
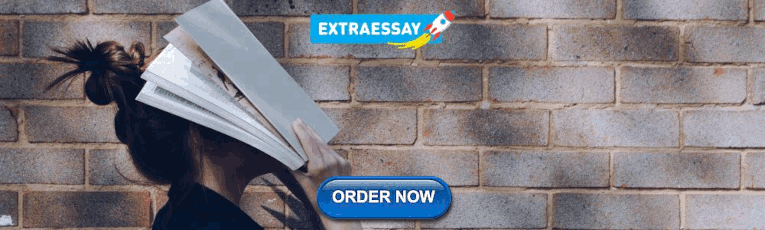
AttributeError: Assignment not allowed to composite field "task" in protocol message object
The error message you're encountering, "AttributeError: Assignment not allowed to composite field 'task' in protocol message object," typically occurs when you try to directly assign a value to a field of a Protocol Buffers (protobuf) message that is marked as a composite field. Composite fields are fields that have a message type.
In protobuf, you cannot directly assign values to composite fields like you would with simple fields (e.g., integers or strings). Instead, you should create an instance of the composite message type and then set its fields individually before assigning it to the parent message.
Here's an example of how to set a composite field correctly:
In this example, we create instances of both the parent message ( ParentMessage ) and the composite message ( CompositeMessage ). We then set the fields of the composite message ( field1 and field2 ) and finally use CopyFrom to assign the composite message to the parent message's task field.
Make sure to replace your_protobuf_module_pb2 with the actual module generated by protoc for your protobuf definition. Additionally, replace ParentMessage , CompositeMessage , and field names with the actual names from your protobuf definition.
roles matlab jsch change-password primefaces firebase-authentication form-data git-config kendo-ui-grid pkcs#11
More Python Questions
- Making square axes plot with log2 scales in matplotlib
- Django Invalid HTTP_HOST header: 'testserver'. You may need to add u'testserver' to ALLOWED_HOSTS
- Typical AngularJS workflow and project structure (with Python Flask)
- Python: How to get values of an array at certain index positions?
- Tensorflow mean squared error loss function
- Fill cells with colors using openpyxl?
- How to write a confusion matrix in python
- Python: Converting string into decimal number
- Previous
- Next

dynamic generation of proto objects and the error: Assignment not allowed to composite field
Tom Lichtenberg
Anton Danilov
google.protobuf.message ¶
Contains an abstract base class for protocol messages.
Exception raised when deserializing messages.
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
Exception raised when serializing messages.
Base error type for this module.
Abstract base class for protocol messages.
Protocol message classes are almost always generated by the protocol compiler. These generated types subclass Message and implement the methods shown below.
Returns the serialized size of this message.
Recursively calls ByteSize() on all contained messages.
The number of bytes required to serialize this message.
Clears all data that was set in the message.
Clears the contents of a given extension.
extension_handle – The handle for the extension to clear.
Clears the contents of a given field.
Inside a oneof group, clears the field set. If the name neither refers to a defined field or oneof group, ValueError is raised.
field_name ( str ) – The name of the field to check for presence.
ValueError – if the field_name is not a member of this message.
Copies the content of the specified message into the current message.
The method clears the current message and then merges the specified message using MergeFrom.
other_msg ( Message ) – A message to copy into the current one.
The google.protobuf.descriptor.Descriptor for this message type.
Clears all fields in the UnknownFieldSet .
This operation is recursive for nested message.
Checks if a certain extension is present for this message.
Extensions are retrieved using the Extensions mapping (if present).
extension_handle – The handle for the extension to check.
Whether the extension is present for this message.
KeyError – if the extension is repeated. Similar to repeated fields, there is no separate notion of presence: a “not present” repeated extension is an empty list.
Checks if a certain field is set for the message.
For a oneof group, checks if any field inside is set. Note that if the field_name is not defined in the message descriptor, ValueError will be raised.
Whether a value has been set for the named field.
Checks if the message is initialized.
The method returns True if the message is initialized (i.e. all of its required fields are set).
Returns a list of (FieldDescriptor, value) tuples for present fields.
A message field is non-empty if HasField() would return true. A singular primitive field is non-empty if HasField() would return true in proto2 or it is non zero in proto3. A repeated field is non-empty if it contains at least one element. The fields are ordered by field number.
field descriptors and values for all fields in the message which are not empty. The values vary by field type.
list [ tuple ( FieldDescriptor , value)]
Merges the contents of the specified message into current message.
This method merges the contents of the specified message into the current message. Singular fields that are set in the specified message overwrite the corresponding fields in the current message. Repeated fields are appended. Singular sub-messages and groups are recursively merged.
other_msg ( Message ) – A message to merge into the current message.
Merges serialized protocol buffer data into this message.
When we find a field in serialized that is already present in this message:
If it’s a “repeated” field, we append to the end of our list.
Else, if it’s a scalar, we overwrite our field.
Else, (it’s a nonrepeated composite), we recursively merge into the existing composite.
serialized ( bytes ) – Any object that allows us to call memoryview(serialized) to access a string of bytes using the buffer interface.
The number of bytes read from serialized . For non-group messages, this will always be len(serialized) , but for messages which are actually groups, this will generally be less than len(serialized) , since we must stop when we reach an END_GROUP tag. Note that if we do stop because of an END_GROUP tag, the number of bytes returned does not include the bytes for the END_GROUP tag information.
DecodeError – if the input cannot be parsed.
Parse serialized protocol buffer data into this message.
Like MergeFromString() , except we clear the object first.
message.DecodeError if the input cannot be parsed. –
Serializes the protocol message to a binary string.
This method is similar to SerializeToString but doesn’t check if the message is initialized.
deterministic ( bool ) – If true, requests deterministic serialization of the protobuf, with predictable ordering of map keys.
A serialized representation of the partial message.
A binary string representation of the message if all of the required fields in the message are set (i.e. the message is initialized).
EncodeError – if the message isn’t initialized (see IsInitialized() ).
Mark this as present in the parent.
This normally happens automatically when you assign a field of a sub-message, but sometimes you want to make the sub-message present while keeping it empty. If you find yourself using this, you may want to reconsider your design.
Returns the UnknownFieldSet.
The unknown fields stored in this message.
UnknownFieldSet
Returns the name of the field that is set inside a oneof group.
If no field is set, returns None.
oneof_group ( str ) – the name of the oneof group to check.
The name of the group that is set, or None.
str or None
ValueError – no group with the given name exists
Table of Contents
- google.protobuf
- google.protobuf.any_pb2
- google.protobuf.descriptor
- google.protobuf.descriptor_database
- google.protobuf.descriptor_pb2
- google.protobuf.descriptor_pool
- google.protobuf.duration_pb2
- google.protobuf.empty_pb2
- google.protobuf.field_mask_pb2
- google.protobuf.internal.containers
- google.protobuf.json_format
- google.protobuf.message
- google.protobuf.message_factory
- google.protobuf.proto_builder
- google.protobuf.reflection
- google.protobuf.service
- google.protobuf.service_reflection
- google.protobuf.struct_pb2
- google.protobuf.symbol_database
- google.protobuf.text_encoding
- google.protobuf.text_format
- google.protobuf.timestamp_pb2
- google.protobuf.type_pb2
- google.protobuf.unknown_fields
- google.protobuf.wrappers_pb2
Related Topics
- Previous: google.protobuf.json_format
- Next: google.protobuf.message_factory
- Show Source

AttributeError: Assignment not allowed to composite field "task" in protocol message object
The error message you're encountering, "AttributeError: Assignment not allowed to composite field 'task' in protocol message object," typically occurs when you try to directly assign a value to a field of a Protocol Buffers (protobuf) message that is marked as a composite field. Composite fields are fields that have a message type.
In protobuf, you cannot directly assign values to composite fields like you would with simple fields (e.g., integers or strings). Instead, you should create an instance of the composite message type and then set its fields individually before assigning it to the parent message.
Here's an example of how to set a composite field correctly:
In this example, we create instances of both the parent message ( ParentMessage ) and the composite message ( CompositeMessage ). We then set the fields of the composite message ( field1 and field2 ) and finally use CopyFrom to assign the composite message to the parent message's task field.
Make sure to replace your_protobuf_module_pb2 with the actual module generated by protoc for your protobuf definition. Additionally, replace ParentMessage , CompositeMessage , and field names with the actual names from your protobuf definition.
roles matlab jsch change-password primefaces firebase-authentication form-data git-config kendo-ui-grid pkcs#11
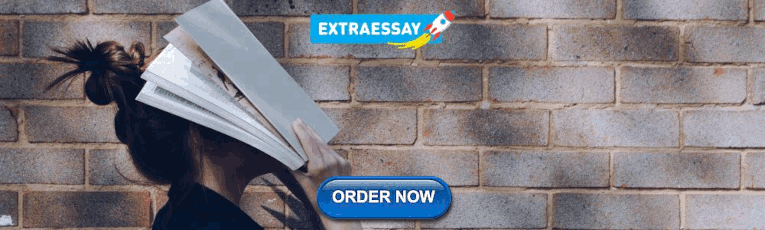
More Python Questions
- Making square axes plot with log2 scales in matplotlib
- Django Invalid HTTP_HOST header: 'testserver'. You may need to add u'testserver' to ALLOWED_HOSTS
- Typical AngularJS workflow and project structure (with Python Flask)
- Python: How to get values of an array at certain index positions?
- Tensorflow mean squared error loss function
- Fill cells with colors using openpyxl?
- How to write a confusion matrix in python
- Python: Converting string into decimal number
- Previous
- Next
python - 属性错误 : Assignment not allowed to composite field "task" in protocol message object
标签 python protocol-buffers
我正在使用protocol-buffers python lib发送数据,但它有一些问题,所以
原型(prototype)文件:
试试 CopyFrom :
关于python - 属性错误 : Assignment not allowed to composite field "task" in protocol message object,我们在Stack Overflow上找到一个类似的问题: https://stackoverflow.com/questions/18376190/
上一篇: Python:我可以有一个带有命名索引的列表吗?
下一篇: python - notepad++ 中 python 的自动缩进.
python - 广播高级索引 numpy
Python subprocess.Popen 作为 Windows 上的不同用户
python - 在 Matplotlib 和 OSX 中使用数学模式和下标时出现错误偏移
xml-serialization - 关于选择不同二进制 xml 工具的建议
go - 使用 dep 时如何正确包含协议(protocol)的 golang protobuf/ptypes?
.net - 我如何在 .net 中使用 SSL 设置 grpc
python - Python 枚举的 Perl 等价物是什么?
python - Python 中的 grep 库输出
c# - 从原始文件生成 C#,反之亦然,解释自定义选项
java - Protocol Buffers : How to parse a . Java 原型(prototype)文件
©2024 IT工具网 联系我们
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Assignment not allowed to repeated field #524
saksham49 commented Nov 1, 2021
BenRKarl commented Nov 2, 2021
Sorry, something went wrong.
techperfect commented Feb 9, 2022
No branches or pull requests
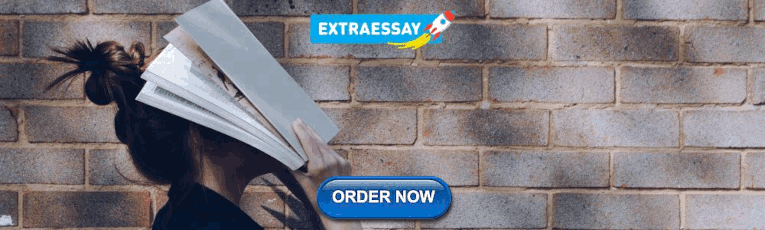
IMAGES
VIDEO
COMMENTS
You cannot assign a value to an embedded message field. Instead, assigning a value to any field within the child message implies setting the message field in the parent. So I'm assuming this should work: task = yacc.task() task.id = 1000 task.msg = u"test" ptask = yacc.task_info() ptask.task.id = task.id ptask.task.msg = task.msg
AttributeError: Assignment not allowed to composite field "id" in protocol message object. Ask Question Asked 5 years, 10 months ago. Modified 5 years, 9 months ago. ... You must either change the field type to a scalar type that maps to a Python int, e.g. int32, or assign an instance of the Identifier message. Share. Improve this answer.
Protobuf python does not support assignment for message field, even it is a normal message field instead of oneof, "test.a =" is still not allowed. "You cannot assign a value to an embedded message field. Instead, assigning a value to any field within the child message implies setting the message field in the parent. " :
Python program to count the pairs of reverse strings; Recursive Functions with Python; ... Assignment not allowed to composite field 'task' in protocol message object," typically occurs when you try to directly assign a value to a field of a Protocol Buffers (protobuf) message that is marked as a composite field. Composite fields are fields ...
Here I get the error: Assignment not allowed to composite field "task" in protocol message object. programatically I can import this module and assign values well enough, for example in the python shell: >> import importlib. >> oobj = importlib.import_module ("dummy.dummy.test_dummy_pb2", package=None)
Environment Python runtime: 3.9.9 OS: MacOS Monterey (version 12.2.1) Arch: M1 (ARM) Steps to reproduce write a python script to test histogram using opentelemetry: from opentelemetry.sdk._metrics....
python - AttributeError: Assignment not allowed to composite field "task" in protocol message object . 0 votes . ... python - AttributeError: Assignment not allowed to composite field "task" in protocol message object . I'm using protocol-buffers python lib to send data,but it's have some problems, so. Traceback (most recent call last): File ...
Contains container classes to represent different protocol buffer types. This file defines container classes which represent categories of protocol buffer field types which need extra maintenance. Currently these categories are: Repeated scalar fields - These are all repeated fields which aren't composite (e.g. they are of simple types like ...
I suppose one is not allowed to assign to repeated fields but I don't see how to proceed. I think appending with request.conversions.append(click_conversion) creates a malformed request. Anyone got a clue on how to get this to work? Thank you for your help
other_msg ( Message) - A message to merge into the current message. Merges serialized protocol buffer data into this message. When we find a field in serialized that is already present in this message: If it's a "repeated" field, we append to the end of our list. Else, if it's a scalar, we overwrite our field.
PYTHON : AttributeError: Assignment not allowed to composite field "task" in protocol message objectTo Access My Live Chat Page, On Google, Search for "hows ...
get the error: Assignment not allowed to composite field "task" in protocol message object. programatically I can import this module and assign values well enough, for
In protobuf, you cannot directly assign values to composite fields like you would with simple fields (e.g., integers or strings). Instead, you should create an instance of the composite message type and then set its fields individually before assigning it to the parent message. Here's an example of how to set a composite field correctly:
AttributeError: Assignment not allowed to repeated field "geo_target_constants" in protocol message object. Can anyone tell me how i can fix this "Assignment not Allowed to repeated field" error? Thanks!
那么,在python中应该怎么赋值呢? 错误示例: search_service. uid = 0. 如果还是和之前一样的赋值,就会报错. AttributeError: Assignment not allowed to repeated field "uid" in protocol message object. 正确示例: search_service. uid. append (1) search_service. uid. append (2)
With Python 3.8 Assignment Expressions have been introduced, allowing to assign values in conditionals and lambdas as such: if x := True: print(x) However it appears this does not extends to attribute assignment, as trying to do something like this. from typing import NamedTuple.
Milestone. No milestone. Development. No branches or pull requests. 1 participant. I have this line in my proto: repeated map<string, string> dependencies = 4; I get this error: Field labels (required/optional/repeated) are not allowed on map fields.
Traceback (most recent call last): File "test_message.py", line 17, in <module> ptask.task = task File "build\bdist.win32\egg\google\protobuf\internal\python_message.py", line 513, in setter AttributeError: Assignment not allowed to composite field "_task" in protocol message object. src如下: 原型(prototype)文件:
What is your question? I was trying to Generate Keyword Ideas by using Google Ads API and I was following this post. However, on running the code I received the following error: Traceback (most recent call last): File ".\keywords.py", li...