Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java booleans.
Very often, in programming, you will need a data type that can only have one of two values, like:
- TRUE / FALSE
For this, Java has a boolean data type, which can store true or false values.
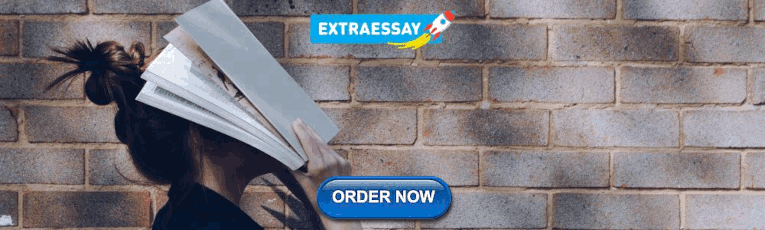
Boolean Values
A boolean type is declared with the boolean keyword and can only take the values true or false :
Try it Yourself »
However, it is more common to return boolean values from boolean expressions, for conditional testing (see below).
Boolean Expression
A Boolean expression returns a boolean value: true or false .
This is useful to build logic, and find answers.
For example, you can use a comparison operator , such as the greater than ( > ) operator, to find out if an expression (or a variable) is true or false:
Or even easier:
In the examples below, we use the equal to ( == ) operator to evaluate an expression:
Real Life Example
Let's think of a "real life example" where we need to find out if a person is old enough to vote.
In the example below, we use the >= comparison operator to find out if the age ( 25 ) is greater than OR equal to the voting age limit, which is set to 18 :
Cool, right? An even better approach (since we are on a roll now), would be to wrap the code above in an if...else statement, so we can perform different actions depending on the result:
Output "Old enough to vote!" if myAge is greater than or equal to 18 . Otherwise output "Not old enough to vote.":
Booleans are the basis for all Java comparisons and conditions.
You will learn more about conditions ( if...else ) in the next chapter.
Test Yourself With Exercises
Fill in the missing parts to print the values true and false :
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
JavaScript disabled. A lot of the features of the site won't work. Find out how to turn on JavaScript HERE .

- Fundamentals
- Objects & Classes
- OO Concepts
- API Contents
- Input & Output
- Collections
- Concurrency
- Swing & RMI
- Certification
Assignment Operators J8 Home « Assignment Operators
- << Relational & Logical Operators
- Bitwise Logical Operators >>
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In the third of five lessons on operators we look at the assignment operators available in Java.
Assignment Operators Overview Top
The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression .
Shorthand Assignment Operators
The shorthand assignment operators allow us to write compact code that is implemented more efficiently.
Automatic Type Conversion, Assignment Rules Top
The following table shows which types can be assigned to which other types, of course we can assign to the same type so these boxes are greyed out.
When using the table use a row for the left assignment and a column for the right assignment. So in the highlighted permutations byte = int won't convert and int = byte will convert.
Casting Incompatible Types Top
The above table isn't the end of the story though as Java allows us to cast incompatible types. A cast instructs the compiler to convert one type to another enforcing an explicit type conversion.
A cast takes the form target = (target-type) expression .
There are a couple of things to consider when casting incompatible types:
- With narrowing conversions such as an int to a short there may be a loss of precision if the range of the int exceeds the range of a short as the high order bits will be removed.
- When casting a floating-point type to an integer type the fractional component is lost through truncation.
- The target-type can be the same type as the target or a narrowing conversion type.
- The boolean type is not only incompatible but also inconvertible with other types.
Lets look at some code to see how casting works and the affect it has on values:
Running the Casting class produces the following output:
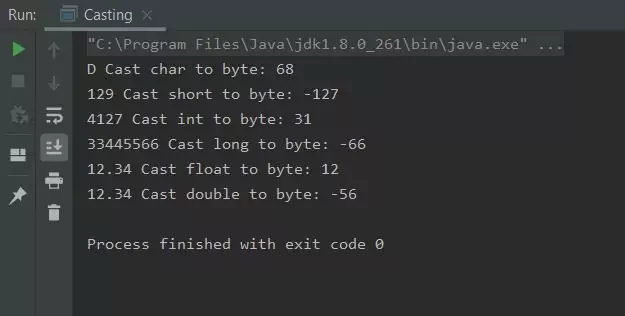
The first thing to note is we got a clean compile because of the casts, all the type conversions would fail otherwise. You might be suprised by some of the results shown in the screenshot above, for instance some of the values have become negative. Because we are truncating everything to a byte we are losing not only any fractional components and bits outside the range of a byte , but in some cases the signed bit as well. Casting can be very useful but just be aware of the implications to values when you enforce explicit type conversion.
Related Quiz
Fundamentals Quiz 8 - Assignment Operators Quiz
Lesson 9 Complete
In this lesson we looked at the assignment operators used in Java.
What's Next?
In the next lesson we look at the bitwise logical operators used in Java.
Getting Started
Code structure & syntax, java variables, primitives - boolean & char data types, primitives - numeric data types, method scope, arithmetic operators, relational & logical operators, assignment operators, assignment operators overview, automatic type conversion, casting incompatible types, bitwise logical operators, bitwise shift operators, if construct, switch construct, for construct, while construct.
Java 8 Tutorials
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
Java Operator Precedence
Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java Math IEEEremainder()
Operators are symbols that perform operations on variables and values. For example, + is an operator used for addition, while * is also an operator used for multiplication.
Operators in Java can be classified into 5 types:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
1. Java Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on variables and data. For example,
Here, the + operator is used to add two variables a and b . Similarly, there are various other arithmetic operators in Java.
Example 1: Arithmetic Operators
In the above example, we have used + , - , and * operators to compute addition, subtraction, and multiplication operations.
/ Division Operator
Note the operation, a / b in our program. The / operator is the division operator.
If we use the division operator with two integers, then the resulting quotient will also be an integer. And, if one of the operands is a floating-point number, we will get the result will also be in floating-point.
% Modulo Operator
The modulo operator % computes the remainder. When a = 7 is divided by b = 4 , the remainder is 3 .
Note : The % operator is mainly used with integers.
2. Java Assignment Operators
Assignment operators are used in Java to assign values to variables. For example,
Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age .
Let's see some more assignment operators available in Java.
Example 2: Assignment Operators
3. java relational operators.
Relational operators are used to check the relationship between two operands. For example,
Here, < operator is the relational operator. It checks if a is less than b or not.
It returns either true or false .
Example 3: Relational Operators
Note : Relational operators are used in decision making and loops.
4. Java Logical Operators
Logical operators are used to check whether an expression is true or false . They are used in decision making.
Example 4: Logical Operators
Working of Program
- (5 > 3) && (8 > 5) returns true because both (5 > 3) and (8 > 5) are true .
- (5 > 3) && (8 < 5) returns false because the expression (8 < 5) is false .
- (5 < 3) || (8 > 5) returns true because the expression (8 > 5) is true .
- (5 > 3) || (8 < 5) returns true because the expression (5 > 3) is true .
- (5 < 3) || (8 < 5) returns false because both (5 < 3) and (8 < 5) are false .
- !(5 == 3) returns true because 5 == 3 is false .
- !(5 > 3) returns false because 5 > 3 is true .
5. Java Unary Operators
Unary operators are used with only one operand. For example, ++ is a unary operator that increases the value of a variable by 1 . That is, ++5 will return 6 .
Different types of unary operators are:
- Increment and Decrement Operators
Java also provides increment and decrement operators: ++ and -- respectively. ++ increases the value of the operand by 1 , while -- decrease it by 1 . For example,
Here, the value of num gets increased to 6 from its initial value of 5 .
Example 5: Increment and Decrement Operators
In the above program, we have used the ++ and -- operator as prefixes (++a, --b) . We can also use these operators as postfix (a++, b++) .
There is a slight difference when these operators are used as prefix versus when they are used as a postfix.
To learn more about these operators, visit increment and decrement operators .
6. Java Bitwise Operators
Bitwise operators in Java are used to perform operations on individual bits. For example,
Here, ~ is a bitwise operator. It inverts the value of each bit ( 0 to 1 and 1 to 0 ).
The various bitwise operators present in Java are:
These operators are not generally used in Java. To learn more, visit Java Bitwise and Bit Shift Operators .
Other operators
Besides these operators, there are other additional operators in Java.
The instanceof operator checks whether an object is an instanceof a particular class. For example,
Here, str is an instance of the String class. Hence, the instanceof operator returns true . To learn more, visit Java instanceof .
The ternary operator (conditional operator) is shorthand for the if-then-else statement. For example,
Here's how it works.
- If the Expression is true , expression1 is assigned to the variable .
- If the Expression is false , expression2 is assigned to the variable .
Let's see an example of a ternary operator.
In the above example, we have used the ternary operator to check if the year is a leap year or not. To learn more, visit the Java ternary operator .
Now that you know about Java operators, it's time to know about the order in which operators are evaluated. To learn more, visit Java Operator Precedence .
Table of Contents
- Introduction
- Java Arithmetic Operators
- Java Assignment Operators
- Java Relational Operators
- Java Logical Operators
- Java Unary Operators
- Java Bitwise Operators
Sorry about that.
Related Tutorials
Java Tutorial
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Summary of Operators
The following quick reference summarizes the operators supported by the Java programming language.
Simple Assignment Operator
Arithmetic operators, unary operators, equality and relational operators, conditional operators, type comparison operator, bitwise and bit shift operators.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
- PyQt5 ebook
- Tkinter ebook
- SQLite Python
- wxPython ebook
- Windows API ebook
- Java Swing ebook
- Java games ebook
- MySQL Java ebook
Java operator
last modified January 27, 2024
In this article we show how to work with operators in Java.
An operator is a special symbol which indicates a certain process is carried out. Operators in programming languages are taken from mathematics. Programmers work with data. The operators are used to process data. An operand is one of the inputs (arguments) of an operator.
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
An operator usually has one or two operands. Those operators that work with only one operand are called unary operators . Those who work with two operands are called binary operators . There is also one ternary operator ?: which works with three operands.
Certain operators may be used in different contexts. For example the + operator. It can be used in different cases. It adds numbers, concatenates strings, or indicates the sign of a number. We say that the operator is overloaded .
Java sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to signal that we have a positive number. It can be omitted and it is mostly done so.
The minus sign changes the sign of a value.
Java assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
Here we assign a number to the x variable.
This expression does not make sense in mathematics, but it is legal in programming. The expression adds 1 to the x variable. The right side is equal to 2 and 2 is assigned to x.
This code line results in syntax error. We cannot assign a value to a literal.
Java concatenating strings
In Java the + operator is also used to concatenate strings.
We join three strings together.
Strings are joined with the + operator.
An alternative method for concatenating strings is the concat method.
Java increment and decrement operators
Incrementing or decrementing a value by one is a common task in programming. Java has two convenient operators for this: ++ and -- .
The above two pairs of expressions do the same.
In the above example, we demonstrate the usage of both operators.
We initiate the x variable to 6. Then we increment x two times. Now the variable equals to 8.
We use the decrement operator. Now the variable equals to 7.
And here is the output of the example.
Java arithmetic operators
The following is a table of arithmetic operators in Java.
The following example shows arithmetic operations.
In the preceding example, we use addition, subtraction, multiplication, division, and remainder operations. This is all familiar from the mathematics.
The % operator is called the remainder or the modulo operator. It finds the remainder of division of one number by another. For example, 9 % 4 , 9 modulo 4 is 1, because 4 goes into 9 twice with a remainder of 1.
Next we show the distinction between integer and floating point division.
In the preceding example, we divide two numbers.
In this code, we have done integer division. The returned value of the division operation is an integer. When we divide two integers the result is an integer.
If one of the values is a double or a float, we perform a floating point division. In our case, the second operand is a double so the result is a double.
We see the result of the program.
Java Boolean operators
In Java we have three logical operators. The boolean keyword is used to declare a Boolean value.
Boolean operators are also called logical.
Many expressions result in a boolean value. For instance, boolean values are used in conditional statements.
Relational operators always result in a boolean value. These two lines print false and true.
The body of the if statement is executed only if the condition inside the parentheses is met. The y > x returns true, so the message "y is greater than x" is printed to the terminal.
The true and false keywords represent boolean literals in Java.
The code example shows the logical and (&&) operator. It evaluates to true only if both operands are true.
Only one expression results in true .
The logical or ( || ) operator evaluates to true if either of the operands is true.
If one of the sides of the operator is true, the outcome of the operation is true.
Three of four expressions result in true .
The negation operator ! makes true false and false true.
The example shows the negation operator in action.
The || , and && operators are short circuit evaluated. Short circuit evaluation means that the second argument is only evaluated if the first argument does not suffice to determine the value of the expression: when the first argument of the logical and evaluates to false, the overall value must be false; and when the first argument of logical or evaluates to true, the overall value must be true. Short circuit evaluation is used mainly to improve performance.
An example may clarify this a bit more.
We have two methods in the example. They are used as operands in boolean expressions. We will see if they are called.
The One method returns false. The short circuit && does not evaluate the second method. It is not necessary. Once an operand is false, the result of the logical conclusion is always false. Only "Inside one" is only printed to the console.
In the second case, we use the || operator and use the Two method as the first operand. In this case, "Inside two" and "Pass" strings are printed to the terminal. It is again not necessary to evaluate the second operand, since once the first operand evaluates to true, the logical or is always true.
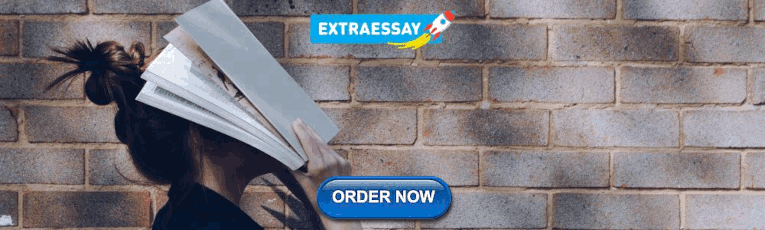
Java relational operators
Relational operators are used to compare values. These operators always result in a boolean value.
Relational operators are also called comparison operators.
In the code example, we have four expressions. These expressions compare integer values. The result of each of the expressions is either true or false. In Java we use the == to compare numbers. (Some languages like Ada, Visual Basic, or Pascal use = for comparing numbers.)
Java bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of a number. Bitwise operators work with bits of a binary number. Bitwise operators are seldom used in higher level languages like Java.
The bitwise negation operator changes each 1 to 0 and 0 to 1.
The operator reverts all bits of a number 7. One of the bits also determines whether the number is negative or not. If we negate all the bits one more time, we get number 7 again.
The bitwise and operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 only if both corresponding bits in the operands are 1.
The first number is a binary notation of 6, the second is 3 and the result is 2.
The bitwise or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if either of the corresponding bits in the operands is 1.
The result is 00110 or decimal 7.
The bitwise exclusive or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if one or the other (but not both) of the corresponding bits in the operands is 1.
The result is 00101 or decimal 5.
Java compound assignment operators
Compound assignment operators are shorthand operators which consist of two operators.
The += compound operator is one of these shorthand operators. The above two expressions are equal. Value 3 is added to the a variable.
Other compound operators are:
The following example uses two compound operators.
We use the += and *= compound operators.
The a variable is initiated to one. Value 1 is added to the variable using the non-shorthand notation.
Using a += compound operator, we add 5 to the a variable. The statement is equal to a = a + 5; .
Using the *= operator, the a is multiplied by 3. The statement is equal to a = a * 3; .
Java instanceof operator
The instanceof operator compares an object to a specified type.
In the example, we have two classes: one base and one derived from the base.
This line checks if the variable d points to the class that is an instance of the Base class. Since the Derived class inherits from the Base class, it is also an instance of the Base class too. The line prints true.
The b object is not an instance of the Derived class. This line prints false.
Every class has Object as a superclass. Therefore, the d object is also an instance of the Object class.
Java lambda operator
Java 8 introduced the lambda operator ( -> ).
This is the basic syntax for a lambda expression in Java. Lambda expression allow to create more concise code in Java.
The declaration of the type of the parameter is optional; the compiler can infer the type from the value of the parameter. For a single parameter the parentheses are optional; for multiple parameters, they are required.
The curly braces are optional if there is only one statement in an expression body. Finally, the return keyword is optional if the body has a single expression to return a value; curly braces are required to indicate that the expression returns a value.
In the example, we define an array of strings. The array is sorted using the Arrays.sort method and a lambda expression.
Lambda expressions are used primarily to define an inline implementation of a functional interface, i.e., an interface with a single method only. Interfaces are abstract types that are used to enforce a contract.
In the example, we create a greeting service with the help of a lambda expression.
Interface GreetingService is created. All objects implementing this interface must implement the greet method.
We create an object that implements GreetingService with a lambda expression. The object has a method that prints a message to the console.
We call the object's greet method, which prints a give message to the console.
There are some common functional interfaces, such as Function , Consumer , or Supplier .
The example uses a lambda expression to compute squares of integers.
Function is a function that accepts one argument and produces a result. The operation of the lamda expression produces a square of the given integer.
Java double colon operator
The double colon operator (::) is used to create a reference to a method.
In the code example, we create a reference to a static method with the double colon operator.
We have a static method that prints a greeting to the console.
Consumer is a functional interface that represents an operation that accepts a single input argument and returns no result. With the double colon operator, we create a reference to the greet method.
We perform the functional operation with the accept method.
Java operator precedence
The operator precedence tells us which operators are evaluated first. The precedence level is necessary to avoid ambiguity in expressions.
What is the outcome of the following expression, 28 or 40?
Like in mathematics, the multiplication operator has a higher precedence than addition operator. So the outcome is 28.
To change the order of evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first. The result of the above expression is 40.
Java operators precedence list
The following table shows common Java operators ordered by precedence (highest precedence first):
Operators on the same row of the table have the same precedence. If we use operators with the same precedence, then the associativity rule is applied.
In this code example, we show a few expressions. The outcome of each expression is dependent on the precedence level.
This line prints 28. The multiplication operator has a higher precedence than addition. First, the product of 5*5 is calculated, then 3 is added.
In this case, the negation operator has a higher precedence than the bitwise OR. First, the initial true value is negated to false, then the | operator combines false and true, which gives true in the end.
Java associativity rule
Sometimes the precedence is not satisfactory to determine the outcome of an expression. There is another rule called associativity . The associativity of operators determines the order of evaluation of operators with the same precedence level.
What is the outcome of this expression, 9 or 1? The multiplication, deletion, and the modulo operator are left to right associated. So the expression is evaluated this way: (9 / 3) * 3 and the result is 9.
Arithmetic, boolean, relational, and bitwise operators are all left to right associated. The assignment operators, ternary operator, increment, decrement, unary plus and minus, negation, bitwise NOT, type cast, object creation operators are right to left associated.
In the example, we have two cases where the associativity rule determines the expression.
The assignment operator is right to left associated. If the associativity was left to right, the previous expression would not be possible.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied.
Java ternary operator
The ternary operator ?: is a conditional operator. It is a convenient operator for cases where we want to pick up one of two values, depending on the conditional expression.
If cond-exp is true, exp1 is evaluated and the result is returned. If the cond-exp is false, exp2 is evaluated and its result is returned.
In most countries the adulthood is based on the age. You are adult if you are older than a certain age. This is a situation for a ternary operator.
First the expression on the right side of the assignment operator is evaluated. The first phase of the ternary operator is the condition expression evaluation. So if the age is greater or equal to 18, the value following the ? character is returned. If not, the value following the : character is returned. The returned value is then assigned to the adult variable.
A 31 years old person is adult.
Calculating prime numbers
In the following example, we are going to calculate prime numbers.
In the above example, we deal with several operators. A prime number (or a prime) is a natural number that has exactly two distinct natural number divisors: 1 and itself. We pick up a number and divide it by numbers from 1 to the selected number. Actually, we do not have to try all smaller numbers; we can divide by numbers up to the square root of the chosen number. The formula will work. We use the remainder division operator.
We will calculate primes from these numbers.
Values 0 and 1 are not considered to be primes.
We skip the calculations for 2 and 3. They are primes. Note the usage of the equality and conditional or operators. The == has a higher precedence than the || operator. So we do not need to use parentheses.
We are OK if we only try numbers smaller than the square root of a number in question.
This is a while loop. The i is the calculated square root of the number. We use the decrement operator to decrease i by one each loop cycle. When i is smaller than 1, we terminate the loop. For example, we have number 9. The square root of 9 is 3. We will divide the 9 number by 3 and 2. This is sufficient for our calculation.
If the remainder division operator returns 0 for any of the i values, then the number in question is not a prime.
In this article we covered Java expressions. We mentioned various types of operators and described precedence and associativity rules in expressions.
Java operators - tutorial
My name is Jan Bodnar and I am a passionate programmer with many years of programming experience. I have been writing programming articles since 2007. So far, I have written over 1400 articles and 8 e-books. I have over eight years of experience in teaching programming.
List all Java tutorials .
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
- Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Java provides many types of operators which can be used according to the need. They are classified based on the functionality they provide. In this article, we will learn about Java Operators and learn all their types.
What are the Java Operators?
Operators in Java are the symbols used for performing specific operations in Java. Operators make tasks like addition, multiplication, etc which look easy although the implementation of these tasks is quite complex.
Types of Operators in Java
There are multiple types of operators in Java all are mentioned below:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
- instance of operator
1. Arithmetic Operators
They are used to perform simple arithmetic operations on primitive data types.
- * : Multiplication
- / : Division
- + : Addition
- – : Subtraction
2. Unary Operators
Unary operators need only one operand. They are used to increment, decrement, or negate a value.
- – : Unary minus , used for negating the values.
- + : Unary plus indicates the positive value (numbers are positive without this, however). It performs an automatic conversion to int when the type of its operand is the byte, char, or short. This is called unary numeric promotion.
- Post-Increment: Value is first used for computing the result and then incremented.
- Pre-Increment: Value is incremented first, and then the result is computed.
- Post-decrement: Value is first used for computing the result and then decremented.
- Pre-Decrement: The value is decremented first, and then the result is computed.
- ! : Logical not operator , used for inverting a boolean value.
3. Assignment Operator
‘=’ Assignment operator is used to assign a value to any variable. It has right-to-left associativity, i.e. value given on the right-hand side of the operator is assigned to the variable on the left, and therefore right-hand side value must be declared before using it or should be a constant.
The general format of the assignment operator is:
In many cases, the assignment operator can be combined with other operators to build a shorter version of the statement called a Compound Statement . For example, instead of a = a+5, we can write a += 5.
- += , for adding the left operand with the right operand and then assigning it to the variable on the left.
- -= , for subtracting the right operand from the left operand and then assigning it to the variable on the left.
- *= , for multiplying the left operand with the right operand and then assigning it to the variable on the left.
- /= , for dividing the left operand by the right operand and then assigning it to the variable on the left.
- %= , for assigning the modulo of the left operand by the right operand and then assigning it to the variable on the left.
4. Relational Operators
These operators are used to check for relations like equality, greater than, and less than. They return boolean results after the comparison and are extensively used in looping statements as well as conditional if-else statements. The general format is,
Some of the relational operators are-
- ==, Equal to returns true if the left-hand side is equal to the right-hand side.
- !=, Not Equal to returns true if the left-hand side is not equal to the right-hand side.
- <, less than: returns true if the left-hand side is less than the right-hand side.
- <=, less than or equal to returns true if the left-hand side is less than or equal to the right-hand side.
- >, Greater than: returns true if the left-hand side is greater than the right-hand side.
- >=, Greater than or equal to returns true if the left-hand side is greater than or equal to the right-hand side.
5. Logical Operators
These operators are used to perform “logical AND” and “logical OR” operations, i.e., a function similar to AND gate and OR gate in digital electronics. One thing to keep in mind is the second condition is not evaluated if the first one is false, i.e., it has a short-circuiting effect. Used extensively to test for several conditions for making a decision. Java also has “Logical NOT”, which returns true when the condition is false and vice-versa
Conditional operators are:
- &&, Logical AND: returns true when both conditions are true.
- ||, Logical OR: returns true if at least one condition is true.
- !, Logical NOT: returns true when a condition is false and vice-versa
6. Ternary operator
The ternary operator is a shorthand version of the if-else statement. It has three operands and hence the name Ternary.
The general format is:
The above statement means that if the condition evaluates to true, then execute the statements after the ‘?’ else execute the statements after the ‘:’.
7. Bitwise Operators
These operators are used to perform the manipulation of individual bits of a number. They can be used with any of the integer types. They are used when performing update and query operations of the Binary indexed trees.
- &, Bitwise AND operator: returns bit by bit AND of input values.
- |, Bitwise OR operator: returns bit by bit OR of input values.
- ^, Bitwise XOR operator: returns bit-by-bit XOR of input values.
- ~, Bitwise Complement Operator: This is a unary operator which returns the one’s complement representation of the input value, i.e., with all bits inverted.
8. Shift Operators
These operators are used to shift the bits of a number left or right, thereby multiplying or dividing the number by two, respectively. They can be used when we have to multiply or divide a number by two. General format-
- <<, Left shift operator: shifts the bits of the number to the left and fills 0 on voids left as a result. Similar effect as multiplying the number with some power of two.
- >>, Signed Right shift operator: shifts the bits of the number to the right and fills 0 on voids left as a result. The leftmost bit depends on the sign of the initial number. Similar effect to dividing the number with some power of two.
- >>>, Unsigned Right shift operator: shifts the bits of the number to the right and fills 0 on voids left as a result. The leftmost bit is set to 0.
9. instanceof operator
The instance of the operator is used for type checking. It can be used to test if an object is an instance of a class, a subclass, or an interface. General format-
Precedence and Associativity of Java Operators
Precedence and associative rules are used when dealing with hybrid equations involving more than one type of operator. In such cases, these rules determine which part of the equation to consider first, as there can be many different valuations for the same equation. The below table depicts the precedence of operators in decreasing order as magnitude, with the top representing the highest precedence and the bottom showing the lowest precedence.
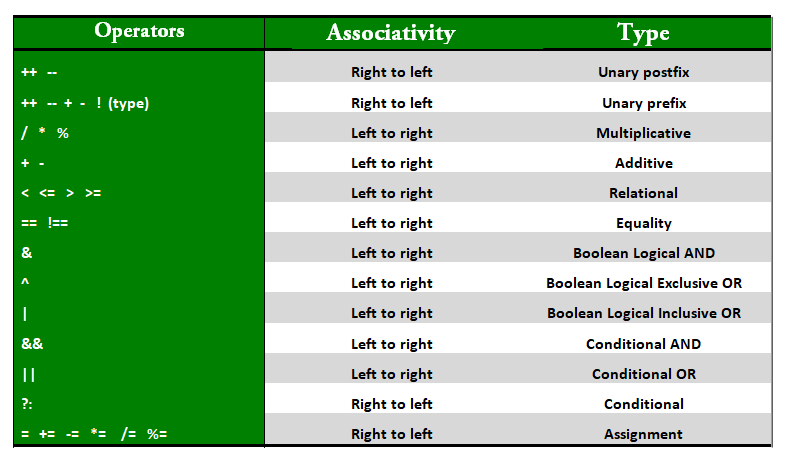
Interesting Questions about Java Operators
1. precedence and associativity:.
There is often confusion when it comes to hybrid equations which are equations having multiple operators. The problem is which part to solve first. There is a golden rule to follow in these situations. If the operators have different precedence, solve the higher precedence first. If they have the same precedence, solve according to associativity, that is, either from right to left or from left to right. The explanation of the below program is well written in comments within the program itself.
2. Be a Compiler:
The compiler in our systems uses a lex tool to match the greatest match when generating tokens. This creates a bit of a problem if overlooked. For example, consider the statement a=b+++c ; too many of the readers might seem to create a compiler error. But this statement is absolutely correct as the token created by lex is a, =, b, ++, +, c. Therefore, this statement has a similar effect of first assigning b+c to a and then incrementing b. Similarly, a=b+++++c; would generate an error as the tokens generated are a, =, b, ++, ++, +, c. which is actually an error as there is no operand after the second unary operand.
3. Using + over ():
When using the + operator inside system.out.println() make sure to do addition using parenthesis. If we write something before doing addition, then string addition takes place, that is, associativity of addition is left to right, and hence integers are added to a string first producing a string, and string objects concatenate when using +. Therefore it can create unwanted results.
Advantages of Operators in Java
The advantages of using operators in Java are mentioned below:
- Expressiveness : Operators in Java provide a concise and readable way to perform complex calculations and logical operations.
- Time-Saving: Operators in Java save time by reducing the amount of code required to perform certain tasks.
- Improved Performance : Using operators can improve performance because they are often implemented at the hardware level, making them faster than equivalent Java code.
Disadvantages of Operators in Java
The disadvantages of Operators in Java are mentioned below:
- Operator Precedence: Operators in Java have a defined precedence, which can lead to unexpected results if not used properly.
- Type Coercion : Java performs implicit type conversions when using operators, which can lead to unexpected results or errors if not used properly.
FAQs in Java Operators
1. what is operators in java with example.
Operators are the special symbols that are used for performing certain operations. For example, ‘+’ is used for addition where 5+4 will return the value 9.
Please Login to comment...
Similar reads.
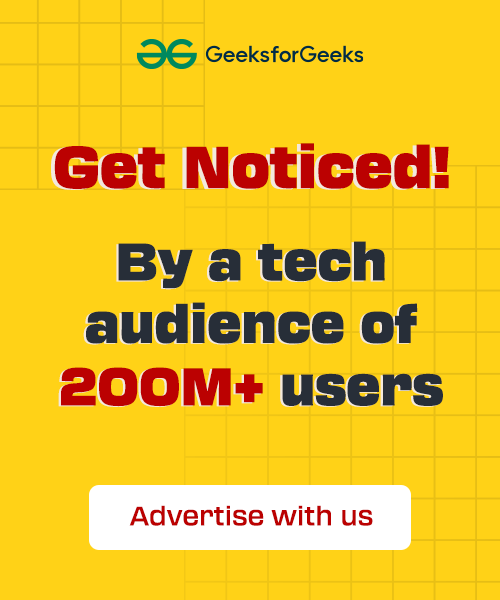
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Free Java Guide
- Programming Tutorials
- History of Java
- Free JAVA Tutorials
- SCJP Notes 1
- SCJP Notes 2
- SCJP Notes 3
- SCJP Notes 4
- SCJP Notes 5
- SCJP Notes 6
- SCJP Notes 7
- SCJP Notes 8
- JDBC Program Tutorial
- Java Source Code
- Java Applet
- Java Servlet
- JavaServer Pages
- Criticism of Java
- SQL Tutorial
- PLSQL Tutorial
- PLSQL Examples
- HTML Tutorial
- What is XML?
- XML Tutorial
- Job Interview Questions
- Java Interview
- SQL Interview
- XML Interview
- HTML Interview
- Partner websites
- Bird Watching
- Haryana Online
- Asia Newscast
- North India Online
These tutorials will introduce you to Java programming Language. You'll compile and run your own Java application, using Sun's JDK. It's very easy to learn java programming skills, and in these parts, you'll learn how to write, compile, and run Java applications. Before you can develop corejava applications, you'll need to download the Java Development Kit ( JDK ).
- Java Arithmetic Operators
- Java Assignment Operators
- Java Increment and Decrement Operators
- Java Relational Operators
Java Boolean Operators
- Java Conditional Operators
The Boolean logical operators are : | , & , ^ , ! , || , && , == , != . Java supplies a primitive data type called Boolean, instances of which can take the value true or false only, and have the default value false. The major use of Boolean facilities is to implement the expressions which control if decisions and while loops. These operators act on Boolean operands according to this table
Example
Previous 1 2 3 4 5 Next
Page 2 of 5
Copyright © 2006-2013 Free Java Guide & Tutorials . All Rights Reserved.
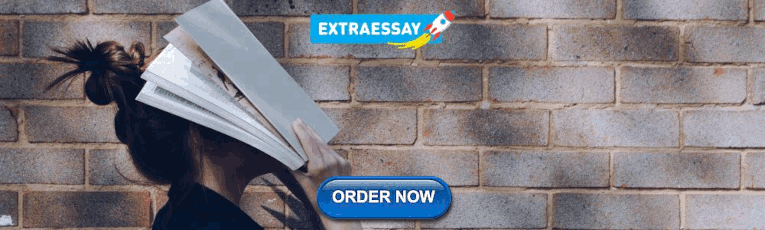
IMAGES
VIDEO
COMMENTS
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
The |= is a compound assignment operator ( JLS 15.26.2) for the boolean logical operator | ( JLS 15.22.2 ); not to be confused with the conditional-or || ( JLS 15.24 ). There are also &= and ^= corresponding to the compound assignment version of the boolean logical & and ^ respectively. In other words, for boolean b1, b2, these two are equivalent:
Learning the operators of the Java programming language is a good place to start. Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result. As we explore the operators of the Java programming language, it may be helpful for you to know ahead of time which operators have the highest ...
Java Comparison Operators. Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The return value of a comparison is either true or false. These values are known as Boolean values, and you will learn more about them in the Booleans and If ...
The Arithmetic Operators. The Java programming language provides operators that perform addition, subtraction, multiplication, and division. ... You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For ... negating an expression, or inverting the value of a boolean. Operator ...
Boolean coercion: Logical operators can sometimes lead to unexpected behavior when used with non-Boolean values. For example, when using the or operator, the expression a or b will evaluate to a if a is truthy, and b otherwise. ... Java Assignment Operators with Examples Java.util.Bitset class | Logical operations Java | Operators | Question 1 ...
Basically, we use these operators to compare two values or variables. 4.1. The "Equal To" Operator. We use the "equal to" operator (==) to compare the values on both sides. If they're equal, the operation returns true: int number1 = 5 ; int number2 = 5 ; boolean theyAreEqual = number1 == number2; Copy.
Boolean Expression. A Boolean expression returns a boolean value: true or false. This is useful to build logic, and find answers. For example, you can use a comparison operator, such as the greater than (>) operator, to find out if an expression (or a variable) is true or false:
Assignment Operators Overview Top. The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression.
Compound Assignment Operators. An assignment operator is a binary operator that assigns the result of the right-hand side to the variable on the left-hand side. The simplest is the "=" assignment operator: int x = 5; This statement declares a new variable x, assigns x the value of 5 and returns 5. Compound Assignment Operators are a shorter ...
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java: 1. += (compound addition assignment operator) 2.
2. Java Assignment Operators. Assignment operators are used in Java to assign values to variables. For example, int age; age = 5; Here, = is the assignment operator. It assigns the value on its right to the variable on its left. That is, 5 is assigned to the variable age. Let's see some more assignment operators available in Java.
There are mainly two types of assignment operators in Java, which are as follows: Simple Assignment Operator ; We use the simple assignment operator with the "=" sign, where the left side consists of an operand and the right side is a value. The value of the operand on the right side must be of the same data type defined on the left side.
The following quick reference summarizes the operators supported by the Java programming language. Simple Assignment Operator = Simple assignment operator Arithmetic Operators ... decrements a value by 1 ! Logical complement operator; inverts the value of a boolean Equality and Relational Operators ...
The operators are used to process data. An operand is one of the inputs (arguments) of an operator. Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of ...
3. Assignment Operator '=' Assignment operator is used to assign a value to any variable. It has right-to-left associativity, i.e. value given on the right-hand side of the operator is assigned to the variable on the left, and therefore right-hand side value must be declared before using it or should be a constant.
The Boolean logical operators are : | , & , ^ , ! , || , && , == , != . Java supplies a primitive data type called Boolean, instances of which can take the value true or false only, and have the default value false. The major use of Boolean facilities is to implement the expressions which control if decisions and while loops. & the AND operator.
32. a |= b; is the same as. a = (a | b); It calculates the bitwise OR of the two operands, and assigns the result to the left operand. To explain your example code: for (String search : textSearch.getValue()) matches |= field.contains(search); I presume matches is a boolean; this means that the bitwise operators behave the same as logical ...
Java also supports a number of Boolean, string, and assignment operators. Boolean operators are used to perform logical comparisons, and always result in one of two values: true or false. Following are the most commonly used Boolean operators :
4. You've got it right. The operator precedence rules make sure that first the == operator is evaluated. That's b1==false, yielding true. After that, the assigned is executed, setting b2 to true. Finally, the assignment operator returns the value as b2, which is evaluated by the if statement. Java usually evaluates the terms from the left to ...
Java is designed to be a language that is easy to learn, read and understand. Adding operators that are designed to do "clever" things in a concise way is liable to make the language harder to learn, and harder to read ... for the average programmer. And, if the operator is only really useful in a tiny number of use-cases, that makes the ...