How to read and write JSON using Gson in Java
Dependencies, create java class, convert java object to json string, convert java object to json file, convert list of java objects to json array, java object to pretty print json file, convert java map to json file, convert json string to java object, convert json file to java object, convert json array to list of java objects, convert json file to java map, json field naming with @serializedname annotation, include null fields, exclude fields with @expose annotation.
In my previous article , we looked at reading and writing JSON files in Java using different open-source libraries like JSON.simple , Jackson , Moshi, and Gson.
In this article, you'll learn how to read and write JSON using Gson in detail. Gson is a popular Java library developed and maintained by Google to convert Java Objects into their JSON representation.
It can also convert a JSON string to an equivalent Java object. Gson can work with arbitrary Java objects, including pre-existing objects you do not modify.
To add Gson to your Gradle project, add the following dependency to the build.gradle file:
For Maven, include the below dependency to your pom.xml file:
Let us first create a simple Java class for holding the Book information:
To convert a Java Object to a JSON string, all you need to do is create a new instance of Gson and then call the toJson() method with Java Object as a parameter:
The above code will produce the following JSON string:
The toJson() method also accepts an instance of Writer as a second parameter that you can use to output the JSON directly to a file, as shown below:
The book.json file should contain the following content:
You can also convert a list of Java Objects to a JSON array using the same toJson() method, as shown below:
The books.json file should look like the below:
Just like Jackson , you can output pretty-print JSON using Gson:
The book.json file will have the following pretty-print JSON:
Using Gson, you can also serialize a Java Map object to a JSON file, as shown below:
To convert a JSON string back to an equivalent Java Object, you can use the fromJson() method from Gson :
The above code will output the following on the console:
The fromJson() method also accepts an instance of Reader to read and parse the JSON data from a file:
The following example shows how to convert a JSON array to a list of Java Objects using the Gson library:
You should then see the following output:
By using Gson, you can also convert a JSON file to a Java Map with the same properties and keys, as shown below:
By default, Gson uses the class field names as JSON property names. With @SerializedName , you can specify a custom JSON property name:
Now if you convert a Book object to JSON, the output should look like the below:
By default, Gson ignores null fields while serializing a Java Object to its JSON representation:
The above code will produce the following JSON without null fields:
To explicitly include the null fields in the final JSON output, you can use the serializeNulls() method from GsonBuilder :
Now the JSON output should include null fields, as shown below:
Gson provides multiple ways to exclude fields while serializing or deserializing JSON. By default, all static and transient fields are excluded.
However, you can change this default behavior with the excludeFieldsWithModifiers() method. For example, the following configuration excludes static fields only:
If you want to exclude both static and transient , use the following (which is equivalent to the default configuration):
If you want to exclude fields explicitly , use the @Expose annotation. It defines the attributes to be excluded from serialization and deserialization to JSON. To enable @Expose , you need to create the Gson object like the below:
Now, all fields without @Expose will be excluded. Let us add this annotation to the Book class:
Now, if you convert the above Java Object to JSON, the output will be like the below:
✌️ Like this article? Follow me on Twitter and LinkedIn . You can also subscribe to RSS Feed .
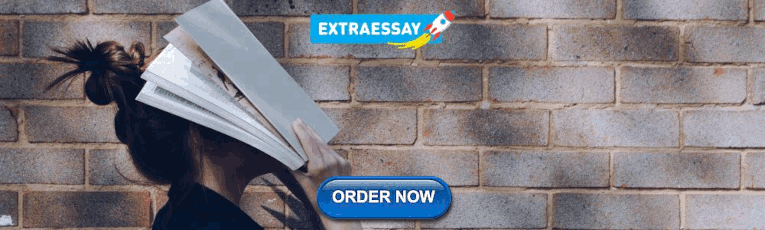
You might also like...
- Calculate days between two OffsetDateTime objects in Java
- Calculate days between two ZonedDateTime objects in Java
- Calculate days between two LocalDateTime objects in Java
- Calculate days between two LocalDate objects in Java
- How to check if an enum value exists in Java
- How to iterate over enum values in Java
The simplest cloud platform for developers & teams. Start with a $200 free credit.
Buy me a coffee ☕
If you enjoy reading my articles and want to help me out paying bills, please consider buying me a coffee ($5) or two ($10). I will be highly grateful to you ✌️
Enter the number of coffees below:
✨ Learn to build modern web applications using JavaScript and Spring Boot
I started this blog as a place to share everything I have learned in the last decade. I write about modern JavaScript, Node.js, Spring Boot, core Java, RESTful APIs, and all things web development.
The newsletter is sent every week and includes early access to clear, concise, and easy-to-follow tutorials, and other stuff I think you'd enjoy! No spam ever, unsubscribe at any time.
- JavaScript, Node.js & Spring Boot
- In-depth tutorials
- Super-handy protips
- Cool stuff around the web
- 1-click unsubscribe
- No spam, free-forever!
Gson Design Document
This document presents issues that we faced while designing Gson. It is meant for advanced users or developers working on Gson. If you are interested in learning how to use Gson, see its user guide.
Some information in this document is outdated and does not reflect the current state of Gson. This information can however still be relevant for understanding the history of Gson.
Navigating the Json tree or the target Type Tree while deserializing
When you are deserializing a Json string into an object of desired type, you can either navigate the tree of the input, or the type tree of the desired type. Gson uses the latter approach of navigating the type of the target object. This keeps you in tight control of instantiating only the type of objects that you are expecting (essentially validating the input against the expected “schema”). By doing this, you also ignore any extra fields that the Json input has but were not expected.
As part of Gson, we wrote a general purpose ObjectNavigator that can take any object and navigate through its fields calling a visitor of your choice.
Supporting richer serialization semantics than deserialization semantics
Gson supports serialization of arbitrary collections, but can only deserialize genericized collections. this means that Gson can, in some cases, fail to deserialize Json that it wrote. This is primarily a limitation of the Java type system since when you encounter a Json array of arbitrary types there is no way to detect the types of individual elements. We could have chosen to restrict the serialization to support only generic collections, but chose not to. This is because often the user of the library are concerned with either serialization or deserialization, but not both. In such cases, there is no need to artificially restrict the serialization capabilities.
Supporting serialization and deserialization of classes that are not under your control and hence can not be modified
Some Json libraries use annotations on fields or methods to indicate which fields should be used for Json serialization. That approach essentially precludes the use of classes from JDK or third-party libraries. We solved this problem by defining the notion of custom serializers and deserializers. This approach is not new, and was used by the JAX-RPC technology to solve essentially the same problem.
Using Checked vs Unchecked exceptions to indicate a parsing error
We chose to use unchecked exceptions to indicate a parsing failure. This is primarily done because usually the client can not recover from bad input, and hence forcing them to catch a checked exception results in sloppy code in the catch() block.
Creating class instances for deserialization
Gson needs to create a dummy class instance before it can deserialize Json data into its fields. We could have used Guice to get such an instance, but that would have resulted in a dependency on Guice. Moreover, it probably would have done the wrong thing since Guice is expected to return a valid instance, whereas we need to create a dummy one. Worse, Gson would overwrite the fields of that instance with the incoming data thereby modifying the instance for all subsequent Guice injections. This is clearly not a desired behavior. Hence, we create class instances by invoking the parameterless constructor. We also handle the primitive types, enums, collections, sets, maps and trees as a special case.
To solve the problem of supporting unmodifiable types, we use custom instance creators. So, if you want to use a library type that does not define a default constructor (for example, Money class), then you can register an instance creator that returns a dummy instance when asked.
Using fields vs getters to indicate Json elements
Some Json libraries use the getters of a type to deduce the Json elements. We chose to use all fields (up the inheritance hierarchy) that are not transient, static, or synthetic. We did this because not all classes are written with suitably named getters. Moreover, getXXX or isXXX might be semantic rather than indicating properties.
However, there are good arguments to support properties as well. We intend to enhance Gson in a later version to support properties as an alternate mapping for indicating Json fields. For now, Gson is fields-based.
Why are most classes in Gson marked as final?
While Gson provides a fairly extensible architecture by providing pluggable serializers and deserializers, Gson classes were not specifically designed to be extensible. Providing non-final classes would have allowed a user to legitimately extend Gson classes, and then expect that behavior to work in all subsequent revisions. We chose to limit such use-cases by marking classes as final, and waiting until a good use-case emerges to allow extensibility. Marking a class final also has a minor benefit of providing additional optimization opportunities to Java compiler and virtual machine.
Why are inner interfaces and classes used heavily in Gson?
Gson uses inner classes substantially. Many of the public interfaces are inner interfaces too (see JsonSerializer.Context or JsonDeserializer.Context as an example). These are primarily done as a matter of style. For example, we could have moved JsonSerializer.Context to be a top-level class JsonSerializerContext , but chose not to do so. However, if you can give us good reasons to rename it alternately, we are open to changing this philosophy.
Why do you provide two ways of constructing Gson?
Gson can be constructed in two ways: by invoking new Gson() or by using a GsonBuilder . We chose to provide a simple no-args constructor to handle simple use-cases for Gson where you want to use default options, and quickly want to get going with writing code. For all other situations, where you need to configure Gson with options such as formatters, version controls etc., we use a builder pattern. The builder pattern allows a user to specify multiple optional settings for what essentially become constructor parameters for Gson.
Comparing Gson with alternate approaches
Note that these comparisons were done while developing Gson so these date back to mid to late 2007.
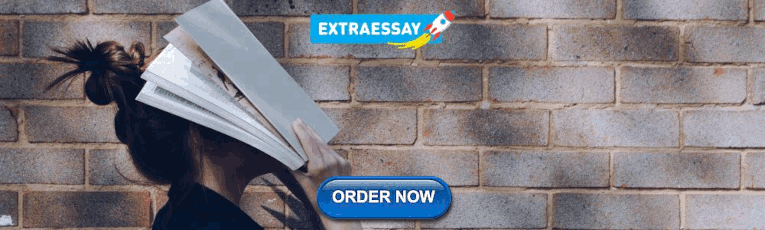
Comparing Gson with org.json library
org.json is a much lower-level library that can be used to write a toJson() method in a class. If you can not use Gson directly (maybe because of platform restrictions regarding reflection), you could use org.json to hand-code a toJson method in each object.
Comparing Gson with org.json.simple library
org.json.simple library is very similar to org.json library and hence fairly low level. The key issue with this library is that it does not handle exceptions very well. In some cases it appeared to just eat the exception while in other cases it throws an “Error” rather than an exception.
- Skip to main content
- Skip to primary sidebar
- Skip to footer
Additional menu

Largest free Technical and Blogging resource site for Beginner. We help clients transform their great ideas into reality!
How to use Gson -> fromJson() to convert the specified JSON into an Object of the Specified Class
Updated on Feb 16, 2023 by App 2
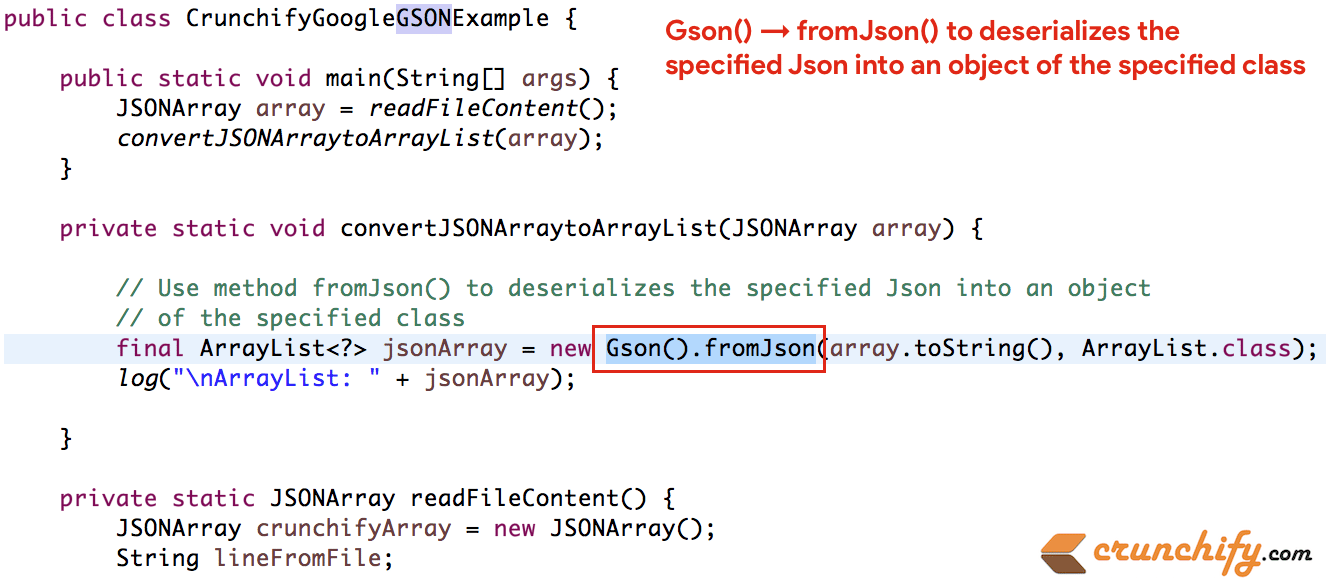
Convert JSON to XML using Gson and JAXB.
As many of you know already Gson is a great Java library that can be used to convert Java Objects into their JSON representation. It works also in reverse order deserializing the specified JSONObject or JSONArray into an object of the specified class.
How to convert Java object to / from JSON (Gson)?
Basically – this same tutorial will help you if you want to convert gson fromjson to map, gson fromjson to list, json fromjson to array, json string to arraylist in java.
In this tutorial we will go over below steps:
- Read File content from file in Java
- We will use regex split operation to bypass any blank space in between words
- Create JSONObject out of each line
- Add each JSONObject to JSONArray
- Print JSONArray
- Now using Gson’s fromJson() method we will deserialize JSONArray to ArrayList
In order to run below code you need below Maven dependency .
Also, here is a file which we are using in below program.
Please download and put it under C: drive or Documents folder and update path below.
Here is a Google Gson Goal from official Github Repository:
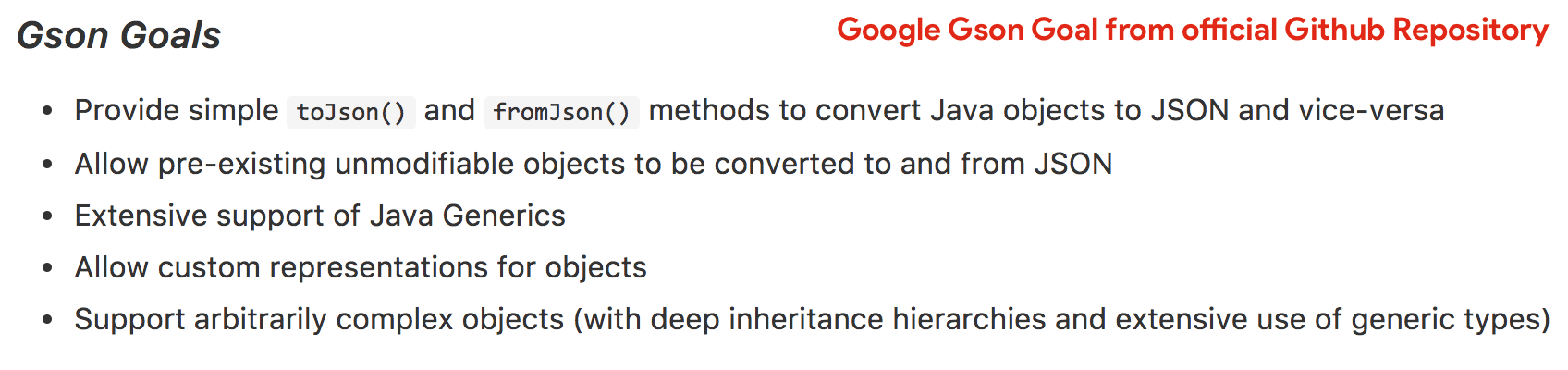
If you liked this article, then please share it on social media. Have a question or suggestion? Please leave a comment to start the discussion.
Suggested Articles...
- How to pretty print JSON in Java using Jackson and Gson both? Example attached
- JSON Manipulation in Java – Examples
- How to Parse JSONObject and JSONArrays in Java? Beginner’s Guide
- In Java How to Convert ArrayList to JSONObject?
- In Java How to Convert Map / HashMap to JSONObject? [4 Different Ways]
- Build RESTful Service in Java using JAX-RS and Jersey (Celsius to Fahrenheit & Fahrenheit to Celsius)
I'm an Engineer by profession, Blogger by passion & Founder of Crunchify, LLC, the largest free blogging & technical resource site for beginners. Love SEO, SaaS, #webperf, WordPress, Java. With over 16 millions+ pageviews/month, Crunchify has changed the life of over thousands of individual around the globe teaching Java & Web Tech for FREE.
Reader Interactions
2 comments....
Aug 1, 2017 at 8:53 pm
Nice article and want to share https:// jsonformatter. org for working with JSON data.
May 12, 2018 at 10:28 am
Thanks Iris for sharing JSONFormatter. I’ll check it out.
Leave a Reply Cancel reply
Comment Policy : We welcome relevant and respectful comments. All comments are manually moderated and those deemed to be spam or solely promotional will be deleted.
Top Tech Categories…
Top blogging categories….
How to fix this unchecked assignment warning?

I got Warning:(31, 46) Unchecked assignment: 'java.lang.Class' to 'java.lang.Class<? extends PACKAGE_NAME.Block>' warning on the line blockRta.registerSubtype(c); , but I can’t figure out how to fix that without supressing it.
ReflectionHelper.getClasses is a static method to get all the classes in that package name, and its return type is Class[] . Block is an interface. RuntimeTypeAdapterFactory is a class in gson extra, and its source code can be viewed here .
Advertisement
Since ReflectionHelper.getClasses returns an array of the raw type Class , the local-variable type inference will use this raw type Class[] for var blks and in turn, the raw type Class for var c . Using the raw type Class for c allows passing it to registerSubtype(Class<? extends Block>) , without any check, but not without any warning. You can use the method asSubclass to perform a checked conversion, but you have to declare an explicit non-raw variable type, to get rid of the raw type, as otherwise, even the result of the asSubclass invocation will be erased to a raw type by the compiler.
There are two approaches. Change the type of blks :
Then, the type of var c changes automatically to Class<?> .
Or just change the type of c :
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Unchecked assignment warning #3
samoylenko commented Sep 30, 2019
No branches or pull requests
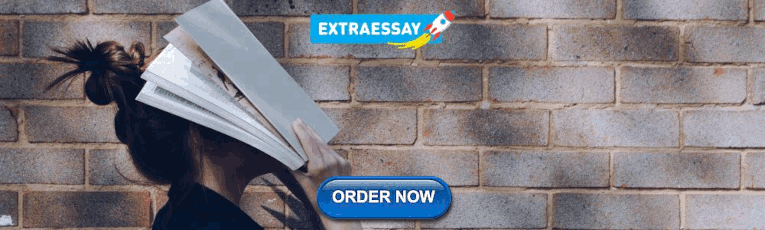
IMAGES
VIDEO
COMMENTS
This will tell Gson which generic type you need to use. See my explanation for why this works here: How does Gson TypeToken work? As an aside, don't name your classes the same thing as anything in the java.lang package.
Troubleshooting Guide. This guide describes how to troubleshoot common issues when using Gson. ClassCastException when using deserialized object. Symptom: ClassCastException is thrown when accessing an object deserialized by Gson Reason: Your code is most likely not type-safe Solution: Make sure your code adheres to the following: Avoid raw types: Instead of calling fromJson(..., List.class ...
Gson is a Java library developed by Google that allows converting Java objects to JSON and vice versa. It simplifies serialization and deserialization tasks, providing customization options and efficient performance for handling complex object structures.. The latest version of the Gson library can be found in the Maven Central Repository.Let's add the dependency to our pom.xml:
Gson is a Java library that can be used to convert Java Objects into their JSON representation. It can also be used to convert a JSON string to an equivalent Java object. Gson can work with arbitrary Java objects including pre-existing objects that you do not have source code of.
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
In this article, you'll learn how to read and write JSON using Gson in detail. Gson is a popular Java library developed and maintained by Google to convert Java Objects into their JSON representation. It can also convert a JSON string to an equivalent Java object. Gson can work with arbitrary Java objects, including pre-existing objects you do ...
Here, we use Gson's TypeToken to determine the correct type to be deserialized - ArrayList<MyClass>. The idiom used to get the listOfMyClassObject actually defines an anonymous local inner class containing a method getType() that returns the fully parameterized type. 3. List of Polymorphic Objects
This document presents issues that we faced while designing Gson. It is meant for advanced users or developers working on Gson. If you are interested in learning how to use Gson, see its user guide. Some information in this document is outdated and does not reflect the current state of Gson. This information can however still be relevant for ...
Do you want to learn how to use Gson, a Java library that can convert Java objects into JSON and vice versa? In this tutorial, you will see how to use Gson -> fromJson() method to parse a JSON string into an object of a specified class. You will also find other useful resources on Gson and JSON on Crunchify.com, a website that provides practical tips and tricks for web development.
MacBook:Homework Brienna$ javac Orders.java -Xlint:unchecked Orders.java:152: warning: [unchecked] unchecked cast orders = (ArrayList<Vehicle>) in.readObject(); ^ required: ArrayList<Vehicle> found: Object 1 warning I always try to improve my code instead of ignoring or suppressing warnings. ... Generic Class for a GSON LinkedHashMap. Hot ...
All modern applications generally fetch data from remote services (e.g. REST or SOAP) that are mostly either XML or JSON format. Gson helps applications in Java-JSON serialization and deserialization automatically as well as manually, if needed, using simple toJson() and fromJson() methods.. Gson can work with arbitrary Java objects including pre-existing objects that we do not have source ...
Answer. Since ReflectionHelper.getClasses returns an array of the raw type Class, the local-variable type inference will use this raw type Class[] for var blks and in turn, the raw type Class for var c. Using the raw type Class for c allows passing it to registerSubtype(Class<? extends Block>), without any check, but not without any warning.
2. Passing Map.class. In general, Gson provides the following API in its Gson class to convert a JSON string to an object: public <T> T fromJson(String json, Class<T> classOfT) throws JsonSyntaxException; From the signature, it's very clear that the second parameter is the class of the object which we intend the JSON to parse into.
Reflection is a powerful feature in Java that allows us to inspect and manipulate classes, interfaces, fields, methods, and other components at runtime. Moreover, it provides the ability to access information about the structure of a class, invoke methods dynamically, and even modify private fields.
For checked exceptions Gson only has MalformedJsonException which is only used by JsonReader so there is probably no need for changes there (and changes would most likely also be source incompatible due to it being a checked exception). Feature description. Define a proper hierarchy for unchecked exceptions.
Java Generics, how to avoid unchecked assignment warning when using class hierarchy? Intellij is giving me the warning below. Not sure how to resolve it, or even if I need to resolve it. The warning details says it only applies to JDK 5, and I am using 6. I am wondering if I need to respond to this, and if so, how? Method call causing warning
This line: Map<String, Map<String, String>> mappingData = Files.exists(path) ? gson.fromJson(Files.newBufferedReader(path), HashMap.class) : new HashMap<>();
I'm using the following code to find a one-dimensional list of unique objects in an n-dimensional list (credits to someone on StackOverflow a while ago for the approach): public static <T> List<T> getUniqueObjectsInArray(List<T> array) {. Integer dimension = getDimensions(array); return getUniqueObjectsInArray(array, dimension);
In this cookbook, we're exploring the various ways to unmarshall JSON into Java objects, using the popular Gson library. 1. Deserialize JSON to Single Basic Object. Let's start simple - we're going to unmarshall a simple json to a Java object - Foo: public class Foo {. public int intValue;