
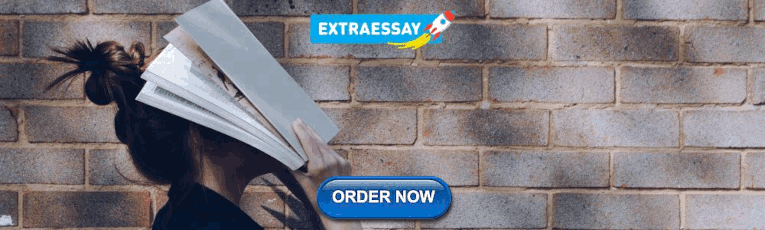
Top 100 C Programming Interview Questions and Answers (PDF)
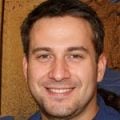
Here are C Programming interview questions and answers for fresher as well as experienced candidates to get their dream job.
Basic C Programming Interview Questions and Answers for Freshers
1) how do you construct an increment statement or decrement statement in c.
There are actually two ways you can do this. One is to use the increment operator ++ and decrement operator –. For example, the statement “x++” means to increment the value of x by 1. Likewise, the statement “x –” means to decrement the value of x by 1. Another way of writing increment statements is to use the conventional + plus sign or – minus sign. In the case of “x++”, another way to write it is “x = x +1”.
👉 Free PDF Download: C Programming Interview Questions & Answers >>
2) What is the difference between Call by Value and Call by Reference?
When using Call by Value, you are sending the value of a variable as parameter to a function, whereas Call by Reference sends the address of the variable. Also, under Call by Value, the value in the parameter is not affected by whatever operation that takes place, while in the case of Call by Reference, values can be affected by the process within the function.
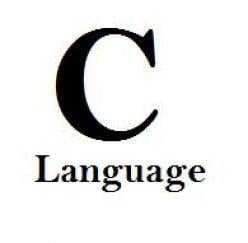
3) Some coders debug their programs by placing comment symbols on some codes instead of deleting it. How does this aid in debugging?
Placing comment symbols /* */ around a code, also referred to as “commenting out”, is a way of isolating some codes that you think maybe causing errors in the program, without deleting the code. The idea is that if the code is in fact correct, you simply remove the comment symbols and continue on. It also saves you time and effort on having to retype the codes if you have deleted it in the first place.
4) What is the equivalent code of the following statement in WHILE LOOP format?
5) what is a stack.
A stack is one form of a data structure. Data is stored in stacks using the FILO (First In Last Out) approach. At any particular instance, only the top of the stack is accessible, which means that in order to retrieve data that is stored inside the stack, those on the upper part should be extracted first. Storing data in a stack is also referred to as a PUSH, while data retrieval is referred to as a POP.
6) What is a sequential access file?
When writing programs that will store and retrieve data in a file, it is possible to designate that file into different forms. A sequential access file is such that data are saved in sequential order: one data is placed into the file after another. To access a particular data within the sequential access file, data has to be read one data at a time, until the right one is reached.
7) What is variable initialization and why is it important?
This refers to the process wherein a variable is assigned an initial value before it is used in the program. Without initialization, a variable would have an unknown value, which can lead to unpredictable outputs when used in computations or other operations.
8 What is spaghetti programming?
Spaghetti programming refers to codes that tend to get tangled and overlapped throughout the program. This unstructured approach to coding is usually attributed to lack of experience on the part of the programmer. Spaghetti programing makes a program complex and analyzing the codes difficult, and so must be avoided as much as possible.
9) Differentiate Source Codes from Object Codes
Source codes are codes that were written by the programmer. It is made up of the commands and other English-like keywords that are supposed to instruct the computer what to do. However, computers would not be able to understand source codes. Therefore, source codes are compiled using a compiler. The resulting outputs are object codes, which are in a format that can be understood by the computer processor. In C programming , source codes are saved with the file extension .C, while object codes are saved with the file extension .OBJ
10) In C programming, how do you insert quote characters (‘ and “) into the output screen?
This is a common problem for beginners because quotes are normally part of a printf statement. To insert the quote character as part of the output, use the format specifiers \’ (for single quote), and \” (for double quote).
11) What is the use of a ‘\0’ character?
It is referred to as a terminating null character, and is used primarily to show the end of a string value.
12) What is the difference between the = symbol and == symbol?
The = symbol is often used in mathematical operations. It is used to assign a value to a given variable. On the other hand, the == symbol, also known as “equal to” or “equivalent to”, is a relational operator that is used to compare two values.
13) What is the modulus operator?
The modulus operator outputs the remainder of a division. It makes use of the percentage (%) symbol. For example: 10 % 3 = 1, meaning when you divide 10 by 3, the remainder is 1.
14) What is a nested loop?
A nested loop is a loop that runs within another loop. Put it in another sense, you have an inner loop that is inside an outer loop. In this scenario, the inner loop is performed a number of times as specified by the outer loop. For each turn on the outer loop, the inner loop is first performed.
15) Which of the following operators is incorrect and why? ( >=, <=, <>, ==)
<> is incorrect. While this operator is correctly interpreted as “not equal to” in writing conditional statements, it is not the proper operator to be used in C programming . Instead, the operator != must be used to indicate “not equal to” condition.
16) Compare and contrast compilers from interpreters.
Compilers and interpreters often deal with how program codes are executed. Interpreters execute program codes one line at a time, while compilers take the program as a whole and convert it into object code, before executing it. The key difference here is that in the case of interpreters, a program may encounter syntax errors in the middle of execution, and will stop from there. On the other hand, compilers check the syntax of the entire program and will only proceed to execution when no syntax errors are found.
17) How do you declare a variable that will hold string values?
The char keyword can only hold 1 character value at a time. By creating an array of characters, you can store string values in it. Example: “char MyName[50]; ” declares a string variable named MyName that can hold a maximum of 50 characters.
18) Can the curly brackets { } be used to enclose a single line of code?
While curly brackets are mainly used to group several lines of codes, it will still work without error if you used it for a single line. Some programmers prefer this method as a way of organizing codes to make it look clearer, especially in conditional statements.
19) What are header files and what are its uses in C programming?
Header files are also known as library files. They contain two essential things: the definitions and prototypes of functions being used in a program. Simply put, commands that you use in C programming are actually functions that are defined from within each header files. Each header file contains a set of functions. For example: stdio.h is a header file that contains definition and prototypes of commands like printf and scanf.
20) What is syntax error?
Syntax errors are associated with mistakes in the use of a programming language. It maybe a command that was misspelled or a command that must was entered in lowercase mode but was instead entered with an upper case character. A misplaced symbol, or lack of symbol, somewhere within a line of code can also lead to syntax error.
21) What are variables and it what way is it different from constants?
Variables and constants may at first look similar in a sense that both are identifiers made up of one character or more characters (letters, numbers and a few allowable symbols). Both will also hold a particular value. Values held by a variable can be altered throughout the program, and can be used in most operations and computations. Constants are given values at one time only, placed at the beginning of a program. This value is not altered in the program. For example, you can assigned a constant named PI and give it a value 3.1415 . You can then use it as PI in the program, instead of having to write 3.1415 each time you need it.
22) How do you access the values within an array?
Arrays contain a number of elements, depending on the size you gave it during variable declaration. Each element is assigned a number from 0 to number of elements-1. To assign or retrieve the value of a particular element, refer to the element number. For example: if you have a declaration that says “intscores[5];”, then you have 5 accessible elements, namely: scores[0], scores[1], scores[2], scores[3] and scores[4].
23) Can I use “int” data type to store the value 32768? Why?
No. “int” data type is capable of storing values from -32768 to 32767. To store 32768, you can use “long int” instead. You can also use “unsigned int”, assuming you don’t intend to store negative values.
24) Can two or more operators such as \n and \t be combined in a single line of program code?
Yes, it’s perfectly valid to combine operators, especially if the need arises. For example: you can have a code like printf (“Hello\n\n\’World\'”) to output the text “Hello” on the first line and “World” enclosed in single quotes to appear on the next two lines.
25) Why is it that not all header files are declared in every C program?
The choice of declaring a header file at the top of each C program would depend on what commands/functions you will be using in that program. Since each header file contains different function definitions and prototype, you would be using only those header files that would contain the functions you will need. Declaring all header files in every program would only increase the overall file size and load of the program, and is not considered a good programming style.
26) When is the “void” keyword used in a function?
When declaring functions, you will decide whether that function would be returning a value or not. If that function will not return a value, such as when the purpose of a function is to display some outputs on the screen, then “void” is to be placed at the leftmost part of the function header. When a return value is expected after the function execution, the data type of the return value is placed instead of “void”.
27) What are compound statements?
Compound statements are made up of two or more program statements that are executed together. This usually occurs while handling conditions wherein a series of statements are executed when a TRUE or FALSE is evaluated. Compound statements can also be executed within a loop. Curly brackets { } are placed before and after compound statements.
28) What is the significance of an algorithm to C programming?
Before a program can be written, an algorithm has to be created first. An algorithm provides a step by step procedure on how a solution can be derived. It also acts as a blueprint on how a program will start and end, including what process and computations are involved.
29) What is the advantage of an array over individual variables?
When storing multiple related data, it is a good idea to use arrays. This is because arrays are named using only 1 word followed by an element number. For example: to store the 10 test results of 1 student, one can use 10 different variable names (grade1, grade2, grade3… grade10). With arrays, only 1 name is used, the rest are accessible through the index name (grade[0], grade[1], grade[2]… grade[9]).
30) Write a loop statement that will show the following output:
C programming interview questions and answers for experienced, 31) what is wrong in this statement scanf(“%d”,whatnumber);.
An ampersand & symbol must be placed before the variable name whatnumber. Placing & means whatever integer value is entered by the user is stored at the “address” of the variable name. This is a common mistake for programmers, often leading to logical errors.
32) How do you generate random numbers in C?
Random numbers are generated in C using the rand() command . For example: anyNum = rand() will generate any integer number beginning from 0, assuming that anyNum is a variable of type integer.
33) What could possibly be the problem if a valid function name such as tolower() is being reported by the C compiler as undefined?
The most probable reason behind this error is that the header file for that function was not indicated at the top of the program. Header files contain the definition and prototype for functions and commands used in a C program. In the case of “tolower()”, the code “#include <ctype.h>” must be present at the beginning of the program.
34) What are comments and how do you insert it in a C program?
35) what is debugging.
Debugging is the process of identifying errors within a program. During program compilation, errors that are found will stop the program from executing completely. At this state, the programmer would look into the possible portions where the error occurred. Debugging ensures the removal of errors, and plays an important role in ensuring that the expected program output is met.
36) What does the && operator do in a program code?
The && is also referred to as AND operator. When using this operator, all conditions specified must be TRUE before the next action can be performed. If you have 10 conditions and all but 1 fails to evaluate as TRUE, the entire condition statement is already evaluated as FALSE
37) In C programming, what command or code can be used to determine if a number of odd or even?
There is no single command or function in C that can check if a number is odd or even. However, this can be accomplished by dividing that number by 2, then checking the remainder. If the remainder is 0, then that number is even, otherwise, it is odd. You can write it in code as:
38) What does the format %10.2 mean when included in a printf statement?
This format is used for two things: to set the number of spaces allotted for the output number and to set the number of decimal places. The number before the decimal point is for the allotted space, in this case it would allot 10 spaces for the output number. If the number of space occupied by the output number is less than 10, addition space characters will be inserted before the actual output number. The number after the decimal point sets the number of decimal places, in this case, it’s 2 decimal spaces.
39) What are logical errors and how does it differ from syntax errors?
Program that contains logical errors tend to pass the compilation process, but the resulting output may not be the expected one. This happens when a wrong formula was inserted into the code, or a wrong sequence of commands was performed. Syntax errors, on the other hand, deal with incorrect commands that are misspelled or not recognized by the compiler.
40) What are the different types of control structures in programming?
There are 3 main control structures in programming: Sequence, Selection and Repetition. Sequential control follows a top to bottom flow in executing a program, such that step 1 is first perform, followed by step 2, all the way until the last step is performed. Selection deals with conditional statements, which mean codes are executed depending on the evaluation of conditions as being TRUE or FALSE. This also means that not all codes may be executed, and there are alternative flows within. Repetitions are also known as loop structures, and will repeat one or two program statements set by a counter.
41) What is || operator and how does it function in a program?
The || is also known as the OR operator in C programming. When using || to evaluate logical conditions, any condition that evaluates to TRUE will render the entire condition statement as TRUE.
42) Can the “if” function be used in comparing strings?
No. “if” command can only be used to compare numerical values and single character values. For comparing string values, there is another function called strcmp that deals specifically with strings.
43) What are preprocessor directives?
Preprocessor directives are placed at the beginning of every C program. This is where library files are specified, which would depend on what functions are to be used in the program. Another use of preprocessor directives is the declaration of constants.Preprocessor directives begin with the # symbol.
44) What will be the outcome of the following conditional statement if the value of variable s is 10?
s >=10 && s < 25 && s!=12
45) Describe the order of precedence with regards to operators in C.
Order of precedence determines which operation must first take place in an operation statement or conditional statement. On the top most level of precedence are the unary operators !, +, – and &. It is followed by the regular mathematical operators (*, / and modulus % first, followed by + and -). Next in line are the relational operators <, <=, >= and >. This is then followed by the two equality operators == and !=. The logical operators && and || are next evaluated. On the last level is the assignment operator =.
46) What is wrong with this statement? myName = “Robin”;
You cannot use the = sign to assign values to a string variable. Instead, use the strcpy function. The correct statement would be: strcpy(myName, “Robin”);
47) How do you determine the length of a string value that was stored in a variable?
To get the length of a string value, use the function strlen(). For example, if you have a variable named FullName, you can get the length of the stored string value by using this statement: I = strlen(FullName); the variable I will now have the character length of the string value.
48) Is it possible to initialize a variable at the time it was declared?
Yes, you don’t have to write a separate assignment statement after the variable declaration, unless you plan to change it later on. For example: char planet[15] = “Earth”; does two things: it declares a string variable named planet, then initializes it with the value “Earth”.
49) Why is C language being considered a middle level language?
This is because C language is rich in features that make it behave like a high level language while at the same time can interact with hardware using low level methods. The use of a well structured approach to programming, coupled with English-like words used in functions, makes it act as a high level language. On the other hand, C can directly access memory structures similar to assembly language routines.
50) What are the different file extensions involved when programming in C?
Source codes in C are saved with .C file extension. Header files or library files have the .H file extension. Every time a program source code is successfully compiled, it creates an .OBJ object file, and an executable .EXE file.
51) What are reserved words?
Reserved words are words that are part of the standard C language library. This means that reserved words have special meaning and therefore cannot be used for purposes other than what it is originally intended for. Examples of reserved words are int, void, and return.
52) What are linked list?
A linked list is composed of nodes that are connected with another. In C programming, linked lists are created using pointers. Using linked lists is one efficient way of utilizing memory for storage.
53) What is FIFO?
In C programming, there is a data structure known as queue. In this structure, data is stored and accessed using FIFO format, or First-In-First-Out. A queue represents a line wherein the first data that was stored will be the first one that is accessible as well.
54) What are binary trees?
Binary trees are actually an extension of the concept of linked lists. A binary tree has two pointers, a left one and a right one. Each side can further branch to form additional nodes, which each node having two pointers as well. Learn more about Binary Tree in Data Structure if you are interested.
55) Not all reserved words are written in lowercase. TRUE or FALSE?
FALSE. All reserved words must be written in lowercase; otherwise the C compiler would interpret this as unidentified and invalid.
56) What is the difference between the expression “++a” and “a++”?
In the first expression, the increment would happen first on variable a, and the resulting value will be the one to be used. This is also known as a prefix increment. In the second expression, the current value of variable a would the one to be used in an operation, before the value of a itself is incremented. This is also known as postfix increment.
57) What would happen to X in this expression: X += 15; (assuming the value of X is 5)
X +=15 is a short method of writing X = X + 15, so if the initial value of X is 5, then 5 + 15 = 20.
58) In C language, the variables NAME, name, and Name are all the same. TRUE or FALSE?
FALSE. C language is a case sensitive language. Therefore, NAME, name and Name are three uniquely different variables.
59) What is an endless loop?
An endless loop can mean two things. One is that it was designed to loop continuously until the condition within the loop is met, after which a break function would cause the program to step out of the loop. Another idea of an endless loop is when an incorrect loop condition was written, causing the loop to run erroneously forever. Endless loops are oftentimes referred to as infinite loops.
60) What is a program flowchart and how does it help in writing a program?
A flowchart provides a visual representation of the step by step procedure towards solving a given problem. Flowcharts are made of symbols, with each symbol in the form of different shapes. Each shape may represent a particular entity within the entire program structure, such as a process, a condition, or even an input/output phase.
61) What is wrong with this program statement? void = 10;
The word void is a reserved word in C language. You cannot use reserved words as a user-defined variable.
62) Is this program statement valid? INT = 10.50;
Assuming that INT is a variable of type float, this statement is valid. One may think that INT is a reserved word and must not be used for other purposes. However, recall that reserved words are express in lowercase, so the C compiler will not interpret this as a reserved word.
63) What are actual arguments?
When you create and use functions that need to perform an action on some given values, you need to pass these given values to that function. The values that are being passed into the called function are referred to as actual arguments.
64) What is a newline escape sequence?
A newline escape sequence is represented by the \n character. This is used to insert a new line when displaying data in the output screen. More spaces can be added by inserting more \n characters. For example, \n\n would insert two spaces. A newline escape sequence can be placed before the actual output expression or after.
65) What is output redirection?
It is the process of transferring data to an alternative output source other than the display screen. Output redirection allows a program to have its output saved to a file. For example, if you have a program named COMPUTE, typing this on the command line as COMPUTE >DATA can accept input from the user, perform certain computations, then have the output redirected to a file named DATA, instead of showing it on the screen.
66) What are run-time errors?
These are errors that occur while the program is being executed. One common instance wherein run-time errors can happen is when you are trying to divide a number by zero. When run-time errors occur, program execution will pause, showing which program line caused the error.
67) What is the difference between functions abs() and fabs()?
These 2 functions basically perform the same action, which is to get the absolute value of the given value. Abs() is used for integer values, while fabs() is used for floating type numbers. Also, the prototype for abs() is under <stdlib.h>, while fabs() is under <math.h>.
68) What are formal parameters?
In using functions in a C program, formal parameters contain the values that were passed by the calling function. The values are substituted in these formal parameters and used in whatever operations as indicated within the main body of the called function.
69) What are control structures?
Control structures take charge at which instructions are to be performed in a program. This means that program flow may not necessarily move from one statement to the next one, but rather some alternative portions may need to be pass into or bypassed from, depending on the outcome of the conditional statements.
70) Write a simple code fragment that will check if a number is positive or negative
71) when is a “switch” statement preferable over an “if” statement.
The switch statement is best used when dealing with selections based on a single variable or expression. However, switch statements can only evaluate integer and character data types.
72) What are global variables and how do you declare them?
Global variables are variables that can be accessed and manipulated anywhere in the program. To make a variable global, place the variable declaration on the upper portion of the program, just after the preprocessor directives section.
73) What are enumerated types?
Enumerated types allow the programmer to use more meaningful words as values to a variable. Each item in the enumerated type variable is actually associated with a numeric code. For example, one can create an enumerated type variable named DAYS whose values are Monday, Tuesday… Sunday.
74) What does the function toupper() do?
It is used to convert any letter to its upper case mode. Toupper() function prototype is declared in <ctype.h>. Note that this function will only convert a single character, and not an entire string.
75) Is it possible to have a function as a parameter in another function?
Yes, that is allowed in C programming. You just need to include the entire function prototype into the parameter field of the other function where it is to be used.
76) What are multidimensional arrays?
Multidimensional arrays are capable of storing data in a two or more dimensional structure. For example, you can use a 2 dimensional array to store the current position of pieces in a chess game, or position of players in a tic-tac-toe program.
77) Which function in C can be used to append a string to another string?
The strcat function. It takes two parameters, the source string and the string value to be appended to the source string.
78) What is the difference between functions getch() and getche()?
Both functions will accept a character input value from the user. When using getch(), the key that was pressed will not appear on the screen, and is automatically captured and assigned to a variable. When using getche(), the key that was pressed by the user will appear on the screen, while at the same time being assigned to a variable.
79) Dothese two program statements perform the same output? 1) scanf(“%c”, &letter); 2) letter=getchar()
Yes, they both do the exact same thing, which is to accept the next key pressed by the user and assign it to variable named letter.
80) What are structure types in C?
Structure types are primarily used to store records. A record is made up of related fields. This makes it easier to organize a group of related data.
81) What does the characters “r” and “w” mean when writing programs that will make use of files?
“r” means “read” and will open a file as input wherein data is to be retrieved. “w” means “write”, and will open a file for output. Previous data that was stored on that file will be erased.
82) What is the difference between text files and binary files?
Text files contain data that can easily be understood by humans. It includes letters, numbers and other characters. On the other hand, binary files contain 1s and 0s that only computers can interpret.
83) is it possible to create your own header files?
Yes, it is possible to create a customized header file. Just include in it the function prototypes that you want to use in your program, and use the #include directive followed by the name of your header file.
84) What is dynamic data structure?
Dynamic data structure provides a means for storing data more efficiently into memory. Using Using dynamic memory allocation , your program will access memory spaces as needed. This is in contrast to static data structure, wherein the programmer has to indicate a fix number of memory space to be used in the program.
85) What are the different data types in C?
The basic data types in C are int, char, and float. Int is used to declare variables that will be storing integer values. Float is used to store real numbers. Char can store individual character values.
86) What is the general form of a C program?
A C program begins with the preprocessor directives, in which the programmer would specify which header file and what constants (if any) to be used. This is followed by the main function heading. Within the main function lies the variable declaration and program statement.
87) What is the advantage of a random access file?
If the amount of data stored in a file is fairly large, the use of random access will allow you to search through it quicker. If it had been a sequential access file, you would have to go through one record at a time until you reach the target data. A random access file lets you jump directly to the target address where data is located.
88) In a switch statement, what will happen if a break statement is omitted?
If a break statement was not placed at the end of a particular case portion? It will move on to the next case portion, possibly causing incorrect output.
89) Describe how arrays can be passed to a user defined function
One thing to note is that you cannot pass the entire array to a function. Instead, you pass to it a pointer that will point to the array first element in memory. To do this, you indicate the name of the array without the brackets.
90) What are pointers?
Pointers point to specific areas in the memory. Pointers contain the address of a variable, which in turn may contain a value or even an address to another memory.
91) Can you pass an entire structure to functions?
Yes, it is possible to pass an entire structure to a function in a call by method style. However, some programmers prefer declaring the structure globally, then pass a variable of that structure type to a function. This method helps maintain consistency and uniformity in terms of argument type.
92) What is gets() function?
The gets() function allows a full line data entry from the user. When the user presses the enter key to end the input, the entire line of characters is stored to a string variable. Note that the enter key is not included in the variable, but instead a null terminator \0 is placed after the last character.
93) The % symbol has a special use in a printf statement. How would you place this character as part of the output on the screen?
You can do this by using %% in the printf statement. For example, you can write printf(“10%%”) to have the output appear as 10% on the screen.
94) How do you search data in a data file using random access method?
Use the fseek() function to perform random access input/ouput on a file. After the file was opened by the fopen() function, the fseek would require three parameters to work: a file pointer to the file, the number of bytes to search, and the point of origin in the file.
95) Are comments included during the compilation stage and placed in the EXE file as well?
No, comments that were encountered by the compiler are disregarded. Comments are mostly for the guidance of the programmer only and do not have any other significant use in the program functionality.
96) Is there a built-in function in C that can be used for sorting data?
Yes, use the qsort() function. It is also possible to create user defined functions for sorting, such as those based on the balloon sort and bubble sort algorithm.
97) What are the advantages and disadvantages of a heap?
Storing data on the heap is slower than it would take when using the stack. However, the main advantage of using the heap is its flexibility. That’s because memory in this structure can be allocated and remove in any particular order. Slowness in the heap can be compensated if an algorithm was well designed and implemented.
98) How do you convert strings to numbers in C?
You can write you own functions to do string to number conversions, or instead use C’s built in functions. You can use atof to convert to a floating point value, atoi to convert to an integer value, and atol to convert to a long integer value.
99) Create a simple code fragment that will swap the values of two variables num1 and num2.
100) what is the use of a semicolon (;) at the end of every program statement.
It has to do with the parsing process and compilation of the code. A semicolon acts as a delimiter, so that the compiler knows where each statement ends, and can proceed to divide the statement into smaller elements for syntax checking.
These interview questions will also help in your viva(orals)
- Bitwise Operators in C: AND, OR, XOR, Shift & Complement
- Dynamic Memory Allocation in C using malloc(), calloc() Functions
- Type Casting in C: Type Conversion, Implicit, Explicit with Example
- C Programming Tutorial PDF for Beginners
- 13 BEST C Programming Books for Beginners (2024 Update)
- Difference Between C and Java
- Difference Between Structure and Union in C
- calloc() Function in C Library with Program EXAMPLE
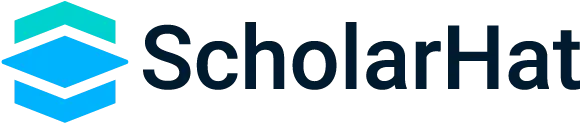
01 Career Opportunities
Top 50 mostly asked c interview questions and answers, 02 beginner.
- Why C is called middle level language?
- If Statement in C
- If-else statement in C Programming
- Understanding realloc() function in C
- Understanding Do while loop in c
- Understanding for loop in C
- if else if statements in C Programming
- Understanding While loop in C
- Beginner's Guide to C Programming
- First C program and Its Syntax
- Escape Sequences and Comments in C
- Keywords in C: List of Keywords
- Identifiers in C: Types of Identifiers
- Data Types in C Programming - A Beginner Guide with examples
- Variables in C Programming - Types of Variables in C ( With Examples )
- 10 Reasons Why You Should Learn C
- Boolean and Static in C Programming With Examples ( Full Guide )
- Operators in C: Types of Operators
- Bitwise Operators in C: AND, OR, XOR, Shift & Complement
- Expressions in C Programming - Types of Expressions in C ( With Examples )
- Conditional Statements in C: if, if..else, Nested if
- Switch Statement in C: Syntax and Examples
- Ternary Operator in C: Ternary Operator vs. if...else Statement
- Loop in C with Examples: For, While, Do..While Loops
- Nested Loops in C - Types of Expressions in C ( With Examples )
- Infinite Loops in C: Types of Infinite Loops
- Jump Statements in C: break, continue, goto, return
- Continue Statement in C: What is Break & Continue Statement in C with Example
03 Intermediate
- Constants in C language
- Functions in C Programming
- Call by Value and Call by Reference in C
- Recursion in C: Types, its Working and Examples
- Storage Classes in C: Auto, Extern, Static, Register
- Arrays in C Programming: Operations on Arrays
- Strings in C with Examples: String Functions
04 Advanced
- How to Dynamically Allocate Memory using malloc() in C?
- How to Dynamically Allocate Memory using calloc() in C?
- Pointers in C: Types of Pointers
- Multidimensional Arrays in C: 2D and 3D Arrays
- Dynamic Memory Allocation in C: Malloc(), Calloc(), Realloc(), Free()
05 Training Programs
- Java Programming Course
- C++ Programming Course
- C Programming Course
- Data Structures and Algorithms Training
- Top 50 Mostly Asked C Int..
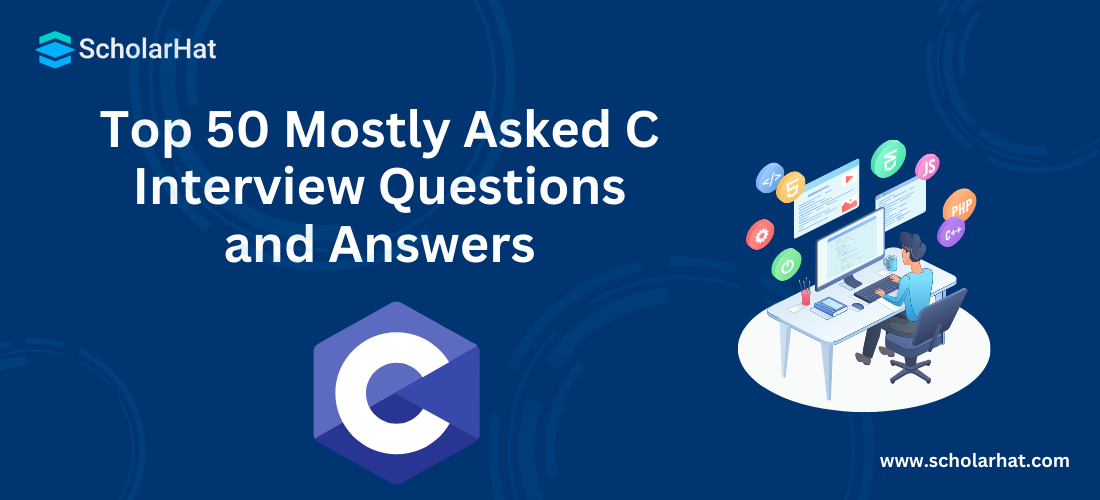
C Programming For Beginners Free Course
C interview questions and answers: an overview.
Are you preparing for a C interview? Feeling excited but quite nervous at the same time? fear not..! This comprehensive guide is here to guide you to demonstrate a solid grasp of C concepts, help you to grow your problem-solving skills, and also make you feel confident to ace your interview.
Why are C Interview Questions Important?
In the Programming world, C remains a foundational language for systems programming, embedded systems, and performance-critical applications. By understanding its complexities, you will conquer half of the programming languages that exist. Interviewers often use C-specific terms to analyze the understanding of your C knowledge such as
- Memory management: C gives you direct control over memory, which can be powerful and challenging. Be prepared to explain concepts like pointers, dynamic allocation, and common memory-related pitfalls.
- Problem-solving and algorithmic thinking: Interviewers want to see how you approach problems analytically, break them down into manageable steps, and write efficient C code to achieve the desired outcome.
- Best practices and coding style: Following good practices demonstrates your professionalism and attention to detail. Be familiar with common C conventions, coding guidelines, and readability tips.
C Interview Questions: A Roadmap to Success
Now that you have a solid understanding of why C interview questions and how to prepare for them, let's see some advanced terms you might encounter in an interview. This guide covers various categories:
- Basics and Syntax: Test your understanding of fundamental C concepts, data types, operators, and control flow.
- Memory Management: Master pointers, dynamic allocation, and memory-related pitfalls.
- Data Structures and Algorithms: Explore common C data structures (arrays, linked lists, stacks, queues, trees, etc.) and demonstrate your ability to implement algorithms using them.
- Advanced Concepts: Get comfortable with preprocessor directives, bitwise operations, unions, structures, and advanced function pointers.
- Problem Solving and Coding: Apply your knowledge to solve real-world programming challenges using efficient and well-structured C code.
Keep in mind for a good communicative interview Sometimes be in the interviewer's shoes too, Don't just answer, ask! Show your genuine interest by crafting insightful questions about the company, role, and team.
Explore the depth of C programming with our comprehensive guide to 50 C Interview Questions and Answers . Whether you're a beginner or an experienced developer, this resource provides valuable insights, helping you master essential concepts and excel in C-related interviews.
C Interview Questions and Answers for Freshers
1. why is c called a mid-level programming language.
C possesses features of both lower-level and higher-level, or assembly-level, languages. C is hence frequently referred to be a middle-level language. One may write both a menu-driven consumer billing system and an operating system in C.
2. What are the features of the C language?
- Middle-level language: Provides low-level memory access and high-level abstractions, balancing efficiency and ease of use.
- Portability: Code written in C can run on different platforms with minimal modifications.
- Efficiency: Offers efficient memory management and execution speed.
- Roch Standard Library: Provides a comprehensive set of functions for various tasks.
- Procedural Language: Follows a structured, step-by-step approach to solving problems.
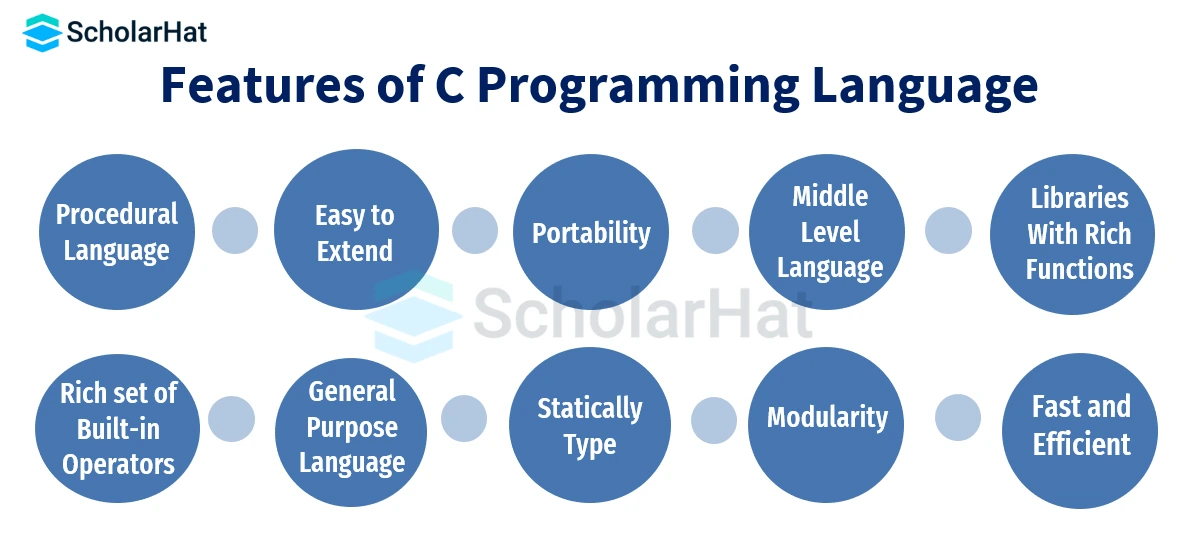
The answer is "f". In C-style languages, the expression 5["zyzuv"] is equivalent to "zyzuv"[5], which accesses the 6th character of the string "zyzuv". Therefore, the value of the expression is "v".
6. What are basic data types supported in the C Programming Language?
In C programming, basic data types include:
- Primitive Data type- There are two other categories of primitive data types: integer and floating.
- User-Defined data types- The user defines these data types to improve program readability.
- Derived data types- Data types that come from pre-built or basic data types.
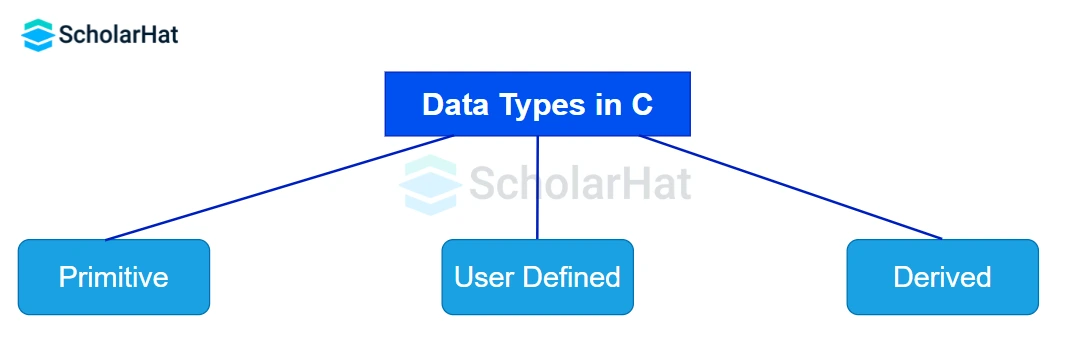
8. What do you mean by the scope of the variable?
In programming languages, scope refers to the block or region where a specified variable will live; if the variable extends outside of that block or region, it will be immediately destroyed. Every variable has a certain range of use. To put it simply, a variable's scope is determined by how long it remains in the program. Three different places can define the variable. They are as follows:
- Local Variables: Located within a certain block or function
- Global Variables: Among all the functions that the program does globally.
- Formal parameters: These are limited to in-function parameters.
9. What is a Preprocessor?
A software application known as a preprocessor is used to handle source files before submitting them to be built. The preprocessor allows for the inclusion of header files, macro expansions, conditional compilation, and line control.
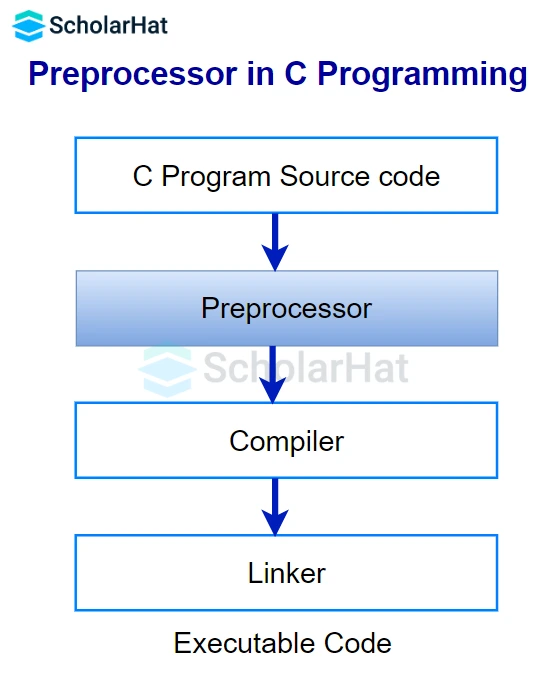
13. What is Recursion in C?
Recursion in C is a programming technique where a function calls itself directly or indirectly to solve a problem. In recursive functions, the function breaks down the problem into smaller subproblems and calls itself with modified arguments until a base case is reached, stopping the recursive calls.
Syntax of recursive function in the C Compiler
14. what is the difference between the local and global variables in c, 15. what is a pointer in c.
A pointer in C is a variable that holds the memory address of another variable. It allows direct manipulation of memory locations, enabling dynamic memory allocation, efficient array handling, and indirect access to data. Pointers are a fundamental feature used for tasks like data structures and dynamic memory management.
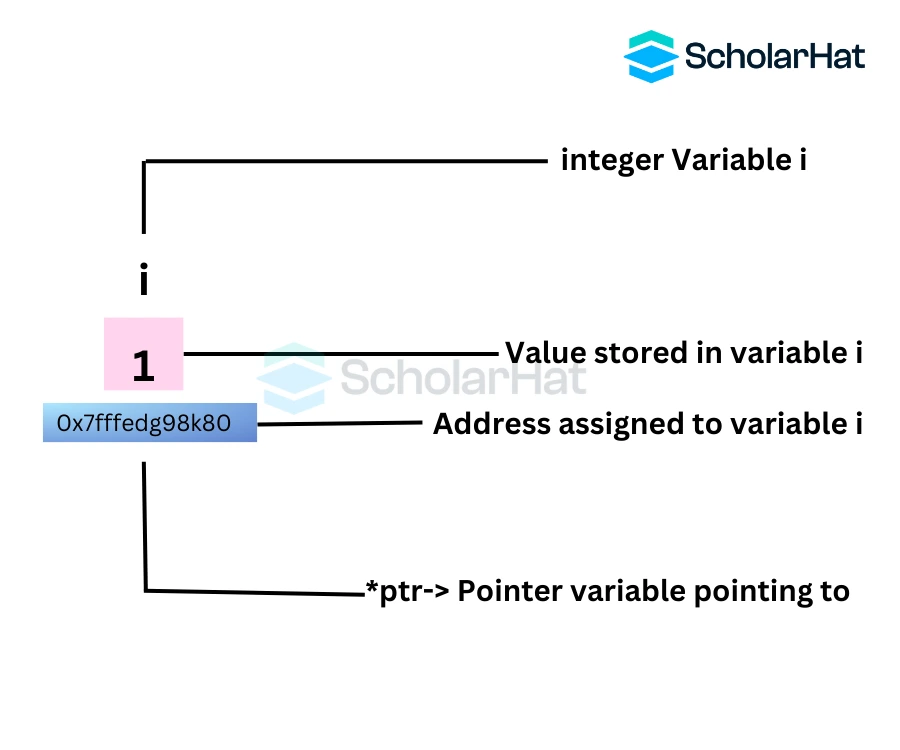
43. Explain Modifiers in C
In the C language, modifiers are keywords that are used to alter the meaning of fundamental data types. They outline how much memory should be set aside for the variable. There are five types of modifiers, which are
44. Write a program to print the factorial of a given number with the help of recursion.
This C program calculates the factorial of a given number using recursion. The factorial function recursively calculates the factorial: n! = n * (n-1)!. In the main function, the program calculates the factorial of 5. The program computes 5! Which is equal to 5 * 4 * 3 * 2 * 1 = 120, and prints the result.
45. What is the difference between #include "..." and #include <...>?
In C programming, both #include "..." and #include <...> are used to include header files, but they differ in their search paths and usage:
#include "..." (Double Quotes): The C preprocessor searches the predefined list of system directories for the filename before checking user-specified directories (directories can be added to the predefined list by using the -I option).
#include <...> (Angle Brackets): The preprocessor follows the search path given for the #include <filename> form after first searching in the same directory as the file containing the directive. Include programmer-defined header files using this way.
Using double quotes ("...") is generally preferred for including project-specific headers, while angle brackets (<...>) are used for standard library or system headers to distinguish between user-defined and system-provided header files.
46. Write a program to check an Armstrong number.
This C program checks if a given number is an Armstrong number or not. It calculates the sum of cubes of its digits and compares it with the original number. If they are equal, it's an Armstrong number. 1^3 + 5^3 + 3^3 = 153, which matches the original number.
47. Merge two sorted Linked Lists in C
In this program, two sorted linked lists (list1 and list2) are merged into a new sorted linked list (mergedList). The merged list is then printed.
48. What is the use of an extern storage specifier?
In the C language, the extern keyword is used to increase the visibility of C variables and functions. External can be shortened to extern. When a specific file wants to access a variable from any other file, it is used. Variables containing the extern keyword are simply declared, not defined, which increases duplication. There is no need to declare or create extern functions because they are visible by default throughout the program.
49. What is the difference between getc(), getchar(), getch() and getche() in C?
- Reads a character from the specified file stream (e.g., stdin, file).
- Requires stdio.h header.
- Offers flexibility for reading from different input sources.
- Reads a character from the standard input stream (stdin).
- Commonly used for simple character input from the keyboard.
- Reads a character from the keyboard without echoing it to the screen.
- Typically used in older MS-DOS and Windows environments.
- Requires conio.h header (non-standard and not portable).
- Reads a character from the keyboard and echoes it to the screen.
- Similar to getch(), but it displays the input character.
Note: getch() and getche() are not standard C functions and are not recommended for portable and modern C programming. Prefer using getc() or getchar() for standard and portable input operations.
50. How is import in Java different from #include in C?
Import is a keyword, but #include is a statement processed by pre-processor software. #include increases in the size of the code.
Related Interview Articles:
- C++ interview questions
- C# interview questions
- Java interview questions
- Javascript Interview Questions
- JQuery Interview Questions
In conclusion, mastering these top 50 C language interview questions is pivotal for aspiring programmers. These insights into fundamental concepts, data structures, and algorithms equip candidates with the knowledge needed to succeed in technical interviews. Practice, understanding, and confidence in these areas will undoubtedly pave the way to a successful C programming career. You can also consider doing our C programming tutorial from ScholarHat to upskill your career.
Q1. What are the different levels of difficulty in C interview questions?
- Beginner: Understanding core concepts like variables, operators, control flow, and functions.
- Intermediate: Pointers, arrays, structures, memory allocation, and recursion.
- Advanced: Algorithms, data structures (linked lists, trees), bit manipulation, and operating system concepts.
Q2. What are some common mistakes candidates make in C interviews?
- Not understanding the question correctly: Listen carefully and rephrase if needed.
- Being unfamiliar with basic C concepts: Brush up on the fundamentals.
- Not explaining their thought process: Talk through your approach even if stuck.
- Poor code quality: Write clean, readable, and well-commented code.
- Lack of confidence: Practice and showcase your enthusiasm for C programming.
Q3. How should I prepare for a C programming interview?
Q4. what types of questions can i expect in a c interview, q5. how can i improve my problem-solving skills for c interview questions, about author.
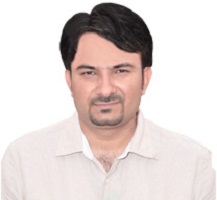
- 22+ Courses
- 750+ Hands-On Labs
- 300+ Quick Notes
- 55+ Skill Tests
- 45+ Interview Q&A
- 10+ Real-world Projects
- Career Coaching
- Email Support
To get full access to all courses
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
Top 100+ C Interview Questions and Answers
Explore our comprehensive guide to the top 100+ C interview questions and answers, designed to help you ace your interviews with confidence and expertise.
C Interview Questions and Answers provides a comprehensive collection of questions and answers for individuals preparing for interviews in the software development field, specifically focusing on the C programming language. C Interview Questions cover a wide range of topics, including syntax, data types, control structures, functions, pointers, memory management, and algorithms.
Basic C Interview Questions and Answers
Basic C Interview Questions cover essential topics and concepts in the C programming language, aiming to prepare individuals for job interviews in the field of software development. Basic C Interview Questions include questions on data types, control structures, syntax, pointers, functions, and memory management, all fundamental to mastering C programming.
What is C programming language?
View Answer
Hide Answer
C programming language is a general-purpose, procedural computer programming language supporting structured programming, lexical variable scope, and recursion, with a static type system.
How do you declare a variable in C?
In Cdeclared a variable by specifying its type followed by the variable name; for example, int myVariable;.
What are the data types available in C?
The data types available in C include int, float, double, char, and void, along with derived types such as arrays, pointers, structures, and unions.
What is the purpose of the main() function in C?
The purpose of the main() function in C is to serve as the entry point for program execution.
How do you print output to the console in C?
Output is printed to the console in C using the printf function.
What is the difference between == and = in C?
In C, == is the equality operator used to compare two values. The = is the assignment operator used to assign a value to a variable.
How do you read input from the user in C?
Input from the user is read in C using the scanf function.
What are loops in C, and what types are there?
Loops in C are used to execute a block of code repeatedly, with the types being for, while, and do-while loops.
How do you comment code in C?
Code is commented in C using // for single-line comments and /* */ for multi-line comments.
What is an array in C, and how is it declared?
An array in C is a collection of items stored at contiguous memory locations, declared by specifying the type of elements followed by the array name and size in square brackets; for example, int myArray[10];.
What is a pointer in C, and why is it used?
A pointer in C is a variable that stores the memory address of another variable. Pointers are used for various purposes, including dynamic memory allocation, arrays, functions, and data structures.
How do you perform arithmetic operations in C?
Arithmetic operations in C are performed using operators such as + for addition, - for subtraction, * for multiplication, / for division, and % for modulus.
What is a function in C, and how do you declare one?
A function in C is a block of code that performs a specific task, declared with a return type, function name, and parameters if any, followed by a body enclosed in braces; for example, int myFunction(int param1, int param2).
What are conditional statements in C?
Conditional statements in C, such as if, else if, else, and switch, control the flow of execution based on specified conditions.
What is a structure in C, and how do you define it?
A structure in C is a user-defined data type that allows to combine data items of different kinds. Structures are defined using the struct keyword followed by the structure name and curly braces enclosing the member definitions.
How do you use the sizeof operator in C?
The sizeof operator in C is used to determine the size, in bytes, of a data type or a variable.
What is the difference between while and do-while loops in C?
The while loop checks the condition before executing the loop body. The do-while loop checks the condition after executing the loop body at least once.
How do you concatenate strings in C?
Strings are concatenated in C using the strcat function, which appends the source string to the destination string.
What is recursion in C, and how do you implement it?
Recursion in C is a technique where a function calls itself directly or indirectly. It is implemented by defining a base case for termination and a recursive case where the function calls itself with a different argument.
How do you handle errors in C programming?
Errors in C programming are handled using error codes returned by functions, errno, and the perror function for reporting error messages to the standard error.
What are command-line arguments in C?
Command-line arguments in C are parameters passed to the program at the time of execution from the command line, accessible within the program through the argc and argv parameters of the main() function.
What is the scope of a variable in C?
The scope of a variable in C determines the part of the program where the variable can be accessed. The scope can be local (inside a block or function) or global (throughout the program).
How do you allocate memory dynamically in C?
Memory is allocated dynamically in C using the malloc, calloc, or realloc functions, which return a pointer to the allocated memory.
What is the use of the const keyword in C?
The const keyword in C is used to declare variables whose value cannot be modified after initialization, making them constant.
How do you declare a global variable in C?
A global variable in C is declared outside of all functions, typically at the top of the program file, making it accessible throughout the program.
Intermediate-level C Interview Questions and Answers
Intermediate C Interview Questions delve into the complexities of the C programming language, targeting programmers with a foundational grasp looking to deepen their understanding. Intermediate C Interview Questions explore nuanced topics such as memory management, data structures, pointers, and algorithms within C.
How do you use pointers to a function in C?
In C, pointers to a function are used by first declaring a pointer with the syntax that mirrors the function it intends to point to, including the return type and parameters. Then, the address of a function is assigned to the pointer. This allows the program to call the function through the pointer, enabling callbacks and higher-order functions.
What is the difference between stack and heap memory allocation in C?
Stack memory allocation in C is managed by the compiler, automatically allocating and deallocating memory as functions are called and returned. It is fast and efficient but limited in size. Heap memory allocation is controlled by the programmer using functions like malloc() and free(), offering dynamic memory management for larger or variable-sized data but requiring manual management to avoid memory leaks.
Can you explain the concept of file handling in C?
File handling in C involves using a set of functions, such as fopen(), fread(), fwrite(), fclose(), etc., to open, read, write, and close files. These operations allow C programs to interact with files stored on the disk, enabling data persistence beyond the program's execution.
How do you use typedef in C for better code readability?
Typedef in C is used to create alias names for existing data types, enhancing code readability and simplifying complex type declarations. It is particularly useful for struct definitions, allowing programmers to refer to them with a single, concise name.
What are volatile variables in C, and when should they be used?
Volatile variables in C are used to tell the compiler that the value of the variable can change at any time, without any action being taken by the code the compiler finds nearby. They are essential in embedded systems or when dealing with hardware where the state can change independently of the program flow, ensuring the compiler does not optimize away direct accesses to them.
How do you implement linked lists in C?
Linked lists in C are implemented by defining a struct that contains at least one member variable pointing to the next node in the list and other data fields. Dynamic memory allocation is used to create new nodes, and pointers are adjusted to add, remove, or traverse the elements in the list.
What is a union in C, and how does it differ from a structure?
A union in C is a data type that allows storing different data types in the same memory location. It differs from a structure because, in a union, only one member can contain a value at any given time, sharing the memory space, whereas in a structure, each member has its own memory location.
How do you perform bit manipulation in C?
Bit manipulation in C is performed using bitwise operators such as AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>). These operators allow direct manipulation of individual bits in an integer's binary representation, enabling efficient data storage and manipulation.
What are static variables in C, and how do they behave?
Static variables in C retain their value between function calls and are initialized only once at compile time. They have a scope where they are defined but persist for the lifetime of the program, making them useful for maintaining the state within a function or across function calls.
How do you use the extern keyword in C?
The extern keyword in C is used to declare a variable or function in another file, indicating to the compiler that the definition is externally defined. It allows for the sharing of variables or functions across multiple files within the same program, facilitating modular programming.
What are the differences between malloc() and calloc() in C?
Malloc() allocates a single block of memory of a specified size, without initializing the memory. Calloc(), on the other hand, allocates memory for an array of elements, initializing all bytes to zero. Both functions return a pointer to the allocated memory, requiring free() to deallocate it.
How can you prevent buffer overflow in C?
To prevent buffer overflow in C, use safe functions that limit the amount of data copied to buffers, such as strncpy() instead of strcpy() and snprintf() instead of sprintf(). Also, always validate the size of inputs and perform bounds checking to ensure data does not exceed buffer limits.
What is a macro in C, and how is it defined?
A macro in C is defined using the #define directive, allowing the creation of constants and function-like macros that perform text substitution before compilation. Macros facilitate code reuse and can make conditional compilation and inline code substitutions more convenient.
How do you implement error handling with errno in C?
Error handling with errno in C involves checking the global variable errno after function calls that can fail. If a function indicates an error, errno will be set to a specific error code, which can be interpreted using perror() or strerror() to display or log a human-readable error message.
What is the significance of the register keyword in C?
The register keyword in C suggests to the compiler that the variable should be stored in a register instead of RAM to optimize access speed. It is a hint, and the compiler may choose to ignore it based on the hardware and optimization settings.
How do you create and manipulate a two-dimensional array in C?
A two-dimensional array in C is created by specifying the size of both dimensions in the declaration. Elements are accessed using row and column indices, and the array can be manipulated by iterating through elements using nested loops for operations like input, output, or algorithmic processing.
What are command-line arguments in C and how do you process them?
Command-line arguments in C are processed using the parameters of the main function, argc for the argument count, and argv, an array of character pointers listing all the arguments. They enable the user to pass runtime arguments to the program, which can be accessed by iterating through argv.
How do you implement a binary tree in C?
Implementing a binary tree in C involves defining a struct for a tree node, which contains data and pointers to the left and right child nodes. Functions are then created to insert, delete, search, and traverse (in-order, pre-order, post-order) the tree nodes.
What is the use of the static keyword in function definitions?
The use of the static keyword in function definitions in C limits the function's scope to the file in which it is defined, making it inaccessible from other files. This is useful for encapsulating functions within a module, enhancing modularity, and preventing namespace collisions.
How do you debug a C program?
Debugging a C program involves using a debugger tool like gdb to step through the code, examine variable values, set breakpoints, and track down the source of errors. Analyzing compiler warnings and using print statements for tracking program flow and variables' states are also common practices.
What are the implications of using goto in C?
Using goto in C can lead to spaghetti code, making the program hard to read, maintain, and debug due to its unstructured jumping from one part of the code to another. It is generally advised to use structured control flow constructs like loops and conditionals instead.
How do you use qsort() function in C?
The qsort() function in C sorts an array of elements using the quicksort algorithm. It requires the base address of the array, the number of elements, the size of each element, and a comparison function that determines the order of sorting. This function allows for efficient and flexible sorting of data.
What are preprocessor directives in C, and how are they used?
Preprocessor directives in C, indicated by the # symbol, include commands like #define, #include, #ifdef, and #ifndef. They are used for defining constants, including header files, and conditional compilation. These directives are processed before compilation, controlling the compilation process and code inclusion.
How do you manage memory leaks in C programs?
Managing memory leaks in C programs involves ensuring that every dynamically allocated memory block with malloc(), calloc(), or realloc() is properly deallocated using free() once it is no longer needed. Tools like Valgrind can help identify memory leaks by analyzing the program's usage.
What is the difference between memcpy() and memmove() in C?
The difference between memcpy() and memmove() in C is that memcpy() assumes that the source and destination memory blocks do not overlap, potentially leading to undefined behavior if they do. Memmove(), however, safely handles overlapping memory blocks, making it a safer choice for moving memory when overlap is possible.
Advanced C Interview Questions and Answers
Advanced C Interview Questions provides an in-depth exploration of complex topics related to the C programming language, designed for individuals seeking to demonstrate their proficiency in this foundational coding language during technical interviews.
How do you optimize C code for memory usage and performance?
Optimizing C code for memory usage involves minimizing variable scope, using data types efficiently, and freeing unused memory. For performance focus on loop optimization, minimizing function calls, and leveraging compiler optimizations. Profiling tools assist in identifying bottlenecks.
What are the implications of using recursive functions in C?
Using recursive functions in C increases stack memory usage, which can lead to stack overflow if not carefully managed. Recursive functions simplify code for problems that naturally fit recursion, such as tree traversals.
Can you explain the process of multi-threading in C and its challenges?
Multi-threading in C involves creating, executing, and managing concurrent threads within a process. Challenges include data synchronization, deadlocks, and race conditions, requiring careful design to ensure thread safety.
How do you implement a mutex in C for thread synchronization?
Implementing a mutex in C for thread synchronization involves using the pthread_mutex_init, pthread_mutex_lock, pthread_mutex_unlock, and pthread_mutex_destroy functions from the POSIX threads library to protect shared data and prevent race conditions.
What is the role of volatile keywords in multi-threaded C programs?
The volatile keyword in multi-threaded C programs prevents the compiler from optimizing away reads and writes to a variable that can change outside the program's control, ensuring memory visibility and ordering between threads.
How do you detect and avoid memory leaks in C applications?
Detecting and avoiding memory leaks in C applications involves using dynamic analysis tools, such as Valgrind, to monitor memory allocation and deallocation. Programmers must ensure every malloc call has a corresponding free call.
What are function pointers and how are they used in C?
Function pointers in C store the address of functions, allowing the dynamic invocation of functions based on runtime conditions. They enable callbacks and passing functions as arguments to other functions.
How can you dynamically load libraries in C?
Dynamically loading libraries in C uses the dlopen, dlsym, dlclose functions from the dlfcn.h header, allowing applications to load shared libraries at runtime and access their symbols.
What is a segmentation fault and how do you debug it in C programs?
A segmentation fault occurs when a C program tries to access memory it is not allowed to. Debugging involves using tools like gdb to trace the faulting access and examining pointer operations and memory allocations.
Can you explain the concept of endianness in C programming?
Endianness in C programming refers to the order in which bytes are stored for multi-byte data types. Big endian stores the most significant byte first, whereas little endian stores the least significant byte first. Understanding endianness is crucial for data serialization and working with different hardware architectures.
How do you implement custom memory allocation functions in C?
Implementing custom memory allocation functions in C involves creating functions that utilize the existing memory allocation APIs (malloc, free) to provide specialized behavior, such as tracking usage, debugging memory leaks, or implementing a memory pool.
What is the use of setjmp and longjmp in C?
The use of setjmp and longjmp in C provides a way to perform non-local jumps, allowing programs to jump from a point in the code to a previously saved state using setjmp, typically used for error handling and implementing coroutines.
How do you use signal handling in C?
Signal handling in C involves defining custom signal handlers using the signal or sigaction functions to catch and respond to asynchronous events or interrupts, allowing programs to gracefully manage unexpected conditions.
Can you explain how to use socket programming in C?
Socket programming in C involves using the socket API to create network connections, enabling communication between processes over TCP/IP. This process includes creating sockets, connecting, sending, and receiving data over the network.
What are the differences between process and thread in C context?
In the C context, a process is an execution environment with its own memory space, while a thread is a lighter, shareable unit of execution within a process. Threads share the same memory space within their parent process, allowing for efficient communication and shared resource access.
How do you ensure cross-platform compatibility of your C code?
Ensuring cross-platform compatibility of C code involves adhering to standard C language features, using conditional compilation for platform-specific code, and relying on portable libraries to abstract away operating system differences.
What is the purpose of the _Atomic keyword in C?
The purpose of the _Atomic keyword in C is to define atomic types, ensuring operations on such types are performed as indivisible units, critical for thread-safe programming to prevent race conditions without explicit locking.
How do you use conditional compilation in C?
Conditional compilation in C uses preprocessor directives (e.g., #if, #ifdef, #ifndef) to include or exclude parts of the code based on specific conditions, facilitating platform-specific code paths or feature toggles.
What are the best practices for writing secure C code?
Best practices for writing secure C code include validating all input, avoiding dangerous functions prone to buffer overflows (e.g., strcpy, strcat), using secure functions (e.g., strncpy, strncat), and performing regular security audits and static code analysis.
How do you interface C programs with other languages?
Interfacing C programs with other languages involves using foreign function interfaces (FFIs) or application programming interfaces (APIs) provided by those languages, along with creating wrapper functions or libraries that expose C functionality in a manner compatible with the target language's calling conventions.
What are the challenges of embedded programming in C?
Challenges of embedded programming in C include dealing with limited hardware resources, real-time constraints, cross-compilation, and ensuring system reliability and stability in constrained environments.
How do you implement a garbage collector in C?
Implementing a garbage collector in C involves creating routines to track memory allocation and usage, identifying unused objects, and reclaiming memory automatically, often through techniques like reference counting or tracing garbage collection.
What techniques do you use for parsing complex data in C?
Parsing complex data in C involves using parsing algorithms, such as finite state machines, recursive descent parsers, or utilizing existing libraries like flex and bison to convert input data into structured, accessible formats.
How can you optimize C code for CPU cache utilization?
Optimizing C code for CPU cache utilization involves organizing data and access patterns to maximize cache hits, such as using data locality, loop transformations, and cache-friendly data structures.
What is the role of context switching in C, and how is it managed?
The role of context switching in C, typically managed by the operating system, involves saving the state of a running thread or process and restoring the state of another, enabling multitasking by switching between different execution contexts efficiently.
C Pointers Interview Questions and Answers
C Pointers Interview Questions is a comprehensive guide that addresses various aspects of using pointers in the C programming language. C Pointers Interview Questions cover fundamental concepts, such as the definition and use of pointers, to more advanced topics like pointer arithmetic, the relationship between arrays and pointers, and the use of pointers with functions and structures.
What is a pointer in C, and why are they used?
A pointer in C is a variable that stores the memory address of another variable. Pointers are used to directly access or manipulate the data stored in memory locations.
How do you declare and initialize a pointer in C?
Declare a pointer in C by specifying the data type of the variable to which it points, followed by an asterisk before the pointer's name. You initialize a pointer by assigning it the address of a variable using the address-of operator (&).
What is the difference between * and & operators in C?
The asterisk (*) operator, when used with a pointer, dereferences the pointer to access the value at the memory address it points to. The ampersand (&) operator is used to obtain the memory address of a variable.
How do you access the value of the variable to which the pointer points?
You access the value of the variable to which the pointer points by using the dereference operator (*), followed by the pointer name.
What are pointer arithmetic operations, and how do they work?
Pointer arithmetic operations include addition, subtraction, increment, and decrement operations on pointers. These operations adjust the pointer's value based on the data type it points to, moving it to point to subsequent or previous memory locations accordingly.
Can you explain the concept of a null pointer in C?
A null pointer in C is a pointer that does not point to any memory location. It is initialized with the value NULL, indicating that it is not intended to point to data.
What happens when you perform arithmetic operations on a null pointer?
Performing arithmetic operations on a null pointer results in undefined behavior, as the null pointer does not point to a valid memory location.
How do you pass a pointer to a function in C?
You pass a pointer to a function in C by specifying the pointer as a parameter in the function definition and then passing the address of a variable to the function call.
What is a pointer to a pointer in C, and how is it useful?
A pointer to a pointer in C is a variable that stores the address of another pointer. It is useful for dynamic data structures like linked lists and for managing multidimensional arrays.
How do you dynamically allocate memory using pointers in C?
You dynamically allocate memory using pointers in C by using memory allocation functions such as malloc() or calloc(), which return a pointer to the allocated memory.
What is the importance of free() function in C, and when should it be used?
The free() function in C is important for releasing memory that was previously allocated dynamically using malloc() or calloc(). It should be used when the dynamically allocated memory is no longer needed to prevent memory leaks.
How can pointer misuse lead to security vulnerabilities in C programs?
Pointer misuse, such as dereferencing uninitialized pointers or performing out-of-bounds access, can lead to security vulnerabilities like buffer overflows, allowing attackers to exploit C programs.
What is the difference between malloc() and calloc() functions in relation to pointers?
The malloc() function allocates a block of memory without initializing it, while calloc() allocates memory and initializes all bits to zero, both returning a pointer to the allocated memory.
How do you use pointers with arrays and what advantages does it offer?
Pointers can be used to iterate through an array by incrementing the pointer to access subsequent elements. This offers advantages in efficiency and simplicity for traversing arrays.
Can you explain the relationship between arrays and pointers in C?
In C, arrays and pointers are closely related, as the name of an array can be used as a pointer to its first element, and pointers can perform array-like operations.
What are function pointers, and how are they utilized in C?
Function pointers in C are pointers that point to functions. They are utilized to invoke functions dynamically, allow passing of functions as arguments to other functions, and enable callback mechanisms.
How do you create a linked list using pointers in C?
You create a linked list using pointers in C by defining a struct for the list nodes that includes data fields and a pointer to the next node, and then dynamically allocating memory for each node while linking them together using the pointers.
What is the significance of using pointers in structures in C?
Using pointers in structures in C allows for dynamic memory allocation for the structure's fields, enabling the creation of flexible and memory-efficient data structures like linked lists and trees.
How do pointers to functions enhance the flexibility of C code?
Pointers to functions enhance the flexibility of C code by enabling functions to be passed as arguments to other functions, facilitating callback mechanisms, and allowing the dynamic invocation of functions.
What precautions should be taken when handling pointers in C to avoid memory leaks?
Precautions include ensuring that every malloc() or calloc() call is paired with a free() call, avoiding dereferencing uninitialized or null pointers, and carefully managing pointer arithmetic to prevent accessing out-of-bounds memory locations.
Design Pattern C Interview Questions and Answers
Design Pattern C Interview Questions focus on evaluating the understanding and application of design patterns in the context of the C programming language. Design Pattern C Interview Questions cover a range of topics, including the implementation of design patterns such as Singleton, Factory, Observer, and Strategy in C.
Can you explain the Singleton design pattern and its implementation in C?
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to it. In C, this pattern is implemented using static variables to store the instance and a function that creates and returns this instance if it does not already exist. The Singleton pattern is useful for managing resources such as database connections.
How do you apply the Factory design pattern in a C program?
The Factory design pattern in C involves defining an interface for creating an object but lets subclasses decide which class to instantiate. This pattern is applied by defining a function pointer in C that represents the factory method and using conditional statements to determine the type of object to create and return.
What is the Observer design pattern, and how can it be used in C?
The Observer design pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. In C, this pattern can be used by maintaining a list of observer pointers and iterating through this list to notify all observers when a change occurs.
Can you describe the implementation of the Strategy design pattern in C?
The Strategy design pattern in C allows the algorithm's behavior to be selected at runtime by defining a family of algorithms, encapsulating each one, and making them interchangeable. This pattern is implemented using function pointers that represent different strategies and a context structure that includes a strategy pointer to the currently selected algorithm.
How is the Adapter design pattern used in C programming?
The Adapter design pattern in C programming makes interfaces of incompatible classes compatible without changing their source code. This pattern is achieved by creating a new structure that wraps the incompatible class and provides an interface expected by the client.
What are the benefits of using the Composite design pattern in C?
The Composite design pattern in C allows clients to treat individual objects and compositions of objects uniformly. This pattern simplifies client code and makes it easier to add new kinds of components by organizing objects into tree structures to represent part-whole hierarchies.
How do you implement the Decorator design pattern in C?
The Decorator design pattern in C adds new responsibilities to objects dynamically by wrapping them in helper structures without altering their interfaces. This pattern is implemented by defining a base structure and specific decorator structures that add functionality and call the base object's function.
Can you explain the use of the Proxy design pattern in C?
The Proxy design pattern in C provides a surrogate or placeholder for another object to control access to it. This pattern is used by creating a structure that contains a pointer to the real object and methods that delegate calls to this object, potentially adding additional logic such as access control or lazy initialization.
How is the Command design pattern implemented in C?
The Command design pattern in C encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. This pattern is implemented by defining a command structure with a function pointer for executing the command and a client that creates and executes commands.
What is the State design pattern, and how can it be applied in C?
The State design pattern in C allows an object to alter its behavior when its internal state changes. This pattern is applied by defining state interface structures with function pointers for state-specific behaviors and a context structure that maintains a pointer to the current state structure.
How do you use the Template Method design pattern in C?
The Template Method design pattern in C defines the skeleton of an algorithm in an operation, deferring some steps to client subclasses. This pattern is used by defining abstract operations with function pointers in a base structure and letting derived structures implement these operations.
Can you implement the Iterator design pattern in C? How?
The Iterator design pattern in C provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. This pattern is implemented by creating an iterator structure with function pointers for accessing and traversing elements and an aggregate structure that creates and returns an appropriate iterator.
What challenges do you face when implementing design patterns in C?
Implementing design patterns in C poses challenges such as managing memory allocation and deallocation manually, ensuring type safety without strong object-oriented support, and dealing with the lack of namespace and templates which can complicate the implementation of complex patterns.
How can the Chain of Responsibility design pattern be applied in C?
The Chain of Responsibility design pattern in C passes the request along a chain of handlers until one of them handles the request. This pattern is applied by defining a handler structure with a function pointer for handling requests and a pointer to the next handler in the chain.
Can you describe a scenario where the Bridge design pattern is useful in C?
The Bridge design pattern in C decouples an abstraction from its implementation so that the two can vary independently. This pattern is useful in scenarios where an application needs to support multiple platform-specific implementations without changing the high-level abstraction code.
How do you ensure memory management when using design patterns in C?
Ensuring memory management when using design patterns in C involves careful allocation and deallocation of memory for objects to prevent memory leaks and ensure efficient use of resources. This requires explicit calls to malloc and free for dynamic memory management and careful tracking of object lifecycles.
What is the role of design patterns in embedded systems programming in C?
Design patterns play a crucial role in embedded systems programming in C by providing proven solutions for common problems, such as resource management, interface abstraction, and system modularity, which are critical in resource-constrained and specialized embedded environments.
How can the Mediator design pattern be used in C to simplify object communication?
The Mediator design pattern in C simplifies object communication by reducing direct connections between objects and letting them communicate indirectly through a mediator object. This pattern is used by creating a mediator structure that encapsulates the interactions between a set of objects.
Can you give an example of the Memento design pattern in C?
The Memento design pattern in C allows the state of an object to be saved and restored without exposing its implementation details. An example involves creating a memento structure that stores the state of another structure and a caretaker structure that manages the memento's lifecycle.
What is the Builder design pattern, and how is it implemented in C?
The Builder design pattern in C separates the construction of a complex object from its representation, allowing the same construction process to create different representations. This pattern is implemented by defining a builder interface with function pointers for each step of the construction process and a director function that executes these steps to construct the object.
Interview Resources
Want to upskill further through more interview questions and resources? Check out our collection of resources curated just for you.
Browse Flexiple's talent pool
While you build a world-class company, we help you assemble a dream tech team. Because, you deserve it.
- Programmers
- React Native
- Ruby on Rails
- Other Developers
Table of Contents
Basic c programming interview questions, intermediate c programming interview questions, advanced c programming interview questions, c programming interview questions and answers [top 30].
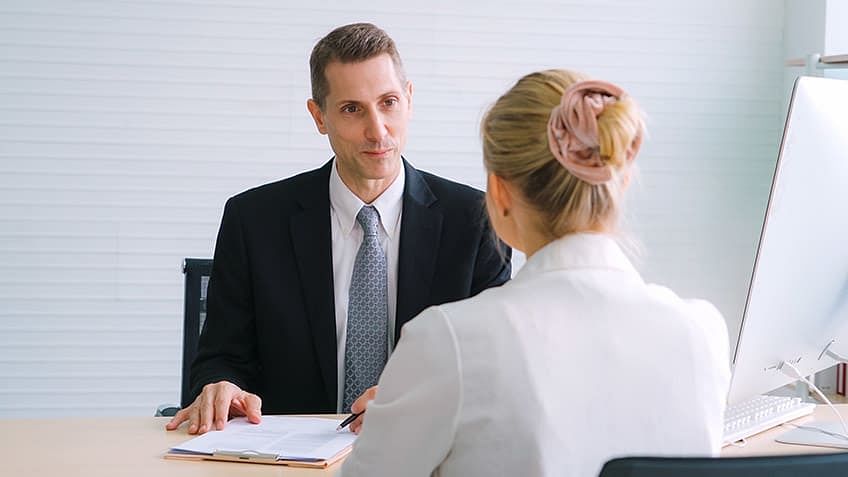
C programming interview questions are a part of most technical rounds conducted by employers. The main goal of questioning a candidate on C programming is to check his/her knowledge about programming and core concepts of the C language. In this article, you will find a mix of C language interview questions designed specially to give you a foundation and build on it. And before going ahead, if you want to know more about C programming, check out what is c programming now?
Let’s start with some basic interview questions on c language:
1. What do you understand by calloc()?
calloc() is a dynamic memory allocation function that loads all the assigned memory locations with 0 value.
2. What happens when a header file is included with-in double quotes ““?
When a header file in c++ is included in double-quotes, the particular header file is first searched in the compiler’s current working directory. If not found, then the built-in include path is also searched.
Want a Top Software Development Job? Start Here!
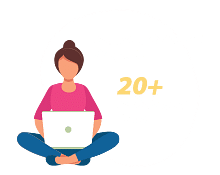
3. Define <stdio.h>.
It is a header file in C that contains prototypes and definitions of commands such as scanf and printf.[2] [MOU3]
4. One of the most common c language interview questions is to define the What is the use of static functions.?
When we want to restrict access to functions, we need to make them static. Making functions static allows us to reuse the same function in C programming name in multiple files.
5. Name the four categories in which data types in the C programming language are divided in.
Basic data types - Arithmetic data types, further divided into integer and floating-point types
Derived datatypes -Arithmetic data types that define variables and assign discrete integer values only
Void data types - no value is available
Enumerated data types -Array types, pointer types, function, structure and union types
6. What is the function of s++ and ++s?
s++ is a single machine instruction used to increment the value of s by 1. (Post increment). ++s is used to carry out pre-increment.
7. What is the use of the ‘==’ symbol?
The ‘==’ symbol or “equivalent to” or “equal to” symbol is a relational operator, i.e., it is used to compare two values or variables.
8. What is the output of the following code snippet?
#include <stdio.h>
void local_static()
static int a;
printf("%d ", a);
local_static();
9. Mention some of the features of the C programming language.
Some of the feature are:
Middle-Level Language - Combined form of both high level language and assembly language
Pointers - Supports pointers
Extensible - Easy to add features to already written program
Recursion - Supports recursion making programs faster
Structured Language - It is a procedural and general purpose language.
10. Name a ternary operator in the C programming language.
The conditional operator (?:)
Here are some frequently asked intermediate interview questions on C language!
1. Why is int known as a reserved word?
As int is a part of standard C language library, and it is not possible to use it for any other activity except its intended functionality, it is known as a reserved word.
2. This is one of the most commonly asked C language interview questions. What will this code snippet return?
void display(unsigned int n)
if(n > 0)
display(n-1);
printf("%d ", n);
Prints number from 1 to n.
3. Another frequent c interview question is what is meant by Call by reference?
When a variable’s value is sent as a parameter to a function, it is known as call by reference. The process can alter the value of the variable within the function.
4. What information is given to the compiler while declaring a prototype function?
The following information is given while declaring a prototype function:
- Name of the function
- Parameters list of the function
- Return type of the function.[6]
5. Why are objects declared as volatile are omitted from optimization?
This is because, at any time, the values of the objects can be changed by code outside the scope of the current code.
6. Give the equivalent FOR LOOP format of the following:
while (a<=10) {
printf ("%d\n", a * a);
for (a=0; a<=10; a++)
7. What is a modifier in the C programming language? Name the five available modifiers.
It is used as a prefix to primary data type to indicate the storage space allocation’s modification to a variable. The available modifiers are:
8. A pointer *a points to a variable v. What can ‘v’ contain?
Pointers is a concept available in C and C++ . The variable ‘v’ might contain the address of another memory or a value.
9. What three parameters fseek() function require to work after the file is opened by the fopen() function?
The number of bytes to search, the point of origin of the file, and a file pointer to the file.
10. Name a type of entry controlled and exit controlled loop in C programming.
Entry controlled loop- For loop (The condition is checked at the beginning)
Exit Controlled loop- do-while loop.[7] (The condition is checked in the end, i.e. loop runs at least once)
Preparing Your Blockchain Career for 2024
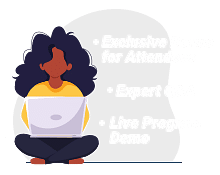
In the next section, we will go through some of the advanced interview questions on C programming:
1. Give the output of the following program:
#include <stdio.h>
int main(void)
int arr[] = {20,40};
int *a = arr;
printf("arr[0] = %d, arr[1] = %d, *a = %d",
arr[0], arr[1], *a);
arr[0] = 20, arr[1] = 40, *p = 40
2. One of the frequently asked c interview questions could be to explain Canif you can we free a block of memory that has been allocated previously? If yes, how?
A block of memory previously allocated can be freed by using free(). The memory can also be released if the pointer holding that memory address is: realloc(ptr,0).
3. How to declare a variable as a pointer to a function that takes a single character-pointer argument and returns a character?
char (*a) (char*);
4. What is the stack area?
The stack area is used to store arguments and local variables of a method. It stays in memory until the particular method is not terminated.
5. What is the function of the following statement?
sscanf(str, “%d”, &i);
To convert a string value to an integer value.
6. Will the value of ‘a’ and ‘b’ be identical or different? Why?
float num = 1.0;
int a = (int) num;
int b = * (int *) #
The variable stores a value of num that has been first cast to an integer pointer and then dereferenced.
7. What are huge pointers?
Huge pointers are 32-bit pointers that can be accessed outside the segment, and the segment part can be modified, unlike far pointers.
8. What will be the output of the following?
#include<stdio.h>
char *a = "abc";
a[2] = 'd';
printf("%c", *a);
The program will crash as the pointer points to a constant string, and the program is trying to change its values.
9. What is a union?
A union is a data type used to store different types of data at the exact memory location. Only one member of a union is helpful at any given time.
10. How is import in Java different from #include in C?
Import is a keyword, but #include is a statement processed by pre-processor software. #include increases the size of the code.
Here’s The Next Step
Go through your self-made notes while preparing for the interview. As a fresher, you aren’t expected to answer complex questions but answer what you know with confidence. One of the essential uses of C programming is full-stack development. If that’s a position you aim to crack in your following interview, check out this comprehensively curated course by Simplilearn and kickstart your web development career now!
If you are looking for a more comprehensive certification course that covers the top programming languages and skills needed to become a Full Stack developer today, Simpliearn’s Post Graduate Program in Full Stack Web Development in collaboration with Caltech CTME should be next on your list. This global online bootcamp offers work-ready training in over 30 in-demand skills and tools. You also get to immediately practice what you learn with 20 lesson-end, 5 phase-end and a Capstone project in 4 domains. This needs to be the next goal in your learning and career journey.
Our Software Development Courses Duration And Fees
Software Development Course typically range from a few weeks to several months, with fees varying based on program and institution.
Recommended Reads
Kubernetes Interview Guide
Top R Programming Interview Questions and Answers
Top 60 C++ Interview Questions and Answers for 2024
Apache Spark Interview Guide
Top 30 C# OOPS Interview Questions and Answers for 2024
180+ Core Java Interview Questions and Answers for 2024
Get Affiliated Certifications with Live Class programs
Post graduate program in full stack web development.
- Live sessions on the latest AI trends, such as generative AI, prompt engineering, explainable AI, and more
- Caltech CTME Post Graduate Certificate
Full Stack Web Developer - MEAN Stack
- Comprehensive Blended Learning program
- 8X higher interaction in live online classes conducted by industry experts
- PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc.
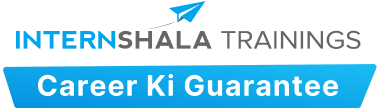
Your favourite senior outside college
Home » Programming » C Interview Questions
Top 50+ C Interview Questions – The 2024 Guide
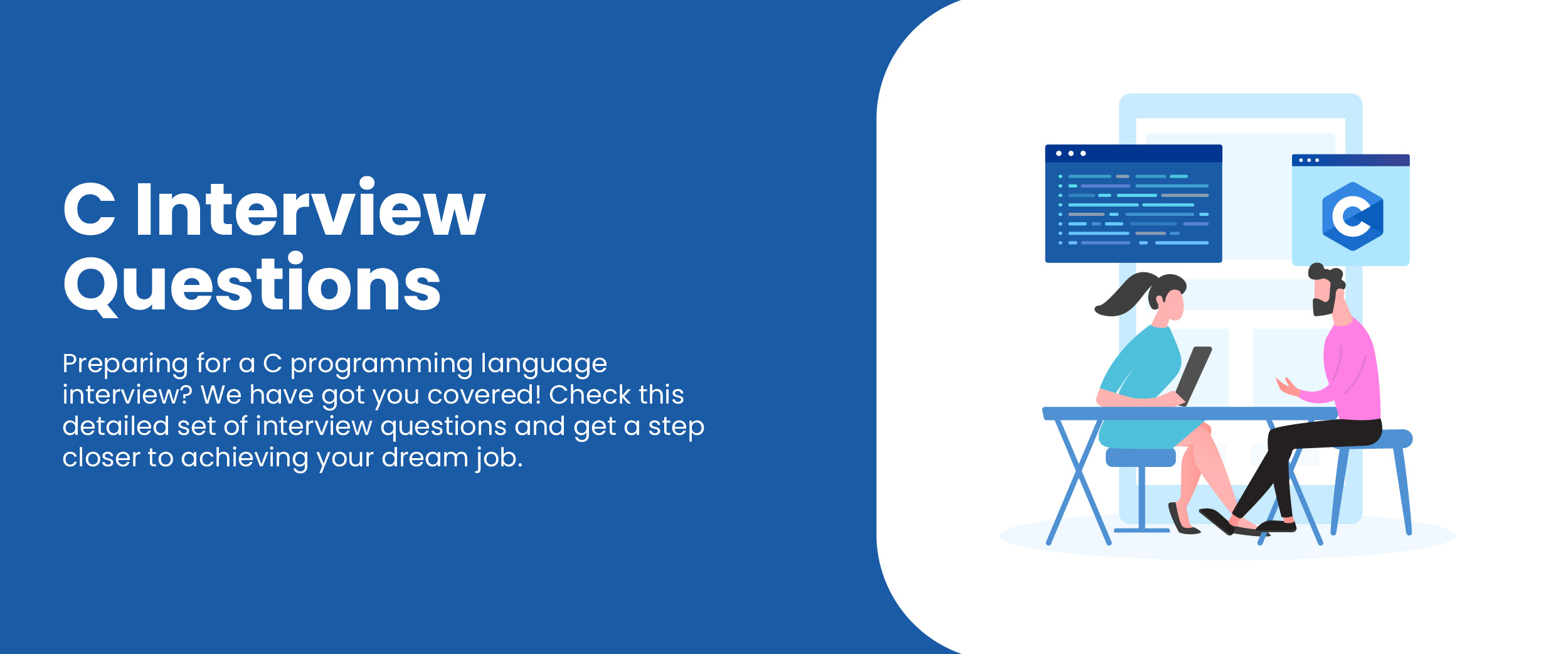
C is a general-purpose, procedural programming language developed in the early 1970s. It is simple, easy to learn, and efficient for computer programs. While C has been superseded by more modern languages, like Java and Python, it remains popular due to its versatility and capability of running on different types of hardware platforms, including embedded systems. In this blog, we will look at some C interview questions for freshers, intermediates, and experienced individuals.
Table of Contents
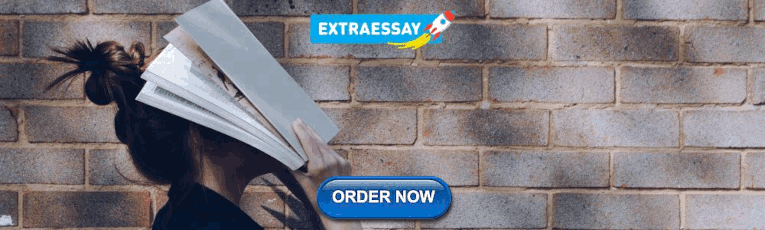
Basic C Interview Questions for Freshers
The following are some simple C interview questions and answers:
1. What is C programming language?
C is a mid-level programming language, also known as the structured programming language. It divides large programs into smaller modules, where each module employs structured code.
2. How is C related to other programming languages?
As the majority of compilers and JVMs are written in C, languages created after C have substantially drawn from it, including C++, Python, Rust, Javascript, etc. It presents fundamental ideas to these languages, including arrays, functions, and file management.
3. Why is C called a mid-level programming language?
Due to its ability to combine low-level and high-level programming, C is referred to as a mid-level programming language. This is because programs written in C are converted into assembly code but are machine-independent, which is a high-level language tendency.
4. When was the C language developed? Name its prominent features.
The C language was developed in 1972 at Bell Laboratories of AT&T by Dennis Ritchie. Some of its prominent features are:
- It is easy and effective.
- It is machine-independent or portable.
- It is a programming language with structure and dynamic memory management.
- It can be expanded and its library is functionally rich.
- In C, pointers can be used.
5. What is the difference between local and global variables in C?
Some of the key differences between local and global variables in C are as follows:
6. What is an array?
A collection of similar-type components is known as an array . It has an uninterrupted memory space. It optimizes the code and makes it simple to sort and navigate through.
7. What is a token?
The individual elements of a program are called tokens. The following 6 types of tokens are available in C:
- Identifiers
- Special Characters
8. What is the use of printf() and scanf() functions?
The printf() and scanf() functions are in-built library functions used for input and output purposes in the C language. The printf() function is used to print the output on the display and the scanf() is used to read formatted data from the keyboard.
9. Explain some data type format specifiers.
- %d: It is used for printing and scanning an integer value.
- %s: It is used for printing and scanning a string.
- %c: It is used for displaying and scanning a character value.
- %f: It is used to display and scan a float value.
10. What is a pointer and NULL pointer in C.
A pointer is a variable that points to a value’s address. It speeds up performance and optimizes the code. A NULL pointer is a pointer that does not point to any address of value other than NULL. Any pointer that receives a value of ‘0’ is converted to a null pointer.
11. What are some of the uses of pointers in C?
The pointers are used in C language for the following purposes:
- Save memory space and execution time.
- To access array elements.
- To pass arguments by reference.
- To implement data structures.
- For dynamic memory allocation.
- For system-level programming that requires memory addresses.
- To return multiple values.
12. What is a built-in function?
The built-in functionality, usually referred to as library functions, is a feature offered by the system that helps developers complete a number of frequently carried out predefined operations. Some of the commonly used built-in functions are scanf(), printf(), strcpy, strlwr, strcmp, etc.
13. What is a preprocessor?
A preprocessor is a software program that processes a source file before sending it to be compiled. It is also known as macro processor as it allows you to define macros or brief abbreviations for longer constructs. It also allows conditional compilation, line control, and inclusion of header files.
14. What is the difference between calloc() and malloc()?
calloc() and malloc() are dynamic memory allocating functions. The sole distinction between them is that malloc() does not load a value into any of the designated memory addresses, but calloc() does.
15. Can a C program be compiled or executed in the absence of a main()?
Although the program won’t run, it will be compiled. Any C program must have main() in order to run because this function acts as the entry point of the program where the execution begins. By default, the return type of main() function is int.
16. Can we use int datatype to store the 32768 value?
No, the integer data type will support the range between -32768 and 32767. Any value exceeding that will not be stored.
17. What is a dangling pointer variable in C?
The term “dangling pointer variable” refers to a pointer variable that has been erased but it still refers to the same position in memory.
18. What is pointer to pointer in C?
A pointer can also be used in C to store another pointer’s address. The first pointer contains the address of a variable, whereas the second pointer contains the address of the first pointer.
19. What are enumerations?
Enumeration is a user-defined data type, often known as enum in C. It is made up of constant integrals or integers with user-assigned names. You can learn more about enumeration through this in-depth C programming course .
Intermediate-Level C Programming Interview Questions
The following are some C language interview questions and answers for intermediate candidates:
20. How is a function defined in C language?
The function in C language is declared as:
21. What is the difference between getc(), getch(), getche(), and getchar()?
The following is the difference between these four functions in C:
getc(): It reads a single character from the input. If it succeeds, it returns an integer value. Otherwise, it returns end-of-file (EOF).
getch(): It reads a single character and does not use any buffer. The entered character is returned immediately without the need to press enter. It is a non-standard function and is mostly used by MS-DOS compilers.
getche(): It reads a character from the keyboard and displays it on the output screen. It does not wait for the enter key.
getchar(): It can read a single character from a standard input stream and return it. You can find this basic function in the stdio.h header file.
22. What is typecasting?
The typecasting process is the change of one data type into another. This conversion is done either manually or automatically. The programmer can manually convert the data type, while the compiler does the automatic conversion.
23. What is dynamic memory allocation?
The process of assigning memory to a program and its variables during execution is known as dynamic memory allocation. Three functions are used in the dynamic memory allocation process to allocate memory and one function is used to release memory that has been used.
24. What are r-value and i-value?
A data value kept in memory at a specific location is referred to as an “r-value”. A value cannot be assigned to an expression that has an r-value. Hence, this expression can only occur on the right side of the assignment operator (=).
The term “i-value” describes a memory address used to identify an object. Either the left or right side of the assignment operator (=) contains the i-value. In many cases, i-value is used as an identification.
25. What is the difference between struct and union in C?
A struct is a collection of intricate data structures kept together in memory and given independent memory locations so that they can all be accessed simultaneously. In contrast, all of the member variables in a union are kept in the same location in memory, which means that changing the value of one member would also modify the values of all the other members.
26. What is a simple example of structure in C language?
Structure or struct in C language is a user-defined data type used to group different variables together in one place. Here is a simple example of structure in C.
The output of the code will depend on the values you input during runtime. For example, if you enter name as John and age as 25, the result will be as follows:
27. What is Call by reference?
Call by reference is the term used when the calling function calls a function without using the addresses of the given parameters. Because all actions in call by reference are conducted on the value stored in the address of real parameters, each operation made on formal parameters has an impact on the value of actual parameters.
28. What is Pass by reference in C?
The callee receives the address in Pass by reference and copies the address of an argument into the formal parameter. The address is used by the callee function to retrieve the actual argument and perform manipulations. The caller function will also be aware of any modifications made to the value referenced at the given address by the callee function.
29. Explain toupper() with an example.
toupper() is a function designed to convert lowercase words/characters into uppercase. Here is an example of this function:
The following is the output for the above program:
The toupper() function does not modify the characters that are not in lowercase. So ‘B’ remains ‘B’ and only ‘a’ changes to ‘A’.
30. What is a memory leak? How can it be avoided?
Memory leaks occur when a program allots dynamic memory to a program but forgets to release or destroy the memory once the code has run its course. To avoid memory leaks, memory allocated on the heap should always be cleared when it is no longer needed.
31. What is typedef?
typedef provides an alias name to the existing complex type definition. With typedef, you can simply create an alias for any type. Whether it is a simple integer to complex function pointer or structure declaration, typedef will shorten your code.
32. What is the difference between ‘g’ and “g” in C?
Single-quoted variables in C are identified as the character and double-quoted variables are identified as a string. The string (double-quoted) variables end with a null terminator that makes it a 2-character array.
33. What is the difference between declaring a header file with < > and ” “?
The compiler looks for the header file in the built-in path if the header file is defined using <>. If the header file is specified with the character ” “, the compiler will first look in the current working directory for the file before moving on to other locations if it cannot be found.
34. When is the register storage specifier used?
If a particular variable is utilized frequently, we use the register storage specifier. Since the variable will be declared in one of the CPU registers, this helps the compiler in finding the variable.
35. Write a program to swap two numbers without using the third variable.
Here is a program to swap two numbers without using the third variable:
36. Which structure is used to link the program and the operating system?
The operating system and the program are joined by the file structure. The file is defined by the “stdio.h” header file (standard input/output header file). It includes details about the file, including its size and placement in memory. It also includes a character pointer that directs the user to the currently opened character. When a file is opened, the connection is made between the program and the operating system.
37. What is a near and far pointer?
A near pointer is used to hold addresses with a maximum size of 16 bits. It is no longer used because it lets you access only 64kb of data at a time, which is seen as insufficient.
A 32-bit pointer is regarded as a far pointer. It can make use of the current segment to access data kept outside of the computer’s memory.
38. What are the two types of loops used in C programming?
Loops in C programming are used for repeated execution of a block of statements. The statement is repeated and executed ‘n’ number of times until the given condition is met. There are two types of loops in C programming. These are:
- Entry-Controlled Loops: Here, the test condition is checked before entering the main body of the loop. Examples: For and While loops.
- Exit-Controlled Loops: Here, the test condition is checked at the end of the loop body. Example: do-while loop.
39. What is the difference between source code and object code?
Some of the key differences between source code and object code are:
C Interview Questions for Experienced Candidates
Here are some C interview questions and answers for experienced candidates:
40. How to remove duplicates in an array?
Here is a program that takes an array of integers as input, removes the duplicate elements, and prints the resulting array.
Suppose you enter the following values during runtime:
You will get the following output:
41. What is a bubble sort algorithm in C? Explain with a sample program.
Bubble sort is a sorting algorithm in C language that is commonly used to sort elements in a list or array. It compares adjacent elements and swaps them if they are in the wrong order. Refer to this example for a better understanding of the bubble sort algorithm:
The output of the code depends on the values you put during runtime. Here is what the output can look like:
42. What are reserved keywords?
Each keyword in C programming is related to a specific task in a program. These are called reserved keywords. They have a defined meaning and you cannot use them for other purposes than already defined. C language has 32 keywords. Some of these are int, auto, switch, else, if, etc.
43. Can a program be compiled without the main() function?
Yes, you can compile a program without the main() function. Instead, you can use macro, which is a small code written to make the code readable by removing repetitive expressions.
The output for the above-given program is:
44. How do you override a macro?
The #ifdef and #undef preprocessors can be used to override a declared macro.
45. What are the limitations of scanf() and how is it avoided?
The scanf() function cannot be used to insert a multi-word string into a single variable. The gets() method is used to prevent this.
46. Write a program to check whether a number is in binary format or not.
You can check whether a number is in binary format or not with the following program.
The output of this program will vary depending on the number you enter. For example, if you enter the number 1010, the output will be:
If you enter the number 1234, the output will be:
47. Write a C program to find out a sum of numbers using recursion.
Recursion in C language is a process in which the program repeats a certain section of code in a similar way. When a program allows you to call a function inside the same function, it is called a recursive call of the function. Here is an example of how this function is used to find out a sum of numbers:
The output will depend on the number you input during runtime. For example, if you enter the number ‘123’, the output will be:
48. What is the use of a semicolon (;) at the end of a program?
The semicolon in C serves as a border between two sets of instructions. It is a key factor in how the compiler analyses (or parses) the complete code and separates it into a series of instructions (or statements).
49. What is the difference between macros and functions?
50. what is a dynamic data structure.
A dynamic data structure (DDS) is an arrangement or grouping of data in memory that can expand or contract in size. It gives a programmer complete control over how much memory is used.
DDS can allocate or deallocate the unused memory according to the requirement. Dynamic memory allocation can be done on both stack and heap as opposed to the static memory allocation, which is done only on the stack. Examples of dynamic data structures are queues, linked lists, stacks, and trees.
51. Write a program to write two numbers without using the addition operator.
Here is a program to add two numbers without using the addition operator:
If we enter values ‘5’ and ‘7’, the output of the above program will be:
52. Write a program to find the nth Fibonacci number.
The following is the code to calculate nth Fibonacci number using a recursive approach. It defines a function ‘fib()’ that takes an integer ‘n’ as input and returns the corresponding Fibonacci number.
The following is the output for the above code:
The output indicates that the 8th Fibonacci number is 21. It is based on the Fibonacci sequence F(n) = F(n-1) + F(n-2) with base values F(0) = 0 and F(1) = 1.
The C interview questions listed in this blog can be a valuable tool for job seekers. They can help you improve your problem-solving abilities and further understand the concepts on a deeper level. A thorough understanding of the key concepts, preparation, and practice will make it extremely simple for you to ace an interview.
Do you think we missed out on any crucial questions here? Let us know in the comments below. For more information about C and how it differs from C++, check out this blog on the differences between C and C++ .
- ← Previous
- Next →
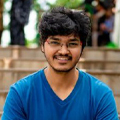
Parinay is an industry expert with over six years of professional experience. He is known for his excellence in Full Stack Development and Web Application Security. The Director of the Tech Team in his college days, Parinay has created websites that have won prestigious awards at national and international events.
Related Post
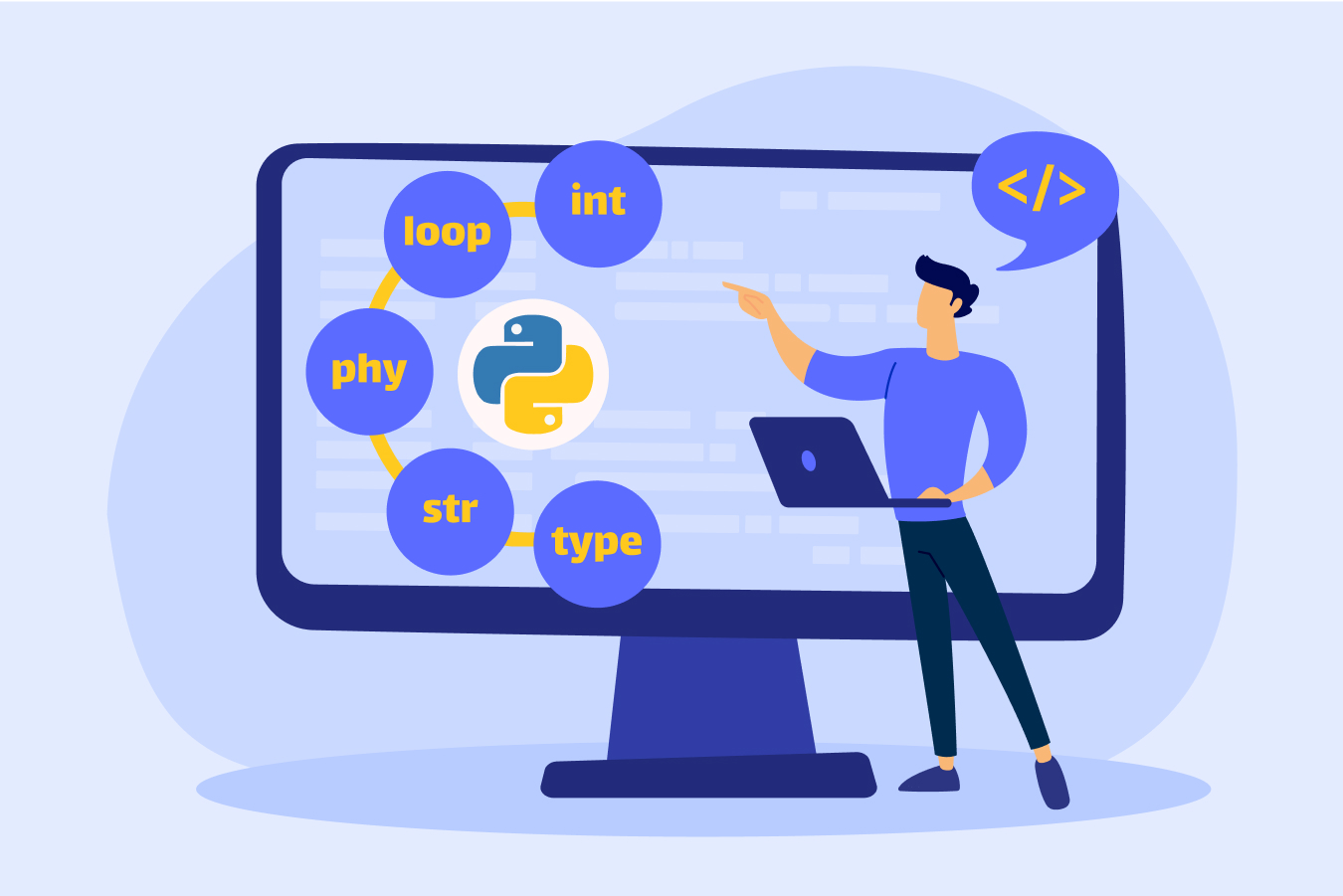
Python Developer Roadmap: Steps to a Successful Career
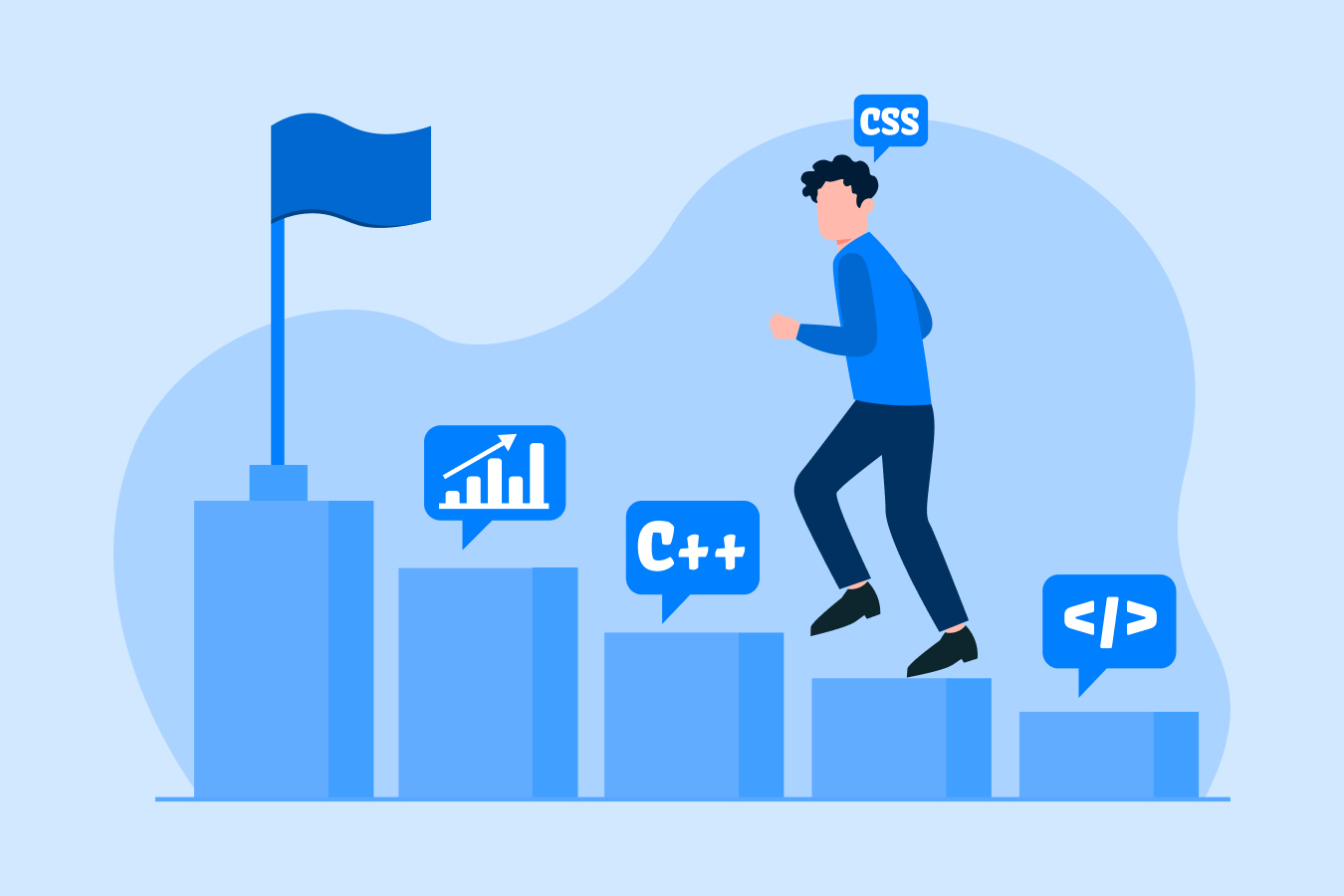
Frontend Roadmap for Beginners: A Guide to Kickstart Your Career
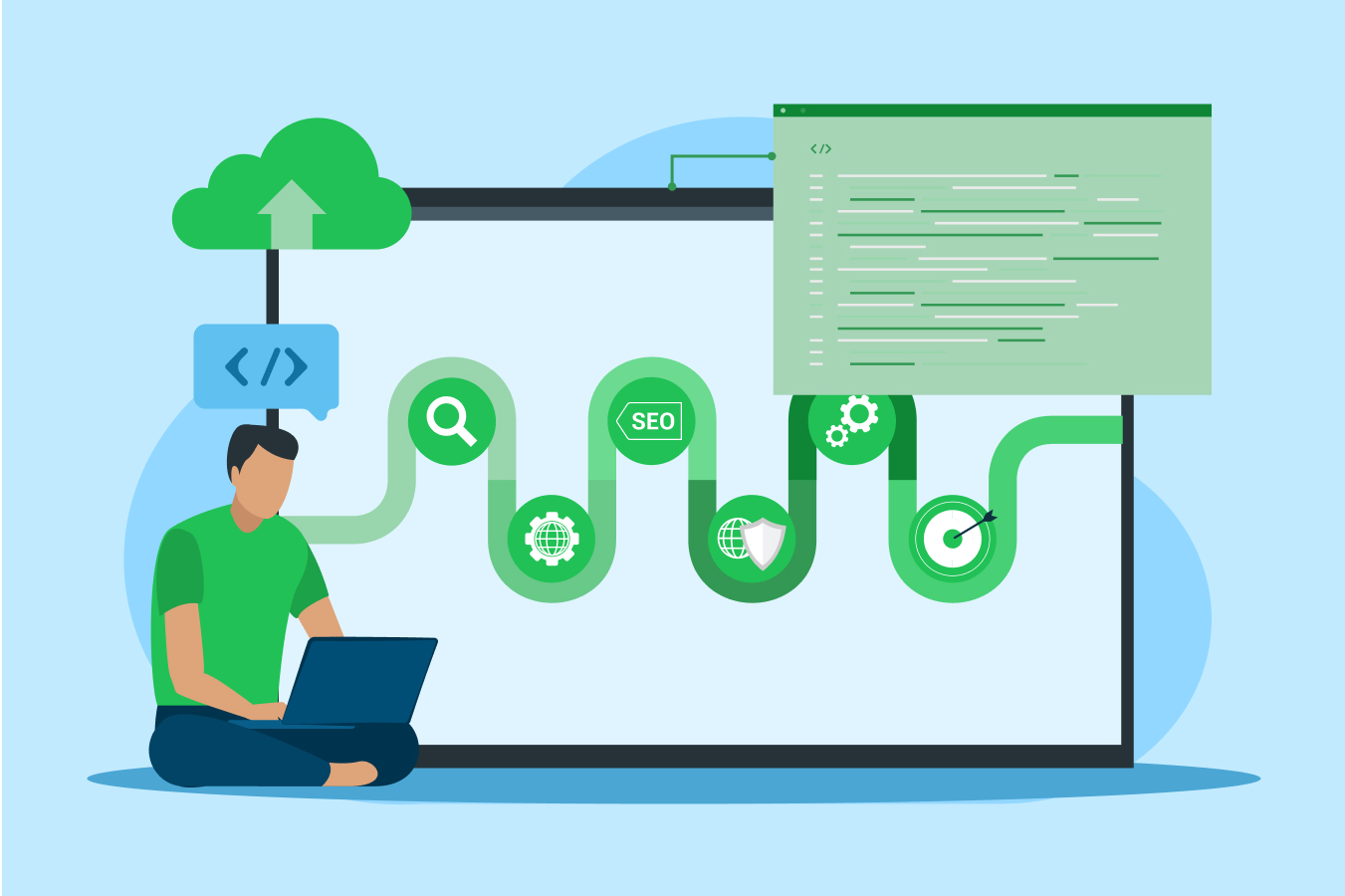
Backend Developer Career Roadmap: The 2024 Guide
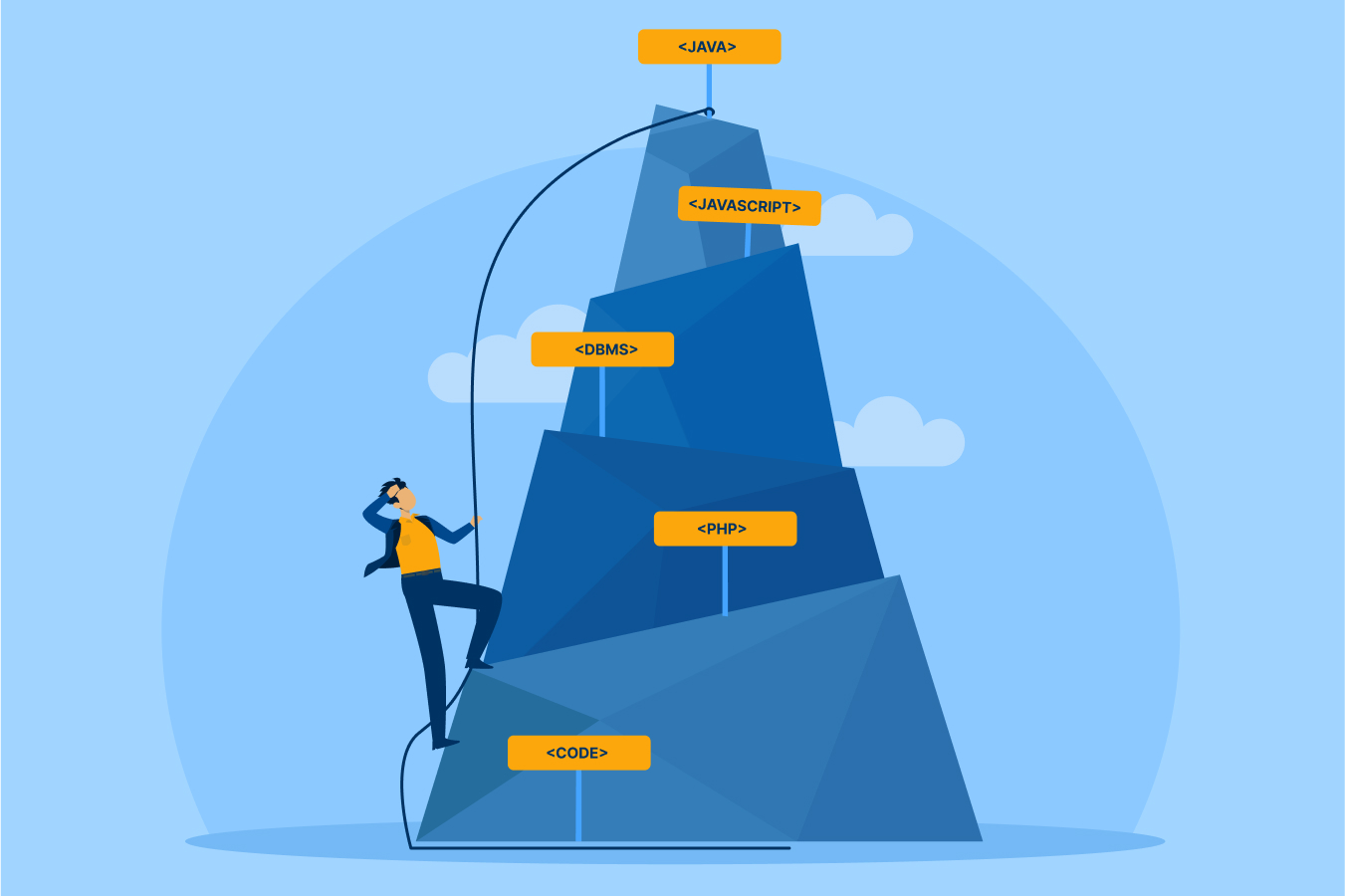
Full Stack Developer Roadmap: A Guide for Building Your Career in 2024
All Courses
- Interview Questions
- Free Courses
- PGP in Data Science and Business Analytics
- PG Program in Data Science and Business Analytics Classroom
- PGP in Data Science and Engineering (Data Science Specialization)
- PGP in Data Science and Engineering (Bootcamp)
- PGP in Data Science & Engineering (Data Engineering Specialization)
- Master of Data Science (Global) – Deakin University
- MIT Data Science and Machine Learning Course Online
- Master’s (MS) in Data Science Online Degree Programme
- MTech in Data Science & Machine Learning by PES University
- Data Analytics Essentials by UT Austin
- Data Science & Business Analytics Program by McCombs School of Business
- MTech In Big Data Analytics by SRM
- M.Tech in Data Engineering Specialization by SRM University
- M.Tech in Big Data Analytics by SRM University
- PG in AI & Machine Learning Course
- Weekend Classroom PG Program For AI & ML
- AI for Leaders & Managers (PG Certificate Course)
- Artificial Intelligence Course for School Students
- IIIT Delhi: PG Diploma in Artificial Intelligence
- Machine Learning PG Program
- MIT No-Code AI and Machine Learning Course
- Study Abroad: Masters Programs
- MS in Information Science: Machine Learning From University of Arizon
- SRM M Tech in AI and ML for Working Professionals Program
- UT Austin Artificial Intelligence (AI) for Leaders & Managers
- UT Austin Artificial Intelligence and Machine Learning Program Online
- MS in Machine Learning
- IIT Roorkee Full Stack Developer Course
- IIT Madras Blockchain Course (Online Software Engineering)
- IIIT Hyderabad Software Engg for Data Science Course (Comprehensive)
- IIIT Hyderabad Software Engg for Data Science Course (Accelerated)
- IIT Bombay UX Design Course – Online PG Certificate Program
- Online MCA Degree Course by JAIN (Deemed-to-be University)
- Cybersecurity PG Course
- Online Post Graduate Executive Management Program
- Product Management Course Online in India
- NUS Future Leadership Program for Business Managers and Leaders
- PES Executive MBA Degree Program for Working Professionals
- Online BBA Degree Course by JAIN (Deemed-to-be University)
- MBA in Digital Marketing or Data Science by JAIN (Deemed-to-be University)
- Master of Business Administration- Shiva Nadar University
- Post Graduate Diploma in Management (Online) by Great Lakes
- Online MBA Programs
- Cloud Computing PG Program by Great Lakes
- University Programs
- Stanford Design Thinking Course Online
- Design Thinking : From Insights to Viability
- PGP In Strategic Digital Marketing
- Post Graduate Diploma in Management
- Master of Business Administration Degree Program
- MS in Business Analytics in USA
- MS in Machine Learning in USA
- Study MBA in Germany at FOM University
- M.Sc in Big Data & Business Analytics in Germany
- Study MBA in USA at Walsh College
- MS Data Analytics
- MS Artificial Intelligence and Machine Learning
- MS in Data Analytics
- Master of Business Administration (MBA)
- MS in Information Science: Machine Learning
- MS in Machine Learning Online
- MIT Data Science Program
- AI For Leaders Course
- Data Science and Business Analytics Course
- Cyber Security Course
- PG Program Online Artificial Intelligence Machine Learning
- PG Program Online Cloud Computing Course
- Data Analytics Essentials Online Course
- MIT Programa Ciencia De Dados Machine Learning
- MIT Programa Ciencia De Datos Aprendizaje Automatico
- Program PG Ciencia Datos Analitica Empresarial Curso Online
- Mit Programa Ciencia De Datos Aprendizaje Automatico
- Online Data Science Business Analytics Course
- Online Ai Machine Learning Course
- Online Full Stack Software Development Course
- Online Cloud Computing Course
- Cybersecurity Course Online
- Online Data Analytics Essentials Course
- Ai for Business Leaders Course
- Mit Data Science Program
- No Code Artificial Intelligence Machine Learning Program
- MS Information Science Machine Learning University Arizona
- Wharton Online Advanced Digital Marketing Program
- C BASIC INTERVIEW QUESTIONS
- C Interview Questions for Freshers
- C Technical Interview Questions
- C data structure Interview Questions
- C Programming Interview Questions
100+ C Interview Questions and Answers for 2024
C programming is a general-purpose language computing programming language and is essential if you want to work in the software development domain. The first set of questions and answers are curated for freshers, but the blog will cover C Interview Questions for experienced, technical interview questions, C data structure interview questions and more. These questions cover all the basics of C and will help showcase your expertise in the subject. The questions are divided into groups as follows.
- C Basic Interview Questions
1. Can you tell me how to check whether a linked list is circular?
First, to find whether the linked list is circular or not, we need to store the header node into some other variable and then transverse the list. A linked list is not circular, if we get null at the next part of any node and if the next node is the same as the stored node, then it is a circular linked list. An empty linked list is also considered a circular linked list.
Algorithm to find whether the given linked list is circular.
An algorithm to determine whether the linked list is circular or not is a very simple way.
Algorithm: –
1. Traverse the linked list.
2. Check if the node is pointing to the head or not.
3. If yes then the linked list is circular.
2. What is the use of a semicolon (;) at the end of every program statement?
A semicolon (;) is used to mark the end of a statement and begin a new statement in the C language. It ensures proper termination of the statement and removes confusion while looking at the code. They are not used between control flow statements but are used to separate conditions in the loop. They are end statements in C programming.
3. Differentiate Source Codes from Object Codes
Source Code: –
- Source code is in the form of Text.
- Created by the Programmer.
- Human readable.
- Can be changed over time.
- Not system specific
- Input to the compiler
- Not CPU executable
Object Codes: –
- Object code is in the form of Binary Numbers.
- Created by the Compiler.
- Machine Readable.
- Needs to compile the Source Code each time a change is to be made.
- System specific
- Output of the compiler
- CPU executable
4. What are header files and what are their uses in C programming?
A header file in C contains predefined standard library functions and declarations to be shared between several source files.
file with extension .h. There are two types of Header files: – Files that the programmer writes and the files that come with your compiler. Header files are library files of your C program. Including a header file means using the content of the header file in your source program. The basic syntax of using header files is: – #include <file> or #include “file”.
Uses of header files: – Header files contain a set of functions that are used to write a program in C.
5. When is the “void” keyword used in a function?
In programming, when you declare functions, you first decide whether that function will declare a value or not. “void” when placed at the leftmost part of the function header, the function will not return a value. The function will only display some outputs on the screen. And in the second case, after the function executes when the return value is excepted then only the data type of the return value is placed instead of “void”.
6. What is dynamic data structure?
Data Structures are of two types. Static Data Structure and Dynamic Data Structure. While performing operations, the size of the structure is not fixed and can vary according to the requirement in the dynamic data structure. This data structure is designed to facilitate the change of data structures in the run time. In DDS, the data in memory has the flexibility to grow or shrink in size. This data structure can “overflow” if it exceeds its limit.
Add Two Numbers Without Using the Addition Operator.
To add two numbers without using the Addition Operator: –
1. Take three variables num1, num2, and x.
2. Take input from the user.
3. Store it in num1 and num2.
4. Increase num1 using the ‘for’ loop.
5. Till the count of the second number for loop will call and, in each call, we will increase the value of num1 by 1.
6. After addition, the result will be stored in num1.
Using this method, we can calculate the sum of two numbers.
Subtract Two Number Without Using Subtraction Operator
To subtract two numbers without using the Subtraction Operator: –
1. Take four variables num1, num2, complement, result.
2. Take input from the user
4. Take two’s complement of a num2 number.
5. Add num1 number with two’s complement.
6. Finally, the difference will be stored in the result.
Using this method, we can easily perform subtraction of two numbers
Multiply an Integer Number by 2 without Using a Multiplication Operator.
We can use multiple approaches to multiply an integer number by 2 without using the Multiplication operator.
- We can easily calculate the product of two numbers using the recursion method and without using the * multiplication operator.
- Also, we can get the product of two numbers without using the * the multiplication operator is using an iterative method.
Check whether the number is EVEN or ODD, without using any arithmetic or relational operators
Even or Odd operation can be performed using the following 3 methods: –
Method 1: Using the bitwise (&) operator.
Method 2: Multiplying and dividing the number by 2.
First, divide the number by 2 and multiply it by 2. If the result is the same as that of the input, then it is an even number otherwise it is an odd number.
Method 3: Switching temporary variable n times with the initial value of the variable being
true. If the flag variable gets its original value (which is true) back, then n is even.
Else, it is false.
Check for Balanced Parentheses using Stack
1. First we need to declare a character stack S.
2. Then traverse the expression string exp.
a) If the character is a starting bracket ‘(‘or ‘{‘or ‘[‘then push it to stack.
b) If the character is a closing bracket ‘)’ or ‘}’ or ‘]’ then pop from the stack.
3. If the popped character is the matching starting bracket, then fine else brackets are not balanced.
4. If there is some starting bracket left after complete traversal in the stack, then it’s “NOT BALANCED”.
1. What is function in C?
A function can be defined as a block of statements which are written to perform a particular task, improve code readability and reusability. The functions can be of two types: Predefined functions and User-defined functions.
Predefined functions are in-built functions which are already present in C library. For example, printf()
A user defined function is declared and defined by the programmer for customized functions. The syntax for the same is:
2. What is recursion in C?
When a function calls itself in its definition then the process is called as Recursion. For example,
3. What is structure in C?
A structure in C is a data type that allows you to list a set of variables within it that can only be accessed by this data type’s object. Structure allows us to emulate data with varied elements.
4. How to declare string in C?
The syntax of declaring a string in C is as follows:
For example: char dog_breed [100];
5. What is string in C?
String is an array of characters whose index starts from 0 and it is terminated with a special character ‘\0’.
6. What is segmentation fault in C?
A segmentation fault in c is a fault that happens when someone tries to access a forbiden memory location or makes use of a technique that is forbidden in the system on which they are working on.
7. What is variable in C?
A variable in c is created in c to store data by reserving memory in the system. You can have different types of variables that store different types of data. for eg. You can store integer data in ‘int’ variable or characters in ‘char’ variables.
8. What is variable in C?
9. how to take string input in c.
There are multiple ways to take a string input in C, such as: – gets:
– Using %s in scanf:
10. What is macro in C?
Macro is c is basically used to replace a block of code in C. You do this by defining it in the start of the program as such:
Eg. #define k 1.44 Here, whenever you use the macro ‘k’ in the code the compile will replace it with the value ‘1.44’.
11. What is union in C?
A union in C is a data type that allows you to list & define a set of variables within it that can only be accessed by this data type’s object. A union is actually very similar to a structure with the one of the difference being that all the elements defined within a union share a memory location whereas in a structure the elements do not share a memory location.
12. How to find prime numbers in C?
A natural number greater than 1 and not a product of two smaller natural numbers other than itself and 1 is called Prime number. For example, 5 (factors of 5 is 1 and 5) is a prime number. The C code to find if the entered number is prime or not is as follows.
13. What is Armstrong number in C?
If the integer is in the form: abc= a*a*a + b*b*b + c*c*c , it is called the Armstrong number. The code to find whether the entered number is Armstrong or not is as follows.
14. What are pointers in C?
Pointers in C are a data type that store the memory address of another variable. For eg.
15. How to round off numbers in C?
The process of making a number simpler to use but keeping the new value close to the old one is called rounding off. For example, 1.76521 round off to 1.7 or 1.76. In C to do the same we have round() function. The usage of the function is depicted in the code below.
16. What is preprocessor in C?
A preprocessor in c is basically used to replace code with values you assign. And this process happens at the start of the program execution hence the name pre-processing. Eg:
17. What is identifier in C?
In identifiers are basically any name user defined name you give to any data type, label, variable or function. Eg. int hello; here hello is an identifier. But there are rules to how you can name an identifier, such as:
The first letter of the identifier has to be an alphabet or an underscore. You can only use alphabets, underscore or digits to write an identifier. There cannot be any whitespace within an identifier, otherwise you will get an error.
18. What is a function in C?
A function in c is a block of code that can be referenced from anywhere in the system and that serves a specific purpose. Eg:
19. What is enum in C?
Enum or Enumeration in C is data type that you can use to assign values to an integral constant. Eg. enum fruits{apple, banana, pineapple};
20. What is stack in C?
A stack in c is a data structure that can be used to store data. as the name suggests a stack allows you store data in the form of a stack – one top of another. You can only insert and remove data from one side that is the top of the stack. We can use operations such as pop(),push() with a stack.
21. What is loop in C?
A loop in C is a set of code that allows the user to repeatedly perform a certain action until the set condition is fulfilled.
22. What is eof in C?
Eof in C defines the end of file, i.e. where the file that you are reading ends.
23. Who invented C?
Dennis Ritchie invented C in 1972.
24. How to return an array in C?
25. what is #include in c.
#include is a pre-processor statement which makes sure about the availability of all the declarative statements and related function calls.
26. What is file in C?
Files in C are a set of bytes that are reserved for storing related data. It’s different than variables as it is used to store data permanently.
27. How to learn C?
To learn C, start with why you should learn C and the features of this language. Then make sure you have the environment set for the same. About programming start with basic syntax, data types, data structure and control structure. After learning basics, then jump to advance concepts.
28. How to call a function in C?
There are two ways in which C function can be called from a program.
1. Call by Value: In this method, the value of the actual parameter can not be modified by formal parameter as both the parameters have different memory allocated to them.
2. Call by Reference: In this method, the value of the actual parameter will be modified by formal parameter as both the parameters have the same memory allocated to them.
29. What is static variable in C?
The variable that is defined only once and the compiler retains its value even after they exit the scope are called Static Variables. They are declared using static keyword. The syntax is:
static data_type variable_name = variable_value;
For example: static int c=9;
30. Who invented C language?
Dennis M. Ritchie at Bell Laboratories (formerly AT&T Bell Laboratories) in 1972 invented the C programming language.
31. How to check if a number is a perfect square in C?
You can check if a number is a perfect square by two ways.
1. Using functions from math.h library. 2. Using for and if loop
The sample code using math.h is as follows:
32. How to find power of a number in C?
The power of a number can be calculated by using pow() function of math.h library. For example:
33. What is compiler in C?
Programmers write code in high level languages and a special program called compiler converts that high level code in machine language.
34. What are identifiers in C?
Identifier refers to unique names given to entities such as variables, functions, structures etc. – Rules for naming identifier: – Can have letters, digits, and underscores – First letter should either alphabet or underscore – Cannot use keywords as identifiers
35. How to use switch case in C?
A switch statement allows a variable to be tested against different values. Each of these values is called a case. Syntax for the same is:
36. How to convert decimal to binary in C?
37. what is linked list in c.
A linked list is a data structure in which nodes are dynamically allocated and arranged where each node contains one value and one pointer. Syntax is as follows:
38. In C, if you pass an array as an argument to a function, what actually gets passed?
When array is passed as argument, then the base address of the array is passed to the function.
39. How to use gets in C?
Gets is a function that reads a line from stdin and stores it in string.
40. What is extern in C?
Extern often referred to as global variables are declared outside the function but are available globally throughout the function execution. The value of this variable can be changed/modified and the default value is zero. These are declared using the “extern” keyword.
41. How to write a C program?
First the appropriate libraries are included using pre-processor. For example, #include<stdio.h>. After this, main() function is written which is the entry point of every program. Inside the main() function code blocks are written using various functions and variables.
Sample Hello World code is as follows:
42. What is token in C?
Smallest elements of a program are called tokens such as keywords, identifiers, strings etc.
43. What is volatile in C?
Volatile is a keyword in C which when applied to a variable at the time of declaration tells the compiler that the value of that variable will change at any time. Syntax for declaring a variable as volatile is as follows:
volatile uint8_t a;
44. What is argument in C?
In C99 Standard, an argument is defined as follows. “An argument is an expression in the comma-separated list bounded by the parentheses in a function call expression, or a sequence of preprocessing tokens in the comma-separated list bounded by the parentheses in a function-like macro invocation.” To explain it through code. Suppose a function:
And while calling the function- sub(5,4) – here 5 and 4 are the arguments of the function sub().
45. How to solve increment and decrement operators in C?
Increment (++) and decrement (–) are unary operators in C. These operators increase and decrease their values by 1.
++a is same as a=a+1 –a is same as a=a-1 a = 5; b = 8; c = ++a + b++; c=++a + 8; c=6+8= 14
46. What is an algorithm in C?
A sequential and well defined instructions for problem solving is called an algorithm. For example, a sample algorithm for subtracting two numbers.
Step 1: Start Step 2: Declare variables a, b and sub. Step 3: Read values a and b. Step 4: Add a and b and assign the result to sub. sub←a – b Step 5: Display sub Step 6: Stop
47. What is the use of C language?
The benefits of C language:
– C has rich library with a large number of inbuilt functions. – C supports dynamic memory allocation. – C facilitates fast computation in programs. – C is highly portable. – C is case sensitive language. – C is used for scripting applications. – C allows free movement of data across the functions.
Due these benefits of C, it can be used/it has the following applications: – Database systems – Graphics packages – Word processors – Operating system development – Compilers and Assemblers – Interpreters
48. How to store values in array in C?
Sample codes for storing values in an array.
49. Why C is called middle level language?
C is called middle level language as some of its features resemble high level language and some resemble low level language. For example, certain instructions in C are like a normal algebraic expressions along with certain English keyword like if, else, for etc. which resembles a high level language. On the other hand C permits the manipulation of individual bits which resembles low level language.
50. What is conditional operator in C?
Conditional operator also known as ternary operator is used for decision making and it uses two symbols- ? and :. The syntax for conditional operator is as follows:
Expression1? expression2: expression3;
Code for the conditional operator is as follows:
51. Why we use conio.h in C?
Conio is a header file which stands for console input output. It contains some functions and methods for formatting the output and getting input in the console which is why it is used.
52. What is null in C?
Null is macro defined in C which has a value equal to zero. It is defined as follows:
53. What is pointer in C?
Every variable is stored at an address in C and pointers are special variables which store addresses. You can declare pointers in 3 different ways.
54. How to print double in C?
Double in C is initialized using the syntax below: double d; To print, double is printed using %lf printf(“%lf”,d);
55. Which data type has more precision in C?
Long double data type has more precision (upto 19 decimal places).
56. What is array in C?
Array can be defined as a collection of the same type of data which can be accessed using the same name. Array can be defined in different dimensions for example, one dimensional array, two dimensional array etc. 1D array is like a list, 2D array is like a table with rows and columns.
57. What is an array in C?
58. how to pass 2d array to function in c.
Passing a 2D array to a function is similar to passing 1D array. A sample code depicting the same is as follows:
59. How to reverse a string in C?
To reverse a string in C, we can make use of 2 variables one starting from the 0th index and one from the last index and making use of a temporary variable reversing the string. The code for the same is as follows:
60. How to compare two strings in C?
We can compare two strings using the in-built function strcmp() which returns 0 if the two strings are equal or identical, negative integer when the ASCII value of first unmatched character is less than the second, and positive integer when the ASCII value of the first unmatched character is greater than the second. Let’s understand through code.
The result of comparing the first and second string will be 0 as both the strings are identical. However, the result of comparing the first and third strings will be 32 as the ASCII code of b is 98 and B is 66.
61. How to run C program in cmd?
The steps to run your c code in command prompt is as follows:
Step-1: Install C compiler (for example gcc) Step-2: Write the c code and save it as .c file. For example, the name of your file is family.c and it is stored in Desktop. Step-3: Open Command Prompt clicking start button → All Apps → Windows System folder → Click Command Prompt. Step-4: Change the directory in command prompt to where you have saved the code file. For example, cd Desktop Step-5: Compile the code file using the compiler you have installed. Use the following syntax gcc -o <name_of_executable> <name_of_source_code> For example, gcc -o fam family.c Step-6: Now run the executable file (for example, fam.exe) by going to the directory of the executable file in command prompt (usually the same as code file until specified otherwise), then type the name of the executable file without the extension
62. How to concatenate two strings in C?
Two strings in C can be concatenated by taking a third string and appending the two strings into third. The code for the same is as follows:
63. What is data type in C?
Data types in c are used to tell the c compiler what type of data is going to be stored in a variable or what type of a function it is going to be.
– for variables: Let’s say there is a variable ‘a’ and we want to store integers in it, so we will make use of the declaration type ‘int’ to tell the compiler that ‘a’.
here ‘int’ is the data type.
– for functions:
Here we are returning an integer value ’11’ and for that purpose we have assigned the data type of this function.
64. How to print a string in C?
The syntax to print the string is as follows:
printf( “%s”, <string name>);
For example: printf (“%s”, dog_breed);
65. How to initialize array in C?
Array is initialized in two ways in C. First if the array elements are user input:
66. How to declare a string in C?
The syntax of declaring a string in C is as follows: char <string name> [size of the string]; For example: char dog_breed [100];
67. How to print string in C?
You can do it in two ways: Using scanf:
or Using gets():
68. How to reverse a number in C?
We can reverse a number using a temporary variable and performing modulus and division operations on the number. The code for reversing a number is as follows:
69. How to return array in C?
The most commonly used way to return an array is by using a pointer. A sample code for returning an array using pointer is mentioned below.
70. What is dangling pointer in C?
If a programmer fails to initialize a pointer with a valid address, then the pointer is known as a dangling pointer in C. Dangling pointers point to a memory location that has been deleted.
71. How to initialize string in C?
There are multiple ways to do so:
Character array Initialization: – Even here we have several ways:
or you can define a macro and use it with the array
Character pointer initialization:
Here Hello-World value is read-only and cannot be edited.
72. What is malloc in C?
Malloc is used for dynamic memory allocation. Malloc, refers to memory allocation, which reserves a block of memory for specific bytes. It initializes each block with default garbage value and returns a pointer of type void. The syntax for malloc is as follows:
Example: ptr = (int*) malloc(10 * sizeof(int)); The size of int is 4 bytes so this statement will allocate 40 bytes of memory. The pointer ptr will hold the address of the first byte in the allocated memory.
73. What is typedef in C?
Typedef is a keyword that assigns alternative names to existing data types. For example, for variables with data type unsigned long or for structures.
74. How to find array length in C?
75. how to declare array in c.
You can do this in multiple ways, such as:
76. How to compile C program in cmd?
Open cmd verify gcc installtion using the command: $ gcc -v then go to your working directory or folder where your code is: $ cd <folder_name> then build the file containing your c code as such: $ gcc main.c then run the executable generated in your system: $ main.exe
77. How to print a sentence in C?
78. how to print ascii value in c.
The code to print ASCII value is as follows:
79. What are storage classes in C?
A storage class represents information about variable such as variable scope, location, value, lifetime etc. There are four types of Storage classes. 1. Auto (default storage class) 2. Extern (global variable) 3. Static 4. Register
80. What are macros in C?
Defined by #define directive, a macro is defined as a segment of code which can be replaced by its initialized value. There are two types of macros. 1. Object-like: For example: #define M=1000 2. Function like: For example: #define Max(x,y) ((x)>(y)?(x):(y))
81. How to use stdin and stdout in C?
STDIN stands for Standard Input. STDOUT stands for Standard Output. There are several functions to both input and output. scanf() [STDIN] and printf() [STDOUT] getchar() [STDIN] and putchar() [STDOUT] gets() [STDIN] and puts() [STDOUT]
82. What is garbage value in C?
In C, when a variable is defined but no value is initialized or assigned to it. Then that variable is assigned to any random computer’s memory which is called Garbage value.
83. What is type casting in C?
Typecasting is a process to convert one data type to another. The syntax for type casting is as follows: (type_name) expression A sample code for the same is as follows:
84. How to print pattern in C?
Suppose you have a half pattern mentioned below to print. * * * * * * The code for the same is:
The * can be replaced by alphabet or number.
For full pattern, the code is: * * * * * * * * *
85. How to convert uppercase to lowercase in C?
To convert a string from uppercase to lowercase, a predefined function tolower() can be used and also by looping it can be achieved. A sample code containing both are as follows:
86. What is flowchart in C?
Graphical representation of an algorithm which as a tool for program planning for solving a problem is used is called flowchart. Symbols are used in flowchart for representing flow of information.
87. How to read a file in C?
The code to read a file in C as follows:
88. What is perfect number in C?
Perfect number is a number which is equal to the sum of its divisor. For example, 6 is a perfect number because 6 divisors: 1,2,3 has sum also equal to 6. Code to check whether a number is perfect or not is as follows:
89. How to convert lowercase to uppercase in C?
Using toupper() functions, one can convert lowercase to uppercase in c. Syntax for the same is as follows. int toupper(int ch);
90. How to stop infinite loop in C?
Break statements (along with a condition) can be used in C to stop infinite loop. Also, keyboard shortcuts such as CTRL-Break, Break, CTRL-C and CTRL-ESC-ESC can be used based on the compiler you are using. Sample code for break statement is as follows:
91. How to swap two numbers in C?
Swapping two numbers using a temporary variable.
92. What is %d in C?
%d means printing and scanning integer variables.
93. What is control statement in C?
A statement that determines whether other statements will be executed or not is called a control statement. There are two types of flow control in C. Branching: it decides what action to take. For example, if and else statement. Looping: it decides a number of times to take certain actions. For example, for, while and do while.
94. What is while loop in C?
A while loop allows the code written inside the code block to be executed a certain number of times depending upon a given Boolean condition. The syntax of while loop in c language: while(condition) { //code to be executed }
95. What is static function in C?
Static in C is a keyword applied before a function to make it static. Syntax for static function is as follows: static int fun(void) { printf(“static function “); }
96. What is pointer to pointer in C?
Pointers are used to store the address of variable. In Pointer to pointer, the first pointer stores the address of the variable and the second pointer is used to store the address of the first pointer. Syntax for declaring pointer to pointer is as follows: int **ptr;
97. What is stderr in C?
There are 3 I/O descriptors: stdin for standard input, stdout for standard output, stderr for error message output. stderr is used for mapping the terminal output and generates the error messages for the output devices. Sample code for the same is as follows:
98. How to declare Boolean in C?
Boolean is a data type containing two values 1 or 0. Syntax for declaring Boolean in C bool variable_name; Code for illustrating the same is as follows:
99. How to find the length of a number in C?
The code to calculate length of a number in C is as follows:
100. Write a program to reverse an integer in C.
101.write a program in c to check whether an integer is an armstrong number or not., 102. write a program in c to check if a given number is prime or not., 104. write a program in c to print the fibonacci series using iteration., 105. write a program in c to print the fibonacci series using recursion., 106. write a program in c to check whether a number is a palindrome or not using iteration., 107. write a program in c to check whether a number is a palindrome or not using recursion., 108. write a program in c to find the greatest among three integers., 109.write a program in c to check if a number is binary., 110. write a program in c to find the sum of digits of a number using recursion..
Q1 What are the basics of C?
Below is the syntax and a few commands to write a basic c program.
Q2 What is C mainly used for?
C is a general-purpose programming language that can be easily read, edited, and understood. It is the language in which operating systems for desktop as well as mobile phones are developed. Flexibility is a property of C that makes it popular for embedded systems programming. Even if you use C language for the embedded systems, you don’t have to pay anything for it as C is a free language.
Q3 Why is C dangerous?
C is unsafe as executing an erroneous operation can cause the entire program to become meaningless. In such a case, the output would become unpredictable as erroneous operations are said to have undefined behavior. Also, C programming allows arbitrary pointer arithmetic with pointers implemented as direct memory addresses having no provision for the bound checking, making C potentially memory-unsafe.
Q4 What is the main() in C?
Every program in C must have a main() function as it is the entry point for any C program. The first function in your program to be executed at the beginning of execution is the main() function, as the execution control goes directly to it.
Q5 What are keywords in C?
Keywords have specific meanings associated with them to the compiler. They have been already defined (pre-defined) and reserved. They cannot be used as identifiers. The 32 keywords in C are:
- auto
- break
- case
- const
- continue
- default
- double
- else
- enum
- float
- for
- goto
- int
- long
- short
- signed
- sizeof
- struct
- switch
- unsigned
- void
Q6 What is c and its features?
C is a widely used, easily read, edited, and understood programming language. Features of C programming language are:
- Procedural Language
- Fast and Efficient
- Statically Type
- General-Purpose Language
- Rich Libraries
- Middle-Level Language
- Machine Independent or Portable
- Easily Extensible
Q7 Is C still used in 2021?
C is a middle-level language; hence it combines features of both the high-level and the low-level languages. It can be used for scripting for drivers & kernels (for low-level programming) & also for the scripting for software applications (for high-level language). C is a language very close to the hardware and is very useful for coding in situations where the speed of execution is critical or only limited resources are available.
Q8 Why is C so popular?
C is known as the mother of all programming languages. It is still widely used and popular due to its features and flexible memory management. For system-level programming, C is considered the best option. Operating system kernels are written in C, so even the smartphones that you have been using every day are running on a C kernel.
Q9 Which is better Python or C?
Both C & Python are useful languages to develop different applications. C is mainly used for the development related to hardware like network drivers & operating systems, whereas Python is used for natural language processing, machine learning, web development, etc. It is not enough for one to master a single programming language in today’s competitive scenario; therefore, learning both will be beneficial to stand strong in the present competitive market.
This brings us to end of the blog on C Interview Questions. We hope you are now well-equipped with the kind of questions that may be asked during an Interview. Wondering where to learn the highly coveted in demand skills for free? Check out the courses on Great Learning Academy .

Top Free Courses

Top 50+Nodejs Interview Question and Answer 2024

Top 30+Embedded C Interview Question and Answers 2024

Top 60+ Cloud Computing Interview Questions (2024)

Top 90+ Tableau Interview Question and Answers in 2024

Top 30 Infosys Interview Questions | 2024

Top 50+ Selenium Interview Questions and Answers in 2024
Leave a comment cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

Table of contents
15 Common Problem-Solving Interview Questions
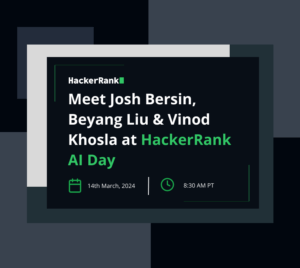
In an interview for a big tech company, I was asked if I’d ever resolved a fight — and the exact way I went about handling it. I felt blindsided, and I stammered my way through an excuse of an answer.
It’s a familiar scenario to fellow technical job seekers — and one that risks leaving a sour taste in our mouths. As candidate experience becomes an increasingly critical component of the hiring process, recruiters need to ensure the problem-solving interview questions they prepare don’t dissuade talent in the first place.
Interview questions designed to gauge a candidate’s problem-solving skills are more often than not challenging and vague. Assessing a multifaceted skill like problem solving is tricky — a good problem solver owns the full solution and result, researches well, solves creatively and takes action proactively.
It’s hard to establish an effective way to measure such a skill. But it’s not impossible.
We recommend taking an informed and prepared approach to testing candidates’ problem-solving skills . With that in mind, here’s a list of a few common problem-solving interview questions, the science behind them — and how you can go about administering your own problem-solving questions with the unique challenges of your organization in mind.
Key Takeaways for Effective Problem-Solving Interview Questions
- Problem solving lies at the heart of programming.
- Testing a candidate’s problem-solving skills goes beyond the IDE. Problem-solving interview questions should test both technical skills and soft skills.
- STAR, SOAR and PREP are methods a candidate can use to answer some non-technical problem-solving interview questions.
- Generic problem-solving interview questions go a long way in gauging a candidate’s fit. But you can go one step further by customizing them according to your company’s service, product, vision, and culture.
Technical Problem-Solving Interview Question Examples
Evaluating a candidates’ problem-solving skills while using coding challenges might seem intimidating. The secret is that coding challenges test many things at the same time — like the candidate’s knowledge of data structures and algorithms, clean code practices, and proficiency in specific programming languages, to name a few examples.
Problem solving itself might at first seem like it’s taking a back seat. But technical problem solving lies at the heart of programming, and most coding questions are designed to test a candidate’s problem-solving abilities.
Here are a few examples of technical problem-solving questions:
1. Mini-Max Sum
This well-known challenge, which asks the interviewee to find the maximum and minimum sum among an array of given numbers, is based on a basic but important programming concept called sorting, as well as integer overflow. It tests the candidate’s observational skills, and the answer should elicit a logical, ad-hoc solution.
2. Organizing Containers of Balls
This problem tests the candidate’s knowledge of a variety of programming concepts, like 2D arrays, sorting and iteration. Organizing colored balls in containers based on various conditions is a common question asked in competitive examinations and job interviews, because it’s an effective way to test multiple facets of a candidate’s problem-solving skills.
3. Build a Palindrome
This is a tough problem to crack, and the candidate’s knowledge of concepts like strings and dynamic programming plays a significant role in solving this challenge. This problem-solving example tests the candidate’s ability to think on their feet as well as their ability to write clean, optimized code.
4. Subarray Division
Based on a technique used for searching pairs in a sorted array ( called the “two pointers” technique ), this problem can be solved in just a few lines and judges the candidate’s ability to optimize (as well as basic mathematical skills).
5. The Grid Search
This is a problem of moderate difficulty and tests the candidate’s knowledge of strings and searching algorithms, the latter of which is regularly tested in developer interviews across all levels.
Common Non-Technical Problem-Solving Interview Questions
Testing a candidate’s problem-solving skills goes beyond the IDE . Everyday situations can help illustrate competency, so here are a few questions that focus on past experiences and hypothetical situations to help interviewers gauge problem-solving skills.
1. Given the problem of selecting a new tool to invest in, where and how would you begin this task?
Key Insight : This question offers insight into the candidate’s research skills. Ideally, they would begin by identifying the problem, interviewing stakeholders, gathering insights from the team, and researching what tools exist to best solve for the team’s challenges and goals.
2. Have you ever recognized a potential problem and addressed it before it occurred?
Key Insight: Prevention is often better than cure. The ability to recognize a problem before it occurs takes intuition and an understanding of business needs.
3. A teammate on a time-sensitive project confesses that he’s made a mistake, and it’s putting your team at risk of missing key deadlines. How would you respond?
Key Insight: Sometimes, all the preparation in the world still won’t stop a mishap. Thinking on your feet and managing stress are skills that this question attempts to unearth. Like any other skill, they can be cultivated through practice.
4. Tell me about a time you used a unique problem-solving approach.
Key Insight: Creativity can manifest in many ways, including original or novel ways to tackle a problem. Methods like the 10X approach and reverse brainstorming are a couple of unique approaches to problem solving.
5. Have you ever broken rules for the “greater good?” If yes, can you walk me through the situation?
Key Insight: “Ask for forgiveness, not for permission.” It’s unconventional, but in some situations, it may be the mindset needed to drive a solution to a problem.
6. Tell me about a weakness you overcame at work, and the approach you took.
Key Insight: According to Compass Partnership , “self-awareness allows us to understand how and why we respond in certain situations, giving us the opportunity to take charge of these responses.” It’s easy to get overwhelmed when faced with a problem. Candidates showing high levels of self-awareness are positioned to handle it well.
7. Have you ever owned up to a mistake at work? Can you tell me about it?
Key Insight: Everybody makes mistakes. But owning up to them can be tough, especially at a workplace. Not only does it take courage, but it also requires honesty and a willingness to improve, all signs of 1) a reliable employee and 2) an effective problem solver.
8. How would you approach working with an upset customer?
Key Insight: With the rise of empathy-driven development and more companies choosing to bridge the gap between users and engineers, today’s tech teams speak directly with customers more frequently than ever before. This question brings to light the candidate’s interpersonal skills in a client-facing environment.
9. Have you ever had to solve a problem on your own, but needed to ask for additional help? How did you go about it?
Key Insight: Knowing when you need assistance to complete a task or address a situation is an important quality to have while problem solving. This questions helps the interviewer get a sense of the candidate’s ability to navigate those waters.
10. Let’s say you disagree with your colleague on how to move forward with a project. How would you go about resolving the disagreement?
Key Insight: Conflict resolution is an extremely handy skill for any employee to have; an ideal answer to this question might contain a brief explanation of the conflict or situation, the role played by the candidate and the steps taken by them to arrive at a positive resolution or outcome.
Strategies for Answering Problem-Solving Questions
If you’re a job seeker, chances are you’ll encounter this style of question in your various interview experiences. While problem-solving interview questions may appear simple, they can be easy to fumble — leaving the interviewer without a clear solution or outcome.
It’s important to approach such questions in a structured manner. Here are a few tried-and-true methods to employ in your next problem-solving interview.
1. Shine in Interviews With the STAR Method
S ituation, T ask, A ction, and R esult is a great method that can be employed to answer a problem-solving or behavioral interview question. Here’s a breakdown of these steps:
- Situation : A good way to address almost any interview question is to lay out and define the situation and circumstances.
- Task : Define the problem or goal that needs to be addressed. Coding questions are often multifaceted, so this step is particularly important when answering technical problem-solving questions.
- Action : How did you go about solving the problem? Try to be as specific as possible, and state your plan in steps if you can.
- Result : Wrap it up by stating the outcome achieved.
2. Rise above difficult questions using the SOAR method
A very similar approach to the STAR method, SOAR stands for S ituation, O bstacle, A ction, and R esults .
- Situation: Explain the state of affairs. It’s important to steer clear of stating any personal opinions in this step; focus on the facts.
- Obstacle: State the challenge or problem you faced.
- Action: Detail carefully how you went about overcoming this obstacle.
- Result: What was the end result? Apart from overcoming the obstacle, did you achieve anything else? What did you learn in the process?
3. Do It the PREP Way
Traditionally used as a method to make effective presentations, the P oint, R eason, E xample, P oint method can also be used to answer problem-solving interview questions.
- Point : State the solution in plain terms.
- Reasons: Follow up the solution by detailing your case — and include any data or insights that support your solution.
- Example: In addition to objective data and insights, drive your answer home by contextualizing the solution in a real-world example.
- Point : Reiterate the solution to make it come full circle.
How to Customize Problem-Solving Interview Questions
Generic problem-solving interview questions go a long way in gauging a candidate’s skill level, but recruiters can go one step further by customizing these problem-solving questions according to their company’s service, product, vision, or culture.
Here are some tips to do so:
- Break down the job’s responsibilities into smaller tasks. Job descriptions may contain ambiguous responsibilities like “manage team projects effectively.” To formulate an effective problem-solving question, envision what this task might look like in a real-world context and develop a question around it.
- Tailor questions to the role at hand. Apart from making for an effective problem-solving question, it gives the candidate the impression you’re an informed technical recruiter. For example, an engineer will likely have attended many scrums. So, a good question to ask is: “Suppose you notice your scrums are turning unproductive. How would you go about addressing this?”
- Consider the tools and technologies the candidate will use on the job. For example, if Jira is the primary project management tool, a good problem-solving interview question might be: “Can you tell me about a time you simplified a complex workflow — and the tools you used to do so?”
- If you don’t know where to start, your company’s core values can often provide direction. If one of the core values is “ownership,” for example, consider asking a question like: “Can you walk us through a project you owned from start to finish?”
- Sometimes, developing custom content can be difficult even with all these tips considered. Our platform has a vast selection of problem-solving examples that are designed to help recruiters ask the right questions to help nail their next technical interview.
Get started with HackerRank
Over 2,500 companies and 40% of developers worldwide use HackerRank to hire tech talent and sharpen their skills.
Recommended topics
- Coding Questions
- Interview Preparation
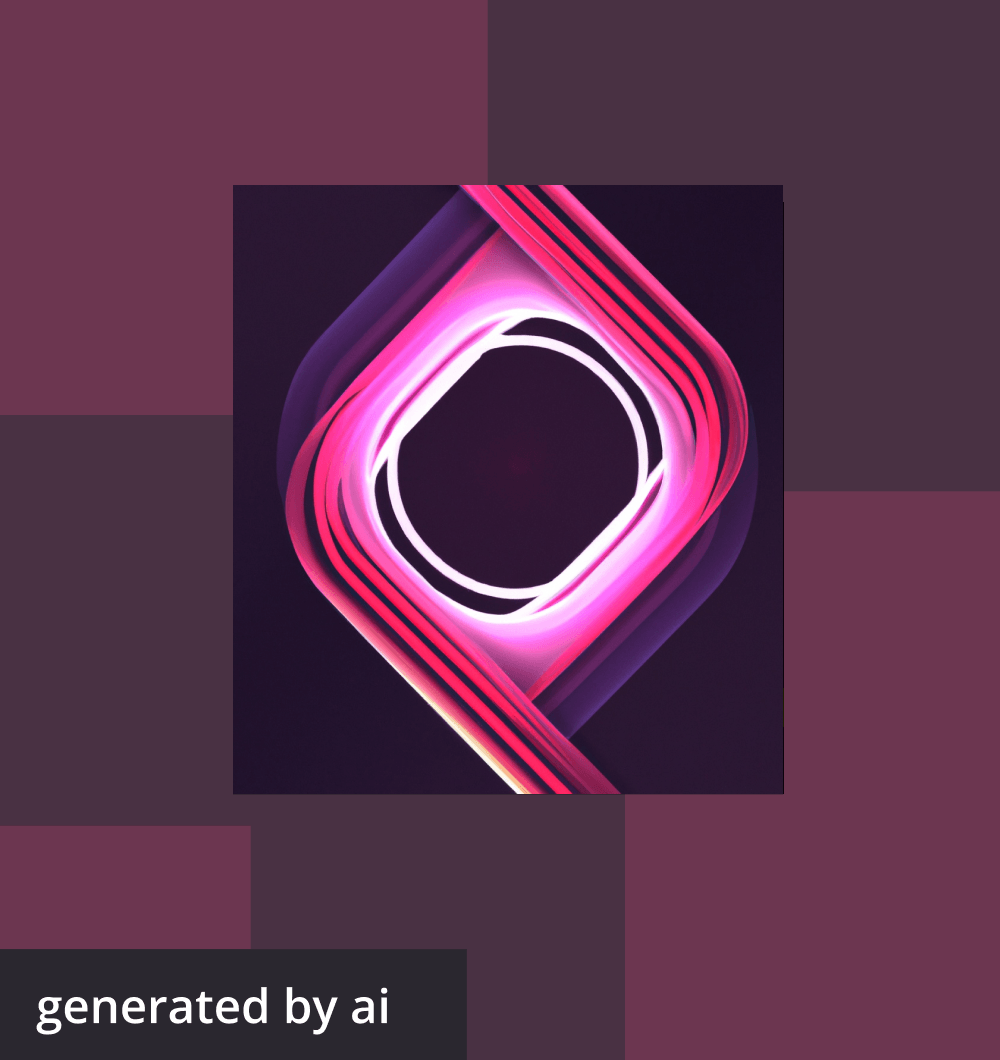
6 REST API Interview Questions Every Developer Should Know
Top 20 Problem Solving Interview Questions (Example Answers Included)
Mike Simpson 0 Comments

By Mike Simpson
When candidates prepare for interviews, they usually focus on highlighting their leadership, communication, teamwork, and similar crucial soft skills . However, not everyone gets ready for problem-solving interview questions. And that can be a big mistake.
Problem-solving is relevant to nearly any job on the planet. Yes, it’s more prevalent in certain industries, but it’s helpful almost everywhere.
Regardless of the role you want to land, you may be asked to provide problem-solving examples or describe how you would deal with specific situations. That’s why being ready to showcase your problem-solving skills is so vital.
If you aren’t sure who to tackle problem-solving questions, don’t worry, we have your back. Come with us as we explore this exciting part of the interview process, as well as some problem-solving interview questions and example answers.
What Is Problem-Solving?
When you’re trying to land a position, there’s a good chance you’ll face some problem-solving interview questions. But what exactly is problem-solving? And why is it so important to hiring managers?
Well, the good folks at Merriam-Webster define problem-solving as “the process or act of finding a solution to a problem.” While that may seem like common sense, there’s a critical part to that definition that should catch your eye.
What part is that? The word “process.”
In the end, problem-solving is an activity. It’s your ability to take appropriate steps to find answers, determine how to proceed, or otherwise overcome the challenge.
Being great at it usually means having a range of helpful problem-solving skills and traits. Research, diligence, patience, attention-to-detail , collaboration… they can all play a role. So can analytical thinking , creativity, and open-mindedness.
But why do hiring managers worry about your problem-solving skills? Well, mainly, because every job comes with its fair share of problems.
While problem-solving is relevant to scientific, technical, legal, medical, and a whole slew of other careers. It helps you overcome challenges and deal with the unexpected. It plays a role in troubleshooting and innovation. That’s why it matters to hiring managers.
How to Answer Problem-Solving Interview Questions
Okay, before we get to our examples, let’s take a quick second to talk about strategy. Knowing how to answer problem-solving interview questions is crucial. Why? Because the hiring manager might ask you something that you don’t anticipate.
Problem-solving interview questions are all about seeing how you think. As a result, they can be a bit… unconventional.
These aren’t your run-of-the-mill job interview questions . Instead, they are tricky behavioral interview questions . After all, the goal is to find out how you approach problem-solving, so most are going to feature scenarios, brainteasers, or something similar.
So, having a great strategy means knowing how to deal with behavioral questions. Luckily, there are a couple of tools that can help.
First, when it comes to the classic approach to behavioral interview questions, look no further than the STAR Method . With the STAR method, you learn how to turn your answers into captivating stories. This makes your responses tons more engaging, ensuring you keep the hiring manager’s attention from beginning to end.
Now, should you stop with the STAR Method? Of course not. If you want to take your answers to the next level, spend some time with the Tailoring Method , too.
With the Tailoring Method, it’s all about relevance. So, if you get a chance to choose an example that demonstrates your problem-solving skills, this is really the way to go.
We also wanted to let you know that we created an amazing free cheat sheet that will give you word-for-word answers for some of the toughest interview questions you are going to face in your upcoming interview. After all, hiring managers will often ask you more generalized interview questions!
Click below to get your free PDF now:
Get Our Job Interview Questions & Answers Cheat Sheet!
FREE BONUS PDF CHEAT SHEET: Get our " Job Interview Questions & Answers PDF Cheat Sheet " that gives you " word-word sample answers to the most common job interview questions you'll face at your next interview .
CLICK HERE TO GET THE JOB INTERVIEW QUESTIONS CHEAT SHEET
Top 3 Problem-Solving-Based Interview Questions
Alright, here is what you’ve been waiting for: the problem-solving questions and sample answers.
While many questions in this category are job-specific, these tend to apply to nearly any job. That means there’s a good chance you’ll come across them at some point in your career, making them a great starting point when you’re practicing for an interview.
So, let’s dive in, shall we? Here’s a look at the top three problem-solving interview questions and example responses.
1. Can you tell me about a time when you had to solve a challenging problem?
In the land of problem-solving questions, this one might be your best-case scenario. It lets you choose your own problem-solving examples to highlight, putting you in complete control.
When you choose an example, go with one that is relevant to what you’ll face in the role. The closer the match, the better the answer is in the eyes of the hiring manager.
EXAMPLE ANSWER:
“While working as a mobile telecom support specialist for a large organization, we had to transition our MDM service from one vendor to another within 45 days. This personally physically handling 500 devices within the agency. Devices had to be gathered from the headquarters and satellite offices, which were located all across the state, something that was challenging even without the tight deadline. I approached the situation by identifying the location assignment of all personnel within the organization, enabling me to estimate transit times for receiving the devices. Next, I timed out how many devices I could personally update in a day. Together, this allowed me to create a general timeline. After that, I coordinated with each location, both expressing the urgency of adhering to deadlines and scheduling bulk shipping options. While there were occasional bouts of resistance, I worked with location leaders to calm concerns and facilitate action. While performing all of the updates was daunting, my approach to organizing the event made it a success. Ultimately, the entire transition was finished five days before the deadline, exceeding the expectations of many.”
2. Describe a time where you made a mistake. What did you do to fix it?
While this might not look like it’s based on problem-solving on the surface, it actually is. When you make a mistake, it creates a challenge, one you have to work your way through. At a minimum, it’s an opportunity to highlight problem-solving skills, even if you don’t address the topic directly.
When you choose an example, you want to go with a situation where the end was positive. However, the issue still has to be significant, causing something negative to happen in the moment that you, ideally, overcame.
“When I first began in a supervisory role, I had trouble setting down my individual contributor hat. I tried to keep up with my past duties while also taking on the responsibilities of my new role. As a result, I began rushing and introduced an error into the code of the software my team was updating. The error led to a memory leak. We became aware of the issue when the performance was hindered, though we didn’t immediately know the cause. I dove back into the code, reviewing recent changes, and, ultimately, determined the issue was a mistake on my end. When I made that discovery, I took several steps. First, I let my team know that the error was mine and let them know its nature. Second, I worked with my team to correct the issue, resolving the memory leak. Finally, I took this as a lesson about delegation. I began assigning work to my team more effectively, a move that allowed me to excel as a manager and help them thrive as contributors. It was a crucial learning moment, one that I have valued every day since.”
3. If you identify a potential risk in a project, what steps do you take to prevent it?
Yes, this is also a problem-solving question. The difference is, with this one, it’s not about fixing an issue; it’s about stopping it from happening. Still, you use problem-solving skills along the way, so it falls in this question category.
If you can, use an example of a moment when you mitigated risk in the past. If you haven’t had that opportunity, approach it theoretically, discussing the steps you would take to prevent an issue from developing.
“If I identify a potential risk in a project, my first step is to assess the various factors that could lead to a poor outcome. Prevention requires analysis. Ensuring I fully understand what can trigger the undesired event creates the right foundation, allowing me to figure out how to reduce the likelihood of those events occurring. Once I have the right level of understanding, I come up with a mitigation plan. Exactly what this includes varies depending on the nature of the issue, though it usually involves various steps and checks designed to monitor the project as it progresses to spot paths that may make the problem more likely to happen. I find this approach effective as it combines knowledge and ongoing vigilance. That way, if the project begins to head into risky territory, I can correct its trajectory.”
17 More Problem-Solving-Based Interview Questions
In the world of problem-solving questions, some apply to a wide range of jobs, while others are more niche. For example, customer service reps and IT helpdesk professionals both encounter challenges, but not usually the same kind.
As a result, some of the questions in this list may be more relevant to certain careers than others. However, they all give you insights into what this kind of question looks like, making them worth reviewing.
Here are 17 more problem-solving interview questions you might face off against during your job search:
- How would you describe your problem-solving skills?
- Can you tell me about a time when you had to use creativity to deal with an obstacle?
- Describe a time when you discovered an unmet customer need while assisting a customer and found a way to meet it.
- If you were faced with an upset customer, how would you diffuse the situation?
- Tell me about a time when you had to troubleshoot a complex issue.
- Imagine you were overseeing a project and needed a particular item. You have two choices of vendors: one that can deliver on time but would be over budget, and one that’s under budget but would deliver one week later than you need it. How do you figure out which approach to use?
- Your manager wants to upgrade a tool you regularly use for your job and wants your recommendation. How do you formulate one?
- A supplier has said that an item you need for a project isn’t going to be delivered as scheduled, something that would cause your project to fall behind schedule. What do you do to try and keep the timeline on target?
- Can you share an example of a moment where you encountered a unique problem you and your colleagues had never seen before? How did you figure out what to do?
- Imagine you were scheduled to give a presentation with a colleague, and your colleague called in sick right before it was set to begin. What would you do?
- If you are given two urgent tasks from different members of the leadership team, both with the same tight deadline, how do you choose which to tackle first?
- Tell me about a time you and a colleague didn’t see eye-to-eye. How did you decide what to do?
- Describe your troubleshooting process.
- Tell me about a time where there was a problem that you weren’t able to solve. What happened?
- In your opening, what skills or traits make a person an exceptional problem-solver?
- When you face a problem that requires action, do you usually jump in or take a moment to carefully assess the situation?
- When you encounter a new problem you’ve never seen before, what is the first step that you take?
Putting It All Together
At this point, you should have a solid idea of how to approach problem-solving interview questions. Use the tips above to your advantage. That way, you can thrive during your next interview.
FREE : Job Interview Questions & Answers PDF Cheat Sheet!
Download our " Job Interview Questions & Answers PDF Cheat Sheet " that gives you word-for-word sample answers to some of the most common interview questions including:
- What Is Your Greatest Weakness?
- What Is Your Greatest Strength?
- Tell Me About Yourself
- Why Should We Hire You?
Click Here To Get The Job Interview Questions & Answers Cheat Sheet

Co-Founder and CEO of TheInterviewGuys.com. Mike is a job interview and career expert and the head writer at TheInterviewGuys.com.
His advice and insights have been shared and featured by publications such as Forbes , Entrepreneur , CNBC and more as well as educational institutions such as the University of Michigan , Penn State , Northeastern and others.
Learn more about The Interview Guys on our About Us page .
About The Author
Mike simpson.

Co-Founder and CEO of TheInterviewGuys.com. Mike is a job interview and career expert and the head writer at TheInterviewGuys.com. His advice and insights have been shared and featured by publications such as Forbes , Entrepreneur , CNBC and more as well as educational institutions such as the University of Michigan , Penn State , Northeastern and others. Learn more about The Interview Guys on our About Us page .
Copyright © 2024 · TheInterviewguys.com · All Rights Reserved
- Our Products
- Case Studies
- Interview Questions
- Jobs Articles
- Members Login

Top 34 C# Coding Interview Questions (SOLVED) To Crack Your Tech Interview
Being powerful, flexible, and well-supported has meant C# has quickly become one of the most popular programming languages available. Today, it is the 4th most popular programming language, with approximately 31% of all developers using it regularly. Follow along and check 34 most common C# Coding Interview Questions (SOLVED) for mid and experienced developers before your next tech interview.
Q1 : Can this be used within a Static method?
We can't use this in static method because keyword this returns a reference to the current instance of the class containing it.
Static methods (or any static member) do not belong to a particular instance. They exist without creating an instance of the class and call with the name of a class not by instance so we can't use this keyword in the body of static Methods, but in case of Extension Methods we can use it as the functions parameters.
Q2 : Implement a Queue using two Stacks
Suppose we have two stacks and no other temporary variable. Is to possible to "construct" a queue data structure using only the two stacks?
Keep two stacks, let's call them inbox and outbox .
- Push the new element onto inbox
- If outbox is empty, refill it by popping each element from inbox and pushing it onto outbox
- Pop and return the top element from outbox
Space: O(1)
In the worst case scenario when outbox stack is empty, the algorithm pops n elements from inbox stack and pushes n elements to outbox, where n is the queue size. This gives 2*n operations, which is O(n) . But when outbox stack is not empty the algorithm has O(1) time complexity that gives amortised O(1) .
Q3 : What are the different types of classes in C#?
The different types of class in C# are:
- Partial class – Allows its members to be divided or shared with multiple .cs files. It is denoted by the keyword Partial.
- Sealed class – It is a class which cannot be inherited. To access the members of a sealed class, we need to create the object of the class. It is denoted by the keyword Sealed .
- Abstract class – It is a class whose object cannot be instantiated. The class can only be inherited. It should contain at least one method. It is denoted by the keyword abstract.
- Static class – It is a class which does not allow inheritance. The members of the class are also static. It is denoted by the keyword static . This keyword tells the compiler to check for any accidental instances of the static class.
Q4 : What is the difference between a Struct and a Class in C#?
Class and struct both are the user defined data type but have some major difference:
- The struct is value type in C# and it inherits from System.Value Type.
- Struct is usually used for smaller amounts of data.
- Struct can't be inherited to other type.
- A structure can't be abstract.
- The class is reference type in C# and it inherits from the System.Object Type.
- Classes are usually used for large amounts of data.
- Classes can be inherited to other class.
- A class can be abstract type.
- We can create a default constructor .
Q5 : Why is the virtual keyword used in code?
The virtual keyword is used while defining a class to specify that the methods and the properties of that class can be overridden in derived classes.
Q6 : Why to use finally block in C#?
Finally block will be executed irrespective of exception . So while executing the code in try block when exception is occurred, control is returned to catch block and at last finally block will be executed. So closing connection to database / releasing the file handlers can be kept in finally block.
Q7 : Explain how does the Sentinel Search work?
The idea behind linear search is to compare the search item with the elements in the list one by one (using a loop) and stop as soon as we get the first copy of the search element in the list. Now considering the worst case in which the search element does not exist in the list of size N then the Simple Linear Search will take a total of 2N+1 comparisons ( N comparisons against every element in the search list and N+1 comparisons to test against the end of the loop condition).
The idea of Sentinel Linear Search is to reduce the number of comparisons required to find an element in a list. Here we replace the last element of the list with the search element itself and run a while loop to see if there exists any copy of the search element in the list and quit the loop as soon as we find the search element. This will reduce one comparison in each iteration.
In that case the while loop makes only one comparison in each iteration and it is sure that it will terminate since the last element of the list is the search element itself. So in the worst case ( if the search element does not exists in the list ) then there will be at most N+2 comparisons ( N comparisons in the while loop and 2 comparisons in the if condition). Which is better than ( 2N+1 ) comparisons as found in Simple Linear Search .
Take note that both the algorithms (Simple Linear and Sentinel) have time complexity of O ( n ) .
Q8 : Given an array of ints, write a C# method to total all the values that are even numbers.
Q9 : how can you prevent a class from overriding in c#.
You can prevent a class from overriding in C# by using the sealed keyword.
Q10 : How to check if two Strings (words) are Anagrams ?
Explain what is space complexity of that solution?
Two words are anagrams of each other if they contain the same number of characters and the same characters. There are two solutions to mention:
- You should only need to sort the characters in lexicographic order, and determine if all the characters in one string are equal to and in the same order as all of the characters in the other string. If you sort either array, the solution becomes O(n log n) .
- Hashmap approach where key - letter and value - frequency of letter,
- for first string populate the hashmap O(n)
- for second string decrement count and remove element from hashmap O(n)
- if hashmap is empty, the string is anagram otherwise not.
The major space cost in your code is the hashmap which may contain a frequency counter for each lower case letter from a to z . So in this case, the space complexity is supposed to be constant O(26) .
To make it more general, the space complexity of this problem is related to the size of alphabet for your input string. If the input string only contains ASCII characters, then for the worst case you will have O(256) as your space cost. If the alphabet grows to the UNICODE set, then the space complexity will be much worse in the worst scenario.
So in general its O(size of alphabet) .
Q11 : Is there a difference between throw and throw ex ?
Yes, there is a difference:
- When you do throw ex , that thrown exception becomes the "original" one. So all previous stack trace will not be there.
If you do throw , the exception just goes down the line and you'll get the full stack trace.
Q12 : Refactor the code
Is there a way to modify ClassA so that you can you call the constructor with parameters, when the Main method is called, without creating any other new instances of the ClassA ?
The this keyword is used to call other constructors, to initialize the class object. The following shows the implementation:
Q13 : Reverse the ordering of words in a String
I have this string
and I want to reverse the order of the words so that
Is it possible to do it in-place (without using additional data structures) and with the time complexity being O(n) ?
Reverse the entire string, then reverse the letters of each individual word.
After the first pass the string will be
and after the second pass it will be
Reversing the entire string is easily done in O(n) , and reversing each word in a string is also easy to do in O(n) . O(n)+O(n) = O(n) . Space complexity is O(1) as reversal done in-place .
Q14 : What is the difference between Equality Operator ( == ) and Equals() Method in C#?
The == Operator (usually means the same as ReferenceEquals , could be overrided) compares the reference identity while the Equals() ( virtual Equals() ) method compares if two objects are equivalent.
Q15 : What is the output of the program below? Explain.
This program will output the number 10 ten times.
Here’s why: The delegate is added in the for loop and “reference” (or perhaps “pointer” would be a better choice of words) to i is stored, rather than the value itself. Therefore, after we exit the loop, the variable i has been set to 10, so by the time each delegate is invoked, the value passed to all of them is 10.
Q16 : What is the use of Null Coalescing Operator ( ?? ) in C#?
The ?? operator is called the null-coalescing operator and is used to define a default value for nullable value types or reference types. It returns the left-hand operand if the operand is not null; otherwise, it returns the right operand.
Q17 : Binet's formula: How to calculate Fibonacci numbers without Recursion or Iteration?
There is actually a simple mathematical formula called Binet's formula for computing the nth Fibonacci number, which does not require the calculation of the preceding numbers:
It features the Golden Ratio (φ) :
The iterative approach scales linearly, while the formula approach is basically constant time.
Time: O(log n)
Space: None
Assuming that the primitive mathematical operations (+, -, * and /) are O(1) you can use this result to compute the n th Fibonacci number in O(log n) time ( O(log n) because of the exponentiation in the formula).
Q18 : Can you create a function in C# which can accept varying number of arguments?
By using the params keyword, you can specify a method parameter that takes a variable number of arguments. No additional parameters are permitted after the params keyword in a method declaration, and only one params keyword is permitted in a method declaration. The declared type of the params parameter must be a single-dimensional array.
Q19 : Does .NET support Multiple Inheritance ?
.NET does not support multiple inheritance directly because in .NET, a class cannot inherit from more than one class. .NET supports multiple inheritance through interfaces.
Q20 : Explain what is Ternary Search?
Like linear search and binary search, ternary search is a searching technique that is used to determine the position of a specific value in an array. In binary search, the sorted array is divided into two parts while in ternary search, it is divided into 3 parts and then you determine in which part the element exists.
Ternary search, like binary search, is a divide-and-conquer algorithm. It is mandatory for the array (in which you will search for an element) to be sorted before you begin the search. In a ternary search, there is a possibility (33.33% chance, actually) that you can eliminate 2/3 of the list, but there is an even greater chance (66.66%) that you will only eliminate 1/3 of the list.
Ternary search has a lesser number of iterations than Binary Search but Ternary search also leads to more comparisons .
Average number of comparisons:
Worst number of comparisons:
Q21 : Explain what is Fibonacci Search technique?
Fibonacci search is a search algorithm based on divide and conquer principle that can find an element in the given sorted array with the help of Fibonacci series in O ( log n ) time complexity.
Compared to binary search where the sorted array is divided into two equal-sized parts, one of which is examined further, Fibonacci search divides the array into two unequal parts that have sizes that are consecutive Fibonacci numbers .
Some facts to note:
- Fibonacci search has the advantage that one only needs addition and subtraction to calculate the indices of the accessed array elements, while classical binary search needs bit-shift, division or multiplication, operations that were less common at the time Fibonacci search was first published
- Binary search works by dividing the seek area in equal parts 1:1 . Fibonacci search can divide it into parts approaching 1:1.618 while using the simpler operations.
- On average, Fibonacci search requires 4% more comparisons than binary search
- Fibonacci Search examines relatively closer elements in subsequent steps. So when input array is big that cannot fit in CPU cache or even in RAM, Fibonacci Search can be useful. Thus Fibonacci search may have the advantage over binary search in slightly reducing the average time needed to access a non-uniform access memory storage.
Let the length of given array be length and the element to be searched be key .
Steps of Fibonacci Search:
- Find the smallest Fibonacci number F(n) that F(n) - 1 >= length
F(n) = F(n-1) + F(n-2) => F(n) - 1 = [ F(n-1) - 1 ] + (1) + [ F(n-2) - 1 ] , where (1) here is for the middle item (the m in below figure).
If key < m , then search key in q = F(n-1) - 1 :
for that set p = F(n-1) - 1, q = F(n-2) - 1, r = F(n-3) - 1 and repeat the process. Here Fibonacci numbers go backward once . These indicate elimination of approximately one-third of the remaining array.
If key > m , then repeat then search key in r = F(n-2) - 1 :
For that, set p = F(n-2) - 1, q = F(n-3) - 1, r = F(n-4) - 1 and repeat the process. Here Fibonacci numbers go backward twice , indicating elimination of approximately two-third of the remaining array.
Fibonacci search has an average- and worst-case complexity of O ( log n ) . The worst case will occur when we have our target in the larger (2/3) fraction of the array, as we proceed to find it. In other words, we are eliminating the smaller (1/3) fraction of the array every time. We call once for n, then for (2/3) n, then for (4/9) n and henceforth.
Q22 : Find Merge (Intersection) Point of Two Linked Lists
Say, there are two lists of different lengths, merging at a point ; how do we know where the merging point is?
Conditions:
- We don't know the length
This arguably violates the "parse each list only once" condition, but implement the tortoise and hare algorithm (used to find the merge point and cycle length of a cyclic list) so you start at List 1, and when you reach the NULL at the end you pretend it's a pointer to the beginning of List 2, thus creating the appearance of a cyclic list. The algorithm will then tell you exactly how far down List 1 the merge is.
Algorithm steps:
- it goes forward every time till the end, and then
- jumps to the beginning of the opposite list, and so on.
- Create two of these, pointing to two heads.
- Advance each of the pointers by 1 every time, until they meet in intersection point ( IP ). This will happen after either one or two passes.
To understand it count the number of nodes traveled from head1-> tail1 -> head2 -> intersection point and head2 -> tail2-> head1 -> intersection point . Both will be equal (Draw diff types of linked lists to verify this). Reason is both pointers have to travel same distances head1-> IP + head2->IP before reaching IP again. So by the time it reaches IP , both pointers will be equal and we have the merging point.
Q23 : Is the comparison of time and null in the if statement below valid or not? Why or why not?
One might think that, since a DateTime variable can never be null (it is automatically initialized to Jan 1, 0001), the compiler would complain when a DateTime variable is compared to null . However, due to type coercion, the compiler does allow it, which can potentially lead to headfakes and pull-out-your-hair bugs.
Specifically, the == operator will cast its operands to different allowable types in order to get a common type on both sides, which it can then compare. That is why something like this will give you the result you expect (as opposed to failing or behaving unexpectedly because the operands are of different types):
However, this can sometimes result in unexpected behavior, as is the case with the comparison of a DateTime variable and null . In such a case, both the DateTime variable and the null literal can be cast to Nullable<DateTime> . Therefore it is legal to compare the two values, even though the result will always be false.
Q24 : Test if a Number belongs to the Fibonacci Series
A positive integer ω is a Fibonacci number if and only one of:
- 5ω 2 + 4 and
is a perfect square or a square number .
A square number is the result when a number has been multiplied by itself.
Note : it involves squaring a Fibonacci number, frequently resulting in a massive product. There are less than 80 Fibonacci numbers that can even be held in a standard 64-bit integer.
Q25 : What is the output of the program below?
TestValue : 10
The static constructor of a class is called before any instance of the class is created. The static constructor called here initializes the TestValue variable first.
Q26 : What is the output of the program below? Explain your answer.
Also, would the answer change if we were to replace await Task.Delay(5); with Thread.Sleep(5) ? Why or why not?
The answer to the first part of the question (i.e., the version of the code with await Task.Delay(5); ) is that the program will just output a blank line ( not “Hello world!”). This is because result will still be uninitialized when Console.WriteLine is called.
Most procedural and object-oriented programmers expect a function to execute from beginning to end, or to a return statement, before returning to the calling function. This is not the case with C# async functions. They only execute up until the first await statement, then return to the caller. The function called by await (in this case Task.Delay ) is executed asynchronously, and the line after the await statement isn’t signaled to execute until Task.Delay completes (in 5 milliseconds). However, within that time, control has already returned to the caller, which executes the Console.WriteLine statement on a string that hasn’t yet been initialized.
Calling await Task.Delay(5) lets the current thread continue what it is doing, and if it’s done (pending any awaits), returns it to the thread pool. This is the primary benefit of the async/await mechanism. It allows the CLR to service more requests with less threads in the thread pool.
Asynchronous programming has become a lot more common, with the prevalence of devices which perform over-the-network service requests or database requests for many activities. C# has some excellent programming constructs which greatly ease the task of programming asynchronous methods, and a programmer who is aware of them will produce better programs.
With regard to the second part of the question, if await Task.Delay(5); was replaced with Thread.Sleep(5) , the program would output Hello world! . An async method without at least one await statement in it operates just like a synchronous method; that is, it will execute from beginning to end, or until it encounters a return statement. Calling Thread.Sleep() simply blocks the currently running thread, so the Thread.Sleep(5) call just adds 5 milliseconds to the execution time of the SaySomething() method.
Q27 : What is the output of the short program below? Explain your answer.
The output will be:
Although both variables are uninitialized, String is a reference type and DateTime is a value type. As a value type, an unitialized DateTime variable is set to a default value of midnight of 1/1/1 (yup, that’s the year 1 A.D.), not null .
Q28 : What's the advantage of using getters and setters - that only get and set - instead of simply using public fields for those variables?
There are actually many good reasons to consider using accessors rather than directly exposing fields of a class - beyond just the argument of encapsulation and making future changes easier.
Here are the some of the reasons I am aware of:
- Encapsulation of behavior associated with getting or setting the property - this allows additional functionality (like validation) to be added more easily later.
- Hiding the internal representation of the property while exposing a property using an alternative representation.
- Insulating your public interface from change - allowing the public interface to remain constant while the implementation changes without affecting existing consumers.
- Controlling the lifetime and memory management (disposal) semantics of the property - particularly important in non-managed memory environments (like C++ or Objective-C).
- Providing a debugging interception point for when a property changes at runtime - debugging when and where a property changed to a particular value can be quite difficult without this in some languages.
- Improved interoperability with libraries that are designed to operate against property getter/setters - Mocking, Serialization, and WPF come to mind.
- Allowing inheritors to change the semantics of how the property behaves and is exposed by overriding the getter/setter methods.
- Allowing the getter/setter to be passed around as lambda expressions rather than values.
- Getters and setters can allow different access levels - for example the get may be public, but the set could be protected.
Q29 : Calculate the circumference of the circle
Given an instance circle of the following class:
The preferred answer would be of the form:
Since we don’t have access to the private radius field of the object, we tell the object itself to calculate the circumference, by passing it the calculation function inline.
A lot of C# programmers shy away from (or don’t understand) function-valued parameters. While in this case the example is a little contrived, the purpose is to see if the applicant understands how to formulate a call to Calculate which matches the method’s definition.
Alternatively, a valid (though less elegant) solution would be to retrieve the radius value itself from the object and then perform the calculation with the result:
Either way works. The main thing we’re looking for here is to see that the candidate is familiar with, and understands how to invoke, the Calculate method.
Q30 : Could you elaborate Polymorphism vs Overriding vs Overloading ?
Polymorphism is the ability of a class instance to behave as if it were an instance of another class in its inheritance tree, most often one of its ancestor classes. For example, in .NET all classes inherit from Object. Therefore, you can create a variable of type Object and assign to it an instance of any class.
An override is a type of function which occurs in a class which inherits from another class. An override function "replaces" a function inherited from the base class, but does so in such a way that it is called even when an instance of its class is pretending to be a different type through polymorphism. Referring to the previous example, you could define your own class and override the toString() function. Because this function is inherited from Object, it will still be available if you copy an instance of this class into an Object-type variable. Normally, if you call toString() on your class while it is pretending to be an Object, the version of toString which will actually fire is the one defined on Object itself. However, because the function is an override, the definition of toString() from your class is used even when the class instance's true type is hidden behind polymorphism.
Overloading is the action of defining multiple methods with the same name, but with different parameters. It is unrelated to either overriding or polymorphism.
Q31 : Implement the Where method in C#. Explain.
The yield keyword actually does quite a lot here. It creates a state machine "under the covers" that remembers where you were on each additional cycle of the function and picks up from there.
The function returns an object that implements the IEnumerable<T> interface. If a calling function starts foreach ing over this object, the function is called again until it "yields" result based on some predicate . This is syntactic sugar introduced in C# 2.0 . In earlier versions you had to create your own IEnumerable and IEnumerator objects to do stuff like this.
Q32 : Why doesn't C# allow static methods to implement an interface ?
My (simplified) technical reason is that static methods are not in the vtable, and the call site is chosen at compile time. It's the same reason you can't have override or virtual static members. For more details, you'd need a CS grad or compiler wonk - of which I'm neither.
You pass an interface to someone, they need to know how to call a method. An interface is just a virtual method table (vtable). Your static class doesn't have that. The caller wouldn't know how to call a method. (Before i read this answer i thought C# was just being pedantic. Now i realize it's a technical limitation, imposed by what an interface is). Other people will talk down to you about how it's a bad design. It's not a bad design - it's a technical limitation.
Q33 : Why prefer Composition over Inheritance ? What trade-offs are there for each approach? When should you choose Inheritance over Composition ?
Think of containment as a has a relationship. A car "has an" engine, a person "has a" name, etc. Think of inheritance as an is a relationship. A car "is a" vehicle, a person "is a" mammal, etc.
Prefer composition over inheritance as it is more malleable / easy to modify later, but do not use a compose-always approach. With composition, it's easy to change behavior on the fly with Dependency Injection / Setters. Inheritance is more rigid as most languages do not allow you to derive from more than one type. So the goose is more or less cooked once you derive from TypeA.
My acid test for the above is:
- Does TypeB want to expose the complete interface (all public methods no less) of TypeA such that TypeB can be used where TypeA is expected? Indicates Inheritance .
e.g. A Cessna biplane will expose the complete interface of an airplane, if not more. So that makes it fit to derive from Airplane.
- Does TypeB want only some/part of the behavior exposed by TypeA? Indicates need for Composition.
e.g. A Bird may need only the fly behavior of an Airplane. In this case, it makes sense to extract it out as an interface / class / both and make it a member of both classes.
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

Download Interview guide PDF
Embedded c interview questions, download pdf.
Embedded C is the most popular choice of language used for developing embedded systems because of its simplicity, efficiency, less time required for development, and its portability from one system to another. As we know that the embedded systems have constraints on hardware resources such as CPU, memory sizes, etc, it becomes very important to use the resources judiciously and responsibly. To achieve this, Embedded C usually can interact with the hardware resources with necessary abstractions.
In this article, we will see the most commonly asked interview questions in Embedded C for both freshers and experienced developers.
Embedded C Interview Questions for Freshers
1. what is embedded c programming how is embedded c different from c language.
Embedded C is a programming language that is an extension of C programming . It uses the same syntax as C and it is called “embedded” because it is used widely in embedded systems. Embedded C supports I/O hardware operations and addressing, fixed-point arithmetic operations, memory/address space access, and various other features that are required to develop fool-proof embedded systems.
Following are the differences between traditional C language and Embedded C:
2. What do you understand by startup code?
A startup code is that piece of code that is called before the execution of the main function. This is used for creating a basic platform for the application and it is written in assembly language.
3. What is ISR?
ISR expands to Interrupt Service Routines. These are the procedures stored at a particular memory location and are called when certain interrupts occur. Interrupt refers to the signal sent to the processor that indicates there is a high-priority event that requires immediate attention. The processor suspends the normal flow of the program, executes the instructions in ISR to cater for the high priority event. Post execution of the ISR, the normal flow of the program resumes. The following diagrams represent the flow of ISR.
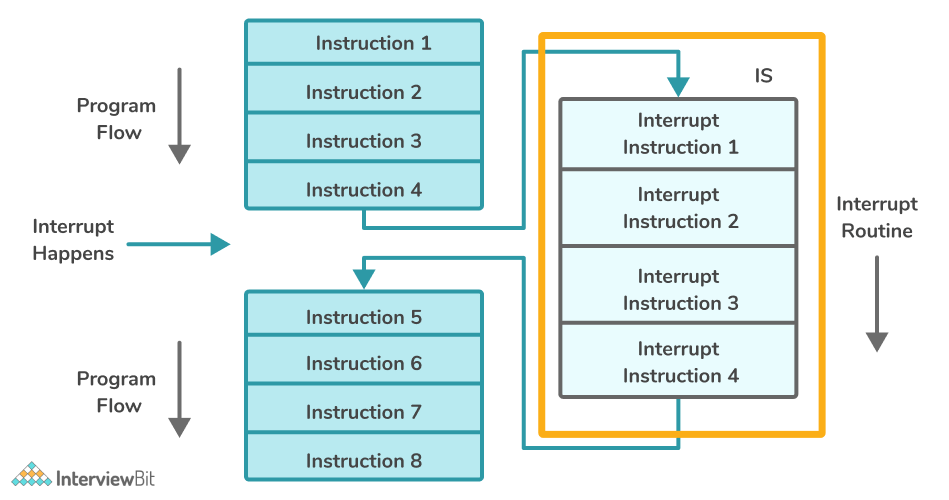
4. What is Void Pointer in Embedded C and why is it used?
Void pointers are those pointers that point to a variable of any type. It is a generic pointer as it is not dependent on any of the inbuilt or user-defined data types while referencing. During dereferencing of the pointer, we require the correct data type to which the data needs to be dereferenced.
For Example:
Void pointers are used mainly because of their nature of re-usability. It is reusable because any type of data can be stored.
5. Why do we use the volatile keyword?
The volatile keyword is mainly used for preventing a compiler from optimizing a variable that might change its behaviour unexpectedly post the optimization. Consider a scenario where we have a variable where there is a possibility of its value getting updated by some event or a signal, then we need to tell the compiler not to optimize it and load that variable every time it is called. To inform the compiler, we use the keyword volatile at the time of variable declaration.
Here, x is an integer variable that is defined as a volatile variable.
- Software Dev
- Data Science
6. What are the differences between the const and volatile qualifiers in embedded C?
7. what is concatenation operator in embedded c.
The Concatenation operator is indicated by the usage of ## . It is used in macros to perform concatenation of the arguments in the macro. We need to keep note that only the arguments are concatenated, not the values of those arguments.
For example, if we have the following piece of code:
We can think of it like this if arguments x and y are passed, then the macro just returns xy -> The concatenation of x and y .
8. What do you understand by Interrupt Latency?
Interrupt latency refers to the time taken by ISR to respond to the interrupt. The lesser the latency faster is the response to the interrupt event.
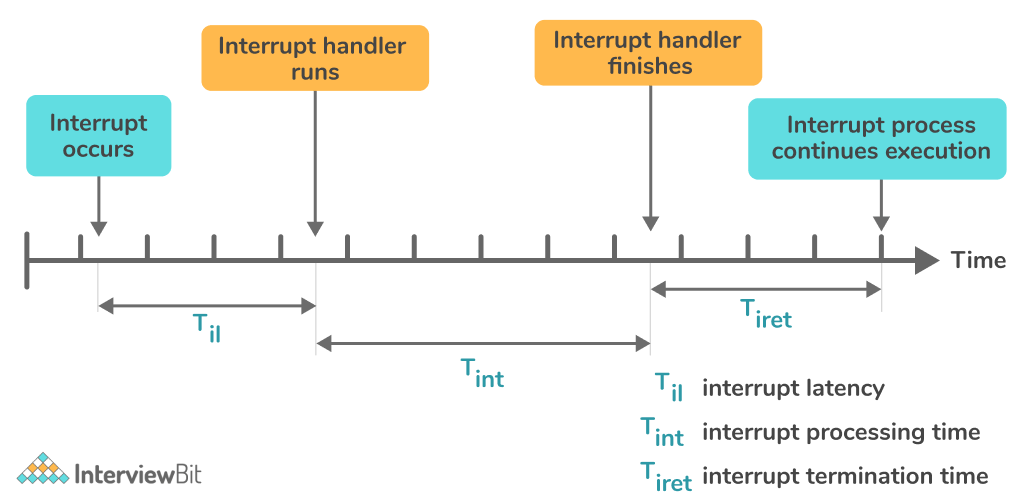
9. How will you use a variable defined in source file1 inside source file2?
We can achieve this by making use of the “extern” keyboard. It allows the variable to be accessible from one file to another. This can be handled more cleanly by creating a header file that just consists of extern variable declarations. This header file is then included in the source files which uses the extern variables. Consider an example where we have a header file named variables.h and a source file named sc_file.c .
10. What do you understand by segmentation fault?
A segmentation fault occurs most commonly and often leads to crashes in the programs. It occurs when a program instruction tries to access a memory address that is prohibited from getting accessed.
11. What are the differences between Inline and Macro Function?
12. is it possible for a variable to be both volatile and const.
The const keyword is used when we want to ensure that the variable value should not be changed. However, the value can still be changed due to external interrupts or events. So, we can use const with volatile keywords and it won’t cause any problem.
13. Is it possible to declare a static variable in a header file?
Variables defined with static are initialized once and persists until the end of the program and are local only to the block it is defined. A static variables declaration requires definition. It can be defined in a header file. But if we do so, a private copy of the variable of the header file will be present in each source file the header is included. This is not preferred and hence it is not recommended to use static variables in a header file.
14. What do you understand by the pre-decrement and post-decrement operators?
The Pre-decrement operator ( --operand ) is used for decrementing the value of the variable by 1 before assigning the variable value.
The Post-decrement operator ( operand-- ) is used for decrementing the value of a variable by 1 after assigning the variable value.
15. What is a reentrant function?
A function is called reentrant if the function can be interrupted in the middle of the execution and be safely called again (re-entered) to complete the execution. The interruption can be in the form of external events or signals or internal signals like call or jump. The reentrant function resumes at the point where the execution was left off and proceeds to completion.
Embedded C Interview Questions for Experienced
1. what kind of loop is better - count up from zero or count down to zero.
Loops that involve count down to zero are better than count-up loops. This is because the compiler can optimize the comparison to zero at the time of loop termination. The processors need not have to load both the loop variable and the maximum value for comparison due to the optimization. Hence, count down to 0 loops are always better.
2. What do you understand by a null pointer in Embedded C?
A null pointer is a pointer that does not point to any valid memory location. It is defined to ensure that the pointer should not be used to modify anything as it is invalid. If no address is assigned to the pointer, it is set to NULL .
One of the uses of the null pointer is that once the memory allocated to a pointer is freed up, we will be using NULL to assign to the pointer so that it does not point to any garbage locations.
3. Following are some incomplete declarations, what do each of them mean?
- The first two declaration points 1 and 2 mean the same. It means that the variable x is a read-only constant integer.
- The third declaration represents that the variable a is a pointer to a constant integer. The integer value can't be modified but the pointer can be modified to point to other locations.
- The fourth declaration means that the variable x is a constant pointer to an integer value. It means that the integer value can be changed, but the pointer can't be made to point to anything else.
- The last declaration means that the variable x is a constant pointer to a constant integer which means that neither the pointer can point to a different location nor the integer value can be modified.
4. Why is the statement ++i faster than i+1?
- ++i instruction uses single machine instruction like INR (Increment Register) to perform the increment.
- For the instruction i+1, it requires to load the value of the variable i and then perform the INR operation on it. Due to the additional load, ++i is faster than the i+1 instruction.
5. What are the reasons for segmentation fault in Embedded C? How do you avoid these errors?
Following are the reasons for the segmentation fault to occur:
- While trying to dereference NULL pointers.
- While trying to write or update the read-only memory or non-existent memory not accessible by the program such as code segment, kernel structures, etc.
- While trying to dereference an uninitialized pointer that might have been pointing to invalid memory.
- While trying to dereference a pointer that was recently freed using the free function.
- While accessing the array beyond the boundary.
Some of the ways where we can avoid Segmentation fault are:
- We can also assign the address of the matrix, vectors or using functions like calloc, malloc etc.
- Only important thing is to assign value to the pointer before accessing it.
- Minimize using pointers: Most of the functions in Embedded C such as scanf, require that address should be sent as a parameter to them. In cases like these, as best practices, we declare a variable and send the address of that variable to that function as shown below:
In the same way, while sending the address of variables to custom-defined functions, we can use the & parameter instead of using pointer variables to access the address.
- Troubleshooting: Make sure that every component of the program like pointers, array subscripts, & operator, * operator, array accessing, etc as they can be likely candidates for segmentation error. Debug the statements line by line to identify the line that causes the error and investigate them.
6. Is it recommended to use printf() inside ISR?
printf() is a non-reentrant and thread-safe function which is why it is not recommended to call inside the ISR.
7. Is it possible to pass a parameter to ISR or return a value from it?
An ISR by nature does not allow anything to pass nor does it return anything. This is because ISR is a routine called whenever hardware or software events occur and is not in control of the code.
8. What Is a Virtual Memory in Embedded C and how can it be implemented?
Virtual memory is a means of allocating memory to the processes if there is a shortage of physical memory by using an automatic allocation of storage. The main advantage of using virtual memory is that it is possible to have larger virtual memory than physical memory. It can be implemented by using the technique of paging.
Paging works as follows:
- Whenever a process needs to be executed, it would be swapped into the memory by using a lazy swapper called a pager.
- The pager tries to guess which page needs to get access to the memory based on a predefined algorithm and swaps that process. This ensures that the whole process is not swapped into the memory, but only the necessary parts of the process are swapped utilizing pages.
- This decreases the time taken to swap and unnecessary reading of memory pages and reduces the physical memory required.
9. What is the issue with the following piece of code?
From the code given, it appears that the function intends to return the square of the values pointed by the pointer p. But, since we have the pointer point to a volatile integer, the compiler generates code as below:
Since the pointer can be changed to point to other locations, it might be possible that the values of the x and y would be different which might not even result in the square of the numbers. Hence, the correct way for achieving the square of the number is by coding as below:
10. The following piece of code uses __interrupt keyword to define an ISR. Comment on the correctness of the code.
Following things are wrong with the given piece of code:
- ISRs are not supposed to return any value. The given code returns a value of datatype double.
- It is not possible to pass parameters to ISRs. Here, we are passing a parameter to the ISR which is wrong.
- It is not advisable to have printf inside the ISR as they are non-reentrant and thereby it impacts the performance.
11. What is the result of the below code?
In Embedded C, we need to know a fact that when expressions are having signed and unsigned operand types, then every operand will be promoted to an unsigned type. Herem the -40 will be promoted to unsigned type thereby making it a very large value when compared to 10. Hence, we will get the statement “Greater than 10” printed on the console.
12. What are the reasons for Interrupt Latency and how to reduce it?
Following are the various causes of Interrupt Latency:
- Hardware : Whenever an interrupt occurs, the signal has to be synchronized with the CPU clock cycles. Depending on the hardware of the processor and the logic of synchronization, it can take up to 3 CPU cycles before the interrupt signal has reached the processor for processing.
- Pipeline : Most of the modern CPUs have instructions pipelined. Execution happens when the instruction has reached the last stage of the pipeline. Once the execution of an instruction is done, it would require some extra CPU cycles to refill the pipeline with instructions. This contributes to the latency.
Interrupt latency can be reduced by ensuring that the ISR routines are short. When a lower priority interrupt gets triggered while a higher priority interrupt is getting executed, then the lower priority interrupt would get delayed resulting in increased latency. In such cases, having smaller ISR routines for lower priority interrupts would help to reduce the delay.
Also, better scheduling and synchronization algorithms in the processor CPU would help minimize the ISR latency.
13. Is it possible to protect a character pointer from accidentally pointing it to a different address?
It can be done by defining it as a constant character pointer. const protects it from modifications.
14. What do you understand by Wild Pointer? How is it different from Dangling Pointer?
A pointer is said to be a wild pointer if it has not been initialized to NULL or a valid memory address. Consider the following declaration:
Here the pointer ptr is not initialized and in the next step, we are trying to assign a valid value to it. If the ptr has a garbage location address, then that would corrupt the upcoming instructions too.
If we are trying to de-allocate this pointer and free it as well using the free function, and again if we are not assigning the pointer as NULL or any valid address, then again chances are that the pointer would still be pointing to the garbage location and accessing from that would lead to errors. These pointers are called dangling pointers.
15. What are the differences between the following 2 statements #include "..." and #include <...>?
Both declarations specify for the files to be included in the current source file. The difference is in how and where the preprocessor looks for including the files. For #include "..." , the preprocessor just searches for the file in the current directory as where the source file is present and if not found, it proceeds to search in the standard directories specified by the compiler. Whereas for the #include <...> declaration, the preprocessor looks for the files in the compiler designated directories where the standard library files usually reside.
16. When does a memory leak occur? What are the ways of avoiding it?
Memory leak is a phenomenon that occurs when the developers create objects or make use of memory to help memory and then forget to free the memory before the completion of the program. This results in reduced system performance due to the reduced memory availability and if this continues, at one point, the application can crash. These are serious issues for applications involving servers, daemons, etc that should ideally never terminate.
Example of Memory Leak:
In this example, we have created pointer p inside the function and we have not freed the pointer before the completion of the function. This causes pointer p to remain in the memory. Imagine 100s of pointers like these. The memory will be occupied unnecessarily and hence resulting in a memory leak.
We can avoid memory leaks by always freeing the objects and pointers when no longer required. The above example can be modified as:
Embedded C Programming
1. write an embedded c program to multiply any number by 9 in the fastest manner..
This can be achieved by involving bit manipulation techniques - Shift left operator as shown below:
2. How will you swap two variables? Write different approaches for the same.
- Using Extra Memory Space:
- Using Arithmetic Operators:
- Using Bit-Wise Operators:
- Using One-liner Bit-wise Operators:
The order of evaluation here is right to left.
- Using One-liner Arithmetic Operators:
Here the order of evaluation is from left to right.
3. Write a program to check if a number is a power of 2 or not.
We can do this by using bitwise operators.
4. Write a program to print numbers from 1 to 100 without making use of conditional operators?
void main (){ int i=0; while (100 – i++) printf ("%d", i); }
5. Write a MIN macro program that takes two arguments and returns the smallest of both arguments.
Useful Resource
- C Interview Questions
C++ Interview Questions
Practice Coding
InterviewBit Blog
- Online C++ Compiler
Embedded C MCQ
Which among the following options best define endianness?
What is the function used for rounding off the value to the nearest value?
Which among the below options are true with regards to the below list of definitions:
Which among the below options are correct for the following declaration of code?
Which among the below options are correct for defining a macro that returns a square of two arguments?
Which among the below options are correct for const and volatile?
What does (void*)ptr represent?
Which among the below options does the task of processing enum types?
Which among the following will happen if we try to assign a value to the array index whose subscript exceeds the array size?
How will you free the allocated memory?
- Privacy Policy
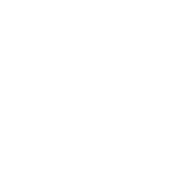
- Practice Questions
- Programming
- System Design
- Fast Track Courses
- Online Interviewbit Compilers
- Online C Compiler
- Online Java Compiler
- Online Javascript Compiler
- Online Python Compiler
- Interview Preparation
- Java Interview Questions
- Sql Interview Questions
- Python Interview Questions
- Javascript Interview Questions
- Angular Interview Questions
- Networking Interview Questions
- Selenium Interview Questions
- Data Structure Interview Questions
- Data Science Interview Questions
- System Design Interview Questions
- Hr Interview Questions
- Html Interview Questions
- Amazon Interview Questions
- Facebook Interview Questions
- Google Interview Questions
- Tcs Interview Questions
- Accenture Interview Questions
- Infosys Interview Questions
- Capgemini Interview Questions
- Wipro Interview Questions
- Cognizant Interview Questions
- Deloitte Interview Questions
- Zoho Interview Questions
- Hcl Interview Questions
- Highest Paying Jobs In India
- Exciting C Projects Ideas With Source Code
- Top Java 8 Features
- Angular Vs React
- 10 Best Data Structures And Algorithms Books
- Best Full Stack Developer Courses
- Best Data Science Courses
- Python Commands List
- Data Scientist Salary
- Maximum Subarray Sum Kadane’s Algorithm
- Python Cheat Sheet
- C++ Cheat Sheet
- Javascript Cheat Sheet
- Git Cheat Sheet
- Java Cheat Sheet
- Data Structure Mcq
- C Programming Mcq
- Javascript Mcq
1 Million +
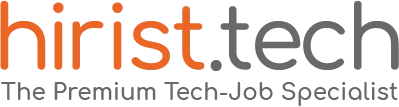
Top 25+ JavaScript Interview Questions and Answers
Research shows that JavaScript is used in 12% of all technical interviews. However, only 42% of candidates pass these interviews. That’s why it is important to prepare in advance. And this is where our guide can help you. We’ve put together a comprehensive list of the top 25+ JavaScript interview questions with clear and straightforward answers.
Whether you’re an experienced developer or a fresher ready for your first interview, these questions cover basic and advanced JavaScript topics.
So, let’s get started!
Table of Contents
About JavaScript
JavaScript is a programming language used in web development to make websites interactive.
It adds behaviour to websites, letting them respond to user actions like form validation, animation, and data fetching without reloading the page.
JavaScript works on both front-end and back-end development , making it versatile for creating responsive and engaging web applications.
According to Statista, JavaScript is the top choice for web development, with about 63.61% of developers worldwide preferring it.
JavaScript is also in high demand in India’s tech industry due to its crucial role in web development and the rise of digital initiatives.
To find the perfect JavaScript job , use Hirist, India’s top IT job portal. Here, you can search for JavaScript jobs, choose your preferred location and experience level, and easily apply for jobs that fit your skills.
JavaScript Interview Questions for Freshers
Here are some commonly asked basic interview questions on JavaScript , along with their answers.
- What is JavaScript?
JavaScript is a programming language used to create interactive elements on websites. It runs in web browsers and can manipulate webpage content, respond to user actions, and interact with servers.
- What are variables in JavaScript?
Variables are used to store data values in JavaScript. They can hold different types of data like numbers, strings (text), and boolean (true/false) values.
- What are the different data types in JavaScript?
JavaScript has several data types, including,
- Number: Represents both integer and decimal (floating-point) numbers.
- String: Represents sequences of characters or alphanumeric values.
- Boolean: Represents true or false values.
- Object: Represents collections of properties and complex values.
- Null: Represents values that are intentionally empty or unknown.
- Undefined: Indicates variables that have been declared but not assigned a value.
- Symbols: Provides unique identifiers for objects and supports special use cases.
- How do you declare a variable in JavaScript?
You can declare a variable using the var, let, or const keywords followed by the variable name.
For example:
var myNumber = 10;
let myName = “Rahul”;
const PI = 3.14;
- What is an event in JavaScript?
An event is an action that occurs on a webpage, such as clicking a button or submitting a form. JavaScript can respond to these events by executing specific functions.
- How do you create a function in JavaScript?
You can create a function using the function keyword followed by the function name and parameters.
function greet(name) {
return “Hello, ” + name + “!”;
- How do you add an event listener in JavaScript?
You can add an event listener to an HTML element using the addEventListener method.
document.getElementById(“myButton”).addEventListener(“click”, function() {
alert(“Button clicked!”);
JavaScript Interview Questions Experienced
We have categorized the commonly asked JS programming interview questions and answers based on candidates’ experience levels.
JavaScript Interview Questions for 2 Year Experience
Here are some common JS interview questions and answers for candidates with 2 years of experience.
- What are closures in JavaScript?
A closure is a feature in JavaScript that allows a function to remember and access its lexical scope even when it’s executed outside that scope. It helps in maintaining private variables and creating modular code.
- Explain the difference between let, const, and var.
var is used to declare variables with function scope, while let and const have block scope (limited to the nearest curly braces). Variables declared with const cannot be changed after assignment, but variables declared with let can.
- What is the difference between synchronous and asynchronous code in JavaScript?
Synchronous code runs line by line and blocks further execution until each line finishes. Asynchronous code allows tasks to run in the background and doesn’t wait for them to complete before moving on.
- How does prototypal inheritance work in JavaScript?
JavaScript uses prototypal inheritance, where objects can inherit properties and methods from other objects. Each object has a prototype object from which it inherits properties.
- Explain the concept of event bubbling and event delegation.
Event bubbling refers to the propagation of an event from the innermost element to the outermost element in the DOM hierarchy. Event delegation is a technique where a parent element handles events triggered by its child elements.
JavaScript Interview Questions for 3 Year Experience
Here are some important JS interview questions for candidates with 3 years of experience.
- What are Promises in JavaScript?
Promises are used for asynchronous programming in JavaScript. They represent the eventual completion or failure of an asynchronous operation, allowing you to handle the result or error once it’s available.
- Explain the concept of higher-order functions.
Higher-order functions are functions that can take other functions as arguments or return functions as their results. They enable functional programming paradigms like map, filter, and reduce.
- Explain the concept of the “this” keyword in JavaScript.
It is one of the most commonly asked JavaScript this interview questions .
In JavaScript, the “this” keyword refers to the current execution context or the “owner” of the function being executed. The value of “this” is determined by how a function is called. It is not fixed and can change based on the invocation context.
- What is the event loop in JavaScript, and how does it work?
The event loop is a mechanism that handles asynchronous operations in JavaScript. It continuously checks the call stack and task queue, moving tasks from the queue to the stack for execution when the stack is empty.
- What is the difference between == and === in JavaScript?
== is used for loose equality comparison and performs type coercion if needed, while === is used for strict equality comparison (checking both value and type) without type coercion.
JavaScript Interview Questions for 5 Years Experience
Here are some important JS interview questions for candidates with 5 years of experience.
- What are generators in JavaScript, and how are they used?
Generators are functions that can be paused and resumed at certain points using the yield keyword. They allow for asynchronous-like behaviour in synchronous code and are often used for iterative algorithms and handling streams of data.
- Explain the concept of ES6 modules in JavaScript.
ES6 modules provide a way to organize and structure code into reusable components. They use import and export statements to define dependencies between modules, allowing for better code organization and maintainability.
- How does JavaScript handle memory management and garbage collection?
JavaScript uses automatic memory management through garbage collection. It allocates memory when objects are created and automatically frees up memory when objects are no longer in use or unreachable by the program.
Common garbage collection algorithms include reference counting and mark-and-sweep.
- What is the difference between function declaration and function expression?
Function declarations are hoisted and can be called before they are defined in the code, while function expressions are not hoisted and must be defined before they are called. Function expressions can also be anonymous (no function name).
- What are some best practices for optimizing JavaScript performance?
Some best practices include,
- Minimizing DOM manipulation
- Using efficient data structures like arrays and objects
- Reducing unnecessary function calls
- Caching variables for reuse
- Using browser developer tools to profile and optimize code
JavaScript Interview Questions for 10 Years Experience
Here are some important JavaScript interview questions for candidates with 10 years of experience.
- What are the differences between classical inheritance and prototypal inheritance in JavaScript?
Classical inheritance involves defining classes and creating instances of those classes using constructors and inheritance hierarchies.
Prototypal inheritance, on the other hand, uses prototypes to share behaviour between objects, allowing for more flexible and dynamic object creation.
- What are the advantages and disadvantages of using closures in JavaScript?
Closures in JavaScript allow functions to retain access to variables from their parent scopes, enabling encapsulation and private data.
However, excessive use of closures can lead to memory leaks if not managed properly, as they maintain references to outer scope variables.
- What are some common design patterns used in JavaScript development?
Common design patterns in JavaScript include the Singleton pattern, Factory pattern, Module pattern, Observer pattern, and Promises pattern.
These patterns help in structuring and organizing code to promote reusability, maintainability, and scalability.
- How does JavaScript handle error handling, and what are the best practices for managing errors in production code?
JavaScript uses try-catch blocks to handle synchronous errors and .catch() methods for handling Promise rejections in asynchronous code.
Best practices for managing errors include,
- Logging errors with relevant information
- Implementing graceful error recovery strategies
- Using tools like error monitoring services to track and analyze production errors.
Advanced JavaScript Interview Questions
Take a look at these advanced JavaScript programming questions and answers .
- Explain the concept of lexical scoping in JavaScript.
Lexical scoping in JavaScript means that the scope of a variable is determined by its position within the source code’s lexical structure. Inner functions have access to variables declared in their outer function’s scope.
- What is the NaN property in JavaScript?
The NaN property in JavaScript represents a value that is “Not-a-Number.” It indicates that a value is not a valid number. If a calculation or operation results in a value that is not a number, it will return NaN.
To check if a value is NaN, you can use the isNaN() function. It’s important to know that isNaN() converts the given value to a number and then checks if it equals NaN.
- What are the various types of errors in JavaScript?
JavaScript errors can be categorized into three types:
- Load time errors : These errors occur while a web page is loading. They include syntax errors that are identified dynamically during the loading process.
- Runtime errors : These errors happen when a command is used incorrectly in HTML.
- Logical errors : These errors result from flawed logic within a function, leading to unexpected outcomes or incorrect behaviour during execution.
- How is DOM used in JavaScript?
The DOM (Document Object Model) in JavaScript controls how different elements in a web page interact with each other.
It allows developers to manipulate objects like links and paragraphs on a web page, enabling actions such as adding or removing them dynamically. Using the DOM API simplifies web development compared to other models.
JavaScript Problem Solving Questions
These are some important problem-solving JavaScript interview questions and answers .
- Find the maximum element in an array.
function findMaxElement(arr) {
if (arr.length === 0) {
return null; // Return null for empty array
}
let max = arr[0]; // Initialize max with the first element
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i]; // Update max if current element is greater
}
return max; // Return the maximum element
// Example usage:
let numbers = [10, 5, 8, 15, 3];
console.log(findMaxElement(numbers)); // Output: 15
- Count the number of vowels in a given string.
function countVowels(str) {
const vowels = ‘aeiouAEIOU’;
let count = 0;
for (let char of str) {
if (vowels.includes(char)) {
count++;
return count;
let text = “Hello World”;
console.log(countVowels(text)); // Output: 3 (e, o, o)
- Calculate the Fibonacci sequence up to n numbers using recursion.
function fibonacci(n) {
if (n <= 0) {
return [];
if (n === 1) {
return [0];
const sequence = [0, 1];
for (let i = 2; i < n; i++) {
sequence.push(sequence[i – 1] + sequence[i – 2]);
return sequence;
let count = 8;
console.log(fibonacci(count)); // Output: [0, 1, 1, 2, 3, 5, 8, 13]
JavaScript Object Oriented Programming Interview Questions
Here are some important JavaScript OOP interview questions and answers.
- What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a way of writing programs that use objects to structure and handle data. This approach emphasizes reusing code and breaking down complex tasks into simpler parts.
- What are some benefits of using OOP in JavaScript?
Using Object-Oriented Programming (OOP) in JavaScript offers several advantages. It enhances code organization, promotes code reuse, and facilitates easier maintenance.
Additionally, OOP allows developers to model real-world objects and concepts more naturally within their codebase.
- What is encapsulation in JavaScript?
Encapsulation is the bundling of data (attributes) and methods (functions) that operate on the data into a single unit called an object. It allows objects to control access to their internal state and hide implementation details from the outside world.
- What is polymorphism in JavaScript?
Polymorphism allows objects of different classes to be treated as objects of a common superclass.
This means that different classes can implement the same method name but behave differently based on the specific class instance. Polymorphism enhances code reusability and flexibility in OOP.
JavaScript Coding Interview Questions
Here are commonly asked coding-related JavaScript practical questions and answers.
- Write a function to check if a given string is a palindrome (reads the same backward as forward).
function isPalindrome(str) {
return str === str.split(”).reverse().join(”);
let text1 = “racecar”;
let text2 = “hello”;
console.log(isPalindrome(text1)); // Output: true
console.log(isPalindrome(text2)); // Output: false
- Write a function to find the factorial of a number.
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
return result;
let num = 5;
console.log(factorial(num)); // Output: 120 (5! = 5 * 4 * 3 * 2 * 1)
- Write a function to remove duplicate elements from an array.
function removeDuplicates(arr) {
return arr.filter((value, index, self) => self.indexOf(value) === index);
let nums = [1, 2, 2, 3, 4, 4, 5];
console.log(removeDuplicates(nums)); // Output: [1, 2, 3, 4, 5]
JavaScript MCQ Question and Answer
Here are some important JavaScript logical questions and answers.
- What will the following code output?
console.log(typeof NaN);
A) “number”
B) “NaN”
C) “undefined”
D) “string”
Answer: A) “number”
- Which keyword is used to declare a constant variable in JavaScript?
B) let
C) const
D) final
Answer: C) const
- What does the setTimeout() function do in JavaScript?
A) Pauses the execution of the script
B) Sets a timer to execute a function after a specified delay
C) Executes a function immediately
D) Stops the execution of a function
Answer: B) Sets a timer to execute a function after a specified delay
- Which of the following is NOT a valid JavaScript data type?
B) boolean
C) undefined
D) decimal
Answer: D) decimal
- Question: What will the following code output?
console.log(2 + “2”);
B) “22”
C) “4”
D) NaN
Answer: B) “22”
Wrapping Up
So, these are the top 25+ JavaScript interview questions and answers that cover essential concepts and topics for JavaScript developers. Learning these questions will boost your confidence and help you impress the interviewers . And if you are looking for the latest JavaScript job opportunities, visit Hirist , the leading IT job portal in India.
Top 25+ Frontend Interview Questions and Answers
Top 35+ nodejs interview questions and answers, you may also like, top 25 sap interview questions and answers, top 25+ agile interview questions and answers, top 25+ sap mm interview questions and answers, top 25+ sap sd interview questions and answers.
- Career Advice
- Interview Questions
- Interview Advice
Userful Links
- Terms of Service
- Privacy Policy
Latest Articles
- Mobile Applications
- Data Science
- Quality Assurance
- Project Management
- UI/UX Design
@2022 - All Right Reserved. Designed and Developed by PenciDesign
Keep me signed in until I sign out
Forgot your password?
A new password will be emailed to you.
Have received a new password? Login here
Are you sure want to unlock this post?
Are you sure want to cancel subscription.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- 30 OOPs Interview Questions and Answers (2024)
- C++ Interview Questions and Answers (2024)
Top 100 C++ Coding Interview Questions and Answers (2024)
- Top 50+ Python Interview Questions and Answers (Latest 2024)
- Java Interview Questions and Answers
- Java Collections Interview Questions and Answers
- Top 20 Java Multithreading Interview Questions & Answers
- Top 100 Data Structure and Algorithms DSA Interview Questions Topic-wise
- Top 50 Array Coding Problems for Interviews
- Most Asked Problems in Data Structures and Algorithms | Beginner DSA Sheet
- Top 10 Algorithms in Interview Questions
- Machine Learning Interview Questions
- Top 50 Problems on Linked List Data Structure asked in SDE Interviews
- Top 50 Problems on Heap Data Structure asked in SDE Interviews
- Data Analyst Interview Questions and Answers
- SQL Query Interview Questions
- Top Linux Interview Questions With Answer
- MySQL Interview Questions
- Top 50 Django Interview Questions and Answers
- Top 50 Networking Interview Questions (2024)
- Software Testing Interview Questions
C++ is one of the most popular languages in the software industry for developing software ranging from operating systems, and DBMS to games. That is why it is also popular to be asked to write C++ Programs in live coding sessions in job placement interviews.
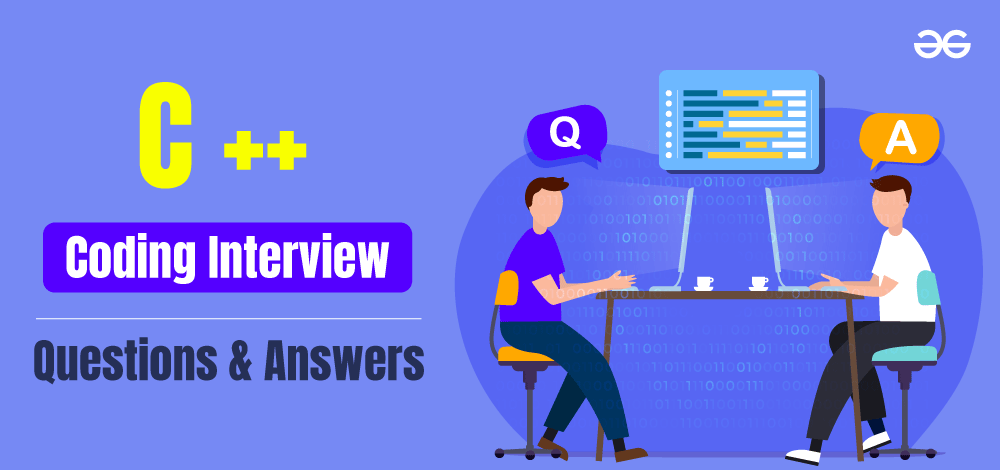
This article provides a list of C++ coding interview questions for beginners as well as experienced professionals. The questions are designed to test candidates’ understanding of the following topics:
- C++ syntax and semantics
- Data structures and algorithms
- Object-oriented programming
- Memory management
List of 100 C++ Coding Interview Questions and Answers
Here is a list of 100 C++ coding interview questions and answers
1. Write a C++ Program to Check Whether a Number is a Positive or Negative Number.
2. write a program to find the greatest of the three numbers., 3. c++ program to check whether number is even or odd.
For more information, refer to the article – C++ Program To Check Whether Number is Even Or Odd
4. Write a Program to Find the ASCII Value of a Character
5. write a program to check whether a character is a vowel or consonant, 6. write a program to print check whether a character is an alphabet or not, 7. write a program to find the length of the string without using strlen() function , 8. write a program to toggle each character in a string , 9. write a program to count the number of vowels , 10. write a program to remove the vowels from a string, 11. write a program to remove all characters from a string except alphabets, 12. write a program to remove spaces from a string, 13. write a program to find the sum of the first n natural numbers, 14. write a program to find the factorial of a number using loops, 15. write a program to find a leap year or not, 16. write a program to check the prime number, 17. write a program to check palindrome, 18. write a program to check whether a number is an armstrong number or not, 19. write a program to find the nth term of the fibonacci series, 20. write a program to calculate the greatest common divisor of two numbers, 21. write a program to calculate the lowest common multiple (lcm) of two numbers, 22. write a program for finding the roots of a quadratic equation, 23. write a program to find the smallest and largest element in an array, 24. write a program to find the second smallest element in an array, 25. write a program to calculate the sum of elements in an array, 26. write a program to check if the given string is palindrome or not, 27. write a program to check if two strings are anagram or not, 28. write a program to print a diamond pattern, 29. write a program to print a pyramid pattern, 30. write a program to print the hourglass pattern, 31. write a program to print the rotated hourglass pattern, 32. write a program to print a simple pyramid pattern, 33. write a program to print an inverted pyramid , 34. write a program to print a triangle star pattern, 35. write a program to print floyd’s triangle, 36. write a program to print the pascal triangle, 37. write a program to print the given string in reverse order , 38. write a c++ program to print the given string in reverse order using recursion, 39. write a program to check if the given string is palindrome or not using recursion, 40. write a program to calculate the length of the string using recursion, 41. write a program to calculate the factorial of a number using recursion, 42. write a program to count the sum of numbers in a string, 43. write a program to print all natural numbers up to n without using a semi-colon, 44. write a program to swap the values of two variables without using any extra variable, 45. write a program to print the maximum value of an unsigned int using one’s complement (~) operator, 46. write a program to check for the equality of two numbers without using arithmetic or comparison operator, 47. write a program to find the maximum and minimum of the two numbers without using the comparison operator, 48. write a program for octal to decimal conversion, 49. write a program for hexadecimal to decimal conversion, 50. write a program for decimal to binary conversion, 51. write a program for decimal octal conversion, 52. write a program for decimal to hexadecimal conversion, 53. write a program for binary to octal conversion , 54. write a program for octal to binary conversion , 55. write a program to implement the use of encapsulation, 56. write a program to implement the concept of abstraction, 57. write a program to implement the concept of compile-time polymorphism or function overloading, 58. write a program to implement the concept of operator overloading, 59. write a program to implement the concept of function overriding or runtime polymorphism, 60. write a program to implement single-level inheritance, 61. write a program to create a class for complex numbers, 62. write a program to implement the inch feet system, 63. write a program to implement bubble sort, 64. write a program to implement insertion sort, 65. write a program to implement selection sort, 66. write a program to implement merge sort, 67. write a program to implement quick sort, 68. write a program to implement linear search, 69. write a program to implement binary search, 70. write a program to find the index of a given element in a vector, 71. write a program to remove duplicate elements in an array using stl, 72. write a program to sort an array in descending order using stl, 73. write a program to calculate the frequency of each word in the given string, 74. write a program to find k maximum elements of an array in the original order, 75. write a program to find all unique subsets of a given set using stl, 76. write a program to iterate over a queue without removing the element, 77. write a program for the implementation of stacks using an array, 78. write a program for the implementation of a queue using an array, 79. write a program implementation of stacks using a queue, 80. write a program to implement a stack using the list in stl, 81. write a program to determine array is a subset of another array or not, 82. write a program for finding the circular rotation of an array by k positions, 83. write a program to sort the first half in ascending order and the second half in descending, 84. write a program to print the given string in reverse order, 85. write a program to print all permutations of a string using recursion, 86. write a program to print all permutations of a given string in lexicographically sorted order, 87. write a program to remove brackets from an algebraic expression, 88. program to perform insert, delete, and print operations singly linked list, 89. program to perform insert, delete, and print operations doubly linked list, 90. program to perform insert, delete, and print operations circular linked list, 91. program for inorder traversal in a binary tree, 92. program to find all its subsets from a set of positive integers, 93. program for preorder traversal in a binary tree, 94. program for postorder traversal in a binary tree, 95. program for level-order traversal in a binary tree, 96. write a program for the top view of a binary tree, 97. write a program to print the bottom view of a binary tree, 98. write a program to print the left view of a binary tree, 99. write a program to print the right view of the binary tree, 100. write a program for the conversion of infix expression to postfix expression.
Coding interviews can be challenging task, but they are also a great opportunity to test your skills and knowledge. By practicing with commonly ask C++ coding interview questions , you can increase your chances of success.
Remember to stay calm, communicate your thought process clearly, and don’t be afraid to ask for clarification if needed. With hard work and preparation, you can ace your interview and land your dream job!
C++ Coding Interview Questions – FAQs
Q: what are the most common c++ coding interview questions.
The most common C++ coding interview questions are designed to test your knowledge of the following topics: C++ syntax and semantics Data structures and algorithms Object-oriented programming Memory management Pointers Templates & More.
Q: What should I do during a C++ coding interview?
When you are asked a coding question in an interview, take a moment to think about the problem before you start coding. Explain your thought process to the interviewer as you are coding. This will help them to understand your approach to solving problems.
Q: What are some tips for writing clean and efficient code?
Here are some tips for writing clean and efficient code: Use meaningful variable names. Use whitespace to make your code readable. Break down complex problems into smaller, more manageable problems. Use comments to explain your code. Test your code thoroughly. Use appropriate data structures and algorithms. Avoid unnecessary duplication of code.
Q: How can I improve my communication skills during C++ coding interviews?
Here are some tips for improving your communication skills during C++ coding interviews: Speak clearly and concisely. Explain your thought process to the interviewer. Ask clarifying questions if needed. Be prepared to answer questions about your code. Be open to feedback. Practicing explaining your code to others is a great way to improve your communication skills. You can also practice by giving yourself mock interviews.
Please Login to comment...
Similar reads.
- interview-questions
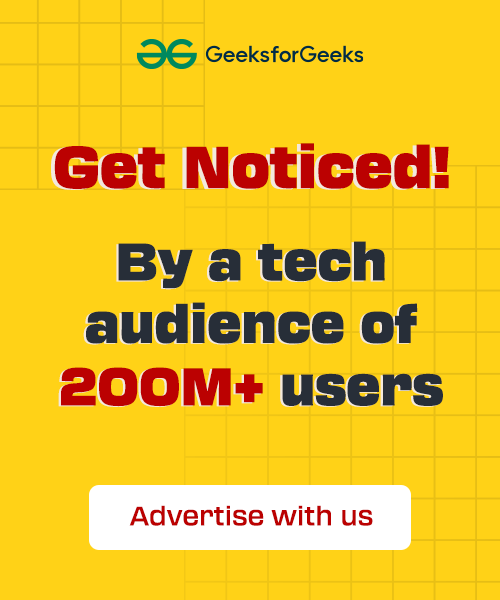
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Share full article
For more audio journalism and storytelling, download New York Times Audio , a new iOS app available for news subscribers.
The Evolving Danger of the New Bird Flu
An unusual outbreak of the disease has spread to dairy herds in multiple u.s. states..
This transcript was created using speech recognition software. While it has been reviewed by human transcribers, it may contain errors. Please review the episode audio before quoting from this transcript and email [email protected] with any questions.
From “The New York Times,” I’m Sabrina Tavernise, and this is “The Daily.”
[MUSIC PLAYING]
The outbreak of bird flu that is tearing through the nation’s poultry farms is the worst in US history. But scientists say it’s now starting to spread into places and species it’s never been before.
Today, my colleague, Emily Anthes, explains.
It’s Monday, April 22.
Emily, welcome back to the show.
Thanks for having me. Happy to be here.
So, Emily, we’ve been talking here on “The Daily” about prices of things and how they’ve gotten so high, mostly in the context of inflation episodes. And one of the items that keeps coming up is eggs. Egg prices were through the roof last year, and we learned it was related to this. Avian flu has been surging in the United States. You’ve been covering this. Tell us what’s happening.
Yes, so I have been covering this virus for the last few years. And the bird flu is absolutely tearing through poultry flocks, and that is affecting egg prices. That’s a concern for everyone, for me and for my family. But when it comes to scientists, egg prices are pretty low on their list of concerns. Because they see this bird flu virus behaving differently than previous versions have. And they’re getting nervous, in particular, about the fact that this virus is reaching places and species where it’s never been before.
OK, so bird flu, though, isn’t new. I mean I remember hearing about cases in Asia in the ‘90s. Remind us how it began.
Bird flu refers to a bunch of different viruses that are adapted to spread best in birds. Wild water birds, in particular, are known for carrying these viruses. And flu viruses are famous for also being shapeshifters. So they’re constantly swapping genes around and evolving into new strains. And as you mentioned back in the ‘90s, a new version of bird flu, a virus known as H5N1, emerged in Asia. And it has been spreading on and off around the world since then, causing periodic outbreaks.
And how are these outbreaks caused?
So wild birds are the reservoir for the virus, which means they carry it in their bodies with them around the world as they fly and travel and migrate. And most of the time, these wild birds, like ducks and geese, don’t even get very sick from this virus. But they shed it. So as they’re traveling over a poultry farm maybe, if they happen to go to the bathroom in a pond that the chickens on the farm are using or eat some of the feed that chickens on the farm are eating, they can leave the virus behind.
And the virus can get into chickens. In some cases, it causes mild illness. It’s what’s known as low pathogenic avian influenza. But sometimes the virus mutates and evolves, and it can become extremely contagious and extremely fatal in poultry.
OK, so the virus comes through wild birds, but gets into farms like this, as you’re describing. How have farms traditionally handled outbreaks, when they do happen?
Well, because this threat isn’t new, there is a pretty well-established playbook for containing outbreaks. It’s sometimes known as stamping out. And brutally, what it means is killing the birds. So the virus is so deadly in this highly pathogenic form that it’s sort of destined to kill all the birds on a farm anyway once it gets in. So the response has traditionally been to proactively depopulate or cull all the birds, so it doesn’t have a chance to spread.
So that’s pretty costly for farmers.
It is. Although the US has a program where it will reimburse farmers for their losses. And the way these reimbursements work is they will reimburse farmers only for the birds that are proactively culled, and not for those who die naturally from the virus. And the thinking behind that is it’s a way to incentivize farmers to report outbreaks early.
So, OK, lots of chickens are killed in a way to manage these outbreaks. So we know how to deal with them. But what about now? Tell me about this new strain.
So this new version of the virus, it emerged in 2020.
After the deadly outbreak of the novel coronavirus, authorities have now confirmed an outbreak of the H5N1 strain of influenza, a kind of bird flu.
And pretty quickly it became clear that a couple things set it apart.
A bald eagle found dead at Carvins Cove has tested positive for the highly contagious bird flu.
This virus, for whatever reason, seemed very good at infecting all sorts of wild birds that we don’t normally associate with bird flu.
[BIRD CRYING]
He was kind of stepping, and then falling over, and using its wing to right itself.
Things like eagles and condors and pelicans.
We just lost a parliament of owls in Minneapolis.
Yeah, a couple of high profile nests.
And also in the past, wild birds have not traditionally gotten very sick from this virus. And this version of the virus not only spread widely through the wild bird population, but it proved to be devastating.
The washing up along the East Coast of the country from Scotland down to Suffolk.
We were hearing about mass die-offs of seabirds in Europe by the hundreds and the thousands.
And the bodies of the dead dot the island wherever you look.
Wow. OK. So then as we know, this strain, like previous ones, makes its way from wild animals to farmed animals, namely to chickens. But it’s even more deadly.
Absolutely. And in fact, it has already caused the worst bird flu outbreak in US history. So more than 90 million birds in the US have died as a result of this virus.
90 million birds.
Yes, and I should be clear that represents two things. So some of those birds are birds who naturally got infected and died from the virus. But the vast majority of them are birds that were proactively culled. What it adds up to is, is 90 million farmed birds in the US have died since this virus emerged. And it’s not just a chicken problem. Another thing that has been weird about this virus is it has jumped into other kinds of farms. It is the first time we’ve seen a bird flu virus jump into US livestock.
And it’s now been reported on a number of dairy farms across eight US states. And that’s just something that’s totally unprecedented.
So it’s showing up at Dairy farms now. You’re saying that bird flu has now spread to cows. How did that happen?
So we don’t know exactly how cows were first infected, but most scientists’ best guess is that maybe an infected wild bird that was migrating shed the virus into some cattle feed or a pasture or a pond, and cattle picked it up. The good news is they don’t seem to get nearly as sick as chickens do. They are generally making full recoveries on their own in a couple of weeks.
OK, so no mass culling of cows?
No, that doesn’t seem to be necessary at this point. But the bad news is that it’s starting to look like we’re seeing this virus spread from cow to cow. We don’t know exactly how that’s happening yet. But anytime you see cow-to-cow or mammal-to-mammal transmission, that’s a big concern.
And why is that exactly?
Well, there are a bunch of reasons. First, it could allow the outbreak to get much bigger, much faster, which might increase the risk to the food supply. And we might also expect it to increase the risk to farm workers, people who might be in contact with these sick cows.
Right now, the likelihood that a farmer who gets this virus passes it on is pretty low. But any time you see mammal-to-mammal transmission, it increases the chance that the virus will adapt and possibly, maybe one day get good at spreading between humans. To be clear, that’s not something that there’s any evidence happening in cows right now. But the fact that there’s any cow-to-cow transmission happening at all is enough to have scientists a bit concerned.
And then if we think more expansively beyond what’s happening on farms, there’s another big danger lurking out there. And that’s what happens when this virus gets into wild animals, vast populations that we can’t control.
We’ll be right back.
So, Emily, you said that another threat was the threat of flu in wild animal populations. Clearly, of course, it’s already in wild birds. Where else has it gone?
Well, the reason it’s become such a threat is because of how widespread it’s become in wild birds. So they keep reintroducing it to wild animal populations pretty much anywhere they go. So we’ve seen the virus repeatedly pop up in all sorts of animals that you might figure would eat a wild bird, so foxes, bobcats, bears. We actually saw it in a polar bear, raccoons. So a lot of carnivores and scavengers.
The thinking is that these animals might stumble across a sick or dead bird, eat it, and contract the virus that way. But we’re also seeing it show up in some more surprising places, too. We’ve seen the virus in a bottle-nosed dolphin, of all places.
And most devastatingly, we’ve seen enormous outbreaks in other sorts of marine mammals, especially sea lions and seals.
So elephant seals, in particular in South America, were just devastated by this virus last fall. My colleague Apoorva Mandavilli and I were talking to some scientists in South America who described to us what they called a scene from hell, of walking out onto a beach in Argentina that is normally crowded with chaotic, living, breathing, breeding, elephant seals — and the beach just being covered by carcass, after carcass, after carcass.
Mostly carcasses of young newborn pups. The virus seemed to have a mortality rate of 95 percent in these elephant seal pups, and they estimated that it might have killed more than 17,000 of the pups that were born last year. So almost the entire new generation of this colony. These are scientists that have studied these seals for decades. And they said they’ve never seen anything like it before.
And why is it so far reaching, Emily? I mean, what explains these mass die-offs?
There are probably a few explanations. One is just how much virus is out there in the environment being shed by wild birds into water and onto beaches. These are also places that viruses like this haven’t been before. So it’s reaching elephant seals and sea lions in South America that have no prior immunity.
There’s also the fact that these particular species, these sea lions and seals, tend to breed in these huge colonies all crowded together on beaches. And so what that means is if a virus makes its way into the colony, it’s very conducive conditions for it to spread. And scientists think that that’s actually what’s happening now. That it’s not just that all these seals are picking up the virus from individual birds, but that they’re actually passing it to each other.
So basically, this virus is spreading to places it’s never been before, kind of virgin snow territory, where animals just don’t have the immunity against it. And once it gets into a population packed on a beach, say, of elephant seals, it’s just like a knife through butter.
Absolutely. And an even more extreme example of that is what we’re starting to see happen in Antarctica, where there’s never been a bird flu outbreak before until last fall, for the first time, this virus reached the Antarctic mainland. And we are now seeing the virus move through colonies of not only seabirds and seals, but penguin colonies, which have not been exposed to these viruses before.
And it’s too soon to say what the toll will be. But penguins also, of course, are known for breeding in these large colonies.
Probably. don’t have many immune defenses against this virus, and of course, are facing all these other environmental threats. And so there’s a lot of fear that you add on the stress of a bird flu virus, and it could just be a tipping point for penguins.
Emily, at this point, I’m kind of wondering why more people aren’t talking about this. I mean, I didn’t know any of this before having this conversation with you, and it feels pretty worrying.
Well, a lot of experts and scientists are talking about this with rising alarm and in terms that are quite stark. They’re talking about the virus spreading through wild animal populations so quickly and so ferociously that they’re calling it an ecological disaster.
But that’s a disaster that sometimes seems distant from us, both geographically, we’re talking about things that are happening maybe at the tip of Argentina or in Antarctica. And also from our concerns of our everyday lives, what’s happening in Penguins might not seem like it has a lot to do with the price of a carton of eggs at the grocery store. But I think that we should be paying a lot of attention to how this virus is moving through animal populations, how quickly it’s moving through animal populations, and the opportunities that it is giving the virus to evolve into something that poses a much bigger threat to human health.
So the way it’s spreading in wild animals, even in remote places like Antarctica, that’s important to watch, at least in part because there’s a real danger to people here.
So we know that the virus can infect humans, and that generally it’s not very good at spreading between humans. But the concern all along has been that if this virus has more opportunities to spread between mammals, it will get better at spreading between them. And that seems to be what is happening in seals and sea lions. Scientists are already seeing evidence that the virus is adapting as it passes from marine mammal to marine mammal. And that could turn it into a virus that’s also better at spreading between people.
And if somebody walks out onto a beach and touches a dead sea lion, if their dog starts playing with a sea lion carcass, you could imagine that this virus could make its way out of marine mammals and into the human population. And if it’s this mammalian adapted version of the virus that makes its way out, that could be a bigger threat to human health.
So the sheer number of hosts that this disease has, the more opportunity it has to mutate, and the more chance it has to mutate in a way that would actually be dangerous for people.
Yes, and in particular, the more mammalian hosts. So that gives the virus many more opportunities to become a specialist in mammals instead of a specialist in birds, which is what it is right now.
Right. I like that, a specialist in mammals. So what can we do to contain this virus?
Well, scientists are exploring new options. There’s been a lot of discussion about whether we should start vaccinating chickens in the US. The government, USDA labs, have been testing some poultry vaccines. It’s probably scientifically feasible. There are challenges there, both in terms of logistics — just how would you go about vaccinating billions of chickens every year. There are also trade questions. Traditionally, a lot of countries have not been willing to accept poultry products from countries that vaccinate their poultry.
And there’s concern about whether the virus might spread undetected in flocks that are vaccinated. So as we saw with COVID, the vaccine can sometimes stop you from getting sick, but it doesn’t necessarily stop infection. And so countries are worried they might unknowingly import products that are harboring the virus.
And what about among wild animals? I mean, how do you even begin to get your head around that?
Yeah, I mean, thinking about vaccinating wild animals maybe makes vaccinating all the chickens in the US look easy. There has been some discussion of limited vaccination campaigns, but that’s not feasible on a global scale. So unfortunately, the bottom line is there isn’t a good way to stop spread in wild animals. We can try to protect some vulnerable populations, but we’re not going to stop the circulation of this virus.
So, Emily, we started this conversation with a kind of curiosity that “The Daily” had about the price of eggs. And then you explained the bird flu to us. And then somehow we ended up learning about an ecological disaster that’s unfolding all around us, and potentially the source of the next human pandemic. That is pretty scary.
It is scary, and it’s easy to get overwhelmed by it. And I feel like I should take a step back and say none of this is inevitable. None of this is necessarily happening tomorrow. But this is why scientists are concerned and why they think it’s really important to keep a very close eye on what’s happening both on farms and off farms, as this virus spreads through all sorts of animal populations.
One thing that comes up again and again and again in my interviews with people who have been studying bird flu for decades, is how this virus never stops surprising them. And sometimes those are bad surprises, like these elephant seal die-offs, the incursions into dairy cattle. But there are some encouraging signs that have emerged recently. We’re starting to see some early evidence that some of the bird populations that survived early brushes with this virus might be developing some immunity. So that’s something that maybe could help slow the spread of this virus in animal populations.
We just don’t entirely know how this is going to play out. Flu is a very difficult, wily foe. And so that’s one reason scientists are trying to keep such a close, attentive eye on what’s happening.
Emily, thank you.
Thanks for having me.
Here’s what else you should know today.
On this vote, the yeas are 366 and the nays are 58. The bill is passed.
On Saturday, in four back-to-back votes, the House voted resoundingly to approve a long-stalled package of aid to Ukraine, Israel and other American allies, delivering a major victory to President Biden, who made aid to Ukraine one of his top priorities.
On this vote, the yeas are 385, and the no’s are 34 with one answering present. The bill is passed without objection.
The House passed the component parts of the $95 billion package, which included a bill that could result in a nationwide ban of TikTok.
On this vote, the yeas are 311 and the nays are 112. The bill is passed.
Oh, one voting present. I missed it, but thank you.
In a remarkable breach of custom, Democrats stepped in to supply the crucial votes to push the legislation past hard-line Republican opposition and bring it to the floor.
The House will be in order.
The Senate is expected to pass the legislation as early as Tuesday.
Today’s episode was produced by Rikki Novetsky, Nina Feldman, Eric Krupke, and Alex Stern. It was edited by Lisa Chow and Patricia Willens; contains original music by Marion Lozano, Dan Powell, Rowan Niemisto, and Sophia Lanman; and was engineered by Chris Wood. Our theme music is by Jim Brunberg and Ben Landsverk of Wonderly. Special thanks to Andrew Jacobs.
That’s it for “The Daily.” I’m Sabrina Tavernise. See you tomorrow.

- April 24, 2024 • 32:18 Is $60 Billion Enough to Save Ukraine?
- April 23, 2024 • 30:30 A Salacious Conspiracy or Just 34 Pieces of Paper?
- April 22, 2024 • 24:30 The Evolving Danger of the New Bird Flu
- April 19, 2024 • 30:42 The Supreme Court Takes Up Homelessness
- April 18, 2024 • 30:07 The Opening Days of Trump’s First Criminal Trial
- April 17, 2024 • 24:52 Are ‘Forever Chemicals’ a Forever Problem?
- April 16, 2024 • 29:29 A.I.’s Original Sin
- April 15, 2024 • 24:07 Iran’s Unprecedented Attack on Israel
- April 14, 2024 • 46:17 The Sunday Read: ‘What I Saw Working at The National Enquirer During Donald Trump’s Rise’
- April 12, 2024 • 34:23 How One Family Lost $900,000 in a Timeshare Scam
- April 11, 2024 • 28:39 The Staggering Success of Trump’s Trial Delay Tactics
- April 10, 2024 • 22:49 Trump’s Abortion Dilemma
Hosted by Sabrina Tavernise
Produced by Rikki Novetsky , Nina Feldman , Eric Krupke and Alex Stern
Edited by Lisa Chow and Patricia Willens
Original music by Marion Lozano , Dan Powell , Rowan Niemisto and Sophia Lanman
Engineered by Chris Wood
Listen and follow The Daily Apple Podcasts | Spotify | Amazon Music
The outbreak of bird flu currently tearing through the nation’s poultry is the worst in U.S. history. Scientists say it is now spreading beyond farms into places and species it has never been before.
Emily Anthes, a science reporter for The Times, explains.
On today’s episode

Emily Anthes , a science reporter for The New York Times.

Background reading
Scientists have faulted the federal response to bird flu outbreaks on dairy farms .
Here’s what to know about the outbreak.
There are a lot of ways to listen to The Daily. Here’s how.
We aim to make transcripts available the next workday after an episode’s publication. You can find them at the top of the page.
Special thanks to Andrew Jacobs .
The Daily is made by Rachel Quester, Lynsea Garrison, Clare Toeniskoetter, Paige Cowett, Michael Simon Johnson, Brad Fisher, Chris Wood, Jessica Cheung, Stella Tan, Alexandra Leigh Young, Lisa Chow, Eric Krupke, Marc Georges, Luke Vander Ploeg, M.J. Davis Lin, Dan Powell, Sydney Harper, Mike Benoist, Liz O. Baylen, Asthaa Chaturvedi, Rachelle Bonja, Diana Nguyen, Marion Lozano, Corey Schreppel, Rob Szypko, Elisheba Ittoop, Mooj Zadie, Patricia Willens, Rowan Niemisto, Jody Becker, Rikki Novetsky, John Ketchum, Nina Feldman, Will Reid, Carlos Prieto, Ben Calhoun, Susan Lee, Lexie Diao, Mary Wilson, Alex Stern, Dan Farrell, Sophia Lanman, Shannon Lin, Diane Wong, Devon Taylor, Alyssa Moxley, Summer Thomad, Olivia Natt, Daniel Ramirez and Brendan Klinkenberg.
Our theme music is by Jim Brunberg and Ben Landsverk of Wonderly. Special thanks to Sam Dolnick, Paula Szuchman, Lisa Tobin, Larissa Anderson, Julia Simon, Sofia Milan, Mahima Chablani, Elizabeth Davis-Moorer, Jeffrey Miranda, Renan Borelli, Maddy Masiello, Isabella Anderson and Nina Lassam.
Advertisement
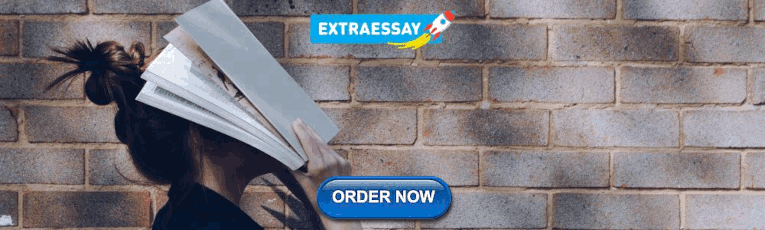
IMAGES
VIDEO
COMMENTS
C Programming Interview Questions - For Freshers. 1. Why is C called a mid-level programming language? Due to its ability to support both low-level and high-level features, C is considered a middle-level language. It is both an assembly-level language, i.e. a low-level language, and a higher-level language.
List of 50 C Coding Interview Questions and Answer. Here is a list of 50 C coding interview questions and answers: 1. Find the largest number among the three numbers. 2. Write a Program to check whether a number is prime or not. 3. Write a C program to calculate Compound Interest. 4.
Likewise, the statement "x -" means to decrement the value of x by 1. Another way of writing increment statements is to use the conventional + plus sign or - minus sign. In the case of "x++", another way to write it is "x = x +1". 👉 Free PDF Download: C Programming Interview Questions & Answers >>.
1. Why is C called a mid-level programming language? C has characteristics of both assembly-level i.e. low-level and higher-level languages. So as a result, C is commonly called a middle-level language. Using C, a user can write an operating system as well as create a menu-driven consumer billing system.
In a C interview, you can expect a mix of theoretical questions to assess your understanding of concepts and practical coding problems to evaluate your problem-solving skills. Questions may range from basic syntax and language features to more complex algorithms and data manipulation tasks.
What a C Programming Interview Looks Like. A C programming interview often involves a range of elements. While the specific format may vary from company to company, you can generally expect a combination of coding exercises, problem-solving questions, and conceptual discussions.
C Interview Questions and Answers provides a comprehensive collection of questions and answers for individuals preparing for interviews in the software development field, specifically focusing on the C programming language. C Interview Questions cover a wide range of topics, including syntax, data types, control structures, functions, pointers ...
C programming interview questions are a part of most technical rounds conducted by employers. The main goal of questioning a candidate on C programming is to check his/her knowledge about programming and core concepts of the C language. In this article, you will find a mix of C language interview questions designed specially to give you a foundation and build on it.
Answer: There are two possible methods to perform this task. Use increment (++) and decrement (-) operator. Example When x=4, x++ returns 5 and x- returns 3. Use conventional + or - sign. Example When x=4, use x+1 to get 5 and x-1 to get 3.
Showing you're an expert with these terms can let them know you have problem-solving skills and can find solutions when these errors occur. If a hiring manager asks you this question during your C programming interview, try to explain why they occur and talk about how they affect a program.
The C interview questions listed in this blog can be a valuable tool for job seekers. They can help you improve your problem-solving abilities and further understand the concepts on a deeper level. A thorough understanding of the key concepts, preparation, and practice will make it extremely simple for you to ace an interview.
Step-2: Write the c code and save it as .c file. For example, the name of your file is family.c and it is stored in Desktop. Step-3: Open Command Prompt clicking start button → All Apps → Windows System folder → Click Command Prompt. Step-4: Change the directory in command prompt to where you have saved the code file.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews. ... For Loop in C. Easy C (Basic) Max Score: 10 Success Rate: 93.65%. Solve Challenge. Sum of Digits of a Five Digit Number. Easy C (Basic) Max Score: 15 Success Rate: 98.66%.
Q2: Write a Program to find the Sum of two numbers entered by the user. In this problem, you have to write a program that adds two numbers and prints their sum on the console screen. For Example, Input: Enter two numbers A and B : 5 2. Output: Sum of A and B is: 7.
Here are a few examples of technical problem-solving questions: 1. Mini-Max Sum. This well-known challenge, which asks the interviewee to find the maximum and minimum sum among an array of given numbers, is based on a basic but important programming concept called sorting, as well as integer overflow.
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies.
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
MIKE'S TIP: When you're answering this question, quantify the details. This gives your answer critical context and scale, showcasing the degree of challenge and strength of the accomplishment. That way, your answer is powerful, compelling, and, above all, thorough. 2. Describe a time where you made a mistake.
Problem-solving interview questions are questions that employers ask related to the candidate's ability to gather data, analyze a problem, weigh the pros and cons and reach a logical decision. Also known as analytical skills interview questions, these questions will often focus on specific instances when the candidate analyzed a situation or ...
Strings 25. Being powerful, flexible, and well-supported has meant C# has quickly become one of the most popular programming languages available. Today, it is the 4th most popular programming language, with approximately 31% of all developers using it regularly. Follow along and check 34 most common C# Coding Interview Questions (SOLVED) for ...
Demonstrating your ability to tackle challenges effectively can set you apart from other applicants. Here are five tips to help you showcase your problem-solving skills during an interview: 1. Use the STAR Method. Structure your responses using the Situation, Task, Action, and Result (STAR) method.
Find the first repeating element in an array of integers. Solve. Find the first non-repeating element in a given array of integers. Solve. Subarrays with equal 1s and 0s. Solve. Rearrange the array in alternating positive and negative items. Solve. Find if there is any subarray with a sum equal to zero.
Here's how you can ace common interview questions for digital strategy roles. Powered by AI and the LinkedIn community. 1. Define Strategy. 2. Analyze Campaigns. Be the first to add your personal ...
Embedded C is a programming language that is an extension of C programming. It uses the same syntax as C and it is called "embedded" because it is used widely in embedded systems. Embedded C supports I/O hardware operations and addressing, fixed-point arithmetic operations, memory/address space access, and various other features that are ...
Here are commonly asked coding-related JavaScript practical questions and answers. Write a function to check if a given string is a palindrome (reads the same backward as forward). function isPalindrome (str) {. return str === str.split (").reverse ().join ("); }
Employers seek specific skills and qualities in business consultants. They want individuals who excel at problem-solving, can articulate complex ideas simply and can work well within diverse teams. Understanding what employers are looking for can guide your responses during the interview process. Strong problem-solving skills
This article provides a list of C++ coding interview questions for beginners as well as experienced professionals. The questions are designed to test candidates' understanding of the following topics: C++ syntax and semantics. Data structures and algorithms. Object-oriented programming.
The Evolving Danger of the New Bird Flu. An unusual outbreak of the disease has spread to dairy herds in multiple U.S. states. April 22, 2024, 6:00 a.m. ET. Share full article. Hosted by Sabrina ...