How to Call By Value and Call By Reference in Python
In this Python tutorial, I will show you how to call by value and call by reference in Python .
Both the concepts of call by value and call by reference are very helpful in memory management, data manipulation, and even API development.
In this tutorial, I have explained the meaning of call by value and reference and, with examples, shown how to implement these concepts and how they differ.
Let’s begin,
Table of Contents
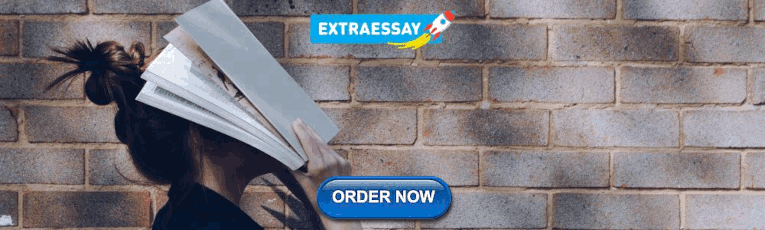
What does Call By Value and Call By Reference in Python Mean?
In several programming languages, you can pass the data to the function argument in two ways: by value and reference (address).
- Calling by value means passing the value to the function’s argument; if any changes are made to that value within the function, then the original value outside the function remains the same.
- Whereas Calling by reference means passing the address of a value to the function’s argument, if any changes are made to that value within the function, they also change the value outside the function.
Understanding these two concepts is very helpful because it allows you to manage your data in a better way.
But I want to tell you one thing: Python does not have the concept ‘call by value’ like languages like C , C++ , Java , etc. Instead, it passes the object reference by value.
This means you pass the immutable objects, such as integers and tuples, to the function argument; it acts like a call by value because the nature of immutable objects prevents any changes within the function from affecting the original objects.
If we talk about the call by reference in Python, mutable objects, such as lists, dictionaries, etc, are passed to the function arguments. Since the function gets the reference to the original object, whatever modification is made to objects changes the original object.
Your concept of call by value and reference must have been clear until now; if not, don’t worry. You will understand it through examples.
Pass By Value and Pass by Reference in Python
Before beginning, remember one thing: the original value doesn’t change in the call by value, but it does change in the call by reference. Also, I will use the words pass or call interchangeably, depending upon the context.
Pass By Reference in Python
As I said, when mutable objects such as lists and dictionaries are passed to the function arguments. Any changes made to these objects within a function by an operation also change the original objects outside the function.
Let’s see, first, create a dictionary named ‘user_profile’ as shown below.
In the above code, the dictionary user_profile contains two key-value pairs: username and email as keys and Tylor and [email protected] as values, respectively.
Now, create a function that updates the user profile email as shown below.
In the above code, the update_user_profile function takes two arguments: the user data through user_info and the user’s new email through user_email that you want to update.
Before calling the function with those values, note the user’s current email; now, let’s call the function with user_profile and new email ‘[email protected]’ as shown below.

Look at the output when you call the function update_user_profile, passing it a dictionary ‘user_profile’ whose email you want to update and the new email ‘[email protected]’. It updates both emails inside and outside the function.
This means it changes the original dictionary ‘user_profile’ outside the function you created.
This is how to call or pass by reference in Python.
Pass By Value in Python
In pass-by-value, when immutable objects such as integers, strings and tuples are passed to the function arguments. Any changes made to these objects within a function by an operation don’t change the original objects outside the function.
To understand this concept, let’s take a simple example.
Create a variable value and initialize it with 5, as shown below.
Create a function named ‘addition’ that accepts the value and increments it by 1, as shown below.
Call the function by passing the variable value (containing 5) you created, as shown below.

Look at the output; the value outside the function is not affected. It contains the same value, which is 5, but the value in the function is incremented by one; it includes the value 6.
Here, when you pass the variable value to function addition(value) , the copy of the variable is passed (in reality, it is an immutable object), so within the function, this line value = value + 1 adds the one to the copy of the variable, not on the original one.
So, only the variable value within a function is incremented, not the variable value outside the function.
This is how to pass by value in Python.
Difference Between Pass By Value and Pass By Reference in Python
Let’s understand the pass-by-reference vs. pass-by-value Python.
In this Python tutorial, you learned how to call by value and call by reference in Python with examples.
Additionally, You learned what call by value and reference means and the difference between them.
You may like to read:
- Write a Program to Find a Perfect Number in Python
- How to Initialize Dictionary Python with 0
- Write a Program to Check Whether a Number is Prime or not in Python

I am Bijay Kumar, a Microsoft MVP in SharePoint. Apart from SharePoint, I started working on Python, Machine learning, and artificial intelligence for the last 5 years. During this time I got expertise in various Python libraries also like Tkinter, Pandas, NumPy, Turtle, Django, Matplotlib, Tensorflow, Scipy, Scikit-Learn, etc… for various clients in the United States, Canada, the United Kingdom, Australia, New Zealand, etc. Check out my profile .
Pass-by-value, reference, and assignment | Pydon't 🐍
When you call a function in Python and give it some arguments... Are they passed by value? No! By reference? No! They're passed by assignment.
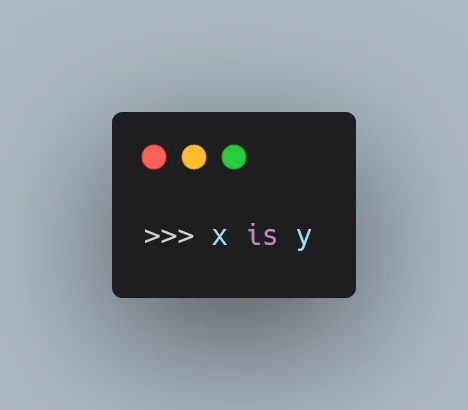
(If you are new here and have no idea what a Pydon't is, you may want to read the Pydon't Manifesto .)
Introduction
Many traditional programming languages employ either one of two models when passing arguments to functions:
- some languages use the pass-by-value model; and
- most of the others use the pass-by-reference model.
Having said that, it is important to know the model that Python uses, because that influences the way your code behaves.
In this Pydon't, you will:
- see that Python doesn't use the pass-by-value nor the pass-by-reference models;
- understand that Python uses a pass-by-assignment model;
- learn about the built-in function id ;
- create a better understanding for the Python object model;
- realise that every object has 3 very important properties that define it;
- understand the difference between mutable and immutable objects;
- learn the difference between shallow and deep copies; and
- learn how to use the module copy to do both types of object copies.
You can now get your free copy of the ebook “Pydon'ts – Write elegant Python code” on Gumroad .
Is Python pass-by-value?
In the pass-by-value model, when you call a function with a set of arguments, the data is copied into the function. This means that you can modify the arguments however you please and that you won't be able to alter the state of the program outside the function. This is not what Python does, Python does not use the pass-by-value model.
Looking at the snippet of code that follows, it might look like Python uses pass-by-value:
This looks like the pass-by-value model because we gave it a 3, changed it to a 4, and the change wasn't reflected on the outside ( a is still 3).
But, in fact, Python is not copying the data into the function.
To prove this, I'll show you a different function:
As we can see, the list l , that was defined outside of the function, changed after calling the function clearly_not_pass_by_value . Hence, Python does not use a pass-by-value model.
Is Python pass-by-reference?
In a true pass-by-reference model, the called function gets access to the variables of the callee! Sometimes, it can look like that's what Python does, but Python does not use the pass-by-reference model.
I'll do my best to explain why that's not what Python does:
If Python used a pass-by-reference model, the function would've managed to completely change the value of l outside the function, but that's not what happened, as we can see.
Let me show you an actual pass-by-reference situation.
Here's some Pascal code:
Look at the last lines of that code:
- we assign 2 to x with x := 2 ;
- we print x ;
- we call foo with x as argument; and
- we print x again.
What's the output of this program?
I imagine that most of you won't have a Pascal interpreter lying around, so you can just go to tio.run and run this code online
If you run this, you'll see that the output is
which can be rather surprising, if the majority of your programming experience is in Python!
The procedure foo effectively received the variable x and changed the value that it contained. After foo was done, the variable x (that lives outside foo ) had a different value. You can't do anything like this in Python.
Python object model
To really understand the way Python behaves when calling functions, it's best if we first understand what Python objects are, and how to characterise them.
The three characteristics of objects
In Python, everything is an object, and each object is characterised by three things:
- its identity (an integer that uniquely identifies the object, much like social security numbers identify people);
- a type (that identifies the operations you can do with your object); and
- the object's content.
Here is an object and its three characteristics:
As we can see above, id is the built-in function you use to query the identity of an object, and type is the built-in function you use to query the type of an object.
(Im)mutability
The (im)mutability of an object depends on its type. In other words, (im)mutability is a characteristic of types, not of specific objects!
But what exactly does it mean for an object to be mutable? Or for an object to be immutable?
Recall that an object is characterised by its identity, its type, and its contents. A type is mutable if you can change the contents of its objects without changing its identity and its type.
Lists are a great example of a mutable data type. Why? Because lists are containers : you can put things inside lists and you can remove stuff from inside those same lists.
Below, you can see how the contents of the list obj change as we make method calls, but the identity of the list remains the same:
However, when dealing with immutable objects, it's a completely different story. If we check an English dictionary, this is what we get for the definition of “immutable”:
adjective: immutable – unchanging over time or unable to be changed.
Immutable objects' contents never change. Take a string as an example:
Strings are a good example for this discussion because, sometimes, they can look mutable. But they are not!
A very good indicator that an object is immutable is when all its methods return something. This is unlike list's .append method, for example! If you use .append on a list, you get no return value. On the other hand, whatever method you use on a string, the result is returned to you:
Notice how obj wasn't updated automatically to "HELLO, WORLD!" . Instead, the new string was created and returned to you.
Another great hint at the fact that strings are immutable is that you cannot assign to its indices:
This shows that, when a string is created, it remains the same. It can be used to build other strings, but the string itself always. stays. unchanged.
As a reference, int , float , bool , str , tuple , and complex are the most common types of immutable objects; list , set , and dict are the most common types of mutable objects.
Variable names as labels
Another important thing to understand is that a variable name has very little to do with the object itself.
In fact, the name obj was just a label that I decided to attach to the object that has identity 2698212637504, has the list type, and contents 1, 2, 3.
Just like I attached the label obj to that object, I can attach many more names to it:
Again, these names are just labels. Labels that I decided to stick to the same object. How can we know it's the same object? Well, all their “social security numbers” (the ids) match, so they must be the same object:
Therefore, we conclude that foo , bar , baz , and obj , are variable names that all refer to the same object.
The operator is
This is exactly what the operator is does: it checks if the two objects are the same .
For two objects to be the same, they must have the same identity:
It is not enough to have the same type and contents! We can create a new list with contents [1, 2, 3] and that will not be the same object as obj :
Think of it in terms of perfect twins. When two siblings are perfect twins, they look identical. However, they are different people!
Just as a side note, but an important one, you should be aware of the operator is not .
Generally speaking, when you want to negate a condition, you put a not in front of it:
So, if you wanted to check if two variables point to different objects, you could be tempted to write
However, Python has the operator is not , which is much more similar to a proper English sentence, which I think is really cool!
Therefore, the example above should actually be written
Python does a similar thing for the in operator, providing a not in operator as well... How cool is that?!
Assignment as nicknaming
If we keep pushing this metaphor forward, assigning variables is just like giving a new nickname to someone.
My friends from middle school call me “Rojer”. My friends from college call me “Girão”. People I am not close to call me by my first name – “Rodrigo”. However, regardless of what they call me, I am still me , right?
If one day I decide to change my haircut, everyone will see the new haircut, regardless of what they call me!
In a similar fashion, if I modify the contents of an object, I can use whatever nickname I prefer to see that those changes happened. For example, we can change the middle element of the list we have been playing around with:
We used the nickname foo to modify the middle element, but that change is visible from all other nicknames as well.
Because they all pointed at the same list object.
Python is pass-by-assignment
Having laid out all of this, we are now ready to understand how Python passes arguments to functions.
When we call a function, each of the parameters of the function is assigned to the object they were passed in. In essence, each parameter now becomes a new nickname to the objects that were given in.
Immutable arguments
If we pass in immutable arguments, then we have no way of modifying the arguments themselves. After all, that's what immutable means: “doesn't change”.
That is why it can look like Python uses the pass-by-value model. Because the only way in which we can have the parameter hold something else is by assigning it to a completely different thing. When we do that, we are reusing the same nickname for a different object:
In the example above, when we call foo with the argument 5 , it's as if we were doing bar = 5 at the beginning of the function.
Immediately after that, we have bar = 3 . This means “take the nickname "bar" and point it at the integer 3 ”. Python doesn't care that bar , as a nickname (as a variable name) had already been used. It is now pointing at that 3 !
Mutable arguments
On the other hand, mutable arguments can be changed. We can modify their internal contents. A prime example of a mutable object is a list: its elements can change (and so can its length).
That is why it can look like Python uses a pass-by-reference model. However, when we change the contents of an object, we didn't change the identity of the object itself. Similarly, when you change your haircut or your clothes, your social security number does not change:
Do you understand what I'm trying to say? If not, drop a comment below and I'll try to help.
Beware when calling functions
This goes to show you should be careful when defining your functions. If your function expects mutable arguments, you should do one of the two:
- do not mutate the argument in any way whatsoever; or
- document explicitly that the argument may be mutated.
Personally, I prefer to go with the first approach: to not mutate the argument; but there are times and places for the second approach.
Sometimes, you do need to take the argument as the basis for some kind of transformation, which would mean you would want to mutate the argument. In those cases, you might think about doing a copy of the argument (discussed in the next section), but making that copy can be resource intensive. In those cases, mutating the argument might be the only sensible choice.
Making copies
Shallow vs deep copies.
“Copying an object” means creating a second object that has a different identity (therefore, is a different object) but that has the same contents. Generally speaking, we copy one object so that we can work with it and mutate it, while also preserving the first object.
When copying objects, there are a couple of nuances that should be discussed.
Copying immutable objects
The first thing that needs to be said is that, for immutable objects, it does not make sense to talk about copies.
“Copies” only make sense for mutable objects. If your object is immutable, and if you want to preserve a reference to it, you can just do a second assignment and work on it:
Or, sometimes, you can just call methods and other functions directly on the original, because the original is not going anywhere:
So, we only need to worry about mutable objects.
Shallow copy
Many mutable objects can contain, themselves, mutable objects. Because of that, two types of copies exist:
- shallow copies; and
- deep copies.
The difference lies in what happens to the mutable objects inside the mutable objects.
Lists and dictionaries have a method .copy that returns a shallow copy of the corresponding object.
Let's look at an example with a list:
First, we create a list inside a list, and we copy the outer list. Now, because it is a copy , the copied list isn't the same object as the original outer list:
But if they are not the same object, then we can modify the contents of one of the lists, and the other won't reflect the change:
That's what we saw: we changed the first element of the copy_list , and the outer_list remained unchanged.
Now, we try to modify the contents of sublist , and that's when the fun begins!
When we modify the contents of sublist , both the outer_list and the copy_list reflect those changes...
But wasn't the copy supposed to give me a second list that I could change without affecting the first one? Yes! And that is what happened!
In fact, modifying the contents of sublist doesn't really modify the contents of neither copy_list nor outer_list : after all, the third element of both was pointing at a list object, and it still is! It's the (inner) contents of the object to which we are pointing that changed.
Sometimes, we don't want this to happen: sometimes, we don't want mutable objects to share inner mutable objects.
Common shallow copy techniques
When working with lists, it is common to use slicing to produce a shallow copy of a list:
Using the built-in function for the respective type, on the object itself, also builds shallow copies. This works for lists and dictionaries, and is likely to work for other mutable types.
Here is an example with a list inside a list:
And here is an example with a list inside a dictionary:
When you want to copy an object “thoroughly”, and you don't want the copy to share references to inner objects, you need to do a “deep copy” of your object. You can think of a deep copy as a recursive algorithm.
You copy the elements of the first level and, whenever you find a mutable element on the first level, you recurse down and copy the contents of those elements.
To show this idea, here is a simple recursive implementation of a deep copy for lists that contain other lists:
We can use this function to copy the previous outer_list and see what happens:
As you can see here, modifying the contents of sublist only affected outer_list indirectly; it didn't affect copy_list .
Sadly, the list_deepcopy method I implemented isn't very robust, nor versatile, but the Python Standard Library has got us covered!
The module copy and the method deepcopy
The module copy is exactly what we need. The module provides two useful functions:
- copy.copy for shallow copies; and
- copy.deepcopy for deep copies.
And that's it! And, what is more, the method copy.deepcopy is smart enough to handle issues that might arise with circular definitions, for example! That is, when an object contains another that contains the first one: a naïve recursive implementation of a deep copy algorithm would enter an infinite loop!
If you write your own custom objects and you want to specify how shallow and deep copies of those should be made, you only need to implement __copy__ and __deepcopy__ , respectively!
It's a great module, in my opinion.
Examples in code
Now that we have gone deep into the theory – pun intended –, it is time to show you some actual code that plays with these concepts.
Mutable default arguments
Let's start with a Twitter favourite:
Python 🐍 is an incredible language but sometimes appears to have quirks 🤪 For example, one thing that often confuses beginners is why you shouldn't use lists as default values 👇 Here is a thread 👇🧵 that will help you understand this 💯 pic.twitter.com/HVhPjS2PSH — Rodrigo 🐍📝 (@mathsppblog) October 5, 2021
Apparently, it's a bad idea to use mutable objects as default arguments. Here is a snippet showing you why:
The function above appends an element to a list and, if no list is given, appends it to an empty list by default.
Great, let's put this function to good use:
We use it once with 1 , and we get a list with the 1 inside. Then, we use it to append a 1 to another list we had. And finally, we use it to append a 3 to an empty list... Except that's not what happens!
As it turns out, when we define a function, the default arguments are created and stored in a special place:
What this means is that the default argument is always the same object. Therefore, because it is a mutable object, its contents can change over time. That is why, in the code above, __defaults__ shows a list with two items already.
If we redefine the function, then its __defaults__ shows an empty list:
This is why, in general, mutable objects shouldn't be used as default arguments.
The standard practice, in these cases, is to use None and then use Boolean short-circuiting to assign the default value:
With this implementation, the function now works as expected:
is not None
Searching through the Python Standard Library shows that the is not operator is used a bit over 5,000 times. That's a lot.
And, by far and large, that operator is almost always followed by None . In fact, is not None appears 3169 times in the standard library!
x is not None does exactly what it's written: it checks if x is None or not.
Here is a simple example usage of that, from the argparse module to create command line interfaces:
Even without a great deal of context, we can see what is happening: when displaying command help for a given section, we may want to indent it (or not) to show hierarchical dependencies.
If a section's parent is None , then that section has no parent, and there is no need to indent. In other words, if a section's parent is not None , then we want to indent it. Notice how my English matches the code exactly!
Deep copy of the system environment
The method copy.deepcopy is used a couple of times in the standard library, and here I'd like to show an example usage where a dictionary is copied.
The module os provides the attribute environ , similar to a dictionary, that contains the environment variables that are defined.
Here are a couple of examples from my (Windows) machine:
The module http.server provides some classes for basic HTTP servers.
One of those classes, CGIHTTPRequestHandler , implements a HTTP server that can also run CGI scripts and, in its run_cgi method, it needs to set a bunch of environment variables.
These environment variables are set to give the necessary context for the CGI script that is going to be ran. However, we don't want to actually modify the current environment!
So, what we do is create a deep copy of the environment, and then we modify it to our heart's content! After we are done, we tell Python to execute the CGI script, and we provide the altered environment as an argument.
The exact way in which this is done may not be trivial to understand. I, for one, don't think I could explain it to you. But that doesn't mean we can't infer parts of it:
Here is the code:
As we can see, we copied the environment and defined some variables. Finally, we created a new subprocess that gets the modified environment.
Here's the main takeaway of this Pydon't, for you, on a silver platter:
“ Python uses a pass-by-assignment model, and understanding it requires you to realise all objects are characterised by an identity number, their type, and their contents. ”
This Pydon't showed you that:
- Python doesn't use the pass-by-value model, nor the pass-by-reference one;
- Python uses a pass-by-assignment model (using “nicknames”);
- its identity;
- its type; and
- its contents.
- the id function is used to query an object's identifier;
- the type function is used to query an object's type;
- the type of an object determines whether it is mutable or immutable;
- shallow copies copy the reference of nested mutable objects;
- deep copies perform copies that allow one object, and its inner elements, to be changed without ever affecting the copy;
- copy.copy and copy.deepcopy can be used to perform shallow/deep copies; and
- you can implement __copy__ and __deepcopy__ if you want your own objects to be copiable.
If you prefer video content, you can check this YouTube video , which was inspired by this article.
If you liked this Pydon't be sure to leave a reaction below and share this with your friends and fellow Pythonistas. Also, subscribe to the newsletter so you don't miss a single Pydon't!
Become a better Python 🐍 developer 🚀
+35 chapters. +400 pages. Hundreds of examples. Over 30,000 readers!
My book “Pydon'ts” teaches you how to write elegant, expressive, and Pythonic code, to help you become a better developer. >>> Download it here 🐍🚀 .
- Python 3 Docs, Programming FAQ, “How do I write a function with output parameters (call by reference)?”, https://docs.python.org/3/faq/programming.html#how-do-i-write-a-function-with-output-parameters-call-by-reference [last accessed 04-10-2021];
- Python 3 Docs, The Python Standard Library, copy , https://docs.python.org/3/library/copy.html [last accessed 05-10-2021];
- effbot.org, “Call by Object” (via “arquivo.pt”), https://arquivo.pt/wayback/20160516131553/http://effbot.org/zone/call-by-object.htm [last accessed 04-10-2021];
- effbot.org, “Python Objects” (via “arquivo.pt”), https://arquivo.pt/wayback/20191115002033/http://effbot.org/zone/python-objects.htm [last accessed 04-10-2021];
- Robert Heaton, “Is Python pass-by-reference or pass-by-value”, https://robertheaton.com/2014/02/09/pythons-pass-by-object-reference-as-explained-by-philip-k-dick/ [last accessed 04-10-2021];
- StackOverflow question and answers, “How do I pass a variable by reference?”, https://stackoverflow.com/q/986006/2828287 [last accessed 04-10-2021];
- StackOverflow question and answers, “Passing values in Python [duplicate]”, https://stackoverflow.com/q/534375/2828287 [last accessed 04-10-2021];
- Twitter thread by @mathsppblog , https://twitter.com/mathsppblog/status/1445148566721335298 [last accessed 20-10-2021];
Previous Post Next Post
Random Article
Stay in the loop, popular tags.
- 6 April 2024
- 5 March 2024
- 2 February 2024
- 8 January 2024
- 1 December 2023
- 22 November 2023
- 4 October 2023
- 6 September 2023
- 7 August 2023
- 12 July 2023
- 4 June 2023
If there is a 50% chance something will go wrong, then 9 times out of 10 it will.
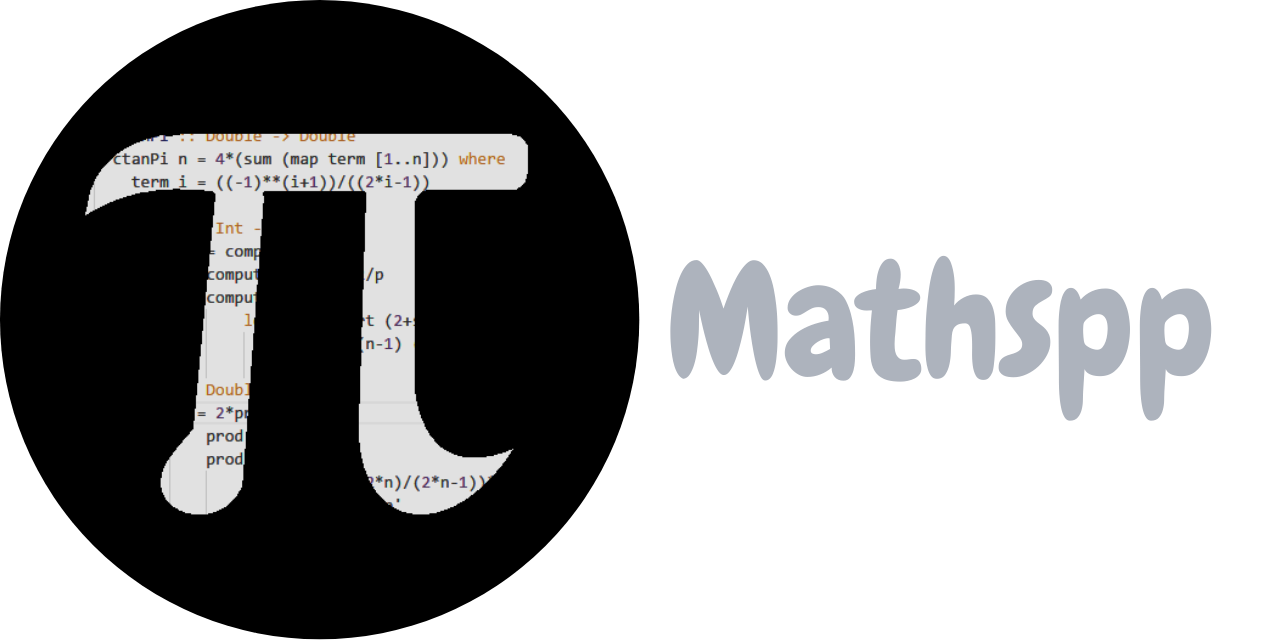
How to pass value by reference in Python?
The concept of a variable in Python is a little different from C, where it is a named memory location with a unique address. In Python, on the other hand, data objects are stored in memory, and a variable is just a label for its easy access. This is why Python is a dynamically typed language, where the type of variable need not be declared before assignment and data decides the type and not other way round (as in C/C++).
Here, an integer object 100 is stored in memory, and for our convenience, a label (which we call as variable) x is assigned to it. The same label assigned to a different object changes its type.
Secondly, the Python interpreter assigns a unique identifier to each object. Python's built-in id() function returns this id, which is roughly equivalent to a memory address.
Note that if x assigned to another variable y , both have same ids, which means both are referring to same object in memory.
As a result, actual and formal arguments involved in a function call have the same id value.
Hence, it can be inferred that in Python, a function is always called by passing a variable by reference. It means, if a function modifies data received from the calling environment, the modification should reflect in the original data. However, this is not always true.
If the actual argument variable represents an immutable object such as int, float, tuple, or a string, any modification inside function will result in creating a different object (which will be local to the called function) and not affect the original variable.
It can be seen that incrementing the received argument (it is an int object which is immutable) creates a new object (with a different id) and doesn't affect the original object referred to x .
Instead, if a mutable object such as a list is passed to function, modification inside a function will be reflected after function call as in the following function.
In the end, we can conclude that even though any object is passed to a function by reference, a function's changes are reflected only in the case of mutable objects and not in immutable objects.
- repr() vs str() in Python
- globals() and locals() Method in Python
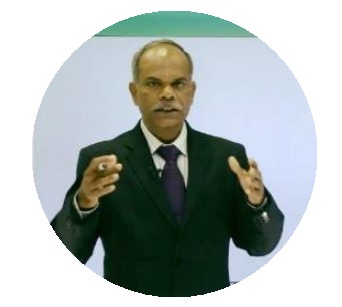
Malhar Lathkar is an independent software professional having 30+ years of experience in various technologies such as Python, Java, Android, PHP, and Databases. He is an author of Python Data Persistence: With SQL and NOSQL Databases
- Entrepreneur
- Productivity
Robert Heaton
Software Engineer / One-track lover / Down a two-way lane
“Suppose I say to Fat, or Kevin says to Fat, “You did not experience God. You merely experienced something with the qualities and aspects and nature and powers and wisdom and goodness of God.” This is like the joke about the German proclivity toward double abstractions; a German authority on English literature declares, “Hamlet was not written by Shakespeare; it was merely written by a man named Shakespeare.” In English the distinction is verbal and without meaning, although German as a language will express the difference (which accounts for some of the strange features of the German mind).”
Valis, p71 (Book-of-the-Month-Club Edition)
Philip K. Dick is not known for his light or digestible prose. The vast majority of his characters are high. Like, really, really, really high. And yet, in the above quote from Valis (published in 1981), he gives a remarkably foresighted explanation of the notoriously misunderstood Python parameter passing paradigm. Plus ça change, plus c’est omnomnomnom drugs.
The two most widely known and easy to understand approaches to parameter passing amongst programming languages are pass-by-reference and pass-by-value. Unfortunately, Python is “pass-by-object-reference”, of which it is often said:
“Object references are passed by value.”
When I first read this smug and overly-pithy definition, I wanted to punch something. After removing the shards of glass from my hands and being escorted out of the strip club, I realised that all 3 paradigms can be understood in terms of how they cause the following 2 functions to behave:
Let’s explore.
The variable is not the object
“Hamlet was not written by Shakespeare; it was merely written by a man named Shakespeare.” Both Python and PKD make a crucial distinction between a thing, and the label we use to refer to that thing. “The man named Shakespeare” is a man. “Shakespeare” is just a name. If we do:
then [] is the empty list. a is a variable that points to the empty list, but a itself is not the empty list. I draw and frequently refer to variables as “boxes” that contain objects; but however you conceive of it, this difference is key.
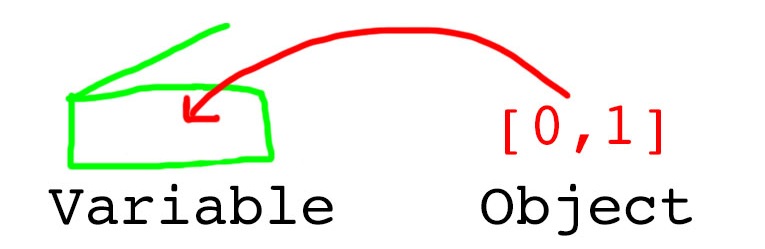
Pass-by-reference
In pass-by-reference, the box (the variable) is passed directly into the function, and its contents (the object represented by the variable) implicitly come with it. Inside the function context, the argument is essentially a complete alias for the variable passed in by the caller. They are both the exact same box, and therefore also refer to the exact same object in memory.
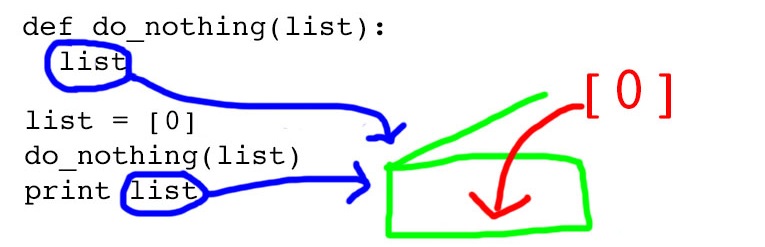
Anything the function does to either the variable or the object it represents will therefore be visible to the caller. For example, the function could completely change the variable’s content, and point it at a completely different object:
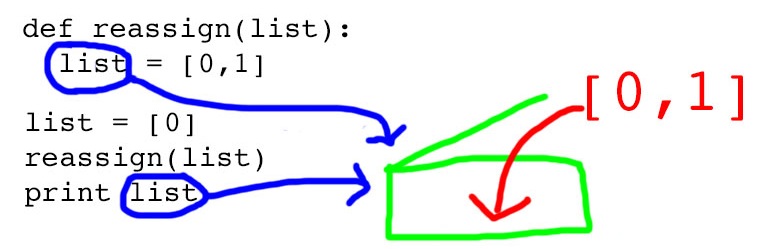
The function could also manipulate the object without reassigning it, with the same effect:
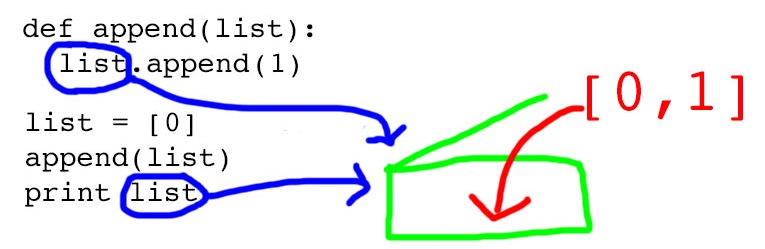
To reiterate, in pass-by-reference, the function and the caller both use the exact same variable and object.
Pass-by-value
In pass-by-value, the function receives a copy of the argument objects passed to it by the caller, stored in a new location in memory.
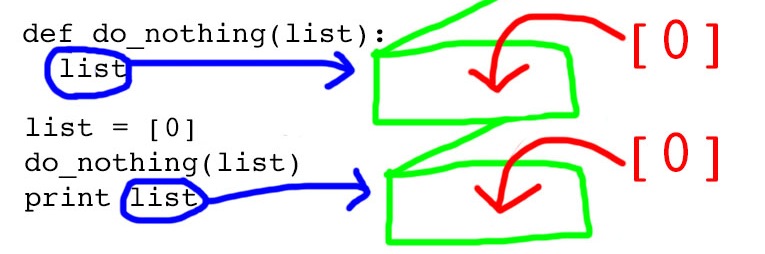
The function then effectively supplies its own box to put the value in, and there is no longer any relationship between either the variables or the objects referred to by the function and the caller. The objects happen to have the same value, but they are totally separate, and nothing that happens to one will affect the other. If we again try to reassign:
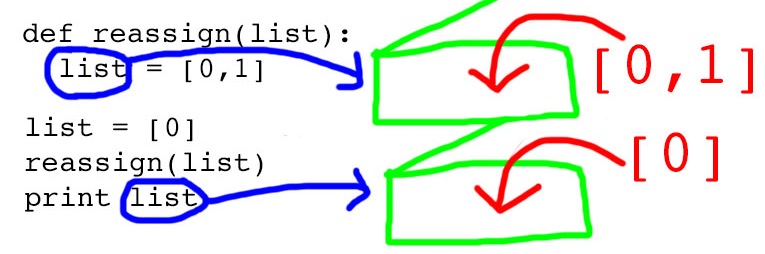
Outside the function, nothing happens. Similarly:
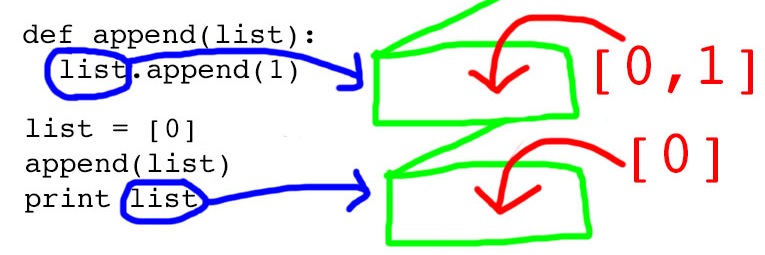
The copies of variables and objects in the context of the caller are completely isolated.
Pass-by-object-reference
Python is different. As we know, in Python, “Object references are passed by value”.
A function receives a reference to (and will access) the same object in memory as used by the caller. However, it does not receive the box that the caller is storing this object in; as in pass-by-value, the function provides its own box and creates a new variable for itself. Let’s try appending again:
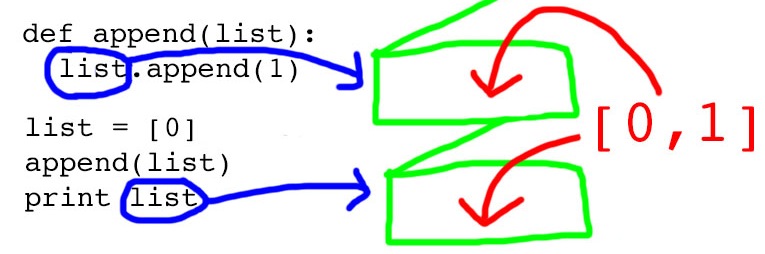
Both the function and the caller refer to the same object in memory, so when the append function adds an extra item to the list, we see this in the caller too! They’re different names for the same thing; different boxes containing the same object. This is what is meant by passing the object references by value - the function and caller use the same object in memory, but accessed through different variables. This means that the same object is being stored in multiple different boxes, and the metaphor kind of breaks down. Pretend it’s quantum or something.
But the key is that they really are different names, and different boxes. In pass-by-reference, they were essentially the same box. When you tried to reassign a variable, and put something different into the function’s box, you also put it into the caller’s box, because they were the same box. But, in pass-by-object-reference:
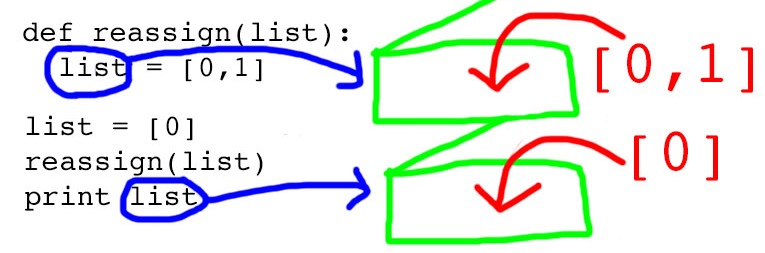
The caller doesn’t care if you reassign the function’s box. Different boxes, same content.
Now we see what Philip K. Dick was trying to tell us. A name and a person are different things. A variable and an object are different things. Armed with this knowledge, you can perhaps start to infer what happens when you do things like
You may also want to read about the interesting interactions these concepts have with mutable and immutable types. But those are stories for another day. Now if you’ll excuse me, I’m going to read “Do Androids Dream Of Electric Sheep?” - my meta-programming is a little rusty.
Useful links
http://foobarnbaz.com/2012/07/08/understanding-python-variables/ http://javadude.com/articles/passbyvalue.htm
Get new essays sent to you
More on programming.
- Hello Deep Learning
- Gamebert: a Game Boy emulator built by Robert
- Gameboy Doctor: debug and fix your gameboy emulator
- What is a self-hosting compiler?
- Migrating bajillions of database records at Stripe
- Code review without your glasses
- Jaccard Similarity and MinHash for winners
What is Call by Value and Call by Reference in Python?
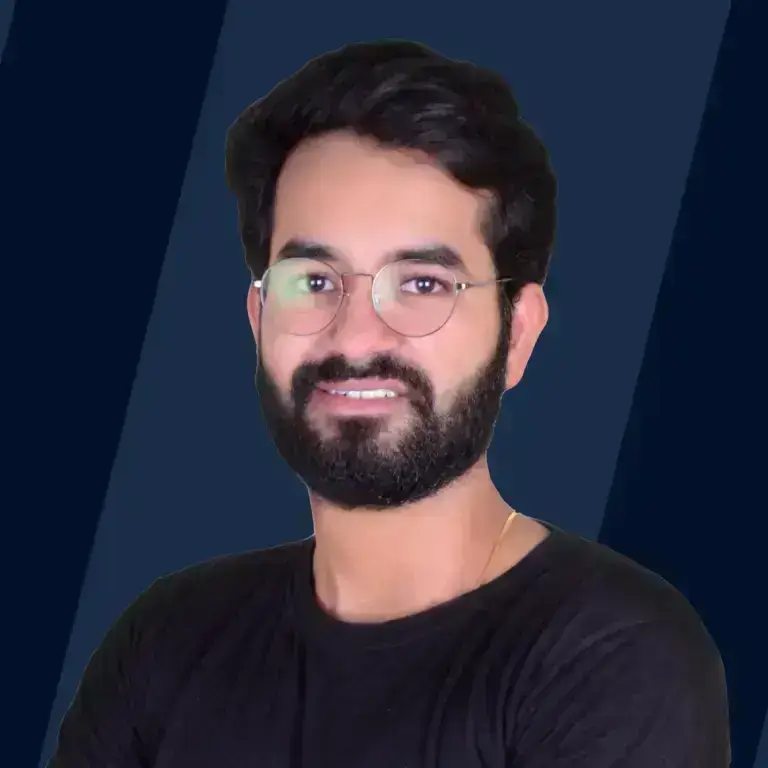
Call by Value
It is a way of passing arguments to a function in which the arguments get copied to the formal parameters of a function and are stored in different memory locations. In short, Any changes made within the function are not reflected in the actual parameters of the function when called.
Call by Reference
It is a way of passing arguments to a function call in which both the actual argument and formal parameters refer to the same memory locations, and any changes made within the function are reflected in the actual parameters of the function when called.
If we consider call by value and call by reference in Python , then we must keep in mind that,
Python variables are not storage containers. Rather Python’s variables are like memory references. They refer to the memory address where the value is stored.
Hence, we should know about Mutable and Immutable Objects.
Mutable objects
An object whose internal state can be changed is called a mutable object. Examples of Mutable objects are Lists, Sets, Dictionaries, bytes and arrays . User-defined classes can be mutable or immutable, depending on whether we can change their internal state.
Note - All operations using mutable objects may or may not change the object.
Immutable objects
An object whose internal state cannot be changed is called an immutable object. Examples of immutable objects are Numbers(int, float, bool, etc), Strings, Tuples, Frozen sets(mutable version of sets are termed as Frozen sets)
Note - All operations using immutable objects make copies .
Code Snippet explaining mutable and immutable objects
Output and Explanation:
Now that we know what Mutable and Immutable Objects are, Lets delve into What is Call by value and Call by reference in Python.
Call by Value in Python
When Immutable objects such as whole numbers, strings, etc are passed as arguments to the function call, the it can be considered as Call by Value.
This is because when the values are modified within the function, then the changes do not get reflected outside the function.
Example showing Call by Value in Python
Explanation: As we can see here, the value of the number changed from 13 to 15 , inside the function, but the value remained 13 itself outside the function. Hence, the changes did not reflect in the value assigned outside the function.
Call by Reference in Python
When Mutable objects such as list, dict, set, etc., are passed as arguments to the function call, it can be called Call by reference in Python. When the values are modified within the function, the change also gets reflected outside the function.
Example 1 showing Call by reference in Python
Explanation: Here we can see that the list value within the function changed from [1] to [1, 3, 1] and the changes got reflected outside the function.
But, Consider this example-
Unlike previous example above , new values are assigned to myList but after function call we see that all the changes do not get reflected outside the function.
Then, is it Call by reference?
Lets take a look into the memory environment for both the examples. We'll print the address of the list before calling the function and after calling the function.
For example 1
Explanation: Here we see the value of the list before calling the function, within the function and after calling the function gets stored in the same memory address. Hence, changes made within function gets reflected outside the function as well.
Lets consider Example 2 now:
Explanation: Here we see that, the address of the list changes when new values are assigned to it within the function but outside the function, the value still refers to the previous memory allocation.Hence, the changes made within the function do not get reflected outside the function .
What do we infer? Well, we see that when mutable objects are passed as arguments to a function then there are two cases:
a.) Change is reflected outside function This is when a mutable object is passed as argument and no new value is assigned to it, ie.,the values refer to the same memory address.
b.) Change is not reflected outside function This is when a mutable object is passed as argument and new values or datatype is assigned to it within the function, ie. when the values outside the function and the values assigned within function refer to different memory addresses .

Difference between Call by Value and Call by Reference
- Depending upon the mutability/immutability of it's data type, a variable behaves differently.
- If a variable refers to an immutable type, then any change in its value will also change the memory address it refers to. Still, if a variable refers to a mutable type , any change in the value of the mutable type will not change the memory address of the variable.
- Arguments are passed by assignment in Python . The assignment creates references to objects.
- Hence, Immutable objects passed as arguments are referred as in Call by Value and Mutable objects , when passed as arguments are referred to as Call by Reference.
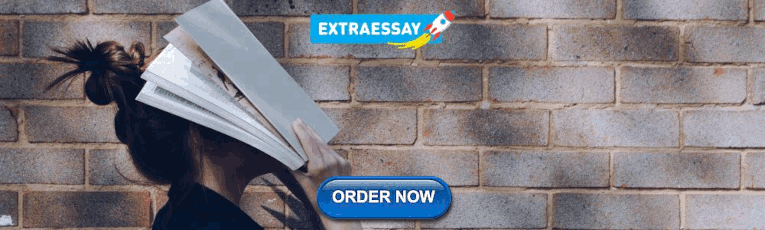
All you need to know on by reference vs by value
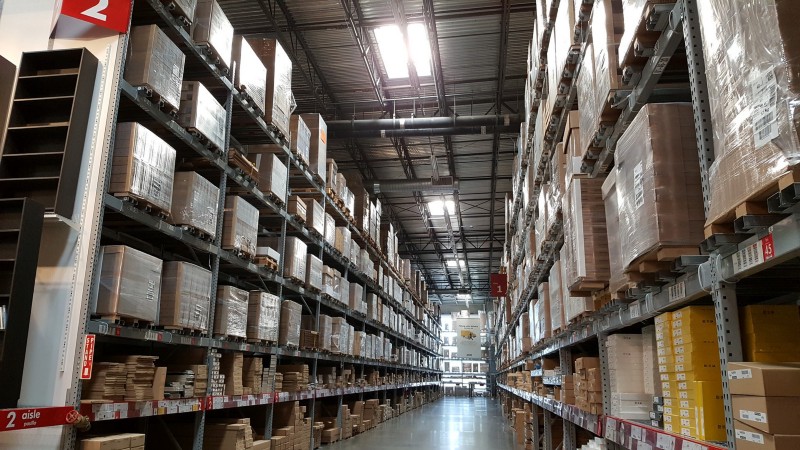
by Szilard Magyar
When it comes to software engineering there are quite a few misunderstood concepts and misused terms. By reference vs by value is definitely one of them.
I remember back in the day when I read up on the topic and every source I went through seemed to contradict the previous one. It took some time to get a solid grasp of it. I had no choice as it is a fundamental subject if you are a software engineer.
I ran into a nasty bug a few weeks back and decided to write an article so other people may have easier time figuring this whole thing out.
I code in Ruby on a daily basis. I also use JavaScript pretty often, so I have chosen these two languages for this presentation.
To understand all the concepts though we will use some Go and Perl examples as well.
To grasp the whole topic you have to understand 3 different things:
- How the underlying data structures are implemented in the language (objects, primitive types, mutability,).
- How variable assignment/copying/reassignment/comparison work
How variables are passed to functions
Underlying data types.
In Ruby there are no primitive types and everything is an object including integers and booleans.
And yes there is a TrueClass in Ruby.
These objects can be either mutable or immutable.
Immutable means there is no way you can change the object once it is created. There is just one instance for a given value with one object_id and it stays the same no matter what you do.
By default in Ruby the immutable object types are: Boolean , Numeric , nil , and Symbol .
In MRI the object_id of an object is the same as the VALUE that represents the object on the C level. For most kinds of objects this VALUE is a pointer to a location in memory where the actual object data is stored.
From now on we will use object_id and memory address interchangeably.
Let’s run some Ruby code in MRI for an immutable Symbol and a mutable String:
As you see while the symbol version keeps the same object_id for the same value, the string values belong to different memory addresses.
Unlike Ruby, JavaScript has primitive types.
They are — Boolean , null , undefined , String , and Number .
The rest of the data types go under the umbrella of Objects ( Array , Function , and Object) . There is nothing fancy here it is way more straightforward than Ruby.
Variable assignment , copying , reassignment and comparison
In Ruby every variable is just a reference to an object (since everything is an object).
When you assign a variable, it is a reference to an object not the object itself. When you copy an object b = a both variables will point to the same address.
This behavior is called copy by reference value .
Strictly speaking in Ruby and JavaScript everything is copied by value.
When it comes to objects though, the values happen to be the memory addresses of those objects. Thanks to this we can modify values that sit in those memory addresses. Again, this is called copy by reference value but most people refer to this as copy by reference.
It would be copy by reference if after reassigning a to ‘new string’, b would also point to the same address and have the same ‘new string’ value.
The same with an immutable type like Integer:
When you reassign a to the same integer, the memory address stays the same since a given integer always has the same object_id.
As you see when you compare any object to another one it is compared by value. If you wanna check out if they are the same object you have to use object_id.
Let’s see the JavaScript version:
Except the comparison — JavaScript uses by value for primitive types and by reference for objects. The behavior looks to be the same just like in Ruby.
Well, not quite.
Primitive values in JavaScript will not be shared between multiple variables . Even if you set the variables equal to each other. Every variable representing a primitive value is guaranteed to belong to a unique memory location.
This means none of the variables will ever point to the same memory address. It is also important that the value itself is stored in a physical memory location.
In our example when we declare b = a , b will point to a different memory address with the same ‘string’ value right away. So you don’t need to reassign a to point to a different memory address.
This is called copied by value since you have no access to the memory address only to the value.
Let’s see a better example where all this matters.
In Ruby if we modify the value that sits in the memory address then all the references that point to the address will have the same updated value:
You might think in JavaScript only the value of a would change but no. You can’t even change the original value as you don’t have direct access to the memory address.
You could say you assigned ‘x’ to a but it was assigned by value so a ’s memory address holds the value ‘x’, but you can’t change it as you have no reference to it.
The behavior of JavaScript objects and implementation are the same like Ruby’s mutable objects. Both copy be reference value.
JavaScript primitive types are copied by value. The behavior is the same like Ruby’s immutable objects which are copied by reference value .
Again, when you copy something by value it means you can’t change (mutate) the original value since there is no reference to the memory address. From the perspective of the writing code this is the same thing like having immutable entities that you can’t mutate.
If you compare Ruby and JavaScript the only data type that ‘behaves’ differently by default is String (that’s why we used String in the examples above).
In Ruby it is a mutable object and it is copied/passed by reference value while in JavaScript it is a primitive type and copied/passed by value.
When you wanna clone (not copy) an object you have to do it explicitly in both languages so you can make sure the original object won’t be modified:
It is crucial to remember this otherwise you will run into some nasty bugs when you invoke your code more than once. A good example would be a recursive function where you use the object as argument.
Another one is React (JavaScript front-end framework) where you always have to pass a new object for updating state as the comparison works based on object id.
This is faster because you don’t have to go through the object line by line to see if it has been changed.
Passing variables to functions is working the same way like copying for the same data types in most of the languages.
In JavaScript primitive types are copied and passed by value and objects are copied and passed by reference value.
I think this is the reason why people only talk about pass by value or pass by reference and never seem to mention copying. I guess they assume copying works the same way.
Now in JavaScript:
If you pass an object (not a primitive type like we did) in JavaScript to the function it works the same way like the Ruby example.
Other languages
We have already seen how copy/pass by value and copy/pass by reference value work. Now we will see what pass by reference is about and we also discover how we can change objects if we pass by value.
As I looked for pass by reference languages I couldn’t find too many and I ended up choosing Perl. Let’s see how copying works in Perl:
Well this seems to be the same just like in Ruby. I haven’t found any proof but I would say Perl is copied by reference value for String.
Now let’s check what pass by reference means:
Since Perl is passed by reference if you do a reassignment within the function it will change the original value of the memory address as well.
For pass by value language I have chosen Go as I intend to deepen my Go knowledge in the foreseeable future:
If you wanna change the value of a memory address you have to use pointers and pass around memory addresses by value. A pointer holds the memory address of a value.
The & operator generates a pointer to its operand and the * operator denotes the pointer’s underlying value. This basically means that you pass the memory address of a value with & and you set the value of a memory address with * .
How to evaluate a language:
- Understand the underlying data types in the language. Read some specifications and play around with them. It usually boils down to primitive types and objects. Then check if those objects are mutable or immutable. Some languages use different copying/passing tactics for different data types.
- Next step is the variable assignment, copying, reassignment and comparison. This is the most crucial part I think. Once you get this you will be able to figure out what’s going on. It helps a lot if you check the memory addresses when playing around.
- Passing variables to functions usually is not special. It usually works the same way like copying in most languages. Once you you know how the variables are copied and reassigned you already know how they are passed to functions.
The languages we used here:
- Go : Copied and passed by value
- JavaScript : Primitive types are copied/passed by value, objects are copied/passed by reference value
- Ruby : Copied and passed by reference value + mutable/immutable objects
- Perl : Copied by reference value and passed by reference
When people say passed by reference they usually mean passed by reference value. Passing by reference value means that variables are passed around by value but those values are references to the objects .
As you saw Ruby only uses pass by reference value while JavaScript uses a mixed strategy. Still, the behavior is the same for almost all the data types due to the different implementation of the data structures.
Most of the mainstream languages are either copied and passed by value or copied and passed by reference value. For the last time: Pass by reference value is usually called pass by reference.
In general pass by value is safer as you won’t run into issues since you can’t accidentally change the original value. It is also slower to write because you have to use pointers if you want to change the objects.
It’s the same idea like with static typing vs dynamic typing — development speed at the cost of safety. As you guessed pass by value is usually a feature of lower level languages like C, Java or Go.
Pass by reference or reference value are usually used by higher level languages like JavaScript, Ruby and Python.
When you discover a new language go through the process like we did here and you will understand how it works.
This is not an easy topic and I am not sure everything is correct what I wrote here. If you think I have made some mistakes in this article, please let me know in the comments.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
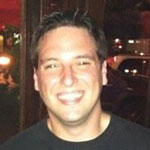
PYTHON PROGRAMMER
Everything i know about python..., learn to write pythonic code.
Check out the book Writing Idiomatic Python !
Looking for Python Tutoring? Remote and local (NYC) slots still available! Email me at [email protected] for more info.
On line 1, we create a binding between a name , some_guy , and a string object containing 'Fred'. In the context of program execution, the environment is altered; a binding of the name some_guy ' to a string object is created in the scope of the block where the statement occurred. When we later say some_guy = 'George' , the string object containing 'Fred' is unaffected. We've just changed the binding of the name some_guy . We haven't, however, changed either the 'Fred' or 'George' string objects . As far as we're concerned, they may live on indefinitely.
With only a single name binding, this may seem overly pedantic, but it becomes more important when bindings are shared and function calls are involved. Let's say we have the following bit of Python code:
So what get's printed in the final line? Well, to start, the binding of some_guy to the string object containing 'Fred' is added to the block's namespace . The name first_names is bound to an empty list object. On line 4, a method is called on the list object first_names is bound to, appending the object some_guy is bound to. At this point, there are still only two objects that exist: the string object and the list object. some_guy and first_names[0] both refer to the same object (Indeed, print(some_guy is first_names[0]) shows this).
Let's continue to break things down. On line 6, a new name is bound: another_list_of_names . Assignment between names does not create a new object. Rather, both names are simply bound to the same object. As a result, the string object and list object are still the only objects that have been created by the interpreter. On line 7, a member function is called on the object another_list_of_names is bound to and it is mutated to contain a reference to a new object: 'George'. So to answer our original question, the output of the code is
This brings us to an important point: there are actually two kinds of objects in Python. A mutable object exhibits time-varying behavior. Changes to a mutable object are visible through all names bound to it. Python's lists are an example of mutable objects. An immutable object does not exhibit time-varying behavior. The value of immutable objects can not be modified after they are created. They can be used to compute the values of new objects, which is how a function like string.join works. When you think about it, this dichotomy is necessary because, again, everything is an object in Python. If integers were not immutable I could change the meaning of the number '2' throughout my program.
It would be incorrect to say that "mutable objects can change and immutable ones can't", however. Consider the following:
Tuples in Python are immutable. We can't change the tuple object name_tuple is bound to. But immutable containers may contain references to mutable objects like lists. Therefore, even though name_tuple is immutable, it "changes" when 'Igor' is appended to first_names on the last line. It's a subtlety that can sometimes (though very infrequently) prove useful.
By now, you should almost be able to intuit how function calls work in Python. If I call foo(bar) , I'm merely creating a binding within the scope of foo to the object the argument bar is bound to when the function is called. If bar refers to a mutable object and foo changes its value, then these changes will be visible outside of the scope of the function.
On the other hand, if bar refers to an immutable object, the most that foo can do is create a name bar in its local namespace and bind it to some other object.
Hopefully by now it is clear why Python is neither "call-by-reference" nor "call-by-value". In Python a variable is not an alias for a location in memory. Rather, it is simply a binding to a Python object. While the notion of "everything is an object" is undoubtedly a cause of confusion for those new to the language, it allows for powerful and flexible language constructs, which I'll discuss in my next post.
« Starting a Django 1.4 Project the Right Way
Like this article?
Why not sign up for Python Tutoring ? Sessions can be held remotely using Google+/Skype or in-person if you're in the NYC area. Email [email protected] if interested.
Sign up for the free jeffknupp.com email newsletter. Sent roughly once a month, it focuses on Python programming, scalable web development, and growing your freelance consultancy. And of course, you'll never be spammed, your privacy is protected, and you can opt out at any time.
- Python »
- 3.14.0a0 Documentation »
- The Python Tutorial »
- 3. An Informal Introduction to Python
- Theme Auto Light Dark |
3. An Informal Introduction to Python ¶
In the following examples, input and output are distinguished by the presence or absence of prompts ( >>> and … ): to repeat the example, you must type everything after the prompt, when the prompt appears; lines that do not begin with a prompt are output from the interpreter. Note that a secondary prompt on a line by itself in an example means you must type a blank line; this is used to end a multi-line command.
You can toggle the display of prompts and output by clicking on >>> in the upper-right corner of an example box. If you hide the prompts and output for an example, then you can easily copy and paste the input lines into your interpreter.
Many of the examples in this manual, even those entered at the interactive prompt, include comments. Comments in Python start with the hash character, # , and extend to the end of the physical line. A comment may appear at the start of a line or following whitespace or code, but not within a string literal. A hash character within a string literal is just a hash character. Since comments are to clarify code and are not interpreted by Python, they may be omitted when typing in examples.
Some examples:
3.1. Using Python as a Calculator ¶
Let’s try some simple Python commands. Start the interpreter and wait for the primary prompt, >>> . (It shouldn’t take long.)
3.1.1. Numbers ¶
The interpreter acts as a simple calculator: you can type an expression at it and it will write the value. Expression syntax is straightforward: the operators + , - , * and / can be used to perform arithmetic; parentheses ( () ) can be used for grouping. For example:
The integer numbers (e.g. 2 , 4 , 20 ) have type int , the ones with a fractional part (e.g. 5.0 , 1.6 ) have type float . We will see more about numeric types later in the tutorial.
Division ( / ) always returns a float. To do floor division and get an integer result you can use the // operator; to calculate the remainder you can use % :
With Python, it is possible to use the ** operator to calculate powers [ 1 ] :
The equal sign ( = ) is used to assign a value to a variable. Afterwards, no result is displayed before the next interactive prompt:
If a variable is not “defined” (assigned a value), trying to use it will give you an error:
There is full support for floating point; operators with mixed type operands convert the integer operand to floating point:
In interactive mode, the last printed expression is assigned to the variable _ . This means that when you are using Python as a desk calculator, it is somewhat easier to continue calculations, for example:
This variable should be treated as read-only by the user. Don’t explicitly assign a value to it — you would create an independent local variable with the same name masking the built-in variable with its magic behavior.
In addition to int and float , Python supports other types of numbers, such as Decimal and Fraction . Python also has built-in support for complex numbers , and uses the j or J suffix to indicate the imaginary part (e.g. 3+5j ).
3.1.2. Text ¶
Python can manipulate text (represented by type str , so-called “strings”) as well as numbers. This includes characters “ ! ”, words “ rabbit ”, names “ Paris ”, sentences “ Got your back. ”, etc. “ Yay! :) ”. They can be enclosed in single quotes ( '...' ) or double quotes ( "..." ) with the same result [ 2 ] .
To quote a quote, we need to “escape” it, by preceding it with \ . Alternatively, we can use the other type of quotation marks:
In the Python shell, the string definition and output string can look different. The print() function produces a more readable output, by omitting the enclosing quotes and by printing escaped and special characters:
If you don’t want characters prefaced by \ to be interpreted as special characters, you can use raw strings by adding an r before the first quote:
There is one subtle aspect to raw strings: a raw string may not end in an odd number of \ characters; see the FAQ entry for more information and workarounds.
String literals can span multiple lines. One way is using triple-quotes: """...""" or '''...''' . End of lines are automatically included in the string, but it’s possible to prevent this by adding a \ at the end of the line. The following example:
produces the following output (note that the initial newline is not included):
Strings can be concatenated (glued together) with the + operator, and repeated with * :
Two or more string literals (i.e. the ones enclosed between quotes) next to each other are automatically concatenated.
This feature is particularly useful when you want to break long strings:
This only works with two literals though, not with variables or expressions:
If you want to concatenate variables or a variable and a literal, use + :
Strings can be indexed (subscripted), with the first character having index 0. There is no separate character type; a character is simply a string of size one:
Indices may also be negative numbers, to start counting from the right:
Note that since -0 is the same as 0, negative indices start from -1.
In addition to indexing, slicing is also supported. While indexing is used to obtain individual characters, slicing allows you to obtain a substring:
Slice indices have useful defaults; an omitted first index defaults to zero, an omitted second index defaults to the size of the string being sliced.
Note how the start is always included, and the end always excluded. This makes sure that s[:i] + s[i:] is always equal to s :
One way to remember how slices work is to think of the indices as pointing between characters, with the left edge of the first character numbered 0. Then the right edge of the last character of a string of n characters has index n , for example:
The first row of numbers gives the position of the indices 0…6 in the string; the second row gives the corresponding negative indices. The slice from i to j consists of all characters between the edges labeled i and j , respectively.
For non-negative indices, the length of a slice is the difference of the indices, if both are within bounds. For example, the length of word[1:3] is 2.
Attempting to use an index that is too large will result in an error:
However, out of range slice indexes are handled gracefully when used for slicing:
Python strings cannot be changed — they are immutable . Therefore, assigning to an indexed position in the string results in an error:
If you need a different string, you should create a new one:
The built-in function len() returns the length of a string:
Strings are examples of sequence types , and support the common operations supported by such types.
Strings support a large number of methods for basic transformations and searching.
String literals that have embedded expressions.
Information about string formatting with str.format() .
The old formatting operations invoked when strings are the left operand of the % operator are described in more detail here.
3.1.3. Lists ¶
Python knows a number of compound data types, used to group together other values. The most versatile is the list , which can be written as a list of comma-separated values (items) between square brackets. Lists might contain items of different types, but usually the items all have the same type.
Like strings (and all other built-in sequence types), lists can be indexed and sliced:
Lists also support operations like concatenation:
Unlike strings, which are immutable , lists are a mutable type, i.e. it is possible to change their content:
You can also add new items at the end of the list, by using the list.append() method (we will see more about methods later):
Simple assignment in Python never copies data. When you assign a list to a variable, the variable refers to the existing list . Any changes you make to the list through one variable will be seen through all other variables that refer to it.:
All slice operations return a new list containing the requested elements. This means that the following slice returns a shallow copy of the list:
Assignment to slices is also possible, and this can even change the size of the list or clear it entirely:
The built-in function len() also applies to lists:
It is possible to nest lists (create lists containing other lists), for example:
3.2. First Steps Towards Programming ¶
Of course, we can use Python for more complicated tasks than adding two and two together. For instance, we can write an initial sub-sequence of the Fibonacci series as follows:
This example introduces several new features.
The first line contains a multiple assignment : the variables a and b simultaneously get the new values 0 and 1. On the last line this is used again, demonstrating that the expressions on the right-hand side are all evaluated first before any of the assignments take place. The right-hand side expressions are evaluated from the left to the right.
The while loop executes as long as the condition (here: a < 10 ) remains true. In Python, like in C, any non-zero integer value is true; zero is false. The condition may also be a string or list value, in fact any sequence; anything with a non-zero length is true, empty sequences are false. The test used in the example is a simple comparison. The standard comparison operators are written the same as in C: < (less than), > (greater than), == (equal to), <= (less than or equal to), >= (greater than or equal to) and != (not equal to).
The body of the loop is indented : indentation is Python’s way of grouping statements. At the interactive prompt, you have to type a tab or space(s) for each indented line. In practice you will prepare more complicated input for Python with a text editor; all decent text editors have an auto-indent facility. When a compound statement is entered interactively, it must be followed by a blank line to indicate completion (since the parser cannot guess when you have typed the last line). Note that each line within a basic block must be indented by the same amount.
The print() function writes the value of the argument(s) it is given. It differs from just writing the expression you want to write (as we did earlier in the calculator examples) in the way it handles multiple arguments, floating point quantities, and strings. Strings are printed without quotes, and a space is inserted between items, so you can format things nicely, like this:
The keyword argument end can be used to avoid the newline after the output, or end the output with a different string:
Table of Contents
- 3.1.1. Numbers
- 3.1.2. Text
- 3.1.3. Lists
- 3.2. First Steps Towards Programming
Previous topic
2. Using the Python Interpreter
4. More Control Flow Tools
- Report a Bug
- Show Source
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
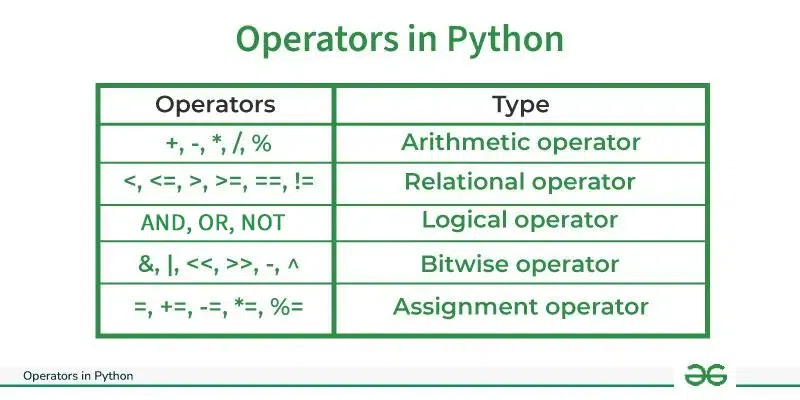
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Proposal: Annotate types in multiple assignment
In the latest version of Python (3.12.3), type annotation for single variable assignment is available:
However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid:
In this case, I propose two new syntaxes to support this feature:
- Annotate directly after each variable:
- Annotate the tuple of return:
In other programming languages, as I know, Julia and Rust support this feature in there approaches:
I’m pretty sure this has already been suggested. Did you go through the mailing list and searched for topics here? Without doing that, there’s nothing to discuss here. (Besides linking to them).
Secondly, try to not edit posts, but post a followup. Some people read these topics in mailing list mode and don’t see your edits.
- https://mail.python.org
- https://mail.python.org/archives

For reference, PEP 526 has a note about this in the “Rejected/Postponed Proposals” section:
Allow type annotations for tuple unpacking: This causes ambiguity: it’s not clear what this statement means: x, y: T Are x and y both of type T , or do we expect T to be a tuple type of two items that are distributed over x and y , or perhaps x has type Any and y has type T ? (The latter is what this would mean if this occurred in a function signature.) Rather than leave the (human) reader guessing, we forbid this, at least for now.
Personally I think the meaning of this is rather clear, especially when combined with an assignment, and I would like to see this.
Thank you for your valuable response, both regarding the discussion convention for Python development and the history of this feature.
I have found a related topic here: https://mail.python.org/archives/list/[email protected]/thread/5NZNHBDWK6EP67HSK4VNDTZNIVUOXMRS/
Here’s the part I find unconvincing:
Under what circumstances will fun() be hard to annotate, but a, b will be easy?
It’s better to annotate function arguments and return values, not variables. The preferred scenario is that fun() has a well-defined return type, and the type of a, b can be inferred (there is no reason to annotate it). This idea is presupposing there are cases where that’s difficult, but I’d like to see some examples where that applies.
Does this not work?
You don’t need from __future__ as of… 3.9, I think?

3.10 if you want A | B too: PEP 604 , although I’m not sure which version the OP is using and 3.9 hasn’t reached end of life yet.
We can’t always infer it, so annotating a variable is sometimes necessary or useful. But if the function’s return type is annotated then a, b = fun() allows type-checkers to infer the types of a and b . This stuff isn’t built in to Python and is evolving as typing changes, so what was inferred in the past might be better in the future.
So my question above was: are there any scenarios where annotating the function is difficult, but annotating the results would be easy? That seems like the motivating use case.
Would it be a solution to put it on the line above? And not allow assigning on the same line? Then it better mirrors function definitions.
It’s a long thread, so it might have been suggested already.
Actually, in cases where the called function differs from the user-defined function, we should declare the types when assignment unpacking.
Here is a simplified MWE:
NOTE: In PyTorch, the __call__ function is internally wrapped from forward .
Can’t you write this? That’s shorter than writing the type annotations.
This is the kind of example I was asking for, thanks. Is the problem that typing tools don’t trace the return type through the call because the wrapping isn’t in python?
I still suggest to read the thread you linked, like I’m doing right now.
The __call__ function is not the same as forward . There might be many other preprocessing and postprocessing steps involved inside it.
Yeah, quite a bit of pre-processing in fact… unless you don’t have hooks by the looks of it:
Related Topics
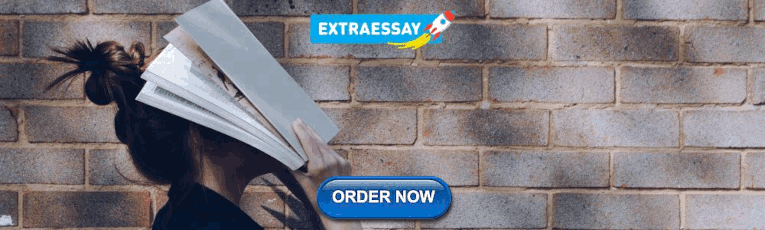
IMAGES
VIDEO
COMMENTS
33. Everything in Python is passed and assigned by value, in the same way that everything is passed and assigned by value in Java. Every value in Python is a reference (pointer) to an object. Objects cannot be values. Assignment always copies the value (which is a pointer); two such pointers can thus point to the same object.
Depending on the type of object you pass in the function, the function behaves differently. Immutable objects show "pass by value" whereas mutable objects show "pass by reference". You can check the difference between pass-by-value and pass-by-reference in the example below: Python3. def call_by_value(x): x = x * 2.
Python passes arguments neither by reference nor by value, but by assignment. Below, you'll quickly explore the details of passing by value and passing by reference before looking more closely at Python's approach. After that, you'll walk through some best practices for achieving the equivalent of passing by reference in Python. Remove ads.
Python utilizes a system, which is known as "Call by Object Reference" or "Call by assignment". If you pass arguments like whole numbers, strings, or tuples to a function, the passing is like a call-by-value because you can not change the value of the immutable objects being passed to the function. Passing mutable objects can be considered as call by reference or Python pass by ...
Pass By Reference vs Pass By Value Python Conclusion. In this Python tutorial, you learned how to call by value and call by reference in Python with examples. Additionally, You learned what call by value and reference means and the difference between them. You may like to read: Write a Program to Find a Perfect Number in Python
Python's Pass by Assignment: Python's behavior is neither purely pass-by value nor pass-by-reference. Instead, it employs a mechanism called "pass by assignment" or "call by object."
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
You'll implement real use cases of pass-by-reference constructs in Python and learn several best practices to avoid pitfalls with your function arguments. In this course, you'll learn: What it means to pass by reference and why you'd want to do so. How passing by reference differs from both passing by value and Python's unique approach.
Python doesn't use the pass-by-value model, nor the pass-by-reference one; Python uses a pass-by-assignment model (using "nicknames"); each object is characterised by. its identity; its type; and. its contents. the id function is used to query an object's identifier; the type function is used to query an object's type;
In Python, on the other hand, data objects are stored in memory, and a variable is just a label for its easy access. This is why Python is a dynamically typed language, where the type of variable need not be declared before assignment and data decides the type and not other way round (as in C/C++).
This mix of pass by value and pass by reference in Python is called pass by assignment. It is essential to keep this concept always in mind when writing Python code. In the previous parts of this Python course, you have seen that it is possible to get a copy of a mutable data type by calling the .copy() function: 1. 2.
The two most widely known and easy to understand approaches to parameter passing amongst programming languages are pass-by-reference and pass-by-value. Unfortunately, Python is "pass-by-object-reference", of which it is often said: "Object references are passed by value.". When I first read this smug and overly-pithy definition, I ...
Call by Reference in Python. When Mutable objects such as list, dict, set, etc., are passed as arguments to the function call, it can be called Call by reference in Python. When the values are modified within the function, the change also gets reflected outside the function. Example 1 showing Call by reference in Python.
The variable name simply points to the object in the memory. Now according to this question, >> a_dict = b_dict = c_dict = {} This creates an empty dictionary and all the variables point to this dict object. So, changing any one would be reflected in the other variables. >> a_dict["key"] = "value" #say. >> print a_dict.
As you saw Ruby only uses pass by reference value while JavaScript uses a mixed strategy. Still, the behavior is the same for almost all the data types due to the different implementation of the data structures. Most of the mainstream languages are either copied and passed by value or copied and passed by reference value. For the last time ...
At a more fundamental level, the confusion arises from a misunderstanding about Python object-centric data model and its treatment of assignment. When asked whether Python function calling model is "call-by-value" or "call-by-reference", the correct answer is: neither. Indeed, to try to shoe-horn those terms into a conversation about Python's ...
Pass by Reference and Pass by Value. One important thing to note is, in Python every variable name is a reference. When we pass a variable to a function Python, a new reference to the object is created. Parameter passing in Python is the same as reference passing in Java. Python3
00:12 Python doesn't use either pass by value or pass by reference. It uses something called pass by assignment. Other names for this include pass by object, pass by object reference, and pass by sharing. 00:25 But I'll be using the phrase "pass by assignment.". It's based on the following: First, everything in Python is an object.
Python (and other languages) calls whatever is needed to refer to a value a reference, despite being pretty unrelated to things like C++ references and pass-by-reference. Assigning to a variable (or object field, or ...) simply makes it refer to another value. The whole model of storage locations does not apply to Python, the programmer never ...
This example introduces several new features. The first line contains a multiple assignment: the variables a and b simultaneously get the new values 0 and 1. On the last line this is used again, demonstrating that the expressions on the right-hand side are all evaluated first before any of the assignments take place.
This is the way Python assignment always works. A = B merely means "the object referred to by the name A is now referred to by the name B as well". Again, ... Arrays in Python are assigned by value or by reference? 3. numpy: pass by reference does not work on itself. 2.
Assignment Operators in Python. Let's see an example of Assignment Operators in Python. Example: The code starts with 'a' and 'b' both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on 'b'.
In the latest version of Python (3.12.3), type annotation for single variable assignment is available: a: int = 1 However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid: from typing import Any def fun() -> Any: # when hard to annotate the strict type return 1, True a: int, b: bool = fun() # INVALID In this case, I ...
Assignment Expressions. For more information on concepts covered in this lesson, you can check out: Here's a feature introduced in version 3.8 of Python, which can simplify the function we're currently working on. It's called an assignment expression, and it allows you to save the return value of a function to a variable while at the same ...
Copying a list is easy ... Just slice it: temp = z[:] This will create a shallow copy -- mutations to elements in the list will show up in the elements in z, but not changes to temp directly. For more general purposes, python has a copy module that you can use: temp = copy.copy(z) Or, possibly: temp = copy.deepcopy(z)