Get full access to Data Structures and Algorithms in Java and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
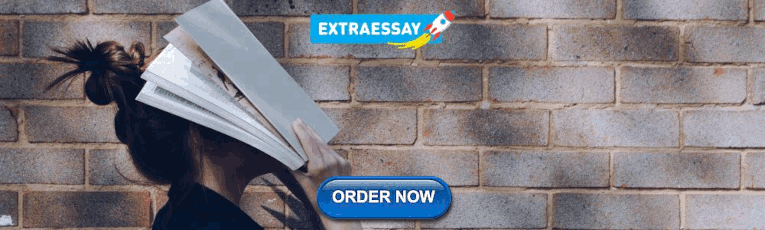
Data Structures and Algorithms in Java
Read it now on the O’Reilly learning platform with a 10-day free trial.
O’Reilly members get unlimited access to books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Book description
Part I: Object-Oriented Programming Part II: Linear Structures Part III: Algorithms Part IV: Trees and Sets Part V: Advanced Topics Part VI: Appendices
Table of contents
- Contents (1/2)
- Contents (2/2)
- 1.1 Software Development
- 1.2 Classes and Objects (1/2)
- 1.2 Classes and Objects (2/2)
- 1.3 Using Objects (1/3)
- 1.3 Using Objects (2/3)
- 1.3 Using Objects (3/3)
- 2.1 Reference Types
- 2.2 Arrays (1/3)
- 2.2 Arrays (2/3)
- 2.2 Arrays (3/3)
- 2.3 Interfaces
- 2.4 Overloading
- 3.1 Extending a Class
- 3.2 The Object Class
- 3.3 Packages and Access Levels
- 4.1 The Stack Interface
- 4.2 The Call Stack
- 4.3 Exceptions (1/2)
- 4.3 Exceptions (2/2)
- 4.4 The Queue Interface (1/2)
- 4.4 The Queue Interface (2/2)
- 5.1 Shrinking and Stretching Arrays
- 5.2 Implementing Stacks and Queues (1/2)
- 5.2 Implementing Stacks and Queues (2/2)
- 5.3 The List Interface (1/2)
- 5.3 The List Interface (2/2)
- 5.4 Iterators (1/3)
- 5.4 Iterators (2/3)
- 5.4 Iterators (3/3)
- 5.5 The Java Collections Framework: A First Look
- 6.1 List Nodes
- 6.2 Stacks and Queues (1/2)
- 6.2 Stacks and Queues (2/2)
- 6.3 The LinkedList Class (1/2)
- 6.3 The LinkedList Class (2/2)
- 6.4 The Java Collections Framework Revisited
- 7.2 Asymptotic Notation (1/2)
- 7.2 Asymptotic Notation (2/2)
- 7.3 Counting Steps
- 7.4 Best, Worst, and Average Case
- 7.5 Amortized Analysis
- 8.1 Linear Search
- 8.2 Binary Search
- 8.3 Insertion Sort
- 8.4 The Comparable Interface
- 8.5 Sorting Linked Lists
- 9.1 Thinking Recursively
- 9.2 Analyzing Recursive Algorithms
- 9.3 Merge Sort
- 9.4 Quicksort
- 9.5 Avoiding Recursion
- 10.1 Binary Trees
- 10.2 Tree Traversal
- 10.3 General Trees (1/2)
- 10.3 General Trees (2/2)
- 11.1 The Set Interface
- 11.2 Ordered Lists
- 11.3 Binary Search Trees (1/2)
- 11.3 Binary Search Trees (2/2)
- 11.4 Hash Tables (1/3)
- 11.4 Hash Tables (2/3)
- 11.4 Hash Tables (3/3)
- 11.5 The Java Collections Framework Again
- 12.1 Bit Vectors
- 12.2 Sparse Arrays
- 12.3 Contiguous Representation of Multidimensional Arrays (1/2)
- 12.3 Contiguous Representation of Multidimensional Arrays (2/2)
- 12.4 Advanced Searching and Sorting
- 13.1 Strings and StringBuilders
- 13.2 String Matching (1/3)
- 13.2 String Matching (2/3)
- 13.2 String Matching (3/3)
- 14.2 Disjoint Set Clusters (1/2)
- 14.2 Disjoint Set Clusters (2/2)
- 14.3 Digital Search Trees (1/2)
- 14.3 Digital Search Trees (2/2)
- 14.4 Red-Black Trees (1/3)
- 14.4 Red-Black Trees (2/3)
- 14.4 Red-Black Trees (3/3)
- 15.1 Terminology
- 15.2 Representation (1/2)
- 15.2 Representation (2/2)
- 15.3 Graph Traversal
- 15.4 Topological Sorting
- 15.5 Shortest Paths
- 15.6 Minimum Spanning Trees
- 16.1 Explicit Memory Management
- 16.2 Automatic Memory Management (1/2)
- 16.2 Automatic Memory Management (2/2)
- 17.1 Interacting with Files
- 17.2 Compression (1/3)
- 17.2 Compression (2/3)
- 17.2 Compression (3/3)
- 17.3 External Sorting (1/2)
- 17.3 External Sorting (2/2)
- 17.4 B-Trees (1/4)
- 17.4 B-Trees (2/4)
- 17.4 B-Trees (3/4)
- 17.4 B-Trees (4/4)
- A.1 The First Program
- A.2 Variables and Types
- A.4 Interacting with the User
- A.5 Branching
- A.6 Methods and Breaking Out
- A.7 Constants
- A.8 Operators
- A.9 Debugging
- A.10 Coding Conventions
- B.1 Class Diagrams
- B.2 Instance Diagrams
- C.1 Sum Notation
- C.2 Sum of Constants
- C.3 Sum of First n Integers
- C.4 Sums of Halves and Doubles
- C.5 Upper Limit on Sum of a Function
- C.6 Constant Factors
- D.1 Data Structures and Algorithms
Product information
- Title: Data Structures and Algorithms in Java
- Author(s): Peter Drake
- Release date: May 2024
- Publisher(s): Pearson India
- ISBN: 9781282650404
You might also like
Data structures & algorithms in python.
by John Canning, Alan Broder, Robert Lafore
LEARN HOW TO USE DATA STRUCTURES IN WRITING HIGH PERFORMANCE PYTHON PROGRAMS AND ALGORITHMS This practical …
Advanced Algorithms and Data Structures
by Marcello La Rocca
As a software engineer, you’ll encounter countless programming challenges that initially seem confusing, difficult, or even …
Learning Java
by Marc Loy, Patrick Niemeyer, Daniel Leuck
Ideal for working programmers new to Java, this best-selling book guides you through the language features …
Java Coding Problems
by Anghel Leonard
Develop your coding skills by exploring Java concepts and techniques such as Strings, Objects and Types, …
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
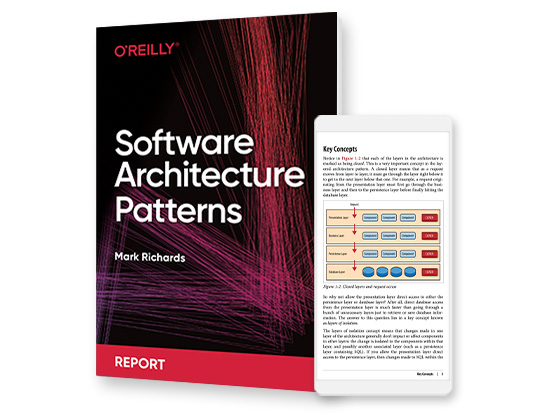
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
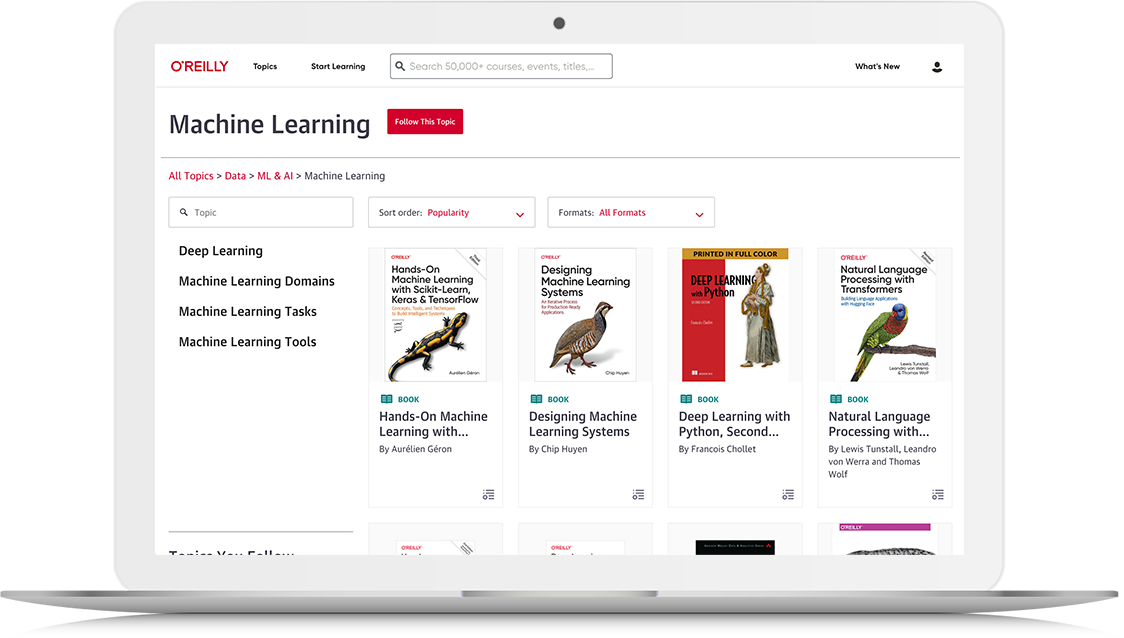
- Buy CP4 - Book 1
- Buy CP4 - Book 2
Competitive Programming
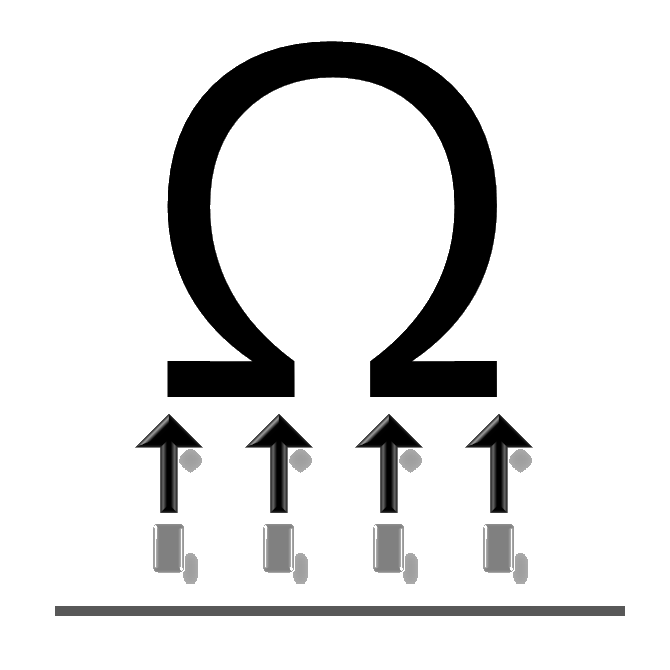
Breaking News (01 February 2023)

One of the authors of Competitive Programming book is part of the launch of the new Centre for Nurturing Computing Excellence (CeNCE)
Breaking News (19 August 2022)
Finally, after two years and one month of exclusive printed-book only (19 July 2020-19 August 2022), we (CP4 book authors, Felix Halim, Suhendry Effendy) have decided to sell the (same) PDF source as eBook. There is no edit compared to the version published in 2020 (i.e., these two PDFs are the original version published back in 19 July 2020), but all known errata has been listed at Errata section.
So if your country/region does not have access to lulu distribution centers or if the shipping cost is too much to bear or if the printed font size is too small for your liking (but you have Internet access and credit card), then pick these alternative options:
- Buy Book 1 (eBook/PDF)
- Buy Book 2 (eBook/PDF)
PS: The eBook versions are 2 USD cheaper than the printed versions.
This is the companion web site for a book: "Competitive Programming 4: The Lower Bound of Programming Contests in the 2020s" (click here for details) and its three past editions written by myself ( Steven Halim - UVa status , Kattis status , and also Clist open kattis status ), my younger brother ( Felix Halim - UVa status , Kattis status ), and my friend (Suhendry Effendy - UVa status , Kattis status ) in 2010 - present. This book is used in my course in School of Computing , National University of Singapore : CS3233 - Competitive Programming (2009-present) and available for wider audiences since 2010. This book contains a collection of competitive programming skills gained by solving more than 3500+ UVa (1999-present) plus Kattis (2015-present) online judge problems and being involved in the ICPCs (since 2001) and the IOIs (since 2009) in various roles: contestants (long ago), coaches, judges, ICPC Regional Contest Director (2015, 2018), and/or IOI International Committee member (2020-2021).
Our objective in writing this book is similar with the IOI objective+ICPC vision: to further improve humanity by training current students to be more competitive in programming contests. The possible long term effect is future Computer Science researchers who are well versed in problem solving skills. We use C++ (primary), Python (secondary), Java (tertiary), and OCaml (optional) code to illustrate the algorithmic concepts, i.e., we dislike vague pseudo-code commonly found in many other Computer Science textbooks. We also built and heavily use our-own visualization tool: VisuAlgo to help explain the data structure and algorithm concepts to our book readers and beyond.
Target Audiences
This Competitive Programming book, 4th edition (CP4) is a must have for every competitive programmer. Mastering the contents of this book is a necessary (but admittedly not sufficient) condition if one wishes to take a leap forward from being just another ordinary coder to being among one of the world's finest competitive programmers.
- Secondary or High School Students who are competing in the annual International Olympiad in Informatics (IOI) (including the National or Provincial Olympiads) as Book 1 covers most of the current IOI Syllabus,
- Casual University students who are using this book as supplementary material for typical Data Structures and Algorithms courses,
- Anyone who wants to prepare for typical fundamental data structure/algorithm part of a job interview at top IT companies.
- University students who are competing in the annual International Collegiate Programming Contest (ICPC) Regional Contests (including the World Finals) as Book 2 covers much more Computer Science topics that have appeared in the ICPCs,
- Teachers or Coaches who are looking for comprehensive training materials,
- Anyone who loves solving problems through computer programs. There are numerous programming contests for those who are no longer eligible for ICPC, including Google CodeJam, Facebook Hacker Cup, TopCoder Open, CodeForces contest, Internet Problem Solving Contest (IPSC), etc.
Forewords for CP4
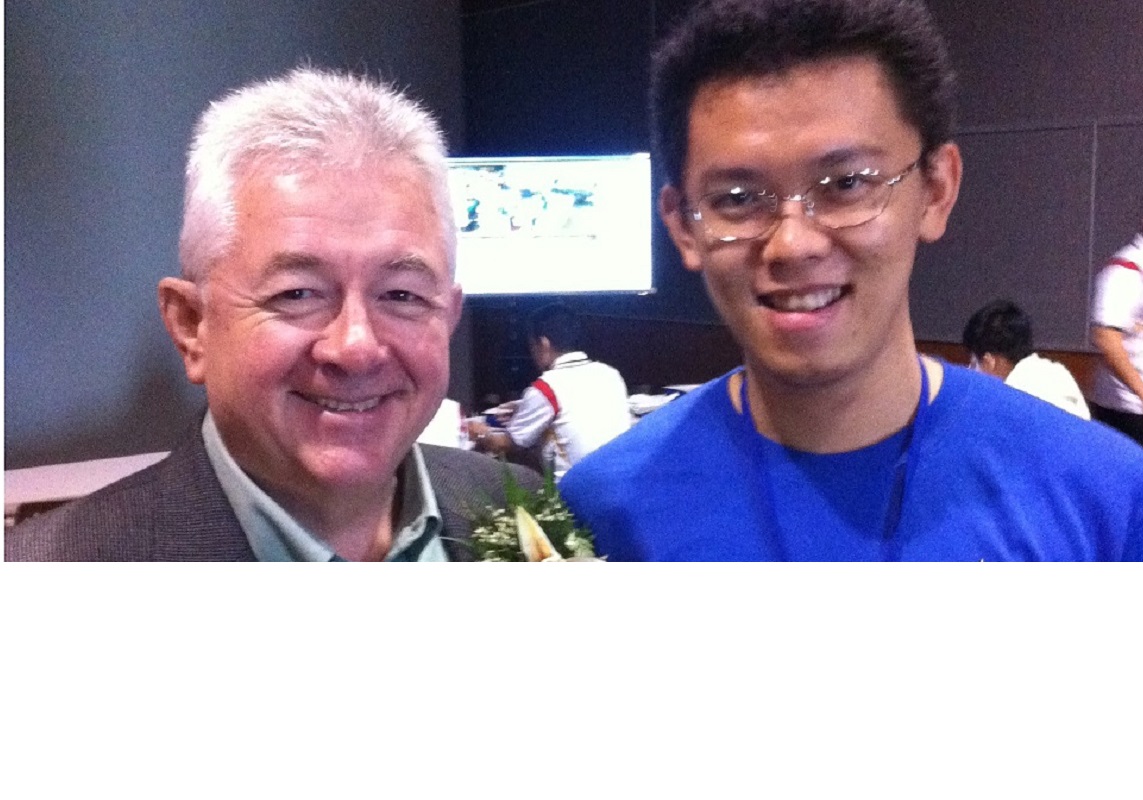
"The authors are seasoned competitive programming experts who have dedicated decades of work to help at all levels of the sport." — Bill Poucher , ICPC Executive Director and President of ICPC Foundation Read
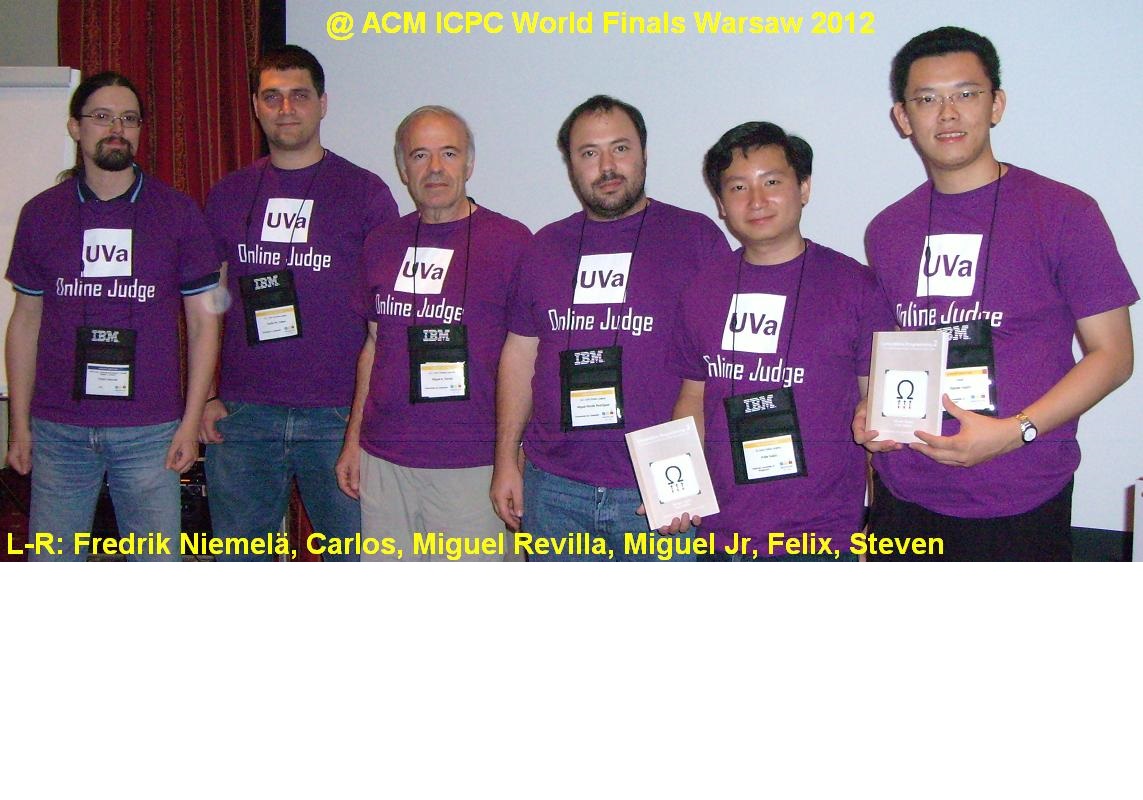
"Steven, Felix, Suhendry in the past two decades, have grown from contestants, to coaches and, finally, masters in the art of competitive programming." — Miguel Revilla Rodríquez , (UVa) Online Judge Manager Read
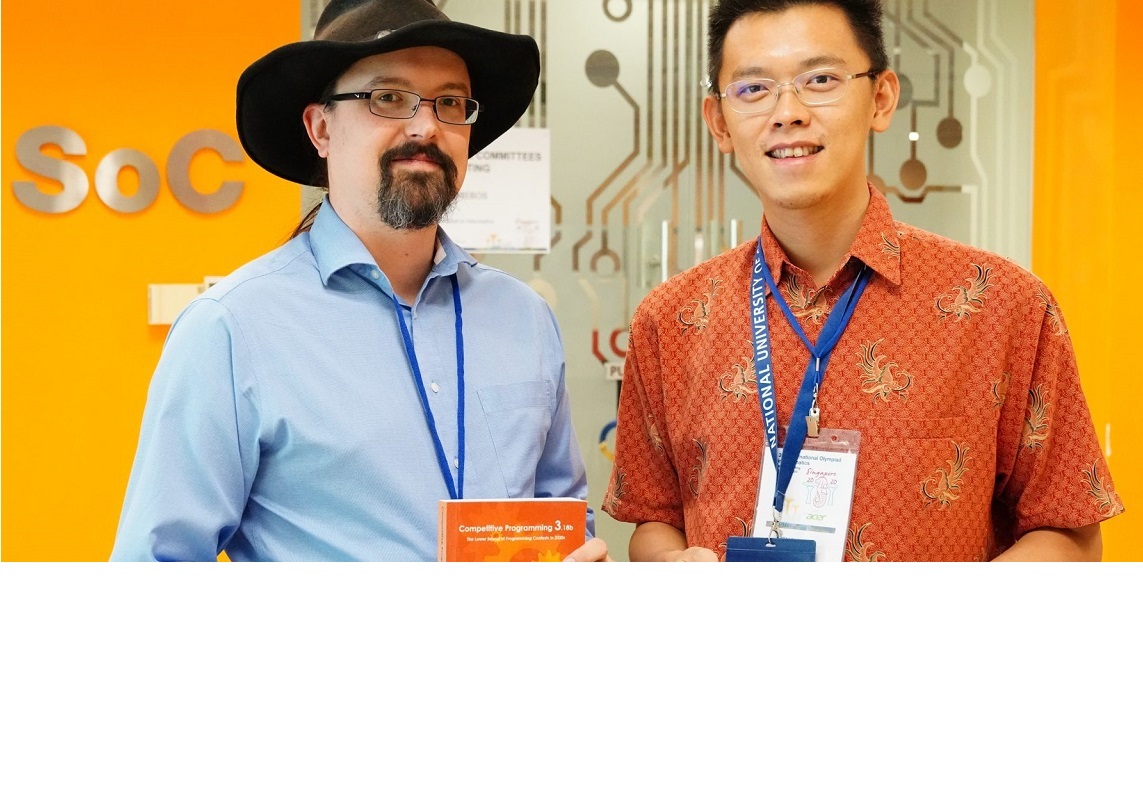
"'Competitive Programming' and Kattis share this motivating principle: to make learning computer science and programming accessible for everyone." — Fredrik Niemelä , Founder of Kattis Read
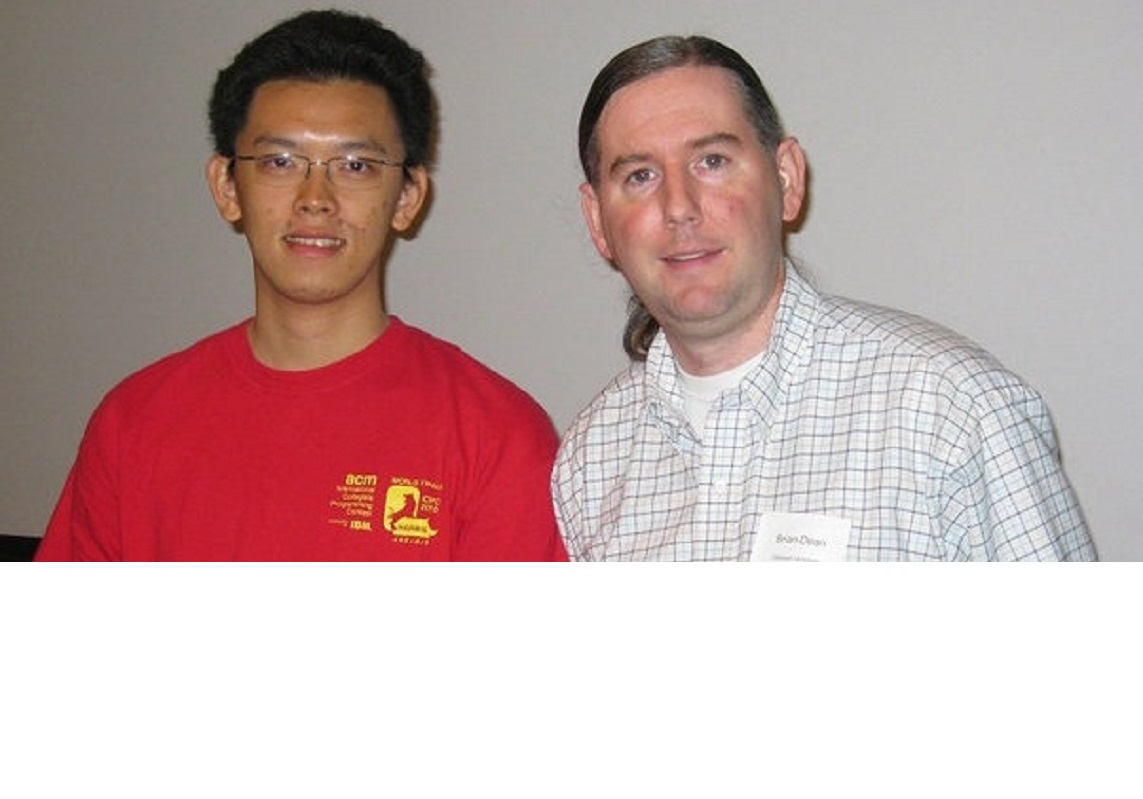
"Both academia and industry are now filled with present-day superstars who were formerly superstars in competitive programming." — Brian Christopher Dean , Director, USA Computing Olympiad Read
Selected Testimonials for CP1/2/3
"Competitive Programming 3 has contributed immensely to my understanding of data structures & algorithms. Steven & Felix have created an incredible book that thoroughly covers every aspect of competitive programming, and have included plenty of practice problems to make sure each topic sinks in. Practicing with CP3 has helped me nail job interviews at Google, and I can't thank Steven & Felix enough!"
"Steven and Felix are passionate about competitive programming. Just as importantly, they are passionate about helping students become better programmers. CP3 is the result: a dauntless dive into the data structures, algorithms, tips, and secrets used by competitive programmers around the world. Yet, when the dust settles on the book, the strongest sillage is likely to be one of confidence---that, yes, this stuff is challenging, but that you can do it."
"CP-Book helped us to train many generations of ICPC and IOI participants for Bolivia. It's the best source to start and reach a good level to be a competitive programmer."
"Reading CP3 has been a major contributor to my growth, not just as a competitive programmer, but also as a computer scientist. My entire approach to problem solving has been improved by doing the exercises in the book; my passion for the art of problem solving, especially in contest environments, has been intensified. I now mentor several students using this book as a guide. It is an invaluable resource to anyone who wants to be a better problem solver."
"I rediscovered CP3 book on 2017-2019 when I come back to Peru after my master in Brazil, I enjoyed, learned and solved many problems, more than during my undergraduate, coaching and learning together in small group of new students that are interesting in competitive programming. It kept me in a constantly competition with them, at the end they have solved more problems than me."
"CP1 helped my preparation during national team training and selection for participating the IOI. When I took the competitive programming course in NUS, CP2 book is extensively used for practice and homework. The good balance between the programming and theoretic exercises for deeper understanding in the book makes CP book a great book to be used for course references, as well as for individual learning. Even at the top competitive programming level, experts can still learn topics they have not learnt before thanks to the rare miscellaneous topics at the end of the book."
"Dr. Steven Halim is one of the best professors I have had in NUS. His intuitive visualizations and clear explanations of highly complex algorithms make it significantly easier for us to grasp difficult concepts. Even though I was never fully into Competitive Programming, his book and his teaching were vital in helping me in job interviews and making me a better coder. Highly recommend CP4 to anyone looking to impress in software engineering job interviews."
"Flunked really hard at IOI 2017, missing medal cutoff by 1 place. Then at the beginning of 2018 Steven Halim gave me a draft copy of CP3.1 / CP4 and I ended up getting a gold medal!"
"As a novice self-learner, CP-book helped me to learn the topics in both fun and challenging ways. As an avid and experienced CP-er, CP-book helped me to find a plentiful and diverse problems. As a trainer, CP-book helped me to plan ahead the materials and tactical strategies or tricks in competition for the students. As the person ever in those three different levels, I must effortlessly say CP-book is a must-have to being a CP master!"
"I've been in CP for three years. A rookie number for all the competitive programmers out there. I have a friend (still chatting with him today) who introduced me to this book. He's my roommate on our National Training Camp for IOI 2018's selection. I finally get a grab of this book in early 2019. To be honest I'm not the 'Adhoc' and good at 'Math' type of CP-er. I love data structures, graph (especially trees) And this CP3 book. Is a leap of knowledge. No joke. I met Dr Felix when I was training in BINUS, I also met Dr Steven when I competed in Singapore's NOI and one of my unforgettable moment is, this legend book got signed by its two authors. Even tho the book is full of marks and stains, truly one of my favorite. Kudos for taking me to this point of my life."
"I bought CP3 on 7th April 2014 on my birthday as a gift for myself and it has been the most worth-it 30USD spent by me on any educational material. In the later years, I was able to compete in IOI and ICPC WF. I think CP3 played a very big factor in igniting the interest and providing a strong technical foundation about all the essential topics required in CP."
"I have always wanted to get involved in competitive programming, but I didn't know how and where to get started. I was introduced to this book while taking Steven's companion course (CS3233) in NUS as an exchange student, and I found the book to be really helpful in helping me to learn competitive programming. It comes with a set of Kattis exercises as well. This book provides a structured content for competitive programming, and can be really useful to anyone ranging from beginners to experts. Just like CLRS for algorithms, CP is THE book for competitive programming."
"My memories about CP3 is me reading it in many places, the bus, my room, the library, the contest floor...not much time had passed since I start in competitive programming reading CP3 until I got qualified to an ICPC World Final"
"My name is Alisia Maria Lupidi and I am an ICPC contestant. I take part in the SWERC and this month (July 2020) I won the gold medal at the Girls ICPC ACPC. My boyfriend, who is a bronze medalist at the SWERC, gave me the CP4 yesterday (30 July) as a present for my birthday (soon). I would like you to know how important your work is for us contestants and to thank you for writing the best birthday present ever!"
Authors' Profiles
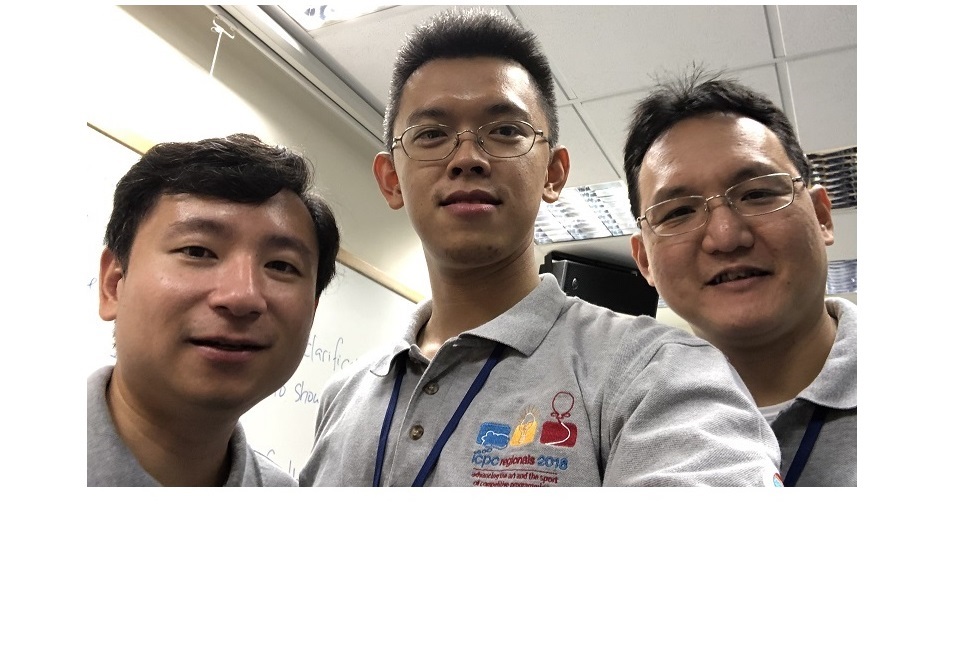
Steven Halim is a Senior Lecturer in the National University of Singapore Read
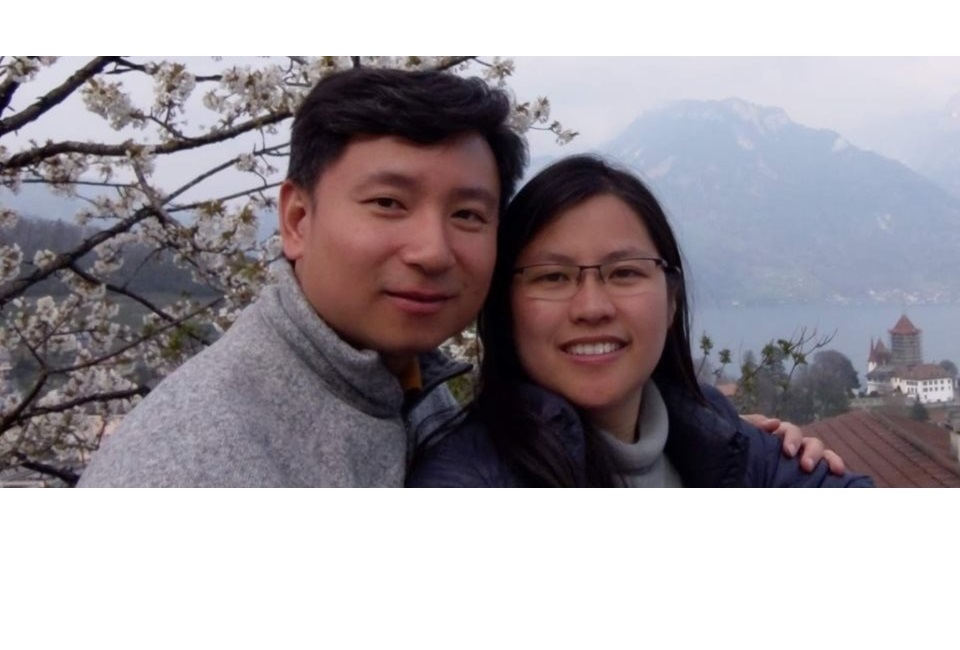
Felix Halim is a Senior Software Engineer at Google, Mountain View, USA Read
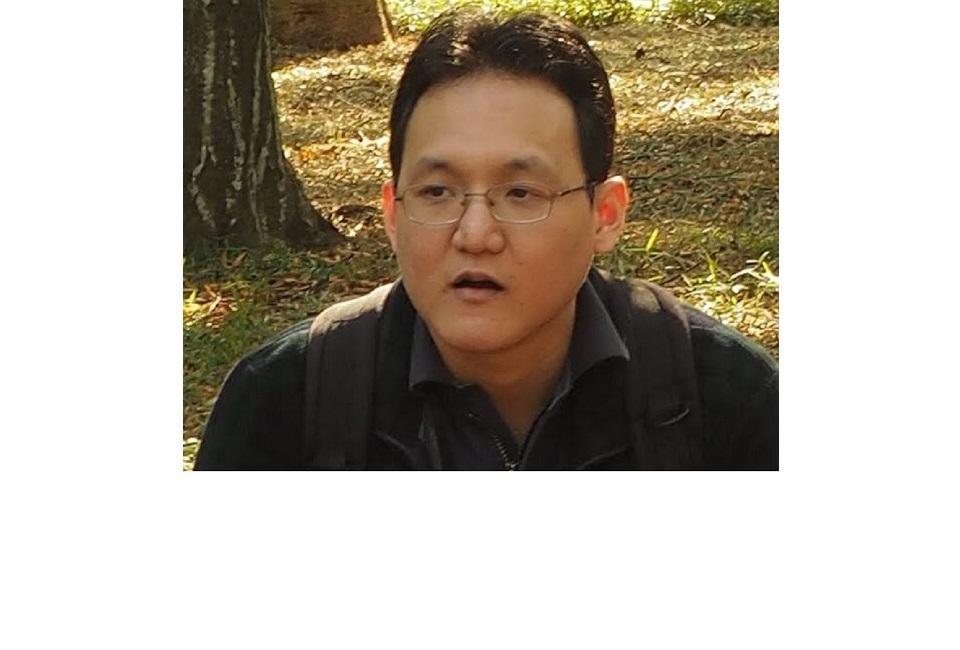
Suhendry Effendy is a Research Fellow in the National University of Singapore Read
Important News
Friday, 11 June 2021: After 1.5 more years of additional translations by Miguel Revilla Rodriquez (since 01 January 2020), CP4 Book 1 and 2 are now available in Spanish language. Here are the Amazon Book 1 link and Amazon Book 2 link .
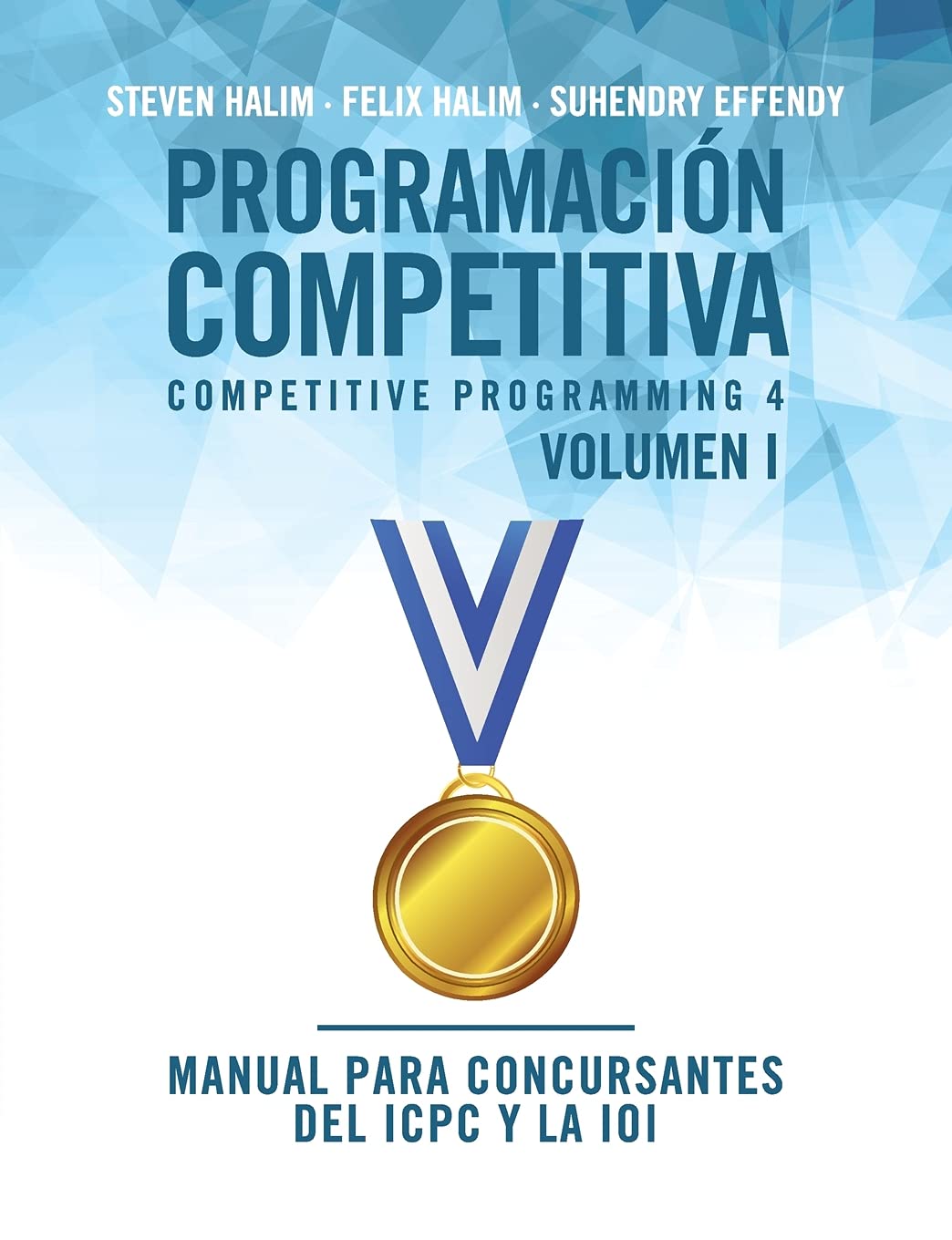
Wednesday, 01 January 2020: CP3 (2013 edition with a bit of 2018 upgrade) is now available in Spanish language. If you are a Spanish-speaking programmer, we recommend that you get the Spanish version that has been translated over the past 1+ year by Miguel Revilla Rodriquez (the current admin of (UVa) Online Judge). Here is the Amazon Link, ISBN: 978-1711024813 . [Updated remarks on 11 June 2021]: Now that CP4 version is fully available in Spanish, I suggest that you go for the latest version :).
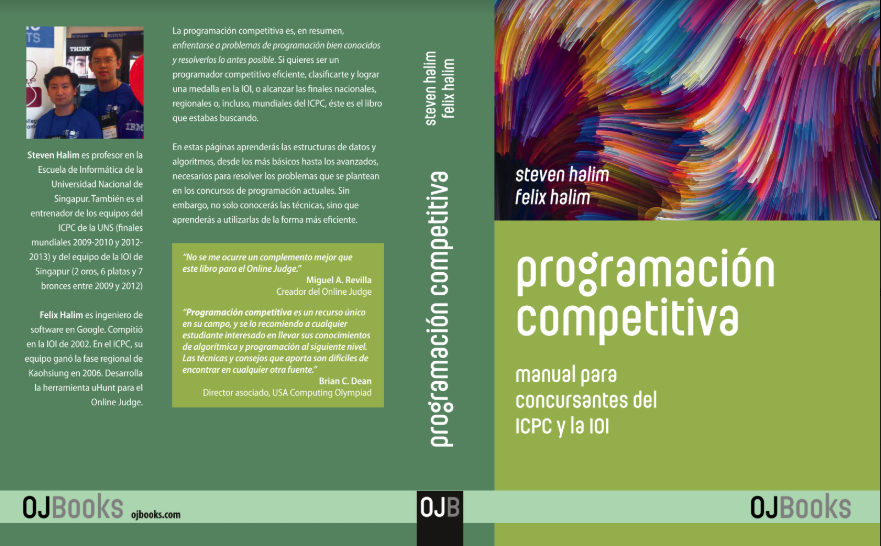
Tuesday, 24 October 2017: CP3 is now available in Korean language. If you are Korean, we recommend that you get the Korean version that has been translated over the past 1+ year by lewha0 . Here is the publisher link: Insight Book, Korea .
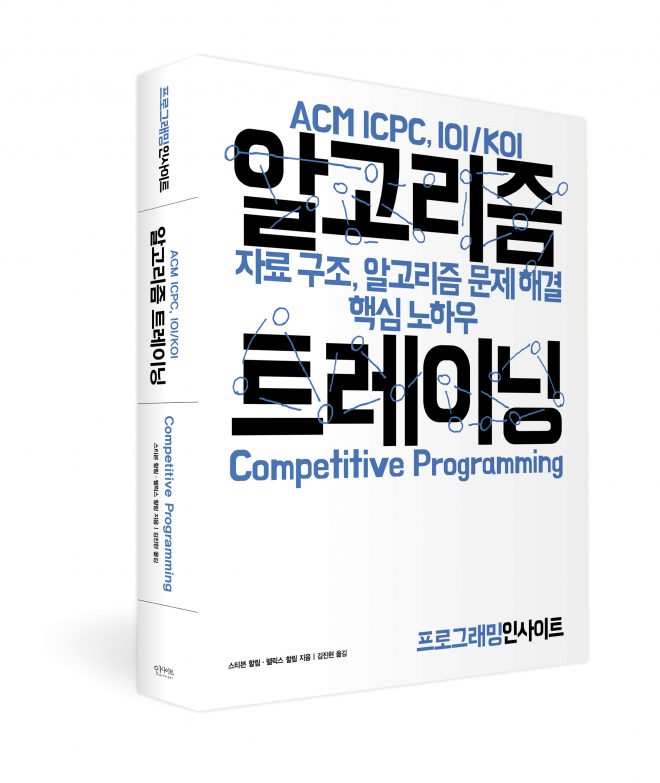
Foreword by Bill Poucher, ICPC Executive Director and President of ICPC Foundation
Introduction.
In 1970, the Texas A&M UPE Honor Society hosted the first university competitive programming competition in the history of the ICPC. The first Finals was held in 1977 in Atlanta in conjunction with the Winter Meeting of the ACM Computer Science Conference. The ICPC International Collegiate Programming Contest hosted regional competitions at 643 sites in 104 countries for 59,000 team members and their 5043 coaches from over 3400 universities that span the globe. The top 135 teams of three will advance to the ICPC World Finals in Moscow hosted by MIPT scheduled for June 2021.
ICPC alumni number over 400,000 worldwide, many playing key roles in building the global digital community for many decades. The ICPC is the root of competitive programming that reaches out through the global digital community to persons from all cultures and in increasingly-younger generations.
The UVa Online Judge opened the doors for online competition and access to ICPC problems under the direction of Professor Miguel Ángel Revilla. Three of the star-studded team are Steven Halim, Felix Halim, and Suhendry Effendy, authors of Competitive Programming 4, Book 1 and Book 2. Their work will be honored at the ICPC World Finals in Moscow hosted by MIPT with a special award from the ICPC Foundation.
What is competitive programming and why should you get involved? First and foremost, it's a mind sport. It more fully develops your algorithmic reasoning skills and bridges the gap between theory and application in bite-sized chunks. Full participation develops problem-solving intuition and competence. Get ready for the Digital Renaissance that will shape your world in the coming decades. To understand the landscape, it is important to shape your mind beyond a swarm of buzzwords. Do it as a team sport.
How do we get started?
Start with Competitive Programming 4, Book 1 and Book 2. Start with Book 1 first :). The authors are seasoned competitive programming experts who have dedicated decades of work to help at all levels of the sport.
In parallel, engage in a culture that develops habits excellence. You are the first generation that has never been disconnected. Being connected is best when we bind our strengths together in common cause. Do that and prepare to meet the challenges that will define your generation.
Life needs you. We are born to compete. We compete best when we compete together, in good faith, in goodwill, and with good deeds. When you come to college, consider the ICPC and the new program ICPC University Commons that will provide a spectrum of activities that happen outside of the classroom. You can visit https://icpc.global for details.
Why get started?
Is developing your problem-solving skills important? Yes. Is preparing for a future engaged in the global digital community important? Yes. Is following T.S. Elliot's advice that to fully develop you must go too far? Yes. Do that in competitive programming. Be careful of pursuits that are not reversible.
Is competitive programming practical? Aristotle asserted that there is nothing more practical than engaging in mental activities and reflections which have their goal in themselves and take pace for their own sake. Let me recommend that you engage your spirit in building a more beautiful world. In the immense scope of life, abundant small kindnesses make a difference. Find friends with common interest and embrace this cycle: "Repeat for a lifetime: Study; Practice; Rehearse; Dress Rehearse; Perform."
It works for athletes. It works for musicians. It works for all performance arts. It will work for you.
Foreword by Miguel Revilla Rodríquez, (UVa) Online Judge Manager
Almost 20 years ago (on November 11th, 2003, to be precise), my father (Miguel Ángel Revilla) received an e-mail with the following message: "I should say in a simple word that with the UVa Site, you have given birth to a new CIVILIZATION and with the books you write (he meant "Programming Challenges: The Programming Contest Training Manual", coauthored with Steven Skiena), you inspire the soldiers to carry on marching. May you live long to serve the humanity by producing super-human programmers."
What, in my father's words, was "clearly an exaggeration" , caused some thinking. And it's not a secret that thoughts can easily lead to dreams. His dream was to create a community around the project he had started, as part of his teaching job at the University of Valladolid, Spain, that gathered people from all around the world working together towards the same ideal, the same quest. With a little searching, on the primitive Internet of the first years of our century, a whole online community of excellent users and tools, built around the UVa site, came to light.
The website Methods to Solve , created by a very young student from Indonesia, was one of the most impressive among them. There was the result of the hard work of a real genius of algorithms and computer science. The seed was planted to believe that the dream could come true. Moreover, it was not only that the leaves of that growing tree were a perfect match, but the root of both projects were exactly the same: to serve the humanity. That young student, the author of the e-mail and the website that put my father to dream, was Steven Halim. Later he would discover that Steven was not alone in his quest, as his younger brother, Felix, shared his view, his interests, and his extraordinary capabilities.
After 15 years of fruitful collaboration and, more important, friendship with Steven and Felix, my father sadly passed away in 2018. His work, and his dreams, now belong to us, the next generation. This book is the living proof that the dream has become true.
"I can't imagine a better complement for the UVa Online Judge" , are my father's words. Now, with this fourth version of Competitive Programming in my hands, I can add that I can't imagine the very existence of the Online Judge without this book. Both projects have grown in parallel and are, no doubt, perfect complements and companions to each other. By practicing and mastering most programming exercises in this book, the reader can learn how to solve hundreds of tasks and find a place in the top 500 best Online Judge coders. You have in your hands over 2000 (yes, two thousand!) selected, classified, and carefully commented problems from the Online Judge.
The authors, in the past two decades, have grown from contestants, to coaches and, finally, masters in the art of competitive programming. They perfectly know every curve and crossroad in that long path, and they can put themselves in the skins of the young IOI contestant, the ICPC newcomer or the seasoned coach, speaking to each in their own language. This book is, for that very reason, the perfect reading for all of them. No matter if you are starting as a competitive programmer in your local IOI, or are coaching in the next ICPC World Finals, no doubt this IS the book for you.
I love movies, I adore classic movies, and I know that what I'm watching is a masterpiece, when, after the film ends, I can't wait to start all over again. In Steven and Felix own words "the book is not meant to be read once, but several times" . And you will find that same feeling, not only because the authors recommend it, but because you will be anxious to read and re-read it as, like in the greatest movies, you will find something new and amazing each time. This book is, by that logic, a masterpiece.
I also have the great honor of being the Spanish language translator of this book. Translating requires a very meticulous process of converting the words while keeping the spirit. You have to think as the author would think, and have to perfectly understand not only what the author is saying, but also what the author is meaning. It is a handcrafting exercise. Having gone forth and back through this text hundreds of times, I have enjoyed every concept, every new idea, and every tip, not only by what is written in it, but also by what it wants to achieve. The quest of making better programmers and, behind that, the quest of serving humanity. This book is, indeed, a truly masterpiece.
Once you've read this book several times, you will realize how much a better programmer you are but, believe it or not, you will realize that you are also a happier person.
Foreword by Fredrik Niemelä, Founder of Kattis
I got my first physical copy of this book from Steven at IOI 2012 in Italy. Like so many other computer scientists, he has a great sense of humor, and named it "Competitive Programming: Increasing the Lower Bound of Programming Contests." It was the second edition of the book and already twice the size of the first edition. Packed with practical advice, it was well-suited to get beginners started and had useful material for the more seasoned algorithmist.
Steven and Felix's vision for their book was to teach everybody how to program (As Gusteau from Ratatouille would put it: "Tout le monde peut programmer"). I had a similar vision, but instead of writing a book, we created Kattis. "Competitive Programming" and Kattis share this motivating principle: to make learning computer science and programming accessible for everyone. In that sense, they are like two of many pieces in the same puzzle.
Kattis is an online tool for teaching computer science and programming, which relies on a curated library of programming tasks. I managed to convince Steven that he should try using Kattis for some of his teaching activities. Over the years he has moved from using Kattis, to pushing us to improve Kattis, to adding high-quality content to Kattis.
From years of teaching algorithms and using similar systems that preceded Kattis, we learned that the quality of the problems, and their absolute correctness, are paramount for learning outcomes. So, this is where we put extra effort into Kattis. (If you ever felt that it's too much work to add problems to Kattis, this is why). What we did back then is now standard practice---both the ICPC and IOI use the same kinds of methods for their finals.
In this fourth edition (more than twice as large as the second edition!), Steven and Felix, now joined by co-author Suhendry, are using problems from Kattis. We are honored to be included. Finally, our puzzle pieces are directly connected, and I am very excited about that.
I hope you will find this book informative and helpful and that you will spend the time it asks of you. You will not be disappointed.
Foreword by Brian Christopher Dean, Director, USA Computing Olympiad
I've had the privilege to be part of the competitive programming world for more than three decades, during which time I've seen the field grow substantially in terms of its impact on modern computing. As director of the USA Computing Olympiad and coach of my University's ICPC teams, I have seen firsthand how competitive programming has become a key part of the global computing talent pipeline - both academia and industry are now filled with present-day superstars who were formerly superstars in competitive programming.
Just as the world of competitive programming has shown tremendous growth in scope, depth, and relevance, so too has this text, now in its fourth edition. Earlier editions of this book provided what I consider to be the gold standard for both an introduction and a thorough reference to the algorithmic concepts most prevalent in competitive programming. The same remains true for this edition.
Competitive programming can be a daunting undertaking for the novice student - learning to code is plenty challenging by itself, and on top of this we add a layer of "standard" algorithms and data structures and then another layer of problem-solving insight and tricks. This text helps the introductory student navigate these challenges in several ways, by its thoughtful organization, extensive practice exercises, and by articulating ideas both in clear prose and code. Competitive programming can also be a daunting prospect for the advanced student due to its rapid pace of evolution - techniques can go from cutting-edge to commonplace in a matter of just a few years, and one must demonstrate not only proficiency but true mastery of a formidable and ever-expanding body of algorithmic knowledge. With its comprehensive algorithmic coverage and its extensive listing of 3458 categorized problems, this text provides the advanced student with years of structured practice that will lead to a high baseline skill level.
I think this is a book that belongs in the library of anyone serious about computing, not just those training for their first or their hundredth programming competition. Ideas from competitive programming can help one develop valuable skills and insight - both in theory and implementation - that can be brought to bear on a wide range of modern computing problems of great importance in practice. Algorithmic problem solving is, after all, truly the heart and soul of computer science! These types of problems are often used in job interviews for a good reason, since they indicate the type of prospective employee who has a skill set that is broadly applicable and that can adapt gracefully to changes in underlying technologies and standards. Studying the concepts in this text is an excellent way to sharpen your skills at problem solving and coding, irrespective of whether you intend to use them in competition or in your other computational pursuits.
I've thoroughly enjoyed reading successive drafts of this updated work shared with me by the authors at recent IOIs, and I commend the authors on the impressive degree to which they have been able extend the scope, clarity, and depth of an already-remarkable text.
Dr Steven Halim, stevenhalim at gmail dot com
Steven Halim is a senior lecturer in the School of Computing, National University of Singapore (SoC, NUS). He teaches several programming courses in NUS, ranging from basic programming methodology, intermediate to hard data structures and algorithms, web programming, and also the 'Competitive Programming' module that uses this book.
He is the coach of both the NUS ICPC teams and the Singapore IOI team. He participated in several ICPC Regionals as a student (Singapore 2001, Aizu 2003, Shanghai 2004). So far, he and other trainers at NUS have successfully groomed various ICPC teams that won twelve different ICPC Regionals (see the list of Regionals wins below), advanced to ICPC World Finals twelve times (2009-2010; 2012-2021) with current best result of Joint-14th in ICPC World Finals Phuket 2016 (see the top 3 World Finals results below), as well as ten gold, twenty-three silver, and sixteen bronze IOI medalists (2009-2021).
He is also the Regional Contest Director of ICPC Asia Singapore 2015+2018 and is the Deputy Director+International Committee member for the IOI 2020+2021 in Singapore (both online competitions due to COVID-19). He has been invited to give international workshops about ICPC/IOI at various countries, e.g., Bolivia ICPC/IOI camp in 2014, Saudi Arabia IOI camp in 2019, Cambodia NOI camp in 2020.
Steven is happily married to Grace Suryani Tioso and has two daughters and one son: Jane Angelina Halim, Joshua Ben Halim, and Jemimah Charissa Halim.
Dr Felix Halim, felix.halim at gmail dot com
Felix Halim is a senior software engineer at Google. While in Google, he worked on distributed system problems, data analysis, indexing, internal tools, and database-related stuff.
Felix has a passion for web development. He created uHunt to help UVa online judge users find the next problems to solve. He also developed a crowdsourcing website, https://kawalpemilu.org , to let the Indonesian public to oversee and actively keep track of the Indonesia general election in 2014 and 2019.
As a contestant, Felix participated in IOI 2002 Korea (representing Indonesia), ICPC Manila 2003-2005, Kaohsiung 2006, and World Finals Tokyo 2007 (representing Bina Nusantara University). He was also one of Google India Code Jam 2005 and 2006 finalists. As a problem setter, Felix set problems for ICPC Jakarta 2010, 2012, 2013, ICPC Kuala Lumpur 2014, and several Indonesian national contests.
Felix is happily married to Siska Gozali. The picture on the right is one of their Europe honeymoon travel photos (in Switzerland) after ICPC World Finals at Porto 2019. For more information about Felix, visit his website at https://felix-halim.net .
Dr Suhendry Effendy, suhendry.effendy dot gmail dot com
Suhendry Effendy is a research fellow in the School of Computing of the National University of Singapore (SoC, NUS). He obtained his bachelor degree in Computer Science from Bina Nusantara University (BINUS), Jakarta, Indonesia, and his PhD degree in Computer Science from National University of Singapore, Singapore. Before completing his PhD, he was a lecturer in BINUS specializing in algorithm analysis and served as the coach for BINUS competitive programming team (nicknamed as "Jollybee").
Suhendry is a recurring problem setter for the ICPC Asia Jakarta since the very first in 2008. From 2010 to 2016, he served as the chief judge for the ICPC Asia Jakarta collaborating with many other problem setters. He also set problems in many other contests, such as the ICPC Asia Kuala Lumpur, the ICPC Asia Singapore, and Olimpiade Sains Nasional bidang Komputer (Indonesia National Science Olympiad in Informatics) to name but a few.
Supporting Material
Sample code for the data structures and algorithms mentioned in the fourth edition of the book can be found below:.
https://github.com/stevenhalim/cpbook-code
Please email Steven ( [email protected] ) if you encounter bugs...
Updated NUS CS3233 - Competitive Programming sample PDF slides will be uploaded later
Dr Steven Halim is the coach of Singapore IOI team and National University of Singapore ACM ICPC team.\ Together with the other coaches and trainers in NUS, he has produced many ACM ICPC World Finalist teams in year 2009-present (2017, but minus 2011)\ and 5 gold/14 silver/11 bronze IOI medals in year 2010-present.
During Steven\'s non-teaching days at National University of Singapore (2nd half of May/June/July or 2nd half of December) Steven is open to visitation by IOI teams outside Singapore\ to do customized (or a joint-training with Singapore IOI team) using mostly the material in Competitive Programming textbook and beyond. There is also a possibility for Steven to travel to your country instead (less preferred due to his busyness) If you are organizing IOI-related activities and interested with this workshop, please do the following:
- Find relevant authorities that might want to sponsor your team\'s trip to NUS School of Computing (especially if your country is very far from Singapore...) and pay for a "small" consultation fee to School of Computing, NUS (this is not significant compared to your traveling cost to Singapore and accommodation+food while you and your team stay in Singapore).
- Let them know that you want the author of Competitive Programming textbook to give a competitive programming workshop to your students (up to > 6500 copies have been sold since the first edition back in August 2010). Note: The workshop will be in English as Steven does not know any other language other than English and Indonesian.
- Contact us (stevenhalim at gmail dot com) to check for Steven\'s availability.
Unfortunately, due to potential conflict of interest between NUS and other Asian Universities, Steven has to decline ACM ICPC-related training requests especially among Asian Universities. Alternative options are:
- Register your students as an exchange student in NUS in Semester 2 (January-April each year) and specifically enroll in CS3233 - Competitive Programming course, officially. NUS has no problems with exchange student taking CS3233 course where I usually share many competition techniques.
- Buy the latest edition of Competitive Programming book and do self-study, perhaps with help of the additional free material (Sample source code and public Lecture slides).
- However if your University is far from Asia and we will not fight each other in ACM ICPC Asia Regional, you may be able to contact Steven on case by case basis.

- Malaysian IOI team (4 students, 1 coach) visited School of Computing on 1-4 July 2013 for IOI 2013 preparation.
- Philippines OI team (1 student) visited School of Computing on 21-26 April 2014 for APIO 2014 preparation.

- CP4 - Book 1 (A5 Paperback)
- CP4 - Book 2 (A5 Paperback)
- CP3 (eBook - will be phased out)
Partner Links

Information
- IOI Workshop
Accessibles
- Testimonials
© 2000-2024 Steven Halim
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
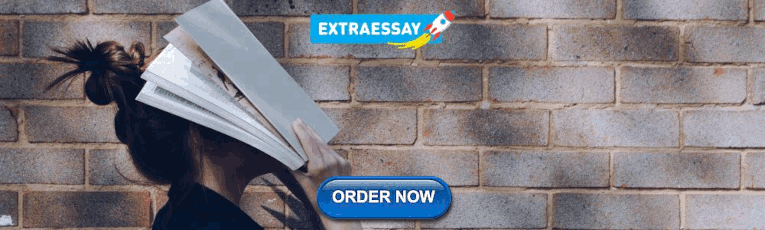
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
kattis-solutions
Here are 45 public repositories matching this topic..., russelldash332 / kattis.
All of my solutions to Kattis archives at https://open.kattis.com/
- Updated May 9, 2024
rambasnet / Kattis-Demos-Testing
Kattis demo solutions with unit testing
- Updated Nov 17, 2023
KentGrigo / Kattis
My solutions to challenges on Kattis
- Updated Feb 6, 2023
ecly / kattis
Solutions to Kattis problems, mostly in Python
- Updated Apr 18, 2024
jaredliw / python-question-bank
Python solutions of problems from various competitive programming platforms.
- Updated Aug 9, 2022
KOBeerose / CP-Solutions
My very bad code solutions for competitive programming problems
- Updated Oct 9, 2022
tadhikari / Kattis-Programming-Problem-Solutions
Kattis Programming Problem Solutions #competitiveprogramming
- Updated Feb 28, 2019
rajitbanerjee / kattis
My solutions to some problems from the Kattis Problem Archive.
- Updated Apr 15, 2022
robertusbagaskara / kattis-solutions
My solutions for some Kattis Problems.
- Updated May 10, 2024
sergeiissaev / Kattis-solutions
My solutions for the programming contest website Kattis : open.kattis.com. Solutions mostly in Python, some in C++.
- Updated Jun 24, 2022
aalekhpatel07 / icpc-ncna-2020
Team localMaxima's solutions for ICPC NCNA 2020. We ranked 9th out of 80 teams competing and have now advanced to ICPC NA Divisional Championship.
- Updated Mar 1, 2021
valentk777 / Competitive-Programming
My personal competitive programming solutions for Codeforces, Codewars, Kattis including fun things like the advent of code, algodaily and others
- Updated Apr 15, 2024
jed1337 / Kattis
Python solutions to Kattis problems
- Updated Dec 31, 2021
theycallmemac / Kattis
My solutions to problems found on https://open.kattis.com/ . Written in Python, Java, C, Go and a small bit of Haskell.
- Updated Dec 23, 2018
ngowran / My_Kattis_Solutions
Kattis solutions in python
- Updated May 8, 2022
renaldyresa / Kattis
Kattis Solution
- Updated Nov 29, 2021
mimseyedi / Kattis
In this repository I have included the problems I have solved from the kattis website. All problems are solved with Python language.
- Updated Nov 7, 2022
Charlot-DEDJINOU / Kattis
Découvrez mes solutions pour quelques exercices de Kattis, une plateforme en ligne populaire pour la pratique de la programmation et de l'algorithmique. Explorez mes approches de résolution de problèmes et ma capacité à coder des solutions efficaces pour une variété de défis algorithmiques
- Updated Apr 25, 2024
arsho / kattis_solutions
Solutions of programming problems from https://open.kattis.com/
- Updated Jan 19, 2022
danieltaylor / kattis-solutions
Solutions to Kattis problems I've solved. For educational use only; do not pass them off as your own work.
- Updated Jun 3, 2022
Improve this page
Add a description, image, and links to the kattis-solutions topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the kattis-solutions topic, visit your repo's landing page and select "manage topics."

- Computers & Technology
- Programming

Enjoy fast, free delivery, exclusive deals, and award-winning movies & TV shows with Prime Try Prime and start saving today with fast, free delivery
Amazon Prime includes:
Fast, FREE Delivery is available to Prime members. To join, select "Try Amazon Prime and start saving today with Fast, FREE Delivery" below the Add to Cart button.
- Cardmembers earn 5% Back at Amazon.com with a Prime Credit Card.
- Unlimited Free Two-Day Delivery
- Streaming of thousands of movies and TV shows with limited ads on Prime Video.
- A Kindle book to borrow for free each month - with no due dates
- Listen to over 2 million songs and hundreds of playlists
- Unlimited photo storage with anywhere access
Important: Your credit card will NOT be charged when you start your free trial or if you cancel during the trial period. If you're happy with Amazon Prime, do nothing. At the end of the free trial, your membership will automatically upgrade to a monthly membership.
Buy new: .savingPriceOverride { color:#CC0C39!important; font-weight: 300!important; } .reinventMobileHeaderPrice { font-weight: 400; } #apex_offerDisplay_mobile_feature_div .reinventPriceSavingsPercentageMargin, #apex_offerDisplay_mobile_feature_div .reinventPricePriceToPayMargin { margin-right: 4px; } -26% $97.67 $ 97 . 67 FREE delivery Friday, May 17 Ships from: Amazon Sold by: bookhunter188
Return this item for free.
Free returns are available for the shipping address you chose. You can return the item for any reason in new and unused condition: no shipping charges
- Go to your orders and start the return
- Select the return method
Save with Used - Good .savingPriceOverride { color:#CC0C39!important; font-weight: 300!important; } .reinventMobileHeaderPrice { font-weight: 400; } #apex_offerDisplay_mobile_feature_div .reinventPriceSavingsPercentageMargin, #apex_offerDisplay_mobile_feature_div .reinventPricePriceToPayMargin { margin-right: 4px; } $78.10 $ 78 . 10 FREE delivery Monday, May 20 Ships from: Amazon Sold by: Star Media USA

Download the free Kindle app and start reading Kindle books instantly on your smartphone, tablet, or computer - no Kindle device required .
Read instantly on your browser with Kindle for Web.
Using your mobile phone camera - scan the code below and download the Kindle app.

Follow the author

Image Unavailable

- To view this video download Flash Player

Data Structures: Abstraction and Design Using Java 4th Edition
Purchase options and add-ons.
Data Structures: Abstraction and Design Using Java offers a coherent and well-balanced presentation of data structure implementation and data structure applications with a strong emphasis on problem solving and software design. Step-by-step, the authors introduce each new data structure as an abstract data type (ADT), explain its underlying theory and computational complexity, provide its specification in the form of a Java interface, and demonstrate its implementation as one or more Java classes. Case studies using the data structures covered in the chapter show complete and detailed solutions to real-world problems, while a variety of software design tools are discussed to help students “Think, then code.”
The book supplements its rigorous coverage of basic data structures and algorithms with chapters on sets and maps, balanced binary search trees, graphs, event-oriented programming, testing and debugging, and other key topics. Now available as an enhanced e-book, the fourth edition of Data Structures: Abstraction and Design Using Java enables students to measure their progress after completing each section through interactive questions, quick-check questions, and review questions.
- ISBN-10 1119703611
- ISBN-13 978-1119703617
- Edition 4th
- Publisher Wiley
- Publication date February 3, 2021
- Language English
- Dimensions 8 x 1.1 x 9.9 inches
- Print length 688 pages
- See all details

Frequently bought together

Customers who viewed this item also viewed

Product details
- Publisher : Wiley; 4th edition (February 3, 2021)
- Language : English
- Paperback : 688 pages
- ISBN-10 : 1119703611
- ISBN-13 : 978-1119703617
- Item Weight : 2.6 pounds
- Dimensions : 8 x 1.1 x 9.9 inches
- #126 in Computer Programming Structured Design
- #629 in Object-Oriented Design
- #679 in Data Modeling & Design (Books)
About the author
Elliot b. koffman.
Discover more of the author’s books, see similar authors, read author blogs and more
Customer reviews
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
- Sort reviews by Top reviews Most recent Top reviews
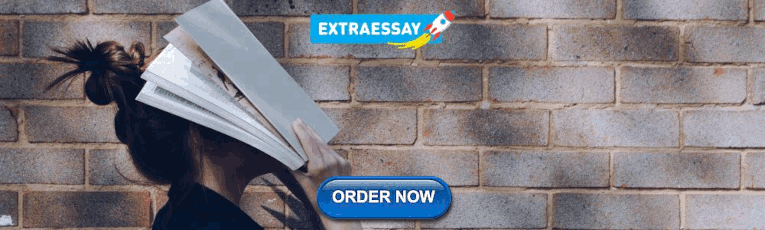
IMAGES
VIDEO
COMMENTS
4th Edition. Data Structures and Problem Solving Using Java takes a practical and unique approach to data structures that separates interface from implementation. It is suitable for the second or third programming course. This book provides a practical introduction to data structures with an emphasis on abstract thinking and problem solving, as ...
cifically; it remains a book that emphasizes data structures and basic problem-solving techniques. Of course, the general techniques used in the design of data structures are applicable to the implementation of the Collections API, so sev-eral chapters in Part Four include Collections API implementations. However,
For the second or third programming course. A practical and unique approach to data structures that separates interface from implementation. This book provides a practical introduction to data structures with an emphasis on abstract thinking and problem solving, as well as the use of Java. It does this through what remains a unique approach that clearly separates each data structure's ...
This book provides a practical introduction to data structures with an emphasis on abstract thinking and problem solving, as well as the use of Java. It does this through what remains a unique approach that clearly separates each data structure's interface (how to use a data structure) from its implementation (how to actually program that ...
Mark Allen Weiss. Pearson/Addison-Wesley, 2006 - Computers - 926 pages. This book provides a practical introduction to data structures from a viewpoint of abstract thinking and problem solving, as well as the use of Java. It does this through what remains a unique approach that clearly separates each data structure's interface (how to use a ...
Data Structures and Problem Solving Using Java takes a practical and unique approach to data structures that separates interface from implementation. It is suitable for the second or third programming course. This book provides a practical introduction to data structures with an emphasis on abstract thinking and problem solving, as well as the use of Java.
Data Structures and Problem Solving Using Java, 4th edition Published by Pearson (July 14, 2021) © 2010. Mark A. Weiss
A Unique Approach. The text takes a unique approach by separating the data structures into their specificatio n (via a Java interface) and subsequent implementation . This approach provides several benefits , including the promotion of abstract thinking . Class interfaces are written and used before th e.
Data Structures and Problem Solving Using Java, Pearson New International Edition, 4th edition. Published by Pearson (July 23, ... personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle. ... Data Structures and Problem Solving Using Java, Pearson New International Edition, 4th edition ...
From the Publisher: Data Structures and Problem Solving Using Java teaches data structures and algorithms from the perspective of abstract thinking and problem solving. In this text, experienced author and educator Mark Allen Weiss takes a unique approach by clearly separating the specification and implementation of data structures. Dr.
Every textbook comes with a 21-day "Any Reason" guarantee. Published by Prentice Hall. Data Structures and Problem Solving Using Java 4th edition solutions are available for this textbook. COUPON: RENT Data Structures and Problem Solving Using Java 4th edition (9780321541406) and save up to 80% on 📚textbook rentals and 90% on 📙used textbooks.
Data Structures & Problem Solving Using Java fourth edition This page intentionally left blank Data Structures & Problem Solving Using Java fourth edition mark allen weiss florida international university Editor-in-Chief Editorial Assistant Managing Editor Senior Production Supervisor Marketing Manager Marketing Coordinator Media Producer Senior Manufacturing Buyer Project Coordination ...
This is the eBook of the printed book and may not include any media, website access codes, or print supplements that may come packaged with the bound book. Data Structures and Problem Solving Using Java takes a practical and unique approach to data structures that separates interface from implementation. It is suitable for the second or third programming course.
It's easier to figure out tough problems faster using Chegg Study. Unlike static PDF Data Structures and Problem Solving Using Java 4th Edition solution manuals or printed answer keys, our experts show you how to solve each problem step-by-step. No need to wait for office hours or assignments to be graded to find out where you took a wrong turn.
Get full access to Data Structures and Algorithms in Java and 60K+ other titles, with a free 10-day trial of O ... O'Reilly members get unlimited access to books, live events, courses curated by job ... of the O'Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
Data Structures and Problem Solving Using Java teaches data structures and algorithms from the perspective of abstract thinking and problem solving. In this text, experienced author and educator Mark Allen Weiss takes a unique approach by clearly separating the specification and implementation of data structures.
"Competitive Programming 3 has contributed immensely to my understanding of data structures & algorithms. Steven & Felix have created an incredible book that thoroughly covers every aspect of competitive programming, and have included plenty of practice problems to make sure each topic sinks in. Practicing with CP3 has helped me nail job interviews at Google, and I can't thank Steven & Felix ...
Specialization - 4 course series. This Specialization starts out by teaching basic concepts in Python and ramps up to more complex subjects such as object-oriented programming and data structures in Java. By the time learners complete this series of four courses, they will be able to write fully-functional programs in both Python and Java, two ...
Data Structures and Problem Solving Using Java teaches data structures and algorithms from the perspective of abstract thinking and problem solving. In this text, experienced author and educator Mark Allen Weiss takes a unique approach by clearly separating the specification and implementation of data structures. Dr.
More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects. ... All 45 C++ 48 Python 45 Java 37 C 6 C# 6 Rust 3 Go 2 Common ... javascript python java algorithm algorithms cpp competitive-programming python3 data-structures python-3 problem-solving kattis algorithms-datastructures ...
Data Structures: Abstraction and Design Using Java offers a coherent and well-balanced presentation of data structure implementation and data structure applications with a strong emphasis on problem solving and software design. Step-by-step, the authors introduce each new data structure as an abstract data type (ADT), explain its underlying theory and computational complexity, provide its ...
In this accessible, comprehensive text, George Luger captures the essence of artificial intelligence-solving the complex problems that arise wherever computer technology is applied. Key representation techniques including logic, semantic and connectionist networks, graphical models, and many more are introduced. Presentation of agent technology and the use of ontologies are added.