Assignment 5: Patient Queue
The patient queue assignment is due Thursday, May 18th at 12:00pm.
- Description
- Implementation
This problem focuses on implementing a priority queue using a Vector and also implementing a priority queue using a singly-linked list.
In the problem, you will implement a collection called a priority queue in two different ways: using a Stanford Library Vector and a linked-list. As an extension, you can also implement a priority queue using a heap (note: most priority queues are implemented as a heap). This problem focuses on linked lists and pointers, along with classes and objects. This is an pair programming assignment . You may choose to work in a pair/group on this program.
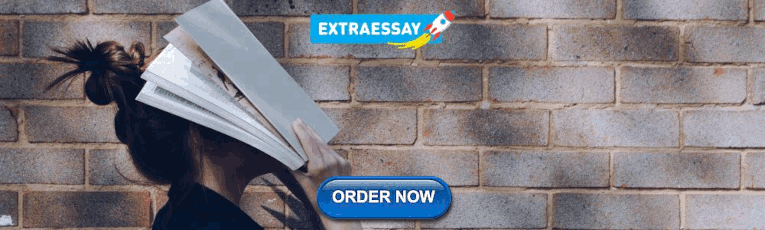
Files and Links:
Problem description:.
In this problem you will write a PatientQueue class that manages a waiting line of patients to be processed at a hospital.
In this class we have learned about queues that process elements in a first-in, first-out (FIFO) order. But FIFO is not the best order to assist patients in a hospital, because some patients have more urgent and critical injuries than others. As each new patient checks in at the hospital, the staff assesses their injuries and gives that patient an integer priority rating, with smaller integers representing greater urgency. (For example, a patient of priority 1 is more urgent and should receive care before a patient of priority 2.)
Once a doctor is ready to see a patient, the patient with the most urgent (smallest) priority is seen first. That is, regardless of the order in which you add/enqueue the elements, when you remove/dequeue them, the one with the lowest priority number comes out first, then the second-smallest, and so on, with the largest priority item coming out last. Priority ties are broken by first dequeuing the same-priority patients in the order they were enqueued. A queue that processes its elements in order of increasing priority like this is also called a priority queue .
Overview of Patient Queues
A priority queue stores a set of keys (priorities) and their associated values. This assignment models a hospital waiting room as a priority queue. If two patients in the queue have the same priority, you will break ties by choosing the patient who arrived earliest with that priority. This means that if a patient arrives at priority K and there are already other patients in the queue with priority K , your new patient should be placed after them.
For example, if the following patients arrive at the hospital in this order:
- "Dolores" with priority 5
- "Bernard" with priority 4
- "Arnold" with priority 8
- "William" with priority 5
- "Teddy" with priority 5
- "Ford" with priority 2
Then if you were to dequeue the patients to process them, they would come out in this order: Ford, Bernard, Dolores, William, Teddy, Arnold.
Implementation Details for both Classes:
Internally a given implementation of a priority queue can store its elements in sorted or unsorted order; all that matters is that when the elements are dequeued, they come out in sorted order by priority. This difference between the external expected behavior of the priority queue and its true internal state can lead to interesting differences in implementation, which you will find while completing this assignment.
You will write a VectorPatientQueue class that uses an un-sorted Vector and you will write a second class, LinkedListPatientQueue that uses a sorted linked list as its internal data storage.
We supply you with a PatientNode structure (in patientnode.h / cpp ) that is a small structure representing a single node of the linked list. Each PatientNode stores a string value and integer priority in it, and a pointer to a next node. In the linked-list implementaiton, you should use this structure to store the elements of your queue along with their priorities. In the Vector Patient Queue, you should probably write your own struct to hold the priorities and elements, and we strongly suggest that you also define an integer "timestamp" parameter that simply increments every time a value is added to the queue. The reason for the timestamp parameter is to enable you to differentiate between patients with the same priority who come in at different times -- in an unsorted list, you need to have the ability to determine who came in first, and a timestamp is a good way to do that. For the linked list implementation, you won't need a timestamp because the list will be sorted, and patients with the same priority will be sorted in the order in which they arrived.
PatientQueue Operations:
Your class must support all of the following operations. For the linked-list implementation, each member must run within the Big-Oh runtime noted; the N in this context means the length of the linked list.
The headers of every member must match those specified above. Do not change the parameters or function names.
Constructor/destructor: Your class must define a parameterless constructor. Since your linked-list implementation allocates dynamic memory (using new ), you must ensure that there are no memory leaks by freeing any such allocated memory at the appropriate time. This will mean that you will need a destructor to free the memory for all of your nodes.
Helper functions: The members listed on the previous page represent your class's required behavior. But you may add other member functions to help you implement all of the appropriate behavior. Any other member functions you provide must be private . Remember that each member function of your class should have a clear, coherent purpose. You should provide private helper members for common repeated operations.
You will also need to do the following:
Add descriptive comments to LinkedListPatientQueue.h/cpp and VectorPatientQueue.h/cpp . All files should have top-of-file headers; one file should have header comments atop each member function, either the .h or .cpp; and the .cpp should have internal comments describing the details of each function's implementation.
Declare your necessary private member variable in PatientQueue.h , along with any private member functions you want to help you implement the required public behavior. Your inner data storage must be a singly linked list of patient nodes; do not use any other collections or data structures in any part of your code.
Implement the bodies of all member functions and constructors in PatientQueue.cpp .
As stated previously, the elements of the patient queue are stored in sorted order internally. As new elements are enqueued, you should add them at the appropriate place in the vector or linked list so as to maintain the sorted order. The primary benefit of this implementation is that when dequeuing, you do not need to search the vector or linked list to find the element with most urgent priority to remove/return it. Enqueuing new patients is slower, because you must search for the proper place to enqueue them, but dequeuing a patient to process them is very fast, because they are at the front of the or Vector list.
Vector Implementation
Your class will use an unsorted Stanford Vector to hold the queue of patients. Because the Vector is unsorted, you will need to traverse the list to find the element with the smallest element, which is, notably, inefficient.
You should create a struct inside the VectorPatientQueue.h file that holds a patient name and a priority, and it should also hold an integer timestamp for breaking ties. This timestamp can be based on an incrementing counter elsewhere in your class, and every time a patient is enqueued you can update the timestamp and add it to the patient's struct.
Do not overthink the Vector patient queue -- this should be a relatively straightforward part of the project so you can get used to the idea of a priority queue.
Linked List Implementation
The following is a diagram of the internal linked list state of a PatientQueue after enqueuing the elements listed previously:
A tricky part of this implementation is inserting a new node in the proper place when a new patient arrives. You must look for the proper insertion point by finding the last element whose priority is at least as large as the new value to insert. Remember that, as shown in class, you must often stop one node early so that you can adjust the next pointer of the preceding node. For example, if you were going to insert the value "Stubbs" with priority 5 into the list shown above, your code should iterate until you have a pointer to the node for "Teddy", as shown below:
Once the current pointer shown above points to the right location, you can insert the new node as shown below:
Here are a few other constraints we expect you to follow in your implementation:
You should not make unnecessary passes over the linked list. For example, when enqueuing an element, a poor implementation would be to traverse the entire list once to count its size and to find the proper index at which to insert, and then make a second traversal to get back to that spot and add the new element. Do not make such multiple passes. Also, keep in mind that your queue class is not allowed to store an integer size member variable; you must use the presence of a null next pointer to figure out where the end of the list is and how long it is.
Duplicate patient names and priorities are allowed in your queue. For example, the upgradePatient operation should affect only a single occurrence of a patient's name (the one with lowest priority). If there are other occurrences of that same value in the queue, a single call to upgradePatient shouldn't affect them all.
You are not allowed to use a sort function or library to arrange the elements of your list, nor are you allowed to create any temporary or auxiliary data structures anywhere in your code. You must implement all behavior using only the one linked list of nodes as specified.
You will need pointers for several of your implementations, but you should not use pointers-to-pointers (for example, PatientNode** ). If you like, you are allowed to use a reference to a pointer (e.g. PQNode*& ).
You should not create any more PatientNode objects than necessary. For example, if a PatientQueue contains 6 elements, there should be exactly 6 PatientNode objects in its internal linked list; no more, no less. You shouldn't, say, have a seventh empty "dummy" node at the front or back of the list that serves only as a marker, nor should you create a new PatientNode that is just thrown away or discarded without being used as part of the linked list. You can declare as many local variable pointers to PatientNode s as you like.
Development Strategy and Hints:
Draw pictures: When manipulating linked lists, it is often helpful to draw pictures of the linked list before, during, and after each of the operations you perform on it. Manipulating linked lists can be tricky, but if you have a picture in front of you as you're coding it can make your job substantially easier.
List states: When processing a linked list, you should consider the following states and transitions between them in your code as you add and remove elements. You should also, for each state below, think about the effect of adding a node in the front, middle, and back of the list.
Heap Implementation (optional extension)
As discussed in -->lecture -->, a binary heap is an unfilled array that maintains a "heap ordering" property where each index i is thought of as having two "child" indexes, i * 2 and i * 2 + 1, and where the elements must be arranged such that "parent" indexes always store more urgent priorities than their "child" indexes. To simplify the index math, we will leave index 0 blank and start the data at an overall parent "root" or "start" index of 1. One very desirable property of a binary heap is that the most urgent-priority element (the one that should be returned from a call to peek or dequeue) is always at the start of the data in index 1. For example, the six elements listed in the previous pages could be put into a binary heap as follows. Notice that the most urgent element, "t":2, is stored at the root index of 1.
index 0 1 2 3 4 5 6 7 8 9 +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ value | | "t":2 | "m":5 | "b":4 | "x":5 | "q":5 | "a":8 | | | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ size = 6 capacity = 10
As discussed in lecture, adding (enqueuing) a new element into a heap involves placing it into the first empty index (7, in this case) and then "bubbling up" or "percolating up" by swapping it with its parent index (i/2) so long as it has a more urgent (lower) priority than its parent. We use integer division, so the parent of index 7 = 7/2 = 3. For example, if we added "y" with priority 3, it would first be placed into index 7, then swapped with "b":4 from index 3 because its priority of 3 is less than b's priority of 4. It would not swap any further because its new parent, "t":2 in index 1, has a lower priority than y. So the final heap array contents after adding "y":3 would be:
index 0 1 2 3 4 5 6 7 8 9 +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ value | | "t":2 | "m":5 | "y":3 | "x":5 | "q":5 | "a":8 | "b":4 | | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ size = 7 capacity = 10
Removing (dequeuing) the most urgent element from a heap involves moving the element from the last occupied index (7, in this case) all the way up to the "root" or "start" index of 1, replacing the root that was there before; and then "bubbling down" or "percolating down" by swapping it with its more urgent-priority child index (i*2 or i*2+1) so long as it has a less urgent (higher) priority than its child. For example, if we removed "t":2, we would first swap up the element "b":4 from index 7 to index 1, then bubble it down by swapping it with its more urgent child, "y":3 because the child's priority of 3 is less than b's priority of 4. It would not swap any further because its new only child, "a":8 in index 6, has a higher priority than b. So the final heap array contents after removing "t":2 would be:
index 0 1 2 3 4 5 6 7 8 9 +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ value | | "y":3 | "m":5 | "b":4 | "x":5 | "q":5 | "a":8 | | | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ size = 6 capacity = 10
A key benefit of using a binary heap to represent a priority queue is efficiency. The common operations of enqueue and dequeue take only O(log N) time to perform, since the "bubbling" jumps by powers of 2 every time. The peek operation takes only O(1) time since the most urgent-priority element's location is always at index 1.
If nodes ever have a tie in priority, break ties by comparing the strings themselves, treating strings that come earlier in the alphabet as being more urgent (e.g. "a" before "b"). Compare strings using the standard relational operators like ,>=,==,and!=. Donotmakeassumptionsaboutthelengthsofthestrings.
Changing the priority of an existing value involves looping over the heap to find that value, then once you find it, setting its new priority and "bubbling up" that value from its present location, somewhat like an enqueue operation.
For both array and heap PQs, when the array becomes full and has no more available indexes to store data, you must resize it to a larger array. Your larger array should be a multiple of the old array size, such as double the size. You must not leak memory; free all dynamically allocated arrays created by your class.
Remember to include the big-O of enqueue and dequeue in the comments in HeapPriorityQueue.h .
Style Details:
As in other assignments, you should follow our Style Guide for information about expected coding style. You are also expected to follow all of the general style constraints emphasized in the Homework 1-4 specs, such as the ones about good problem decomposition, parameters, redundancy, using proper C++ idioms, and commenting. The following are additional points of emphasis and style contraints specific to this problem. (Some of these may seem overly strict or arbitrary, but we need to constrain the assignment to force you to properly practice pointers and linked lists as intended.)
Commenting: Add descriptive comments to your .h and .cpp files. Both files should have top-of-file headers. One file should have header comments atop each member function (either the .h or .cpp; your choice). The .cpp file should have internal comments describing the details of each function's implementation.
Restrictions on pointers: The whole point of this assignment is to practice pointers and linked lists. Therefore, do not declare or use any other collections in any part of your code; all data should be stored in your linked list of nodes. There are some C++ "smart pointer" libraries that manage pointers and memory automatically, allocating and freeing memory as needed; you should not use any such libraries on this assignment.
Restrictions on private member variables: As stated previously, the only member variable (a.k.a. instance variable; private variable; field) you are allowed to have is a PatientNode* pointer to the front of your list. You may not have any other member variables. Do not declare an int for the list size. Do not declare members that are pointers to any other nodes in the list. Do not declare any collections or data structures as members.
Restrictions on modifying member function headers: Please do not make modifications to the PatientQueue class's public constructor or public member functions' names, parameter types, or return types. Our client code should be able to call public member functions on your queue successfully without any modification.
Restrictions on creation and usage of nodes: The only place in your code where you should be using the new keyword is in the newPatient function. No other members should use new or create new nodes under any circumstances. You also should not be modifying or swapping nodes' name values after they are added to the queue. In other words, you should implement all of the linked list / patient queue operations like upgradePatient by manipulating node pointers, not by creating entirely new nodes and not by modifying "data" of existing nodes.
You also should not create any more PatientNode structures than necessary. For example, if the client has called newPatient 6 times, your code should have created exactly 6 total PatientNode objects; no more, no less. You shouldn't, say, have a seventh empty "dummy" node at the front or back of the list that serves only as a marker, and you shouldn't accidentally create a temporary node object that is lost or thrown away. You can declare as many local variable pointers to nodes as you like.
Restrictions on traversal of the list: Any function that modifies your linked list should do so by traversing across your linked list a single time , not multiple times. For example, in functions like newPatient , do not make one traversal to find a node or place of interest and then a second traversal to manipulate/modify the list at that place. Do the entire job in a single pass.
Do not traverse the list farther than you need to. That is to say, once you have found the node of interest, do not unnecessarily process the rest of the list; break/return out of code as needed once the work is done.
Avoiding memory leaks: This item is listed under Style even though it is technically a functional requirement, because memory leakage is not usually visible while a program is running. To ensure that your class does not leak memory, you must delete all of the node objects in your linked list whenever data is removed or cleared from the list. You must also properly implement a destructor that deletes the memory used by all of the linked list nodes inside your PatientQueue object.
Frequently Asked Questions (FAQ):
For each assignment problem, we receive various frequent student questions. The answers to some of those questions can be found by clicking the link below.
Or, you can pass any of the value , priority , and next values on construction:
You *must* declare all your nodes as pointers; do not declare them as regular objects like the following code, because it will break when the list node goes out of scope:
Possible Extra Features:
For this problem, most good extra feature ideas involve adding operations to your queue beyond those specified. Here are some ideas for extra features that you could add to your program:
- Known list of diseases: Instead of, or in addition to, asking for each new patient's priority, ask what illness or disease they have, and use that to initialize the priority of newly checked-in patients. Then keep a table of known diseases and their respective priorities. For example, if the patient says that they have the flu, maybe the priority is 5, but if they say they have a broken arm, the priority is 2.
- Merge two queues: Write a member function that accepts another patient queue of the same type and adds all of its elements into the current patient queue. Do this merging "in place" as much as possible; for example, if you are merging two linked lists, directly connect the node pointers of one to the other as appropriate.
- Deep Copy: Make your queue properly support the = assignment statement, copy constructor, and deep copying. Google about the C++ "Rule of Three" and follow that guideline in your implementation.
- Iterator: Write a class that implements the STL iterator type and begin and end member functions in your queue, which would enable "for-each" over your PQ. This requires knowledge of the C++ STL library.
- Other: If you have your own creative idea for an extra feature, ask your SL and/or the instructor about it.
Indicating that you have done extra features: If you complete any extra features, then in the comment heading on the top of your program, please list all extra features that you worked on and where in the code they can be found (what functions, lines, etc. so that the grader can look at their code easily).
Submitting a program with extra features: Since we use automated testing for part of our grading process, it is important that you submit a program that conforms to the preceding spec, even if you want to do extra features. If your feature(s) cause your program to change the output that it produces in such a way that it no longer matches the expected sample output test cases provided, you should submit two versions of your program file: a first one with the standard file name without any extra features added (or with all necessary features disabled or commented out), and a second one whose file name has the suffix -extra.cpp with the extra features enabled. Please distinguish them in by explaining which is which in the comment header. Our turnin system saves every submission you make, so if you make multiple submissions we will be able to view all of them; your previously submitted files will not be lost or overwritten.
© Stanford 2017 | Created by Chris Gregg. CS106B has been developed over decades by many talented teachers.
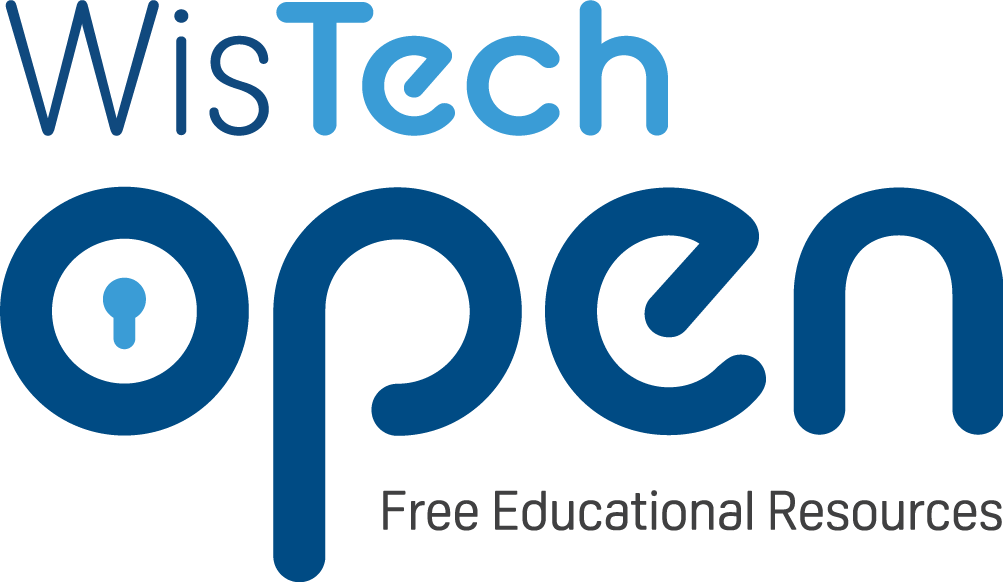
Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
2.3 Tools for Prioritizing
Prioritization of care for multiple patients while also performing daily nursing tasks can feel overwhelming in today’s fast-paced health care system. Because of the rapid and ever-changing conditions of patients and the structure of one’s workday, nurses must use organizational frameworks to prioritize actions and interventions. These frameworks can help ease anxiety, enhance personal organization and confidence, and ensure patient safety.
Acuity and intensity are foundational concepts for prioritizing nursing care and interventions. Acuity refers to the level of patient care that is required based on the severity of a patient’s illness or condition. For example, acuity may include characteristics such as unstable vital signs, oxygenation therapy, high-risk IV medications, multiple drainage devices, or uncontrolled pain. A “high-acuity” patient requires several nursing interventions and frequent nursing assessments.
Intensity addresses the time needed to complete nursing care and interventions such as providing assistance with activities of daily living (ADLs), performing wound care, or administering several medication passes. For example, a “high-intensity” patient generally requires frequent or long periods of psychosocial, educational, or hygiene care from nursing staff members. High-intensity patients may also have increased needs for safety monitoring, familial support, or other needs. [1]
Many health care organizations structure their staffing assignments based on acuity and intensity ratings to help provide equity in staff assignments. Acuity helps to ensure that nursing care is strategically divided among nursing staff. An equitable assignment of patients benefits both the nurse and patient by helping to ensure that patient care needs do not overwhelm individual staff and safe care is provided.
Organizations use a variety of systems when determining patient acuity with rating scales based on nursing care delivery, patient stability, and care needs. See an example of a patient acuity tool published in the American Nurse in Table 2.3. [2] In this example, ratings range from 1 to 4, with a rating of 1 indicating a relatively stable patient requiring minimal individualized nursing care and intervention. A rating of 2 reflects a patient with a moderate risk who may require more frequent intervention or assessment. A rating of 3 is attributed to a complex patient who requires frequent intervention and assessment. This patient might also be a new admission or someone who is confused and requires more direct observation. A rating of 4 reflects a high-risk patient. For example, this individual may be experiencing frequent changes in vital signs, may require complex interventions such as the administration of blood transfusions, or may be experiencing significant uncontrolled pain. An individual with a rating of 4 requires more direct nursing care and intervention than a patient with a rating of 1 or 2. [3]
Table 2.3 Example of a Patient Acuity Tool [4]
Read more about using a patient acuity tool on a medical-surgical unit.
Rating scales may vary among institutions, but the principles of the rating system remain the same. Organizations include various patient care elements when constructing their staffing plans for each unit. Read more information about staffing models and acuity in the following box.
Staffing Models and Acuity
Organizations that base staffing on acuity systems attempt to evenly staff patient assignments according to their acuity ratings. This means that when comparing patient assignments across nurses on a unit, similar acuity team scores should be seen with the goal of achieving equitable and safe division of workload across the nursing team. For example, one nurse should not have a total acuity score of 6 for their patient assignments while another nurse has a score of 15. If this situation occurred, the variation in scoring reflects a discrepancy in workload balance and would likely be perceived by nursing peers as unfair. Using acuity-rating staffing models is helpful to reflect the individualized nursing care required by different patients.
Alternatively, nurse staffing models may be determined by staffing ratio. Ratio-based staffing models are more straightforward in nature, where each nurse is assigned care for a set number of patients during their shift. Ratio-based staffing models may be useful for administrators creating budget requests based on the number of staff required for patient care, but can lead to an inequitable division of work across the nursing team when patient acuity is not considered. Increasingly complex patients require more time and interventions than others, so a blend of both ratio and acuity-based staffing is helpful when determining staffing assignments. [5]
As a practicing nurse, you will be oriented to the elements of acuity ratings within your health care organization, but it is also important to understand how you can use these acuity ratings for your own prioritization and task delineation. Let’s consider the Scenario B in the following box to better understand how acuity ratings can be useful for prioritizing nursing care.
You report to work at 6 a.m. for your nursing shift on a busy medical-surgical unit. Prior to receiving the handoff report from your night shift nursing colleagues, you review the unit staffing grid and see that you have been assigned to four patients to start your day. The patients have the following acuity ratings:
Patient A: 45-year-old patient with paraplegia admitted for an infected sacral wound, with an acuity rating of 4.
Patient B: 87-year-old patient with pneumonia with a low grade fever of 99.7 F and receiving oxygen at 2 L/minute via nasal cannula, with an acuity rating of 2.
Patient C: 63-year-old patient who is postoperative Day 1 from a right total hip replacement and is receiving pain management via a PCA pump, with an acuity rating of 2.
Patient D: 83-year-old patient admitted with a UTI who is finishing an IV antibiotic cycle and will be discharged home today, with an acuity rating of 1.
Based on the acuity rating system, your patient assignment load receives an overall acuity score of 9. Consider how you might use their acuity ratings to help you prioritize your care. Based on what is known about the patients related to their acuity rating, whom might you identify as your care priority? Although this can feel like a challenging question to answer because of the many unknown elements in the situation using acuity numbers alone, Patient A with an acuity rating of 4 would be identified as the care priority requiring assessment early in your shift.
Although acuity can a useful tool for determining care priorities, it is important to recognize the limitations of this tool and consider how other patient needs impact prioritization.
Maslow’s Hierarchy of Needs
When thinking back to your first nursing or psychology course, you may recall a historical theory of human motivation based on various levels of human needs called Maslow’s Hierarchy of Needs. Maslow’s Hierarchy of Needs reflects foundational human needs with progressive steps moving towards higher levels of achievement. This hierarchy of needs is traditionally represented as a pyramid with the base of the pyramid serving as essential needs that must be addressed before one can progress to another area of need. [6] See Figure 2.1 [7] for an illustration of Maslow’s Hierarchy of Needs.
Maslow’s Hierarchy of Needs places physiological needs as the foundational base of the pyramid. [8] Physiological needs include oxygen, food, water, sex, sleep, homeostasis, and excretion. The second level of Maslow’s hierarchy reflects safety needs. Safety needs include elements that keep individuals safe from harm. Examples of safety needs in health care include fall precautions. The third level of Maslow’s hierarchy reflects emotional needs such as love and a sense of belonging. These needs are often reflected in an individual’s relationships with family members and friends. The top two levels of Maslow’s hierarchy include esteem and self-actualization. An example of addressing these needs in a health care setting is helping an individual build self-confidence in performing blood glucose checks that leads to improved self-management of their diabetes.
So how does Maslow’s theory impact prioritization? To better understand the application of Maslow’s theory to prioritization, consider Scenario C in the following box.
You are an emergency response nurse working at a local shelter in a community that has suffered a devastating hurricane. Many individuals have relocated to the shelter for safety in the aftermath of the hurricane. Much of the community is still without electricity and clean water, and many homes have been destroyed. You approach a young woman who has a laceration on her scalp that is bleeding through her gauze dressing. The woman is weeping as she describes the loss of her home stating, “I have lost everything! I just don’t know what I am going to do now. It has been a day since I have had water or anything to drink. I don’t know where my sister is, and I can’t reach any of my family to find out if they are okay!”
Despite this relatively brief interaction, this woman has shared with you a variety of needs. She has demonstrated a need for food, water, shelter, homeostasis, and family. As the nurse caring for her, it might be challenging to think about where to begin her care. These thoughts could be racing through your mind:
Should I begin to make phone calls to try and find her family? Maybe then she would be able to calm down.
Should I get her on the list for the homeless shelter so she wouldn’t have to worry about where she will sleep tonight?
She hasn’t eaten in awhile; I should probably find her something to eat.
All of these needs are important and should be addressed at some point, but Maslow’s hierarchy provides guidance on what needs must be addressed first. Use the foundational level of Maslow’s pyramid of physiological needs as the top priority for care. The woman is bleeding heavily from a head wound and has had limited fluid intake. As the nurse caring for this patient, it is important to immediately intervene to stop the bleeding and restore fluid volume. Stabilizing the patient by addressing her physiological needs is required before undertaking additional measures such as contacting her family. Imagine if instead you made phone calls to find the patient’s family and didn’t address the bleeding or dehydration – you might return to a severely hypovolemic patient who has deteriorated and may be near death. In this example, prioritizing emotional needs above physiological needs can lead to significant harm to the patient.
Although this is a relatively straightforward example, the principles behind the application of Maslow’s hierarchy are essential. Addressing physiological needs before progressing toward additional need categories concentrates efforts on the most vital elements to enhance patient well-being. Maslow’s hierarchy provides the nurse with a helpful framework for identifying and prioritizing critical patient care needs.
Airway, breathing, and circulation, otherwise known by the mnemonic “ABCs,” are another foundational element to assist the nurse in prioritization. Like Maslow’s hierarchy, using the ABCs to guide decision-making concentrates on the most critical needs for preserving human life. If a patient does not have a patent airway, is unable to breathe, or has inadequate circulation, very little of what else we do matters. The patient’s ABCs are reflected in Maslow’s foundational level of physiological needs and direct critical nursing actions and timely interventions. Let’s consider Scenario D in the following box regarding prioritization using the ABCs and the physiological base of Maslow’s hierarchy.
You are a nurse on a busy cardiac floor charting your morning assessments on a computer at the nurses’ station. Down the hall from where you are charting, two of your assigned patients are resting comfortably in Room 504 and Room 506. Suddenly, both call lights ring from the rooms, and you answer them via the intercom at the nurses’ station.
Room 504 has an 87-year-old male who has been admitted with heart failure, weakness, and confusion. He has a bed alarm for safety and has been ringing his call bell for assistance appropriately throughout the shift. He requires assistance to get out of bed to use the bathroom. He received his morning medications, which included a diuretic about 30 minutes previously, and now reports significant urge to void and needs assistance to the bathroom.
Room 506 has a 47-year-old woman who was hospitalized with new onset atrial fibrillation with rapid ventricular response. The patient underwent a cardioversion procedure yesterday that resulted in successful conversion of her heart back into normal sinus rhythm. She is reporting via the intercom that her “heart feels like it is doing that fluttering thing again” and she is having chest pain with breathlessness.
Based upon these two patient scenarios, it might be difficult to determine whom you should see first. Both patients are demonstrating needs in the foundational physiological level of Maslow’s hierarchy and require assistance. To prioritize between these patients’ physiological needs, the nurse can apply the principles of the ABCs to determine intervention. The patient in Room 506 reports both breathing and circulation issues, warning indicators that action is needed immediately. Although the patient in Room 504 also has an urgent physiological elimination need, it does not overtake the critical one experienced by the patient in Room 506. The nurse should immediately assess the patient in Room 506 while also calling for assistance from a team member to assist the patient in Room 504.
Prioritizing what should be done and when it can be done can be a challenging task when several patients all have physiological needs. Recently, there has been professional acknowledgement of the cognitive challenge for novice nurses in differentiating physiological needs. To expand on the principles of prioritizing using the ABCs, the CURE hierarchy has been introduced to help novice nurses better understand how to manage competing patient needs. The CURE hierarchy uses the acronym “CURE” to guide prioritization based on identifying the differences among Critical needs, Urgent needs, Routine needs, and Extras. [9]
“Critical” patient needs require immediate action. Examples of critical needs align with the ABCs and Maslow’s physiological needs, such as symptoms of respiratory distress, chest pain, and airway compromise. No matter the complexity of their shift, nurses can be assured that addressing patients’ critical needs is the correct prioritization of their time and energies.
After critical patient care needs have been addressed, nurses can then address “urgent” needs. Urgent needs are characterized as needs that cause patient discomfort or place the patient at a significant safety risk. [10]
The third part of the CURE hierarchy reflects “routine” patient needs. Routine patient needs can also be characterized as “typical daily nursing care” because the majority of a standard nursing shift is spent addressing routine patient needs. Examples of routine daily nursing care include actions such as administering medication and performing physical assessments. [11] Although a nurse’s typical shift in a hospital setting includes these routine patient needs, they do not supersede critical or urgent patient needs.
The final component of the CURE hierarchy is known as “extras.” Extras refer to activities performed in the care setting to facilitate patient comfort but are not essential. [12] Examples of extra activities include providing a massage for comfort or washing a patient’s hair. If a nurse has sufficient time to perform extra activities, they contribute to a patient’s feeling of satisfaction regarding their care, but these activities are not essential to achieve patient outcomes.
Let’s apply the CURE mnemonic to patient care in the following box.
If we return to Scenario D regarding patients in Room 504 and 506, we can see the patient in Room 504 is having urgent needs. He is experiencing a physiological need to urgently use the restroom and may also have safety concerns if he does not receive assistance and attempts to get up on his own because of weakness. He is on a bed alarm, which reflects safety considerations related to his potential to get out of bed without assistance. Despite these urgent indicators, the patient in Room 506 is experiencing a critical need and takes priority. Recall that critical needs require immediate nursing action to prevent patient deterioration. The patient in Room 506 with a rapid, fluttering heartbeat and shortness of breath has a critical need because without prompt assessment and intervention, their condition could rapidly decline and become fatal.
In addition to using the identified frameworks and tools to assist with priority setting, nurses must also look at their patients’ data cues to help them identify care priorities. Data cues are pieces of significant clinical information that direct the nurse toward a potential clinical concern or a change in condition. For example, have the patient’s vital signs worsened over the last few hours? Is there a new laboratory result that is concerning? Data cues are used in conjunction with prioritization frameworks to help the nurse holistically understand the patient’s current status and where nursing interventions should be directed. Common categories of data clues include acute versus chronic conditions, actual versus potential problems, unexpected versus expected conditions, information obtained from the review of a patient’s chart, and diagnostic information.
Acute Versus Chronic Conditions
A common data cue that nurses use to prioritize care is considering if a condition or symptom is acute or chronic. Acute conditions have a sudden and severe onset. These conditions occur due to a sudden illness or injury, and the body often has a significant response as it attempts to adapt. Chronic conditions have a slow onset and may gradually worsen over time. The difference between an acute versus a chronic condition relates to the body’s adaptation response. Individuals with chronic conditions often experience less symptom exacerbation because their body has had time to adjust to the illness or injury. Let’s consider an example of two patients admitted to the medical-surgical unit complaining of pain in Scenario E in the following box.
As part of your patient assignment on a medical-surgical unit, you are caring for two patients who both ring the call light and report pain at the start of the shift. Patient A was recently admitted with acute appendicitis, and Patient B was admitted for observation due to weakness. Not knowing any additional details about the patients’ conditions or current symptoms, which patient would receive priority in your assessment? Based on using the data cue of acute versus chronic conditions, Patient A with a diagnosis of acute appendicitis would receive top priority for assessment over a patient with chronic pain due to osteoarthritis. Patients experiencing acute pain require immediate nursing assessment and intervention because it can indicate a change in condition. Acute pain also elicits physiological effects related to the stress response, such as elevated heart rate, blood pressure, and respiratory rate, and should be addressed quickly.
Actual Versus Potential Problems
Nursing diagnoses and the nursing care plan have significant roles in directing prioritization when interpreting assessment data cues. Actual problems refer to a clinical problem that is actively occurring with the patient. A risk problem indicates the patient may potentially experience a problem but they do not have current signs or symptoms of the problem actively occurring.
Consider an example of prioritizing actual and potential problems in Scenario F in the following box.
A 74-year-old woman with a previous history of chronic obstructive pulmonary disease (COPD) is admitted to the hospital for pneumonia. She has generalized weakness, a weak cough, and crackles in the bases of her lungs. She is receiving IV antibiotics, fluids, and oxygen therapy. The patient can sit at the side of the bed and ambulate with the assistance of staff, although she requires significant encouragement to ambulate.
Nursing diagnoses are established for this patient as part of the care planning process. One nursing diagnosis for this patient is Ineffective Airway Clearance . This nursing diagnosis is an actual problem because the patient is currently exhibiting signs of poor airway clearance with an ineffective cough and crackles in the lungs. Nursing interventions related to this diagnosis include coughing and deep breathing, administering nebulizer treatment, and evaluating the effectiveness of oxygen therapy. The patient also has the nursing diagnosis Risk for Skin Breakdown based on her weakness and lack of motivation to ambulate. Nursing interventions related to this diagnosis include repositioning every two hours and assisting with ambulation twice daily.
The established nursing diagnoses provide cues for prioritizing care. For example, if the nurse enters the patient’s room and discovers the patient is experiencing increased shortness of breath, nursing interventions to improve the patient’s respiratory status receive top priority before attempting to get the patient to ambulate.
Although there may be times when risk problems may supersede actual problems, looking to the “actual” nursing problems can provide clues to assist with prioritization.
Unexpected Versus Expected Conditions
In a similar manner to using acute versus chronic conditions as a cue for prioritization, it is also important to consider if a client’s signs and symptoms are “expected” or “unexpected” based on their overall condition. Unexpected conditions are findings that are not likely to occur in the normal progression of an illness, disease, or injury. Expected conditions are findings that are likely to occur or are anticipated in the course of an illness, disease, or injury. Unexpected findings often require immediate action by the nurse.
Let’s apply this tool to the two patients previously discussed in Scenario E. As you recall, both Patient A (with acute appendicitis) and Patient B (with weakness and diagnosed with osteoarthritis) are reporting pain. Acute pain typically receives priority over chronic pain. But what if both patients are also reporting nausea and have an elevated temperature? Although these symptoms must be addressed in both patients, they are “expected” symptoms with acute appendicitis (and typically addressed in the treatment plan) but are “unexpected” for the patient with osteoarthritis. Critical thinking alerts you to the unexpected nature of these symptoms in Patient B, so they receive priority for assessment and nursing interventions.
Handoff Report/Chart Review
Additional data cues that are helpful in guiding prioritization come from information obtained during a handoff nursing report and review of the patient chart. These data cues can be used to establish a patient’s baseline status and prioritize new clinical concerns based on abnormal assessment findings. Let’s consider Scenario G in the following box based on cues from a handoff report and how it might be used to help prioritize nursing care.
Imagine you are receiving the following handoff report from the night shift nurse for a patient admitted to the medical-surgical unit with pneumonia:
At the beginning of my shift, the patient was on room air with an oxygen saturation of 93%. She had slight crackles in both bases of her posterior lungs. At 0530, the patient rang the call light to go to the bathroom. As I escorted her to the bathroom, she appeared slightly short of breath. Upon returning the patient to bed, I rechecked her vital signs and found her oxygen saturation at 88% on room air and respiratory rate of 20. I listened to her lung sounds and noticed more persistent crackles and coarseness than at bedtime. I placed the patient on 2 L/minute of oxygen via nasal cannula. Within 5 minutes, her oxygen saturation increased to 92%, and she reported increased ease in respiration.
Based on the handoff report, the night shift nurse provided substantial clinical evidence that the patient may be experiencing a change in condition. Although these changes could be attributed to lack of lung expansion that occurred while the patient was sleeping, there is enough information to indicate to the oncoming nurse that follow-up assessment and interventions should be prioritized for this patient because of potentially worsening respiratory status. In this manner, identifying data cues from a handoff report can assist with prioritization.
Now imagine the night shift nurse had not reported this information during the handoff report. Is there another method for identifying potential changes in patient condition? Many nurses develop a habit of reviewing their patients’ charts at the start of every shift to identify trends and “baselines” in patient condition. For example, a chart review reveals a patient’s heart rate on admission was 105 beats per minute. If the patient continues to have a heart rate in the low 100s, the nurse is not likely to be concerned if today’s vital signs reveal a heart rate in the low 100s. Conversely, if a patient’s heart rate on admission was in the 60s and has remained in the 60s throughout their hospitalization, but it is now in the 100s, this finding is an important cue requiring prioritized assessment and intervention.
Diagnostic Information
Diagnostic results are also important when prioritizing care. In fact, the National Patient Safety Goals from The Joint Commission include prompt reporting of important test results. New abnormal laboratory results are typically flagged in a patient’s chart or are reported directly by phone to the nurse by the laboratory as they become available. Newly reported abnormal results, such as elevated blood levels or changes on a chest X-ray, may indicate a patient’s change in condition and require additional interventions. For example, consider Scenario H in which you are the nurse providing care for five medical-surgical patients.
You completed morning assessments on your assigned five patients. Patient A previously underwent a total right knee replacement and will be discharged home today. You are about to enter Patient A’s room to begin discharge teaching when you receive a phone call from the laboratory department, reporting a critical hemoglobin of 6.9 gm/dL on Patient B. Rather than enter Patient A’s room to perform discharge teaching, you immediately reprioritize your care. You call the primary provider to report Patient B’s critical hemoglobin level and determine if additional intervention, such as a blood transfusion, is required.
- Oregon Health Authority. (2021, April 29). Hospital nurse staffing interpretive guidance on staffing for acuity & intensity . Public Health Division, Center for Health Protection. https://www.oregon.gov/oha/PH/PROVIDERPARTNERRESOURCES/HEALTHCAREPROVIDERSFACILITIES/HEALTHCAREHEALTHCAREREGULATIONQUALITYIMPROVEMENT/Documents/NSInterpretiveGuidanceAcuity.pdf ↵
- Ingram, A., & Powell, J. (2018). Patient acuity tool on a medical surgical unit. American Nurse . https://www.myamericannurse.com/patient-acuity-medical-surgical-unit/ ↵
- Kidd, M., Grove, K., Kaiser, M., Swoboda, B., & Taylor, A. (2014). A new patient-acuity tool promotes equitable nurse-patient assignments. American Nurse Today, 9 (3), 1-4. https://www.myamericannurse.com/a-new-patient-acuity-tool-promotes-equitable-nurse-patient-assignments / ↵
- Welton, J. M. (2017). Measuring patient acuity. JONA: The Journal of Nursing Administration, 47 (10), 471. https://doi.org/10.1097/nna.0000000000000516 ↵
- Maslow, A. H. (1943). A theory of human motivation. Psychological Review , 50 (4), 370–396. https://doi.org/10.1037/h0054346 ↵
- " Maslow's_hierarchy_of_needs.svg " by J. Finkelstein is licensed under CC BY-SA 3.0 ↵
- Stoyanov, S. (2017). An analysis of Abraham Maslow's A Theory of Human Motivation (1st ed.). Routledge. https://doi.org/10.4324/9781912282517 ↵
- Kohtz, C., Gowda, C., & Guede, P. (2017). Cognitive stacking: Strategies for the busy RN. Nursing2021, 47 (1), 18-20. https://doi.org/10.1097/01.nurse.0000510758.31326.92 ↵
The level of patient care that is required based on the severity of a patient’s illness or condition.
A staffing model used to make patient assignments that reflects the individualized nursing care required for different types of patients.
A staffing model used to make patient assignments in terms of one nurse caring for a set number of patients.
Prioritization strategies often reflect the foundational elements of physiological needs and safety and progress toward higher levels.
Airway, breathing, and circulation.
Pieces of clinical information that direct the nurse toward a potential “actual problem” or a change in condition.
Conditions having a sudden and severe onset.
Have a slow onset and may gradually worsen over time.
Nursing problems currently occurring with the patient.
A nursing problem that reflects that a patient may experience a problem but does not currently have signs reflecting the problem is actively occurring.
Conditions that are not likely to occur in the normal progression of an illness, disease or injury.
Conditions that are likely to occur or anticipated in the course of an illness, disease, or injury.
Nursing Management and Professional Concepts Copyright © by Chippewa Valley Technical College is licensed under a Creative Commons Attribution 4.0 International License , except where otherwise noted.
Share This Book

An official website of the United States government
The .gov means it’s official. Federal government websites often end in .gov or .mil. Before sharing sensitive information, make sure you’re on a federal government site.
The site is secure. The https:// ensures that you are connecting to the official website and that any information you provide is encrypted and transmitted securely.
- Publications
- Account settings
Preview improvements coming to the PMC website in October 2024. Learn More or Try it out now .
- Advanced Search
- Journal List

A systematic review of patient prioritization tools in non-emergency healthcare services
Julien déry.
1 Department of Rehabilitation, Université Laval, Québec, Canada
2 Centre interdisciplinaire de recherche en réadaptation et intégration sociale (CIRRIS), Centre intégré universitaire de santé et de services sociaux de la Capitale-Nationale, Québec, Canada
3 Faculty of Business Administration, Université Laval, Québec, Canada
4 Centre interuniversitaire de recherche sur les réseaux d’entreprise, la logistique et le transport (CIRRELT), Université de Montréal, Montréal, Canada
François Routhier
Valérie bélanger.
5 Department of Logistics and Operations Management, HEC Montréal, Montréal, Canada
André Côté
6 Centre de recherche du CHU de Québec, Université Laval, Québec, Canada
7 Centre de recherche en gestion des services de santé, Université Laval, Québec, Canada
Daoud Ait-Kadi
8 Department of Mechanical Engineering, Université Laval, Québec, Canada
Marie-Pierre Gagnon
9 Faculty of Nursing, Université Laval, Québec, Canada
Simon Deslauriers
Ana tereza lopes pecora, eduardo redondo, anne-sophie allaire, marie-eve lamontagne, associated data.
All data generated or analyzed during this study are included in this published article and its supplementary information files.
Patient prioritization is a strategy used to manage access to healthcare services. Patient prioritization tools (PPT) contribute to supporting the prioritization decision process, and to its transparency and fairness. Patient prioritization tools can take various forms and are highly dependent on the particular context of application. Consequently, the sets of criteria change from one context to another, especially when used in non-emergency settings. This paper systematically synthesizes and analyzes the published evidence concerning the development and challenges related to the validation and implementation of PPTs in non-emergency settings.
We conducted a systematic mixed studies review. We searched evidence in five databases to select articles based on eligibility criteria, and information of included articles was extracted using an extraction grid. The methodological quality of the studies was assessed by using the Mixed Methods Appraisal Tool. The article selection process, data extraction, and quality appraisal were performed by at least two reviewers independently.
We included 48 studies listing 34 different patient prioritization tools. Most of them are designed for managing access to elective surgeries in hospital settings. Two-thirds of the tools were investigated based on reliability or validity. Inconclusive results were found regarding the impact of PPTs on patient waiting times. Advantages associated with PPT use were found mostly in relationship to acceptability of the tools by clinicians and increased transparency and equity for patients.
Conclusions
This review describes the development and validation processes of PPTs used in non-urgent healthcare settings. Despite the large number of PPTs studied, implementation into clinical practice seems to be an open challenge. Based on the findings of this review, recommendations are proposed to develop, validate, and implement such tools in clinical settings.
Systematic review registration
PROSPERO CRD42018107205
Patients from countries with publicly funded healthcare systems frequently experience excessive wait times [ 1 ], with sometimes dramatic consequences. Patients waiting for non-urgent health services such as elective surgeries and rehabilitation services can suffer physical and psychological sequelae [ 2 ]. To reduce these negative effects, waiting lists should be managed as fairly as possible to ensure that patients with greater or more serious needs are given priority for treatment [ 3 ].
Patient prioritization, defined as the process of ranking referrals in a certain order based on criteria, is one of the possible strategies to improve fairness in waiting list management [ 4 ]. This practice is used in many settings, and it differs from a first-in first-out (FIFO) approach that ranks patients on waiting lists chronologically, according to their arrival. It also differs from triage methods used in emergency departments where patients are sorted into broader categories (e.g., low/moderate/high priority or service/no service). Prioritization is related to non-urgent services involving a broader range of timeframes and patient types [ 2 , 5 ]. The use of prioritization is widely reported, yet not fully described, in services provided by allied health professionals, including physical therapists, occupational therapists, or psychologists as well as multidisciplinary allied health teams [ 2 ]. In practice, however, assessing patients’ priority on the basis of explicit criteria is complex and, to a certain degree, inconsistent [ 6 – 8 ]. Moreover, most prioritization criteria are subjectively defined, and comparing patients’ needs and referrals can be challenging [ 8 ].
Patient prioritization tools are designed to support the decision process leading to patient sorting in an explicit, transparent, and fair manner. Such tools or systems are usually set up to help clinicians or managers to make decisions about which patients should be seen first when demand is great and resources are limited [ 2 ]. We define patient prioritization tools as paper-and-pencil or computer-based instruments that support patient prioritization processes, either by stating explicit and standardized prioritization criteria, or by enabling easier or better calculation of priority scores, or because they automatically include the patients into a ranked list. PPTs are mainly built around sets of general criteria that encompass personal factors (i.e., age), social factors (i.e., ability to work), clinical factors (i.e., patients’ quality of life), and any other factor deemed relevant [ 1 , 3 , 5 ]. Since tools can take various forms and they are very dependent on the particular context of application, some of the literature reports a lack of consistency in the way they are developed [ 5 , 6 ].
In healthcare, the process of validating a new tool to measure an abstract phenomenon such as quality of life, patient adherence, and urgency/needs in the case of PPTs, is in large part oriented toward the evaluation and the reduction of errors in the measurement process [ 9 ]. The process of a measure, a tool, or an instrument’s quality assessment involves investigating their reliability and validity. Reliability estimates are used to evaluate the stability of measures gathered at different time intervals on the same individuals (intra-rater reliability), the link and coherence of items from the same test (internal consistency), or the stability of scores for a behavior or event using the same instrument by different observers (inter-rater reliability) [ 9 ]. There are many approaches to measure validity of a tool and in the context of our review, we identified those more relevant. Construct validity is the extent to which the instrument measures what it says it measures [ 10 ]. Content validity addresses the representativeness of the measurement tool in capturing the major elements or different facets relevant to the construct being measured, which can be in part assessed by stakeholders’ opinion (face validity) [ 10 ]. Criterion-related validity evaluates how closely the results of a tool correspond to the results of another tool. As in the case of patient prioritizing, much of the research conducted in healthcare involves quantifying abstract concepts that cannot be measured precisely, such as the severity of a disease and patient satisfaction. Validity evidence is built over time, with validations occurring in a variety of populations [ 9 ].
Aside from the validation process, there is no consensus in the literature about the effect of PPTs on healthcare service delivery, patient flow, or stakeholders. Some studies state that the prioritization process is associated with lower waiting times [ 11 – 13 ], but a systematic review of triage systems indicates mixed results on waiting time reduction [ 14 ]. It is difficult to assess these findings considering the variance between research settings and the nature of the considered prioritization system, tool, or process used in the studies. For example, Harding et al. [ 14 ] included studies about any system that either ranked patients in order of priority or sorted patients into the most appropriate service, which are two completely different systems. These authors also merged results from emergency and non-emergency settings, which are contrasting contexts of healthcare. Emergency refers to contexts where patients present life-threatening symptoms requiring immediate clinical action. In the case of non-urgent healthcare services, also referred to as elective services, access is organized according to priorities and level of need [ 3 ]. Besides the outcome related to review evidence that highlights other indicators of quality related to PPTs and their effects on the process of care and users.
The prioritization process in various non-urgent settings may vary significantly according to the kind of PPT used. The heterogeneity of the tools is reflected in the array of outcomes used to evaluate the effectiveness of PPT, which makes it difficult to have a broader and systematic understanding of their real impact on clinical practices and patient health outcomes.
The goal of this paper is to systematically review and synthesize the published evidence concerning PPTs in non-emergency settings in order to (1) describe PPT characteristics, such as format, scoring description, population, setting, purpose, criteria, developers, and benefits/limitations, (2) identify the validation approaches proposed to enhance the quality of the tools in practice, and (3) describe their effect or outcome measures (e.g., shorter wait times).
The detailed methods of this systematic review are reported in a published protocol [ 15 ], but some key elements are the following. The review has been registered in the PROPERO database (CRD42018107205). This review is based on the stages proposed by Pluye and Hong [ 16 ] to guide systematic reviews and it is reported in accordance with the Preferred Reporting Items for Systematic Reviews and Meta-Analyses (PRISMA) statement [ 17 ]. The PRISMA 2009 checklist is joined in Additional file 1 .
Search strategy
Two of the authors (JD and MEL), helped by a professional librarian, identified the search strategy for this systematic review in accordance with the research objectives. An example of a search query in the Medline (Ovid) database is presented in Additional file 2 . First, JD and SD imported into a reference management software (Endnote) the records from five source databases: Medline, Embase, CINAHL, Web of Science, and the Cochrane Library. We did not apply any restrictions on the papers’ publication date. A secondary search was performed according to the following four steps: (1) screening of the lists of references in the articles identified; (2) citation searches performed using Google Scholar for records that meet the inclusion criteria; (3) screening of 25 similar references suggested by the databases, where available; and (4) contacting the researchers who authored two articles or more included in our review.
Eligibility criteria
We selected articles that met the following inclusion criteria: (1) peer-reviewed quantitative/qualitative/mixed methods empirical studies, which includes all qualitative research methods (i.e., phenomenological, ethnographic, grounded theory, case study, and narrative) and quantitative research designs (i.e., randomized controlled studies, cohort studies, case control, cross sectional, descriptive, and longitudinal); (2) published in English or French; (3) reporting the use of a PPT in a non-emergency healthcare setting. We excluded references based on the following four exclusion criteria: (1) studies focusing on strategies/methods of waiting list management not using a prioritization tool or system; (2) studies conducted to fit an emergency setting; (3) articles dealing with critical or life-threatening situations (i.e., organ transplants); and (4) literature reviews.
Screening and selection process
Two steps were applied to remove duplicates. First, we used the software command “Find duplicates” on the “title” field of the references. Then, JD and MEL independently screened all the references identified from the search and manually deleted remaining duplicates. In the screening process, first, titles and abstracts were analyzed to extract relevant articles. Whenever a mismatch on the relevance of an article arose between JD and MEL, the authors discussed the paper until a consensus was reached. Second, JD read the full texts of all the extracted articles to select those that were relevant based on our eligibility criteria. MEL, AR, and VB validated the article selection, and disagreements were resolved by discussion.
Data extraction
All articles in the final list were reviewed by JD using an extraction grid. First, JD extracted information related to the studies, such as the authors, title, year of publication, country, population, purpose of the study, and setting. Second, JD documented the information about the tool used in the study, including format, description, developers, development process, criteria, reliability, validity, and outcome assessment, relevant results, and implementation process. The grid and information extracted by JD were validated independently by six research team members.
Data synthesis
We used a data-based convergent qualitative synthesis method to describe the results of the systematic mixed studies review [ 16 ]. As described by Hong et al. [ 18 ], all included studies are analyzed using the same synthesis method and results are presented together. Since only one synthesis method is used for all evidence, data transformation is involved. In our review, results from all studies were transformed into qualitative findings [ 18 ]. We extracted qualitative data related to the objectives of the review from all the manuscripts included. In the extraction grid, we conducted a hybrid deductive-inductive approach [ 18 ] using predefined themes (e.g., developers and prioritization criteria), then we assigned data to themes, and new themes derived from data (e.g., types of outcome measured in the included studies). Quantitative data presented in our review are the numbers of occurrences of the qualitative data extracted in the included studies.
Critical appraisal
The methodological quality of the studies selected was assessed by three independent assessors (JD, ATP, ASA) using the mixed methods appraisal tool (MMAT-version 2018) [ 19 , 20 ], which, on its own, allows for concomitantly appraising all types of empirical studies, whether mixed, quantitative, or qualitative [ 19 ]. Each study was assigned a score, from 0 to 5, based on the number of criteria met. We did not exclude studies with low MMAT scores.
Description of included articles
The database search was conducted from 24 September 2018, to 14 January 2019. It was updated on 4 November 2019. We screened a total of 12,828 records after removing duplicates. We assessed 115 full-text articles for eligibility and from those, 67 were excluded based on at least one exclusion criterion. Figure Figure1 1 presents the PRISMA flow diagram for inclusion of the 48 relevant papers [ 13 , 21 – 67 ].

PRISMA flow diagram from Moher et al. [ 17 ]
The articles included were published between 1989 and 2019. Most of the studies were conducted in Canada [ 22 , 23 , 25 , 26 , 30 – 33 , 40 , 42 , 48 , 50 , 53 , 57 , 59 , 61 , 67 ], Spain [ 21 , 28 , 29 , 37 , 38 , 41 , 52 , 54 – 56 , 60 , 62 ], and New Zealand [ 27 , 34 – 36 , 49 , 58 , 63 ]. As presented in Table Table1, 1 , these studies’ stated goals were mainly related to evaluating validity and reliability, developing prioritization criteria, and creating prioritization tools.
Study characteristics of the included articles related to each PPT
a Quality score ranged from meeting none of five criteria (0) to meeting all criteria (5)
Three development processes of similar tools stand out in our review based on the number of studies conducted, one from the Western Canada Waiting List Project [ 68 ], which produced 12 studies included in our review [ 22 , 26 , 30 – 33 , 40 , 42 , 48 , 57 , 59 , 61 ], one from Spanish research groups including 10 studies [ 21 , 28 , 29 , 37 , 38 , 41 , 55 , 56 , 60 , 62 ], and one from four New Zealand researcher studies [ 34 – 36 , 49 ]. They are reviewed in more detail in the following paragraphs.
Western Canada Waiting List (WCWL) project
The WCWL project was a collaborative initiative undertaken by 19 partner organizations to address five areas where waiting lists were considered to be problematic: cataract surgery, general surgery, hip and knee replacement, magnetic resonance imaging (MRI), and children’s mental health services [ 68 ]. Hadorn’s team developed point-count systems using statistical linear models [ 68 ]. These types of systems have been developed for many clinical and non-clinical contexts such as predicting mortality in intensive care units (APACHE scoring system [ 69 ]) and neonatal assessment (Apgar score [ 70 ]). In this project, the researchers developed tools using priority scores in the 0-100 range based on weighted prioritization criteria. Based on the studies included in our review, a panel of experts adopted a set of criteria, incorporated them in a questionnaire to rate a series of consecutive patients in their practices, and then used regression analysis to determine the statistically optimal set of weights on each criterion to best predict (or correlate with) overall urgency [ 42 , 57 , 59 , 61 ]. Reliability [ 22 , 33 , 40 , 42 , 57 , 61 ] and validity [ 26 , 30 – 33 ] were also assessed for most of the tools created by this research team and the key results are presented in Additional file 4 .
A group of researchers in Spain developed a four-step approach to designing prioritization tools. The four steps included (1) systematic review to gather available evidence about waiting list problems and prioritization criteria used, (2) compilation of clinical scenarios, (3) expert panel consultations provided with the literature review and the list of scenarios, (4) rating of the scenarios and criteria weighting, carried out in two rounds using a modified Delphi method. The researchers then evaluated the reliability of the tool in the context of hip and knee surgeries [ 21 , 38 , 55 ] as well as its validity for cataract surgeries [ 21 , 37 , 41 , 56 ] and these results are detailed in Additional file 4 .
New Zealand
The third tool detailed in our review was developed by New Zealand researchers. Clinical priority assessment criteria (CPAC) were defined for multiple elective surgery settings [ 35 , 36 , 49 ] based on a previous similar work [ 34 ]. Validity results of these tools are presented in Additional file 4 . These tools were part of an appointment system aimed at reforming the access to elective surgery policy in order to improve equity, provide clarity for patients, and achieve a paradigm shift by relating likely benefits from surgery to the available resources [ 36 ]. The development process was not described in detail in the studies included. As discussed in one study, implementation of the system encountered some difficulties, mostly in achieving consensus on the components and the weighting of the various categories of prioritization criteria [ 36 ].
Designs and quality of included studies
We appraised the methodological quality of the 48 articles using MMAT, which allowed for quality appraisal based on the design of the studies assessed. One was a mixed methods study [ 50 ], four were qualitative studies [ 36 , 43 , 48 , 60 ], five were quantitative descriptive studies [ 35 , 37 , 49 , 52 , 66 ], none were randomized controlled trials, and the remaining 38 were quantitative non-randomized studies. From these quantitative studies, most were cross sectional, prospective, or retrospective design studies. The overall methodological quality of the articles was good, with a mean score of 3.81/5 (range from 0 to 5). Score associated with each study is presented in Table Table1 1 .
Characteristics of the prioritization tools
We listed 34 distinct PPTs from 46 articles reviewed. Two articles [ 46 , 48 ] were included even though no specific PPT was used or developed in these studies. In Kingston and Masterson’s study [ 46 ] (MMAT score = 0), the Harris Hip Score and the American Knee Society Score were used as the scoring instruments to determine priority in the waiting list, and in McGurran et al.’s article [ 48 ], the (MMAT score = 2) authors consulted the general public to collect their opinion on appropriateness, acceptability, and implementation of waiting list PPTs. Table Table1 1 shows that PPTs were mostly used in hospital settings (19/34) for arthroplasty (9/34), cataract surgery (5/34), and other elective surgeries (4/34). We found that a different set of tools support prioritization in 14 other healthcare services. Three tools were designed for primary care, three for outpatient clinics, one for community-based care, and one for rehabilitation. Three studies [ 26 , 43 , 52 ] portrayed the use of a PPT in multiple settings, and four studies [ 39 , 42 , 51 , 64 ] did not specify the setting. The PPTs reviewed were mostly (17/34) tools attributing scores ranging from 0 to 100 to patients based on weighted criteria. Other tools (8/34) used priority scores that sorted patients into broad categories (e.g., low, intermediate, high priority). The format of the PPTs reported in the studies were mostly unspecified (26/34), but some explicitly specified that the tool was either in paper (4/34) or electronic (2/34) format.
Several stakeholders were involved in the development of the 34 PPT retrieved, such as clinicians (50% of the PPT), specialists (35%), surgeons (29%), general practitioners (26%), and others (Fig. (Fig.2). 2 ). It is worth mentioning that patients and caregivers were involved in only 15% of the PPTs developed [ 21 , 38 , 52 , 60 , 63 , 65 ], while for 21% of the PPTs, authors did not specify who participated in their development.

Stakeholders participating in the PPTs development
Regarding the development process, we have not identified guidelines or standardized procedures explaining how the proposed PPTs were created. Some authors reported relying on literature reviews (44%) and stakeholder consultations (53%) to inform PPT design, but most provided very little information about the other steps of development.
Below is a review of the criteria elected to produce the different PPTs found in this synthesis. First, the number of criteria ranges from 2 to 17 (mean: 7.6, SD: 3.8). As regards their nature or orientation, some PPTs are related to generic criteria, others are specific to a disease, a service, or a population, as reported in Table Table2. 2 . The criteria of each PPT are listed in Additional file 3 .
Type of criteria reported at least once in PPTs
In summary, PPTs are typically used in hospital settings for managing access to hip, knee, cataract, and other elective surgeries. Their format is undefined, their development process is non-standardized, and they are mostly developed by consulting clinicians and physicians (surgeons, general practitioners, and specialists).
Reliability and validity of PPTs
Only 26 out of the 48 articles included in this synthesis, representing 23 tools (68%), reported an investigation of at least one of the qualities of the measuring instrument, i.e., reliability and validity. Figure Figure3 3 displays the scope of aspects that were assessed.

Portion of the 34 PPTs assessed for each aspect of quality
Inter-rater [ 21 – 24 , 33 , 38 , 40 , 42 , 45 , 47 , 50 , 55 , 57 , 61 , 65 ] and intra-rater [ 22 , 24 , 33 , 40 , 42 , 47 , 55 , 57 , 61 ] reliability were evaluated by comparing the priority ratings of two groups of raters (inter) and by comparing priority ratings by the same raters at two different points in time (intra). Face validity [ 33 , 38 , 41 , 47 , 52 , 55 ] was determined by consultation with stakeholders (e.g., surgeons, clinicians, patients, etc.). Other validity assessments, such as content [ 41 , 47 , 55 ], construct [ 21 , 23 , 26 , 27 , 30 – 32 , 34 , 35 , 37 , 52 , 63 ], and criterion [ 26 , 32 , 35 , 41 , 45 , 47 , 52 , 55 ] validity were appraised using correlations between PPT results and other measures. In fact, some studies compared PPT scores with a generic health-related quality of life measure such as the Short Form Health Survey (both SF-36 and SF-12) [ 35 , 55 , 63 ]. Aside from correlations with other measures, PPT validity was evaluated using two other means: disease-specific questionnaires (e.g., the Western Ontario and McMaster Universities Arthritis Index [ 27 , 30 , 31 , 37 , 55 ] and the Visual Function Index [ 35 , 41 ]) or another measure of urgency/priority (e.g., the Visual Analog Scale [ 21 , 22 , 26 , 30 – 32 , 42 , 61 ] or a traditional method [ 47 ]).
One of the objectives of this review was to synthesize results about the quality features of each PPT. The diversity of contexts, settings, and formats PPTs adopted made a fair and reasonable comparison almost impossible. We observed various methods of assessing reliability and validity of PPTs across a number of settings. All the findings relating to the features reported in the articles are presented in Additional file 4 . We can conclude that the reliability and the validity of PPTs have generally been assessed as acceptable to good.
Effects on the waiting list process
Assessing actual benefits remains, in our opinion, one of the most important drawbacks of the reported PPTs. In fact, we found that only 10 studies [ 13 , 28 , 29 , 39 , 44 , 46 , 50 , 58 , 62 , 64 ] investigated the effects or outcomes of the proposed PPTs, while six other studies [ 23 , 36 , 48 – 50 , 67 ] merely reported opinions expressed by stakeholders about essential benefits and limitations of PPTs.
Waiting time is the most studied outcome assessment [ 13 , 28 , 29 , 39 , 58 , 62 , 64 ]. Four studies [ 28 , 29 , 39 , 62 ] used a computer simulation model to evaluate the impact of the PPT on waiting times. In their simulations, the authors compared the use of a PPT to the FIFO model and reported mixed findings. Comas et al. [ 28 , 29 ] showed that prioritization systems produced better results than a FIFO strategy in the contexts of cataract and knee surgeries. They concluded that the waiting times weighted by patient priority produced by prioritization systems were 1.54 and 4.5 months shorter than the ones produced by FIFO in the case of cataract and knee surgeries, respectively. Another study [ 39 ] revealed, in regard to cataract surgery, that the prioritization system concerned made it possible for patients with the highest priority score (91-100) to wait 52.9 days less than if the FIFO strategy were used. In contrast, patients with the lowest priority score (1-10) saw their mean waiting time increase from 193.3 days (FIFO) to 303.6 days [ 39 ]. Tebé et al. [ 62 ] noted that the application of a system of prioritization seeks to reorder the list so that patients with a higher priority are operated on earlier. However, this does not necessarily mean an overall reduction in waiting times. These authors concluded that although waiting times for knee arthroplasty dropped to an average wait of between 3 and 4 months throughout the period studied, they could not ascertain that it was directly related to the use of the prioritization system [ 62 ].
The other three studies conducted a retrospective analysis of patients on waiting lists [ 13 , 58 , 64 ]. With a PPT [ 13 ] using a total score of priority then sorting patients in groups (group 1 having the greatest need for surgery and group 4 the least need), the mean waiting time for surgery was 3 years shorter across all indication groups. In a study with patients waiting for cardiac surgery, the clinician’s classification was compared to the New Zealand priority scores (0-100) based on clinical features [ 58 ]. According to this study, it is difficult to determine whether waiting times were reduced as a result of the use of the PPT, because findings only showed the reorganization of the waiting list based on each category and priority scores. However, waiting times were reduced for the least urgent patients in both groups. Waiting times before surgery were between 161 and 1199 days based on the clinician’s classifications compared to waiting times related to New Zealand priority scores, which were between 58 and 486 days [ 58 ]. In addition, Valente et al. [ 6 ] studied a model to prioritize access to elective surgery and found no evident effects in terms of reduction or increase of the overall waiting list length.
Effects on the care process
Some studies address the effects of PPT on the demand for healthcare services [ 44 , 46 , 50 , 64 ]. The introduction of a new need-based prioritization system for hip and knee arthroplasty has reduced the number of inquiries and cancelations [ 46 ]. Changes were also observed after the implementation of a PPT for physiotherapy services with an increase of 38% of high priority clients in their caseload [ 50 ]. PPTs also had an impact on the healthcare delivery process. For example, Mifflin and Bzdell [ 50 ] reported improvement in communication between physiotherapists and other health professionals in remote areas. Furthermore, Isojoki et al. [ 44 ] demonstrated that the priority ratings made by experts in adolescent psychiatry were correlated with the type and duration of the treatment received. This suggests that the PPT identified adolescents with the greatest need of psychiatric care and that it might, to some extent, predict the intensity of the treatment to be delivered [ 44 ]. The system proposed by Valente et al. [ 64 ] enabled easy and coherent scheduling and reduced postponements [ 64 ].
Other outcomes related to the use of PPTs
Although some attempts have been made to assess the positive impacts of PPTs on patients, the results reported are not consistent enough to confirm such benefits. Seddon et al. [ 58 ] stated that priority scores for cardiac surgery prioritize patients as accurately as clinician assessments do according to the patients’ risk of cardiac events (cardiac death, myocardial infarction, and cardiac readmission). In their study concerning a PPT for patients waiting for hip and knee arthroplasty, Kingston and Masterson [ 46 ] used two instruments to measure patients’ priority scores (the Harris Hip Score and the American Knee Society Score), and the mean joint score for patients on the waiting list remained unchanged a year after the introduction of the new system.
Benefits and limitations of PPTs
The challenge of producing long-term assessments of PPT benefits has led researchers to rely on qualitative methods, i.e., stakeholder perceptions concerning acceptability and benefits of PPTs. Focus groups including the general public indicated that the tools presented in five different clinical areas 1 were appropriate and acceptable [ 48 ]. Other studies [ 23 , 36 , 49 , 50 , 67 ] examined perceptions of PPT stakeholders—clinicians, managers, and surgeons—about the tools. Clinicians using a PPT in clinical practice stated that it promotes a shared and more homogeneous vision of patients’ needs and that it helps to gather relevant information about them [ 23 ]. It also improved transparency and equity for patients, as well as accuracy of waiting times [ 36 ]. In another study, physiotherapists reported increased job satisfaction, decreased job stress, and less time spent triaging referrals [ 50 ]. They also commented that, compared to the methods used before the tool was introduced [ 50 ], PPT allowed physiotherapy services to be delivered more equitably. In Rahimi et al.’s study [ 67 ], surgeons reported that the PPT provides a precise and reliable prioritization that is more effective than the prioritization method currently in use.
On a less positive note, some authors reported that PPTs were perceived as lacking flexibility, which limited their acceptance by surgeons [ 36 , 49 ]. In a study surveying surgeons about the use of PPTs, only 19.5% agreed that current PPTs were an effective method of prioritizing patients, and 44.8% felt that further development of surgical scoring tools had the potential to provide an effective way of prioritizing patients [ 49 ]. In fact, most surgeons felt that their clinical judgment was the most effective way of prioritizing patients [ 49 ]. Many studies mentioned the need to support implementation of PPTs in clinical practice and to involve potential tool users in the implementation process [ 21 , 31 , 48 , 57 , 60 ]. In this vein, another recommendation concerning implementation was to secure agreement and to assess acceptability of the criteria and the tool in clinical settings [ 60 ]. A panel of experts recommended that a set of operational definitions and instructions be prepared to accompany the criteria in order to make the tool more reliable [ 57 ]. Implementation should also involve continuous monitoring and an evaluation of the effects of implementation on patient outcomes, on resource use, and on the patient-provider relationship [ 31 ].
The aim of this systematic review was to gather and synthesize information about PPTs in non-emergency healthcare contexts in order to develop a better understanding of their characteristics and to find out to what extent they have been proven to be reliable, valid, and effective methods of managing access to non-urgent healthcare services. A significant number of PPTs are discussed in the literature, most of them designed to prioritize patients waiting for elective surgeries such as, but not limited to, cataract, knee, and hip operations. We also discovered a broad range of studies assessing PPT reliability and validity. The wide range of methods underlying the tools and contexts in which they are used to make a fair assessment of their quality very difficult. We nonetheless noted that the overall assessment of PPT reliability and validity were reported as being acceptable to good. We have also synthesized the quality assessment processes conducted in the literature to apprise interested readers and encourage further research on PPT development and validation. The overall effectiveness of PPTs was difficult to demonstrate according to our findings, essentially because the articles showed mixed results about reduction of waiting times and because of the lack of studies measuring PPT impact on patients. However, a few studies demonstrated benefits according to the general public, clinicians, and physicians, in terms of a more equitable and reliable delivery of services. PPTs are used to manage access to a given healthcare service by helping service providers to prioritize patients on a waiting list. The use of PPTs as scoring measures provides a relatively transparent and standardized method for assigning priority to patients on waiting lists [ 68 ]. Measuring abstract constructs, such as the relative priority of the patients’ need to obtain access to a healthcare service is, indeed, a complex task.
The findings of this review show that PPT development processes are, at best, heterogeneous. However, our systematic review demonstrates that certain development steps are present in most studies. Based on these results, and supported by studies that showed reliable and valid PPTs, we can draw some recommendations or guidelines for the development of reliable, valid, user-friendly, and acceptable tools (see Table Table3). 3 ). Recommendations are formulated regarding preliminary steps, development, evaluation, and finally the implementation of PPTs.
Recommendations and guidelines for PPT development projects
Our systematic review broadens earlier research reviewing priority scoring tools used for prioritizing patients in elective surgery settings [ 5 ] to more general patient prioritization tools and to all non-urgent healthcare settings.
Harding et al. conducted two systematic reviews, which focused specifically on triage methods [ 6 ] and their impact on patient flow in a broad spectrum of health services [ 14 ]. Our review distinguishes prioritization tools and triage systems. Triage and prioritization are often used interchangeably because both terms refer to allocating services to patients at the point of service delivery [ 2 ]. In fact, triage was traditionally associated with emergency services, but it was also used in other healthcare settings, and it relates to the process of making a decision about the type of service needed (for example, the need for a physical therapist versus a therapy assistant) and whether there is such a need at all [ 2 ]. Prioritization is a process of ranking patient needs for non-urgent services and as such, it involves a broader range of timeframes and patient types [ 2 , 5 ]. Whereas triage sorts patients into broader categories (e.g., low/moderate/high priority or service/no service), it can nevertheless imply a prioritization process, as presented in our results.
Harding et al.’s review [ 14 ] included any system that either ranked patients in order of priority, or sorted patients into the most appropriate service. Additionally, 64% of the studies included were conducted in acute care hospital emergency departments [ 14 ]. Since both terms coexist in the literature, we included studies that used prioritization tools, which only rank patients in order of priority. Based on this, we obtained a better picture of the prioritization tools used, and we found that prioritization is more often used in non-urgent healthcare settings, without using a sorting (triage) process.
By excluding studies in emergency and life-threatening settings, we can assume that the evidence reviewed relates more to an assessment of patient priority based on needs and social characteristics, and less to an urgency to receive a given service [ 3 ]. As demonstrated by the wide range of PPTs reviewed, prioritization is used in managing access to care across many healthcare settings other than emergency departments, such as elective surgeries, rehabilitation, and mental health services.
We listed 16 specific types of non-urgent healthcare services and we found that most of the criteria used are generic, such as the threat to the patient’s ability to play a role, functional limitations, pain, and probability of recovery. Two PPTs used only specific criteria related to the disease, i.e., varicose vein surgery [ 52 ] and orthodontic treatment [ 51 ]. We demonstrated that even prioritization tools used in specific health condition services included generic criteria to prioritize patients on waiting lists. These findings suggest that generic criteria, such as non-clinical or social factors, could be added to condition-specific criteria in PPTs to represent more fairly and precisely patients’ needs to receive healthcare services.
One of the limitations of this systematic review is that the search strategy was restricted to the English and French languages. The definition of PPT is neither precise nor systematic in the literature as some authors use other terms such as triage systems and priority scoring tools. This explains why our database search yielded a substantial number of references. However, we believe that our search strategy was rigorously conducted in order to include all relevant studies. Heterogeneity between healthcare services and settings in our review highlighted the wide variety of PPT uses, but it could limit the generalizability of the results. Considering the large number of studies and PPTs found in the literature, we presented our synthesis descriptively in order to define the current state of knowledge, but we did not intend to compare the tools reviewed to one another. Although we found that PPTs are broadly used in non-urgent healthcare services, we did not find any evidence about the prevalence of PPT use in current practice. We are aware that other PPTs may exist in very specific healthcare organizations, and that a grey literature search would surely benefit this review.
Long waiting times and other problems of access to healthcare services are important challenges that public healthcare systems face. Patient prioritization could help to manage access to care in an equitable manner. Development and validation processes are widely described for PPTs in non-urgent healthcare settings, mainly in the contexts of elective surgeries, but implementation into clinical practice seems to be a challenge. Although we were able to put forward some recommendations to support the development of reliable and valid PPTs for non-urgent services, we believe that more standardized projects need to be conducted and supported in order to evaluate facilitators and barriers to the implementation of such innovations. Further research is also needed to explore the outcomes of PPT use—other than their effects on waiting times—in clinical settings.
Supplementary information
Acknowledgements.
The authors would like to thank Marie Denise Lavoie for helping to design the search strategy for this review. They also want to thank Aora El Horani for language corrections of the text.
Abbreviations
Authors’ contributions.
JD conceptualized the study, analyzed data, and was the lead author of the manuscript. MEL, AR, AC, and FR contributed to concept development, protocol development, and manuscript writing. VB, AR, MEL, AC, ER, and ASA participated in data validation. All authors read and approved the final manuscript.
This study was funded by the program Recherche en Équipe of Fonds de recherche du Québec Nature et Technologie . JD received study grants from Centre interdisciplinaire de recherche en réadaptation et intégration sociale (CIRRIS) and from Fonds de recherche en réadaptation de l’Université Laval to conduct this research. FR and MEL are both Fonds de recherche du Québec Santé Research Scholar and MPG is the chairholder of The Canada Research Chair on Technologies and Practices in Health.
Availability of data and materials
Ethics approval and consent to participate.
Not applicable.
Consent for publication
Competing interests.
The authors declare that they have no competing interests.
1 Hip and knee joint replacement; cataract removal surgery; general surgery; children’s mental health services; and MRI scanning.
Publisher’s Note
Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Contributor Information
Julien Déry, Email: [email protected] .
Angel Ruiz, Email: [email protected] .
François Routhier, Email: [email protected] .
Valérie Bélanger, Email: [email protected] .
André Côté, Email: [email protected] .
Daoud Ait-Kadi, Email: [email protected] .
Marie-Pierre Gagnon, Email: [email protected] .
Simon Deslauriers, Email: [email protected] .
Ana Tereza Lopes Pecora, Email: [email protected] .
Eduardo Redondo, Email: [email protected] .
Anne-Sophie Allaire, Email: [email protected] .
Marie-Eve Lamontagne, Email: [email protected] .
Supplementary information accompanies this paper at 10.1186/s13643-020-01482-8.

____________________________________________________________
The information, including but not limited to, audio, video, text, and graphics contained on this website are for educational purposes only. No content on this website is intended to guide nursing practice and does not supersede any individual healthcare provider’s scope of practice or any nursing school curriculum. Additionally, no content on this website is intended to be a substitute for professional medical advice, diagnosis or treatment.
20 Secrets of Successful Nursing Students
Being a successful nursing student is more than just study tips and test strategies. It’s a way of life.
you may also like...

#337: An End to Case Study Confusion

#334: Use the ABCs and Maslow to Prioritize Nursing Care

Tips for Prioritizing with ABCs and Maslow

#331: Know These 12 Drugs Before Your First Clinical Day

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
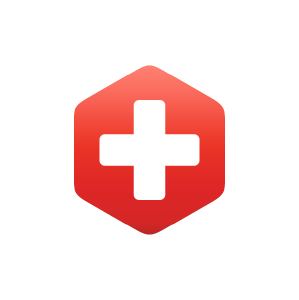
2.3: Tools for Prioritizing
- Last updated
- Save as PDF
- Page ID 63139
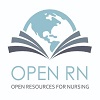
- Ernstmeyer & Christman (Eds.)
- Chippewa Valley Technical College via OpenRN
Prioritization of care for multiple patients while also performing daily nursing tasks can feel overwhelming in today’s fast-paced health care system. Because of the rapid and ever-changing conditions of patients and the structure of one’s workday, nurses must use organizational frameworks to prioritize actions and interventions. These frameworks can help ease anxiety, enhance personal organization and confidence, and ensure patient safety.
Acuity and intensity are foundational concepts for prioritizing nursing care and interventions. Acuity refers to the level of patient care that is required based on the severity of a patient’s illness or condition. For example, acuity may include characteristics such as unstable vital signs, oxygenation therapy, high-risk IV medications, multiple drainage devices, or uncontrolled pain. A “high-acuity” patient requires several nursing interventions and frequent nursing assessments.
Intensity addresses the time needed to complete nursing care and interventions such as providing assistance with activities of daily living (ADLs), performing wound care, or administering several medication passes. For example, a “high-intensity” patient generally requires frequent or long periods of psychosocial, educational, or hygiene care from nursing staff members. High-intensity patients may also have increased needs for safety monitoring, familial support, or other needs. [1]
Many health care organizations structure their staffing assignments based on acuity and intensity ratings to help provide equity in staff assignments. Acuity helps to ensure that nursing care is strategically divided among nursing staff. An equitable assignment of patients benefits both the nurse and patient by helping to ensure that patient care needs do not overwhelm individual staff and safe care is provided.
Organizations use a variety of systems when determining patient acuity with rating scales based on nursing care delivery, patient stability, and care needs. See an example of a patient acuity tool published in the American Nurse in Table 2.3. [2] In this example, ratings range from 1 to 4, with a rating of 1 indicating a relatively stable patient requiring minimal individualized nursing care and intervention. A rating of 2 reflects a patient with a moderate risk who may require more frequent intervention or assessment. A rating of 3 is attributed to a complex patient who requires frequent intervention and assessment. This patient might also be a new admission or someone who is confused and requires more direct observation. A rating of 4 reflects a high-risk patient. For example, this individual may be experiencing frequent changes in vital signs, may require complex interventions such as the administration of blood transfusions, or may be experiencing significant uncontrolled pain. An individual with a rating of 4 requires more direct nursing care and intervention than a patient with a rating of 1 or 2. [3]
Read more about using a patient acuity tool on a medical-surgical unit.
Rating scales may vary among institutions, but the principles of the rating system remain the same. Organizations include various patient care elements when constructing their staffing plans for each unit. Read more information about staffing models and acuity in the following box.
Staffing Models and Acuity
Organizations that base staffing on acuity systems attempt to evenly staff patient assignments according to their acuity ratings. This means that when comparing patient assignments across nurses on a unit, similar acuity team scores should be seen with the goal of achieving equitable and safe division of workload across the nursing team. For example, one nurse should not have a total acuity score of 6 for their patient assignments while another nurse has a score of 15. If this situation occurred, the variation in scoring reflects a discrepancy in workload balance and would likely be perceived by nursing peers as unfair. Using acuity-rating staffing models is helpful to reflect the individualized nursing care required by different patients.
Alternatively, nurse staffing models may be determined by staffing ratio. Ratio-based staffing models are more straightforward in nature, where each nurse is assigned care for a set number of patients during their shift. Ratio-based staffing models may be useful for administrators creating budget requests based on the number of staff required for patient care, but can lead to an inequitable division of work across the nursing team when patient acuity is not considered. Increasingly complex patients require more time and interventions than others, so a blend of both ratio and acuity-based staffing is helpful when determining staffing assignments. [5]
As a practicing nurse, you will be oriented to the elements of acuity ratings within your health care organization, but it is also important to understand how you can use these acuity ratings for your own prioritization and task delineation. Let’s consider the Scenario B in the following box to better understand how acuity ratings can be useful for prioritizing nursing care.
You report to work at 6 a.m. for your nursing shift on a busy medical-surgical unit. Prior to receiving the handoff report from your night shift nursing colleagues, you review the unit staffing grid and see that you have been assigned to four patients to start your day. The patients have the following acuity ratings:
Patient A: 45-year-old patient with paraplegia admitted for an infected sacral wound, with an acuity rating of 4.
Patient B: 87-year-old patient with pneumonia with a low grade fever of 99.7 F and receiving oxygen at 2 L/minute via nasal cannula, with an acuity rating of 2.
Patient C: 63-year-old patient who is postoperative Day 1 from a right total hip replacement and is receiving pain management via a PCA pump, with an acuity rating of 2.
Patient D: 83-year-old patient admitted with a UTI who is finishing an IV antibiotic cycle and will be discharged home today, with an acuity rating of 1.
Based on the acuity rating system, your patient assignment load receives an overall acuity score of 9. Consider how you might use their acuity ratings to help you prioritize your care. Based on what is known about the patients related to their acuity rating, whom might you identify as your care priority? Although this can feel like a challenging question to answer because of the many unknown elements in the situation using acuity numbers alone, Patient A with an acuity rating of 4 would be identified as the care priority requiring assessment early in your shift.
Although acuity can a useful tool for determining care priorities, it is important to recognize the limitations of this tool and consider how other patient needs impact prioritization.
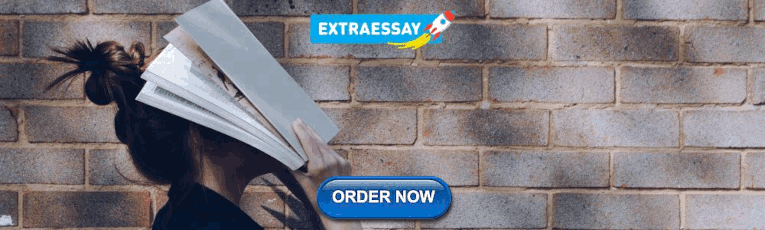
Maslow’s Hierarchy of Needs
When thinking back to your first nursing or psychology course, you may recall a historical theory of human motivation based on various levels of human needs called Maslow’s Hierarchy of Needs. Maslow’s Hierarchy of Needs reflects foundational human needs with progressive steps moving towards higher levels of achievement. This hierarchy of needs is traditionally represented as a pyramid with the base of the pyramid serving as essential needs that must be addressed before one can progress to another area of need. [6] See Figure 2.1 [7] for an illustration of Maslow’s Hierarchy of Needs.
Maslow’s Hierarchy of Needs places physiological needs as the foundational base of the pyramid. [8] Physiological needs include oxygen, food, water, sex, sleep, homeostasis, and excretion. The second level of Maslow’s hierarchy reflects safety needs. Safety needs include elements that keep individuals safe from harm. Examples of safety needs in health care include fall precautions. The third level of Maslow’s hierarchy reflects emotional needs such as love and a sense of belonging. These needs are often reflected in an individual’s relationships with family members and friends. The top two levels of Maslow’s hierarchy include esteem and self-actualization. An example of addressing these needs in a health care setting is helping an individual build self-confidence in performing blood glucose checks that leads to improved self-management of their diabetes.
So how does Maslow’s theory impact prioritization? To better understand the application of Maslow’s theory to prioritization, consider Scenario C in the following box.
You are an emergency response nurse working at a local shelter in a community that has suffered a devastating hurricane. Many individuals have relocated to the shelter for safety in the aftermath of the hurricane. Much of the community is still without electricity and clean water, and many homes have been destroyed. You approach a young woman who has a laceration on her scalp that is bleeding through her gauze dressing. The woman is weeping as she describes the loss of her home stating, “I have lost everything! I just don’t know what I am going to do now. It has been a day since I have had water or anything to drink. I don’t know where my sister is, and I can’t reach any of my family to find out if they are okay!”
Despite this relatively brief interaction, this woman has shared with you a variety of needs. She has demonstrated a need for food, water, shelter, homeostasis, and family. As the nurse caring for her, it might be challenging to think about where to begin her care. These thoughts could be racing through your mind:
Should I begin to make phone calls to try and find her family? Maybe then she would be able to calm down.
Should I get her on the list for the homeless shelter so she wouldn’t have to worry about where she will sleep tonight?
She hasn’t eaten in awhile; I should probably find her something to eat.
All of these needs are important and should be addressed at some point, but Maslow’s hierarchy provides guidance on what needs must be addressed first. Use the foundational level of Maslow’s pyramid of physiological needs as the top priority for care. The woman is bleeding heavily from a head wound and has had limited fluid intake. As the nurse caring for this patient, it is important to immediately intervene to stop the bleeding and restore fluid volume. Stabilizing the patient by addressing her physiological needs is required before undertaking additional measures such as contacting her family. Imagine if instead you made phone calls to find the patient’s family and didn’t address the bleeding or dehydration – you might return to a severely hypovolemic patient who has deteriorated and may be near death. In this example, prioritizing emotional needs above physiological needs can lead to significant harm to the patient.
Although this is a relatively straightforward example, the principles behind the application of Maslow’s hierarchy are essential. Addressing physiological needs before progressing toward additional need categories concentrates efforts on the most vital elements to enhance patient well-being. Maslow’s hierarchy provides the nurse with a helpful framework for identifying and prioritizing critical patient care needs.
Airway, breathing, and circulation, otherwise known by the mnemonic “ABCs,” are another foundational element to assist the nurse in prioritization. Like Maslow’s hierarchy, using the ABCs to guide decision-making concentrates on the most critical needs for preserving human life. If a patient does not have a patent airway, is unable to breathe, or has inadequate circulation, very little of what else we do matters. The patient’s ABCs are reflected in Maslow’s foundational level of physiological needs and direct critical nursing actions and timely interventions. Let’s consider Scenario D in the following box regarding prioritization using the ABCs and the physiological base of Maslow’s hierarchy.
You are a nurse on a busy cardiac floor charting your morning assessments on a computer at the nurses’ station. Down the hall from where you are charting, two of your assigned patients are resting comfortably in Room 504 and Room 506. Suddenly, both call lights ring from the rooms, and you answer them via the intercom at the nurses’ station.
Room 504 has an 87-year-old male who has been admitted with heart failure, weakness, and confusion. He has a bed alarm for safety and has been ringing his call bell for assistance appropriately throughout the shift. He requires assistance to get out of bed to use the bathroom. He received his morning medications, which included a diuretic about 30 minutes previously, and now reports significant urge to void and needs assistance to the bathroom.
Room 506 has a 47-year-old woman who was hospitalized with new onset atrial fibrillation with rapid ventricular response. The patient underwent a cardioversion procedure yesterday that resulted in successful conversion of her heart back into normal sinus rhythm. She is reporting via the intercom that her “heart feels like it is doing that fluttering thing again” and she is having chest pain with breathlessness.
Based upon these two patient scenarios, it might be difficult to determine whom you should see first. Both patients are demonstrating needs in the foundational physiological level of Maslow’s hierarchy and require assistance. To prioritize between these patients’ physiological needs, the nurse can apply the principles of the ABCs to determine intervention. The patient in Room 506 reports both breathing and circulation issues, warning indicators that action is needed immediately. Although the patient in Room 504 also has an urgent physiological elimination need, it does not overtake the critical one experienced by the patient in Room 506. The nurse should immediately assess the patient in Room 506 while also calling for assistance from a team member to assist the patient in Room 504.
Prioritizing what should be done and when it can be done can be a challenging task when several patients all have physiological needs. Recently, there has been professional acknowledgement of the cognitive challenge for novice nurses in differentiating physiological needs. To expand on the principles of prioritizing using the ABCs, the CURE hierarchy has been introduced to help novice nurses better understand how to manage competing patient needs. The CURE hierarchy uses the acronym “CURE” to guide prioritization based on identifying the differences among Critical needs, Urgent needs, Routine needs, and Extras. [9]
“Critical” patient needs require immediate action. Examples of critical needs align with the ABCs and Maslow’s physiological needs, such as symptoms of respiratory distress, chest pain, and airway compromise. No matter the complexity of their shift, nurses can be assured that addressing patients’ critical needs is the correct prioritization of their time and energies.
After critical patient care needs have been addressed, nurses can then address “urgent” needs. Urgent needs are characterized as needs that cause patient discomfort or place the patient at a significant safety risk. [10]
The third part of the CURE hierarchy reflects “routine” patient needs. Routine patient needs can also be characterized as “typical daily nursing care” because the majority of a standard nursing shift is spent addressing routine patient needs. Examples of routine daily nursing care include actions such as administering medication and performing physical assessments. [11] Although a nurse’s typical shift in a hospital setting includes these routine patient needs, they do not supersede critical or urgent patient needs.
The final component of the CURE hierarchy is known as “extras.” Extras refer to activities performed in the care setting to facilitate patient comfort but are not essential. [12] Examples of extra activities include providing a massage for comfort or washing a patient’s hair. If a nurse has sufficient time to perform extra activities, they contribute to a patient’s feeling of satisfaction regarding their care, but these activities are not essential to achieve patient outcomes.
Let’s apply the CURE mnemonic to patient care in the following box.
If we return to Scenario D regarding patients in Room 504 and 506, we can see the patient in Room 504 is having urgent needs. He is experiencing a physiological need to urgently use the restroom and may also have safety concerns if he does not receive assistance and attempts to get up on his own because of weakness. He is on a bed alarm, which reflects safety considerations related to his potential to get out of bed without assistance. Despite these urgent indicators, the patient in Room 506 is experiencing a critical need and takes priority. Recall that critical needs require immediate nursing action to prevent patient deterioration. The patient in Room 506 with a rapid, fluttering heartbeat and shortness of breath has a critical need because without prompt assessment and intervention, their condition could rapidly decline and become fatal.
In addition to using the identified frameworks and tools to assist with priority setting, nurses must also look at their patients’ data cues to help them identify care priorities. Data cues are pieces of significant clinical information that direct the nurse toward a potential clinical concern or a change in condition. For example, have the patient’s vital signs worsened over the last few hours? Is there a new laboratory result that is concerning? Data cues are used in conjunction with prioritization frameworks to help the nurse holistically understand the patient’s current status and where nursing interventions should be directed. Common categories of data clues include acute versus chronic conditions, actual versus potential problems, unexpected versus expected conditions, information obtained from the review of a patient’s chart, and diagnostic information.
Acute Versus Chronic Conditions
A common data cue that nurses use to prioritize care is considering if a condition or symptom is acute or chronic. Acute conditions have a sudden and severe onset. These conditions occur due to a sudden illness or injury, and the body often has a significant response as it attempts to adapt. Chronic conditions have a slow onset and may gradually worsen over time. The difference between an acute versus a chronic condition relates to the body’s adaptation response. Individuals with chronic conditions often experience less symptom exacerbation because their body has had time to adjust to the illness or injury. Let’s consider an example of two patients admitted to the medical-surgical unit complaining of pain in Scenario E in the following box.
As part of your patient assignment on a medical-surgical unit, you are caring for two patients who both ring the call light and report pain at the start of the shift. Patient A was recently admitted with acute appendicitis, and Patient B was admitted for observation due to weakness. Not knowing any additional details about the patients’ conditions or current symptoms, which patient would receive priority in your assessment? Based on using the data cue of acute versus chronic conditions, Patient A with a diagnosis of acute appendicitis would receive top priority for assessment over a patient with chronic pain due to osteoarthritis. Patients experiencing acute pain require immediate nursing assessment and intervention because it can indicate a change in condition. Acute pain also elicits physiological effects related to the stress response, such as elevated heart rate, blood pressure, and respiratory rate, and should be addressed quickly.
Actual Versus Potential Problems
Nursing diagnoses and the nursing care plan have significant roles in directing prioritization when interpreting assessment data cues. Actual problems refer to a clinical problem that is actively occurring with the patient. A risk problem indicates the patient may potentially experience a problem but they do not have current signs or symptoms of the problem actively occurring.
Consider an example of prioritizing actual and potential problems in Scenario F in the following box.
A 74-year-old woman with a previous history of chronic obstructive pulmonary disease (COPD) is admitted to the hospital for pneumonia. She has generalized weakness, a weak cough, and crackles in the bases of her lungs. She is receiving IV antibiotics, fluids, and oxygen therapy. The patient can sit at the side of the bed and ambulate with the assistance of staff, although she requires significant encouragement to ambulate.
Nursing diagnoses are established for this patient as part of the care planning process. One nursing diagnosis for this patient is Ineffective Airway Clearance . This nursing diagnosis is an actual problem because the patient is currently exhibiting signs of poor airway clearance with an ineffective cough and crackles in the lungs. Nursing interventions related to this diagnosis include coughing and deep breathing, administering nebulizer treatment, and evaluating the effectiveness of oxygen therapy. The patient also has the nursing diagnosis Risk for Skin Breakdown based on her weakness and lack of motivation to ambulate. Nursing interventions related to this diagnosis include repositioning every two hours and assisting with ambulation twice daily.
The established nursing diagnoses provide cues for prioritizing care. For example, if the nurse enters the patient’s room and discovers the patient is experiencing increased shortness of breath, nursing interventions to improve the patient’s respiratory status receive top priority before attempting to get the patient to ambulate.
Although there may be times when risk problems may supersede actual problems, looking to the “actual” nursing problems can provide clues to assist with prioritization.
Unexpected Versus Expected Conditions
In a similar manner to using acute versus chronic conditions as a cue for prioritization, it is also important to consider if a client’s signs and symptoms are “expected” or “unexpected” based on their overall condition. Unexpected conditions are findings that are not likely to occur in the normal progression of an illness, disease, or injury. Expected conditions are findings that are likely to occur or are anticipated in the course of an illness, disease, or injury. Unexpected findings often require immediate action by the nurse.
Let’s apply this tool to the two patients previously discussed in Scenario E. As you recall, both Patient A (with acute appendicitis) and Patient B (with weakness and diagnosed with osteoarthritis) are reporting pain. Acute pain typically receives priority over chronic pain. But what if both patients are also reporting nausea and have an elevated temperature? Although these symptoms must be addressed in both patients, they are “expected” symptoms with acute appendicitis (and typically addressed in the treatment plan) but are “unexpected” for the patient with osteoarthritis. Critical thinking alerts you to the unexpected nature of these symptoms in Patient B, so they receive priority for assessment and nursing interventions.
Handoff Report/Chart Review
Additional data cues that are helpful in guiding prioritization come from information obtained during a handoff nursing report and review of the patient chart. These data cues can be used to establish a patient’s baseline status and prioritize new clinical concerns based on abnormal assessment findings. Let’s consider Scenario G in the following box based on cues from a handoff report and how it might be used to help prioritize nursing care.
Imagine you are receiving the following handoff report from the night shift nurse for a patient admitted to the medical-surgical unit with pneumonia:
At the beginning of my shift, the patient was on room air with an oxygen saturation of 93%. She had slight crackles in both bases of her posterior lungs. At 0530, the patient rang the call light to go to the bathroom. As I escorted her to the bathroom, she appeared slightly short of breath. Upon returning the patient to bed, I rechecked her vital signs and found her oxygen saturation at 88% on room air and respiratory rate of 20. I listened to her lung sounds and noticed more persistent crackles and coarseness than at bedtime. I placed the patient on 2 L/minute of oxygen via nasal cannula. Within 5 minutes, her oxygen saturation increased to 92%, and she reported increased ease in respiration.
Based on the handoff report, the night shift nurse provided substantial clinical evidence that the patient may be experiencing a change in condition. Although these changes could be attributed to lack of lung expansion that occurred while the patient was sleeping, there is enough information to indicate to the oncoming nurse that follow-up assessment and interventions should be prioritized for this patient because of potentially worsening respiratory status. In this manner, identifying data cues from a handoff report can assist with prioritization.
Now imagine the night shift nurse had not reported this information during the handoff report. Is there another method for identifying potential changes in patient condition? Many nurses develop a habit of reviewing their patients’ charts at the start of every shift to identify trends and “baselines” in patient condition. For example, a chart review reveals a patient’s heart rate on admission was 105 beats per minute. If the patient continues to have a heart rate in the low 100s, the nurse is not likely to be concerned if today’s vital signs reveal a heart rate in the low 100s. Conversely, if a patient’s heart rate on admission was in the 60s and has remained in the 60s throughout their hospitalization, but it is now in the 100s, this finding is an important cue requiring prioritized assessment and intervention.
Diagnostic Information
Diagnostic results are also important when prioritizing care. In fact, the National Patient Safety Goals from The Joint Commission include prompt reporting of important test results. New abnormal laboratory results are typically flagged in a patient’s chart or are reported directly by phone to the nurse by the laboratory as they become available. Newly reported abnormal results, such as elevated blood levels or changes on a chest X-ray, may indicate a patient’s change in condition and require additional interventions. For example, consider Scenario H in which you are the nurse providing care for five medical-surgical patients.
You completed morning assessments on your assigned five patients. Patient A previously underwent a total right knee replacement and will be discharged home today. You are about to enter Patient A’s room to begin discharge teaching when you receive a phone call from the laboratory department, reporting a critical hemoglobin of 6.9 gm/dL on Patient B. Rather than enter Patient A’s room to perform discharge teaching, you immediately reprioritize your care. You call the primary provider to report Patient B’s critical hemoglobin level and determine if additional intervention, such as a blood transfusion, is required.
- Oregon Health Authority. (2021, April 29). Hospital nurse staffing interpretive guidance on staffing for acuity & intensity . Public Health Division, Center for Health Protection. https://www.oregon.gov/oha/PH/PROVIDERPARTNERRESOURCES/HEALTHCAREPROVIDERSFACILITIES/HEALTHCAREHEALTHCAREREGULATIONQUALITYIMPROVEMENT/Documents/NSInterpretiveGuidanceAcuity.pdf ↵
- Ingram, A., & Powell, J. (2018). Patient acuity tool on a medical surgical unit. American Nurse . https://www.myamericannurse.com/patient-acuity-medical-surgical-unit/ ↵
- Kidd, M., Grove, K., Kaiser, M., Swoboda, B., & Taylor, A. (2014). A new patient-acuity tool promotes equitable nurse-patient assignments. American Nurse Today, 9 (3), 1-4. https://www.myamericannurse.com/a-new-patient-acuity-tool-promotes-equitable-nurse-patient-assignments / ↵
- Welton, J. M. (2017). Measuring patient acuity. JONA: The Journal of Nursing Administration, 47 (10), 471. https://doi.org/10.1097/nna.0000000000000516 ↵
- Maslow, A. H. (1943). A theory of human motivation. Psychological Review , 50 (4), 370–396. https://doi.org/10.1037/h0054346 ↵
- " Maslow's_hierarchy_of_needs.svg " by J. Finkelstein is licensed under CC BY-SA 3.0 ↵
- Stoyanov, S. (2017). An analysis of Abraham Maslow's A Theory of Human Motivation (1st ed.). Routledge. https://doi.org/10.4324/9781912282517 ↵
- Kohtz, C., Gowda, C., & Guede, P. (2017). Cognitive stacking: Strategies for the busy RN. Nursing2021, 47 (1), 18-20. https://doi.org/10.1097/01.nurse.0000510758.31326.92 ↵
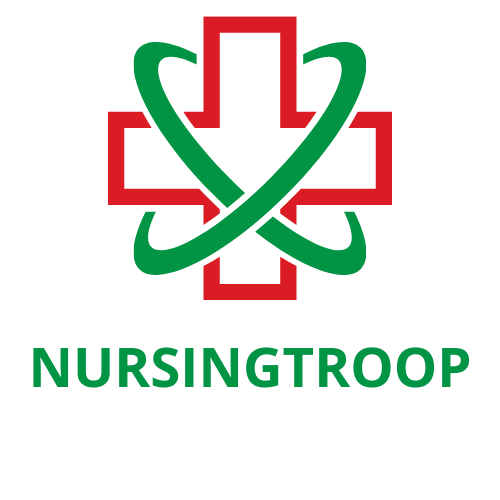
NursingTroop
Accessories for Nursing Students & Working Nurses.
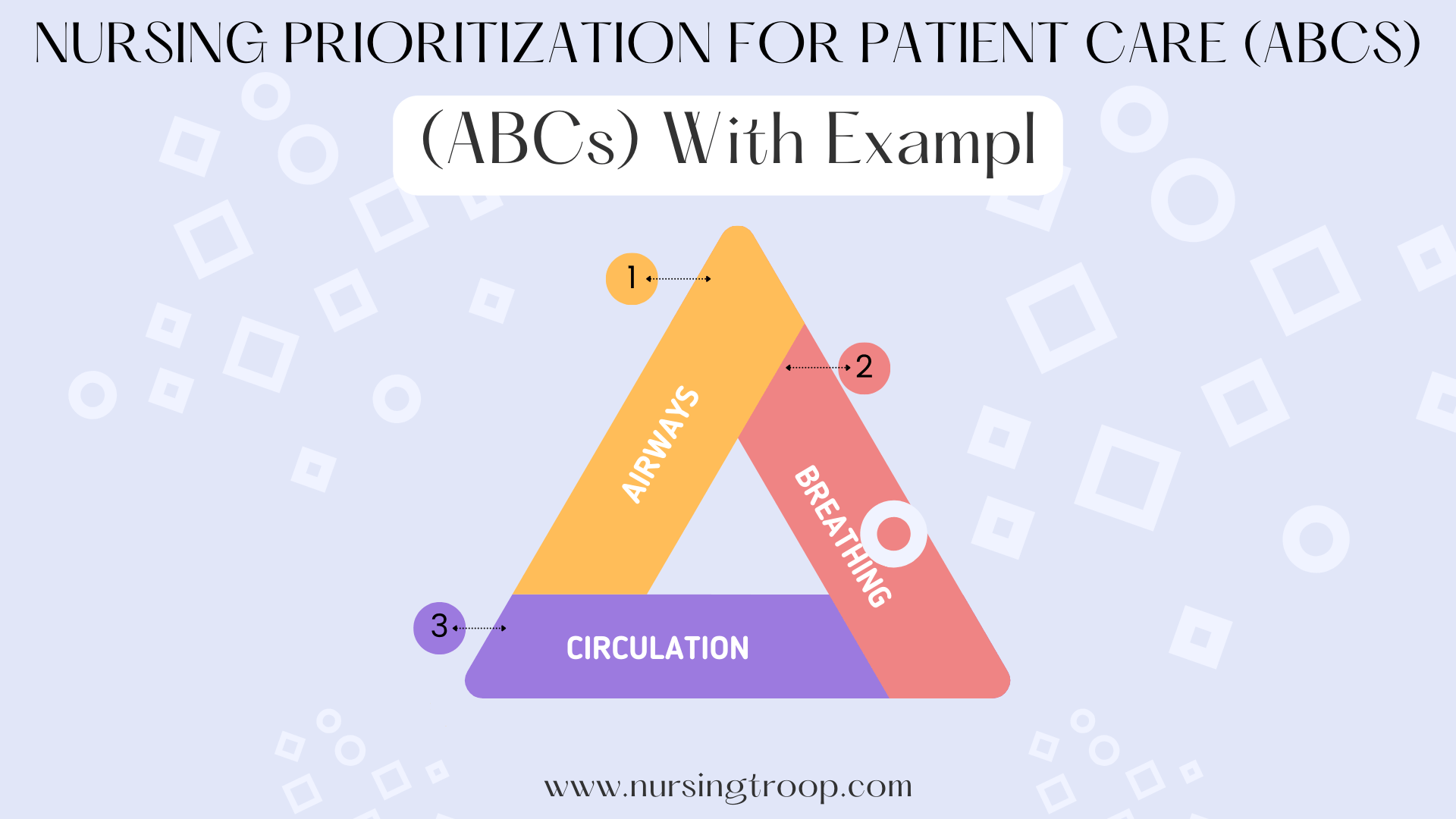
Nursing Prioritization for Patient Care (ABCs) with Examples
Are you a nurse who often feels overwhelmed by all your job’s competing tasks and demands? Do you ever need help prioritizing patient care when so much seems urgent? If so, then this blog post is for you! We all know that nurses play a vital role in providing quality health care, but learning how to manage their workload is an essential skill.
This blog will discuss the fundamental principles of nursing prioritization—including ABCs (Airway, Breathing, and Circulation) and reliability strategies—to help nurses better identify patients’ needs and balance their professional obligations. Through this post, we hope to provide resources to help build confident prioritization skills for any experience level. Read on for more information about the basics of nursing prioritization.
Table of Contents
Nursing Prioritization For Patient Care (ABCs)
The key to effective nursing prioritization is understanding the patient’s needs. Nurses must always be aware of how their patients are doing and ensure they are providing appropriate care. The ABCs are a simple way to remember the steps nurses should take when determining a patient’s condition:
- Airway: Is the patient’s airway free from obstruction? Are they able to breathe normally?
- Breathing: Is the patient breathing adequately? Are there any signs of distress or difficulty with respiration?
- Circulation: Is the patient’s heart rate and rhythm average? Are their blood pressure and oxygen saturation levels okay?
Using these factors, nurses can quickly assess the patient’s condition and prioritize their care accordingly.
Maslow’s Hierarchy of Needs
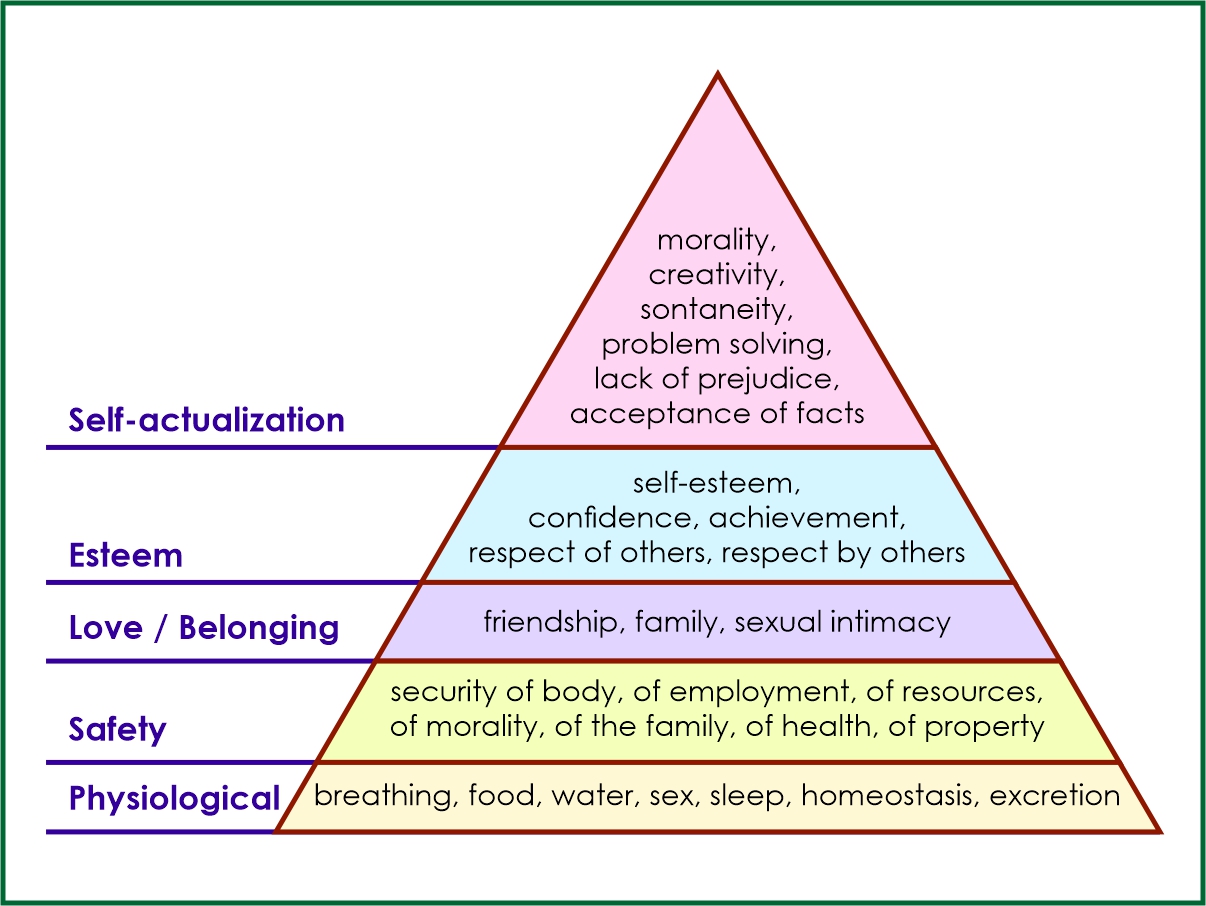
Current nurses are likely familiar with Maslow’s Hierarchy of Needs. Abraham Maslow established that humans have specific needs which must be met before progressing to other, higher needs. This concept is depicted in a pyramid, where foundational conditions at the bottom must be fulfilled before addressing more complex requirements at the top.
Maslow’s hierarchy is essential to keep in mind when prioritizing patient care. Nurses should prioritize treatments that meet the most basic physical and safety needs at the bottom of the pyramid. This includes ensuring proper airway, breathing, and circulation and providing a therapeutic environment for the patient. Once these basics are taken care of, nurses can move on to addressing more complex needs, such as emotional support or activities of daily living.
- Physiological needs
- Safety needs
- Love and belonging needs
- Esteem needs
- Self-actualization
Physiological needs –
This is the foundation of Maslow’s hierarchy and includes the most basic needs for survival, such as air, water, food, shelter, sleep, and reproduction. Nursing prioritization would include ensuring that a patient has a clear airway, adequate breathing, and proper circulation.
Safety needs –
The next level in Maslow’s hierarchy deals with the need for safety and security. This includes physical safety, emotional stability, financial security, and protection from harm. In terms of nursing prioritization, this would involve addressing any potential threats to a patient’s well-being, such as preventing falls or providing appropriate pain management.
Love and belonging needs –
Individuals need love, affection, and a sense of belonging at this level. This includes relationships with family, friends, and a community. In terms of nursing prioritization, this could involve providing emotional support or facilitating communication between the patient and their loved ones.
Esteem needs –
The fourth level in Maslow’s hierarchy deals with self-esteem, self-respect, and recognition from others. In terms of nursing prioritization, this could involve promoting patient autonomy and independence and acknowledging their accomplishments and contributions.
Self-actualization –
The top level of Maslow’s hierarchy is self-actualization, which involves reaching one’s full potential and achieving personal growth. Nursing prioritization could involve supporting a patient’s goals for recovery or providing resources to help them cope with their illness or condition.
Examples of Maslow’s hierarchy of needs
Aside from its psychological application, numerous disciplines have found Maslow’s model to be a valuable pedagogical tool. In education, professionals refer to the hierarchy when assessing challenges in students’ education or behavior, aiming to identify unmet needs.
Similarly, the hierarchy is utilized in certain business leadership theories as a guide to support employees and foster a healthy organization.
Advocates for race and social justice employ this hierarchy as a model to elucidate how fundamental disparities impede the upward mobility of underserved individuals.
“Experiencing food insecurity, inadequate housing, or being overworked does not inherently preclude us from experiencing moments of genuine happiness, contentment, and ease,” states the YWCA, a women’s rights organization, in an article on race, poverty, and well-being.
“However, these experiences create disadvantages in pursuing a well-lived life. They present barriers that must be overcome, challenges that must be faced, and instill worry regarding the possibility of our most basic needs going unmet, jeopardizing our primary aspiration: to live.”
Within this sphere, some individuals reframe Maslow’s hierarchy as one of rights or inequities to effectively illustrate how discrimination leads to the denial of these needs.
Why the hierarchy of needs is important
While Maslow’s hierarchy of needs is widely recognized and helpful, it is important to acknowledge that it represents just one perspective on human psychology and is not considered an absolute scientific truth. Critics of Maslow’s model argue that it may be too arbitrary and suggest that human needs exist on a more fluid spectrum.
Nevertheless, many experts still find the concept valuable for understanding personal well-being and exploring broader questions of human potential. “It encompasses both our deepest deficiencies and challenges, as well as our highest strengths,” explained psychologist Scott Barry Kaufman in an interview with mindbodygreen.
Writing for the Berkeley Well-Being Institute , Charlie Huntington highlighted that Maslow saw his hierarchy as part of a motivational theory. We can gain insights into our motivations by examining how our behaviors align or deviate from the hierarchy.
Prioritization without the ABCs
The ABCs are a great starting point for prioritizing care, but there are other considerations. In addition to the patient’s current condition, nurses must consider potential risks or threats that could affect their health and safety. This includes things like allergies, medication interactions, and other medical conditions.
Nurses should also keep in mind the importance of communication and documentation. Documenting all patient care activities is essential so other healthcare team members can stay informed and provide necessary follow-up care. Communication between nurses and physicians ensures patients receive the best possible care.
Reliability Strategies for Nursing Prioritization
In addition to Maslow’s Hierarchy of Needs, nurses can use several strategies to ensure reliable care. These include:
- Being aware of your limitations – nurses must recognize their strengths and weaknesses to manage their workload responsibly.
- Documenting all care given to the patient – documenting each step of care helps ensure the patient receives the best possible treatment.
- Utilizing evidence-based practices – utilizing research-backed methods can help nurses make more informed decisions about prioritizing patient care.
- Communicating effectively with other health professionals – nurses need to communicate clearly and effectively with other healthcare team members to ensure coordinated care.
- Asking questions when appropriate – nurses should never be afraid to ask questions if something seems unclear or uncertain; this could prevent mistakes from occurring later on.
- Being mindful of the patient’s needs – nurses should always be aware of the unique aspects of each patient’s situation and adjust their approach accordingly.
These strategies help nurses stay organized, on top of their tasks, and provide the best quality care for patients.
The Nursing Process
The nursing process is an essential tool for prioritizing care. This process includes five steps:
- Assessment – The first step of this process— assessment —involves collecting information about the patient’s condition to identify needs and problems.
- Diagnosis – During the diagnosis stage, nurses use the collected data to identify the root causes of the problem.
- Planning – The planning stage is then used to create a care plan to address the patient’s needs and goals.
- Implementation – In the implementation step, nurses begin carrying out the care plan.
- Evaluation – Finally, in evaluation , nurses assess how well their interventions have worked.
Family nurse practitioners utilize the nursing process to provide care for their patients. This includes assessing, diagnosing, planning, implementing, and evaluating treatments. For example, suppose a patient is prescribed pharmacologic therapy for an infection but does not experience symptom resolution after treatment. In that case, the family nurse practitioner will reassess the situation and potentially prescribe an alternative therapeutic regimen.
In certain states, nurse practitioners have the autonomy to prescribe medications independently. Elsewhere, they are pushing for full practice authority. In any case, the nursing process is used to structure and prioritize patient care.
The nursing process helps guide nurses in providing the most appropriate care for each patient. This process allows nurses to prioritize the right treatments and interventions for their patients’ needs.
Tools for Prioritizing Care in Nursing
In addition to the nursing process, several tools help nurses prioritize care. One example is the Nursing Prioritization Model (NPM), which allows nurses to identify and prioritize patient needs by assessing their condition. This model also considers the patient’s physical and psychological conditions to determine a course of action.
The NPM is based on Maslow’s hierarchy of needs, with each level representing a different set of patient needs. Using this model, nurses can determine how to address patients’ needs and provide the most effective care.
Another tool is the Nursing Task Prioritization System (NTPS), which helps nurses prioritize tasks related to patient care. The NTPS uses a numerical rating system from 0-10, with higher numbers indicating more essential tasks. This system allows nurses to quickly identify and prioritize the most pressing needs of their patients.
Finally, tools such as the Emergency Severity Index (ESI) and the Rapid Emergency Assessment of Needs (REAN) can help nurses determine a patient’s condition’s urgency or priority level. These tools use a rating system that allows nurses to prioritize care based on the acuity or complexity of the situation.
Professionals Standard for Prioritizing
Prioritizing tasks can be a challenging aspect of daily life. Whether it’s managing workload at your job, school assignments, or even personal to-do lists, it can be overwhelming to determine what needs to be done first. Finding a system that works best for you and helps you stay organized and productive.
This paragraph will discuss LEVEL PRIORITY and how it can help you prioritize your tasks effectively.
FIRST-LEVEL PRIORITY PROBLEMSAirway, Breathing, Circulation (ABCs)
ABC’s
The highest priority issues are health problems that immediately threaten life and demand urgent attention. These encompass critical matters related to the ABCs: airway, breathing, and circulation. Examples include securing an airway, providing respiratory support, and managing sudden perfusion and cardiac concerns.
SECOND-LEVEL PRIORITY PROBLEMSMAA-U-AR
“MAA U ARe 2nd”
Secondary-level issues encompass critical health conditions requiring prompt intervention to prevent further deterioration. Prioritization can be achieved by employing the MAA-U-AR method, which stands for mental status change, acute pain, acute impaired urinary elimination, untreated medical problems, abnormal diagnostic test results, and risks.
Mental Status Change
Brain and Delta-sign
Alterations in mental status signify broad alterations in brain function, encompassing confusion, amnesia, diminished alertness, impaired judgment or cognition, and more. Failure to address changes in mental status may result in harm, falls, or irreversible brain damage.
Acute-sign Pain-bolt
Acute pain may indicate the existence of actual or potential tissue damage, serving as an indication that something is amiss. Untreated pain can result in muscle tension, limited mobility, skin breakdown, or infection.
Acute Urinary Elimination Problems
Acute-sign Urine Retained in the Bladder
Acute urinary elimination problems may indicate obstructions in the bladder or urethra. Untreated urinary retention can lead to complications such as urinary tract infections, bladder damage, and chronic kidney failure.
Untreated Medical Problems
Unsolved Medical Problem-cube
Untreated medical conditions can result in rapid deterioration of a patient’s health or even mortality. A prime example is a diabetic patient who requires insulin; the absence of insulin may lead to the development of diabetic ketoacidosis.
Abnormal Laboratory Values
Abnormal Test-tubes
Abnormal laboratory values may indicate potentially significant issues and warrant further investigation. They could suggest underlying concerns related to the liver, heart, or kidneys.
Risk of Infection, Safety or Security
Infectious bacteria, Safety-pin and Security
It is crucial to address infections promptly to prevent further deterioration. Infections can result in inflammation, pain, impaired wound healing, sepsis, and even mortality. Safety and security concerns can also significantly heighten the risk of falls, injuries, and trauma.
THIRD-LEVEL PRIORITY PROBLEMSLong-Term Treatments
Long-ruler Treat
The third-level priorities encompass crucial aspects of patient health that can be addressed after addressing more critical health issues. Treating these problems requires long-term interventions, including addressing knowledge gaps, mobility limitations, family coping, activity levels, and rest.
What is an example of Prioritising in nursing?
For example, if a nurse cares for an elderly patient who has just been admitted to the hospital, they would first assess the patient’s condition and identify any immediate needs. The nurse’s priorities may be care based on Maslow’s hierarchy of needs and ensuring that the patient is provided with adequate food, water, and rest. Once the patient’s physiological needs have been met, the nurse can provide emotional and psychological support by talking to them and helping them feel safe and secure.
To determine a care plan, the nurse may assess potential medical issues, such as pain or other symptoms. The nurse can prioritize tasks related to this plan by utilizing the Nursing Task Prioritization System (NTPS). The nurse may also use the Emergency Severity Index (ESI) or Rapid Emergency Assessment of Needs (REAN) to determine if urgent care is needed.
Final Words
Patient prioritization is a critical component of nursing care. It helps nurses ensure that they provide the most appropriate care for their patients in the most efficient manner possible.
Nurses can effectively prioritize patient needs and provide necessary treatments by using tools such as the Nursing Process, Nursing Prioritization Model (NPM), and Nursing Task Prioritization System (NTPS). Utilizing these tools can help nurses provide the best possible care to their patients.
This content is written for educational purposes and does not constitute medical advice. If you have any questions, please consult a licensed healthcare professional.
References:
https://online.marymount.edu/blog/abcs-nursing-prioritization
https://edition.cnn.com/world/maslows-hierarchy-of-needs-explained-wellness-cec/index.html
https://simplypsychology.org/maslows.html
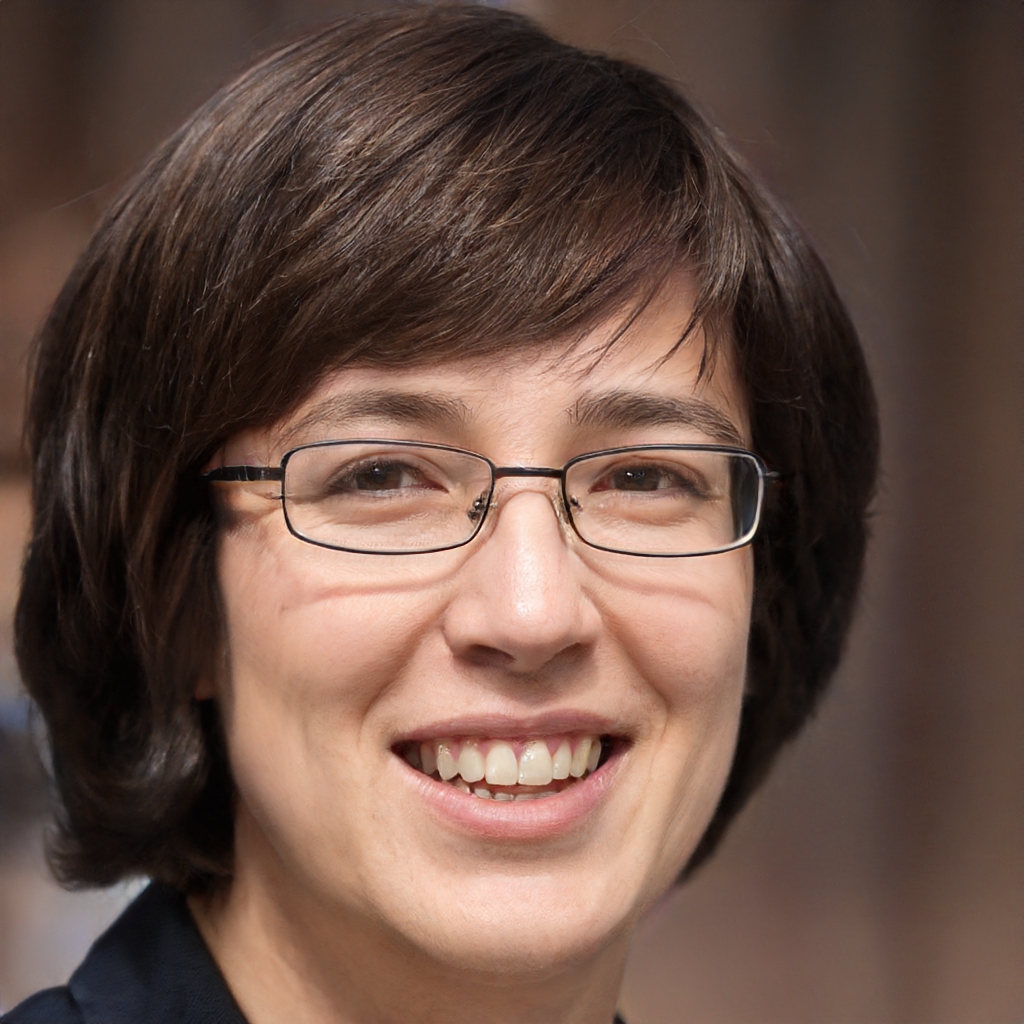
Mrs. Marie Brown has been a registered nurse for over 25 years. She began her nursing career at a Level I Trauma Center in downtown Chicago, Illinois. There she worked in the Emergency Department and on the Surgical Intensive Care Unit. After several years, she moved to the Midwest and continued her nursing career in a critical care setting. For the last 10 years of her nursing career, Mrs. Brown worked as a flight nurse with an air ambulance service. During this time, she cared for patients throughout the United States.
More Related Post
Leave a comment cancel reply.
Save my name, email, and website in this browser for the next time I comment.
- General Nursing
- Nursing Specialties
- Nursing Students
- United States Nursing
- World Nursing
- Boards of Nursing
- Breakroom / Clubs
- Nurse Q&A
- Student Q&A
- Fastest BSN
- Most Affordable BSN
- Fastest MSN
- Most Affordable MSN
- Best RN to BSN
- Fastest RN to BSN
- Most Affordable RN to BSN
- Best LPN/LVN
- Fastest LPN/LVN
- Most Affordable LPN/LVN
- Fastest DNP
- Most Affordable DNP
- Medical Assistant
- Best Online Medical Assistant
- Best Accelerated Medical Assistant
- Most Affordable Medical Assistant
- Nurse Practitioner
- Pediatric NP
- Neonatal NP
- Oncology NP
- Acute Care NP
- Aesthetic NP
- Women's Health NP
- Adult-Gerontology NP
- Emergency NP
- Best RN to NP
- Psychiatric-Mental Health NP
- RN Specialties
- Best RN Jobs and Salaries
- Aesthetic Nurse
- Nursing Informatics
- Nurse Case Manager
- Forensic Nurse
- Labor and Delivery Nurse
- Psychiatric Nurse
- Pediatric Nurse
- Travel Nurse
- Telemetry Nurse
- Dermatology Nurse
- Best NP Jobs and Salaries
- Family NP (FNP)
- Orthopedic NP
- Psychiatric-Mental Health NP (PMHNP)
- Nurse Educator
- Nurse Administrator
- Certified Nurse Midwife (CNM)
- Clinical Nurse Specialist (CNS)
- Certified Registered Nurse Anesthetist (CRNA)
- Best Free Online NCLEX-RN Study Guide
- The Nursing Process
- Question Leveling
- NCLEX-RN Question Identification
- Expert NCLEX-RN Test-Taking Strategies
- Best Scrubs for Nurses
- Best Shoes for Nurses
- Best Stethoscopes for Nurses
- Best Gifts for Nurses
- Undergraduate
- How to Become an LPN/LVN
- How to Earn an ADN
- Differences Between ADN, ASN, AAS
- How to Earn a BSN
- Best MSN Concentrations
- Is an MSN Worth It?
- How to Earn a DNP
- MSN vs. DNP
Next-Gen NCLEX-RN: Identifying Prioritization, Delegation, and Scope of Practice Questions
This section of allnurses' Next-Gen NCLEX-RN Study Guide focuses on questions relating to prioritization, delegation, and scope of practice. Resources
- Next Gen Nclex
- Table of Contents:
- Prioritization Questions
- Delegation Questions
- Scope of Practice Questions
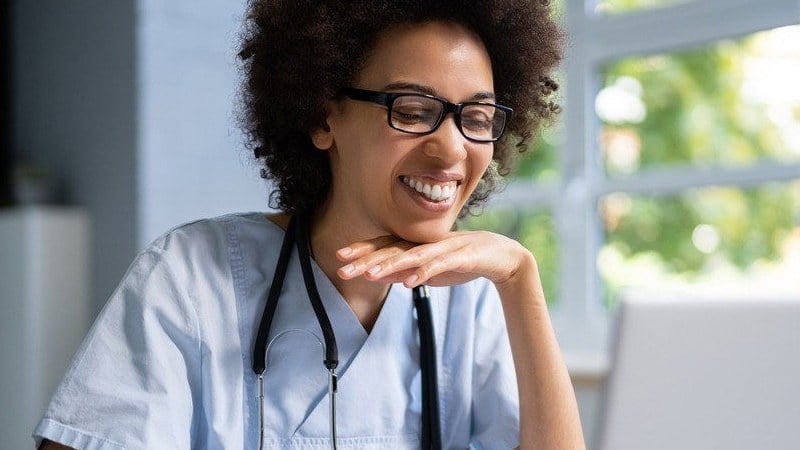
Prioritization , delegation , and scope of practice questions are some of the most difficult questions to answer for NCLEX candidates. These questions are plentiful on the exam and often challenge test-takers to make safe and sound decisions about the care they are providing. Since these questions ask candidates to make decisions, these are typically higher-level questions and require a great deal of concentration and understanding of the nursing concepts that guide nursing practice. To help you focus on what's most important, let's look at how each of these high-level nursing concepts requires a bit of strategy to stay focused on the bigger picture.
This article is part of a more extensive study guide for the Next-Gen NCLEX-RN:
- Best Free Online Next-Gen NCLEX-RN Study Guide
- The Nursing Process: Everything Next-Gen NCLEX-RN Test-Takers Need to Know
- Next-Gen NCLEX-RN Question Leveling: Recognition, Comprehension, Application, and Analysis
- Next-Gen NCLEX-RN Expert Test-Taking Strategies
Prioritization
When you see the words:
These indicate that the test question is focusing on the nursing concept of prioritization. This concept challenges candidates to understand the criteria requiring the nurse to shift priorities as they move through care delivery. To make it easier, the following principles will help set guidelines that should help make decisions and priorities.
Acute vs Chronic
The principle of acute versus chronic can be interpreted as all acute issues or problems always taking priority over chronic issues or problems. For example, a patient that is experiencing a sudden onset of shortness of breath will take priority over a patient that is short of breath due to a COPD exacerbation. The "sudden onset" is a key term that lets the test-taker know it is a new status change. If the question offers several acute issues as possible answer choices, then you must think about the issues separately and determine which one is more severe and requires immediate attention.
Actual vs Potential
The principle of actual versus potential can be interpreted as all actual issues or problems taking priority over potential issues or problems. For example, a patient that is complaining of pain will take priority over a patient that has right-sided weakness and is requesting to go to the commode. Of course, the candidate may consider the possibility of the patient with right-sided weakness falling if they attempt to get up to go to the commode on their own, but as it stands, it remains a potential problem and therefore does not take priority over the person in pain. It is important to always consider the fact that the test questions want the test-taker to focus on the immediate need that needs to be addressed at this moment. One of the biggest challenges for new grad nurses is thinking too deeply about the "what ifs," which can quickly get them to choose the incorrect answer.
Physical vs Psychosocial
The principle of physical versus psychosocial can be interpreted as all physical issues or problems taking priority over psychosocial issues or problems. For example, a patient that is complaining of chills takes priority over a patient that is complaining of pain. Both of these are actual problems; however, on the NCLEX, pain is considered a psychosocial issue. Chills could be related to infection and, therefore, the priority at this moment. Of all the physical needs that a patient may have, you can prioritize those by using Maslow's Hierarchy of Needs.
Physical needs include:
- Circulation
- Elimination
- Temperature
Psychosocial needs include:
- Emotional support
Unstable vs Stable
The principle of unstable versus stable is a little less cut and dry in regard to interpretation. The word unstable in itself is pretty vague and could involve many considerations and factors. To make it easier for candidates to determine if a patient is unstable or not, consider the following:
- Is there a sudden or new onset of a status change? If so, then the patient is unstable.
- Are there any actual issues that require the skill set of the nurse? If so, that patient is unstable.
- Is there a question about the outcome of the patient? If so, then the patient is unstable.
- Is the patient brand new or just returned from a procedure? If so, then the patient is unstable.
- Is the patient emotionally distraught, angry, or fearful? If so, the patient is unstable.
- Does the patient have abnormal vital signs that put them at risk for injury? If so, then the patient is unstable.
- Is the patient at risk of losing life or limb? If so, the patient is unstable.
Stable patients have predictable outcomes, have normal vital signs, are not demonstrating any actual issues or problems at the moment, and are within normal limits of the care plan .
Just remember that if a patient requires a great deal of nursing judgment and close assessment, then that patient can be considered unstable and requires the RN to tend to them first. When establishing priority, you can utilize all of the above principles to help you determine which answer choice best meets the criteria for the prioritization rules.
Nursing students often struggle with delegation simply because they do not get enough opportunities throughout their schooling to practice the art and science of safe and effective delegation. Despite having first-hand experience or not, if candidates understand the fundamental principles and guidelines of delegation, then they can correctly answer NCLEX questions surrounding this concept.
Rules of Delegation
Nursing delegation can begin anytime after the RN has assessed the patient, and after the patient's condition and needs have been considered. The RN will prioritize the patient's needs based on their condition and differentiate between nursing and non-nursing tasks.
Tasks such as obtaining a set of vital signs on a stable patient could be delegated to allow the RN to do other tasks that require the skill set of an RN.
The NCLEX is mainly concerned with the candidate's understanding of what the nurse should not delegate. The functions of the nurse that cannot be delegated include:
- Nursing Judgement
For RNs, this is limited to initial assessment, initial evaluation, and initial teaching. PNs are perfectly capable of doing ongoing assessments, reinforcing patient education, and evaluating the effectiveness of the care they are providing. However, they must follow the same principles as an RN and not delegate the functions of the nurse to a nursing assistant or unlicensed assistive personnel.
Another thing to consider when determining if it is safe to delegate is the person's ability to complete the task safely and whether or not the delegating nurse will be available to supervise and intervene if necessary. If the nurse is not available to assist in the event that there is a complication or unexpected outcome, then the nurse should not delegate the task as it would be considered to violate the rules of safe and effective delegation.
When In Doubt, Check It Out
Sometimes situations are difficult and require much discernment to determine if we are upholding the highest standards of patient safety and nursing practice. NCLEX questions can be very challenging and require candidates to carefully consider the best, safest, and most appropriate actions related to the situation provided. In many cases, this task is difficult because there may be a lot of questions or there isn't enough information to make a sound decision about what to do.
When nurses run into situations where they do not know what to do next, they rely on assessment to figure it out. So when test-takers are confused or uncertain of what to do next, they can rely on assessment to help guide them.
Let's take a look at how being unsure or not having enough information can guide a candidate into using assessment to select the correct answer:
A mother calls the clinic to report that her child has been nauseated and vomiting and that her child has type I diabetes. What should the nurse tell the mother?
- Give the child foods with simple sugars
- Give all medications as prescribed
- Check the blood glucose every three to four hours
- Give small, frequent meals
The correct answer is 3.
This question does not provide enough information for the candidate to make a safe and informed decision regarding the care of the patient. Several things could relate to nausea and vomiting that may or may not be related to diabetes. Therefore, the only answer that would help the nurse make a better, informed decision is to use assessment to obtain more data that can be analyzed.
Frequent blood sugar readings will help the nurse determine if the patient is stable enough to be treated at home or if they need to report to the nearest healthcare facility for treatment.
Assessment is always the first step in The Nursing Process and therefore becomes the default when nurses are unsure what to do next or if they require more information to make an informed decision.
Here is another example of how being unsure or not having enough information can guide a candidate into using assessment to select the correct answer:
The nurse responds to a Rapid Response. When the nurse arrives to assist in the rapid response, a nursing assistant tells the nurse that the patient started to complain of chest pain before they fell to the floor. Which of the following actions, if taken by the nurse, would be most appropriate?
- Help the nursing assistant move the patient from the floor to the bed
- Ask the nursing assistant what the patient was doing before the chest pain started
- Tell the nursing assistant to get supplies for supplemental oxygen therapy
- Ask the patient if they are okay
The correct answer is 4.
This question does not provide enough information for the candidate to make a safe and informed decision regarding the care of the patient. When nurses do not have enough information, they should always default to assessment. In this case, the nurse needs to assess the patient to determine what level of care they may need. Are they alert? Responsive? Do they look injured? Do they require emergent intervention? The nurse must first assess the patient before they can decide what to do next.
Scope of Practice
Nurses have a very special role within the healthcare team. Nurses spend the most time with their patients. They are with their patients during the most intimate of situations. Nurses are on the front lines of patient care delivery, patient satisfaction, patient safety, and patient outcomes.
The NCLEX challenges candidates to fully understand the scope of practice, including ability and limitations. Just as test-takers must be able to answer questions about The Nursing Process correctly, they must also be able to answer scope of practice questions successfully. RNs and PNs must be able to distinguish between the differences within their scope of practice.
Let's review the key differences between each scope and give examples of how the NCLEX may challenge this nursing concept.
Rules of Management (RNs)
RNs are considered to be "managers" of patient care. With this responsibility falling under the scope of practice for the RNs, candidates taking the NCLEX-RN must be well-versed in the rules that guide their practice. In addition to understanding concepts of nursing leadership, conflict resolution, customer service, and other administrative skills, the NCLEX wants RNs to understand the rules that help them make careful decisions about managing patient care.
Here are the rules of management for RN candidates:
- Always follow the rules of delegation (never delegate what you can E.A.T.). Remember that RNs must do all of the initial assessment, evaluation, and teaching.
- Nursing practice and decision-making must always stem from evidence-based practice and textbook nursing.
- Always follow the rules of Prioritization (ABCs, Maslow's, Actual vs. Potential, etc.)
- RNs are responsible for verifying, validating, and following up with all status changes, new patients, complaints, and unexpected outcomes.
- RNs must never leave their patients until they have been determined to be stable.
Here is an example of how the NCLEX could challenge the RN candidate to answer a question about the rules of management:
A 37-year-old patient is being admitted to the unit for a fracture of the right femur. Which of the following actions by the nurse is best?
- Tell the nursing assistant to obtain the vital signs while the RN obtains a health history from the patient
- Tell the PN to go obtain phone orders from the physician while the RN assesses the pedial pulses
- Tell the PN to stay with the client while the RN goes to get supplies to care for the patient
- Tell the nursing assistant to fill out the admission forms while the RN goes on a lunch break
The correct answer is 2.
This question challenges the candidate to be able to identify the correct action that falls within the scope of practice for the RN. Following the rules of management as a guide really helps test-takers make decisions about correct and incorrect answer choices. Answer choice 1 violates the rules of management of care because the RN is responsible for all initial assessments. Answer choice 3 violates the rules of management because the RN should never leave the patient until they are determined to be stable. Answer choice 4 violates the rules of management of care because the RN is responsible for documenting their findings during the admission assessment. Also, the RN should not leave the patient until they are determined to be stable.
Here is another example of how the NCLEX could challenge the RN candidate to answer a question about the rules of management:
A nurse is creating the unit assignment for the day. Which of the following clients would be appropriate to assign to an LPN?
- A 33-year-old patient who had an appendectomy yesterday and is scheduled to be discharged later today
- A 42-year-old patient who had a bowel resection two days ago and has a nasogastric tube inserted and set to intermittent suction
- A 68-year-old patient who has lower abdominal pain and is scheduled to have an exploratory endoscopy in the afternoon
- A 98-year-old patient who is on hospice and is demonstrating agonal breathing
This question challenges the candidate to identify the correct action that falls with-in the scope of practice for the RN—which, in this case, is appropriate delegation. Following the rules of management as a guide helps test-takers make decisions about correct and incorrect answer choices. Answer choice 1 violates the rules of management of care because the RN is responsible for all initial teaching. Discharge teaching is the responsibility of the RN. Answer choice 3 violates the rules of management of care because the RN is responsible for all initial teaching, assessment, and evaluation. Patients going for procedures should be managed by the RN for these reasons. Answer choice 4 violates the rules of management because the RN should keep patients with complex needs and/or who have unexpected outcomes. Although hospice patients demonstrating agonal breathing is expected in the stages of death and dying, each person's response to death can vary. These patients and their family members may require additional teaching, assessment, and evaluation to provide the best care possible.
Rules of Coordination (PNs)
PNs are considered to be the coordinators of patient care. With this responsibility falling under the scope of practice for the practical nurse, candidates taking the NCLEX-PN must be well-versed in the rules that guide their practice. In addition to understanding concepts of nursing leadership, conflict resolution, customer service, and other administrative skills, the NCLEX wants PNs to understand the rules that help them make careful decisions about coordinating patient care.
Here are the rules of management for PN candidates:
- Always follow the rules of delegation (never delegate what you can E.A.T.). This also applies to PNs, as they cannot delegate these functions to unlicensed assistive personnel. Remember that PNs can do ongoing assessments, evaluation, and teaching after the RN has completed the initial.
- Always follow the rules of prioritization (ABCs, Maslow's, Actual vs. Potential, etc.)
- PNs are responsible for reporting changes in status and unexpected outcomes to the RN so the RN can verify, validate, and follow up.
- PNs must never leave their patients until they have been determined to be stable or unless an RN comes in to take over.
Here is an example of how the NCLEX could challenge the PN candidate to answer a question about the rules of management:
The nurse is caring for a 22-year-old patient who has been admitted for a fracture of the right femur. The nursing assistant reports to the nurse that the patient is complaining of a headache. Which of the following actions by the nurse is best?
- Have the nursing assistant obtain vital signs while the nurse calls the physician
- The nurse obtains a full set of vital signs, assesses the patient, and reports the findings to the RN in charge
- The nurse tells the nursing assistant to stay with the patient while the nurse goes to get medication for the patient
- Have the nursing assistant go give a report to the RN in charge while the nurse goes on a lunch break.
This question challenges the candidate to identify the correct action that falls within the scope of practice for the PN. Following the rules of coordination as a guide helps test-takers make decisions about correct and incorrect answer choices. Answer choice 1 violates the rules of coordination of care because the PN is responsible for all initial assessments before reporting to the RN to validate their findings. Answer choice 3 violates the rules of coordination because the PN should never leave the patient until they are determined to be stable. Answer choice 4 violates the rules of coordination of care because the PN is responsible for reporting their findings to the RN so that the RN can assess the patient and be determined stable before the PN goes on a lunch break.
Here is another example of how the NCLEX could challenge the PN candidate to answer a question about the rules of management:
The nurse is caring for an 87-year-old patient admitted for failure to thrive. Which care tasks would be appropriate for the PN to delegate to an experienced nursing assistant?
- Have the nursing assistant ask the patient's family how much the patient has eaten in the past 24 hours.
- Ask the nursing assistant to look at the patient's skin for breakdown or open areas.
- Tell the nursing assistant to provide information to the patient and family regarding the use of the remote, call bell, and bed controls.
- Have the nursing assistant gather equipment that will be needed to care for the patient.
This question challenges the candidate to identify the correct action that falls within the scope of practice for the PN. Following the rules of coordination as a guide helps test-takers decide on correct and incorrect answer choices. Answer choice 1 violates the rules of coordination of care because the PN is responsible for all initial assessments before reporting to the RN to validate their findings. Answer choice 2 violates the rules of coordination because the PN cannot delegate the functions of assessment. Answer choice 3 violates the rules of coordination of care because the PN cannot delegate the function of teaching.
Therapeutic Communication
Both RNs and PNs are responsible for responding to others in a professional and therapeutic manner. Whether the nurse is speaking with patients, family members, visitors, colleagues, or other members of the healthcare team, they must follow the rules of therapeutic communication at all times. The NCLEX challenges candidates to understand these rules and often places distractors and traps in questions that challenge this nursing concept. To help candidates better understand how to answer therapeutic communication questions successfully, let's first list out the answer types that are always incorrect.
The following answer choice options are incorrect for therapeutic communication questions:
- Authoritative answers (telling the patient or others what to do because you know best)
- Asking "why?” (asking "why?” causes patients and others to become defensive)
- Focusing on self rather than the patient or other (it's not about the nurse, it's about the patient or other person)
- False reassurance (everything will be fine, you're going to be okay)
- Asking closed-ended questions (limits the opportunity for the patient or other to talk about what they want to)
None of the above answer types should even be considered as an option for the correct answer to therapeutic communication questions. These responses typically cause further emotional conflict and do not promote a positive outcome.
Documentation
All nursing students leave school understanding the importance of documentation. It serves a legal purpose, as well as serves as the primary method of communication for all members of the healthcare team. The NCLEX challenges candidates to understand this concept fully and often writes questions that ask test-takers to identify good or bad documentation samples.
Although this nursing concept is pretty straightforward, test anxiety or feelings of test fatigue can often be a barrier when candidates try to pull from their memory banks. To help, we've listed out the rules of "good" documentation to make it easier to recall when needed.
Here are the rules of "good" nursing documentation:
- Documentation must be objective
- Documentation must be specific
- Documentation must be descriptive
- Documentation must be measurable
Here is an example of how an NCLEX question can challenge the nurse regarding nursing documentation:
The nurse is caring for a 12-year-old patient brought to the emergency department by their parents with a fractured pelvic bone. When asked how the injury occurred, the parents state that the child fell down the stairs while chasing their family dog. Upon assessment, the nurse notes several large bruises and three large scratches on the child's inner thighs. How should the nurse document these findings?
- "Multiple marks on child's legs"
- "Three large purple-colored bruises and one large scratch noted on right inner thigh, and two large purple-colored bruises and two large scratches noted on left inner thigh"
- "Several large bruises and scratches caused by abuse"
- "Multiple bruises and scratches noted on bilateral inner thighs, caused by falling down the stairs"
This question challenges candidates to follow the rules of good documentation when choosing the correct answer. The only option that meets the criteria for the rules is answer choice 2 because it is objective, specific, descriptive, and measurable. That means that anyone else could read that documentation and validate these findings.
Answer choice 1 is not descriptive enough. Answer choice 3 is assuming that it was abuse, and nurses must remain objective. We do not know for sure whether it was caused by abuse or not, so one cannot document that in the chart. Answer choice 4 also assumes that the injuries were sustained from falling down the stairs, which the nurse cannot prove or disprove, so therefore, it should not be documented as a definitive reason.
Caring And Compassion
While providing safe and effective patient care, it is part of the nurse's role also to provide that care in a caring and compassionate manner. Although caring and compassion can come at varying degrees of personal investment, a fundamental rule must be followed at all times. On the NCLEX, this fundamental rule isn't so much about compassion as it is about caring. It is important that candidates understand that no matter how complex and overwhelming patient care can become with all the various care equipment, such as heart monitors, traction, wound vacs, IV pumps, chest tubes, central lines, surgical drains, etc.—always take care of the patient first.
Here is an example of how the NCLEX can take this fundamental rule and put it into a test question:
The nurse is caring for a 48-year-old patient in skeletal traction who sustained a fractured femur from a car accident. The patient reports to the nurse that they have terrible pain in the affected extremity. Which action by the nurse is best?
- Check that the weights are in line and hanging free
- Check that the traction sling is correctly positioned
- Ask the client to describe the location and characteristics of their pain
- Ask the nursing assistant to reposition the client to ease their pain
The principle of caring for the patient first can be related to the first step of The Nursing Process and the statement, "When in doubt, check it out." When a patient states that they are having pain, difficulty breathing, dizziness, or provides any other subjective symptom, it is the responsibility of the nurse to assess further so that they can address the patient's complaints and immediately begin working towards meeting the patient's needs. Answer 1 is incorrect because the equipment may or may not be causing the pain, so asking the patient is more important. Answer 2 is incorrect for the same reason as answer 1. Answer 4 is incorrect because the nurse has not yet determined if the patient is stable and therefore should not delegate tasks to unstable patients.
When thinking about caring for patients, always remember to "Put the needs of your patients second to the needs of your own, and in all other possible circumstances, take care of your patients first,” according to Damion Keith Jenkins, RN, MSN.
Teaching And Learning Considerations
Since much of what nurses do is teaching and counseling patients, families, communities, students, and staff, the NCLEX challenges candidates' understanding of teaching and learning principles.
The first way to ensure that a nurse will teach effectively is to be confident in speaking to the content. Many of the NCLEX questions surrounding this nursing concept are written in a way that asks test-takers to identify correct or incorrect information regarding a particular topic. This type of question helps to determine if a candidate is competent in determining if teaching is effective or if further teaching is necessary.
Here is an example of how the NCLEX can write questions that challenge candidates to understand teaching and learning principles:
The nurse is caring for a patient admitted to the labor suite for premature contractions. The treatment was successful, and the patient is scheduled to be discharged later today. The nurse provides discharge teaching regarding a new prescription for terbutaline. The nurse understands that teaching was effective if the patient states which of the following?
- I can be certain that I will not have my baby prematurely while on this medication
- I will take this medication every day unless I am feeling really tired
- I will remain on bedrest so this medication can work
- I may feel mild muscle tremors while on this medication
This question challenges the candidate to know the correct information regarding the medication terbutaline. If the candidate is unfamiliar with this medication, then it is possible that they may answer this question incorrectly. The best way to approach these questions is to carefully look at each answer choice for clues. Answer choice 1 is incorrect because it includes the absolute term - "certain." We cannot be certain of anything in healthcare as each person responds differently to treatment. This is similar to giving false reassurance, which we cannot do. Answer choice 2 is incorrect because the medication should be taken all the time unless the doctor says otherwise. Answer choice 3 is incorrect because bedrest does not affect whether or not medication will be effective. Additionally, this patient is being discharged home, which rarely includes mandatory bed rest.
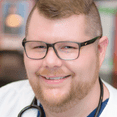
About Damion Jenkins, MSN, RN
Damion Jenkins has 14 years experience as a MSN, RN and specializes in NCLEX Prep Expert - 100% Pass Rate!.
More Like This
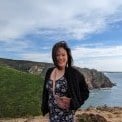
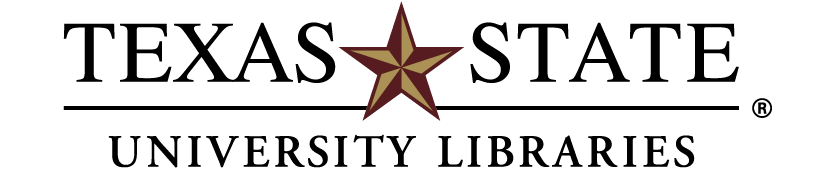
Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
2.3 Tools for Prioritizing
Prioritization of care for multiple patients while also performing daily nursing tasks can feel overwhelming in today’s fast-paced health care system. Because of the rapid and ever-changing conditions of patients and the structure of one’s workday, nurses must use organizational frameworks to prioritize actions and interventions. These frameworks can help ease anxiety, enhance personal organization and confidence, and ensure patient safety.
Acuity and intensity are foundational concepts for prioritizing nursing care and interventions. Acuity refers to the level of patient care that is required based on the severity of a patient’s illness or condition. For example, acuity may include characteristics such as unstable vital signs, oxygenation therapy, high-risk IV medications, multiple drainage devices, or uncontrolled pain. A “high-acuity” patient requires several nursing interventions and frequent nursing assessments.
Intensity addresses the time needed to complete nursing care and interventions such as providing assistance with activities of daily living (ADLs), performing wound care, or administering several medication passes. For example, a “high-intensity” patient generally requires frequent or long periods of psychosocial, educational, or hygiene care from nursing staff members. High-intensity patients may also have increased needs for safety monitoring, familial support, or other needs. [1]
Many health care organizations structure their staffing assignments based on acuity and intensity ratings to help provide equity in staff assignments. Acuity helps to ensure that nursing care is strategically divided among nursing staff. An equitable assignment of patients benefits both the nurse and patient by helping to ensure that patient care needs do not overwhelm individual staff and safe care is provided.
Organizations use a variety of systems when determining patient acuity with rating scales based on nursing care delivery, patient stability, and care needs. See an example of a patient acuity tool published in the American Nurse in Table 2.3. [2] In this example, ratings range from 1 to 4, with a rating of 1 indicating a relatively stable patient requiring minimal individualized nursing care and intervention. A rating of 2 reflects a patient with a moderate risk who may require more frequent intervention or assessment. A rating of 3 is attributed to a complex patient who requires frequent intervention and assessment. This patient might also be a new admission or someone who is confused and requires more direct observation. A rating of 4 reflects a high-risk patient. For example, this individual may be experiencing frequent changes in vital signs, may require complex interventions such as the administration of blood transfusions, or may be experiencing significant uncontrolled pain. An individual with a rating of 4 requires more direct nursing care and intervention than a patient with a rating of 1 or 2. [3]
Table 2.3 Example of a Patient Acuity Tool [4]
Read more about using a patient acuity tool on a medical-surgical unit.
Rating scales may vary among institutions, but the principles of the rating system remain the same. Organizations include various patient care elements when constructing their staffing plans for each unit. Read more information about staffing models and acuity in the following box.
Staffing Models and Acuity
Organizations that base staffing on acuity systems attempt to evenly staff patient assignments according to their acuity ratings. This means that when comparing patient assignments across nurses on a unit, similar acuity team scores should be seen with the goal of achieving equitable and safe division of workload across the nursing team. For example, one nurse should not have a total acuity score of 6 for their patient assignments while another nurse has a score of 15. If this situation occurred, the variation in scoring reflects a discrepancy in workload balance and would likely be perceived by nursing peers as unfair. Using acuity-rating staffing models is helpful to reflect the individualized nursing care required by different patients.
Alternatively, nurse staffing models may be determined by staffing ratio. Ratio-based staffing models are more straightforward in nature, where each nurse is assigned care for a set number of patients during their shift. Ratio-based staffing models may be useful for administrators creating budget requests based on the number of staff required for patient care, but can lead to an inequitable division of work across the nursing team when patient acuity is not considered. Increasingly complex patients require more time and interventions than others, so a blend of both ratio and acuity-based staffing is helpful when determining staffing assignments. [5]
As a practicing nurse, you will be oriented to the elements of acuity ratings within your health care organization, but it is also important to understand how you can use these acuity ratings for your own prioritization and task delineation. Let’s consider the Scenario B in the following box to better understand how acuity ratings can be useful for prioritizing nursing care.
You report to work at 6 a.m. for your nursing shift on a busy medical-surgical unit. Prior to receiving the handoff report from your night shift nursing colleagues, you review the unit staffing grid and see that you have been assigned to four patients to start your day. The patients have the following acuity ratings:
Patient A: 45-year-old patient with paraplegia admitted for an infected sacral wound, with an acuity rating of 4.
Patient B: 87-year-old patient with pneumonia with a low grade fever of 99.7 F and receiving oxygen at 2 L/minute via nasal cannula, with an acuity rating of 2.
Patient C: 63-year-old patient who is postoperative Day 1 from a right total hip replacement and is receiving pain management via a PCA pump, with an acuity rating of 2.
Patient D: 83-year-old patient admitted with a UTI who is finishing an IV antibiotic cycle and will be discharged home today, with an acuity rating of 1.
Based on the acuity rating system, your patient assignment load receives an overall acuity score of 9. Consider how you might use their acuity ratings to help you prioritize your care. Based on what is known about the patients related to their acuity rating, whom might you identify as your care priority? Although this can feel like a challenging question to answer because of the many unknown elements in the situation using acuity numbers alone, Patient A with an acuity rating of 4 would be identified as the care priority requiring assessment early in your shift.
Although acuity can a useful tool for determining care priorities, it is important to recognize the limitations of this tool and consider how other patient needs impact prioritization.
Maslow’s Hierarchy of Needs
When thinking back to your first nursing or psychology course, you may recall a historical theory of human motivation based on various levels of human needs called Maslow’s Hierarchy of Needs. Maslow’s Hierarchy of Needs reflects foundational human needs with progressive steps moving towards higher levels of achievement. This hierarchy of needs is traditionally represented as a pyramid with the base of the pyramid serving as essential needs that must be addressed before one can progress to another area of need. [6] See Figure 2.1 [7] for an illustration of Maslow’s Hierarchy of Needs.
Maslow’s Hierarchy of Needs places physiological needs as the foundational base of the pyramid. [8] Physiological needs include oxygen, food, water, sex, sleep, homeostasis, and excretion. The second level of Maslow’s hierarchy reflects safety needs. Safety needs include elements that keep individuals safe from harm. Examples of safety needs in health care include fall precautions. The third level of Maslow’s hierarchy reflects emotional needs such as love and a sense of belonging. These needs are often reflected in an individual’s relationships with family members and friends. The top two levels of Maslow’s hierarchy include esteem and self-actualization. An example of addressing these needs in a health care setting is helping an individual build self-confidence in performing blood glucose checks that leads to improved self-management of their diabetes.
So how does Maslow’s theory impact prioritization? To better understand the application of Maslow’s theory to prioritization, consider Scenario C in the following box.
You are an emergency response nurse working at a local shelter in a community that has suffered a devastating hurricane. Many individuals have relocated to the shelter for safety in the aftermath of the hurricane. Much of the community is still without electricity and clean water, and many homes have been destroyed. You approach a young woman who has a laceration on her scalp that is bleeding through her gauze dressing. The woman is weeping as she describes the loss of her home stating, “I have lost everything! I just don’t know what I am going to do now. It has been a day since I have had water or anything to drink. I don’t know where my sister is, and I can’t reach any of my family to find out if they are okay!”
Despite this relatively brief interaction, this woman has shared with you a variety of needs. She has demonstrated a need for food, water, shelter, homeostasis, and family. As the nurse caring for her, it might be challenging to think about where to begin her care. These thoughts could be racing through your mind:
Should I begin to make phone calls to try and find her family? Maybe then she would be able to calm down.
Should I get her on the list for the homeless shelter so she wouldn’t have to worry about where she will sleep tonight?
She hasn’t eaten in awhile; I should probably find her something to eat.
All of these needs are important and should be addressed at some point, but Maslow’s hierarchy provides guidance on what needs must be addressed first. Use the foundational level of Maslow’s pyramid of physiological needs as the top priority for care. The woman is bleeding heavily from a head wound and has had limited fluid intake. As the nurse caring for this patient, it is important to immediately intervene to stop the bleeding and restore fluid volume. Stabilizing the patient by addressing her physiological needs is required before undertaking additional measures such as contacting her family. Imagine if instead you made phone calls to find the patient’s family and didn’t address the bleeding or dehydration – you might return to a severely hypovolemic patient who has deteriorated and may be near death. In this example, prioritizing emotional needs above physiological needs can lead to significant harm to the patient.
Although this is a relatively straightforward example, the principles behind the application of Maslow’s hierarchy are essential. Addressing physiological needs before progressing toward additional need categories concentrates efforts on the most vital elements to enhance patient well-being. Maslow’s hierarchy provides the nurse with a helpful framework for identifying and prioritizing critical patient care needs.
Airway, breathing, and circulation, otherwise known by the mnemonic “ABCs,” are another foundational element to assist the nurse in prioritization. Like Maslow’s hierarchy, using the ABCs to guide decision-making concentrates on the most critical needs for preserving human life. If a patient does not have a patent airway, is unable to breathe, or has inadequate circulation, very little of what else we do matters. The patient’s ABCs are reflected in Maslow’s foundational level of physiological needs and direct critical nursing actions and timely interventions. Let’s consider Scenario D in the following box regarding prioritization using the ABCs and the physiological base of Maslow’s hierarchy.
You are a nurse on a busy cardiac floor charting your morning assessments on a computer at the nurses’ station. Down the hall from where you are charting, two of your assigned patients are resting comfortably in Room 504 and Room 506. Suddenly, both call lights ring from the rooms, and you answer them via the intercom at the nurses’ station.
Room 504 has an 87-year-old male who has been admitted with heart failure, weakness, and confusion. He has a bed alarm for safety and has been ringing his call bell for assistance appropriately throughout the shift. He requires assistance to get out of bed to use the bathroom. He received his morning medications, which included a diuretic about 30 minutes previously, and now reports significant urge to void and needs assistance to the bathroom.
Room 506 has a 47-year-old woman who was hospitalized with new onset atrial fibrillation with rapid ventricular response. The patient underwent a cardioversion procedure yesterday that resulted in successful conversion of her heart back into normal sinus rhythm. She is reporting via the intercom that her “heart feels like it is doing that fluttering thing again” and she is having chest pain with breathlessness.
Based upon these two patient scenarios, it might be difficult to determine whom you should see first. Both patients are demonstrating needs in the foundational physiological level of Maslow’s hierarchy and require assistance. To prioritize between these patients’ physiological needs, the nurse can apply the principles of the ABCs to determine intervention. The patient in Room 506 reports both breathing and circulation issues, warning indicators that action is needed immediately. Although the patient in Room 504 also has an urgent physiological elimination need, it does not overtake the critical one experienced by the patient in Room 506. The nurse should immediately assess the patient in Room 506 while also calling for assistance from a team member to assist the patient in Room 504.
Prioritizing what should be done and when it can be done can be a challenging task when several patients all have physiological needs. Recently, there has been professional acknowledgement of the cognitive challenge for novice nurses in differentiating physiological needs. To expand on the principles of prioritizing using the ABCs, the CURE hierarchy has been introduced to help novice nurses better understand how to manage competing patient needs. The CURE hierarchy uses the acronym “CURE” to guide prioritization based on identifying the differences among Critical needs, Urgent needs, Routine needs, and Extras. [9]
“Critical” patient needs require immediate action. Examples of critical needs align with the ABCs and Maslow’s physiological needs, such as symptoms of respiratory distress, chest pain, and airway compromise. No matter the complexity of their shift, nurses can be assured that addressing patients’ critical needs is the correct prioritization of their time and energies.
After critical patient care needs have been addressed, nurses can then address “urgent” needs. Urgent needs are characterized as needs that cause patient discomfort or place the patient at a significant safety risk. [10]
The third part of the CURE hierarchy reflects “routine” patient needs. Routine patient needs can also be characterized as “typical daily nursing care” because the majority of a standard nursing shift is spent addressing routine patient needs. Examples of routine daily nursing care include actions such as administering medication and performing physical assessments. [11] Although a nurse’s typical shift in a hospital setting includes these routine patient needs, they do not supersede critical or urgent patient needs.
The final component of the CURE hierarchy is known as “extras.” Extras refer to activities performed in the care setting to facilitate patient comfort but are not essential. [12] Examples of extra activities include providing a massage for comfort or washing a patient’s hair. If a nurse has sufficient time to perform extra activities, they contribute to a patient’s feeling of satisfaction regarding their care, but these activities are not essential to achieve patient outcomes.
Let’s apply the CURE mnemonic to patient care in the following box.
If we return to Scenario D regarding patients in Room 504 and 506, we can see the patient in Room 504 is having urgent needs. He is experiencing a physiological need to urgently use the restroom and may also have safety concerns if he does not receive assistance and attempts to get up on his own because of weakness. He is on a bed alarm, which reflects safety considerations related to his potential to get out of bed without assistance. Despite these urgent indicators, the patient in Room 506 is experiencing a critical need and takes priority. Recall that critical needs require immediate nursing action to prevent patient deterioration. The patient in Room 506 with a rapid, fluttering heartbeat and shortness of breath has a critical need because without prompt assessment and intervention, their condition could rapidly decline and become fatal.
In addition to using the identified frameworks and tools to assist with priority setting, nurses must also look at their patients’ data cues to help them identify care priorities. Data cues are pieces of significant clinical information that direct the nurse toward a potential clinical concern or a change in condition. For example, have the patient’s vital signs worsened over the last few hours? Is there a new laboratory result that is concerning? Data cues are used in conjunction with prioritization frameworks to help the nurse holistically understand the patient’s current status and where nursing interventions should be directed. Common categories of data clues include acute versus chronic conditions, actual versus potential problems, unexpected versus expected conditions, information obtained from the review of a patient’s chart, and diagnostic information.
Acute Versus Chronic Conditions
A common data cue that nurses use to prioritize care is considering if a condition or symptom is acute or chronic. Acute conditions have a sudden and severe onset. These conditions occur due to a sudden illness or injury, and the body often has a significant response as it attempts to adapt. Chronic conditions have a slow onset and may gradually worsen over time. The difference between an acute versus a chronic condition relates to the body’s adaptation response. Individuals with chronic conditions often experience less symptom exacerbation because their body has had time to adjust to the illness or injury. Let’s consider an example of two patients admitted to the medical-surgical unit complaining of pain in Scenario E in the following box.
As part of your patient assignment on a medical-surgical unit, you are caring for two patients who both ring the call light and report pain at the start of the shift. Patient A was recently admitted with acute appendicitis, and Patient B was admitted for observation due to weakness. Not knowing any additional details about the patients’ conditions or current symptoms, which patient would receive priority in your assessment? Based on using the data cue of acute versus chronic conditions, Patient A with a diagnosis of acute appendicitis would receive top priority for assessment over a patient with chronic pain due to osteoarthritis. Patients experiencing acute pain require immediate nursing assessment and intervention because it can indicate a change in condition. Acute pain also elicits physiological effects related to the stress response, such as elevated heart rate, blood pressure, and respiratory rate, and should be addressed quickly.
Actual Versus Potential Problems
Nursing diagnoses and the nursing care plan have significant roles in directing prioritization when interpreting assessment data cues. Actual problems refer to a clinical problem that is actively occurring with the patient. A risk problem indicates the patient may potentially experience a problem but they do not have current signs or symptoms of the problem actively occurring.
Consider an example of prioritizing actual and potential problems in Scenario F in the following box.
A 74-year-old woman with a previous history of chronic obstructive pulmonary disease (COPD) is admitted to the hospital for pneumonia. She has generalized weakness, a weak cough, and crackles in the bases of her lungs. She is receiving IV antibiotics, fluids, and oxygen therapy. The patient can sit at the side of the bed and ambulate with the assistance of staff, although she requires significant encouragement to ambulate.
Nursing diagnoses are established for this patient as part of the care planning process. One nursing diagnosis for this patient is Ineffective Airway Clearance . This nursing diagnosis is an actual problem because the patient is currently exhibiting signs of poor airway clearance with an ineffective cough and crackles in the lungs. Nursing interventions related to this diagnosis include coughing and deep breathing, administering nebulizer treatment, and evaluating the effectiveness of oxygen therapy. The patient also has the nursing diagnosis Risk for Skin Breakdown based on her weakness and lack of motivation to ambulate. Nursing interventions related to this diagnosis include repositioning every two hours and assisting with ambulation twice daily.
The established nursing diagnoses provide cues for prioritizing care. For example, if the nurse enters the patient’s room and discovers the patient is experiencing increased shortness of breath, nursing interventions to improve the patient’s respiratory status receive top priority before attempting to get the patient to ambulate.
Although there may be times when risk problems may supersede actual problems, looking to the “actual” nursing problems can provide clues to assist with prioritization.
Unexpected Versus Expected Conditions
In a similar manner to using acute versus chronic conditions as a cue for prioritization, it is also important to consider if a client’s signs and symptoms are “expected” or “unexpected” based on their overall condition. Unexpected conditions are findings that are not likely to occur in the normal progression of an illness, disease, or injury. Expected conditions are findings that are likely to occur or are anticipated in the course of an illness, disease, or injury. Unexpected findings often require immediate action by the nurse.
Let’s apply this tool to the two patients previously discussed in Scenario E. As you recall, both Patient A (with acute appendicitis) and Patient B (with weakness and diagnosed with osteoarthritis) are reporting pain. Acute pain typically receives priority over chronic pain. But what if both patients are also reporting nausea and have an elevated temperature? Although these symptoms must be addressed in both patients, they are “expected” symptoms with acute appendicitis (and typically addressed in the treatment plan) but are “unexpected” for the patient with osteoarthritis. Critical thinking alerts you to the unexpected nature of these symptoms in Patient B, so they receive priority for assessment and nursing interventions.
Handoff Report/Chart Review
Additional data cues that are helpful in guiding prioritization come from information obtained during a handoff nursing report and review of the patient chart. These data cues can be used to establish a patient’s baseline status and prioritize new clinical concerns based on abnormal assessment findings. Let’s consider Scenario G in the following box based on cues from a handoff report and how it might be used to help prioritize nursing care.
Imagine you are receiving the following handoff report from the night shift nurse for a patient admitted to the medical-surgical unit with pneumonia:
At the beginning of my shift, the patient was on room air with an oxygen saturation of 93%. She had slight crackles in both bases of her posterior lungs. At 0530, the patient rang the call light to go to the bathroom. As I escorted her to the bathroom, she appeared slightly short of breath. Upon returning the patient to bed, I rechecked her vital signs and found her oxygen saturation at 88% on room air and respiratory rate of 20. I listened to her lung sounds and noticed more persistent crackles and coarseness than at bedtime. I placed the patient on 2 L/minute of oxygen via nasal cannula. Within 5 minutes, her oxygen saturation increased to 92%, and she reported increased ease in respiration.
Based on the handoff report, the night shift nurse provided substantial clinical evidence that the patient may be experiencing a change in condition. Although these changes could be attributed to lack of lung expansion that occurred while the patient was sleeping, there is enough information to indicate to the oncoming nurse that follow-up assessment and interventions should be prioritized for this patient because of potentially worsening respiratory status. In this manner, identifying data cues from a handoff report can assist with prioritization.
Now imagine the night shift nurse had not reported this information during the handoff report. Is there another method for identifying potential changes in patient condition? Many nurses develop a habit of reviewing their patients’ charts at the start of every shift to identify trends and “baselines” in patient condition. For example, a chart review reveals a patient’s heart rate on admission was 105 beats per minute. If the patient continues to have a heart rate in the low 100s, the nurse is not likely to be concerned if today’s vital signs reveal a heart rate in the low 100s. Conversely, if a patient’s heart rate on admission was in the 60s and has remained in the 60s throughout their hospitalization, but it is now in the 100s, this finding is an important cue requiring prioritized assessment and intervention.
Diagnostic Information
Diagnostic results are also important when prioritizing care. In fact, the National Patient Safety Goals from The Joint Commission include prompt reporting of important test results. New abnormal laboratory results are typically flagged in a patient’s chart or are reported directly by phone to the nurse by the laboratory as they become available. Newly reported abnormal results, such as elevated blood levels or changes on a chest X-ray, may indicate a patient’s change in condition and require additional interventions. For example, consider Scenario H in which you are the nurse providing care for five medical-surgical patients.
You completed morning assessments on your assigned five patients. Patient A previously underwent a total right knee replacement and will be discharged home today. You are about to enter Patient A’s room to begin discharge teaching when you receive a phone call from the laboratory department, reporting a critical hemoglobin of 6.9 gm/dL on Patient B. Rather than enter Patient A’s room to perform discharge teaching, you immediately reprioritize your care. You call the primary provider to report Patient B’s critical hemoglobin level and determine if additional intervention, such as a blood transfusion, is required.
- Oregon Health Authority. (2021, April 29). Hospital nurse staffing interpretive guidance on staffing for acuity & intensity . Public Health Division, Center for Health Protection. https://www.oregon.gov/oha/PH/PROVIDERPARTNERRESOURCES/HEALTHCAREPROVIDERSFACILITIES/HEALTHCAREHEALTHCAREREGULATIONQUALITYIMPROVEMENT/Documents/NSInterpretiveGuidanceAcuity.pdf ↵
- Ingram, A., & Powell, J. (2018). Patient acuity tool on a medical surgical unit. American Nurse . https://www.myamericannurse.com/patient-acuity-medical-surgical-unit/ ↵
- Kidd, M., Grove, K., Kaiser, M., Swoboda, B., & Taylor, A. (2014). A new patient-acuity tool promotes equitable nurse-patient assignments. American Nurse Today, 9 (3), 1-4. https://www.myamericannurse.com/a-new-patient-acuity-tool-promotes-equitable-nurse-patient-assignments / ↵
- Welton, J. M. (2017). Measuring patient acuity. JONA: The Journal of Nursing Administration, 47 (10), 471. https://doi.org/10.1097/nna.0000000000000516 ↵
- Maslow, A. H. (1943). A theory of human motivation. Psychological Review , 50 (4), 370–396. https://doi.org/10.1037/h0054346 ↵
- " Maslow's_hierarchy_of_needs.svg " by J. Finkelstein is licensed under CC BY-SA 3.0 ↵
- Stoyanov, S. (2017). An analysis of Abraham Maslow's A Theory of Human Motivation (1st ed.). Routledge. https://doi.org/10.4324/9781912282517 ↵
- Kohtz, C., Gowda, C., & Guede, P. (2017). Cognitive stacking: Strategies for the busy RN. Nursing2021, 47 (1), 18-20. https://doi.org/10.1097/01.nurse.0000510758.31326.92 ↵
Intentional causation of harmful or offensive contact with another's person without that person's consent.
An act of restraining another person causing that person to be confined in a bounded area. Restraints can be physical, verbal, or chemical.
The right of an individual to have personal, identifiable medical information kept private.
An act of making negative, malicious, and false remarks about another person to damage their reputation.
An act of deceiving an individual for personal gain.
The failure to exercise the ordinary care a reasonable person would use in similar circumstances.
A specific term used for negligence committed by a professional with a license.
The person bringing the lawsuit.
The parties named in a lawsuit.
Legal obligations nurses have to their patients to adhere to current standards of practice.
State law providing protections against negligence claims to individuals who render aid to people experiencing medical emergencies outside of clinical environments.
Leadership and Management of Nursing Care Copyright © 2022 by Kim Belcik and Open Resources for Nursing is licensed under a Creative Commons Attribution 4.0 International License , except where otherwise noted.
Share This Book
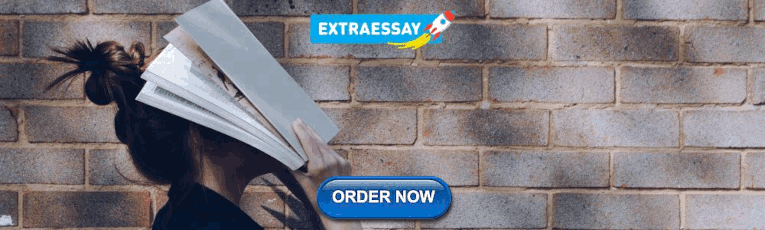
IMAGES
VIDEO
COMMENTS
Programming Assignments. Unless otherwise noted, all assignments are due at 11:59 pm Seattle time. Programming assignments will be posted here as they are released. P3 - It's Data Time! Initial Submission by Friday 12/09 at 11:59 pm. See Details. P2 - Prioritizing Patients. Initial Submission by Tuesday 11/15 at 11:59 pm.
• Programming Assignment 2 deadline extended ... Prioritizing Patients Lesson 12 - Spring 2023 5 onsider the specification where we determine a "priority score" for a patient. The specification asks you to use a formula that takes into consideration:
Step 1. Create a Patient class that implements the Comparable interface. This class will repres... In this week's assignment, you are to create a simple application which manages a priority queue of patients. Your application will use console input / output as shown in the example below. The system will give the user options to add patients to ...
To prioritizing queue, if it possible separate the Collection you want to process, so you must sort with simple sort algorithm e.g quicksort and pass the sort data to another collection e.q Queue, Stack. i think this method good to be in ER Class. edited Mar 18, 2013 at 4:12. answered Mar 18, 2013 at 3:44. Dion Dirza.
The health care system faces a significant challenge in balancing the ever-expanding task of meeting patient care needs with scarce nursing resources that has even worsened as a result of the COVID-19 pandemic. With a limited supply of registered nurses, nurse managers are often challenged to implement creative staffing practices such as sending staff to units where they do not normally work ...
Extras. This problem focuses on implementing a priority queue using a Vector and also implementing a priority queue using a singly-linked list. In the problem, you will implement a collection called a priority queue in two different ways: using a Stanford Library Vector and a linked-list. As an extension, you can also implement a priority queue ...
2.3 Tools for Prioritizing. Prioritization of care for multiple patients while also performing daily nursing tasks can feel overwhelming in today's fast-paced health care system. Because of the rapid and ever-changing conditions of patients and the structure of one's workday, nurses must use organizational frameworks to prioritize actions ...
Prioritization is a process of ranking patient needs for non-urgent services and as such, it involves a broader range of timeframes and patient types [2, 5]. Whereas triage sorts patients into broader categories (e.g., low/moderate/high priority or service/no service), it can nevertheless imply a prioritization process, as presented in our results.
Programming Assignment Unit 2: University of the People CS 2203 - Databases 1 Chinu Singla, Instructor June 25, 2023. 2. E-R diagram of the hospital software system, based on relations created for Programming Assignment in Unit 1. 1: Doctor vs Patient relationship: Doctor can have zero or many patient, whereas Patient can have one and only one ...
Prioritize nursing care based on patient acuity. Use principles of time management to organize work. Analyze effectiveness of time management strategies. Use critical thinking to prioritize nursing care for patients. Apply a framework for prioritization (e.g., Maslow, ABCs) "So much to do, so little time.". This is a common mantra of today ...
Without utilization of appropriate prioritization strategies, nurses can experience time scarcity, a feeling of racing against a clock that is continually working against them. Functioning under the burden of time scarcity can cause feelings of frustration, inadequacy, and eventually burnout. Time scarcity can also impact patient safety ...
Prioritizing like a pro. Looking at your list of real and potential problems, you're going to assign each item a rating. A: Things that need to be addressed now (if you don't, the patient will suffer serious harm) B: Things that need to be addressed soon (you definitely can't ignore these issues) C: Things that need to be addressed today ...
Acuity. Acuity and intensity are foundational concepts for prioritizing nursing care and interventions. Acuity refers to the level of patient care that is required based on the severity of a patient's illness or condition. For example, acuity may include characteristics such as unstable vital signs, oxygenation therapy, high-risk IV medications, multiple drainage devices, or uncontrolled pain.
The Nursing Process. The nursing process is an essential tool for prioritizing care. This process includes five steps: Assessment- The first step of this process—assessment—involves collecting information about the patient's condition to identify needs and problems. Diagnosis- During the diagnosis stage, nurses use the collected data ...
The RN will prioritize the patient's needs based on their condition and differentiate between nursing and non-nursing tasks. ... A nurse is creating the unit assignment for the day. Which of the following clients would be appropriate to assign to an LPN? ... so asking the patient is more important. Answer 2 is incorrect for the same reason as ...
Module 02 Assignment - Assigning, Delegating, Supervising, and Prioritizing Care assigning, delegating, supervising, and prioritizing care list of clients and. Skip to document. University; High School. Books; ... Describe the patients you will be assigning to the LPN 1. Client number 2: 46-year-old female with full-thickness burns to the leg ...
Study with Quizlet and memorize flashcards containing terms like The nursing instructor asks a nursing student to identify the priorities of care for an assigned client. Which statement indicates that the student correctly identifies the priority client needs? 1. actual or life-threatening concerns 2. completing care in a reasonable time frame 3. time constraints related to the client's needs ...
1. Introduction. The advent of patient-centered care summons healthcare organizations to focus patient treatment using individual patient preferences, needs and values as a guide [1, 2].Relationships form one of the essential grounding requirements: "… the concept, at its core, encapsulates healing relationships grounded in strong communication and trust" [2,p.1489].
Decide on the process. Now that you've gathered the information you need, you're ready to develop your plan for assigning nurses. This step usually combines the unit layout with your patient flow. Nurses typically use one of three processes—area, direct, or group—to make assignments. (See Choose your process.)
2.3 Tools for Prioritizing. Prioritization of care for multiple patients while also performing daily nursing tasks can feel overwhelming in today's fast-paced health care system. Because of the rapid and ever-changing conditions of patients and the structure of one's workday, nurses must use organizational frameworks to prioritize actions ...
Module 2 assignment-delegation, supervision and prioritizing. Course. Leadership and Professional identity (NUR 2832) 183 ... and ambulating patients. The RN will prioritize the care of the clients according to their needs. The RN will assess each client and determine the care that is needed. The RN will then delegate the care to the LPN/LVN ...