Create PowerPoint presentations with JavaScript
The most popular powerpoint+js library on npm with 1,800 stars on github, works everywhere.
- Every modern desktop and mobile browser is supported
- Integrates with Node, Angular, React, and Electron
- Compatible with Microsoft PowerPoint, Apple Keynote, and many others
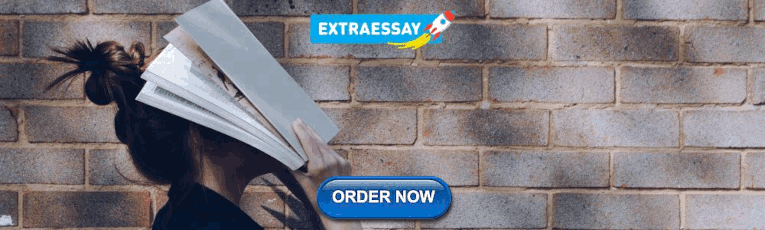
Full Featured
- All major objects are available (charts, shapes, tables, etc.)
- Master Slide support for academic/corporate branding
- Animated gifs, SVG images, YouTube videos, RTL text, and Asian fonts
Simple And Powerful
- The absolute easiest PowerPoint library to use
- Learn as you code using the built-in typescript definitions
- Tons of sample code comes included (75+ slides of demos)
Export Your Way
- Exports files direct to client browsers with proper MIME-type
- Other export formats available: base64, blob, stream, etc.
- Presentation compression options and more
An introduction to the npm package manager
- Introduction to npm
npm is the standard package manager for Node.js.
In September 2022 over 2.1 million packages were reported being listed in the npm registry, making it the biggest single language code repository on Earth, and you can be sure there is a package for (almost!) everything.
It started as a way to download and manage dependencies of Node.js packages, but it has since become a tool used also in frontend JavaScript.
Yarn and pnpm are alternatives to npm cli. You can check them out as well.
npm manages downloads of dependencies of your project.
- Installing all dependencies
If a project has a package.json file, by running
it will install everything the project needs, in the node_modules folder, creating it if it's not existing already.
- Installing a single package
You can also install a specific package by running
Furthermore, since npm 5, this command adds <package-name> to the package.json file dependencies . Before version 5, you needed to add the flag --save .
Often you'll see more flags added to this command:
- --save-dev installs and adds the entry to the package.json file devDependencies
- --no-save installs but does not add the entry to the package.json file dependencies
- --save-optional installs and adds the entry to the package.json file optionalDependencies
- --no-optional will prevent optional dependencies from being installed
Shorthands of the flags can also be used:
- -D: --save-dev
- -O: --save-optional
The difference between devDependencies and dependencies is that the former contains development tools, like a testing library, while the latter is bundled with the app in production.
As for the optionalDependencies the difference is that build failure of the dependency will not cause installation to fail. But it is your program's responsibility to handle the lack of the dependency. Read more about optional dependencies .
- Updating packages
Updating is also made easy, by running
npm will check all packages for a newer version that satisfies your versioning constraints.
You can specify a single package to update as well:
In addition to plain downloads, npm also manages versioning , so you can specify any specific version of a package, or require a version higher or lower than what you need.
Many times you'll find that a library is only compatible with a major release of another library.
Or a bug in the latest release of a lib, still unfixed, is causing an issue.
Specifying an explicit version of a library also helps to keep everyone on the same exact version of a package, so that the whole team runs the same version until the package.json file is updated.
In all those cases, versioning helps a lot, and npm follows the semantic versioning (semver) standard.
You can install a specific version of a package, by running
- Running Tasks
The package.json file supports a format for specifying command line tasks that can be run by using
For example:
It's very common to use this feature to run Webpack:
So instead of typing those long commands, which are easy to forget or mistype, you can run
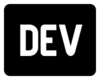
DEV Community

Posted on Jul 14, 2023
A Comprehensive Beginner's Guide to NPM: Simplifying Package Management
In the vast landscape of web development, efficiently managing project dependencies is crucial for seamless development workflows. Enter NPM (Node Package Manager), a robust package manager designed for JavaScript projects, primarily used in conjunction with Node.js. This fully beginner-friendly guide will take you through the fundamentals of NPM, providing you with a solid foundation for simplifying package management and streamlining your development process.
NPM is a command-line tool that facilitates the installation, management, and sharing of reusable JavaScript code modules, known as packages, within your projects. As the default package manager for Node.js, NPM comes bundled with the Node.js installation, making it readily available.
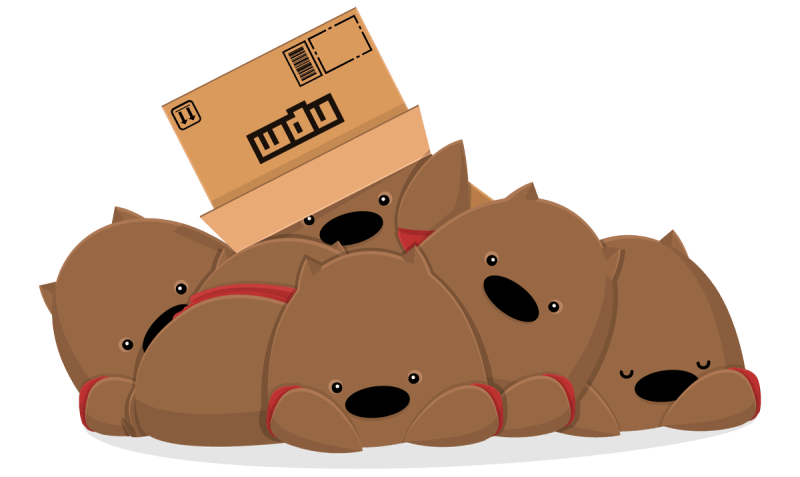
Installing NPM:
Before diving into NPM, you'll need to have Node.js installed on your system. Simply head over to the official Node.js website (nodejs.org) and download the appropriate version for your operating system. Once Node.js is successfully installed, NPM will be at your fingertips through your command prompt or terminal.
And also, npm includes a CLI (Command Line Client) that can be used to download and install the software:
Windows Example C:\>npm install <package>
Mac OS Example >npm install <package>
Here are some beginner-level NPM commands:
npm init This command initializes a new NPM package within your project directory. It creates a package.json file, where you can define project metadata, dependencies, and other configurations.
npm install <package-name> This command installs a specific NPM package and its dependencies into your project. Replace <package-name> with the name of the package you want to install. The package and its dependencies will be downloaded and saved in the node_modules folder.
npm install Running npm install without specifying a package name will install all the dependencies listed in the package.json file. It ensures that all required packages for your project are installed and up to date.
npm uninstall <package-name> This command removes a specific NPM package from your project. Replace <package-name> with the name of the package you want to uninstall. The package and its associated files will be removed from the node_modules folder.
npm update Running npm update updates all the packages listed in the package.json file to their latest versions. It checks for new versions of packages and updates them accordingly. It's important to test your code after running this command to ensure compatibility with the updated packages.
npm outdated The npm outdated command displays a list of installed packages that have newer versions available. It helps you identify which packages are outdated and need to be updated to their latest versions.
npm run <script-name> NPM allows you to define custom scripts in the scripts section of your package.json file. The npm run <script-name> command executes a specific script defined in the package.json file. Replace <script-name> with the name of the script you want to run.
npm publish If you have developed a package and want to make it available to others, the npm publish command helps you publish your package to the NPM registry. It allows other developers to install and use your package in their projects.
You can See a simple NPM Basics Cheat Sheet through this link
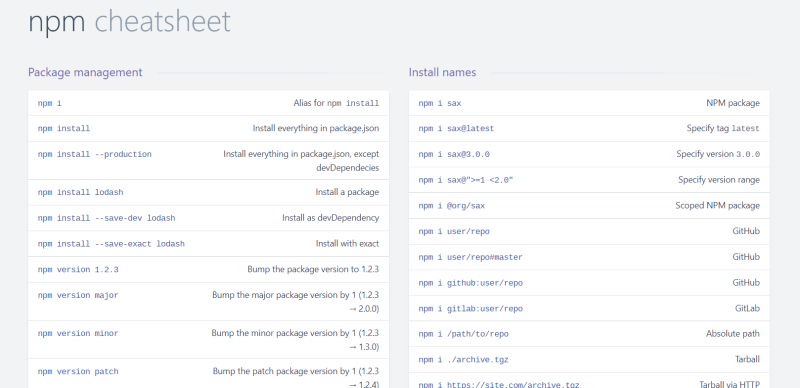
Free Courses :
NPM Full Course For Beginners - Learn NPM fundamentals and basics Node.js Essential Training: Web Servers, Tests, and Deployment
There are some additional points.
Managing Dependencies: NPM simplifies dependency management by allowing you to specify desired package versions and ranges directly within the package.json file. With this approach, NPM ensures that all required packages are installed correctly, thereby avoiding version conflicts and ensuring the stability of your project. To update or remove packages, NPM provides dedicated commands like npm update or npm uninstall, further streamlining the management process.
Unleashing the Power of NPM Scripts: NPM Scripts are a powerful feature that empowers developers to define custom scripts within the package.json file. These scripts can be easily executed via the command line, using the npm run syntax. By harnessing NPM Scripts, you can automate a wide range of tasks, such as running tests, building your project, or deploying to a server, greatly enhancing your development workflow.
Publishing Your Packages: NPM goes beyond consuming packages and enables developers to publish their packages to the NPM registry, thereby contributing to the vibrant JavaScript ecosystem. By creating an account on the NPM website and following a few straightforward steps, you can share your code with the community, receive feedback, and make your mark within the development community.
Introducing the Package.json File:
At the core of every NPM project lies the essential "package.json" file. This file acts as the project's manifesto, housing crucial metadata such as the project name, version, dependencies, and other essential configurations. You can manually create a package.json file or generate one effortlessly by executing the npm init command within your project directory.
NPM serves as a robust and indispensable tool for simplifying package management within JavaScript projects. By familiarizing yourself with NPM's fundamentals, you gain the ability to effortlessly install, manage, and share packages, ultimately improving your development workflow. Armed with this knowledge, you can harness the benefits of NPM to create efficient and maintainable projects, all while enhancing your web development skill set.
Additional resources
NPM website: https://www.npmjs.com/ NPM documentation: https://docs.npmjs.com/ Node.js website: https://nodejs.org/en/ JavaScript tutorial: https://www.w3schools.com/js/
Okay, that’s it for this article. Also, if you have any questions about this or anything else, please feel free to let me know in a comment below or on Instagram , Facebook or Twitter .
Thank you for reading this article, and see you soon in the next one! ❤️
Top comments (7)
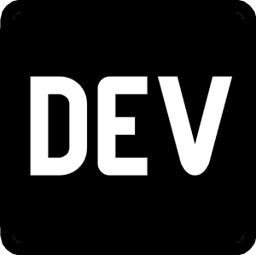
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Colombo, Sri Lanka
- Education Uva Wellassa University
- Pronouns He/Him
- Work ᯤ Graphic Designer @WhiteBox
- Joined Dec 25, 2022

- Email [email protected]
- Location Rathnapura, Sri Lanka
- Education University of Sri Jayewardenepura
- Pronouns she/her
- Joined Feb 7, 2023
Great article.

- Joined Nov 4, 2020
Well written, I super love the writing style!!!

- Location Brazil
- Joined Oct 8, 2019
Abishek Haththakage, Thanks for sharing Great article

- Email [email protected]
- Location Bihar , India
- Joined Nov 18, 2022
Great Article .

- Work Junior Front End Engineer
- Joined Jan 11, 2021
+1 for npm outdated .
Some comments may only be visible to logged-in visitors. Sign in to view all comments. Some comments have been hidden by the post's author - find out more
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
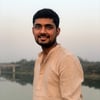
Angular Material Update Guide
Dharmen Shah - Mar 30
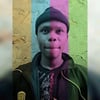
Optimizing React Apps for Performance: A Comprehensive Guide
Humjerry⚓ - Apr 2
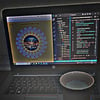
Mastering the Core Concepts of Angular
Muhammad Tanveer - Apr 2
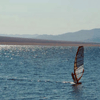
JavaScript News, Updates, and Tutorials: March 2024 Edition
Pavel Lazarev - Apr 2
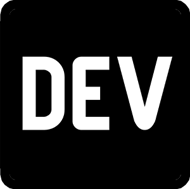
We're a place where coders share, stay up-to-date and grow their careers.
How to Create and Publish an NPM Package – a Step-by-Step Guide
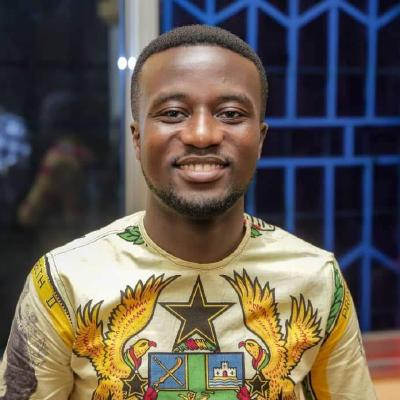
NPM is the largest software registry on the internet. There are over a million packages in the NPM Library.
Developers publish packages on NPM to share their code with others. And organisations also use NPM to share code internally.
In this article, you will learn how to create a package. And you will also learn how to publish your package on NPM so others can download and use it.
Let's get started!
How to Choose a Name For Your NPM Package
The first thing you need to do before creating your package is to choose a name. This is important because your package needs to have a unique name. You should choose a name that has not been used already.
When you decide on a name, go to the NPM registry and run a search. Be sure there's no exact match to the name you chose (or a match that is too similar).
For example, if there's a package called hellonpmpackage and you decide to call yours hello-npm-package , NPM will throw an error when you attempt to publish it.
If there's already a package in the NPM registry with the same you want to use, then you have two options.
- You can choose a different name.
- You can publish your package as a scoped package (see the section "Publishing scoped packages" below).
How to Create a NPM Package
Follow the steps below to create your package.
1. Install Node
If you do not already have Node installed, you should go ahead and install it. You can visit the official website to download and install Node.js . NPM comes pre-installed with Node.
2. Initialize a Git Repository
Create a new project folder for your package and navigate into the folder. Then, run the following command in your terminal:
This will help you track the changes you make to your package. Also, make sure you have a remote version of your repository on GitHub (or your preferred hosting service).
3. Initialize NPM in Your Project
To do this, navigate to the root directory of your project and run the following command:
This command will create a package.json file. You will get prompts to provide the following information:
- package-name : As you learned earlier in this tutorial, the name of your package must be unique. Also it must be lowercase. It may include hyphens.
- version : The initial value is 1.0.0. You update the number when you update your package using semantic versioning .
- description : You can provide a description of your package here. Indicate what your package does and how to use it.
- entry point : The entry file for your code. The default value is index.js .
- test command : Here, you can add the command you want to run when a user runs npm run test .
- git repository : The link to your remote repository on GitHub.
- keywords : Add relevant keywords that will help others find your package on the NPM registry.
- author : Add your name.
- license : You can add a license or use the default license (Internet Systems Consortium (ISC) License).
See the screenshot below for an example of how to answer the prompt questions:
Note: I left the test command blank because there is no test command for the package in this tutorial.
4. Add Your Code
Now, you can go ahead and add the code for your package.
First, you need to create the file that will be loaded when your module is required by another application. For this tutorial, that will be the index.js file.
Inside the index.js file, add the code for your package.
For this tutorial, I will be creating a simple package called first-hello-npm . This package returns the string "Hello NPM!" .
After creating your function, you should export it like in the example above. That way, anyone who downloads your package can load and use it in their code.
If you have been following along, you should now have your package created. But before you publish, you need to test your package. Testing your package reduces the chances of publishing bugs to the NPM registry.
How to Test Your NPM Package
Testing ensures that your NPM package works as expected. There are many ways to test your package. In this tutorial, you will learn one of the simplest ways of testing.
First, navigate to the root of your package project. Then, run the following command:
This will make your package available globally. And you can require the package in a different project to test it out.
Create a test folder. And inside that test folder, add a script.js file.
In the example above, the test folder contains only the script.js file. It does not yet contain the package. To add the package you created to your test folder, run the command below:
In the case of the test folder for this tutorial, I will run the following command:
This will create a node-modules folder. And it'll add all the files and folders from your package – see the screenshot below:
In the script.js file, you can now require your package and use it for the test.
The first-hello-npm package is expected to return the string "hello NPM!" . As you can see from the screenshot below, the package works as expected when I run the script.
After testing your package and ensuring it works as expected, you can now publish it on the NPM registry.
How to Publish Your NPM Package
To publish your package on the NPM registry, you need to have an account. If you don't have an account, visit the NPM sign up page to create one.
After creating the account, open your terminal and run the following command in the root of your package:
You will get a prompt to enter your username and password . If login is successful, you should see a message like this:
Logged in as <your-username> on https://registry.npmjs.org/.
You can now run the following command to publish your package on the NPM registry:
If all goes well, you should get results similar to the screenshot below:
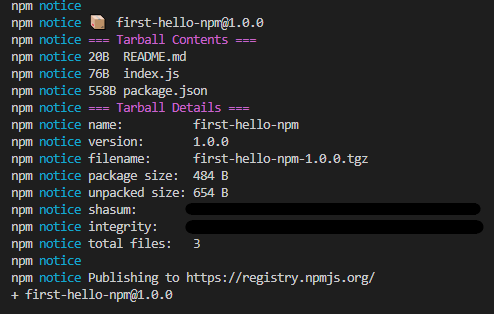
If you have been following along, then congratulations! You just published your first NPM package.
You can visit the NPM website and run a search for your package. You should see your package show up in the search results.
For example, from the screenshot below, you can see the first-hello-npm package is now available on NPM.
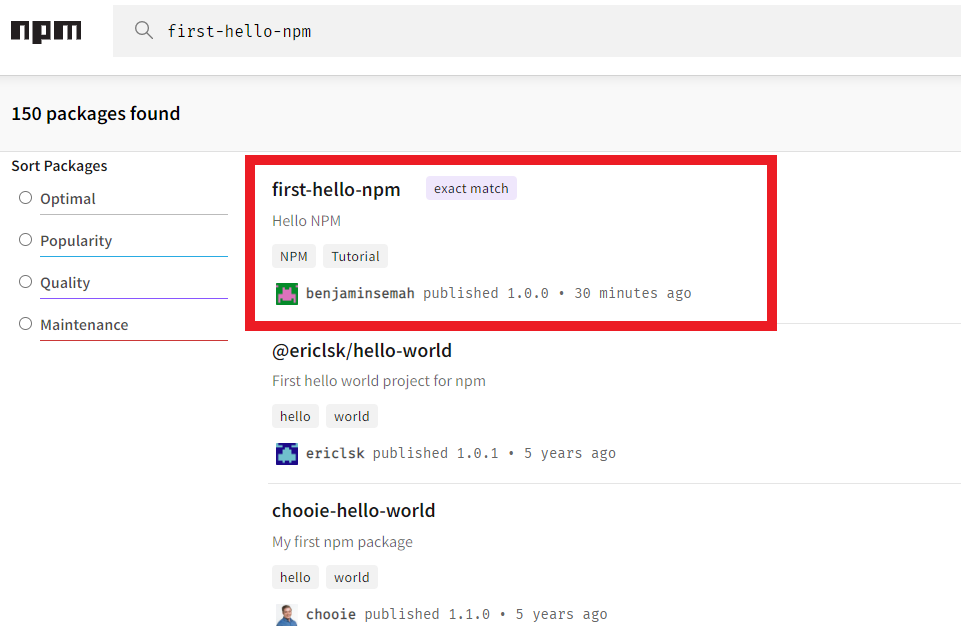
How to Publish Scoped NPM Packages
If an existing package has the same name you would like to use, the workaround is to publish a scoped package.
When you publish a scoped package, you have the option to make it public or private. If it's private, you can choose who you want to share the package with.
How to Create a Scoped NPM Package
To create a scoped package, first navigate to the root of your package directory.
Then, run the npm init command and pass your username as the value to the scope flag:
Respond to the prompts to create a package.json file. For your package name, the format should be @your-username/package-name .
For example @benjaminsemah/first-hello-npm .
You can now add the code for your package and test it. The process is the same as already explained above.
Then, to publish your scoped package, run the following command in your terminal.
You can change from public to private if you don't want to make the package available for public use.
You should see a response similar to this.
Congratulations if you followed along. You've published your scoped package. You should see your scoped package on NPM if you search for it. For example in the screenshot below, you can see the scoped package I created in this tutorial.
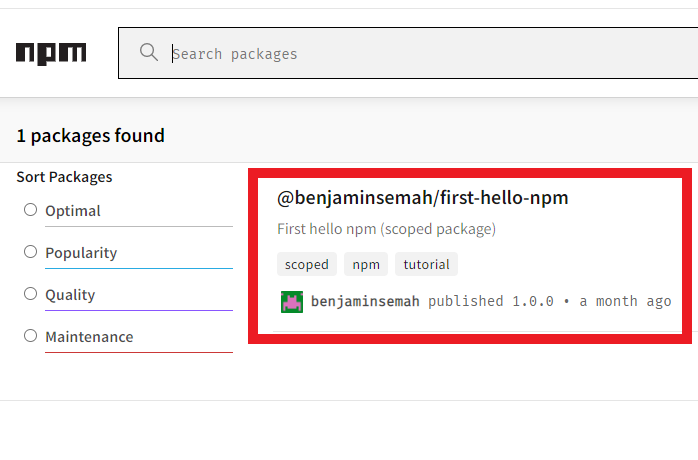
Packages helps developers work faster. And they also improve collaboration. When you figure out a smarter way of doing things, one way you can share with the community is to create and publish your solution as a package.
In this article, you learned what packages are and why they are useful. You also learned how to create and publish packages on the NPM registry. The developer community awaits all the beautiful packages you will create and share.
Thanks for reading. And happy coding!
Software Developer | Technical Writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Site navigation
Packages and modules.
- About the public npm registry
- About packages and modules
- About scopes
- About public packages
- About private packages
- npm package scope, access level, and visibility
- Creating a package.json file
- Creating Node.js modules
- About package README files
- Creating and publishing unscoped public packages
- Creating and publishing scoped public packages
- Creating and publishing private packages
- Package name guidelines
- Specifying dependencies and devDependencies in a package.json file
- About semantic versioning
- Adding dist-tags to packages
- Changing package visibility
- Adding collaborators to private packages owned by a user account
- Updating your published package version number
- Deprecating and undeprecating packages or package versions
- Transferring a package from a user account to another user account
- Unpublishing packages from the registry
- Searching for and choosing packages to download
- Downloading and installing packages locally
- Downloading and installing packages globally
- Resolving EACCES permissions errors when installing packages globally
- Updating packages downloaded from the registry
- Using npm packages in your projects
- Using deprecated packages
- Uninstalling packages and dependencies
- About audit reports
- Auditing package dependencies for security vulnerabilities
- Generating provenance statements
- About ECDSA registry signatures
- Verifying ECDSA registry signatures
- Requiring 2FA for package publishing and settings modification
- Reporting malware in an npm package

Last updated on February 20th, 2024 at 02:32 pm
30 Most Popular NPM Packages for Node JS Developers
What are the best and most popular NPM packages? What package does Node js use ? What is the most used NPM package? What are the most downloaded NPM packages? Looking for answers to these questions? Keep reading.
Node js can handle huge traffic while maintaining seamless performance. As a result, some major tech companies and new-age startups have adopted Node js to scale their businesses. Node is the market leader in asynchronous frameworks due to its popular demand. What’s more, Node isn’t slowing down anytime soon. Starting with Node js is pretty straightforward. Clear guidelines exist and hundreds of projects are available on GitHub for developers to read, review and analyze.
Also, Node js has the most popular package manager – NPM. NPM is the default package manager for the JS runtime environment Node js. Thanks to the modules and libraries available through the NPM package manager, creating a website takes only a few minutes.
Table of Contents
Why should developers use NPM packages for Node.js projects?
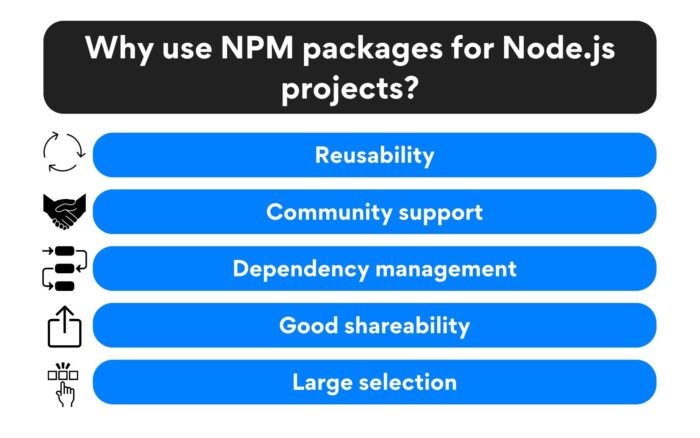
Why use NPM packages for Node.js projects
Using NPM packages for Node.js allows developers to easily include and manage external modules in their projects. These packages, which are published on the NPM registry, can provide additional functionality or utilities that would be time-consuming or difficult to implement from scratch. Additionally, using packages from the NPM registry allows developers to benefit from the work of other developers and easily share and collaborate on their own code.
Using NPM packages in a Node.js project can provide several benefits, including:
- Reusability: NPM packages allow developers to use pre-existing code, which can save time and effort in development.
- Community support: Many popular NPM packages have a large and active community of developers who contribute to and maintain the package, providing bug fixes, new features, and overall improvements.
- Dependency management: NPM automatically manages dependencies between packages, ensuring that the correct versions of dependencies are installed and updated.
- Good shareability: NPM packages can be easily shared and installed across different projects, making collaboration and code sharing more efficient.
- Large selection: NPM has a large selection of packages available, covering a wide range of functionality, including libraries for server-side rendering, data validation, and more.
Is it safe to use NPM packages for Node.js?
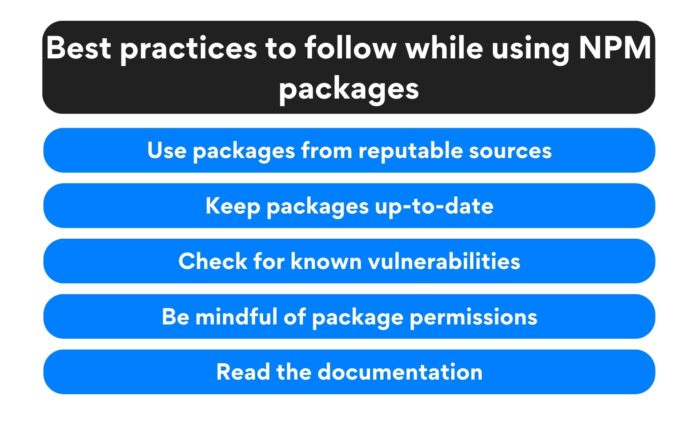
Best practices to follow while using NPM packages
In general, it is safe to use NPM packages for Node.js, as long as you take certain precautions. Here are some best practices to follow when using NPM packages:
- Use packages from reputable sources: Make sure you are using packages from reputable sources, such as well-established and widely-used packages, or packages that have been recommended by trusted developers.
- Keep packages up-to-date: Regularly update your packages to ensure that they are using the latest version, which may include security fixes.
- Check for known vulnerabilities: Use a tool like NPM audit to check for known vulnerabilities in your packages, and take steps to address any issues that are found.
- Be mindful of package permissions: Be aware of the permissions that a package is requesting, and only install packages that have the minimum permissions required for your application.
- Read the documentation: Before installing a package, read the documentation to understand what it does and whether it is suitable for your project.
It’s worth noting that, as with any open-source software , it’s always a good idea to check the code of the package you’re using and see if it contains any suspicious or malicious code.
By following these best practices, you can ensure that your use of NPM packages is as safe as possible.
30 most popular NPM packages
Developers love NPM packages and use them extensively in web development . However, they also get overwhelmed because there are thousands of packages available in the market. So, what should you pick? We’re here to help you make the best choice.
Here is a list of the 30 most popular NPM packages for Node js developers .
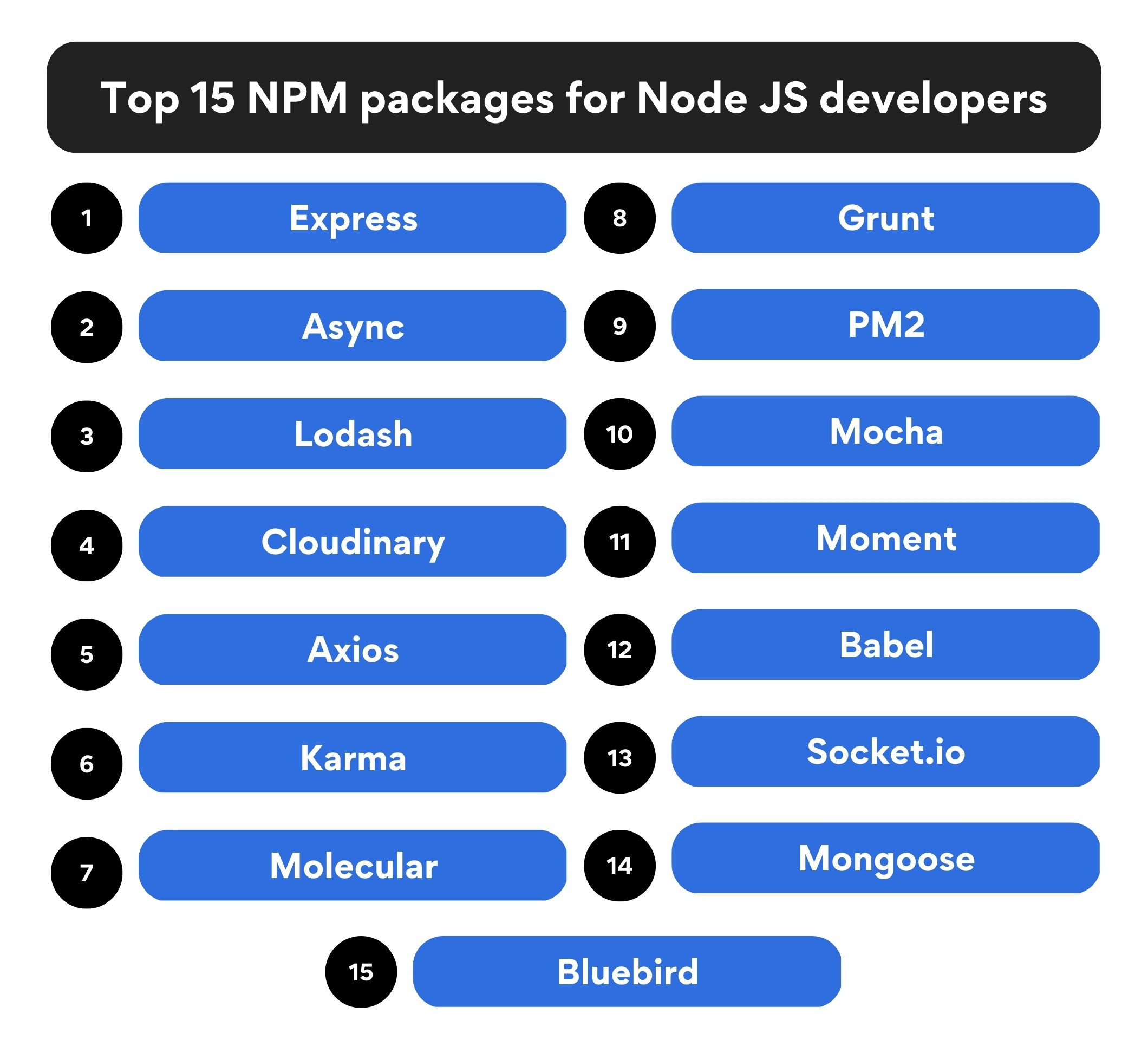
Top 15 most popular NPM packages for Node JS developers
Features of Express js
- Robust routing
- Focus on high-quality performance
- Super high test coverage
- HTTP helpers (such as redirection and caching)
- Content negation
- Executable for developing apps and APIs
Features of Async
- Async supports inline functions and text strings.
- Error handling from the dependency queue.
- The use of AsyncLocalStorge within Async js creates asynchronous states within callbacks and promise chains.
- A collection of Async functions controls the flow through the script.
- Async helps developers avoid memory leaks.
- Async helps developers integrate AsyncResource with EvenEmitter.
- Async supports asynchronous functions.
Features of Lodash
- Keep the Node js code minimal and neat.
- Develop ers only need to remember Lodash functions. This action makes coding easier.
- New developers can understand Lodash quickly.
Features of Cloudinary
- Remote fetch, auto-backup, and revision tracking.
- Image and video transformations.
- Video transcoding and adaptive streaming.
- High-performance delivery through a CDN.
- Support for forums, tickets, and email.
- Access to free add-ons.
Features of Axios
- Allows developers to make simultaneous requests.
- Axios supports promised base requests.
- Transforms responses and requests to JSON data.
- Requests and responses to interceptions.
- Support HTTP requests for Node js apps
- Supports HTTP requests from browsers.
Features of Karma
- Tests code in real browsers.
- Tests code in multiple browsers (mobile phones, tablets, and desktops).
- Controls the whole testing workflow from command or IDE.
- Executes tests after every save.
- Executes tests locally during development.
- Executes tests on a continuous integration server.
Features of Molecular
- Molecular uses promise-based solutions.
- Molecular supports event-driven architecture with balancing.
- Molecular supports versioned services.
- Molecular has built-in caching solutions such as memory and Reds.
- Molecular has built-in metrics features with reporters, such as Console, CSV, Datagod, and StatsD.
- Molecular has a built-in service registry and dynamic service discovery.
- Molecular employs a request-reply concept.
- Molecular has fault tolerance features such as Fallback, Timeout, Retry, Bullhead, and Circuit Breaker.
Features of Grunt
- Grunt has a plethora of plugins that can automate even the most monotonous jobs.
- Grunt allows developers to publish Grunt-specific plugins through NPM .
Features of PM2
- Behavior Configuration
- Container Integration
- Watch & Reload
- Log management
- Max memory reload
- Multiple browser support.
- Simple async support, including promises.
- Test coverage reporting.
- String diff support.
- JavaScript API for running tests.
- Auto-detects and disables coloring for non-TTYs.
- Async test timeout support.
Moment offers a lot of customization and flexibility. With functions like add, subtract, startOf, endOf, and others, dates can be easily manipulated while supporting a wide range of date and time formats as well as timezones.
Key features of Moment:
Portable and simple to use
Various date and time formats that are supported
Comprehensive timezone support
Powerful formatting and manipulation tools
Compatible with web browsers and Node.js.
Babel has many configuration options and may be set up to accommodate a variety of plugins and presets. It is a potent tool for contemporary web development because it also has capabilities for debugging and code optimization.
Key features of Babel:
Creates code that is backward compatible with contemporary JavaScript.
Utilizing presets and plugins to customize
Supports code optimization and debugging
Popular in today’s web development.
Since Socket.io supports a variety of protocols and fallback options, clients and servers can communicate without interruption even when there are problems with the network. With a wide range of setup options and support for different events kinds, it is also quite flexible.
Key features of Socket.io:
Bidirectional, real-time interaction between clients and servers
Includes fallback options and supports several protocols
Highly adaptable and accommodating of different event types
Widely used, especially for real-time applications, in web development.
Mongoose is perfect for complicated data models since it allows a wide variety of data types and connections between them. With support for plugins that add new features, it is also quite extensible.
Key features of Mongoose:
Offers a layer for object data modeling for MongoDB databases.
Makes it easier for Node.js apps to interface with MongoDB databases
Enables a variety of data kinds and associations
Highly extensible and plug-in compatible.
Bluebird can function easily alongside other JavaScript libraries and frameworks since it is so compatible with them. Large-scale Node.js applications are frequently powered by it in production situations.
Key features of Bluebird:
Provides a powerful and efficient implementation of Promises
Provides a variety of features and performance improvements.
Extremely compatible with other JavaScript frameworks and libraries Widely used in production environments.
- Component-based architecture for building reusable UI elements.
- Virtual DOM for efficient rendering and performance optimization.
- The declarative syntax for describing UI state and handling UI updates.
- A large ecosystem with extensive community support and third-party libraries.
- Centralized state management for predictable application behavior.
- Immutable state updates for easier debugging and time-travel debugging.
- Middleware support for intercepting and extending functionality.
- Compatibility with different UI frameworks.
Support for both synchronous and asynchronous testing.
Snapshot testing for detecting unintended changes in UI components.
Mocking and spying capabilities for isolating dependencies.
Built-in code coverage reporting.
Also, read: 10 Popular Software Testing Trends in 2024
- Bundling of JavaScript, CSS, and other assets for efficient delivery.
- Support for module-based development using import/export syntax.
- Code splitting for optimized loading and lazy loading of resources.
- Extensive plugin system for customizing the build process.
Declarative data fetching, enabling clients to request only the specific data they need.
Strong typing and schema definition for robust and self-documented APIs.
Efficient and performant data retrieval through batched requests.
Support for real-time updates and subscriptions.
- A declarative approach to handling complex asynchronous flows.
- Generator-based functions for expressing async logic in a sequential and testable manner.
- Fine-grained control over the handling of async actions and their effects.
- Integration with Redux DevTools for debugging and time-travel debugging.
- Support for various email delivery methods, including SMTP, Sendmail, and Amazon SES.
- Comprehensive email composition capabilities, including HTML content and attachments.
- Templating support for dynamic email generation.
- Error handling and event-based notifications.
React-Router
- Declarative routing with dynamic matching and rendering of components.
- Support for nested routes and route parameters.
- Browser history management for navigation and URL synchronization.
- Code-splitting and lazy loading of route components.
React Native
- Cross-platform development with reusable components and codebase.
- Access to native device APIs and functionalities.
- Hot reloading for fast development and instant UI updates.
- Performance is comparable to native apps.
- Has jQuery-like syntax for traversing and manipulating HTML as well as XML documents.
- Lightweight and efficient, designed for server-side rendering and scraping tasks.
- Support for CSS selectors for easy element selection.
- Integration with other libraries for data extraction and manipulation.
- Simplifies configuration management by storing sensitive information in a .env file.
- Seamless integration with Node.js applications.
- Support for different environments (development, production, etc.) with separate .env files.
- Variable expansion and interpolation for complex configurations.
Passport.js
- Support for multiple authentication strategies, including local, OAuth, OpenID, and more.
- Easy integration with Express and other Node.js frameworks.
- Session management and persistent login sessions.
- Customizable and extensible to fit different authentication requirements.
- Support for multiple log transports, including console, file, database, and more.
- Configurable log levels and formatting options.
- Integration with popular frameworks and libraries.
- Asynchronous logging for performance optimization.
- Efficient image resizing and transformation operations.
- Support for various image formats, including JPEG, PNG, and WebP.
- Advanced image manipulation, such as overlaying, watermarking, and applying filters.
- Optimized for speed and memory usage.
- Automated web scraping and crawling.
- Web testing and UI interaction automation.
- PDF generation and screenshot capturing.
- Performance monitoring and analysis.
Open-source frameworks help developers improve their skills in delivering professional applications that are lightweight and reliable. They make the overall development process smooth and efficient. Therefore, knowing these packages is absolutely necessary for developers.
We hope you found this list of the most popular NPM packages useful.
If you are an experienced Node js developer who wishes to work on projects with major US-based tech companies and enjoy excellent pay, visit Turing.com.
With Turing, you can apply to remote US software jobs from the comfort of your home. Also, you can join a network of the world’s best developers and get full-time, long-term remote software jobs with solid compensation and career growth.
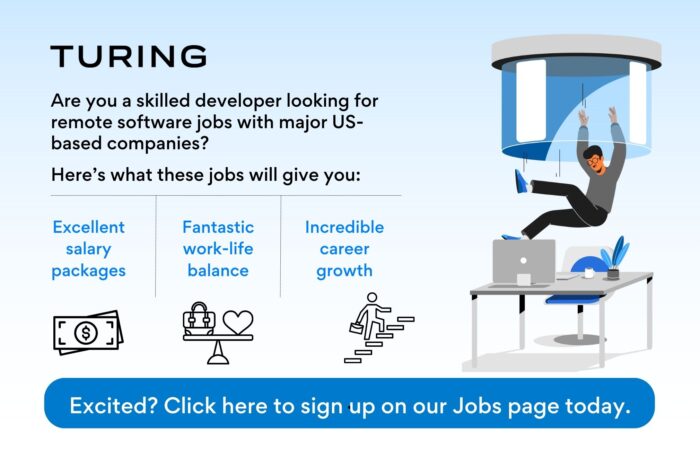
- How many packages are there in NPM? Over 1.3 million packages are available in the main NPM registry.
- What is the NPM package in Node JS? NPM Inc. maintains NPM, a package manager for the JavaScript programming language. For the JS runtime environment Node js, NPM is the default package manager.
- How do NPM packages work? NPM install is a command that downloads a package. NPM install can be used with or without parameters. NPM install downloads dependencies defined in a package.json file and creates a node module folder with the installed modules when called without parameters.
- What are the most popular NPM packages? The largest software registry in the world, NPM has more than 1.5 million packages. Express, React, Lodash, Async, and Request are some of the NPM packages that are most frequently used. With the help of these packages, Node.js and JavaScript programmers may create apps more quickly and effectively.
- How to find the best NPM packages? It’s critical to take into account unique project requirements, package popularity and usage, update frequency, community support, and documentation quality while looking for the finest NPM packages. Check the package’s GitHub repository and issue tracker for bugs and security issues. Research and contrast related packages. Analyze the overall stability and dependability of the package. Follow new trends and experiment with various packages.
- Is NPM package an API? No, an NPM package is not an API. A group of JavaScript files and assets known as an “NPM package” offer a particular feature, such as libraries or tools. On the other hand, APIs are collections of rules and guidelines that let various software programs talk to one another. NPM packages themselves are not APIs, even though some of them may contain APIs or offer interfaces for interacting with external APIs.
- What are the three components of NPM? The registry, command-line interface (CLI), and package.json file are the three parts of NPM (short for Node Package Manager). Over a million packages of open-source code for Node.js are housed in the registry, a public database. The CLI is a tool that enables developers to interact with the registry and install, publish, and manage packages. The package.json file is a metadata file used to automate operations like installation and build procedures. It contains facts about the project, including its dependencies, scripts, and other configuration information.
Tell us the skills you need and we'll find the best developer for you in days, not weeks.
Hire Developers
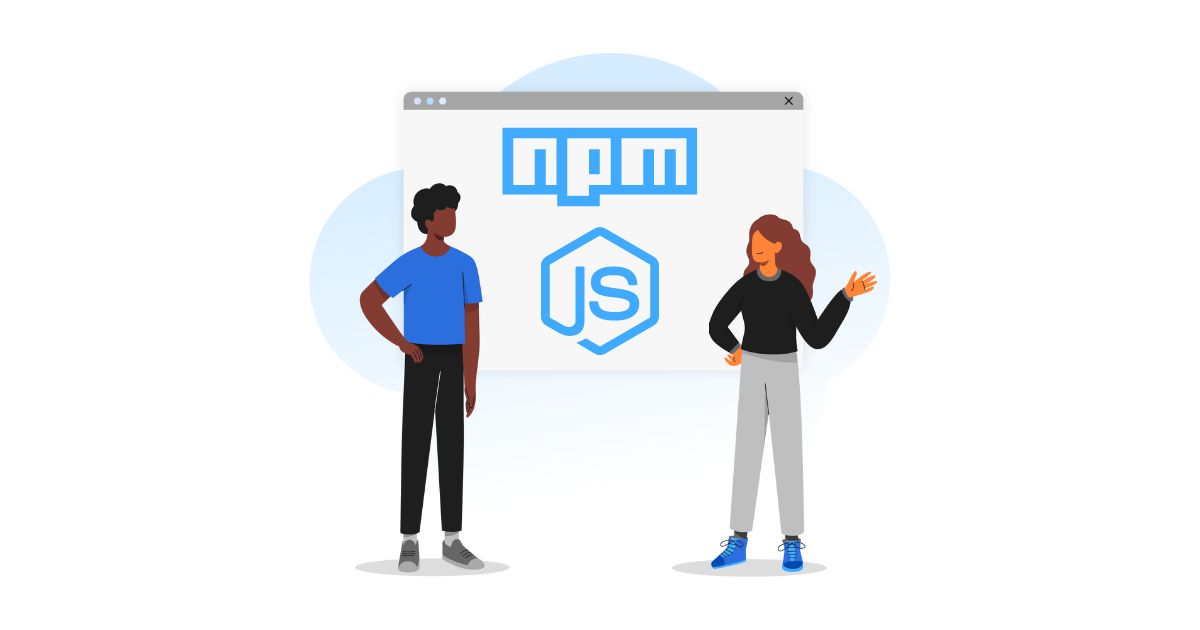
Ankit is a writer and editor who has contributed his expertise to Govt of India, Reebok, Abbott, TimesPro, Chitale Bandhu, InsideAIML, Kolte Patil Dev., etc.
View all posts

Ritvik is a copywriter and content writer who has worked with fast-scaling startups such as GoMechanic and Pitstop. He runs his own automotive blog as well.

Cancel Reply
- Takata Recall
- Crown Signia
- Grand Highlander
- Land Cruiser
- Concept Vehicles
- Historic Vehicles
2025 Toyota 4Runner
- CALTY 50th Anniversary
- 2024 Toyota Land Cruiser
- 2024 Toyota Tacoma
- New Product Showcase
- Los Angeles
- Global Shows
- What’s New for 2025
- What’s New for 2024
- What’s New for 2023
- What’s New for 2022
- Images & Videos
Latest News
- Sales & Financial
- Voluntary Recalls
- Takata Info
- Environmental
- Community Engagement
- Diversity & Inclusion
- Finance, Insurance & Banking
- Advanced Technology
- Research & Development
- Safety Technology
- Motorsports
- Company History
- Executive Bios
- Media Contacts

2025 Toyota 4Runner Refines Adventure Ready Heritage
SAN DIEGO, Calif. (April 9, 2024) – The instant name recognition that 4Runner has built over the past four decades is undeniable. This legend has long established itself as a North American off-road icon, ready to tackle tough terrain in its way. The all-new 2025 Toyota 4Runner stays true to its original concept, as an extremely capable, dynamic, and durable off-roader and will continue to provide owners a window to bigger worlds. The new generation introduces a bold new look and adds new technology, premium materials, options, and safety. In fact, this all-new sixth generation 4Runner raises the bar in terms of go-anywhere capability and high-end refinement. The 2025 4Runner will be built in Toyota Motor Corporation’s Tahara plant in Japan and will arrive in the U.S. in the fall of 2024.
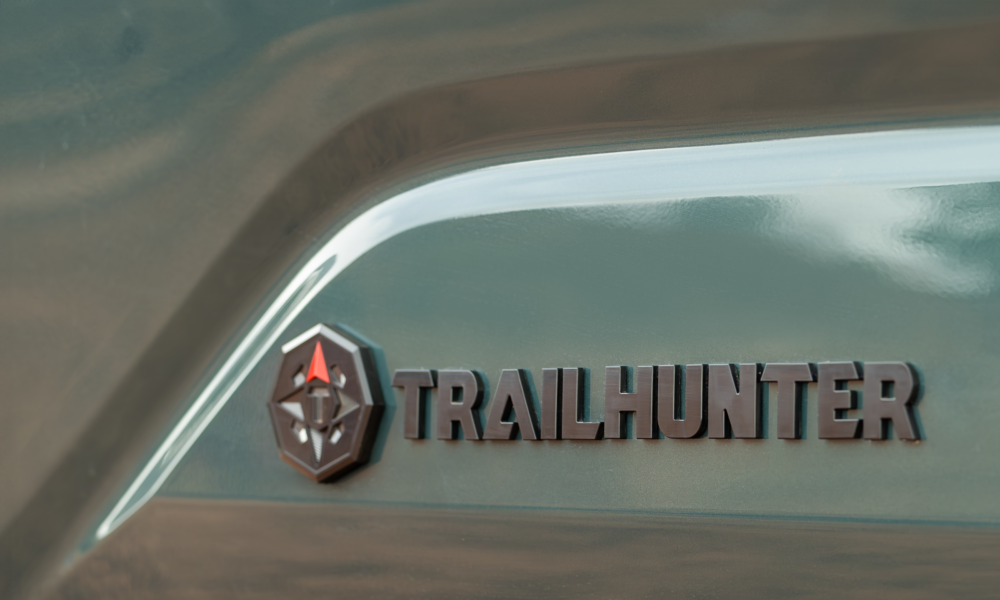
The All-New 4Runner Answers the Call of the Wild. Coming April 9
PLANO, Texas (April 8, 2024) – Toyota’s Trailhunter line up is expanding. Learn more when the all-new 2025 4Runner makes its world debut, April 9, at 7:15pm Pacific Daylight Time.

Fresh Air for Your Wild Side in the Next Generation 4Runner
PLANO, Texas (April 4, 2024) – A window to bigger worlds and adventures is just around the corner. Stay tuned for more #4Runner updates.

- + Select All
- - DeSelect All

2025_Toyota_4Runner_Group_01

2025_Toyota_4Runner_Limited_BrownLeather_01

2025_Toyota_4Runner_Limited_BrownLeather_02

2025_Toyota_4Runner_Limited_Brownleather_03

2025_Toyota_4Runner_Limited_Brownleather_04

2025_Toyota_4Runner_Limited_HeritageBlue_01

2025_Toyota_4Runner_TRDPro_Black_01

2025_Toyota_4Runner_TRDPro_Black_05

2025_Toyota_4Runner_TRDPro_Black_06

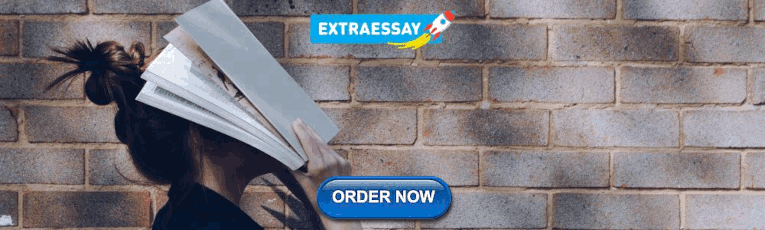
2025_Toyota_4Runner_TRDPro_Black_07

2025_Toyota_4Runner_TRDPro_Black_08

2025_Toyota_4Runner_TRDPro_Mudbath_01

2025_Toyota_4Runner_TRDPro_Mudbath_05

2025_Toyota_4Runner_TRDPro_Mudbath_03

2025_Toyota_4Runner_Trailhunter_Everest_01

2025_Toyota_4Runner_Trailhunter_Everest_02

2025_Toyota_4Runner_Trailhunter_Everest_03

2025_Toyota_4Runner_Trailhunter_Everest_04

2025_Toyota_4Runner_TRDPro_Mudbath_02

2025_Toyota_4Runner_TRD Pro_Black_INT_B_Roll

2025_Toyota_4Runner_TRD Pro_Mudbath_EXT_B_Roll

2025_Toyota_4Runner_Trailhunter_Everest_EXT_B_Roll

2025_Toyota_4Runner_Trailhunter_Mineral_INT_B_Roll

2025_Toyota_4Runner_YT
Email Sign Up
Enter your email address below to sign up for email alerts.
* Indicates Required
Thank you for subscribing. Please check your email to validate your sign up.
You are already subscribed, your mailing lists have been updated.
Copyright Notice
All materials on this site are for editorial use only. The use of these materials for advertising, marketing or any other commercial purpose is prohibited. They may be cropped but not otherwise modified. To download these materials, you must agree to abide by these terms.
- JavaScript packages
presentation-models
The augmented.js next - presentation dom module. for more information about how to use this package see readme.

Ensure you're using the healthiest npm packages
Snyk scans all the packages in your projects for vulnerabilities and provides automated fix advice
Package Health Score
- security No known security issues
- popularity Limited
- maintenance Inactive
- community Limited
Explore Similar Packages
- augmentedjs-next 46
- augmentedjs-next-presentation 46
- augmentedjs-service 36
Security and license risk for significant versions
Is your project affected by vulnerabilities.
Scan your projects for vulnerabilities. Fix quickly with automated fixes. Get started with Snyk for free.
Weekly Downloads (38)
Direct usage popularity.
The npm package presentation-models receives a total of 38 downloads a week. As such, we scored presentation-models popularity level to be Limited.
Based on project statistics from the GitHub repository for the npm package presentation-models, we found that it has been starred ? times.
Downloads are calculated as moving averages for a period of the last 12 months, excluding weekends and known missing data points.
This project has seen only 10 or less contributors.
We found a way for you to contribute to the project! Looks like presentation-models is missing a Code of Conduct.
Embed Package Health Score Badge
Maintenance, commit frequency.
Further analysis of the maintenance status of presentation-models based on released npm versions cadence, the repository activity, and other data points determined that its maintenance is Inactive.
An important project maintenance signal to consider for presentation-models is that it hasn't seen any new versions released to npm in the past 12 months , and could be considered as a discontinued project, or that which receives low attention from its maintainers.
In the past month we didn't find any pull request activity or change in issues status has been detected for the GitHub repository.
Keep your project healthy
Check your package.json, snyk vulnerability scanner.
presentation-models has more than a single and default latest tag published for the npm package. This means, there may be other tags available for this package, such as next to indicate future releases, or stable to indicate stable releases.
Augmented.js Presentation Models & Collections Module
Table of Contents
Previouspage, paginationfactory.
Extends AbstractModel
Model Supports:
- Validation and Schemas
- options (optional, default {} )
- args ...any
crossOrigin
Cross Origin property
- crossOrigin boolean Cross Origin property
- uri string The uri for the datasource (if applicable)
sync - Sync model data to bound REST call
- method (optional, default "READ" )
Fetch the model
- options object Any options to pass
Save the model
Update the model
Destroy the model
Extends AbstractCollection
Collection Class
Sync collection data to bound REST call
- method string The method to Unsuccessfull
- model Model The model to Sync
- options object The options to pass (optional, default {} )
Returns function Returns a request function
Fetch the collection
Save the collection
Update the collection
Destroy the collection
LocalStorageCollection
A local storage-based Collection
Base key name for the collection (simular to url for rest-based)
- key string The key
is Persistant or not
- persist boolean Persistant property
The namespace
- namespace boolean
Initialize the model with needed wireing
Custom init method for the model (called at inititlize)
Sync method for Collection
PaginatedCollection
Extends Collection
Paginated Collection Class - A Collection that handles pagination from client or server-side
setPageSize
Current page for the collection
Total pages for the collection
Sets the number of items in a page
- size number Number of items in each page
setCurrentPage
Sets the current page
- page number Current page in collection
Collection.fetch - rewritten fetch method from Backbone.Collection.fetch
Moves to the next page
Moves to the previous page
Goes to page
- page number Page to go to
Moves to the first page
Moves to the last page
Refreshes the collection
Pagination factory for returning pagination collections of an API type
PAGINATION_API_TYPE
Types of pagination API
- GITHUB Symbol GitHub API
- SOLR Symbol SOLR API
- DATABASE Symbol Database-like API
getPaginatedCollection
Get a pagination collection of type
- apiType Pagination.PAGINATION_API_TYPE The API type to return an instance of
- data object Collection models
- options object Collection options
presentation-models dependencies
Presentation-models development dependencies, what is presentation-models.
The Augmented.js Next - Presentation Dom Module. Visit Snyk Advisor to see a full health score report for presentation-models, including popularity, security, maintenance & community analysis.
Is presentation-models popular?
The npm package presentation-models receives a total of 38 weekly downloads. As such, presentation-models popularity was classified as limited . Visit the popularity section on Snyk Advisor to see the full health analysis.
Is presentation-models well maintained?
We found indications that presentation-models is an Inactive project. See the full package health analysis to learn more about the package maintenance status.
Is presentation-models safe to use?
The npm package presentation-models was scanned for known vulnerabilities and missing license, and no issues were found. Thus the package was deemed as safe to use . See the full health analysis review .
Build a secure application checklist
Select a recommended open source package.
Minimize your risk by selecting secure & well maintained open source packages
Scan your app for vulnerabilities
Scan your application to find vulnerabilities in your: source code, open source dependencies, containers and configuration files
Source Code
Open source, fix identified vulnerabilities.
Easily fix your code by leveraging automatically generated PRs
Monitor for new issues
New vulnerabilities are discovered every day. Get notified if your application is affected
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[BUG] failed optional dependency builds should not prevent global install of packages #7355
rigor789 commented Apr 9, 2024
Sorry, something went wrong.
wraithgar commented Apr 9, 2024
- 👍 1 reaction
No branches or pull requests
presentation-models
- 3 Dependencies
- 11 Dependents
- 21 Versions
Augmented.js Presentation Models & Collections Module
Table of Contents
Previouspage, paginationfactory.
Extends AbstractModel
Model Supports:
- Validation and Schemas
- options (optional, default {} )
- args ...any
crossOrigin
Cross Origin property
- crossOrigin boolean Cross Origin property
- uri string The uri for the datasource (if applicable)
sync - Sync model data to bound REST call
- method (optional, default "READ" )
Fetch the model
- options object Any options to pass
Save the model
Update the model
Destroy the model
Extends AbstractCollection
Collection Class
Sync collection data to bound REST call
- method string The method to Unsuccessfull
- model Model The model to Sync
- options object The options to pass (optional, default {} )
Returns function Returns a request function
Fetch the collection
Save the collection
Update the collection
Destroy the collection
LocalStorageCollection
A local storage-based Collection
Base key name for the collection (simular to url for rest-based)
- key string The key
is Persistant or not
- persist boolean Persistant property
The namespace
- namespace boolean
Initialize the model with needed wireing
Custom init method for the model (called at inititlize)
Sync method for Collection
PaginatedCollection
Extends Collection
Paginated Collection Class - A Collection that handles pagination from client or server-side
setPageSize
Current page for the collection
Total pages for the collection
Sets the number of items in a page
- size number Number of items in each page
setCurrentPage
Sets the current page
- page number Current page in collection
Collection.fetch - rewritten fetch method from Backbone.Collection.fetch
Moves to the next page
Moves to the previous page
Goes to page
- page number Page to go to
Moves to the first page
Moves to the last page
Refreshes the collection
Pagination factory for returning pagination collections of an API type
PAGINATION_API_TYPE
Types of pagination API
- GITHUB Symbol GitHub API
- SOLR Symbol SOLR API
- DATABASE Symbol Database-like API
getPaginatedCollection
Get a pagination collection of type
- apiType Pagination.PAGINATION_API_TYPE The API type to return an instance of
- data object Collection models
- options object Collection options
- augmentedjs
- presentation
Package Sidebar
npm i presentation-models
Git github.com/Augmentedjs/presentation-models
www.augmentedjs.com
Downloads Weekly Downloads
Unpacked size, total files, last publish.
4 years ago
Collaborators
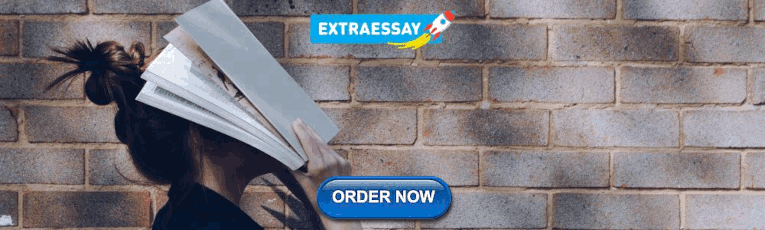
IMAGES
VIDEO
COMMENTS
Create JavaScript PowerPoint Presentations. Latest version: 3.12.0, last published: a year ago. Start using pptxgenjs in your project by running `npm i pptxgenjs`. There are 58 other projects in the npm registry using pptxgenjs.
Start using react-use-presentation in your project by running `npm i react-use-presentation`. There are no other projects in the npm registry using react-use-presentation. Create pure HTML (React enriched if you will) presentations.. Latest version: 1.4.3, last published: 10 months ago. ... Package Sidebar Install. npm i react-use-presentation ...
Create PowerPoint presentations with JavaScript The most popular powerpoint+js library on npm with 1,800 stars on GitHub. Get Started. Demos. HTML to PPTX. Works Everywhere. Every modern desktop and mobile browser is supported; Integrates with Node, Angular, React, and Electron;
NOTE: Someone else registered the node-pptx npm package out from under us. Use this package instead: $ npm i nodejs-pptx. Let's create a very simple presentation with one slide. ... Presentation.getSlide() also supports integer slide numbers (slide numbers are base-1). For example, to grab the very first slide of a PPTX, you would call ...
Presentation slides for developers. Latest version: .48.-beta.14, last published: 7 hours ago. Start using @slidev/cli in your project by running `npm i @slidev/cli`. ... 🎨 Themable - theme can be shared and used with npm packages. 🌈 Stylish - on-demand utilities via UnoCSS. 🤹 Interactive - embedding Vue components seamlessly.
Create PowerPoint presentations with a powerful, concise JavaScript API. - gitbrent/PptxGenJS. ... Automate any workflow Packages. Host and manage packages Security. Find and fix vulnerabilities Codespaces. Instant dev environments Copilot. Write better code with AI Code review ... Dzmitry Dulko - Getting the project published on NPM;
Features. 🎨 Themable - theme can be shared and used with npm packages. 🌈 Stylish - on-demand utilities via UnoCSS. 🤹 Interactive - embedding Vue components seamlessly. 🎙 Presenter Mode - use another window, or even your phone to control your slides. 🧮 LaTeX - built-in LaTeX math equations support.
Introduction to npm. npm is the standard package manager for Node.js. In September 2022 over 2.1 million packages were reported being listed in the npm registry, making it the biggest single language code repository on Earth, and you can be sure there is a package for (almost!) everything. It started as a way to download and manage dependencies ...
Node Package Manager (NPM) is the world's largest software registry, housing over a million packages that enable developers to share and borrow packages, and assemble them in powerful new ways. An NPM package is a reusable piece of code that you can download and install into your projects, encapsulating functionality that can be easily shared ...
Here are some beginner-level NPM commands: npm init. This command initializes a new NPM package within your project directory. It creates a package.json file, where you can define project metadata, dependencies, and other configurations. npm install <package-name>. This command installs a specific NPM package and its dependencies into your project.
To publish your npm package, you run the well-named command: npm publish. So we have an empty package.json in our folder and we'll give it a try: npm publish. Whoops! We got an error: npm ERR! file package.json npm ERR! code EJSONPARSE npm ERR! Failed to parse json npm ERR! Unexpected end of JSON input while parsing near '' npm ERR!
To do this, navigate to the root directory of your project and run the following command: npm init. This command will create a package.json file. You will get prompts to provide the following information: package-name: As you learned earlier in this tutorial, the name of your package must be unique.
About private packages. npm package scope, access level, and visibility. Contributing packages to the registry. Creating a package.json file. Creating Node.js modules. About package README files. Creating and publishing unscoped public packages. Creating and publishing scoped public packages.
Community support: Many popular NPM packages have a large and active community of developers who contribute to and maintain the package, providing bug fixes, new features, and overall improvements. Dependency management: NPM automatically manages dependencies between packages, ensuring that the correct versions of dependencies are installed and ...
If no package name is provided, it will search for a package.json in the current folder and use the name property. Syntax : - npm bugs <pkgname> npm bugs (with no args in a package dir) npm - build. This is the plumbing command called by npm link and npm install. It should generally not be called directly.
Explore over 1 million open source packages. Learn more about presentation-application: package health score, popularity, security, maintenance, versions and more. presentation-application - npm package | Snyk
To further understand the popularity of each one, I summarized the downloads (last month) of each packages and came up with this graphic: For instance Coffee-script package last month downloads is 2,478,652. After adding all those packages, I come up to a total 13,213,401 of downloads. This graph shows that for Coffee-script for instance ...
The All-New 4Runner Answers the Call of the Wild. Coming April 9. April 08, 2024. PLANO, Texas (April 8, 2024) - Toyota's Trailhunter line up is expanding. Learn more when the all-new 2025 4Runner makes its world debut, April 9, at 7:15pm Pacific Daylight Time. Read More.
The Augmented.js Next - Presentation SASS/CSS Module.. Latest version: 4.16.1, last published: 10 days ago. Start using presentation-css in your project by running `npm i presentation-css`. There are 11 other projects in the npm registry using presentation-css.
This package can automatically use the azureauth CLI to fetch tokens and update a user's .npmrc file for authenticating to Azure DevOps npm feeds. - microsoft/ado-npm-auth
The npm package presentation receives a total of 1 downloads a week. As such, we scored presentation popularity level to be Limited. Based on project statistics from the GitHub repository for the npm package presentation, we found that it has been starred 3 times. Downloads are calculated as moving averages for a period of the last 12 months ...
This lightweight npm package provides a utility for checking the strength of passwords based on various criteria such as presence of uppercase and lowercase characters, digits, special characters, and minimum length. It returns a detailed result object containing information about the password strength and any missing criteria.
IIIF Presentation v3.0 typescript types. Latest version: 2.1.3, last published: 2 months ago. Start using @iiif/presentation-3 in your project by running `npm i @iiif/presentation-3`. There are 18 other projects in the npm registry using @iiif/presentation-3.
The npm package presentation-models receives a total of 43 downloads a week. As such, we scored presentation-models popularity level to be Limited. Based on project statistics from the GitHub repository for the npm package presentation-models, we found that it has been starred ? times.
Expected Behavior. Global installs should not fail. fsevents is an optional peerDependency, and installing it should not trigger a rebuild, nor fail on unsupported systems (like Linux/Windows).. Steps To Reproduce. install npm 10.4 or newer; run npm i -g nativescript; the install will fail; To confirm the linked change breaks it:
I have been working with whatsapp-wb.js for a while. Recently the package is not working anymore. Firstly, when I try their latest package i.e. "whatsapp-web.js": "^1.23.0", even before the qr code shows up, it throws:
The Augmented.js Next - Presentation Dom Module.. Latest version: 3.1.6, last published: 3 years ago. Start using presentation-models in your project by running `npm i presentation-models`. There are 11 other projects in the npm registry using presentation-models.