Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
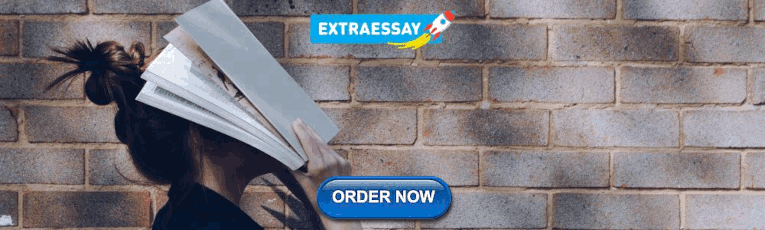
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Ternary Operator
JavaScript switch...case Statement
- JavaScript try...catch...finally Statement
- JavaScript if...else Statement
The JavaScript if...else statement is used to execute/skip a block of code based on a condition.
Here's a quick example of the if...else statement. You can read the rest of the tutorial if you want to learn about if...else in greater detail.
In the above example, the program displays You passed the examination. if the score variable is equal to 50 . Otherwise, it displays You failed the examination.
In computer programming, the if...else statement is a conditional statement that executes a block of code only when a specific condition is met. For example,
Suppose we need to assign different grades to students based on their scores.
- If a student scores above 90 , assign grade A .
- If a student scores above 75 , assign grade B .
- If a student scores above 65 , assign grade C .
These conditional tasks can be achieved using the if...else statement.
- JavaScript if Statement
We use the if keyword to execute code based on some specific condition.
The syntax of if statement is:
The if keyword checks the condition inside the parentheses () .
- If the condition is evaluated to true , the code inside { } is executed.
- If the condition is evaluated to false , the code inside { } is skipped.
Note: The code inside { } is also called the body of the if statement.
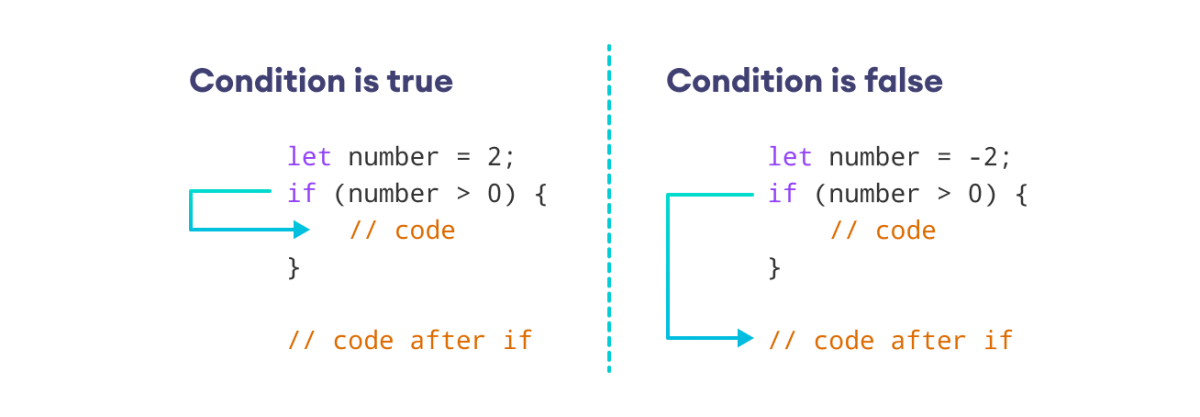
Example 1: JavaScript if Statement
Sample Output 1
In the above program, when we enter 5 , the condition number > 0 evaluates to true . Thus, the body of the if statement is executed.
Sample Output 2
Again, when we enter -1 , the condition number > 0 evaluates to false . Hence, the body of the if statement is skipped.
Since console.log("nice number"); is outside the body of the if statement, it is always executed.
Note: We use comparison and logical operators in our if conditions. To learn more, you can visit JavaScript Comparison and Logical Operators .
- JavaScript else Statement
We use the else keyword to execute code when the condition specified in the preceding if statement evaluates to false .
The syntax of the else statement is:
The if...else statement checks the condition and executes code in two ways:
- If condition is true , the code inside if is executed. And, the code inside else is skipped.
- If condition is false , the code inside if is skipped. Instead, the code inside else is executed.
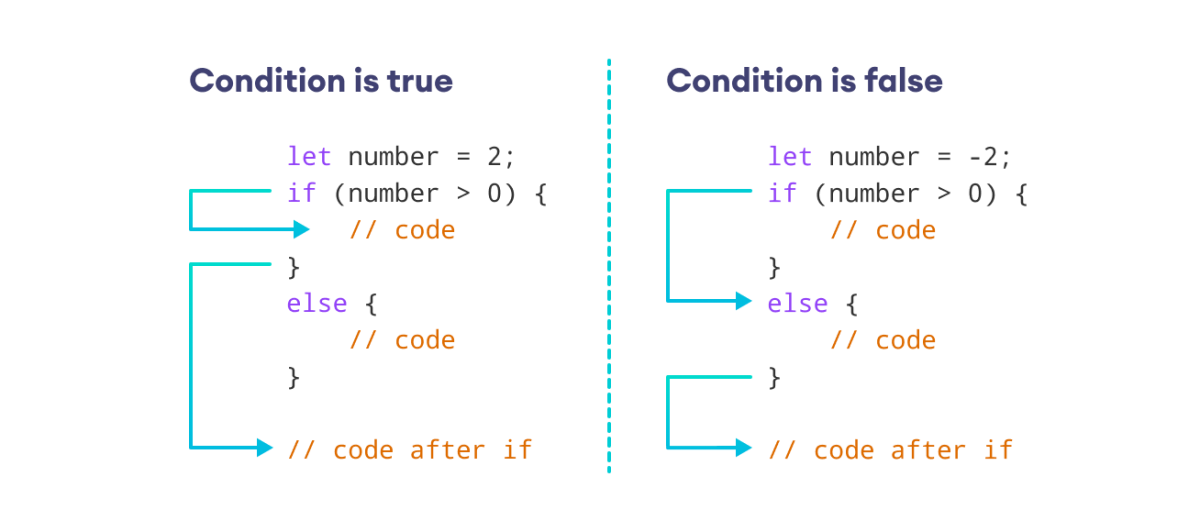
Example 2: JavaScript if…else Statement
In the above example, the if statement checks for the condition age >= 18 .
Since we set the value of age to 17 , the condition evaluates to false .
Thus, the code inside if is skipped. And, code inside else is executed.
We can omit { } in if…else statements when we have a single line of code to execute. For example,
- JavaScript else if Statement
We can use the else if keyword to check for multiple conditions.
The syntax of the else if statement is:
- First, the condition in the if statement is checked. If the condition evaluates to true , the body of if is executed, and the rest is skipped.
- Otherwise, the condition in the else if statement is checked. If true , its body is executed and the rest is skipped.
- Finally, if no condition matches, the block of code in else is executed.
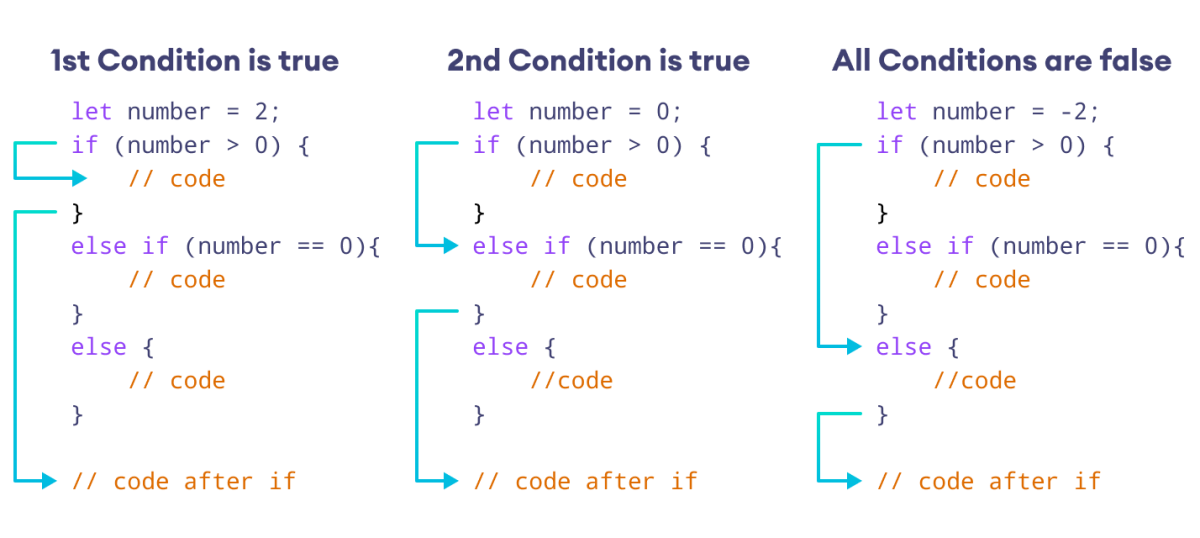
Example 3: JavaScript if...else if Statement
In the above example, we used the if statement to check for the condition rating <= 2 .
Likewise, we used the else if statement to check for another condition, rating >= 4 .
Since the else if condition is satisfied, the code inside it is executed.
We can use the else if keyword as many times as we want. For example,
In the above example, we used two else if statements.
The second else if statement was executed as its condition was satisfied.
- Nested if...else Statement
When we use an if...else statement inside another if...else statement, we create a nested if...else statement. For example,
Outer if...else
In the above example, the outer if condition checks if a student has passed or failed using the condition marks >= 40 . If it evaluates to false , the outer else statement will print Failed .
On the other hand, if marks >= 40 evaluates to true , the program moves to the inner if...else statement.
Inner if...else statement
The inner if condition checks whether the student has passed with distinction using the condition marks >= 80 .
If marks >= 80 evaluates to true , the inner if statement will print Distinction .
Otherwise, the inner else statement will print Passed .
Note: Avoid nesting multiple if…else statements within each other to maintain code readability and simplify debugging.
More on JavaScript if...else Statement
We can use the ternary operator ?: instead of an if...else statement if the operation we're performing is very simple. For example,
can be written as
We can replace our if…else statement with the switch statement when we deal with a large number of conditions.
For example,
In the above example, we used if…else to evaluate five conditions, including the else block.
Now, let's use the switch statement for the same purpose.
As you can see, the switch statement makes our code more readable and maintainable.
In addition, switch is faster than long chains of if…else statements.
We can use logical operators such as && and || within an if statement to add multiple conditions. For example,
Here, we used the logical operator && to add two conditions in the if statement.
Table of Contents
- Introduction
Video: JavaScript if...else
Sorry about that.
Related Tutorials
JavaScript Tutorial
- Skip to main content
- Select language
- Skip to search
Assignment within the conditional expression
The if statement executes a statement if a specified condition is truthy. If the condition is falsy, another statement can be executed.
Description
Multiple if...else statements can be nested to create an else if clause. Note that there is no elseif (in one word) keyword in JavaScript.
To see how this works, this is how it would look like if the nesting were properly indented:
To execute multiple statements within a clause, use a block statement ( { ... } ) to group those statements. In general, it is a good practice to always use block statements, especially in code involving nested if statements:
Do not confuse the primitive boolean values true and false with truthiness or falsiness of the Boolean object. Any value that is not undefined , null , 0 , NaN , or the empty string ( "" ), and any object, including a Boolean object whose value is false, is considered truthy when used as the condition. For example:
Using if...else
Using else if.
Note that there is no elseif syntax in JavaScript. However, you can write it with a space between else and if :
It is advisable to not use simple assignments in a conditional expression, because the assignment can be confused with equality when glancing over the code. For example, do not use the following code:
If you need to use an assignment in a conditional expression, a common practice is to put additional parentheses around the assignment. For example:
Specifications
Browser compatibility.
- conditional operator
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Home » JavaScript Tutorial » JavaScript if else if
JavaScript if else if
Summary : In this tutorial, you will learn how to use the JavaScript if...else...if statement to check multiple conditions and execute the corresponding block if a condition is true .
Introduction to the JavaScript if else if statement
The if an if…else statements accepts a single condition and executes the if or else block accordingly based on the condition.
To check multiple conditions and execute the corresponding block if a condition is true , you use the if...else...if statement like this:
In this syntax, the if...else...if statement has three conditions. In theory, you can have as many conditions as you want to, where each else...if branch has a condition.
The if...else...if statement checks the conditions from top to bottom and executes the corresponding block if the condition is true .
The if...else...if statement stops evaluating the remaining conditions as soon as a condition is true . For example, if the condition2 is true , the if...else...if statement won’t evaluate the condition3 .
If all the conditions are false , the if...else...if statement executes the block in the else branch.
The following flowchart illustrates how the if...else...if statement works:
JavaScript if else if examples
Let’s take some examples of using the if...else...if statement.
1) A simple JavaScript if…else…if statement example
The following example uses the if...else...if statement to get the month name from a month number:
In this example, we compare the month with 12 numbers from 1 to 12 and assign the corresponding month name to the monthName variable.
Since the month is 6 , the expression month==6 evaluates to true . Therefore, the if...else...if statement assigns the literal string 'Jun' to the monthName variable. Therefore, you see Jun in the console.
If you change the month to a number that is not between 1 and 12, you’ll see the Invalid Month in the console because the else clause will execute.
Conditional branching: if, '?'
Sometimes, we need to perform different actions based on different conditions.
To do that, we can use the if statement and the conditional operator ? , that’s also called a “question mark” operator.
The “if” statement
The if(...) statement evaluates a condition in parentheses and, if the result is true , executes a block of code.
For example:
In the example above, the condition is a simple equality check ( year == 2015 ), but it can be much more complex.
If we want to execute more than one statement, we have to wrap our code block inside curly braces:
We recommend wrapping your code block with curly braces {} every time you use an if statement, even if there is only one statement to execute. Doing so improves readability.
Boolean conversion
The if (…) statement evaluates the expression in its parentheses and converts the result to a boolean.
Let’s recall the conversion rules from the chapter Type Conversions :
- A number 0 , an empty string "" , null , undefined , and NaN all become false . Because of that they are called “falsy” values.
- Other values become true , so they are called “truthy”.
So, the code under this condition would never execute:
…and inside this condition – it always will:
We can also pass a pre-evaluated boolean value to if , like this:
The “else” clause
The if statement may contain an optional else block. It executes when the condition is falsy.
Several conditions: “else if”
Sometimes, we’d like to test several variants of a condition. The else if clause lets us do that.
In the code above, JavaScript first checks year < 2015 . If that is falsy, it goes to the next condition year > 2015 . If that is also falsy, it shows the last alert .
There can be more else if blocks. The final else is optional.
Conditional operator ‘?’
Sometimes, we need to assign a variable depending on a condition.
For instance:
The so-called “conditional” or “question mark” operator lets us do that in a shorter and simpler way.
The operator is represented by a question mark ? . Sometimes it’s called “ternary”, because the operator has three operands. It is actually the one and only operator in JavaScript which has that many.
The syntax is:
The condition is evaluated: if it’s truthy then value1 is returned, otherwise – value2 .
Technically, we can omit the parentheses around age > 18 . The question mark operator has a low precedence, so it executes after the comparison > .
This example will do the same thing as the previous one:
But parentheses make the code more readable, so we recommend using them.
In the example above, you can avoid using the question mark operator because the comparison itself returns true/false :
Multiple ‘?’
A sequence of question mark operators ? can return a value that depends on more than one condition.
It may be difficult at first to grasp what’s going on. But after a closer look, we can see that it’s just an ordinary sequence of tests:
- The first question mark checks whether age < 3 .
- If true – it returns 'Hi, baby!' . Otherwise, it continues to the expression after the colon “:”, checking age < 18 .
- If that’s true – it returns 'Hello!' . Otherwise, it continues to the expression after the next colon “:”, checking age < 100 .
- If that’s true – it returns 'Greetings!' . Otherwise, it continues to the expression after the last colon “:”, returning 'What an unusual age!' .
Here’s how this looks using if..else :
Non-traditional use of ‘?’
Sometimes the question mark ? is used as a replacement for if :
Depending on the condition company == 'Netscape' , either the first or the second expression after the ? gets executed and shows an alert.
We don’t assign a result to a variable here. Instead, we execute different code depending on the condition.
It’s not recommended to use the question mark operator in this way.
The notation is shorter than the equivalent if statement, which appeals to some programmers. But it is less readable.
Here is the same code using if for comparison:
Our eyes scan the code vertically. Code blocks which span several lines are easier to understand than a long, horizontal instruction set.
The purpose of the question mark operator ? is to return one value or another depending on its condition. Please use it for exactly that. Use if when you need to execute different branches of code.
if (a string with zero)
Will alert be shown?
Yes, it will.
Any string except an empty one (and "0" is not empty) becomes true in the logical context.
We can run and check:
The name of JavaScript
Using the if..else construct, write the code which asks: ‘What is the “official” name of JavaScript?’
If the visitor enters “ECMAScript”, then output “Right!”, otherwise – output: “You don’t know? ECMAScript!”
Demo in new window
Show the sign
Using if..else , write the code which gets a number via prompt and then shows in alert :
- 1 , if the value is greater than zero,
- -1 , if less than zero,
- 0 , if equals zero.
In this task we assume that the input is always a number.
Rewrite 'if' into '?'
Rewrite this if using the conditional operator '?' :
Rewrite 'if..else' into '?'
Rewrite if..else using multiple ternary operators '?' .
For readability, it’s recommended to split the code into multiple lines.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
JS Reference
Html events, html objects, other references, javascript if...else.
If the hour is less than 20, output "Good day":
Output "Good day" or "Good evening":
More examples below.
Description
The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed.
The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions.
In JavaScript we have the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true
- Use else to specify a block of code to be executed, if the same condition is false
- Use else if to specify a new condition to test, if the first condition is false
- Use switch to select one of many blocks of code to be executed
The if statement specifies a block of code to be executed if a condition is true:
The else statement specifies a block of code to be executed if the condition is false:
The else if statement specifies a new condition if the first condition is false:
Parameter Values
Advertisement
More Examples
If time is less than 10:00, create a "Good morning" greeting, if not, but time is less than 20:00, create a "Good day" greeting, otherwise a "Good evening":
If the first <div> element in the document has an id of "myDIV", change its font-size:
Change the value of the source attribute (src) of an <img> element, if the user clicks on the image:
Display a message based on user input:
Validate input data:
Related Pages
JavaScript Tutorial: JavaScript If...Else Statements
JavaScript Tutorial: JavaScript Switch Statement
Browser Support
if...else is an ECMAScript1 (ES1) feature.
ES1 (JavaScript 1997) is fully supported in all browsers:

COLOR PICKER

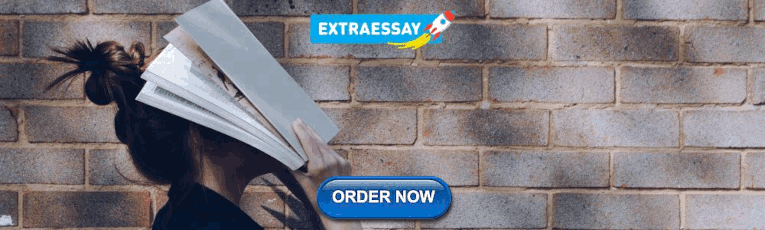
Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
How to Use If Statements in JavaScript – a Beginner's Guide

JavaScript is a powerful and versatile programming language that is widely used for creating dynamic and interactive web pages.
One of the fundamental building blocks of JavaScript programming is the if statement. if statements allow developers to control the flow of their programs by making decisions based on certain conditions.
In this article, we will explore the basics of if statements in JavaScript, understand their syntax, and see how they can be used to create more responsive and intelligent code.
What is an if Statement?
An if statement is a conditional statement that allows you to execute a block of code only if a specified condition is true. In other words, it provides a way to make decisions in your code.
For example, you might want to display a message to the user if they have entered the correct password, or you might want to perform a certain action only if a variable has a specific value.
Here's a simple example to illustrate the basic structure of an if statement:
In this example, the if statement checks whether the value of the temperature variable is greater than 20. If the condition is true, the code inside the curly braces ( {} ) is executed, and the message "It's a warm day!" is logged to the console.
Syntax of if Statements
The syntax of an if statement in JavaScript is straightforward. It consists of the if keyword followed by a set of parentheses containing a condition. If the condition evaluates to true , the code inside the curly braces is executed. Here is the basic syntax:
Let's break down the components of the if statement:
- if keyword: This is the keyword that starts the if statement and is followed by a set of parentheses.
- Condition: Inside the parentheses, you provide the condition that you want to evaluate. This condition should result in a boolean value ( true or false ).
- Code block: The code block is enclosed in curly braces {} . If the condition is true, the code inside the block is executed.
It's important to note that the curly braces are essential, even if the block contains only one statement. Including the braces makes your code more readable and helps prevent bugs that can occur when adding more statements to the block later.
Simple if Statements
Let's dive deeper into simple if statements by exploring some examples. Simple if statements consist of a single condition and a block of code that executes when the condition is true.
Example 1: Checking a Number
In this example, the if statement checks whether the value of the number variable is greater than 0. If the condition is true, the message "The number is positive." is logged to the console.
Example 2: Verifying User Input
This example uses the prompt function to get the user's age as input. The if statement then checks whether the entered age is greater than or equal to 18. If true, the message "You are eligible to vote." is logged to the console.
Example 3: Checking Equality
In this example, the if statement checks whether the value of the password variable is exactly equal to the string "secure123". If the condition is true, the message "Access granted." is logged to the console.
If-Else Statements
While simple if statements are useful, often you'll want to provide an alternative action when the condition is false. This is where the if-else statement comes in handy. The else block contains code that executes when the if condition is false.
Example: If-Else Statement
In this example, the if statement checks whether the value of the hour variable is less than 12. If true, it logs "Good morning!" to the console. Otherwise, it logs "Good afternoon!".
If-Else If Statements
Sometimes, you may have multiple conditions to check. In such cases, you can use else if statements to add additional conditions. The code inside the first true condition block encountered will be executed, and subsequent conditions will be ignored.
Example: If-Else If Statement
In this example, the code determines a student's grade based on their score. It first checks if the grade is greater than or equal to 90, and if true, it logs "A". If not, it moves to the next condition and checks if the grade is greater than or equal to 80, and so on. If none of the conditions are true, it logs "D".
Nested if Statements
You can also nest if statements inside other if statements to create more complex decision structures. Each if statement within the nested structure adds an additional layer of conditions.
Example: Nested if Statement
In this example, the outer if statement checks if it's summer. If true, it enters the nested structure and checks the temperature. Depending on the temperature, it logs different messages. If it's not summer, it logs "It's not summer."
Logical Operators in if Statements
JavaScript provides logical operators ( && , || , ! ) that allow you to combine multiple conditions in a single if statement.
Example: Logical Operators
In this example, the && (logical AND) operator combines two conditions: the person's age being 18 or older and having a valid license. If both conditions are true, it logs "You are eligible to drive." Otherwise, it logs "You are not eligible to drive."
Switch Statements
In situations where you have multiple possible conditions to check against a single value, a switch statement can be more concise than a series of if-else if statements.
Example: Switch Statement
In this example, the switch statement checks the value of dayOfWeek and executes the corresponding block of code. If none of the cases match, the default block is executed.
Common Mistakes with if Statements
While using if statements, beginners often make some common mistakes. Let's address a few of them:
Mistake 1: Forgetting the Equality Operator
The above code is incorrect because it uses the assignment operator = instead of the equality operator === inside the if condition. The correct code should be if (x === 10) .
Mistake 2: Misplacing Curly Braces
In this example, only the first console.log statement is part of the if block because the curly braces are missing. To include both statements in the if block, use curly braces:
Mistake 3: Confusing = and ==
The above code is incorrect because it uses the assignment operator = instead of the equality operator == inside the if condition. The correct code should be if (z == 5) .
Understanding and mastering if statements is essential for any JavaScript developer. These conditional statements provide the logic that enables your programs to make decisions and respond dynamically to different situations.
Whether you're validating user input, controlling the flow of your application, or handling different cases, if statements are a fundamental tool in your programming arsenal.
As you continue your journey in JavaScript development, practice using if statements in various scenarios. Experiment with different conditions, nesting structures, and logical operators to become comfortable and proficient in utilizing these powerful tools.
With a solid understanding of if statements, you'll be well-equipped to build more dynamic and responsive web applications.
frontend developer || technical writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript if-else
- JavaScript if else else if
- JavaScript Basics
- JavaScript Boolean
- Code Golfing in JavaScript
- Difference Between == & === in JavaScript
- Why “0” is equal to false in JavaScript ?
- JavaScript isFinite() Function
- JavaScript isNaN() Function
- Advanced JavaScript Backend Basics
- What are the Gotchas in JavaScript ?
- NOT(~) Bitwise Operator in JavaScript
- Control Statements in JavaScript
- JavaScript Quiz | Set-3
- JavaScript Quiz | Set-2
- JavaScript Set has() Method
- 7 Loops of JavaScript
- Swift - If-else Statement
- Bash Scripting - Else If Statement
- Swift - If-else-if Statement
JavaScript if-else or conditional statements will perform some action for a specific condition. If the condition is true then a particular block of action will be executed otherwise it will execute another block of action that satisfies that particular condition.
Such control statements are used to cause the flow of execution to advance and branch based on changes to the state of a program.
Example: This example describes the if-statement in JavaScript.
JavaScript’s conditional statements
Table of Content
JavaScript if-statement
Javascript if-else statement, javascript nested-if statement, javascript if-else-if ladder statement.
We will understand each conditional statement, its syntax, flowchart, and examples. Please refer to the Switch Case in JavaScript article to understand the switch case. Let’s begin with the if-statement.
It is a conditional statement used to decide whether a certain statement or block of statements will be executed or not i.e. if a certain condition is true then a block of statements is executed otherwise not.
The if statement accepts boolean values – if the value is true then it will execute the block of statements under it. If we do not provide the curly braces ‘{‘ and ‘}’ after if( condition ) then by default if statement considers the immediate one statement to be inside its block. For example,
Flow chart:
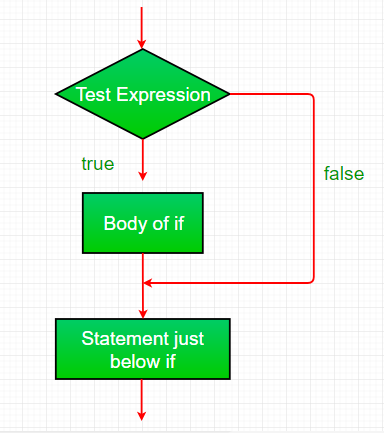
if-condition statement
Example: Here is a simple example demonstrating the if statement.
The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won’t. But what if we want to do something else if the condition is false? Here comes the else statement. We can use the else statement with the if statement to execute a block of code when the condition is false.
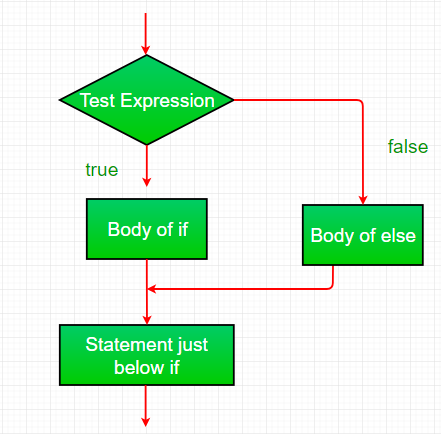
if-else statement
Example: This example describes the if-else statement in Javascript.
JavaScript allows us to nest if statements within if statements. i.e, we can place an if statement inside another if statement. A nested if is an if statement that is the target of another if or else.
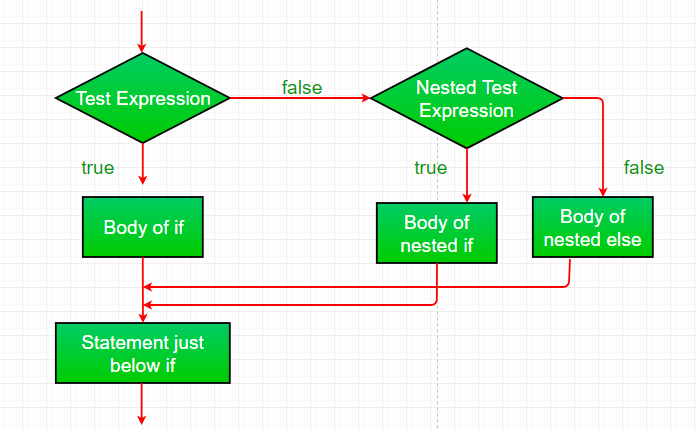
nested-if statement
Example : This example describes the nested-if statement in Javascript.
Here, a user can decide among multiple options. The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed.
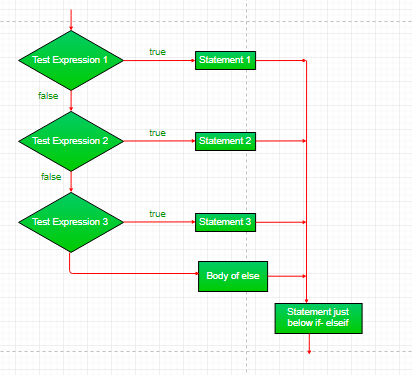
if-else-if ladder statement
Example: This example describes the if-else-if ladder statement in Javascript.
Supported Browsers
- Google Chrome 1.0
- Firefox 1.0
- Microsoft Edge 12.0
Please Login to comment...
Similar reads.
- javascript-basics
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Contact Mentor
Projects + Tutorials on React JS & JavaScript
10 JavaScript If else exercises with solution
1. check if a number is odd or even in javascript.
Function `isEvenOrOdd()` checks if input number is even or odd by using “%” operator in JavaScript.
- Print “Number is even” if the number is divisible by 2.
- Else print “Number is odd” if the number returns a remainder when divided by 2.
2. Check if input variable is a number or not
Function `isNumber()` checks if input variable is a number by using isNaN() in-built JavaScript function. Read more about isNan() from w3schools.com/jsref/jsref_isnan.asp .
- Print “Variable is not a number” if isNaN() returns true.
- Else print “Variable is a valid number” if isNaN() returns false.
3. Find the largest of two number
Function `findLargest()` finds the largest between two number by using “>” and “=” operator in JavaScript.
- Print num1 is the largest if num1>num2.
- Print num2 is the largest if num1<num2.
- Else print num1 and num2 are equal when num1==num2.
4. Find the largest of three number
Function `findLargest()` finds the largest of three number by using “>” and “&&” operator in JavaScript.
- Print num1 is the largest if num1>num2 and num1>num3.
- Print num2 is the largest if num2<num3.
- Else print num3.
5. Check if a triangle is equilateral, scalene, or isosceles
Function `findTriangleType()` finds the type of the triangle for given side values by using “==” and “&&” operator in JavaScript.
- Print “Equilateral triangle.” if values for all side1, side2 and side3 are equal.
- Print “Isosceles triangle.” if values for side1 is equal to side2 or side2 is equal to side3
- Else “Scalene triangle.” since values of all sides are unequal.
6. Find the a number is present in given range
Function `checkInRange()` finds if the given number is within the provided start and end range using >=, <= and && operators in JavaScript.
- Print “Between the range” if num is between start and end values
- Else Print “Outside the range” since num is outside start and end values.
7. Perform arithmetic operations on two numbers
Function `evalNumbers()` prints the result after evaluating arithmetic operations between two numbers of addition, multiplication, division, and modulus in JavaScript.
- Print result of num1+num2 if operation is “add”
- Print result of num1-num2 if operation is “subtract”
- Print result of num1*num2 if operation is “multiply”
- Print result of num1/num2 if operation is “divide”
- Print result of num1%num2 if operation is “modulus”
- Else print “Invalid operation”
8. Find check if a year is leap year or not
Function `checkLeapYear()` find if the given year is a leap year or not by using %, !=, && and || operators in JavaScript.
- If year is divisble by 4 and not divisble by 100 then print “leap year”.
- Or if year is divisible by 400 then print “leap year”.
- Else print “not a leap year”.
9. Find the grade for input marks
Function `findGrade()` to find the grade of the student based on the input marks.
- Print “S grade” if marks is between 90 and 100.
- Print “A grade” if marks is between 80 and 90.
- Print “B grade” if marks is between 70 and 80.
- Print “C grade” if marks is between 60 and 70.
- Print “D grade” if marks is between 50 and 60.
- Print “E grade” if marks is between 40 and 50.
- Print “Student has failed” if marks is between 0 and 40.
- Else print “Invalid marks”.
10. Find number of days in a given month
Function `findDaysInMonth()` finds the number of days in a given month of a year.
- If month is outside the range of 1 and 12 print “Invalid month”.
- If month is equal to 2 ie, February print “29 days” if leap year else print “28 days” .
- Else if month is equal to 4, 6, 9 or 11 print “30 days”.
- Else print “31 days”.
Video for Reference

Related Articles
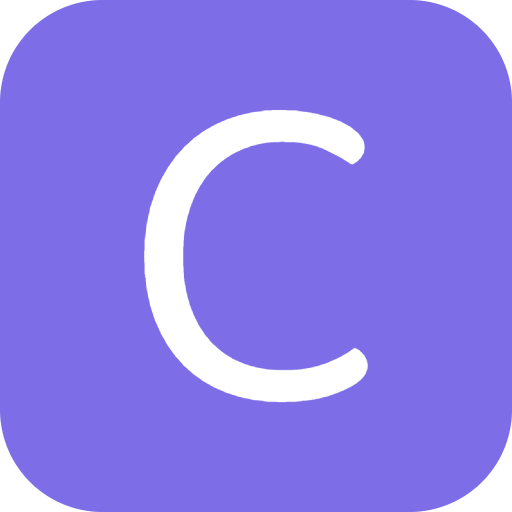
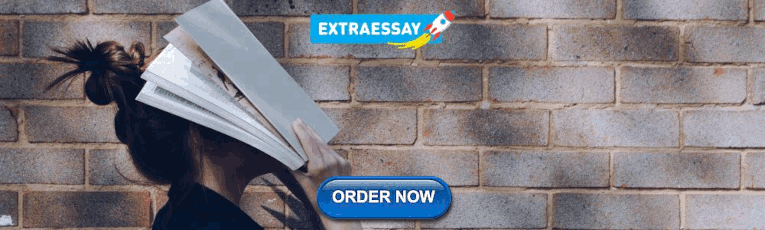
IMAGES
VIDEO
COMMENTS
statement1. else. statement2. An expression that is considered to be either truthy or falsy. Statement that is executed if condition is truthy. Can be any statement, including further nested if statements. To execute multiple statements, use a block statement ( { /* ... */ }) to group those statements.
This is a one-line shorthand for an if-else statement. It's called the conditional operator. 1. ... but it contain two or more lines and cannot assign to a variable. Javascript have a solution for this Problem Ternary Operator. Ternary Operator can write in one line and assign to a variable. Example:
In JavaScript we have the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false.
The JavaScript if...else statement is used to execute/skip a block of code based on a condition. Here's a quick example of the if...else statement. You can read the rest of the tutorial if you want to learn about if...else in greater detail. Example. let score = 45;
Summary: in this tutorial, you will learn how to use the JavaScript if...else statement to execute a block based on a condition.. Introduction to the JavaScript if…else statement. The if statement executes a block if a condition is true.When the condition is false, it does nothing.But if you want to execute a statement if the condition is false, you can use an if...else statement.
Note that there is no elseif syntax in JavaScript. However, you can write it with a space between else and if ... (x > 50) { /* do the right thing */ } else { /* do the right thing */ } Assignment within the conditional expression. It is advisable to not use simple assignments in a conditional expression, because the assignment can be confused ...
Introduction to the JavaScript if else if statement. The if an if ... In this example, we compare the month with 12 numbers from 1 to 12 and assign the corresponding month name to the monthName variable. Since the month is 6, the expression month==6 evaluates to true. Therefore, the if ...
It may be difficult at first to grasp what's going on. But after a closer look, we can see that it's just an ordinary sequence of tests: The first question mark checks whether age < 3.; If true - it returns 'Hi, baby!'.Otherwise, it continues to the expression after the colon ":", checking age < 18.; If that's true - it returns 'Hello!'. ...
The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed. The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions. In JavaScript we have the following conditional ...
"Else" statements: where if the same condition is false it specifies the execution for a block of code. "Else if" statements: this specifies a new test if the first condition is false. Now that you have the basic JavaScript conditional statement definitions, let's show you examples of each.
There are times in JavaScript where you might consider using a switch statement instead of an if else statement. switch statements can have a cleaner syntax over complicated if else statements. Take a look at the example below - instead of using this long if else statement, you might choose to go with an easier to read switch statement.
JavaScript is a powerful and versatile programming language that is widely used for creating dynamic and interactive web pages. One of the fundamental building blocks of JavaScript programming is the if statement.if statements allow developers to control the flow of their programs by making decisions based on certain conditions.. In this article, we will explore the basics of if statements in ...
The JavaScript if, else, and else if statements are part of the conditional statements available in JavaScript. These conditional statements are used to execute a particular block of code based on the passed condition. It will execute the code statements only if the condition turns to true.
0. Instead of the ternary operator, you could use Logical AND assignment (&&=). Basic examples. let output = false; output &&= true; console.log(output) Reassignment is not possible because output has been defined as false. const b = 10; const max = 1;
JavaScript if-else or conditional statements will perform some action for a specific condition. If the condition is true then a particular block of action will be executed otherwise it will execute another block of action that satisfies that particular condition. Such control statements are used to cause the flow of execution to advance and ...
10 JavaScript If else exercises with solution. 1. Check if a number is odd or even in JavaScript. Function `isEvenOrOdd ()` checks if input number is even or odd by using "%" operator in JavaScript. Print "Number is even" if the number is divisible by 2.
JavaScript Assignment - Conditionals (IF/Else Statements) 6. Javascript if expression evaluation. 1. JavaScript assignment and conditional on one line. 36. Variable assignment inside an 'if' condition in JavaScript. 2. Assigning the value of a variable inside the condition of an if statemment. 0.
Henry Longfellow still in 2,000 Guineas, but Royal Ascot assignment more likely Updated / Tuesday, 21 May 2024 17:06. Henry Longfellow winning last season's National Stakes at the Curragh.
Here's the assignment: Write the JavaScript code in one HTML document using IF, and IF/Else statements for the following three situations. For each one make sure to write comments for each section. Determine tax rate based on income and what the tax would be on the income. Variable declarations section 1.
If you just want an inline IF (without the ELSE), you can use the logical AND operator: (a < b) && /*your code*/; If you need an ELSE also, use the ternary operation that the other people suggested. edited Jul 15, 2015 at 20:31. answered Jul 14, 2015 at 3:51. Nahn.