Presentation Model
Represent the state and behavior of the presentation independently of the GUI controls used in the interface
Also Known as: Application Model
19 July 2004
This is part of the Further Enterprise Application Architecture development writing that I was doing in the mid 2000’s. Sadly too many other things have claimed my attention since, so I haven’t had time to work on them further, nor do I see much time in the foreseeable future. As such this material is very much in draft form and I won’t be doing any corrections or updates until I’m able to find time to work on it again.
GUIs consist of widgets that contain the state of the GUI screen. Leaving the state of the GUI in widgets makes it harder to get at this state, since that involves manipulating widget APIs, and also encourages putting presentation behavior in the view class.
Presentation Model pulls the state and behavior of the view out into a model class that is part of the presentation. The Presentation Model coordinates with the domain layer and provides an interface to the view that minimizes decision making in the view. The view either stores all its state in the Presentation Model or synchronizes its state with Presentation Model frequently
Presentation Model may interact with several domain objects, but Presentation Model is not a GUI friendly facade to a specific domain object. Instead it is easier to consider Presentation Model as an abstract of the view that is not dependent on a specific GUI framework. While several views can utilize the same Presentation Model , each view should require only one Presentation Model . In the case of composition a Presentation Model may contain one or many child Presentation Model instances, but each child control will also have only one Presentation Model .
Presentation Model is known to users of Visual Works Smalltalk as Application Model
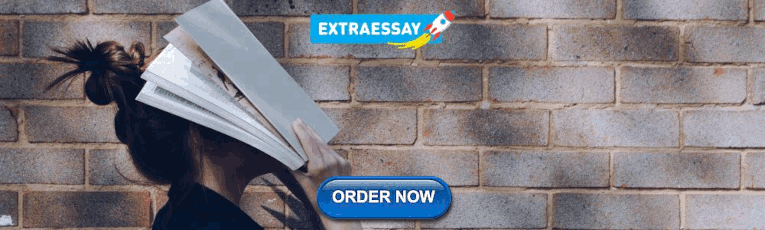
How it Works
The essence of a Presentation Model is of a fully self-contained class that represents all the data and behavior of the UI window, but without any of the controls used to render that UI on the screen. A view then simply projects the state of the presentation model onto the glass.
To do this the Presentation Model will have data fields for all the dynamic information of the view. This won't just include the contents of controls, but also things like whether or not they are enabled. In general the Presentation Model does not need to hold all of this control state (which would be lot) but any state that may change during the interaction of the user. So if a field is always enabled, there won't be extra data for its state in the Presentation Model .
Since the Presentation Model contains data that the view needs to display the controls you need to synchronize the Presentation Model with the view. This synchronization usually needs to be tighter than synchronization with the domain - screen synchronization is not sufficient, you'll need field or key synchronization.
To illustrate things a bit better, I'll use the aspect of the running example where the composer field is only enabled if the classical check box is checked.
Figure 1: Classes showing structure relevant to clicking the classical check box
Figure 2: How objects react to clicking the classical check box.
When someone clicks the classical check box the check box changes its state and then calls the appropriate event handler in the view. This event handler saves the state of the view to Presentation Model and then updates itself from the Presentation Model (I'm assuming a coarse-grained synchronization here.) The Presentation Model contains the logic that says that the composer field is only enabled if the check box is checked, so the when the view updates itself from the Presentation Model , the composer field control changes its enablement state. I've indicated on the diagram that the Presentation Model would typically have a property specifically to mark whether the composer field should be enabled. This will, of course, just return the value of the isClassical property in this case - but the separate property is important because that property encapsulates how the Presentation Model determines whether the composer field is enabled - clearly indicating that that decision is the responsibility of the Presentation Model .
This small example is illustrates the essence of the idea of the Presentation Model - all the decisions needed for presentation display are made by the Presentation Model leaving the view to be utterly simple.
Probably the most annoying part of Presentation Model is the synchronization between Presentation Model and view. It's simple code to write, but I always like to minimize this kind of boring repetitive code. Ideally some kind of framework could handle this, which I'm hoping will happen some day with technologies like .NET's data binding.
A particular decision you have to make with synchronization in Presentation Model is which class should contain the synchronization code. Often, this decision is largely based on the desired level of test coverage and the chosen implementation of Presentation Model . If you put the synchronization in the view, it won't get picked up by tests on the Presentation Model . If you put it in the Presentation Model you add a dependency to the view in the Presentation Model which means more coupling and stubbing. You could add a mapper between them, but adds yet more classes to coordinate. When making the decision of which implementation to use it is important to remember that although faults do occur in synchronization code, they are usually easy to spot and fix (unless you use fine-grained synchronization).
An important implementation detail of Presentation Model is whether the View should reference the Presentation Model or the Presentation Model should reference the View. Both implementations provide pros and cons.
A Presentation Model that references a view generally maintains the synchronization code in the Presentation Model . The resulting view is very dumb. The view contains setters for any state that is dynamic and raises events in response to user actions. The views implement interfaces allowing for easy stubbing when testing the Presentation Model . The Presentation Model will observe the view and respond to events by changing any appropriate state and reloading the entire view. As a result the synchronization code can be easily tested without needing the actual UI class.
A Presentation Model that is referenced by a view generally maintains the synchronization code in the view. Because the synchronization code is generally easy to write and easy to spot errors it is recommended that the testing occur on the Presentation Model and not the View. If you are compelled to write tests for the view this should be a clue that the view contains code that should belong in the Presentation Model . If you prefer to test the synchronization, a Presentation Model that references a view implementation is recommended.
When to Use It
Presentation Model is a pattern that pulls presentation behavior from a view. As such it's an alternative to to Supervising Controller and Passive View . It's useful for allowing you to test without the UI, support for some form of multiple view and a separation of concerns which may make it easier to develop the user interface.
Compared to Passive View and Supervising Controller , Presentation Model allows you to write logic that is completely independent of the views used for display. You also do not need to rely on the view to store state. The downside is that you need a synchronization mechanism between the presentation model and the view. This synchronization can be very simple, but it is required. Separated Presentation requires much less synchronization and Passive View doesn't need any at all.
Example: Running Example (View References PM) (C#)
Here's a version of the running example , developed in C# with Presentation Model .
Figure 3: The album window.
I'll start discussing the basic layout from the domain model outwards. Since the domain isn't the focus of this example, it's very uninteresting. It's essentially just a data set with a single table holding the data for an album. Here's the code for setting up a few test albums. I'm using a strongly typed data set.
The Presentation Model wraps this data set and provides properties to get at the data. There's a single instance of the Presentation Model for the whole table, corresponding to the single instance of the window. The Presentation Model has fields for the data set and also keeps track of which album is currently selected.
class PmodAlbum...
PmodAlbum provides properties to get at the data in the data set. Essentially I provide a property for each bit of information that the form needs to display. For those values which are just pulled directly out of the data set, this property is pretty simple.
The title of the window is based on the album title. I provide this through another property.
I have a property to see if the composer field should be enabled.
This is just a call to the public IsClassical property. You may wonder why the form doesn't just call this directly - but this is the essence of the encapsulation that the Presentation Model provides. PmodAlbum decides what the logic is for enabling that field, the fact that it's simply based on a property is known to the Presentation Model but not to the view.
The apply and cancel buttons should only be enabled if the data has changed. I can provide this by checking the state of that row of the dataset, since data sets record this information.
The list box in the view shows a list of the album titles. PmodAlbum provides this list.
So that covers the interface that PmodAlbum presents to the view. Next I'll look at how I do the synchronization between the view and the Presentation Model . I've put the synchronization methods in the view and am using coarse-grained synchronization. First I have a method to push the state of the view into the Presentation Model .
class FrmAlbum...
This method is very simple, just assigning the mutable parts of the view to the Presentation Model . The load method is a touch more complicated.
The complication here is avoiding a infinite recursion since synchronizing causes fields on the form to update which triggers synchronization.... I guard against that with a flag.
With these synchronization methods in place, the next step is just to call the right bit of synchronization in event handlers for the controls. Most of the time this easy, just call SyncWithPmod when data changes.
Some cases are more involved. When the user clicks on a new item in the list we need to navigate to a new album and show its data.
Notice that this method abandons any changes if you click on the list. I've done this awful bit of usability to keep the example simple, the form should really at least pop up a confirmation box to avoid losing the changes.
The apply and cancel buttons delegate what to do to the Presentation Model .
So although I can move most of the behavior to the Presentation Model , the view still retains some intelligence. For the testing aspect of Presentation Model to work better, it would be nice to move more. Certainly you can move more into the Presentation Model by moving the synchronization logic there, at the expense of having the Presentation Model know more about the view.
Example: Data Binding Table Example (C#)
As I first looked at Presentation Model in the .NET framework, it seemed that data binding provided excellent technology to make Presentation Model work simply. So far limitations in the current version of data binding holds it back from places that I'm sure it will eventually go. One area where data binding can work very well is with read-only data, so here is an example that shows this as well as how tables can fit in with a Presentation Model design.
Figure 4: A list of albums with the rock ones highlighted.
This is just a list of albums. The extra behavior is that each rock album should have it's row colored in cornsilk.
I'm using a slightly different data set to the other example. Here is the code for some test data.
The presentation model in this case reveals its internal data set as a property. This allows the form to data bind directly to the cells in the data set.
To support the highlighting, the presentation model provides an additional method that indexes into the table.
This method is similar to the ones in a simple example, the difference being that methods on table data need cell coordinates to pick out parts of the table. In this case all we need is a row number, but in general we may need row and column numbers.
From here on I can use the standard data binding facilities that come with visual studio. I can bind table cells easily to data in the data set, and also to data on the Presentation Model .
Getting the color to work is a little bit more involved. This is straying a little bit away from the main thrust of the example, but the whole thing gets its complication because there's no way to have row by row highlighting on the standard WinForms table control. In general the answer to this need is to buy a third party control, but I'm too cheap to do this. So for the curious here's what I did (the idea was mostly ripped off from http://www.syncfusion.com/FAQ/WinForms/). I'm going to assume you're familiar with the guts of WinForms from now on.
Essentially I made a subclass of DataGridTextBoxColumn which adds the color highlighting behavior. You link up the new behavior by passing in a delegate that handles the behavior.
class ColorableDataGridTextBoxColumn...
The constructor takes the original DataGridTextBoxColumn as well as the delegate. What I'd really like to do here is to use the decorator pattern to wrap the original but the original, like so many classes in WinForms, is all sealed up. So instead I copy over all the properties of the original into my subclass. This won't work is there are vital properties that can't be copied because you can't read or write to them. It seems to work here for now.
Fortunately the paint method is virtual (otherwise I would need a whole new data grid.) I can use it to insert the appropriate background color using the delegate.
To put this new table in place, I replace the columns of the data table in the page load after the controls have been built on the form.
class FrmAlbums...
It works, but I'll admit it's a lot more messy than I would like. If I were doing this for real, I'd want to look into a third party control. However I've seen this done in a production system and it worked just fine.
Application Architecture Guide - Chapter 10 - Presentation Layer Guidelines
Note - The patterns & practices Microsoft Application Architecture Guide, 2nd Edition is now live at http://msdn.microsoft.com/en-us/library/dd673617.aspx .
- J.D. Meier, Alex Homer, David Hill, Jason Taylor, Prashant Bansode, Lonnie Wall, Rob Boucher Jr, Akshay Bogawat
- 1 Objectives
- 3 Presentation Layer Components
- 5 Design Considerations
- 6 Presentation Layer Frame
- 8 Composition
- 9 Exception Management
- 12 Navigation
- 13 Presentation Entities
- 14 Request Processing
- 15 User Experience
- 16 UI Components
- 17 UI Process Components
- 18 Validation
- 19 Pattern Map
- 20 Pattern Descriptions
- 21.1 Mobile Applications
- 21.2 Rich Client Applications
- 21.3 Rich Internet Applications (RIA)
- 21.4 Web Applications
- 22 patterns & practices Solution Assets
- 23 Additional Resources
- Understand how the presentation layer fits into typical application architecture.
- Understand the components of the presentation layer.
- Learn the steps for designing the presentation layer.
- Learn the common issues faced while designing the presentation layer.
- Learn the key guidelines for designing the presentation layer.
- Learn the key patterns and technology considerations for designing the presentation layer.
The presentation layer contains the components that implement and display the user interface and manage user interaction. This layer includes controls for user input and display, in addition to components that organize user interaction. Figure 1 shows how the presentation layer fits into a common application architecture.
Figure 1 A typical application showing the presentation layer and the components it may contain
Presentation Layer Components
- User interface (UI) components . User interface components provide a way for users to interact with the application. They render and format data for users. They also acquire and validate data input by the user.
- User process components . User process components synchronize and orchestrate user interactions. Separate user process components may be useful if you have a complicated UI. Implementing common user interaction patterns as separate user process components allows you to reuse them in multiple UIs.
The following steps describe the process you should adopt when designing the presentation layer for your application. This approach will ensure that you consider all of the relevant factors as you develop your architecture:
- Identify your client type . Choose a client type that satisfies your requirements and adheres to the infrastructure and deployment constraints of your organization. For instance, if your users are on mobile devices and will be intermittently connected to the network, a mobile rich client is probably your best choice.
- Determine how you will present data . Choose the data format for your presentation layer and decide how you will present the data in your UI.
- Determine your data-validation strategy . Use data-validation techniques to protect your system from untrusted input.
- Determine your business logic strategy . Factor out your business logic to decouple it from your presentation layer code.
- Determine your strategy for communication with other layers . If your application has multiple layers, such as a data access layer and a business layer, determine a strategy for communication between your presentation layer and other layers.
Design Considerations
There are several key factors that you should consider when designing your presentation layer. Use the following principles to ensure that your design meets the requirements for your application, and follows best practices:
- Choose the appropriate UI technology. Determine if you will implement a rich (smart) client, a Web client, or a rich Internet application (RIA). Base your decision on application requirements, and on organizational and infrastructure constraints.
- Use the relevant patterns. Review the presentation layer patterns for proven solutions to common presentation problems.
- Design for separation of concerns. Use dedicated UI components that focus on rendering and display. Use dedicated presentation entities to manage the data required to present your views. Use dedicated UI process components to manage the processing of user interaction.
- Consider human interface guidelines. Review your organization’s guidelines for UI design. Review established UI guidelines based on the client type and technologies that you have chosen.
- Adhere to user-driven design principles. Before designing your presentation layer, understand your customer. Use surveys, usability studies, and interviews to determine the best presentation design to meet your customer’s requirements.
Presentation Layer Frame
There are several common issues that you must consider as your develop your design. These issues can be categorized into specific areas of the design. The following table lists the common issues for each category where mistakes are most often made.
Table 1 Presentation Layer Frame
Caching is one of the best mechanisms you can use to improve application performance and UI responsiveness. Use data caching to optimize data lookups and avoid network round trips. Cache the results of expensive or repetitive processes to avoid unnecessary duplicate processing.
Consider the following guidelines when designing your caching strategy:
- Do not cache volatile data.
- Consider using ready-to-use cache data when working with an in-memory cache. For example, use a specific object instead of caching raw database data.
- Do not cache sensitive data unless you encrypt it.
- If your application is deployed in Web farm, avoid using local caches that need to be synchronized; instead, consider using a transactional resource manager such as Microsoft SQL Server® or a product that supports distributed caching.
- Do not depend on data still being in your cache. It may have been removed.
Composition
Consider whether your application will be easier to develop and maintain if the presentation layer uses independent modules and views that are easily composed at run time. Composition patterns support the creation of views and the presentation layout at run time. These patterns also help to minimize code and library dependencies that would otherwise force recompilation and redeployment of a module when the dependencies change. Composition patterns help you to implement sharing, reuse, and replacement of presentation logic and views.
Consider the following guidelines when designing your composition strategy:
- Avoid using dynamic layouts. They can be difficult to load and maintain.
- Be careful with dependencies between components. For example, use abstraction patterns when possible to avoid issues with maintainability.
- Consider creating templates with placeholders. For example, use the Template View pattern to compose dynamic Web pages in order to ensure reuse and consistency.
- Consider composing views from reusable modular parts. For example, use the Composite View pattern to build a view from modular, atomic component parts.
- If you need to allow communication between presentation components, consider implementing the Publish/Subscribe pattern. This will lower the coupling between the components and improve testability.
Exception Management
Design a centralized exception-management mechanism for your application that catches and throws exceptions consistently. Pay particular attention to exceptions that propagate across layer or tier boundaries, as well as exceptions that cross trust boundaries. Design for unhandled exceptions so they do not impact application reliability or expose sensitive information.
Consider the following guidelines when designing your exception management strategy:
- Use user-friendly error messages to notify users of errors in the application.
- Avoid exposing sensitive data in error pages, error messages, log files, and audit files.
- Design a global exception handler that displays a global error page or an error message for all unhandled exceptions.
- Differentiate between system exceptions and business errors. In the case of business errors, display a user-friendly error message and allow the user to retry the operation. In the case of system exceptions, check to see if the exception was caused by issues such as system or database failure, display a user-friendly error message, and log the error message, which will help in troubleshooting.
- Avoid using exceptions to control application logic.
Design a user input strategy based on your application input requirements. For maximum usability, follow the established guidelines defined in your organization, and the many established industry usability guidelines based on years of user research into input design and mechanisms.
Consider the following guidelines when designing your input collection strategy:
- Use forms-based input controls for normal data-collection tasks.
- Use a document-based input mechanism for collecting input in Microsoft Office–style documents.
- Implement a wizard-based approach for more complex data collection tasks, or for input that requires a workflow.
- Design to support localization by avoiding hard-coded strings and using external resources for text and layout.
- Consider accessibility in your design. You should consider users with disabilities when designing your input strategy; for example, implement text-to-speech software for blind users, or enlarge text and images for users with poor sight. Support keyboard-only scenarios where possible for users who cannot manipulate a pointing device.
Design your UI layout so that the layout mechanism itself is separate from the individual UI components and UI process components. When choosing a layout strategy, consider whether you will have a separate team of designers building the layout, or whether the development team will create the UI. If designers will be creating the UI, choose a layout approach that does not require code or the use of development-focused tools.
Consider the following guidelines when designing your layout strategy:
- Use templates to provide a common look and feel to all of the UI screens.
- Use a common look and feel for all elements of your UI to maximize accessibility and ease of use.
- Consider device-dependent input, such as touch screens, ink, or speech, in your layout. For example, with touch-screen input you will typically use larger buttons with more spacing between them than you would with mouse or keyboard inputs.
- When building a Web application, consider using Cascading Style Sheets (CSS) for layout. This will improve rendering performance and maintainability.
- Use design patterns, such as Model-View-Presenter (MVP), to separate the layout design from interface processing.
Design your navigation strategy so that users can navigate easily through your screens or pages, and so that you can separate navigation from presentation and UI processing. Ensure that you display navigation links and controls in a consistent way throughout your application to reduce user confusion and hide application complexity.
Consider the following guidelines when designing your navigation strategy:
- Use well-known design patterns to decouple the UI from the navigation logic where this logic is complex.
- Design toolbars and menus to help users find functionality provided by the UI.
- Consider using wizards to implement navigation between forms in a predictable way.
- Determine how you will preserve navigation state if the application must preserve this state between sessions.
- Consider using the Command Pattern to handle common actions from multiple sources.
Presentation Entities
Use presentation entities to store the data you will use in your presentation layer to manage your views. Presentation entities are not always necessary; use them only if your datasets are sufficiently large and complex to require separate storage from the UI controls.
Consider the following guidelines when designing presentation entities:
- Determine if you require presentation entities. Typically, you may require presentation entities only if the data or the format to be displayed is specific to the presentation layer.
- If you are working with data-bound controls, consider using custom objects, collections, or datasets as your presentation entity format.
- If you want to map data directly to business entities, use a custom class for your presentation entities.
- Do not add business logic to presentation entities.
- If you need to perform data type validation, consider adding it in your presentation entities.
Request Processing
Design your request processing with user responsiveness in mind, as well as code maintainability and testability.
Consider the following guidelines when designing request processing:
- Use asynchronous operations or worker threads to avoid blocking the UI for long-running actions.
- Avoid mixing your UI processing and rendering logic.
- Consider using the Passive View pattern (a variant of MVP) for interfaces that do not manage a lot of data.
- Consider using the Supervising Controller pattern (a variant of MVP) for interfaces that manage large amounts of data.
User Experience
Good user experience can make the difference between a usable and unusable application. Carry out usability studies, surveys, and interviews to understand what users require and expect from your application, and design with these results in mind.
Consider the following guidelines when designing for user experience:
- When developing a rich Internet application (RIA), avoid synchronous processing where possible.
- When developing a Web application, consider using Asynchronous JavaScript and XML (AJAX) to improve responsiveness and to reduce post backs and page reloads.
- Do not design overloaded or overly complex interfaces. Provide a clear path through the application for each key user scenario.
- Design to support user personalization, localization, and accessibility.
- Design for user empowerment. Allow the user to control how he or she interacts with the application, and how it displays data to them.
UI Components
UI components are the controls and components used to display information to the user and accept user input. Be careful not to create custom controls unless it is necessary for specialized display or data collection.
Consider the following guidelines when designing UI components:
- Take advantage of the data-binding features of the controls you use in the UI.
- Create custom controls or use third-party controls only for specialized display and data-collection tasks.
- When creating custom controls, extend existing controls if possible instead of creating a new control.
- Consider implementing designer support for custom controls to make it easier to develop with them.
- Consider maintaining the state of controls as the user interacts with the application instead of reloading controls with each action.
UI Process Components
UI process components synchronize and orchestrate user interactions. UI processing components are not always necessary; create them only if you need to perform significant processing in the presentation layer that must be separated from the UI controls. Be careful not to mix business and display logic within the process components; they should be focused on organizing user interactions with your UI.
Consider the following guidelines when designing UI processing components:
- Do not create UI process components unless you need them.
- If your UI requires complex processing or needs to talk to other layers, use UI process components to decouple this processing from the UI.
- Consider dividing UI processing into three distinct roles: Model, View, and Controller/Presenter, by using the MVC or MVP pattern.
- Avoid business rules, with the exception of input and data validation, in UI processing components.
- Consider using abstraction patterns, such as dependency inversion, when UI processing behavior needs to change based on the run-time environment.
- Where the UI requires complex workflow support, create separate workflow components that use a workflow system such as Windows Workflow or a custom mechanism.
Designing an effective input and data-validation strategy is critical to the security of your application. Determine the validation rules for user input as well as for business rules that exist in the presentation layer.
Consider the following guidelines when designing your input and data validation strategy:
- Validate all input data on the client side where possible to improve interactivity and reduce errors caused by invalid data.
- Do not rely on client-side validation only. Always use server-side validation to constrain input for security purposes and to make security-related decisions.
- Design your validation strategy to constrain, reject, and sanitize malicious input.
- Use the built-in validation controls where possible, when working with .NET Framework.
- In Web applications, consider using AJAX to provide real-time validation.
Pattern Map
Key patterns are organized by key categories, as detailed in the Presentation Layer Frame in the following table. Consider using these patterns when making design decisions for each category.
Table 2 Pattern Map
- For more information on the Page Cache pattern, see “Enterprise Solution Patterns Using Microsoft .NET” at http://msdn.microsoft.com/en-us/library/ms998469.aspx
- For more information on the Model-View-Controller (MVC), Page Controller, Front Controller, Template View, Transform View, and Two-Step View patterns, see “Patterns of Enterprise Application Architecture (P of EAA)” at http://martinfowler.com/eaaCatalog/
- For more information on the Composite View, Supervising Controller, and Presentation Model patterns, see “Patterns in the Composite Application Library” at http://msdn.microsoft.com/en-us/library/cc707841.aspx
- For more information on the Chain of responsibility and Command pattern, see “data & object factory” at http://www.dofactory.com/Patterns/Patterns.aspx
- For more information on the Asynchronous Callback pattern, see “Creating a Simplified Asynchronous Call Pattern for Windows Forms Applications” at http://msdn.microsoft.com/en-us/library/ms996483.aspx
- For more information on the Exception Shielding and Entity Translator patterns, see “Useful Patterns for Services” at http://msdn.microsoft.com/en-us/library/cc304800.aspx
Pattern Descriptions
- Asynchronous Callback. Execute long-running tasks on a separate thread that executes in the background, and provide a function for the thread to call back into when the task is complete.
- Cache Dependency. Use external information to determine the state of data stored in a cache.
- Chain of Responsibility. Avoid coupling the sender of a request to its receiver by giving more than one object a chance to handle the request.
- Composite View . Combine individual views into a composite representation.
- Command Pattern. Encapsulate request processing in a separate command object with a common execution interface.
- Entity Translator. An object that transforms message data types into business types for requests, and reverses the transformation for responses.
- Exception Shielding. Prevent a service from exposing information about its internal implementation when an exception occurs.
- Front Controller . Consolidate request handling by channeling all requests through a single handler object, which can be modified at run time with decorators.
- Model-View-Controller . Separate the UI code into three separate units: Model (data), View (interface), and Presenter (processing logic), with a focus on the View. Two variations on this pattern include Passive View and Supervising Controller, which define how the View interacts with the Model.
- Page Cache. Improve the response time for dynamic Web pages that are accessed frequently but change less often and consume a large amount of system resources to construct.
- Page Controller . Accept input from the request and handle it for a specific page or action on a Web site.
- Passive View . Reduce the view to the absolute minimum by allowing the controller to process user input and maintain the responsibility for updating the view.
- Presentation Model . Move all view logic and state out of the view, and render the view through data-binding and templates.
- Supervising Controller . A variation of the MVC pattern in which the controller handles complex logic, in particular coordinating between views, but the view is responsible for simple view-specific logic.
- Template View . Implement a common template view, and derive or construct views using this template view.
- Transform View . Transform the data passed to the presentation tier into HTML for display in the UI.
- Two-Step View . Transform the model data into a logical presentation without any specific formatting, and then convert that logical presentation to add the actual formatting required.
Technology Considerations
The following guidelines will help you to choose an appropriate implementation technology. The guidelines also contain suggestions for common patterns that are useful for specific types of application and technology.
Mobile Applications
Consider the following guidelines when designing a mobile application:
- If you want to build full-featured connected, occasionally connected, and disconnected executable applications that run on a wide range of Microsoft Windows®–based devices, consider using the Microsoft Windows Compact Framework.
- If you want to build connected applications that require Wireless Application Protocol (WAP), compact HTML (cHTML), or similar rendering formats, consider using ASP.NET Mobile Forms and Mobile Controls.
- If you want to build applications that support rich media and interactivity, consider using Microsoft Silverlight® for Mobile.
Rich Client Applications
Consider the following guidelines when designing a rich client application:
- If you want to build applications with good performance and interactivity, and have design support in Microsoft Visual Studio®, consider using Windows Forms.
- If you want to build applications that fully support rich media and graphics, consider using Windows Presentation Foundation (WPF).
- If you want to build applications that are downloaded from a Web server and then execute on the client, consider using XAML Browser Applications (XBAP).
- If you want to build applications that are predominantly document-based, or are used for reporting, consider designing a Microsoft Office Business Application.
- If you decide to use Windows Forms and you are designing composite interfaces, consider using the Smart Client Software Factory.
- If you decide to use WPF and you are designing composite interfaces, consider using the Composite Application Guidance for WPF.
- If you decide to use WPF, consider using the Presentation Model (Model-View-ViewModel) pattern.
- If you decide to use WPF, consider using WPF Commands to communicate between your View and your Presenter or ViewModel.
- If you decide to use WPF, consider implementing the Presentation Model pattern by using DataTemplates over User Controls to give designers more control.
Rich Internet Applications (RIA)
Consider the following guidelines when designing an RIA:
- If you want to build browser-based, connected applications that have broad cross-platform reach, are highly graphical, and support rich media and presentation features, consider using Silverlight.
- If you decide to use Silverlight, consider using the Presentation Model (Model-View-ViewModel) pattern.
Web Applications
Consider the following guidelines when designing a Web application:
- If you want to build applications that are accessed through a Web browser or specialist user agent, consider using ASP.NET.
- If you want to build applications that provide increased interactivity and background processing, with fewer page reloads, consider using ASP.NET with AJAX.
- If you want to build applications that include islands of rich media content and interactivity, consider using ASP.NET with Silverlight controls.
- If you are using ASP.NET and want to implement a control-centric model with separate controllers and improved testability, consider using the ASP.NET MVC Framework.
- If you are using ASP.NET, consider using master pages to simplify development and implement a consistent UI across all pages.
patterns & practices Solution Assets
- Web Client Software Factory at http://msdn.microsoft.com/en-us/library/bb264518.aspx
- Smart Client Software Factory at http://msdn.microsoft.com/en-us/library/aa480482.aspx
- Composite Application Guidance for WPF at http://msdn.microsoft.com/en-us/library/cc707819.aspx
- Smart Client - Composite UI Application Block at http://msdn.microsoft.com/en-us/library/aa480450.aspx
Additional Resources
- For more information, see Microsoft Inductive User Interface Guidelines at http://msdn.microsoft.com/en-us/library/ms997506.aspx .
- For more information, see User Interface Control Guidelines at http://msdn.microsoft.com/en-us/library/bb158625.aspx .
- For more information, see User Interface Text Guidelines at http://msdn.microsoft.com/en-us/library/bb158574.aspx .
- For more information, see Design and Implementation Guidelines for Web Clients at http://msdn.microsoft.com/en-us/library/ms978631.aspx .
- For more information, see Web Presentation Patterns at http://msdn.microsoft.com/en-us/library/ms998516.aspx .
Navigation menu
Page actions.
- View source
Personal tools
- Community portal
- Current events
- Recent changes
- Random page
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information

- This page was last edited on 22 January 2010, at 02:50.
- Privacy policy
- About Guidance Share
- Disclaimers
Presentation Models by Example
- Conference paper
- Cite this conference paper
- Pablo Castells 3 &
- Pedro Szekely 4
Part of the book series: Eurographics ((EUROGRAPH))
106 Accesses
1 Citations
Interface builders and multi-media authoring tools only support the construction of static displays where the components of the display are known at design time (e.g., buttons, menus). High-level UIMSs and automated designers support more sophisticated displays but are not easy to use as they require dealing explicitly with elaborate abstract concepts. This paper describes a GUI development environment, HandsOn, where complex displays of dynamically changing data can be constructed by direct manipulation. HandsOn integrates principles of graphic design, supports constraint-based layout, and has facilities for easily specifying the layout of collections of data. The system incorporates Programming By Example techniques to relieve the designer from having to deal with abstractions, and relies on a model-based language for the representation of the displays being constructed and as a means to provide information for the tool to reason about.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Unable to display preview. Download preview PDF.
R. Ballinger. Layout and Graphic Design . Van Nostrand Reinhold, New York, 1970.
Google Scholar
Bauer, B. Generating User Interfaces from Formal Specifications of the Application . Proceedings of 2nd International Workshop on Computer-Adied Design of User Interfaces (CADUI’96). Presses Universitaires de Namur, 1996.
F. Bodart, A.-M. Hennebert, J.-M. Leheureux, I. Provot, B. Sacre, J. Vanderdonckt. Towards a Systematic Building of Software Architectures: the Trident Methodological Guide . Proceedings of 2nd Eurographics Workshop on Design, Specification, Verification of Interactive Systems (DSV-IS’95). Springer-Verlag, 1995.
P. Castells, P. Szekely and E. Salcher. Declarative Models of Presentation . Proceedings of International Conference on Intelligent Interfaces (IUI’96). Orlando, Florida, 1997.
P. Castells and P. Szekely. HandsOn: Dynamic Interface Presentations by Example . To appear in Proceedings of 8th International Conference on Human-Computer Interaction (HCI International ’ 99 ). Munich, Germany, 1999.
A. Cypher (ed.). Watch What I Do: Programming by Demonstration . The MIT Press, 1993.
B. A. Myers et al. The Amulet 2.0 Reference Manual . Carnegie Mellon University Tech. Report, 1996.
B. A. Myers. User Interface Software Tools . ACM Transactions on Computer Human Interaction, v2, nl, March 1995, pp. 64–103.
B. A. Myers. Creating User Interfaces by Demonstration . Academic Press, San Diego, 1988.
MATH Google Scholar
B. A. Myers, J. Goldstein, M. Goldberg. Creating Charts by Demonstration . Proceedings of the CHI’94 Conference. ACM Press, Boston, April 1994.
S. F. Roth and J. Mattis Data Characterization for Intelligent Graphics Presentation . Proceedings of SIGCHI’90 Human Factors in Computing Systems. ACM press, Seattle,WA, April 1990, pp. 193–200.
S.F. Roth, J. Kolojejchick, J. Mattis, and J. Goldstein. Interactive Graphic Design Using Automatic Presentation Knowledge . Proceedings of the CHI’94 Conference. ACM Press, Boston, April 1994, pp. 112–117
P. Szekely, P. Luo, and R. Neches. Beyond Interface Builders: Model-Based Interface Tools . Proceedings of INTERCHI’93, April 1993.
P. Szekely, P. Sukaviriya, P. Castells, J. Muthukumarasamy and E. Salcher. Declarative Interface Models for User Interface Construction: The Mastermind Approach . In Engineering for Human-Computer Interaction, L. Bass and C. Unger (eds.). Chapman & Hall, 1996.
A. Wiecha, W. Bennett, S. Boies, J. Gould and S. Greene. ITS: A Tool For Rapidly Developing Interactive Applications . ACM Transactions on Information Systems 8 (3), July 1990, pp. 204–236.
Article Google Scholar
R. Williams. The Non-Designer Design Book . Peachpit Press Inc., Berkeley, California, 1994.
Download references
Author information
Authors and affiliations.
E.T.S.I. Informatica, Universidad Autonoma de Madrid, Ctra. de Colmenar Viejo km. 17, 28049, Madrid, Spain
Pablo Castells
Information Sciences Institute, University of Southern California, 4676 Admiralty Way, #1001, Marina del Rey, CA, 90292, USA
Pedro Szekely
You can also search for this author in PubMed Google Scholar
Editor information
Editors and affiliations.
Department of Computer Science, University of York, York, UK
Red Whale Software, Palo Alto, USA
Angel Puerta
Rights and permissions
Reprints and permissions
Copyright information
© 1999 Springer-Verlag/Wien
About this paper
Cite this paper.
Castells, P., Szekely, P. (1999). Presentation Models by Example. In: Duke, D., Puerta, A. (eds) Design, Specification and Verification of Interactive Systems ’99. Eurographics. Springer, Vienna. https://doi.org/10.1007/978-3-7091-6815-8_8
Download citation
DOI : https://doi.org/10.1007/978-3-7091-6815-8_8
Publisher Name : Springer, Vienna
Print ISBN : 978-3-211-83405-3
Online ISBN : 978-3-7091-6815-8
eBook Packages : Springer Book Archive
Share this paper
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Home Blog Guide to Presenting Using the Pyramid Principle
Guide to Presenting Using the Pyramid Principle
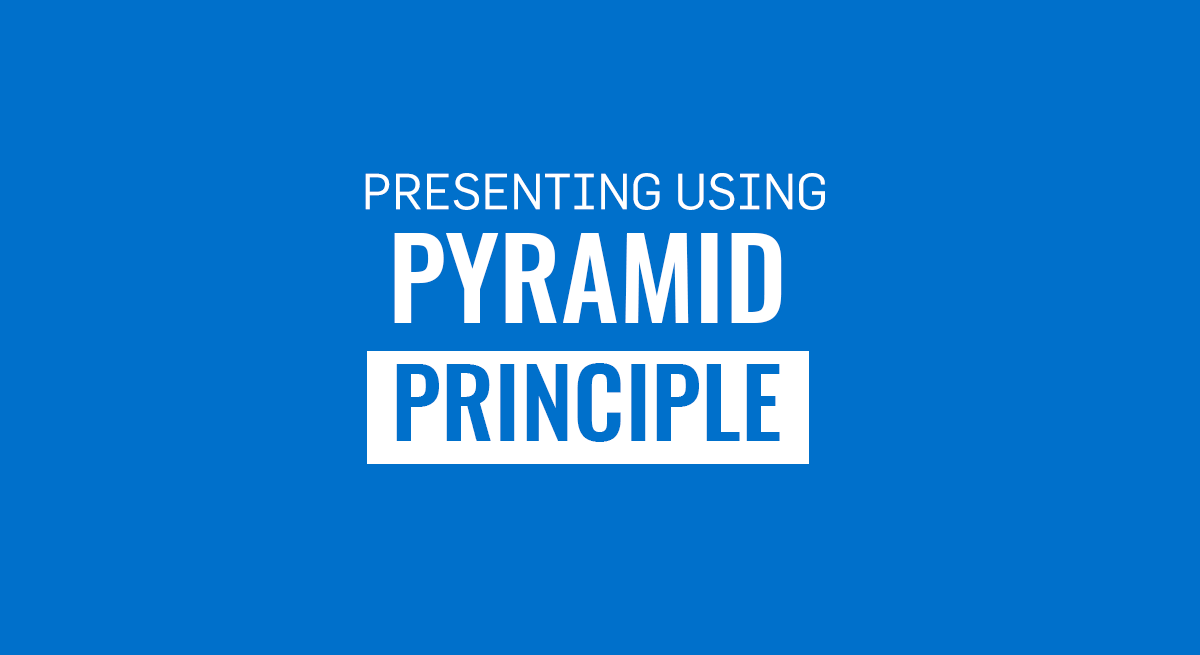
The conventional method for presenting a PowerPoint presentation entails reflecting upon the facts and fine details to draw the audience towards a conclusion. This can result in a lengthy Q&A session at the end of the presentation, where the audience might appear confused, unsatisfied, and at times, feel manipulated into being led towards a conclusion of the presenter’s choosing. A better alternative can be to use the Pyramid Principle .
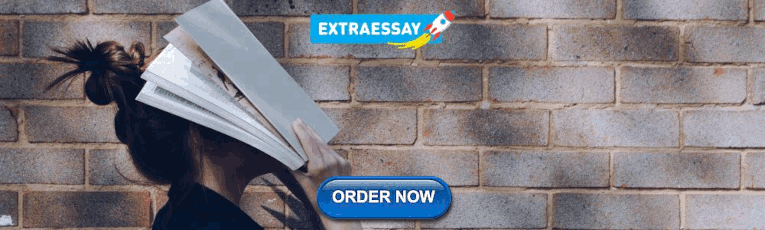
Present using the Pyramid Principle: An Excellent Tool to Communicate Logical Information
The Pyramid Principle can be used for structuring communication to have a meaningful impact. Whether you’re making reports, delivering a presentation, or preparing an analysis, the Pyramid Principle can be an excellent tool to communicate logical information . The structured format in which the communication relays the answer prior to facts and data can help create an environment where critical thinking can be stimulated at the very start, instead of the end.
What is the Pyramid Principle?
Developed by an ex-McKinsey consultant, Barbara Minto, the Pyramid Principle is considered as one of the most important concepts of executive communication often taught in strategic communications and leadership programs . Unlike conventional modes of presenting information, the Pyramid Principle presents the answer at the beginning, followed by supporting arguments, data, and facts. The concept was documented in her book published in 1985 titled; The Pyramid Principle: Logic in Writing and Thinking .
The information is presented in the form of a pyramid, with the core idea at the top, which is then broken down by revealing fine details. The top of the pyramid contains the answer, which is the starting point. The middle of the pyramid represents supporting arguments. Whereas the bottom of the pyramid gives the supporting data and facts.
What are the 3 Rules When Creating Pyramid Structure?
When sitting through a lengthy PowerPoint presentation bloated with facts and data and a leading conclusion, one can feel unsatisfied with the presenter. However, when given the answer at the very beginning, you might feel a need to think through its merits from the very start. As you are presented with supporting arguments and facts, you can determine whether you agree or disagree with the statement or feel a need to raise important questions.
This approach makes it possible to aid structured thinking and stimulate critical thinking at the very start of a presentation or when reading through a report or research. Rather than feeling that you are being led towards a conclusion with convoluted information.
Supporting Arguments
Once the audience has been given the answer or hypothesis at the start, they can begin critically analyzing the supporting argument that follows. This is the second stage of the Pyramid Principle, where the answer is supported with relevant arguments to help test the validity of the hypothesis or to present it for critical analysis.
Supporting Data and Facts
Unlike conventional approaches to presenting data, the Pyramid Principle makes it possible to see supporting facts and data after a hypothesis. Rather than wondering what the long bits of information might be leading to. The one reading through the information or sitting in the audience does not need to wonder about the suggested conclusion, as it has already been presented at the start. Enabling critical analysis of the data and facts, as they are presented.
Why Does the Pyramid Principle Work?
Before the Pyramid Principle, Barbara Minto developed the MECE principle in the 1960s, which is a grouping principle that separates items into subsets. These subsets are mutually exclusive and collectively exhaustive, hence the name MECE. This concept underlies what was later known as the Pyramid Principle during the 1980s. Providing a mechanism to structure information for maximum impact. Making the principle practical and useful.
The Pyramid Principle suggests that ideas should be presented as a pyramid. Using the pyramid structure, the information is grouped together with similar, low-level facts, drawing insights from the similarity, and forming a group of related insights.
How is the Pyramid Principle used for Effective Writing?
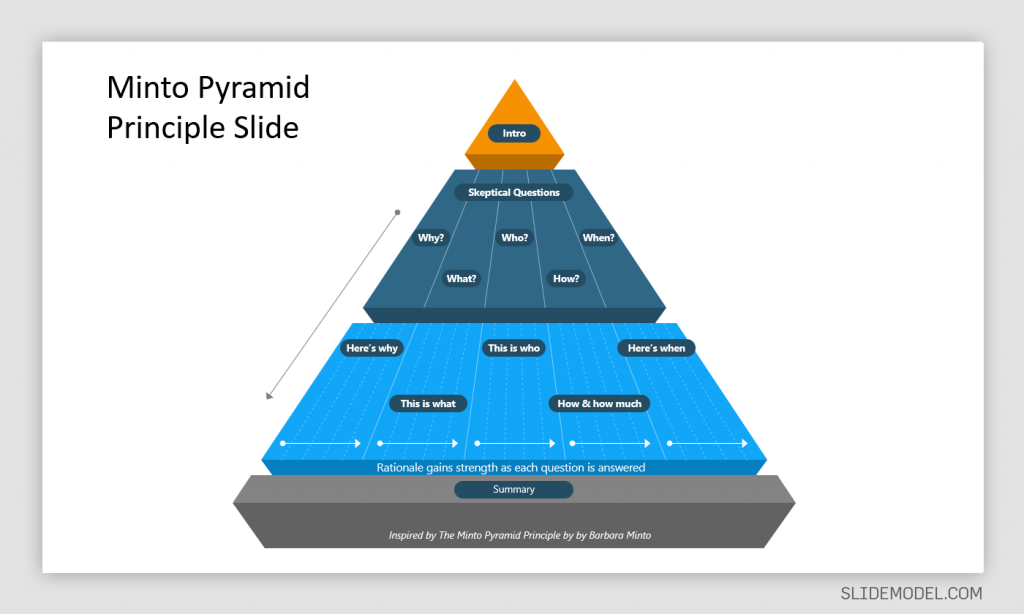
Effective writing produces content that is clear, accurate, and concise. The writing is focused, coherent, correct, supporting the central idea. When incorporating the Pyramid Principle for effective writing, the same rules apply. You need to start with the central idea. The ‘answer’ serves as a single thought, supported by arguments, data, and facts. You should present the ideas using the pyramid structure, summarizing the ideas grouped below each other, while remaining true to the ‘single thought’, i.e. the central idea (the answer).
An example of the structure for content produced using the Pyramid Principle would be as follows:
Answer -> Supporting Argument -> Evidence.
The answer shall remain at the top or at the heart of the content you are writing, whereas the supporting argument will be backed by evidence at each instance. If you have more than one supporting argument, you should take the time to structure each argument. For example, write the first supporting argument, followed by its evidence, before moving on to the second supporting argument and its related evidence.
How to Apply the Pyramid Principle in Preparing Presentations
When applying the Pyramid Principle, you must first start with the hypothesis and break it down with supporting arguments, backed by facts and data. It’s quite likely that during the course of the presentation, your audience would want to ask tough questions. Using this concept, you can enable those questions to be asked sooner and provide your breakdown of the information in a structured manner to ensure all your arguments are covered.
Begin with the Hypothesis
It is common to present the hypothesis at the very end after data, facts, and different ideas related to the potential hypothesis have been presented. The Pyramid Principle flips this conventional approach by presenting the hypothesis (answer) at the beginning.
Example: In our example, a mobile operator called ABC Telecom wants to enter a new market in Country X, located in Central Africa. The conventional approach would be to provide data and facts before mentioning why it’s a good idea to invest in a specific country. The presenter might even take the time to mention the country at the very end. The Pyramid Principle would require this information to be shared at the very beginning instead. In our example, the presenter would start the presentation by mentioning the hypothesis or answer.
In this case, the hypothesis might be something as follows: ‘investing in Country X would be profitable and lead to 30% increase in revenue for ABC Telecom over the next 5 years’.
Presenting the hypothesis at the start will stimulate critical thinking and aid structured communication, where there might be people for and against the argument scrambling to ask tough questions. That’s one of the benefits of the Pyramid Principle, as it helps bring out critical questions from the very start instead of at the end of a bloated presentation or report.
Present Arguments to Support Your Answer
It is essential to back the answer with supporting arguments to enable a meaningful discussion or to raise key questions regarding the accuracy of the hypothesis. For a business, this can have dire implications and major investment decisions might hinge on such information.
Example: The presenter proceeds to mention why investing in Country X by ABC Telecom is important for the long-term sustainability of the company. We assume that the argument for this investment is that ABC Telecom is already operating in a saturated market, where profit margins are projected to decline, and it is essential to move into a new market to increase revenue and profitability.
Present the Data to Support Your Argument
A supporting argument is only as good as the data and facts that are presented to back it up. The bottom of the pyramid, therefore, is the foundation of the Pyramid Principle. The foundation needs to contain accurate and reliable information that can back the hypothesis.
Example: In our example, ABC Telecom conducted research across 3 potential markets (Country X, Country Y, and Country Z) to look for countries to expand their operations in. During the research, it was revealed that only Country X appeared to be a lucrative market for investment.
The research revealed that Country Y and Country Z already have a saturated telecom industry with heavy taxes, rigid government policies, and very low ease of doing business ratings. Moreover, the population and telecom density of both these countries does not appear to indicate the potential for growth. On the contrary, Country X with a large population and low competition serves as a lucrative market. The market competition is slim, the government is looking to expand its telecom infrastructure, giving tax concessions to companies looking to set up their operations. The country also has a better ease of doing business ranking.
Another factor that goes in favor of ABC Telecom investing in Country X is that they are already operating in the neighboring country (Country W), making it easier to expand operations due to familiarity with the region. Furthermore, other global telecom operators are looking towards expanding into Asia Pacific, instead of Central Africa, leaving the market open for a new operator to rapidly expand. The recent rise in purchasing power, increased use of smartphones, and the demand for 4G and 5G services (currently not available in Country X) make another compelling argument for an efficient mobile operator to start operations in the country.
What are the Benefits of Applying the Pyramid Principle?
1. be better at structured thinking.
The idea behind structured thinking is to be efficient at problem-solving and critically analyzing things in an organized manner. The Pyramid Principle provides this formula in its pyramid-like structure, where important questions can be asked right from the start.
2. Focus on Core issues
Lengthy reports and presentations can lead to a lot of confusion and might even deviate the people in charge of making decisions from the core issue. By placing the core issue at the very heart and elaborating upon it at the start, the Pyramid Principle can help keep everyone involved in the discussion on point.
3. Placing the Solutions at the Start Initiates Critical Analysis
When exploring solutions, such as in our example above, (the expansion of a telecom operator into a new market), it is essential to initiate critical analysis. Key decisions related to investment, expansion into new markets, or changes to products or services can make or break a business. Placing the solution at the start of the discussion leaves ample room for critical analysis to see if the presented solution can be applied or if better alternatives can be explored.
4. Hypothesis Backed by Data can Aid Better Decision Making
How would you feel if you are presented with 1 hour of slides filled with data, with a solution at the end? It is likely that such a presentation would leave you weary and tired, unable to connect the data with the solution at the end. Now imagine, you are given the solution at the start of the presentation, and each bit of information related to the answer that you would see after that can be connected with the solution when analyzing its practical implications. The latter is an approach that will help you connect with the arguments, facts, and data, as they are presented. Since you are already aware of the answer or hypothesis that you need to focus on.
Is Barbara Minto’s Pyramid Principle Still Valid?
The short answer to the question would be, yes! Barbara Minto’s Pyramid Principle is considered as one of the most important methodologies for structured communication. Since its initial revelation during 1985 to the revised edition of Minto’s book in 1996; ‘The Minto Pyramid Principle: Logic in Writing, Thinking and Problem Solving’, the principle still remains effective. It is widely used for making business executives absorb information quickly, in a structured manner, and aiding executive communication.
Final Words
The Pyramid Principle can be used effectively for structured thinking, problem-solving, and presenting information in a palatable format for busy business executives. Moreover, presenting the information true to the core idea presented at the very start can help make it easier to keep the audience abreast with the arguments, data, and facts that follow.
At the very core of decision making, be it decisions made by businesses or individuals, everyone wants to find the solution that works best for them. But complex data analysis and information overload can hinder good decisions and obscure solutions. By starting with the potential solution, its merits and demerits can be critically analyzed with ease.
1. Minto Pyramid Principle PowerPoint Template
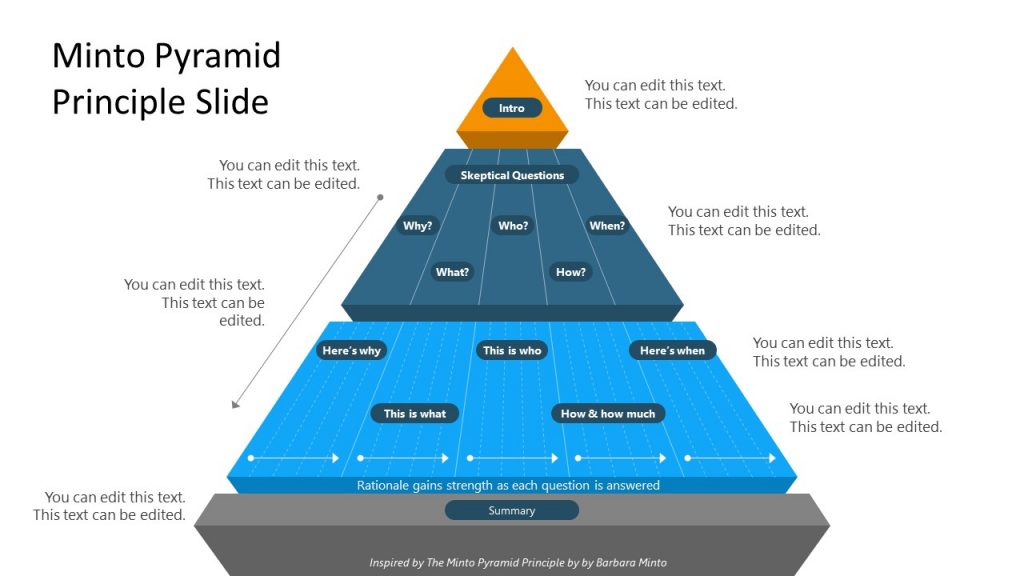
Use This Template
2. 3 Levels 3D Pyramid Template for PowerPoint
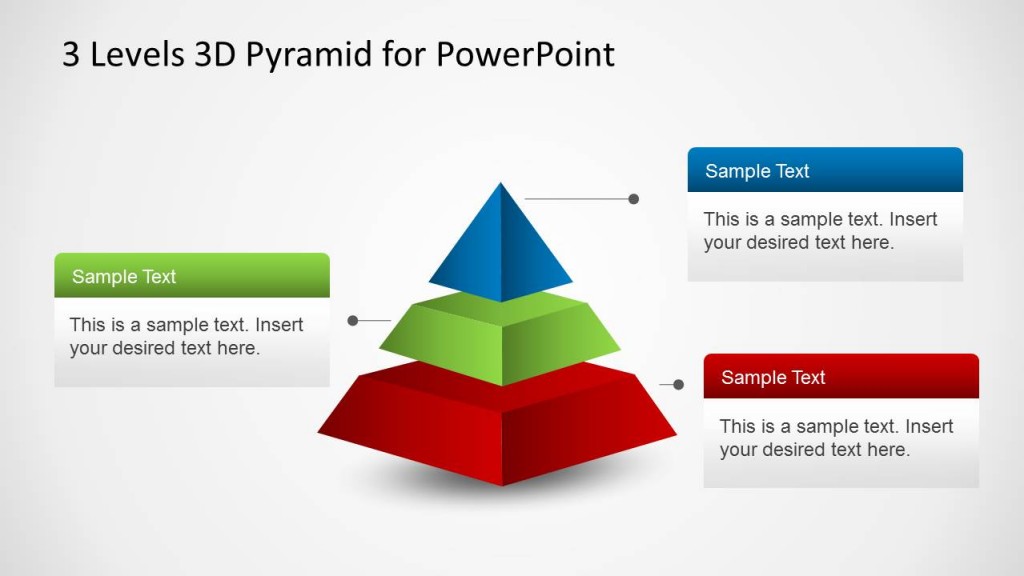
Like this article? Please share
Communication, Communication Skills, Presentation Approaches, Presentations
Related Articles
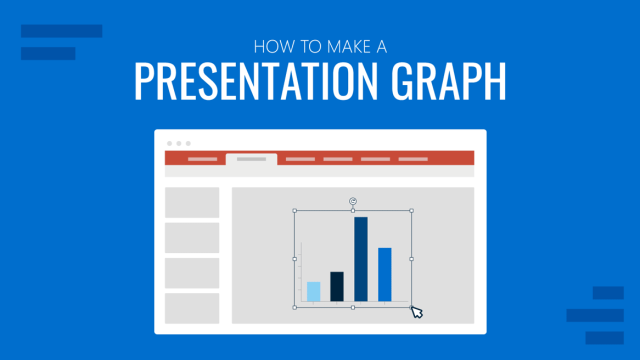
Filed under Design • March 27th, 2024
How to Make a Presentation Graph
Detailed step-by-step instructions to master the art of how to make a presentation graph in PowerPoint and Google Slides. Check it out!
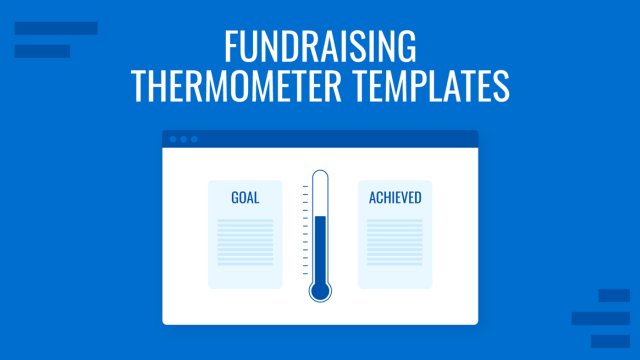
Filed under Presentation Ideas • February 29th, 2024
How to Make a Fundraising Presentation (with Thermometer Templates & Slides)
Meet a new framework to design fundraising presentations by harnessing the power of fundraising thermometer templates. Detailed guide with examples.
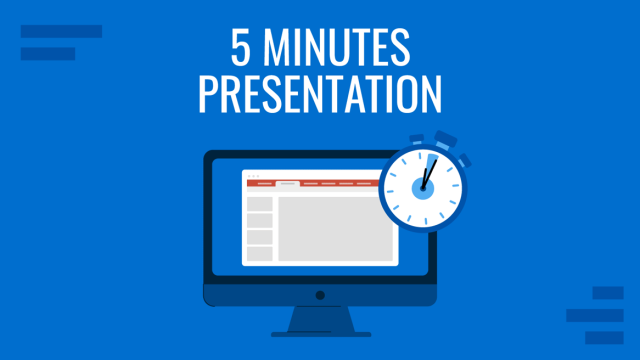
Filed under Presentation Ideas • February 15th, 2024
How to Create a 5 Minutes Presentation
Master the art of short-format speeches like the 5 minutes presentation with this article. Insights on content structure, audience engagement and more.
Leave a Reply
These are Sankey diagrams that should hopefully illustrate the factors involved that results in a favorable presentation model where latency is the lowest.
The presentation model Windows ends up using for a game can be inspected using either Special K’s control panel or framepacing widget, or by using the excellent PresentMon tool from Intel.
The diagrams were created using SankeyMATIC .
A simplification that has been made in the diagrams is that even with MPOs enabled a game can still be reported as using Hardware: Independent Flip on some systems, though when this happens it tend to act and function identical to Hardware Composed: Independent Flip .
¶ Flip (D3D11/12*)
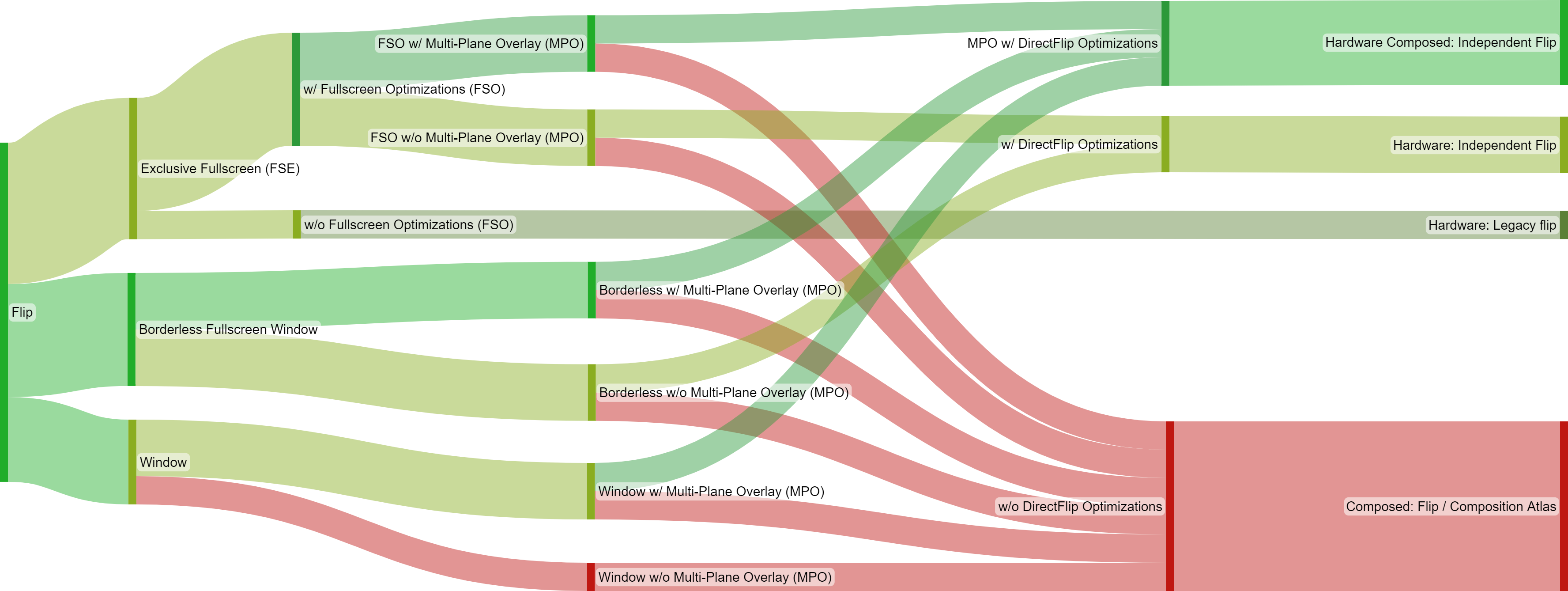
For a game using a Flip swap effect, there are various factors that determine which presentation model will be used:
- (*) The Exclusive Fullscreen (FSE) section is not applicable to DirectX 12 games as its “exclusive mode” is merely an “emulated” mode where the Windows desktop temporarily changes resolution, refresh rate, and colorspace to the one requested by the game, but otherwise acts exactly like a regular borderless fullscreen window.
- Fullscreen Optimizations (FSO) toggle (only relevant for games running in Exclusive Fullscreen (FSE) mode).
- Whether Multi-Plane Overlay (MPO) are available on the hardware.
- DirectFlip : Your swapchain buffers match the screen dimensions, and your window client region covers the screen. Instead of using the DWM swapchain to display on the screen, the application swapchain is used.
- DirectFlip with panel fitters (requires MPO ) : Your window client region covers the screen, and your swapchain buffers are within some hardware-dependent scaling factor (for example, 0.25x to 4x) of the screen. The GPU scanout hardware is used to scale your buffer while sending it to the display.
- DirectFlip with multi-plane overlay (requires MPO ) : Your swapchain buffers are within some hardware-dependent scaling factor of your window dimensions. The DWM is able to reserve a dedicated hardware scanout plane for your application, which is then scanned out and potentially stretched to an alpha-blended sub-region of the screen.
¶ BitBlt (D3D11)
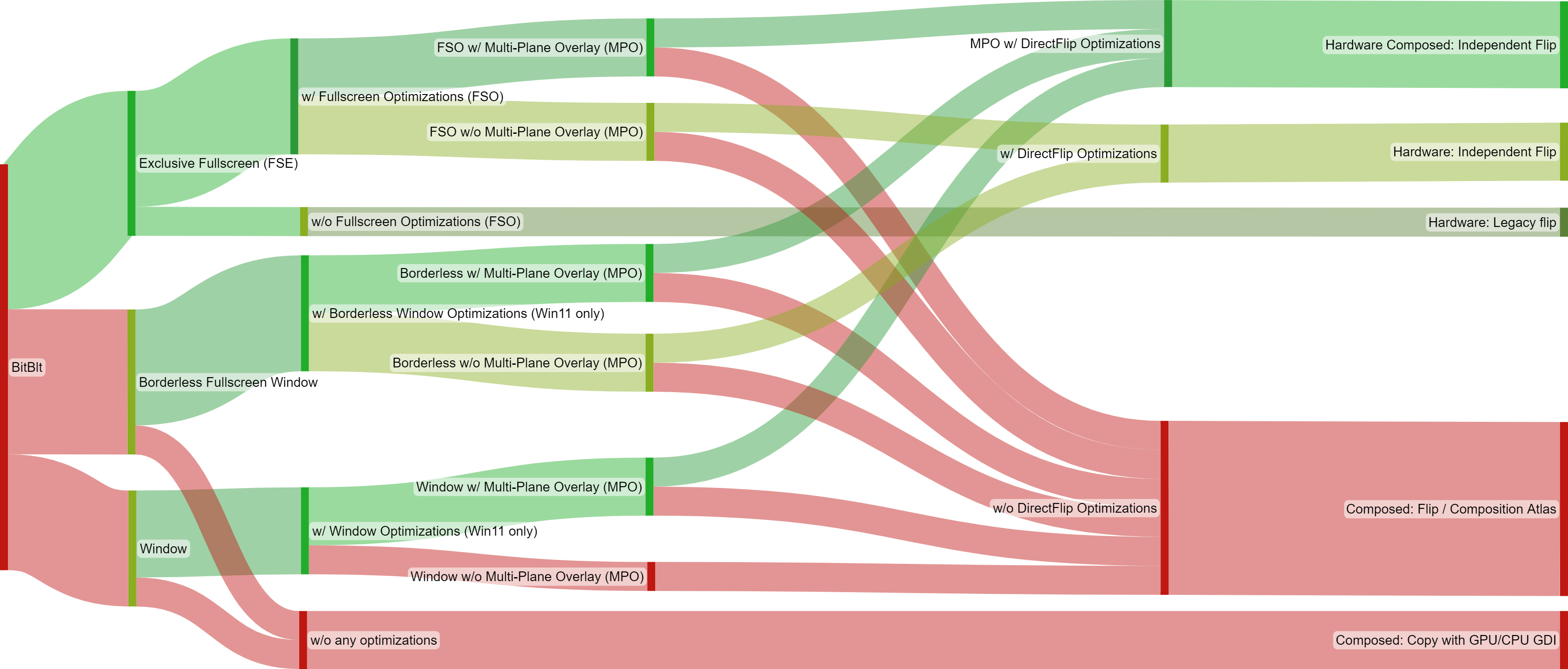
For a game using a legacy BitBlt swap effect, there are various factors that determine which presentation model will be used:
- The requested display mode of the game.
- Special K’s automatic flip model override performs this functionality on Windows 10 and earlier versions of Windows 11 where the OS provided override is not available.
- DirectFlip (requires Fullscreen/Windowed Optimizations) : Your swapchain buffers match the screen dimensions, and your window client region covers the screen. Instead of using the DWM swapchain to display on the screen, the application swapchain is used.
- DirectFlip with panel fitters (requires MPO + Fullscreen/Windowed Optimizations) : Your window client region covers the screen, and your swapchain buffers are within some hardware-dependent scaling factor (for example, 0.25x to 4x) of the screen. The GPU scanout hardware is used to scale your buffer while sending it to the display.
- DirectFlip with multi-plane overlay (requires MPO + Fullscreen/Windowed Optimizations) : Your swapchain buffers are within some hardware-dependent scaling factor of your window dimensions. The DWM is able to reserve a dedicated hardware scanout plane for your application, which is then scanned out and potentially stretched to an alpha-blended sub-region of the screen.
OpenAI's big event: CTO Mira Murati announces GPT-4o, which gives ChatGPT a better voice and eyes
- OpenAI's "Spring Update" revealed new updates to ChatGPT.
- OpenAI CTO Mira Murati kicked off the event.
- She announced GPT-4o, its next flagship AI model, with improved voice and vision capabilities.

OpenAI just took the wraps off a big new update to ChatGPT.
Cofounder and CEO Sam Altman had teased "new stuff" coming to ChatGPT and GPT-4 , the AI model that powers its chatbot, and told his followers to tune in Monday at 1 p.m. ET for its "Spring Update" to learn more.
Also ahead of time, Altman ruled that the event would reveal GPT-5 or a new OpenAI search engine, which is reportedly in the works. OpenAI is reportedly planning to eventually take on internet search giant Google with its own AI-powered search product.
But the big news on Monday was OpenAI's new flagship AI model, GPT-4o, which will be free to all users and "can reason across audio, vision, and text in real time." It was CTO Mira Murati who delivered the updates with no appearance on the livestream from Altman.
There were a ton of demos intended to demonstrate the real-time smarts of GPT-4o.
OpenAI researchers showed how the new ChatGPT can quickly translate speech and help with basic linear algebra using its visual capabilities. The use of the tech on school assignments has been a polarizing topic in education since it first launched.
Say hello to GPT-4o, our new flagship model which can reason across audio, vision, and text in real time: https://t.co/MYHZB79UqN Text and image input rolling out today in API and ChatGPT with voice and video in the coming weeks. pic.twitter.com/uuthKZyzYx — OpenAI (@OpenAI) May 13, 2024
OpenAI posted another example to X of how one can interact with the new ChatGPT bot. It resembled a video call, and it got pretty meta.
In the video, ChatGPT takes in the room around it, discerns it's a recording setup, figures it might have something to do with OpenAI since the user is wearing a hoodie, and then gets told that the announcement has to do with the AI — it is the AI. It reacts with a voice that sounds more emotive.
OpenAI also announced the desktop version of ChatGPT, and a new and improved user interface.
In addition to GPT-4o and ChatGPT, OpenAI's other products include its AI-powered image generator DALL-E , its unreleased text-to-video generator Sora , and its GPT app store.
You can catch up on our liveblog of the event below.
That’s a wrap! OpenAI concludes the event without an appearance from Altman.
OpenAI says text and image input for GPT-4o-powered ChatGPT is launching today. Meanwhile, voice and video options will drop in the coming weeks, the company said.
Although Altman didn't step in front of the camera, the CEO posted videos from the audience on X.
He also teases "more stuff to share soon."
GPT-4o can also break down charts
The new AI model can interact with code bases, the OpenAI execs say. The next demo shows it analyzing a chart from some data.
It's a plot of global temperatures. GPT-4o gives some takeaways from what it sees, and CTO Mira Murati asks about the Y axis, which the AI explains.
ChatGPT reads human emotions — with a stumble
For the last live demo of the day, Zoph holds his phone up to his face and asks ChatGPT to tell him how he looks. Initially, it identifies him as a "wooden surface" — a reference to an earlier photo he had shared.
But after a second try, the model gives a better answer.
"It looks like you're feeling pretty happy and cheerful," ChatGPT says, noting the small smile on Zoph's face.
In one of the final tests, ChatGPT becomes a translator
In response to a request from an X user, Murati speaks to ChatGPT in Italian.
In turn, the bot translates her query into English for Zoph and Chen.
"Mike, she wonders if whales could talk, what would they tell us?" she said in English after hearing Murati's Italian.
It's pretty impressive.
The video demo shows how it could help with math homework, including basic linear algebra
OpenAI Research Lead Barret Zoph walks through an equation on a whiteboard (3x+1=4), and ChatGPT gives him hints as he finds the value of x — making it basically a real-time math tutor.
At the beginning, the bot jumped the gun.
"Whoops, I got too excited," it said after it tried to solve the math problem hadn't been uploaded yet.
But it then walked him through each step, recognizing his written work as he tried to solve the equation.
It was able to recognize math symbols, and even a heart.
OpenAI's first demo: Talking to GPT-4o
It's demo time!
The new bot has a voice that sounds like an American female, but no word yet if you can change it.
OpenAI Research Lead Mark Chen pulled out ChatGPT on his phone and asks for advice on giving a live presentation using Voice Mode.
"Mark, you're not a vacuum cleaner," it responds when he hyperventilates, appearing to perceive his nervousness. It then tells him to moderate his breathing.
Some big changes, you can interrupt the AI now, and there shouldn't be the usual 2 or 3-second delay with GPT-4o.
It can also detect emotion, according to OpenAI.
GPT-4o will have improved voice capabilities
Murati emphasizes the necessity of safety with the real-time voice and audio capabilities of the new GPT-4o model.
She says OpenAI is "continuing our iterative deployment to bring all the capabilities to you."
Murati says the big news is a "new flagship model" called GPT-4o.
The new model is called GPT-4o, and Murati says that OpenAI is making a "huge step forward" with ease of use with the new model.
It's free for users, and "allows us to bring GPT-4 class intelligence to our free users," Murati says.
And we're off!
The livestream began with CTO Mira Murati at OpenAI's offices.
OpenAI is going to be announcing 3 things today, she says. "That's it."
For those who want to watch live, you can view the whole event here.
OpenAI will be livestreaming its spring update, which kicks off in less than an hour.
Axel Springer, Business Insider's parent company, has a global deal to allow OpenAI to train its models on its media brands' reporting.
- Main content
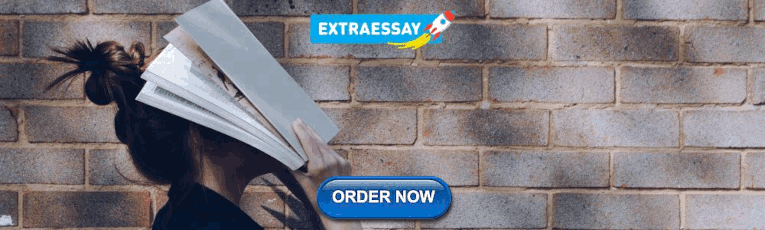
IMAGES
VIDEO
COMMENTS
Model-view-presenter. Model-view-presenter ( MVP) is a derivation of the model-view-controller (MVC) architectural pattern, and is used mostly for building user interfaces. In MVP, the presenter assumes the functionality of the "middle-man". In MVP, all presentation logic is pushed to the presenter.
4. The main difference is that in MVC the Controller does not pass any data from the Model to the View. It just notifies the View to get the data from the Model itself. However, in MVP, there is no connection between the View and Model. The Presenter itself gets any data needed from the Model and passes it to the View to show.
Presentation Model is known to users of Visual Works Smalltalk as Application Model. How it Works. The essence of a Presentation Model is of a fully self-contained class that represents all the data and behavior of the UI window, but without any of the controls used to render that UI on the screen. A view then simply projects the state of the ...
The Open System Interconnection (OSI) Model is a seven layer model developed by International Organization for Standardization (ISO) in the late 1970s. It is a layered, abstract definition for communications protocol and computer network protocol design. ... The Presentation Layer exists to make sure that the Application Layer of the sender ...
Overview. The presentation layer contains the components that implement and display the user interface and manage user interaction. This layer includes controls for user input and display, in addition to components that organize user interaction. Figure 1 shows how the presentation layer fits into a common application architecture.
3. The model The presentation model specifies the structure and graphical components of displays, how the components are connected to application data, the visual appearance of each component, and how the components are laid out. 3.1 Basic constructs The model consists of a part-whole hierarchy of graphical objects that is defined by
Declarative Models of Presentation. Proceedings of International Conference on Intelligent Interfaces (IUI'96). Orlando, Florida, 1997. Google Scholar. P. Castells and P. Szekely. HandsOn: Dynamic Interface Presentations by Example. To appear in Proceedings of 8th International Conference on Human-Computer Interaction (HCI International ' 99 ).
Model-view-controller (MVC) is a software design pattern commonly used for developing user interfaces that divides the related program logic into three interconnected elements. These elements are: ... e.g. multiple views on one model simultaneously, composable presentation widgets, e.g. one view used as a subview of another, ...
Guide to Presenting Using the Pyramid Principle. • October 1st, 2021. The conventional method for presenting a PowerPoint presentation entails reflecting upon the facts and fine details to draw the audience towards a conclusion. This can result in a lengthy Q&A session at the end of the presentation, where the audience might appear confused ...
The presentation model Windows ends up using for a game can be inspected using either Special K's control panel or framepacing widget, or by using the excellent PresentMon tool from Intel. The diagrams were created using SankeyMATIC. A simplification that has been made in the diagrams is that even with MPOs enabled a game can still be ...
Model-View-Controller (MVC) is a software architecture [1], currently considered as an architectural pattern used in software engineering. The pattern isolates "domain logic" (the application logic for the user) from input and presentation (GUI), permitting independent development, testing and maintenance of each.
0. The presentation layer delivers information to the application layer for display. The presentation layer, in some cases, handles data translation to allow use on a particular system. The user interface shows you the data once the presentation layer has done any translations it needs to.
OpenAI on Monday announced its latest artificial intelligence large language model that it says will be easier and more intuitive to use. The new model, called GPT-4o, is an update from the ...
Berlo's model includes a detailed discussion of the four main components of communication and their different aspects. Berlo's model is a linear transmission model of communication. It was published by David Berlo in 1960 and was influenced by earlier models, such as the Shannon-Weaver model and Schramm's model.
A Presentation Model that references a view generally maintains the synchronization code in the Presentation Model. The resulting view is very dumb. The view contains setters for any state that is dynamic and raises events in response to user actions. The views implement interfaces allowing for easy stubbing when testing the Presentation Model.
OpenAI is reportedly planning to eventually with its own AI-powered search product. But the big news on Monday was OpenAI's new flagship AI model, GPT-4o, which will be free to all users and "can ...
Presentation technology consists of tools used to assist in conveying information during a presentation. [1] When a speaker is verbally addressing an audience, it is often necessary to use supplementary equipment and media to clarify the point. If the audience is large or the speaker is soft-spoken, a public address system may be employed.
4. What are the naming conventions you use for application domain model classes and presentation layer classes? Postfixes/Prefixes to distinguish the aim of the class from the first sight: For example information about the person that is returned from DAL can be. public class PersonEntity. {. public Guid Id { get; set; }
Archived from the original on October 8, 2015. Retrieved August 25, 2017. Microsoft PowerPoint, virtual presentation software developed by Robert Gaskins and Dennis Austin for the American computer software company Forethought, Inc. The program, initially named Presenter, was released for the Apple Macintosh in 1987.
Free presentation. In algebra, a free presentation of a module M over a commutative ring R is an exact sequence of R -modules: Note the image under g of the standard basis generates M. In particular, if J is finite, then M is a finitely generated module. If I and J are finite sets, then the presentation is called a finite presentation; a module ...
Claude. Claude was the initial version of Anthropic's language model released in March 2023, [8] Claude demonstrated proficiency in various tasks but had certain limitations in coding, math, and reasoning capabilities. [9] Anthropic partnered with companies like Notion (productivity software) and Quora (to help develop the Poe chatbot).