What's the Difference Between the Assignment (`=`) and Equality (`==`, `===`) Operators?
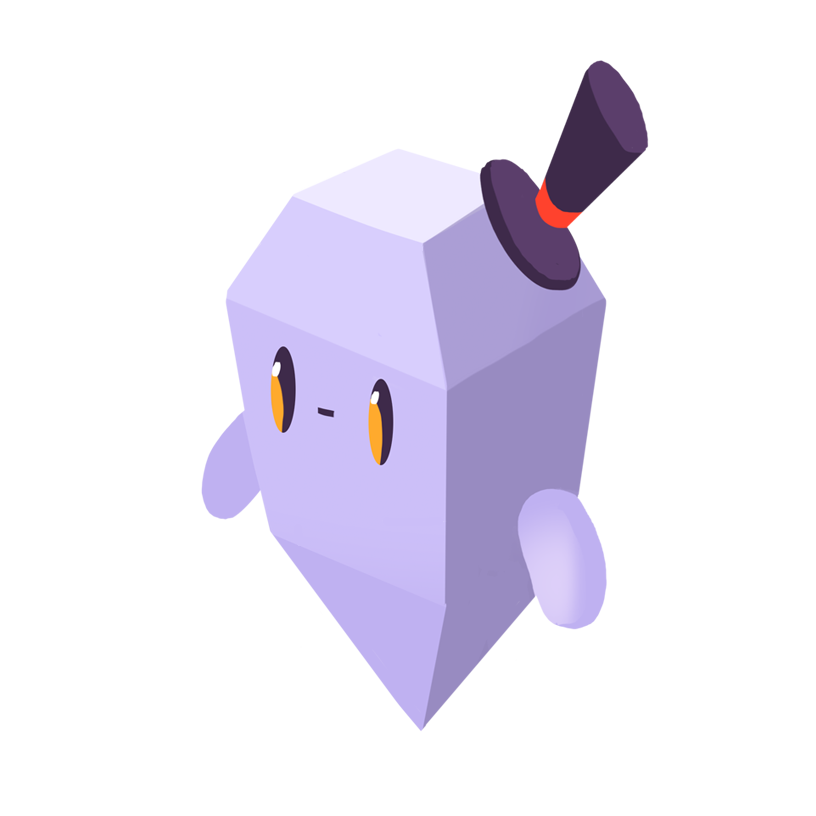
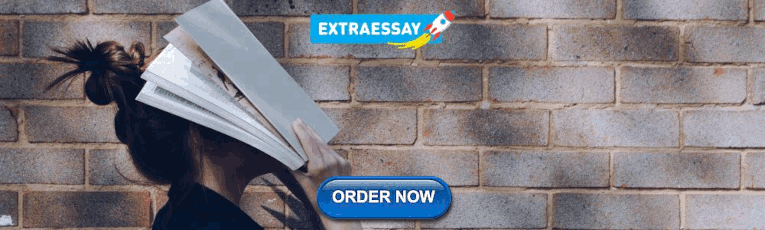
.css-13lojzj{position:absolute;padding-right:0.25rem;margin-left:-1.25rem;left:0;height:100%;display:-webkit-inline-box;display:-webkit-inline-flex;display:-ms-inline-flexbox;display:inline-flex;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;display:none;} .css-b94zdx{width:1rem;height:1rem;} The Problem
People who are new to programming often find it difficult to understand the difference between = , == , and === .
In mathematics we only use = , so what do the other two mean?
The Solution
The = is an assignment operator, while == and === are called equality operators.
Assignment Operator ( = )
In mathematics and algebra, = is an equal to operator. In programming = is an assignment operator , which means that it assigns a value to a variable.
For example, the following code will store a value of 5 in the variable x :
We can combine the declaration and assignment in one line:
It may look like the assignment operator works the same way as algebra’s equal to operator, but that’s not the case.
For example, the following doesn’t make any sense in algebra:
But it is acceptable in JavaScript (and other programming languages). JavaScript will take the expression on the right-hand side of the operator x + 4 and store this value in x again.
Equality Operator ( == )
In JavaScript, the operator that compares two values is written like this: == . It is called an equality operator . The equality operator is one of the many comparison operators in JavaScript that are used in logical and conditional statements.
The equality operator returns true or false based on whether the operands (the values being compared) are equal.
For example, the following code will return false :
Interestingly, if we compare an integer 5 and a string "5" it returns true .
That is because in most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for comparison. This behavior generally results in comparing the operands numerically.
Strict Equality Operator ( === )
Like the equality operator above, the strict equality operator compares the two values. But unlike the equality operator, the strict equality operator compares both the content and the type of the operands.
So using the strict equality operator, 5 and "5" are not equal.
It is better to use the strict equality operator to prevent type conversions, which may result in unexpected bugs. But if you’re certain the types on both sides will be the same, there is no problem with using the shorter operator.
Get Started With Sentry
Get actionable, code-level insights to resolve JavaScript performance bottlenecks and errors.
Create a free Sentry account
Create a JavaScript project and note your DSN
Grab the Sentry JavaScript SDK
- Configure your DSN
Loved by over 4 million developers and more than 90,000 organizations worldwide, Sentry provides code-level observability to many of the world’s best-known companies like Disney, Peloton, Cloudflare, Eventbrite, Slack, Supercell, and Rockstar Games. Each month we process billions of exceptions from the most popular products on the internet.
Related Answers
- Are there any issues with using `async`/`await` with `forEach()` loops in JavaScript?
- Asynchronous Callbacks in JavaScript
- Capturing JavaScript Errors
- How do I check if an element is hidden in jQuery?
- Check if a variable is a string in JavaScript
- Fixing ChunkLoadErrors in JavaScript
- Comments in JSON
- Warning: componentWillMount has been Renamed, and is not Recommended for Use
- Convert Unix timestamp to date and time in JavaScript
- Default function parameter values in JavaScript
A better experience for your users. An easier life for your developers.
A peek at your privacy.
Here’s a quick look at how Sentry handles your personal information (PII).
Who we collect PII from
We collect PII about people browsing our website, users of the Sentry service, prospective customers, and people who otherwise interact with us.
What if my PII is included in data sent to Sentry by a Sentry customer (e.g., someone using Sentry to monitor their app)? In this case you have to contact the Sentry customer (e.g., the maker of the app). We do not control the data that is sent to us through the Sentry service for the purposes of application monitoring.
PII we may collect about you
- PII provided by you and related to your
- Account, profile, and login
- Requests and inquiries
- PII collected from your device and usage
- PII collected from third parties (e.g., social media)
How we use your PII
- To operate our site and service
- To protect and improve our site and service
- To provide customer care and support
- To communicate with you
- For other purposes (that we inform you of at collection)
Third parties who receive your PII
We may disclose your PII to the following type of recipients:
- Subsidiaries and other affiliates
- Service providers
- Partners (go-to-market, analytics)
- Third-party platforms (when you connect them to our service)
- Governmental authorities (where necessary)
- An actual or potential buyer
We use cookies (but not for advertising)
- We do not use advertising or targeting cookies
- We use necessary cookies to run and improve our site and service
- You can disable cookies but this can impact your use or access to certain parts of our site and service
Know your rights
You may have the following rights related to your PII:
- Access, correct, and update
- Object to or restrict processing
- Opt-out of marketing
- Be forgotten by Sentry
- Withdraw your consent
- Complain about us
If you have any questions or concerns about your privacy at Sentry, please email us at [email protected] .
If you are a California resident, see our Supplemental notice .
- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
Assignment vs Equality
Kenneth Leroy Busbee
Assignment sets and/or re-sets the value stored in the storage location denoted by a variable name. [1] Equality is a relational operator that tests or defines the relationship between two entities. [2]
Most control structures use a test expression that executes either selection (as in the: if then else) or iteration (as in the while; do while; or for loops) based on the truthfulness or falseness of the expression. Thus, we often talk about the Boolean expression that is controlling the structure. Within many programming languages, this expression must be a Boolean expression and is governed by a tight set of rules. However, in many programming languages, each data type can be used as a Boolean expression because each data type can be demoted into a Boolean value by using the rule/concept that zero and nothing represent false and all non-zero values represent true.
Within various languages, we have the potential added confusion of the equals symbol = as an operator that does not represent the normal math meaning of equality that we have used for most of our life. The equals symbol typically means: assignment. To get the equality concept of math we often use two equal symbols to represent the relational operator of equality. Let’s consider:
The test expression of the control structure will always be true because the expression is an assignment (not the relational operator of == ). It assigns the ‘y’ to the variable pig, then looks at the value in pig and determines that it is not zero; therefore the expression is true. And it will always be true and the else part will never be executed. This is not what the programmer had intended. The correct syntax for a Boolean expression is:
This example reminds you that you must be careful in creating your test expressions so that they are indeed a question, usually involving the relational operators. Some programming languages will generate a warning or an error when an assignment is used in a Boolean expression, and some do not.
Don’t get caught using assignment for equality.
- cnx.org: Programming Fundamentals – A Modular Structured Approach using C++
- Wikipedia: Assignment (computer science) ↵
- Wikipedia: Relational operator ↵
Programming Fundamentals Copyright © 2018 by Kenneth Leroy Busbee is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License , except where otherwise noted.
Share This Book
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript operators.
Javascript operators are used to perform different types of mathematical and logical computations.
The Assignment Operator = assigns values
The Addition Operator + adds values
The Multiplication Operator * multiplies values
The Comparison Operator > compares values
JavaScript Assignment
The Assignment Operator ( = ) assigns a value to a variable:
Assignment Examples
Javascript addition.
The Addition Operator ( + ) adds numbers:
JavaScript Multiplication
The Multiplication Operator ( * ) multiplies numbers:
Multiplying
Types of javascript operators.
There are different types of JavaScript operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- String Operators
- Logical Operators
- Bitwise Operators
- Ternary Operators
- Type Operators
JavaScript Arithmetic Operators
Arithmetic Operators are used to perform arithmetic on numbers:
Arithmetic Operators Example
Arithmetic operators are fully described in the JS Arithmetic chapter.
Advertisement
JavaScript Assignment Operators
Assignment operators assign values to JavaScript variables.
The Addition Assignment Operator ( += ) adds a value to a variable.
Assignment operators are fully described in the JS Assignment chapter.
JavaScript Comparison Operators
Comparison operators are fully described in the JS Comparisons chapter.
JavaScript String Comparison
All the comparison operators above can also be used on strings:
Note that strings are compared alphabetically:
JavaScript String Addition
The + can also be used to add (concatenate) strings:
The += assignment operator can also be used to add (concatenate) strings:
The result of text1 will be:
When used on strings, the + operator is called the concatenation operator.
Adding Strings and Numbers
Adding two numbers, will return the sum, but adding a number and a string will return a string:
The result of x , y , and z will be:
If you add a number and a string, the result will be a string!
JavaScript Logical Operators
Logical operators are fully described in the JS Comparisons chapter.
JavaScript Type Operators
Type operators are fully described in the JS Type Conversion chapter.
JavaScript Bitwise Operators
Bit operators work on 32 bits numbers.
The examples above uses 4 bits unsigned examples. But JavaScript uses 32-bit signed numbers. Because of this, in JavaScript, ~ 5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
Bitwise operators are fully described in the JS Bitwise chapter.
Test Yourself With Exercises
Multiply 10 with 5 , and alert the result.
Start the Exercise
Test Yourself with Exercises!
Exercise 1 » Exercise 2 » Exercise 3 » Exercise 4 » Exercise 5 »

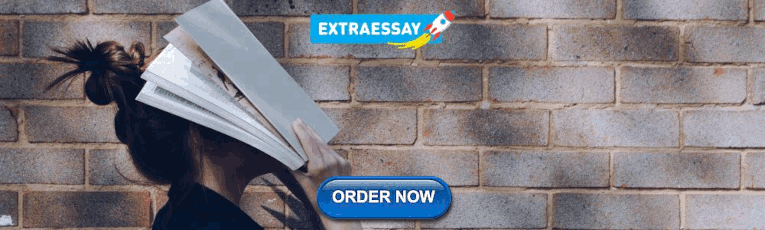
COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
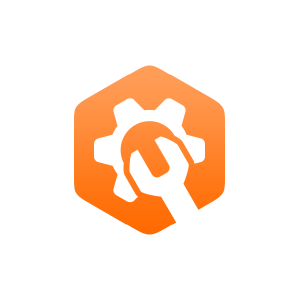
4.6: Assignment Operator
- Last updated
- Save as PDF
- Page ID 29038
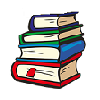
- Patrick McClanahan
- San Joaquin Delta College
Assignment Operator
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
As we have seen, assignment operators are used to assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=” : This is the simplest assignment operator, which was discussed above. This operator is used to assign the value on the right to the variable on the left. For example: a = 10; b = 20; ch = 'y';
If initially the value 5 is stored in the variable a, then: (a += 6) is equal to 11. (the same as: a = a + 6)
If initially value 8 is stored in the variable a, then (a -= 6) is equal to 2. (the same as a = a - 6)
If initially value 5 is stored in the variable a,, then (a *= 6) is equal to 30. (the same as a = a * 6)
If initially value 6 is stored in the variable a, then (a /= 2) is equal to 3. (the same as a = a / 2)
Below example illustrates the various Assignment Operators:
Definitions
Adapted from: "Assignment Operator" by Kenneth Leroy Busbee , (Download for free at http://cnx.org/contents/[email protected] ) is licensed under CC BY 4.0
Difference between equal to (==) and assignment operator (=) in Python
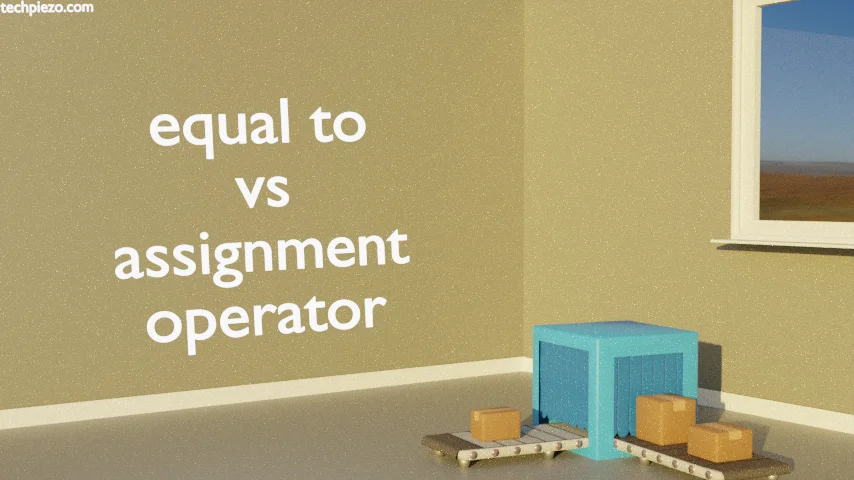
In this article, we would discuss the difference between equal to (==) and assignment operator (=) in Python. In programming languages, we need to assign values to the variables this is achieved through assignment operator . Furthermore, to compare values stored in variables we use comparison operators. Want to know about comparison operators in Python ? equal to comes under the category of comparison operators.
If you have just started with Python then it may look confusing in the beginning. But, these are pretty easy to use once we understand what we are trying to achieve with both these operators. So, we start with the basic introduction followed by relevant examples.
Firstly, equal to (==) operator – as already discussed it is a comparison operator. It is used to check if two values/variables are equal. For instance, string “abc” is equal to string “abc” but not equal to string “xyz”. If the condition satisfies then, it returns with True otherwise False. In addition to, it won’t always be plain True/False outcome. At times, we would ask the interpreter to execute a specific code if a condition satisfies otherwise something else. Even for those cases, the equal to (==) operator works just fine.
Now, understand it with couple of examples next. We compare an integer with integer and then string with a string.
For both, it would return with True .
As expected, it returns with False . We go a step further and use if..else statement .
It would return with –
Note: Please take care of indentation in above code otherwise it would result in an error.
Here, if..else statement checks whether x is equal to 1 or not. And, shows the outcome accordingly. If you want to know more about if..else statement in Python .
Secondly, assignment (=) operator – these are used to assign values to the variable. If we want to write an efficient and clutter-free code then, we have to assign values to the variables. And, these variables can later be used to perform various operations. So, this is how it works –
Clearly, we assigned some value to x and y variables. Later, these we printed using print() method.
In conclusion , we have covered difference between equal to and assignment operators operator in Python here.
Similar Posts
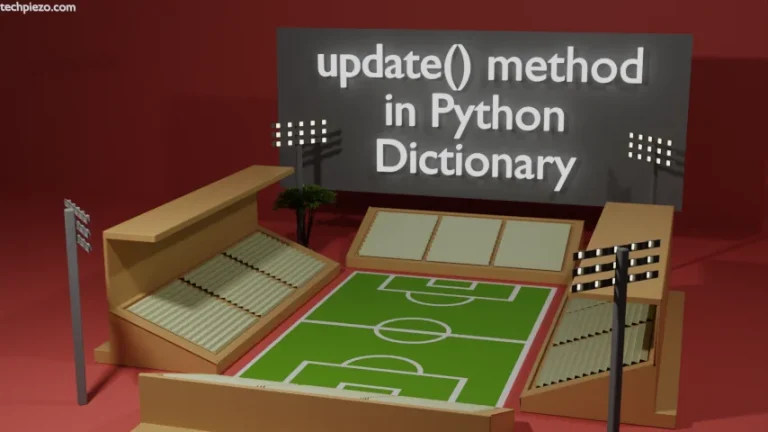
update() method in Python Dictionary
If you have been following us along, then you would have noticed that we couldn’t make changes to the value…
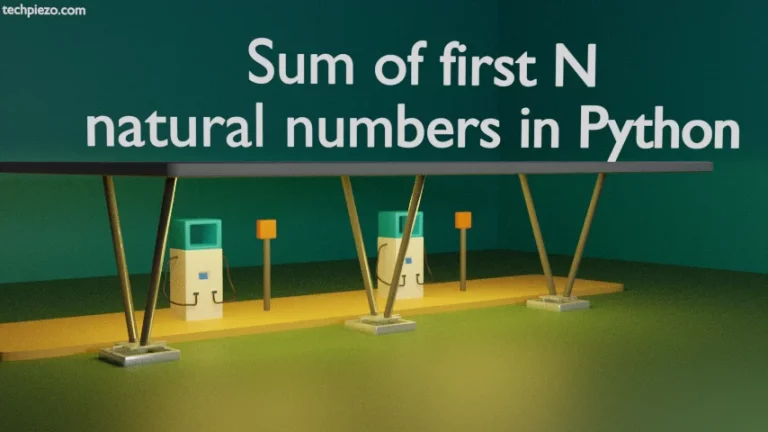
Sum of first N natural numbers in Python
In this article, we cover how to write a Python program that helps us calculate the sum of the first…
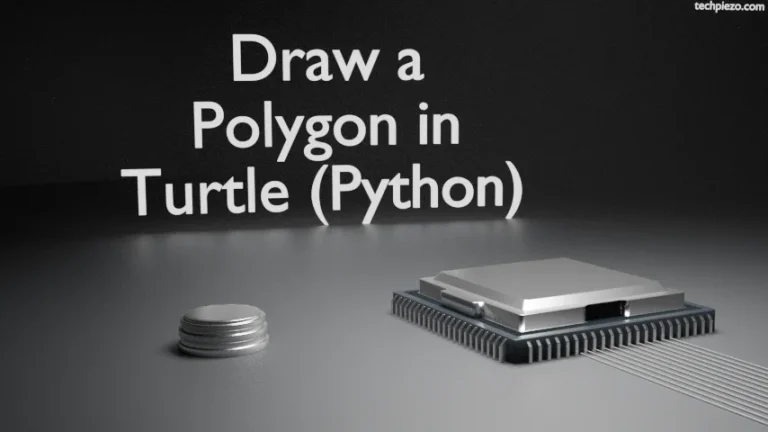
Draw a polygon in Turtle (Python)
In this article, we would discuss how to draw a polygon in Turtle. Turtle is basically an inbuilt module in…
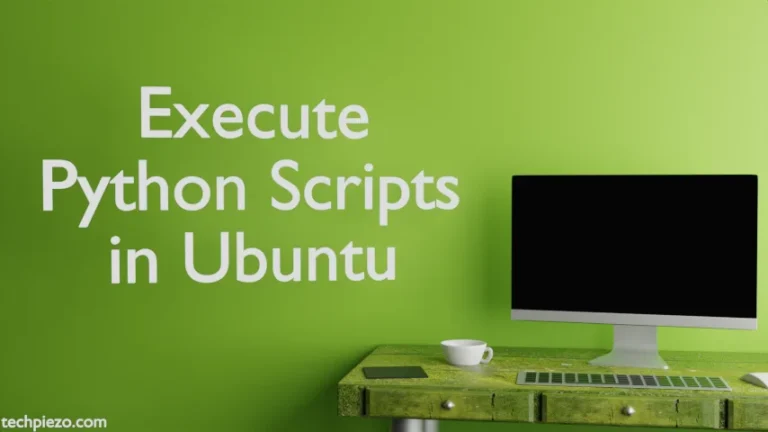
Execute Python Scripts in Ubuntu
In this article, we would discuss how to execute Python scripts in Ubuntu. A Python script contains an instruction set….
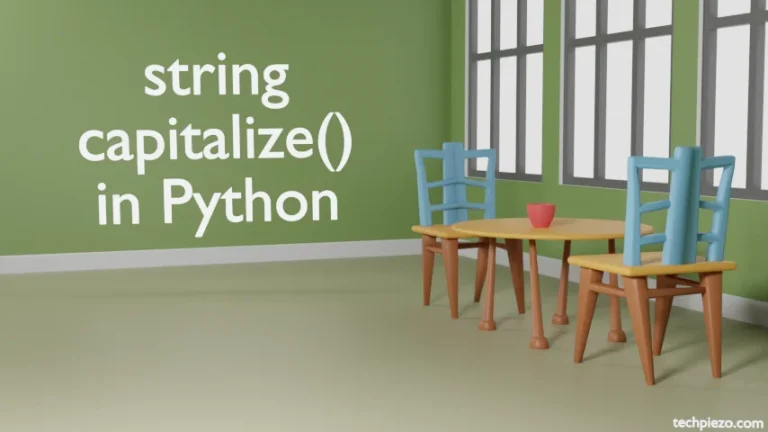
string capitalize() in Python
In this article, we would cover string capitalize() method in Python. The capitalize() method returns a string wherein all the…
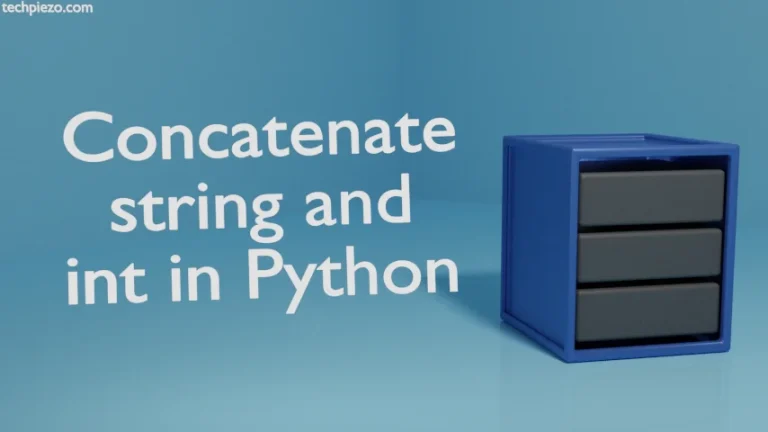
Concatenate String and Int in Python
We consider this article to be an extension to the article – Concatenate Strings in Python. In that article, we…
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
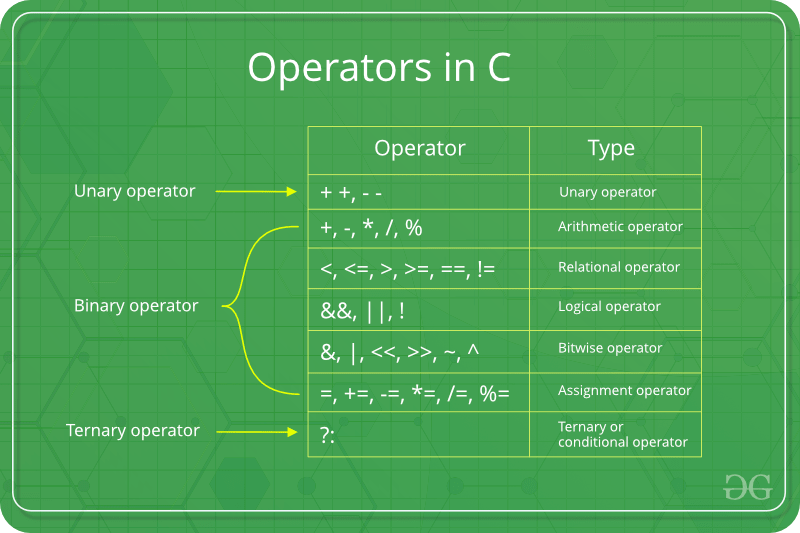
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
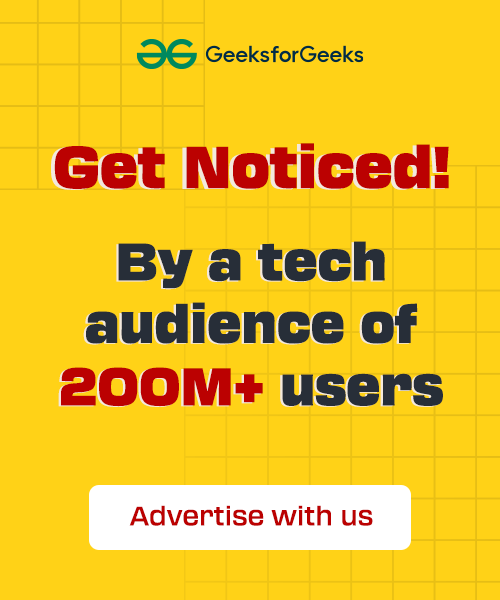
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
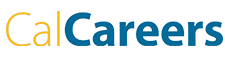
- Site Search:
- Site Search
- Help/Tutorials
- Settings
- Messages
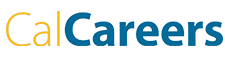
Job Posting: Caltrans Equipment Operator II
Department of Transportation
$4,741.00 - $5,720.00 per Month
Final Filing Date: 5/8/2024
Job Description and Duties
Under the direction of a Caltrans Maintenance Supervisor, the Caltrans Equipment Operator II (CEO II) will operate and service vehicles requiring a Class A commercial driver's license with tank (N) endorsement, and performs work related to highway maintenance, structures maintenance, construction, and Landscape Maintenance. When not operating the specified equipment, the Equipment Operator II may perform any of the duties outlined under Caltrans Equipment Operator I, Caltrans Highway or Landscape Maintenance Worker, and perform other related highway maintenance work. The CEO II maintains knowledge of all current mandated and applicable safety training and standards, policies, practices, directives, and expectations, and complies with all departmental training and safe work practice requirements. The CEO II may be assigned to other operations units and/or geographical areas as operations needs dictate. Overnight travel out of town up to 80 percent of the time may be required to meet operational needs. The incumbent will operate, and service highway maintenance equipment identified as Levels of Equipment for Caltrans Equipment Operator I and II. The incumbent will operate appropriate equipment to conduct asphalt and concrete repair and preventative maintenance operations related to SB-1. Will carry out the installation and repair of signs, fence and guardrail and may perform striping, pavement marking, culvert, and bridge-related work. The incumbent may be required to perform snow and ice control tasks including truck, grader, or rotary plowing, repair and removal, chain control operations, apply de-icing materials, and the construction and repair of traction control devices. May be required to operate a mobile sweeper as the designated operator for all cost centers within the Superintendents' area. The incumbent maintains knowledge of all current mandated and applicable safety training and standards, policies, practices, directives, and expectations, and complies with all departmental training and safe work practice requirements.
Eligibility for hire may be determined by your score on the Caltrans Equipment Operator II Exam. For those who do not have current eligibility (e.g., transfer, permissive reinstatement, or voluntary demotions) and/or who will be new to state civil services employment, you must be on the state examination list to be eligible for these positions. The Caltrans Equipment Operator II Exam is located here: https://www.calcareers.ca.gov/CalHrPublic/Exams/Bulletin.aspx?examCD=9PB20
T he Human Resources Contact is available to answer questions regarding the application process. The Hiring Unit Contact is available to answer questions regarding the position.
Permanent, intermittent positions are not subject to Post and Bid. A permanent, intermittent employee may work up to 1,500 hours in any 12 consecutive months. The number of hours and schedule of work shall be determined based upon the operational needs of each department.
Apply today to join our team! We especially encourage applicants to check out our Caltrans Career Compass tool! The Caltrans Career Compass is a tool to assist and help candidates understand and succeed in applying to Caltrans positions.
You will find additional information about the job in the Duty Statement .
Working Conditions
This position is located in Jamestown/ Sonora, Groveland and/or Long Barn, Tuolumne County
The incumbent may be exposed to and work in loud noise, dust, chemicals, extreme weather conditions, great heights, confined spaces, uneven and unstable terrain, and next to vehicle traffic. The incumbent will be required to wear all personal protective equipment and follow all policies, and procedures. Will be required to work rotating or irregular shifts, including weekends, nights, holidays, overtime, and be able to respond to after-hour emergencies.
New to State candidates will be hired into the minimum salary of the classification or minimum of alternate range when applicable.
Minimum Requirements
- CALTRANS EQUIPMENT OPERATOR II
Additional Documents
- Job Application Package Checklist
- Duty Statement
Position Details
Department information.
Caltrans Mission : Provide a safe and reliable transportation network that serves all people and respects the environment.
Caltrans Vision: A brighter future for all through a world-class transportation network.
The Caltrans workforce is made up of diverse and unique individuals who contribute to our organizational success. Caltrans is about celebrating diversity, valuing one another, and recognizing that Caltrans is strong not in spite of the diverse attributes of our workforce, but because of our diversity.
Department Website: www.dot.ca.gov
Frequently Asked Questions for an Applicant: http://dot.ca.gov/jobs/docs/faq-ct-applicants-081617.pdf
Director’s EEO Policy : https://dot.ca.gov/programs/equal-employment-opportunity
Director’s EEO Policy Statement: https://dot.ca.gov/programs/equal-employment-opportunity
Special Requirements
- The position(s) require(s) a valid California Drivers License (CDL). You must answer the questions addressing your CDL on your application. Ensure you provide your CDL number, class, expiration date, and any endorsements and/or restrictions.
- The position(s) require(s) a Drug Screening be passed prior to being hired.
The position(s) require(s) a valid unrestricted Class A Commercial Driver’s License, With Tank endorsement [N].
Possession of Minimum Qualifications will be verified prior to interview and/or appointment. If you are basing your eligibility on education, you must include your unofficial transcript(s)/diploma for verification. Unofficial, original, or official sealed transcripts will be accepted and may be required upon appointment. Applicants with foreign transcripts/degrees must provide a transcript/degree U.S. equivalency report evaluation that indicates the number of units and degree to which the foreign coursework is equivalent. Here is a list of evaluation agencies: https://www.naces.org/members . Please redact birthdates and social security numbers.
Application Instructions
Completed applications and all required documents must be received or postmarked by the Final Filing Date in order to be considered. Dates printed on Mobile Bar Codes, such as the Quick Response (QR) Codes available at the USPS, are not considered Postmark dates for the purpose of determining timely filing of an application.
Who May Apply
How To Apply
Address for Mailing Application Packages
You may submit your application and any applicable or required documents to:
Address for Drop-Off Application Packages
You may drop off your application and any applicable or required documents at:
Required Application Package Documents
The following items are required to be submitted with your application. Applicants who do not submit the required items timely may not be considered for this job:
- Current version of the State Examination/Employment Application STD Form 678 (when not applying electronically), or the Electronic State Employment Application through your Applicant Account at www.CalCareers.ca.gov. All Experience and Education relating to the Minimum Qualifications listed on the Classification Specification should be included to demonstrate how you meet the Minimum Qualifications for the position.
- Resume is optional. It may be included, but is not required.
Click HERE to view the Benefits Summary for Civil Service Employees in the State of California.
Important Application Instructions
The STD. 678 is required, and each section must be filled out completely and thoroughly. For mailed or hand delivered applications to be considered for this position, you are required to include the Job Control (JC-427170), (PARF #10-4-023) and title of the position (Caltrans Equipment Operator II) on the State application (STD. 678) form.
Electronic applications through your CalCareers account are highly recommended and encouraged.
Candidates that meet the minimum qualifications based on possession of EDUCATION, LICENSE, OR CERTIFICATE must include a copy of your DEGREE/TRANSCRIPTS, LICENSE, or CERTIFICATE, along with your State application (STD. 678), to be considered for this position.
NOTE: Do not submit the “Equal Employment Opportunity” questionnaire (page 5) with your completed State application (STD. 678). This page is for examination use only. Do not include any confidential information on any documents you submit for this job vacancy, such as your state application, resume, or educational transcripts. Confidential information that should be excluded or removed from these documents includes, but is not limited to, your Social Security Number, birth date, driver’s license number, examination results, LEAP status, marital status, and age. The job application packet checklist is not required to apply for this position. Failure to follow these instructions may result in your application not being considered for this position.
Please take this 1-minute Caltrans Recruitment survey to tell us how you found out about this job!
https://www.surveymonkey.com/r/HMQ7LDR
https://youtu.be/oC9wIp8QalI
Equal Opportunity Employer
The State of California is an equal opportunity employer to all, regardless of age, ancestry, color, disability (mental and physical), exercising the right to family care and medical leave, gender, gender expression, gender identity, genetic information, marital status, medical condition, military or veteran status, national origin, political affiliation, race, religious creed, sex (includes pregnancy, childbirth, breastfeeding and related medical conditions), and sexual orientation.
It is an objective of the State of California to achieve a drug-free work place. Any applicant for state employment will be expected to behave in accordance with this objective because the use of illegal drugs is inconsistent with the law of the State, the rules governing Civil Service, and the special trust placed in public servants.
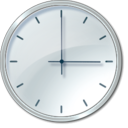
Automatic log out in
Select 'Stay Logged In' below to resume your activity.
You must enable Javascript to use this site.
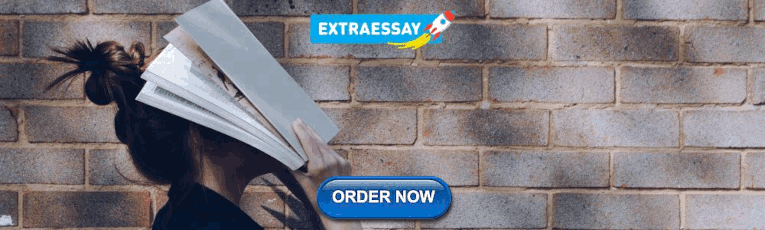
IMAGES
VIDEO
COMMENTS
The differences can be shown in tabular form as follows: =. ==. It is an assignment operator. It is a relational or comparison operator. It is used for assigning the value to a variable. It is used for comparing two values. It returns 1 if both the values are equal otherwise returns 0. Constant term cannot be placed on left hand side.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
The = operator is an assignment operator. You are assigning an object to a value. The == operator is a conditional equality operation. You are confirming whether two things have equal values. There is also a === operator. This compares not only value, but also type. Assignment Operators. Comparison Operators
The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand. That is, x = f() is an assignment expression that assigns the value of f() to x. There are also compound assignment operators that are shorthand for the operations listed in the following table: Name Shorthand operator
The = is an assignment operator, while == and === are called equality operators. Assignment Operator (=) In mathematics and algebra, = is an equal to operator. In programming = is an assignment operator, which means that it assigns a value to a variable. For example, the following code will store a value of 5 in the variable x:
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
The simple assignment operator is equal (=), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x. There are also compound assignment operators that are shorthand for the operations listed in the following table: Compound assignment operators; Name Shorthand operator Meaning;
Assignment vs Equality Kenneth Leroy Busbee. Overview. Assignment sets and/or re-sets the value stored in the storage location denoted by a variable name. [1] Equality is a relational operator that tests or defines the relationship between two entities. [2] Discussion. Most control structures use a test expression that executes either selection (as in the: if then else) or iteration (as in the ...
The Addition Assignment Operator (+=) adds a value to a variable. ... x = x ** y: Note. Assignment operators are fully described in the JS Assignment chapter. JavaScript Comparison Operators. Operator Description == equal to === equal value and equal type!= not equal!== not equal value or not equal type > greater than < less than >= greater ...
Assignment Operator. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Difference between equal to (==) and assignment operator (=) in Python. Firstly, equal to (==) operator - as already discussed it is a comparison operator. It is used to check if two values/variables are equal. For instance, string "abc" is equal to string "abc" but not equal to string "xyz". If the condition satisfies then, it ...
That said, to mean assignment, the =, :=, <-and ← operators are common across many languages descending from C, Pascal and BASIC. Vale uses a set keyword to mean reassignment. In languages where the concept of "(re)assignment" does not exist, like most Functional and Logical ones, it is not uncommon to see = be used both for bindings and as ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
Description. Logical AND assignment short-circuits, meaning that x &&= y is equivalent to x && (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not truthy, due to short-circuiting of the logical AND operator. For example, the following does not throw an error, despite x being const:
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
The assignment operator "is" the equal operator, and that is exactly what you are using. You might be getting confused by the order of operations in C. On your printf line, the expression y = x happens first, and the resulting value ( y) is used as a parameter in your printf function, which is why you see the value of y printed.
The State of California is an equal opportunity employer to all, regardless of age, ancestry, color, disability (mental and physical), exercising the right to family care and medical leave, gender, gender expression, gender identity, genetic information, marital status, medical condition, military or veteran status, national origin, political ...
Reading from "Introducing Monte Carlo Methods with R", by Robert and Casella: "The assignment operator is =, not to be confused with ==, which is the Boolean operator for equality.An older assignment operator is <-and, for compatibility reasons, it still remains functional, but it should be ignored to ensure cleaner programming. (As pointed out by Spector, P. (2009).
Job Description and Duties. Under the direction of a Caltrans Maintenance Supervisor, the Caltrans Equipment Operator II (CEO II) will operate and service vehicles requiring a Class A commercial driver's license with tank (N) endorsement, and performs work related to highway maintenance, structures maintenance, construction, and Landscape Maintenance.
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.