- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
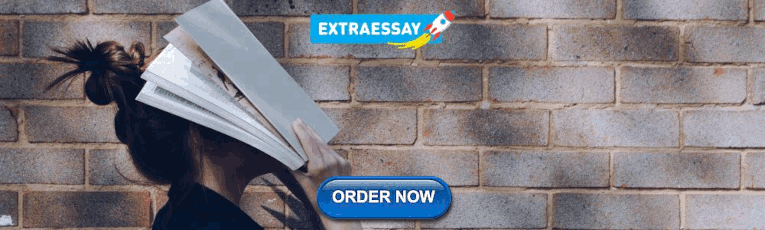
JavaScript ReferenceError – Assignment to undeclared variable
This JavaScript exception Assignment to undeclared variable occurs in strict-mode If the value has been assigned to an undeclared variable.
Error Type:
Cause of the error: Somewhere in the code, there is an assignment without the var , let, or const keywords. When a value is assigned to an undeclared variable this error occurs.
Example 1: In this example, the const keyword is used with the variable assignment, So the error has not occurred.
Output:
Example 2: In this example, the var , let or const keyword is not used with the variable assignment, So the error has occurred.
Similar Reads
- Web Technologies
- JavaScript-Errors
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?
- Skip to main content
- Select language
- Skip to search
ReferenceError: assignment to undeclared variable "x"
Valid cases.
ReferenceError warning in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
To make "bar" a declared variable, you can add the var keyword in front of it.
- Strict mode
Document Tags and Contributors
- ReferenceError
- Strict Mode
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting a property that has only a getter
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

- Get Started
Dec 28, 2016 8:00:56 AM | JavaScript Error Handling - ReferenceError: assignment to undeclared variable “x”
The Assignment to Undeclared Variable error crops up anytime code attempts to assign a value to a variable that has yet to be declared.
Today as we progress through our JavaScript Error Handling series we're going to take a closer look at the Assignment to Undeclared Variable error in all its magnificent glory. The Assignment to Undeclared Variable error crops up anytime code attempts to assign a value to a variable that has yet to be declared (via the var keyword).
Below we'll go over a few code examples that will illustrate reproduction of a typical Assignment to Undeclared Variableerror, as well as outline how to handle this error when it comes about. Let's get to it!
The Technical Rundown
- All JavaScript error objects are descendants of the Error object, or an inherited object therein.
- The ReferenceError object is inherited from the Error object.
- The Assignment to Undeclared Variable error is a specific type of ReferenceError object.
When Should You Use It?
As mentioned in the introduction, an Assignment to Undeclared Variable error will occur when attempting to assign a value to a variable which has not previously been declared using the var keyword. However, beyond this cause, there is also one strict limitation within JavaScript itself, which can cause Assignment to Undeclared Variable errors to be suppressed in many circumstances: An Assignment to Undeclared Variable error will only fire when Strict Mode is active within the executing JavaScript.
When Strict Mode is activated, it is a way to programmatically opt in to a restricted variant of JavaScript that has intentionally different semantics and behaviors in a number of cases. While the entirety of Strict Mode is out of the scope of this article, for the purposes of analyzing errors like the Assignment to Undeclared Variable error, it's critical to understand that Strict Mode causes JavaScript to disallow the creation of accidental global variables.
For example, in normal JavaScript, the following code would be allowed, as the JavaScript engine assumes the intention is to create a new global variable, names, and assign it to the value of splitting the full name string of "Bob Smith" that was provided:
Sure enough, in normal JavaScript, this executes properly without any thrown errors and gives us the expected output:
Therefore, in order for JavaScript to assume it is not the intention of the developer and the code to automatically generate a new global variable anytime an assignment is made to a previously undeclared variable, Strict Mode must be enabled. This is done by including the line 'use strict'; somewhere in the code, preferably at the same scope as the checks for undeclared assignments.
In this example, we are expanding a bit on the concept above, by creating a simple getFirstName() function, which takes a single full_name parameter, enters into Strict Mode, splits the full_name, and returns the first value (i.e. the first name). By assigning Strict Mode within the function only, we ensure our strict behavior is only within the scope we want, rather than globally across our entire script:
var getFirstName = function(full_name) { // Setting strict mode 'use strict'; // Assigning to `names`, which is undefined names = full_name.split(' '); return names[0]; }
try { var first_name = getFirstName("Bob Smith"); console.log(`First name is ${first_name}.`) } catch (e) { if (e instanceof ReferenceError) { printError(e, true); } else { printError(e, false); } }
Now that Strict Mode is enabled, the line that worked previously and created a new names global variable now, instead, produces an Assignment to Undeclared Variable error as expected. The specific error message produced will differ slightly depending on the JavaScript engine, but the idea is the same:
// CHROME [EXPLICIT] ReferenceError: names is not defined
The obvious solution to our issue is to ensure that anytime a variable assignment is made, it must already be declared with proper scope, or we must always specify this new variable declaration with the var keyword preceding it:
var getFirstName = function(full_name) { // Setting strict mode 'use strict'; // Assigning to `names`, which is now defined var names = full_name.split(' '); return names[0]; }
With no Assignment to Undeclared Variable error produced, as expected, we get our first name output:
These built-in exception classes are helpful JavaScript error handling tools! Used with Airbrake’s JavaScript Error Handler your debugging process will be a breeze. Good luck!
Written By: Frances Banks
You may also like.
Jan 3, 2017 11:34:16 AM | JavaScript Error Handling - ReferenceError: "x" is not defined
Jan 26, 2017 6:00:03 am | javascript - referenceerror: invalid assignment left-hand side, may 13, 2017 8:00:02 am | javascript error handling - syntaxerror: redeclaration of formal parameter "x".
© Airbrake. All rights reserved. Terms of Service | Privacy Policy | DPA
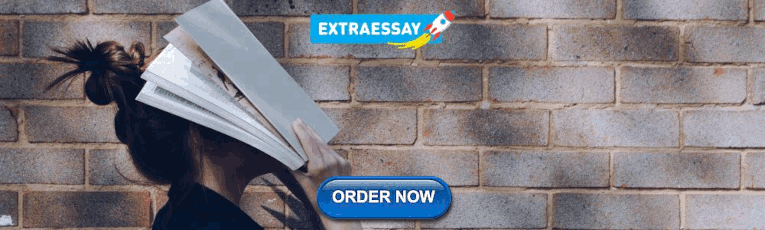
How to Fix the ‘ReferenceError: assignment to undeclared variable “x”‘ Error in Our JavaScript App?
- Post author By John Au-Yeung
- Post date August 20, 2021
- No Comments on How to Fix the ‘ReferenceError: assignment to undeclared variable “x”‘ Error in Our JavaScript App?
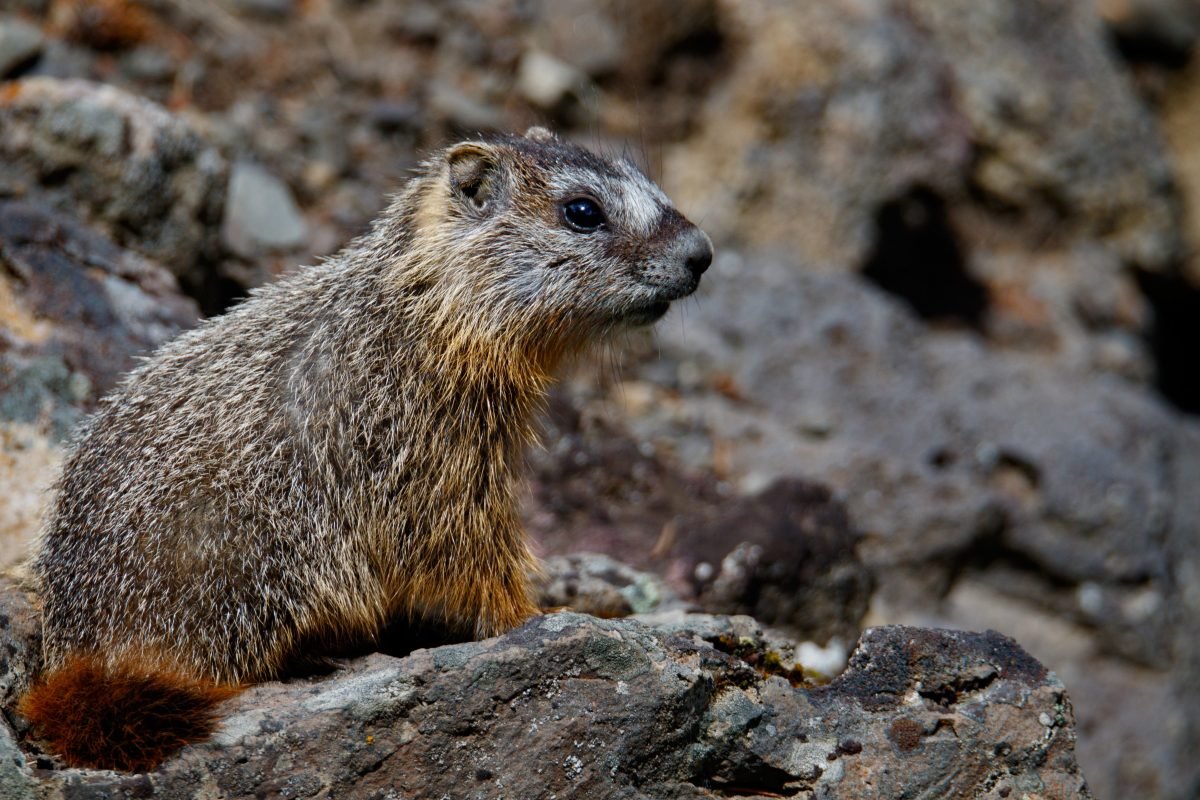
Sometimes, we may run into the ‘ReferenceError: assignment to undeclared variable "x"’ when we’re developing JavaScript apps.
In this article, we’ll look at how to fix the ‘ReferenceError: assignment to undeclared variable "x"’ when we’re developing JavaScript apps.
Fix the ‘ReferenceError: assignment to undeclared variable "x"’ When Developing JavaScript Apps
To fix the ‘ReferenceError: assignment to undeclared variable "x"’ when we’re developing JavaScript apps, we should make sure that we’re assigning values to variables that have already been declared.
The error message for this error is ReferenceError: "x" is not defined in Chrome.
And in Edge, the error message for this error is ReferenceError: Variable undefined in strict mode .
For instance, we shouldn’t write code like:
since bar hasn’t been declared in the foo function.
Instead, we should declare the bar variable by writing:
Now the error should be fixed.
Related Posts
Sometimes, we may run into the 'ReferenceError: "x" is not defined' when we're developing JavaScript…
It is easy to convert anything a boolean in JavaScript. Truthy values will be converted…
To throw errors in our JavaScript apps, we usually through an object that’s the instance…
By John Au-Yeung
Web developer specializing in React, Vue, and front end development.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Skip to main content
- Skip to search
- Skip to select language
- English (US)
ReferenceError: assignment to undeclared variable "x"
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add a let , const , or var keyword in front of it.
- Strict mode
ReferenceError: assignment to undeclared variable "x"
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
ReferenceError in strict mode only.
What went wrong?
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
Three things to note about declared and undeclared variables:
- Declared variables are constrained in the execution context in which they are declared. Undeclared variables are always global.
- Declared variables are created before any code is executed. Undeclared variables do not exist until the code assigning to them is executed.
- Declared variables are a non-configurable property of their execution context (function or global). Undeclared variables are configurable (e.g. can be deleted).
For more details and examples, see the var reference page.
Errors about undeclared variable assignments occur in strict mode code only. In non-strict code, they are silently ignored.
Invalid cases
In this case, the variable "bar" is an undeclared variable.
Valid cases
To make "bar" a declared variable, you can add a let , const , or var keyword in front of it.
- Strict mode
© 2005–2023 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Errors/Undeclared_var
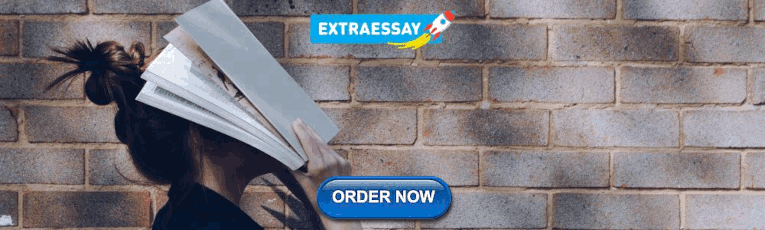
IMAGES
VIDEO
COMMENTS
The JavaScript strict mode-only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
The code never declares an i variable, but then tries to assign a value to it (in the initialization part of the for loop). Apparently you're using strict mode (good!), and so the engine is giving you an error rather than creating an implicit global. Declare i using var in the function, e.g.: function listinputs() {.
ReferenceError: Variable undefined in strict mode (Edge) Error Type: ReferenceError. Cause of the error: Somewhere in the code, there is an assignment without the var, let, or const keywords. When a value is assigned to an undeclared variable this error occurs.
A value has been assigned to an undeclared variable. In other words, there was an assignment without the var keyword. There are some differences between declared and undeclared variables, which might lead to unexpected results and that's why JavaScript presents an error in strict mode.
The Assignment to Undeclared Variable error crops up anytime code attempts to assign a value to a variable that has yet to be declared (via the var keyword).
ReferenceError. What went wrong? There is a non-existent variable referenced somewhere. This variable needs to be declared, or you need to make sure it is available in your current script or scope. Note: When loading a library (such as jQuery), make sure it is loaded before you access library variables, such as "$".
When any value is assigned to undeclared variable or assignment without the var keyword or variable is not in your current scope, it might lead to unexpected results and that’s why JavaScript presents a ReferenceError: assignment to undeclared variable "x" in strict mode.
To fix the ‘ReferenceError: assignment to undeclared variable "x"’ when we’re developing JavaScript apps, we should make sure that we’re assigning values to variables that have already been declared. The error message for this error is ReferenceError: "x" is not defined in Chrome.
The JavaScript strict mode-only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.
The JavaScript strict mode -only exception "Assignment to undeclared variable" occurs when the value has been assigned to an undeclared variable.