Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
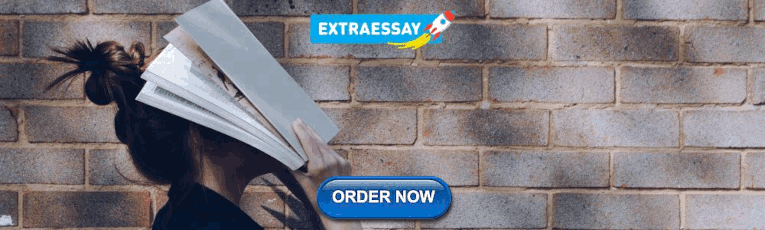
Related Pages

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
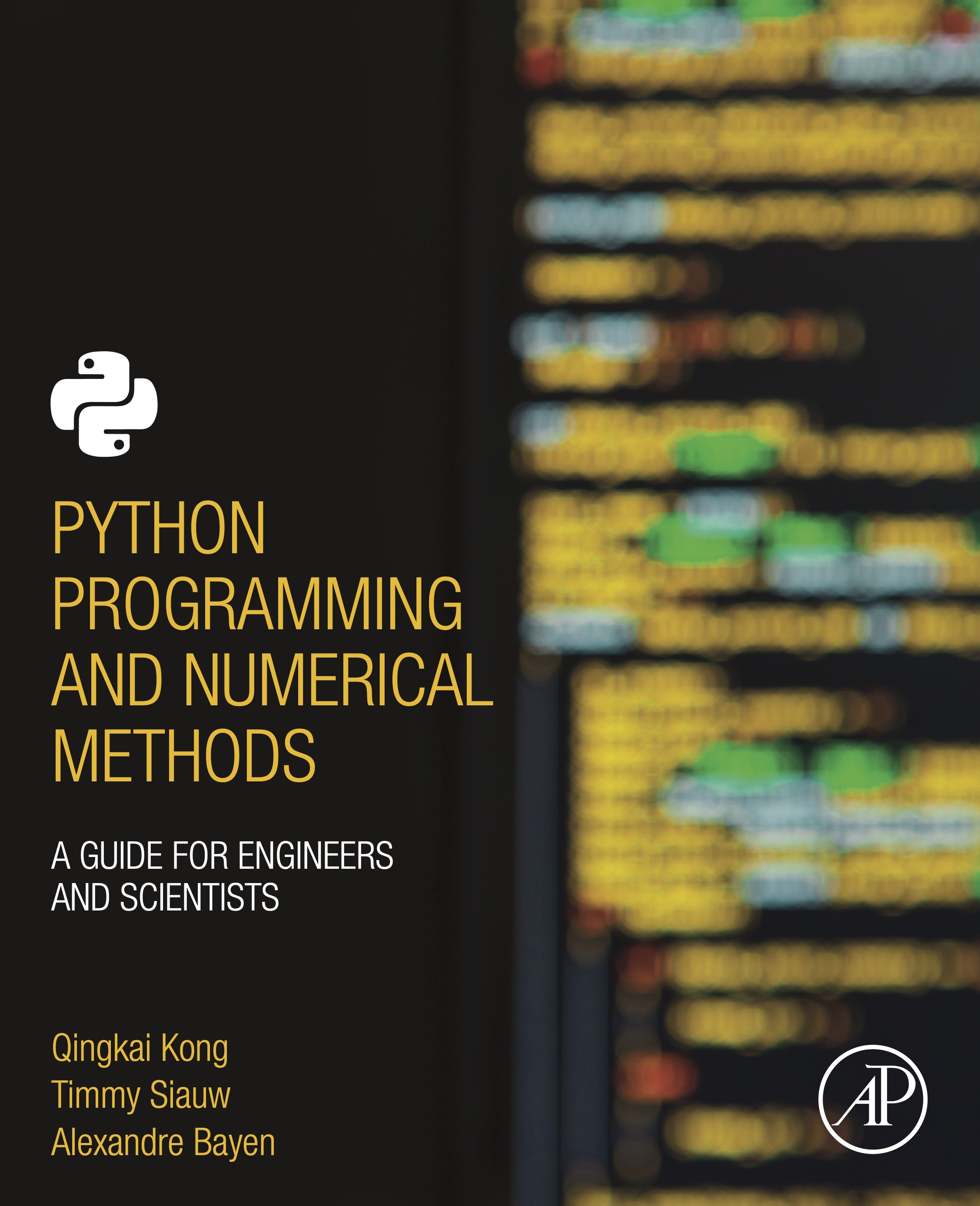
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
- Python »
- 3.12.3 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |
7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment expression like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
For expressions as assignment targets, the annotations are evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
New in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python Tokens and Character Sets
- Fraud Prevention and Privacy Laws
- What is Online Privacy and how is it affected?
- What is Plagiarism? Definition, Types, How to Avoid, Laws
- Effects of Child Pornography
- What is Digital Rights Management? Definition, Working, Benefits
- Expressions in Python
- How to Browse the Internet Safely?
- What is Internet Trolling and How to deal with it?
- What is Decomposition Computational Thinking?
- What is the Difference between Interactive and Script Mode in Python Programming?
- What is Phishing?
- Open Source and Open Data
- What is E-Waste?
- What is Licensing?
- Cloud Deployment Models
- What is Cyber Bullying? Definition, Types, Effects, Laws
- Cyber Forensics
- What is a Scam?
Augmented Assignment Operators in Python
An assignment operator is an operator that is used to assign some value to a variable. Like normally in Python, we write “ a = 5 “ to assign value 5 to variable ‘a’. Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. So assume if we need to add 7 to a variable “a” and assign the result back to “a”, then instead of writing normally as “ a = a + 7 “, we can use the augmented assignment operator and write the expression as “ a += 7 “. Here += has combined the functionality of arithmetic addition and assignment.
So, augmented assignment operators provide a short way to perform a binary operation and assigning results back to one of the operands. The way to write an augmented operator is just to write that binary operator and assignment operator together. In Python, we have several different augmented assignment operators like +=, -=, *=, /=, //=, **=, |=, &=, >>=, <<=, %= and ^=. Let’s see their functioning with the help of some exemplar codes:
1. Addition and Assignment (+=): This operator combines the impact of arithmetic addition and assignment. Here,
a = a + b can be written as a += b
2. Subtraction and Assignment (-=): This operator combines the impact of subtraction and assignment.
a = a – b can be written as a -= b
Example:
3. Multiplication and Assignment (*=): This operator combines the functionality of multiplication and assignment.
a = a * b can be written as a *= b
4. Division and Assignment (/=): This operator has the combined functionality of division and assignment.
a = a / b can be written as a /= b
5. Floor Division and Assignment (//=): It performs the functioning of floor division and assignment.
a = a // b can be written as a //= b
6. Modulo and Assignment (%=): This operator combines the impact of the modulo operator and assignment.
a = a % b can be written as a %= b
7. Power and Assignment (**=): This operator is equivalent to the power and assignment operator together.
a = a**b can be written as a **= b
8. Bitwise AND & Assignment (&=): This operator combines the impact of the bitwise AND operator and assignment operator.
a = a & b can be written as a &= b
9. Bitwise OR and Assignment (|=): This operator combines the impact of Bitwise OR and assignment operator.
a = a | b can be written as a |= b
10. Bitwise XOR and Assignment (^=): This augmented assignment operator combines the functionality of the bitwise XOR operator and assignment operator.
a = a ^ b can be written as a ^= b
11. Bitwise Left Shift and Assignment (<<=): It puts together the functioning of the bitwise left shift operator and assignment operator.
a = a << b can be written as a <<= b
12. Bitwise Right Shift and Assignment (>>=): It puts together the functioning of the bitwise right shift operator and assignment operator.
a = a >> b can be written as a >>= b
Please Login to comment...
Similar reads.
- School Learning
- School Programming
- 10 Best Slack Integrations to Enhance Your Team's Productivity
- 10 Best Zendesk Alternatives and Competitors
- 10 Best Trello Power-Ups for Maximizing Project Management
- Google Rolls Out Gemini In Android Studio For Coding Assistance
- 30 OOPs Interview Questions and Answers (2024)
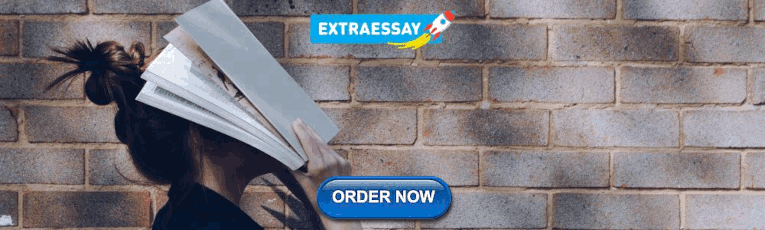
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
A Comprehensive Guide to Augmented Assignment Operators in Python
Augmented assignment operators are a vital part of the Python programming language. These operators provide a shortcut for assigning the result of an operation back to a variable in an expressive and efficient manner.
In this comprehensive guide, we will cover the following topics related to augmented assignment operators in Python:
Table of Contents
What are augmented assignment operators, arithmetic augmented assignments, bitwise augmented assignments, sequence augmented assignments, advantages of augmented assignment operators, augmented assignment with mutable types, operator precedence and order of evaluation, updating multiple references, augmented assignment vs normal assignment, comparisons to other languages, best practices and style guide.
Augmented assignment operators are a shorthand technique that combines an arithmetic or bitwise operation with an assignment.
The augmented assignment operator performs the operation on the current value of the variable and assigns the result back to the same variable in a compact syntax.
For example:
Here x += 3 is equivalent to x = x + 3 . The += augmented assignment operator adds the right operand 3 to the current value of x , which is 2 . It then assigns the result 5 back to x .
This shorthand allows you to reduce multiple lines of code into a concise single line expression.
Some key properties of augmented assignment operators in Python:
- Operators act inplace directly modifying the variable’s value
- Work on mutable types like lists, sets, dicts unlike normal operators
- Generally have equivalent compound statement forms using standard operators
- Have right-associative evaluation order unlike arithmetic operators
- Available for arithmetic, bitwise, and sequence operations
Now let’s look at the various augmented assignment operators available in Python.
Augmented Assignment Operators List
Python supports augmented versions of all the arithmetic, bitwise, and sequence assignment operators.
These perform the standard arithmetic operations like addition or exponentiation and assign the result back to the variable.
These allow you to perform bitwise AND, OR, XOR, right shift, and left shift operations combined with assignment.
The += operator can also be used to concatenate sequences like lists, tuples, and strings.
These operators provide a shorthand for sequence concatenation.
Some key advantages of using augmented assignment operators:
Conciseness : Performs an operation and assignment in one concise expression rather than multiple lines or steps.
Readability : The operator itself makes the code’s intention very clear. x += 3 is more readable than x = x + 3 .
Efficiency : Saves executing multiple operations and creates less intermediate objects compared to chaining or sequencing the operations. The variable is modified in-place.
For these reasons, augmented assignments should be preferred over explicit expansion into longer compound statements in most cases.
Some examples of effective usage:
- Incrementing/decrementing variables: index += 1
- Accumulating sums: total += price
- Appending to sequences: names += ["Sarah", "John"]
- Bit masking tasks: bits |= 0b100
So whenever you need to assign the result of some operation back into a variable, consider using the augmented version.
Common Mistakes to Avoid
While augmented assignment operators are very handy, some common mistakes can occur:
Augmented assignments act inplace and modify the existing object. This can be problematic with mutable types like lists:
In contrast, normal operators with immutable types create a new object:
So be careful when using augmented assignments with mutable types, as they modify the object in-place rather than creating a new object.
Augmented assignment operators have right-associativity. This can cause unexpected results:
The right-most operation y += 1 is evaluated first updating y. Then x += y uses the new value of y.
To avoid this, use parenthesis to control order of evaluation:
When you augmented assign to a variable, it updates all references to that object:
y also reflects the change since it points to the same mutable list as x .
To avoid this, reassign the variable rather than using augmented assignment:
While the augmented assignment operators provide a shorthand, they differ from standard assignment in some key ways:
Inplace modification : Augmented assignment acts inplace and modifies the existing variable rather than creating a new object.
Mutable types : Works directly on mutable types like lists, sets, and dicts unlike normal assignment.
Order of evaluation : Has right-associativity unlike left-associativity of normal assignment.
Multiple references : Affects all references to a mutable object unlike normal assignment.
In summary, augmented assignment operators combine both an operation and assignment but evaluate differently than standard operators.
Augmented assignments exist in many other languages like C/C++, Java, JavaScript, Go, Rust, etc. Some key differences to Python:
In C/C++ augmented assignments return the assigned value allowing usage in expressions unlike Python which returns None .
Java and JavaScript don’t allow augmented assignment with strings unlike Python which supports += for concatenation.
Go doesn’t have an increment/decrement operator like ++ and -- . Python’s += 1 and -= 1 serves a similar purpose.
Rust doesn’t allow built-in types like integers to be reassigned with augmented assignment and requires mutable variables be defined with mut .
So while augmented assignment is common across languages, Python provides some unique behaviors to be aware of.
Here are some best practices when using augmented assignments in Python:
Use whitespace around the operators: x += 1 rather than x+=1 for readability.
Limit chaining augmented assignments like x = y = 0 . Use temporary variables if needed for clarity.
Don’t overuse augmented assignment especially with mutable types. Reassignment may be better if the original object shouldn’t be changed.
Watch the order of evaluation with multiple augmented assignments on one line due to right-associativity.
Consider parentheses for explicit order of evaluation: x += (y + z) rather than relying on precedence.
For increments/decrements, prefer += 1 and -= 1 rather than x = x + 1 and x = x - 1 .
Use normal assignment for updating multiple references to avoid accidental mutation.
Following PEP 8 style, augmented assignments should have the same spacing and syntax as normal assignment operators. Just be mindful of potential pitfalls.
Augmented assignment operators provide a compact yet expressive shorthand for modifying variables in Python. They combine an operation and assignment into one atomic expression.
Key takeaways:
Augmented operators perform inplace modification and behave differently than standard operators in some cases.
Know the full list of arithmetic, bitwise, and sequence augmented assignment operators.
Use augmented assignment to write concise and efficient updates to variables and sequences.
Be mindful of right-associativity order of evaluation and behavior with mutable types to avoid bugs.
I hope this guide gives you a comprehensive understanding of augmented assignment in Python. Use these operators appropriately to write clean, idiomatic Python code.
- python
- variables-and-operators
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar

PythonForBeginners.com
Learn By Example
List of Lists in Python
Author: Aditya Raj Last Updated: March 25, 2022
Lists are used in python to store data when we need to access them sequentially. In this article, we will discuss how we can create a list of lists in python. We will also implement programs to perform various operations like sorting, traversing, and reversing a list of lists in python.
What Is a List of Lists in Python?
List of lists using the append() method in python, create list of lists using list comprehension in python, access elements in a list of lists in python, traverse a list of lists in python, delete an element from a list of lists using the pop() method, remove an element from a list of lists using the remove() method, flatten list of lists in python, reverse the order of inner list in list of lists in python, reversing the order of elements of inner list in list of lists in python, sort list of lists in python using the sort() method, sorting list of lists in python using the sorted() function, concatenate two lists of lists in python, shallow copy list of lists in python, deep copy list of lists in python.
A list of lists in python is a list that contains lists as its elements. Following is an example of a list of lists.
Here, myList contains five lists as its elements. Hence, it is a list of lists.
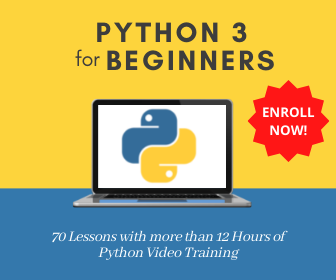
Create a List of Lists in Python
To create a list of lists in python, you can use the square brackets to store all the inner lists. For instance, if you have 5 lists and you want to create a list of lists from the given lists, you can put them in square brackets as shown in the following python code.
In the above example, you can observe that we have created a list of lists using the given lists.
We can also create a list of lists using the append() method in python. The append() method, when invoked on a list, takes an object as input and appends it to the end of the list. To create a list of lists using the append() method, we will first create a new empty list. For this, you can either use the square bracket notation or the list() constructor. The list() constructor, when executed without input arguments, returns an empty list.
After creating the empty list, we can append all the given lists to the created list using the append() method to create a list of lists in python as shown in the following code snippet.
If you want to create a list of lists like a 2-d array using only integer data types, you can use nested for loops with the append() method to create a list of lists.
In this approach, we will first create a new list, say myList . After that, we will use nested for loop to append other lists to myList . In the outer for loop of the nested loop, we will create another empty list, say tempList . In the inner for loop, we will append the numbers to tempList using the append() method.
After appending the numbers to tempList , we will get a list of integers. After that, we will come to the outer for loop and will append tempList to myList . In this way, we can create a list of lists.
For instance, suppose that we have to create a 3×3 array of numbers. For this, we will use the range() function and the for loop to create a list of lists in python as follows.
Instead of using the for loop, you can use list comprehension with the range() function to create a list of lists in a concise way as shown in the following example.
We can access the contents of a list using the list index. In a flat list or 1-d list, we can directly access the list elements using the index of the elements. For instance, if we want to use positive values as the index for the list elements, we can access the 1st item of the list using index 0 as shown below.
Similarly, if we use the negative values as the list indices, we can access the last element of the list using the index -1 as shown below.
If you want to access the inner lists from a list of lists, you can use list indices in a similar way as shown in the above example.
If you are using positive numbers as list indices, you can access the first inner list from the list of lists using index 0 as shown below.
Similarly, if you are using negative numbers as list indices, you can access the last inner list from the list of lists as shown below.
To access the elements of the inner lists, you need to use double square brackets after the list name. Here, the first square bracket denotes the index of the inner list, and the second square bracket denotes the index of the element in the inner list.
For example, you can access the third element of the second list from the list of lists using square brackets as shown below.
To traverse the elements of a list of lists, we can use a for a loop. To print the inner lists, we can simply iterate through the list of lists as shown below.
Instead of printing the whole list while traversing the list of lists, we can also print the elements of the list. For this, we will use another for loop in addition to the for loop shown in the previous example. In the inner for loop, we will iterate through the inner lists and print their elements as shown below.
Delete an Element From a List of Lists in Python
To delete an inner list from a list of lists, we can use different methods of list-objects.
We can use the pop() method to delete the last item from the list of lists. The pop() method, when invoked on the list of lists, deletes the last element and returns the list present at the last position. We can understand this using a simple example shown below.
To delete any other inner list, we will need to know its index. For instance, we can delete the second element of the list of lists using the pop() method. For this, we will invoke the pop() method on the list and will pass the index of the second list i.e. 1 to the pop() method. After execution, the pop() method will delete the second inner list from the list of lists and will return it as shown in the following example.
If we know the element that has to be deleted, we can also use the remove() method to delete an inner list. The remove() method, when invoked on a list, takes the element to be deleted as its input argument. After execution, it deletes the first occurrence of the element passed as the input argument. To delete any inner list, we can use the remove() method as shown below.
Sometimes, we need to flatten a list of lists to create a 1-d list. To flatten the list of lists, we can use a for loop and the append() method. In this approach, we will first create an empty list say outputList .
After creating outputList , we will use a nested for loop to traverse the list of lists. In the outer for loop, we will select an inner list. After that, we will traverse the elements of the inner list in the inner for loop. In the inner for loop, we will invoke the append() method on outputList and will pass the elements of the inner for loop as an input argument to the append() method.
After execution of the for loops, we will get a flat list created from the list of lists as shown in the following code.
Instead of using the for loop, you can also use list comprehension to flatten a list of lists as shown below.
Reverse List of Lists in Python
We can reverse a list of lists in two ways. One approach is to reverse the order of the inner lists only and leave the order of the elements in the inner lists intact. Another approach is to reverse the order of the elements in the inner lists too.
To simplify reverse the order of the inner lists, we will first create an empty list, say outputList . After that, we will traverse through the list of lists in reverse order. While traversal, we will append the inner lists to outputList . In this way, we will get the reversed list of lists outputList after the execution of the for loop. You can observe this in the following example.
Instead of using the for loop, you can use the reverse() method to reverse the list of lists. The reverse() method, when invoked on a list, reverses the order of the elements in the list. When we will invoke the reverse() method on the list of lists, it will reverse the order of the inner lists as shown in the following example.
In the above approach, the original list is modified. However, that wasn’t the situation in the previous example. Thus, you can choose an approach depending on whether you have to modify the original list or not.
In addition to reversing the order of the inner lists, you can also reverse the order of the elements in the inner lists. For this, we will first create an empty list, say outputList . After that, we will traverse through the list of lists in reverse order using a for a loop. Inside the for loop, we will create an empty list, say tempList . After that, we will traverse through the elements of the inner list in reverse order using another for loop. While traversing the elements of the inner list, we will append the elements to the tempList . Outside the inner loop, we will append the tempList to outputList .
After execution of the for loops, we will get a list with all the elements in reverse order as shown in the following code.
Instead of using the for loop to reverse the elements of the inner lists, you can use the reverse() method as shown below.
Here, we have first reversed the inner list inside the for loop. After that, we have appended it to outputList . In this way, we have obtained the list of lists in which the inner lists, as well as the elements of the inner lists, are present in the reverse order as compared to the original list.
Sort List of Lists in Python
To sort a list of lists in python, we can use the sort() method or the sorted function.
The sort() method, when invoked on a list, sorts the elements of the list in increasing order. When we invoke the sort() method on a list of lists, it sorts the inner lists according to the first element of the inner lists.
In other words, the inner list whose first element is smallest among the first element of all the inner lists is assigned the first position in the list of lists. Similarly, the inner list whose first element is largest among the first element of all the inner lists is assigned the last position.
Also, if two inner lists have the same element at the first position, their position is decided on the basis of the second element. If the second element of the inner lists is also the same, the position of the lists will be decided on the basis of the third element, and so on. You can observe this in the following example.
You can also change the behavior of the sort() method. For this, you can use the ‘ key ’ parameter of the sort() method. The ‘ key ’ method takes an operator or a function as an input argument. For example, if you want to sort the list of lists according to the third element of the inner lists, you can pass an operator that uses the third element of the inner list as follows.
If you want to sort the list of lists according to the last elements of the inner lists, you can do it as follows.
Similarly, if you want to sort the list of lists according to the length of the inner lists, you can pass the len() function to the parameter ‘ key ’ of the sort() method. After execution, the sort() method will sort the list of lists using the length of the inner lists. You can observe this in the following example.
If you are not allowed to modify the original list of lists, you can use the sorted() function to sort a list of lists. The sorted() function works in a similar manner to the sort() method. However, instead of sorting the original list, it returns a sorted list.
To sort a list of lists, you can pass the list to the sorted() function. After execution, the sorted() function will return the sorted list as shown in the following example.
You can also use the key parameter to sort the list of lists using the sorted() function. For instance, you can sort the list of lists according to the third element of the inner lists using the sorted() function as shown below.
Similarly, if you want to sort the list of lists according to the length of the inner lists, you can pass the len() function to the parameter ‘ key ’ of the sorted() function. After execution, the sorted() function will return the sorted list of lists using the length of the inner lists. You can observe this in the following example.
If you are given two lists of lists and you want to concatenate the list of lists, you can do it using the + operator as shown below.
Here, the inner elements of both lists are concatenated into a single list of lists.
Copy List of Lists in Python
To copy a list of lists in python, we can use the copy() and the deepcopy() method provided in the copy module.
The copy() method takes a nested list as an input argument. After execution, it returns a list of lists similar to the original list. You can observe this in the following example.
The operation discussed in the above example is called shallow copy. Here, the inner elements in the copied list and the original list point to the same memory location. Thus, whenever we make a change in the copied list, it is reflected in the original list. Similarly, if we make a change in the original list, it is reflected in the copied list. To avoid this, you can use the deepcopy() method.
The deepcopy() method takes a nested list as its input argument. After execution, it creates a copy of all the elements of the nested list at a different location and then returns the copied list. Thus, whenever we make a change in the copied list, it is not reflected in the original list. Similarly, if we make a change in the original list, it is not reflected in the copied list. You can observe this in the following example.
In this article, we have discussed the list of lists in python. We have discussed how we can perform various operations on a list of lists. We have also discussed how shallow copy and deep copy work with a list of lists. Additionally, we have discussed how to sort, reverse, flatten, and traverse a list of lists in python, To know more about the python programming language, you can read this article on dictionary comprehension in python . You might also like this article on file handling in python .
Recommended Python Training
Course: Python 3 For Beginners
Over 15 hours of video content with guided instruction for beginners. Learn how to create real world applications and master the basics.
More Python Topics
Python Lab Assignments
You can not learn a programming language by only reading the language construct. It also requires programming - writing your own code and studying those of others. Solve these assignments, then study the solutions presented here.

Python Tutorial
Python oops, python mysql, python mongodb, python sqlite, python questions, python tkinter (gui), python web blocker, related tutorials, python programs.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Introduction
- Get started
- Import external data
- Create plots and charts
- Open-source Python libraries
- Keyboard shortcuts
- Troubleshoot errors
- Availability
- Data security
- PY function
Use Power Query to import data for Python in Excel
Python in Excel is currently in preview and is subject to change based on feedback. To use this feature, join the Microsoft 365 Insider Program and choose the Beta Channel Insider level.
Python in Excel is gradually rolling out to Excel for Windows customers using the Beta Channel. At this time, the feature is not available on other platforms.
If you encounter any issues with Python in Excel, please report them by selecting Help > Feedback in Excel.
New to Python in Excel? Start with Introduction to Python in Excel and Get started with Python in Excel
What is Power Query?
Power Query is a data transformation and preparation tool designed to help you shape your data, and it’s available in Excel and other Microsoft products.
Use Power Query with Python in Excel to import external data into Excel and then analyze that data with Python.
Important: Power Query is the only way to import external data for use with Python in Excel.
To learn more about Power Query, see Power Query for Excel Help .
How to use Power Query to import data for Python in Excel
Take the following steps to learn how to import a sample external data set and to use with Python in Excel.
Tip: If you’re already familiar with Power Query and want to work with existing queries in your workbook, skip to Use Power Query data with Python in Excel later in this article.
To see the Get & Transform Data and Queries & Connections groups, select the Data tab. Choose Get Data to select your data source. You can import data from multiple sources, including comma-separated values (CSV) files. Every import creates a query. This example imports data from the Northwind OData service , a test data source. To do this, select Get Data > From Other Sources > From OData Feed .
Next, enter the following link to the Northwind OData service and select OK.
https://services.odata.org/northwind/northwind.svc/

You can also select Transform Data from the Power Query preview dialog. This allows you to edit the data in the Power Query Editor before importing it into Excel.
If you want to view the data on the Excel grid, select the Load button. This will load the selected table directly to the Excel grid.
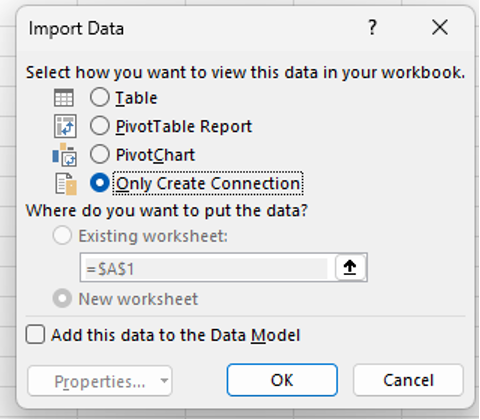
The Queries & Connections pane opens automatically, listing the query created by Power Query for the selected data source—in this case, the Categories table from the Northwind OData sample dataset. To manually open the Queries & Connections pane, on the Data tab select Queries & Connections .
Tip: Hover over the queries in the task pane to get a preview of the data.
The next section describes how to analyze the Categories data with Python in Excel.
Use Power Query data with Python in Excel
The following procedure assumes that you’ve created a Power Query connection with the Categories data from the Northwind OData service described in the preceding article section. These steps show how to analyze the Categories data with Python in Excel.
To work with external data with Python in Excel, enable Python in a cell by entering the =PY function. Next, use the Python in Excel xl() formula to reference Excel elements, like a Power Query query. For this example, enter xl("Categories") into the Python in Excel cell.
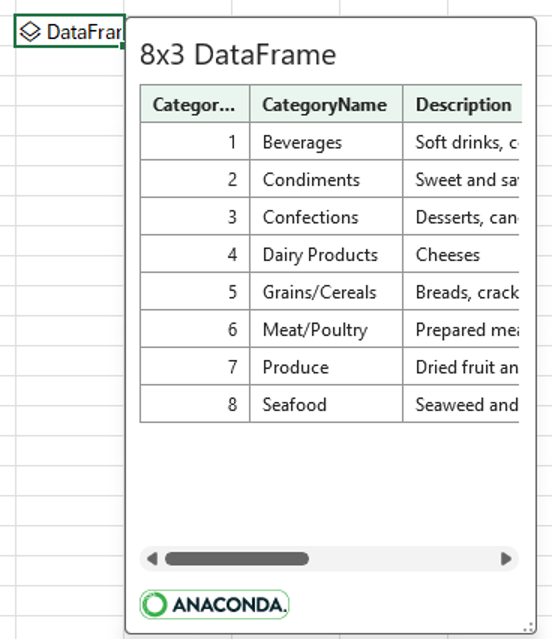
Tip: Resize the DataFrame dialog using the icon in the bottom right corner.
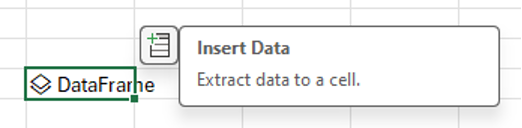
Now that you’ve used Power Query to import external data and processed that data with Python in Excel, you’re ready to start analyzing data with Python in Excel. To learn how to create Python plots and charts with your data, see Create Python in Excel plots and charts .
Related articles
About Power Query in Excel
Create Python in Excel plots and charts

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
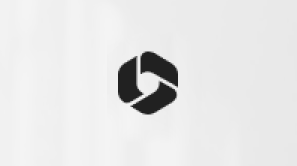
Microsoft 365 subscription benefits
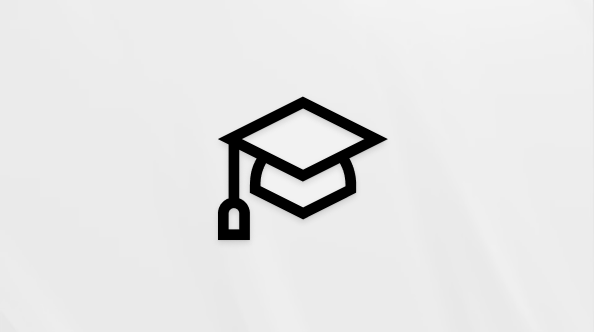
Microsoft 365 training
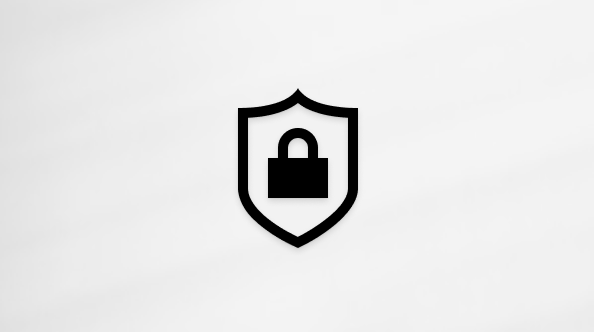
Microsoft security
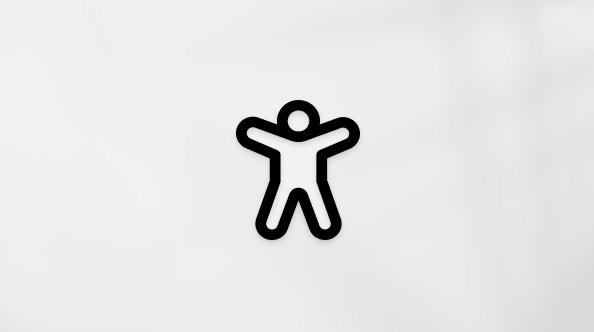
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
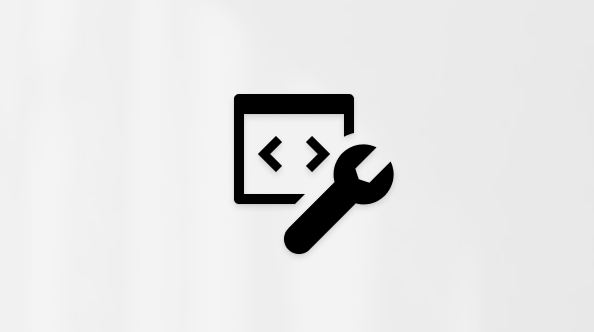
Microsoft Tech Community
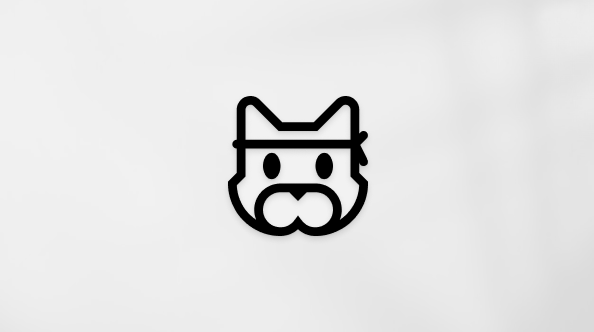
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
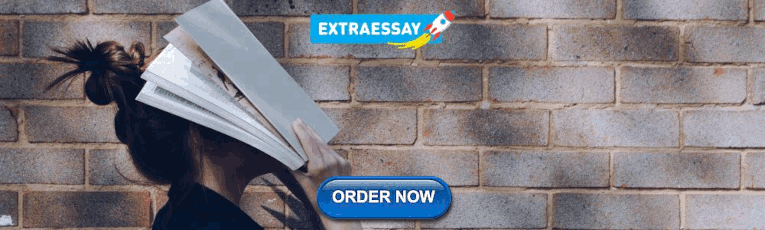
IMAGES
VIDEO
COMMENTS
Implicit Assignments in Python. Python implicitly runs assignments in many different contexts. In most cases, these implicit assignments are part of the language syntax. In other cases, they support specific behaviors. Whenever you complete an action in the following list, Python runs an implicit assignment for you: Define or call a function
good question! the answer is simple: my 3 lists in one list were only an example. originally, i wanted to put 15 lists into a list. of course, your way is working, but it looks somewhat weird if i implement it that way. i just wanted to learn if there is an advanced way of doing this. but thanks ;)
Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms :-. 1. Basic form: Unmute.
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
Assignment Operators in Python. Python assignment operators play a crucial role by allocating a specific value to a variable. This operator is symbolized by the equals sign (=), marking its significance as one of the most frequently used operators in Python programming.
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables. Operator. Description. Syntax = Assign value of right side of expression to left side operand: x = y + z +=
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right. Assignment is defined recursively depending on the form of the target (list).
Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. So assume if we need to add 7 to a variable "a" and assign the result back to "a", then instead of writing normally as " a = a + 7 ", we can use ...
The Python language has distinct concepts for expressions and statements. Assignment is a statement even if the syntax sometimes tricks you into thinking it's an expression (e.g. a=b=99 works but is a special syntax case and doesn't mean that the b=99 is an expression like it is for example in C). List comprehensions are instead expressions because they return a value, in a sense the loop they ...
Here are some best practices when using augmented assignments in Python: Use whitespace around the operators: x += 1 rather than x+=1 for readability. Limit chaining augmented assignments like x = y = 0. Use temporary variables if needed for clarity. Don't overuse augmented assignment especially with mutable types.
To copy a list of lists in python, we can use the copy() and the deepcopy() method provided in the copy module. Shallow Copy List of Lists in Python. The copy() method takes a nested list as an input argument. After execution, it returns a list of lists similar to the original list. You can observe this in the following example.
python programs with output for class 12 and 11 students. Simple Assignements are for beginners starting from basics hello world program to game development using class and object concepts. A list of assignment solutions with source code are provided to learn concept by examples in an easy way.
There are different types of operators arithmetic, logical, relational, assignment, and bitwise, etc. Python has an assignment operator that helps to assign values or expressions to the left-hand-side variable. The assignment operator is represented as the "=" symbol used in assignment statements and assignment expressions.
List out a few services which use python. Enlist 5 programming languages with their use in a specific domain; Assignment - 03: [1]. Consider variables A = 8 and B = 6. Write a program to demonstrate various arithmetic operators [2]. Consider x = 30 and y = 30. Write a program to demonstrate various comparison operators. Assignment - 04: [1].
So always use the == operator to check if any two Python objects have the same value. 4. Tuple Assignment and Mutable Objects . If you're familiar with built-in data structures in Python, you know that tuples are immutable. So you cannot modify them in place. Data structures like lists and dictionaries, on the other hand, are mutable.
3. a is a pointer to the list [1,2]. When you do the assignment b = a the value of b is the address of the list [1,2]. So when you do a.append(3) you are not actually changing a, you are changing the list that a points to. Since a and b both point to the same list, they both appear to change when you modify the other.
Hi everyone! I am here to write global assignments like; Java, Python, C++, Articles, Thesis, Projects and more. plagiarism free💯 whatsapp : +923176293311
To see the Get & Transform Data and Queries & Connections groups, select the Data tab. Choose Get Data to select your data source. You can import data from multiple sources, including comma-separated values (CSV) files. Every import creates a query. This example imports data from the Northwind OData service, a test data source.To do this, select Get Data > From Other Sources > From OData Feed.
As you have written: list1=[1,2,3,4] list2=list1. The above code snippet will map list1 to list2. To check whether two variables refer to the same object, you can use is operator. >>> list1 is list2. # will return "True". In your example, Python created one list, reference by list1 & list2. So there are two references to the same object.
The Pittsburgh Pirates today ended the rehab assignment of right-handed pitcher Colin Holderman and reinstated him from the 15-day injured list (illness). Holderman allowed one hit in 3.0 scoreless innings in his final three rehab appearances with Triple-A Indianapolis after giving up three runs in 0.2 inning in his first
4. Python employs assignment unpacking when you have an iterable being assigned to multiple variables like above. In Python3.x this has been extended, as you can also unpack to a number of variables that is less than the length of the iterable using the star operator: >>> a,b,*c = [1,2,3,4] >>> a. 1. >>> b. 2.
I'm new to python but I will try my best to explain what's happening. So i want to write a python script which detects VID,PID and NAME of a keyboard and mouse using pywin32. I already wrote a script for detecting a mouse and it works.