Learn Java practically and Get Certified .
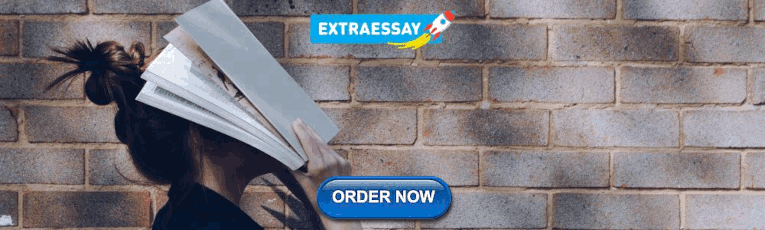
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
Java Expressions, Statements and Blocks
Java Flow Control
Java if...else statement.
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
Java break Statement
- Java continue Statement
Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
In programming, we use the if..else statement to run a block of code among more than one alternatives.
For example, assigning grades (A, B, C) based on the percentage obtained by a student.
- if the percentage is above 90 , assign grade A
- if the percentage is above 75 , assign grade B
- if the percentage is above 65 , assign grade C
1. Java if (if-then) Statement
The syntax of an if-then statement is:
Here, condition is a boolean expression such as age >= 18 .
- if condition evaluates to true , statements are executed
- if condition evaluates to false , statements are skipped
Working of if Statement
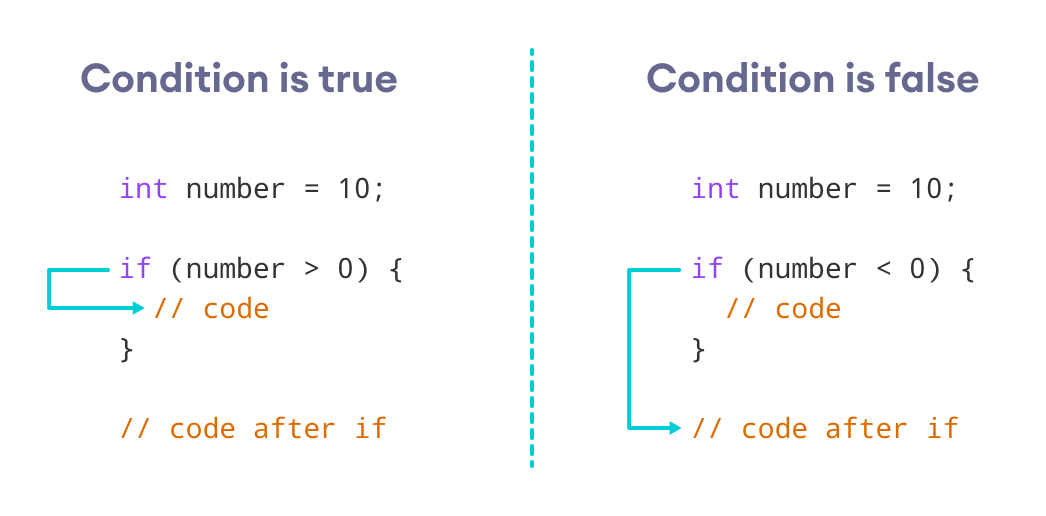
Example 1: Java if Statement
In the program, number < 0 is false . Hence, the code inside the body of the if statement is skipped .
Note: If you want to learn more about about test conditions, visit Java Relational Operators and Java Logical Operators .
We can also use Java Strings as the test condition.
Example 2: Java if with String
In the above example, we are comparing two strings in the if block.
2. Java if...else (if-then-else) Statement
The if statement executes a certain section of code if the test expression is evaluated to true . However, if the test expression is evaluated to false , it does nothing.
In this case, we can use an optional else block. Statements inside the body of else block are executed if the test expression is evaluated to false . This is known as the if-...else statement in Java.
The syntax of the if...else statement is:
Here, the program will do one task (codes inside if block) if the condition is true and another task (codes inside else block) if the condition is false .
How the if...else statement works?
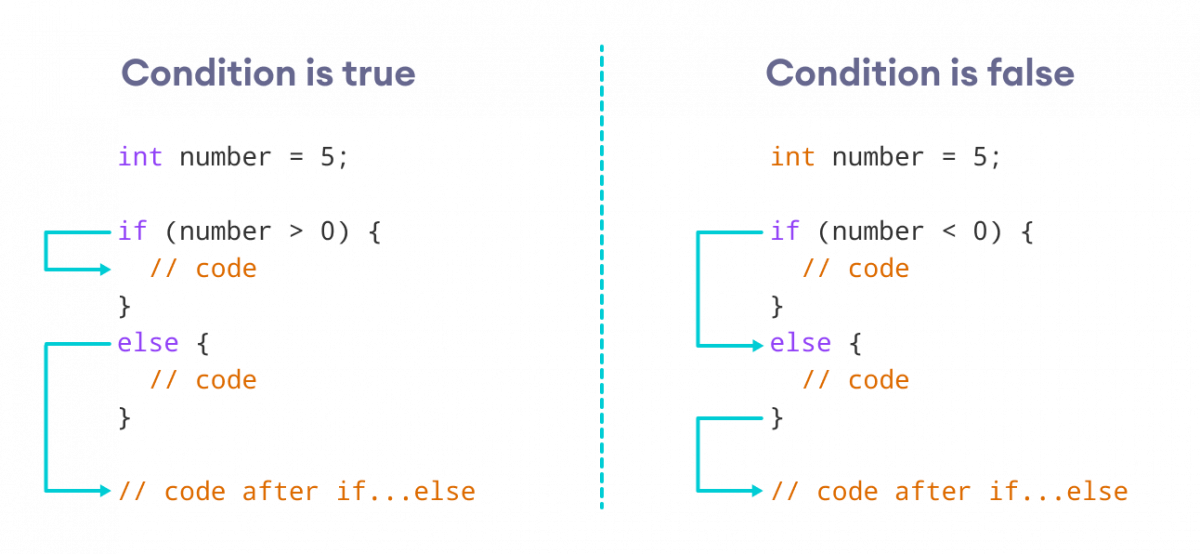
Example 3: Java if...else Statement
In the above example, we have a variable named number . Here, the test expression number > 0 checks if number is greater than 0.
Since the value of the number is 10 , the test expression evaluates to true . Hence code inside the body of if is executed.
Now, change the value of the number to a negative integer. Let's say -5 .
If we run the program with the new value of number , the output will be:
Here, the value of number is -5 . So the test expression evaluates to false . Hence code inside the body of else is executed.
3. Java if...else...if Statement
In Java, we have an if...else...if ladder, that can be used to execute one block of code among multiple other blocks.
Here, if statements are executed from the top towards the bottom. When the test condition is true , codes inside the body of that if block is executed. And, program control jumps outside the if...else...if ladder.
If all test expressions are false , codes inside the body of else are executed.
How the if...else...if ladder works?
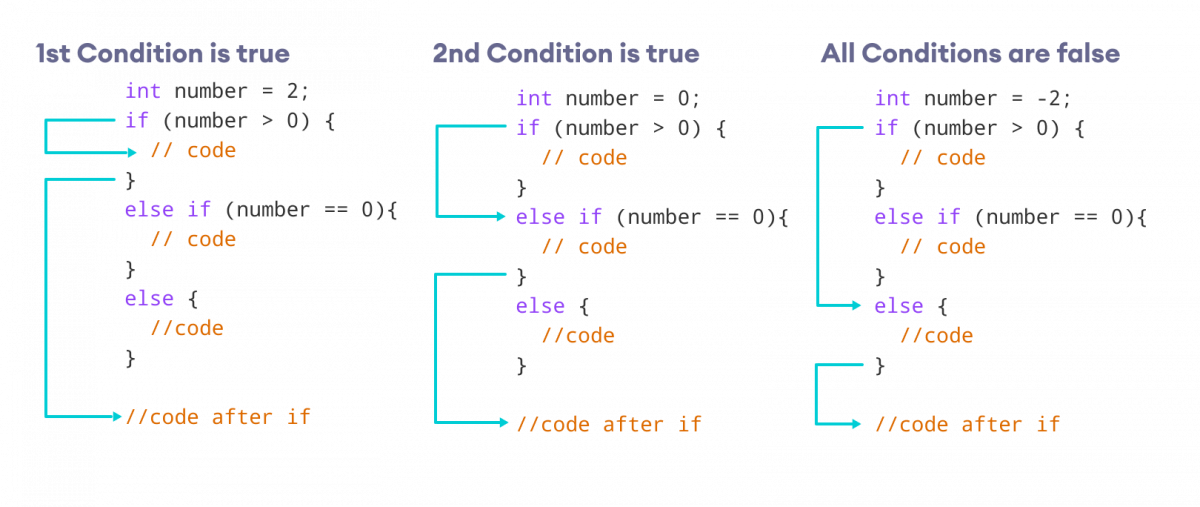
Example 4: Java if...else...if Statement
In the above example, we are checking whether number is positive, negative, or zero . Here, we have two condition expressions:
- number > 0 - checks if number is greater than 0
- number < 0 - checks if number is less than 0
Here, the value of number is 0 . So both the conditions evaluate to false . Hence the statement inside the body of else is executed.
Note : Java provides a special operator called ternary operator , which is a kind of shorthand notation of if...else...if statement. To learn about the ternary operator, visit Java Ternary Operator .
4. Java Nested if..else Statement
In Java, it is also possible to use if..else statements inside an if...else statement. It's called the nested if...else statement.
Here's a program to find the largest of 3 numbers using the nested if...else statement.
Example 5: Nested if...else Statement
In the above programs, we have assigned the value of variables ourselves to make this easier.
However, in real-world applications, these values may come from user input data, log files, form submission, etc.
Table of Contents
- Introduction
- Java if (if-then) Statement
- Example: Java if Statement
- Java if...else (if-then-else) Statement
- Example: Java if else Statement
- Java if..else..if Statement
- Example: Java if..else..if Statement
- Java Nested if..else Statement
Sorry about that.
Related Tutorials
Java Tutorial
Java Language
- Getting started with Java Language
- Top 10 Java Errors with Solutions
- Awesome Book
- Awesome Community
- Awesome Course
- Awesome Tutorial
- Awesome YouTube
- 2D Graphics in Java
- Alternative Collections
- Annotations
- Apache Commons Lang
- AppDynamics and TIBCO BusinessWorks Instrumentation for Easy Integration
- Atomic Types
- Auto-unboxing may lead to NullPointerException
- Different Cases When Integer and int can be used interchangeably
- Memory and Computational Overhead of Autoboxing
- Using Boolean in if statement
- Using int and Integer interchangeably
- Basic Control Structures
- Bit Manipulation
- BufferedWriter
- Bytecode Modification
- C++ Comparison
- Calendar and its Subclasses
- Character encoding
- Choosing Collections
- Class - Java Reflection
- Classes and Objects
- Classloaders
- Collection Factory Methods
- Collections
- Command line Argument Processing
- Common Java Pitfalls
- Comparable and Comparator
- CompletableFuture
- Concurrent Collections
- Concurrent Programming (Threads)
- Console I/O
- Constructors
- Converting to and from Strings
- Creating Images Programmatically
- Currency and Money
- Dates and Time (java.time.*)
- Default Methods
- Dequeue Interface
- Disassembling and Decompiling
- Documenting Java Code
- Dynamic Method Dispatch
- Encapsulation
- Enum starting with number
- EnumSet class
- Exceptions and exception handling
- Executor, ExecutorService and Thread pools
- Expressions
- FileUpload to AWS
- Fluent Interface
- FTP (File Transfer Protocol)
- Functional Interfaces
- Generating Java Code
- Getters and Setters
- HttpURLConnection
- Immutable Class
- Immutable Objects
- Inheritance
- InputStreams and OutputStreams
- Installing Java (Standard Edition)
- Iterator and Iterable
- Java Agents
- Java Compiler - 'javac'
- Java deployment
- Java Editions, Versions, Releases and Distributions
- Java Floating Point Operations
- Java Memory Management
- Java Memory Model
- Java Native Access
- Java Native Interface
- Java Performance Tuning
- Java Pitfalls - Exception usage
- Java Pitfalls - Language syntax
- Java Pitfalls - Nulls and NullPointerException
- Java Pitfalls - Performance Issues
- Java Pitfalls - Threads and Concurrency
- Java plugin system implementations
- Java Print Service
- Java SE 7 Features
- Java SE 8 Features
- Java Sockets
- Java Virtual Machine (JVM)
- JSON in Java
- Just in Time (JIT) compiler
- JVM Tool Interface
- Lambda Expressions
- LinkedHashMap
- List vs SET
- Local Inner Class
- Localization and Internationalization
- log4j / log4j2
- Logging (java.util.logging)
- Multi-Release JAR Files
- Nashorn JavaScript engine
- Nested and Inner Classes
- New File I/O
- NIO - Networking
- Non-Access Modifiers
- NumberFormat
- Object Class Methods and Constructor
- Object Cloning
- Object References
- Oracle Official Code Standard
- Parallel programming with Fork/Join framework
- Polymorphism
- Preferences
- Primitive Data Types
- Properties Class
- Queues and Deques
- Random Number Generation
- Readers and Writers
- Reference Data Types
- Reference Types
- Reflection API
- Regular Expressions
- Remote Method Invocation (RMI)
- Resources (on classpath)
- RSA Encryption
- Runtime Commands
- Secure objects
- Security & Cryptography
- SecurityManager
- Serialization
- ServiceLoader
- Splitting a string into fixed length parts
- Stack-Walking API
- String Tokenizer
- StringBuffer
- StringBuilder
- sun.misc.Unsafe
- super keyword
- The Classpath
- The Java Command - 'java' and 'javaw'
- The java.util.Objects Class
- ThreadLocal
- TreeMap and TreeSet
- Type Conversion
- Unit Testing
- Using Other Scripting Languages in Java
- Using the static keyword
- Using ThreadPoolExecutor in MultiThreaded applications.
- Varargs (Variable Argument)
- Visibility (controlling access to members of a class)
- WeakHashMap
- XML Parsing using the JAXP APIs
- XML XPath Evaluation
- XOM - XML Object Model
Java Language Autoboxing Using Boolean in if statement
Fastest entity framework extensions.
Due to auto unboxing, one can use a Boolean in an if statement:
That works for while , do while and the condition in the for statements as well.
Note that, if the Boolean is null , a NullPointerException will be thrown in the conversion.
Got any Java Language Question?

- Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks.
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
Mastering If Statements in Java: Basics and Beyond
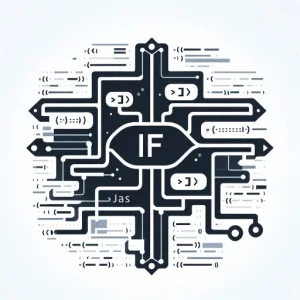
Are you finding it challenging to get your head around if statements in Java? You’re not alone. Many developers, especially beginners, often find themselves puzzled when it comes to handling if statements in Java. Think of an if statement as a traffic cop, directing the flow of your program based on certain conditions. It’s a fundamental concept that forms the backbone of many programming tasks.
This guide will walk you through the ins and outs of using if statements in Java , from the basics to more advanced techniques. We’ll cover everything from the syntax of if statements, how to use them in different scenarios, to common pitfalls and how to avoid them.
So, let’s dive in and start mastering if statements in Java!
TL;DR: How Do I Use an If Statement in Java?
In Java, an “if statement” is used to test a condition. The syntax is if (condition) {code to execute} . If the condition is true, the code within the curly braces {} is executed.
Here’s a simple example:
In this example, we’ve used an if statement to compare two variables, x and y . The condition x > y is true, so the code within the if statement is executed, and ‘x is greater than y’ is printed to the console.
This is a basic way to use if statements in Java, but there’s much more to learn about controlling the flow of your program. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of If Statements in Java
Intermediate-level if statements, exploring alternatives to if statements in java, troubleshooting if statements in java, unveiling java control flow, if statements in the larger context, wrapping up: mastering if statements in java.
The if statement in Java is a fundamental control flow statement, which allows the program to make decisions based on certain conditions. It follows a simple syntax:
The condition in an if statement is a boolean expression, which means it can either be true or false . If the condition is true, the code block inside the if statement gets executed. If it’s false, the code block is skipped.
Here’s a simple code example:
In this example, the condition age >= 18 is true, so the string ‘You are eligible to vote.’ is printed to the console.
While if statements are incredibly useful, they do come with some potential pitfalls. For example, if you forget to use braces {} , only the first line of code after the if statement will be considered part of the if block. This can lead to unexpected results. Always remember to enclose the code you want to execute within braces.
In the next section, we’ll take a look at more complex uses of if statements in Java.
Once you’ve mastered the basics of if statements in Java, it’s time to explore more complex uses. Two such techniques are nested if statements and the use of logical operators.
Nested If Statements
A nested if statement is an if statement inside another if statement. It allows you to test for multiple conditions and execute different code blocks depending on the outcome. Here’s an example:
In this example, we first check if the score is greater than or equal to 50. If it is, we then check if it’s also greater than or equal to 75. Depending on the results of these tests, we print different messages to the console.
If Statements with Logical Operators
Logical operators such as && (and), || (or), and ! (not) can be used in the condition of an if statement to test multiple criteria at once. Here’s an example:
In this example, the if statement checks two conditions: whether age is greater than or equal to 18, and whether isCitizen is true. Only if both conditions are true does it print ‘You are eligible to vote.’ to the console.
These advanced techniques allow for more complex decision-making in your Java programs. However, they also require careful attention to detail to avoid errors and unexpected behavior.
While if statements are a crucial part of controlling the flow of your program, Java offers other control flow statements that can serve as alternatives or complements to if statements. Two such alternatives are switch statements and ternary operators.
The Power of Switch Statements
A switch statement can be used instead of a complex if-else chain when you need to select one of many code blocks to be executed.
In this example, the switch statement checks the value of day , and depending on that value, assigns a different string to dayOfWeek . This is much cleaner and more readable than a long chain of if-else statements.
Ternary Operators: A Shorter Alternative
The ternary operator is a shorter form of an if-else statement. It’s a one-liner that tests a condition and returns a value based on whether the condition is true or false.
In this example, the ternary operator checks if score is greater than or equal to 50. If it is, it assigns the string ‘Pass’ to result . If not, it assigns ‘Fail’. This is much shorter and more concise than an equivalent if-else statement.
While these alternatives offer their own advantages, they also have their own drawbacks. Switch statements can be more verbose for simple conditions, and ternary operators can be harder to read for complex conditions. It’s essential to choose the right tool for the job based on your specific situation.
While if statements are a cornerstone of Java, they can sometimes lead to unexpected results. Let’s explore some common pitfalls and best practices to avoid them.
The Importance of Braces
Forgetting to use braces {} is a common mistake. In Java, if you don’t use braces after an if statement, only the first line of code will be considered part of the if block. Here’s an example:
In this example, the second print statement executes regardless of whether x is greater than y because it’s not enclosed in braces. Always remember to use braces when you want multiple lines of code to be part of the if block.
Using the Correct Comparison Operator
Another common pitfall is using the wrong comparison operator. For example, using = (assignment) instead of == (equality) can lead to unexpected results.
In this example, using x = 5 in the if statement will cause a compile error because = is an assignment operator, not a comparison operator. To check if x is equal to 5, you should use x == 5 .
Being aware of these common mistakes and following these best practices can help you avoid errors and write more robust Java code.
Control flow is a fundamental concept in programming that refers to the order in which the program’s code executes. In Java, this is primarily controlled by control flow statements, which include if statements, switch statements, for loops, while loops, and more.
If Statements: The Traffic Cops of Java
Among these, if statements play a pivotal role. They act like traffic cops, directing the flow of your program based on certain conditions. If a condition is true, the code within the if statement executes. If not, the code is skipped, and the program moves on to the next part of the code.
In this example, the condition temperature > 30 is false, so the print statement within the if statement is skipped, and there’s no output.
The Role of Boolean Expressions
The conditions in if statements are boolean expressions, meaning they evaluate to either true or false . These expressions can involve comparisons (like x > y ), logical operators (like x > y && x < z ), or method calls that return a boolean value (like string.isEmpty() ).
Understanding control flow and the role of if statements in Java is crucial to writing effective and efficient code. As you gain more experience, you’ll find that mastering these concepts can greatly enhance your problem-solving skills in programming.
If statements don’t exist in isolation. They’re often used in conjunction with other control flow statements, such as loops and methods, to create more complex and powerful programs.
If Statements and Loops
For example, you can use if statements within loops to perform certain actions only when specific conditions are met.
In this example, the if statement checks if the current number i is even. If it is, it prints a message to the console. This happens on each iteration of the loop, allowing us to check and respond to different conditions at each step.
If Statements and Methods
If statements can also be used within methods to control the method’s behavior based on its inputs.
In this example, the greet method checks if the input name is null or empty. If it is, it prints a generic greeting. If not, it prints a personalized greeting. This allows the method to respond appropriately to different inputs.
Further Resources for Mastering Java Control Flow
If you’re interested in diving deeper into control flow in Java, here are some resources that you might find helpful:
- Demystifying Loops in Java – Discover how loops contribute to code modularity and reusability in Java.
Java Else-If – Explore the else-if ladder in Java for handling multiple conditional branches.
Using Break in Java – Learn how to use break to terminate loop execution based on certain conditions.
Oracle’s Java Tutorials covers all aspects of Java, including in-depth discussions on control flow.
Java Conditions and If Statements by W3Schools explains how to use conditions and ‘if’ statements in Java.
Learn Java course includes several lessons and projects that will help you master control flow in Java.
In this comprehensive guide, we’ve navigated through the world of if statements in Java, a cornerstone of control flow in programming.
We began with the basics, understanding the syntax and usage of if statements, and how they serve as the traffic cops of your Java programs. We then delved into more complex uses, exploring nested if statements and the use of logical operators to test multiple conditions simultaneously.
Along the way, we’ve tackled common pitfalls, such as forgetting to use braces or using the wrong comparison operator, and provided best practices to avoid these errors. We’ve also explored alternative control flow statements, such as switch statements and ternary operators, giving you a broader perspective on controlling the flow of your program.
Here’s a quick comparison of the methods we’ve discussed:
Whether you’re a beginner just starting out with if statements or an experienced developer looking to brush up on your skills, we hope this guide has been a helpful journey through the ins and outs of if statements in Java.
Mastering if statements and control flow is a crucial step in becoming a proficient Java programmer. Now, you’re well equipped to handle any condition your program might throw at you. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
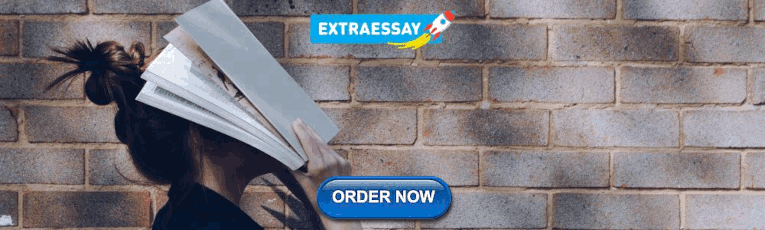
Related Posts
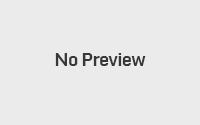
- Prev Class
- Next Class
- No Frames
- All Classes
- Summary:
- Nested |
- Field |
- Constr |
- Detail:
Class Boolean
- java.lang.Object
- java.lang.Boolean
Field Summary
Constructor summary, method summary, methods inherited from class java.lang. object, field detail, constructor detail, method detail, parseboolean, booleanvalue.
Submit a bug or feature For further API reference and developer documentation, see Java SE Documentation . That documentation contains more detailed, developer-targeted descriptions, with conceptual overviews, definitions of terms, workarounds, and working code examples. Copyright © 1993, 2024, Oracle and/or its affiliates. All rights reserved. Use is subject to license terms . Also see the documentation redistribution policy .
Scripting on this page tracks web page traffic, but does not change the content in any way.
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java booleans.
Very often, in programming, you will need a data type that can only have one of two values, like:
- TRUE / FALSE
For this, Java has a boolean data type, which can store true or false values.
Boolean Values
A boolean type is declared with the boolean keyword and can only take the values true or false :
Try it Yourself »
However, it is more common to return boolean values from boolean expressions, for conditional testing (see below).
Boolean Expression
A Boolean expression returns a boolean value: true or false .
This is useful to build logic, and find answers.
For example, you can use a comparison operator , such as the greater than ( > ) operator, to find out if an expression (or a variable) is true or false:
Or even easier:
In the examples below, we use the equal to ( == ) operator to evaluate an expression:
Real Life Example
Let's think of a "real life example" where we need to find out if a person is old enough to vote.
In the example below, we use the >= comparison operator to find out if the age ( 25 ) is greater than OR equal to the voting age limit, which is set to 18 :
Cool, right? An even better approach (since we are on a roll now), would be to wrap the code above in an if...else statement, so we can perform different actions depending on the result:
Output "Old enough to vote!" if myAge is greater than or equal to 18 . Otherwise output "Not old enough to vote.":
Booleans are the basis for all Java comparisons and conditions.
You will learn more about conditions ( if...else ) in the next chapter.
Test Yourself With Exercises
Fill in the missing parts to print the values true and false :
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Java One Line if Statement
- Java Howtos
Ternary Operator in Java
One line if-else statement using filter in java 8.
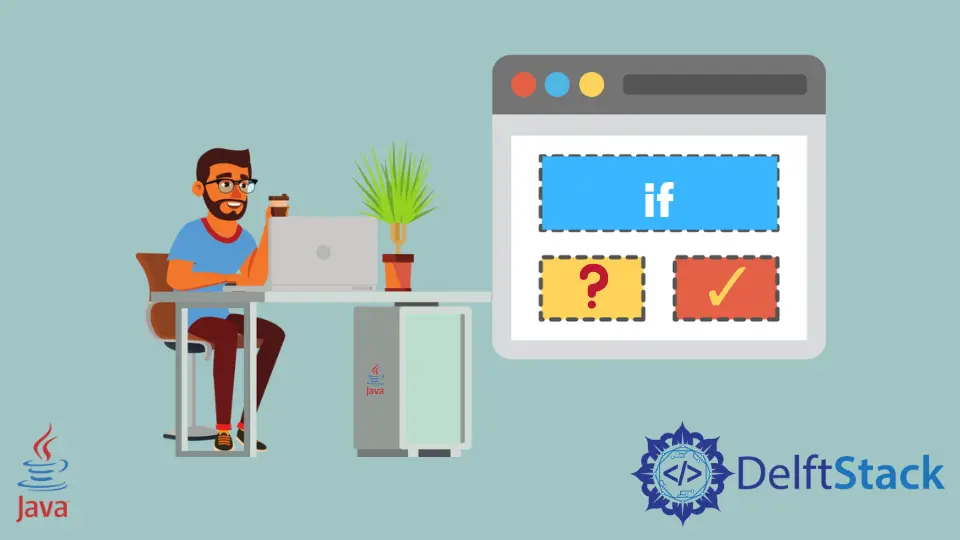
The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code.
Fortunately, Java provides a shorthand form called the ternary operator, which allows us to write a one-line if-else statement.
The ternary operator in Java, denoted as ? : , provides a compact way to represent an if-else statement. Its syntax is as follows:
Here, condition is evaluated first. If condition is true, expression1 is executed; otherwise, expression2 is executed.
Example 1: Assigning a Value Based on a Condition
This code uses the ternary operator to determine if a student has made a distinction based on their exam marks.
If marks is greater than 70 , the string Yes is assigned to the distinction variable and the output will be:
If marks is less than or equal to 70 , the string No is assigned to the distinction variable and the output will be:
Example 2: Printing a Message Conditionally
This code defines a Boolean variable isRaining with the value true and uses a ternary operator to print a message based on the value of isRaining .
If isRaining is true , it prints Bring an umbrella ; otherwise, it prints No need for an umbrella .
Example 3: Returning a Value from a Method
In this code, we’re assigning the maximum of a and b to the variable max using a ternary operator. The maximum value is then printed to the console.
You can try it yourself by replacing the values of a and b with your desired values to find the maximum between them.
Java 8 introduced streams and the filter method, which operates similarly to an if-else statement. It allows us to filter elements based on a condition.
Here’s its syntax:
Predicate is a functional interface that takes an argument and returns a Boolean. It’s often used with lambda expressions to define the condition for filtering.
Here’s a demonstration of a one-line if-else -like usage using the filter method in Java 8 streams.
As we can see, this example demonstrates a one-line usage of an if-else -like structure using the filter method. It first defines a list of words and then applies a stream to this list.
Within the map function, a lambda expression is used to check if each word starts with the letter b . If a word meets this condition, it remains unchanged; otherwise, it is replaced with the string Not available .
Finally, the resulting stream is collected back into a list using collect(Collectors.toList()) . The output shows the effect of this one-line if-else -like usage, modifying the words based on the specified condition.
Let’s see another example.
This example code also showcases the use of Java 8 streams to filter and print elements from a list based on a condition. The code begins by importing necessary packages and defining a class named Java8Streams .
Inside the main method, a list of strings, stringList , is created with elements 1 and 2 . The stream is then created from this list using the stream() method.
Next, the filter method is applied to this stream, utilizing a lambda expression as the predicate. The lambda expression checks if the string is equal to 1 .
If this condition is true, the string is allowed to pass through the filter; otherwise, it is discarded.
Finally, the forEach method is used to iterate over the filtered stream and print each element that passed the filter. It uses a method reference System.out::println to achieve this.
In summary, one-line if statements in Java offer a compact and efficient way to handle simple conditional logic. By understanding the syntax and appropriate usage, you can write concise code while maintaining readability and clarity.
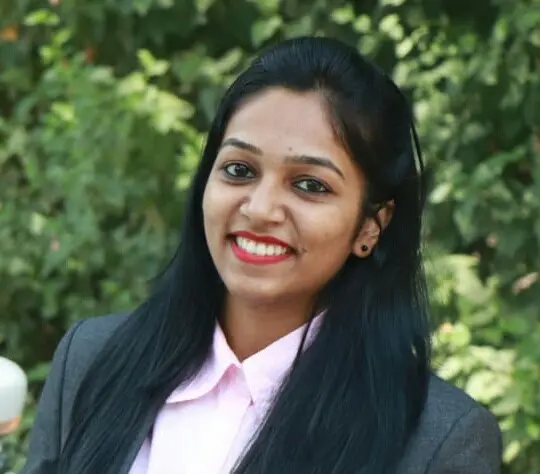
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
Related Article - Java Statement
- Difference Between break and continue Statements in Java
- The continue Statement in Java
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
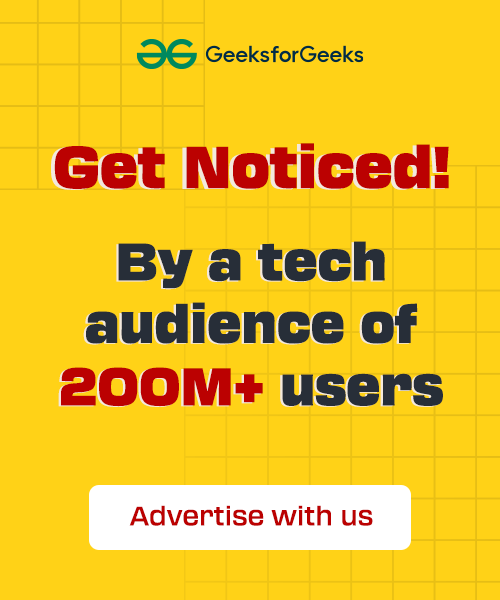
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
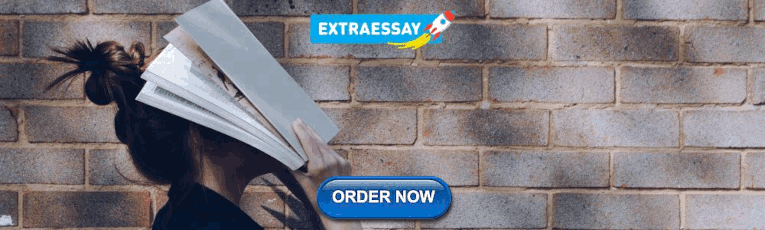
COMMENTS
Because I know it's possible in while conditions, but I'm not sure if I'm doing it wrong for the if-statement or if it's just not possible. HINT: what type while and if condition should be ?? If it can be done with while, it can be done with if statement as weel, as both of them expect a boolean condition.
The if statement will evaluate whatever code you put in it that returns a boolean value, and if the evaluation returns true, you enter the first block. Else (if the value is not true, it will be false, because a boolean can either be true or false) it will enter the - yep, you guessed it - the else {} block.
Output. The number is positive. Statement outside if...else block. In the above example, we have a variable named number.Here, the test expression number > 0 checks if number is greater than 0.. Since the value of the number is 10, the test expression evaluates to true.Hence code inside the body of if is executed.. Now, change the value of the number to a negative integer.
The if-else statement is the most basic of all control structures, and it's likely also the most common decision-making statement in programming. It allows us to execute a certain code section only if a specific condition is met. 2. Syntax of If-Else. The if statement always needs a boolean expression as its parameter.
Boolean Type The simplest and most common form of boolean expression is the use a < in an if-statement as shown above. However, boolean is a full primitive type in Java, just like int and double. In the boolean type, there are only two possible values: true and false. We can have variables and expressions of type boolean, just has we have ...
There could be multiple if conditions, although, for simplicity, we'll assume just one if / else statement. 4. Use Switch. An alternative to using an if logic is the switch command. Let's see how we can use it in our example: boolean switchMonth(Month month) {. switch (month) {. case OCTOBER: case NOVEMBER:
Java if-else. Decision-making in Java helps to write decision-driven statements and execute a particular set of code based on certain conditions. The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won't. In this article, we will learn about Java if-else.
Learn Java Language - Using Boolean in if statement. Learn Java Language - Using Boolean in if statement. RIP Tutorial. Tags; Topics; Examples; eBooks; Download Java Language (PDF) ... That works for while, do while and the condition in the for statements as well. Note that, if the Boolean is null, a NullPointerException will be thrown in the ...
Java Else-If - Explore the else-if ladder in Java for handling multiple conditional branches. Using Break in Java - Learn how to use break to terminate loop execution based on certain conditions. Oracle's Java Tutorials covers all aspects of Java, including in-depth discussions on control flow. Java Conditions and If Statements by ...
Working of if statement: Control falls into the if block. The flow jumps to Condition. Condition is tested. If Condition yields true, goto Step 4. If Condition yields false, goto Step 5. The if-block or the body inside the if is executed. Flow steps out of the if block. Flowchart if statement:
Java has the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false. Use switch to specify many alternative blocks of ...
When you run this code in jshell, you will get the following output: Output. x ==> 1. y ==> 0. 1 is bigger than 0. The first two lines of the output confirm the values of x and y. Line 3 reads 1 is bigger than 0, which means that the conditional statement is true: x is bigger than y.
The Boolean class wraps a value of the primitive type boolean in an object. An object of type Boolean contains a single field whose type is boolean . In addition, this class provides many methods for converting a boolean to a String and a String to a boolean, as well as other constants and methods useful when dealing with a boolean.
Conditional statements in programming are used to control the flow of a program based on certain conditions. These statements allow the execution of different code blocks depending on whether a specified condition evaluates to true or false, providing a fundamental mechanism for decision-making in algorithms. In this article, we will learn about the basics of Conditional Statements along with ...
15. You can use java ternary operator, this statement can be read as, If testCondition is true, assign the value of value1 to result; otherwise, assign the value of value2 to result. simple example would be, In above code, if the variable a is less than b, minVal is assigned the value of a; otherwise, minVal is assigned the value of b.
For this, Java has a boolean data type, which can store true or false values. ... However, it is more common to return boolean values from boolean expressions, for conditional testing (see below). Boolean Expression. A Boolean expression returns a boolean value: true or false.
The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code. Fortunately, Java provides a shorthand form called the ternary ...
Tricky Java: boolean assignment in if condition. Ask Question Asked 10 years, 3 months ago. Modified 10 years, 3 months ago. ... Not only does it have assignment within conditional, it also uses bitwise OR. I assume the cert exams intentionally throw stuff like this on there, but if I received this code in a review, I'd send it back. We don't ...
1. Your problem is with your. b=false. = is an assignment operator. What you need is == which will compare. If want to check whether a boolean is false you can also use !b which is equivalent to b== false. answered Aug 2, 2017 at 20:01. ecain. 1,292 5 24 54.
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
zeeshan. 13 Answers. Answer. + 8. Yes you can put assignment operator (=) inside if conditional statement (C/C++) and its boolean type will be always evaluated to true since it will generate side effect to variables inside in it. And if you use equality relational operator (==) then its boolean type will be evaluated to true or false depending ...