- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
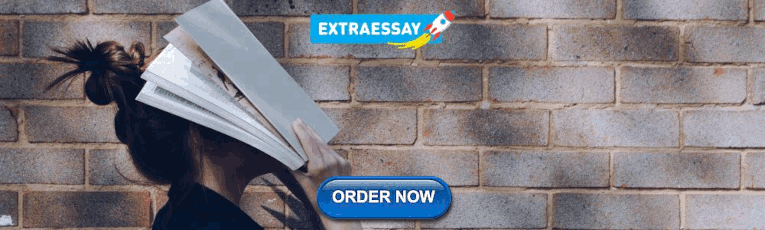
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Comparisons
We know many comparison operators from maths.
In JavaScript they are written like this:
- Greater/less than: a > b , a < b .
- Greater/less than or equals: a >= b , a <= b .
- Equals: a == b , please note the double equality sign == means the equality test, while a single one a = b means an assignment.
- Not equals: In maths the notation is ≠ , but in JavaScript it’s written as a != b .
In this article we’ll learn more about different types of comparisons, how JavaScript makes them, including important peculiarities.
At the end you’ll find a good recipe to avoid “JavaScript quirks”-related issues.
Boolean is the result
All comparison operators return a boolean value:
- true – means “yes”, “correct” or “the truth”.
- false – means “no”, “wrong” or “not the truth”.
For example:
A comparison result can be assigned to a variable, just like any value:
String comparison
To see whether a string is greater than another, JavaScript uses the so-called “dictionary” or “lexicographical” order.
In other words, strings are compared letter-by-letter.
The algorithm to compare two strings is simple:
- Compare the first character of both strings.
- If the first character from the first string is greater (or less) than the other string’s, then the first string is greater (or less) than the second. We’re done.
- Otherwise, if both strings’ first characters are the same, compare the second characters the same way.
- Repeat until the end of either string.
- If both strings end at the same length, then they are equal. Otherwise, the longer string is greater.
In the first example above, the comparison 'Z' > 'A' gets to a result at the first step.
The second comparison 'Glow' and 'Glee' needs more steps as strings are compared character-by-character:
- G is the same as G .
- l is the same as l .
- o is greater than e . Stop here. The first string is greater.
The comparison algorithm given above is roughly equivalent to the one used in dictionaries or phone books, but it’s not exactly the same.
For instance, case matters. A capital letter "A" is not equal to the lowercase "a" . Which one is greater? The lowercase "a" . Why? Because the lowercase character has a greater index in the internal encoding table JavaScript uses (Unicode). We’ll get back to specific details and consequences of this in the chapter Strings .
Comparison of different types
When comparing values of different types, JavaScript converts the values to numbers.
For boolean values, true becomes 1 and false becomes 0 .
It is possible that at the same time:
- Two values are equal.
- One of them is true as a boolean and the other one is false as a boolean.
From JavaScript’s standpoint, this result is quite normal. An equality check converts values using the numeric conversion (hence "0" becomes 0 ), while the explicit Boolean conversion uses another set of rules.
Strict equality
A regular equality check == has a problem. It cannot differentiate 0 from false :
The same thing happens with an empty string:
This happens because operands of different types are converted to numbers by the equality operator == . An empty string, just like false , becomes a zero.
What to do if we’d like to differentiate 0 from false ?
A strict equality operator === checks the equality without type conversion.
In other words, if a and b are of different types, then a === b immediately returns false without an attempt to convert them.
Let’s try it:
There is also a “strict non-equality” operator !== analogous to != .
The strict equality operator is a bit longer to write, but makes it obvious what’s going on and leaves less room for errors.
Comparison with null and undefined
There’s a non-intuitive behavior when null or undefined are compared to other values.
These values are different, because each of them is a different type.
There’s a special rule. These two are a “sweet couple”: they equal each other (in the sense of == ), but not any other value.
null/undefined are converted to numbers: null becomes 0 , while undefined becomes NaN .
Now let’s see some funny things that happen when we apply these rules. And, what’s more important, how to not fall into a trap with them.
Strange result: null vs 0
Let’s compare null with a zero:
Mathematically, that’s strange. The last result states that " null is greater than or equal to zero", so in one of the comparisons above it must be true , but they are both false.
The reason is that an equality check == and comparisons > < >= <= work differently. Comparisons convert null to a number, treating it as 0 . That’s why (3) null >= 0 is true and (1) null > 0 is false.
On the other hand, the equality check == for undefined and null is defined such that, without any conversions, they equal each other and don’t equal anything else. That’s why (2) null == 0 is false.
An incomparable undefined
The value undefined shouldn’t be compared to other values:
Why does it dislike zero so much? Always false!
We get these results because:
- Comparisons (1) and (2) return false because undefined gets converted to NaN and NaN is a special numeric value which returns false for all comparisons.
- The equality check (3) returns false because undefined only equals null , undefined , and no other value.
Avoid problems
Why did we go over these examples? Should we remember these peculiarities all the time? Well, not really. Actually, these tricky things will gradually become familiar over time, but there’s a solid way to avoid problems with them:
- Treat any comparison with undefined/null except the strict equality === with exceptional care.
- Don’t use comparisons >= > < <= with a variable which may be null/undefined , unless you’re really sure of what you’re doing. If a variable can have these values, check for them separately.
- Comparison operators return a boolean value.
- Strings are compared letter-by-letter in the “dictionary” order.
- When values of different types are compared, they get converted to numbers (with the exclusion of a strict equality check).
- The values null and undefined equal == each other and do not equal any other value.
- Be careful when using comparisons like > or < with variables that can occasionally be null/undefined . Checking for null/undefined separately is a good idea.
What will be the result for these expressions?
Some of the reasons:
- Obviously, true.
- Dictionary comparison, hence false. "a" is smaller than "p" .
- Again, dictionary comparison, first char "2" is greater than the first char "1" .
- Values null and undefined equal each other only.
- Strict equality is strict. Different types from both sides lead to false.
- Similar to (4) , null only equals undefined .
- Strict equality of different types.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Currently Reading :
Currently reading:
Table of Contents - JavaScript Not Equal:
Javascript not equal and comparison operators explained.
Ancil Eric D'Silva
Software Developer
Published on Fri Mar 11 2022
In this tutorial, you will learn about JavaScript not equal to operator, and the other Comparison operators along with examples.
What are Comparison Operators in JS?
What is “=” in js.
- Other Comparison Operators
- Closing thoughts
Comparison operators in programming languages are used to compare two values. These operators return a boolean value (true or false) based on the condition. Hence these operators are used in decision making or as conditional statements for loops.
Given its vast usage, every developer should understand the functionality of each operator. This article is a good starting point for the same, however, we do emphasize more on the JavaScript not equal (!= & !==) operators.
The JavaScript not equal or inequality operator (!=) checks whether two values are not equal and returns a boolean value. This operator tries to compare values irrespective of whether they are of different types.
However, the “!==” or Strict inequality operator does not attempt to do so and returns false if the values are unequal or of different types.
Both these operators solve different purposes and hence I would recommend practicing them to facilitate further understanding.
Code and Explanation:
In the first case, it returned true as the values were different. In the second and third cases, it returned a false cause the values are the same. Do note that in the latter case even though we passed 10 as a string the operator was able to compare both the values.
In the last case, we used the strict inequality operator and it returned true as the values were of different types.
Other Comparison Operators:
Apart from the JavaScript not equal and Strict inequality operators, we have a few other operators that solve different use cases. We have added a brief about them below.
- Equal to (==) - Check if two values are equal
- Strict equal to (===) - Checks is two values are equal and of similar type
- Greater than (>) - Checks if the value on the left is greater than the value on the right
- Greater than or equal to (>=) - Checks if the value is greater than or equal to the value on the right
- Less than (<) - Checks if the value on the left is less than the value on the right
- Less than or equal to (<=) - Checks if the value is less than or equal to the value on the right
Closing thoughts - JavaScript not equal:
In this tutorial, we covered the JavaScript not equal and the other comparison operators. As a next step do spend some quality time practicing the operators understand how they differ from each other and also try breaking them.
Once you are done with comparison operators do have a look at logical operators.
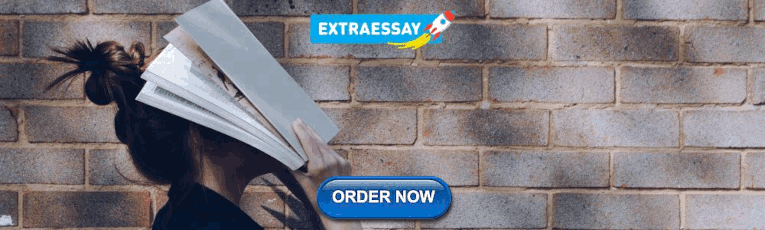
About the author
Ancil Eric D'Silva is a software development engineer known for his proficiency in full-stack development and his ability to integrate complex systems with ease, enhancing product functionality and user experience.
Related Blogs
How to use the JavaScript filter array method?
How to Link JavaScript to HTML?

Siddharth Khuntwal
JavaScript parseFloat() Method
Deep Dive into JavaScript Array findIndex() Method

Harsh Pandey
How to Make JavaScript Wait 1 Second
JavaScript instanceof operator
Vinayaka Tadepalli
Browse Flexiple's talent pool
Explore our network of top tech talent. Find the perfect match for your dream team.
- Programmers
- React Native
- Ruby on Rails
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Comparison operators
Comparison operators compare the values of two operands and evaluate whether the statement they form is true or false . The following example uses the strict equality operator ( === ) to compare two operands: the expression 2 + 2 and the value 4 . Because the result of the expression and the number value 4 are the same, this expression evaluates to true :
Type coercion and equality
Two of the most frequently-used comparison operators are == for loose equality and === for strict equality. == performs a loose comparison between two values by coercing the operands to matching data types, if possible. For example, 2 == "2" returns true , even though the comparison is being made between a number value and a string value.
The same is true of != , which returns true only if the operands being compared aren't loosely equal.
Strict comparisons using === or !== don't perform type coercion. For a strict comparison to evaluate to true , the values being compared must have the same data type. Because of this, 2 == "2" returns true , but 2 === "2" returns false :
To remove any ambiguity that might result from auto-coercion, use === whenever possible.
Truthy and falsy
All values in JavaScript are implicitly true or false , and can be coerced to the corresponding boolean value—for example, by using the "loosely equal" comparator. A limited set of values coerce to false :
- An empty string ( "" )
All other values coerce to true , including any string containing one or more characters and all nonzero numbers. These are commonly called "truthy" and "falsy" values.
Logical operators
Use the logical AND ( && ), OR ( || ), and NOT ( ! ) operators to control the flow of a script based on the evaluation of two or more conditional statements:
A logical NOT ( ! ) expression negates the truthy or falsy value of an operand, evaluating to true if the operand evaluates to false , and false if the operand evaluates to true :
Using the logical NOT operator ( ! ) in front of another data type, like a number or a string, coerces that value to a boolean and reverses the truthy or falsy value of the result.
It's common practice to use two NOT operators to quickly coerce data to its matching boolean value:
The logical AND and OR operators don't perform any coercion by themselves. They return the value of one of the two operands being evaluated, with the chosen operand determined by that evaluation.
Logical AND ( && ) returns the first of its two operands only if that operand evaluates to false , and the second operand otherwise. In comparisons that evaluate to boolean values, it returns true only if the operands on both sides of the logical AND evaluate to true . If either side evaluates to false , it returns false .
When && is used with two non-boolean operands, the first operand is returned unchanged if it can be coerced to false . If the first operand can be coerced to true , the second operand is returned unchanged:
Logical OR ( || ) returns the first of its two operands only if that operand evaluates to true , and the second operand otherwise. In comparisons that evaluate to boolean values, this means it returns true if either operand evaluates to true , and if neither side evaluates to true , it returns false :
When using || with two non-boolean operands, it returns the first operand unchanged if it could be coerced to true . If the first operand can be coerced to false , the second operand is returned unchanged:
Nullish coalescing operator
Introduced in ES2020 , the "nullish coalescing operator" ( ?? ) returns the first operand only if that operand has any value other than null or undefined . Otherwise, it returns the second operand.
?? is similar to a logical OR, but stricter in how the first operand is evaluated. || returns the second operand for any expression that can be coerced to false , including undefined and null . ?? returns the second operand when the first operand is anything but null or undefined , even if it could be coerced to false :
Logical assignment operators
Use assignment operators to assign the value of a second operator to a first operator. The most common example of this is a single equals sign ( = ), used to assign a value to a declared variable .
Use logical assignment operators to conditionally assign a value to a variable based on the truthy or falsy value of that variable.
The logical AND assignment ( &&= ) operator evaluates the second operand and assigns to the first operand if the only if the first operand would evaluate to true —effectively, "if the first operand is true, assign it the value of the second operand instead:"
The truthy or falsy value of the first operand determines whether an assignment is performed. However, trying to evaluate the first operand using a comparison operator results in a true or false boolean, which can't be assigned a value:
The logical OR assignment ( ||= ) operator evaluates the second operand and assign to the first operand if the first operand evaluates to false — effectively "if the first operand is false, assign it the value of the second operand instead:"
Check your understanding
Which operator indicates "strictly equal"?
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-03-31 UTC.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript variables, variables are containers for storing data.
JavaScript Variables can be declared in 4 ways:
- Automatically
- Using const
In this first example, x , y , and z are undeclared variables.
They are automatically declared when first used:
It is considered good programming practice to always declare variables before use.
From the examples you can guess:
- x stores the value 5
- y stores the value 6
- z stores the value 11
Example using var
The var keyword was used in all JavaScript code from 1995 to 2015.
The let and const keywords were added to JavaScript in 2015.
The var keyword should only be used in code written for older browsers.
Example using let
Example using const, mixed example.
The two variables price1 and price2 are declared with the const keyword.
These are constant values and cannot be changed.
The variable total is declared with the let keyword.
The value total can be changed.
When to Use var, let, or const?
1. Always declare variables
2. Always use const if the value should not be changed
3. Always use const if the type should not be changed (Arrays and Objects)
4. Only use let if you can't use const
5. Only use var if you MUST support old browsers.
Just Like Algebra
Just like in algebra, variables hold values:
Just like in algebra, variables are used in expressions:
From the example above, you can guess that the total is calculated to be 11.
Variables are containers for storing values.
Advertisement
JavaScript Identifiers
All JavaScript variables must be identified with unique names .
These unique names are called identifiers .
Identifiers can be short names (like x and y) or more descriptive names (age, sum, totalVolume).
The general rules for constructing names for variables (unique identifiers) are:
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter.
- Names can also begin with $ and _ (but we will not use it in this tutorial).
- Names are case sensitive (y and Y are different variables).
- Reserved words (like JavaScript keywords) cannot be used as names.
JavaScript identifiers are case-sensitive.
The Assignment Operator
In JavaScript, the equal sign ( = ) is an "assignment" operator, not an "equal to" operator.
This is different from algebra. The following does not make sense in algebra:
In JavaScript, however, it makes perfect sense: it assigns the value of x + 5 to x.
(It calculates the value of x + 5 and puts the result into x. The value of x is incremented by 5.)
The "equal to" operator is written like == in JavaScript.
JavaScript Data Types
JavaScript variables can hold numbers like 100 and text values like "John Doe".
In programming, text values are called text strings.
JavaScript can handle many types of data, but for now, just think of numbers and strings.
Strings are written inside double or single quotes. Numbers are written without quotes.
If you put a number in quotes, it will be treated as a text string.
Declaring a JavaScript Variable
Creating a variable in JavaScript is called "declaring" a variable.
You declare a JavaScript variable with the var or the let keyword:
After the declaration, the variable has no value (technically it is undefined ).
To assign a value to the variable, use the equal sign:
You can also assign a value to the variable when you declare it:
In the example below, we create a variable called carName and assign the value "Volvo" to it.
Then we "output" the value inside an HTML paragraph with id="demo":
It's a good programming practice to declare all variables at the beginning of a script.
One Statement, Many Variables
You can declare many variables in one statement.
Start the statement with let and separate the variables by comma :
A declaration can span multiple lines:
Value = undefined
In computer programs, variables are often declared without a value. The value can be something that has to be calculated, or something that will be provided later, like user input.
A variable declared without a value will have the value undefined .
The variable carName will have the value undefined after the execution of this statement:
Re-Declaring JavaScript Variables
If you re-declare a JavaScript variable declared with var , it will not lose its value.
The variable carName will still have the value "Volvo" after the execution of these statements:
You cannot re-declare a variable declared with let or const .
This will not work:
JavaScript Arithmetic
As with algebra, you can do arithmetic with JavaScript variables, using operators like = and + :
You can also add strings, but strings will be concatenated:
Also try this:
If you put a number in quotes, the rest of the numbers will be treated as strings, and concatenated.
Now try this:
JavaScript Dollar Sign $
Since JavaScript treats a dollar sign as a letter, identifiers containing $ are valid variable names:
Using the dollar sign is not very common in JavaScript, but professional programmers often use it as an alias for the main function in a JavaScript library.
In the JavaScript library jQuery, for instance, the main function $ is used to select HTML elements. In jQuery $("p"); means "select all p elements".
JavaScript Underscore (_)
Since JavaScript treats underscore as a letter, identifiers containing _ are valid variable names:
Using the underscore is not very common in JavaScript, but a convention among professional programmers is to use it as an alias for "private (hidden)" variables.
Test Yourself With Exercises
Create a variable called carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
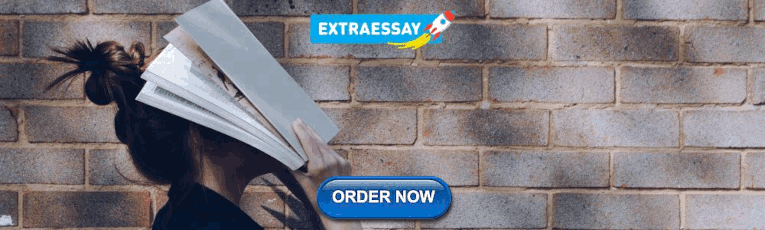
IMAGES
VIDEO
COMMENTS
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = f() is an assignment expression that assigns the value of f() to x. There are also compound assignment operators that are shorthand for the operations listed in the ...
When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
The = operator is an assignment operator. You are assigning an object to a value. The == operator is a conditional equality operation. You are confirming whether two things have equal values. There is also a === operator. This compares not only value, but also type. Assignment Operators. Comparison Operators
JavaScript Assignment. The Assignment Operator (=) assigns a value to a variable: ... not equal value or not equal type > greater than < less than >= greater than or equal to <= less than or equal to? ternary operator: Note. Comparison operators are fully described in the JS Comparisons chapter.
The strict inequality operator checks whether its operands are not equal. It is the negation of the strict equality operator so the following two lines will always give the same result: js. x !== y; !(x === y); For details of the comparison algorithm, see the page for the strict equality operator. Like the strict equality operator, the strict ...
Expressions and operators. This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. A complete and detailed list of operators and expressions is also available in the reference.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Comparisons. We know many comparison operators from maths. In JavaScript they are written like this: Greater/less than: a > b, a < b. Greater/less than or equals: a >= b, a <= b. Equals: a == b, please note the double equality sign == means the equality test, while a single one a = b means an assignment.
Learn how to use the "not equal" operator in Javascript with syntax, examples, and best practices. Avoid common mistakes and explore types of comparison operators. ... The "=" sign is the assignment operator in JavaScript. It is used to assign a value to a variable or property. On the other hand, the "!=" sign is the not equal operator.
Other Comparison Operators: Apart from the JavaScript not equal and Strict inequality operators, we have a few other operators that solve different use cases. We have added a brief about them below. Equal to (==) - Check if two values are equal. Strict equal to (===) - Checks is two values are equal and of similar type.
Truthy and falsy. All values in JavaScript are implicitly true or false, and can be coerced to the corresponding boolean value—for example, by using the "loosely equal" comparator.A limited set of values coerce to false:. 0; null; undefined; NaN; An empty string ("")All other values coerce to true, including any string containing one or more characters and all nonzero numbers.
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.
The Assignment Operator. In JavaScript, the equal sign (=) is an "assignment" operator, not an "equal to" operator. This is different from algebra. The following does not make sense in algebra: x = x + 5 In JavaScript, however, it makes perfect sense: it assigns the value of x + 5 to x.
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
Look at the 3rd line of the code. Isn't there supposed to be == instead of = after classname? Because = is an assignment operator. What I need is true which == or === should give and it indeed does from the console.log. However when I use === inside the if statement the function no longer works. But it works with the = which is not making any ...
If they are of the same type, compare them using step 1. If one of the operands is a Symbol but the other is not, return false. If one of the operands is a Boolean but the other is not, convert the boolean to a number: true is converted to 1, and false is converted to 0. Then compare the two operands loosely again.
Reference: JavaScript Tutorial: Comparison Operators. The == operator will compare for equality after doing any necessary type conversions. The === operator will not do the conversion, so if two values are not the same type === will simply return false. Both are equally quick.
Returns false if its single operand can be converted to true; otherwise, returns true.. If a value can be converted to true, the value is so-called truthy.If a value can be converted to false, the value is so-called falsy.. Examples of expressions that can be converted to false are: null;; NaN;; 0;; empty string ("" or '' or ``);undefined.