This page aims to provide an overview and some details on how to perform arithmetic between matrices, vectors and scalars with Eigen .
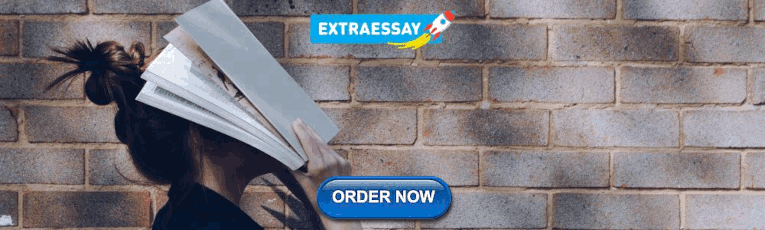
Introduction
Eigen offers matrix/vector arithmetic operations either through overloads of common C++ arithmetic operators such as +, -, *, or through special methods such as dot(), cross(), etc. For the Matrix class (matrices and vectors), operators are only overloaded to support linear-algebraic operations. For example, matrix1 * matrix2 means matrix-matrix product, and vector + scalar is just not allowed. If you want to perform all kinds of array operations, not linear algebra, see the next page .
Addition and subtraction
The left hand side and right hand side must, of course, have the same numbers of rows and of columns. They must also have the same Scalar type, as Eigen doesn't do automatic type promotion. The operators at hand here are:
- binary operator + as in a+b
- binary operator - as in a-b
- unary operator - as in -a
- compound operator += as in a+=b
- compound operator -= as in a-=b
Scalar multiplication and division
Multiplication and division by a scalar is very simple too. The operators at hand here are:
- binary operator * as in matrix*scalar
- binary operator * as in scalar*matrix
- binary operator / as in matrix/scalar
- compound operator *= as in matrix*=scalar
- compound operator /= as in matrix/=scalar
A note about expression templates
This is an advanced topic that we explain on this page , but it is useful to just mention it now. In Eigen , arithmetic operators such as operator+ don't perform any computation by themselves, they just return an "expression object" describing the computation to be performed. The actual computation happens later, when the whole expression is evaluated, typically in operator= . While this might sound heavy, any modern optimizing compiler is able to optimize away that abstraction and the result is perfectly optimized code. For example, when you do:
Eigen compiles it to just one for loop, so that the arrays are traversed only once. Simplifying (e.g. ignoring SIMD optimizations), this loop looks like this:
Thus, you should not be afraid of using relatively large arithmetic expressions with Eigen : it only gives Eigen more opportunities for optimization.
Transposition and conjugation
The transpose \( a^T \), conjugate \( \bar{a} \), and adjoint (i.e., conjugate transpose) \( a^* \) of a matrix or vector \( a \) are obtained by the member functions transpose() , conjugate() , and adjoint() , respectively.
For real matrices, conjugate() is a no-operation, and so adjoint() is equivalent to transpose() .
As for basic arithmetic operators, transpose() and adjoint() simply return a proxy object without doing the actual transposition. If you do b = a.transpose() , then the transpose is evaluated at the same time as the result is written into b . However, there is a complication here. If you do a = a.transpose() , then Eigen starts writing the result into a before the evaluation of the transpose is finished. Therefore, the instruction a = a.transpose() does not replace a with its transpose, as one would expect:
This is the so-called aliasing issue . In "debug mode", i.e., when assertions have not been disabled, such common pitfalls are automatically detected.
For in-place transposition, as for instance in a = a.transpose() , simply use the transposeInPlace() function:
There is also the adjointInPlace() function for complex matrices.
Matrix-matrix and matrix-vector multiplication
Matrix-matrix multiplication is again done with operator* . Since vectors are a special case of matrices, they are implicitly handled there too, so matrix-vector product is really just a special case of matrix-matrix product, and so is vector-vector outer product. Thus, all these cases are handled by just two operators:
- binary operator * as in a*b
- compound operator *= as in a*=b (this multiplies on the right: a*=b is equivalent to a = a*b )
Note: if you read the above paragraph on expression templates and are worried that doing m=m*m might cause aliasing issues, be reassured for now: Eigen treats matrix multiplication as a special case and takes care of introducing a temporary here, so it will compile m=m*m as:
If you know your matrix product can be safely evaluated into the destination matrix without aliasing issue, then you can use the noalias() function to avoid the temporary, e.g.:
For more details on this topic, see the page on aliasing .
Note: for BLAS users worried about performance, expressions such as c.noalias() -= 2 * a.adjoint() * b; are fully optimized and trigger a single gemm-like function call.
Dot product and cross product
For dot product and cross product, you need the dot() and cross() methods. Of course, the dot product can also be obtained as a 1x1 matrix as u.adjoint()*v.
Remember that cross product is only for vectors of size 3. Dot product is for vectors of any sizes. When using complex numbers, Eigen 's dot product is conjugate-linear in the first variable and linear in the second variable.
Basic arithmetic reduction operations
Eigen also provides some reduction operations to reduce a given matrix or vector to a single value such as the sum (computed by sum() ), product ( prod() ), or the maximum ( maxCoeff() ) and minimum ( minCoeff() ) of all its coefficients.
The trace of a matrix, as returned by the function trace() , is the sum of the diagonal coefficients and can also be computed as efficiently using a.diagonal().sum() , as we will see later on.
There also exist variants of the minCoeff and maxCoeff functions returning the coordinates of the respective coefficient via the arguments:
Validity of operations
Eigen checks the validity of the operations that you perform. When possible, it checks them at compile time, producing compilation errors. These error messages can be long and ugly, but Eigen writes the important message in UPPERCASE_LETTERS_SO_IT_STANDS_OUT. For example:
Of course, in many cases, for example when checking dynamic sizes, the check cannot be performed at compile time. Eigen then uses runtime assertions. This means that the program will abort with an error message when executing an illegal operation if it is run in "debug mode", and it will probably crash if assertions are turned off.
For more details on this topic, see this page .

Browse Course Material
Course info.
- Prof. Gilbert Strang
Departments
- Mathematics
As Taught In
- Linear Algebra
Learning Resource Types
Lecture 21: eigenvalues and eigenvectors.
- Download video
- Download transcript

You are leaving MIT OpenCourseWare

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
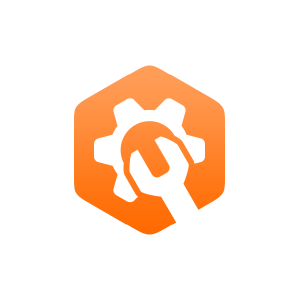
10.3: Eigenvalues and Eigenvectors
- Last updated
- Save as PDF
- Page ID 22501
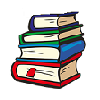
- Peter Woolf et al.
- University of Michigan
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
What are Eigenvectors and Eigenvalues?

Definition: Eigenvector and Eigenvalues
- An Eigenvector is a vector that maintains its direction after undergoing a linear transformation.
- An Eigenvalue is the scalar value that the eigenvector was multiplied by during the linear transformation.
Eigenvectors and Eigenvalues are best explained using an example. Take a look at the picture below.
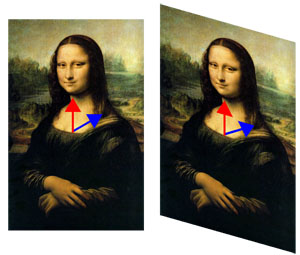
In the left picture, two vectors were drawn on the Mona Lisa. The picture then under went a linear transformation and is shown on the right. The red vector maintained its direction; therefore, it’s an eigenvector for that linear transformation. The blue vector did not maintain its director during the transformation; thus, it is not an eigenvector. The eigenvalue for the red vector in this example is 1 because the arrow was not lengthened or shortened during the transformation. If the red vector, on the right, were twice the size than the original vector then the eigenvalue would be 2. If the red vector were pointing directly down and remained the size in the picture, the eigenvalue would be -1.
Now that you have an idea of what an eigenvector and eigenvalue are we can start talking about the mathematics behind them.
Fundamental Equation
The following equation must hold true for Eigenvectors and Eigenvalues given a square matrix \(\mathrm{A}\):
\[\mathrm{A} \cdot \mathrm{v}=\lambda \cdot \mathrm{v} \label{eq1} \]
- \(\mathrm{A}\) is a square matrix
- \(\mathrm{v}\) is the Eigenvector
- \(\lambda\) is the Eigenvalue
Let's go through a simple example so you understand the fundamental equation better.
Example \(\PageIndex{1}\)
Is \(\mathbf{v}\) an eigenvector with the corresponding \(λ = 0\) for the matrix \(\mathbf{A}\)?
\[\mathbf{v}=\left[\begin{array}{c} 1 \\ -2 \end{array}\right] \nonumber \nonumber \]
\[\mathbf{A}=\left[\begin{array}{cc} 6 & 3 \\ -2 & -1 \end{array}\right] \nonumber \nonumber \]
\[\begin{align*} A \cdot \mathbf{v} &= \lambda \cdot \mathbf{v} \\[4pt] \left[\begin{array}{cc} 6 & 3 \\ -2 & -1 \end{array}\right] \cdot\left[\begin{array}{c} 1 \\ -2 \end{array}\right] &=0\left[\begin{array}{c} 1 \\ -2 \end{array}\right] \\[4pt] \left[\begin{array}{l} 0 \\ 0 \end{array}\right] &=\left[\begin{array}{l} 0 \\ 0 \end{array}\right] \end{align*}\nonumber \]
Therefore, it is true that \(\mathbf{v}\) and \(λ = 0\) are an eigenvector and eigenvalue respectively, for \(\mathbf{A}\). (See section on Matrix operations, i.e. matrix multiplication)
Calculating Eigenvalues and Eigenvectors
Calculation of the eigenvalues and the corresponding eigenvectors is completed using several principles of linear algebra. This can be done by hand, or for more complex situations a multitude of software packages (i.e. Mathematica) can be used. The following discussion will work for any n x n matrix; however for the sake of simplicity, smaller and more manageable matrices are used. Note also that throughout this article, boldface type is used to distinguish matrices from other variables.
Linear Algebra Review
For those who are unfamiliar with linear algebra, this section is designed to give the necessary knowledge used to compute the eigenvalues and eigenvectors. For a more extensive discussion on linear algebra, please consult the references.
Basic Matrix Operations
An m x n matrix A is a rectangular array of \(mn\) numbers (or elements) arranged in horizontal rows ( m ) and vertical columns ( n ):
\[\boldsymbol{A}=\left[\begin{array}{lll} a_{11} & a_{1 j} & a_{1 n} \\ a_{i 1} & a_{i j} & a_{i n} \\ a_{m 1} & a_{m j} & a_{m n} \end{array}\right]\nonumber \]
To represent a matrix with the element aij in the i th row and j th column, we use the abbreviation A = [ aij ]. Two m x n matrices A = [ aij ] and B = [ bij ] are said to be equal if corresponding elements are equal.
Addition and subtraction
We can add A and B by adding corresponding elements:
\[A + B = [a_{ij}] + [b_{ij}] = [a_{ij} + b_{ij}\nonumber \]
This will give the element in row i and column j of C = A + B to have
\[c_{ij} = a_{ij} + b_{ij}.\nonumber \]
More detailed addition and subtraction of matrices can be found in the example below.
\[\left[\begin{array}{ccc} 1 & 2 & 6 \\ 4 & 5 & 10 \\ 5 & 3 & 11 \end{array}\right]+\left[\begin{array}{ccc} 8 & 3 & 5 \\ 5 & 4 & 4 \\ 3 & 0 & 6 \end{array}\right]=\left[\begin{array}{ccc} 1+8 & 2+3 & 6+5 \\ 4+5 & 5+4 & 10+4 \\ 5+3 & 3+0 & 11+6 \end{array}\right]=\left[\begin{array}{ccc} 9 & 5 & 11 \\ 9 & 9 & 14 \\ 8 & 3 & 17 \end{array}\right]\nonumber \]
Multiplication
Multiplication of matrices are NOT done in the same manner as addition and subtraction. Let's look at the following matrix multiplication:
\[A \times B=C\nonumber \]
\(A\) is an \(m \times n\) matrix, \(B\) is an \(n \times p\) matrix, and \(C\) is an \(m \times p\) matrix. Therefore the resulting matrix, \(C\), has the same number of rows as the first matrix and the same number of columns as the second matrix. Also the number of columns in the first is the same as the number of rows in the second matrix.
The value of an element in C (row i, column j) is determined by the general formula:
\[c_{i, j}=\sum_{k=1}^{n} a_{i, k} b_{k, j}\nonumber \]
\[\begin{align*} \left[\begin{array}{ccc} 1 & 2 & 6 \\ 4 & 5 & 10 \\ 5 & 3 & 11 \end{array}\right]\left[\begin{array}{cc} 3 & 0 \\ 0 & 1 \\ 5 & 1 \end{array}\right] &=\left[\begin{array}{cc} 1 \times 3+2 \times 0+6 \times 5 & 1 \times 0+2 \times 1+6 \times 1 \\ 4 \times 3+5 \times 0+10 \times 5 & 4 \times 0+5 \times 1+10 \times 1 \\ 5 \times 3+3 \times 0+11 \times 5 & 5 \times 0+3 \times 1+11 \times 1 \end{array}\right] \\[4pt] &=\left[\begin{array}{cc} 33 & 8 \\ 62 & 15 \\ 70 & 14 \end{array}\right]\end{align*} \nonumber \]
It can also be seen that multiplication of matrices is not commutative ( A B ≠ B A ). Multiplication of a matrix by a scalar is done by multiplying each element by the scalar. c A = A c =[ caij ]
\[2\left[\begin{array}{ccc} 1 & 2 & 6 \\ 4 & 5 & 10 \\ 5 & 3 & 11 \end{array}\right]=\left[\begin{array}{ccc} 2 & 4 & 12 \\ 8 & 10 & 20 \\ 10 & 6 & 22 \end{array}\right]\nonumber \]
Identity Matrix
The identity matrix is a special matrix whose elements are all zeroes except along the primary diagonal, which are occupied by ones. The identity matrix can be any size as long as the number of rows equals the number of columns.
\[\mathbf{I}=\left[\begin{array}{llll} 1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 1 & 0 \\ 0 & 0 & 0 & 1 \end{array}\right]\nonumber \]
Determinant
The determinant is a property of any square matrix that describes the degree of coupling between equations. For a 2x2 matrix the determinant is:
\[\operatorname{det}(\mathbf{A})=\left|\begin{array}{ll} a & b \\ c & d \end{array}\right|=a d-b c\nonumber \]
Note that the vertical lines around the matrix elements denotes the determinant. For a 3x3 matrix the determinant is:
\[\operatorname{det}(\mathbf{A})=\left|\begin{array}{lll} a & b & c \\ d & e & f \\ g & h & i \end{array}\right|=a\left|\begin{array}{cc} e & f \\ h & i \end{array}\right|-b\left|\begin{array}{cc} d & f \\ g & i \end{array}\right|+c\left|\begin{array}{cc} d & e \\ g & h \end{array}\right|=a(e i-f h)-b(d i-f g)+c(d h-e g)\nonumber \]
Larger matrices are computed in the same way where the element of the top row is multiplied by the determinant of matrix remaining once that element’s row and column are removed. Terms where the top elements in odd columns are added and terms where the top elements in even rows are subtracted (assuming the top element is positive). For matrices larger than 3x3 however; it is probably quickest to use math software to do these calculations since they quickly become more complex with increasing size.
Solving for Eigenvalues and Eigenvectors
The eigenvalues (λ) and eigenvectors ( v ), are related to the square matrix A by the following equation. (Note: In order for the eigenvalues to be computed, the matrix must have the same number of rows as columns.)
\[(\mathbf{A}-\lambda \mathbf{I}) \cdot \mathbf{v}=0\nonumber \]
This equation is just a rearrangement of the Equation \ref{eq1}. To solve this equation, the eigenvalues are calculated first by setting det( A -λ I ) to zero and then solving for λ. The determinant is set to zero in order to ensure non-trivial solutions for v , by a fundamental theorem of linear algebra.
\[A=\left[\begin{array}{lll} 4 & 1 & 4 \\ 1 & 7 & 1 \\ 4 & 1 & 4 \end{array}\right]\nonumber \]
\[A-\lambda I=\left[\begin{array}{lll} 4 & 1 & 4 \\ 1 & 7 & 1 \\ 4 & 1 & 4 \end{array}\right]+\left[\begin{array}{ccc} -\lambda & 0 & 0 \\ 0 & -\lambda & 0 \\ 0 & 0 & -\lambda \end{array}\right]\nonumber \]
\[\operatorname{det}(A-\lambda I)=\left|\begin{array}{ccc} 4-\lambda & 1 & 4 \\ 1 & 7-\lambda & 1 \\ 4 & 1 & 4-\lambda \end{array}\right|=0\nonumber \]
\[\begin{array}{l} -54 \lambda+15 \lambda^{2}-\lambda^{3}=0 \\ -\lambda(\lambda-6)(\lambda-9)=0 \\ \lambda=0,6,9 \end{array}\nonumber \]
For each of these eigenvalues, an eigenvector is calculated which will satisfy the equation ( A -λ I ) v =0 for that eigenvalue. To do this, an eigenvalue is substituted into A -λ I , and then the system of equations is used to calculate the eigenvector. For \(λ = 6\)
\[(\mathbf{A}-6 \mathbf{I}) \mathbf{v}=\left[\begin{array}{ccc} 4-6 & 1 & 4 \\ 1 & 7-6 & 1 \\ 4 & 1 & 4-6 \end{array}\right]\left[\begin{array}{l} x \\ y \\ z \end{array}\right]=\left[\begin{array}{ccc} -2 & 1 & 4 \\ 1 & 1 & 1 \\ 4 & 1 & -2 \end{array}\right]\left[\begin{array}{l} x \\ y \\ z \end{array}\right]=0\nonumber \]

Calculating Eigenvalues and Eigenvectors using Numerical Software
Eigenvalues in mathematica.
For larger matrices (4x4 and larger), solving for the eigenvalues and eigenvectors becomes very lengthy. Therefore software programs like Mathematica are used. The example from the last section will be used to demonstrate how to use Mathematica. First we can generate the matrix A . This is done using the following syntax:
- \(A = \{\{4,1,4\},\{1,7,1\},\{4,1,4\}\}\)
It can be seen that the matrix is treated as a list of rows. Elements in the same row are contained in a single set of brackets and separated by commas. The set of rows are also contained in a set of brackets and are separated by commas. A screenshot of this is seen below. (Note: The "MatrixForm[]" command is used to display the matrix in its standard form. Also in Mathematica you must hit Shift + Enter to get an output.)
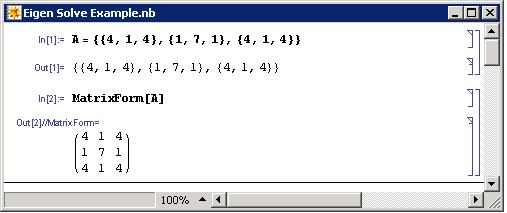
Next we find the determinant of matrix A -λ I , by first subtracting the matrix λ I from A (Note: This new matrix, A -λ I , has been called A2).
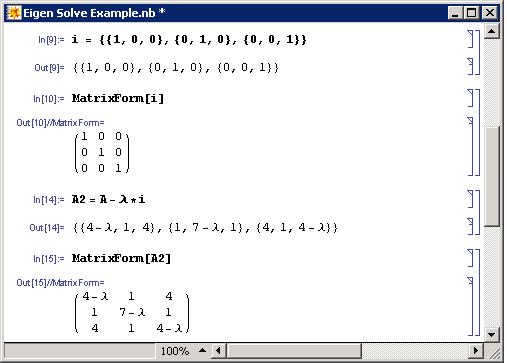
The command to find the determinant of a matrix A is:
For our example the result is seen below. By setting this equation to 0 and solving for λ, the eigenvalues are found. The Solve[] function is used to do this. Notice in the syntax that the use of two equal signs (==) is used to show equivalence whereas a single equal sign is used for defining a variable.
- Solve[{set of equations},{variables being solved}]
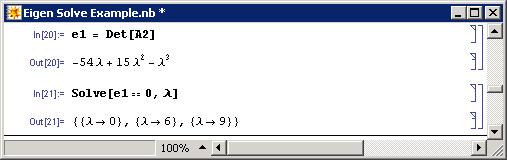
Alternatively the eigenvalues of a matrix A can be solved with the Mathematica Eigenvalue[] function:
- Eigenvalues[A]
Note that the same results are obtained for both methods.

To find the eigenvectors of a matrix A , the Eigenvector[] function can be used with the syntax below.
- Eigenvectors[A]
The eigenvectors are given in order of descending eigenvalues.

One more function that is useful for finding eigenvalues and eigenvectors is Eigensystem[]. This function is called with the following syntax.
- Eigensystem[A]
In this function, the first set of numbers are the eigenvalues, followed by the sets of eigenvectors in the same order as their corresponding eigenvalues.

The Mathematica file used to solve the example can be found at this link.Media:Eigen Solve Example.nb
Microsoft Excel
Microsoft Excel is capable of solving for Eigenvalues of symmetric matrices using its Goal Seek function. A symmetric matrix is a square matrix that is equal to its transpose and always has real, not complex, numbers for Eigenvalues. In many cases, complex Eigenvalues cannot be found using Excel. Goal Seek can be used because finding the Eigenvalue of a symmetric matrix is analogous to finding the root of a polynomial equation. The following procedure describes how to calculate the Eigenvalue of a symmetric matrix in the Mathematica tutorial using MS Excel.
(1) Input the values displayed below for matrix A then click menu INSERT-NAME-DEFINE “matrix_A” to name the matrix.
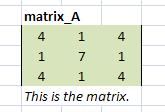
(2) Similarly, define identity matrix I by entering the values displayed below then naming it “matrix_I.”
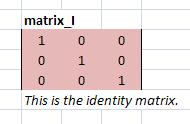
(3) Enter an initial guess for the Eigenvalue then name it “lambda.”

(4) In an empty cell, type the formula =matrix_A-lambda*matrix_I. Highlight three cells to the right and down, press F2, then press CRTL+SHIFT+ENTER. Name this matrix “matrix_A_lambda_I.”
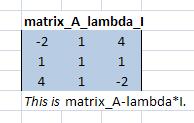
(5) In another cell, enter the formula =MDETERM(matrix_A_lambda_I). This is the determinant formula for matrix_A_lambda_I.

(6) Click menu Tools-Goal Seek… and set the cell containing the determinant formula to zero by changing the cell containing lambda.
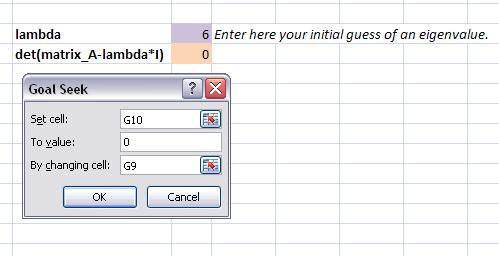
(7) To obtain all three Eigenvalues for matrix A, re-enter different initial guesses. Excel calculates the Eigenvalue nearest to the value of the initial guess. The Eigenvalues for matrix A were determined to be 0, 6, and 9. For instance, initial guesses of 1, 5, and 13 will lead to Eigenvalues of 0, 6, and 9, respectively.
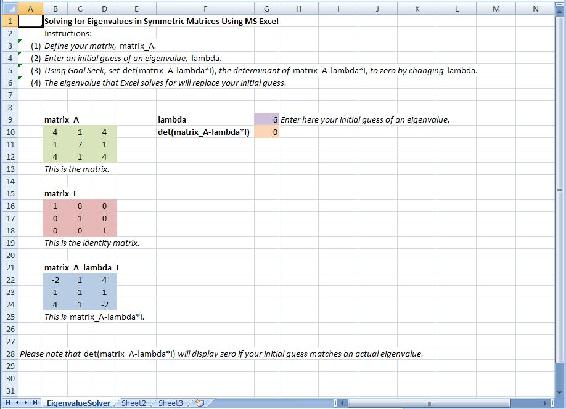
The MS Excel spreadsheet used to solve this problem, seen above, can be downloaded from this link: Media:ExcelSolveEigenvalue.xls.
Chemical Engineering Applications
The eigenvalue and eigenvector method of mathematical analysis is useful in many fields because it can be used to solve homogeneous linear systems of differential equations with constant coefficients. Furthermore, in chemical engineering many models are formed on the basis of systems of differential equations that are either linear or can be linearized and solved using the eigenvalue eigenvector method. In general, most ODEs can be linearized and therefore solved by this method. Linearizing ODEs For example, a PID control device can be modeled with ODEs that may be linearized where the eigenvalue eigenvector method can then be implemented. If we have a system that can be modeled with linear differential equations involving temperature, pressure, and concentration as they change with time, then the system can be solved using eigenvalues and eigenvectors:
\[\frac{d P}{d t}=4 P-4 T+C\nonumber \]
\[\frac{d T}{d t}=4 P-T+3 C\nonumber \]
\[\frac{d C}{d t}=P+5 T-C\nonumber \]
Note: This is not a real model and simply serves to introduce the eigenvalue and eigenvector method.

\[\mathbf{A}=\left[\begin{array}{ccc} 4 & -4 & 1 \\ 4 & -1 & 3 \\ 1 & 5 & -1 \end{array}\right]\nonumber \]
It is noteworthy that matrix A is only filled with constants for a linear system of differential equations. This turns out to be the case because each matrix component is the partial differential of a variable (in this case P, T, or C). It is this partial differential that yields a constant for linear systems. Therefore, matrix A is really the Jacobian matrix for a linear differential system.
Now, we can rewrite the system of ODE's above in matrix form.

\[\mathbf{x}(t)=\left[\begin{array}{l} P(t) \\ T(t) \\ C(t) \end{array}\right]\nonumber \]
We guess trial solutions of the form
\[\mathbf{x}=\mathbf{v} e^{\lambda t}\nonumber \]
since when we substitute this solution into the matrix equation, we obtain
\[\lambda \mathbf{v} e^{\lambda t}=\mathbf{A} \mathbf{v} e^{\lambda t}\nonumber \]
After cancelling the nonzero scalar factor e λt , we obtain the desired eigenvalue problem.
\[\mathbf{A} \mathbf{v}=\lambda \mathbf{v}\nonumber \]
Thus, we have shown that
will be a nontrivial solution for the matrix equation as long as v is a nonzero vector and λ is a constant associated with v that satisfies the eigenvalue problem.
In order to solve for the eigenvalues and eigenvectors, we rearrange the Equation \ref{eq1} to obtain the following:
\[\left(\begin{array}{lllll} \boldsymbol{\Lambda} & \lambda \mathbf{I}) \mathbf{v}=0 & & {\left[\begin{array}{ccc} 4-\lambda & -4 & 1 \\ 4 & 1 & \lambda & 3 \\ 1 & 5 & -1-\lambda \end{array}\right] \cdot\left[\begin{array}{l} x \\ y \\ z \end{array}\right]=0} \end{array}\right.\nonumber \]
For nontrivial solutions for v , the determinant of the eigenvalue matrix must equal zero, \(\operatorname{det}(\mathbf{A}-\lambda \mathbf{I})=0\). This allows us to solve for the eigenvalues, λ. You should get, after simplification, a third order polynomial, and therefore three eigenvalues. (see section on Solving for Eigenvalues and Eigenvectors for more details) Using the calculated eignvalues, one can determine the stability of the system when disturbed (see following section).

The solution will look like the following:
\[\left[\begin{array}{l} P(t) \\ T(t) \\ C(t) \end{array}\right]=c_{1}\left[\begin{array}{l} x_{1} \\ y_{1} \\ z_{1} \end{array}\right] e^{\lambda_{1} t}+c_{2}\left[\begin{array}{l} x_{2} \\ y_{2} \\ z_{2} \end{array}\right] e^{\lambda_{2} t}+c_{3}\left[\begin{array}{l} x_{3} \\ y_{3} \\ z_{3} \end{array}\right] e^{\lambda_{3} t}\nonumber \] where x 1 , x 2 , x 3 , y 1 , y 2 , y 3 , z 1 , z 2 , z 3 are all constants from the three eigenvectors. The general solution is a linear combination of these three solution vectors because the original system of ODE's is homogeneous and linear. It is homogeneous because the derivative expressions have no cross terms, such as PC or TC , and no dependence on t . It is linear because the derivative operator is linear. To solve for c 1 , c 2 , c 3 there must be some given initial conditions (see Worked out Example 1). This Wiki does not deal with solving ODEs. It only deals with solving for the eigenvalues and eigenvectors. In Mathematica the Dsolve[] function can be used to bypass the calculations of eigenvalues and eigenvectors to give the solutions for the differentials directly. See Using eigenvalues and eigenvectors to find stability and solve ODEs for solving ODEs using the eigenvalues and eigenvectors method as well as with Mathematica. This section was only meant to introduce the topic of eigenvalues and eigenvectors and does not deal with the mathematical details presented later in the article.
Using Eigenvalues to Determine Effects of Disturbing a System
Eigenvalues can help determine trends and solutions with a system of differential equations. Once the eigenvalues for a system are determined, the eigenvalues can be used to describe the system’s ability to return to steady-state if disturbed.
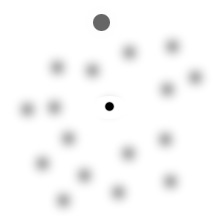
The above picture is of a plinko board with only one nail position known. Without knowing the position of the other nails, the Plinko disk's fall down the wall is unpredictable.
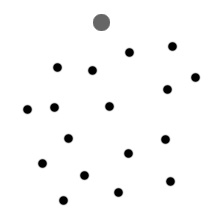
Knowing the placement of all of the nails on this Plinko board allows the player to know general patterns the disk might follow.
Repeated Eigenvalues
A final case of interest is repeated eigenvalues. While a system of \(N\) differential equations must also have \(N\) eigenvalues, these values may not always be distinct. For example, the system of equations:
\[\begin{align*} &\frac{d C_{A}}{d t}=f_{A, in} \rho C_{A}=f_{out} \rho C_{A} \sqrt{V_{1}}-V_{1} k_{1} C_{A} C_{B}\\[4pt] &\frac{d C_{B}}{d t}=f_{B, in} \rho C_{B in}-f_{out} \rho C_{B} \sqrt{V_{1}}-V_{1} k_{1} C_{A} C_{B}\\[4pt] &\frac{d C_{C}}{d t}=-f_{out} \rho C_{c} \sqrt{V_{1}}+V_{1} k_{1} C_{A} C_{B}\\[4pt] &\frac{d V_{1}}{d t}=f_{A, in}+f_{B, in}-f_{out} \sqrt{V_{1}}\\[4pt] &\frac{d V_{2}}{d t}=f_{out} \sqrt{V_{1}}-f_{customer} \sqrt{V_{2}}\\[4pt] &\frac{d C_{C 2}}{d t}=f_{\text {out}}, \rho C_{C} \sqrt{V_{1}}-f_{\text {customer}}, \rho C_{\mathrm{C} 2} \sqrt{V_{2}} \end{align*} \nonumber \]
May yield the eigenvalues: {-82, -75, -75, -75, -0.66, -0.66}, in which the roots ‘-75’ and ‘-0.66’ appear multiple times. Repeat eigenvalues bear further scrutiny in any analysis because they might represent an edge case, where the system is operating at some extreme. In mathematical terms, this means that linearly independent eigenvectors cannot be generated to complete the matrix basis without further analysis. In “real-world” engineering terms, this means that a system at an edge case could distort or fail unexpectedly.
However, for the general solution:
\[Y(t)=k_{1} \exp (\lambda t) V_{1}+k_{2} \exp (\lambda t)\left(t V_{1}+V_{2}\right)\nonumber \]
If \(λ < 0\), as \(t\) approaches infinity, the solution approaches 0, indicating a stable sink, whereas if λ > 0, the solution approaches infinity in the limit, indicating an unstable source. Thus the rules above can be roughly applied to repeat eigenvalues, that the system is still likely stable if they are real and less than zero and likely unstable if they are real and positive. Nonetheless, one should be aware that unusual behavior is possible. This course will not concern itself with resultant behavior of repeat eigenvalues, but for further information, see:
- http://math.rwinters.com/S21b/supplements/newbasis.pdf
- http://www.sosmath.com/diffeq/system/linear/eigenvalue/repeated/repeated.html
Your immediate supervisor, senior engineer Captain Johnny Goonewadd, has brought you in on a project dealing with a new silcone-based sealant that is on the ground level of research. Your job is to characterize the thermal expansion of the sealant with time given a constant power supply. Luckily, you were given a series of differential equations that relate temperature and volume in terms of one another with respect to time (Note: T and V are both dimensionless numbers with respect to their corresponding values at t=0). Solve the system of differentials and determine the equations for both Temperature and Volume in terms of time.
You are given the initial condition at time t=0, T=1 and V=1
\[\frac{d T}{d t}=4 T-3 V\nonumber \]
\[\frac{d V}{d t}=3 T+4 V\nonumber \]
By defining a matrix for both the coefficients and dependant variables we are able to rewrite the above series of differentials in matrix form
\[A=\left[\begin{array}{cc} 4 & -3 \\ 3 & 4 \end{array}\right]\nonumber \]
\[X=\left[\begin{array}{l} T \\ V \end{array}\right]\nonumber \]
\[A * X=\left[\begin{array}{l} \frac{d T}{d V} \\ \frac{d V}{d t} \end{array}\right]\nonumber \]
Lambda is inserted into the A matrix to determine the Eigenvalues

For each eigenvalue, we must find the eigenvector. Let us start with λ 1 = 4 − 3 i

Now we find the eigenvector for the eigenvalue λ 2 = 4 + 3 i
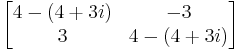
The general solution is in the form

A mathematical proof, Euler's formula, exists for transforming complex exponentials into functions of sin(t) and cos(t)

Simplifying

Since we already don't know the value of c 1 , let us make this equation simpler by making the following substitution

Thus, we get have our solution in terms of real numbers

Or, rewriting the solution in scalar form

Now that we have our solutions, we can use our initial conditions to find the constants c 3 and c 4
First initial condition: t=0, T=1

First initial condition: t=0, V=1

We have now arrived at our solution

See Using eigenvalues and eigenvectors to find stability and solve ODEs_Wiki for solving ODEs using the eigenvalues and eigenvectors.
Example \(\PageIndex{2}\)
Process Engineer, Dilbert Pickel, has started his first day for the Helman's Pickel Brine Factory. His first assignment is with a pre-startup team formulated to start up a new plant designed to make grousley sour pickle brine. Financial constraints have demanded that the process begin to produce good product as soon as possible. However, you are forced to reflux the process until you reach the set level of sourness. You have equations that relate all of the process variable in terms of one another with respect to time. Therefore, it is Dill Pickles job to characterize all of the process variables in terms of time (dimensionless Sourness, Acidity, and Water content; S, A, & W respectively). Below is the set of differentials that will be used to solve the equation.
\[\\begin{array}{l} \frac{d S}{d t}=S+A+10 W \\ \frac{d A}{d t}=S+5 A+2 W \\ \frac{d W}{d t}=4 S+3 A+8 W \end{array}]
Thus the coefficient matrix
\[\mathbf{A}=\left[\begin{array}{lll} 1 & 1 & 10 \\ 1 & 5 & 2 \\ 4 & 3 & 8 \end{array}\right]\nonumber \]
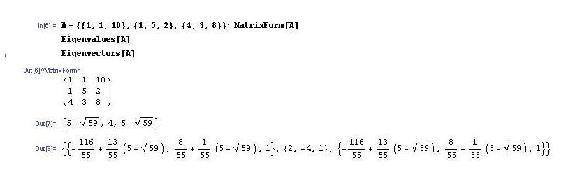
The eigenvectors can then be used to determine the final solution to the system of differentials. Some data points will be necessary in order to determine the constants.
\[\left[\begin{array}{l} S \\ A \\ W \end{array}\right]=C_{1}\left[\begin{array}{c} 0.88 \\ 0.38 \\ 1 \end{array}\right] e^{(5+\sqrt{89} k}+C_{2}\left[\begin{array}{c} 2 \\ -4 \\ 1 \end{array}\right] e^{4 t}+C_{3}\left[\begin{array}{c} -2.74 \\ 0.10 \\ 1 \end{array}\right]\nonumber \]
Example \(\PageIndex{3}\)
It is possible to find the Eigenvalues of more complex systems than the ones shown above. Doing so, however, requires the use of advanced math manipulation software tools such as Mathematica. Using Mathematica, it is possible to solve the system of ODEs shown below.
\[\begin{aligned} \frac{d X}{d t} &=8 X+\frac{10 X Y F}{X+Z} \\ \frac{d Y}{d t} &=4 F-Y-Z-\frac{3 X Y}{X+Y} \\ \frac{d Z}{d t} &=9 X-2 Z+F \end{aligned}\nonumber \]
Obviously, this system of ODEs has 4 variables and only 3 equations.
Obviously, this is a more complex set of ODEs than the ones shown above. And even though they will create a more complex set of Eigenvalues, they are solved for in the same way when using Mathematica.
Using the code shown below:
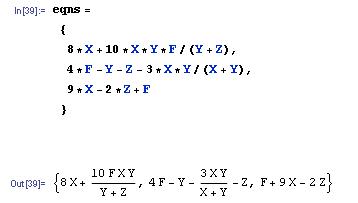
The equations can be entered into Mathematica. The equations are shown again in the output
Then, using the next bit of code:
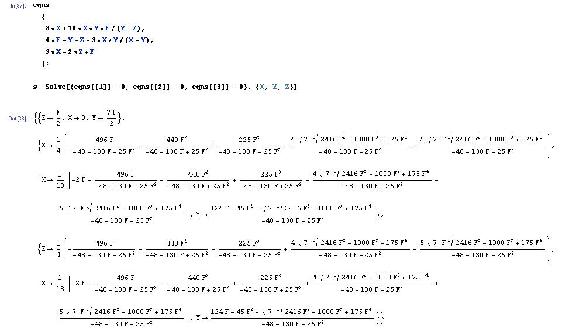
The it is possible to find where the equations are equal to 0 (i.e. the fixed points). The results of this is also shown in the image above. It's notable that 3 solutions are found. This makes sense as the system is 3 ODEs.
The Jacobian can then be found by simply using the code shown below.
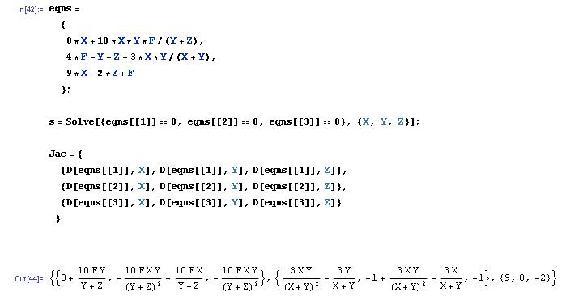
The ersults of finding the Jacobian are shown in the equation above.
Finally, to find one of the Eigenvalues, one can simply use the code shown below.
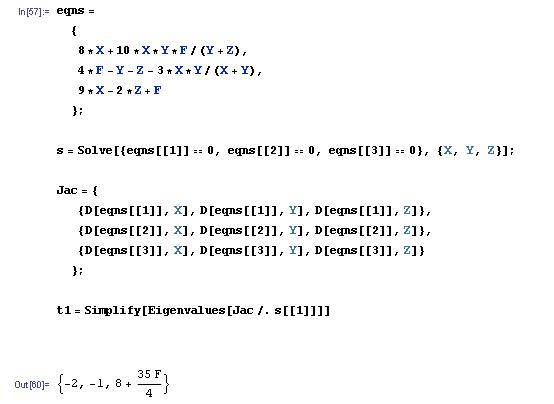
This gives the Eigenvalue when the first fixed point (the first solution found for "s") is applied. The other two solutions could be found by simply changing the fixed blade that is referred to when finding t1. The other Eigenvalues are not shown because of their large size.
Exercise \(\PageIndex{1}\): Calculating Eigenvalues and Eigenvectors using Numerical Software
What are the eigenvalues for the matrix A ?
\[\mathbf{A}=\left[\begin{array}{cc} 4 & 2 \\ 3 & -1 \end{array}\right]\nonumber \]
- \(\lambda_{1}=-2\) and \(\lambda_{1}=5\)
- \(\lambda_{1}=2\) and \(\lambda_{1}=-5\)
- \(\lambda_{1}=2\) and \(\lambda_{1}=5\)
- \(\lambda_{1}=-2\) and \(\lambda_{1}=-5\)
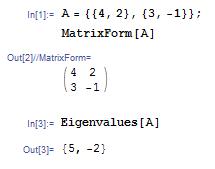
Exercise \(\PageIndex{2}\): Using Eigenvalues to Determine Effects of Disturbing a System
When a differential system with a real negative eigenvalue is disturbed, the system is...
- Driven away from the steady state value
- Unchanged and remains at the disturbed value
- Driven back to the steady state value
- Unpredictable and the effects can not be determined
c. A real negative eigenvalue is indicative of a stable system that will return to the steady state value after it is disturbed.
- Kravaris, Costas: Chemical Process Control: A Time-Domain Approach. Ann Arbor: The University of Michigan, pp 1-23, A.1-A.7.
- Bhatti, M. Asghar: Practical Optimization Methods with Mathematica Applications. Springer, pp 75-85, 677-691.
- Strang, Prof. Gilbert: “Eigenvalues and Eigenvectors.” Math 18.06. Lord Foundation of Massachusetts. Fall 1999.
- Edwards, C. Henry and David E. Penney: Differential Equations: Computing and Modeling. Upper Saddle River: Pearson Education, Inc, pp 299-365.
- Teknomo, Kardi. Finding Eigen Value of Symmetric matrix Using Microsoft Excel. http:\\people.revoledu.com\kardi\ tutorial\Excel\EigenValue.html
Contributors and Attributions
- Authors: (October 19, 2006) Tommy DiRaimondo, Rob Carr, Marc Palmer, Matt Pickvet
- Stewards: (October 22, 2007) Shoko Asei, Brian Byers, Alexander Eng, Nicholas James, Jeffrey Leto
Eigenvector and Eigenvalue
They have many uses!
A simple example is that an eigenvector does not change direction in a transformation:
How do we find that vector?
The Mathematics Of It
For a square matrix A , an Eigenvector and Eigenvalue make this equation true:
Let us see it in action:
Let's do some matrix multiplies to see if that is true.
Av gives us:
λv gives us :
Yes they are equal!
So we get Av = λv as promised.
Notice how we multiply a matrix by a vector and get the same result as when we multiply a scalar (just a number) by that vector .
How do we find these eigen things?
We start by finding the eigenvalue . We know this equation must be true:
Next we put in an identity matrix so we are dealing with matrix-vs-matrix:
Bring all to left hand side:
Av − λIv = 0
If v is non-zero then we can (hopefully) solve for λ using just the determinant :
| A − λI | = 0
Let's try that equation on our previous example:
Example: Solve for λ
Start with | A − λI | = 0
Calculating that determinant gets:
(−6−λ)(5−λ) − 3×4 = 0
Which simplifies to this Quadratic Equation :
λ 2 + λ − 42 = 0
And solving it gets:
λ = −7 or 6
And yes, there are two possible eigenvalues.
Now we know eigenvalues , let us find their matching eigenvectors .
Example (continued): Find the Eigenvector for the Eigenvalue λ = 6 :
Start with:
After multiplying we get these two equations:
Bringing all to left hand side:
Either equation reveals that y = 4x , so the eigenvector is any non-zero multiple of this:
And we get the solution shown at the top of the page:
... and also ...
So Av = λv , and we have success!
Now it is your turn to find the eigenvector for the other eigenvalue of −7
What is the purpose of these?
One of the cool things is we can use matrices to do transformations in space, which is used a lot in computer graphics.
In that case the eigenvector is "the direction that doesn't change direction" !
And the eigenvalue is the scale of the stretch:
- 1 means no change,
- 2 means doubling in length,
- −1 means pointing backwards along the eigenvector's direction
There are also many applications in physics, etc.
Why "Eigen"
Eigen is a German word meaning "own" or "typical"
"das ist ihnen eigen " is German for "that is typical of them"
Sometimes in English we use the word "characteristic", so an eigenvector can be called a "characteristic vector".
Not Just Two Dimensions
Eigenvectors work perfectly well in 3 and higher dimensions.
First calculate A − λI :
Now the determinant should equal zero:
(2−λ) [ (4−λ)(3−λ) − 5×4 ] = 0
This ends up being a cubic equation, but just looking at it here we see one of the roots is 2 (because of 2−λ), and the part inside the square brackets is Quadratic, with roots of −1 and 8 .
So the Eigenvalues are −1 , 2 and 8
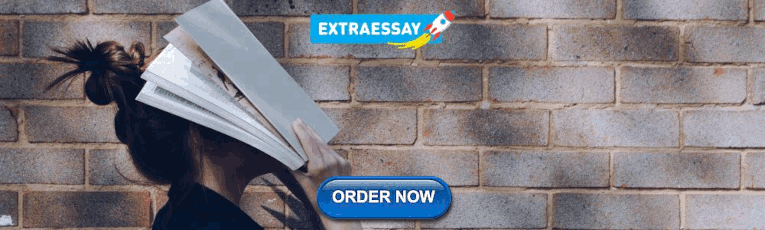
Example (continued): find the Eigenvector that matches the Eigenvalue −1
Put in the values we know:
After multiplying we get these equations:
So x = 0 , and y = −z and so the eigenvector is any non-zero multiple of this:
So Av = λv , yay!
(You can try your hand at the eigenvalues of 2 and 8 )
Back in the 2D world again, this matrix will do a rotation by θ:
Example: Rotate by 30°
cos(30°) = √3 2 and sin(30°) = 1 2 , so:
But if we rotate all points , what is the "direction that doesn't change direction"?
Let us work through the mathematics to find out:
( √3 2 −λ)( √3 2 −λ) − ( −1 2 )( 1 2 ) = 0
Which becomes this Quadratic Equation:
λ 2 − (√3)λ + 1 = 0
Whose roots are:
λ = √3 2 ± i 2
The eigenvalues are complex!
I don't know how to show you that on a graph, but we still get a solution.
Eigenvector
So, what is an eigenvector that matches, say, the √3 2 + i 2 root?
√3 2 x − 1 2 y = √3 2 x + i 2 x
1 2 x + √3 2 y = √3 2 y + i 2 y
Which simplify to:
And the solution is any non-zero multiple of:
Wow, such a simple answer!
Is this just because we chose 30°? Or does it work for any rotation matrix? I will let you work that out! Try another angle, or better still use "cos(θ)" and "sin(θ)".
Oh, and let us check at least one of those solutions:
Does it match this?
Oh yes it does!
Want Better Math Grades?
✅ Unlimited Solutions
✅ Step-by-Step Answers
✅ Available 24/7
➕ Free Bonuses ($1085 value!)
On this page
- Matrices and Determinants
- 1. Determinants
- Systems of 3x3 Equations interactive applet
- 2. Large Determinants
- 3. Matrices
- 4. Multiplication of Matrices
- 4a. Matrix Multiplication examples
- 4b. Add & multiply matrices applet
- 5. Finding the Inverse of a Matrix
- 5a. Simple Matrix Calculator
- 5b. Inverse of a Matrix using Gauss-Jordan Elimination
- 6. Matrices and Linear Equations
- Matrices and Linear Transformations
- Eigenvalues and eigenvectors - concept applet
- 7. Eigenvalues and Eigenvectors
8. Applications of Eigenvalues and Eigenvectors
- Eigenvalues and eigenvectors calculator
Related Sections
Math Tutoring
Need help? Chat with a tutor anytime, 24/7.
Online Algebra Solver
Solve your algebra problem step by step!
IntMath Forum
Get help with your math queries:
Examples on this page
A. google's pagerank, b. electronics: rlc circuits.
c. Repeated applications of a matrix
Why are eigenvalues and eigenvectors important? Let's look at some real life applications of the use of eigenvalues and eigenvectors in science, engineering and computer science.
Google's extraordinary success as a search engine was due to their clever use of eigenvalues and eigenvectors . From the time it was introduced in 1998, Google's methods for delivering the most relevant result for our search queries has evolved in many ways, and PageRank is not really a factor any more in the way it was at the beginning.
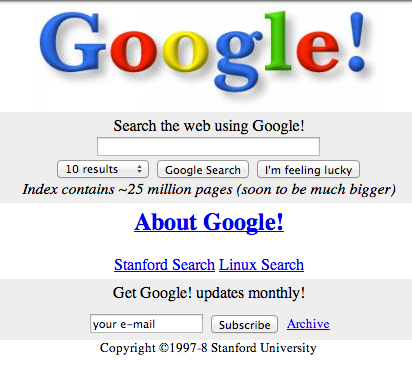
But for this discussion, let's go back to the original idea of PageRank.
Let's assume the Web contains 6 pages only. The author of Page 1 thinks pages 2, 4, 5, and 6 have good content, and links to them. The author of Page 2 only likes pages 3 and 4 so only links from her page to them. The links between these and the other pages in this simple web are summarised in this diagram.
A simple Internet web containing 6 pages
Google engineers assumed each of these pages is related in some way to the other pages, since there is at least one link to and from each page in the web.
Their task was to find the "most important" page for a particular search query, as indicated by the writers of all 6 pages. For example, if everyone linked to Page 1, and it was the only one that had 5 incoming links, then it would be easy - Page 1 would be returned at the top of the search result.
However, we can see some pages in our web are not regarded as very important. For example, Page 3 has only one incoming link. Should its outgoing link (to Page 5) be worth the same as Page 1's outgoing link to Page 5?
The beauty of PageRank was that it regarded pages with many incoming links (especially from other popular pages) as more important than those from mediocre pages, and it gave more weighting to the outgoing links of important pages.
Google's use of eigenvalues and eigenvectors
For the 6-page web illustrated above, we can form a "link matrix" representing the relative importance of the links in and out of each page.
Considering Page 1, it has 4 outgoing links (to pages 2, 4, 5, and 6). So in the first column of our "links matrix", we place value `1/4` in each of rows 2, 4, 5 and 6, since each link is worth `1/4` of all the outgoing links. The rest of the rows in column 1 have value `0`, since Page 1 doesn't link to any of them.
Meanwhile, Page 2 has only two outgoing links, to pages 3 and 4. So in the second column we place value `1/2` in rows 3 and 4, and `0` in the rest. We continue the same process for the rest of the 6 pages.
`bb(A)=[(0,0,0,0,1/2,0),(1/4,0,0,0,0,0),(0,1/2,0,0,0,0),(1/4,1/2,0,0,1/2,0),(1/4,0,1,1,0,1),(1/4,0,0,0,0,0)]`
Next, to find the eigenvalues.
`| bb(A) -lambda I |=|(-lambda,0,0,0,1/2,0),(1/4,-lambda,0,0,0,0),(0,1/2,-lambda,0,0,0),(1/4,1/2,0,-lambda,1/2,0),(1/4,0,1,1,-lambda,1),(1/4,0,0,0,0,-lambda)|`
`=lambda^6 - (5lambda^4)/8 - (lambda^3)/4 - (lambda^2)/8`
This expression is zero for `lambda = -0.72031,` `-0.13985+-0.39240j,` `0,` `1`. (I expanded the determinant and then solved it for zero using Wolfram|Alpha.)
We can only use non-negative, real values of `lambda` (since they are the only ones that will make sense in this context), so we conclude `lambda=1.` (In fact, for such PageRank problems we always take `lambda=1`.)
We could set up the six equations for this situation, substitute and choose a "convenient" starting value, but for vectors of this size, it's more logical to use a computer algebra system. Using Wolfram|Alpha, we find the corresponding eigenvector is:
`bb(v)_1=[4\ \ 1\ \ 0.5\ \ 5.5\ \ 8\ \ 1]^"T"`
As Page 5 has the highest PageRank (of 8 in the above vector), we conclude it is the most "important", and it will appear at the top of the search results.
We often normalize this vector so the sum of its elements is `1.` (We just add up the amounts and divide each amount by that total, in this case `20`.) This is OK because we can choose any "convenient" starting value and we want the relative weights to add to `1.` I've called this normalized vector `bb(P)` for "PageRank".
`bb(P)=[0.2\ \ 0.05\ \ 0.025\ \ 0.275\ \ 0.4\ \ 0.05]^"T"`
Search engine reality checks
- Our example web above has 6 pages, whereas Google (and Bing and other sesarch engines) needs to cope with billions of pages. This requires a lot of computing power, and clever mathematics to optimize processes.
- PageRank was only one of many ranking factors employed by Google from the beginning. They also looked at key words in the search query and compared that to the number of times those search words appeared on a page, and where they appeared (if they were in headings or page descriptions they were "worth more" than if the words were lower down the page). All of these factors were fairly easy to "game" once they were known about, so Google became more secretive about what it uses to rank pages for any particular search term.
- Google currenly use over 200 different signals when analyzing Web pages, including page speed, whether local or not, mobile friendliness, amount of text, authority of the overall site, freshness of the content, and so on. They constantly revise those signals to beat "black hat" operators (who try to game the system to get on top) and to try to ensure the best quality and most authoritative pages are presented at the top.
References and further reading
- How Google Finds Your Needle in the Web's Haystack (a good explanation of the basics of PageRank and consideration of cases that don't quite fit the model)
- The Anatomy of a Large-Scale Hypertextual Web Search Engine (the original Stanford research paper by Sergey Brin and Lawrence Page presenting the concepts behind Google search, using eigenvalues and eigenvectors)
- The $25,000,000,000 Eigenvector The Linear Algebra Behind Google (PDF, containing further explanation)
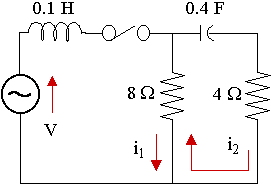
An electical circuit consists of 2 loops, one with a 0.1 H inductor and the second with a 0.4 F capacitor and a 4 Ω resistor, and sharing an 8 Ω resistor, as shown in the diagram. The power supply is 12 V. (We'll learn how to solve such circuits using systems of differential equations in a later chapter, beginning at Series RLC Circuit .)
Let's see how to solve such a circuit (that means finding the currents in the two loops) using matrices and their eigenvectors and eigenvalues. We are making use of Kirchhoff's voltage law and the definitions regarding voltage and current in the differential equations chapter linked to above.
NOTE: There is no attempt here to give full explanations of where things are coming from. It's just to illustrate the way such circuits can be solved using eigenvalues and eigenvectors.
For the left loop: `0.1(di_1)/(dt) + 8(i_1 - i_2) = 12`
Muliplying by 10 and rearranging gives: `(di_1)/(dt) = - 80i_1 + 80i_2 +120` ... (1)
For the right loop: `4i_2 + 2.5 int i_2 dt + 8(i_2 - i_1) = 12`
Differentiating gives: `4(di_2)/(dt) + 2.5i_2 + 8((di_2)/(dt) - (di_1)/(dt)) = 12`
Rearranging gives: `12(di_2)/(dt) = 8(di_1)/(dt) - 2.5i_2 + 12`
Substituting (1) gives: `12(di_2)/(dt)` ` = 8(- 80i_1 + 80i_2 +120) - 2.5i_2 + 12` ` = - 640i_1 + 637.5i_2 + 972`
Dividing through by 12 and rearranging gives: `(di_2)/(dt) = - 53.333i_1 + 53.125i_2 + 81` ...(2)
We can write (1) and (2) in matrix form as:
`(dbb(K))/(dt) = bb(AK) + bb(v)`, where `bb(K)=[(i_1),(i_2)],` `bb(A) = [(-80, 80),(-53.333, 53.125)],` `bb(v)=[(120),(81)]`
The characteristic equation for matrix A is `lambda^2 + 26.875lambda + 16.64 = 0` which yields the eigenvalue-eigenvector pairs `lambda_1=-26.2409,` `bb(v)_1 = [(1.4881),(1)]` and `lambda_2=-0.6341,` `bb(v)_2 = [(1.008),(1)].`
The eigenvectors give us a general solution for the system:
`bb(K)` `=c_1[(1.4881),(1)]e^(-1.4881t) + c_2[(1.008),(1)]e^(-1.008t)`
c. Repeated applications of a matrix: Markov processes
Scenario: A market research company has observed the rise and fall of many technology companies, and has predicted the future market share proportion of three companies A, B and C to be determined by a transition matrix P, at the end of each monthly interval:
`bb(P)=[(0.8,0.1,0.1),(0.03,0.95,0.02),(0.2,0.05,0.75)]`
The first row of matrix P represents the share of Company A that will pass to Company A, Company B and Company C respectively. The second row represents the share of Company B that will pass to Company A, Company B and Company C respectively, while the third row represents the share of Company C that will pass to Company A, Company B and Company C respectively. Notice each row adds to 1.
The initial market share of the three companies is represented by the vector `bb(s_0)=[(30),(15),(55)]`, that is, Company A has 30% share, Company B, 15% share and Company C, 55% share.
We can calculate the predicted market share after 1 month, s 1 , by multiplying P and the current share matrix:
`bb(s)_1` `=bb(Ps_0)` `=[(0.8,0.1,0.1),(0.03,0.95,0.02),(0.2,0.05,0.75)][(30),(15),(55)]` `= [(35.45),(20),(44.55)]`
Next, we can calculate the predicted market share after the second month, s 2 , by squaring the transition matrix (which means applying it twice) and multiplying it by s 0 :
`bb(s)_2` `=bb(P)^2bb(s_0)` `=[(0.663,0.18,0.157),(0.0565,0.9065,0.037),(0.3115,0.105,0.5835)][(30),(15),(55)]` `= [(37.87),(24.7725),(37.3575)]`
Continuing in this fashion, we see that after a period of time, the market share of the three companies settles down to around 23.8%, 61.6% and 14.5%. Here's a table with selected values.
This type of process involving repeated multiplication of a matrix is called a Markov Process , after the 19th century Russian mathematician Andrey Markov.
Here's the graph of the change in proportions over a period of 40 months.
Proportion of Company A (green), Company B (magenta) and Company C (blue) over time
Next, we'll see how to find these terminating values without the bother of multiplying matrices over and over.
Using eigenvalues and eigenvectors to calculate the final values when repeatedly applying a matrix
First, we need to consider the conditions under which we'll have a steady state. If there is no change of value from one month to the next, then the eigenvalue should have value 1 . It means multiplying by matrix P N no longer makes any difference. It also means the eigenvector will be `[(1),(1),(1)].`
We need to make use of the transpose of matrix P , that is P T , for this solution. (If we use P , we get trivial solutions since each row of P adds to 1.) The eigenvectors of the transpose are the same as those for the original matrix.
Solving `[bb(P)^"T"-lambda bb(I)]bb(x)` gives us:
`[bb(P)^"T"-lambda bb(I)]bb(x) = [(0.8-1,0.03,0.2),(0.1,0.95-1,0.05),(0.1,0.02,0.75-1)][(x_1),(x_2),(x_3)]`
`= [(-0.2,0.03,0.2),(0.1,-0.05,0.05),(0.1,0.02,-0.25)][(x_1),(x_2),(x_3)]`
`=[(0),(0),(0)]`
Written out, the last two lines give us:
`-0.2 x_1 + 0.03x_2 + 0.2x_3 = 0` `0.1x_1 - 0.05 x_2 + 0.05 x_3 = 0` `0.1x_1 + 0.02x_2 - 0.25x_3 = 0`
Choosing `x_1=1`, we solve rows 1 and 2 simultaneously to give: `x_2=2.6087` and then `x_3=0.6087.`
We now normalize these 3 values, by adding them up, dividing each one by the total and multiplying by 100. We obtain:
`bb(v) = [23.7116,61.8564, 14.4332]`
This value represents the "limiting value" of each row of the matrix P as we multiply it by itself over and over. More importantly, it gives us the final market share of the 3 companies A, B and C.
We can see these are the values for the market share are converging to in the above table and graph.
For interest, here is the result of multiplying matrix P by itself 40 times. We see each row is the same as we obtained by the procedure involving the transpose above.
`bb(A)^40=[(0.23711623272314,0.61856408536471,0.14433161991843),(0.23711623272314,0.61856408536471,0.14433161991843),(0.23711623272314,0.61856408536471,0.14433161991843)]`
Tips, tricks, lessons, and tutoring to help reduce test anxiety and move to the top of the class.
Email Address Sign Up

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
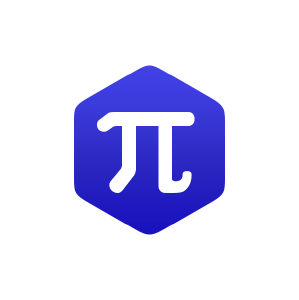
11.6.1: Eigenvalues and Eigenvectors (Exercises)
- Last updated
- Save as PDF
- Page ID 120068
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
In Exercises \(\PageIndex{1}\) - \(\PageIndex{6}\), a matrix \(A\) and one of its eigenvectors are given. Find the eigenvalue of \(A\) for the given eigenvector.
Exercise \(\PageIndex{1}\)
\(A=\left[\begin{array}{cc}{9}&{8}\\{-6}&{-5}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{-4}\\{3}\end{array}\right]\)
\(\lambda =3\)
Exercise \(\PageIndex{2}\)
\(A=\left[\begin{array}{cc}{19}&{-6}\\{48}&{-15}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{1}\\{3}\end{array}\right]\)
\(\lambda =1\)
Exercise \(\PageIndex{3}\)
\(A=\left[\begin{array}{cc}{1}&{-2}\\{-2}&{4}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{2}\\{1}\end{array}\right]\)
\(\lambda =0\)
Exercise \(\PageIndex{4}\)
\(A=\left[\begin{array}{ccc}{-11}&{-19}&{14}\\{-6}&{-8}&{6}\\{-12}&{-22}&{15}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{3}\\{2}\\{4}\end{array}\right]\)
\(\lambda =-5\)
Exercise \(\PageIndex{5}\)
\(A=\left[\begin{array}{ccc}{-7}&{1}&{3}\\{10}&{2}&{-3}\\{-20}&{-14}&{1}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{1}\\{-2}\\{4}\end{array}\right]\)
Exercise \(\PageIndex{6}\)
\(A=\left[\begin{array}{ccc}{-12}&{-10}&{0}\\{15}&{13}&{0}\\{15}&{18}&{-5}\end{array}\right]\quad\vec{x}=\left[\begin{array}{c}{-1}\\{1}\\{1}\end{array}\right]\)
\(\lambda =-2\)
In Exercises \(\PageIndex{7}\) – \(\PageIndex{11}\), a matrix \(A\) and one of its eigenvalues are given. Find an eigenvector of \(A\) for the given eigenvalue.
Exercise \(\PageIndex{7}\)
\(A=\left[\begin{array}{cc}{16}&{6}\\{-18}&{-5}\end{array}\right]\quad\lambda =4\)
\(\vec{x}=\left[\begin{array}{c}{-1}\\{2}\end{array}\right]\)
Exercise \(\PageIndex{8}\)
\(A=\left[\begin{array}{cc}{-2}&{6}\\{-9}&{13}\end{array}\right]\quad\lambda =7\)
\(\vec{x}=\left[\begin{array}{c}{2}\\{3}\end{array}\right]\)
Exercise \(\PageIndex{9}\)
\(A=\left[\begin{array}{ccc}{-16}&{-28}&{-19}\\{42}&{69}&{46}\\{-42}&{-72}&{-49}\end{array}\right]\quad\lambda =5\)
\(\vec{x}=\left[\begin{array}{c}{3}\\{-7}\\{7}\end{array}\right]\)
Exercise \(\PageIndex{10}\)
\(A=\left[\begin{array}{ccc}{7}&{-5}&{-10}\\{6}&{2}&{-6}\\{2}&{-5}&{-5}\end{array}\right]\quad\lambda =-3\)
\(\vec{x}=\left[\begin{array}{c}{1}\\{0}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{11}\)
\(A=\left[\begin{array}{ccc}{4}&{5}&{-3}\\{-7}&{-8}&{3}\\{1}&{-5}&{8}\end{array}\right]\quad\lambda =2\)
\(\vec{x}=\left[\begin{array}{c}{-1}\\{1}\\{1}\end{array}\right]\)
In Exercises \(\PageIndex{12}\) – \(\PageIndex{28}\), find the eigenvalues of the given matrix. For each eigenvalue, give an eigenvector.
Exercise \(\PageIndex{12}\)
\(\left[\begin{array}{cc}{-1}&{-4}\\{-3}&{-2}\end{array}\right]\)
\(\lambda_{1}=-5\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{1}\end{array}\right];\)
\(\lambda_{2}=2\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-4}\\{3}\end{array}\right]\)
Exercise \(\PageIndex{13}\)
\(\left[\begin{array}{cc}{-4}&{72}\\{-1}&{13}\end{array}\right]\)
\(\lambda_{1}=4\) with \(\vec{x_{1}}=\left[\begin{array}{c}{9}\\{1}\end{array}\right];\)
\(\lambda_{2}=5\) with \(\vec{x_{2}}=\left[\begin{array}{c}{8}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{14}\)
\(\left[\begin{array}{cc}{2}&{-12}\\{2}&{-8}\end{array}\right]\)
\(\lambda_{1}=-4\) with \(\vec{x_{1}}=\left[\begin{array}{c}{2}\\{1}\end{array}\right];\)
\(\lambda_{2}=-2\) with \(\vec{x_{2}}=\left[\begin{array}{c}{3}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{15}\)
\(\left[\begin{array}{cc}{3}&{12}\\{1}&{-1}\end{array}\right]\)
\(\lambda_{1}=-3\) with \(\vec{x_{1}}=\left[\begin{array}{c}{-2}\\{1}\end{array}\right];\)
\(\lambda_{2}=5\) with \(\vec{x_{2}}=\left[\begin{array}{c}{6}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{16}\)
\(\left[\begin{array}{cc}{5}&{9}\\{-1}&{-5}\end{array}\right]\)
\(\lambda_{1}=-4\) with \(\vec{x_{1}}=\left[\begin{array}{c}{-1}\\{1}\end{array}\right];\)
\(\lambda_{2}=4\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-9}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{17}\)
\(\left[\begin{array}{cc}{3}&{-1}\\{-1}&{3}\end{array}\right]\)
\(\lambda_{1}=2\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{1}\end{array}\right];\)
\(\lambda_{2}=4\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-1}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{18}\)
\(\left[\begin{array}{cc}{0}&{1}\\{25}&{0}\end{array}\right]\)
\(\lambda_{1}=-5\) with \(\vec{x_{1}}=\left[\begin{array}{c}{-1}\\{5}\end{array}\right];\)
\(\lambda_{2}=5\) with \(\vec{x_{2}}=\left[\begin{array}{c}{1}\\{5}\end{array}\right]\)
Exercise \(\PageIndex{19}\)
\(\left[\begin{array}{cc}{-3}&{1}\\{0}&{-1}\end{array}\right]\)
\(\lambda_{1}=-1\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{2}\end{array}\right];\)
\(\lambda_{2}=-3\) with \(\vec{x_{2}}=\left[\begin{array}{c}{1}\\{0}\end{array}\right]\)
Exercise \(\PageIndex{20}\)
\(\left[\begin{array}{ccc}{1}&{-2}&{-3}\\{0}&{3}&{0}\\{0}&{-1}&{-1}\end{array}\right]\)
\(\lambda_{1}=-1\) with \(\vec{x_{1}}=\left[\begin{array}{c}{3}\\{0}\\{2}\end{array}\right];\)
\(\lambda_{2}=1\) with \(\vec{x_{2}}=\left[\begin{array}{c}{1}\\{0}\\{0}\end{array}\right]\)
\(\lambda_{3}=3\) with \(\vec{x_{3}}=\left[\begin{array}{c}{5}\\{-8}\\{2}\end{array}\right]\)
Exercise \(\PageIndex{21}\)
\(\left[\begin{array}{ccc}{5}&{-2}&{3}\\{0}&{4}&{0}\\{0}&{-1}&{3}\end{array}\right]\)
\(\lambda_{1}=3\) with \(\vec{x_{1}}=\left[\begin{array}{c}{-3}\\{0}\\{2}\end{array}\right];\)
\(\lambda_{2}=4\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-5}\\{-1}\\{1}\end{array}\right]\)
\(\lambda_{3}=5\) with \(\vec{x_{3}}=\left[\begin{array}{c}{1}\\{0}\\{0}\end{array}\right]\)
Exercise \(\PageIndex{22}\)
\(\left[\begin{array}{ccc}{1}&{0}&{12}\\{2}&{-5}&{0}\\{1}&{0}&{2}\end{array}\right]\)
\(\lambda_{1}=-5\) with \(\vec{x_{1}}=\left[\begin{array}{c}{0}\\{1}\\{0}\end{array}\right];\)
\(\lambda_{2}=-2\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-12}\\{-8}\\{3}\end{array}\right]\)
\(\lambda_{3}=5\) with \(\vec{x_{3}}=\left[\begin{array}{c}{15}\\{3}\\{5}\end{array}\right]\)
Exercise \(\PageIndex{23}\)
\(\left[\begin{array}{ccc}{1}&{0}&{-18}\\{-4}&{3}&{-1}\\{1}&{0}&{-8}\end{array}\right]\)
\(\lambda_{1}=-5\) with \(\vec{x_{1}}=\left[\begin{array}{c}{24}\\{13}\\{8}\end{array}\right];\)
\(\lambda_{2}=-2\) with \(\vec{x_{2}}=\left[\begin{array}{c}{6}\\{5}\\{1}\end{array}\right]\)
\(\lambda_{3}=3\) with \(\vec{x_{3}}=\left[\begin{array}{c}{0}\\{1}\\{0}\end{array}\right]\)
Exercise \(\PageIndex{24}\)
\(\left[\begin{array}{ccc}{-1}&{18}&{0}\\{1}&{2}&{0}\\{5}&{-3}&{-1}\end{array}\right]\)
\(\lambda_{1}=-4\) with \(\vec{x_{1}}=\left[\begin{array}{c}{-6}\\{1}\\{11}\end{array}\right];\)
\(\lambda_{2}=-1\) with \(\vec{x_{2}}=\left[\begin{array}{c}{0}\\{0}\\{1}\end{array}\right]\)
\(\lambda_{3}=5\) with \(\vec{x_{3}}=\left[\begin{array}{c}{3}\\{1}\\{2}\end{array}\right]\)
Exercise \(\PageIndex{25}\)
\(\left[\begin{array}{ccc}{5}&{0}&{0}\\{1}&{1}&{0}\\{-1}&{5}&{-2}\end{array}\right]\)
\(\lambda_{1}=-2\) with \(\vec{x_{1}}=\left[\begin{array}{c}{0}\\{0}\\{1}\end{array}\right];\)
\(\lambda_{2}=1\) with \(\vec{x_{2}}=\left[\begin{array}{c}{0}\\{3}\\{5}\end{array}\right]\)
\(\lambda_{3}=5\) with \(\vec{x_{3}}=\left[\begin{array}{c}{28}\\{7}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{26}\)
\(\left[\begin{array}{ccc}{2}&{-1}&{1}\\{0}&{3}&{6}\\{0}&{0}&{7}\end{array}\right]\)
\(\lambda_{1}=2\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{0}\\{0}\end{array}\right];\)
\(\lambda_{2}=3\) with \(\vec{x_{2}}=\left[\begin{array}{c}{-1}\\{1}\\{0}\end{array}\right]\)
\(\lambda_{3}=7\) with \(\vec{x_{3}}=\left[\begin{array}{c}{-1}\\{15}\\{10}\end{array}\right]\)
Exercise \(\PageIndex{27}\)
\(\left[\begin{array}{ccc}{3}&{5}&{-5}\\{-2}&{3}&{2}\\{-2}&{5}&{0}\end{array}\right]\)
\(\lambda_{1}=-2\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{0}\\{1}\end{array}\right];\)
\(\lambda_{2}=3\) with \(\vec{x_{2}}=\left[\begin{array}{c}{1}\\{1}\\{1}\end{array}\right];\)
\(\lambda_{3}=5\) with \(\vec{x_{3}}=\left[\begin{array}{c}{0}\\{1}\\{1}\end{array}\right]\)
Exercise \(\PageIndex{28}\)
\(\left[\begin{array}{ccc}{1}&{2}&{1}\\{1}&{2}&{3}\\{1}&{1}&{1}\end{array}\right]\)
\(\lambda_{1}=0\) with \(\vec{x_{1}}=\left[\begin{array}{c}{1}\\{3}\\{1}\end{array}\right];\)
\(\lambda_{2}=-1\) with \(\vec{x_{2}}=\left[\begin{array}{c}{2}\\{2}\\{1}\end{array}\right];\)
\(\lambda_{3}=2\) with \(\vec{x_{3}}=\left[\begin{array}{c}{1}\\{1}\\{1}\end{array}\right]\)
Eigenvalue-generalized eigenvector assignment by output feedback
Ieee account.
- Change Username/Password
- Update Address
Purchase Details
- Payment Options
- Order History
- View Purchased Documents
Profile Information
- Communications Preferences
- Profession and Education
- Technical Interests
- US & Canada: +1 800 678 4333
- Worldwide: +1 732 981 0060
- Contact & Support
- About IEEE Xplore
- Accessibility
- Terms of Use
- Nondiscrimination Policy
- Privacy & Opting Out of Cookies
A not-for-profit organization, IEEE is the world's largest technical professional organization dedicated to advancing technology for the benefit of humanity. © Copyright 2024 IEEE - All rights reserved. Use of this web site signifies your agreement to the terms and conditions.
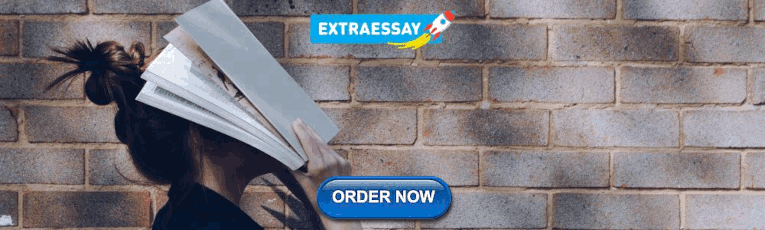
IMAGES
VIDEO
COMMENTS
The comma initializer. Eigen offers a comma initializer syntax which allows the user to easily set all the coefficients of a matrix, vector or array. Simply list the coefficients, starting at the top-left corner and moving from left to right and from the top to the bottom. The size of the object needs to be specified beforehand.
Eigenvalues are a special set of scalars associated with a square matrix that are sometimes also known as characteristic roots, characteristic values (Hoffman and Kunze 1971), proper values, or latent roots (Marcus and Minc 1988, p. 144). The determination of the eigenvalues and eigenvectors of a matrix is extremely important in physics and ...
This page aims to provide an overview and some details on how to perform arithmetic between matrices, vectors and scalars with Eigen.. Introduction. Eigen offers matrix/vector arithmetic operations either through overloads of common C++ arithmetic operators such as +, -, *, or through special methods such as dot(), cross(), etc. For the Matrix class (matrices and vectors), operators are only ...
Lecture 21: Eigenvalues and eigenvectors. If the product Ax points in the same direction as the vector x, we say that x is an eigenvector of A. Eigenvalues and eigenvectors describe what happens when a matrix is multiplied by a vector. In this session we learn how to find the eigenvalues and eigenvectors of a matrix.
Steps for finding the eigenvalues and eigenvectors. We want to find λ λ and non-zero vector x x such that Ax = λx A x = λ x for a n × n n × n matrix. We introduce an identity matrix I I of n × n n × n. Then the equation becomes: Ax = λIx A x = λ I x. Ax − λIx = 0 A x − λ I x = 0. (A − λI)x = 0 ( A − λ I) x = 0.
Lecture 21: Eigenvalues and Eigenvectors. Transcript. Download video. Download transcript. MIT OpenCourseWare is a web based publication of virtually all MIT course content. OCW is open and available to the world and is a permanent MIT activity.
Key Idea 4.1.1: Finding Eigenvalues and Eigenvectors. Let A be an n × n matrix. To find the eigenvalues of A, compute p(λ), the characteristic polynomial of A, set it equal to 0, then solve for λ. To find the eigenvectors of A, for each eigenvalue solve the homogeneous system (A − λI)→x = →0. Example 4.1.3.
Most 2 by 2 matrices have two eigenvector directions and two eigenvalues. We will show that det(A − λI)=0. This section explains how to compute the x's and λ's. It can come early in the course. We only need the determinant ad − bc of a 2 by 2 matrix. Example 1 uses to find the eigenvalues λ = 1 and λ = det(A−λI)=0 1.
In order to solve for the eigenvalues and eigenvectors, we rearrange the Equation 10.3.1 to obtain the following: (Λ λI)v = 0 [4 − λ − 4 1 4 1 λ 3 1 5 − 1 − λ] ⋅ [x y z] = 0. For nontrivial solutions for v, the determinant of the eigenvalue matrix must equal zero, det(A − λI) = 0. This allows us to solve for the eigenvalues, λ.
According to Eigen Doc, Vector is a typedef for Matrix, and the Matrix has a constructor with the following signature: Matrix (const Scalar *data) Constructs a fixed-sized matrix initialized with coefficients starting at data. And vector reference defines the std::vector::data as:. std::vector::data T* data(); const T* data() const;
For a square matrix A, an Eigenvector and Eigenvalue make this equation true: Let us see it in action: Let's do some matrix multiplies to see if that is true. Av gives us: λv gives us : Yes they are equal! So we get Av = λv as promised. Notice how we multiply a matrix by a vector and get the same result as when we multiply a scalar (just a ...
Let's look at some real life applications of the use of eigenvalues and eigenvectors in science, engineering and computer science. a. Google's PageRank. Google's extraordinary success as a search engine was due to their clever use of eigenvalues and eigenvectors. From the time it was introduced in 1998, Google's methods for delivering the most ...
In a recent paper [1], a characterization has been given for the class of all closed-loop eigenvector sets which can be obtained with a given set of distinct closed-loop eigenvalues. This note extends these results to characterize the class of generalized eigenvector chains which can be obtained with a given set of nondistinct eigenvalues. Included is an algorithm for computing a feedback ...
Eigenvector assignment. Abstract: Eigenstructure assignment techniques have typically focused on the assignment of eigenvalues and in some MIMO cases, the additional assignment of best fit eigenvectors. While eigenvalue specification determines the stability and speed of response, eigenvector specification determines the shape of the transients.
Download Page (PDF) Download Full Book (PDF) Resources expand_more. Periodic Table. Physics Constants. Scientific Calculator. Reference expand_more. Reference & Cite. Tools expand_more.
eigenvalue/eigenvector assignment problem is to determine the number of eigen- values in equation (4) that can be arbitrarily assigned and to determine the freedom available in the selection of the associated eigenvectors. In order to see what freedom exists in the choice of eigenvectors, write equation (4) in partitioned form as
Abstract: The problem of pole-assignment in a linear time-invariant multivariable system using output feedback is considered. New sufficient conditions are derived to assign an almost arbitrary set of min (n,m+r- 1) distinct eigenvalues, where n, m, and r are the number of states, inputs, and outputs, respectively. The analysis also highlights the freedom in selection of closed-loop ...
How exactly do we set the value of an Eigen Vector or Matrix by index. I'm trying to do something similar to: // Assume row major matrix[i][j] = value // or vector[i] = value I might have missed it, but could not find anything in the quick reference guide. c++; c++11; eigen; eigen3; Share.
This note generalizes the previous results of the closed-loop eigenstructure assignment via output feedback in linear multivariable systems. Necessary and sufficient conditions for the closed-loop eigenstructure assignment by output feedback are presented. Some known results on entire eigenstructure assignment are deduced from this result.
The more useful takeaway from this thread is the demonstration of copying a std::vector to all rows or columns of an Eigen Matrix, in the accepted answer. I want to copy vectors into the columns of a matrix, like the following: int m = 10; std::vector<Eigen::VectorXd> v(m); Eigen::MatrixXd S(m,m); for (int i = 0; i != m; ++i) {.