Set and Check User Rights Assignment via Powershell
You can add, remove, and check user rights assignment (remotely / locally) with the following powershell scripts..
Posted by : blakedrumm on Jan 5, 2022

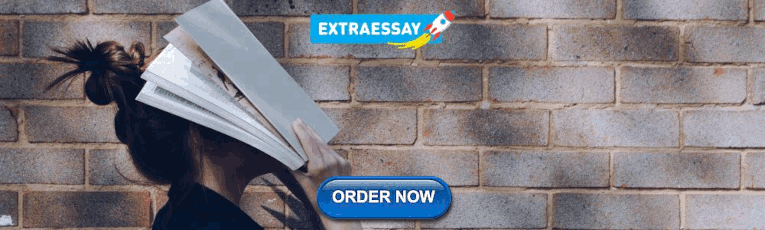
How to get it

Local Computer
Remote computer, output types.
This post was last updated on August 29th, 2022
I stumbled across this gem ( weloytty/Grant-LogonAsService.ps1 ) that allows you to grant Logon as a Service Right for a User. I modified the script you can now run the Powershell script against multiple machines, users, and user rights.
Set User Rights

All of the User Rights that can be set:
Note You may edit line 437 in the script to change what happens when the script is run without any arguments or parameters, this also allows you to change what happens when the script is run from the Powershell ISE.
Here are a few examples:
Add Users Single Users Example 1 Add User Right “Allow log on locally” for current user: . \Set-UserRights.ps1 -AddRight -UserRight SeInteractiveLogonRight Example 2 Add User Right “Log on as a service” for CONTOSO\User: . \Set-UserRights.ps1 -AddRight -Username CONTOSO\User -UserRight SeServiceLogonRight Example 3 Add User Right “Log on as a batch job” for CONTOSO\User: . \Set-UserRights.ps1 -AddRight -Username CONTOSO\User -UserRight SeBatchLogonRight Example 4 Add User Right “Log on as a batch job” for user SID S-1-5-11: . \Set-UserRights.ps1 -AddRight -Username S-1-5-11 -UserRight SeBatchLogonRight Add Multiple Users / Rights / Computers Example 5 Add User Right “Log on as a service” and “Log on as a batch job” for CONTOSO\User1 and CONTOSO\User2 and run on, local machine and SQL.contoso.com: . \Set-UserRights.ps1 -AddRight -UserRight SeServiceLogonRight , SeBatchLogonRight -ComputerName $ env : COMPUTERNAME , SQL.contoso.com -UserName CONTOSO\User1 , CONTOSO\User2
Remove Users Single Users Example 1 Remove User Right “Allow log on locally” for current user: . \Set-UserRights.ps1 -RemoveRight -UserRight SeInteractiveLogonRight Example 2 Remove User Right “Log on as a service” for CONTOSO\User: . \Set-UserRights.ps1 -RemoveRight -Username CONTOSO\User -UserRight SeServiceLogonRight Example 3 Remove User Right “Log on as a batch job” for CONTOSO\User: . \Set-UserRights.ps1 -RemoveRight -Username CONTOSO\User -UserRight SeBatchLogonRight Example 4 Remove User Right “Log on as a batch job” for user SID S-1-5-11: . \Set-UserRights.ps1 -RemoveRight -Username S-1-5-11 -UserRight SeBatchLogonRight Remove Multiple Users / Rights / Computers Example 5 Remove User Right “Log on as a service” and “Log on as a batch job” for CONTOSO\User1 and CONTOSO\User2 and run on, local machine and SQL.contoso.com: . \Set-UserRights.ps1 -RemoveRight -UserRight SeServiceLogonRight , SeBatchLogonRight -ComputerName $ env : COMPUTERNAME , SQL.contoso.com -UserName CONTOSO\User1 , CONTOSO\User2
Check User Rights
In order to check the Local User Rights, you will need to run the above (Get-UserRights), you may copy and paste the above script in your Powershell ISE and press play.
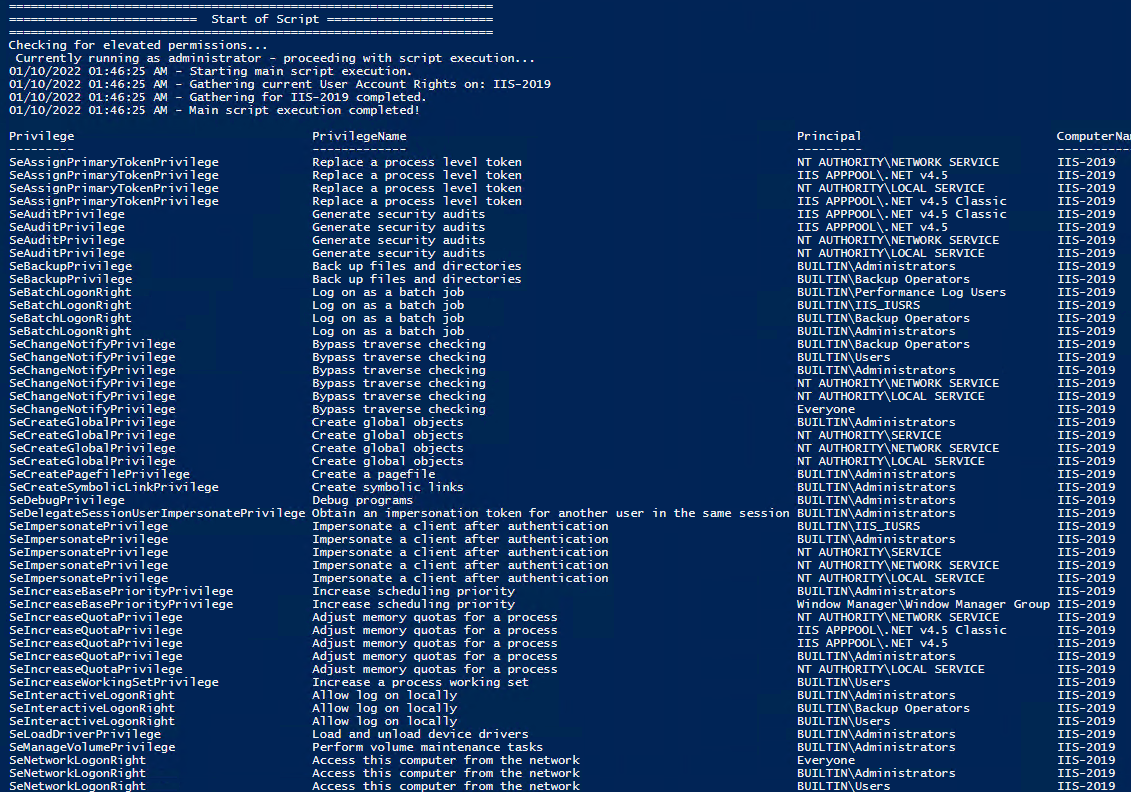
Note You may edit line 467 in the script to change what happens when the script is run without any arguments or parameters, this also allows you to change what happens when the script is run from the Powershell ISE.
Get Local User Account Rights and output to text in console:
Get Remote SQL Server User Account Rights:
Get Local Machine and SQL Server User Account Rights:
Output Local User Rights on Local Machine as CSV in ‘C:\Temp’:
Output to Text in ‘C:\Temp’:
PassThru object to allow manipulation / filtering:

I like to collaborate and work on projects. My skills with Powershell allow me to quickly develop automated solutions to suit my customers, and my own needs.
Email : [email protected]
Website : https://blakedrumm.com
My name is Blake Drumm, I am working on the Azure Monitoring Enterprise Team with Microsoft. Currently working to update public documentation for System Center products and write troubleshooting guides to assist with fixing issues that may arise while using the products. I like to blog on Operations Manager and Azure Automation products, keep checking back for new posts. My goal is to post atleast once a month if possible.
- operationsManager
- troubleshooting
- certificates

Managing User Rights in Powershell
Managing User Rights Assignments in Powershell
Windows User Rights, also known as Windows Privileges, are traditionally managed via GPO or in the simplest of cases via the server’s Local Security Policy. These assignments control special permissions that are often needed by IIS applications or other application hosting on Windows Servers.
So how can we manage these assignments in Powershell? There’s no obvious solution provided in Powershell, but there are several options are available. None of which are a pure Powershell solution, but some are close.
- Wrap the ntrights.exe process in Powershell. This is relatively simple, but the downside is having an external dependency on the ntrights.exe file.
- Embed a wrapper class to the LSA API in your script. This is a pretty good solution but certainly bloats your script.
- Load and Reference the Carbon DLL (If you haven’t already checked out this Powershell library, you should it is very powerful and regularly updated. I choose this approach because it keeps the script clean and compact, it returns an array of strings for easy interrogation. It does require a dependency on carbon.dll, but this library provides a ton of functionality beyond just this.
I like the 3rd option, its very clean, and I like working with the Carbon library.
Now lets take this script to the next level and wrap it into a DSC Script implementation. We can use this same logic in a DSC configuration to make sure our desired User Rights Assignments are kept in place.
What else can we do? We could also create a Custom DSC Resource to have a cleaner DSC Configuration.
Related Posts
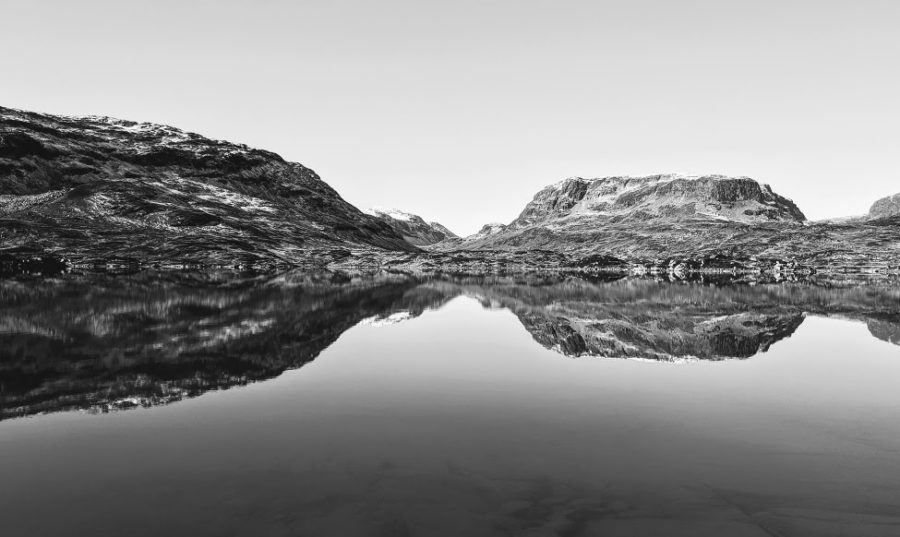
Local Administrator Audit Script
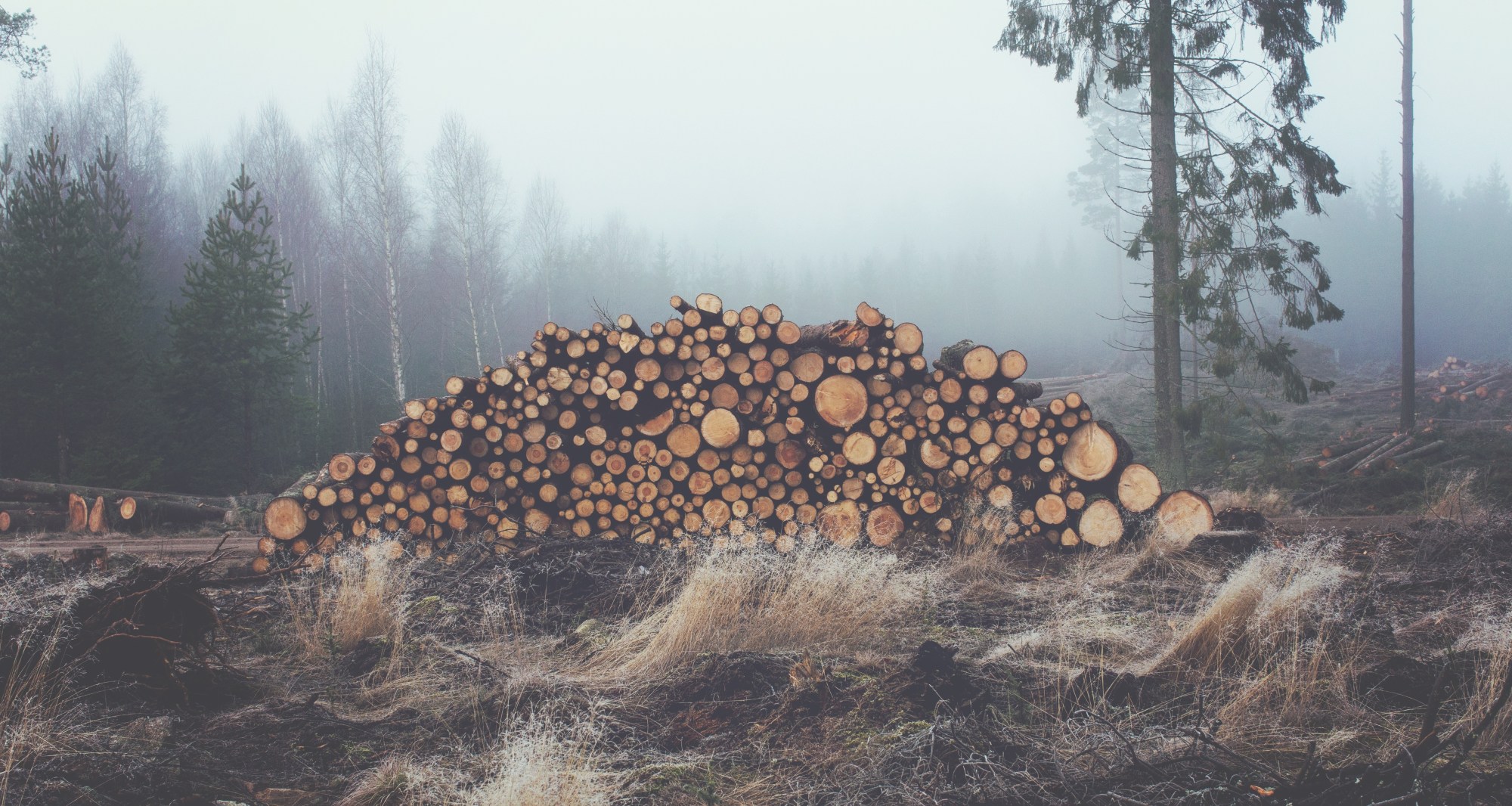
Powershell Log Archival Script
My new stories.
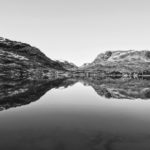
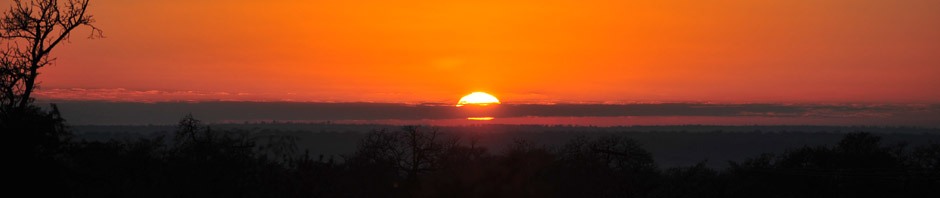
- PowerShell Forum Directory
- Publications
Managing Privileges using PoshPrivilege
A recent project of mine has been to write a module to manage privileges on a local system. What I came up is a module called PoshPrivilege that allows you to not only look at what user rights are available on a local or remote system, but also provide the ability to Add, Remove, Enable and Disable the privileges as well.
If you are running PowerShell V5, you can download this module from the PowerShell Gallery:
Otherwise, check out my GitHub page where I am maintaining this project:
https://github.com/proxb/PoshPrivilege
I won’t spend time talking about how I wrote this module and my reasons behind it. What I will say is that instead of writing out C# code and then using Add-Type to compile it, I went with the Reflection approach of building out everything from the pinvoke signatures for methods to the Structs and even the Enums.
Let’s get started by looking at what is available in this module. The first function that is available is Get-Privilege and it comes with a few parameters. This function’s purpose is to let you view what privileges are currently available on the system (local or remote) as well as what is currently applied to your current process token.

A quick run through of using this function with various parameters:

If this one looks familiar, then it is probably likely that you have used the following command:

I opted for boolean values instead to determine the state for easier filtering if needed.
Up next are the Enable/Disable-Privilege functions. These work to Enable or Disable the privileges that are currently available on your local system to your process token. This means that if something like SeDebugPrivilege isn’t available on your system (such as being removed via Group Policy), then you cannot use Enable-Privilege to add your process token to this privilege. As in the previous image where we can see what is enabled and disabled, these are the only privileges that are available for me to work with.
To show this point, I am going to enable both SeSecurityPrivilege and SeDebugPrivilege so you can see that while the first privilege will show as Enabled, the other will not appear as it has not been made available.

As you can see from the picture, SeSecurityPrivilege has been enabled as expected, but SeDebugPrivilege is nowhere to be found. If we want SeDebugPrivilege, we will need to go about this another way which will be shown shortly.
Disabling a privilege can be done using Disable-Privilege as shown in the example below.

Now that I have covered Enabling and Disabling of the privileges and their limitations, I will move onto the Add/Remove-Privilege functions which allow you to add a privilege for a user or group or remove them on a local system. Note that this only works up until it gets reverted if set by group policy. This will also note show up if you look at the privileges available on your current process token (you will log off and log back in to see it).
Remember that I do not have SeDebugPrivilege available to use? Well, now we can add it to my own account using Add-Privilege.

We can see it is now available, but as I mentioned before, it doesn’t show up in my current process. A logoff and login now shows that it is not only available, but already enabled.

With this now enabled, we could disable it as well if needed using Disable-Privilege. I added my account for show, but we can also add groups this was as well.
As with Adding a privilege, we can remove privileges as well using Remove-Privilege.

As with Add-Privilege, you will need to log off and log back in to see the change take effect on your account.
Again, you can install this module using Install-Module if running PowerShell V5 and this project is out on GitHub to download (and contribute to as well). Enjoy!
Share this:
4 responses to managing privileges using poshprivilege.
I downloaded the scripts from Github, but getting compile errors.
Specifically the errors are around the WInOS Structures listed below:
Unable to find type [LUID]: make sure that the assembly containing this type is loaded. Unable to find type [LSA_UNICODE_STRING]: make sure that the assembly containing this type is loaded. Unable to find type [LARGE_INTEGER]: make sure that the assembly containing this type is loaded. Unable to find type [LUID_AND_ATTRIBUTES]: make sure that the assembly containing this type is loaded. Unable to find type [TokPriv1Luid]: make sure that the assembly containing this type is loaded.
Unable to find type [TOKEN_INFORMATION_CLASS]: make sure that the assembly containing this type is loaded.
Unable to find type [ProcessAccessFlags]: make sure that the assembly containing this type is loaded.
BTW, I have posted the full error log @ https://docs.google.com/document/d/18boeWSbvlLwpoIAMTJAp0ooNaLxe6kniYrJr_q3ZNMQ/edit?usp=sharing
Just a question, how can I grant the SESecurityPrivilege to the Set-Acl process ? If I do a whoami /priv I can see my useraccount (PS –> run as administrator) I can see the privilege is enabled, but when I try to run the script I have I get the following error :
Set-Acl : The process does not possess the ‘SeSecurityPrivilege’ privilege which is required for this operation. At C:\Scripts\SESOG\ImportACLSEv2.ps1:16 char:16 + $acl | Set-Acl $path + ~~~~~~~~~~~~~ + CategoryInfo : PermissionDenied: (P:\Common:String) [Set-Acl], PrivilegeNotHeldException + FullyQualifiedErrorId : System.Security.AccessControl.PrivilegeNotHeldException,Microsoft.PowerShell.Commands.SetAclCommand
The script looks like this : $par = Import-Csv -Path “c:\scripts\sesog\ImportMainCC.csv” -Delimiter “;”
foreach ( $i in $par ) { $path= $i.Path $IdentityReference= $i.IdentityReference $AccessControlType=$i.AccessControlType $InheritanceFlags= $i.InheritanceFlags $PropagationFlags=$i.PropagationFlags $FileSystemRights=$i.FileSystemRights echo $path $IdentityReference $acl = Get-Acl $path $permission = $IdentityReference, $FileSystemRights, $InheritanceFlags, $PropagationFlags, $AccessControlType $accessRule = new-object System.Security.AccessControl.FileSystemAccessRule $permission $acl.SetAccessRule($accessRule) $acl | Set-Acl $path }
In the import csv a path is set and exported export rights from the original location (I am doing a fileserver migration) but on each of the folders mentioned the inherentance flag has been removed.
Pingback: PowerShell Magazine » The case of potentially broken PackageManagement for Windows 10 Insiders
You are a lifesaver! I have been fretting over how to manage service account rights on remote servers – each OU has a corresponding AD security group and GPO, and doing this manually is both time-consuming and fraught with error. I can’t wait to try this out. First PoshWSUS, then this …you rock.
Leave a comment Cancel reply
Translate this blog.
- Search for:
Recent Posts
- Dealing with Runspacepool Variable Scope Creep in PowerShell
- 2018 PowerShell Resolutions
- Quick Hits: Getting the Local Computer Name
- Recent Articles on MCPMag
- Quick Hits: Finding all Hyperlinks in an Excel Workbook
- Querying UDP Ports with PowerShell
- Changing Ownership of File or Folder Using PowerShell
- Avoiding System.Object[] (or Similar Output) when using Export-Csv
- Quick Hits: Ping Sweep One Liner
- Starting,Stopping and Restarting Remote Services with PowerShell
- Locating Mount Points Using PowerShell
- Building a Chart Using PowerShell and Chart Controls
- PowerShell and Excel: Adding Some Formatting To Your Report
- Quick Hits: Finding Exception Types with PowerShell
- Using PowerShell Parameter Validation to Make Your Day Easier
- background jobs
- Internet Explorer
- performance
- powerscripting
- Regular Expressions
- scripting games 2012
- scripting games 2013
- scripting guy
- winter scriting games 2014
Email Subscription
Enter your email address to subscribe to this blog and receive notifications of new posts by email.
Email Address:
Sign me up!
- 5,515,901 Visitors Since August 5, 2010
- Entries feed
- Comments feed
- WordPress.com
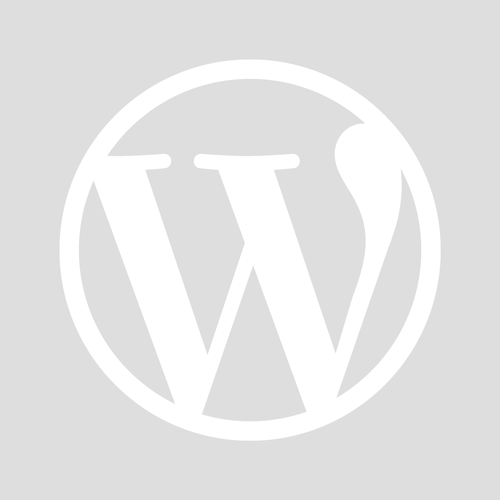
- Already have a WordPress.com account? Log in now.
- Subscribe Subscribed
- Copy shortlink
- Report this content
- View post in Reader
- Manage subscriptions
- Collapse this bar
List all user rights assignment permissions in domain for specific account
Hi, this might be an overwhelming task but it is good to know whether this is possible. I want to list all permissions which are given to specific account via GPOs (default domain policy and default domain controller policy). It is account used by backup software and in order to work properly this account had to be given bunch of permissions to (Create a token object, Log on as a batch job, etc.). Of course given permissions can be seen in Group Policy MMC but I am interested only in getting permissions given to that account and exporting these permissions to some file for the purpose of documenting them.
Thanks in advance.
You are talking about UserRights Assignments, not permissions. With that said, here is a nice looking module someone wrote that can do what you are asking. I have not tested it, but looks very promising. Of course you should evaluate their code and confirm it will pose not threat to your environment before using anything taken from the internet.
https://userrights.codeplex.com/
Maybe I am wrong but it seems this module does the job only for local user rights assignment on given computer (local security policy). I need PowerShell code to retrieve user rights assignments given to specific account via GPOs (rights assigned to account in both Default Domain Policy and Default Domain Controller Policy).
Well, the rights on the local computer will be the culmination of the applied GPO’s, so it would be the effective rights after the GPOs have applied. If you are wanting to specifically look at the GPOs themselves, you can use the GPO cmdlets. Specifically, if you are good at XML, you can use the Get-GPOReport cmdlet.
[xml]$report = Get-GPOReport -Name “Default Domain Policy” -ReportType XML
I know that Curtis :-), rights on local computer will be result of applied GPOs on that computer. If used, this module has to be copied to one domain member machine and one domain controller and tested on both of them. Since you can do virtually anything with PowerShell nowadays I assume there is some way to pull out user rights assignments from GPO and filter them based on specific account.
The only way I can think to get the gpo settings was in the second part of my previous statement. …
If you are wanting to specifically look at the GPOs themselves, you can use the GPO cmdlets. Specifically, if you are good at XML, you can use the Get-GPOReport cmdlet.
Related Topics
UserRightsAssignment
Analyze the effective User Rights Assignments on a computer and compare results
Minimum PowerShell version
Installation options.
- Install Module
- Install PSResource
- Azure Automation
- Manual Download
Copy and Paste the following command to install this package using PowerShellGet More Info
Copy and Paste the following command to install this package using Microsoft.PowerShell.PSResourceGet More Info
You can deploy this package directly to Azure Automation. Note that deploying packages with dependencies will deploy all the dependencies to Azure Automation. Learn More
Manually download the .nupkg file to your system's default download location. Note that the file won't be unpacked, and won't include any dependencies. Learn More
Copyright (c) 2021 Friedrich Weinmann
Package Details
- Friedrich Weinmann
Compare-UserRightsAssignment ConvertTo-UserRightsAssignmentSummary Get-DomainUserRightsAssignment Get-UserRightsAssignment Import-UserRightsAssignment
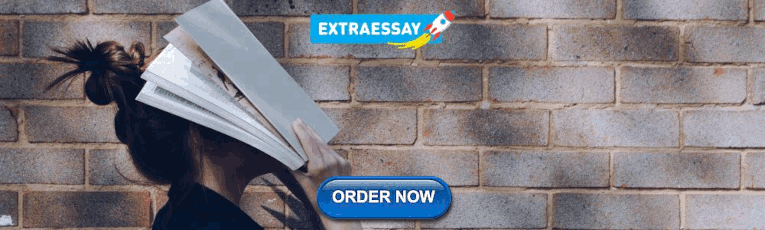
Dependencies
- PSFramework (>= 1.6.201)
- UserRightsAssignment.nuspec
- changelog.md
- UserRightsAssignment.psd1
- UserRightsAssignment.psm1
- bin\readme.md
- en-us\about_UserRightsAssignment.help.txt
- en-us\strings.psd1
- functions\Compare-UserRightsAssignment.ps1
- functions\ConvertTo-UserRightsAssignmentSummary.ps1
- functions\Get-DomainUserRightsAssignment.ps1
- functions\Get-UserRightsAssignment.ps1
- functions\Import-UserRightsAssignment.ps1
- functions\readme.md
- internal\configurations\configuration.ps1
- internal\configurations\readme.md
- internal\functions\readme.md
- internal\scriptblocks\scriptblocks.ps1
- internal\scripts\license.ps1
- internal\scripts\postimport.ps1
- internal\scripts\preimport.ps1
- internal\scripts\strings.ps1
- internal\tepp\assignment.ps1
- internal\tepp\example.tepp.ps1
- internal\tepp\readme.md
- tests\pester.ps1
- tests\readme.md
- tests\functions\readme.md
- tests\general\FileIntegrity.Exceptions.ps1
- tests\general\FileIntegrity.Tests.ps1
- tests\general\Help.Exceptions.ps1
- tests\general\Help.Tests.ps1
- tests\general\Manifest.Tests.ps1
- tests\general\PSScriptAnalyzer.Tests.ps1
- tests\general\strings.Exceptions.ps1
- tests\general\strings.Tests.ps1
- xml\readme.md
- xml\UserRightsAssignment.Format.ps1xml
- xml\UserRightsAssignment.Types.ps1xml
Version History
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
How to add a user group in the "Shut down the system" group policy in Windows Server by CMD or PowerShell
I've read some documentation on Microsoft and other sites. Some of them suggest GPRegistryValue for registry-based policies and other recommended third-party software.
The full path of the key is: "Computer Configuration\Windows Settings\Security Settings\Local Policies\User Rights Assignment"
But in my case I cannot use other packages except CMD or PowerShell (UI not available).
- group-policy
- windows-server

- superuser.com/questions/1254253/… and blakedrumm.com/blog/set-and-check-user-rights-assignment might help you for a starting point to play with. – Vomit IT - Chunky Mess Style Nov 25, 2022 at 21:25
- This is just local security policy settings. What did you search for as this is a common task? powershell 'Local User Rights Management' – postanote Nov 25, 2022 at 21:37
Windows provides the secedit.exe tool for this and or custom code, as per the link provided in my comment to you.
Also, did you check the mspowershellgallery.com site for modules that assist with local user security policy?
Update as per '@Vomit IT - Chunky Mess Style', suggestion.
The more succinct/elegant option.
FYI --- Update for '@Vomit IT - Chunky Mess Style'. Using the PS_LSA.Wrapper
- 1 @VomitIT-ChunkyMessStyle... update provided. – postanote Nov 25, 2022 at 21:43
- Oh yeah, now you're talking!!! I saw github examples of that Indented.SecurityPolicy you suggested listed there. I like it! – Vomit IT - Chunky Mess Style Nov 25, 2022 at 21:55
- 1 Yeppers, I've got a bunch of these I've collected, refactored, and written over the years in different engagements. Even one using the underlying OS PS_LSA Windows library. – postanote Nov 25, 2022 at 22:06
- Thanks for helping me.The module of 'SecurityPolicy' is available, but when I try to find its modules "Get-Command -Module 'SecurityPolicy'" nothing is listed. Thus, I can't execute 'Add-UserRightsAssignment'. – Daniel Teodoro Nov 29, 2022 at 13:38
- If you did this Get-Command -Module 'SecurityPolicy' , and you see nothing? If so, that means it's not installed/in your PSModulePath. Did you install the module as I show in my suggested answer? If not, then you need to. Then you use Get-Module -ListAvailable to validate it's on your system. – postanote Nov 30, 2022 at 6:37
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged powershell group-policy windows-server ..
- The Overflow Blog
- Climbing the GenAI decision tree sponsored post
- Diverting more backdoor disasters
- Featured on Meta
- New Focus Styles & Updated Styling for Button Groups
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network
- Google Cloud will be Sponsoring Super User SE
Hot Network Questions
- Late IRA contribution with changing filing status and income limits
- Is an LED driver IC just a boost conv with voltage across Rsense fed into FB?
- What were the major things that caused TCP/IP to become the internet standard protocol?
- How does a wireless charger work if there is no transfer of electrons?
- Does damage that "can't be reduced" bypass temporary hit points?
- How do I attach this master link on the chain?
- Integrality of a quotient of Fermat numbers
- Formula to count rows between positive values
- Why do Chinese people complicate the matter in choosing the words for translation
- Is there something between Debug and Release build?
- Why is there increased fear of Russia's attack against Latvia, Lithuania, Estonia after the invasion of Ukraine started?
- Replicate a "thermometer" with color gradient
- Get cumulative sums in a list without using a for loop
- What is a word for battery "longevity"?
- Can falsehood be measured? If so, would it be continuous or discrete?
- dashed line in tikz
- How to write a paper with word count limit?
- Ryanair seating policy: will two passengers be seated apart if you don't pay for a seat?
- Who has the right to examine a will?
- False information on Florida Criminal History Report, can I sue the state?
- Position of the text within a table
- Interpreting a notation in calculus of variations (differentiating with respect to a derivative)
- Linking number and intersection number
- Haste creature under The Akroan War chapter 2 ability
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Get-Cs User Policy Assignment
This cmdlet is used to return the policy assignments for a user, both directly assigned and inherited from a group.
Description
This cmdlets returns the effective policies for a user, based on either direct policy assignment or inheritance from a group policy assignment. For a given policy type, if an effective policy is not returned, this indicates that the effective policy for the user is either the tenant global default policy (if set) or the system global default policy.
This cmdlet does not currently support returning policies for multiple users.
This examples returns the policies that are either directly assigned to a user or that the user inherited from a group.
The PolicySource property can be expanded to show more details for a particular policy type. The PolicySource property indicates whether a particular policy was directly assigned to the user or inherited from a group.
In this example, the details for the TeamsBroadcastMeetingPolicy are shown. The user was directly assigned the "Employees Events" policy, which took precedence over the "Vendor Live Events" policy which is assigned to a group (566b8d39-5c5c-4aaa-bc07-4f36278a1b38) that the user belongs to.
In this example, the details for the TeamsMeetingPolicy are shown. The user is a member of two groups, each of which is assigned a TeamsMeetingPolicy. The user has inherited the "AllOn" policy because the priority of the assignment is higher than that of the "Kiosk" policy assignment to the other group.
Wait for .NET debugger to attach
-HttpPipelineAppend
SendAsync Pipeline Steps to be appended to the front of the pipeline
-HttpPipelinePrepend
SendAsync Pipeline Steps to be prepended to the front of the pipeline
The identify of the user whose policy assignments will be returned.
The -Identity parameter can be in the form of the users ObjectID (taken from Microsoft Entra ID) or in the form of the UPN ([email protected])
-InputObject
Identity Parameter To construct, see NOTES section for INPUTOBJECT properties and create a hash table.
-PolicyType
Use to filter to a specific policy type.
The URI for the proxy server to use
-ProxyCredential
Credentials for a proxy server to use for the remote call
-ProxyUseDefaultCredentials
Use the default credentials for the proxy
Microsoft.Teams.Config.Cmdlets.Models.IIc3AdminConfigRpPolicyIdentity
Microsoft.Teams.Config.Cmdlets.Models.IEffectivePolicy
COMPLEX PARAMETER PROPERTIES
To create the parameters described below, construct a hash table containing the appropriate properties. For information on hash tables, run Get-Help about_Hash_Tables.
INPUTOBJECT <IIc3AdminConfigRpPolicyIdentity>: Identity Parameter [GroupId <String>]: The ID of a group whose policy assignments will be returned. [Identity <String>]: [OperationId <String>]: The ID of a batch policy assignment operation. [PolicyType <String>]: The policy type for which group policy assignments will be returned.
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
User Rights Assignment Policy
Check that your User Rights Assignment policy settings comply with security standards in both your Group Policy Objects and on all your Windows computers at once with our reporting tool XIA Configuration .
Use the built-in Windows compliance benchmark to expose servers and workstations that do not meet your security policies.
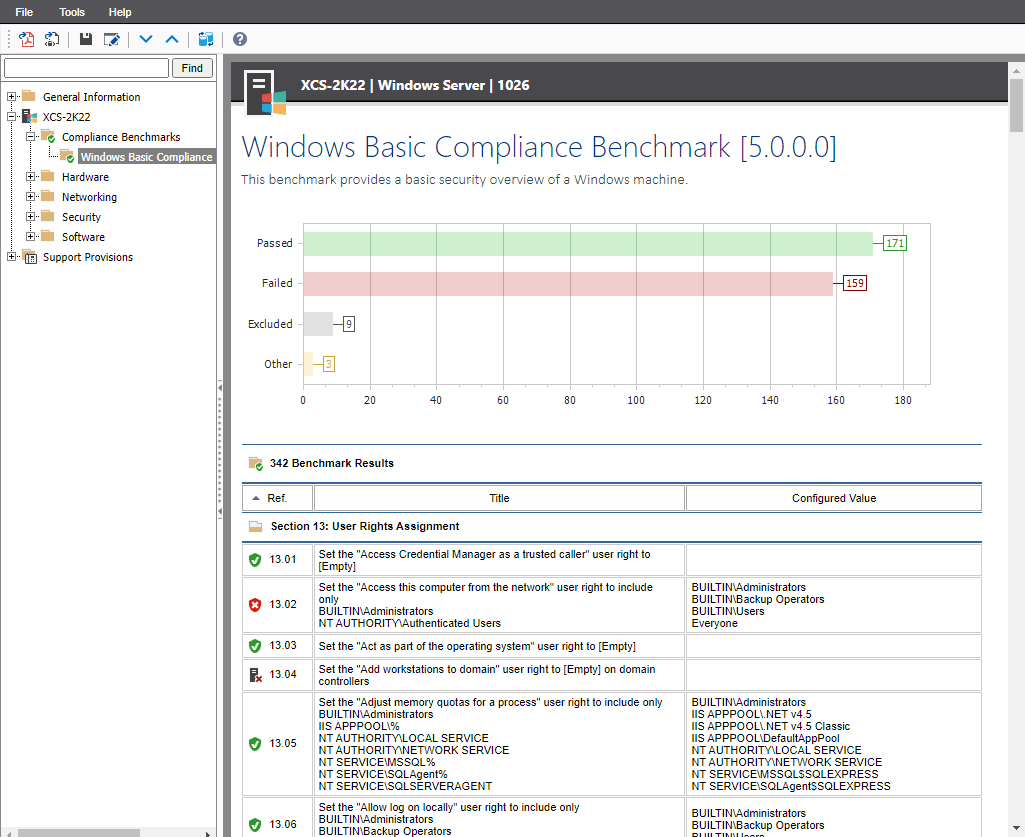
XIA Configuration has a non-intrusive architecture with agentless data collection and PowerShell support .
User Rights Assignment in Group Policy
User Rights Assignment policy settings are typically viewed in the Group Policy Management Console in the following section:
Computer Configuration > Windows Settings > Security Settings > Local Policies > User Rights Assignment
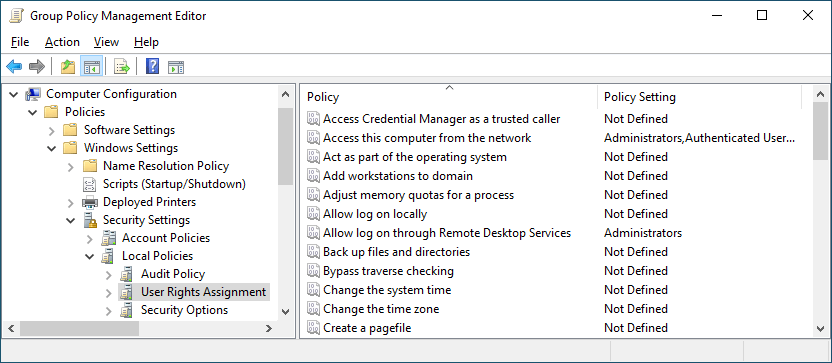
XIA Configuration presents this data in a modern, intuitive web interface and provides reporting functionality allowing you to audit all your Group Policy Objects at once.
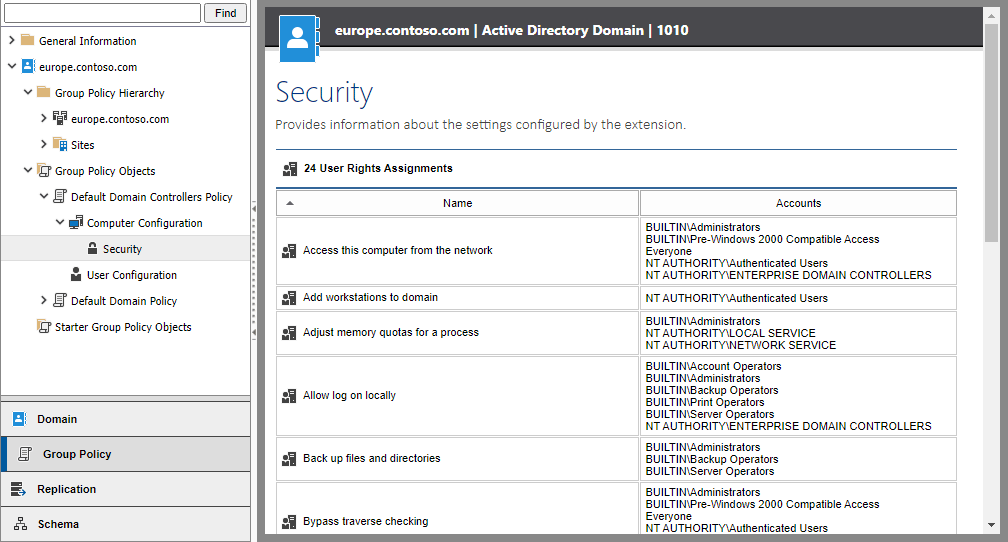
Audit and document your User Rights Assignment settings
If you're performing a security audit on your network, checking group policy settings is essential. As well as getting data from Group Policy, XIA Configuration also automates the retrieval of applied security settings across all your Windows computers.
- The reporting feature allows you to query all your computers at once
- The Windows compliance benchmark allows you to check your data complies with security standards
- Report filters allow you to get the data you need for external auditors
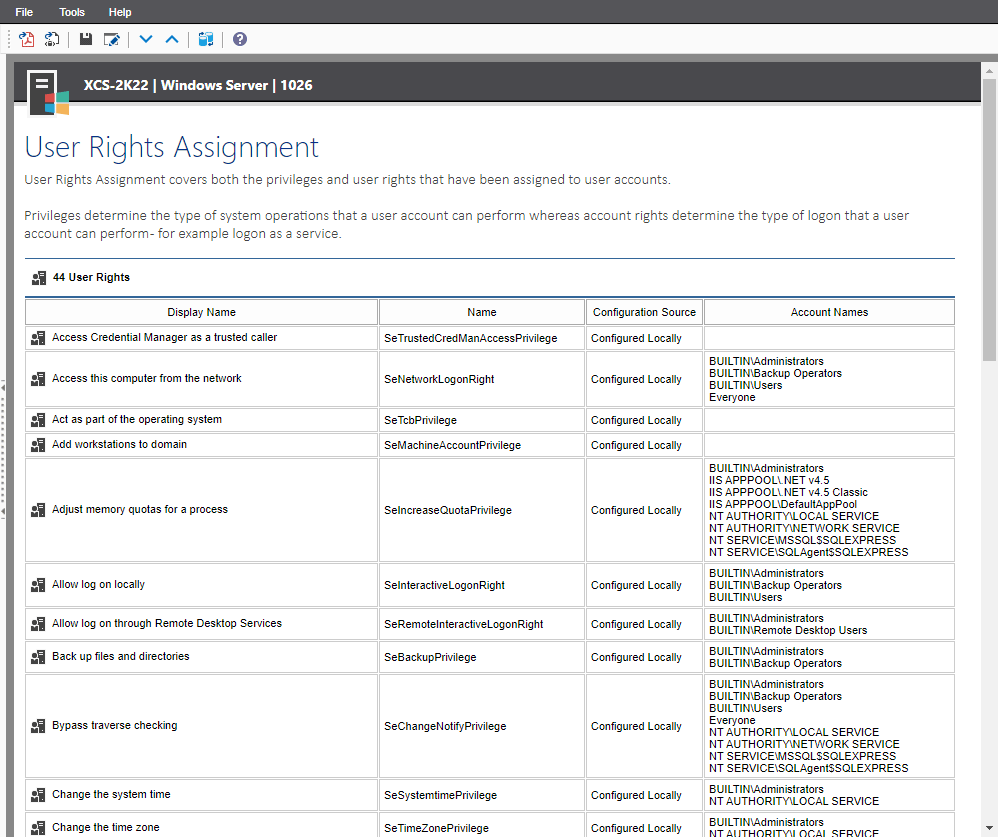
Check your User Rights Assignment security settings
Both the privileges and the user rights that have been assigned to user accounts are covered.
- Display name - for example "Access to this computer from the network"
- Internal right or privilege name - for example "SeNetworkLogonRight"
- Configuration Source (Local / Group Policy (GPO))
- Users and groups with this right assigned
Press the Show details link to view all the user rights retrieved by XIA Configuration.
- Access Credential Manager as a trusted caller
- Access this computer from the network
- Act as part of the operating system
- Add workstations to domain
- Adjust memory quotas for a process
- Allow log on locally
- Allow log on through Remote Desktop Services
- Back up files and directories
- Bypass traverse checking
- Change the system time
- Change the time zone
- Create a pagefile
- Create a token object
- Create global objects
- Create permanent shared objects
- Create symbolic links
- Debug programs
- Deny access to this computer from the network
- Deny log on as a batch job
- Deny log on as a service
- Deny log on locally
- Deny log on through Remote Desktop Services
- Enable computer and user accounts to be trusted for delegation
- Force shutdown from a remote system
- Generate security audits
- Impersonate a client after authentication
- Increase a process working set
- Increase scheduling priority
- Load and unload device drivers
- Lock pages in memory
- Log on as a batch job
- Log on as a service
- Manage auditing and security log
- Modify an object label
- Modify firmware environment values
- Obtain an impersonation token for another user in the same session
- Perform volume maintenance tasks
- Profile single process
- Profile system performance
- Remove computer from docking station
- Replace a process-level token
- Restore files and directories
- Shut down the system
- Synchronize directory service data
- Take ownership of files or other objects
To see all the Windows settings supported by XIA Configuration, navigate up to Windows .
Try auditing your user rights assignment settings for free
No commitments. No costs. Try XIA Configuration today.
Up to Windows
By continuing to browse, you are agreeing to our use of cookies as explained in our Cookie Policy .
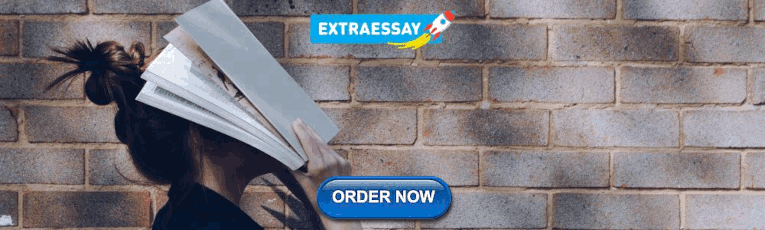
COMMENTS
Personal File Server - Get-UserRights.ps1 Alternative Download Link. or. Personal File Server - Get-UserRights.txt Text Format Alternative Download Link. In order to check the Local User Rights, you will need to run the above (Get-UserRights), you may copy and paste the above script in your Powershell ISE and press play.
To view a specific account (user or group) privileges/rights, you would use: PrivMan -a username --list. The output will be the list of privileges/rights (e.g., SeServiceLogonRight, etc.) directly assigned to that account. edited Feb 6 at 19:03. answered Jan 22 at 21:15. Bill_Stewart. 23.7k 5 51 65.
9. You can use AccessChk in accomplish this task. Accesschk "domain\user" -a * will list all the permissions of a given domain user. You can call this program within a PowerShell script, concatenate the results into a text file, then filter out just the permissions you want to know about. Share.
Get-Management Role Assignment. Get-Management. Role. Assignment. This cmdlet is available in on-premises Exchange and in the cloud-based service. Some parameters and settings may be exclusive to one environment or the other. Use the Get-ManagementRoleAssignment cmdlet to retrieve management role assignments.
1. 0. Managing User Rights Assignments in Powershell. Windows User Rights, also known as Windows Privileges, are traditionally managed via GPO or in the simplest of cases via the server's Local Security Policy. These assignments control special permissions that are often needed by IIS applications or other application hosting on Windows Servers.
As with Adding a privilege, we can remove privileges as well using Remove-Privilege. Remove-Privilege -Privilege SeDebugPrivilege -AccountName boe-pc\proxb. As with Add-Privilege, you will need to log off and log back in to see the change take effect on your account. Again, you can install this module using Install-Module if running ...
User rights are managed in Group Policy under the User Rights Assignment item. Each user right has a constant name and a Group Policy name associated with it. The constant names are used when referring to the user right in log events. You can configure the user rights assignment settings in the following location within the Group Policy ...
Local Policies/User Rights Assignment. User rights assignments are settings applied to the local device. They allow users to perform various system tasks, such as local logon, remote logon, accessing the server from network, shutting down the server, and so on. In this section, I will explain the most important settings and how they should be ...
1 Press the Win + R keys to open Run, type secpol.msc into Run, and click/tap on OK to open Local Security Policy. 2 Expand open Local Policies in the left pane of Local Security Policy, and click/tap on User Rights Assignment. (see screenshot below step 3) 3 In the right pane of User Rights Assignment, double click/tap on the policy (ex: "Shut down the system") you want to add users and/or ...
Manual steps: Open Group Policy Management. Navigate to the following path in the Group Policy Object. Select Policy. Right click & Edit: Computer Configuration\Windows Settings\Security Settings\Local Policies\User Rights Assignment. Add/remove the necessary users. Windows. Active Directory.
What is an equivalent for ntrights.exe on Windows 10? Set and Check User Rights Assignment via Powershell You can add, remove, and check User Rights Assignment (remotely / locally) with the following Powershell scripts.
I need to check that only the administrators group (sid: S-1-5-32-544) has the privilege to take ownership of files or folders (SeTakeOwnershipPrivilege). How can I get an overview of all users/groups that have this privilege? What I already found and tried is the following command: secedit /export /areas USER_RIGHTS /cfg output.txt
I want to edit security settings of user rights assignment of local security policy using powershell or cmd. Eg: policy = "change the system time". default_security_settings = "local service,Administrators". i want to remove everything except Administrators. i have tried ntrights command, but seems like not working Any command will be appreciated.
Hi, this might be an overwhelming task but it is good to know whether this is possible. I want to list all permissions which are given to specific account via GPOs (default domain policy and default domain controller policy). It is account used by backup software and in order to work properly this account had to be given bunch of permissions to (Create a token object, Log on as a batch job ...
To find the permissions required to run any cmdlet or parameter in your organization, see Find the permissions required to run any Exchange cmdlet. Examples Example 1 Get-RoleAssignmentPolicy. This example returns a list of all the existing role assignment policies. Example 2 Get-RoleAssignmentPolicy "End User Policy" | Format-List
Analyze the effective User Rights Assignments on a computer and compare results. Minimum PowerShell version. 5.1. Installation Options. Install Module Install PSResource Azure Automation Manual Download Copy and Paste the following command to install this package using PowerShellGet More Info. Install-Module -Name UserRightsAssignment ...
I've asked a similar question like this before to get the Local User right in PowerShell of a certain domain user, now I would like to enable the right. There are a few rights I am looking to enable, but for this example I will use Logon as a Batch Job. Ntrights does not come with Windows Server 2008 by default, so I cannot use that method.
Find-Module -Name '*sec*pol*' # Results <# Version Name Repository Description ----- ---- ----- ----- 2.10.0.0 SecurityPolicyDsc PSGallery This module is a wrapper around secedit.exe which provides the ability to configure user rights assignments 1.3.2 Indented.SecurityPolicy PSGallery Security management functions and resources 0.0.12 ...
This cmdlets returns the effective policies for a user, based on either direct policy assignment or inheritance from a group policy assignment. For a given policy type, if an effective policy is not returned, this indicates that the effective policy for the user is either the tenant global default policy (if set) or the system global default policy. This cmdlet does not currently support ...
Check your User Rights Assignment security settings. Both the privileges and the user rights that have been assigned to user accounts are covered. Press the Show details link to view all the user rights retrieved by XIA Configuration. To see all the Windows settings supported by XIA Configuration, navigate up to Windows. No commitments. No costs.
I have a script that needs to check the user' rights on the remote machine in order to confirm the user has the permissions to copy their files. When this part of the script runs, it fails 90% of the ... Powershell check if user is local-user and have admin rights from username and password on local windows machine (Not active directory) 0.