- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python datetime module
- Python DateTime - Date Class
- ctime() Function Of Datetime.date Class In Python
- fromisoformat() Function Of Datetime.date Class In Python
- Fromordinal() Function Of Datetime.date Class In Python
- fromtimestamp() Function Of Datetime.date Class In Python
- isocalendar() Function Of Datetime.date Class In Python
- Isoformat() Function Of Datetime.date Class In Python
- Isoweekday() Function Of Datetime.date Class In Python
- timetuple() Function Of Datetime.date Class In Python
- Get current date using Python
- toordinal() Function Of Datetime.date Class In Python
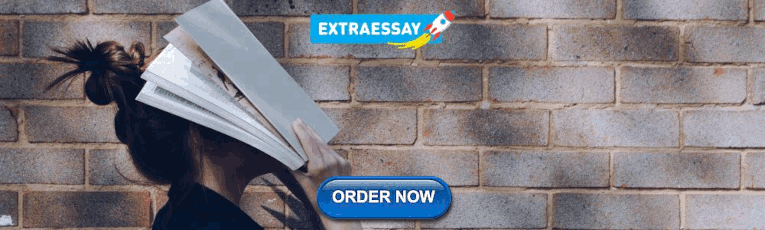
weekday() Function Of Datetime.date Class In Python
- Python DateTime - Time Class
- Python time.daylight() Function
- Python time.tzname() Function
Datetime class
- Python DateTime - DateTime Class
- Python DateTime astimezone() Method
- Python - time.ctime() Method
- Isocalendar() Method Of Datetime Class In Python
- Isoformat() Method Of Datetime Class In Python
- Isoweekday() Method Of Datetime Class In Python
- Datetime.replace() Function in Python
- Python DateTime - strptime() Function
- Python | time.time() method
- Python datetime.timetz() Method with Example
- Python - datetime.toordinal() Method with Example
- Get UTC timestamp in Python
- Python datetime.utcoffset() Method with Example
Timedelta class
- Python DateTime - Timedelta Class
- Python - Timedelta object with negative values
- Python | Difference between two dates (in minutes) using datetime.timedelta() method
- Python | datetime.timedelta() function
- Python timedelta total_seconds() Method with Example
- Python - datetime.tzinfo()
- Handling timezone in Python
The weekday() of datetime.date class function is used to return the integer value corresponding to the specified day of the week.
Syntax: weekday() Parameters: This function does not accept any parameter. Return values: This function returns the integer mapping corresponding to the specified day of the week. Below table shows the integer values corresponding to the day of the week.
Example 1: Getting the integer values corresponding to the specified days of the week.
Example 2: Getting the date and time along with the day’s name of the entire week.
Please Login to comment...
Similar reads.
- Python-datetime
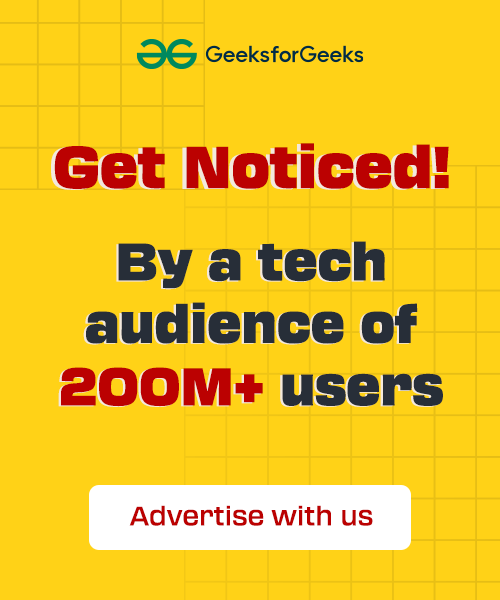
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
5 Pythonic Ways to Check if a Date is a Weekend Using datetime
💡 Problem Formulation: When working with dates in Python, it’s common to need to check whether a given date falls on a weekend. This is crucial for applications that require different logic for weekdays versus weekends, such as scheduling software or automated reports that only run on business days. For example, given a date input, we want to determine whether it’s a Saturday or Sunday (the typical weekend in many regions).
Method 1: Using weekday() Function
The weekday() function of Python’s datetime module returns an integer corresponding to the day of the week, where Monday is 0 and Sunday is 6. By checking if this integer is 5 or 6, we can determine if the date is a weekend.
Here’s an example:
Output: True
This method is straightforward and readable. It utilizes the convenience of Python’s built-in functions to perform the check with minimal code.
Method 2: Using the strftime() Function
The strftime() function formats a date into a string based on a directive given to it. By formatting the date to return the day of the week as a string and comparing it to “Saturday” or “Sunday”, we can determine if it is a weekend day.
This method is particularly useful when you need the day of the week as a string for other purposes as well, although it can be less efficient than numerical comparison due to string operations.
Method 3: Using isoweekday() Function
Similar to weekday() , the isoweekday() function returns an integer representing the day of the week, but according to the ISO standard where Monday is 1 and Sunday is 7. Checking for 6 and 7 will tell us if it’s a weekend.
This approach operates almost identically to the first method but adheres to the ISO standard for the days of the week, which may be preferable in some international contexts.
Method 4: Using timedelta and weekday
This method involves determining if the given date is within the range between the last Friday and the next Monday. It’s a bit more complex but useful if your weekend definition might vary (e.g., Friday evening to Monday morning).
This method might be an overkill for basic weekend checking but allows flexibility if different definitions of the weekend are needed.
Bonus One-Liner Method 5: Lambda Function
For a quick, in-place way to check if a date is a weekend, a lambda function can be employed. This is best when you need a simple check done once without needing a full function definition elsewhere in your code.
The lambda function here is a concise and direct way to check for a weekend, great for one-off checks within other expressions or functions.
Summary/Discussion
- Method 1: weekday() Function. Straightforward and efficient. Best for those who prefer numerical comparisons over string comparisons. Might be less clear to readers who don’t remember which number corresponds to which day.
- Method 2: strftime() Function. Clear and human-readable. Offers string-based comparison, which may be less efficient and could potentially lead to errors with different locale settings.
- Method 3: isoweekday() Function. Similar benefits to Method 1 but uses the ISO standard for the days of the week. It is suitable for international contexts.
- Method 4: Using timedelta and weekday. Flexible and powerful, allowing for a customizable definition of the weekend. However, it is more complex and hence may be less clear at a glance.
- Bonus Method 5: Lambda Function. Quick and elegant for one-off checks. However, due to the lack of explicit function definition, it may not be as clear to maintain for those unfamiliar with lambda functions or pythonic shortcuts.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
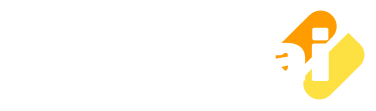
- Latest Posts
Get Dates of Working Days Between Two Dates in Python (Exclude Weekends)
Here I am giving an example to show you how to get dates of working days between two dates in Python by excluding weekends.
Python Workdays Example
In the below Python program, it will take three parameters, 1st is the start date, 2nd is the end date, and the third is default specified the day numbers 6 and 7, for Saturday and Sundays.
You can see in the above result that the weekend dates 26th (Saturday) and 27th (Sunday) of January 2019 are not present.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Sign in to your account
Username or Email Address
Remember Me
Assignments » Conditional Structures » Set 1 » Solution 6
Write a program that prompts the user to input a number. The program should display the corresponding day for the given number. For example, if the user types 1, the output should be Sunday. If the user types 7, the output should be Saturday.
Source Code
Enter a number from 1 to 7: 5 5 is Thursday
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
- Weekend in Python
Checking whether a date is a weekday or weekend in Python is very easy. If you don’t know, weekend days are Saturday and Sunday . Check out the following code to learn how to find a date is a weekday or weekend.
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
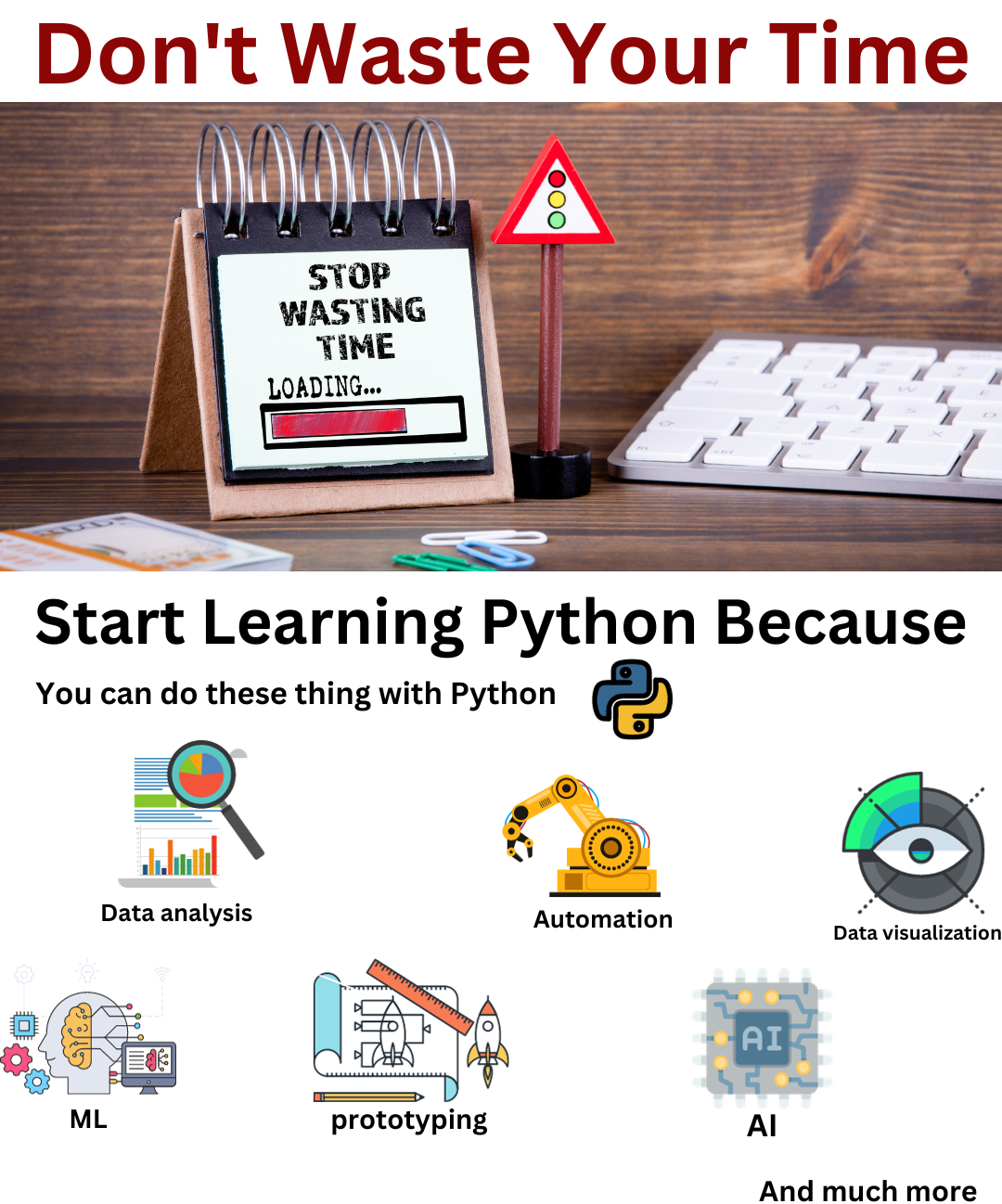
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
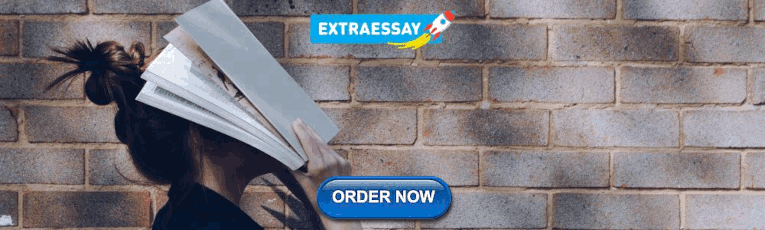
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Entry task assignment for python weekend | https://pythonweekend.cz/
kiwicom/python-weekend-entry-task
Folders and files, repository files navigation, python weekend entry task.
Welcome to Europython! To experience the attendee selection process for Kiwi.com Python Weekend and win some flight vouchers for your next trip, you can try solving this entry task and receiving feedback. Just email the solution in a zip file to [email protected] with the subject starting with PW . Happy coding!
Write a python script/module/package, that for a given flight data in a form of csv file (check the examples), prints out a structured list of all flight combinations for a selected route between airports A -> B, sorted by the final price for the trip.
Description
You've been provided with some semi-randomly generated example csv datasets you can use to test your solution. The datasets have following columns:
- flight_no : Flight number.
- origin , destination : Airport codes.
- departure , arrival : Dates and times of the departures/arrivals.
- base_price , bag_price : Prices of the ticket and one piece of baggage.
- bags_allowed : Number of allowed pieces of baggage for the flight.
In addition to the dataset, your script will take some additional arguments as input:
Search restrictions
- By default you're performing search on ALL available combinations, according to search parameters.
- In case of a combination of A -> B -> C, the layover time in B should not be less than 1 hour and more than 6 hours .
- A -> B -> A -> C is not a valid combination for search A -> C.
- Output is sorted by the final price of the trip.
Optional arguments
You may add any number of additional search parameters to boost your chances to attend. Here are 2 recommended ones:
Performing return trip search
Example input (assuming solution.py is the main module):
will perform a search RFZ -> WIW -> RFZ for flights which allow at least 1 piece of baggage.
- NOTE: Since WIW is in this case the final destination for one part of the trip, the layover rule does not apply.
The output will be a json-compatible structured list of trips sorted by price. The trip has the following schema:
For more information, check the example section.
Points of interest
Assuming your solution is working, we'll be additionally judging based on following skills:
- input, output - what if we input garbage?
- modules, packages & code structure (hint: it's easy to overdo it)
- usage of standard library and built-in data structures
- code readability, clarity, used conventions, documentation and comments
Requirements and restrictions
- Your solution needs to contain a README file describing what it does and how to run it.
- Only the standard library is allowed, no 3rd party packages, notebooks, specialized distros (Conda) etc.
- The code should run as is, no environment setup should be required.
Example behaviour
Let's imagine we wrote our solution into one file, solution.py and our datatset is in data.csv . We want to test the script by performing a flight search on route BTW -> REJ (we know the airports are present in the dataset) with one bag. We run the thing:
and get the following result:
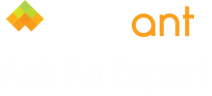
Python_Assignment
You found an exciting summer job for five weeks. Suppose it pays $15.50 per hour.
Suppose that: total_tax is 14% of the total pay for five weeks.
After paying taxes you spend 10% of your net income to buy new clothes and accessories for the next school year.
After buying cloth and accessories you spend 25% of the remaining money to buy savings bonds.
For each dollar spent buying savings bonds your parents spend $0.50 to buy additional savings bonds for you.
Write a program that prompts the user to enter the pay rate for an hour of work and the number of hours worked each week.
The program outputs the following:
Your income before and after taxes from your five-week summer job.
Money spent on cloth and accessories
Money spent to buy savings bonds
Money your parents spend to buy additional savings bonds
Money left after taxes, cloth, and accessories, savings bonds(you bought).
Notes for the assignment:
Code should include the header file as usual, along with comments that are brief, specific, and meaningful(no rambling).
Please be sure blank lines are used between sections of code to make it easier to read and variables well named.
No function calls, arithmetic calculations, rounding, or type conversions can be done in print statements.
Create a function for the savings bond calculation based on what the parents spent. The function must return what the parents spent to buy a savings bond.
All dollar values need to be rounded in two decimal places.
1 Expert Answer
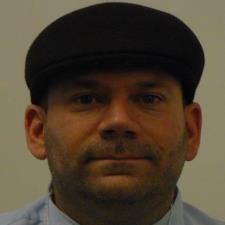
Patrick B. answered 01/18/21
Math and computer tutor/teacher
def CalculateSavingsBonds( x):
return(int(x) * 0.50)
hourlyWage = input("Please input the hourly wage :>")
weeklyHoursWorked = input("Please # of hours worked each week :>")
print("hourly wage is " + str(hourlyWage))
print(" weekly hours worked is " + str(weeklyHoursWorked))
grossSalary = 5 * float(weeklyHoursWorked)*float(hourlyWage)
taxAmount = grossSalary * 0.14
balance = float(grossSalary) - float(taxAmount)
print(" gross Salary is " + str(grossSalary))
print(" tax amount is " + str(taxAmount))
print(" net salary after taxes is " + str(balance))
schoolSupplies = 0.10 * float(balance)
print(" Amount spent on school supplies " + str(schoolSupplies))
balance = float(balance) - float(schoolSupplies)
savingsBonds = 0.25 * float(balance)
print("Amount spend on savings bonds " + str(savingsBonds))
balance = float(balance) - float(savingsBonds)
print("Remaining balance is " + str(balance))
parentsSavingsBonds = CalculateSavingsBonds(savingsBonds)
print(" Amount Parents spend on additional savings bonds " + str(parentsSavingsBonds))
Still looking for help? Get the right answer, fast.
Get a free answer to a quick problem. Most questions answered within 4 hours.
Choose an expert and meet online. No packages or subscriptions, pay only for the time you need.
RELATED TOPICS
Related questions, write an employee payroll program that uses polymorphism to calculate and print the weekly payroll for your company.
Answers · 2
Hey, I have this question for Matlab, and I am completely lost on what I need to do .
Answers · 1
Under what circumstances would you use a sequential file over a database?
Answers · 4
Help writing this program homework
Using visual basic write program, recommended tutors.
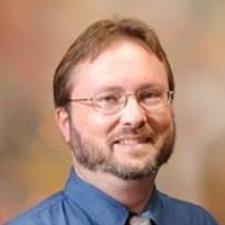
find an online tutor
- Computer Science tutors
- Object Oriented Programming tutors
- Coding tutors
- Software Engineering tutors
- User Interface Programming tutors
- Computer Architecture tutors
- Medical Coding tutors
- Functional Programming tutors
related lessons
- What is a Robot? Etymology and History
- Need help with something else? Try one of our lessons.
- How it works
- Homework answers
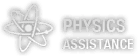
Python Answers
Questions: 5 831
Answers by our Experts: 5 728
Ask Your question
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Search & Filtering
Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not.
11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
11.2 Create an instance of the triangle class and call all the defined methods.
11.3 Create three child classes of triangle class - isosceles_triangle, right_triangle and equilateral_triangle.
11.4 Define methods which check for their properties.
Create an empty dictionary called Car_0 . Then fill the dictionary with Keys : color , speed , X_position and Y_position.
car_0 = {'x_position': 10, 'y_position': 72, 'speed': 'medium'} .
a) If the speed is slow the coordinates of the X_pos get incremented by 2.
b) If the speed is Medium the coordinates of the X_pos gets incremented by 9
c) Now if the speed is Fast the coordinates of the X_pos gets incremented by 22.
Print the modified dictionary.
Create a simple Card game in which there are 8 cards which are randomly chosen from a deck. The first card is shown face up. The game asks the player to predict whether the next card in the selection will have a higher or lower value than the currently showing card.
For example, say the card that’s shown is a 3. The player chooses “higher,” and the next card is shown. If that card has a higher value, the player is correct. In this example, if the player had chosen “lower,” they would have been incorrect. If the player guesses correctly, they get 20 points. If they choose incorrectly, they lose 15 points. If the next card to be turned over has the same value as the previous card, the player is incorrect.
Consider an ongoing test cricket series. Following are the names of the players and their scores in the test1 and 2.
Test Match 1 :
Dhoni : 56 , Balaji : 94
Test Match 2 :
Balaji : 80 , Dravid : 105
Calculate the highest number of runs scored by an individual cricketer in both of the matches. Create a python function Max_Score (M) that reads a dictionary M that recognizes the player with the highest total score. This function will return ( Top player , Total Score ) . You can consider the Top player as String who is the highest scorer and Top score as Integer .
Input : Max_Score({‘test1’:{‘Dhoni’:56, ‘Balaji : 85}, ‘test2’:{‘Dhoni’ 87, ‘Balaji’’:200}}) Output : (‘Balaji ‘ , 200)
Write a Python program to demonstrate Polymorphism.
1. Class Vehicle with a parameterized function Fare, that takes input value as fare and
returns it to calling Objects.
2. Create five separate variables Bus, Car, Train, Truck and Ship that call the Fare
3. Use a third variable TotalFare to store the sum of fare for each Vehicle Type. 4. Print the TotalFare.
Write a Python program to demonstrate multiple inheritance.
1. Employee class has 3 data members EmployeeID , Gender (String) , Salary and
PerformanceRating ( Out of 5 ) of type int. It has a get() function to get these details from
2. JoiningDetail class has a data member DateOfJoining of type Date and a function
getDoJ to get the Date of joining of employees.
3. Information Class uses the marks from Employee class and the DateOfJoining date
from the JoiningDetail class to calculate the top 3 Employees based on their Ratings and then Display, using readData , all the details on these employees in Ascending order of their Date Of Joining.
You are given an array of numbers as input: [10,20,10,40,50,45,30,70,5,20,45] and a target value: 50. You are required to find pairs of elements (indices of two numbers) from the given array whose sum equals a specific target number. Your solution should not use the same element twice, thus it must be a single solution for each input
1.1 Write a Python class that defines a function to find pairs which takes 2 parameters (input array and target value) and returns a list of pairs whose sum is equal to target given above. You are required to print the list of pairs and state how many pairs if found. Your solution should call the function to find pairs, then return a list of pairs.
1.2 Given the input array nums in 1.1 above. Write a second program to find a set of good pairs from that input array nums. Here a pair (i,j) is said to be a good pair if nums[i] is the same as nums[j] and i < j. You are required to display an array of good pairs indices and the number of good pairs.
How to find largest number inn list in python
Given a list of integers, write a program to print the sum of all prime numbers in the list of integers.
Note: one is neither prime nor composite number
Using the pass statement
how to get a input here ! example ! 2 4 5 6 7 8 2 4 5 2 3 8 how to get it?
- Programming
- Engineering
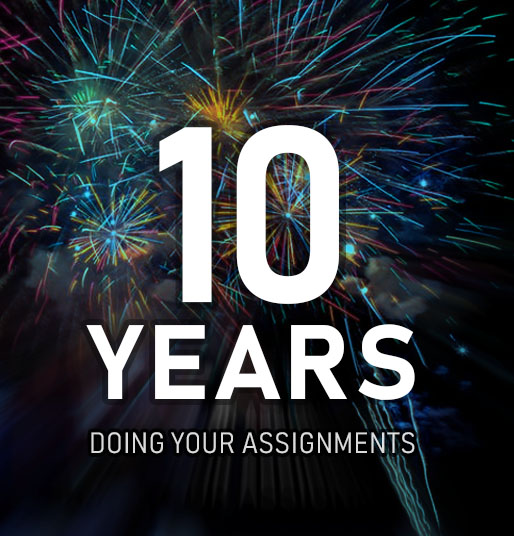
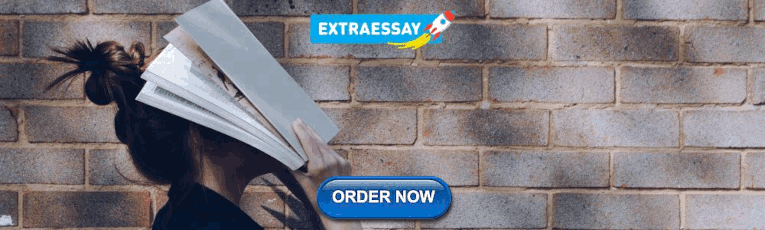
IMAGES
VIDEO
COMMENTS
Question #176131. Weekends. Given two dates D1 and D2, write a program to count the number of Saturdays and Sundays from D1 to D2 (including D1 and D2). The date in string format is like "8 Feb 2021".Input. The first line of input will contain date D1 in the string format. The second line of input will contain date D2 in the string format.Output.
Given two dates D1 and D2, write a program to count number of Saturdays and Sundays from D1 to D2 (including D1 and D2). The date in string format like "8 Feb 2021". Input. The first line of input will contain date D1 in string format. The second line of input will contain date D2 in string format. Output.
Python. Question #174121. Weekends. Given two dates D1 and D2, write a program to count the number of Saturdays and Sundays from D1 to D2 (including D1 and D2). The date in string format is like "8 Feb 2021".Input. The first line of input will contain date D1 in the string format. The second line of input will contain date D2 in the string format.
How to find whether a particular day is weekday or weekend in Python? python; Share. Improve this question. Follow edited Aug 29, 2022 at 14:27. Karl Knechtel. 61.7k 12 12 gold badges 117 117 silver badges 162 162 bronze badges. asked Apr 1, 2015 at 6:58. vinay h vinay h.
Assignment Operators; Relational Operators; Logical Operators; Ternary Operators ... we will discuss the weekday() function in the DateTime module. weekday() function is used to get the week number based on given DateTime. It will return the number in the range of 0-6. ... Python program to get the name of weekday. Python3 # create a list of ...
Exercise 1: Print current date and time in Python. Exercise 2: Convert string into a datetime object. Exercise 3: Subtract a week (7 days) from a given date in Python. Exercise 4: Print a date in a the following format. Exercise 5: Find the day of the week of a given date. Exercise 6: Add a week (7 days) and 12 hours to a given date.
The weekday () of datetime.date class function is used to return the integer value corresponding to the specified day of the week. Syntax: weekday () Parameters: This function does not accept any parameter. Return values: This function returns the integer mapping corresponding to the specified day of the week.
Method 1: Using weekday () Function. The weekday() function of Python's datetime module returns an integer corresponding to the day of the week, where Monday is 0 and Sunday is 6. By checking if this integer is 5 or 6, we can determine if the date is a weekend. Here's an example: from datetime import datetime. def is_weekend(date):
Here I am giving an example to show you how to get dates of working days between two dates in Python by excluding weekends. Python Workdays Example In the below Python program, it will take three parameters, 1st is the start date, 2nd is the end date, and the third is default specified the day numbers 6 and 7, for Saturday and Sundays.
Python Assignment - Week of the Day. Assignments » Conditional Structures » Set 1 » Solution 6. Write a program that prompts the user to input a number. The program should display the corresponding day for the given number. For example, if the user types 1, the output should be Sunday. If the user types 7, the output should be Saturday.
If you don't know, weekend days are Saturday and Sunday. Check out the following code to learn how to find a date is a weekday or weekend. Code: from datetime import datetime d = datetime (year, month, date); if d.weekday () > 4: print 'Date is weekend.' else: print 'Data is weekday.'. Also Read:
Assignment Write a python script/module/package, that for a given flight data in a form of csv file (check the examples), prints out a structured list of all flight combinations for a selected route between airports A -> B, sorted by the final price for the trip.
Write a Python program to find the day of the week for any particular date in the past or future. Let the input be in the format "dd mm yyyy".Examples: Approach #2 : Using strftime () methodThe strftime () method takes one or more format codes as an argument and returns a formatted string based on it. Here we will pass the directive "%A ...
Python_Assignment. You found an exciting summer job for five weeks. Suppose it pays $15.50 per hour. Suppose that: total_tax is 14% of the total pay for five weeks. After paying taxes you spend 10% of your net income to buy new clothes and accessories for the next school year. After buying cloth and accessories you spend 25% of the remaining ...
Question #246593. Weekends. Given two dates D1 and D2, write a program to count the number of Saturdays and Sundays from D1 to D2 (including D1 and D2).The date in string format is like "8 Feb 2021". Input: The first line of input will contain date D1 in the string format.
Question #189798. Given two dates D1 and D2, write a program to count the number of Saturdays and Sundays from D1 to D2 (including D1 and D2). The date in string format is like "8 Feb 2021" . The first line of input will contain date D1 in the string format . The second line of input will contain date D2 in the string format .
This week's assignment involves writing a Python program to collect all the data of a road trip and calculate each person's share of the cost. Prompt the user for each of the following: . The number of people on the trip. • The number of days of the trip. . For each day of the trip: o Cost of food.
ALL Answered. Question #350996. Python. Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not. 11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
The food and gas costs should be stored in two. This week's assignment involves writing a Python program to collect all the data of a road trip and calculate each person's share of the cost. Prompt the user for each of the following: - The number of people on the trip. - The number of days of the trip. - For each day of the trip: Cost of food ...
This week's assignment involves writing a Python program to compute the weekly pay for a paper carrier. Your program should prompt the user for the following numeric values: the number of papers on the route • the number of days the paper is delivered per week • the amount of tips received for the week Your program should define (not prompt) for the following: • the cost of each ...
The food and gas costs should be stored in two. This week's assignment involves writing a Python program to collect all the data of a road trip and calculate each person's share of the cost. Prompt the user for each of the following: The number of people on the trip. The number of days of the trip. For each day of the trip: Cost of food.