Why solve a problem twice? Design patterns let you apply existing solutions to your code
Software design patterns are like best practices employed by many experienced software developers. You can use design patterns to make your application scalable and flexible.
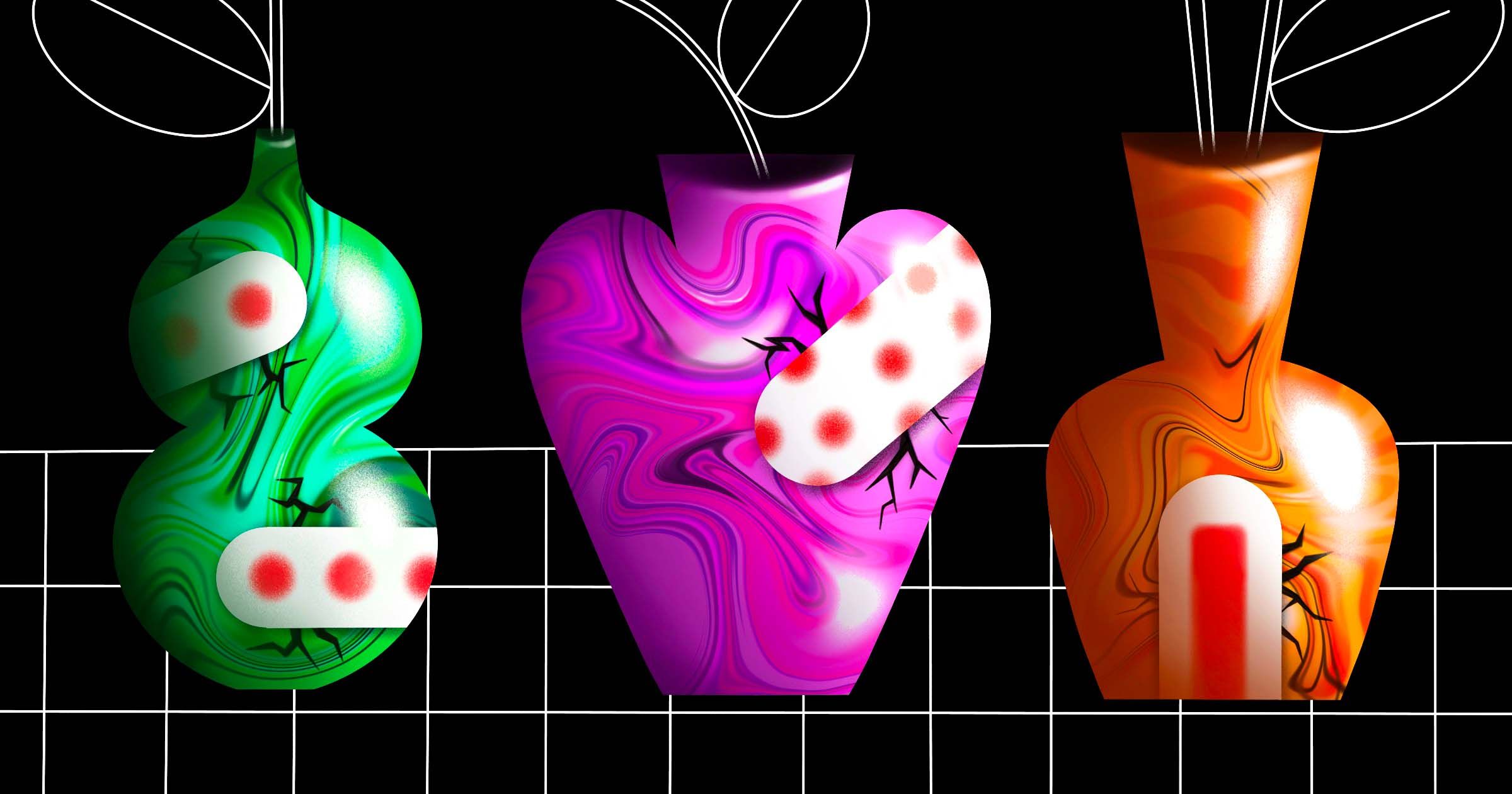
The most satisfying problems in software engineering are those that no one has solved before. Cracking a unique problem is something that you can use in job interviews and talk about in conferences. But the reality is that the majority of challenges you face will have already been solved. You can use those solutions to better your own software.
Software design patterns are typical solutions for the reoccurring design problems in software engineering. They're like the best practices employed by many experienced software developers. You can use design patterns to make your application scalable and flexible .
In this article, you'll discover what design patterns are and how you can apply them to develop better software applications, either from the start or through refactoring your existing code.
Note: Before learning design patterns, you should have a basic understanding of object-oriented programming.
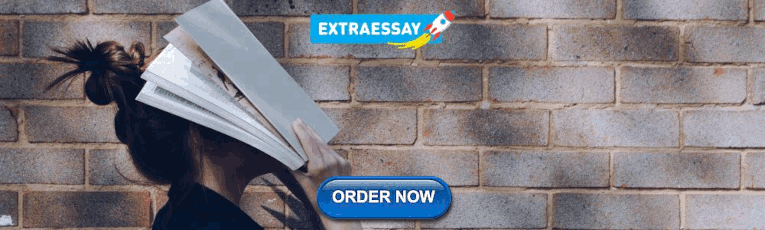
What are design patterns?
Design patterns are solutions to commonly occurring design problems in developing flexible software using object-oriented programming . Design patterns typically use classes and objects, but you can also implement some of them using functional programming . They define how classes should be structured and how they should communicate with one another in order to solve specific problems.
Some beginners may mix up design patterns and algorithms . While an algorithm is a well-defined set of instructions, a design pattern is a higher-level description of a solution. You can implement a design pattern in various ways, whereas you must follow the specific instructions in an algorithm. They don’t solve the problem; they solve the design of the solution.
Design patterns are not blocks of code you can copy and paste to implement. They are like frameworks of solutions with which one can solve a specific problem.
Classification of design patterns
The book, Design Patterns- Elements of Reusable Object-Oriented Software written by the Gang of Four (Erich Gamma, John Vlissides, Ralph Johnson, and Richard Helm) introduced the idea of design patterns in software development. The book contains 23 design patterns to solve a variety of object-oriented design problems. These patterns are a toolbox of tried and tested solutions for various common problems that you may encounter while developing software applications.
Design patterns vary according to their complexity, level of detail, and scope of applicability for the whole system. They can be classified into three groups based on their purpose:
- Creational patterns describe various methods for creating objects to increase code flexibility and reuse.
- Structural patterns describe relations between objects and classes in making them into complex structures while keeping them flexible and efficient.
- Behavioral patterns define how objects should communicate and interact with one another.
Why should you use design patterns?
You can be a professional software developer even if you don't know a single design pattern. You may be using some design patterns without even knowing them. But knowing design patterns and how to use them will give you an idea of solving a particular problem using the best design principles of object-oriented programming. You can refactor complex objects into simpler code segments that are easy to implement, modify, test, and reuse. You don’t need to confine yourself to one specific programming language; you can implement design patterns in any programming language. They represent the idea, not the implementation.
Design patterns are all about the code. They make you follow the best design principles of software development, such as the open/closed principle ( objects should be open for extension but closed for modification ) and the single responsibility principle ( A class should have only one reason to change ). This article discusses design principles in greater detail.
You can make your application more flexible by using design patterns that break it into reusable code segments. You can add new features to your application without breaking the existing code at any time. Design patterns also enhance the readability of code; if someone wants to extend your application, they will understand the code with little difficulty.
What are useful design patterns?
Every design pattern solves a specific problem. You can use it in that particular situation. When you use design patterns in the wrong context, your code appears complex, with many classes and objects. The following are some examples of the most commonly used design patterns.
Singleton design pattern
Object oriented code has a bad reputation for being cluttered. How can you avoid creating large numbers of unnecessary objects? How can you limit the number of instances of a class? And how can a class control its instantiation?
Using a singleton pattern solves these problems. It’s a creational design pattern that describes how to define classes with only a single instance that will be accessed globally. To implement the singleton pattern, you should make the constructor of the main class private so that it is only accessible to members of the class and create a static method (getInstance) for object creation that acts as a constructor.
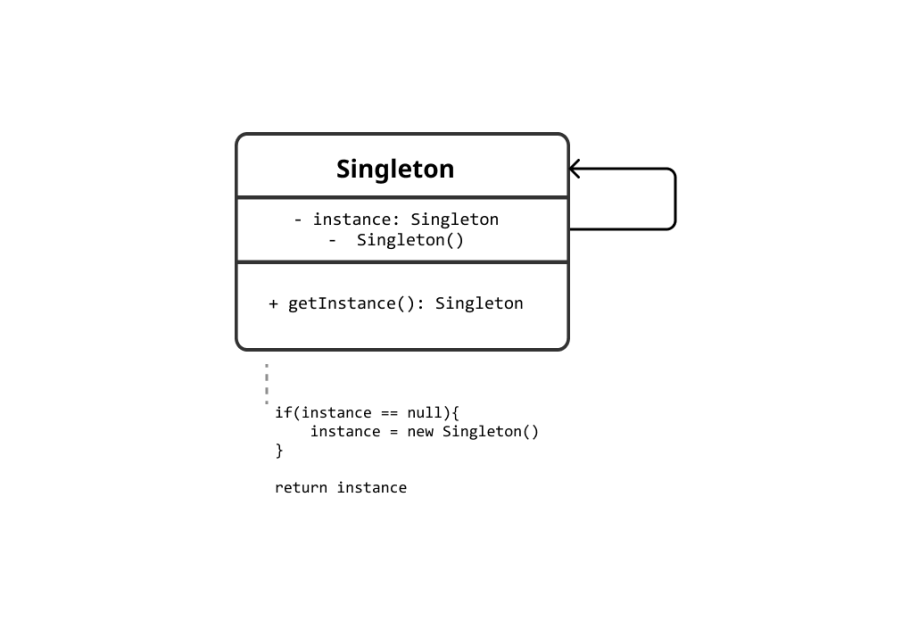
Here’s the implementation of the singleton pattern in Python.
The above code is the traditional way to implement the singleton pattern, but you can make it easier by using __new__ or creating a metaclass).
You should use this design pattern only when you are 100% certain that your application requires only a single instance of the main class. Singleton pattern has several drawbacks compared to other design patterns:
- You should not define something in the global scope but singleton pattern provides globally accessible instance.
- It violates the Single-responsibility principle.
Check out some more drawbacks of using a singleton pattern .
Decorator design pattern
If you’re following SOLID principles (and in general, you should), you’ll want to create objects or entities that are open for extension but closed for modification. How can you extend the functionality of an object at run-time? How can you extend an object’s behavior without affecting the other existing objects? You might consider using inheritance to extend the behavior of an existing object. However, inheritance is static. You can’t modify an object at runtime. Alternatively, you can use the decorator pattern to add additional functionality to objects (subclasses) at runtime without changing the parent class. The decorator pattern ( also known as a wrapper ) is a structural design pattern that lets you cover an existing class with multiple wrappers.
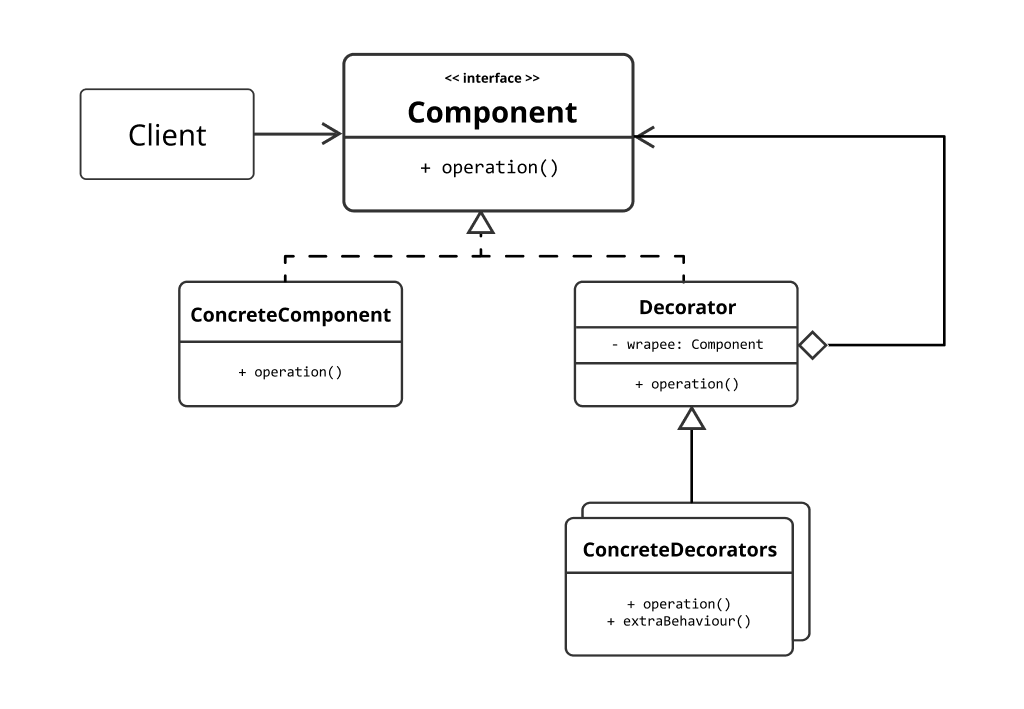
For wrappers, it employs abstract classes or interfaces through composition (instead of inheritance). In composition, one object contains an instance of other classes that implement the desired functionality rather than inheriting from the parent class. Many design patterns, including the decorator, are based on the principle of composition. Check out why you should use composition over inheritance .
The above code is the classic way of implementing the decorator pattern. You can also implement it using functions.
The decorator pattern implements the single-responsibility principle. You can split large classes into several small classes, each implementing a specific behavior and extend them afterward. Wrapping the decorators with other decorators increases the complexity of code with multiple layers. Also, it is difficult to remove a specific wrapper from the wrappers' stack.
Strategy design pattern
How can you change the algorithm at the run-time? You might tend to use conditional statements. But if you have many variants of algorithms, using conditionals makes our main class verbose. How can you refactor these algorithms to be less verbose?
The strategy pattern allows you to change algorithms at runtime. You can avoid using conditional statements inside the main class and refactor the code into separate strategy classes. In the strategy pattern, you should define a family of algorithms, encapsulate each one and make them interchangeable at runtime.
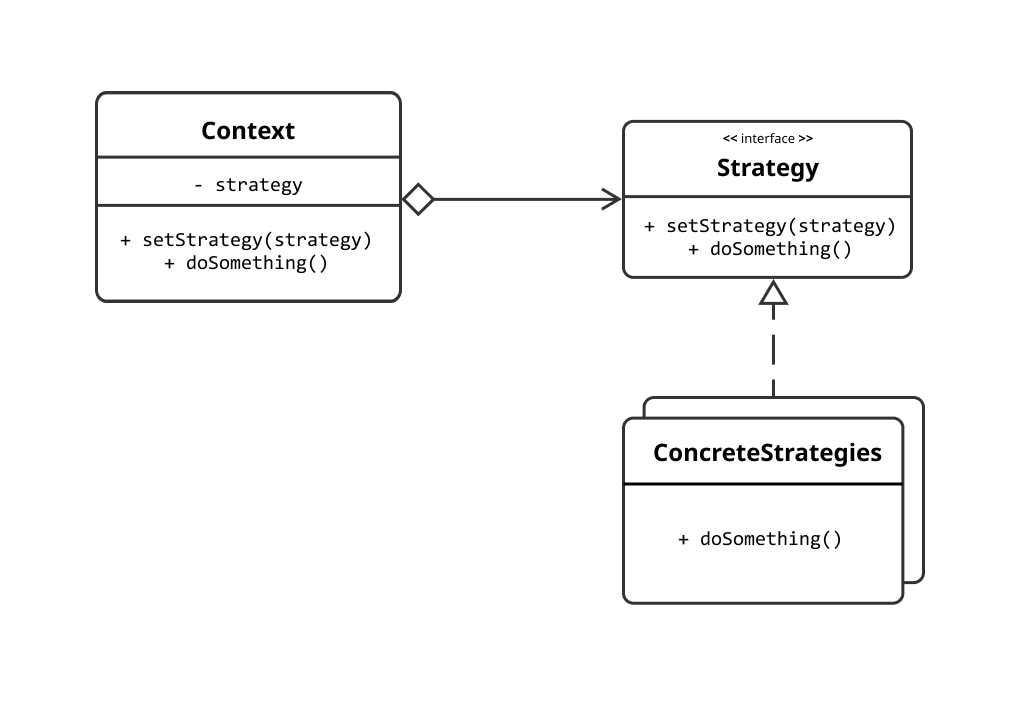
You can easily implement the strategy pattern by creating separate classes for algorithms. You can also implement different strategies as functions instead of using classes.
Here’s a typical implementation of the strategy pattern:
In the above code snippet, the client code is simple and straightforward. But in real-world application, the context changes depend on user actions, like when they click a button or change the level of the game. For example, in a chess application, the computer uses different strategy when you select the level of difficulty.
It follows the single-responsibility principle as the massive content main (context) class is divided into different strategy classes. You can add as many additional strategies as you want while keeping the main class unchanged (open/closed principle). It increases the flexibility of our application. It would be best to use this pattern when your main class has many conditional statements that switch between different variants of the same algorithm. However, if your code contains only a few algorithms, there is no need to use a strategy pattern. It just makes your code look complicated with all of the classes and objects.
State design pattern
Object oriented programming in particular has to deal with the state that the application is currently in. How can you change an object’s behavior based on its internal state? What is the best way to define state-specific behavior?
The state pattern is a behavioral design pattern. It provides an alternative approach to using massive conditional blocks for implementing state-dependent behavior in your main class. Your application behaves differently depending on its internal state, which a user can change at runtime. You can design finite state machines using the state pattern. In the state pattern, you should define separate classes for each state and add transitions between them.
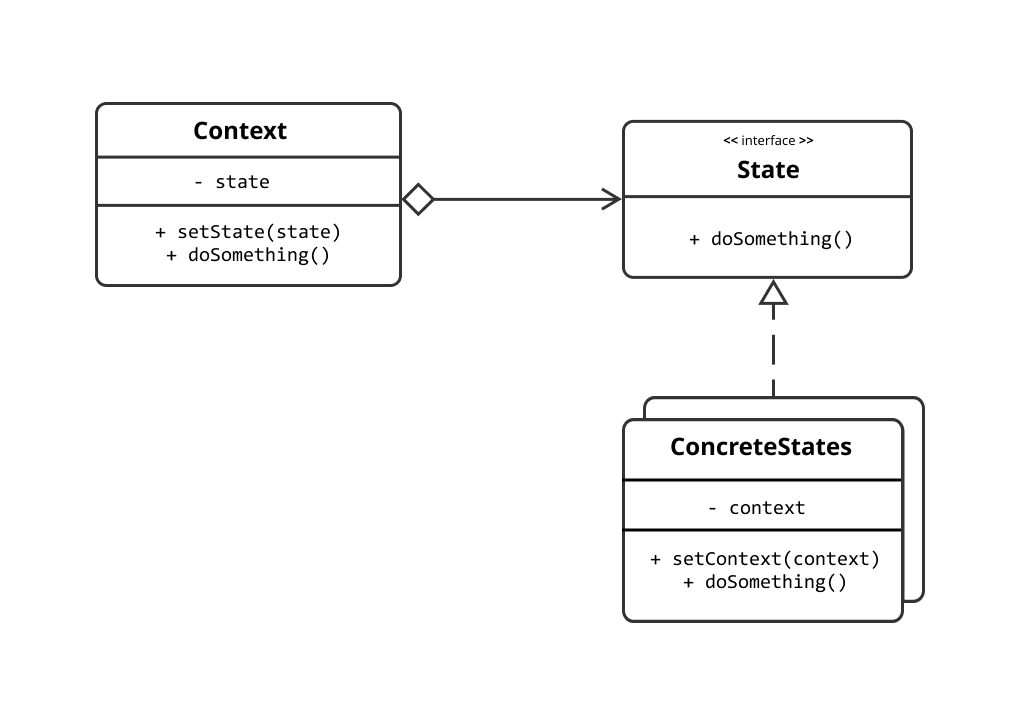
State pattern follows both the single-responsibility principle as well as the open/closed principle. You can add as many states and transitions as you want without changing the main class. The state pattern is very similar to the strategy pattern, but a strategy is unaware of other strategies, whereas a state is aware of other states and can switch between them. If your class (or state machine) has a few states or rarely changes, you should avoid using the state pattern.
Command design pattern
The command pattern is a behavioral design pattern that encapsulates all the information about a request into a separate command object. Using the command pattern, you can store multiple commands in a class to use them over and over. It lets you parameterize methods with different requests, delay or queue a request’s execution, and support undoable operations. It increases the flexibility of your application.
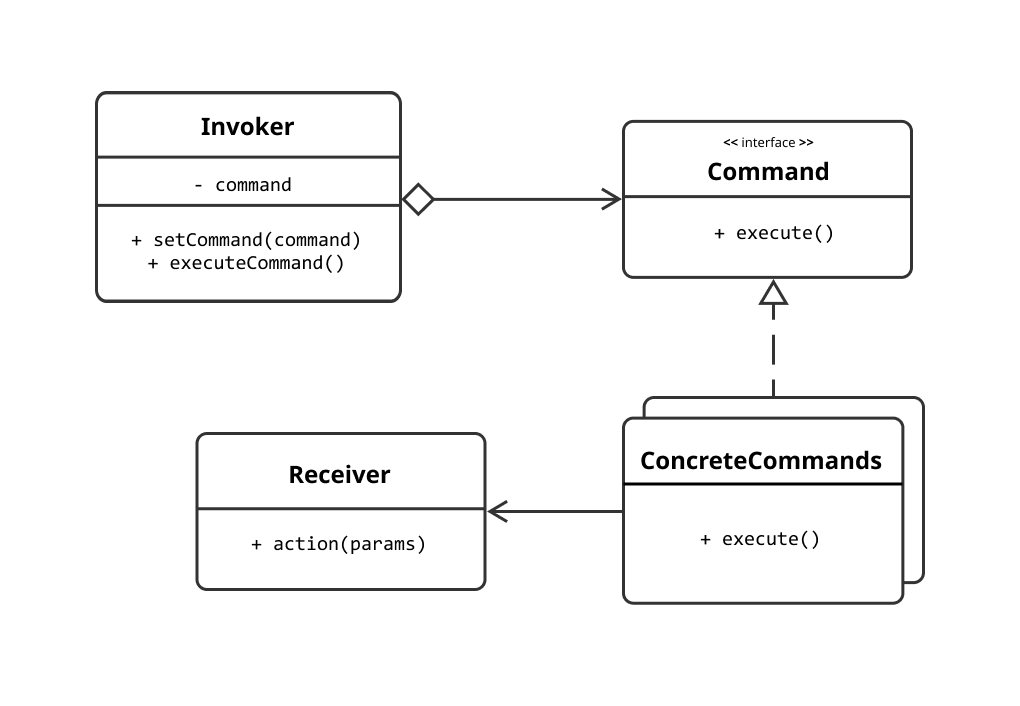
A command pattern implements the single-responsibility principle, as you have divided the request into separate classes such as invokers, commands, and receivers. It also follows the open/closed principle. You can add new command objects without changing the previous commands.
Suppose you want to implement reversible operations (like undo/redo) using a command pattern. In that case, you should maintain a command history: a stack containing all executed command objects and the application’s state. It consumes a lot of RAM, and sometimes it is impossible to implement an efficient solution. You should use the command pattern if you have many commands to execute; otherwise, the code may become more complicated since you’re adding a separate layer of commands between senders and receivers.
According to most software design principles including the well-established SOLID principles, you should write reusable code and extendable applications. Design patterns allow you to develop flexible, scalable, and maintainable object-oriented software using best practices and design principles. All the design patterns are tried and tested solutions for various recurring problems. Even if you don't use them right away, knowing about them will give you a better understanding of how to solve different types of problems in object-oriented design. You can implement the design patterns in any programming language as they are just the description of the solution, not the implementation.
If you’re going to build large-scale applications, you should consider using design patterns because they provide a better way of developing software. If you’re interested in getting to know these patterns better, consider implementing each design pattern in your favorite programming language.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Latest commit
File metadata and controls, practical python design patterns.
Welcome to the Practical Python Design Patterns repository! Here, you'll find practical examples and solutions from the book "Practical Python Design Patterns: Pythonic Solutions to Common Problems" by Wessel Badenhorst, published in 2017. This repository serves as a valuable resource to explore and understand various design patterns in Python.
About the Book
"Practical Python Design Patterns" is a comprehensive guide that provides you with all the essential constructs you need to become proficient in Python. Whether you're a seasoned software engineer or just starting your journey with Python, this book is a must-have resource.
Patterns Covered
In this repository, you'll find examples and implementations of a wide range of design patterns covered in the book. These patterns are powerful tools for solving common programming challenges efficiently. Here's a quick reference to the patterns you'll discover:
Singleton Pattern
Prototype Pattern
Factory Pattern
Builder Pattern
Adapter Pattern
Decorator Pattern
Facade Pattern
Proxy Pattern
Chain of Responsibility Pattern
Command Pattern
Interpreter Pattern
Iterator Pattern
Observer Pattern
State Pattern
Strategy Pattern
Template Method Pattern
Visitor Pattern
Model-View-Controller Pattern
Publisher-Subscriber Pattern
Getting Started
To explore these design patterns, simply navigate through the repository's folders. Each pattern has its dedicated folder containing Python code examples and explanations. You can clone or download this repository to access the code and start experimenting with these patterns in your own Python projects.
Contribution
If you have any insights, improvements, or additional examples related to the design patterns covered in the book, feel free to contribute to this repository. Your contributions are highly valued and can help other Python enthusiasts and developers better understand and apply these design patterns.
The code and content in this repository are provided under the terms of the MIT License . Please review the license before using or contributing to this project.
Happy coding and exploring the world of Python design patterns!
Practice Problems
Problems to be submitted (20 points).
- ITERATOR pattern?
- OBSERVER pattern?
- STRATEGY pattern?
- COMPOSITE pattern?
- ADAPTER pattern?
If a design pattern was used somewhere in the code, please indicate where/how it was used.
Note/Hint: Please include any design patterns employed by the various Java classes used in the code - e.g. JPanel , etc.
- SceneEditor.java
- SceneComponent.java
- CarShape.java
- HouseShape.java
- CustomShape.java
- SelectableShape.java
- SceneShape.java
- Create a new class called CompositeShape , which will serve as our "Composite" class. For simplicity, have it extend our "Leaf" class, CustomShape (there's no rule that the "Composite" can't extend the "Leaf" class...) .
- draw all the aggregated SceneShapes,
- translate all the aggregated SceneShapes by the desired (dx,dy) , and
- check all the aggregated SceneShape to see if any of them contain the specified point
- Create a new class called WindowShape that creates a small window graphic. Make it more interesting than just a simple square... For example, one option would be to draw horizontal and vertical pane separators (which would look something like 2x2 grid of squares...).
- Add another button in SceneEditor that creates an instance of this WindowShape. Make sure that you can select and de-select it, as expected.
- Finally, add another button that creats a CompositeShape that includes both a House and a Window (positioned appropriately inside the House). So, in the button's listener, first create a CompositeShape. Then create a HouseShape object and add it to that CompositeShape. Last, repeat with a WindowShape, but be sure to supply an offset to addShape() to position it in a reasonable location with the House. Finally, add the CompositeShape to the scene, and make sure that the entirety of it can be selected with a single mouse click, as expected.
Extra Credit
- (1 point) In part 3g, we used the Integer.parse() method to convert Strings to integers. However, if the String contains non-numeric characters, this parsing will throw an exception. Apply Java's try-catch structure (discussed in Chap. 1, among other places) around the parsing command(s), and have it generate a pop-up window (in a JOptionPane ) that conveys an appropriate error message when such an error occurs.
- (1 point) If you implementation in part 3g used String's split() method to read the string from a single text field and then convert to an array of strings that are then each parsed into numbers, there's also the potential problem of not having enough numbers (in this case we're expecting two numbers, separated by a comma). If too few numbers are given, another exception can occur. Catch this exception and convey an appropriate error message in a pop-up window.
- System Design Tutorial
- What is System Design
- System Design Life Cycle
- High Level Design HLD
- Low Level Design LLD
- Design Patterns
- UML Diagrams
- System Design Interview Guide
- Crack System Design Round
- System Design Bootcamp
- System Design Interview Questions
- Microservices
- Scalability
Design Patterns in Object-Oriented Programming (OOP)
- Best Practices of Object Oriented Programming (OOP)
- Perl - Attributes in Object Oriented Programming
- Object Oriented Programming | HCI
- OOPs | Object Oriented Design
- R - Object Oriented Programming
- Object Oriented Paradigm in Object Oriented Analysis & Design(OOAD)
- How to design a parking lot using object-oriented principles?
- Object Oriented Programming (OOPs) in Perl
- Design Goals and Principles of Object Oriented Programming
- Object Oriented Programming in C++
- Object Oriented Programming (OOPs) in MATLAB
- Introduction of Object Oriented Programming
- Object Oriented Programming (OOPs) Concept in Java
- Object Oriented Programming System (OOPs) For Kids
- Differences between Procedural and Object Oriented Programming
- Object-Oriented Programming in Ruby | Set 1
- Object Oriented Programming in Ruby | Set-2
- Four Main Object Oriented Programming Concepts of Java
- Object Oriented Programming | Set 1
Software Development is like putting together a puzzle. Object-oriented programming (OOP) is a popular way to build complex software, but it can be tricky when you face the same design problems repeatedly. That’s where design patterns come in.
Design patterns are like well-known recipes for common problems in software development. They’re not step-by-step instructions, but more like guidelines to help you solve these problems in a flexible and efficient way. These patterns gather the wisdom of the software development community, making it easier for developers to work together and create software that’s easy to maintain, adapt, and reuse.
Important Topics for the Design patterns in object-oriented programming
Singleton Pattern
Factory method pattern, abstract factory method pattern, builder pattern, adapter pattern, proxy pattern, decorator pattern, composite pattern, observer pattern, strategy pattern, command pattern, state pattern, template method pattern, visitor pattern, memento pattern.
The Singleton Pattern is a design pattern in object-oriented programming that ensures a class has only one instance and provides a global point of access to that instance. This means that regardless of how many times the class is instantiated, there will always be only one instance, which can be accessed from any part of the program.
Explanation with Real-World Example
Consider a real-world analogy of a government in a country. In this context:
- Singleton Class: The government can be represented as a Singleton class. Only one government exists for a particular country.
- Instance Creation: When you create a government, you’re essentially creating the one and only instance of that government for the country.
- Access Point: To interact with the government, you don’t create a new government every time you need something from it. Instead, you access the existing government. This ensures that the decisions and actions of the government are consistent and centralized.
The Factory Method Pattern is a creational design pattern in object-oriented programming. It defines an interface for creating an object but lets subclasses alter the type of objects that will be created. It provides an abstract method for creating an object, and the concrete subclasses of the factory class implement this method to produce objects of a specific type.
Consider a real-world example of a vehicle manufacturing company. The company produces different types of vehicles such as cars, motorcycles, and bicycles. In this context
- Factory Class: The vehicle manufacturing company can be seen as the factory class, which defines an abstract method.
- Concrete Factories: There will be multiple concrete factory classes, each responsible for creating a specific type of vehicle. For example, CarFactory, MotorcycleFacotry and BicycleFactory.
- Products: The products are the vehicles themselves- cars, motorcycles, or bicycles.
The Factory Method Pattern is used in software development when you need to decouple the object creation from the object’s usage.
The Abstract Factory Pattern is a creational design pattern in object-oriented programming that provies an interface for creating families of related or dependent objects without specifying their concrete classes. It’s a higher-level pattern compared to the Factory Method Pattern, where you have not just one factory but a family of factories that work together to create related objects.
Explanation with Real World Example
Let’s consider a real-world example of a furniture manufacturer. This manufacturer produces various types of furniture sets, such as modern and vintage sets. Each set consists of several pieces of furniture, including a table, chiars, and a sofa. In this context:
- Abstract Factory: The abstract factory represents the furniture factory, which defines methods for creating different types of furniture products.
- Concrete Factories: There are two concrete factories – ModernFurnitureFactory and VintageFurnitureFactory. Each of these factoreis creates a complete set of furniture in a consistent style.
- Product Families: The product families are the sets of furniture (e.g., modern or vintage) with individual objects (e.g., table, chairs, sofa).
The Abstract Factory Pattern is used when you need to ensure that the created objects are compatible and belong to a consistent family.
The Builder Pattern is a creational desing pattern in object-oriented prgramming that is used to construct a complex obect step by step. It separates the construction of a complex object from its representation, allowing the same construction process to create different representations of an object. It is particularly used when an object has a large number of parameters, some of which are optional, and it provides a more readable and maintainable way to create objects with different configurations.
Imagien a real-world scenario of building a custom computeer. When ordering a custom computer, you have various components to choose from, such as the processor, memory, storage, graphics card, and so on. This customization process can be represented using the Builder Pattern:
- Director Pattern: In this case, the computer seller acts as the director. They understand the configuration options and guide you through the customization process.
- Builder: The builder is responsible for constructing the computer step by steo. It takes your choices for each component and assembles the computer accordingly.
- Product: The end result is your custiom-build computer, which can have various configurations based on your device.
The Builder Pattern is used in situations where you need to create complexo objects with multiple configuration options, especially when some of these optins are optional or can have default values.
The Adapter Pattern is a structural design pattern in object-oriented programming that allows objects with incompatilbe interfaces to work together. It acts as a bridge between two incompatible interfaces, making them compatible without changing acutal classes. The Adapter Pattern involves a single class called the adapter that is responsible for joining functionalities from independent or incompatible interfaces.
Let’s consider a real-world scenario involving electrical outlets. In different countries, you often find different types of electrical outlets and plugs. For instance the United States uses Type A and Type B outlets with flat pins, while Europe uses Type C and Type F outlets with round pins. If you have an appliance with a U.S. plug and want to use it in Europe, you’ll need an adapter:
- Clinet: The appliance (e.g., a laptio charger) is the client, designed for a U.S. outlet.
- Target Interface: The European outlet is the target interface. It’s what the client needs to connect to.
- Adapter: The adpater, in this case, is the physical device that converts the U.S. plug to the European outlet.
The Adapter Pattern is used when you want to make two incompatible interfaces work together.
The Proxy Pattern is a structural design pattern in object-oriented programming. It provides a surrogate or placeholder for another object to control access to it. In other words, a proxy acts as an intermediary or representative for an object, allowing you to add extra funtionality, such as lazy loading, access control, or caching, without altering the underlying object.
Consider a real-world example of a proxy in the context of a smart card access system:
- Real Object: The real object in this scenario is the physical access control system, which authenticates users and grants or denies access based on their credentials.
- Proxy: The proxy, in this case, is a security guard who stands between users and the access control system. The security guard check user credentials, verify identities, and decide whether to grant access. Users interact with the security guard rather than the access control system.
- Client: Users who want to enter a secure area. Instead of interacting directly with the access control system, they interact with the security guard (proxy).
The security guard (proxy) can provide additional services, such as logging access, verifying IDs, or controlling the flow of people, without the users (client) needing to be aware of these operations. This is analogous to how a proxy in software provide caching, lazy loading, or access control without affecting the underlying object.
The Proxy Pattern is used in various scenarios where you want to control access to an object or add additional functionality without changing the object’s code.
The Decorator Pattern is a structural desing pattern in object-oriented programming. It allows you to dynamically add new behavior or responsibilities to objects without altering their code. This pattern is acheived by creating a set of decorator classes that are used to wrap the original object. Each decorator addis its own functionality while delegating the rest of the work to the wrapped object.
Explantion with Real World Example
Imagine you are ordering a coffee from a cafe. You can choose a base coffee, such as espresso, and then add various toppings to enhance the coffee. Each topping adds a specific flavor or characteristic to the baes coffeee without changing the coffee itself. In this context:
- Component: The base coffee, such as espresso, represents the core component that you want to enhance.
- Concrete Component: Espresso is a concrete component, as it’s the initial coffee you start with.
- Decorator: The toppings like caramel, whipped cream, or cinnamon represent decorators. They add extra flavor or features to the core coffee.
- Concrete Decorator: Each topping is a concrete decorator that extends the base coffee with specific features.
The Decorator Pattern is used in situations where you need to add responsibilities or behaviors to objects dynamically and without altering their underlying class.
The Composite Pattern is a structural design pattern in object-oriented programming that allows you to compose objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly. In other words, you can work with individual objects and their collections in a consistent way, abstracting away the differences between them.
In a file system, you have a hierarchical structure with directories (composites) containing files (leaves). This structure can be effectively modeled using the Composite Pattern:
- Component: The component represehts the common interface for all file system elements.
- Leaf (File): A leaf is a concrete file, which cannot have child elements.
- Composite (Directory): A composite is a directory that can contain child elments, including other directories and files.
Here’s how this example works:
- Client Code: When you want to work with the file system, you don’t need to differentiate between individual files and directoreis. You can treat all elements uniformly.
- Tree Structure: The components, whether leaf of compsite, are organized in a tree structure to represent the file system’s hierarchical nature.
The Observer Pattern is a behavioral desing pattern in object-oriented programming. It defiens a one-to-many dependency between objects so that when one object (the subject) changes its state, all its dependents (observers) are notified and updated automatically. In other words, the observer pattern allows one object to inform a list of other objects about changes without knowing who or what those objects are.
Think of a real-world scenario of a news agency and its subscribers:
- Subject (News Ajency): The news agency is the subject. It generates news and broadcasts it.
- Observer (Subscribers): Subscribers are the observers. They subscribe to the news agency to receive updates.
- Update Mechanism: When the news agency publishes a new article or breaking news, all subscribers receive the updates without the news agency knowing who they are or how many there are.
This real-wrold analogy demonstrates how the observer patten works. The news agenchy (subject) informs its subscribers (observers) about changes without needing to be aware of the specific subscribers.
The Observer Pattern is used in various scenarios where a one-to-many relationship exists between objects, and one subject needs to notify multiple others about changes.
The Strategy Pattern is a behafvioral design pattern in object-oriented programming. It defines a family of algorithms, encapsulates each one, and makes them interchangeable. The pattern allows a client to choose and use an algorithm from a family of algorithms at runtime. It separates the algorithm from the client, making the algorithm’s implementation details hidden from the detail.
Let’s consider a real-world example involving a navigation application that provides various routing options to users:
- Context (Navigation Application): The navigation applications is the context that requires different routing algorithms. It represents the client.
- Strategy (Routing Algorithms): The routing algorithms are the strategies. They encapsulate various methods to calculates notes, such as Shortest Route, Scenic Route and Avoid Traffic Route.
- Client’s Choice: The user of the navigation application can choose the routing strategy they prefer. For example, if they select Avoid Traffic Routhe the application employs that specific routing algorithm.
This real world example illustrates how the Strategy Pattern allows users to select a specific routing algorithm without needing to understand the inner workings of the algorithm or the application.
The Strategy Pattern is used in situations where multiple algorithms or strategies are available for a specific task, and you want to select and use one of them at runtime.
The Command Pattern is a behaviroal desing pattern in object-oriented programming that encapsulats a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. It turns a request into a standalone object with its own class, parameters, and methods to invoke the request. This pattern decouples the sender and receiver of a request. allowing for the sender to issue requests without needing to know the specific operations or the receiver’s class.
Let’s consider a real-world scenario involving a remote control for a television:
- Command: In this context, a command is a representation of a specific operation, like turning the TV on, changing the channel, or adjusting the volume. Each command is encapsulated as an object with a defined method.
- Invoker (Remote Control): The remote control is the invoker. It holds a list of commands and can execute them without needing to know the details of the TV’s internal workings.
- Receiver (TV): The television is the receiver. It understandsthe individual commands, such as how to turn on or change channels. The commands act as intermediaries to invoke the appropriate methods on the receiver.
In this example, the Command Pattern allows the remote control (invoker) to issue various commands to the TV (receiver) without requiring a deep understanding of the TV’s internal operations. The remote control interacts with commands that encapsualte specific actions, providing a more flexible and decoupled way to control the TV.
The Command Pattern is used in various scenarios where you want to encapsulate requests as objects and decouple the sender and receiver of the requests.
The State Pattern is a behavioral desing pattern in object-oriented programing. It allows one object to alter its behavior when its internal state changes. The pattern acheives this by representing each state as an object and delegating the state-specific behavior to these state objects. The context (the object that uses the state) holds a reference to the curreent state object and delegates operations to it.
Let’s consider a real-world example involving a vending machine, which can have different states based on its current condition:
- Context (Vending Machine): The vending machine represnts the context. It can be in different states like idle, payment in progress, and item dispensed. The context is responsible for maintaining the current state and delegating actions accordingly.
- State (State of Vending Machine): Each state is represented as on object (e.g., idle state, payment in progress state, item dispensed state). These state objects encapsulate the behavior associated with that particular state.
- Transition: When a user interacts with the vending machine (e.g., inserts money or select an item), the context switches from one state to another. Each state knows how to handle actions specific to that state.
In this example, the State Pattern allows the vending machine to change its behavior based on its current state without using a complex conditional structure. It simplifies the code, making it more modular and maintainable.
The State Pattern is used in scenarios where object can exist in multiple states, and the behavior of the object must change dynamically based on its state.
The Template Method Pattern is a behavioral desing pattern in object-oriented programming. It defines the skeleton of an algorithm in a method, deferring some steps to subclasses. It allows subclasses to redefine certain steps of an algorithm wihout altering the algorithm’s structure. This pattern promotes reusability by providing a common structure for a family of related algorithms.
Let’s consider a real-world example involving the prepartaion of a hot beverage such as coffee or tea:
- Template Method (Beverage Recipe): The template method represents the common structure of making a hot beverage. It outlines the algorithm, including steps like boiling water, brewing the beverage, and serving it. Some steps are defined in the template method, while others are left as abstract methods to be implemented by concrete subclasses.
- Concrete Sublclasses (Coffee, Tea): Concrete subclasses represent specific beverages (e.g., Coffee, Tea). They inherit the common structure from the template method but provide their own implementation for steps like brewing. For example, Coffee and Tea will have different methods for brewing coffee and tea, respectively.
- Hook Methods: The template method may include hook methods, which are methods with default implementation that can be overridden by concrete subclasses. For example, a hook method can allow the addition of sugar or milk, which is optional and may vary between beverages.
In this example, the Template Method Pattern provides a common structure for making hot beverages, but it allows individual beverages to customize specific steps while preserving the overall algorithm.
The Template Method Pattern is used in scenarios where you want to define the outline of an algorithm and allows subclasses to provide specific implementations for certain steps.
The Visitor Pattern is a behavioral design pattern in object-oriented programming that allows you to add further operations to objects without having to modify them. It i used when you have a set of objects with different types, and you want to perfrom a common operation on them without modifying their classes. The pattern achieves this by defining a separate visitor class or interface, which is responsible for performing operations on these objecs. Each object accepts the visitor and delegates the operation to it.
Consider a real-world example of a tax calculation system in a finance application:
- Elements (Taxable Items): The elements are different taxable items such as products, services, and investments. Each taxable item is a distinct class with its properties and methods.
- Visitor (Tax Calculator): The visitor, in this case is the tax calculator. It’s a separate class or set of classes responsible for performing tax calculations on various taxable items.
- Operation (Tax Calculation): The operation is the tax calculation itself. It’s a specific operation that needs to be performed on each taxable item. The tax calculator class defines methods for different types of taxable items to calculate taxes.
In this example the Visitor Pattern allows you to add new tax calculation functionality to your finance application without modifying the existing classes of taxable items. Each taxable item can accept the tax calculator (visitor) and delegate the tax calculation operation on it.
The Visitor Pattern used in situations where you have a set of objects with different types or classes and you want to perform a common operation on all of them.
The Memento Pattern is a behavioral desing pattern in object-oriented programmming that provides a way to capture and externalize an object’s interal state without violating its encapsulation. It allows an object to capture its current state in a memenot object, which can later be used to restore the object to that state. The pattern is particularly useful when you need to implement undo/redo functionality or save and restore an object’s state.
Consider a real-world example involving a text editor:
- Originator (Text Editor): The originator is the text editor. It’s the object whose state you want to save and restore. The text editor has a current document with text.
- Memento (Snapshot): The memento represents a snapshot of the text editor’s state. It captures the content of the current document.
- Caretaker (History Manager): The caretaker, in this case, is the history manager. It keeps a stack of mementos (snapshots) taken at various points in the text editor’s history.
In this scenario, the Memento Pattern allows the text editor to save its state by creating a memnto object (snapshot) and pushing it onto the history manager’s stack. Later, if the users wants to undo an action, the history manager can pop the latest memento from the stack and use it to restore the text editor’s state to a previous point in time.
Desing pattern in object-oriented programming are like blueprints for solving common problems. By understanding and using these patterns, developers can write more maintainable, scalable, and efficient code. Whether you’re creating a complex GUI, managing database connection, or designing a game, there’s a design pattern that can help you build better software. So, keep these patterns in mind as valuable tools for your programming journey. Happy Coding!
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Design Pattern
- Geeks Premier League
- System Design
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Assignment 4: Design Patterns
Assignment 4a:.
- what kind of problem(s) you can solve with that pattern and when you use it, maybe with a short example
- how the pattern works, what the basic idea of the pattern is
- what the main advantage and what the the main disadvantage is of using this pattern
Assignment 4b:
Credit card problem :, assignment 4c:, starting point:.
- Think in small objects: each field might be an object, you might have subclasses of fields (mined fields, save fields), you might have classes for the different kind of covers (plain cover, question mark cover, flag cover).....
- Distinguish between the visual things and the effective domain specific things (what concepts would remain if your connection to the computer were not a graphical user interface?)
- If you are not sure if you should include a certain pattern or not, include it and mention in the drawbacks what makes you doubt if the design pattern is really appropriate.
Deliverables:
- Model of the minesweeper:
- Class diagram showing essential classes, associations with names, important attributes, important operations available to other classes. Add descriptions etc. where necessary to make the diagram easily understandable for another person while keeping it as short as possible.
- Design patterns:
- Use class-, object- or interaction-diagrams to show how you used the pattern, if adequate also short textual description how you used the pattern.
- List advantages of using the pattern (e.g., "gives clean solution to.....", "enhances maintenability because....."). This should also describe the problem you have solved by using the pattern and the positive consequences of using the pattern.
- List drawbacks and negative consequences of using the pattern (e.g., "overkill in this situation").
- Reviews / Why join our community?
- For companies
- Frequently asked questions
User Interface (UI) Design Patterns
What are user interface (ui) design patterns.
User interface (UI) design patterns are reusable/recurring components which designers use to solve common problems in user interface design.
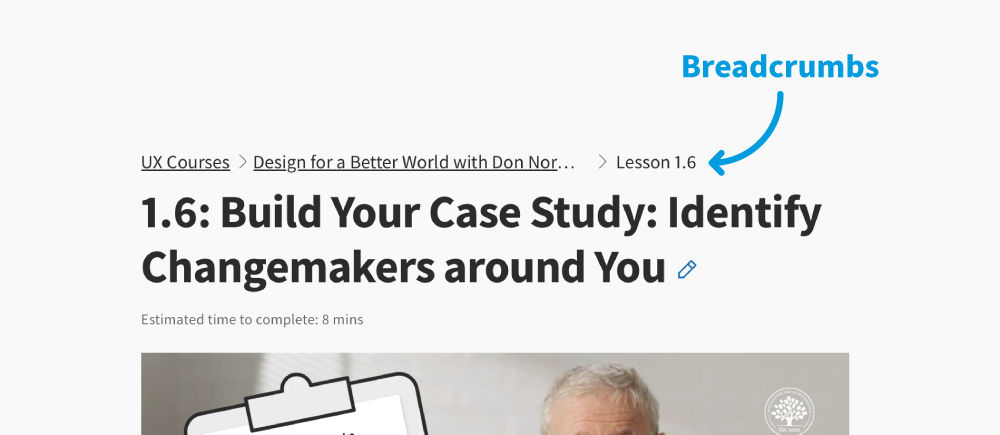
© Interaction Design Foundation, CC BY-SA 4.0
For example, the breadcrumbs design pattern lets users retrace their steps.
Designers can apply them to a broad range of cases, but must adapt each to the specific context of use.
Why Design Patterns Are Such Powerful Design Aids
Websites and apps have a conventional look and feel because of design patterns such as global navigation and tab bars. In UI design , you can use design patterns as a quick way to build interfaces that solve a problem; for instance, a filter pattern is a versatile tool that helps the user extract, enhance, or manipulate data to achieve specific goals.
- Transcript loading…
UI design patterns serve as design blueprints that allow designers to choose the best and most commonly used interfaces for the user's specific context. Each pattern typically contains:
A user’s usability -related problem .
The context / situation where that problem happens.
The principle involved—e.g., error management.
The proven solution for the designer to implement to address the core of the problem.
Why —the reason for the pattern’s existence and how it can affect usability.
Examples —which show the pattern’s successful real-life application (e.g., screenshots and descriptions).
Implementation —some patterns include detailed instructions.
Common UI Design Patterns
Some of the most common UI design patterns are:
Breadcrumbs: Use linked labels to provide secondary navigation that shows the path from the front to the current site page in the hierarchy.
Lazy Registration: Forms can put users off registration. So, use this sign-up pattern to let users sample what your site/app offers for free or familiarize themselves with it. Then, you show them a sign-up form. For example, Amazon allows unrestricted navigation and cart-loading before it prompts users to register for an account. Note:
When content is accessible only to registered users or users must keep entering details, offer them simplified/low-effort sign-up forms .
Minimize/Avoid optional information fields . Use the Required Field Markers pattern to guide users to enter needed data.
Forgiving Format: Let users enter data in various formats (e.g., city/town/village or zip code).
Clear Primary Actions: Make buttons stand out with color so users know what to do (e.g., “Submit”). You may have to decide which actions take priority.
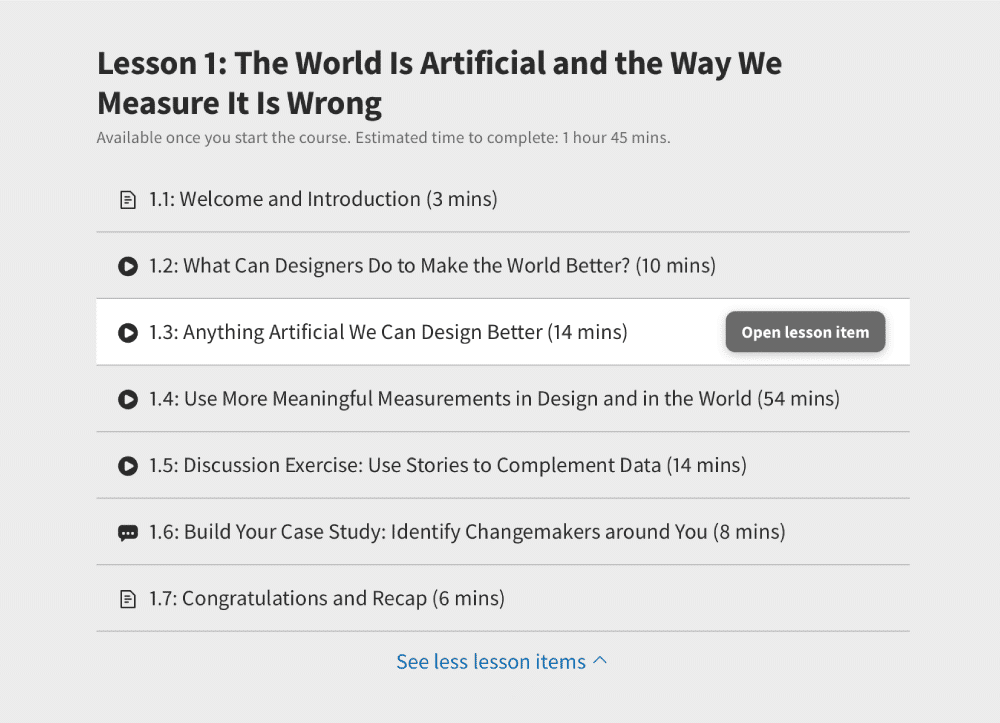
Here, we use the design pattern “clear primary action” to clearly show users where they can click.
Progressive Disclosure: Show users only features relevant for the task at hand, one per screen. If you break input demands into sections, you’ll reduce cognitive load (e.g., “Show More”).
Hover Controls: Hide nonessential information on detailed pages to let users find relevant information more easily.
Steps Left: Designers typically combine this with a wizard pattern. It shows how many steps a user has to take to complete a task. You can use gamification (an incentivizing design pattern) here to enhance engagement.
Subscription Plans: Offer users an options menu (including “Sign-up” buttons) for joining at certain rates.
Leaderboard: You can boost engagement if you use this social media pattern.
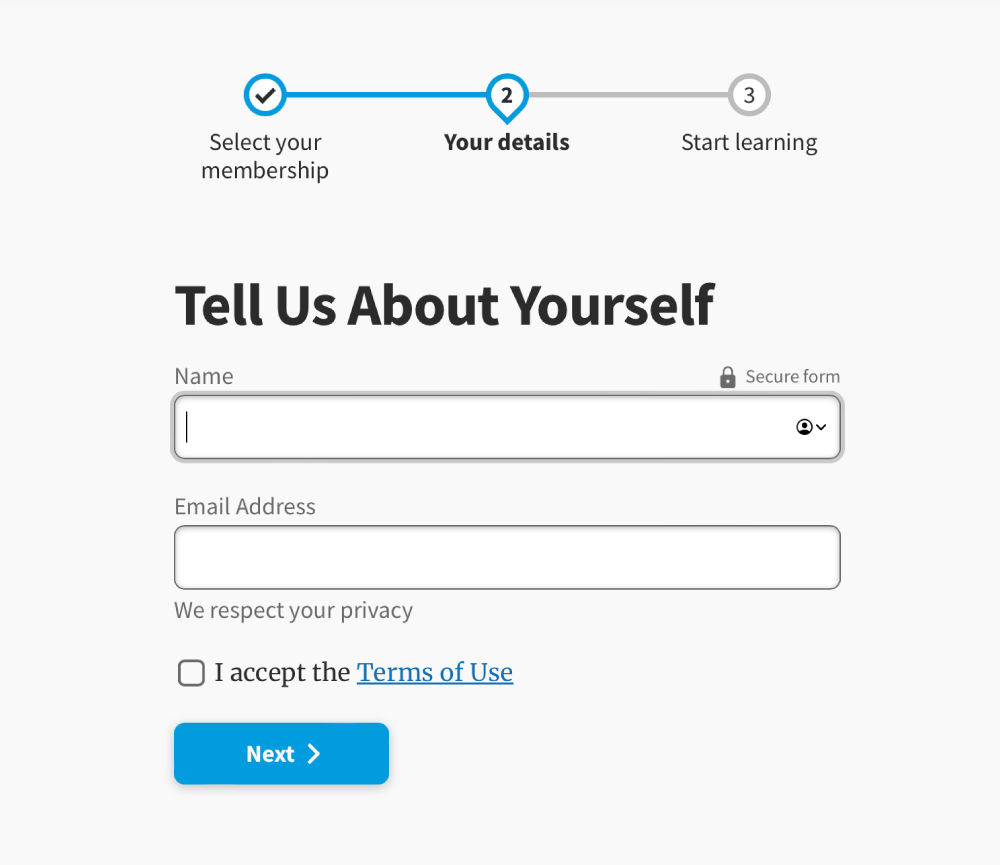
This page uses the design patterns progress tracker, clear primary actions and progressive disclosure.
Dark Patterns: Some designers use these to lead or trick users into performing certain actions, typically in e-commerce, so they spend more or surrender personal information. Dark patterns range in harmfulness. Some designers leave an unchecked opt-out box as a default to secure customer information. Others slip items into shopping carts. To use dark patterns responsibly, you must be ethical and have empathy with your users. Dark patterns are risky because user mistrust and feedback can destroy a brand’s reputation overnight.
How to Use Design Patterns
Freely available, UI design patterns let you save time and money since you can copy and adapt them into your design—instead of reinventing the wheel for every new interface. They also facilitate faster prototyping and user familiarity . However, you should use them carefully . The wrong choices can prove costly – for example, if you:
Approach problems incorrectly because you’re over-relying on patterns.
Don’t fine-tune patterns to specific contexts.
Don’t customize a distinct brand image (e.g., your website ultimately resembles Facebook).
Overlook management requirements. If you create your own patterns, you must clearly define how to use them and with what types of problems, version-control them, and store them for team access.
Overall, give users familiar frameworks that maximize convenience and prevent confusion while they interact with your unique-looking brand.
Future-Focused UI Patterns for Mobile
Let’s look at mobile UX design , more specifically, common mobile UI patterns. These can be used to design intuitive interfaces and speed up your design process.
Learn More about UI Design Patterns
Take our UI Design Patterns course .
Take our Mobile UI Design course .
Register to Complex UI Design: Practical Techniques Master Class webinar with Smashing Magazine creative lead Vitaly Friedman.
Explore UI Patterns in this library of real-life examples.
Read UX content strategist Jerry Cao’s detailed examination of mobile UI Design patterns .
Questions related to User Interface (UI) Design Patterns
A UI pattern is a repeatable solution to a standard design problem, ensuring consistent user experience. This video highlights the 'OK/Cancel' pattern, emphasizing the concept of proximity .
The pattern uses grouping to indicate that items, like the 'OK' and 'Cancel' buttons, relate to each other. This means users instinctively understand their relationship and function when they see these buttons together. Following a recognizable UI pattern, such as this, can significantly enhance a user's navigation experience. Check out the full video for insights on essential mobile UX design patterns.
Grid and Layout : The foundation of any design is its grid and layout. Proper alignment and spacing ensure that content is organized and easily digestible by users.
Typography : Text plays a vital role in conveying information. Choosing the right font and maintaining readability is paramount.
Hierarchy : This defines the order and prominence of elements, guiding users through the interface and highlighting important content.
Simplicity and Order : Our brains prefer order over chaos. A well-organized interface, free from clutter, allows faster processing and a more pleasant user experience.
By mastering these rules, designers can craft aesthetically pleasing and functional interfaces.
UI Design Patterns are essential, recurring solutions designers employ to address common interface dilemmas. These patterns simplify complex environments, ensuring users view vital content when needed.
For instance, in the clip, the Galaxus product search uses an, efficient UI patterns to provide a better filtering experience. Such designs prioritize user convenience, eliminating unnecessary auto-refreshes and incorporating intuitive features, especially in mobile views. Whether through slide-in filters or accordions, these patterns enhance navigation. Crucially, well-designed UI patterns improve user experience, mitigate usability problems, and bolster a product's success. They remain a cornerstone of user-centric design and product innovation.
UI patterns streamline user experience and can be classified into various categories. Some essential UI pattern categories include:
Onboarding : Engaging users with compelling visual design, like the Down Dog app.
Gestures : Intuitive actions like tap, swipe, and unique gestures made familiar by apps like Tinder.
Integration : Seamlessly connecting apps with other platforms, ensuring fluid transitions.
Back Buttons : Allowing users to navigate to previous content or sections without feeling lost.
Accordions : Efficient navigation tools, but must ensure users don't get lost within.
Contrast and Affordance : Showcasing navigational cues like open/close indicators.
Hamburger Menus : Simplified navigation menus, optimizing visibility and accessibility.
Search : Allowing users to search content within specified parameters effectively.
Videos : Engaging content that should be easily scannable, captioned, and accessible.
Customization : Providing users control of their data and avoiding random, non-desirable features.
Touch Targets : Ensuring clear signifiers for tapable items and preventing accidental touches.
These categories are essential for creating user-friendly, effective interfaces.
UI patterns and components are essential to interface design but serve distinct purposes. UI patterns are recurring solutions that address common design challenges in user interfaces. They provide consistent, efficient ways to solve usability issues, like how filtering should function in e-commerce sites without causing disruptive refreshes. On the other hand, components are the building blocks used within those patterns. For instance, in an e-commerce filter pattern, buttons, drop-down menus, and checkboxes are the components. Simply put, UI patterns guide how components are organized and interact to deliver an optimal user experience. By understanding and leveraging both, designers can create functional and user-friendly interfaces.
A design pattern solves common user interface challenges, focusing on specific interaction or design problems. It's a repeatable solution applied within different contexts. On the other hand, a design system is a comprehensive set of standards meant to guide the creation of products. It encompasses a library of patterns, components, guidelines, and best practices, ensuring consistency across an organization's designs. While design patterns offer singular solutions, design systems provide a holistic framework and a shared vocabulary for teams, streamlining the product development process. Design patterns can be seen as individual elements within the broader architecture of a design system.
UI pattern libraries are curated collections of user interface design solutions that address recurring challenges in product development. These libraries offer a repository of proven, practical design components that can be reused across different projects. Leveraging UI pattern libraries can help designers maintain consistency, speed up the design process, and ensure best practices in usability. As highlighted in this article from Interaction Design Foundation, there are numerous resources online where designers can access and get inspired by extensive UI pattern libraries, streamlining their design workflow and enhancing user experience.
Design system patterns are reusable, standardized solutions to commonly occurring design challenges. Within a design system, they ensure consistency across products by providing a shared language for both designers and developers. These patterns encompass visual elements, interactions, and even code snippets. By utilizing them, teams can improve design cohesiveness, speed up the design process, and ensure a smoother design handoff between designers and developers.
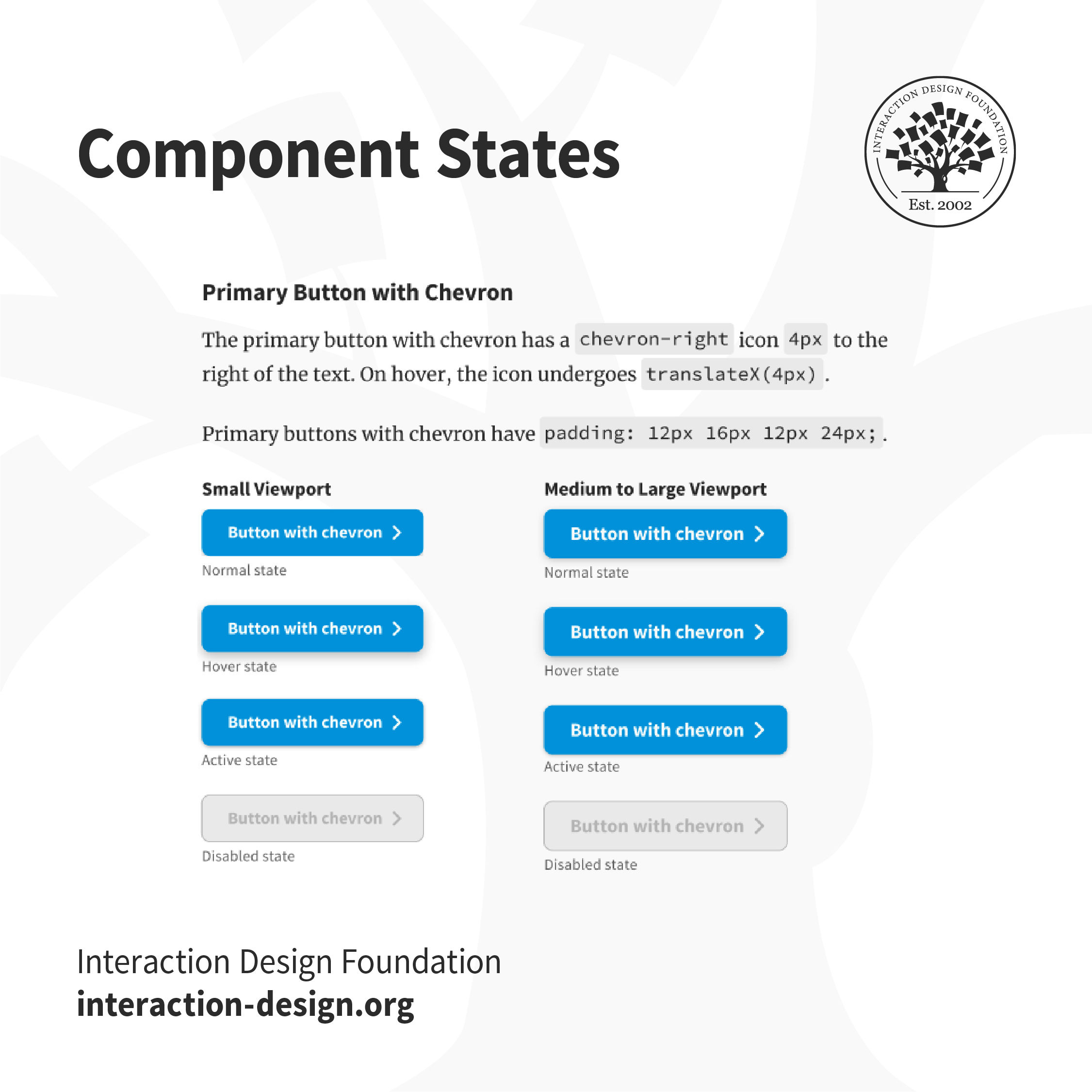
Incorporating design system patterns promotes efficiency and maintains brand integrity throughout different projects.
The 60-30-10 rule in UI design is a color distribution guideline for creating balanced and visually appealing interfaces.
This rule suggests using three primary colors:
Background color (60%): This dominates the design, typically a neutral tone.
Foreground (text) color (30%): Provides visual contrast, often a darker shade.
Accent color (10%): Draws attention to essential elements or actions.
Starting with these primary colors is essential, ensuring harmony and reducing visual clutter. For a cohesive look, the foreground color can sometimes hint at the accent color. This rule simplifies the design process and ensures a consistent, user-friendly experience.
For a comprehensive understanding of UI design patterns, consider these courses from Interaction Design Foundation:
UI Design Patterns for Successful Software : Delve into effective patterns, their psychology, and real-world applications in design.
Mobile UI Design Course : Learn mobile-specific design patterns and best practices for intuitive mobile interfaces.
If you're short on time, the UI Design Skills Masterclass provides a swift yet thorough overview of crucial UI design concepts. Enhance your design acumen and stay abreast of evolving UI trends with these invaluable resources.
Literature on User Interface (UI) Design Patterns
Here’s the entire UX literature on User Interface (UI) Design Patterns by the Interaction Design Foundation, collated in one place:
Learn more about User Interface (UI) Design Patterns
Take a deep dive into User Interface (UI) Design Patterns with our course Mobile UI Design .
In the “Build Your Portfolio” project, you’ll find a series of practical exercises that will give you first-hand experience of the methods we cover. You will build on your project in each lesson so once you have completed the course you will have a thorough case study for your portfolio.
Mobile User Experience Design: UI Design is built on evidence-based research and practice. Your expert facilitator is Frank Spillers, CEO of ExperienceDynamics.com, author, speaker and internationally respected Senior Usability practitioner.
All open-source articles on User Interface (UI) Design Patterns
10 great sites for ui design patterns.
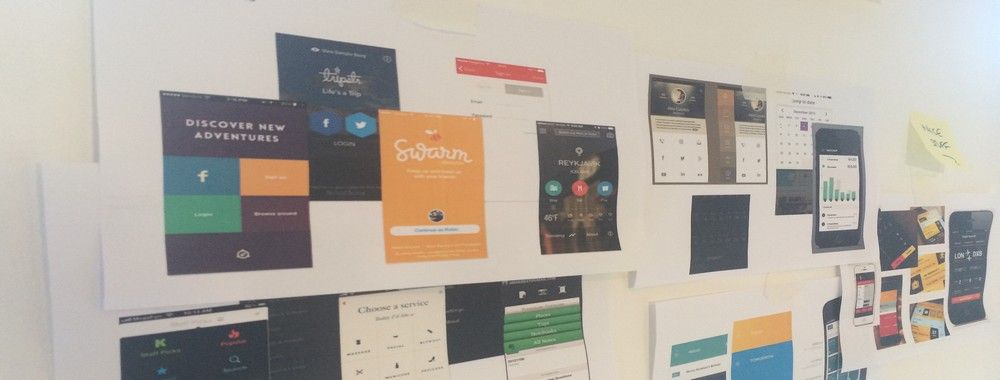
- 1.4k shares
Interaction Design Patterns
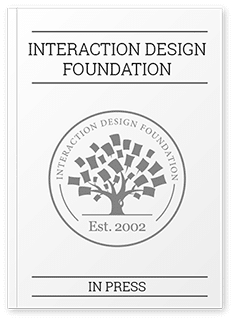
Split the Contents of a Website with the Pagination Design Pattern
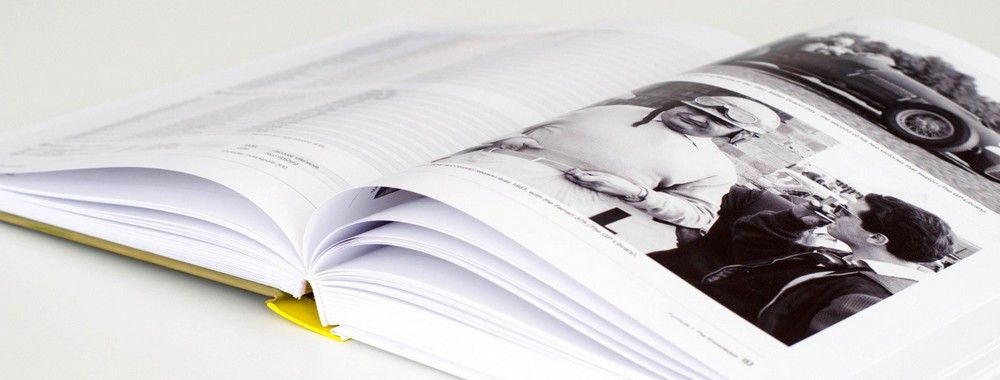
- 3 years ago
Implement Global Navigation to Improve Website Usability
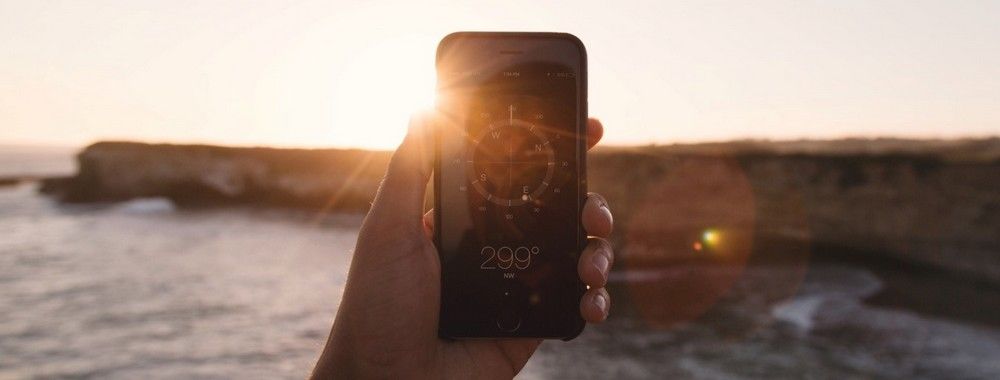
Make it Easy on the User: Designing for Discoverability within Mobile Apps
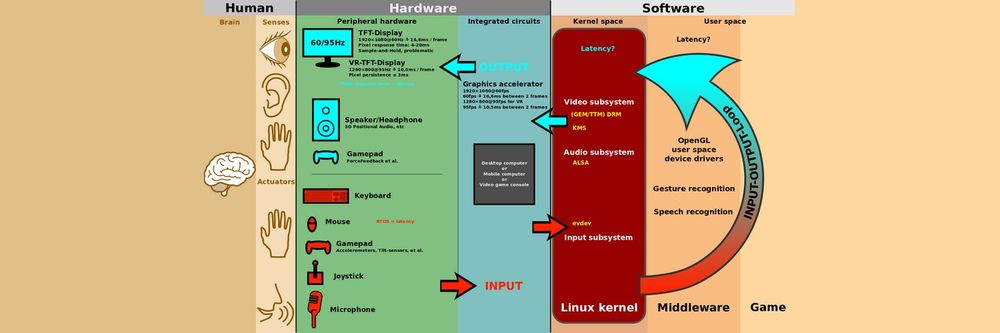
Display Achievements to Encourage Website Usage
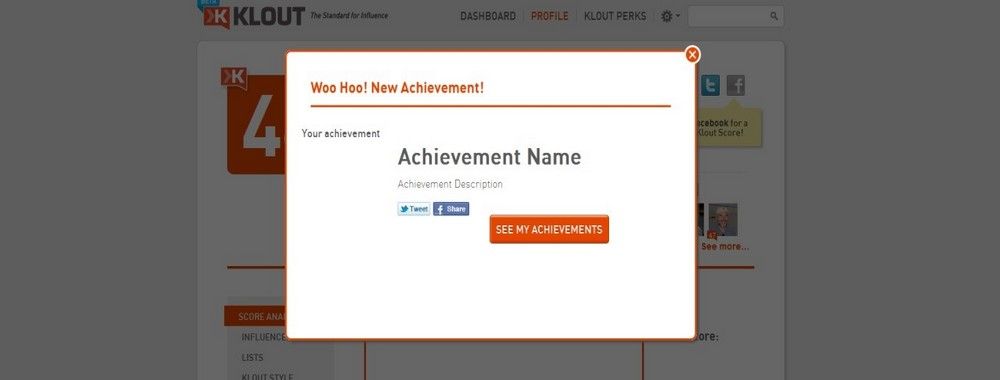
How to Apply Search Boxes to Increase Efficiency
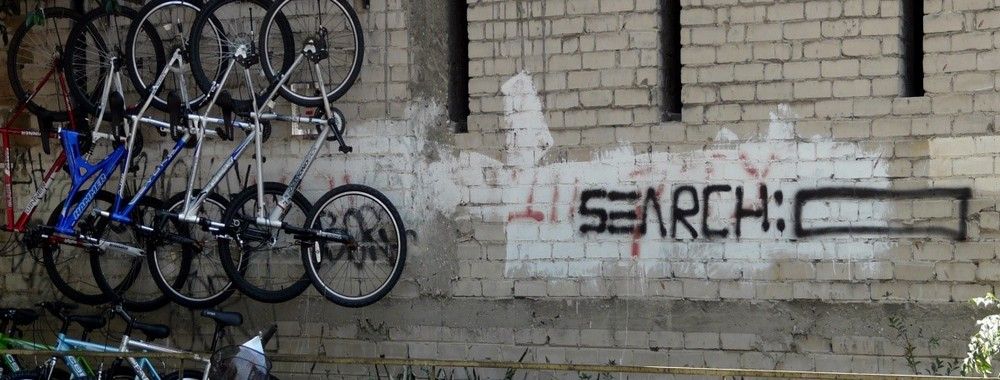
How to Implement Sitemap Footers to Keep Users Going
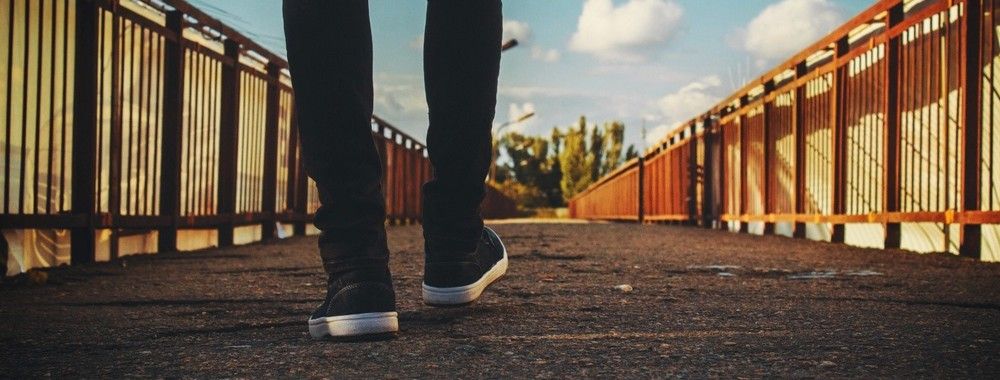
Embrace the Mental Models of Users by Implementing Tabs
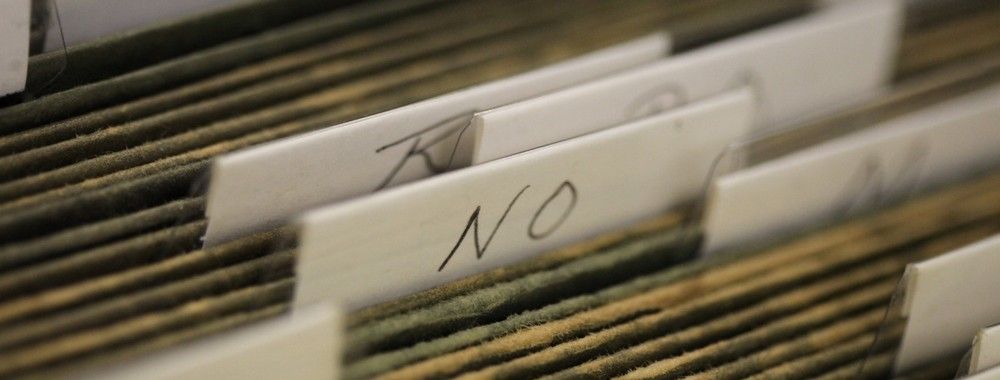
Giving Your Users Freedom with Editable Input Fields
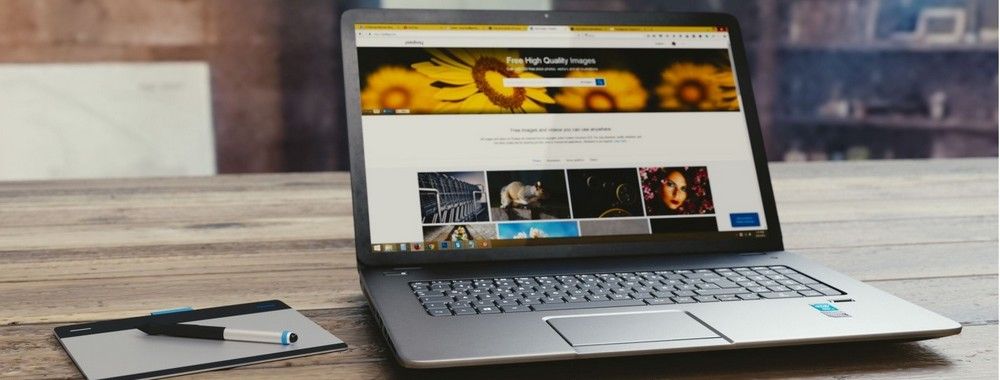
Design Patterns for Fluid Navigation – How to Use Inline Linking
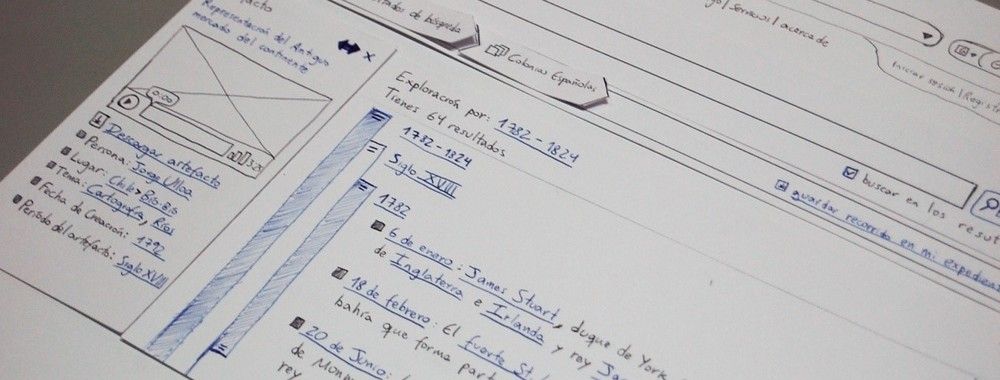
Best Practices and UI Design Patterns for Help in Mobile
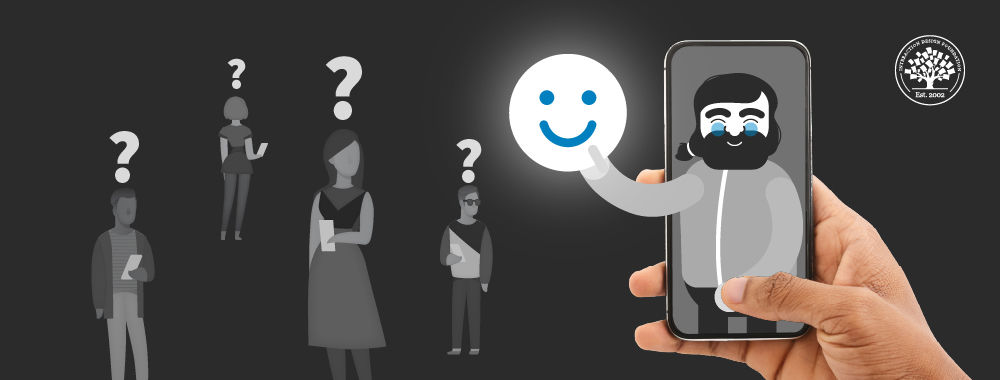
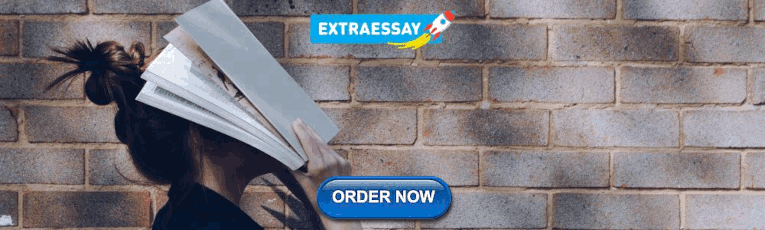
Using Design Patterns: Doing It Again Without The Hard Work
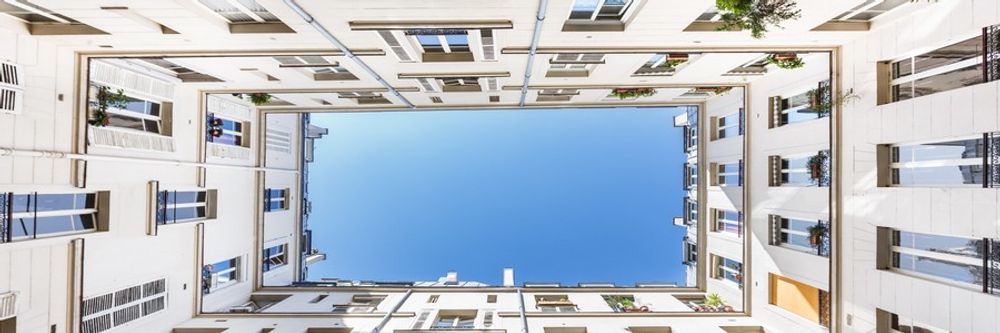
Use Color to Prevent Confusion and Help Your Users
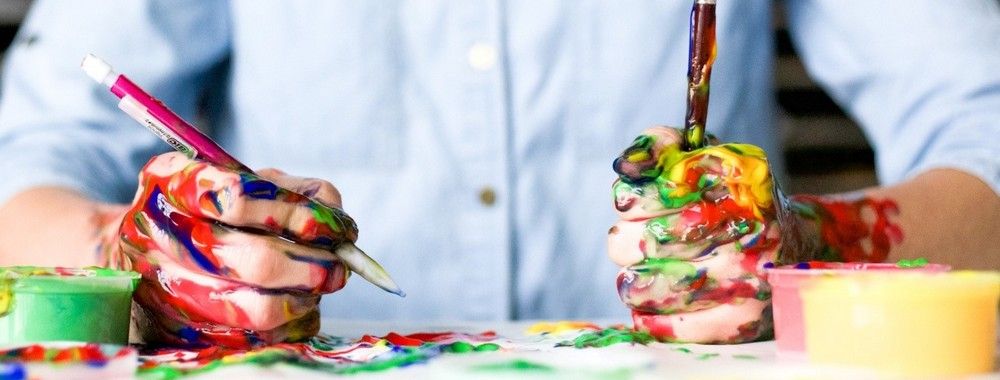
Search for Items with Scrolling Lists, Archive Lists or List Inlays
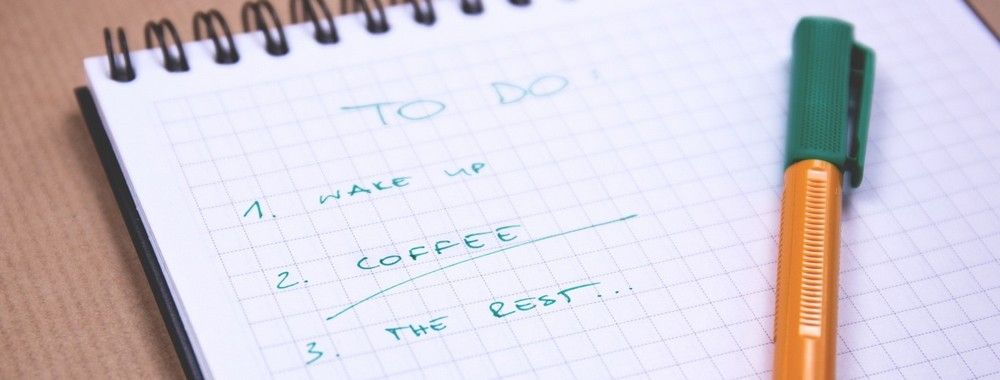
Two-Panel Selectors for Easy Access of Content
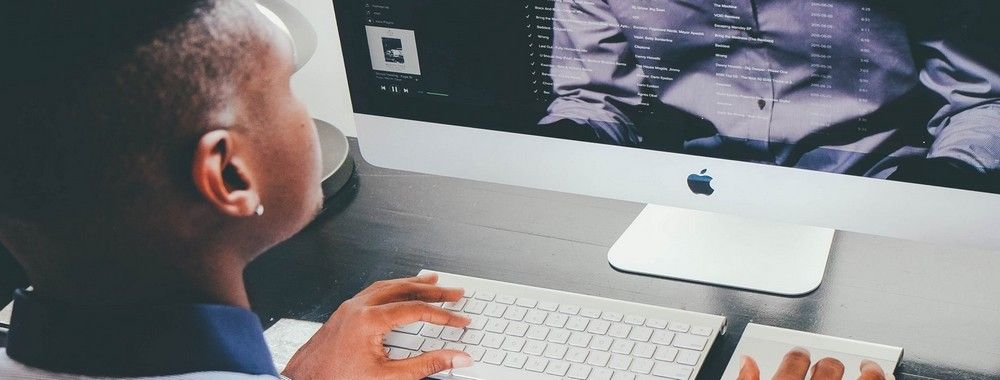
- 4 years ago
Display Contents the Classic Way with Dropdown Menus
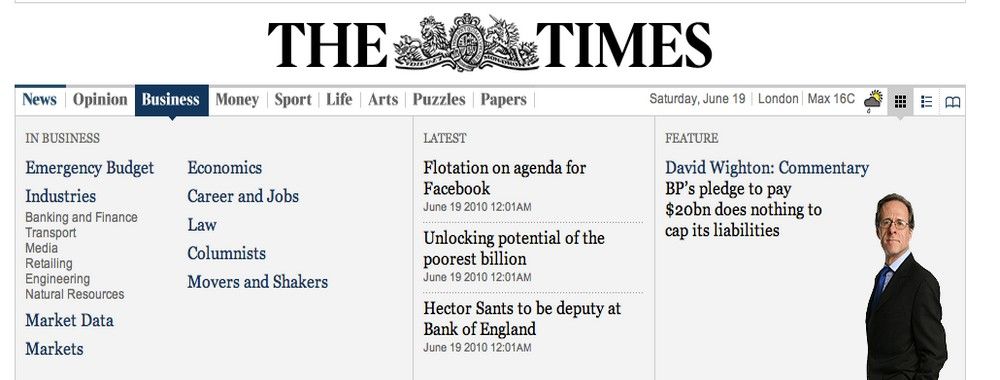
How to Implement a Forgiving Format to Accommodate Users’ Mistakes
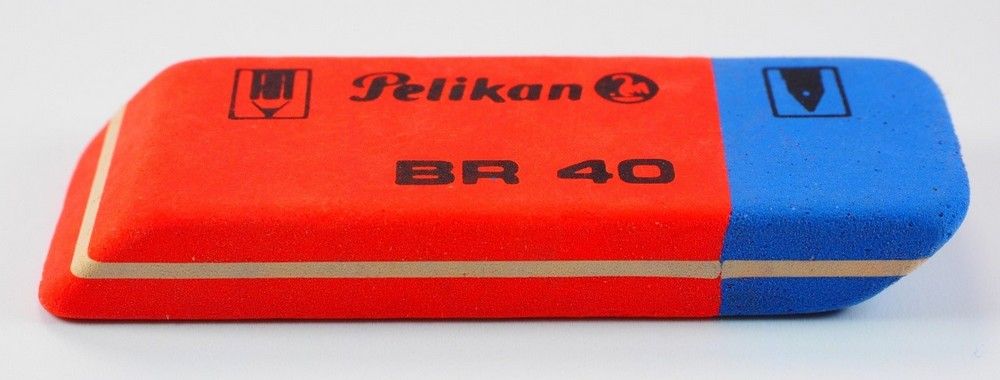
Speed up the User’s Process by Adding an Event Calendar
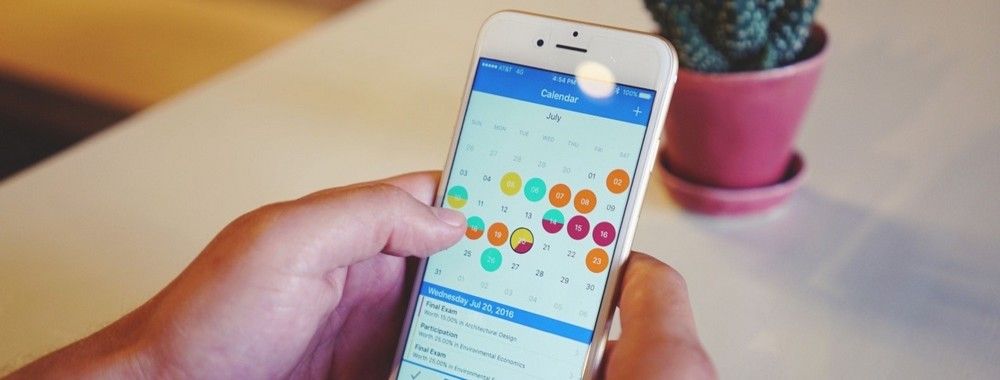
- 2 years ago
How to Get Users’ Agreement with the Opt-in/Opt-out Dance
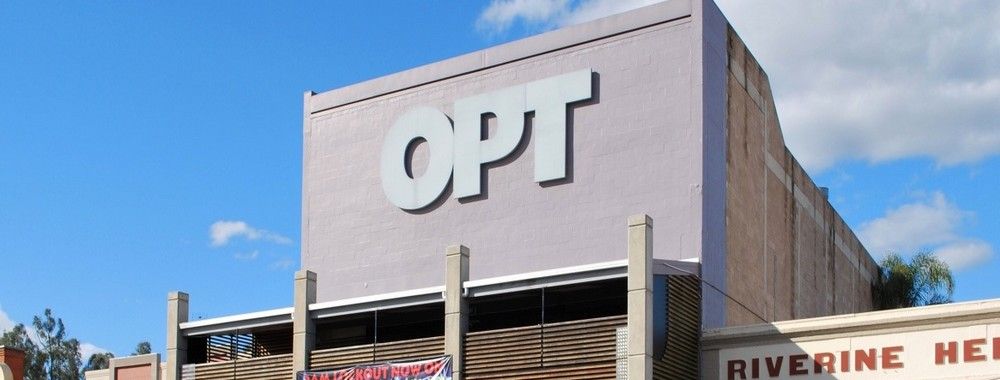
Open Access—Link to us!
We believe in Open Access and the democratization of knowledge . Unfortunately, world-class educational materials such as this page are normally hidden behind paywalls or in expensive textbooks.
If you want this to change , cite this page , link to us, or join us to help us democratize design knowledge !
Privacy Settings
Our digital services use necessary tracking technologies, including third-party cookies, for security, functionality, and to uphold user rights. Optional cookies offer enhanced features, and analytics.
Experience the full potential of our site that remembers your preferences and supports secure sign-in.
Governs the storage of data necessary for maintaining website security, user authentication, and fraud prevention mechanisms.
Enhanced Functionality
Saves your settings and preferences, like your location, for a more personalized experience.
Referral Program
We use cookies to enable our referral program, giving you and your friends discounts.
Error Reporting
We share user ID with Bugsnag and NewRelic to help us track errors and fix issues.
Optimize your experience by allowing us to monitor site usage. You’ll enjoy a smoother, more personalized journey without compromising your privacy.
Analytics Storage
Collects anonymous data on how you navigate and interact, helping us make informed improvements.
Differentiates real visitors from automated bots, ensuring accurate usage data and improving your website experience.
Lets us tailor your digital ads to match your interests, making them more relevant and useful to you.
Advertising Storage
Stores information for better-targeted advertising, enhancing your online ad experience.
Personalization Storage
Permits storing data to personalize content and ads across Google services based on user behavior, enhancing overall user experience.
Advertising Personalization
Allows for content and ad personalization across Google services based on user behavior. This consent enhances user experiences.
Enables personalizing ads based on user data and interactions, allowing for more relevant advertising experiences across Google services.
Receive more relevant advertisements by sharing your interests and behavior with our trusted advertising partners.
Enables better ad targeting and measurement on Meta platforms, making ads you see more relevant.
Allows for improved ad effectiveness and measurement through Meta’s Conversions API, ensuring privacy-compliant data sharing.
LinkedIn Insights
Tracks conversions, retargeting, and web analytics for LinkedIn ad campaigns, enhancing ad relevance and performance.
LinkedIn CAPI
Enhances LinkedIn advertising through server-side event tracking, offering more accurate measurement and personalization.
Google Ads Tag
Tracks ad performance and user engagement, helping deliver ads that are most useful to you.
Share Knowledge, Get Respect!
or copy link
Cite according to academic standards
Simply copy and paste the text below into your bibliographic reference list, onto your blog, or anywhere else. You can also just hyperlink to this page.
New to UX Design? We’re Giving You a Free ebook!

Download our free ebook The Basics of User Experience Design to learn about core concepts of UX design.
In 9 chapters, we’ll cover: conducting user interviews, design thinking, interaction design, mobile UX design, usability, UX research, and many more!
Written Assignment 4: Design Patterns
Due on November 29th at 11:59 PM .
This is an individual assignment. You are to complete this assignment on your own, although you may discuss the lab concepts with your classmates. Remember the Academic Integrity Policy : do not show your work to anyone outside of the course staff and do not look at anyone else’s work for this lab. If you need help, please post on the Piazza forum or contact the instructor . Note that no exception is made for your group members ; collaboration with your group members is a violation of the Academic Integrity Policy as much as collaboration with other members of the class. If you have any doubts about what is okay and what is not, it’s much safer to ask than to risk violating the Academic Integrity Policy .
For this assignment, write answers to the questions below and upload them to your personal GitHub repository for the assignment. You can find your repository at:
where <username> should be replaced by your Swarthmore username. You may add whatever materials to this repository you please, but your answers to each question must be clearly identified.
Design Patterns
This assignment requires you to demonstrate understand of design patterns. You’ll want to keep the course’s Design Patterns Guide handy. Only design patterns from that guide are necessary to complete this assignment.
Part I: Identifying Patterns
For each of the following code snippets, identify the design pattern best represented by that code. Briefly explain your reasoning.
- public class Employee { ... } public class Manager extends Employee { private List<Employee> directSubordinates; public Manager(List<Employee> directSubordinates) { this.directSubordinates = directSubordinates; } public List<Employee> getDirectSubordinates() { return this.directSubordinates; } }
- public class TreeWalker<T> { private List<TreeNode<T>> remainingNodes; public TreeWalker(TreeNode<T> root) { this.remainingNodes = new LinkedList<TreeNode<T>>(); this.remainingNodes.add(root); } public boolean finishedWalking() { return this.remainingNodes.isEmpty(); } public T getNextNodeData() { TreeNode<T> node = this.remainingNodes.remove(0); if (node.getLeft() != null) { this.remainingNodes.add(node.getLeft()); } if (node.getRight() != null) { this.remainingNodes.add(node.getRight()); } return node.getData(); } }
- public interface Combiner<T> { public T combine(T a, T b); } ... public T accumulate(List<T> items, T baseValue, Combiner<T> combiner) { T accumulator = baseValue; for (T t : items) { accumulator = combiner.combine(accumulator, t); } return accumulator; }
Part II: Correcting Problems
For each of the following code snippets, identify which design pattern should have been used to improve the quality of the code. Briefly explain why that design pattern would’ve led to better code.
- public void startWork() { worker.setJob(someJob); Thread finishCleanupThread = new Thread(new Runnable() { public void run() { while (worker.isWorking()) { } worker.cleanup(); } }); finishCleanupThread.start(); worker.start(); // non-blocking call which starts work }
- public class FitnessCustomer { private static enum Level { BRONZE, SILVER, GOLD } private Level level; public void setLevel(Level level) { this.level = level; } public double getFees() { switch (level) { case BRONZE: return CustomerConstants.BRONZE_FEES; case SILVER: return CustomerConstants.SILVER_FEES; case GOLD: return CustomerConstants.GOLD_FEES; } throw new IllegalStateException("How did I get here?"); } public boolean canAccessPool() { return (level == Level.SILVER || level == Level.GOLD); } public boolean hasOwnLocker() { return (level == Level.GOLD); } public double getEquipmentDiscount() { switch (level) { case BRONZE: return CustomerConstants.BRONZE_DISCOUNT; case SILVER: return CustomerConstants.SILVER_DISCOUNT; case GOLD: return CustomerConstants.GOLD_DISCOUNT; } throw new IllegalStateException("How did I get here?"); } ... }
Part III: API Examples
Examine the following members of the Java API. For each one, identify the design pattern that the member represents and briefly explain why.
The AbstractList.get(int) method.
The PushbackReader class.
Objects returned by the Collections.unmodifiableSet method.
Part IV: Design Scenarios
Consider each of the scenarios below and identify the design pattern which is most directly addresses the problem described. Briefly explain your reasoning.
You’ve developed a new implementation of the List interface and you’d like to test the behavior of your new data structure. You’ve written an algorithm to perform your speed tests, but the algorithm needs to make a great many instances of your list class. You want to test the performance of your list against the performance of ArrayList and LinkedList , but you don’t want to have to write your algorithm three times in order for it to be able to create the right kind of list to test.
You’ve completed a compiler for a new language! There are many parts to your compilation process: parsing, transformation, assembly code generation, and so forth. You’d like to allow other programmers to use an interface to compile their code without resorting to system calls or other command-line invocations – your compiler can just run in their processes – but you don’t want those users to have to know how to bring all of the steps of compilation together in order to use your compiler.
Computer Science Design Patterns
The term Design Patterns can confuse you at first, or it can seem like something incredibly difficult. In fact it is nothing more than convenient ways of identifying, labelling and coding general solutions to recurring design problems.
So design patterns are nothing more than commonly occurring patterns in design that are repeatable and generalist enough to be written down and named as software design constructs that all can commonly identify and apply. Note however that a design pattern refers to the logical structure of the code, what it does and how it addresses the issues, not on direct code portability across projects, but in the portability of the way design issues can be addressed.
Imagine yourself working in a project team and someone just wrote a class and is trying to explain to you that only one instance is allowed. Instead the person could simply say "The class I just wrote is a Singleton".
Patterns can be classified in different categories, of which the main categories are: Creational Patterns, Structural Patterns and Behavioral Patterns.
- Abstract Factory
- Chain of responsibility
- Factory method
- Interpreter
- Model–view–controller
- Template method
- Development stages
External links [ edit | edit source ]
- Java Design Patterns tutorial
- History of Design Patterns
- Book:Computer Science Design Patterns
- Shelf:Computer science
- Books with print version
- Books with PDF version
- Subject:Computer science
- Subject:Computer science/all books
- Subject:Computing/all books
- Subject:Books by subject/all books
- Book:Wikibooks Stacks/Books
- Shelf:Computer science/all books
- Department:Computing/all books
- Alphabetical/C
- Partly developed books
- Books by completion status/all books
Navigation menu
- CPA NEW SYLLABUS 2021
- KCSE MARKING SCHEMES
- ACTS OF PARLIAMENT
- UNIVERSITY RESOURCES PDF
- CPA Study Notes
- INTERNATIONAL STANDARDS IN AUDITING (ISA)
- Teach Yourself Computers
KNEC / TVET CDACC STUDY MATERIALS, REVISION KITS AND PAST PAPERS
Quality and Updated
KNEC, TVET CDACC NOTES AND PAST PAPERS
DIPLOMA MATERIALS
- KNEC NOTES – Click to download
- TVET CDACC PAST PAPERS – Click to download
CERTIFICATE MATERIALS
- KNEC CERTIFICATE NOTES – Click to download
Routing and Wavelength Assignment Algorithm for Mesh-based Multiple Multicasts in Optical Network-on-chip
- Published: 23 May 2024
Cite this article
- Fei Gao 1 ,
- Yawen Chen 2 &
- Boyong Gao 1
19 Accesses
Explore all metrics
Optical network-on-chip (ONoC) is an emerging architecture to realize high-performance and low power consumption for many-core processors. Multicast in ONoC is a vital communication pattern widely used in parallel computing and computational genomics. This paper proposes an efficient scheme for multiple multicast requests in ONoC. Firstly, we design an algorithm to construct two trees for two multicasts without duplicate edges in a mesh topology, and it only uses one wavelength. We propose a graph to represent the relationship between each multicast. Secondly, a group-based wavelength assignment scheme is designed to assign wavelengths for multiple multicasts. Finally, we used data analysis to extract helpful simulation results to form conclusions. The results indicate that our algorithm is superior to other tree-based schemes in communication latency and wavelength number usage.
This is a preview of subscription content, log in via an institution to check access.
Access this article
Price includes VAT (Russian Federation)
Instant access to the full article PDF.
Rent this article via DeepDyve
Institutional subscriptions
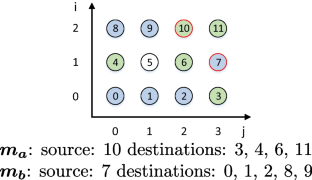
Similar content being viewed by others
A Survey of Multicast Communication in Optical Network-on-Chip (ONoC)
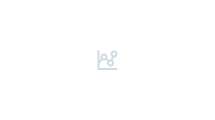
A survey of routing algorithm for mesh Network-on-Chip
A low-cost and latency bypass channel-based on-chip network.
Sugumaran, S., Lakshmi, D.N., Choudhary S.: An Overview of FTTH for optical network. Advances in Smart Communication and Imaging Systems pp. 41–51 (2021)
Barzaq, A., Ashour, I., Shbair, W., et al.: 2 Tbit/s based coherent wavelength division multiplexing passive optical network for 5G transport. Optoelectron. Lett. 17 (5), 308–312 (2021)
Article Google Scholar
Asadinia, S., Mehrabi, M., Yaghoubi, E.: Surix: Non-blocking and low insertion loss micro-ring resonator-based optical router for photonic network on chip. J. Supercomput. 77 (5), 4438–4460 (2021)
Mishra, P., Charles S.: The future of secure and trustworthy network-on-chip architectures. Network-on-Chip Security and Privacy, p. 483 (2021)
Gowd, R., Thenappan, S., GiriPrasad, M.N.: A traffic delay and bandwidth based multipath scheduling approach for optimal routing in underwater optical network. Wirel. Pers. Commun. 116 (2), 1009–1023 (2021)
Yadav, D.S.: RDRSA: A reactive defragmentation based on rerouting and spectrum assignment (RDRSA) for spectrum convertible elastic optical network. Opt. Commun. 127144 (2021)
Abdollahi, M., Mohammadi, S.: Vulnerability assessment of fault-tolerant optical network-on-chips. J. Parallel Distrib. Comput. 145 , 140–159 (2020)
Article MATH Google Scholar
Yu, C., Yang, X., Yang, L.X., et al.: Routing and wavelength assignment for 3-ary n-cube in array-based optical network. Inf. Process. Lett. 112 (6), 252–256 (2012)
Article MathSciNet MATH Google Scholar
Preston, K., Sherwood-Droz, N., Levy, J.S., et al.: Performance guidelines for WDM interconnects based on silicon microring resonators. 2011-Laser Science to Photonic Applications, pp. 1-2. IEEE (2011)
Chaudhari, B.S., Patil, S.S.: Optimized designs of low loss non-blocking optical router for ONoC applications. Int. J. Inf. Technol. 12 (1), 91–96 (2020)
MATH Google Scholar
Uçtu, G., Alkan, M., Doğru, İ.A., et al.: A suggested testbed to evaluate multicast network and threat prevention performance of next generation firewalls. Futur. Gener. Comput. Syst. (2021)
Abadal, S., Mestres, A., Martinez, R., et al.: Multicast on-chip traffic analysis targeting manycore NoC design, Euromicro International Conference on Parallel. Distributed, and Network-Based Processing, pp. 370-378. IEEE (2015)
Bogdan, P., Majumder, T., Ramanathan, A., et al.: NoC architectures as enablers of biological discovery for personalized and precision medicine. Proceedings of the 9th International Symposium on Networks-on-Chip, pp. 1–11. (2015)
Nasiri, F., Sarbazi-Azad, H., Khademzadeh, A.: Reconfigurable multicast routing for networks on chip. Microprocess. Microsyst. 42 , 180–189 (2016)
Ramya, S., Indumathi, T.S.A.: Comparative study on the routing and wavelength assignment in WDM. Computing and Communication Technologies (CONECCT), pp. 1-5. IEEE (2020)
Hu, W., Lu, Z., Jantsch, A., et al.: Power-efficient tree-based multicast support for networks-on-chip. Asia and South Pacific Design Automation Conference, pp. 363-368. IEEE, (2011)
Yang, W., Chen, Y., Huang, Z., et al.: RWADMM: routing and wavelength assignment for distribution-based multiple multicasts in ONoC. In: IEEE International Conference ISPA/IUCC pp. 550-557. (2017)
Yang, W., Chen, Y., Huang, Z., et al.: Path-based routing and wavelength assignment for multiple multicasts in optical network-on-chip. International Conference on High Performance Computing and Communications pp. 1155-1162. (2019)
Choudhury, P.D., Reddy, P.V.R., Chatterjee, B.C., et al.: Performance of routing and spectrum allocation approaches for multicast traffic in elastic optical networks. Opt. Fiber Technol. 58 , 102247 (2020)
Gao, F., Yu, C., Gao, B., et al.: Dynamic multiple multicasts routing and wavelength assignment for realizing modified artificial fish model in mesh-based ONoC. Opt. Switch. Netw. 50 , 100744 (2023)
Maurya, R.K., Thangaraj, J., Priye, V.: Dynamic routing and wavelength assignment using cost based heuristics in WDM optical networks. Wirel. Pers. Commun. 115 (2), 971–992 (2020)
Hadfield, R.H.: Single-photon detectors for optical quantum information applications. Nat. Photonics 3 (12), 696–705 (2009)
Jerger, N.E., Peh, L.S., Lipasti, M.: Virtual circuit tree multicasting: A case for on-chip hardware multicast support. International Symposium on Computer Architecture, pp. 229-240. . IEEE, (2008)
Download references
Acknowledgements
We thank the editors for their rigorous and efficient work, and we also thank the referees for their helpful comments.
This work is supported by the National Key Research and Development Plan “NQI Integrated Services Common Technologies Research” special project (No. 2021YFF0600100) and the Natural Science Foundation of Zhejiang Province (No. LQ18F020004).
Author information
Authors and affiliations.
Key Laboratory of Electromagnetic Wave Information Technology and Metrology of Zhejiang Province, College of Information Engineering, China Jiliang University, Hangzhou, 310018, China
Fei Gao, Cui Yu & Boyong Gao
Department of Computer Science, University of Otago, Dunedin, 9016, New Zealand
You can also search for this author in PubMed Google Scholar
Contributions
Fei Gao and Cui Yu wrote the main manuscript text. All authors reviewed the manuscript.
Corresponding author
Correspondence to Cui Yu .
Ethics declarations
Conflicts of interest.
The authors declare that they have no conflicts of interest to report regarding the present study.
Competing interests
The authors declare no competing interests.
Additional information
Publisher's note.
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Rights and permissions
Springer Nature or its licensor (e.g. a society or other partner) holds exclusive rights to this article under a publishing agreement with the author(s) or other rightsholder(s); author self-archiving of the accepted manuscript version of this article is solely governed by the terms of such publishing agreement and applicable law.
Reprints and permissions
About this article
Gao, F., Yu, C., Chen, Y. et al. Routing and Wavelength Assignment Algorithm for Mesh-based Multiple Multicasts in Optical Network-on-chip. Theory Comput Syst (2024). https://doi.org/10.1007/s00224-024-10177-9
Download citation
Accepted : 20 April 2024
Published : 23 May 2024
DOI : https://doi.org/10.1007/s00224-024-10177-9
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Mesh topology
- Optical network-on-chip
- Routing algorithm
- Find a journal
- Publish with us
- Track your research
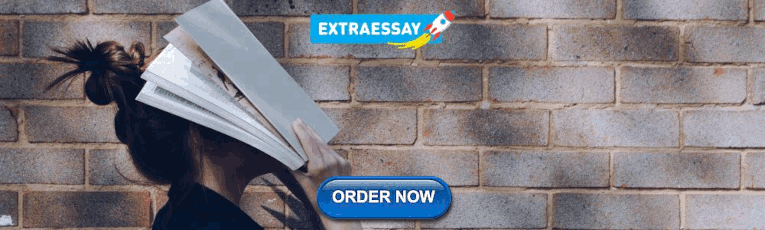
COMMENTS
Introduction to Design Patterns: Creational & Structural Patterns. Module 1 • 4 hours to complete. Design patterns help to solve common design issues in object-oriented software. You will learn what they are and how they can be applied. In this module you will learn the creational and structural design patterns.
Behavioral design patterns are concerned with algorithms and the assignment of responsibilities between objects. Lets you pass requests along a chain of handlers. Upon receiving a request, each handler decides either to process the request or to pass it to the next handler in the chain. Turns a request into a stand-alone object that contains ...
Behavioral design patterns are concerned with algorithms and the assignment of responsibilities between objects. Design patterns are solutions to commonly occurring problems in software design.
GRASP, which stands for General Responsibility Assignment Software Patterns (or Principles), is a collection of nine best practices that assist in assigning responsibilities to classes and objects.
A composite design pattern is meant to achieve two goals: To compose nested structures of objects, and; To deal with the classes for theses objects uniformly. The following basic design. Think of composite design patterns as trees: The composite design pattern is used to address two issues.
Check out our ebook on design patterns and principles. It's available in PDF/ePUB/MOBI formats and includes the archive with code examples in Java, C#, C++, PHP, Python, Ruby, Go, Swift, & TypeScript. Learn more about the book. Design Patterns are typical solutions to commonly occurring problems in software design.
General Responsibility Assignment Software Patterns (or Principles), abbreviated GRASP, is a set of "nine fundamental principles in object design and responsibility assignment": 6 first published by Craig Larman in his 1997 [citation needed] book Applying UML and Patterns.. The different patterns and principles used in GRASP are controller, creator, indirection, information expert, low ...
Design patterns allow you to develop flexible, scalable, and maintainable object-oriented software using best practices and design principles. All the design patterns are tried and tested solutions for various recurring problems. Even if you don't use them right away, knowing about them will give you a better understanding of how to solve ...
Design Patterns. Composite Pattern We'll consider the example of a file system. Need to represent directories and files Directories can contain other files or directories Files are "leaf" nodes, probably contain pointers to data. This example will also use the factory pattern. Andre Kessler. 6.S096 Lecture 6 - Design Patterns 13 /17
The most universal and high-level patterns are architectural patterns. Developers can implement these patterns in virtually any language. Unlike other patterns, they can be used to design the architecture of an entire application. In addition, all patterns can be categorized by their intent, or purpose.
CSE 403, Spring 2008, Alverson. Desiggpn patterns. A design patterndesign patternis a timeis a time-tested solution to atested solution to a common software problem. yPatterns enable a common design vocabulary, improving communication, easing implementation andd t tid documentation.
Welcome to the Practical Python Design Patterns repository! Here, you'll find practical examples and solutions from the book "Practical Python Design Patterns: Pythonic Solutions to Common Problems" by Wessel Badenhorst, published in 2017. This repository serves as a valuable resource to explore and understand various design patterns in Python.
By rotating the design, these shapes look less like Ys and give the pattern a different effect. 5. Black-and-White Lines. A simple black-and-white geometric design by Peter Bone. By repeating and mirroring a simple square block containing thick black-and-white lines, a mesmerizing effect is created. 6.
According to this Design Patterns Cheat Sheet, choose creational design patterns when object creation is complex, involves multiple steps, or requires specific initialization. They're useful for promoting reusability, encapsulating creation logic, and decoupling client code from classes it instantiates. Creational patterns enhance flexibility ...
Note:For this assignment you are allowed, even encouraged, to work in groups of two (i.e. two students work together and turn in one set of answers). (2 points) Exercise #6.18 at the end of Chapter 6 (p. 259) (2 points) As noted on p. 252 of the textbook, the Rectangle2D and Rectangle2D.Double classes use the TEMPLATE pattern.
These patterns can be categorized into three main groups: 1. Creational Design Patterns. Singleton Pattern. The Singleton method or Singleton Design pattern is one of the simplest design patterns. It ensures a class only has one instance, and provides a global point of access to it. Factory Method Pattern.
Assignment 5: Design Patterns Spring 2015 Exercise 1 Examine the listed Java APIs and identify some of the design patterns present. For each pattern found describe why the API follows it. Each bullet-point group corresponds to one pattern to be identi ed. Note that most of the patterns have not been covered on lectures.
The Composite Pattern is a structural design pattern in object-oriented programming that allows you to compose objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly. In other words, you can work with individual objects and their collections in a ...
Sep 24, 2017. --. 1. Design Patterns are incredibly important tools for software developers. Above solving common problems, they give people a standard vernacular to communicate with, and offer ...
CS446 Assignment 4: Design Patterns Assignment 4a: Give a summary description of six design patterns that you choose from the following design patterns: Adapter, Bridge, Builder, Composite, Decorator, Flyweight, Interpreter, Iterator, Mediator, Memento, Observer, Prototype, Proxy, Template Method.In your summaries say: what kind of problem(s) you can solve with that pattern and when you use it ...
Assignment #5 Design Patterns CS 3354.251 and 252 Spring 2017 Instructor: Jill Seaman Due: before class Wednesday, 4/12/2017 (upload electronic copy by 10:30am, bring paper copy of the UML diagrams to class). We want to implement a Java class to represent a student and their scores from a class
UI design patterns serve as design blueprints that allow designers to choose the best and most commonly used interfaces for the user's specific context. Each pattern typically contains: A user's usability -related problem. The context / situation where that problem happens.
Software Engineering Written Assignment 4: Design Patterns. Due on November 29th at 11:59 PM.. This is an individual assignment. You are to complete this assignment on your own, although you may discuss the lab concepts with your classmates. Remember the Academic Integrity Policy: do not show your work to anyone outside of the course staff and do not look at anyone else's work for this lab.
Computer Science Design Patterns. The term Design Patterns can confuse you at first, or it can seem like something incredibly difficult. In fact it is nothing more than convenient ways of identifying, labelling and coding general solutions to recurring design problems. So design patterns are nothing more than commonly occurring patterns in ...
In software engineering, the composite pattern is a partitioning design pattern.The composite pattern describes a group of objects that are treated the same way as a single instance of the same type of object. The intent of a composite is to "compose" objects into tree structures to represent part-whole hierarchies.
These are Design Pattern Assignments. Course. Design Patterns (CSE354) 11 Documents. Students shared 11 documents in this course. University COMSATS University Islamabad. Academic year: 2018/2019. Uploaded by: Waseem Azad. COMSATS University Islamabad. 0 followers. 8 Uploads 83 upvotes. Follow. Recommended for you. 3.
DIPLOMA MATERIALS. KNEC NOTES - Click to download. TVET CDACC PAST PAPERS - Click to download. CERTIFICATE MATERIALS. KNEC CERTIFICATE NOTES - Click to download. (Visited 109,430 times, 35 visits today)
Optical network-on-chip (ONoC) is an emerging architecture to realize high-performance and low power consumption for many-core processors. Multicast in ONoC is a vital communication pattern widely used in parallel computing and computational genomics. This paper proposes an efficient scheme for multiple multicast requests in ONoC. Firstly, we design an algorithm to construct two trees for two ...