- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- C Exercises - Practice Questions with Solutions for C Programming
- C Programming Interview Questions (2024)
- C | Storage Classes and Type Qualifiers | Question 7
- C | Storage Classes and Type Qualifiers | Question 3
- C | Storage Classes and Type Qualifiers | Question 8
- Top 25 C Projects with Source Code in 2023
- C | Storage Classes and Type Qualifiers | Question 19
- C++ Exercises - C++ Practice Set with Solutions
- Top | MCQs on Dynamic Programming with Answers | Question 19
- C++ Programming Multiple Choice Questions
- Data Structures & C Programming - GATE CSE Previous Year Questions
- Class 8 NCERT Solutions - Chapter 16 Playing with Numbers - Exercise 16.1
- QA - Placement Quizzes | SP Contest 2 | Question 9
- Monotype Solutions Interview Experience
- QA - Placement Quizzes | SP Contest 2 | Question 3
- GATE | Quiz for Sudo GATE 2021 | Question 18
- QA - Placement Quizzes | SP Contest 2 | Question 8
- Mentor Graphics Interview Experience| Set 6 (On-Campus for Freshers)
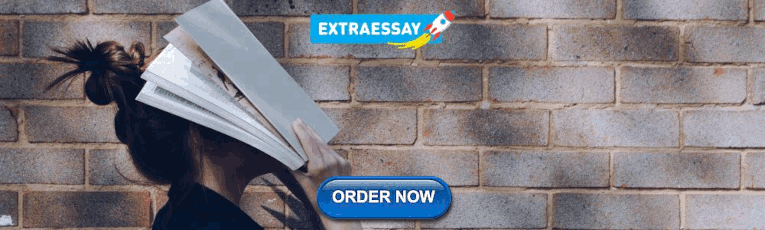
C Exercises – Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
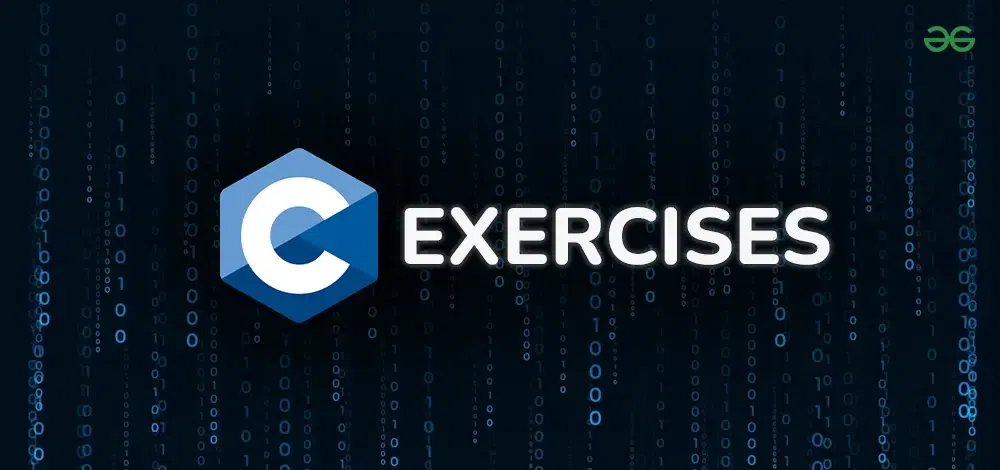
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
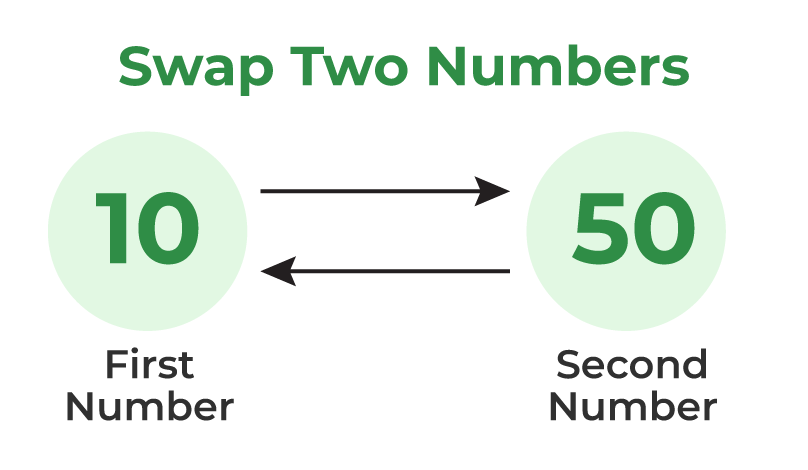
Swap two numbers
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
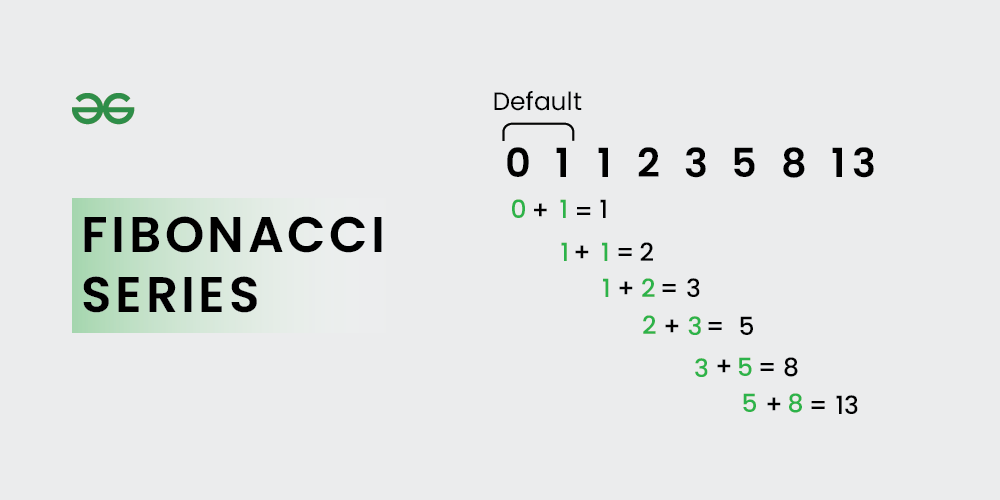
Fibonacci Series
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
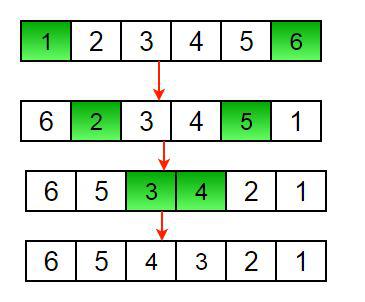
Reverse an array
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array ‘.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
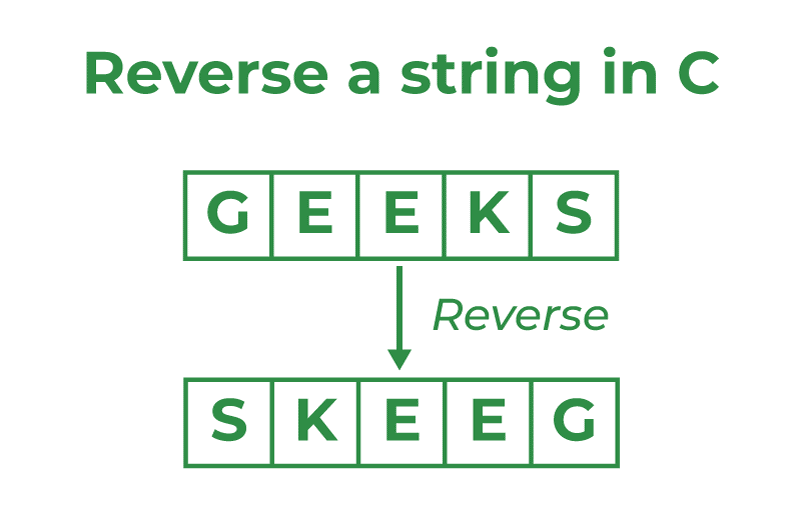
reverse a string
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
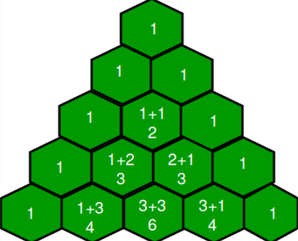
Pascal’s Triangle
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
"Hello World!" in C Easy C (Basic) Max Score: 5 Success Rate: 85.71%
Playing with characters easy c (basic) max score: 5 success rate: 84.34%, sum and difference of two numbers easy c (basic) max score: 5 success rate: 94.61%, functions in c easy c (basic) max score: 10 success rate: 95.99%, pointers in c easy c (basic) max score: 10 success rate: 96.57%, conditional statements in c easy c (basic) max score: 10 success rate: 96.94%, for loop in c easy c (basic) max score: 10 success rate: 93.70%, sum of digits of a five digit number easy c (basic) max score: 15 success rate: 98.66%, bitwise operators easy c (basic) max score: 15 success rate: 94.91%, printing pattern using loops medium c (basic) max score: 30 success rate: 95.91%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website

Problem Solving Through Programming in C
In this lesson, we are going to learn Problem Solving Through Programming in C. This is the first lesson while we start learning the C language.
So let’s start learning the C language.
Table of Contents
Introduction to Problem Solving Through Programming in C
Regardless of the area of the study, computer science is all about solving problems with computers. The problem that we want to solve can come from any real-world problem or perhaps even from the abstract world. We need to have a standard systematic approach to problem solving through programming in c.
computer programmers are problem solvers. In order to solve a problem on a computer, we must know how to represent the information describing the problem and determine the steps to transform the information from one representation into another.
In this chapter, we will learn problem-solving and steps in problem-solving, basic tools for designing solution as an algorithm, flowchart , pseudo code etc.
A computer is a very powerful and versatile machine capable of performing a multitude of different tasks, yet it has no intelligence or thinking power.
The Computer performs many tasks exactly in the same manner as it is told to do. This places responsibility on the user to instruct the computer in a correct and precise manner so that the machine is able to perform the required job in a proper way. A wrong or ambiguous instruction may sometimes prove dangerous.
The computer cannot solve the problem on its own, one has to provide step by step solutions of the problem to the computer. In fact, the task of problem-solving is not that of the computer.
It is the programmer who has to write down the solution to the problem in terms of simple operations which the computer can understand and execute.
Problem-solving is a sequential process of analyzing information related to a given situation and generating appropriate response options.
In order to solve a problem with the computer, one has to pass through certain stages or steps. They are as follows:
Steps to Solve a Problem With the Computer
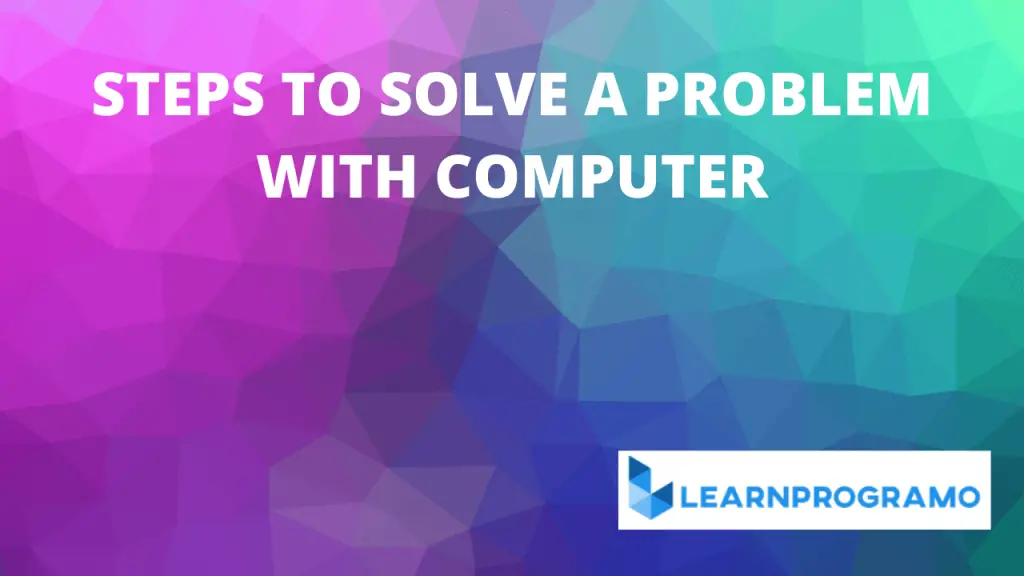
Step 1: Understanding the Problem:
Here we try to understand the problem to be solved in totally. Before with the next stage or step, we should be absolutely sure about the objectives of the given problem.
Step 2: Analyzing the Problem:
After understanding thoroughly the problem to be solved, we look at different ways of solving the problem and evaluate each of these methods.
The idea here is to search for an appropriate solution to the problem under consideration. The end result of this stage is a broad overview of the sequence of operations that are to be carried out to solve the given problem.
Step 3: Developing the solution:
Here, the overview of the sequence of operations that was the result of the analysis stage is expanded to form a detailed step by step solution to the problem under consideration.
Step 4: Coding and Implementation:
The last stage of problem-solving is the conversion of the detailed sequence of operations into a language that the computer can understand. Here, each step is converted to its equivalent instruction or instructions in the computer language that has been chosen for the implantation.
The vehicle for the computer solution to a problem is a set of explicit and unambiguous instructions expressed in a programming language. This set of instruction is called a program with problem solving through programming in C .
A program may also be thought of as an algorithm expressed in a programming language. an algorithm, therefore, corresponds to a solution to a problem that is independent of any programming language .
To obtain the computer solution to a problem once we have the program we usually have to supply the program with input or data. The program then takes this input and manipulates it according to its instructions. Eventually produces an output which represents the computer solution to the problem.
The problem solving is a skill and there are no universal approaches one can take to solving problems. Basically one must explore possible avenues to a solution one by one until she/he comes across the right path to a solution.
In general, as one gains experience in solving problems, one develops one’s own techniques and strategies, though they are often intangible. Problem-solving skills are recognized as an integral component of computer programming.
Note: Practice C Programs for problem solving through programming in C.
Problem Solving Steps
Problem-solving is a creative process which defines systematization and mechanization. There are a number of steps that can be taken to raise the level of one’s performance in problem-solving.
A problem-solving technique follows certain steps in finding the solution to a problem. Let us look into the steps one by one:
1. Problem Definition Phase:
The success in solving any problem is possible only after the problem has been fully understood. That is, we cannot hope to solve a problem, which we do not understand. So, the problem understanding is the first step towards the solution of the problem.
In the problem definition phase, we must emphasize what must be done rather than how is it to be done. That is, we try to extract the precisely defined set of tasks from the problem statement.
Inexperienced problem solvers too often gallop ahead with the task of the problem – solving only to find that they are either solving the wrong problem or solving the wrong problem or solving just one particular problem.
2. Getting Started on a Problem:
There are many ways of solving a problem and there may be several solutions. So, it is difficult to recognize immediately which path could be more productive. Problem solving through programming in C.
Sometimes you do not have any idea where to begin solving a problem, even if the problem has been defined. Such block sometimes occurs because you are overly concerned with the details of the implementation even before you have completely understood or worked out a solution.
The best advice is not to get concerned with the details. Those can come later when the intricacies of the problem have been understood.
3. Use of Specific Examples:
To get started on a problem, we can make use of heuristics i.e the rule of thumb. This approach will allow us to start on the problem by picking a specific problem we wish to solve and try to work out the mechanism that will allow solving this particular problem.
It is usually much easier to work out the details of a solution to a specific problem because the relationship between the mechanism and the problem is more clearly defined.
This approach of focusing on a particular problem can give us the foothold we need for making a start on the solution to the general problem.
4. Similarities Among Problems:
One way to make a start is by considering a specific example. Another approach is to bring the experience to bear on the current problems. So, it is important to see if there are any similarities between the current problem and the past problems which we have solved.
The more experience one has the more tools and techniques one can bring to bear in tackling the given problem. But sometimes, it blocks us from discovering a desirable or better solution to the problem.
A skill that is important to try to develop in problem-solving is the ability to view a problem from a variety of angles.
One must be able to metaphorically turn a problem upside down, inside out, sideways, backwards, forwards and so on. Once one has developed this skill it should be possible to get started on any problem.
5. Working Backwards from the Solution:
In some cases, we can assume that we already have the solution to the problem and then try to work backwards to the starting point. Even a guess at the solution to the problem may be enough to give us a foothold to start on the problem.
We can systematize the investigations and avoid duplicate efforts by writing down the various steps taken and explorations made.
Another practice that helps to develop the problem-solving skills, once we have solved a problem, to consciously reflect back on the way we went about discovering the solution.
General Problem Solving Strategies:
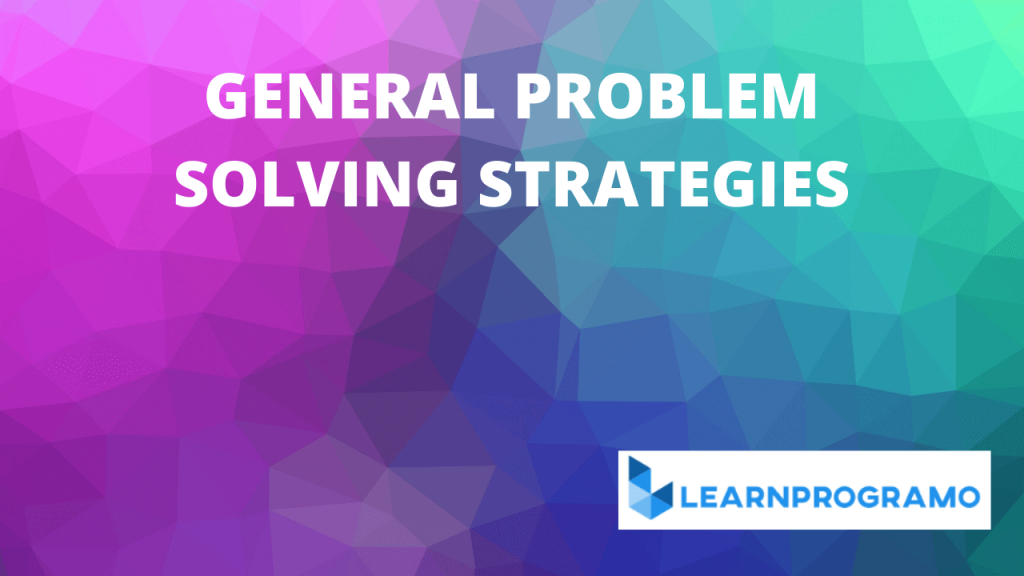
There are a number of general and powerful computational strategies that are repeatedly used in various guises in computer science.
Often it is possible to phrase a problem in terms of one of these strategies and achieve considerable gains in computational efficiency.
1. Divide and Conquer:
The most widely known and used strategy, where the basic idea is to break down the original problem into two or more sub-problems, which is presumably easier or more efficient to solve.
The Splitting can be carried on further so that eventually we have many sub-problems, so small that further splitting is no necessary to solve them. We shall see many examples of this strategy and discuss the gain in efficiency due to its application.
2. Binary Doubling:
This is the reverse of the divide and conquers strategy i.e build-up the solution for a larger problem from solutions and smaller sub-problems.
3. Dynamic Programming:
Another general strategy for problem-solving which is useful when we can build-up the solution as a sequence of the intermediate steps. Problem Solving through programming in C.
The travelling salesman problem falls into this category. The idea here is that a good or optimal solution to a problem can be built-up from good or optimal solutions of the sub-problems.
4. General Search, Back Tracking and Branch-and-Bound:
All of these are variants of the basic dynamic programming strategy but are equally important.
Share This Story, Choose Your Platform!
Related posts.
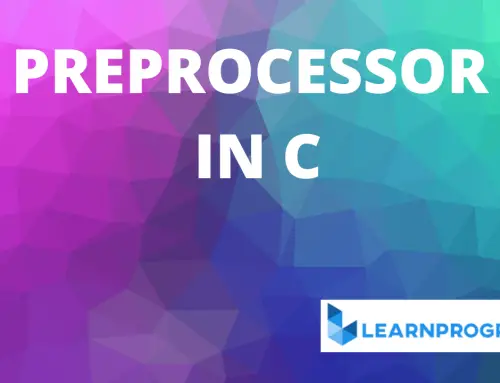
What is Preprocessor in C
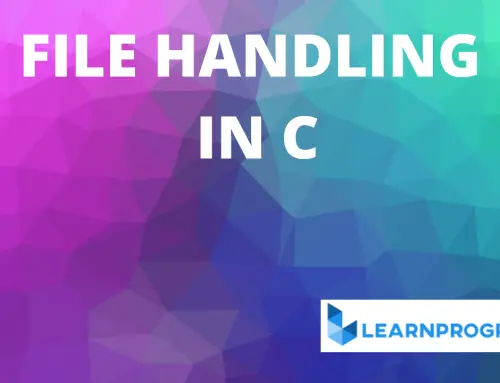
What is File Handling in C
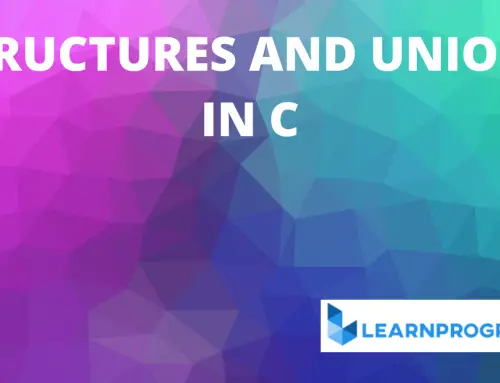
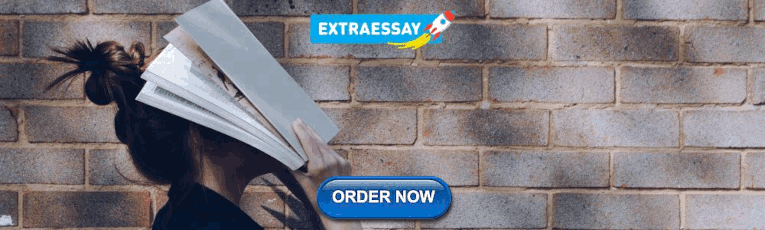
Structures and Unions in C

- Top Courses
- Online Degrees
- Find your New Career
- Join for Free

C for Everyone: Programming Fundamentals
This course is part of Coding for Everyone: C and C++ Specialization
Taught in English
Some content may not be translated

Instructor: Ira Pohl
Financial aid available
286,425 already enrolled

(6,326 reviews)
What you'll learn
Write a simple program.
Compile, debug, and run a program.
Apply concepts related to arrays & pointers, functions & storage classes, logic operators & various question types, lexical elements & data types.
Details to know

Add to your LinkedIn profile
See how employees at top companies are mastering in-demand skills
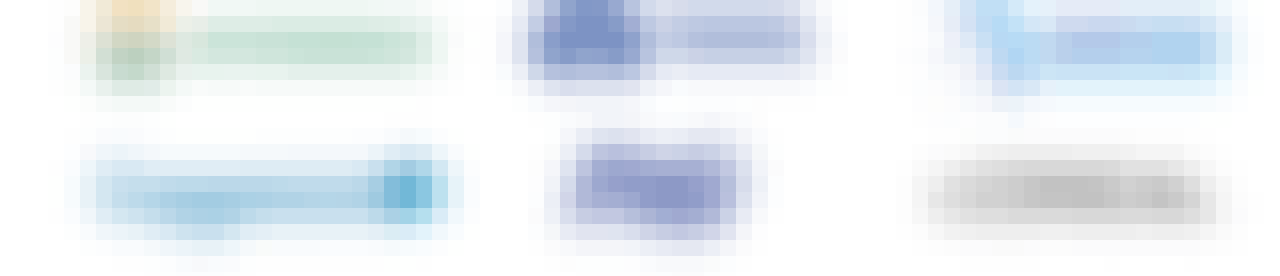
Build your subject-matter expertise
- Learn new concepts from industry experts
- Gain a foundational understanding of a subject or tool
- Develop job-relevant skills with hands-on projects
- Earn a shareable career certificate
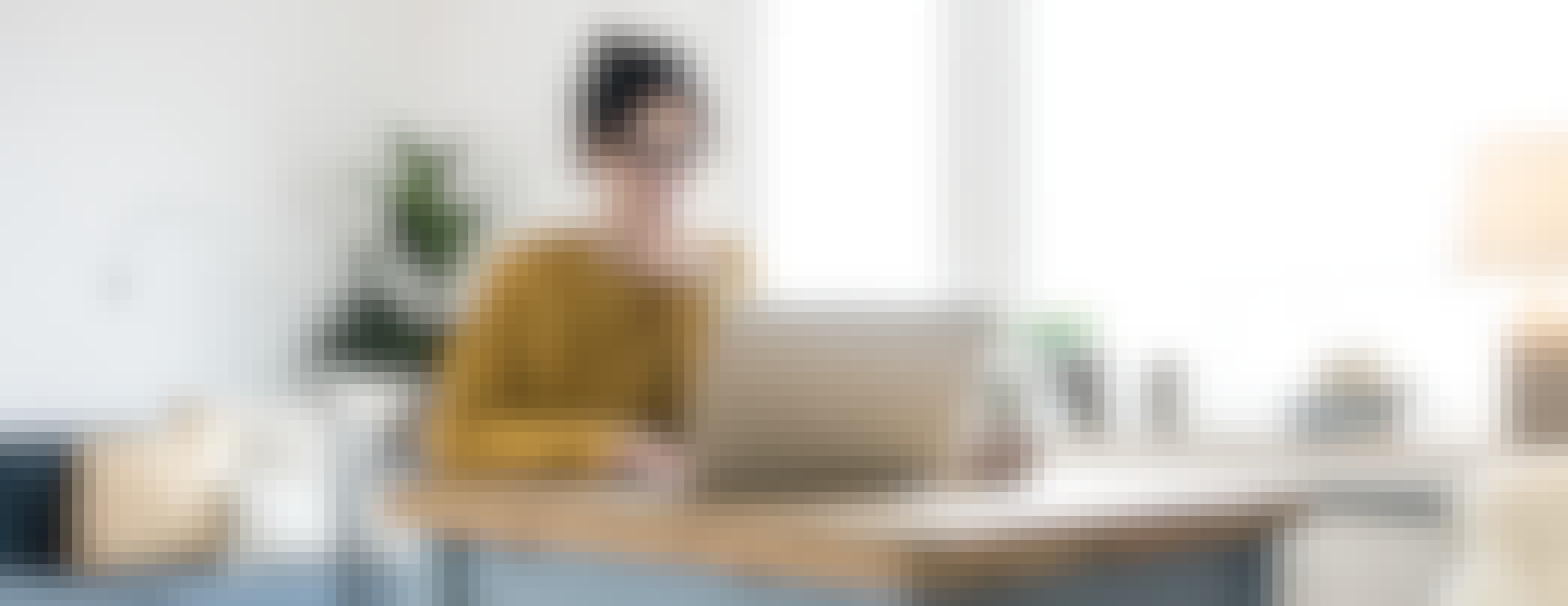
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
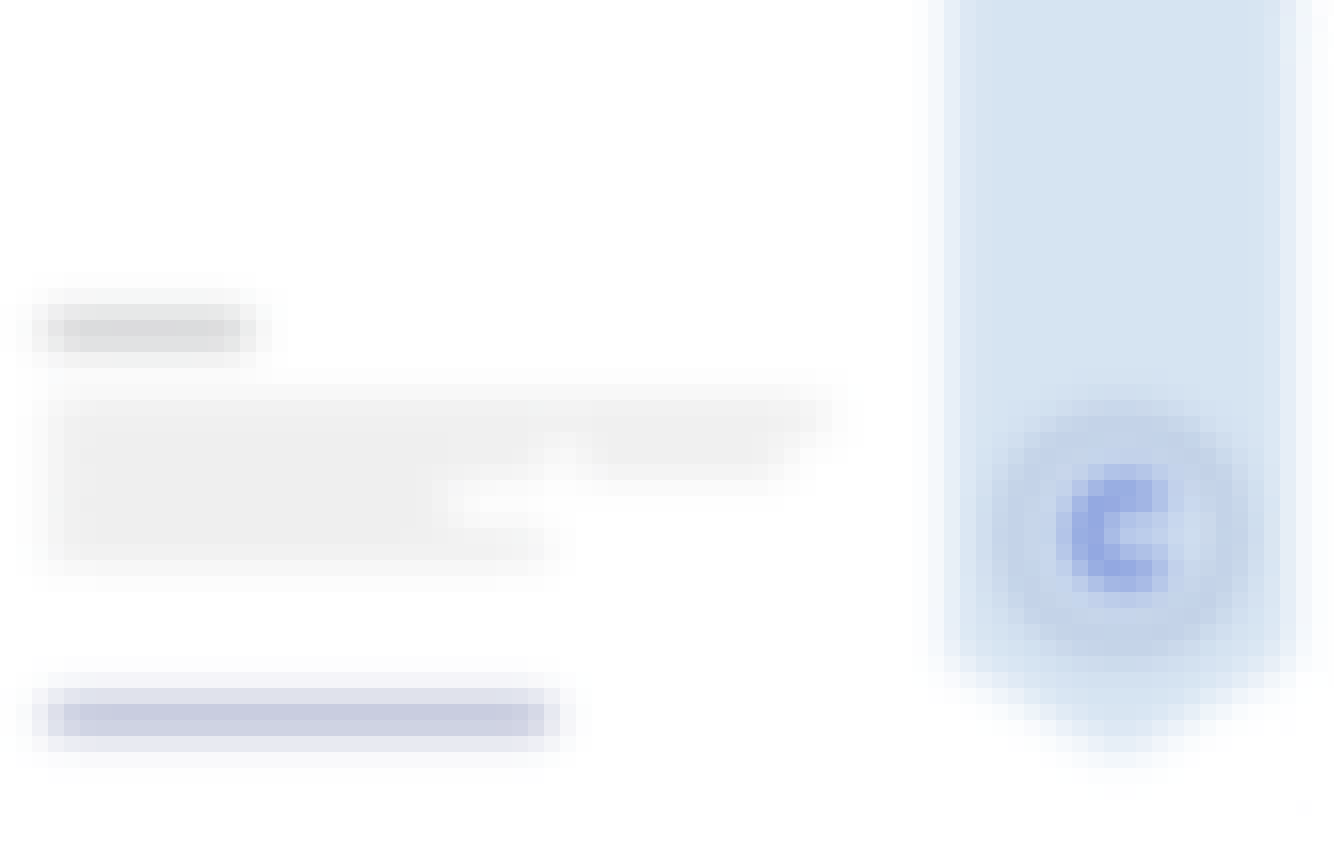
There are 6 modules in this course
This course is for everyone. In the new world we live in, coding is a universally valuable skill, whether you're a scientist, artist, or a humanist. Algorithms are everywhere, and we all have to understand how they work. The C language is particularly well suited as an introduction to coding: It's a tried-and-true language, and it allows you to understand computing processes at a deep level.
No prior knowledge of coding is needed for this course. We'll start at the beginning. The time estimated time commitment for this course is five hours a week for five weeks.
Introduction
An overview of the course, a history of the C language, and a first set of programming activities.
What's included
9 videos 2 peer reviews
9 videos • Total 67 minutes
- Overview • 4 minutes • Preview module
- History of C • 2 minutes
- Compiling, debugging, and running a program, part 1 • 8 minutes
- Compiling, debugging, and running a program part 2 (File included ➕) • 12 minutes
- First Program • 7 minutes
- Example - Circle code • 7 minutes
- Example - Marathon • 8 minutes
- Simple input/output - fahrenheit • 6 minutes
- Simple input/output - miles • 9 minutes
2 peer reviews • Total 120 minutes
- Fix Dr. P’s mistake (week 1) • 60 minutes
- Print a poem • 60 minutes
Lexical Elements and Data Types
Lexical elements and data types, programming activities of increasing sophistication, and an optional discussion of more advanced issues.
12 videos 2 quizzes 2 peer reviews 1 discussion prompt
12 videos • Total 102 minutes
- Character sets and tokens • 6 minutes • Preview module
- Comments • 4 minutes
- Keywords • 8 minutes
- Identifiers • 11 minutes
- Operators • 8 minutes
- Expressions and precedence (File included ➕) • 7 minutes
- Expression and evaluation • 9 minutes
- Declarations • 6 minutes
- Fundamental types and sizeof • 9 minutes
- The char type (File included ➕) • 8 minutes
- The int type • 10 minutes
- The integer and floating point types (Files included ➕) • 10 minutes
- int quiz • 0 minutes
- Expressions quiz • 0 minutes
- Fix Dr. P’s mistake (week 2) • 60 minutes
- Write a program that prints the sine function for an input x between (0, 1) • 60 minutes
1 discussion prompt • Total 10 minutes
- Problems from Chapter 1 of "A Book on C" (Optional Advanced Activity) • 10 minutes
Flow of Control and Simple Functions
Flow of control and simple functions, even more sophisticated programming activities, and an optional discussion of more advanced issues.
10 videos 4 quizzes 2 peer reviews 1 discussion prompt
10 videos • Total 61 minutes
- Logical operators, expressions, and short-circuit evaluation • 6 minutes • Preview module
- The conditional statement if and if-else • 7 minutes
- The iterative statement while • 6 minutes
- while-cnt-char-explained • 6 minutes
- while-code - example • 5 minutes
- The for statement and its while analog • 8 minutes
- for statement code example • 6 minutes
- oddball operators-conditional and comma • 7 minutes
- ternary-operator code example • 3 minutes
- Break and continue and switch (File included ➕) • 4 minutes
- Logic operators quiz • 0 minutes
- While loop questions • 0 minutes
- Switch questions • 0 minutes
- Cond-comma-ops quiz • 0 minutes
- Fix D. P's mistake (week 3) • 60 minutes
- Write a function that prints a table of values for sine and cosine between (0, 1) • 60 minutes
- Problem 23 from Chapter 2 of "A Book on C" (Optional Advanced Activity) • 10 minutes
Advanced Functions, Recursion, Arrays, and Pointers
A continuation of functions, recursion, arrays, and pointers.
11 videos • Total 65 minutes
- Function definition • 6 minutes • Preview module
- Function code example • 4 minutes
- Return statement • 4 minutes
- Function prototype • 4 minutes
- Function Prototype - code example • 5 minutes
- Function variables—with call-by-value explained • 7 minutes
- Function definitions and scope rules • 5 minutes
- Storage class code example • 7 minutes
- Simple recursion • 5 minutes
- Recursion- factorial code • 6 minutes
- Recursion Fibonacci code (File included ➕) • 9 minutes
Arrays and pointers
Further treatment of arrays and pointers and an interesting programming activity.
11 videos 1 peer review
11 videos • Total 84 minutes
- Pointers and simple arrays • 6 minutes • Preview module
- initialize arrays • 8 minutes
- array-grade-code example • 4 minutes
- What is a pointer • 9 minutes
- Pointer code example • 10 minutes
- Call-by-reference simulated • 7 minutes
- array as a parameter • 4 minutes
- array-bubble-sort code • 13 minutes
- merge sort overview • 7 minutes
- merge code -example 1 • 5 minutes
- merge code example 2 (File included ➕) • 7 minutes
1 peer review • Total 60 minutes
- Compute the average weight for a population of elephant seals read into an array • 60 minutes
The end of the first part of C for Everyone and an opportunity to assess your learning.
1 quiz • Total 90 minutes
- Final exam • 90 minutes
Instructor ratings
We asked all learners to give feedback on our instructors based on the quality of their teaching style.

UC Santa Cruz is an outstanding public research university with a deep commitment to undergraduate education. It’s a place that connects people and programs in unexpected ways while providing unparalleled opportunities for students to learn through hands-on experience.
Recommended if you're interested in Software Development
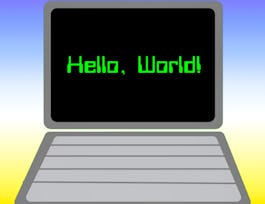
University of California, Santa Cruz
Coding for Everyone: C and C++
Specialization
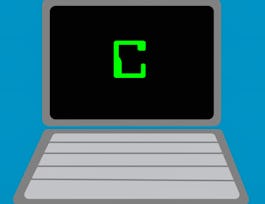
C for Everyone: Structured Programming
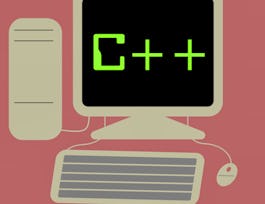
C++ For C Programmers, Part A
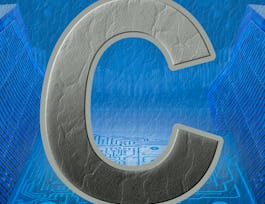
University of Michigan
Exploring C
Why people choose coursera for their career.

Learner reviews
Showing 3 of 6326
6,326 reviews
Reviewed on Mar 2, 2022
I encourage everyone if you do not yet join this course yes you can join you will run many new things whether you are a beginner or any other skills in programming. You will enjoy it for sure.
Reviewed on Apr 28, 2020
e will know all the essential knowledge to moe forward. It is a very logical and steady building up procedure. It is an absolutely stunning course. Thanks for professor Iran Pol.
Reviewed on Aug 31, 2020
The course is great , and it's cover all the basic of C language .Without coursera I can't get knowledge from the world best teacher , Thank you Coursera and also the instructor.
New to Software Development? Start here.

Open new doors with Coursera Plus
Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
When will i have access to the lectures and assignments.
Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. If you don't see the audit option:
The course may not offer an audit option. You can try a Free Trial instead, or apply for Financial Aid.
The course may offer 'Full Course, No Certificate' instead. This option lets you see all course materials, submit required assessments, and get a final grade. This also means that you will not be able to purchase a Certificate experience.
What will I get if I subscribe to this Specialization?
When you enroll in the course, you get access to all of the courses in the Specialization, and you earn a certificate when you complete the work. Your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. If you only want to read and view the course content, you can audit the course for free.
What is the refund policy?
If you subscribed, you get a 7-day free trial during which you can cancel at no penalty. After that, we don’t give refunds, but you can cancel your subscription at any time. See our full refund policy Opens in a new tab .
Is financial aid available?
Yes. In select learning programs, you can apply for financial aid or a scholarship if you can’t afford the enrollment fee. If fin aid or scholarship is available for your learning program selection, you’ll find a link to apply on the description page.
More questions
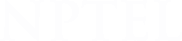
- Announcements
- Explore Courses
Problem solving through Programming In C
- BE/BTech in all disciplines
- BCA/MCA/M. Sc
- All IT Industries
65741 students have enrolled already!!
01. PLAY WITH PRINTF()
02. data type & variables, 03. user input scanf(), 04. apply your conditions, 05. magic of loop, 06. uses of array, 07. fun of function, 08. start with string, 09. pointer de complexity, 10. structur of union, 11. basic of file i/o, 12. math & coding.
Problem Solving with Computer
By Bipin Tiwari
Problem Solving is a scientific technique to discover and implement the answer to a problem. The computer is the symbol manipulating device that follows the set of commands known as program.
Program is the set of instructions which is run by the computer to perform specific task. The task of developing program is called programming.
Problem Solving Technique:
Sometimes it is not sufficient just to cope with problems. We have to solve that problems. Most people are involving to solve the problem. These problem are occur while performing small task or making small decision. So, Here are the some basic steps to solve the problems
Step 1: Identify and Define Problem
Explain you problem clearly as possible as you can.
Step 2: Generate Possible Solutions
- List out all the solution that you find. Don’t focus on the quality of the solution
- Generate the maximum number of solution as you can without considering the quality of the solution
Step 3: Evaluate Alternatives
After generating the maximum solution, Remove the undesired solutions.
Step 4: Decide a Solution
After filtering all the solution, you have the best solution only. Then choose on of the best solution and make a decision to make it as a perfect solution.
Step 5: Implement a Solution:
After getting the best solution, Implement that solution to solve a problem.
Step 6: Evaluate the result
After implementing a best solution, Evaluate how much you solution solve the problem. If your solution will not solve the problem then you can again start with Step 2 .
Algorithm is the set of rules that define how particular problem can be solved in finite number of steps. Any good algorithm must have following characteristics
- Input: Specify and require input
- Output: Solution of any problem
- Definite: Solution must be clearly defined
- Finite: Steps must be finite
- Correct: Correct output must be generated
Advantages of Algorithms:
- It is the way to sole a problem step-wise so it is easy to understand.
- It uses definite procedure.
- It is not dependent with any programming language.
- Each step has it own meaning so it is easy to debug
Disadvantage of Algorithms:
- It is time consuming
- Difficult to show branching and looping statement
- Large problems are difficult to implement
The solution of any problem in picture form is called flowchart. It is the one of the most important technique to depict an algorithm.
Advantage of Flowchart:
- Easier to understand
- Helps to understand logic of problem
- Easy to draw flowchart in any software like MS-Word
- Complex problem can be represent using less symbols
- It is the way to documenting any problem
- Helps in debugging process
Disadvantage of Flowchart:
- For any change, Flowchart have to redrawn
- Showing many looping and branching become complex
- Modification of flowchart is time consuming
Symbol Used in Flowchart:
Example: Algorithm and Flowchart to check odd or even
Coding, Compiling and Execution
Question's answer.
Share this link via
Or copy link
Copyright 2022 | HAMROCSIT.COM | All Right Reserved
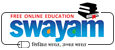
Problem Solving through Programming in C
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
Note: This exam date is subjected to change based on seat availability. You can check final exam date on your hall ticket.
Page Visits
Course layout, books and references, instructor bio.

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :
We will keep fighting for all libraries - stand with us!
Internet Archive Audio
- This Just In
- Grateful Dead
- Old Time Radio
- 78 RPMs and Cylinder Recordings
- Audio Books & Poetry
- Computers, Technology and Science
- Music, Arts & Culture
- News & Public Affairs
- Spirituality & Religion
- Radio News Archive
- Flickr Commons
- Occupy Wall Street Flickr
- NASA Images
- Solar System Collection
- Ames Research Center
- All Software
- Old School Emulation
- MS-DOS Games
- Historical Software
- Classic PC Games
- Software Library
- Kodi Archive and Support File
- Vintage Software
- CD-ROM Software
- CD-ROM Software Library
- Software Sites
- Tucows Software Library
- Shareware CD-ROMs
- Software Capsules Compilation
- CD-ROM Images
- ZX Spectrum
- DOOM Level CD

- Smithsonian Libraries
- FEDLINK (US)
- Lincoln Collection
- American Libraries
- Canadian Libraries
- Universal Library
- Project Gutenberg
- Children's Library
- Biodiversity Heritage Library
- Books by Language
- Additional Collections
- Prelinger Archives
- Democracy Now!
- Occupy Wall Street
- TV NSA Clip Library
- Animation & Cartoons
- Arts & Music
- Computers & Technology
- Cultural & Academic Films
- Ephemeral Films
- Sports Videos
- Videogame Videos
- Youth Media
Search the history of over 866 billion web pages on the Internet.
Mobile Apps
- Wayback Machine (iOS)
- Wayback Machine (Android)
Browser Extensions
Archive-it subscription.
- Explore the Collections
- Build Collections
Save Page Now
Capture a web page as it appears now for use as a trusted citation in the future.
Please enter a valid web address
- Donate Donate icon An illustration of a heart shape
Problem solving using C : structured programing techniques
Bookreader item preview, share or embed this item, flag this item for.
- Graphic Violence
- Explicit Sexual Content
- Hate Speech
- Misinformation/Disinformation
- Marketing/Phishing/Advertising
- Misleading/Inaccurate/Missing Metadata
![[WorldCat (this item)] [WorldCat (this item)]](https://archive.org/images/worldcat-small.png)
plus-circle Add Review comment Reviews
28 Previews
2 Favorites
DOWNLOAD OPTIONS
No suitable files to display here.
PDF access not available for this item.
IN COLLECTIONS
Uploaded by station18.cebu on January 18, 2024
SIMILAR ITEMS (based on metadata)
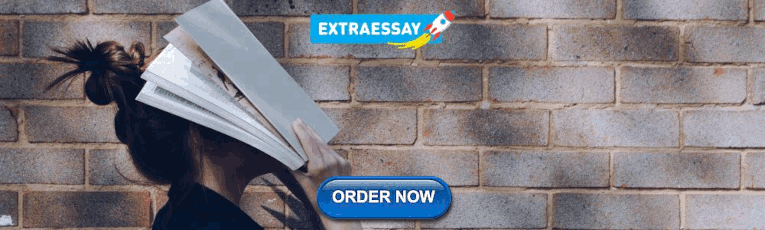
IMAGES
VIDEO
COMMENTS
Q2: Write a Program to find the Sum of two numbers entered by the user. In this problem, you have to write a program that adds two numbers and prints their sum on the console screen. For Example, Input: Enter two numbers A and B : 5 2. Output: Sum of A and B is: 7.
Practice C programming skills and solve real-world problems on HackerRank, the leading platform for competitive coding.
Learners enrolled: 29073. ABOUT THE COURSE : This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
Note: Practice C Programs for problem solving through programming in C. Problem Solving Steps. Problem-solving is a creative process which defines systematization and mechanization. There are a number of steps that can be taken to raise the level of one's performance in problem-solving. A problem-solving technique follows certain steps in ...
Use C to kickstart your journey in the world of logic building, basic data structures and algorithms. Courses. Learn Python 10 courses. Learn C++ 9 ... Simple math concepts required to solve programming problems. Lesson. Addition and multiplication. Lesson. Subtraction and division. 3. Conditional statements. Simple conditional concepts ...
In addition to two newly introduced chapters on 'Graphics using C' and 'Searching and Sorting', all other chapters of the previous edition have been thoroughly revised and updated. The usage of pseudocodes as a problem-solving tool has been explored throughout the book before providing C programming solutions for the problems, wherever necessary.
Specialization - 4 course series. This specialization develops strong programming fundamentals for learners who want to solve complex problems by writing computer programs. Through four courses, you will learn to develop algorithms in a systematic way and read and write the C code to implement them. This will prepare you to pursue a career in ...
This course is part of the Coding for Everyone: C and C++ Specialization. When you enroll in this course, you'll also be enrolled in this Specialization. Learn new concepts from industry experts. Gain a foundational understanding of a subject or tool. Develop job-relevant skills with hands-on projects.
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
Share your videos with friends, family, and the world
Documentation - Techniques of Problem Solving - Problem solving aspects - Top- Down aspects - Implementation of algorithms - Program verification - Flowcharting, decision table, algorithms, Structured programming concepts, Programming methodologies viz. top-down and bottom-up programming. Basic Concepts of Computer
Problem solving through Programming In C. ABOUT THE COURSE This course is aimed at enabling the students to. ·formulate simple algorithms for arithmetic and logical problems·translate the algorithms to programs (in C language)·test and execute the programs and correct syntax and logical errors·implement conditional branching, iteration and ...
This course is aimed at enabling the students toFormulate simple algorithms for arithmetic and logical problems.Translate the algorithms to programs (in C la...
Programming for problem solving using C Notes Unit - I Computer History, Hardware, Software, Programming Languages and Algorithms: Components andfunctions of a Computer System, Concept of Hardware and Software Programming Languages: Low- level and High-level Languages, Program Design Tools: Algorithm, Flowchart, Pseudo code.
Course abstract. This course is aimed at enabling the students to 1. Formulate simple algorithms for arithmetic and logical problems 2. Translate the algorithms to programs (in C language) 3. Test and execute the programs and correct syntax and logical errors 4. Implement conditional branching, iteration and recursion 5.
Jones and Harrow present programming concepts in the context of solving problems. Each chapter introduces a problem first, and then covers the C language elements needed to solve it. Students can see how a program is built from its simplest beginning to its final polished form. This book introduces beginning programming concepts using the C language.
How to Prepare for Problem Solving through Programming in C NPTEL Exam ( Proctored Exam )Tips and Tricks for cracking Proctored Exam of Problem Solving throu...
101 C programming. 01. PLAY WITH PRINTF () PROBLEM [01-05] 02. DATA TYPE & VARIABLES. PROBLEM [06-12] 03. USER INPUT SCANF ()
NPTEL :: Computer Science and Engineering - NOC:Problem Solving through Programming in C. Courses. Computer Science and Engineering. NOC:Problem Solving through Programming in C (Video) Syllabus. Co-ordinated by : IIT Kharagpur. Available from : 2017-12-21. Lec : 1. Watch on YouTube.
Step 1: Identify and Define Problem. Explain you problem clearly as possible as you can. Step 2: Generate Possible Solutions. List out all the solution that you find. Don't focus on the quality of the solution. Generate the maximum number of solution as you can without considering the quality of the solution.
Problem solving through Programming In C. By Prof. Anupam Basu | IIT Kharagpur. Learners enrolled: 61372. This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors.
Learners enrolled: 25190. This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
Problem solving using C : structured programing techniques ... IBM PC or compatible, with Microsoft Windows 3.1 or higher and C programming language Includes index Access-restricted-item true Addeddate 2024-02-14 00:36:18 Autocrop_version ..17_books-serials-20230720-.3 Boxid IA41211503 ...