cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
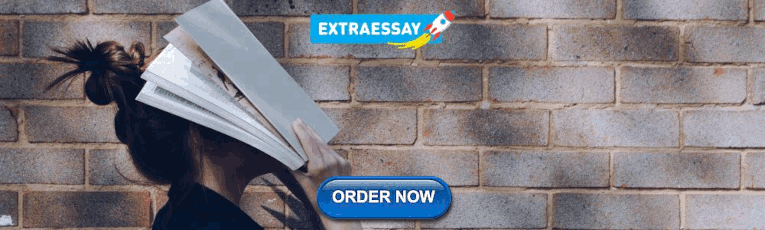
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 09:36.
- This page has been accessed 58,085 times.
- Privacy policy
- About cppreference.com
- Disclaimers


21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Assignment operators
Assignment operators modify the value of the object.
Explanation
copy assignment operator replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is a special member function, described in copy assignment operator .
move assignment operator replaces the contents of the object a with the contents of b , avoiding copying if possible ( b may be modified). For class types, this is a special member function, described in move assignment operator . (since C++11)
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
compound assignment operators replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
Builtin direct assignment
The direct assignment expressions have the form
For the built-in operator, lhs may have any non-const scalar type and rhs must be implicitly convertible to the type of lhs .
The direct assignment operator expects a modifiable lvalue as its left operand and an rvalue expression or a braced-init-list (since C++11) as its right operand, and returns an lvalue identifying the left operand after modification.
For non-class types, the right operand is first implicitly converted to the cv-unqualified type of the left operand, and then its value is copied into the object identified by left operand.
When the left operand has reference type, the assignment operator modifies the referred-to object.
If the left and the right operands identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same)
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
Builtin compound assignment
The compound assignment expressions have the form
The behavior of every builtin compound-assignment expression E1 op = E2 (where E1 is a modifiable lvalue expression and E2 is an rvalue expression or a braced-init-list (since C++11) ) is exactly the same as the behavior of the expression E1 = E1 op E2 , except that the expression E1 is evaluated only once and that it behaves as a single operation with respect to indeterminately-sequenced function calls (e.g. in f ( a + = b, g ( ) ) , the += is either not started at all or is completed as seen from inside g ( ) ).
In overload resolution against user-defined operators , for every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2, where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
Operator precedence
Operator overloading
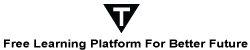
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Proposal: Annotate types in multiple assignment
In the latest version of Python (3.12.3), type annotation for single variable assignment is available:
However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid:
In this case, I propose two new syntaxes to support this feature:
- Annotate directly after each variable:
- Annotate the tuple of return:
In other programming languages, as I know, Julia and Rust support this feature in there approaches:
I’m pretty sure this has already been suggested. Did you go through the mailing list and searched for topics here? Without doing that, there’s nothing to discuss here. (Besides linking to them).
Secondly, try to not edit posts, but post a followup. Some people read these topics in mailing list mode and don’t see your edits.
- https://mail.python.org
- https://mail.python.org/archives

For reference, PEP 526 has a note about this in the “Rejected/Postponed Proposals” section:
Allow type annotations for tuple unpacking: This causes ambiguity: it’s not clear what this statement means: x, y: T Are x and y both of type T , or do we expect T to be a tuple type of two items that are distributed over x and y , or perhaps x has type Any and y has type T ? (The latter is what this would mean if this occurred in a function signature.) Rather than leave the (human) reader guessing, we forbid this, at least for now.
Personally I think the meaning of this is rather clear, especially when combined with an assignment, and I would like to see this.
Thank you for your valuable response, both regarding the discussion convention for Python development and the history of this feature.
I have found a related topic here: https://mail.python.org/archives/list/[email protected]/thread/5NZNHBDWK6EP67HSK4VNDTZNIVUOXMRS/
Here’s the part I find unconvincing:
Under what circumstances will fun() be hard to annotate, but a, b will be easy?
It’s better to annotate function arguments and return values, not variables. The preferred scenario is that fun() has a well-defined return type, and the type of a, b can be inferred (there is no reason to annotate it). This idea is presupposing there are cases where that’s difficult, but I’d like to see some examples where that applies.
Does this not work?
You don’t need from __future__ as of… 3.9, I think?

3.10 if you want A | B too: PEP 604 , although I’m not sure which version the OP is using and 3.9 hasn’t reached end of life yet.
We can’t always infer it, so annotating a variable is sometimes necessary or useful. But if the function’s return type is annotated then a, b = fun() allows type-checkers to infer the types of a and b . This stuff isn’t built in to Python and is evolving as typing changes, so what was inferred in the past might be better in the future.
So my question above was: are there any scenarios where annotating the function is difficult, but annotating the results would be easy? That seems like the motivating use case.
Would it be a solution to put it on the line above? And not allow assigning on the same line? Then it better mirrors function definitions.
It’s a long thread, so it might have been suggested already.
Actually, in cases where the called function differs from the user-defined function, we should declare the types when assignment unpacking.
Here is a simplified MWE:
NOTE: In PyTorch, the __call__ function is internally wrapped from forward .
Can’t you write this? That’s shorter than writing the type annotations.
This is the kind of example I was asking for, thanks. Is the problem that typing tools don’t trace the return type through the call because the wrapping isn’t in python?
I still suggest to read the thread you linked, like I’m doing right now.
The __call__ function is not the same as forward . There might be many other preprocessing and postprocessing steps involved inside it.
Yeah, quite a bit of pre-processing in fact… unless you don’t have hooks by the looks of it:
Related Topics
- Skip to main content
- Keyboard shortcuts for audio player
The huge solar storm is keeping power grid and satellite operators on edge

Geoff Brumfiel
Willem Marx

NASA's Solar Dynamics Observatory captured this image of solar flares early Saturday afternoon. The National Oceanic and Atmospheric Administration says there have been measurable effects and impacts from the geomagnetic storm. Solar Dynamics Observatory hide caption
NASA's Solar Dynamics Observatory captured this image of solar flares early Saturday afternoon. The National Oceanic and Atmospheric Administration says there have been measurable effects and impacts from the geomagnetic storm.
Planet Earth is getting rocked by the biggest solar storm in decades – and the potential effects have those people in charge of power grids, communications systems and satellites on edge.
The National Oceanic and Atmospheric Administration says there have been measurable effects and impacts from the geomagnetic storm that has been visible as aurora across vast swathes of the Northern Hemisphere. So far though, NOAA has seen no reports of major damage.

The Picture Show
Photos: see the northern lights from rare, solar storm.
There has been some degradation and loss to communication systems that rely on high-frequency radio waves, NOAA told NPR, as well as some preliminary indications of irregularities in power systems.
"Simply put, the power grid operators have been busy since yesterday working to keep proper, regulated current flowing without disruption," said Shawn Dahl, service coordinator for the Boulder, Co.-based Space Weather Prediction Center at NOAA.
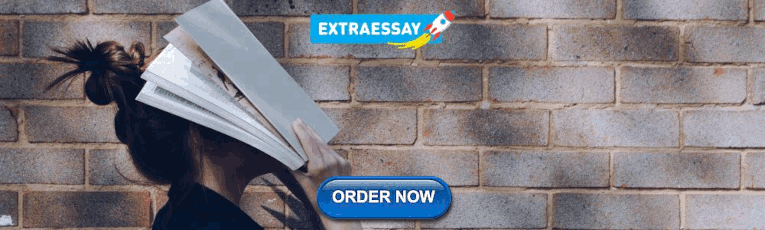
NOAA Issues First Severe Geomagnetic Storm Watch Since 2005

- LISTEN & FOLLOW
- Apple Podcasts
- Google Podcasts
- Amazon Music
- Amazon Alexa
Your support helps make our show possible and unlocks access to our sponsor-free feed.
"Satellite operators are also busy monitoring spacecraft health due to the S1-S2 storm taking place along with the severe-extreme geomagnetic storm that continues even now," Dahl added, saying some GPS systems have struggled to lock locations and offered incorrect positions.
NOAA's GOES-16 satellite captured a flare erupting occurred around 2 p.m. EDT on May 9, 2024.
As NOAA had warned late Friday, the Earth has been experiencing a G5, or "Extreme," geomagnetic storm . It's the first G5 storm to hit the planet since 2003, when a similar event temporarily knocked out power in part of Sweden and damaged electrical transformers in South Africa.
The NOAA center predicted that this current storm could induce auroras visible as far south as Northern California and Alabama.
Extreme (G5) geomagnetic conditions have been observed! pic.twitter.com/qLsC8GbWus — NOAA Space Weather Prediction Center (@NWSSWPC) May 10, 2024
Around the world on social media, posters put up photos of bright auroras visible in Russia , Scandinavia , the United Kingdom and continental Europe . Some reported seeing the aurora as far south as Mallorca, Spain .
The source of the solar storm is a cluster of sunspots on the sun's surface that is 17 times the diameter of the Earth. The spots are filled with tangled magnetic fields that can act as slingshots, throwing huge quantities of charged particles towards our planet. These events, known as coronal mass ejections, become more common during the peak of the Sun's 11-year solar cycle.
A powerful solar storm is bringing northern lights to unusual places
Usually, they miss the Earth, but this time, NOAA says several have headed directly toward our planet, and the agency predicted that several waves of flares will continue to slam into the Earth over the next few days.
While the storm has proven to be large, predicting the effects from such incidents can be difficult, Dahl said.
Shocking problems
The most disruptive solar storm ever recorded came in 1859. Known as the "Carrington Event," it generated shimmering auroras that were visible as far south as Mexico and Hawaii. It also fried telegraph systems throughout Europe and North America.

Stronger activity on the sun could bring more displays of the northern lights in 2024
While this geomagnetic storm will not be as strong, the world has grown more reliant on electronics and electrical systems. Depending on the orientation of the storm's magnetic field, it could induce unexpected electrical currents in long-distance power lines — those currents could cause safety systems to flip, triggering temporary power outages in some areas.
my cat just experienced the aurora borealis, one of the world's most radiant natural phenomena... and she doesn't care pic.twitter.com/Ee74FpWHFm — PJ (@kickthepj) May 10, 2024
The storm is also likely to disrupt the ionosphere, a section of Earth's atmosphere filled with charged particles. Some long-distance radio transmissions use the ionosphere to "bounce" signals around the globe, and those signals will likely be disrupted. The particles may also refract and otherwise scramble signals from the global positioning system, according to Rob Steenburgh, a space scientist with NOAA. Those effects can linger for a few days after the storm.
Like Dahl, Steenburgh said it's unclear just how bad the disruptions will be. While we are more dependent than ever on GPS, there are also more satellites in orbit. Moreover, the anomalies from the storm are constantly shifting through the ionosphere like ripples in a pool. "Outages, with any luck, should not be prolonged," Steenburgh said.

What Causes The Northern Lights? Scientists Finally Know For Sure
The radiation from the storm could have other undesirable effects. At high altitudes, it could damage satellites, while at low altitudes, it's likely to increase atmospheric drag, causing some satellites to sink toward the Earth.
The changes to orbits wreak havoc, warns Tuija Pulkkinen, chair of the department of climate and space sciences at the University of Michigan. Since the last solar maximum, companies such as SpaceX have launched thousands of satellites into low Earth orbit. Those satellites will now see their orbits unexpectedly changed.
"There's a lot of companies that haven't seen these kind of space weather effects before," she says.
The International Space Station lies within Earth's magnetosphere, so its astronauts should be mostly protected, Steenburgh says.
In a statement, NASA said that astronauts would not take additional measures to protect themselves. "NASA completed a thorough analysis of recent space weather activity and determined it posed no risk to the crew aboard the International Space Station and no additional precautionary measures are needed," the agency said late Friday.

People visit St Mary's lighthouse in Whitley Bay to see the aurora borealis on Friday in Whitley Bay, England. Ian Forsyth/Getty Images hide caption
People visit St Mary's lighthouse in Whitley Bay to see the aurora borealis on Friday in Whitley Bay, England.
While this storm will undoubtedly keep satellite operators and utilities busy over the next few days, individuals don't really need to do much to get ready.
"As far as what the general public should be doing, hopefully they're not having to do anything," Dahl said. "Weather permitting, they may be visible again tonight." He advised that the largest problem could be a brief blackout, so keeping some flashlights and a radio handy might prove helpful.
I took these photos near Ranfurly in Central Otago, New Zealand. Anyone can use them please spread far and wide. :-) https://t.co/NUWpLiqY2S — Dr Andrew Dickson reform/ACC (@AndrewDickson13) May 10, 2024
And don't forget to go outside and look up, adds Steenburgh. This event's aurora is visible much further south than usual.
A faint aurora can be detected by a modern cell phone camera, he adds, so even if you can't see it with your eyes, try taking a photo of the sky.
The aurora "is really the gift from space weather," he says.
- space weather
- solar flares
- solar storm
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
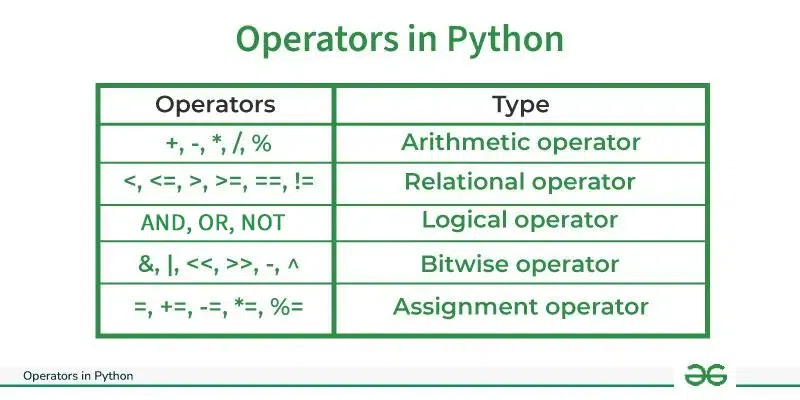
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
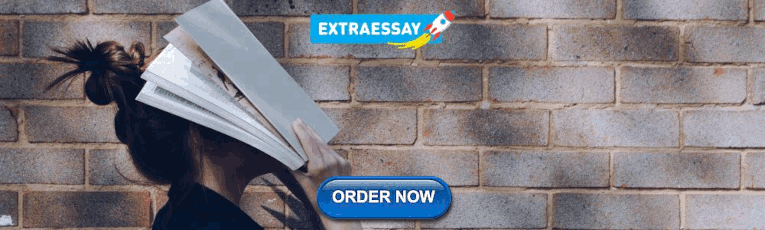
IMAGES
VIDEO
COMMENTS
The rule is to return the right-hand operand of = converted to the type of the variable which is assigned to. int a; float b; a = b = 4.5; // 4.5 is a double, it gets converted to float and stored into b. // this returns a float which is converted to an int and stored in a. // the whole expression returns an int.
The return type is important for chaining operations. Consider the following construction: a = b = c;. This should be equal to a = (b = c), i.e. c should be assigned into b and b into a. Rewrite this as a.operator=(b.operator=(c)). In order for the assignment into a to work correctly the return type of b.operator=(c) must be reference to the ...
All built-in assignment operators return * this, and most user-defined overloads also return * this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return type (including void). T2 can be any type including T.
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
The copy assignment operator, often just called the "assignment operator", is a special case of assignment operator where the source (right-hand side) and destination ... The language permits an overloaded assignment operator to have an arbitrary return type (including void). However, the operator is usually defined to return a reference to the ...
Are there any practical uses of the assignment operator's return value that could not be trivially rewritten? Generally speaking, no. The idea of having the value of an assignment expression be the value that was assigned means that we have an expression which may be used for both its side effect and its value , and that is considered by many ...
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return type ...
What is the return type of the assignment operator? Select one: a. a class object. b. *this. c. a reference of the same type as the invoking object. d. void. What happens if you open a file for output and the file doesn't exist? Select one: a. The program crashes. b. The file fails to open, but the program does not crash. c. The file is ...
Always return a reference to the newly altered left hand side, return *this. This is to allow operator chaining, e.g. a = b = c;. Always check for self assignment (this == &rhs). This is especially important when your class does its own memory allocation. MyClass& MyClass::operator=(const MyClass &rhs) {.
Simple Assignment Operator (=) To assign a value to a variable, use the basic assignment operator (=). It is the most fundamental assignment operator in Java. It assigns the value on the right side of the operator to the variable on the left side. In the above example, the variable x is assigned the value 10.
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
In the latest version of Python (3.12.3), type annotation for single variable assignment is available: a: int = 1 However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid: from typing import Any def fun() -> Any: # when hard to annotate the strict type return 1, True a: int, b: bool = fun() # INVALID In this case, I ...
That is a good convention to follow to be consistent with the behavior of operator= for built-in types. With built-in types you can do something like this: int a, b, c; // ... a = b = c = 10; If you do not return the reference to *this, the assignment chain won't be supported by your type.
Planet Earth is getting rocked by the biggest solar storm in decades - and the potential effects have those people in charge of power grids, communications systems and satellites on edge.
The first version allows to do further operations on the returned object in one line as in. (refobj = a).do_something(); When you don't return a reference to the object, this is not possible. You may think this is silly, but think about output operators. void operator<<(std::ostream &out, const Obj1 &obj1); std::ostream& operator<<(std::ostream ...
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient. There are two types of division operators: Float division; Floor division; Float division