
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
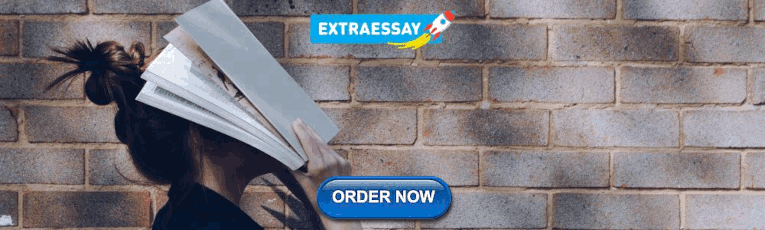
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
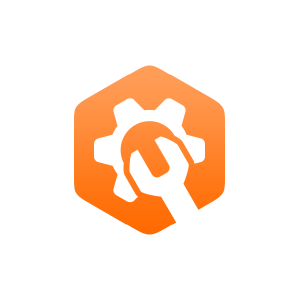
4.6: Assignment Operator
- Last updated
- Save as PDF
- Page ID 29038
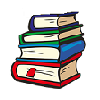
- Patrick McClanahan
- San Joaquin Delta College
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
Assignment Operator
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
As we have seen, assignment operators are used to assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=” : This is the simplest assignment operator, which was discussed above. This operator is used to assign the value on the right to the variable on the left. For example: a = 10; b = 20; ch = 'y';
If initially the value 5 is stored in the variable a, then: (a += 6) is equal to 11. (the same as: a = a + 6)
If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
If initially value 5 is stored in the variable a,, then (a *= 6) is equal to 30. (the same as a = a * 6)
If initially value 6 is stored in the variable a, then (a /= 2) is equal to 3. (the same as a = a / 2)
Below example illustrates the various Assignment Operators:
Definitions
Adapted from: "Assignment Operator" by Kenneth Leroy Busbee , (Download for free at http://cnx.org/contents/[email protected] ) is licensed under CC BY 4.0
Assignment operators
Assignment operators modify the value of the object.
Explanation
copy assignment operator replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is a special member function, described in copy assignment operator .
move assignment operator replaces the contents of the object a with the contents of b , avoiding copying if possible ( b may be modified). For class types, this is a special member function, described in move assignment operator . (since C++11)
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
compound assignment operators replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
Builtin direct assignment
The direct assignment expressions have the form
For the built-in operator, lhs may have any non-const scalar type and rhs must be implicitly convertible to the type of lhs .
The direct assignment operator expects a modifiable lvalue as its left operand and an rvalue expression or a braced-init-list (since C++11) as its right operand, and returns an lvalue identifying the left operand after modification.
For non-class types, the right operand is first implicitly converted to the cv-unqualified type of the left operand, and then its value is copied into the object identified by left operand.
When the left operand has reference type, the assignment operator modifies the referred-to object.
If the left and the right operands identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same)
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
Builtin compound assignment
The compound assignment expressions have the form
The behavior of every builtin compound-assignment expression E1 op = E2 (where E1 is a modifiable lvalue expression and E2 is an rvalue expression or a braced-init-list (since C++11) ) is exactly the same as the behavior of the expression E1 = E1 op E2 , except that the expression E1 is evaluated only once and that it behaves as a single operation with respect to indeterminately-sequenced function calls (e.g. in f ( a + = b, g ( ) ) , the += is either not started at all or is completed as seen from inside g ( ) ).
In overload resolution against user-defined operators , for every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2, where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
Operator precedence
Operator overloading
C++ Tutorial
C++ functions, c++ classes, c++ reference, c++ examples, c++ assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Operator precedence
Operator precedence determines how operators are parsed concerning each other. Operators with higher precedence become the operands of operators with lower precedence.
Precedence and associativity
Consider an expression describable by the representation below, where both OP1 and OP2 are fill-in-the-blanks for OPerators.
The combination above has two possible interpretations:
Which one the language decides to adopt depends on the identity of OP1 and OP2 .
If OP1 and OP2 have different precedence levels (see the table below), the operator with the higher precedence goes first and associativity does not matter. Observe how multiplication has higher precedence than addition and executed first, even though addition is written first in the code.
Within operators of the same precedence, the language groups them by associativity . Left-associativity (left-to-right) means that it is interpreted as (a OP1 b) OP2 c , while right-associativity (right-to-left) means it is interpreted as a OP1 (b OP2 c) . Assignment operators are right-associative, so you can write:
with the expected result that a and b get the value 5. This is because the assignment operator returns the value that is assigned. First, b is set to 5. Then the a is also set to 5 — the return value of b = 5 , a.k.a. right operand of the assignment.
As another example, the unique exponentiation operator has right-associativity, whereas other arithmetic operators have left-associativity.
Operators are first grouped by precedence, and then, for adjacent operators that have the same precedence, by associativity. So, when mixing division and exponentiation, the exponentiation always comes before the division. For example, 2 ** 3 / 3 ** 2 results in 0.8888888888888888 because it is the same as (2 ** 3) / (3 ** 2) .
For prefix unary operators, suppose we have the following pattern:
where OP1 is a prefix unary operator and OP2 is a binary operator. If OP1 has higher precedence than OP2 , then it would be grouped as (OP1 a) OP2 b ; otherwise, it would be OP1 (a OP2 b) .
If the unary operator is on the second operand:
Then the binary operator OP2 must have lower precedence than the unary operator OP1 for it to be grouped as a OP2 (OP1 b) . For example, the following is invalid:
Because + has higher precedence than yield , this would become (a + yield) 1 — but because yield is a reserved word in generator functions, this would be a syntax error. Luckily, most unary operators have higher precedence than binary operators and do not suffer from this pitfall.
If we have two prefix unary operators:
Then the unary operator closer to the operand, OP2 , must have higher precedence than OP1 for it to be grouped as OP1 (OP2 a) . It's possible to get it the other way and end up with (OP1 OP2) a :
Because await has higher precedence than yield , this would become (await yield) 1 , which is awaiting an identifier called yield , and a syntax error. Similarly, if you have new !A; , because ! has lower precedence than new , this would become (new !) A , which is obviously invalid. (This code looks nonsensical to write anyway, since !A always produces a boolean, not a constructor function.)
For postfix unary operators (namely, ++ and -- ), the same rules apply. Luckily, both operators have higher precedence than any binary operator, so the grouping is always what you would expect. Moreover, because ++ evaluates to a value , not a reference , you can't chain multiple increments together either, as you may do in C.
Operator precedence will be handled recursively . For example, consider this expression:
First, we group operators with different precedence by decreasing levels of precedence.
- The ** operator has the highest precedence, so it's grouped first.
- Looking around the ** expression, it has * on the right and + on the left. * has higher precedence, so it's grouped first. * and / have the same precedence, so we group them together for now.
- Looking around the * / / expression grouped in 2, because + has higher precedence than >> , the former is grouped.
Within the * / / group, because they are both left-associative, the left operand would be grouped.
Note that operator precedence and associativity only affect the order of evaluation of operators (the implicit grouping), but not the order of evaluation of operands . The operands are always evaluated from left-to-right. The higher-precedence expressions are always evaluated first, and their results are then composed according to the order of operator precedence.
If you are familiar with binary trees, think about it as a post-order traversal .
After all operators have been properly grouped, the binary operators would form a binary tree. Evaluation starts from the outermost group — which is the operator with the lowest precedence ( / in this case). The left operand of this operator is first evaluated, which may be composed of higher-precedence operators (such as a call expression echo("left", 4) ). After the left operand has been evaluated, the right operand is evaluated in the same fashion. Therefore, all leaf nodes — the echo() calls — would be visited left-to-right, regardless of the precedence of operators joining them.
Short-circuiting
In the previous section, we said "the higher-precedence expressions are always evaluated first" — this is generally true, but it has to be amended with the acknowledgement of short-circuiting , in which case an operand may not be evaluated at all.
Short-circuiting is jargon for conditional evaluation. For example, in the expression a && (b + c) , if a is falsy , then the sub-expression (b + c) will not even get evaluated, even if it is grouped and therefore has higher precedence than && . We could say that the logical AND operator ( && ) is "short-circuited". Along with logical AND, other short-circuited operators include logical OR ( || ), nullish coalescing ( ?? ), and optional chaining ( ?. ).
When evaluating a short-circuited operator, the left operand is always evaluated. The right operand will only be evaluated if the left operand cannot determine the result of the operation.
Note: The behavior of short-circuiting is baked in these operators. Other operators would always evaluate both operands, regardless if that's actually useful — for example, NaN * foo() will always call foo , even when the result would never be something other than NaN .
The previous model of a post-order traversal still stands. However, after the left subtree of a short-circuiting operator has been visited, the language will decide if the right operand needs to be evaluated. If not (for example, because the left operand of || is already truthy), the result is directly returned without visiting the right subtree.
Consider this case:
Only C() is evaluated, despite && having higher precedence. This does not mean that || has higher precedence in this case — it's exactly because (B() && A()) has higher precedence that causes it to be neglected as a whole. If it's re-arranged as:
Then the short-circuiting effect of && would only prevent C() from being evaluated, but because A() && C() as a whole is false , B() would still be evaluated.
However, note that short-circuiting does not change the final evaluation outcome. It only affects the evaluation of operands , not how operators are grouped — if evaluation of operands doesn't have side effects (for example, logging to the console, assigning to variables, throwing an error), short-circuiting would not be observable at all.
The assignment counterparts of these operators ( &&= , ||= , ??= ) are short-circuited as well. They are short-circuited in a way that the assignment does not happen at all.
The following table lists operators in order from highest precedence (18) to lowest precedence (1).
Several general notes about the table:
- Not all syntax included here are "operators" in the strict sense. For example, spread ... and arrow => are typically not regarded as operators. However, we still included them to show how tightly they bind compared to other operators/expressions.
- Some operators have certain operands that require expressions narrower than those produced by higher-precedence operators. For example, the right-hand side of member access . (precedence 17) must be an identifier instead of a grouped expression. The left-hand side of arrow => (precedence 2) must be an arguments list or a single identifier instead of some random expression.
- Some operators have certain operands that accept expressions wider than those produced by higher-precedence operators. For example, the bracket-enclosed expression of bracket notation [ … ] (precedence 17) can be any expression, even comma (precedence 1) joined ones. These operators act as if that operand is "automatically grouped". In this case we will omit the associativity.
- The operand can be any expression.
- The "right-hand side" must be an identifier.
- The "right-hand side" can be any expression.
- The "right-hand side" is a comma-separated list of any expression with precedence > 1 (i.e. not comma expressions).
- The operand must be a valid assignment target (identifier or property access). Its precedence means new Foo++ is (new Foo)++ (a syntax error) and not new (Foo++) (a TypeError: (Foo++) is not a constructor).
- The operand must be a valid assignment target (identifier or property access).
- The operand cannot be an identifier or a private property access.
- The left-hand side cannot have precedence 14.
- The operands cannot be a logical OR || or logical AND && operator without grouping.
- The "left-hand side" must be a valid assignment target (identifier or property access).
- The associativity means the two expressions after ? are implicitly grouped.
- The "left-hand side" is a single identifier or a parenthesized parameter list.
- Only valid inside object literals, array literals, or argument lists.
operator-assignment
Require or disallow assignment operator shorthand where possible
Some problems reported by this rule are automatically fixable by the --fix command line option
JavaScript provides shorthand operators that combine variable assignment and some simple mathematical operations. For example, x = x + 4 can be shortened to x += 4 . The supported shorthand forms are as follows:
Rule Details
This rule requires or disallows assignment operator shorthand where possible.
The rule applies to the operators listed in the above table. It does not report the logical assignment operators &&= , ||= , and ??= because their short-circuiting behavior is different from the other assignment operators.
This rule has a single string option:
- "always" (default) requires assignment operator shorthand where possible
- "never" disallows assignment operator shorthand
Examples of incorrect code for this rule with the default "always" option:
Examples of correct code for this rule with the default "always" option:
Examples of incorrect code for this rule with the "never" option:
Examples of correct code for this rule with the "never" option:
When Not To Use It
Use of operator assignment shorthand is a stylistic choice. Leaving this rule turned off would allow developers to choose which style is more readable on a case-by-case basis.
This rule was introduced in ESLint v0.10.0.
- Rule source
- Tests source

21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
cppreference.com
Copy assignment operator.
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . For a type to be CopyAssignable , it must have a public copy assignment operator.
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used.
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used (non-swappable type or degraded performance).
- Forcing a copy assignment operator to be generated by the compiler.
- Avoiding implicit copy assignment.
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
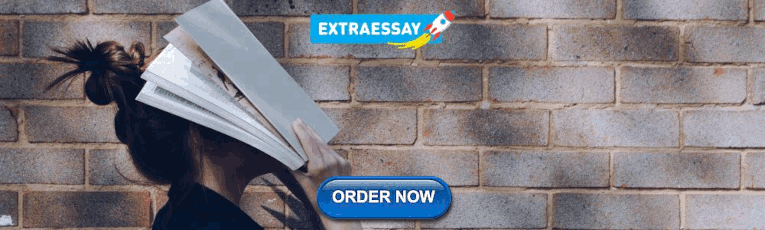
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) exception specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
A implicitly-declared copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a user-declared move constructor;
- T has a user-declared move assignment operator.
Otherwise, it is defined as defaulted.
A defaulted copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a non-static data member of non-class type (or array thereof) that is const ;
- T has a non-static data member of a reference type;
- T has a non-static data member or a direct or virtual base class that cannot be copy-assigned (overload resolution for the copy assignment fails, or selects a deleted or inaccessible function);
- T is a union-like class , and has a variant member whose corresponding assignment operator is non-trivial.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted) , , and if it is defaulted, its signature is the same as implicitly-defined (until C++14) ;
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial;
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
- Pages with unreviewed CWG DR marker
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 9 January 2019, at 07:16.
- This page has been accessed 570,566 times.
- Privacy policy
- About cppreference.com
- Disclaimers

trending now in US News

Family's $15K Carnival Cruise vacation canceled just 2 days...

Gabby Petito's mom forgives killer Brian Laundrie but calls out...

6-week-old mauled to death by family husky during 'completely...

Serial killer who brought dozens of female victims to his pig...

Fetterman dramatically whips off Harvard hood at Yeshiva...

Kids of missing Conn. mom break silence as dad’s girlfriend...

'The View' co-host's daughter celebrates graduation from swanky...

Columbia students set up new anti-Israel encampment on campus...
Rep. stefanik slaps judge juan merchan with misconduct complaint over ‘random’ assignment to trump ‘hush money’ trial.
- View Author Archive
- Get author RSS feed
Thanks for contacting us. We've received your submission.
Rep. Elise Stefanik (R-NY) filed a misconduct complaint Tuesday against the judge overseeing Donald Trump’s Manhattan hush money trial , alleging that his selection to handle the former president’s case — and others involving his allies — is “not random at all.”
The House Republican Conference chairwoman’s complaint with the inspector general of the New York State Unified Court System called for an investigation into Justice Juan Merchan “to determine whether the required random selection process was in fact followed.”
“The potential misconduct pertains to the repeated assignment of Acting Justice Juan Merchan, a Democrat Party donor, to criminal cases related to President Donald J. Trump and his allies,” Stefanik wrote .

“Acting Justice Merchan currently presides over the criminal case against President Trump brought by Manhattan District Attorney Alvin Bragg,” she said.
“Acting Justice Merchan also presided over the criminal trial against the Trump Organization and will be presiding over the criminal trial of Steve Bannon, a senior advisor in President Trump’s White House and a prominent advocate for President Trump,” Stefanik continued, noting that there were at least two dozen sitting justices eligible to oversee the cases, but Merchan — an acting justice — was selected for all three related to the presumptive 2024 GOP nominee for president and his allies.
“If justices were indeed being randomly assigned in the Criminal Term, the probability of two specific criminal cases being assigned to the same justice is quite low, and the probability of three specific criminal cases being assigned to the same justice is infinitesimally small. And yet, we see Acting Justice Merchan on all three cases,” Stefanik argued.
The congresswoman also highlighted the judge’s political donations, for which he was cleared of misconduct last July by the New York State Commission on Judicial Conduct.
Merchan contributed $15 earmarked for the “Biden for President” campaign on July 26, 2020, and then the following day made $10 contributions to the Progressive Turnout Project and Stop Republicans, Federal Election Commission records show.

The donations were made through ActBlue, the Democratic Party’s preferred online fundraising platform.
The Progressive Turnout Project’s stated mission is to “rally Democrats to vote,” according to the group’s website.
Stop Republicans is a subsidiary of the Progressive Turnout Project and describes itself as “a grassroots-funded effort dedicated to resisting the Republican Party and Donald Trump’s radical right-wing legacy.”

The judge’s daughter, Loren Merchan, is more involved in Democratic politics — through her work as head of the consulting firm Authentic Campaigns — and Stefanik argued in her missive that Loren Merchan’s “firm stands to profit greatly if Donald Trump is convicted.”
“One cannot help but suspect that the ‘random selection’ at work in the assignment of Acting Justice Merchan, a Democrat Party donor, to these cases involving prominent Republicans, is in fact not random at all,” the New York Republican lawmaker wrote.
Stefanik demanded an investigation into the “anomaly” and asked that anyone found to be involved in any sort of “scheme” to get Merchan on the three cases face discipline.
The New York State Office of Court Administration said Merchan’s assignment followed typical procedure.
“As we’ve said repeatedly, including in April 2023, Judge Merchan was assigned to supervise the special grand juries that investigated the Trump Organization and Allen Weisselberg as well as Donald Trump. He was, in turn, assigned the indictments that arose from those investigations, which is common practice since the judge supervising the grand jury investigation already has some familiarity with these often-complex cases and can manage them more efficiently,” OCA spokesperson Al Baker said in a statement.
Share this article:

Advertisement
- C++ Classes and Objects
- C++ Polymorphism
- C++ Inheritance
- C++ Abstraction
- C++ Encapsulation
- C++ OOPs Interview Questions
- C++ OOPs MCQ
- C++ Interview Questions
- C++ Function Overloading
- C++ Programs
- C++ Preprocessor
- C++ Templates
The Rule of Five in C++
- Rule Of Three in C++
- Virtual Function in C++
- real() function in C++
- Order of Evaluation in C++17
- Output in C++
- std::all_of() in C++
- Nested Classes in C++
- multimap size() function in C++ STL
- norm() function in C++ with Examples
- Removed Features of C++17
- Conversion Operators in C++
- Clockwise/Spiral Rule in C/C++ with Examples
- C++ STL - Vector in Reverse Order
- Function Overloading in C++
- Power Function in C/C++
- < iomanip > Header in C++
- How to Use the Not-Equal (!=) Operator in C++?
- Structure of C++ Program
- Chain of Pointers in C with Examples
The “ Rule of Five” is a guideline for efficient and bug-free programming in C++. The Rule of Five states that,
If any of the below functions is defined for a class, then it is better to define all of them.
It includes the following functions of a class:
- Copy Constructor
- Copy Assignment Operator
- Move Constructor
- Move Assignment Operator
The Rule of Big Five is an extension of the Rule of Three to include move semantics. The Rule of Three, consists of a destructor, copy constructor, and, copy assignment operator, use all these three functions when you are dealing with dynamically allocated resources Whereas The Rule of Five includes two more functions i.e. move constructor and move assignment operator .
Need for Rule of Five in C++
In C++ “The Rule of Big Five” is needed to properly manage the resources and efficiently handle objects. Following are the important reasons why we should use the Rule of the Big Five:
- Assume that you have some dynamically allocated resources in the object and you copy them using shallow copy. This will lead to problems like segmentation faults when the source object is destroyed. Similar thing will happen with copy assignment, move constructor and move assignment operator.
- By implementing Rule of Big Five properly we can make sure that there are no resource leaks. This can be achieved by ensuring that all the dynamically allocated memory or any other resources are released appropriately.
Let’s discuss each of the five special member functions that a class should define in detail
1. Destructor
The Destructor is used for removing/freeing up all the resources that an object has taken throughout its lifetime.With the help of this destructor, we make sure that any resources taken by objects are properly released before the object is no longer in scope.
2. Copy Constructor
Copy Constructor is used to make a new object by copying an existing object. Copy constructor is invoked when we use it to pass an object by value or when we make a copy explicitly. mainly we use copy constructor to replicate an already existing object.
3. Copy Assignment Operator
Copy Assignment Operator is a special type of function that takes care of assigning the data of one object to another object. It gets called when you use this assignment operator (=) between objects.
4. Move Constructor
Move Constructor is one of the member functions that is used to transfer the ownership of resources from one object to another. This job can easily be done by this move constructor by using a temporary object called rvalue
Explanation: In the above syntax, “noexcept” is used to indicate that the given function, like the move constructor in this case, does not throw any kind of exceptions. The move constructor is specifically designed to handle temporary objects and it requires an rvalue reference (ClassName&& other) as a parameter.
5. Move Assignment Operator
The Move Assignment Operator is comparable to the Move Constructor. It is used when an existing object is assigned the value of an rvalue. It is activated when you use the assignment operator (=) to assign the data of a temporary object(value) to an existing object.
The below example demonstrates the use of all five member functions: Destructor, Copy Constructor, Copy Assignment Operator, Move Constructor, and Move Assignment Operator.
In C++ “The Rule of Big Five” is used to ensure that the memory is properly handled i.e. memory allocation and deallocation is done properly and also resource management. It is an extension of Rule of Three. This rule says that we should use or try to use all the five functions (Destructor, Copy Constructor, Copy Assignment Operator, Move Constructor, and Move Assignment Operator) even if initially we require only one so that we can achieve application optimization, less bugs, efficient code and manage the resources efficiently.
Please Login to comment...
Similar reads.
- C++-Class and Object
- C++-Constructors
- C++-Destructors
- cpp-constructor
- Geeks Premier League
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
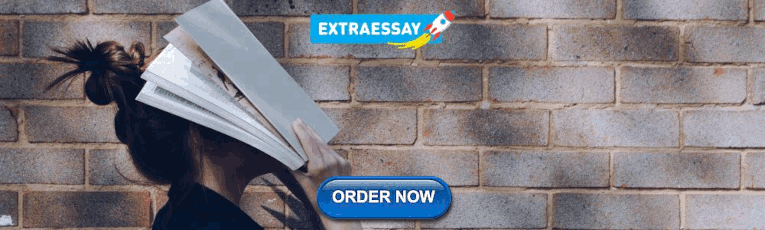
COMMENTS
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Variants. Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return ...
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left. Example: (a -= b) can be written as (a = a - b) If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
In the C++ programming language, the assignment operator, =, is the operator used for assignment. Like most other operators in C++, it can be overloaded . The copy assignment operator, often just called the "assignment operator", is a special case of assignment operator where the source (right-hand side) and destination (left-hand side) are of ...
Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return type ...
Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator ( =) to assign the value 10 to a variable called x:
This rule grammatically forbids some expressions that would be semantically invalid anyway. Some compilers ignore this rule and detect the invalidity semantically. ... In C++, the conditional operator has the same precedence as assignment operators, and prefix ++ and --and assignment operators don't have the restrictions about their operands.
This is because the assignment operator returns the value that is assigned. First, b is set to 5. Then the a is also set to 5 — the return value of b = 5, a.k.a. right operand of the assignment. As another example, the unique exponentiation operator has right-associativity, whereas other arithmetic operators have left-associativity.
Use of operator assignment shorthand is a stylistic choice. Leaving this rule turned off would allow developers to choose which style is more readable on a case-by-case basis. Version
Assignment Operator. There's a lot to be said about assignment. However, most of it has already been said in GMan's famous Copy-And-Swap FAQ, so I'll skip most of it here, only listing the perfect assignment operator for reference: X& X::operator=(X rhs) { swap(rhs); return *this; } Stream Insertion and Extraction
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
In those situations where copy assignment cannot benefit from resource reuse (it does not manage a heap-allocated array and does not have a (possibly transitive) member that does, such as a member std::vector or std::string), there is a popular convenient shorthand: the copy-and-swap assignment operator, which takes its parameter by value (thus working as both copy- and move-assignment ...
Assignment Operator. Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand. Python. # Assigning values using # Assignment Operator a = 3 b = 5 c = a + b # Output print(c) Output. 8.
This is a list of operators in the C and C++ programming languages.All the operators (except typeof) listed exist in C++; the column "Included in C", states whether an operator is also present in C. Note that C does not support operator overloading.. When not overloaded, for the operators &&, ||, and , (the comma operator), there is a sequence point after the evaluation of the first operand.
Implicitly-declared copy assignment operator. If no user-defined copy assignment operators are provided for a class type (struct, class, or union), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T:: operator = (const T &) if all of the following is ...
The Assistant can help you find answers or contact an agent. Ask the Assistant. Users reported that Category Assignment Rules show wrong information when filtered with a search in Vault Client. The information shown is representing rules that are not included in the filter.
In April, Amazon announced it would begin using drone delivery in Arizona. The FAA approval allows Amazon's commercial drone operators to employ Beyond Visual Line of Sight or BVLOS tactics when ...
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
Rep. Elise Stefanik filed a misconduct complaint against the judge overseeing Donald Trump's hush money trial, alleging that his selection to handle the former president's case is "not ...
Operator Associativity in Assignment Operators: Assignment operators, like the simple assignment (=) and compound assignments (e.g., +=), typically have right-to-left associativity. ... It is similar to the BODMAS rule we have learned in our school mathematics. I. 3 min read.
Local bus operator KMB has said commuters should avoid bringing onboard items with a total volume exceeding 0.1 cubic metres or those with a weight of more than 5kg. ... The rules for the firm's ...
Move Assignment Operator; The Rule of Big Five is an extension of the Rule of Three to include move semantics. The Rule of Three, consists of a destructor, copy constructor, and, copy assignment operator, use all these three functions when you are dealing with dynamically allocated resources Whereas The Rule of Five includes two more functions ...