to store multiple values in a single variable.
Step 2: Use the list() function
You can use the list() function to assign the values of the array to variables:
Step 3: Check the Result
You can now use these variables in your code. For example:
Note: The list() function is not used as much in newer versions of PHP (from PHP 7.1 onwards) because you can now use the shorthand array destructuring syntax, which works in exactly the same way:
This code does the same thing as the list() function in the previous example. It assigns the values of the array to the variables $fruit1 , $fruit2 , and $fruit3 .
That's it! This is a basic tutorial on how to use the list() function in PHP. It's a handy function when you need to assign the values of an array to a list of variables.
How to Use list() in PHP to Assign Array Values to Variables:
- Description: list() is used to assign values from an array to individual variables in one operation.
- Example Code: $coordinates = [10, 20, 30]; list($x, $y, $z) = $coordinates; echo "X: $x, Y: $y, Z: $z"; // Outputs: X: 10, Y: 20, Z: 30
Destructuring Arrays with list() in PHP:
- Description: list() facilitates array destructuring, allowing easy assignment of values to variables.
- Example Code: $person = ['John', 25, 'New York']; list($name, $age, $city) = $person; echo "Name: $name, Age: $age, City: $city"; // Outputs: Name: John, Age: 25, City: New York
PHP list() with Indexed Arrays:
- Description: list() works seamlessly with indexed arrays, assigning values based on the order of elements.
- Example Code: $colors = ['Red', 'Green', 'Blue']; list($first, $second, $third) = $colors; echo "First: $first, Second: $second, Third: $third"; // Outputs: First: Red, Second: Green, Third: Blue
Using list() with Associative Arrays in PHP:
- Description: list() can also be used with associative arrays, assigning values based on the key names.
- Example Code: $person = ['name' => 'Alice', 'age' => 30, 'city' => 'London']; list('name' => $name, 'age' => $age, 'city' => $city) = $person; echo "Name: $name, Age: $age, City: $city"; // Outputs: Name: Alice, Age: 30, City: London
PHP list() vs Individual Variable Assignment:
- Description: list() provides a concise way to assign multiple values from an array in a single line.
- Example Code: $coordinates = [5, 10, 15]; // Using list() list($x, $y, $z) = $coordinates; echo "X: $x, Y: $y, Z: $z"; // Individual variable assignment $x = $coordinates[0]; $y = $coordinates[1]; $z = $coordinates[2]; echo "X: $x, Y: $y, Z: $z"; // Outputs are the same: X: 5, Y: 10, Z: 15
Unpacking Array Values with list() in PHP:
- Description: list() can be used to quickly unpack array values into variables, enhancing code readability.
- Example Code: $point = [25, 30, 40]; list($x, $y, $z) = $point; echo "X: $x, Y: $y, Z: $z"; // Outputs: X: 25, Y: 30, Z: 40
Advanced Usage of list() in PHP:
- Description: list() can be used in more advanced scenarios, such as nested arrays or function returns.
- Example Code: $data = [ 'person' => ['Alice', 25, 'London'], 'scores' => [90, 85, 92] ]; list('person' => list($name, $age, $city), 'scores' => list($math, $english, $science)) = $data; echo "Name: $name, Age: $age, City: $city, Math: $math, English: $english, Science: $science"; // Outputs: Name: Alice, Age: 25, City: London, Math: 90, English: 85, Science: 92
PHP list() for Skipping Unwanted Array Elements:
- Description: list() allows skipping unwanted elements when assigning values, improving flexibility.
- Example Code: $coordinates = [10, 20, 30, 40]; list(, $y, , $z) = $coordinates; echo "Y: $y, Z: $z"; // Outputs: Y: 20, Z: 40
Handling Mismatched Variable Counts with list() in PHP:
- Description: list() handles mismatched variable counts gracefully, assigning values up to the available variables.
- Example Code: $values = [1, 2, 3]; list($a, $b, $c, $d) = $values; echo "A: $a, B: $b, C: $c, D: $d"; // Outputs: A: 1, B: 2, C: 3, D:
list() Function in PHP and Its Alternatives:
- Description: list() is a convenient way for array value assignment. Alternatives include individual variable assignments and array destructuring.
- Example Code: $colors = ['Red', 'Green', 'Blue']; // Using list() list($first, $second, $third) = $colors; echo "First: $first, Second: $second, Third: $third"; // Individual variable assignment $first = $colors[0]; $second = $colors[1]; $third = $colors[2]; echo "First: $first, Second: $second, Third: $third"; // Outputs are the same: First: Red, Second: Green, Third: Blue
Common Mistakes and Pitfalls with PHP list() :
- Description: Common mistakes include mismatched variable counts and attempting to use list() with non-array values.
- Example Code: $data = [10, 20, 30]; // Mistake: Mismatched variable count list($x, $y) = $data; // Mistake: Using list() with non-array value list($value) = 42;
Tips for Efficient Variable Assignment Using list() in PHP:
- Description: Use list() when dealing with multiple values from an array, enhancing code readability.
- Example Code: $point = [15, 25, 30]; // Less efficient alternative $x = $point[0]; $y = $point[1]; $z = $point[2]; // More efficient with list() list($x, $y, $z) = $point;
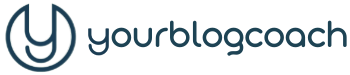
Home > Tips & Tricks > PHP list() Method – Multiple Variable Assignment from an Array
PHP list() Method – Multiple Variable Assignment from an Array
The list() method in PHP is a versatile function that allows developers to assign variables in a single operation, streamlining code and enhancing readability.
This method is particularly useful when working with arrays. It is also useful when enabling the extraction of values effortlessly and assigning them to variables in one go.
To utilize the PHP list() method , an array is required on the right-hand side of the assignment, and the variables to be assigned are listed on the left. The number of variables on the left should match the number of elements in the array.
This method is an effective way to destructure arrays and access their individual elements without the need for explicit indexing.
Let’s delve into a practical example to illustrate the usage of the list() method:
In this example, the list() method efficiently assigns the values from the $person array to the variables $firstName , $lastName , and $age . This results in concise and readable code, especially when dealing with arrays with a considerable number of elements.
Transitioning from traditional array assignment to the list() method enhances code clarity and reduces redundancy. It provides a cleaner and more expressive way to work with arrays, promoting better code organization and maintainability.
In conclusion, the list() method in PHP is a powerful tool for array manipulation, allowing developers to assign array elements to variables seamlessly. With this method, code becomes more readable, and the process of extracting values from arrays becomes more efficient and elegant.
More Tricks
- Variables Variable in PHP – Double Dollar ($$) Variable
- Extract Email Addresses From Text with PHP
- Important Tips to Sanitizing Input Data in PHP
- Increase Maximum PHP File Upload Size
- Send Email with PHP
Being Tricky 😉
Related Posts
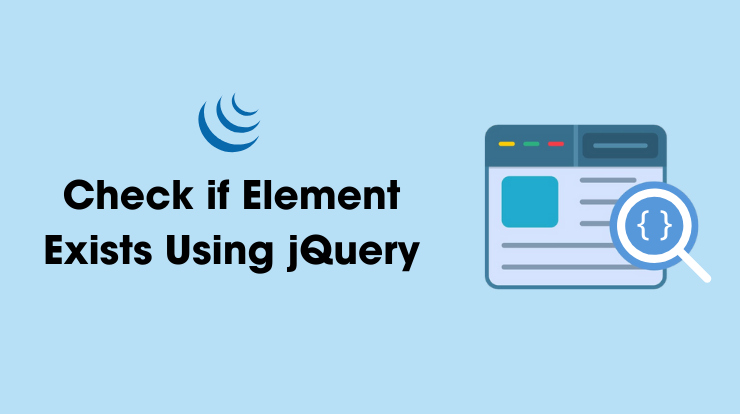
How to Check if Element Exists Using jQuery?
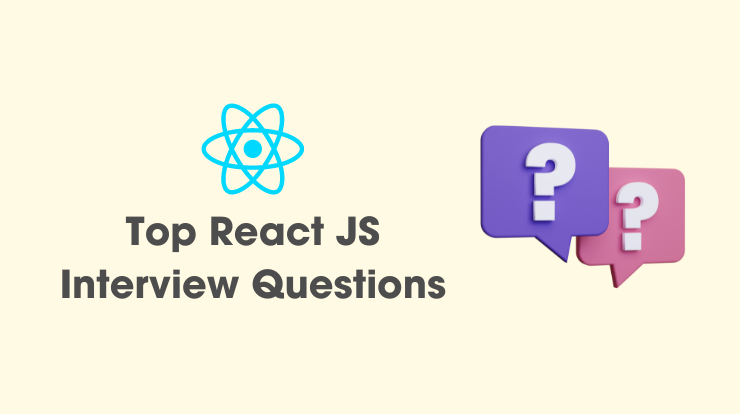
Top 100+ React JS Interview Questions And Answers
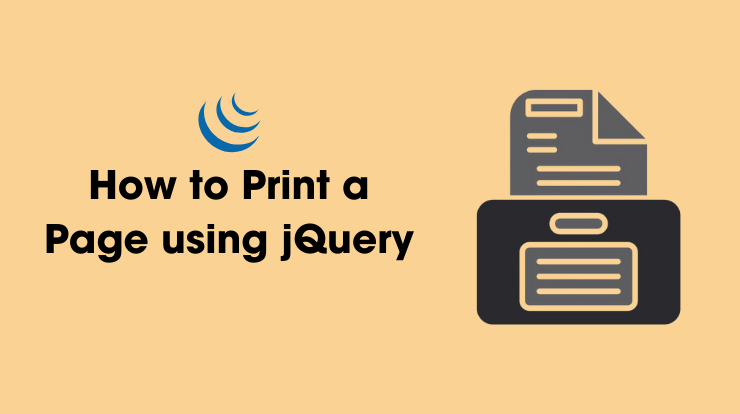
How to Print a Page using jQuery?
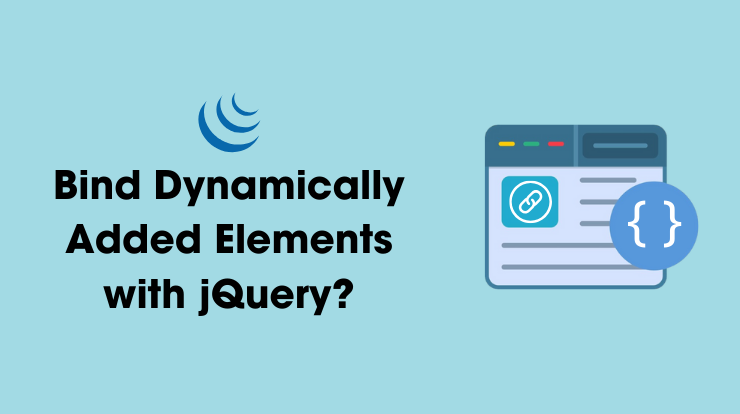
How to Bind Dynamically Added Elements with jQuery?
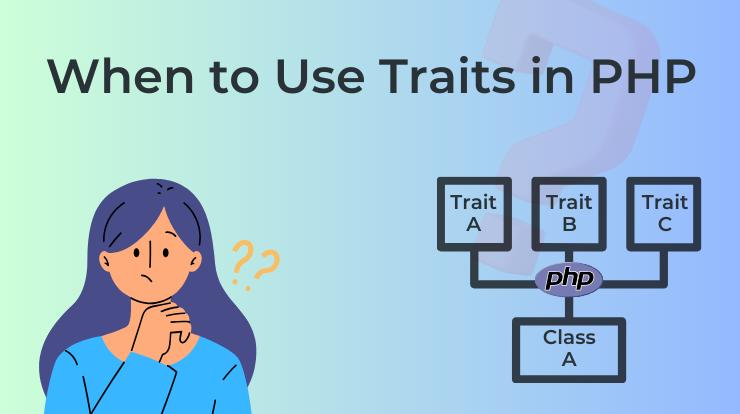
When to Use Traits in PHP – A Comprehensive Guide
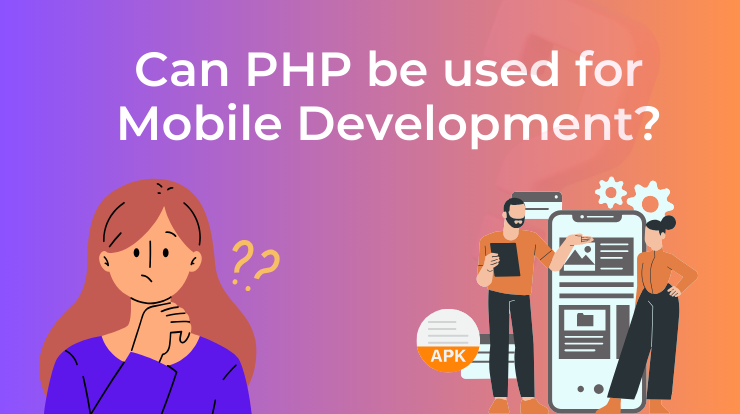
Can PHP be used for Mobile Applications Development?

About Aman Mehra
Leave a comment cancel reply.
Save my name, email, and website in this browser for the next time I comment.
Privacy Overview
PHP RFC: Default Value in List Assignment Syntax
- Version: 0.1
- Date: 2015-11-08
- Author: Reeze Xia, [email protected]
- Status: Under Discussion
- First Published at: http://wiki.php.net/rfc/list_default_value
- Introduction
We could destruct variables from an array with list constuct , it may be nested or a simple array. But there is no guarantee that the array can fulfill all variables, it will be simple assigned with null with notice error (not good). We will need several redundant code to handle the possible cases if we want to check it and assign it with default values.
For the similar reason we introduced Null coalesce "??" and Ternary Operator "?:" to help write clean code.
Some clever users figured out some workaround like this User contributed notes . But that is not good enough and ugly.
Support new syntax to set default values for list elements, when the requested index it is not found in array:
The assignment could be considered as a shortcut for:
This seems not a lot of work than the new syntax. But sometimes we need to destruct nested array and there are morn than two variables, anyway list() itself is designed to avoid assign by hand for short and clean code.
The syntax is:
The syntax is same as function's default value for parameters.
We could also set default value in foreach list context:
More case could be found from PR's tests: https://github.com/php/php-src/pull/1623/files
This feature could also be found in Clojure and Javascript for destructing.
- Backward Incompatible Changes
No BC break.
- Proposed PHP Version(s)
I am working on opcache compatibility.
- Open Issues
None for now
- Future Scope
- Proposed Voting Choices
* Whether accept the RFC for PHP 7.1
This project requires a 2/3 majority (see voting )
- Patches and Tests
* Patch: https://github.com/php/php-src/pull/1623
I am working on opcache compatibility
- Implementation
After the project is implemented, this section should contain
- the version(s) it was merged to
- a link to the git commit(s)
- a link to the PHP manual entry for the feature
- Javascript destructing: https://hacks.mozilla.org/2015/05/es6-in-depth-destructuring/
- Clojure destructing https://clojurebridge.github.io/community-docs/docs/clojure/destructuring/
- Rejected Features
- v0.1 - Initial version
- Show pagesource
- Old revisions
- Back to top
Table of Contents
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.

PHP Exercises
Tutorials Class provides you exercises on various PHP Topics such as PHP basics, variables, operators, loops, forms, and database. This page is an Index for PHP Exercises.
Choose the PHP Exercise type that you want to practice. Practice your PHP skills using PHP Exercises & Assignments.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.
Write a program to count 5 to 15 using PHP loop
Write a program to print “hello world” using echo, write a program to print “hello php” using variable, write a program to print a string using echo+variable., write a program to print two variables in single echo, write a program to check student grade based on marks, write a program to show day of the week using switch, write a factorial program using for loop in php, factorial program in php using recursive function, write a program to create chess board in php using for loop, write a program to create given pattern with * using for loop, simple tips for php beginners, experiment with basic php syntax errors, using phpinfo() – display php configuration & modules, write a php program to add two numbers, write a program to calculate electricity bill in php, write a simple calculator program in php using switch case, remove specific element by value from an array in php, write a php program to check if a person is eligible to vote, write a php program to calculate area of rectangle.
- PHP Exercises Categories
- PHP All Exercises & Assignments
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
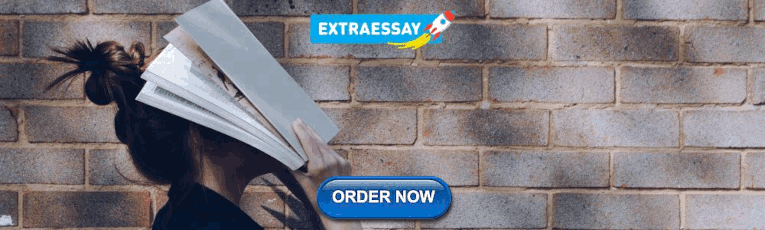
IMAGES
VIDEO
COMMENTS
Support for reference assignments in array destructuring was added. 7.1.0: It is now possible to specify keys in list(). This enables destructuring of arrays with non-integer or non-sequential keys. ... As of PHP 7.1.0 list() can now also contain explicit keys, which can be given as arbitrary expressions. Mixing of integer and string keys is ...
Definition and Usage. The list () function is used to assign values to a list of variables in one operation. Note: Prior to PHP 7.1, this function only worked on numerical arrays.
In this tutorial, you'll learn how to use the PHP list syntax to assign multiple variables in one operation.
The PHP Package Repository, Like array(), this is not really a function, but a language construct. list() is used to assign a list of variables in one operation. Strings can not be unpacked and list() expressions can not be completely empty.. Note: . Before PHP 7.1.0, list() only worked on numerical arrays and assumes the numerical indices start at 0.
The list () construct in PHP is used to assign a list of variables in one operation. The list () construct only works with numeric arrays and assumes the numerical indices start at 0. The list () construct cannot be used with associative arrays. The list () construct can be used with arrays only. The list () construct removes elements from the ...
The list () function is a unique construct in PHP that allows you to assign variables to the elements of an array in a single line of code. It follows this basic syntax: Here's how it works: The list of variables on the left side of the assignment operator (=) will be assigned values from the array on the right side.
The list() function is an inbuilt function in PHP which is used to assign array values to multiple variables at a time. This function will only work on numerical arrays. When the array is assigned to multiple values, then the first element in the array is assigned to the first variable, second to the second variable, and so on, till the number of variables.
Get the Leng of a String: strlen() Search for a Substring in a String: substr() Locate the first Occurrence of a Substring: strpos() Replace All Occurrences of a Substring: str_replace()
As you can see, the list() function can handle complex assignments involving multiple dimensions. Tips and Common Error-Prone Cases While PHP List is a powerful tool, there are some common mistakes that developers often make: Using list() with associative arrays: The list() function does not work with associative arrays.
I found that the `list()` function saves me from writing additional lines of code to assign variables manually. It really simplifies the process of extracting data from arrays and makes my code more concise. I hope sharing this example helps you understand how the `list()` function can be utilized in real-world projects.
The shorthand array syntax ([]) may now be used to destructure arrays for assignments (including within foreach), as an alternative to the existing list() syntax, which is still supported. ... The following function implements both PHP 7.1 list construction and allows to extract properties from objects with renaming support.
The list () function is used to assign values to a list of variables. Like array (), this is not really a function, but a language construct. list () is used to assign a list of variables in one operation. Version: (PHP 4 and above) Syntax: list (varname1, varname2.... varnamen ) Parameters: Name.
Step 1: Create an Indexed Array. First, you need to create an array. An array is a special variable that allows you to store multiple values in a single variable. Step 2: Use the list() function. You can use the list() function to assign the values of the array to variables: Step 3: Check the Result.
The examples on the PHP manual page for list() are especially illuminating: Basically, your list can be as long as you want, but it is an absolute list. In other words the order of the items in the array obviously matters, and to skip things, you have to leave the corresponding spots empty in your list(). Finally, you can't list string.
The list() method in PHP is a versatile function that allows developers to assign variables in a single operation, streamlining code and enhancing readability.. This method is particularly useful when working with arrays. It is also useful when enabling the extraction of values effortlessly and assigning them to variables in one go.
This RFC proposes introducing a second syntax form for destructuring assignment, where list (and ) ... Nikita Popov's Abstract Syntax Tree RFC, which was accepted into PHP 7, noted that a short list syntax like this would not be possible without having the abstract syntax tree.
Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database. Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions. Here, you will find a list of PHP programs, along with problem description and solution.
But sometimes we need to destruct nested array and there are morn than two variables, anyway list() itself is designed to avoid assign by hand for short and clean code. The syntax is: assignment_list_element: variable + | variable '=' expr # the new syntax. value could be any expression | T_LIST '(' assignment_list ')' | /* empty */
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
Weekly Trends and Language Statistics. PHP Exercises, Practice, Solution: PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.
Tutorials Class provides you exercises on various PHP Topics such as PHP basics, variables, operators, loops, forms, and database. This page is an Index for PHP Exercises. Choose the PHP Exercise type that you want to practice. Practice your PHP skills using PHP Exercises & Assignments. Here, you will find a list of PHP programs, along with ...
Houston Astros outfielder Chas McCormick, sidelined since May 1, was activated from the injured list after a six-game minor league rehab assignment.