Function expressions
In JavaScript, a function is not a “magical language structure”, but a special kind of value.
The syntax that we used before is called a Function Declaration :
There is another syntax for creating a function that is called a Function Expression .
It allows us to create a new function in the middle of any expression.
For example:
Here we can see a variable sayHi getting a value, the new function, created as function() { alert("Hello"); } .
As the function creation happens in the context of the assignment expression (to the right side of = ), this is a Function Expression .
Please note, there’s no name after the function keyword. Omitting a name is allowed for Function Expressions.
Here we immediately assign it to the variable, so the meaning of these code samples is the same: "create a function and put it into the variable sayHi ".
In more advanced situations, that we’ll come across later, a function may be created and immediately called or scheduled for a later execution, not stored anywhere, thus remaining anonymous.
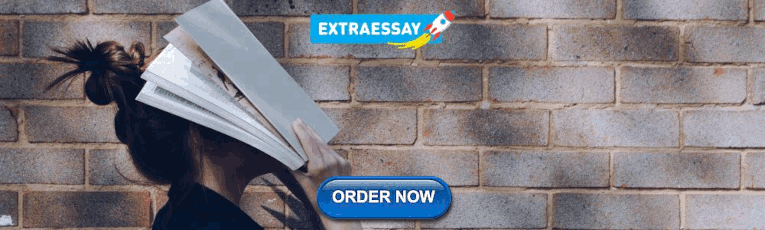
Function is a value
Let’s reiterate: no matter how the function is created, a function is a value. Both examples above store a function in the sayHi variable.
We can even print out that value using alert :
Please note that the last line does not run the function, because there are no parentheses after sayHi . There are programming languages where any mention of a function name causes its execution, but JavaScript is not like that.
In JavaScript, a function is a value, so we can deal with it as a value. The code above shows its string representation, which is the source code.
Surely, a function is a special value, in the sense that we can call it like sayHi() .
But it’s still a value. So we can work with it like with other kinds of values.
We can copy a function to another variable:
Here’s what happens above in detail:
- The Function Declaration (1) creates the function and puts it into the variable named sayHi .
- Line (2) copies it into the variable func . Please note again: there are no parentheses after sayHi . If there were, then func = sayHi() would write the result of the call sayHi() into func , not the function sayHi itself.
- Now the function can be called as both sayHi() and func() .
We could also have used a Function Expression to declare sayHi , in the first line:
Everything would work the same.
You might wonder, why do Function Expressions have a semicolon ; at the end, but Function Declarations do not:
The answer is simple: a Function Expression is created here as function(…) {…} inside the assignment statement: let sayHi = …; . The semicolon ; is recommended at the end of the statement, it’s not a part of the function syntax.
The semicolon would be there for a simpler assignment, such as let sayHi = 5; , and it’s also there for a function assignment.
Callback functions
Let’s look at more examples of passing functions as values and using function expressions.
We’ll write a function ask(question, yes, no) with three parameters:
The function should ask the question and, depending on the user’s answer, call yes() or no() :
In practice, such functions are quite useful. The major difference between a real-life ask and the example above is that real-life functions use more complex ways to interact with the user than a simple confirm . In the browser, such functions usually draw a nice-looking question window. But that’s another story.
The arguments showOk and showCancel of ask are called callback functions or just callbacks .
The idea is that we pass a function and expect it to be “called back” later if necessary. In our case, showOk becomes the callback for “yes” answer, and showCancel for “no” answer.
We can use Function Expressions to write an equivalent, shorter function:
Here, functions are declared right inside the ask(...) call. They have no name, and so are called anonymous . Such functions are not accessible outside of ask (because they are not assigned to variables), but that’s just what we want here.
Such code appears in our scripts very naturally, it’s in the spirit of JavaScript.
Regular values like strings or numbers represent the data .
A function can be perceived as an action .
We can pass it between variables and run when we want.
Function Expression vs Function Declaration
Let’s formulate the key differences between Function Declarations and Expressions.
First, the syntax: how to differentiate between them in the code.
Function Declaration: a function, declared as a separate statement, in the main code flow:
Function Expression: a function, created inside an expression or inside another syntax construct. Here, the function is created on the right side of the “assignment expression” = :
The more subtle difference is when a function is created by the JavaScript engine.
A Function Expression is created when the execution reaches it and is usable only from that moment.
Once the execution flow passes to the right side of the assignment let sum = function… – here we go, the function is created and can be used (assigned, called, etc. ) from now on.
Function Declarations are different.
A Function Declaration can be called earlier than it is defined.
For example, a global Function Declaration is visible in the whole script, no matter where it is.
That’s due to internal algorithms. When JavaScript prepares to run the script, it first looks for global Function Declarations in it and creates the functions. We can think of it as an “initialization stage”.
And after all Function Declarations are processed, the code is executed. So it has access to these functions.
For example, this works:
The Function Declaration sayHi is created when JavaScript is preparing to start the script and is visible everywhere in it.
…If it were a Function Expression, then it wouldn’t work:
Function Expressions are created when the execution reaches them. That would happen only in the line (*) . Too late.
Another special feature of Function Declarations is their block scope.
In strict mode, when a Function Declaration is within a code block, it’s visible everywhere inside that block. But not outside of it.
For instance, let’s imagine that we need to declare a function welcome() depending on the age variable that we get during runtime. And then we plan to use it some time later.
If we use Function Declaration, it won’t work as intended:
That’s because a Function Declaration is only visible inside the code block in which it resides.
Here’s another example:
What can we do to make welcome visible outside of if ?
The correct approach would be to use a Function Expression and assign welcome to the variable that is declared outside of if and has the proper visibility.
This code works as intended:
Or we could simplify it even further using a question mark operator ? :
As a rule of thumb, when we need to declare a function, the first thing to consider is Function Declaration syntax. It gives more freedom in how to organize our code, because we can call such functions before they are declared.
That’s also better for readability, as it’s easier to look up function f(…) {…} in the code than let f = function(…) {…}; . Function Declarations are more “eye-catching”.
…But if a Function Declaration does not suit us for some reason, or we need a conditional declaration (we’ve just seen an example), then Function Expression should be used.
- Functions are values. They can be assigned, copied or declared in any place of the code.
- If the function is declared as a separate statement in the main code flow, that’s called a “Function Declaration”.
- If the function is created as a part of an expression, it’s called a “Function Expression”.
- Function Declarations are processed before the code block is executed. They are visible everywhere in the block.
- Function Expressions are created when the execution flow reaches them.
In most cases when we need to declare a function, a Function Declaration is preferable, because it is visible prior to the declaration itself. That gives us more flexibility in code organization, and is usually more readable.
So we should use a Function Expression only when a Function Declaration is not fit for the task. We’ve seen a couple of examples of that in this chapter, and will see more in the future.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Difference between function expression vs declaration in JavaScript
- Difference between Function Declarations & Function Expressions in JavaScript
- Difference between ‘function declaration’ and ‘function expression' in JavaScript
- Difference between Methods and Functions in JavaScript
- Difference between First-Class and Higher-Order Functions in JavaScript
- Explain the Different Function States in JavaScript
- Difference between AngularJS Expressions and JavaScript Expressions
- JavaScript async function expression
- Difference Between Static and Const in JavaScript
- Difference between Anonymous and Named functions in JavaScript
- Using the function* Declaration in JavaScript
- Difference between "var functionName = function() {}" and "function functionName() {}" in JavaScript
- Difference Between Scope and Closures in JavaScript
- Difference between declaration of user defined function inside main() and outside of main()
- JavaScript Function Expression
- Function Declaration vs. Function Definition
- JavaScript function* expression
- Difference between var and let in JavaScript
- Difference between var, let and const keywords in JavaScript
- How to declare variables in different ways in JavaScript?
Function Declaration: A Function Declaration ( or a Function Statement) defines a function with the specified parameters without requiring a variable assignment. They exist on their own, i.e, they are standalone constructs and cannot be nested within a non-function block. A function is declared using the function keyword.
Function Expression: A Function Expression works just like a function declaration or a function statement, the only difference is that a function name is NOT started in a function expression, that is, anonymous functions are created in function expressions. The function expressions run as soon as they are defined.
Example 1: Using a Function Declaration
Example 2: Using a Function Expression
Please Login to comment...
Similar reads.
- Web Technologies - Difference Between
- Difference Between
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Function Declaration vs Function Expression
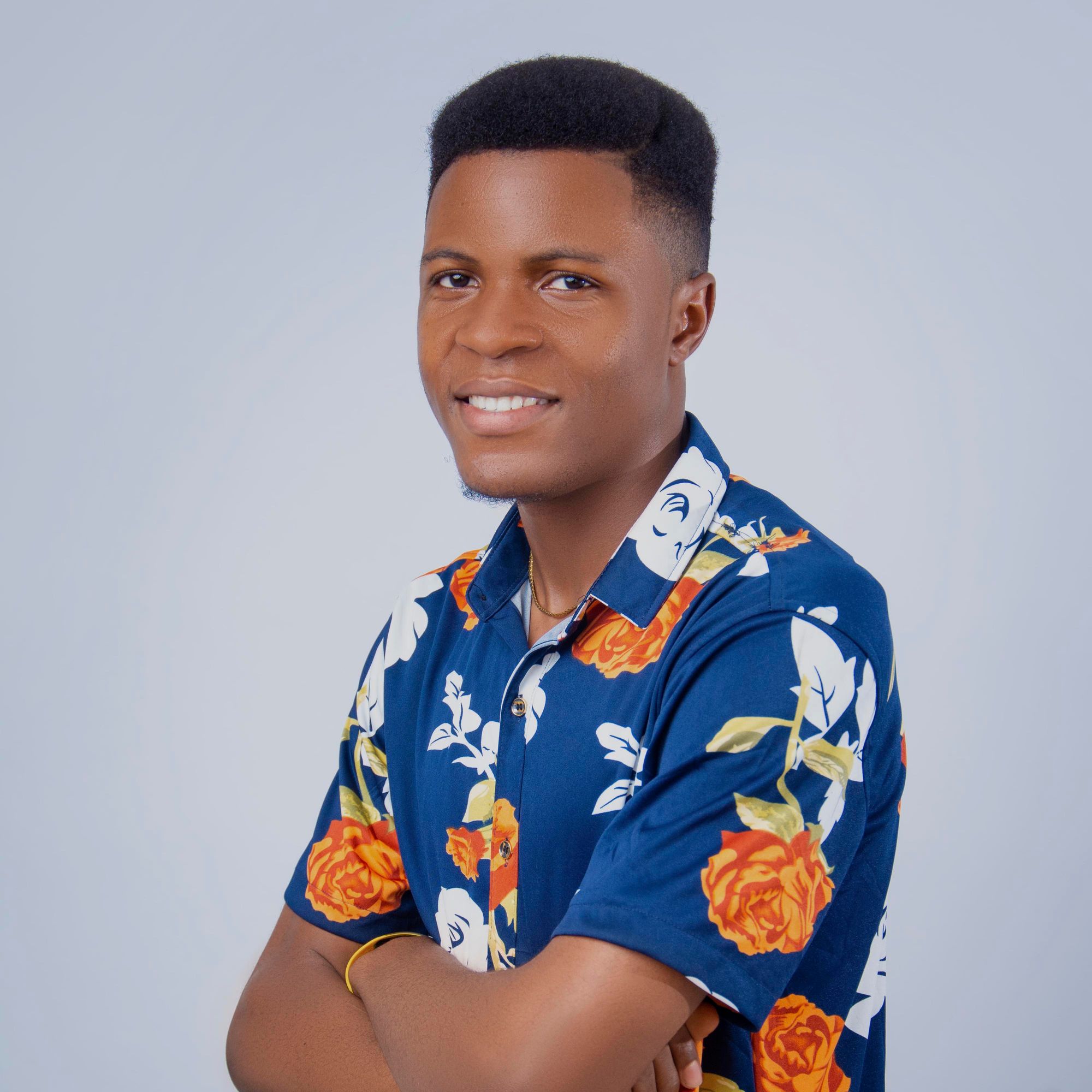
When creating functions, you can use two approaches: declaration and expression . What's the difference?
When talking about functions in JavaScript, you would often hear function declarations and function expressions. Though these approaches are almost similar, they have notable differences.
We'll look at the differences in this article.
I have a video version of this topic you can also check out.
Function Declaration
To declare a function, you use the function keyword and specify a name for the function. For example:
Here, we have "declared" a function called generateIntro . You see we use the function keyword followed by the name of the function: "generateIntro"
Now let's look at function expression.
Function Expression
Here, you create a function expression and assign it to a variable that can be called. You can do this in two ways.
Function Expressions with the function keyword
One way to do this is to use the function keyword without a name, which makes it an anonymous function. Here's how:
As you see here, we have the function keyword without a name for the function. This makes it an expression, which you have to assign to a variable (as we have done to generateIntro here).
Note: you can use const , let or var to declare the variable. You can learn more about the differences between these keywords in this article
If we use the function keyword without a name, we create a function expression, which we have to assign to a variable, else we get an error. Here's what I mean:
We get an error: SyntaxError: Function statements require a function name . Without assigning it to a variable, JavaScript assumes it is a statement, and as the error says, you must provide a function name.
But when you assign it to a variable, you assign the expression, and when you call the variable ( variable() ), it will execute the logic of the function expression assigned to it.
Arrow function expressions
You can also create function expressions with arrow functions. Arrow functions, introduced in ES6 allow you to write functions in a short manner. But arrow functions cannot be declared; they can only be expressed . Here's an example:
The arrow function here is (args) => {...} . This is a function expression that we have assigned to generateIntro .
For the rest of this article, I'll focus on function expressions created with the function keyword but know that it also applies to arrow functions.
Function Declarations vs Function Expressions
So what's the difference between these ways of creating functions and why does it matter?
It matters because these functions have different behaviors. And depending on what you want to achieve, one may be preferred over the other.
1. Expressed functions cannot be used before initialization
You can use a declared function before the line it was initialized. Here's what I mean:
As you see here, we used sum on line 1, which is actually before the line was declared. What happens here is hoisting . sum is hoisted to the top of the code before the whole code is executed. This makes sum accessible before the line where it was actually created in the code.
When it comes to hoisting, all functions and variables are hoisted . But, functions created with function expressions cannot be "used" before their initialization.
Let's see an example using a function expression created with the function keyword:
We get an error: ReferenceError: Cannot access 'sum' before initialization . We get this error because when you declare variables with let or const (like we did for sum here), they are hoisted, but without a default initialization. You can learn more about what happens here in this article: the hoisting behavior in let and const
Let's say we create the variable with var instead:
Now we get a new error: TypeError: sum is not a function . Although var variables are hoisted, they are hoisted with a default initialization of undefined . So attempting to call it like a function, that is undefined() , throws the error that "sum is not a function".
The same error would occur if it were an arrow function.
Therefore, only declared functions can be used before initialization .
2. Expressed functions need to be assigned to be used later
With declared functions, you already have the name: function name... . So you can use the function later: name() . But with function expressions, there's no name as we saw. It would be impossible to use such a function later unless we assigned it to a variable:
Here, we assigned the function expression to printName . Now we can use that function logic later by calling printName() .
3. Anonymous functions are useful for anonymous operations
There are cases where you do not need to use a function later. You can execute the function instantly. In such cases, you do not need a name, so you can use a function expression instead of a declaration.
Let's see some examples.
Immediately Invoked Function Expressions (IIFEs)
IIFEs are functions that are immediately invoked after creation. Here's an example:
Or the arrow function equivalent:
This is an IIFE where we create a function that executes console.log('deeecode') . Immediately after creating the function, we execute it as you see at the end ( () ). Here, we do not intend to use the function later, so a function expression works fine.
Using a function declaration here will not throw an error, but the name of the function will be inaccessible:
Using a function declaration, the IIFE is executed, but you cannot access the name of the function outside the parenthesis.
Callback Functions
When using callback functions, you can also pass anonymous functions (function expressions). For example, using the forEach method of arrays which expects a callback function, we can use an anonymous function.
The syntax of the forEach method is:
forEach loops through each item in an array and executes the callback function on them. Let's see how we use a function expression for this:
As you see here, we passed a function expression (anonymous function) as an argument to forEach .
You can pass a function declaration instead, and the callback function will work. But, as we saw earlier, you won't be able to access the function later:
As you see here, print is declared and used as the callback function, but you cannot access print afterward.
Function declarations and function expressions are terms you would hear a lot around functions in JavaScript You can use function expressions to perform similar logic with function declarations, but it is worth noting the differences.
In this article, we've seen how function expressions and different from function declarations.
If you enjoyed this article, please give it a share :)
Developer Advocate and Content Creator passionate about sharing my knowledge on Tech. I simplify JavaScript / ReactJS / NodeJS / Frameworks / TypeScript / et al My YT channel: youtube.com/c/deeecode
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Skip to main content
- Select language
- Skip to search
- function declaration
Function declaration hoisting
Using function.
The function declaration defines a function with the specified parameters.
You can also define functions using the Function constructor and a function expression .
Description
A function created with a function declaration is a Function object and has all the properties, methods and behavior of Function objects. See Function for detailed information on functions.
A function can also be created using an expression (see function expression ).
By default, functions return undefined . To return any other value, the function must have a return statement that specifies the value to return.
Conditionally created functions
Functions can be conditionally declared, that is, a function statement can be nested within an if statement. Most browsers other than Mozilla will treat such conditional declarations as an unconditional declaration and create the function whether the condition is true or not, see this article for an overview. For this reason, they should not be used — for conditional creation use function expressions instead.
Function declarations in JavaScript are hoisting the function definition. You can use the function before you declared it:
Note that function expressions are not hoisted:
The following code declares a function that returns the total amount of sales, when given the number of units sold of products a , b , and c .
Specifications
Browser compatibility.
- Functions and function scope
- function expression
- function* statement
- function* expression
- Arrow functions
- GeneratorFunction
- async function
- async function expression
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- class expression
- delete operator
- in operator
- new operator
- void operator
- Legacy generator function
- for each...in
- try...catch
- Arguments object
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
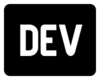
DEV Community

Posted on May 15
Understanding JavaScript Function Declarations: 'function' vs 'const'
JavaScript , as a versatile and dynamic language, offers multiple ways to define functions. Among these, 'function' declarations and 'const' function expressions stand out as two common approaches. Understanding the differences between them is crucial for writing efficient and error-free JavaScript code. In this article, we'll explore the nuances of 'function' declarations and 'const' function expressions, their implications, and best practices.
Exploring the Basics ' function ' Declarations: These are traditional function definitions that are hoisted within their scope. This means you can call them before they are declared in the code.
' const ' Function Expressions: When defining functions using 'const', it creates a function expression. Unlike 'function' declarations, 'const' function expressions are not hoisted and result in an error when called before declaration.
Understanding Hoisting in JavaScript Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope during compilation. ' function ' declarations are hoisted, allowing them to be called before their declaration in the code. Conversely, ' const ' function expressions are not hoisted, and calling them before declaration will result in an error.
Practical Example

Conclusion Understanding the differences between ' function ' declarations and ' const ' function expressions enables cleaner, more efficient JavaScript code. By mastering hoisting implications and choosing the right method, developers enhance code reliability and maintainability. Whether leveraging hoisting or constancy, mastering these concepts is essential for JavaScript proficiency.
Top comments (1)
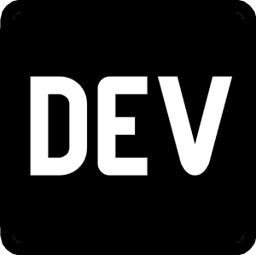
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Bangkok 🇹🇭
- Joined Jun 1, 2018
When defining functions using 'const', it creates a function expression
You can't 'define' a function using const - the act of using const in this way merely assigns the function (defined by the function expression) to the declared variable. You could just as well use let - it would have the same effect...
A 'function expression' is an expression that has a function as its value. In the examples above, the function expressions are everything following the = in the declaration and subsequent assignment of add1 and add2 .
The function keyword can be used both to create a function declaration statement, and also a function expression. If the keyword is used in a position in the code where a statement is invalid, then it will create a function expression. Conversely, if the keyword is used in a position where a statement is valid - it will be interpreted as a function declaration statement. A function declaration statement requires a name for the function. We can see evidence of the 'function' keyword being interpreted as a statement if we try to use it without a name in the console (note the use of 'function statement' in the error):
Now some examples of using the 'function' keyword in both ways:
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

How to use Promise chaining in Javascript.
Makoto Tsuga - May 23
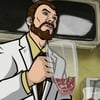
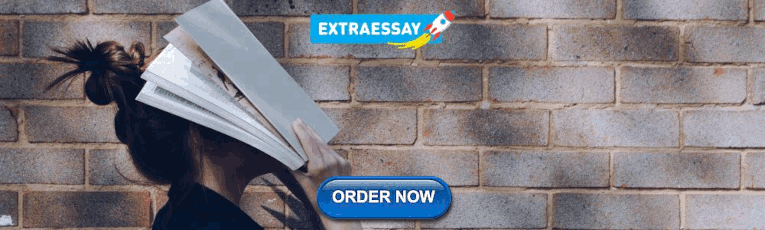
Make your sites more accessible by setting max icon widths
CodeWithCaen - May 23
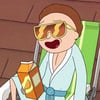
How to Create a User using Tinker in Laravel
saim - May 23

8 Reasons Why Flutter is the Future of App Development
Nilesh Payghan - May 23
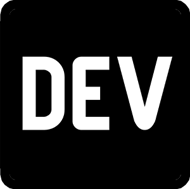
We're a place where coders share, stay up-to-date and grow their careers.
JavaScript Function Declarations vs Function Expressions
Author: WittCode
Created: 10/31/2022

Learn what function declarations and function expressions are and how they are similar/different with hoisting , blocks, scope, and global space pollution.
Function Declaration
Function declarations start with the word function, similar to how variable declarations start with either var , let , or const .
Function declarations are functions with a name, here the name of the function is myDeclaration .
Function Expression
A function expression is a function that is part of an assignment expression. For example, capturing the function in a variable .
This is a named function expression as the function is given a name, myExpressionFunction . Named function expressions are only available inside the scope of the newly defined function.
The main purpose of named function expressions is for debugging as they allow us to check the stack trace for the name of the function. Anonymous functions , or functions that have no name, are often used in function expressions .
However, function expressions are most often created with the ES6 arrow syntax.
Hoisting is when the JavaScript interpreter , prior to executing code, moves the declaration of functions, variables , and classes to the top of their scope . In other words, hoisting determines when functions, variables , and classes are accessible after being declared. Function declarations are hoisted, meaning we can call them before they are declared.
Function expressions are not hoisted, meaning they can only be called after they have been declared.
Blocks and Function Declarations
Function declarations are not statements, meaning using them inside a block should be considered a syntax error. This is because historically, different browsers would parse these functions differently leading to inconsistencies.
This is because these blocks can only contain statements. However, we can use function expressions in blocks.
However, the introduction of strict mode in ES5 resolved this issue by scoping function declarations to their block. To use strict mode , place use strict before any other statement.
Global Space Pollution
When it comes to scope, function declarations are always local to the current scope.
We can use a function expression with the keywords let or const to avoid polluting the global namespace. Polluting the global scope is bad because we only want to have code accessible when and where we need it.
However, if we use a function expression with the var keyword in the outermost scope , it will still pollute the global namespace.
But there we go! If you enjoyed this article please consider supporting me by downloading my chrome extension WittCepter from the chrome web store or checking out my YouTube channel WittCode!
- Console Output
- Read from Text File
- Variable Declaration
- Global Variables
- Determine Type
- If Statement
- Switch Case
- Ternary Operator
- Do-While Loop
- Try Catch Block
- Function Declaration
- Anonymous Functions
- Function Assignment
- Object Declaration
- Create Object using Constructor
- Constructor
Version: 1.8.5
Function assignment in javascript.
Used to call and/or provide the context (object) for a function that is dependant on an object. Often, functions are assigned to objects and access object members using the 'this' keyword.
Typically used when a function depends on different object types, or for passing parameters.
Function.prototype.call - JavaScript | MDN Function.prototype.apply - JavaScript | MDN Function.prototype.bind - JavaScript | MDN
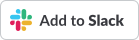
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript variables, variables are containers for storing data.
JavaScript Variables can be declared in 4 ways:
- Automatically
- Using const
In this first example, x , y , and z are undeclared variables.
They are automatically declared when first used:
It is considered good programming practice to always declare variables before use.
From the examples you can guess:
- x stores the value 5
- y stores the value 6
- z stores the value 11
Example using var
The var keyword was used in all JavaScript code from 1995 to 2015.
The let and const keywords were added to JavaScript in 2015.
The var keyword should only be used in code written for older browsers.
Example using let
Example using const, mixed example.
The two variables price1 and price2 are declared with the const keyword.
These are constant values and cannot be changed.
The variable total is declared with the let keyword.
The value total can be changed.
When to Use var, let, or const?
1. Always declare variables
2. Always use const if the value should not be changed
3. Always use const if the type should not be changed (Arrays and Objects)
4. Only use let if you can't use const
5. Only use var if you MUST support old browsers.
Just Like Algebra
Just like in algebra, variables hold values:
Just like in algebra, variables are used in expressions:
From the example above, you can guess that the total is calculated to be 11.
Variables are containers for storing values.
Advertisement
JavaScript Identifiers
All JavaScript variables must be identified with unique names .
These unique names are called identifiers .
Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume).
The general rules for constructing names for variables (unique identifiers) are:
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter.
- Names can also begin with $ and _ (but we will not use it in this tutorial).
- Names are case sensitive (y and Y are different variables).
- Reserved words (like JavaScript keywords) cannot be used as names.
JavaScript identifiers are case-sensitive.
The Assignment Operator
In JavaScript, the equal sign ( = ) is an "assignment" operator, not an "equal to" operator.
This is different from algebra. The following does not make sense in algebra:
In JavaScript, however, it makes perfect sense: it assigns the value of x + 5 to x.
(It calculates the value of x + 5 and puts the result into x. The value of x is incremented by 5.)
The "equal to" operator is written like == in JavaScript.
JavaScript Data Types
JavaScript variables can hold numbers like 100 and text values like "John Doe".
In programming, text values are called text strings.
JavaScript can handle many types of data, but for now, just think of numbers and strings.
Strings are written inside double or single quotes. Numbers are written without quotes.
If you put a number in quotes, it will be treated as a text string.
Declaring a JavaScript Variable
Creating a variable in JavaScript is called "declaring" a variable.
You declare a JavaScript variable with the var or the let keyword:
After the declaration, the variable has no value (technically it is undefined ).
To assign a value to the variable, use the equal sign:
You can also assign a value to the variable when you declare it:
In the example below, we create a variable called carName and assign the value "Volvo" to it.
Then we "output" the value inside an HTML paragraph with id="demo":
It's a good programming practice to declare all variables at the beginning of a script.
One Statement, Many Variables
You can declare many variables in one statement.
Start the statement with let and separate the variables by comma :
A declaration can span multiple lines:
Value = undefined
In computer programs, variables are often declared without a value. The value can be something that has to be calculated, or something that will be provided later, like user input.
A variable declared without a value will have the value undefined .
The variable carName will have the value undefined after the execution of this statement:
Re-Declaring JavaScript Variables
If you re-declare a JavaScript variable declared with var , it will not lose its value.
The variable carName will still have the value "Volvo" after the execution of these statements:
You cannot re-declare a variable declared with let or const .
This will not work:
JavaScript Arithmetic
As with algebra, you can do arithmetic with JavaScript variables, using operators like = and + :
You can also add strings, but strings will be concatenated:
Also try this:
If you put a number in quotes, the rest of the numbers will be treated as strings, and concatenated.
Now try this:
JavaScript Dollar Sign $
Since JavaScript treats a dollar sign as a letter, identifiers containing $ are valid variable names:
Using the dollar sign is not very common in JavaScript, but professional programmers often use it as an alias for the main function in a JavaScript library.
In the JavaScript library jQuery, for instance, the main function $ is used to select HTML elements. In jQuery $("p"); means "select all p elements".
JavaScript Underscore (_)
Since JavaScript treats underscore as a letter, identifiers containing _ are valid variable names:
Using the underscore is not very common in JavaScript, but a convention among professional programmers is to use it as an alias for "private (hidden)" variables.
Test Yourself With Exercises
Create a variable called carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
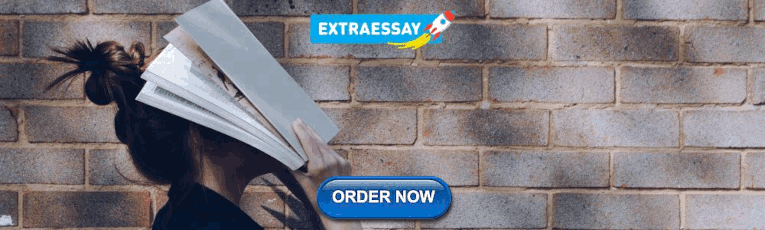
IMAGES
VIDEO
COMMENTS
Function declaration vs. function expression is the real reason why there is a difference demonstrated by Greg. Fun fact: var xyz = function abc(){}; console.log(xyz.name); // Prints "abc" Personally, I prefer the "function expression" declaration because this way I can control the visibility. When I define the function like. var abc = function
The first difference: a name. When you create a function with a name, that is a function declaration. The name may be omitted in function expressions, making that function "anonymous". Function declaration: function doStuff() {}; Function expression: const doStuff = function() {} We often see anonymous functions used with ES6 syntax like so:
Oct 2, 2014 at 18:29. 4. It's actually considered a best practice to use function expressions as then the behavior is more intuitive than with declarations. It reads better as it follows a logical flow, You define it and then call it, if you don't you get an error, which is the expected behavior.
A function declaration must have a function name. A function expression is similar to a function declaration without the function name. Function declaration does not require a variable assignment. Function expressions can be stored in a variable assignment. These are executed before any other code.
A Function Expression is created when the execution reaches it and is usable only from that moment. Once the execution flow passes to the right side of the assignment let sum = function… - here we go, the function is created and can be used (assigned, called, etc. ) from now on. Function Declarations are different.
The preceding statement calls the function with an argument of 5.The function executes its statements and returns the value 25.. Functions must be in scope when they are called, but the function declaration can be hoisted (appear below the call in the code). The scope of a function declaration is the function in which it is declared (or the entire program, if it is declared at the top level).
A function declaration creates a Function object. Each time when a function is called, it returns the value specified by the last executed return statement, or undefined if the end of the function body is reached. See functions for detailed information on functions.. function declarations behave like a mix of var and let:. Like let, in strict mode, function declarations are scoped to the most ...
Function Declaration: A Function Declaration( or a Function Statement) defines a function with the specified parameters without requiring a variable assignment. They exist on their own, i.e, they are standalone constructs and cannot be nested within a non-function block. A function is declared using the function keyword.. Syntax:
Expressed functions need to be assigned to be used later. With declared functions, you already have the name: function name.... So you can use the function later: name(). But with function expressions, there's no name as we saw. It would be impossible to use such a function later unless we assigned it to a variable:
By default, if a function's execution doesn't end at a return statement, or if the return keyword doesn't have an expression after it, then the return value is undefined.The return statement allows you to return an arbitrary value from the function. One function call can only return one value, but you can simulate the effect of returning multiple values by returning an object or array and ...
Description. A function created with a function declaration is a Function object and has all the properties, methods and behavior of Function objects. See Function for detailed information on functions. A function can also be created using an expression (see function expression ). By default, functions return undefined.
A 'function expression' is an expression that has a function as its value. In the examples above, the function expressions are everything following the = in the declaration and subsequent assignment of add1 and add2. The function keyword can be used both to create a function declaration statement, and also a function expression. If the keyword ...
Function Declaration ... here the name of the function is myDeclaration. Function Expression A function expression is a function that is part of an assignment expression. For example, capturing the function in a variable. ... Hoisting is when the JavaScript interpreter, prior to executing code, moves the declaration of functions, ...
There are three common ways of using functions and each has its own name — "Function Declaration" and "Function Expression". There is also "Arrow Function", which has the shortest syntax. The syntactical differences between them are like so: Function Declaration is declared as a separate statement, in the main code flow. return a + b;
When that happens only one is available. The innermost reference (the function name) is hidden (unavailable) under the outermost reference (the one declared by var). If the two references are different then both are available. When a function is declared and assigned you get the benefit of both approaches.
Remove the word "function" and place arrow between the argument and opening body brace (a) => { return a + 100; }; // 2. Remove the body braces and word "return" — the return is implied. (a) => a + 100; // 3. Remove the parameter parentheses a => a + 100; In the example above, both the parentheses around the parameter and the braces around ...
Function Assignment in JavaScript. Used to call and/or provide the context (object) for a function that is dependant on an object. Often, functions are assigned to objects and access object members using the 'this' keyword.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Variable declared without a value will have the value undefined. The variable carname will have the value undefined after the execution of the following statement: var carname; var hoisting. In JavaScript, a variable can be declared after being used. bla = 2.
All JavaScript variables must be identified with unique names. These unique names are called identifiers. Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume). The general rules for constructing names for variables (unique identifiers) are: Names can contain letters, digits, underscores, and dollar signs.
The boolean operators in JavaScript can return an operand, and not always a boolean result as in other languages. The Logical OR operator ( ||) returns the value of its second operand, if the first one is falsy, otherwise the value of the first operand is returned. For example: "foo" || "bar"; // returns "foo".