- Assignments
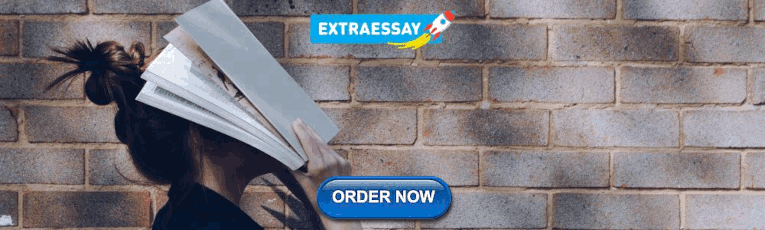
CPS 114 Introduction to Computer Networks: Labs
Lab 2: simple router, introduction.
In this lab assignment, you will write a simple router with a static routing table. We will pass to your code raw Ethernet frames and give you a function that can send a raw Ethernet frame. It is up to you to implement the forwarding logic between getting a frame and sending it out over another interface.

Figure 1: sample topology
Your router will route real packets from any machine to application servers sitting behind your router. The servers are running HTTP applications. When you have finished the forwarding path of your router, you should be able to access these servers using regular client software. In addition, you should be able to ping and traceroute to and through your functioning router. If the router is functioning correctly, all of the following operations should work:
This lab runs on top of Stanford's Virtual Network System . VNS allows you to build virtual network topologies consisting of nodes that operate on actual Ethernet frames. You don't have to know how VNS works to complete this assignment.
Getting started
First download and untar the assignment code .
You will also need to download your testing topology and auth_key file from class Forum ( DO use your own topology and auth_key file). Please
Here is one sample rtable file for topology in Figure 1. The base rtable file only has a default route to the firewall.
You can then build and run the assignment code (TOPOLOGY_ID and USER_NAME are in the topology file): xwy@linux21$ make xwy@linux21$ ./sr -s vns-2.stanford.edu -t <TOPOLOGY_ID> -u <USER_NAME>
From another terminal or another machine, try pinging one of the router's interfaces. Currently, all the assignment code does is print that it gets a packet to the command line.
Understanding the code
Data structure.
sr_router.[h|c] : The full context of the router is housed in the struct sr_instance (sr_router.h). sr_instance contains information about the topology the router is routing for as well as the routing table and the list of interfaces.
sr_if.[h|c] : After connecting, the server will send the client the hardware information for that host. The assignment code uses this to create a linked list of interfaces in the router instance at member if_list. Utility methods for handling the interface list can be found at sr_if.[h|c].
sr_rt.[h|c] : The routing table in the stub code is read on from a file (default filename "rtable", can be set with command line option -r ) and stored in a linked list of routing entries in the current routing instance.
sr_arpcache.[h|c] : You will need to add ARP requests and packets waiting on responses to those ARP requests to the ARP request queue. When an ARP response arrives, you will have to remove the ARP request from the queue and place it onto the ARP cache, forwarding any packets that were waiting on that ARP request. Pseudocode for these operations is provided in sr_arpcache.h. The base code already creates a thread that times out ARP cache entries 15 seconds after they are added for you. You must fill out the sr_arpcache_sweepreqs function in sr_arpcache.c that gets called every second to iterate through the ARP request queue and re-send ARP requests if necessary. Psuedocode for this is provided in sr_arpcache.h.
Important Functions
void sr_handlepacket(struct sr_instance* sr, uint8_t * packet, unsigned int len, char* interface)
This function receives a raw Ethernet frame and sends raw Ethernet frames when sending a reply to the sending host or forwarding the frame to the next hop. This method, located in sr_router.c, is called by the router each time a packet is received. The "packet" argument points to the packet buffer which contains the full packet including the ethernet header. The name of the receiving interface is passed into the method as well.
int sr_send_packet(struct sr_instance* sr, uint8_t* buf, unsigned int len, const char* iface)
This method, located in sr_vns_comm.c, will send an arbitrary packet of length, len, to the network out of the interface specified by iface.
void sr_arpcache_sweepreqs(struct sr_instance *sr)
The assignment requires you to send an ARP request about once a second until a reply comes back or we have sent five requests. This function is defined in sr_arpcache.c and called every second, and you should add code that iterates through the ARP request queue and re-sends any outstanding ARP requests that haven't been sent in the past second. If an ARP request has been sent 5 times with no response, a destination host unreachable should go back to all the sender of packets that were waiting on a reply to this ARP request.
Requirements
- The router enforces guarantees on timeouts--that is, if an ARP request is not responded to within a fixed period of time, the ICMP host unreachable message is generated even if no more packets arrive at the router.
Functions and data structures you need to implement
There is no specific requirement which function you can/cannot modify. To make the simple router work, you need to handle 4 kinds of packets correctly: Ethernet , IP , ICMP and ARP . For the actual specifications, there are also the RFC's for ARP (RFC826) , IP (RFC791) , and ICMP (RFC792) .
In our reference implementation, we developed sr_ip.[h|c], sr_icmp.[h|c] (you need to add these source files to the Makefile). We also modified sr_router.[h|c], protocol.h and sr_arpcache.[h|c]. Total work load is around 550 lines of c code.
Understanding Protocols
You are given a raw Ethernet frame and have to send raw Ethernet frames. You should understand source and destination MAC addresses and the idea that we forward a packet one hop by changing the destination MAC address of the forwarded packet to the MAC address of the next hop's incoming interface.
Internet Protocol
You should understand how to find the longest prefix match of a destination IP address in the routing table. If you determine that a datagram should be forwarded, you should correctly decrement the TTL field of the header and recompute the checksum over the changed header before forwarding it to the next hop.
Internet Control Message Protocol
ICMP is used to send control information back to the sending host. You will need to properly generate the following ICMP messages (including the ICMP header checksum) in response to the sending host under the following conditions:
Address Resolution Protocol
ARP is needed to determine the next-hop MAC address that corresponds to the next-hop IP address stored in the routing table. Without the ability to generate an ARP request and process ARP replies, your router would not be able to fill out the destination MAC address field of the raw Ethernet frame you are sending over the outgoing interface. Analogously, without the ability to process ARP requests and generate ARP replies, no other router could send your router Ethernet frames. Therefore, your router must generate and process ARP requests and replies. To lessen the number of ARP requests sent out, you are required to cache ARP replies. Cache entries should time out after 15 seconds to minimize staleness. The provided ARP cache class already times the entries out for you.
When forwarding a packet to a next-hop IP address, the router should first check the ARP cache for the corresponding MAC address before sending an ARP request. In the case of a cache miss, an ARP request should be sent to a target IP address about once every second until a reply comes in. If the ARP request is sent five times with no reply, an ICMP destination host unreachable is sent back to the source IP as stated above. The provided ARP request queue will help you manage the request queue.
In the case of an ARP request, you should only send an ARP reply if the target IP address is one of your router's IP addresses. In the case of an ARP reply, you should only cache the entry if the target IP address is one of your router's IP addresses. Note that ARP requests are sent to the broadcast MAC address (ff-ff-ff-ff-ff-ff). ARP replies are sent directly to the requester's MAC address.
Testing and Debugging
The above test case does not include Destination Host Unreachable , Destination Unreachable and Protocol Unreachable tests. To do these three tests, you may use test4 at cps114.cod.cs.duke.edu . Before you start these tests, you need to reconfigure your router/rtable. Let's take Figure 1 as an example.
Suppose your topology is shown in Figure 1, the allocated IP block is 171.67.243.176/29 (8 IP addresses). Packets heading to this IP block will be routed to your router. This IP block consists of 4 /31 blocks. They are 171.67.243.176/31 (connected to eth0), 171.67.243.178/31, 171.67.243.180/31 (connected to eth1) and 171.67.243.182/31 (connected to eth2). 171.67.243.178/31 is not connected to any interface.
171.67.243.179 171.67.243.178 255.255.255.254 eth1
to your routing table, once your router receives a packet heading to 171.67.243.179, it will send ARP request for 171.67.243.178 through eth1. There will be no reply since 171.67.243.178 does not exist at all. After 5 ARP requests without any reply, a Destination Host Unreachable ICMP packet will be generated by your router. At this point, your route/rtable should look like
0.0.0.0 172.24.74.17 0.0.0.0 eth0 171.67.243.181 171.67.243.181 255.255.255.255 eth1 171.67.243.183 171.67.243.183 255.255.255.255 eth2 171.67.243.179 171.67.243.178 255.255.255.254 eth1
For the Destination Unreachable test, We do following changes to the above routing table. First, we remove the default route. Second we remove the routing table entry for 171.67.243.179. Third, we add a entry for any PC in Figure 1. For example, if any PC is cps114.cod.cs.duke.edu , we add a entry for its IP address (152.3.145.121) to the routing table. After these three changes, your routing table should look like.
152.3.145.121 172.24.74.17 255.255.255.0 eth0 171.67.243.181 171.67.243.181 255.255.255.255 eth1 171.67.243.183 171.67.243.183 255.255.255.255 eth2
Right now, your router/rtable is ready for the Destination Unreachable test. You may simply send a UDP packet heading for 171.67.243.179 from cps114.cod.cs.duke.edu (152.3.145.121). Your router should be able to generate a Destination Unreachable ICMP packet and send it to cps114.cod.cs.duke.edu . At this point, you may think about why do we do the above three modifications. Try not to delete the default route, what do you find?
For the Protocol Unreachable test, you may simply send one UDP packet to any of your router interfaces. Your router should be able to generate a protocol unreachable packt and send it to you.
For the Destination Host Unreachable test, Destination Unreachable test and Protocol Unreachable tests, you can log on to cps114.cod.cs.duke.edu with your cs appartment account and use test4 to send UDP packets and receive ICMP feedbacks. You need to create and modify config.xml . <dst> stands for your UDP packet's destination address, <intf> is the interface IP addresses of your router. Then run the test case as following. xwy@cps114:~$ test4 config.xml This test4 sends 3 UDP packets to your specified IP address, grabs received ICMP packets and check these packets' source addresses, ICMP type and code fields.
- Run the command make submit , this should create a file called router.tar.gz.
- Upload router.tar.gz to the lab2 assignment on Blackboard .
Collaboration policy
You can discuss with anyone, but you must not look at each other's code, or copy code from others.
Acknowledgement
Administrivia
Lectures and assignments, second half.
This course is an introduction to the implementation and design of computer networks. The goal of this course is learn the standard protocols of modern networks and to learn how to interact with networks through standard programming practices. We will cover the entire network stack: from physical and link layer properties; (inter-) network and transport protocols; application and socket programming; and network security. The programming assignments in this course will be a mix of C and Python programming.
Course Goals/Promisses
Homework policy, participation policy, academic integrity.
Academic honesty is required in all work you submit to be graded. With the exception of your lab partner on approved lab assignments, you may not submit work done with (or by) someone else, or examine or use work done by others to complete your own work. Your code should never be shared with anyone; you may not examine or use code belonging to someone else, nor may you let anyone else look at or make a copy of your code. This includes sharing solutions after the due date of the assignment.
Discussing ideas and approaches to problems with others on a general level is fine (in fact, we encourage you to discuss general strategies with each other), but you should never read anyone else's code or let anyone else read your code. You may discuss assignment specifications and requirements with others in the class to be sure you understand the problem. In addition, you are allowed to work with others to help learn the course material. However, with the exception of your lab partner, you may not work with others on your assignments in any capacity.
``It is the opinion of the faculty that for an intentional first offense, failure in the course is normally appropriate. Suspension for a semester or deprivation of the degree in that year may also be appropriate when warranted by the seriousness of the offense.'' - Swarthmore College Bulletin (2008-2009, Section 7.1.2)
Class Schedule
Computer Networks
COS 461, Princeton University Spring 2019
Assignments
This course studies computer networks and protocols, the services built on top of them, and some topics relating to Internet policy. Topics include: packet switching, routing, congestion control, quality-of-service, network security, network measurement, network management, and network applications.
This course is "flipped", with mandatory instructional videos and optional class meetings with discussions, applications of course material to current Internet development and events, group work, and practice problems. Students will learn:
- Internet protocols used in Internet access networks, local area networks, wide area networks
- Internet services including Internet content delivery
- Measurement, data analysis, and machine learning in managing network performance and security.
Contact: Prof. Nick Feamster 310 Sherrerd Hall <my last name>@cs.princeton.edu
- Austin Hounsel
- Arunesh Mathur
- Trisha Datta
- Leora Huebner
- Nathan Mytelka
Class Location and Time: Lectures: Mondays and Wednesdays, 1:30-2:50 pm, Frist Campus Center 302
- Nick: Mondays and Wednesdays 11a-12p (310 Sherrerd; sign up in advance )
- Austin: TTR 2-3p (Sherrerd Hall Third Floor Open Space)
- Arunesh: MW 4-5p (Sherrerd Hall Third Floor Open Space)
Course Format
The course will meet twice a week for 80-minute class meetings .
Each class meeting will contain discussion, activities, and material that assumes that you have watched the preparation videos in advance. Preparation may be tested without warning.
The primary assignments for the course will be lab-based programming assignments. We will also release weekly problem set exercises whose primary purpose is to help in preparation for the midterm and final exam; these problem sets may be graded at random.
Recommended Background
Prerequisite: COS 217. Although not required, and one of either COS 333, COS 418, or COS 432 prior to enrolling in the course (concurrent enrollement does not count). Note well: The assignments in this class are in Go, C, and Python. You do not need to have experience with all of these languages before taking the course. However, you should be comfortable with learning new programming languages and finding resources on your own, independently as you attack problems.
You will be exposed to new programming languages and environments. If you are not comfortable learning new programming languages and familiarizing yourself with new programming environments on your own, you will have difficulty in this course.
- an in-class midterm (15%)
- an in-class end-of-term exam (20%)
- seven programming assignments (8% each, 16% for the last assignment)
- participation (Piazza and in-class), in-class quizzes, pencil-and-paper "practice" assignments (1%)
Late Policy
- You start the term with a grace period "balance" of 96 hours.
- Each assignment will be due at 5:00 p.m. (Princeton Local Time) on the due date.
- For each assignment, every hour late (or fraction thereof) that you turn in the assignment will subtract one hour from your grace-period balance. For example, if you turn in your assignment at 6:02 p.m. on the due date, we will count this as two hours against your grace period.
- As long as your grace period balance is positive, you can turn in any assignment late without penalty.
- Once your grace period balance reaches zero, you will receive half credit for any assignment that you turn in, as long as you turn it in within one week of the due date. If your grace period balance is zero and you turn in an assignment more than one week late, you will receive no credit for the assignment. Important: You must still turn in all assignments to pass the course, even if you receive zero points on an assignment. Turning in all assignments is a necessary condition for passing.
Students are expected to abide by the Princeton University Honor Code. Honest and ethical behavior is expected at all times. All incidents of suspected dishonesty will be reported to and handled by the office of student affairs. You are to do all assignments yourself, unless explicitly told otherwise. You may discuss the assignments with your classmates, but you may not copy any solution (or part of a solution) from a classmate.
Reading and Videos
Supplementary Videos: Prof. Feamster's Networking Videos .
- Computer Networking (5th edition) , by Andrew Tanenbaum and David Wetherall
- Computer Networking: A Top-Down Approach (6th edition) , by Jim Kurose and Keith Ross
- Computer Networks: A Systems Approach , Larry Peterson and Bruce Davie
- TCP/IP Illustrated, Volume 1: The Protocols and Unix Network Programming, Volume 1: The Sockets Networking API (3rd Edition)
This schedule and syllabus is preliminary and subject to change. Edits will be ongoing, but you can assume that all updates for the subsequent week will be completed by 5p ET of the Sunday prior to the week of instruction.
Readings: Abbreviations refer to the following:
- TW: Tanenbaum/Wetherall (6th Edition - Draft)
- KR: Kurose/Ross (6th edition)
- PD: Peterson/Davie (open-source edition)
For Tanenbaum, the relevant excerpts will be posted on Blackboard, as part of revisions for the upcoming 6th edition. These readings are required. KW and PD readings are optional, unless otherwise specified.
Videos: In addition to the readings, we recommend watching the corresponding videos from the YouTube Playlist , before lecture.
Slides: All slides will be posted on Blackboard before class meeting.
Course Resources
- Piazza Online Discussion Forum
- Course Blackboard Page (Slides, readings, old midterms and exams (with solutions), and other course resources are posted here.)
Go Resources
- Go Language Blog
- defer and exception management
- arrays and slices
- formatting code
- concurreny in practice
- builtin identifiers
- race detection
- Go Language FAQ
- Go Lecture by Rob Pike
- Packet Processing in Go
- Understanding Go Internals
Software Resources
- VirtualBox Environment
- Official virtual machine image (To be posted.)
- Mininet Walkthrough
Going Further (Optional)
- Various Online Reading from Professor Feamster
- Connection Management Blog : Blog where various topics will be discussed during the course.
- A Research "How To" Blog
- Udacity Course Modules (some may be useful for additional explanation)
The following assignments below have been officially released.
We have linked the public repository, which contains assignments as they were released last year, for reference. Although the assignments will be similar, we advise against starting early on (last year's) assignments in the repository that haven't been officially released as these are still subject to change and update. Course staff will not answer questions on any assignments that have not yet been officially released.
- Programming Assignment 1
- Programming Assignment 2
- Programming Assignment 3
- Programming Assignment 4
- Programming Assignment 5
- Programming Assignment 6
- Programming Assignment 7
Extra Credit Opportunities
Assignment maintenance.
All assignments are weighted according to the syllabus. There are opportunities for extra credit on this year's assignments, to encourage student contributions to keeping the material current.
- One instructor endorsed Piazza post: 1% single assignment point bonus, up to a maximum of 10% bonus for each assignment.
All assignment concepts should be proposed and approved in advance by the instructors. Point bonuses are "all or nothing" and will be conferred upon successful pull request to the COS 461 github repository.
Reading Maintenance
Professor Feamster is revising the Tanenbaum and Wetherall book, and this term we will be using draft sections of the book for our readings.
Each error you discover in the reading material, typographical, conceptual, or otherwise will be worth a 1% assignment point bonus.
If you find a missing topic that you would like to see better covered in the book, we are open to suggestions. If you offer sufficient resources and material, extra credit will be conferred at the instructors' discretion, not to exceed a 5% assignment point bonus per suggestion.
Network Fundamentals Lab 1
Lesson Contents
Congratulations! You’ve been selected as the chief network engineer for NovaTech Pioneers, an emerging leader in the realm of digital innovation. With the company poised for unprecedented growth, a brand-new campus is on the horizon to house its expanding operations and ambitious projects.
Your pivotal role involves constructing a sophisticated campus network from the ground up. This network is the heartbeat of NovaTech Pioneers, designed to underpin a wide array of activities, from cutting-edge research to global collaboration.
As you embark on this comprehensive journey, here’s what you can expect to engage with in bringing the NovaTech Pioneers campus network to life:
- LAN Switching Technologies : Your first steps involve VLAN setup for network segmentation and security, trunking to facilitate efficient switch connections, and implementing Spanning Tree Protocol (STP) for network resilience and loop prevention.
- Layer 3 Implementations : Advance to configuring IP addresses for device communication and routing, ensuring packets navigate the network efficiently. You’ll work with both static routes and dynamic routing protocols.
- IP Services : Set up Dynamic Host Configuration Protocol (DHCP) for dynamic IP address assignment, Network Address Translation (NAT) for secure internet access, and Virtual Router Redundancy Protocol (VRRP) for router failover and high availability.
- Network Management : Implement Network Time Protocol (NTP) to synchronize network devices, essential for accurate logging and event management. Syslog for reliable logging. Security will be a continuous focus, safeguarding every network layer.
- Security : Improve the network’s security such as using access-lists to restrict traffic, configure SSH for secure remote login, and more.
This lab is a proving ground where theory meets practice, designed to challenge you and foster a deep understanding of network component interactions. You’ll develop a robust set of skills and best practices vital for a network engineer.
Your expertise will drive NovaTech Pioneers into a new era. It’s time to unleash your potential and create a network that is not only scalable and reliable but also a foundation for the company’s visionary goals. Welcome to NovaTech Pioneers, where your journey in shaping the future of networking begins.
Here is the topology for this lab:
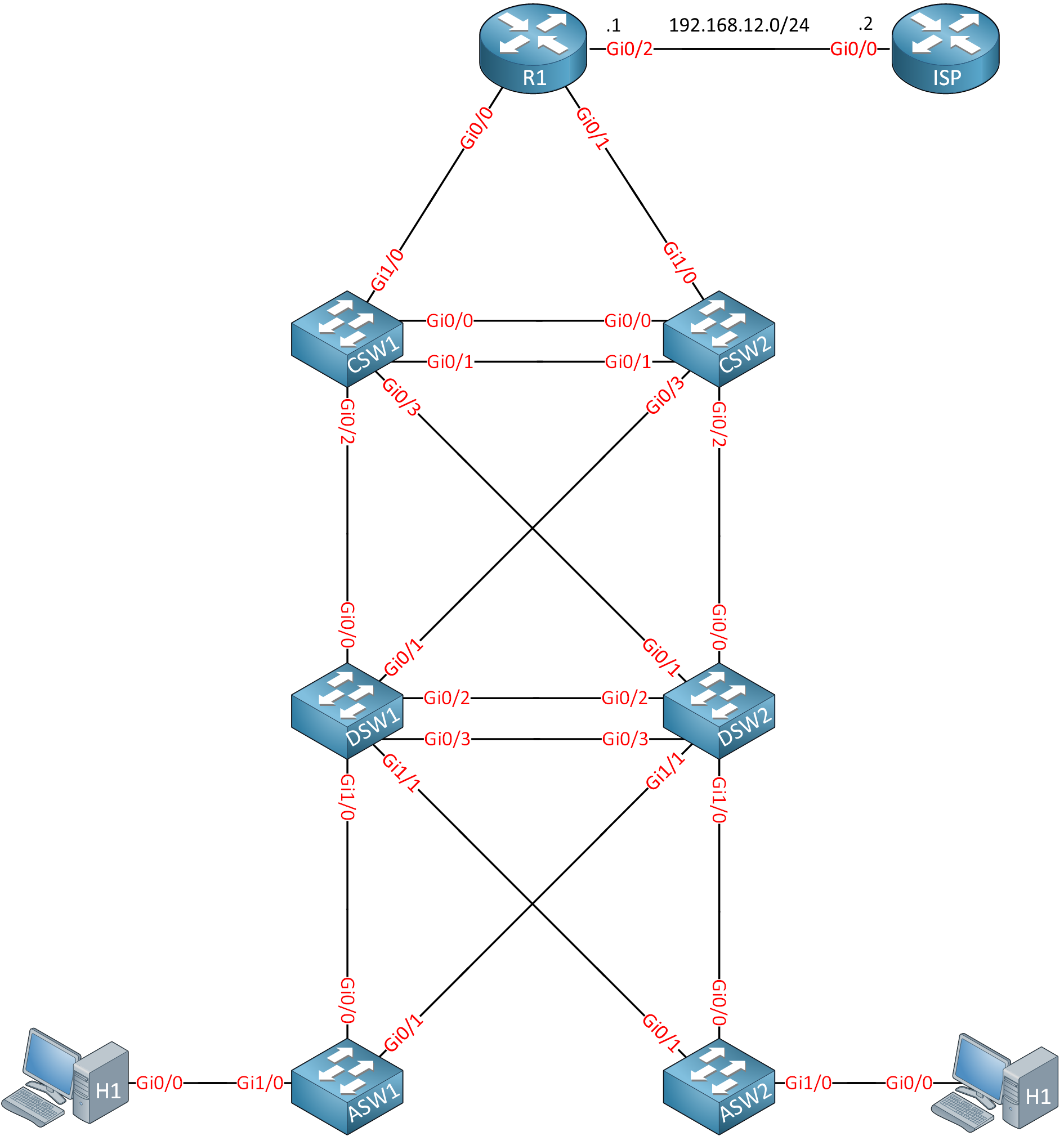
Startup Configs
Configurations
Want to take a look for yourself? Here you will find the startup configuration of the ISP router.
The tasks are described as if you are on the job, and someone asks you to configure this network. It’s not a cookbook where we tell exactly what to do and in what order. This helps you think through what is really needed instead of just typing in commands.
- Each device should have a configured hostname.
- Logging messages on R1 should never interrupt what you are typing on the CLI.
- The console log on R1 should never timeout if you are idle.
- The interfaces on the core switches that connect to R1 require a description so you know what the interfaces are for.
- When you mistype a command on R1, it shouldn’t attempt to connect to a hostname.
LAN Switching Technologies
- VLAN 10: CLIENTS
- VLAN 20: VOICE
- VLAN 30: PRINTERS
- VLAN 40: MANAGEMENT
- H1 and H2 should be assigned to VLAN 10.
- Prepare interface GigabitEthernet 0/2 on ASW1 so that the interface can connect to a computer in VLAN 10 and a phone in VLAN 20.
- The access layer switches should use a dynamic method to negotiate the trunk link.
- The distribution layer switches should use a static method to create a trunk.
- You need to use a negotiation, but due to company policy, you are not allowed to use a proprietary protocol.
- ASW1 should actively negotiate, while DSW1 should only respond to requests.
- You are not allowed to use any protocols to establish this link.
- Configure the network so that we use the fastest spanning tree protocol option. A spanning tree should be calculated for every VLAN.
- The DSW1 switch has to be the root bridge for all VLANs. When this switch fails, SW2 should take over.
- The hosts are modern computers that boot up in a couple of seconds. Make sure that when they are connected, they can send traffic immediately, and they don’t have to wait for the network to converge.
- When a host sends a BPDU, the interface should go down immediately.
- The interface should recover automatically after 10 minutes.
- Spanning tree BPDUs should be sent every three seconds.
- The port state should transition after ten seconds when a new configuration BPDU propagates throughout the network.
- A configuration BPDU should expire after 15 seconds.
- Make sure these timers are always used, even when another switch becomes the root bridge.
IP Addressing
The campus network requires IPv4 and IPv6 addressing.
Here are the requirements for IPv4:
- The campus network has to use the 10.0.0.0/8 prefix.
- VLAN 10: contains a maximum of 100 devices.
- VLAN 20: contains a maximum of 40 devices.
- VLAN 30: contains a maximum of 12 devices.
- VLAN 40: contains a maximum of 6 devices.
- Each device requires a loopback interface with a /32 IP address.
- Point-to-point links require IP addresses (when needed)
- R1: 172.16.1.1
- ISP: 172.16.1.2
You must be as efficient as possible with IP address space while considering future growth for new network devices and VLANs. The number of devices per VLAN will not change in the future. Configure all IPv4 addresses.
Here are the requirements for IPv6:
- You must use the 2001:db8::/32 prefix.
- VLANs: use a /64 prefix.
- Loopback interfaces: Use a /128 prefix.
- Point-to-point links: Use a /127 prefix.
Configure all IPv6 addresses.
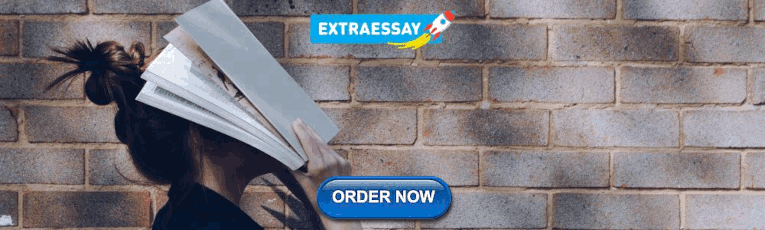
Routing Technologies
The campus network requires IPv4 and IPv6 routing.
Here are the IPv4 requirements:
- Configure R1 so that you can reach 1.1.1.1 or any other network behind the ISP router. You are not allowed to make any changes to the ISP router.
- You must use a non-proprietary link-state routing protocol that converges quickly.
- All devices should be in a single area.
- Distribution layer switches shouldn’t have any unnecessary neighbor adjacencies.
- The core layer switches should not have a DR/BDR election on their bundled interface.
- A hello packet should be sent every 5 seconds.
- The neighbor adjacency should be declared down after not receiving hello packets for 15 seconds.
- All loopback and VLAN interfaces should be reachable.
Here are the IPv6 requirements:
- Configure R1 so that you can reach 2001:DB8::1:1:1:1 or any other network behind the ISP router. You are not allowed to make any changes to the ISP router.
- Use the IPv4 address on the loopback interfaces as the router ID.
- Use a single area.
- Ensure there are no unneeded neighbor adjacencies between the distribution layer switches.
- Advertise a default route on R1 in the routing protocol.
Network Services
Users in VLAN 10 have been complaining that their default gateway sometimes becomes unreachable. This happens because of stability issues on DSW1 and DSW2.
- Configure a virtual default gateway address for VLAN 10 so that this won’t cause any more issues.
Hosts should be able to receive IP addresses automatically:
- Use the virtual gateway address as the default gateway.
- Use DNS server 1.1.1.1.
- Use domain name MYDOMAIN.LOCAL
- Lease time 48 hours.
- Configure a pool on R1 for VLAN 20 and include the IP address of TFTP server 10.0.0.135.
- Test the IP address assignment by disabling routing on H1 and H2 and enable the DHCP client.
Users request access to the server on 1.1.1.1:
- Configure R1 so that all campus switches and hosts can reach 1.1.1.1.
- You are not allowed to make any changes to the ISP router.
The security officer noticed that the logging information was inconsistent. To solve this, all clocks on the switches have to be synchronized:
- R1 should use 1.1.1.1 as the time source.
- Core layer switches should use R1 as the time source.
- Distribution layer switches should use the core switches as a time source.
- Access layer switches should use the distribution layer switches as a time source.
The company policy requires some security measures:
- When someone logs into R1, it should show an “authorized users only” message.
- CSW1 should show a MOTD message “This is CSW1”.
- Only a single MAC address is allowed.
- The first learned MAC address should be stored.
- When there is a violation, the interface should be shut.
- Hosts in VLAN 10 are not allowed to reach the HTTP server on 1.1.1.1. All other traffic is permitted.
Network Management
- DSW1 requires an external syslog server located at 10.0.0.240.
- Messages should be sent every five seconds.
- When a device doesn’t receive any messages, information should be discarded after 60 seconds.
- Messages should not be sent to hosts or the ISP.
- Don’t send or receive messages on the interfaces that connect to the distribution layer switches.
- Don’t send any messages to hosts but you should receive them.
- Use username “cisco” with password “cisco”.
- The password should not be stored in clear text.
- Telnet traffic should not be permitted.
This lab was configured using these images:
- Switches : Cisco IOS Software, vios_l2 Software (vios_l2-ADVENTERPRISEK9-M), Experimental Version 15.2(20200924:215240) [sweickge-sep24-2020-l2iol-release 135]
- Routers : Cisco IOS Software, IOSv Software (VIOS-ADVENTERPRISEK9-M), Version 15.9(3)M6, RELEASE SOFTWARE (fc1)
We’ll start with the basics. Use enable and configure terminal to start the configuration. If you are unsure about how the Cisco CLI works, take a look at our Introduction to Cisco IOS CLI lesson .
We’ll start with the hostname on all devices:
After changing the hostname, it shows up right away on the CLI:
The hostname also shows up in the running configuration:
Logging Synchronous
Logging synchronous appends commands to a new line. This can be useful if you don’t want to interrupt what you are typing on the CLI:
Exec Timeout
If you don’t want the console line to log out because it’s idle, you can use the exec timeout command. Here is the default setting:
The default idle timeout is 10 minutes. We’ll set it to 0 which disables it:
Now it shows up as “never”:
Interface Description
Interface descriptions can be useful to quickly see what the interface is used for. This is how to configure one:
You can see the description here:
DNS Lookups
When you mistype a command, Cisco IOS thinks you want to connect to a hostname. For example:
This can be annoying when you don’t have a DNS server configured because it takes time. You can disable it like this:
Once configured, Cisco IOS won’t attempt to do a DNS lookup anymore:
This is where we configure all L2 technologies.
We need to configure VLANs.
We’ll manually create all VLANs on the required switches:
We only use VLANs on the access and distribution layer switches.
This is how you can check the VLANs you create:
The VLANs with their names are created.
Switchport VLAN Assignment
The interfaces that connect to H1 and H2 need to be in VLAN 10:
Because these are interfaces that connect to hosts, it’s best to change the switchport mode to access. If you leave them at the default, a host could try to create a trunk to your switch.
You can see the assigned interfaces to each VLAN in this overview:
Or you can look at the switchport settings per interface:
You can assign a Voice VLAN to an interface using the switchport voice command. Here is how to do this:
We can verify the voice VLAN per interface like this:
We need trunks between the access layer and distribution layer switches. The default switchport state looks like this:
It is dynamic auto , and the operational mode becomes access. We’ll change the interfaces to dynamic desirable:
The end result will be a trunk port:
The interfaces on the distribution layer switches that connect to the access layer switches have to be configured in static trunk mode:
This won’t change the end result because the interfaces are already in trunk mode, but it was a requirement.
Let’s verify our work:
This interface is configured as a static trunk.
Etherchannel
We can configure L2 or L3 Etherchannels .
L2 Etherchannel
We start with the Etherchannel between DSW1 and DSW2. This has to be an L2 Etherchannel that uses LACP:
The two switches will actively negotiate using LACP. We’ll set the encapsulation type to 802.1Q and make it a static trunk. Here’s how to verify it:
The port-channel interface works, and we use LACP. You can also verify it like this:
The output above tells us that the interface is active and uses LACP. Here is one more command:
This gives us a similar output. We can verify the trunk state by looking at the switchport information:
The port-channel 1 interface is in trunk mode.
L3 Etherchannel
The interfaces that connect the two core switches require an L3 EtherChannel and shouldn’t use LACP. Here’s how to configure it:
The no switchport command turns the interfaces into routed ports. Using mode on for the channel-group command creates a static Etherchannel. Here’s how to verify it:
The output above tells us the port-channel 1 interface is active and that it is an L3 EtherChannel.
Spanning Tree (STP)
Let’s look at some spanning tree configurations.
The default spanning tree mode is PVST . You can verify it like this:
Or you can use this command:
Let’s change it on all access layer and distribution layer switches where we use spanning tree:
This changes the spanning tree mode to RPVST .
Primary and Secondary Root
We need to configure DSW1 always to be the root bridge for all VLANs . We can enforce this by changing its priority to 0:
We can verify it here:
DSW2 should be the root bridge in case something happens with DSW1. We can achieve this by setting the priority to the second best value:
We can see this priority here:
The access layer switches will have the default priority so they won’t become the root bridge:
STP Portfast and BPDU Guard
The interfaces that connect to the hosts require spanning tree portfast and BPDU guard . Here’s how to configure this:
You can verify that these two options are enabled with this command:
When the interface goes into error-disabled mode because of BPDU guard, it should automatically recover in 10 minutes. We can configure this with the errdisable command:
We can check that this has been enabled:
And you can see the timer here:
Spanning Tree Timers
We need to tune some of the spanning tree timers. Here are the requirements:
- hello-delay timer to 3 seconds
- forward-delay timer to 10 seconds
- maximum-aging timer to 20 seconds
Here is how you can configure this:
You only need to configure these on the root bridge but our task requires us that we use these timers, even if another switch becomes the root bridge. You can verify these timers here:
We have to configure IPv4 and IPv6.
We have the 10.0.0.0/8, and we have these requirements:
- VLAN 40: containers a maximum of 6 devices.
- Loopback interfaces: each device requires a loopback interface with an IP address.
- Point-to-Point links: we need two IP addresses, one on each end.
We should be as efficient as possible and take future growth into account. There are many ways how you can solve this. Since this is a lab about networking fundamentals, we’ll throw in some subnetting and VLSM .
We’ll start with the VLANs. We’ll use the address space up to 10.0.127.x in case we want to create more VLANs in the future.
- Has a maximum of 100 devices:
- A /25 subnet offers 126 usable IP addresses.
- network address: 10.0.0.0/25
- usable IP addresses: 10.0.0.1 -to 10.0.0.126
- broadcast address: 10.0.0.127
- Has a maximum of 40 devices
- A /26 subnet offers 62 usable IP addresses:
- network address: 10.0.0.128/26
- usable IP addresses: 10.0.0.129 -to 10.0.0.190
- broadcast address: 10.0.0.191
- Has a maximum of 12 devices.
- A /28 subnet offers 14 usable IP addresses.
- network address: 10.0.0.192/28
- usable IP addresses: 10.0.0.193 -to 10.0.0.206
- broadcast address: 10.0.0.207
- Has a maximum of 6 devices.
- A /29 subnet offers 6 usable IP addresses.
- network address: 10.0.0.208/29
- usable IP addresses: 10.0.0.209 -to 10.0.0.214
- broadcast address: 10.0.0.215
The distribution layer switches are the default gateways for our VLANs. We’ll need an IP address on both switches , for each VLAN. I’ll use the first IP address in each subnet:
- DSW1: 10.0.0.1/25
- DSW2: 10.0.0.2/25
- DSW1: 10.0.0.129/26
- DSW2: 10.0.0.130/26
- DSW1: 10.0.0.193/28
- DSW2: 10.0.0.194/28
- DSW1: 10.0.0.209/29
- DSW2: 10.0.0.210/29
I’ll use these IP addresses on the access layer switches:
- ASW1: 10.0.0.211/29
- ASW2: 10.0.0.212/29
Let’s configure all these IP addresses.
Loopback Addresses
We reserve IP addresses up to 10.0.127.x for VLANs, so that means we can continue with 10.0.128.x. We’ll use an IP address for each device:
- R1: 10.0.128.1/32
- CSW1: 10.0.128.2/32
- CSW2: 10.0.128.3/32
- DSW1: 10.0.128.4/32
- DSW2: 10.0.128.5/32
- ASW1: 10.0.128.6/32
- ASW2: 10.0.128.7/32
We’ll reserve some address space for future devices. We’ll use the range 10.0.128.x to 10.0.130.x for the loopback address.
Let’s configure these loopback interfaces:
Point-to-Point Links
On the point-to-point links, we only need two usable IP addresses. We’ll reserve the address space from 10.0.131.0 to 10.134.255 for this.
Within this range, we allocate /30 subnets for each link. The first /30 subnet would be 10.0.131.0/30, the next 10.0.131.4/30, and so on. This provides enough space for numerous /30 subnets, each of which can support a point-to-point link between switches.
Here are all the point-to-point links we need with possible subnets:
- DSW1: 10.0.131.1
- CSW1: 10.0.131.2
- DSW1: 10.0.131.5
- CSW2: 10.0.131.6
- DSW2: 10.0.131.9
- CSW1: 10.0.131.10
- DSW2: 10.0.131.13
- CSW2: 10.0.131.14
- CSW1: 10.0.131.17
- CSW2: 10.0.131.18
- CSW1: 10.0.131.21
- R1: 10.0.131.22
- CSW2: 10.0.131.25
- R1: 10.0.131.26
Let’s configure all of these IP addresses.
Distribution layer switches:
Core switches:
We can verify all IP addresses per device like this:
Before you continue, it might be wise to ping all IP addresses between devices to make sure you configured everything correctly:
This works. the first ping fails because of ARP .
We have the 2001:db8::/32 prefix, which is used in documentation. Perfect for a lab. Similar to IPv4 addressing, we need to think about the addresses we are going to use. We’ll use similar reservations as we used for the IPv4 addressing scheme.
For the VLANs, we’ll use /64 subnets:
- DSW1: 2001:DB8:0:10::1/64
- DSW2: 2001:DB8:0:10::2/64
- DSW1: 2001:DB8:0:20::1/64
- DSW2: 2001:DB8:0:20::2/64
- DSW1: 2001:DB8:0:30::1/64
- DSW2: 2001:DB8:0:30::2/64
- DSW1: 2001:DB8:0:40::1/64
- DSW2: 2001:DB8:0:40::2/64
- ASW1: 2001:DB8:0:40::6/64
- ASW2: 2001:DB8:0:40::7/64
Let’s configure these.
Loopback Interfaces
We’ll use the 2001:DB8:0:128:: up to 2001:DB8:0:131:: range for loopback interfaces. For example:
- R1: 2001:DB8:0:128::1/128
- CSW1: 2001:DB8:0:128::2/128
- CSW2: 2001:DB8:0:128::3/128
- DSW1: 2001:DB8:0:128::4/128
- DSW2: 2001:DB8:0:128::5/128
- ASW1: 2001:DB8:0:128::6/128
- ASW2: 2001:DB8:0:128::7/128
Let’s configure these addresses:
Point-to-Point links
For the point-to-point links, we can use /127 subnets. We don’t need to configure any addresses on the L3 interfaces between the distribution and core layer switches because IPv6 uses link-local addresses for router neighbor adjacencies. We do need to configure addresses on the link between R1 and the ISP. For example:
- R1: 2001:DB8:0:131::1E/127
- ISP: 2001:DB8:0:131::1F/127
We’ll configure this address on R1 and use ipv6 enable on all other required interfaces. Here’s R1:
We can verify our IPv6 addresses with this command:
It’s a good idea to test reachability between two devices. Let’s try a ping between two link-local addresses:
When you ping between link-local addresses, you need to specify the outgoing interface:
We configured all IPv4 and IPv6 addresses, so now we can continue with routing.
We’ll start with IPv4 routing.
Static Routing
To reach the network behind the ISP, we’ll configure a static default route on R1:
To verify that the default route works, we’ll send a ping from R1 to the ISP loopback:
For the internal networks, we’ll configure OSPF and advertise all of our networks:
We have a requirement that the distribution layer switches shouldn’t have any unnecessary neighbor adjacencies. To accomplish this, we need to make sure they don’t establish a neighbor adjacency on their SVI interfaces:
We use the passive-interface command for this. If you don’t do this, DSW1 and DSW2 establish neighbor adjacencies on all SVI interfaces.
We also advertise the default route from R1 into OSPF:
We now have all OSPF neighbor adjacencies. Let’s verify those:
We're Sorry, Full Content Access is for Members Only...
If you like to keep on reading, Become a Member Now! Here is why:
- Learn any CCNA, CCNP and CCIE R&S Topic . Explained As Simple As Possible.
- Try for Just $1 . The Best Dollar You’ve Ever Spent on Your Cisco Career!
- Full Access to our 786 Lessons . More Lessons Added Every Week!
- Content created by Rene Molenaar (CCIE #41726)
687 Sign Ups in the last 30 days
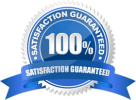
Forum Replies
2 of the interfaces coming from R1 are labeled Gi0/0.
Hello Andrew
Yes, thanks for pointing that out, I’ll let Rene know to make the fix.
Hello Markus
Looking over the lab it does seem that the loopbacks assigned to the ASW devices will not be reachable from the rest of the topology. As it stands, OSPF is not configured on the AWS devices. So either OSPF must be enabled, and the loopbacks advertised, or the DSWs must be configured with a static route to those addresses, and those static routes should then be redistributed into OSPF. I will let Rene know so he can make any changes he deems necessary. Thanks for pointing this out!
You are right. We need something on ASW1 and ASW2 to make these loopback interfaces reachable. To make this work, we need to run a routing protocol and establish an OSPF neighbor adjacency in VLAN 10, 20, or 30 to advertise the loopback.
I’m making a change to the lab. We’ll add one more VLAN named “MANAGEMENT” that we can use for the L2 switches. We’ll establish OSPF neighbor adjacencies within this VLAN so that we can advertise the ASW1+ASW2 loopback interfaces.
Instead of OSPF, you could also configure a default gateway on ASW1+ASW2 but in that ca
Hello Steven
You are indeed correct, strictly speaking, you don’t need to enable routing on the access switches. The configuration of the default gateway should be enough for the ASW switches to achieve reachability to the rest of the network. However
2 more replies! Ask a question or join the discussion by visiting our Community Forum
- Popular Professionals
- Design & Planning
- Construction & Renovation
- Finishes & Fixtures
- Landscaping & Outdoor
- Systems & Appliances
- Interior Designers & Decorators
- Architects & Building Designers
- Design-Build Firms
- Kitchen & Bathroom Designers
- General Contractors
- Kitchen & Bathroom Remodelers
- Home Builders
- Roofing & Gutters
- Cabinets & Cabinetry
- Tile & Stone
- Hardwood Flooring Dealers
- Landscape Contractors
- Landscape Architects & Landscape Designers
- Home Stagers
- Swimming Pool Builders
- Lighting Designers and Suppliers
- 3D Rendering
- Sustainable Design
- Basement Design
- Architectural Design
- Universal Design
- Energy-Efficient Homes
- Multigenerational Homes
- House Plans
- Home Remodeling
- Home Additions
- Green Building
- Garage Building
- New Home Construction
- Basement Remodeling
- Stair & Railing Contractors
- Cabinetry & Cabinet Makers
- Roofing & Gutter Contractors
- Window Contractors
- Exterior & Siding Contractors
- Carpet Contractors
- Carpet Installation
- Flooring Contractors
- Wood Floor Refinishing
- Tile Installation
- Custom Countertops
- Quartz Countertops
- Cabinet Refinishing
- Custom Bathroom Vanities
- Finish Carpentry
- Cabinet Repair
- Custom Windows
- Window Treatment Services
- Window Repair
- Fireplace Contractors
- Paint & Wall Covering Dealers
- Door Contractors
- Glass & Shower Door Contractors
- Landscape Construction
- Land Clearing
- Garden & Landscape Supplies
- Deck & Patio Builders
- Deck Repair
- Patio Design
- Stone, Pavers, & Concrete
- Paver Installation
- Driveway & Paving Contractors
- Driveway Repair
- Asphalt Paving
- Garage Door Repair
- Fence Contractors
- Fence Installation
- Gate Repair
- Pergola Construction
- Spa & Pool Maintenance
- Swimming Pool Contractors
- Hot Tub Installation
- HVAC Contractors
- Electricians
- Appliance Services
- Solar Energy Contractors
- Outdoor Lighting Installation
- Landscape Lighting Installation
- Outdoor Lighting & Audio/Visual Specialists
- Home Theater & Home Automation Services
- Handyman Services
- Closet Designers
- Professional Organizers
- Furniture & Accessories Retailers
- Furniture Repair & Upholstery Services
- Specialty Contractors
- Color Consulting
- Wine Cellar Designers & Builders
- Home Inspection
- Custom Artists
- Columbus, OH Painters
- New York City, NY Landscapers
- San Diego, CA Bathroom Remodelers
- Minneapolis, MN Architects
- Portland, OR Tile Installers
- Kansas City, MO Flooring Contractors
- Denver, CO Countertop Installers
- San Francisco, CA New Home Builders
- Rugs & Decor
- Home Improvement
- Kitchen & Tabletop
- Bathroom Vanities
- Bathroom Vanity Lighting
- Bathroom Mirrors
- Bathroom Fixtures
- Nightstands & Bedside Tables
- Kitchen & Dining
- Bar Stools & Counter Stools
- Dining Chairs
- Dining Tables
- Buffets and Sideboards
- Kitchen Fixtures
- Wall Mirrors
- Living Room
- Armchairs & Accent Chairs
- Coffee & Accent Tables
- Sofas & Sectionals
- Media Storage
- Patio & Outdoor Furniture
- Outdoor Lighting
- Ceiling Lighting
- Chandeliers
- Pendant Lighting
- Wall Sconces
- Desks & Hutches
- Office Chairs
- View All Products
- Designer Picks
- Side & End Tables
- Console Tables
- Living Room Sets
- Chaise Lounges
- Ottomans & Poufs
- Bedroom Furniture
- Nightstands
- Bedroom Sets
- Dining Room Sets
- Sideboards & Buffets
- File Cabinets
- Room Dividers
- Furniture Sale
- Trending in Furniture
- View All Furniture
- Bath Vanities
- Single Vanities
- Double Vanities
- Small Vanities
- Transitional Vanities
- Modern Vanities
- Houzz Curated Vanities
- Best Selling Vanities
- Bathroom Vanity Mirrors
- Medicine Cabinets
- Bathroom Faucets
- Bathroom Sinks
- Shower Doors
- Showerheads & Body Sprays
- Bathroom Accessories
- Bathroom Storage
- Trending in Bath
- View All Bath
- Houzz x Jennifer Kizzee
- Houzz x Motivo Home
- How to Choose a Bathroom Vanity
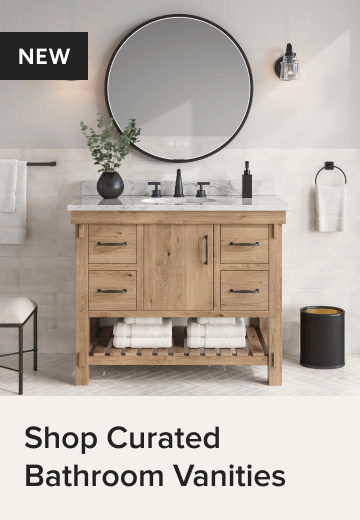
- Patio Furniture
- Outdoor Dining Furniture
- Outdoor Lounge Furniture
- Outdoor Chairs
- Adirondack Chairs
- Outdoor Bar Furniture
- Outdoor Benches
- Wall Lights & Sconces
- Outdoor Flush-Mounts
- Landscape Lighting
- Outdoor Flood & Spot Lights
- Outdoor Decor
- Outdoor Rugs
- Outdoor Cushions & Pillows
- Patio Umbrellas
- Lawn & Garden
- Garden Statues & Yard Art
- Planters & Pots
- Outdoor Sale
- Trending in Outdoor
- View All Outdoor
- 8 x 10 Rugs
- 9 x 12 Rugs
- Hall & Stair Runners
- Home Decor & Accents
- Pillows & Throws
- Decorative Storage
- Faux Florals
- Wall Panels
- Window Treatments
- Curtain Rods
- Blackout Curtains
- Blinds & Shades
- Rugs & Decor Sale
- Trending in Rugs & Decor
- View All Rugs & Decor
- Pendant Lights
- Flush-Mounts
- Ceiling Fans
- Track Lighting
- Wall Lighting
- Swing Arm Wall Lights
- Display Lighting
- Table Lamps
- Floor Lamps
- Lamp Shades
- Lighting Sale
- Trending in Lighting
- View All Lighting
- Bathroom Remodel
- Kitchen Remodel
- Kitchen Faucets
- Kitchen Sinks
- Major Kitchen Appliances
- Cabinet Hardware
- Backsplash Tile
- Mosaic Tile
- Wall & Floor Tile
- Accent, Trim & Border Tile
- Whole House Remodel
- Heating & Cooling
- Building Materials
- Front Doors
- Interior Doors
- Home Improvement Sale
- Trending in Home Improvement
- View All Home Improvement
- Cups & Glassware
- Kitchen & Table Linens
- Kitchen Storage and Org
- Kitchen Islands & Carts
- Food Containers & Canisters
- Pantry & Cabinet Organizers
- Kitchen Appliances
- Gas & Electric Ranges
- Range Hoods & Vents
- Beer & Wine Refrigerators
- Small Kitchen Appliances
- Cookware & Bakeware
- Tools & Gadgets
- Kitchen & Tabletop Sale
- Trending in Kitchen & Tabletop
- View All Kitchen & Tabletop
- Storage & Organization
- Baby & Kids
- Housekeeping & Laundry
- Pet Supplies
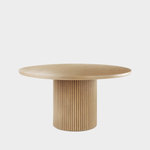
- View all photos
- Dining Room
- Breakfast Nook
- Family Room
- Bed & Bath
- Powder Room
- Storage & Closet
- Outdoor Kitchen
- Bar & Wine
- Wine Cellar
- Home Office
- Popular Design Ideas
- Kitchen Backsplash
- Deck Railing
- Privacy Fence
- Small Closet
- Stories and Guides
- Popular Stories
- Renovation Cost Guides
- Fence Installation Cost Guide
- Window Installation Cost Guide
- Discussions
- Design Dilemmas
- Before & After
- Houzz Research
- View all pros
- View all services
- View all products
- View all sales
- Living Room Chairs
- Dining Room Furniture
- Coffee Tables
- Home Office Furniture
- Join as a Pro
- Interior Design Software
- Project Management
- Custom Website
- Lead Generation
- Invoicing & Billing
- Landscape Contractor Software
- General Contractor Software
- Remodeler Software
- Builder Software
- Roofer Software
- Architect Software
- Takeoff Software
- Lumber & Framing Takeoffs
- Steel Takeoffs
- Concrete Takeoffs
- Drywall Takeoffs
- Insulation Takeoffs
- Stories & Guides
- LATEST FROM HOUZZ
- HOUZZ DISCUSSIONS
- SHOP KITCHEN & DINING
- Kitchen & Dining Furniture
- Sinks & Faucets
- Kitchen Cabinets & Storage
- Knobs & Pulls
- Kitchen Knives
- KITCHEN PHOTOS
- FIND KITCHEN PROS
- Bath Accessories
- Bath Linens
- BATH PHOTOS
- FIND BATH PROS
- SHOP BEDROOM
- Beds & Headboards
- Bedroom Decor
- Closet Storage
- Bedroom Vanities
- BEDROOM PHOTOS
- Kids' Room
- FIND DESIGN PROS
- SHOP LIVING
- Fireplaces & Accessories
- LIVING PHOTOS
- SHOP OUTDOOR
- Pool & Spa
- Backyard Play
- OUTDOOR PHOTOS
- FIND LANDSCAPING PROS
- SHOP LIGHTING
- Bathroom & Vanity
- Flush Mounts
- Kitchen & Cabinet
- Outdoor Wall Lights
- Outdoor Hanging Lights
- Kids' Lighting
- Decorative Accents
- Artificial Flowers & Plants
- Decorative Objects
- Screens & Room Dividers
- Wall Shelves
- About Houzz
- Houzz Credit Cards
- Privacy & Notice
- Cookie Policy
- Your Privacy Choices
- Mobile Apps
- Copyright & Trademark
- For Professionals
- Houzz vs. Houzz Pro
- Houzz Pro vs. Ivy
- Houzz Pro Advertising Reviews
- Houzz Pro 3D Floor Planner Reviews
- Trade Program
- Buttons & Badges
- Your Orders
- Shipping & Delivery
- Return Policy
- Houzz Canada
- Review Professionals
- Suggested Professionals
- Accessibility
- Houzz Support
- COUNTRY COUNTRY
New & Custom Home Builders in Elektrostal'
Location (1).
- Use My Current Location
Popular Locations
- Albuquerque
- Cedar Rapids
- Grand Rapids
- Indianapolis
- Jacksonville
- Kansas City
- Little Rock
- Los Angeles
- Minneapolis
- New Orleans
- Oklahoma City
- Orange County
- Philadelphia
- Portland Maine
- Salt Lake City
- San Francisco
- San Luis Obispo
- Santa Barbara
- Washington D.C.
- Elektrostal', Moscow Oblast, Russia
Professional Category (1)
- Accessory Dwelling Units (ADU)
Featured Reviews for New & Custom Home Builders in Elektrostal'
- Reach out to the pro(s) you want, then share your vision to get the ball rolling.
- Request and compare quotes, then hire the Home Builder that perfectly fits your project and budget limits.
Before choosing a Builder for your residential home project in Elektrostal', there are a few important steps to take:
- Define your project: Outline your desired home type, features, and layout. Provide specific details and preferences to help the builder understand your vision.
- Establish a budget: Develop a comprehensive budget, including construction expenses and material costs. Communicate your budgetary constraints to the builder from the beginning.
- Timeline: Share your estimated timeline or desired completion date.
- Site conditions: Inform the builder about any unique site conditions or challenges.
- Local regulations: Make the builder aware of any building regulations or permits required.
- Land Surveying
What do new home building contractors do?
Questions to ask a prospective custom home builder in elektrostal', moscow oblast, russia:.
If you search for Home Builders near me you'll be sure to find a business that knows about modern design concepts and innovative technologies to meet the evolving needs of homeowners. With their expertise, Home Builders ensure that renovation projects align with clients' preferences and aspirations, delivering personalized and contemporary living spaces.
BUSINESS SERVICES
Connect with us.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
computer-networking-lab-assignments
Here is 1 public repository matching this topic..., iamrohitsuthar / cnl.
SPPU COMP Codes of CNL
- Updated Oct 15, 2019
Improve this page
Add a description, image, and links to the computer-networking-lab-assignments topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the computer-networking-lab-assignments topic, visit your repo's landing page and select "manage topics."
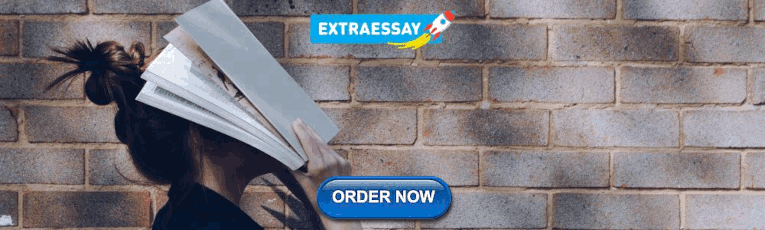
IMAGES
VIDEO
COMMENTS
Introduction. In this lab assignment, you will write a simple router with a static routing table. We will pass to your code raw Ethernet frames and give you a function that can send a raw Ethernet frame. It is up to you to implement the forwarding logic between getting a frame and sending it out over another interface. Figure 1: sample topology.
imsiddhant / Computer-Networking-Project-1. PROJECT 1: With this Project/Assignment, I designed a sample network for an Enterprise. This Enterprise has 2 Locations (Location A & Location B). It is a of Secure Campus Area Network where in Location B, there is a Main Server with IP of 172.18.51.1.
Please don't post source code to lab solutions. Archived lecture videos: Canvas Panopto Course Videos: Optional course texts: Kaashoek & Saltzer, "Principles of Computer System Design," Chapters 7-8 (Networks & Fault Tolerance) Kurose & Ross, "Computer Networking: A Top-Down Approach" Peterson & Davie, "Computer Networks: A Systems Approach"
Computer Networks Lab Manual. Search Ctrl + K. COURSE INFORMATION. Welcome. Lab Session and Etiquette. Submission Requirements. Team Setup. Automated Testing. Assignments. ... The table below gives an overview of all Lab assignments and their associated rewards. Assignment Mandatory Reward [points] Chat Client. 100. TCP Trace Analysis. 100 ...
Therefore, you can be sure that each program will surely run and perform the task it is supposed to do. Although, you must be aware that there might be some corner cases which might get left out. If you just want the codes you can go to each assignment's folder and get it. If you are doing this while your lab is going on / you're studying for ...
Welcome to CS144: Introduction to Computer Networking. In this warmup lab, you will set up an installation of Linux on your computer, learn how to perform some tasks over the Internet by hand, write a small program in C++ that fetches a Web page over the Internet, ... lab assignments: • It's a good idea to skim the whole lab before diving in!
Introduction to Computer Networks Fall 2013. ... Most of the assignments will be done in the context of the Open Network Lab a network testbed that allows you to define your own private network configuration and run applications within it. You will be working with multiple computers at the same time, as well as network components such as ...
All assignments are on the web page Text: Kurose & Ross, Computer Networking: A Top-Down Approach, 4th or 5th edition-Instructors working from 4th edition, either OK-Don't need lab manual or Ethereal (used book OK) Syllabus on web page-Gives which textbook chapters correspond to lectures (Lectures and book topics will mostly overlap)
Programming/Lab Assignments: 40% (~8-10 labs, some labs may be worth more than others) Homework: 10% (~10 homeworks) Class and Lab Participation: 5% Lab Policy All assignments are due at the time and date specified on the lab write-up. Lab submissions will be done electronically, and I will provide more instruction later.
Modules 1 - 3: Basic Network Connectivity and Communications Exam Answers. Test. Modules 4 - 7: Ethernet Concepts Exam Answers. Test. Modules 8 - 10: Communicating Between Networks Exam Answers. Test. Modules 11 - 13: IP Addressing Exam Answers. Test. Modules 14 - 15: Network Application Communications Exam Answers.
These were the Computer Networks Assignments given in my sixth semester at IIT Guwahati. The topics covered in the assignments are as follows: Assignment 01: Exploring the various network diagnostic tools, an end user makes use of these tools to discover how a machine is connected to the network and how the network looks like beyond the first hop.
CSE 473S Lab Assignment 1. Due date: TBA. Goals. To learn the basic infrastructure computer networks. of layered architecture and service primitives in. To design a simplified datalink layer. To get familiar with the simulator environment used for this and the next lab. Layered architecture. For the purpose of this lab, assume that each node in ...
through this computer's Internet connectioncheck box. 9. If you are sharing a dial-up Internet connection, select the Establish a dial-up connection whenever a computer on my network attempts to access the Internet check box if you want to permit your computer to automatically connect to the Internet. 10. Click OK. You receive the following ...
The primary assignments for the course will be lab-based programming assignments. We will also release weekly problem set exercises whose primary purpose is to help in preparation for the midterm and final exam; these problem sets may be graded at random. ... Computer Networking: A Top-Down Approach (6th edition), by Jim Kurose and Keith Ross ...
Network Fundamentals Lab 1. OSPFv2. Congratulations! You've been selected as the chief network engineer for NovaTech Pioneers, an emerging leader in the realm of digital innovation. With the company poised for unprecedented growth, a brand-new campus is on the horizon to house its expanding operations and ambitious projects.
hours to complete (future labs will take more of your time). Three quick points about the lab assignment: • It's a good idea to read the whole document before diving in! • Over the course of this 8-part lab assignment, you'll be building up your own imple-mentation of a significant portion of the Internet—a router, a network interface ...
Solutions to assignments of Stanford CS144, Introduction to Computer Networking, 2020 Fall. - wine99/cs144-20fa. Solutions to assignments of Stanford CS144, Introduction to Computer Networking, 2020 Fall. - wine99/cs144-20fa. Skip to content. Navigation Menu Toggle navigation. ... CS144 Lab Assignments - 手写TCP - LAB0. CS 144: ...
Before you hire a custom home builder in Elektrostal', Moscow Oblast, browse through our network of over 1,121 local custom home builders. Read through customer reviews, check out their past projects and then request a quote from the best custom home builders near you. Finding custom home builders in my area is easy on Houzz.
Find company research, competitor information, contact details & financial data for AVANGARD, OOO of Elektrostal, Moscow region. Get the latest business insights from Dun & Bradstreet.
Collection of assignments for CS252: Computer Networks Lab course at IIT Bombay. Team members: Yash Shah , Rupesh (@dungeon-masterRupesh) NOTE: Individual assignments are not added. About. Course assignments for CS252: Computer Networks Lab at IIT Bombay Resources. Readme Activity. Stars. 1 star Watchers. 1 watching
Find detailed information on Computer and Peripheral Equipment Manufacturing companies in Elektrostal, Russian Federation, including financial statements, sales and marketing contacts, top competitors, and firmographic insights. Dun & Bradstreet gathers Computer and Peripheral Equipment Manufacturing business information from trusted sources to ...
Add a description, image, and links to the computer-networking-lab-assignments topic page so that developers can more easily learn about it. Curate this topic Add this topic to your repo To associate your repository with the ...