Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
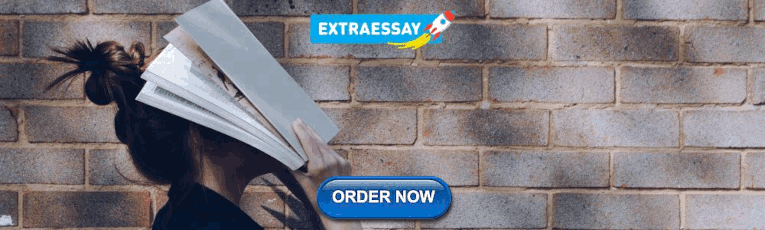
Mutable, unmodifiable, and immutable empty Map in Java
This article will discuss different ways to create a mutable, unmodifiable, immutable, and fixed-length empty map in Java.
Mutable maps supports modification operations such as add, remove, and clear on it. Unmodifiable Maps are “read-only” wrappers over other maps. They do not support add, remove, and clear operations, but we can modify their underlying map. Maps that guarantee that no change in the map will ever be visible (even if their underlying map is modified) are referred to as immutable .
Please note that making a map final will not make it unmodifiable or immutable. We can still add key-value pairs or remove key-value pairs from them. Only the reference to the map is final.
1. Mutable Empty Map
⮚ Using Plain Java
We can simply use the HashMap constructor, which constructs an empty resizable-array implementation of the Map interface. The following code takes advantage of the new “diamond” syntax introduced in Java SE 7.
⮚ Using Guava
Guava’s Maps.newHashMap() creates a mutable, empty HashMap instance. This method is deprecated in Java 7 and above.
⮚ Java 8
We can use the Java 8 Stream to construct empty collections by combining stream factory methods and collectors. Collectors.toMap() returns a Collector that accumulates the input key-value pairs into a new Map. To create an empty map, we can pass an empty 2D array.
2. Unmodifiable Empty Map
⮚ Using Collections
Collections unmodifiableMap() returns an unmodifiable view of the specified map.
⮚ Using Apache Collections: MapUtils Class
Apache Commons Collections MapUtils.unmodifiableMap() returns an unmodifiable map backed by the specified map.
Both the above methods throw a NullPointerException if the specified map is null. If we try to modify the returned map, the map will throw an UnsupportedOperationException . However, any changes in the original mutable map will be visible in the unmodifiable map.
3. Immutable Empty Map
There are several ways to create an immutable empty map in Java. The map will throw an UnsupportedOperationException if any modification operation is performed on it.
We can use Collections.EMPTY_MAP that returns a serializable and immutable empty map.
The above method might throw an unchecked assignment warning. The following example demonstrates the type-safe way to obtain an empty map:
⮚ In Java 8
We could adapt the Collectors.toMap() collector discussed earlier to always produce an immutable empty map, as shown below:
Guava provides several static utility methods that can be used to obtain an immutable empty map.
1. ImmutableMap.copyOf returns an immutable empty map if specified map is empty.
The method will throw a NullPointerException if the specified map is null, and any changes in the underlying mutable map will not be visible in the immutable map.
2. Guava also provides a builder that can create an immutable empty map instance similarly.
3. ImmutableMap.of() can also be used to return an immutable empty map.
⮚ Using Apache Collections
Apache Commons Collections provides MapUtils.EMPTY_MAP that returns an immutable empty map.
⮚ In Java 9
Java 9 comes with static factory methods on the Map interface that can create compact, unmodifiable instances.
We can use Map.of() to create the structurally immutable empty map. Keys and values cannot be added to it, and calling any mutator method will always cause UnsupportedOperationException to be thrown.
4. Fixed Length Empty Map
There is another type of empty map possible in Java apart from mutable, unmodifiable, and immutable maps called fixed-length map.
Apache Commons Collections MapUtils class has a fixedSizeMap() method that can return a fixed-length empty map backed by the specified empty map. We cannot add elements to the returned map, and the map will throw an UnsupportedOperationException if any resize operation is performed on it.
That’s all about mutable, unmodifiable, and immutable empty Map in Java.
Unmodifiable Map in Java
Mutable, unmodifiable, and immutable empty Set in Java
Mutable, unmodifiable, and immutable empty List in Java
Rate this post
Average rating 4.71 /5. Vote count: 7
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Unchecked assignment: 'java.util.Map<java.lang.String,java.lang.Object>' to 'java.util.Map<java.lang.String,java.lang.String>' #1
weituotian commented Apr 23, 2018
Sorry, something went wrong.
No branches or pull requests
- About TedBlob
- Privacy Policy
- Terms and Conditions
Jackson Java convert between JSON and Map
- November 27, 2021 November 29, 2021
1. Overview
In this article, we will learn to convert between JSON and Map in Java using Jackson library.
To learn more about Jackson, refer to our articles .
By encoding your Java object as a JSON string, you can transfer the Java Map object over a network or store it in a database or a file.
Serialization is converting your Java object in your application to the JSON format , whereas deserialization converts the JSON back into the original Java object .
ObjectMapper class of Jackson helps you with the serialization and deserialization process in Java. Let’s understand how to configure your Jackson to convert between Map and JSON.
2. Dependencies
To get started, you must add the Jackson library as a dependency in your project.

This ObjectMapper class is available in the Jackson databind project.
The com.fasterxml.jackson.core.databind library dependency pulls in all other required dependencies into your project:
- jackson-annotations
- jackson-core
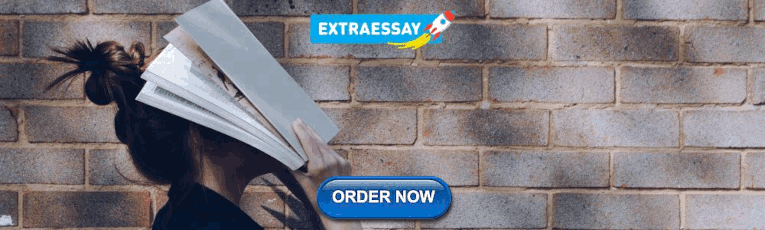
3. Jackson convert between JSON and Map<String, String>
Let’s see serialization and deserialization between JSON and Map<String, String>.
3.1. Serialization – convert Map<String, String> to a JSON
There is no special configuration required to convert Map<String, String> to JSON. You can simply use the ObjectMapper class to convert the Map to JSON.
For example, the below test method contains a Map of users and their corresponding age .
If you run the above code, the ObjectMapper converts the Map to JSON and prints the following result:
3.2. Deserialization – convert JSON to Map<String, String>
You can deserialize the JSON with an array of objects to Map<String, String> by using the ObjectMapper's readValue method and passing Map.class as a parameter. However, IDE throws unchecked assignment Map to Map<String, String>.
Though the above code works fine and provides the expected result, the IDE would show the following warning
Type safety: The expression of type Map needs unchecked conversion to conform to Map
3.2.1. Configure TypeReference for Map<String, String> deserialization
You can prevent the unchecked assignment error by using the TypeReference , a class of Jackson library that allows to provide the type information to Jackson. Therefore, Jackson resolves to the provided type.
3.2.2. Configure TypeFactory for Map<String, String> deserialization
Alternatively, you can use the overloaded readValue function that accepts the JavaType as input:
The JavaType is the base class that contains type information for deserializer. To create a JavaType , you first need to get the configured and readily available TypeFactory instance from the ObjectMapper object.
You can create the MapType (subclass of JavaType ) to deserialize the JSON array of objects as a Map object.
4. Jackson convert between JSON and Map<String, Object>
Let’s see serialization and deserialization between JSON and Map<String, Object>.
4.1. Serialization – convert Map<String, Object > to a JSON
Here, no special configuration is required to convert Map<String, Object > to JSON. You can simply use the ObjectMapper class to convert the Map to JSON.
For example, the below test method contains an Map of students sorted by their rank.
4.2. Deserialize to Map<String, Object> from JSON
You can deserialize the JSON with an array of objects to Map<String, Object> by using the ObjectMapper'sreadValue method and passing Map.class as a parameter. However, IDE throws unchecked assignment Map to Map<String, Object>.
If you run the above code, then the ObjectMapper converts the JSON to Map<String, Student> and prints the following result.
3.2.1. Configure TypeReference for Map<String, Object> deserialization
You can prevent the unchecked assignment error by using the TypeReference , a class of Jackson library that allows providing the type information to Jackson. Therefore, Jackson resolves to the provided type.
You can create the MapType (subclass of JavaType ) to deserialize the JSON array of objects as a Map<String, Student> object.
5. Conclusion
To sum up, we have learned to serialize or deserialize maps using the Jackson library.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
{{#message}}{{{message}}}{{/message}}{{^message}}Your submission failed. The server responded with {{status_text}} (code {{status_code}}). Please contact the developer of this form processor to improve this message. Learn More {{/message}}
{{#message}}{{{message}}}{{/message}}{{^message}}It appears your submission was successful. Even though the server responded OK, it is possible the submission was not processed. Please contact the developer of this form processor to improve this message. Learn More {{/message}}
Submitting…
- Prev Class
- Next Class
- No Frames
- All Classes
- Summary:
- Nested |
- Field |
- Constr |
- Detail:
Interface MapChangeListener<K,V>
- Type Parameters: K - the key element type V - the value element type All Known Implementing Classes: WeakMapChangeListener Functional Interface: This is a functional interface and can therefore be used as the assignment target for a lambda expression or method reference. @FunctionalInterface public interface MapChangeListener<K,V> Interface that receives notifications of changes to an ObservableMap. Since: JavaFX 2.0
Nested Class Summary
Method summary, method detail.
Copyright (c) 2008, 2015, Oracle and/or its affiliates. All rights reserved.
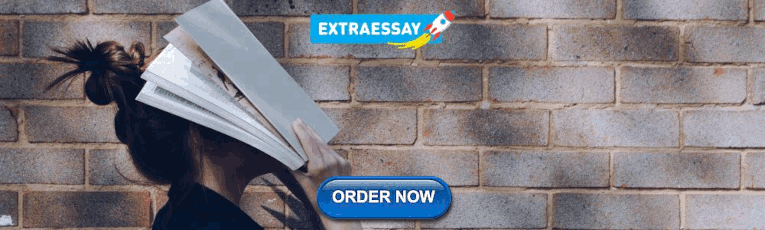
IMAGES
VIDEO
COMMENTS
Map<Integer, String> map = a.getMap(); gets you a warning now: "Unchecked assignment: 'java.util.Map to java.util.Map<java.lang.Integer, java.lang.String>'. Even though the signature of getMap is totally independent of T, and the code is unambiguous regarding the types the Map contains. I know that I can get rid of the warning by reimplementing ...
Of course that's an unchecked assignment. You seem to be implementing a heterogeneous map. Java (and any other strongly-typed language) has no way to express the value type of your map in a statically-type-safe way. That is because the element types are only known at runtime.
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
The above method might throw an unchecked assignment warning. The following example demonstrates the type-safe way to obtain an empty map: ... We can use the Java 8 Stream to construct small maps by obtaining stream from static factory methods like Stream.of() or Arrays.stream() and accumulating the input elements into a new map using collectors.
Jun 1, 2023. Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler. This operation is unchecked because the ...
Casting, instanceof, and @SuppressWarnings("unchecked") are noisy. It would be nice to stuff them down into a method where they won't need to be looked at. CheckedCast.castToMapOf() is an attempt to do that. castToMapOf() is making some assumptions: (1) The map can't be trusted to be homogeneous (2) Redesigning to avoid need for casting or instanceof is not viable
The map will throw an UnsupportedOperationException if any modification operation is performed on it. ... The above method might throw an unchecked assignment warning. The following example demonstrates the type-safe way to obtain an empty map: 1. Map < String, String > immutableMap = Collections. emptyMap (); ⮚ In Java 8.
With a few extra steps, we can also serialize a map containing a custom Java class. Let's create a MyPair class to represent a pair of related String objects.. Note: the getters/setters should be public, and we annotate toString() with @JsonValue to ensure Jackson uses this custom toString() when serializing:. public class MyPair { private String first; private String second; @Override ...
5.2. Checking Type Conversion Before Using the Raw Type Collection. The warning message " unchecked conversion " implies that we should check the conversion before the assignment. To check the type conversion, we can go through the raw type collection and cast every element to our parameterized type.
public static class AbstractMap.SimpleEntry<K,V>. extends Object. implements Map.Entry <K,V>, Serializable. An Entry maintaining a key and a value. The value may be changed using the setValue method. This class facilitates the process of building custom map implementations. For example, it may be convenient to return arrays of SimpleEntry ...
The type checker is marking a real issue here. To visualise this, replace your RecursiveElement<T> with a generic Iterable<T>, which provides identical type guarantees.. When different layers mix different types, RecursiveIterator unfortunately breaks down. Here is an example:
Unchecked assignment: java.util.List to java.util.List<String> Unfortunately, there's no way to fix that problem. I've tried adding <String>before the dot and after, but neither works.
An unchecked cast warning in Java occurs when the compiler cannot verify that a cast is safe at compile time. This can happen when you are casting an object to a type that is not a supertype or subtype of the object's actual type. To address an unchecked cast warning, you can either suppress the warning using the @SuppressWarnings("unchecked ...
private Map<String, String> getVendorProperties(DataSource dataSource) { return jpaProperties.getHibernateProperties(dataSource); } 多数据源那里
In this tutorial, we'll explore three different approaches to converting an Object to a Map in Java using reflection, Jackson, and Gson APIs. 2. Using Reflection. Reflection is a powerful feature in Java that allows us to inspect and manipulate classes, interfaces, fields, methods, and other components at runtime. Moreover, it provides the ...
个人博客导航页(点击右侧链接即可打开个人博客):大牛带你入门技术栈 . 问题. 日常在写 Java 代码时对警告 Type safety: Unchecked cast from XXX to YYY 一定不会陌生,例如 Type safety: Unchecked cast from Object to Map<String,String>。如果仔细观察的话,可以注意到,YYY 从来不会是一个非泛型的类型。
You can deserialize the JSON with an array of objects to Map<String, Object> by using the ObjectMapper'sreadValue method and passing Map.class as a parameter. However, IDE throws unchecked assignment Map to Map<String, Object>.
Java Generics, how to avoid unchecked assignment warning when using class hierarchy? Intellij is giving me the warning below. Not sure how to resolve it, or even if I need to resolve it. The warning details says it only applies to JDK 5, and I am using 6. I am wondering if I need to respond to this, and if so, how? Method call causing warning
WeakMapChangeListener. Functional Interface: This is a functional interface and can therefore be used as the assignment target for a lambda expression or method reference. @FunctionalInterface. public interface MapChangeListener<K,V>. Interface that receives notifications of changes to an ObservableMap. Since: