7 Ways to Loop Through a List in Python

Lists are one of the six fundamental data types in the Python programming language. To work effectively with Python, you need to know the functions and methods that work with lists. And that’s what we’ll explain in this article.
In Python, lists can be used to store multiple elements in a single variable. Moreover, a single Python iterate list can harbor elements of multiple data types. Lists (like arrays in other programming languages) can also be nested – i.e. lists can contain other lists.
Python provides multiple ways to iterate over lists; each one has its benefits and drawbacks. In this article, we shall look at how Python lists are iterated and present an example for each method. If this all seems new, we recommend trying our Learn Programming with Python track to get a head start in Python programming. This track will help you understand the fundamentals of programming, including lists and iteration.
Without further delay, let's dive right in!
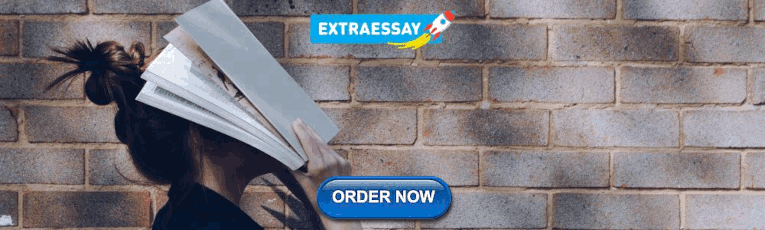
7 Ways You Can Iterate Through a List in Python
1. a simple for loop.
Using a Python for loop is one of the simplest methods for iterating over a list or any other sequence (e.g. tuples, sets, or dictionaries ).
Python for loops are a powerful tool , so it is important for programmers to understand their versatility. We can use them to run the statements contained within the loop once for each item in a list. For example:
Running the function results in the following output:
Here, the for loop has printed each of the list items. In other words, the loop has called the print() function four times, each time printing the current item in the list – i.e. the name of a fruit.
2. List Comprehension
List comprehension is similar to the for loop; however, it allows us to create a list and iterate through it in a single line. Due to its utter simplicity, this method is considered one of the most robust ways of iterating over Python lists. Check out this article on lists and list comprehension in Python for more details. For now, let’s look at an example:
You’ll notice that we’re using what looks like another for loop: for fruit in fruits . The key here is that the command and the for..in structure are enclosed with the print() command in square brackets; that’s what makes it a list comprehension.
Here’s the output:
As you can see, we created the fruits list just as we did in the previous example. However, this time we used list comprehension to do two things: add the word ‘juice’ to the end of the list item and print it.
3. A for Loop with range()
Another method for looping through a Python list is the range() function along with a for loop. range() generates a sequence of integers from the provided starting and stopping indexes. (An index refers to the position of elements in a list. The first item has an index of 0, the second list item is 1, and so on.) The syntax of the range function is as follows:
The start and step arguments are optional; only the stop argument is required. The step determines if you skip list items; this is set as 1 by default, meaning no items are skipped. If you only specify one parameter (i.e. the stop index), the function constructs a range object containing all elements from 0 to stop-1 .
Here’s an example that will print the fruit name and its index in the list:
This results in the following output:
A slightly different approach would be to print only some of the fruits based on their index. We’d do this by specifying the starting and ending index for the for loop using the range() function:
Here’s the output:
As we asked, it’s returned only those fruits at index 1 and 2; remember, 3 is the stopping point, and 0 is the first index in Python.
4. A for Loop with enumerate()
Sometimes you want to know the index of the element you are accessing in the list. The enumerate() function will help you here; it adds a counter and returns it as something called an ‘enumerate object’. This object contains elements that can be unpacked using a simple Python for loop. Thus, an enumerate object reduces the overhead of keeping a count of the number of elements in a simple iteration.
Here’s an example:
Running the above code returns this list of the elements and their indexes:
5. A for Loop with lambda
Python’s lambda function is an anonymous function in which a mathematical expression is evaluated and then returned. As a result, lambda can be used as a function object. Let’s see how to use lambda as we loop through a list.
We’ll make a for loop to iterate over a list of numbers, find each number's square, and save or append it to the list. Finally, we’ll print a list of squares. Here’s the code:
We use lambda to iterate through the list and find the square of each value. To iterate through lst1 , a for loop is used. Each integer is passed in a single iteration; the append() function saves it to lst2 .
We can make this code even more efficient using the map() function:
After applying the provided function to each item in a specified iterable, map() produces a map object (which is an iterator) of the results.
Both these codes give the exact same output:
6. A while Loop
We can also iterate over a Python list using a while loop. This is one of the first loops beginning programmers meet. It's also one of the easiest to grasp. If you consider the name of the loop, you'll soon see that the term "while" has to do with an interval or time period. The term "loop" refers to a piece of code that is executed repeatedly. So, a while loop executes until a certain condition is met.
In the code below, that condition is the length of the list; the i counter is set to zero, then it adds 1 every time the loop prints one item in the list. When i becomes greater than the number of items in the list, the while loop terminates. Check out the code:
Can you guess what the output will be?
It is important to note the i = i + 1 in the code above can also be shortened as i += 1 .
Our code ensures that the condition i < len( fruits ) will be satisfied after a certain number of iterations. Ending while loops properly is critical; you can learn more about how to end loops in Python here .
7. The NumPy Library
The methods we’ve discussed so far used a small lists. However, efficiency is essential when you’re working with larger amounts of data. Suppose you have large single-dimensional lists with a single data type. In this case, an external library like NumPy is the best way to loop through big lists.
NumPy reduces the overhead by making iteration more efficient. This is done by converting the lists into NumPy arrays . As with lists, the for loop can also be used to iterate over these arrays.
It is important to note that the method we present here can only be used for arrays of single data types.
Running the code above gives the following output:
Although we’ve used for num in nums : for its simplicity in this example, it’s usually better to use for num in np.nditer(nums): when you’re working with large lists. The np.nditer function returns an iterator that can traverse the NumPy array, which is computationally more efficient than using a simple for loop.
Time To Practice Lists and Loops in Python!
Python loops are useful because they allow you to repeat a piece of code. You'll frequently find yourself in circumstances where you'll need to perform the same operations over and over again; loops help you do so efficiently.
You now know many ways to use Python to loop through a list. If you want to practice what you’ve learned (and solidify your understanding of Python) check out our Python Practice Set . The exercises are straightforward and intuitive. Plus, there aren’t many tricky questions, and you will always be able to count on help and hints. So visit this course now and embark on your journey to become a Pythonista.
You may also like
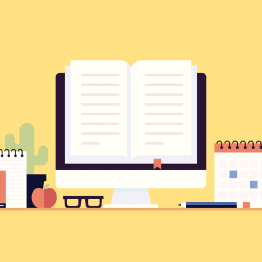
How Do You Write a SELECT Statement in SQL?
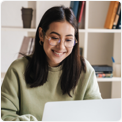
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Home » Python Basics » How to Use a For Loop to Iterate over a List
How to Use a For Loop to Iterate over a List
Summary : in this tutorial, you’ll learn how to use the Python for loop to iterate over a list in Python.
Using Python for loop to iterate over a list
To iterate over a list , you use the for loop statement as follows:
In this syntax, the for loop statement assigns an individual element of the list to the item variable in each iteration.
Inside the body of the loop, you can manipulate each list element individually.
For example, the following defines a list of cities and uses a for loop to iterate over the list:
In this example, the for loop assigns an individual element of the cities list to the city variable and prints out the city in each iteration.
Using Python for loop to iterate over a list with index
Sometimes, you may want to access indexes of elements inside the loop. In these cases, you can use the enumerate() function.
The enumerate() function returns a tuple that contains the current index and element of the list.
The following example defines a list of cities and uses a for loop with the enumerate() function to iterate over the list:
To access the index, you can unpack the tuple within the for loop statement like this:
The enumerate() function allows you to specify the starting index which defaults to zero.
The following example uses the enumerate() function with the index that starts from one:
- Use a for loop to iterate over a list.
- Use a for loop with the enumerate() function to access indexes.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python for loops.
A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).
This is less like the for keyword in other programming languages, and works more like an iterator method as found in other object-orientated programming languages.
With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc.
Print each fruit in a fruit list:
The for loop does not require an indexing variable to set beforehand.
Looping Through a String
Even strings are iterable objects, they contain a sequence of characters:
Loop through the letters in the word "banana":
The break Statement
With the break statement we can stop the loop before it has looped through all the items:
Exit the loop when x is "banana":
Exit the loop when x is "banana", but this time the break comes before the print:
Advertisement
The continue Statement
With the continue statement we can stop the current iteration of the loop, and continue with the next:
Do not print banana:
The range() Function
The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and ends at a specified number.
Using the range() function:
Note that range(6) is not the values of 0 to 6, but the values 0 to 5.
The range() function defaults to 0 as a starting value, however it is possible to specify the starting value by adding a parameter: range(2, 6) , which means values from 2 to 6 (but not including 6):
Using the start parameter:
The range() function defaults to increment the sequence by 1, however it is possible to specify the increment value by adding a third parameter: range(2, 30, 3 ) :
Increment the sequence with 3 (default is 1):
Else in For Loop
The else keyword in a for loop specifies a block of code to be executed when the loop is finished:
Print all numbers from 0 to 5, and print a message when the loop has ended:
Note: The else block will NOT be executed if the loop is stopped by a break statement.
Break the loop when x is 3, and see what happens with the else block:
Nested Loops
A nested loop is a loop inside a loop.
The "inner loop" will be executed one time for each iteration of the "outer loop":
Print each adjective for every fruit:
The pass Statement
for loops cannot be empty, but if you for some reason have a for loop with no content, put in the pass statement to avoid getting an error.
Test Yourself With Exercises
Loop through the items in the fruits list.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
Python for Loop
Python while Loop
Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
- Python Sets
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python range() Function
- Python enumerate()
In Python, a for loop is used to iterate over sequences such as lists , strings , tuples , etc.
In the above example, we have created a list called languages . As the list has 3 elements, the loop iterates 3 times.
The value of i is
- Swift in the first iteration.
- Python in the second iteration.
- Go in the third iteration.
- for loop Syntax
Here, val accesses each item of the sequence on each iteration. The loop continues until we reach the last item in the sequence.
- Flowchart of Python for Loop
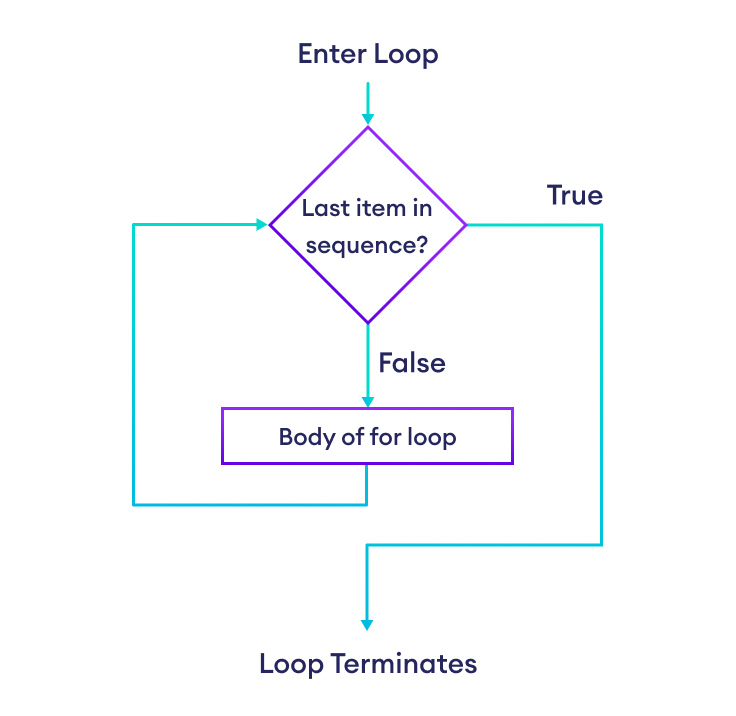
- Example: Loop Through a String
Here, we have printed each character of the string language using a for loop.
- for Loop with Python range()
In Python, the range() function returns a sequence of numbers. For example,
Here, range(4) returns a sequence of 0 , 1 , 2 ,and 3 .
Since the range() function returns a sequence of numbers, we can iterate over it using a for loop. For example,
Here, we used the for loop to iterate over a range from 0 to 3 .
This is how the above program works.
More on Python for Loop
A for loop can have an optional else clause. This else clause executes after the iteration completes.
Here, the for loop prints all the items of the digits list. When the loop finishes, it executes the else block and prints No items left .
Note : The else block will not execute if the for loop is stopped by a break statement.
We can also use for loop to repeat an action a certain number of times. For example,
Here, we used the list languages to run the loop three times. However, we didn't use any of the elements of the list.
In such cases, it is clearer to use the _ (underscore) as the loop variable. The _ indicates that a loop variable is a placeholder and its value is intentionally being ignored.
For example,
Here, the loop still runs three times because there are three elements in the languages list. Using _ indicates that the loop is there for repetition and not for accessing the elements.
A for loop can also have another for loop inside it. For each cycle of the outer loop, the inner loop completes its entire sequence of iterations. For example,
Also Read :
- Python while loop
Table of Contents
- Introduction
Write a function to calculate the factorial of a number.
- The factorial of a non-negative integer n is the product of all positive integers less than or equal to n .
- For example, if n is 5 , the return value should be 120 because 1*2*3*4*5 is 120 .
Video: Python for Loop
Sorry about that.
Related Tutorials
Python Library
Python Tutorial
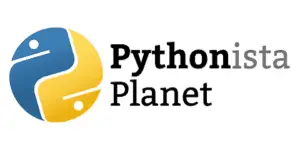
21 Python for Loop Exercises and Examples
In Python programming, we use for loops to repeat some code a certain number of times. It allows us to execute a statement or a group of statements multiple times by reducing the burden of writing several lines of code.
To get a clear idea about how a for loop works, I have provided 21 examples of using for loop in Python. You can go through these examples and understand the working of for loops in different scenarios.
Let’s dive right in.
1. Python for loop to iterate through the letters in a word
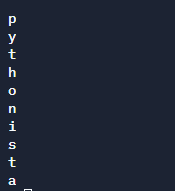
2. Python for loop using the range() function
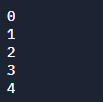
3. Python for loop to iterate through a list
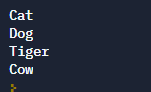
4. Python for loop to iterate through a dictionary
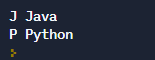
5. Python for loop using the zip() function for parallel iteration
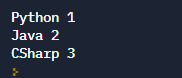
6. Using else statement inside a for loop in Python
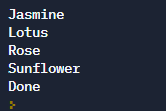
7. Nested for loops in Python (one loop inside another loop)
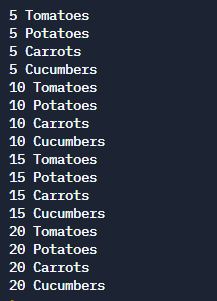
8. Using break statement inside a for loop in Python
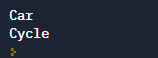
9. Using continue statement inside a for loop in Python
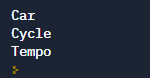
10. Python for loop to count the number of elements in a list
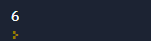
11. Python for loop to find the sum of all numbers in a list
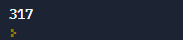
12. Python for loop to find the multiples of 5 in a list
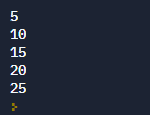
13. Python for loop to print a triangle of stars
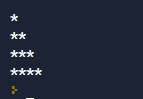
14. Python for loop to copy elements from one list to another

15. Python for loop to find the maximum element in a list
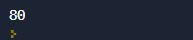
16. Python for loop to find the minimum element in a list
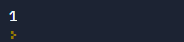
17. Python for loop to sort the numbers in a list in ascending order

18. Python for loop to sort the numbers in a list in descending order

19. Python for loop to print the multiples of 3 using range() function
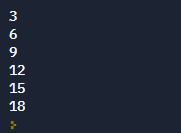
20. Python for loop to print the multiples of 5 using range() function
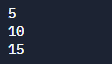
21. Python for loop to print the numbers in reverse order using range() function
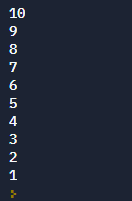
I hope this article was helpful. Check out my post on 18 Python while Loop Examples .
I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology. On this site, I share everything that I've learned about computer programming.
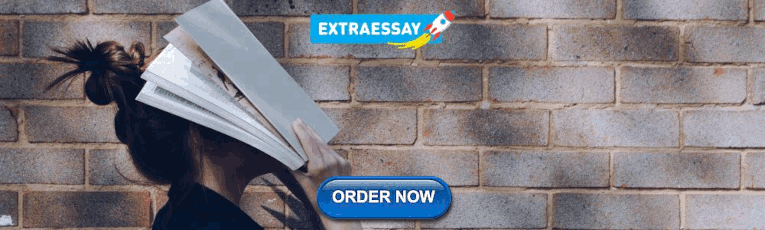
3 thoughts on “ 21 Python for Loop Exercises and Examples ”
we need some more difficult problems. please give some different questions.
These are some examples of implementing for loop in Python. If you want more coding problems, check out this post: https://pythonistaplanet.com/python-programming-exercises-and-solutions/
myfamily = { “child1” : { “name” : “Emil”, “year” : 2004 }, “child2” : { “name” : “Tobias”, “year” : 2007 }, “child3” : { “name” : “Linus”, “year” : 2011 } }
Find the child the year greater than 2007. Output = child3
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name and email in this browser for the next time I comment.
Recent Posts
Introduction to Modular Programming with Flask
Modular programming is a software design technique that emphasizes separating the functionality of a program into independent, interchangeable modules. In this tutorial, let's understand what modular...
Introduction to ORM with Flask-SQLAlchemy
While Flask provides the essentials to get a web application up and running, it doesn't force anything upon the developer. This means that many features aren't included in the core framework....
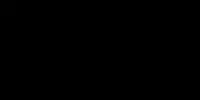

- Online Python Compiler
- Hello World
- Console Operations
- Conditional Statements
- Loop Statements
- Builtin Functions
- Type Conversion
Collections
- Classes and Objects
- File Operations
- Global Variables
- Regular Expressions
- Multi-threading
- phonenumbers
- Breadcrumbs
- ► Python Examples
- ► ► List Operations
- ► ► ► Python List For Loop
- Python Strings
- Python List Tutorials
- Python Lists
- Python List Operations
- Create Lists
- Python – Create an empty list
- Python – Create a list of size n
- Python – Create a list of numbers from 1 to n
- Python – Create a list of strings
- Python – Create a list of objects
- Python – Create a list of empty lists
- Access Lists
- Python List – Access elements
- Python List – Get item at specific index
- Python List – Get first element
- Python List – Get last element
- Python List – Iterate with index
- Python List – While loop
- Python List – For loop
- Python List – Loop through items
- Python – Unpack List
- List Properties
- Python – List length
- List Methods
- Python List Methods
- Python List append()
- Python List clear()
- Python List copy()
- Python List count()
- Python List extend()
- Python List index()
- Python List insert()
- Python List pop()
- Python List remove()
- Python List reverse()
- Python List sort()
- Update / Transform Lists
- Python – Add item to list
- Python – Remove specific item from list
- Python – Remove item at specific index from list
- Python – Remove all occurrences of an item from list
- Python – Remove duplicates from list
- Python – Append a list to another list
- Python – Reverse list
- Python – Sort list
- Python – Sort list in descending order
- Python – Shuffle list
- Python – Convert list into list of lists
- Checking Operations on Lists
- Python - Check if list is empty
- Python - Check if element is present in list
- Python - Check if value is in list using "in" operator
- Python - Check if list contains all elements of another list
- List Finding Operations
- Python – Count the occurrences of items in a List with a specific value
- Python – Find duplicates in list
- Python – Find unique elements in list
- Python – Find index of specific item in list
- Python – Insert item at specific position in list
- Python – Find element with most number of occurrences in the list
- Python – Find element with least number of occurrences in the list
- List Comprehension Operations
- Python List Comprehension
- Python List Comprehension with two lists
- Python List Comprehension with If condition
- Python List Comprehension with multiple conditions
- Python List Comprehension with List of Lists
- Python List Comprehension with nested For loops
- List Filtering
- Python – Filter list
- Python – Filter list of strings that start with specific prefix string
- Python – Filter list based on datatype
- Python – Get list without last element
- Python – Traverse list except last element
- Python List – Get first N elements
- Python List – Get last N elements
- Python List – Slice
- Python List – Slice first N elements
- Python List – Slice last N elements
- Python List – Slice all but last
- Python List – Slice reverse
- List Conversions to other Types
- Python – Convert List to Dictionary
- Python – Convert dictionary keys to list
- Python – Convert dictionary values to list
- Python – Convert list to tuple
- Python – Convert tuple to list
- Python – Convert list to set
- Python – Convert set to list
- Python – Convert list to string
- Python – Convert CSV string to list
- Python – Convert list to CSV string
- Python – Convert string (numeric range) to a list
- Python – Convert list of lists to a flat list
- Python - Convert list of strings into list of integers
- Python - Convert list of strings into list of floats
- Python - Convert list of integers into list of strings
- Python - Convert list of floats into list of strings
- Numeric Lists
- Python – Find average of numbers in list
- Python – Find largest number in list
- Python – Find smallest number in list
- Python – Find sum of all numbers in numeric list
- Python – Find product of all numbers in numeric list
- Python – Sort numbers
- String Lists
- Python – Compare lists lexicographically
- Python – Find shortest string in list
- Python – Find longest string in list
- Python – Find most occurred string in a list
- Python – Find least occurred string in a list
- Python – Sort list of strings based on length
- Python – Sort a list alphabetically
- Python - Join list with new line
- Python - Join list with comma
- Python - Join list with space
- Printing Lists
- Python - Print list
- Python - Print list without brackets
- Python - Print list without commas
- Python - Print list without quotes
- Python - Print list without spaces
- Python - Print list without brackets and commas
- Python - Print list without brackets and quotes
- Python - Print list without quotes and commas
- Python - Print list in reverse order
- Python - Print list in JSON format
- Python - Print list with double quotes
- Python - Print list with index
- Python - Print list as column
- Python - Print list as lines
- Python - Print elements at even indices in List
- Python - Print elements at odd indices in List
- Exceptions with Lists
- Python List ValueError: too many values to unpack
- Python List ValueError: not enough values to unpack
- List Other Topics
- Python List of Lists
- Python – Print a list
- Python List vs Set
- Python Dictionary
Python List For Loop
Syntax of list for loop.
- Iterate over List using For Loop
- Iterate over list of numbers using For loop
Python List is a collection of items. For loop can be used to execute a set of statements for each of the element in the list.
In this tutorial, we will learn how to use For loop to traverse through the elements of a given list.
In general, the syntax to iterate over a list using for loop is
- element contains value of the this element in the list. For each iteration, next element in the list is loaded into this variable.
- list is the Python List over which we would like to iterate.
- statement(s) are block are set of statement(s) which execute for each of the element in the list.
1. Iterate over List using For Loop
In this example, we take a list of strings, and iterate over each string using for loop, and print the string length.
Python Program
Reference tutorials for the above program
- Python print()
- Python len()
2. Iterate over list of numbers using For loop
In this example, we will take a list of numbers, and iterate over each number using for loop, and in the body of for loop, we will check if the number is even or odd.
- Python If-Else
In this tutorial of Python Examples , we learned how to use For Loop to iterate over Python List elements.
Related Tutorials
Lists and for-loops
So far, we’ve needed a new variable name for each new piece of information we wanted to store. A list is a Python data type that can store multiple pieces of information, in order, and with a single variable name. The list name, together with a non-negative integer can then be used to refer to the individual items of data. Let’s start with an example:
Notice that we can use moons[0] and moons[1] as if they were variables in their own right.
There are two main uses of lists:
- Storing large amounts of similar data using just one name for the list.
- Applying similar operations to many pieces of data.
Creating lists
You can type a list in using brackets and commas.
When the Python interpreter reaches the brackets, Python allocates space in memory for the items in the list. Of course, usually there’s not much point in just printing out a list created this way; we’d like to be able to refer to the list with a variable name. The assignment operator = assigns the list to the variable on the left-hand side:
Strictly speaking, it’s incorrect to say “ characters is a list.” Instead, the correct thing to say is “ characters holds the address in memory of a list.” Although the difference between “is a list” and “holds the address in memory of a list” might seem subtle right now, we’ll see later that there’s definitely a difference.
You can also create an empty list.
For now, we don’t have much use for empty lists, but we’ll soon see that we can start with an empty list and then add items to it.
Exercise: star power
Objective: Create a list with data and make use of that list by passing it to a function.
The vertices of a star centered the middle of a small drawing area are (161, 86), (119, 80), (100, 43) (81, 80), (39, 86), (70, 116), (63, 158), (100, 138), (137, 158), (130, 116).
In the code below, the polygon function takes two parameters. The first parameter is a reference to a list containing the x coordinates of a polygon. The second parameter is a reference to a list containing the y coordinates of a polygon. Draw a star on the screen.
Here is a solution .
Accessing items of a list
Once a list has been created and its address assigned to a variable, you will want to do things with individual items of the list. We use square brackets to index into a list. The first list element is at index 0 (because that’s how computer scientists like to count: starting from 0), so to get the 0th item in the list, follow the name of the list variable with square brackets containing the number 0.
What goes within the brackets can be any expression that evaluates to an int that is in the range of the indices:
Notice that list items can really be treated as variables. You can use the assignment operator to change an item in a list ( characters[1] = "Thing One" ). Here is another example:
some_numbers[2] has the value 8 (remember, we start counting, or indexing, the list, from 0). some_number[3] has the value 12. After adding, we get 20, and assign that value to the 0th item of the list. Run the program to see the result.
Common programming error: List index out of range
If you forget that the list is indexed from 0, or for some other reason try to access an item of the list at an index beyond the end of the list, Python will abort your program and print an error message. The following code fails, because there are only items at indices 0, 1, and 2:
This is a very common type of programming error. If you get an error like
from Python, step through your code by hand near the line where Python reports an error, and figure out how you managed to ask for a list index that isn’t in the list. There are two cases:
- You asked for an item at the index you intended, but somehow didn’t originally put the item into the list.
- You put the correct items into the list, but made a mistake in computing or choosing the index.
The length of a list
You can find out how many items are in a list using the Python built-in function len . Here’s an example:
Iterating over a list with a while-loop
A list is a way of organizing, or structuring, data that your program will use. We say that a list is a data structure , and we will see many other examples of data structures later in the course. One of the key principles of a data structure is that the data structure allows you to apply operations to some or all of the data in a unified way.
Let’s take an example. You decide to start a new website, www.warmhanoverweather.com . Initially, daily temperatures are recorded in Fahrenheit.
But then next month, the United States decides to convert to the Celsius scale, leaving the Bahamas, Belize, the Cayman Islands, and Jamaica as the only countries using the Fahrenheit scale. You decide to convert all of your temperatures to Celsius. You could do it this way:
(Notice the floating-point inaccuracies. I told you so.)
You are doing the same type of operation over and over again. Convert temperatures[0] . Convert temperatures[1] . Convert temperatures[2] . And so on. You could use a loop, and replace six statements like so,
This while-loop would work for even a very long list of temperatures.
Exercise: falling star
Objective: Modify the data in a list using a while loop.
The code below draws a star in the center of the screen. Re-write the code so that the star slowly falls from its starting position downwards.
An algorithm design and implementation example: reversing a list
Let’s look at an example in which we want to reverse a list. Recall from the first chapter that a fully specified step-by-step strategy for solving a problem in computer science is called an algorithm . The first step to solving a problem is to think about how we might solve it as a human, as precisely as possible. Once each step has been fully specified, we have designed an algorithm. We then translate that algorithm into runnable code, thereby implementing the algorithm.
There are two choices for our reverse_list algorithm:
Create a new, reversed copy of the list, leaving the original list intact.
Reverse the list “in place.” If we do it this way, there is only one list, and at the end of the operation, the items of the list are in reversed order. We say that this operation is in place because the items never leave the list they started in.
We’ll use the second approach and reverse the list in place, since I haven’t yet told you how to make a new list of the correct size.
Now let’s think about how to reverse the list in place. What’s our target?
We want the first item to be in the last item’s spot, and vice versa. We want the second item to be in the current second-to-last item’s spot, and vice versa. So here is a first attempt at our algorithm specification, in English (or pseudo-code):
Will it work? Let’s walk through it in our heads for a list of length 6. Start by swapping the first item with the sixth. Good. Swap the second item with the fifth. Good. Swap the third item with the fourth. Good. The next item is the fourth item. Houston, we have a problem. After we swapped the third item with the fourth, we actually were done—the list was reversed. If we then swap the fourth with the third, as our first attempt at an algorithm seems to specify, we put them back to where they were when we started. We have begun to undo our reversal of the list items. We need to modify our algorithm so that it stops after swapping only half of the items in the list.
There’s one more thing we should be nervous about. A list of length 6 has a first half and a second half, each of size 3. What if we had a list of length 7? What’s the first half? What’s the second? How many swaps should we do? Well, the item that is precisely in the middle of an odd-length list doesn’t get changed by reversing the list. So for a list of length 7, we need to do only 3 swaps. If the list length is odd, the number of swaps is the length of the list, divided by 2, and rounded down. If the list length is even, the number of swaps is the length of the list divided by 2. So our final algorithm design is:
Now let’s implement the reverse_list algorithm in Python code. We need to loop through the first half (rounded down) of the list. We can use a while-loop for that.
There are a few tricky things to notice. First, we can accomplish the rounding down of the index of the middle item of the list by just using integer division ( while index < len(l) // 2: ). In Python 2, we could have just used the / operator, but the // integer division operator makes it more specific and works for Python 3, too.
Also, because indices in a list start at 0, we have to be careful about computing the index into the second half of the list. It’s good to check an example. Suppose the list l has length 8. Then the index of the last item should be 7. So we should use len(l) - 1 , and then subtract the current index to get the index into the second half of the list. Work it out: if the index is 0, then len(l) - 1 - 0 gives 7. If the index is 1, then len(l) - 1 - 1 gives 6. If the index is 2, then len(l) - 1 - 2 gives 5. And so on. So it works out.
The final thing to notice is how we swap items. We need a temporary variable to hold one of the values. Suppose you tried something like this:
The both items of the list would end up having the same value after the first line, and the original value of l[index] has been irrevocably lost.
If needing a temporary variable confuses you, think of it this way. Suppose that Nicole and Tom want to exchange the positions of their two cars in their two-car garage. Tom’s Chevy starts on the left side, and Nicole’s Honda starts on the right. Tom can’t just drive his Chevy out onto the right side. No, he has to put his Chevy in the driveway (the temporary storage), then put Nicole’s Honda in the left side, and finally move his Chevy to the right side.
Appending to an existing list
If you have an existing list and would like to add an item to the end, you can do that with the append function:
The syntax for append is probably not what you expected. You might have expected something like append(dwarfs, "Grumpy") , since append clearly needs two things: the variable referring to the list, dwarfs , and the data to append, "Grumpy" .
What’s the period doing in dwarfs.append("Grumpy") ? This syntax shows how we call a special kind of function called a method . Methods change things called objects . We’ll see objects in detail in an upcoming chapter, and this syntax for calling append indicates that lists are a kind of object. In this case, we are calling the method append on the list dwarfs , passing to the method the parameter "Grumpy" . If this discussion makes no sense to you now, fear not: it will. In the meantime, just think that the way to append an item, say new_item , to a list, say my_list , is to call my_list.append(new_item) .
Exercise: circle art
Objective: Append items to a list in response to user input.
Modify the following program so that it lets the user draw circles on the screen while dragging the mouse with the button pressed. Do not delete or in any other way prevent the clear() function from being called each time circle_art is called.
Inserting into and deleting from lists
There are a couple more list operations that you should know about.
You can insert an item into a list and delete an item from the list using the insert method and the del operation, as demonstrated in
To delete an item, you just write del followed by the name of the list and an index to the item you want to delete, so that the line del new_england[1] deletes the item at index 1. You can also delete a contiguous portion of a list, using colon-notation. In our example, del new_england[1:4] deletes the items starting at index 1 and going up to—but not including—index 4. In general, if you write del some_list[i:j] , you delete the items from index i through index j − 1 .
To insert into a list, you call the insert method. This method takes two parameters: the index of the item you want to insert before , and the item to insert. So when we call new_england.insert(3, "Newyorkachusetts") , we’re inserting before the item at index 3 ( "Vermont" ). If you give insert an index beyond the end of the list, as in new_england.insert(17, "New Brunswick") , then it’s just like an append operation.
We often use a loop to work with items of a list when programming. This section introduces a new type of loop, called a for-loop, that is particularly well suited to dealing with items of a list. Anything you can accomplish with a for-loop you can also accomplish with a while-loop, so we are not getting any more power out of for-loops. But we’ll see that they are easier to read and to type. Alternate, briefer ways of expressing the same thing in code are called syntactic sugar , because they’re just a sweet extra.
Before we get to for-loops, let’s look at another example with a while-loop. A particularly common case is when you would like to use each item in a list, but do not want to actually change the list. Here is an example of simply printing out all of the items of a list:
You might notice that in the above loop, the variable index is never used on its own; it’s a variable that is introduced solely for the purpose of getting items. You never print out index , you don’t compute anything with it, and you really don’t care what its value is. You just want to get each item of the moons list and print it. You also don’t really care about len(moons) . Yes, you need to use it to know when to terminate the while loop, but who cares what the actual value is? (I also introduced a variable moon to store the list item, and then worked with that temporary variable. There was no real reason not to just print out moons[index] directly, but this temporary variable will help clarify the explanation of for-loops.)
If you were describing the method for printing out the names of moons at a high level, you might say (in English) something like, “For every item of the moons list, print out that item.” You might not tell me about initializing index , about the mechanical detail of incrementing index each time through the loop, or about comparing index to len(moons) . Humans don’t think like that. We can hide these details with a for-loop.
A for-loop iterates over items in a list, making each item available in sequence. Here is an example, in moons_for.py , that acts identically to the example with the while-loop.
With a for-loop, you don’t need to create an index variable, increment the index variable, or compare the index variable to the length of the list. Python does that stuff for you internally, behind the scenes. There are a couple of key things to notice.
If you actually want the value of the index, you can’t determine it during the for-loop. You’ll need to use a while-loop.
You cannot change the moons list during a for-loop. Each item is copied into the temporary variable moon . You can change moon if you like, but that won’t change the list. If you want to change the list, you’ll need to use a while-loop.
Because for-loops are simpler and easier to read, you should use a for-loop in your code wherever the goal is to refer to the items of a list, but without changing the list. If you want to change which items the list contains, you should use a while-loop.
Using range to create a list of int values
Some functions return lists. For example, the built-in Python function range gives ints in a specified range. (By built-in, I mean that you don’t even have to import it from anywhere.) For example, range(3, 10) gives the list
Notice that the last number in the list is 9, not 10.
range can save you some typing if you want to count over integers. Here’s an example.
If you only give the range function just one parameter, then the list will start at 0:
By calling len and range , you can use a for-loop to index into a list. Then you can change the values in the list, since you have an index. For example, here are two ways to double each number in a list:
Exercise: bubbles
Objective: Access and modify lists in a for loop using an index variable.
The following program adds x and y coordinates to lists to represent bubbles in water. Write a for-loop that draws all of the bubbles, and moves every bubble upwards one pixel after it is drawn. Notice that since the bubbles are originally placed below the bottom of the screen, you will not see anything at first; your program might need to run for a while. For debugging, you could increase the frequency of bubbles and place them higher up on the screen.
You can treat a string like a list (sort of)
You can get a character from a string almost as though the string were a list:
You can even loop over characters in a string,
You might think you could change a character in a string this way too. It won’t work. mystring[3] = 'r' will give an error. Python strings are immutable ; their values cannot be changed.
If you wanted to do something like changing the third letter to r , you would have to build an entirely new string, using the old string and the new letter. We’ll see how to do that later in the course.
How it works: The list in memory
Consider when we first assign to a variable, such as x = 5 . Python knows that x is an integer, and Python knows that four bytes of memory are typically enough to store that integer. Python allocates those four bytes, and it copies a binary code corresponding to the value 5 into those bytes. We say that the value 5 is stored in the variable.
Something different happens when you create a list. Space in memory is allocated for the list somewhere other than at the variable’s address . The variable just stores the address of the list. Let’s look at an example:
Here is what happens. First, Python sees a list literal on the right-hand side of the equals sign. Python finds memory space for the list data ["Minnie", "Mickey", "Mighty"] in an area of memory called the heap . This new list now has an address. Let’s say the address of that list data is 1000.
Now Python looks at the left-hand side of the assignment operator. Python has to create a new variable, mice . Python copies the value 1000—the address of the list—into the variable mice . Here’s how you should think of it:
I drew the arrow to indicate that the variable mice is really just telling us that the list itself is somewhere else, in this case at address 1000.
Important note. It would be incorrect to say that the variable mice contains the list. In our earlier example, x = 5 , the variable x really did contain the value 5 (or at least its binary representation), not an address or anything else. For a list, however, the variable naming the list— mice in this example—contains only the address of the list data, not the list data itself.
We cannot store a list in a variable, because the list could have any size at all. But just like integer values, all addresses have the same size, so we know how much space in a variable to use for an address.
Aliasing and the assignment operator
Because the address of a list is stored in a variable, rather than actually storing the list data itself in the variable, an important property follows: any variable that stores that address can be used to change the list. Look at this example:
This program prints
Notice that ivies[3] is no longer 'Cornell' ; it has become 'Stanford' , even though we made the change to expensive_schools[3] , not to ivies[3] .
What happened here?
The assignment operator always copies the value of a variable. But in this case, the value of the variable ivies is some address (perhaps 2000), not the list itself. The list is not copied by the assignment operator in expensive_schools = ivies . Only the address is copied. There are not two different lists in the heap; there’s still just one list, but both ivies and expensive_schools hold its address:
Now, when the item at index 3 in the list whose address is held in expensive_schools is changed to "Stanford" , the one and only list at address 2000 changes:
I wrote the item at index 3 in italics just to highlight it. When we print the list using the ivies variable (which contains the address of the same list), we see that the list has changed.
You can think of it like this. Let’s say you have a savings account number. You can think of that number as the address used to refer to a pile of money in some bank vault somewhere. Now if the bank assigns me the same address (savings account number), then I can use that number to withdraw half of the money. When you go to look at the account, half of the money is gone.
When two names refer to the same thing, those names are called aliases of each other. Dr. Dre and Andre Ramelle Young both refer to the same person. Aliasing can be a source of tricky-to-find bugs. (You might view it as a bug if I withdrew half of your money from your savings account, and you didn’t even know that we had the same account number.)
For integers, floats, or booleans, the assignment operator makes a new copy of the data, not a copy of the address, so for these primitive types , changing the value of one variable never has an effect on the value of another variable. For strings, the assignment operator copies the address, but because strings are immutable , you’ll never notice that aliasing is occurring.
Why the difference between how primitive types and lists are treated? Lists can be long, and copying a long list can take a long time, relatively speaking. Copying the address of the list might be enough. We’ll see later that we can still tell Python that we really do want to copy the list itself, and not just the address, using a special function list .
Passing lists to functions (and aliasing)
If you pass the address of a list to a function, then the function can change the data at that address. The function does not change the value of the parameter which is passed to it, which is just some address of a list. But the data contained within the list itself is changed.
Exercise: function for reversing a list
Objective: Take an implementation of a list algorithm, and wrap it in a function that takes the list as a parameter.
Reversing a list seems like a useful thing to do; we already wrote the code to do it. Aliasing lets us move that code into a function. Write a function reverse_list that takes one parameter, the address of a list to reverse, and reverses that list in place. Test your function by calling reverse_list on a test list of numbers.
Copying a list with list
Sometimes, you really do want a copy of a list. You can use the function list to make a copy of a list. The list function copies all the data in the list into a new area in the heap, and returns the address of the new data.
Sorting a list with sorted
Python contains a useful built-in function, sorted , which makes a sorted copy of the original list, and returns that list. The sorted function does not change the original list. Look at this example:
Run it, and look at the output.
How does the sorted function work? Over the years, computer scientists have developed dozens of different algorithms for sorting. We’ll see a little later a simple sorting algorithm called selection sort .
Good programming practice: Lists should contain only data of similar types
It is possible to store different types of items in a single list. However, you should avoid doing so most of the time.
The problem is that if you want to apply some operation to all items of the list, you can’t be sure that it makes sense to do so. Imagine you tried to sort weird_list using the sorted function. What would the result be? It turns out Python will do it, but I just can’t remember or predict the behavior. It’s terrible programming practice to rely on surprising behavior that you cannot predict!
Loops with Lists
One great way to work with lists in Python is using a loop. Recall from an earlier lab that a for loop actually iterates over a list itself, and that the range() function is simply used to generate a list that the for loop can use. Likewise, while loops can also be used in a similar way.
Let’s look at a quick example of how we can iterate through the items in a list using a loop in Python.
Consider the following example program in Python:
In this example, the for loop will iterate through the values in the nums list instead of a given range. So, during the first iteration, i will store the value $ 3 $ , then $ 1 $ , then $ 4 $ , and so on until it reaches the end of the list. When the program is executed, we should receive this output:
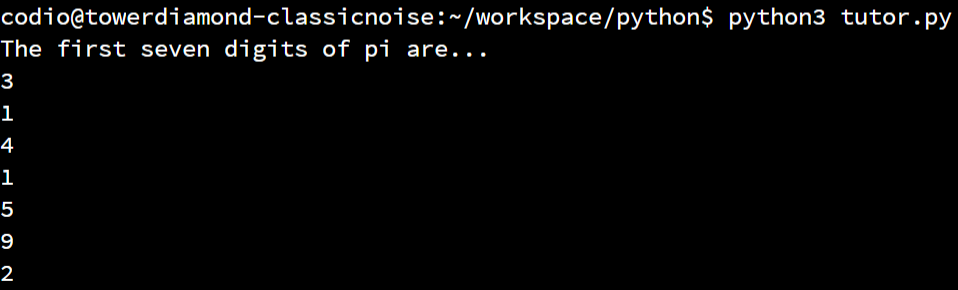
Using a list in a for loop is an excellent way to go through each element quickly, and it allows us to build for loops that don’t require the use of the range() function to generate a list that is in some sort of sequential order. We’ll see this pattern used very often in our programs using lists.
Finally, it is not recommended to edit the contents of the list while inside of the for loop using this method. Since we are only getting the individual values from each list element, we cannot easily make changes to the list itself without causing issues in the loop itself. Instead, if we want to make changes to the list while we are iterating through it, it is highly recommended to use a while loop structure as shown below.
It is also possible to iterate through a list in Python using a while loop. However, instead of iterating through the items themselves, we can use an iterator variable that references the indexes of the elements in the list. Consider this example program:
This program is effectively the same as the one above, except that it uses a while loop to iterate through the items in the nums list. We start by setting the iterator variable i to be $ 0 $ , the first index in the list. Then, in the while loop, we use the special len() function, which is used to find the size of the list. Since the list contains seven items, the length of the list is $ 7 $ . Another way to think about the length of the list is that it is always one more than the highest index - if the last item in the list is at index $ 6 $ , then the length of the list overall must be $ 7 $ .
Then, inside of the loop, we’ll use the iterator variable i inside of square brackets to access each individual element in the nums list and print it to the terminal. We’ll also need to remember to increment i by one each time.
This method is very useful if we want to do more with the list inside of our loop, such as edit individual elements or make changes to the overall structure of the list. This works because we can directly manipulate the value in the iterator variable i , and then use it to access individual elements in the list and update them. Also, if the size of the list changes, it will be checked using the len() function after each iteration of the while loop.
So, as a general rule, we should use a for loop when we just want to iterate through the list and not make any changes to the list while inside of the loop. If we do need to make changes, it is better to use a while loop and an iterator variable to access the list elements directly.
Last modified by: Russell Feldhausen Jun 7, 2023
My code as a for loop that don't work
I am a newbie and I had an exercise to do, but as I am trying to make a for loop that supposed to check all data in a .csv with the same “entity” as the user inputed to finally make an average of all life expectancy with the same entity over the years, the count is always = 0. (It’s on the country based research option, and the not working for loop is line 65 to 76)
Help I tried everything with my current knowledge:
(The rest of the code work it’s just for the context)
It is because you are consuming the f iterator twice . Or in Layman’s terms: when you open a file and use for ... in f then that loop goes through entire file and so when you start yet another loop on the same variable, then that loop has nothing to iterate (because it is at the end of a file).
You have 2 options:
- open a file twice
- “Be Kind To Rewind”: f.seek(0)
Oh ok thank you! I didn’t know that! You saved me!
Related Topics
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
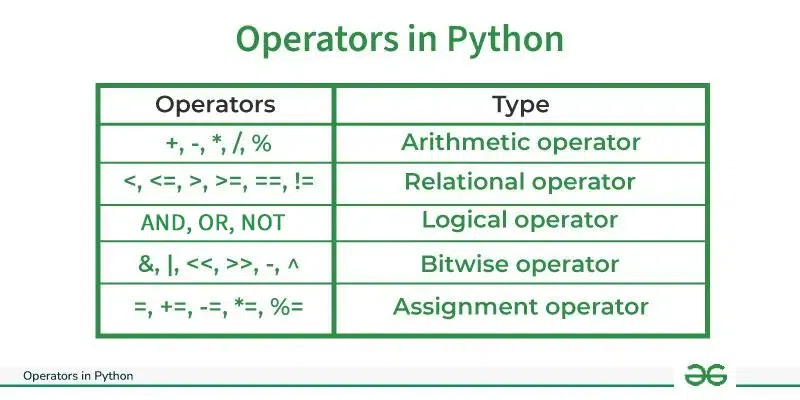
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
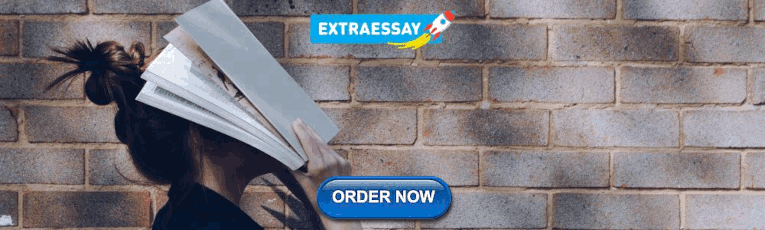
IMAGES
VIDEO
COMMENTS
print(x) (Note I expanded x += 3 to x = x + 3 to increase visibility for the name accesses - read and write.) First, you bind the list [1, 2, 3] to the name a. Then, you iterate over the list. During each iteration, the value is bound to the name x in the current scope. Your assignment then assigns another value to x.
7 Ways You Can Iterate Through a List in Python. 1. A Simple for Loop. Using a Python for loop is one of the simplest methods for iterating over a list or any other sequence (e.g. tuples, sets, or dictionaries ). Python for loops are a powerful tool, so it is important for programmers to understand their versatility.
Whenever you complete an action in the following list, Python runs an implicit assignment for you: Define or call a function; Define or instantiate a class; Use the current ... The memory address of control_variable changes on each iteration of the loop. This is because Python internally reassigns a new value from the loop iterable to the loop ...
To iterate over a list, you use the for loop statement as follows: for item in list: # process the item Code language: Python (python) In this syntax, the for loop statement assigns an individual element of the list to the item variable in each iteration. Inside the body of the loop, you can manipulate each list element individually.
This loop is interpreted as follows: Initialize i to 1.; Continue looping as long as i <= 10.; Increment i by 1 after each loop iteration.; Three-expression for loops are popular because the expressions specified for the three parts can be nearly anything, so this has quite a bit more flexibility than the simpler numeric range form shown above. These for loops are also featured in the C++ ...
Python For Loops. A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string).. This is less like the for keyword in other programming languages, and works more like an iterator method as found in other object-orientated programming languages.. With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc.
In Python, a for loop is used to iterate over sequences such as lists, strings, tuples, etc. languages = ['Swift', 'Python', 'Go'] # access elements of the list one by one for i in languages: print(i) Output. Swift Python Go. In the above example, we have created a list called languages. As the list has 3 elements, the loop iterates 3 times.
To get a clear idea about how a for loop works, I have provided 21 examples of using for loop in Python. You can go through these examples and understand the working of for loops in different scenarios. Let's dive right in. 1. Python for loop to iterate through the letters in a word. for i in "pythonista":
Auxiliary Space: O (1), as we're using additional space res other than the input list itself with the same size of input list. Method #2 : Using itemgetter () itemgetter function can also be used to perform this particular task. This function accepts the index values and the container it is working on and assigns to the variables.
Python List For Loop. Python List is a collection of items. For loop can be used to execute a set of statements for each of the element in the list. In this tutorial, we will learn how to use For loop to traverse through the elements of a given list. Syntax of List For Loop. In general, the syntax to iterate over a list using for loop is
Use nums[-1] to represent the last item in the list, since Python supports negative indexing :) Note: other solutions are more elegant, and this is not how I would solve the problem, but for a beginner it is sometimes easier to understand if working with simple constructs like for loop.
Syntax of for loop. for i in range/sequencee: statement 1 statement 2 statement n Code language: Python (python). In the syntax, i is the iterating variable, and the range specifies how many times the loop should run. For example, if a list contains 10 numbers then for loop will execute 10 times to print each number.; In each iteration of the loop, the variable i get the current value.
First, Python sees a list literal on the right-hand side of the equals sign. Python finds memory space for the list data ["Minnie", "Mickey", "Mighty"] in an area of memory called the heap. This new list now has an address. Let's say the address of that list data is 1000. Now Python looks at the left-hand side of the assignment operator.
In this tutorial, you used assignment expressions to make compact sections of Python code that assign values to variables inside of if statements, while loops, and list comprehensions. For more information on other assignment expressions, you can view PEP 572 —the document that initially proposed adding assignment expressions to Python.
Let's see all the different ways to iterate over a list in Python and the performance comparison between them. Using for loop. Using for loop and range () Using a while loop. Using list comprehension. Using enumerate () method. Using the iter function and the next function. Using the map () function.
Example with List, Tuple, String, and Dictionary Iteration Using for Loops in Python. We can use for loop to iterate lists, tuples, strings and dictionaries in Python. The code showcases different ways to iterate through various data structures in Python.
This program is effectively the same as the one above, except that it uses a while loop to iterate through the items in the nums list. We start by setting the iterator variable i to be $ 0 $, the first index in the list.Then, in the while loop, we use the special len() function, which is used to find the size of the list. Since the list contains seven items, the length of the list is $ 7 $.
1. I want to make an assignment to an item of a list in a single line for loop. First i have a list, where each list items are dictionary object. Then, i do for loop over each item of the list and compare if 'nodeid' field of dictionary is 106. If yes, i add new field to that dictionary. Code is below. K= []
Table of contents. Exercise 1: Print First 10 natural numbers using while loop. Exercise 2: Print the following pattern. Exercise 3: Calculate the sum of all numbers from 1 to a given number. Exercise 4: Write a program to print multiplication table of a given number. Exercise 5: Display numbers from a list using loop.
Hi, I am a newbie and I had an exercise to do, but as I am trying to make a for loop that supposed to check all data in a .csv with the same "entity" as the user inputed to finally make an average of all life expectancy with the same entity over the years, the count is always = 0. (It's on the country based research option, and the not working for loop is line 65 to 76) Help I tried ...
Computer Science CSCI 141: Computational Problem-Solving Spring 2024 Assignment 2: (10 points) Lists, Loops, and Numbering Systems Due: Sunday, February 11 th by 11:55pm to Blackboard Rules of Engagement: This is an open book, open computer, open notes, individual assignment. You cannot get assistance from any humans (besides DrChap and the section TA), AI tools, or previously completed ...
Assignment Operators in Python. Let's see an example of Assignment Operators in Python. Example: The code starts with 'a' and 'b' both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on 'b'.
If I call container_list [ index ][ 0 ] the first element of all nested lists is being referenced instead of the first element in successive nested lists. If I write the container_list manually then the for-loop works but because each nested list is recognised as inside_list, I cannot reference an element in a nested list.
We recommend you have a high school level of mathematics (functions, basic algebra) and familiarity with a programming language (loops, functions, if/else statements, lists/dictionaries, importing libraries). Assignments and labs are written in Python but the course introduces all the machine learning libraries you'll use. Applied Learning ...
3. This solution might be more complicated and not a simple list-comprehension but it is also more efficient (O (n) instead of O (n 2 )). The reason is that we no longer have go through the entire list D for every item e we get from C but only once. As a result, the benefits of this approach scale with increasing size of D or C.
The assignments and lectures in the new Specialization have been rebuilt to use Python rather than Octave, like in the original course. Before the graded programming assignments, there are additional ungraded code notebooks with sample code and interactive graphs to help you visualize what an algorithm is doing and make it easier to complete ...
is it possible to for-loop through a list with integers and use the += operator to add the integers to a variable with 0? eg: length = [3, 2] grades = [1, 1, 1, 5, 4] avg_grade = [] c = 0 d= 0 r =...