Browse Course Material
Course info, instructors.
- Dr. Ana Bell
- Prof. Eric Grimson
- Prof. John Guttag
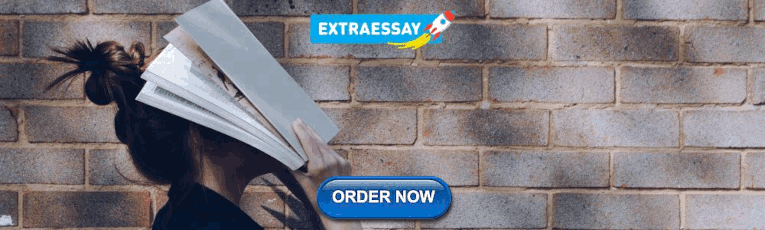
Departments
- Electrical Engineering and Computer Science
As Taught In
- Algorithms and Data Structures
- Programming Languages
Learning Resource Types
Introduction to computer science and programming in python, lecture slides and code.
The slides and code from each lecture are available below.

You are leaving MIT OpenCourseWare
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Introduction to Programming Languages
- Introduction to Visual Programming Language
- Introduction to the C99 Programming Language : Part III
- The Evolution of Programming Languages
- Bhailang - A Toy Programming Language
- Lisp vs Erlang Programming Language
- Introduction of Object Oriented Programming
- 5 Best Languages for Competitive Programming
- Introduction to C++ Programming Language
- Ruby Programming Language (Introduction)
- Go Programming Language (Introduction)
- Introduction to Scripting Languages
- Introduction to Go Programming
- Introduction of Programming Paradigms
- Generation of Programming Languages
- Introduction to GUI Programming in C++
- Introduction to Processing | Java
- C Programming Language Standard
- GFact | Bootstrapped Programming languages
- Introduction to LISP
Introduction:
A programming language is a set of instructions and syntax used to create software programs. Some of the key features of programming languages include:
- Syntax : The specific rules and structure used to write code in a programming language.
- Data Types : The type of values that can be stored in a program, such as numbers, strings, and booleans.
- Variables : Named memory locations that can store values.
- Operators : Symbols used to perform operations on values, such as addition, subtraction, and comparison.
- Control Structures : Statements used to control the flow of a program, such as if-else statements, loops, and function calls.
- Libraries and Frameworks: Collections of pre-written code that can be used to perform common tasks and speed up development.
- Paradigms : The programming style or philosophy used in the language, such as procedural, object-oriented, or functional.
Examples of popular programming languages include Python, Java, C++, JavaScript, and Ruby. Each language has its own strengths and weaknesses and is suited for different types of projects.
A programming language is a formal language that specifies a set of instructions for a computer to perform specific tasks. It’s used to write software programs and applications, and to control and manipulate computer systems. There are many different programming languages, each with its own syntax, structure, and set of commands. Some of the most commonly used programming languages include Java, Python, C++, JavaScript, and C#. The choice of programming language depends on the specific requirements of a project, including the platform being used, the intended audience, and the desired outcome. Programming languages continue to evolve and change over time, with new languages being developed and older ones being updated to meet changing needs.
Are you aiming to become a software engineer one day? Do you also want to develop a mobile application that people all over the world would love to use? Are you passionate enough to take the big step to enter the world of programming? Then you are in the right place because through this article you will get a brief introduction to programming. Now before we understand what programming is, you must know what is a computer. A computer is a device that can accept human instruction, processes it, and responds to it or a computer is a computational device that is used to process the data under the control of a computer program. Program is a sequence of instruction along with data.
The basic components of a computer are:
- Central Processing Unit(CPU)
- Output unit
The CPU is further divided into three parts-
- Memory unit
- Control unit
- Arithmetic Logic unit
Most of us have heard that CPU is called the brain of our computer because it accepts data, provides temporary memory space to it until it is stored(saved) on the hard disk, performs logical operations on it and hence processes(here also means converts) data into information. We all know that a computer consists of hardware and software. Software is a set of programs that performs multiple tasks together. An operating system is also software (system software) that helps humans to interact with the computer system. A program is a set of instructions given to a computer to perform a specific operation. or computer is a computational device that is used to process the data under the control of a computer program. While executing the program, raw data is processed into the desired output format. These computer programs are written in a programming language which are high-level languages. High level languages are nearly human languages that are more complex than the computer understandable language which are called machine language, or low level language. So after knowing the basics, we are ready to create a very simple and basic program. Like we have different languages to communicate with each other, likewise, we have different languages like C, C++, C#, Java, python, etc to communicate with the computers. The computer only understands binary language (the language of 0’s and 1’s) also called machine-understandable language or low-level language but the programs we are going to write are in a high-level language which is almost similar to human language. The piece of code given below performs a basic task of printing “hello world! I am learning programming” on the console screen. We must know that keyboard, scanner, mouse, microphone, etc are various examples of input devices, and monitor(console screen), printer, speaker, etc are examples of output devices.
At this stage, you might not be able to understand in-depth how this code prints something on the screen. The main() is a standard function that you will always include in any program that you are going to create from now onwards. Note that the execution of the program starts from the main() function. The clrscr() function is used to see only the current output on the screen while the printf() function helps us to print the desired output on the screen. Also, getch() is a function that accepts any character input from the keyboard. In simple words, we need to press any key to continue(some people may say that getch() helps in holding the screen to see the output). Between high-level language and machine language, there are assembly languages also called symbolic machine code. Assembly languages are particularly computer architecture specific. Utility program ( Assembler ) is used to convert assembly code into executable machine code. High Level Programming Language is portable but requires Interpretation or compiling to convert it into a machine language that is computer understood. Hierarchy of Computer language –

There have been many programming languages some of them are listed below:
Most Popular Programming Languages –
Characteristics of a programming Language –
- A programming language must be simple, easy to learn and use, have good readability, and be human recognizable.
- Abstraction is a must-have Characteristics for a programming language in which the ability to define the complex structure and then its degree of usability comes.
- A portable programming language is always preferred.
- Programming language’s efficiency must be high so that it can be easily converted into a machine code and its execution consumes little space in memory.
- A programming language should be well structured and documented so that it is suitable for application development.
- Necessary tools for the development, debugging, testing, maintenance of a program must be provided by a programming language.
- A programming language should provide a single environment known as Integrated Development Environment(IDE).
- A programming language must be consistent in terms of syntax and semantics.
Basic Terminologies in Programming Languages:
- Algorithm : A step-by-step procedure for solving a problem or performing a task.
- Variable : A named storage location in memory that holds a value or data.
- Data Type : A classification that specifies what type of data a variable can hold, such as integer, string, or boolean.
- Function : A self-contained block of code that performs a specific task and can be called from other parts of the program.
- Control Flow : The order in which statements are executed in a program, including loops and conditional statements.
- Syntax : The set of rules that govern the structure and format of a programming language.
- Comment : A piece of text in a program that is ignored by the compiler or interpreter, used to add notes or explanations to the code.
- Debugging : The process of finding and fixing errors or bugs in a program.
- IDE : Integrated Development Environment, a software application that provides a comprehensive development environment for coding, debugging, and testing.
- Operator : A symbol or keyword that represents an action or operation to be performed on one or more values or variables, such as + (addition), – (subtraction), * (multiplication), and / (division).
- Statement : A single line or instruction in a program that performs a specific action or operation.
Basic Example Of Most Popular Programming Languages:
Here the basic code for addition of two numbers are given in some popular languages (like C, C++,Java, Python, C#, JavaScript etc.).
Advantages of programming languages:
- Increased Productivity: Programming languages provide a set of abstractions that allow developers to write code more quickly and efficiently.
- Portability: Programs written in a high-level programming language can run on many different operating systems and platforms.
- Readability : Well-designed programming languages can make code more readable and easier to understand for both the original author and other developers.
- Large Community: Many programming languages have large communities of users and developers, which can provide support, libraries, and tools.
Disadvantages of programming languages:
- Complexity : Some programming languages can be complex and difficult to learn, especially for beginners.
- Performance : Programs written in high-level programming languages can run slower than programs written in lower-level languages.
- Limited Functionality : Some programming languages may not have built-in support for certain types of tasks or may require additional libraries to perform certain functions.
- Fragmentation: There are many different programming languages, which can lead to fragmentation and make it difficult to share code and collaborate with other developers.
Tips for learning new programming language:
- Start with the fundamentals : Begin by learning the basics of the language, such as syntax, data types, variables, and simple statements. This will give you a strong foundation to build upon.
- Code daily : Like any skill, the only way to get good at programming is by practicing regularly. Try to write code every day, even if it’s just a few lines.
- Work on projects : One of the best ways to learn a new language is to work on a project that interests you. It could be a simple game, a web application, or anything that allows you to apply what you’ve learned that is the most important part.
- Read the documentation : Every programming language has documentation that explains its features, syntax, and best practices. Make sure to read it thoroughly to get a better understanding of the language.
- Join online communities : There are many online communities dedicated to programming languages, where you can ask questions, share your code, and get feedback. Joining these communities can help you learn faster and make connections with other developers.
- Learn from others : Find a mentor or someone who is experienced in the language you’re trying to learn. Ask them questions, review their code, and try to understand how they solve problems.
- Practice debugging : Debugging is an essential skill for any programmer, and you’ll need to do a lot of it when learning a new language. Make sure to practice identifying and fixing errors in your code.
Please Login to comment...
Similar reads.
- Programming Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Home PowerPoint Templates Programming
Programming PowerPoint Templates & Slide Designs for Presentations
Download 100% editable presentation templates on programming. Our programming slide designs can help prepare presentations for various topics, such as slides for learning to code, development frameworks, and more. Download editable programming presentations to prepare presentations to learn programming topics and software development, or prepare programming training courses.
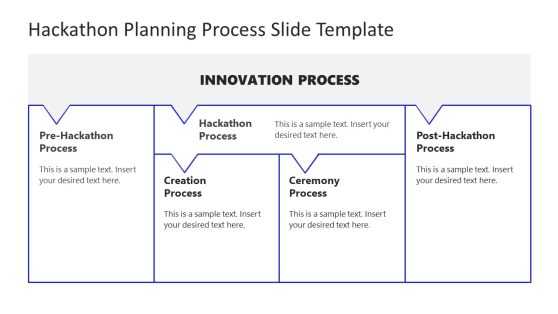
Hackathon Planning Process PowerPoint Template
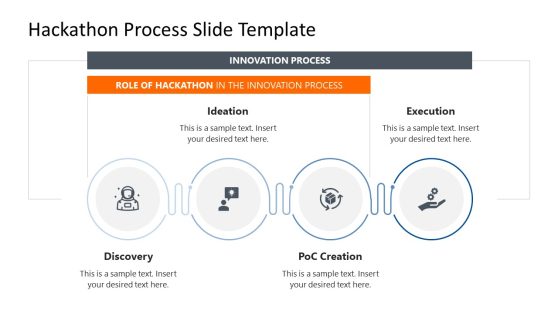
Hackathon Slide Template for PowerPoint
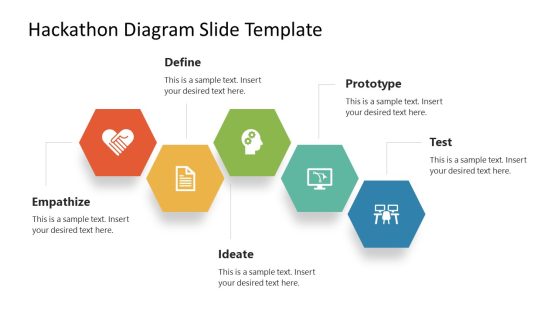
Hackathon Diagram Slide Template for PowerPoint
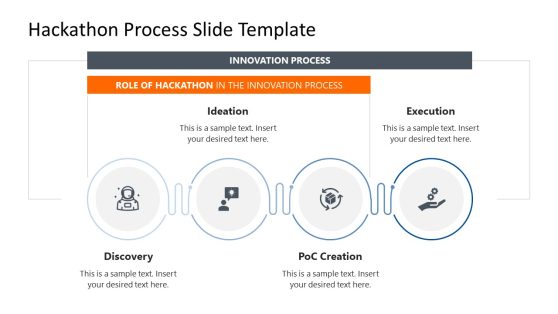
Hackathon Process PowerPoint Template
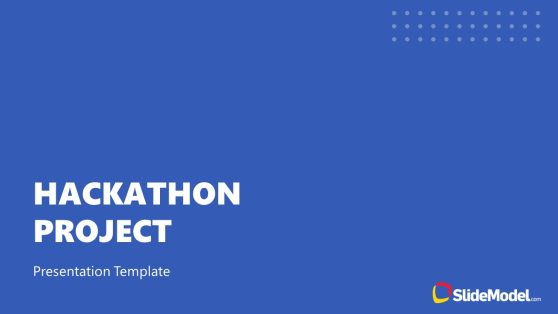
Hackathon Project PowerPoint Template
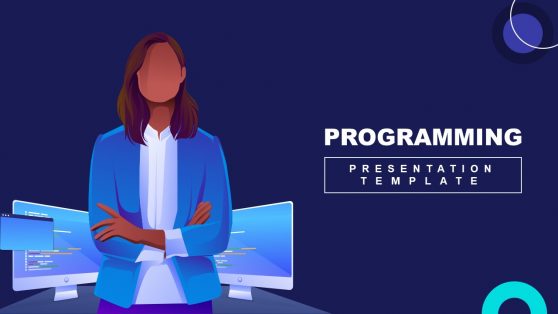
Programming PowerPoint Template
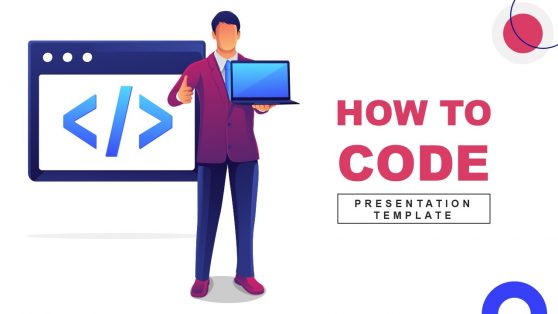
How to Code Presentation Template
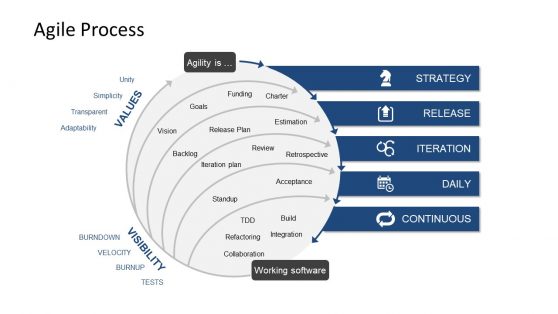
Extreme Programming PowerPoint Templates
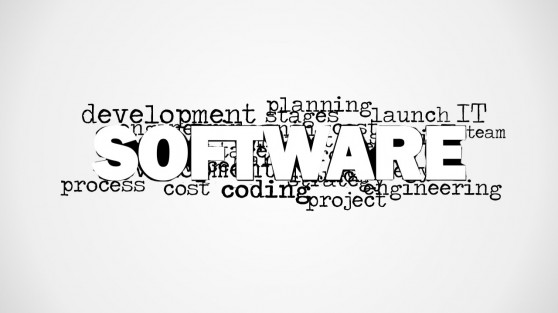
Software Word Cloud Picture for PowerPoint
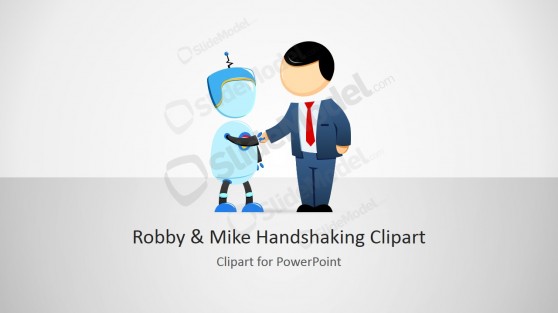
Robby & Mike Cartoon Handshaking Clipart
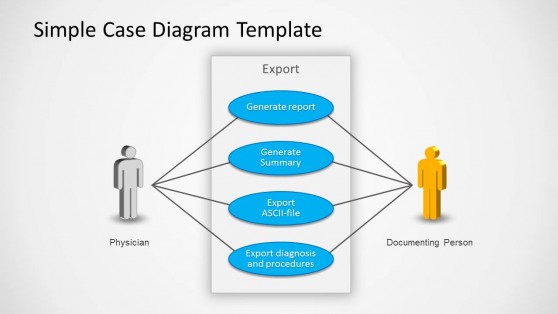
Use Case PowerPoint Diagram
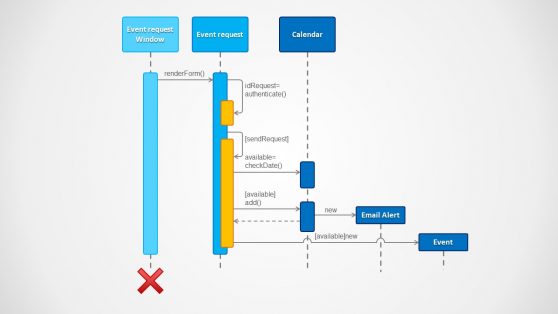
Sequence Diagram for PowerPoint
Computer programming involves a specific logical process, typically designing and developing a command-line computer program. Because the world now revolves around technology, programming is a highly valued and in-demand skill. A variety of institutions frequently offer programming or coding courses and diplomas. As a result, we have a helpful How to Code Template for every programmer. Programming presentations will require Well-presented Programming templates. Creating a visual slide for your project defense, proposals, product pitching, or other purposes using our Programming Powerpoint Templates will make your defense easier. All you need to do is edit one of our fully customizable templates.
A programmer will usually illustrate the project’s progress from start to finish. Check out our fully editable Extreme Programming PowerPoint Templates to save time. These PPT Slides are simple to use in terms of download and editing. Using a UML Diagram can help you make your message more understandable.
On the other hand, the inclusion of codes and other programming languages has made creating a Programming PowerPoint presentation somewhat tricky. We have compiled a list of Programming Presentation Templates that are visually appealing to the audience and effectively convey knowledge in our catalog. Our PowerPoint slides and layouts with creative programming backgrounds, PowerPoint shapes, diagrams, and other relevant icons efficiently represent codes and other programming languages.
What is programming used for?
Programming aims to find instructions that will automate the execution of a task on a computer, often to solve a specific problem. Also, the act of programming is used in software development.
Is there a distinction to be made between programming and coding?
Yes, Programming is a process that involves the ratification of codes to create programs. In contrast, coding is a subset of programming that deals with writing codes that a machine can understand. As a result, coding necessitates basic programming knowledge without using any software tools.
What is the importance of a Pre-designed PowerPoint template presentation?
Powerpoint Templates are ready-to-use presentations that can insert your information. It has a distinct theme color, style, effects, fonts, shapes, and template background. Visit SlideModel to find more visually appealing pre-designed presentation templates.
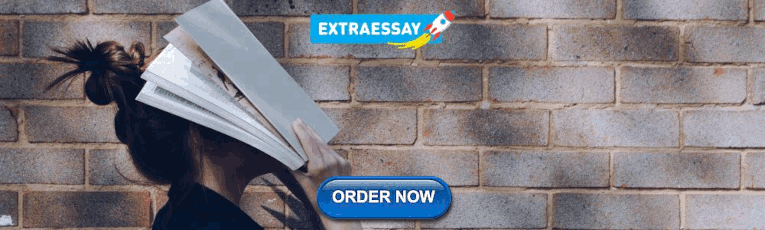
Download Unlimited Content
Our annual unlimited plan let you download unlimited content from slidemodel. save hours of manual work and use awesome slide designs in your next presentation..
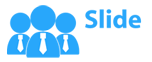
- Programming Language
- Popular Categories
Powerpoint Templates
Icon Bundle
Kpi Dashboard
Professional
Business Plans
Swot Analysis
Gantt Chart
Business Proposal
Marketing Plan
Project Management
Business Case
Business Model
Cyber Security
Business PPT
Digital Marketing
Digital Transformation
Human Resources
Product Management
Artificial Intelligence
Company Profile
Acknowledgement PPT
PPT Presentation
Reports Brochures
One Page Pitch
Interview PPT
All Categories
Powerpoint Templates and Google slides for Programming Language
Save your time and attract your audience with our fully editable ppt templates and slides..
Item 1 to 60 of 215 total items
- You're currently reading page 1

Introduce your topic and host expert discussion sessions with this Programming Language Powerpoint Ppt Template Bundles. This template is designed using high-quality visuals, images, graphics, etc, that can be used to showcase your expertise. Different topics can be tackled using the Fourteen slides included in this template. You can present each topic on a different slide to help your audience interpret the information more effectively. Apart from this, this PPT slideshow is available in two screen sizes, standard and widescreen making its delivery more impactful. This will not only help in presenting a birds-eye view of the topic but also keep your audience engaged. Since this PPT slideshow utilizes well-researched content, it induces strategic thinking and helps you convey your message in the best possible manner. The biggest feature of this design is that it comes with a host of editable features like color, font, background, etc. So, grab it now to deliver a unique presentation every time.
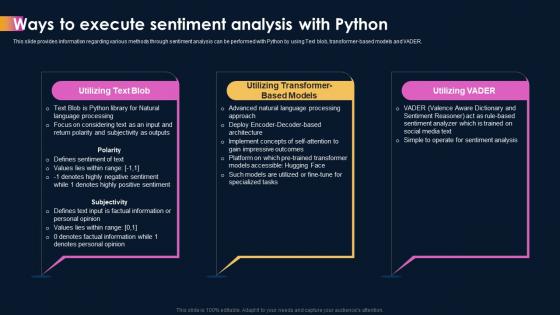
This slide provides information regarding various methods through sentiment analysis can be performed with Python by using Text blob, transformer-based models and VADER. Introducing Ai Powered Sentiment Analysis Ways To Execute Sentiment Analysis With Python AI SS to increase your presentation threshold. Encompassed with three stages, this template is a great option to educate and entice your audience. Dispence information on Sentiment, Analysis, Transformer, using this template. Grab it now to reap its full benefits.
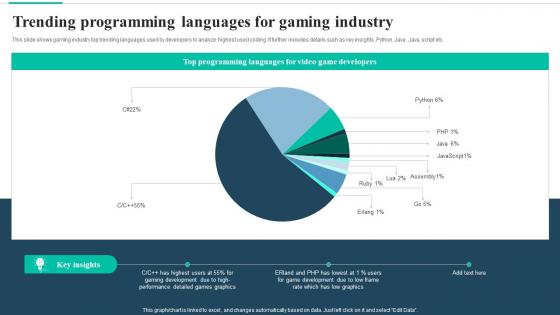
This slide shows gaming industry top trending languages used by developers to analyze highest used coding .It further includes details such as key insights ,Python ,Java ,Java script etc. Presenting our well structured Trending Programming Languages For Gaming Industry. The topics discussed in this slide are Java, Python, Scala. This is an instantly available PowerPoint presentation that can be edited conveniently. Download it right away and captivate your audience.
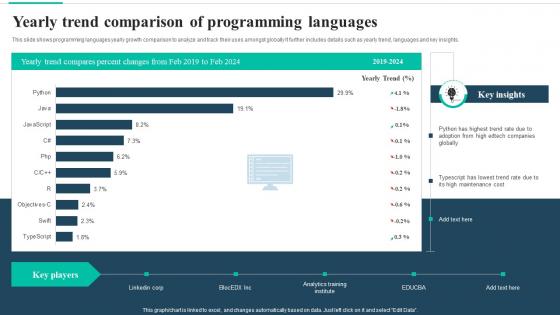
This slide shows programming languages yearly growth comparison to analyze and track their uses amongst globally It further includes details such as yearly trend, languages and key insights. Presenting our well structured Yearly Trend Comparison Of Programming Languages. The topics discussed in this slide are Edtech Companies Globally, Maintenance Cost. This is an instantly available PowerPoint presentation that can be edited conveniently. Download it right away and captivate your audience.
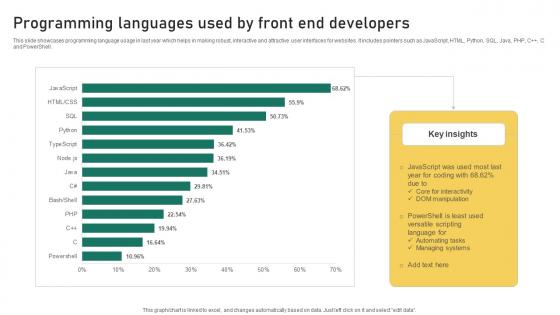
This slide showcases programming language usage in last year which helps in making robust, interactive and attractive user interfaces for websites. It includes pointers such as JavaScript, HTML, Python, SQL, Java, PHP, C, C and PowerShell. Introducing our Programming Languages Used By Front End Developers set of slides. The topics discussed in these slides are Programming Languages Used, Front End Developers. This is an immediately available PowerPoint presentation that can be conveniently customized. Download it and convince your audience.
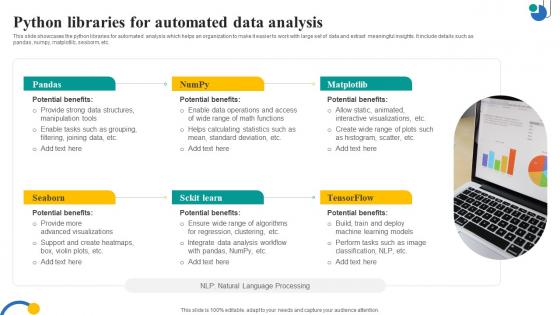
This slide showcases the python libraries for automated analysis which helps an organization to make it easier to work with large set of data and extraxt meaningful insights. It include details such as pandas, numpy, matplotlib, seaborm, etc. Introducing our premium set of slides with Python Libraries For Automated Data Analysis. Ellicudate the six stages and present information using this PPT slide. This is a completely adaptable PowerPoint template design that can be used to interpret topics like Pandas, Sckit Learn, Tensorflow. So download instantly and tailor it with your information.
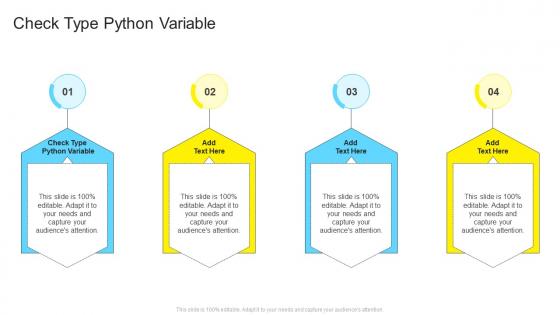
Presenting Check Type Python Variable In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Check Type Python Variable. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
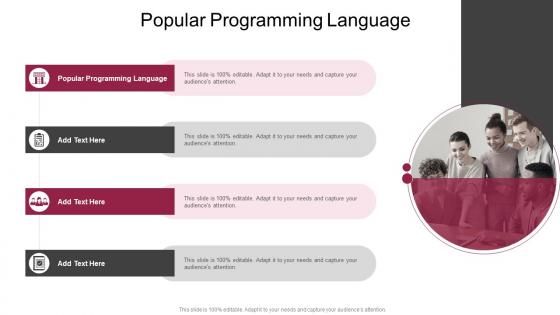
Presenting Popular Programming Language In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase Four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Popular Programming Language. This well-structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
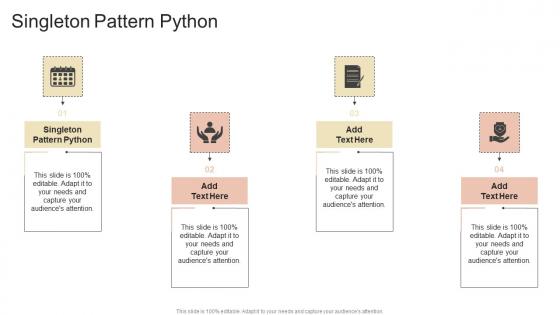
Presenting Singleton Pattern Python In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Singleton Pattern Python. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
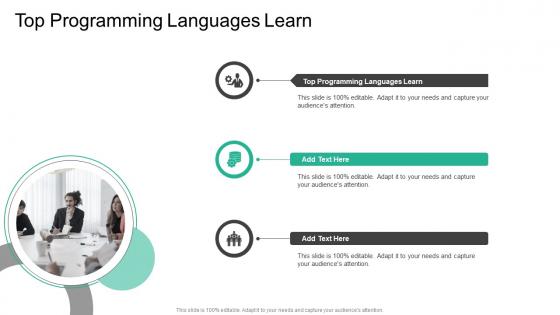
Presenting Top Programming Languages Learn In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase five stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Top Programming Languages Learn. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
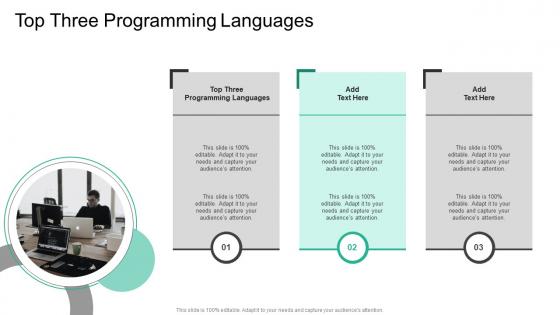
Presenting Top Three Programming Languages In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase five stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Top Three Programming Languages. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
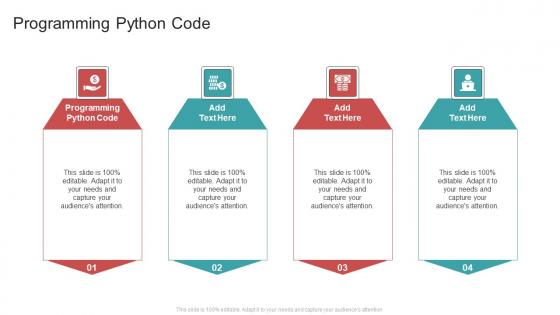
Presenting Programming Python Code In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Programming Python Code. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
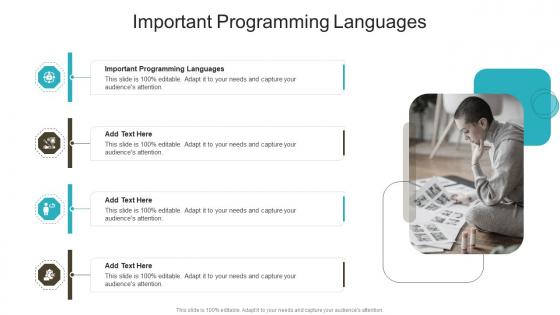
Presenting Important Programming Languages In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Important Programming Languages. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
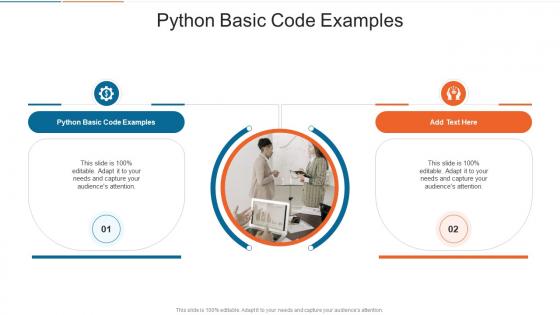
Presenting Python Basic Code Examples In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase two stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Python Basic Code Examples. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
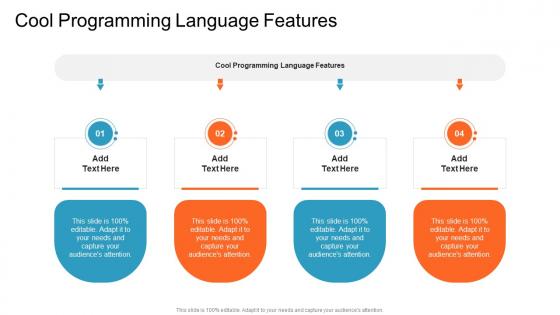
Presenting our Cool Programming Language Features In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Cool Programming Language Features. This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
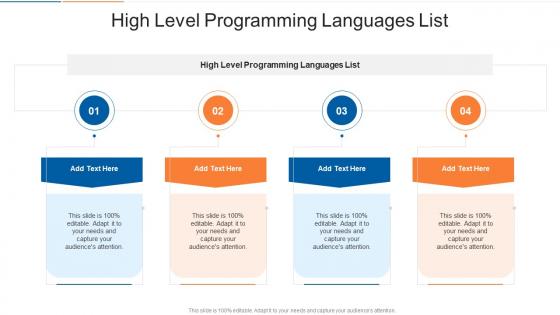
Presenting High Level Programming Languages List In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like High Level Programming Languages List. This well-structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
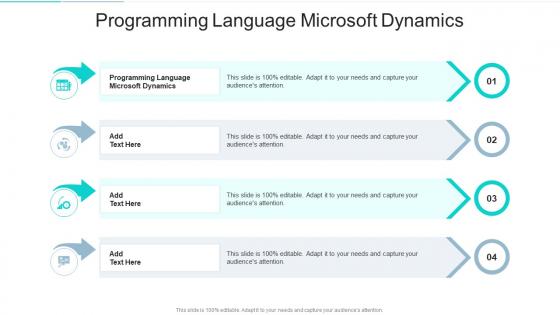
Presenting Programming Language Microsoft Dynamics In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Programming Language Microsoft Dynamics. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
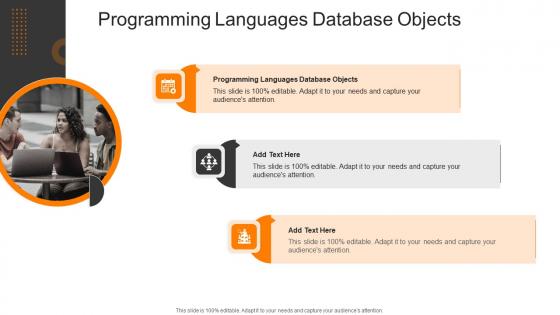
Presenting our Programming Languages Database Objects In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Programming Languages Database Objects This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.

Presenting our Best Places Learn Python In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Best Places Learn Python. This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
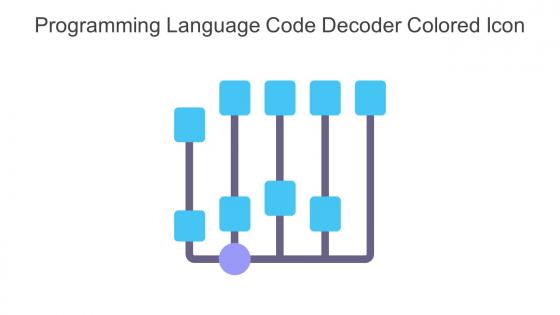
This powerpoint icon is a vibrant, multi coloured symbol that conveys the idea of decoding and problem solving. It is perfect for presentations, websites and other digital projects that require a modern, eye catching icon. The icon is available in a variety of colours and sizes, making it easy to customise to your projects needs.
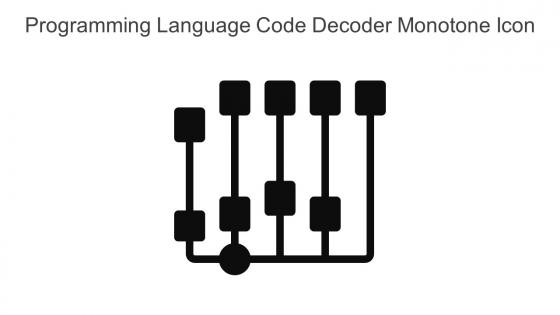
Monotone Powerpoint Icon is a perfect choice for presentations. It is a professional looking icon that is easy to use and customize. It can be used to create a modern and stylish look for any presentation. It is also available in different sizes and colors to suit your needs.
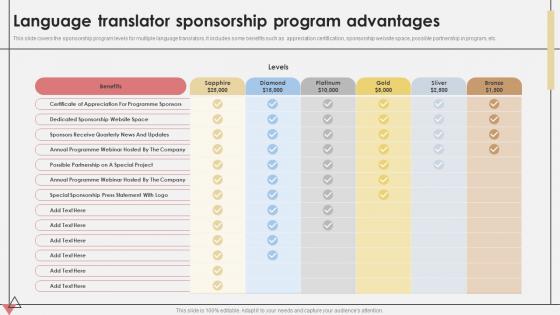
This slide covers the sponsorship program levels for multiple language translators. It includes some benefits such as appreciation certification, sponsorship website space, possible partnership in program, etc. Presenting our well structured Language Translator Sponsorship Program Advantages. The topics discussed in this slide are Programme, Website, Company. This is an instantly available PowerPoint presentation that can be edited conveniently. Download it right away and captivate your audience.
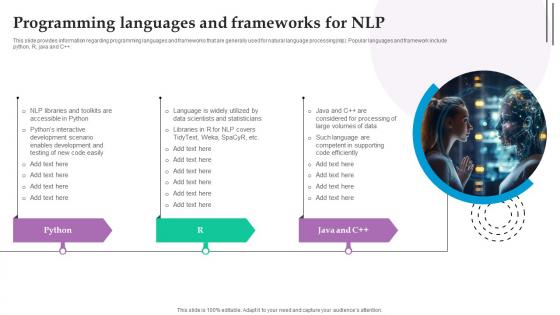
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingnlp. Popular languages and framework include python, R, java and C. Deliver an outstanding presentation on the topic using this Programming Languages And Frameworks NLP Role Of NLP In Text Summarization And Generation AI SS V. Dispense information and present a thorough explanation of Python, NLP Libraries, Language Widely Utilized using the slides given. This template can be altered and personalized to fit your needs. It is also available for immediate download. So grab it now.
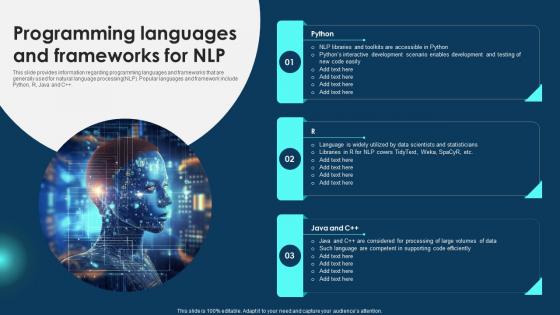
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and C. Deliver an outstanding presentation on the topic using this Programming Languages And Frameworks Zero To NLP Introduction To Natural Language Processing AI SS V. Dispense information and present a thorough explanation of Python, Development Scenario, Considered Processing using the slides given. This template can be altered and personalized to fit your needs. It is also available for immediate download. So grab it now.
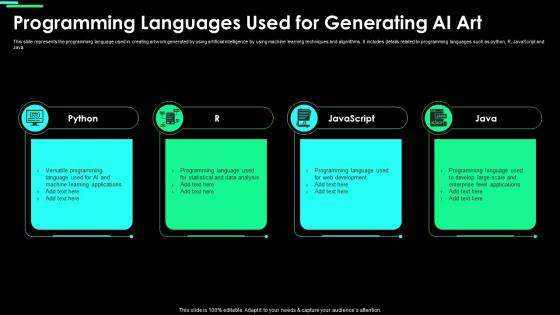
This slide represents the programming language used in creating artwork generated by using artificial intelligence by using machine learning techniques and algorithms. It includes details related to programming languages such as python, R, JavaScript and Java. Introducing Programming Languages Used For Generating AI Art Using Chatgpt For Generating Chatgpt SS to increase your presentation threshold. Encompassed with four stages, this template is a great option to educate and entice your audience. Dispence information on Programming Languages, Python, Javascript using this template. Grab it now to reap its full benefits.
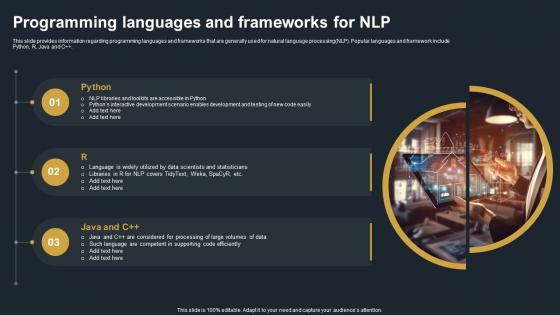
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and C plus. Increase audience engagement and knowledge by dispensing information using Programming Languages And Frameworks For NLP Decoding Natural Language AI SS V This template helps you present information on three stages. You can also present information on Python, Programming Languages, Frameworks using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
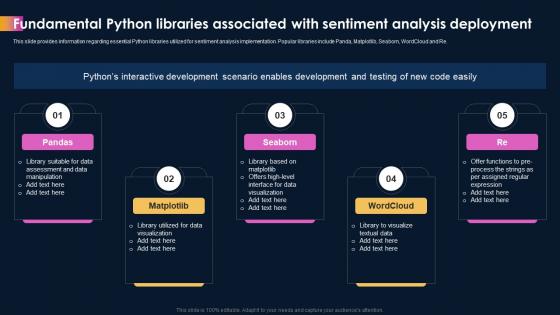
This slide provides information regarding essential Python libraries utilized for sentiment analysis implementation. Popular libraries include Panda, Matplotlib, Seaborn, WordCloud and Re. Increase audience engagement and knowledge by dispensing information using Ai Powered Sentiment Analysis Fundamental Python Libraries Associated With Sentiment Analysis AI SS. This template helps you present information on five stages. You can also present information on Analysis, Deployment, Fundamental using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
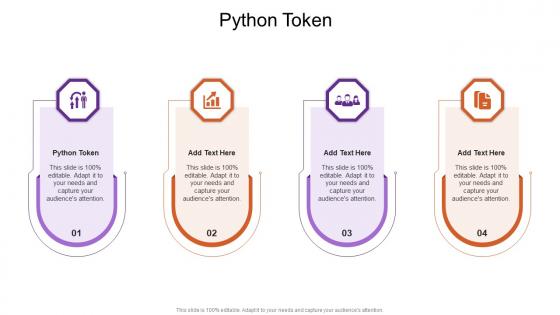
Presenting Python Token In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase Four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Python Token This well-structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
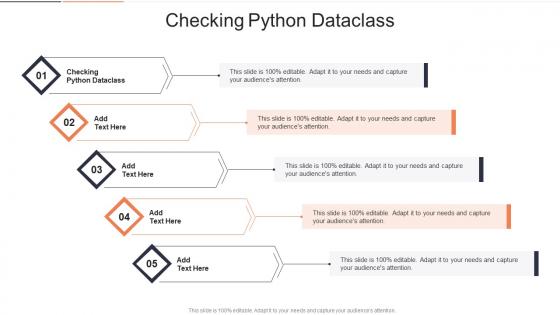
Presenting our Checking Python Dataclass In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases five stages. It is useful to share insightful information on Checking Python Dataclass. This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
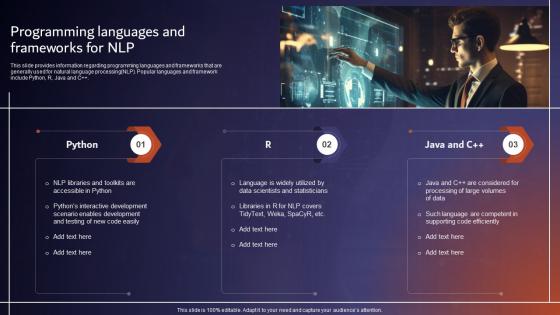
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and C plus plus.Introducing Programming Languages And Frameworks For NLP Comprehensive Tutorial About AI SS V to increase your presentation threshold. Encompassed with three stages, this template is a great option to educate and entice your audience. Dispence information on Interactive Development, Data Scientists, Supporting Code Efficiently, using this template. Grab it now to reap its full benefits.
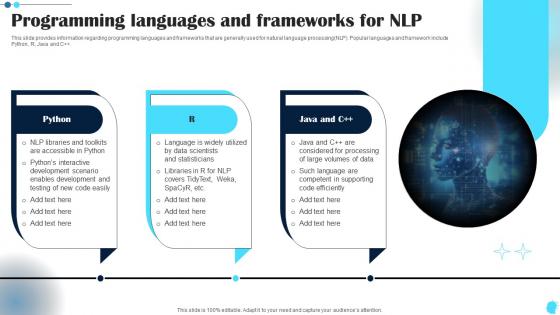
This slide provides information regarding programming languages frameworks that are generally used for natural language processingNLP. Popular languages framework include Python, R, Java C plus plus.Present the topic in a bit more detail with this Programming Languages Frameworks Power Of Natural Language Processing AI SS V. Use it as a tool for discussion navigation on Development Scenario, Enables Development, Data Scientists. This template is free to edit as deemed fit for your organization. Therefore download it now.
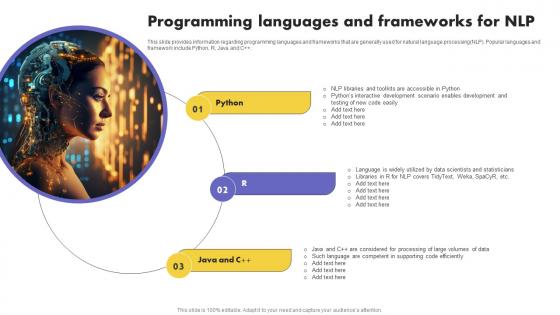
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and C Plus plus.Increase audience engagement and knowledge by dispensing information using Programming Languages And Frameworks For NLP What Is NLP And How It Works AI SS V This template helps you present information on three stages. You can also present information on Considered Processing, Competent Supporting, Scientists Statisticians using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
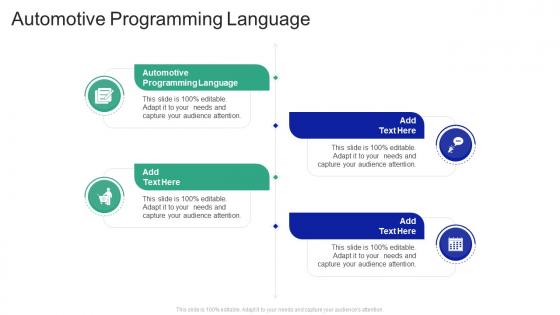
Presenting our Automotive Programming Language In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Automotive Programming Language This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
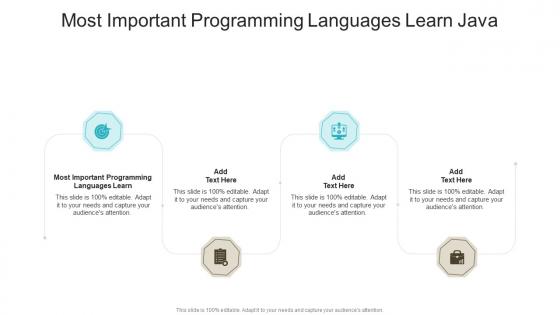
Presenting Most Important Programming Languages Learn Java In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Most Important Programming Languages Learn Java. This well structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.

Presenting our Programing Language Types In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Programing Language Types This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
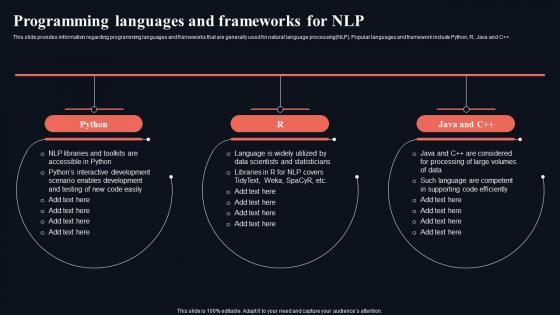
This slide provides information regarding programming languages and frameworks that are generally used for natural language processing NLP. Popular languages and framework include Python, R, Java and C plus plus. Increase audience engagement and knowledge by dispensing information using Programming Languages And Frameworks Gettings Started With Natural Language AI SS V. This template helps you present information on three stages. You can also present information on Languages, Frameworks, Programming using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
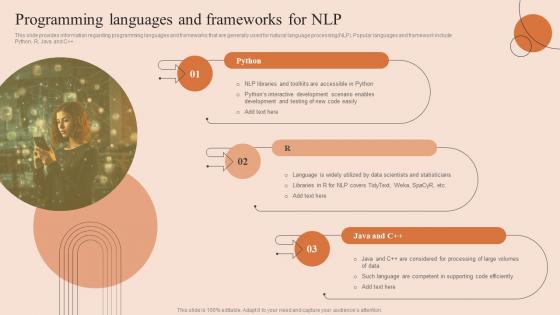
This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and Cplusplus. Increase audience engagement and knowledge by dispensing information using Natural Language Processing Programming Languages And Frameworks For NLP AI SS V. This template helps you present information on three stages. You can also present information on Python, Java using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
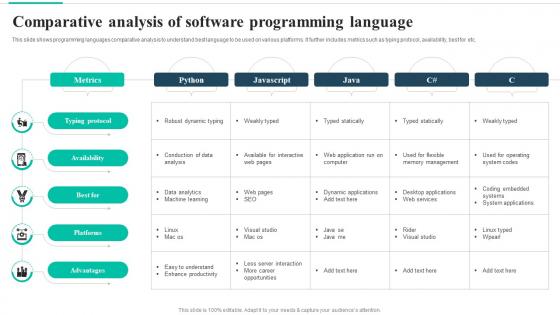
This slide shows programming languages comparative analysis to understand best language to be used on various platforms. It further includes metrics such as typing protocol, availability, best for etc. Introducing our Comparative Analysis Of Software Programming Language set of slides. The topics discussed in these slides are Typing Protocol, Availability, Platforms. This is an immediately available PowerPoint presentation that can be conveniently customized. Download it and convince your audience.
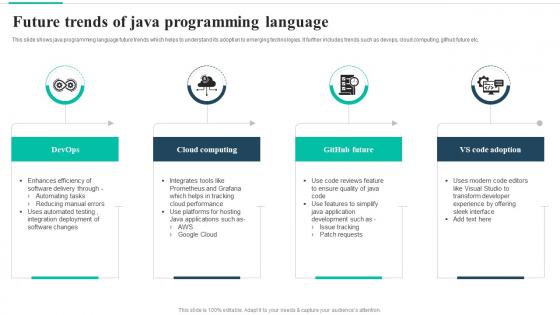
This slide shows java programming language future trends which helps to understand its adoption to emerging technologies. It further includes trends such as devops, cloud computing, github future etc. Presenting our set of slides with Future Trends Of Java Programming Language. This exhibits information on Four stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Cloud Computing, Github Future, Devops.
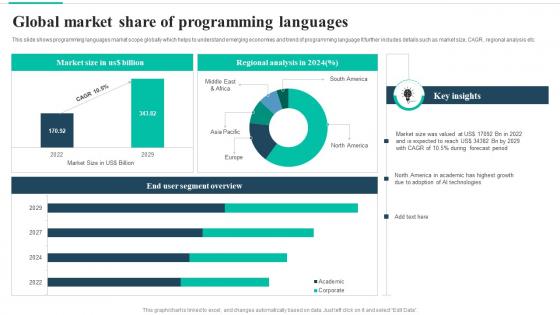
This slide shows programming languages market scope globally which helps to understand emerging economies and trend of programming language It further includes details such as market size, CAGR, regional analysis etc. Introducing our premium set of slides with Global Market Share Of Programming Languages. Ellicudate the Four stages and present information using this PPT slide. This is a completely adaptable PowerPoint template design that can be used to interpret topics like End User Segment Overview, Forecast Period, AI Technologies. So download instantly and tailor it with your information.
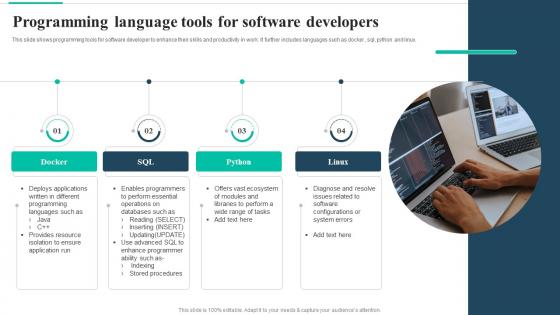
This slide shows server framework for sql programming language to understand queries and storage engine that manages database files. It further includes details such as share memory, external protocols, stored procedure etc. Introducing our Programming Language Tools For Software Developers set of slides. The topics discussed in these slides are Docker, SQL, Python. This is an immediately available PowerPoint presentation that can be conveniently customized. Download it and convince your audience.

This slide shows artificial intelligence programming languages with features and comparison of uses It further includes languages such as python, lisp, java, cPlus Plus and R. Presenting our well structured SQL Programming Language Server Architecture. The topics discussed in this slide are External Protocols, Database Engine, Query Executor. This is an instantly available PowerPoint presentation that can be edited conveniently. Download it right away and captivate your audience.
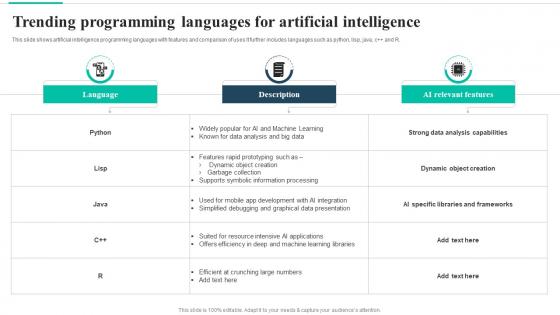
Introducing our Trending Programming Languages For Artificial Intelligence set of slides. The topics discussed in these slides are Low Graphics, Games Graphics. This is an immediately available PowerPoint presentation that can be conveniently customized. Download it and convince your audience.

This slide shows various types of programming languages uses for software development to know different function of all coding It further includes languages such as java, CPlusPlus, python and scala. Presenting our set of slides with Types Of Programming Language For Software Development. This exhibits information on Four stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Operating Systems, Games, GUI Based Apps.
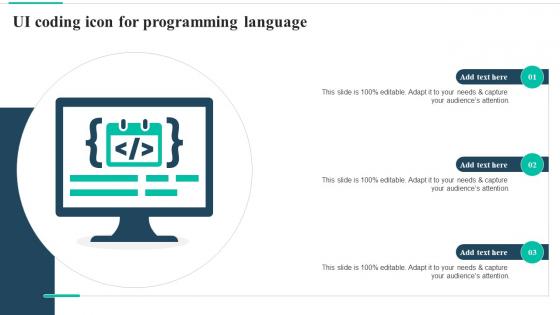
Introducing our premium set of slides with UI Coding Icon For Programming Language. Ellicudate the Three stages and present information using this PPT slide. This is a completely adaptable PowerPoint template design that can be used to interpret topics like UI Coding Icon, Programming Language. So download instantly and tailor it with your information.
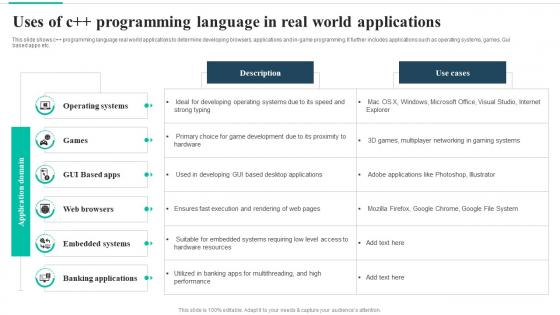
This slide shows cPlusPlus programming language real world applications to determine developing browsers, applications and in-game programming. It further includes applications such as operating systems, games, Gui based apps etc. Presenting our set of slides with Uses Of C Plus Plus Programming Language In Real World Applications. This exhibits information on Five stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Real World Applications, Uses Of C Plus Plus
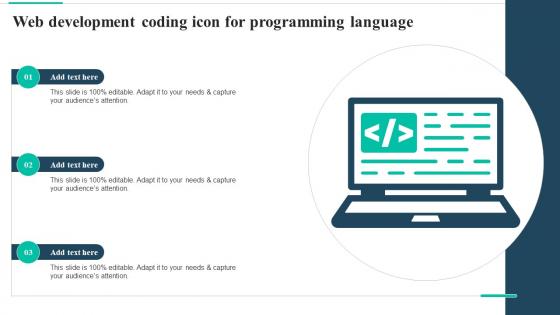
Introducing our premium set of slides with Web Development Coding Icon For Programming Language. Ellicudate the Three stages and present information using this PPT slide. This is a completely adaptable PowerPoint template design that can be used to interpret topics like Web Development Coding Icon, Programming Language. So download instantly and tailor it with your information.
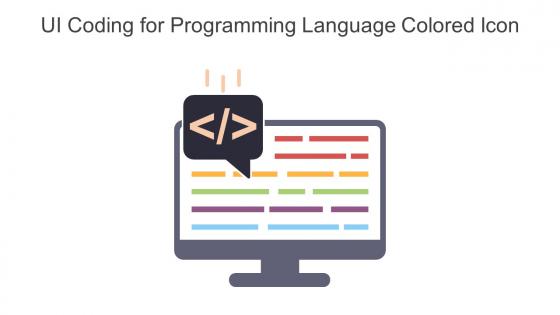
This vibrant PowerPoint icon features a coding language symbol, perfect for presentations on UI programming and development. The bold colors and clean design make it easy to incorporate into any slide, while the detailed image accurately represents the topic at hand. Add a touch of professionalism to your slides with this eye-catching icon.
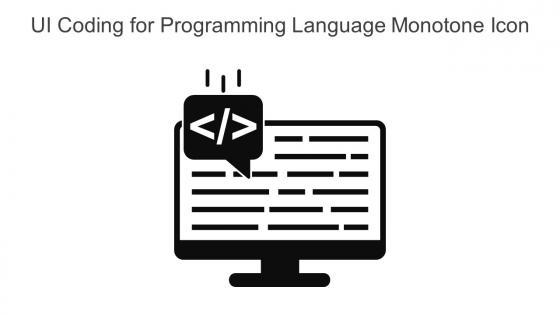
This Monotone powerpoint icon is perfect for representing UI coding in programming language presentations. With its sleek and minimalist design, it conveys the technical aspect of UI coding while maintaining a professional and modern look. Use it to enhance your slides and make a strong visual impact.
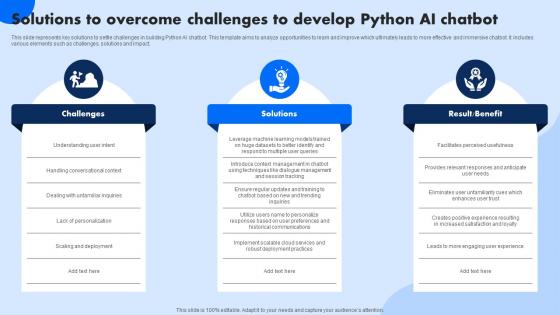
This slide represents key solutions to settle challenges in building Python AI chatbot. This template aims to analyze opportunities to learn and improve which ultimately leads to more effective and immersive chatbot. It includes various elements such as challenges, solutions and impact. Presenting our set of slides with Solutions To Overcome Challenges To Develop Python AI Chatbot This exhibits information on three stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Challenges, Solutions, Result Benefit
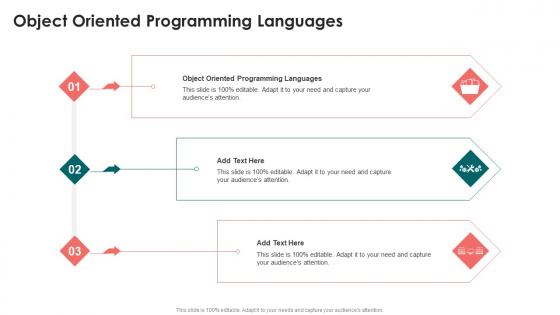
Presenting our Object Oriented Programming Languages In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Object Oriented Programming Languages. This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.

This slide provides information regarding programming languages and frameworks that are generally used for natural language processing nlp. Popular languages and framework include python, R, java and C. Increase audience engagement and knowledge by dispensing information using Programming Languages And Explore Natural Language Processing NLP AI SS V. This template helps you present information on three stages. You can also present information on Accessible, Scientists, Supporting using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
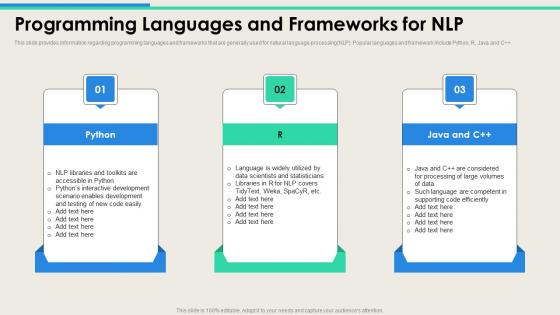
This slide provides information regarding programming languages and frameworks that are generally used for natural language processing NLP. Popular languages and framework include Python, R, Java and C Introducing Programming Languages And Frameworks Technologies And Associated With NLP AI SS to increase your presentation threshold. Encompassed with three stages, this template is a great option to educate and entice your audience. Dispence information on Accessible, Statisticians, Processing, using this template. Grab it now to reap its full benefits.
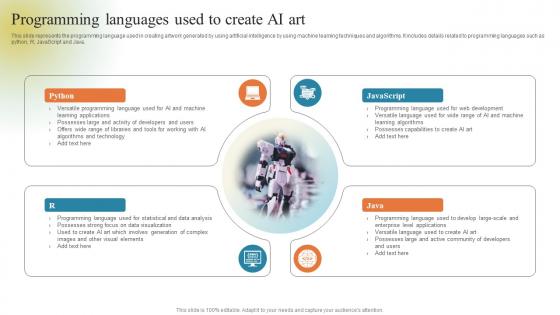
This slide represents the programming language used in creating artwork generated by using artificial intelligence by using machine learning techniques and algorithms. It includes details related to programming languages such as python, R, JavaScript and Java. Deliver an outstanding presentation on the topic using this GPT Chatbots For Generating Programming Languages Used To Create AI Art ChatGPT SS V. Dispense information and present a thorough explanation of Programming Language, Web Development, AI And Machine Learning Algorithms using the slides given. This template can be altered and personalized to fit your needs. It is also available for immediate download. So grab it now.
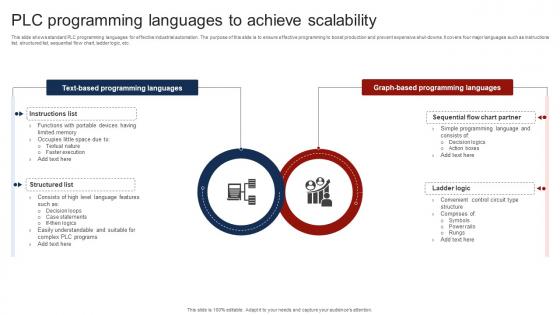
This slide shows standard PLC programming languages for effective industrial automation. The purpose of this slide is to ensure effective programming to boost production and prevent expensive shut downs. It covers four major languages such as instructions list, structured list, sequential flow chart, ladder logic, etc.Introducing our premium set of slides with PLC Programming Languages To Achieve Scalability. Ellicudate the two stages and present information using this PPT slide. This is a completely adaptable PowerPoint template design that can be used to interpret topics like Simple Programming, Easily Understandable, Limited Memory. So download instantly and tailor it with your information.

This slide provides information regarding programming languages and frameworks that are generally used for natural language processingNLP. Popular languages and framework include Python, R, Java and C Plus plus.Increase audience engagement and knowledge by dispensing information using Natural Language Programming Languages And Frameworks For NLP AI SS V. This template helps you present information on Three stages. You can also present information on Competent Supporting, Interactive Development, Enables Development using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
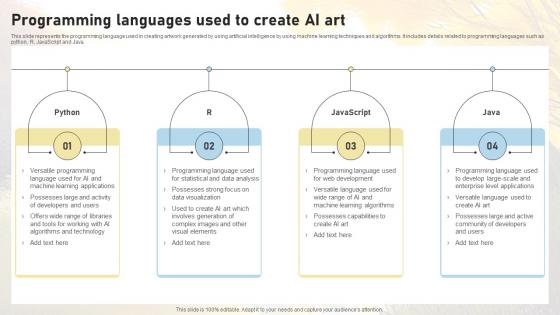
This slide represents the programming language used in creating artwork generated by using artificial intelligence by using machine learning techniques and algorithms. It includes details related to programming languages such as python, R, JavaScript and Java. Increase audience engagement and knowledge by dispensing information using Programming Languages Used To Create AI Art Comprehensive Guide On AI ChatGPT SS V This template helps you present information on four stages. You can also present information on Python, Javascript, Development, Technology using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
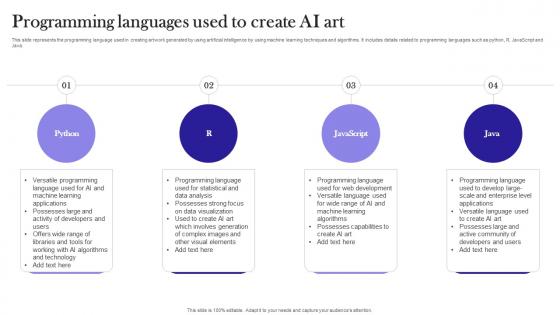
This slide represents the programming language used in creating artwork generated by using artificial intelligence by using machine learning techniques and algorithms. It includes details related to programming languages such as python, R, JavaScript and Java. Introducing Programming Languages Strategies For Using Chatgpt To Generate AI Art Prompts Chatgpt SS V to increase your presentation threshold. Encompassed with four stages, this template is a great option to educate and entice your audience. Dispence information on JavaScript, Java, Python, using this template. Grab it now to reap its full benefits.
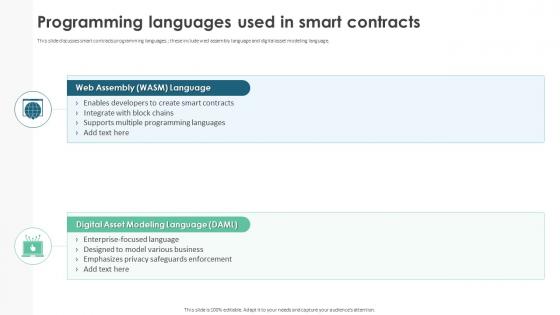
This slide discusses smart contracts programming languages, these include wed assembly language and digital asset modeling language. Increase audience engagement and knowledge by dispensing information using Programming Languages Used In Smart Contracts Ppt Show. This template helps you present information on two stages. You can also present information on Programming Languages, Smart Contracts, Digital Asset Modeling Language using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.

This slide describes the training program for Natural Language Generation systems. The purpose of this slide is to highlight the training schedule for NLG systems and the main components include agenda, name of trainer, system requirements, mode and cost of the training. Deliver an outstanding presentation on the topic using this Automated Narrative Generation Training Program To Build Natural Language. Dispense information and present a thorough explanation of Agenda, Trainer, System Requirements using the slides given. This template can be altered and personalized to fit your needs. It is also available for immediate download. So grab it now.

Introduction to �Object Oriented Programming�
Gayathri Namasivayam
Introduction to OOP
- Why use OOP?
- Building blocks of OOP
- What is OOP?
- OOP concepts
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
- Advantages vs Disadvantages
- Object Oriented Programming (OOP) is one of the most widely used programming paradigm
- Why is it extensively used?
- Well suited for building trivial and complex applications
- Allows re-use of code thereby increasing productivity
- New features can be easily built into the existing code
- Reduced production cost and maintenance cost
- Common programming languages used for OOP include C++, Java, and C#
Building Blocks of OOP: Objects & Classes
- Object: models a
- Real world object (ex. computer, book, box)
- Concept (ex. meeting, interview)
- Process (ex. sorting a stack of papers or comparing two computers to measure their performance)
- Class: prototype or blueprint from which objects are created
- Set of attributes or properties that describes every object
- Set of behavior or actions that every object can perform
- Set of data (value for each of its attribute)
- Set of actions that it can perform
- An identity
- Every object belongs to a class
Real World Example of Objects & Classes
Object: FordCar1
Start, Accelerate, Reverse, Stop
Color: Yellow
Type: Coupe
Model: Mustang
Cylinder: 6
Color, Type, Model, Cylinder
Class: FordCar
Color: Orange
Model: Focus
Cylinder: 4
Object: FordCar2
Another Real World Example..
Name, Height, Age
Speak, Listen, Eat, Run, Walk
Class: Person
Object: Person1
Height: 5’ 4”
Height: 5’ 9”
Object: Person2
- A class is a set of variables (to represent its attributes) and functions (to describe its behavior) that act on its variables
Class ShippingBox
int shipping_cost() {
return cost_per_pound*weight;
sender_name : string
receiver_name : string
cost_per_pound : int
weight : int
shipping_cost() : int
- Object is an instance of a class that holds data (values) in its variables. Data can be accessed by its functions
Objects of ShippingBox class
sender_name = Jim
receiver_name = John
cost_per_pound = 5
weight = 10
shipping_cost()
Object BoxB
Object BoxA
sender_name = Julie
receiver_name = Jill
cost_per_pound = 2
- Paradigm for problem solving by interaction among objects
- It follows a natural way of solving problems
- Ex. Ann wants to start her car
(1) Ann walks to her car
(2) Ann sends a message to the car to start by turning on the ignition
(3)The car starts
Problem Solving in OOP
Problem: Ann wants to start her car
Color = Yellow
Type = Coupe
Model = Mustang
Cylinder = 6
Accelerate()
Object Ann’s car
- Extracting essential properties and behavior of an entity
- Class represents such an abstraction and is commonly referred to as an abstract data type
Ex. In an application that computes the shipping cost of a box, we extract its properties: cost_per_pound, weight and its behavior: shipping_cost()
Shipping Box
Sender’s name,
Receiver’s name,
Cost of shipping per pound,
Calculate shipping cost
sender_name
receiver_name
cost_per_pound
shipping_cost ()
- Mechanism by which we combine data and the functions that manipulate the data into one unit
- Objects & Classes enforce encapsulation
- Create new classes (derived classes) from existing classes (base classes)
- The derived class inherits the variables and functions of the base class and adds additional ones!
- Provides the ability to re-use existing code
Inheritance Example
BankAccount CheckingAccount SavingsAccount
customer_name : string
account_type : string
balance : int
insufficient_funds_fee : int
deposit() : int
withdrawal() : int
process_deposit() : int
interest_rate : int
calculate_interest() : int
CheckingAccount
SavingsAccount
BankAccount
- Polymorphism*
(* To be covered in the next class)
Disadvantages of OOP
- Initial extra effort needed in accurately modeling the classes and sub-classes for a problem
- Suited for modeling certain real world problems as opposed to some others
- Classes & Objects
- Concepts in OOP
- Advantages & Disadvantages
- Be prepared for an in-class activity (based on topics covered today)!
- Polymorphism in OOP!
- Top Courses
- Online Degrees
- Find your New Career
- Join for Free
5 Types of Programming Languages
Learn more about some common categories of programming languages to get inspired to start coding.
![programing language presentation [Featured image] A woman sits in front of two computer monitors writing code in a programming language.](https://d3njjcbhbojbot.cloudfront.net/api/utilities/v1/imageproxy/https://images.ctfassets.net/wp1lcwdav1p1/69aHNX9jkz3vnIvD5LDOhS/12a92e14a1f9dd8b5175ffb9447f549f/GettyImages-1322050853__1_.jpg?w=1500&h=680&q=60&fit=fill&f=faces&fm=jpg&fl=progressive&auto=format%2Ccompress&dpr=1&w=1000)
Programming is a skill that is becoming increasingly sought after in the job market. Having at least a basic understanding of how software functions is helpful for anyone who interacts with technology. With a background in programming, you can get a job coding, designing software, data architecture, or creating intuitive user interfaces.
But what programming language should you learn? You'll find a seemingly infinite number of programming languages that are free to learn and develop projects with online. With the field of technology growing exponentially each year, the internet is a great place to start when trying to explore the latest developments or discover a new skill.
In this article, we'll explore some of the most common types of programming languages and give you some resources you can use to start learning.
Whichever language you learn, you may find it helpful to take notes or draw diagrams detailing the steps you're taking and why. It's important for programmers to be able to communicate their process to non-technical stakeholders, but taking notes can also be a great learning tool. "Turns out this often comes in handy not only for the sake of creating documentation, but often helps in solving a single task or issue where the underlying tech is challenging to understand," offers Eric Hartzog, a software engineer at Meta . You can learn software engineering from Meta staff by enrolling in one of their online courses or certificate programs, for example, the Meta Front-End Developer Professional Certificate , which focuses on JavaScript, HTML, and CSS.
5 major types of programming languages
While you'll find dozens of ways to classify various programming languages, they generally fall into five major categories. Keep in mind that some languages may fall under more than one type:
1. Procedural programming languages
A procedural language follows a sequence of statements or commands in order to achieve a desired output. Each series of steps is called a procedure, and a program written in one of these languages will have one or more procedures within it. Common examples of procedural languages include:
C++ is a great programming language to learn if you're also interested in learning more about how computers function. While it may not be as readable as other high-level programming languages like Python, it can still be beginner-friendly. You can give it a try for free by enrolling in Codio's introductory online program, Programming in C++: A Hands-on Introduction Specialization . After a month, you'll have created and ran your first program in C++.
2. Functional programming languages
Rather than focusing on the execution of statements, functional languages focus on the output of mathematical functions and evaluations. Each function–a reusable module of code–performs a specific task and returns a result. The result will vary depending on what data you input into the function. Some popular functional programming languages include:
3. Object-oriented programming languages (OOP)
This type of language treats a program as a group of objects composed of data and program elements, known as attributes and methods. Objects can be reused within a program or in other programs. This makes it a popular language type for complex programs, as code is easier to reuse and scale. Some common object-oriented languages include:
If you're teaching yourself Python but you're not yet ready to commit to an online course or program, you might consider bookmarking some of the following tutorials:
How to Use For Loops in Python: Step-By-Step
How to Use Python Break
How to Write and Use Python While Loops
Python Loops Cheat Sheet
4. Scripting languages
Programmers use scripting languages to automate repetitive tasks, manage dynamic web content, or support processes in larger applications. Some common scripting languages include:
Linux operating system is the primary choice for those who prefer open-source software [ 1 ]. Familiarizing yourself with Linux can be useful for someone who is interested in learning scripting languages.
5. Logic programming languages
Instead of telling a computer what to do, a logic programming language expresses a series of facts and rules to instruct the computer on how to make decisions. Some examples of logic languages include:
Other ways to classify programming languages
You'll find many more ways to categorize languages beyond the five listed above. Let's take a closer look at there other ways you can think about programming languages:
Front-end vs. back-end languages
Front-end languages are primarily concerned with the ‘user’ aspect of the software. The front end deals with all of the text, colors, buttons, images, and navigation that the user will face when navigating your website or application. Anyone with a background in graphic design or art may be more inspired to begin learning one of the front-end languages.
Some examples of front-end programming languages include:
JavaScript
Back-end languages deal with storage and manipulation of the server side of software. This is the part of the software that the user does not directly come into contact with but supports their experience behind the scenes. This includes data architecture, scripting, and communication between applications and underlying databases.
Anyone with experience in mathematics or engineering may find more interest in back-end development.
Some examples of back-end programming languages include:
A full-stack developer combines their knowledge of both front- and back-end languages, along with other technical skills and expertise, to work on any part of the development process.
High-level vs. low-level languages
The biggest factor that differentiates high- and low-level programming languages is whether the language is meant to be easily understood by a human programmer or a computer. Low-level languages are machine-friendly, which makes them highly efficient in terms of memory usage but difficult to understand without the help of an assembler. Since they're not very people-friendly because they don't use human language, they're also not widely used to code. Examples of these machine languages include machine code, binary code, and assembly languages.
High-level languages , on the other hand, are less memory efficient but much more human-friendly. This programming style makes it easier to write, understand, maintain, and debug. Most popular programming languages in use today are considered high-level languages.
Interpreted vs. compiled languages
The distinction between interpreted and compiled languages has to do with how they convert high-level code and make it readable by a computer. With interpreted languages , code goes through a program called an interpreter, which reads and executes the code line by line. This tends to make these languages more flexible and platform independent.
Examples of interpreted languages include:
Compiled languages go through a build step where the entire program is converted into machine code. This makes it faster to execute, but it also means that you have to compile or "build" the program again anytime you need to make a change.
Examples of compiled languages include:
C, C++, and C#
Markup language
Often, markup languages such as Hypertext Markup Language (HTML) are classified as programming languages. Technically, markup languages are not considered to be the same as programming languages. Instead, they are text-encoding systems made up of symbols that control the formatting and structure of content on a page or document.
Start programming today on Coursera
Learning to program is easier now than ever. Start writing programs in Python with Python for Everybody from the University of Michigan, learn the basics of web development with HTML, CSS, and JavaScript for Web Developers from Johns Hopkins University, or prepare for a career in IT with Google IT Automation with Python .
Article sources
Statista. " Market share held by the leading computer (desktop/tablet/console) operating systems worldwide from January 2012 to February 2024 , https://www.statista.com/statistics/268237/global-market-share-held-by-operating-systems-since-2009/." Accessed March 19, 2024.
Keep reading
Coursera staff.
Editorial Team
Coursera’s editorial team is comprised of highly experienced professional editors, writers, and fact...
This content has been made available for informational purposes only. Learners are advised to conduct additional research to ensure that courses and other credentials pursued meet their personal, professional, and financial goals.
The Canva Windows app lets you enjoy all the features you love in a dedicated program. Launch Canva instantly from your desktop. Dive into deep work without the tab overload. WORK SMARTER WITH THE VISUAL SUITE A complete suite of tools for our visual world - Craft professional content with 250,000+ free templates. - Design visual Docs with videos, charts, or linked Canva designs. - Capture your team’s best ideas with Whiteboards. - Present with confidence. Wow your audience with visual slides. - Design, schedule, and track your social posts in one place. - Print anything from t-shirts to mugs, posters, and packaging. - Turn your designs into a website. Save on domain costs. - Collaborate in real time with your team, from anywhere. - Connect your favorite work apps for a seamless workflow. PHOTO & VIDEO EDITING MADE SIMPLE Fresh content at your fingertips - Edit photos instantly. Auto enhance, focus, or blur to add depth. - Personalize with ease. Filter photos, add text, and adjust image lighting. - Need to remove photo clutter? Add, replace, or modify details with AI tools. - Restore photos or customize emojis. Discover new possibilities with Canva apps. - Play with video editing. Crop, split, or speed up videos. - Finish with the perfect audio track. Sync to the beat in a snap. MEET MAGIC STUDIO All the power of AI. All in one place. - Find the right words, fast, with Magic Write. - Create custom presentations and posts in seconds with Magic Design. - Turn ideas into images and videos with Magic Media. - Swap design formats, languages, or dimensions with Magic Switch. - Extend an image in any direction with Magic Expand. - Instantly add transitions to your design with Magic Animate. CANVA PRO GIVES YOU MORE MAGIC Unlock premium templates, powerful tools, and AI-powered magic. - Unlimited access to 100+ million premium templates and content. - Full access to 20+ AI-powered tools with Magic Studio. - Set up, manage, and grow your brand with Brand Kit. - Resize designs without limits with Magic Switch. - Remove image and video backgrounds in a click. - Turn slides and brainstorms into a doc with Magic Switch. - Schedule social media posts to 8 platforms with Content Planner. - Working with a team? Collaborate faster with Canva for Teams. Canva Pro - $14.99/month or $119.99/year Canva for Teams - $29.99/month or $300/year for the first 5 team members Prices in USD. Localized pricing applies. Subscription auto-renews unless turned off at least 24 hours before the renewal date. Any unused portion of a trial period, if offered, will be forfeited when you purchase a paid subscription. https://about.canva.com/terms-of-use https://about.canva.com/privacy-policy
Https://about.canva.com/terms-of-use https://about.canva.com/privacy-policy https://www.canva.com/policies/license-agreements.
Got any suggestions?
We want to hear from you! Send us a message and help improve Slidesgo
Top searches
Trending searches

memorial day
12 templates
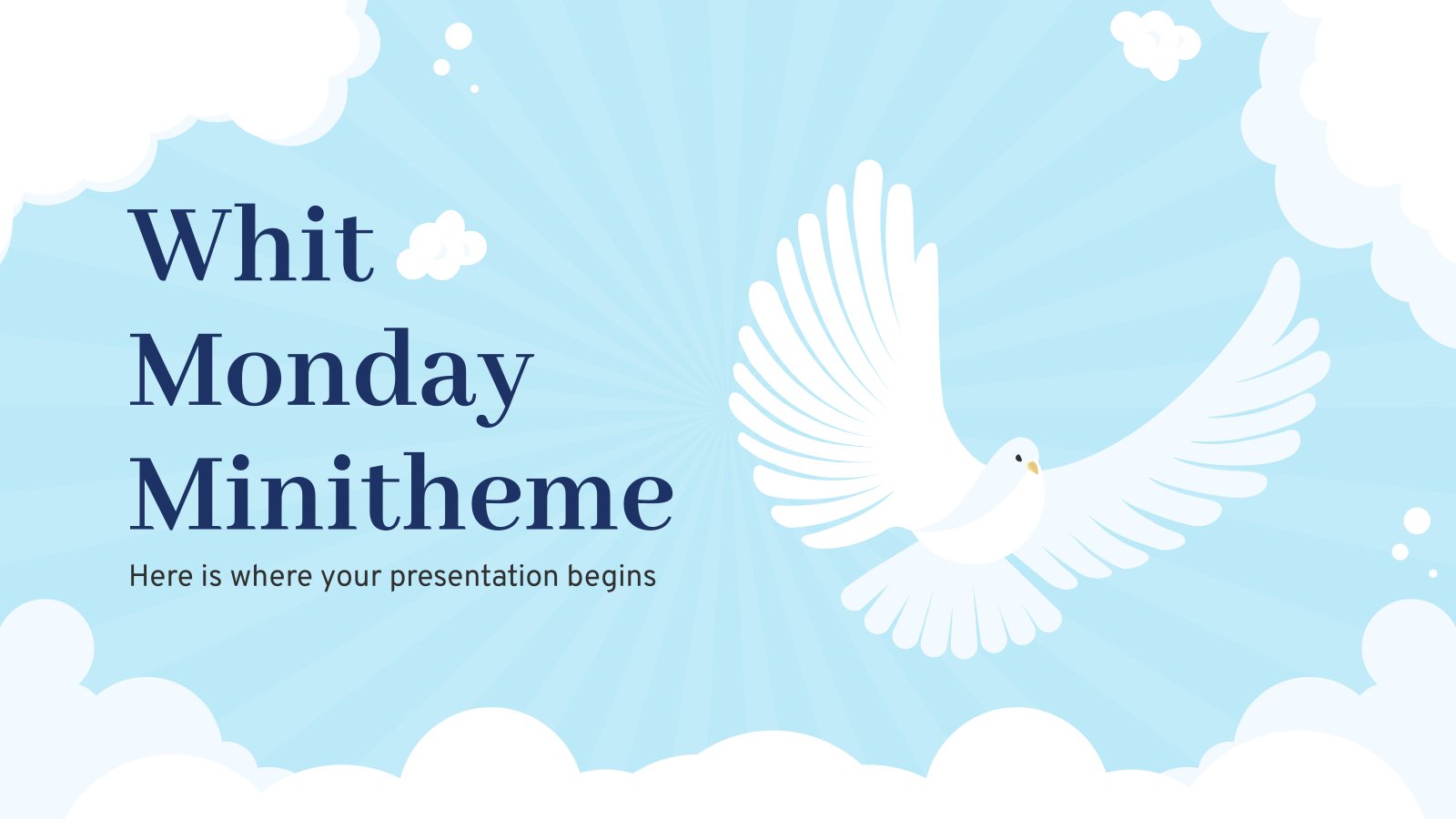
holy spirit
36 templates
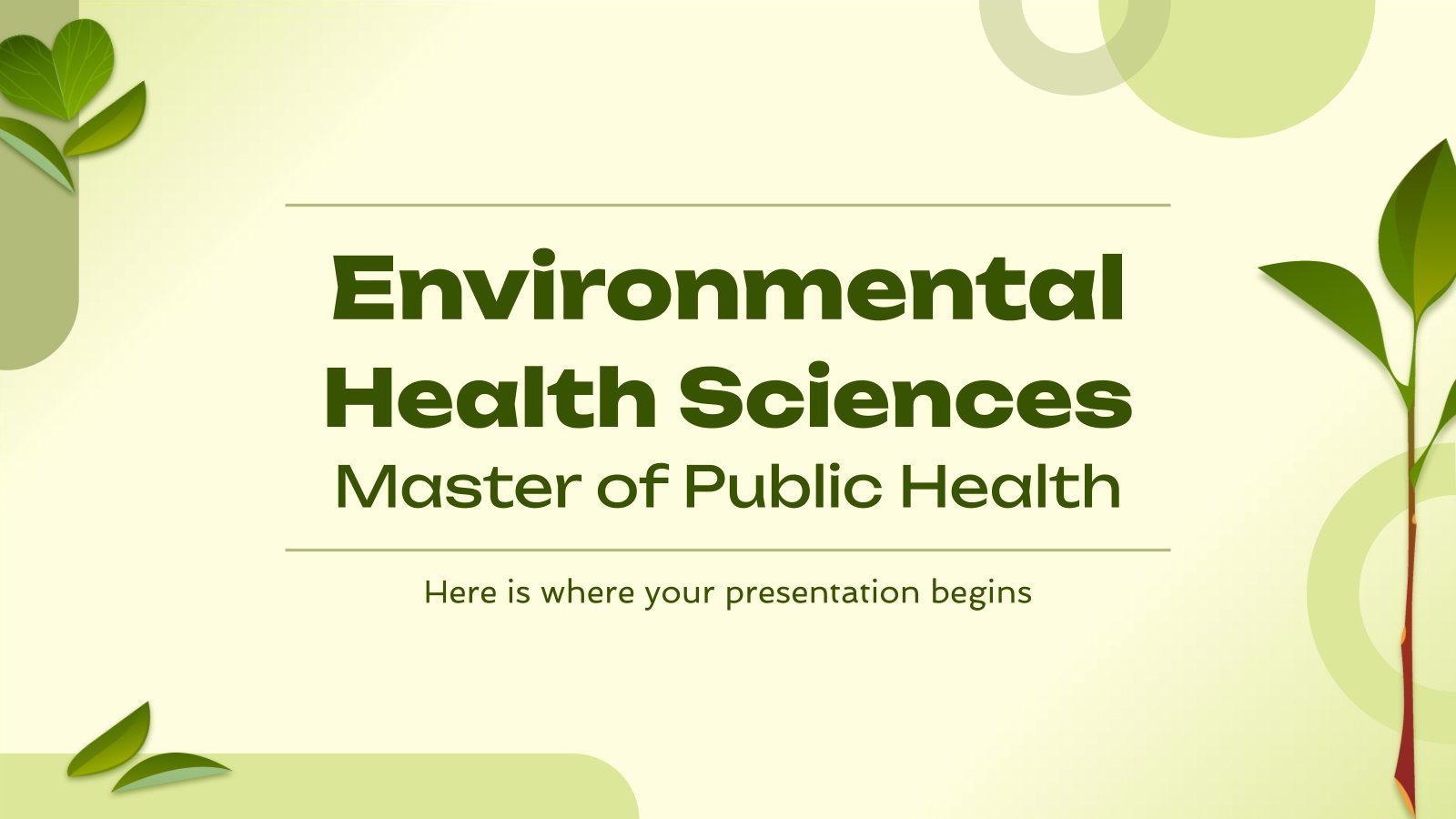
environmental science
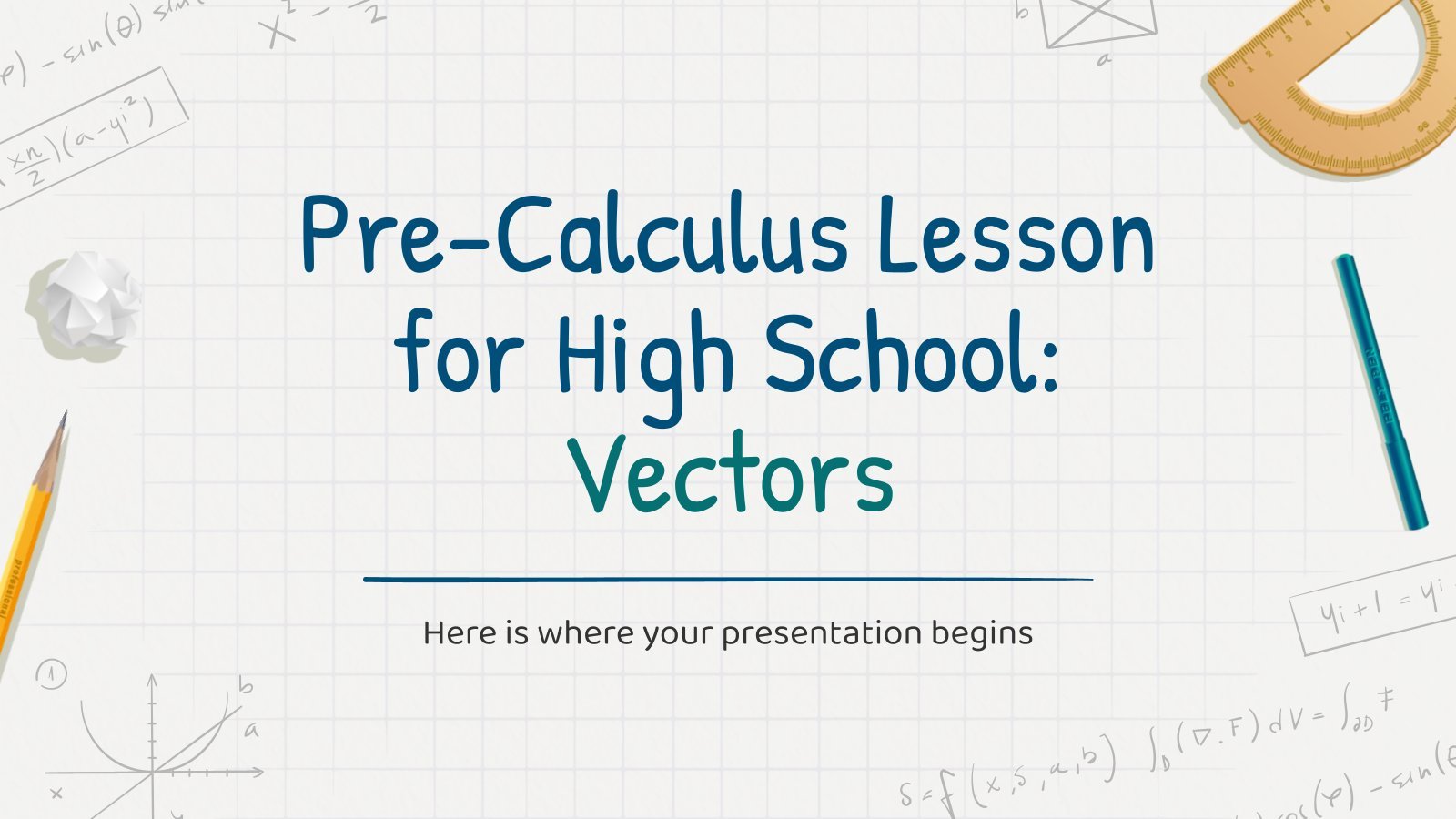
21 templates
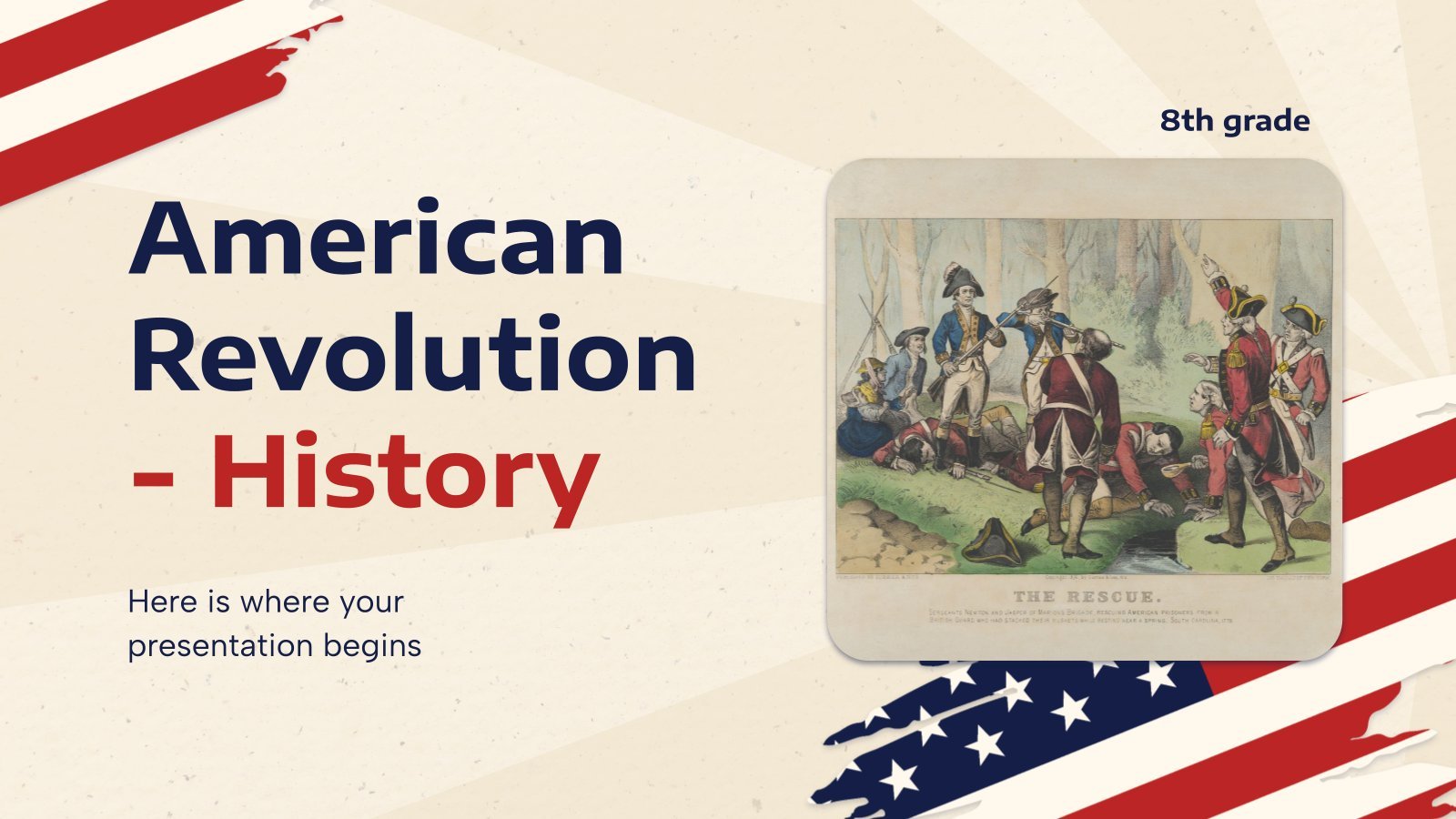
american history
74 templates
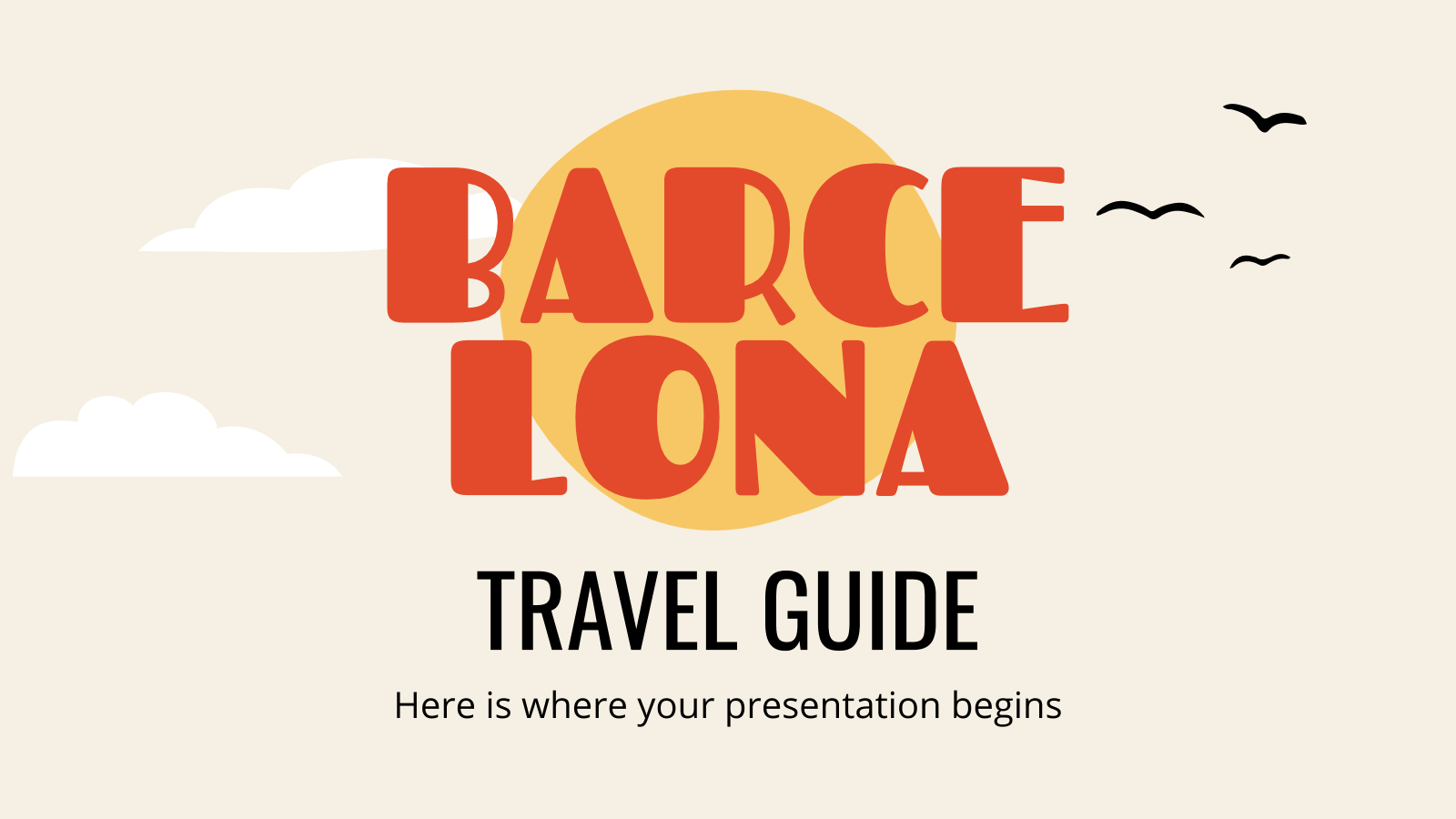
13 templates
Programming Language Workshop for Beginners Infographics
It seems that you like this template, free google slides theme, powerpoint template, and canva presentation template.
We propose you a challenge: to get the code out of our website. Well, better not, you're a real hacker! Joking aside, we have something for you. If you teach programming workshops and use our designs, this set of infographics can help you organize your data. You'll find graphs, tables or diagrams, all in a very "computer" style. It looks like the slides were written in code!
Features of these infographics
- 100% editable and easy to modify
- 32 different infographics to boost your presentations
- Include icons and Flaticon’s extension for further customization
- Designed to be used in Google Slides, Canva, and Microsoft PowerPoint
- 16:9 widescreen format suitable for all types of screens
- Include information about how to edit and customize your infographics
- Supplemental infographics for the template Programming Language Workshop for Beginners
How can I use the infographics?
Am I free to use the templates?
How to attribute the infographics?
Combines with:
This template can be combined with this other one to create the perfect presentation:
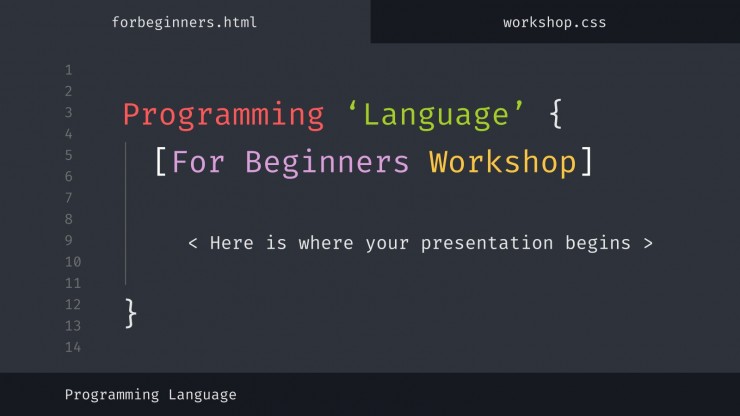
Attribution required If you are a free user, you must attribute Slidesgo by keeping the slide where the credits appear. How to attribute?
Related posts on our blog.
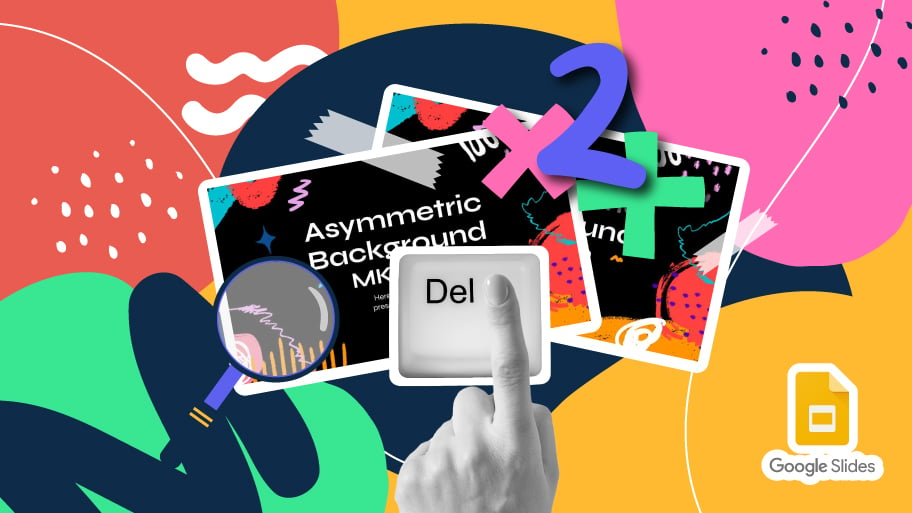
How to Add, Duplicate, Move, Delete or Hide Slides in Google Slides

How to Change Layouts in PowerPoint
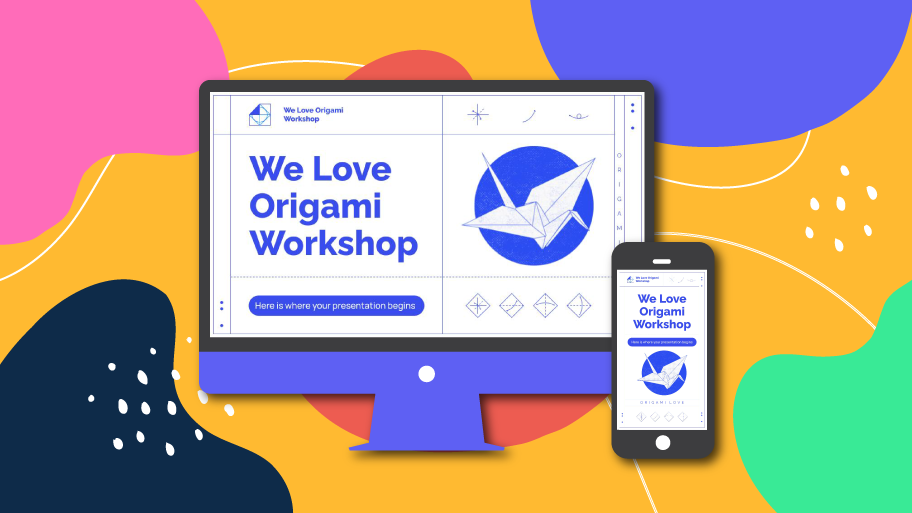
How to Change the Slide Size in Google Slides
Related presentations.
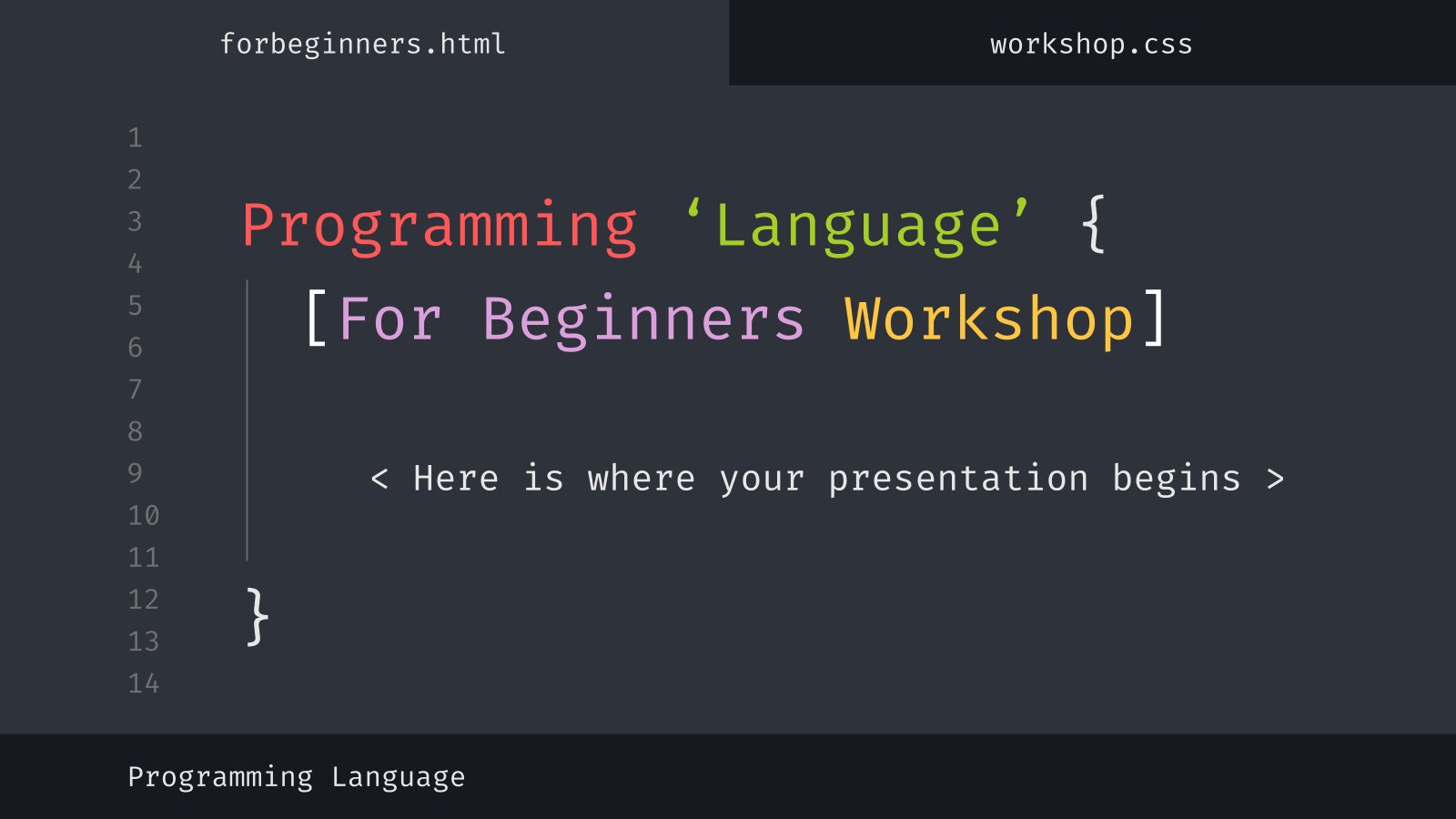
Premium template
Unlock this template and gain unlimited access
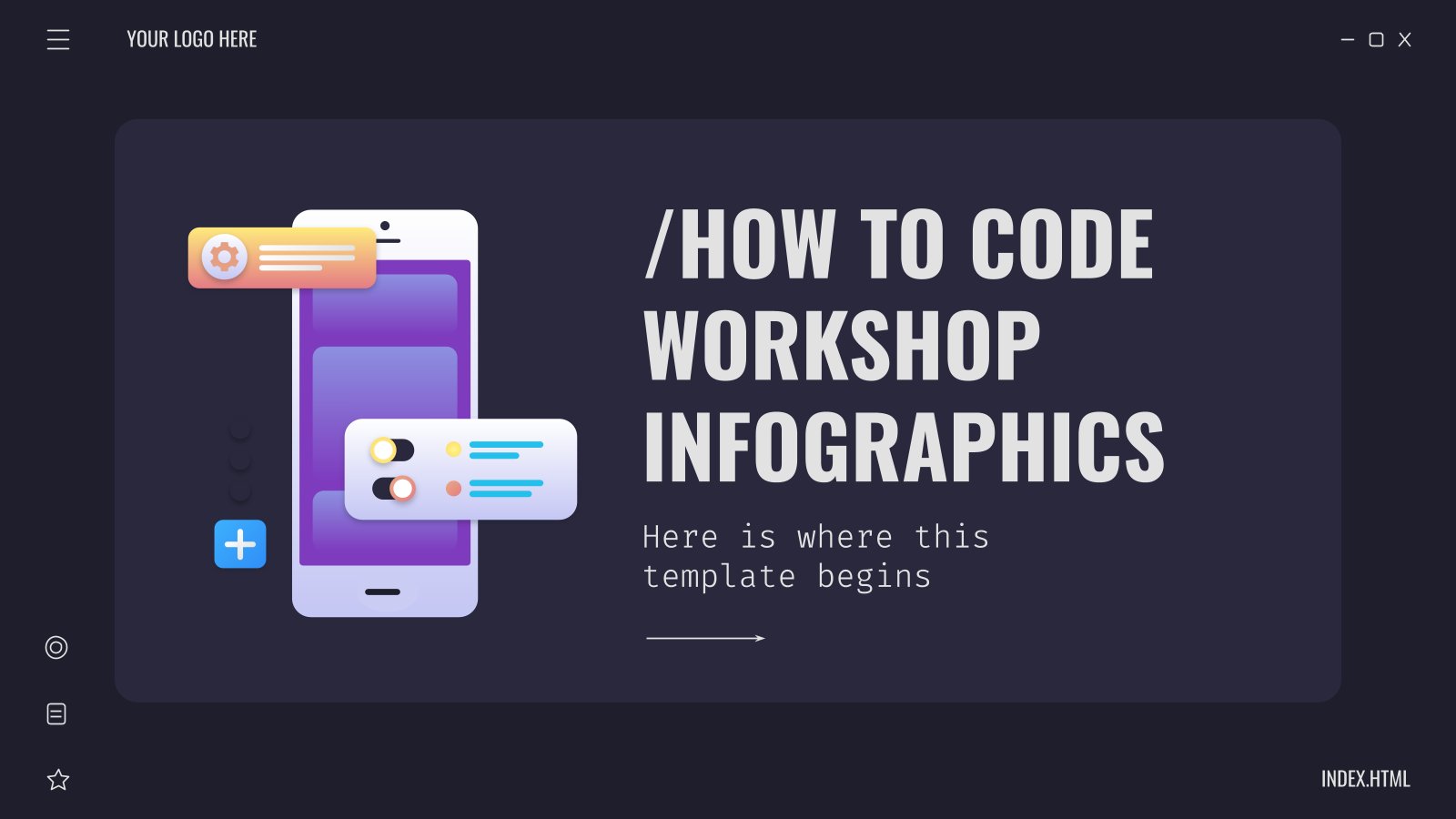
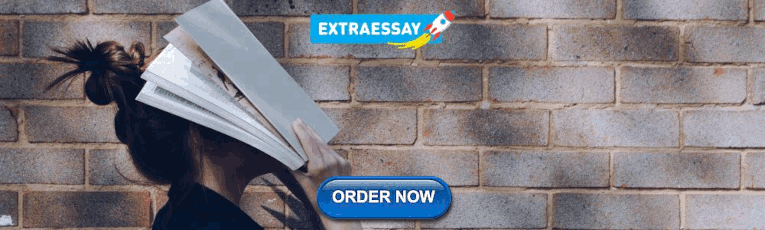
IMAGES
VIDEO
COMMENTS
The language is the set of sentences containing only terminal symbols that can be generated by applying the rewriting rules starting from the root symbol (let's call such sentences strings) Consider the following grammar G: N = {S;X; Y} S = S. Σ = {a; b; c} δ consists of the following rules: S -> b.
All About Programming in Java. Download the All About Programming in Java presentation for PowerPoint or Google Slides. High school students are approaching adulthood, and therefore, this template's design reflects the mature nature of their education. Customize the well-defined sections, integrate multimedia and interactive elements and ...
Object Oriented Programming Slides for Lecture 8 (PDF) Code for Lecture 8 (PY) 9 Python Classes and Inheritance Slides for Lecture 9 (PDF - 1.6MB) Code for Lecture 9 (PY) 10 Understanding Program Efficiency, Part 1 Slides for Lecture 10 (PDF) Code for Lecture 10 (PY) 11 Understanding Program Efficiency, Part 2 Slides for Lecture 11 (PDF)
4. PS — Introduction A programming language is a notational system for describing computation in a machine-readable and human-readable form. A programming language is a tool for developing executable models for a class of problem domains. 5. English is a natural language.
A programming language is a formal language that specifies a set of instructions for a computer to perform specific tasks. It's used to write software programs and applications, and to control and manipulate computer systems. There are many different programming languages, each with its own syntax, structure, and set of commands.
UNDERSTAND TRADE-OFFS/IMPLEMENTATION COSTS BASED ON UNDERSTANDING OF LANGUAGE INTERNALS. EXAMPLES: USE X*X RATHER THAN X**2. USE C POINTERS OR PASCAL 'WITH' STATEMENT TO FACTOR ADDRESS CALCULATIONS. AVOID CALL-BY-VALUE WITH LARGE ARGUMENTS IN PASCAL. AVOID THE USE OF CALL-BY-NAME IN ALGOL-60.
The templates provide a visually appealing and professional design to engage and inform the audience about programming topics. Download your presentation as a PowerPoint template or use it online as a Google Slides theme. 100% free, no registration or download limits. Get these programming templates to create dynamic and engaging presentations ...
Object-oriented (e.g., Smalltalk, Java) Scripting languages (e.g., Ruby, JavaScript) Markup (e.g., HTML, CSS) Note that these categories are loose and many languages bleed, or do not really fit, in various categories. Some authors claim various of these categories are not really categories, while others disagree.
The amazing design works perfect for a programming workshop because it includes editable resources and a layout that makes understanding code lines a very easy and visual experience. In addition, the color combination of the fonts will help you guide your students though the different functions and types of your favourite programming language ...
These PPT Slides are simple to use in terms of download and editing. Using a UML Diagram can help you make your message more understandable. On the other hand, the inclusion of codes and other programming languages has made creating a Programming PowerPoint presentation somewhat tricky.
Why study programming languages? ITo improve the ability to develop effective algorithms. ITo improve the use of familiar languages. ITo increase the vocabulary of useful programming constructs. ITo allow a better choice of programming language. ITo make it easier to learn a new language. ITo make it easier to design a new language. ITo simulate useful features in languages that lack them.
Free Google Slides theme, PowerPoint template, and Canva presentation template. If you teach programming languages and related issues, download and personalize this template to prepare your lesson. The fonts look computer-like, and it's very creative. Insert some info about the features of the topic, assignments and support them with visual ...
This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like High Level Programming Languages List. This well-structured design can be downloaded in different formats like PDF, JPG, and PNG.
The following PPT is an Introduction to Python as a Programming Language and its Applications. It covers all the basic info about python and its applications. This is an interactive presentation created using PowerPoint Online.
Introduction to OOP. Building Blocks of OOP: Objects & Classes. Object: models a. Real world object (ex. computer, book, box) Concept (ex. meeting, interview) Process (ex. sorting a stack of papers or comparing two computers to measure their performance) . Class: prototype or blueprint from which objects are created. Introduction to OOP.
Introduction To Programming Languages. A programming language is a formal constructed language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs to control the behavior of a machine or to express algorithms.
Simple C Program Line 1: #include o As part of compilation, the C compiler runs a program called the C preprocessor. The preprocessor is able to add and remove code from your source file. In this case, the directive #include tells the preprocessor to include code from the file stdio.h. This file contains declarations for functions that the ...
Cp Sc 1110 - Programming in C 4th Edition. Slides and Handouts. Chapter. Slides. Handouts. 01 Introduction to C. Slides. Handouts. 02 Your First Program.
Programming Languages Presentation By Nick Colvin Computer Languages There are many different computer languages, and all of them have different uses. It takes a lot of time to master one language, let alone all of them. In this presentation we will explore five different
Some popular functional programming languages include: Scala. Erlang. Haskell. Elixir. F#. 3. Object-oriented programming languages (OOP) This type of language treats a program as a group of objects composed of data and program elements, known as attributes and methods.
Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely. A programming language is a set of words, symbols and codes that enables humans to communicate with computers. 4. 5. Computer programming language is an language used to write computer programs, which involve a ...
You need high-quality business presentation software to take your slides to the next level. Some of the best presentation software include Visme, Haiku Deck, Prezi, Microsoft Powerpoint, Canva and Google Slides. In this comparison guide, we'll analyze each of these tools and many more to understand what the difference is between them so you ...
The Canva Windows app lets you enjoy all the features you love in a dedicated program. Launch Canva instantly from your desktop. Dive into deep work without the tab overload. WORK SMARTER WITH THE VISUAL SUITE A complete suite of tools for our visual world - Craft professional content with 250,000+ free templates. - Design visual Docs with videos, charts, or linked Canva designs. - Capture ...
If we tell you Java or Python you might be closer to the answer. All right, programming languages! Thanks to this template you will be able to present the content of a master's degree in programming languages. Show the world your information in an original way, through slides with illustrations related to the subject.
Prior to GPT-4o, you could use Voice Mode to talk to ChatGPT with latencies of 2.8 seconds (GPT-3.5) and 5.4 seconds (GPT-4) on average. To achieve this, Voice Mode is a pipeline of three separate models: one simple model transcribes audio to text, GPT-3.5 or GPT-4 takes in text and outputs text, and a third simple model converts that text back to audio.
Joking aside, we have something for you. If you teach programming workshops and use our designs, this set of infographics can help you organize your data. You'll find graphs, tables or diagrams, all in a very "computer" style. It looks like the slides were written in code!