- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
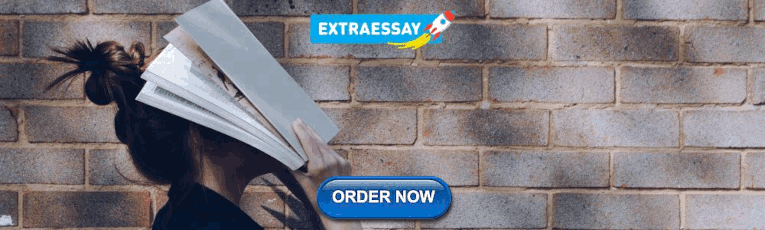
Nullish coalescing assignment (??=)
The nullish coalescing assignment ( ??= ) operator, also known as the logical nullish assignment operator, only evaluates the right operand and assigns to the left if the left operand is nullish ( null or undefined ).
Description
Nullish coalescing assignment short-circuits , meaning that x ??= y is equivalent to x ?? (x = y) , except that the expression x is only evaluated once.
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
Neither would the following trigger the setter:
In fact, if x is not nullish, y is not evaluated at all.
Using nullish coalescing assignment
You can use the nullish coalescing assignment operator to apply default values to object properties. Compared to using destructuring and default values , ??= also applies the default value if the property has value null .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Nullish coalescing operator ( ?? )

Nullish Coalescing: The ?? Operator in TypeScript
TypeScript 3.7 added support for the ?? operator, which is known as the nullish coalescing operator . We can use this operator to provide a fallback value for a value that might be null or undefined .
# Truthy and Falsy Values in JavaScript
Before we dive into the ?? operator, let's recall that JavaScript values can either be truthy or falsy : when coerced to a Boolean, a value can either produce the value true or false . In JavaScript, the following values are considered to be falsy:
All other JavaScript values will produce the value true when coerced to a Boolean and are thus considered truthy.
# Providing Fallback Values with the ?? Operator
The ?? operator can be used to provide a fallback value in case another value is null or undefined . It takes two operands and is written like this:
If the left operand is null or undefined , the ?? expression evaluates to the right operand:
Otherwise, the ?? expression evaluates to the left operand:
Notice that all left operands above are falsy values. If we had used the || operator instead of the ?? operator, all of these expressions would've evaluated to their respective right operands:
This behavior is why you shouldn't use the || operator to provide a fallback value for a nullable value. For falsy values, the result might not be the one you wanted or expected. Consider this example:
The expression options.prettyPrint ?? true lets us provide the default value true in case that the prettyPrint property contains the value null or undefined . If prettyPrint contains the value false , the expression false ?? true still evaluates to false , which is exactly the behavior we want here.
Note that using the || operator here would lead to incorrect results. options.prettyPrint || true would evaluate to true for the values null and undefined , but also for the value false . This would clearly not be intended. I've seen this happen in practice a handful of times, so make sure to keep this case in mind and use towards the ?? operator instead.
# Compiled Output: ES2020 and Newer
The nullish coalescing operator has reached Stage 4 ("Finished") of the TC39 process and is now officially part of ES2020 . Therefore, the TypeScript compiler will emit the ?? operator as is without any downleveling when you're targeting "ES2020" (or a newer language version) or "ESNext" in your tsconfig.json file:
So, this simple expression will be emitted unchanged:
If you're planning on using the ?? operator while targeting "ES2020" or a newer language version, head over to caniuse.com and node.green and make sure that all the JavaScript engines you need to support have implemented the operator.
# Compiled JavaScript Output: ES2019 and Older
If you're targeting "ES2019" or an older language version in your tsconfig.json file, the TypeScript compiler will rewrite the nullish coalescing operator into a conditional expression. That way, we can start using the ?? operator in our code today and still have the compiled code successfully parse and execute in older JavaScript engines.
Let's look at the same simple ?? expression again:
Assuming we're targeting "ES2019" or a lower language version, the TypeScript compiler will emit the following JavaScript code:
The value variable is compared against both null and undefined (the result of the expression void 0 ). If both comparisons produce the value false , the entire expression evaluates to value ; otherwise, it evaluates to fallbackValue .
Now, let's look at a slightly more complex example. Instead of a simple value variable, we're going to use a getValue() call expression as the left operand of the ?? operator:
In this case, the compiler will emit the following JavaScript code (modulo whitespace differences):
You can see that the compiler generated an intermediate variable _a to store the return value of the getValue() call. The _a variable is then compared against null and void 0 and (potentially) used as the resulting value of the entire expression. This intermediate variable is necessary so that the getValue function is only called once.
# Compiled Output: Checking for null and undefined
You might be wondering why the compiler emits the following expression to check the value variable against null and undefined :
Couldn't the compiler emit the following shorter check instead?
Unfortunately, it can't do that without sacrificing correctness. For almost all values in JavaScript, the comparison value == null is equivalent to value === null || value === undefined . For those values, the negation value != null is equivalent to value !== null && value !== undefined . However, there is one value for which these two checks aren't equivalent, and that value is document.all :
The value document.all is not considered to be strictly equal to either null or undefined , but it is considered to be loosely equal to both null and undefined . Because of this anomaly, the TypeScript compiler can't emit value != null as a check because it would produce incorrect results for document.all .
You can read more about this curious behavior in an answer to the Why is document.all falsy? question on Stack Overflow. Oh, the things we do for web compatibility.
This article and 44 others are part of the TypeScript Evolution series. Have a look!
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
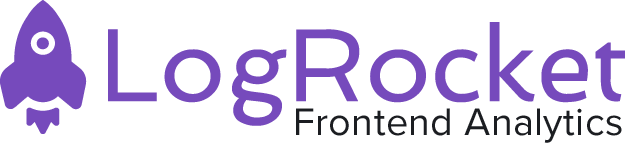
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Optional chaining and nullish coalescing in TypeScript

In TypeScript, optional chaining is defined as the ability to immediately stop running an expression if a part of it evaluates to either null or undefined . It was introduced in TypeScript 3.7 with the ?. operator.
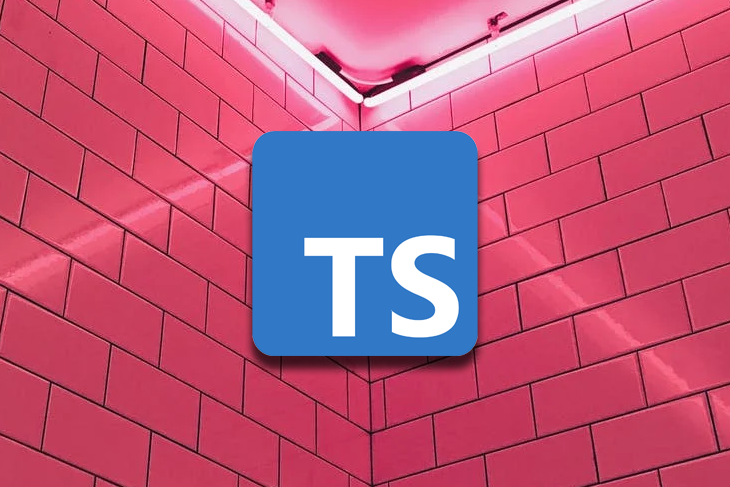
Optional chaining is often used together with nullish coalescing, which is the ability to fall back to a default value when the primary expression evaluates to null or undefined . In this case, the operator to be used is ?? .
In this article, we’re going to explore the way we can use those two operators, comparing them with the legacy TypeScript 3.6 code.
Table of contents:
null and undefined in the type system
Explicit checking for null and undefined, optional property access and optional call, optional element access, a note on short-circuiting, nullish coalescing, conclusions.
TypeScript’s type system defines two very special types, null and undefined , to represent the values null and undefined , respectively. However, by default, TypeScript’s type checker considers null and undefined as legal values for any type. For instance, we can assign null to a value of type string :
However, if we enable the strictNullChecks flag, null and undefined types are taken into account during type checks. In this case, the snippet above won’t type check anymore and we’ll receive the following error message: “Type null is not assignable to type string .”
The types null and undefined are treated differently to match the same semantics used by JavaScript: string | null is a different type than string | undefined , which is, in turn, different than string | null | undefined . For example, the following expression will not type check, and the error message will be “Type undefined is not assignable to type string | null .”:
Until version 3.7, TypeScript did not contain any operators to help us deal with null or undefined values. Hence, before accessing a variable that could assume them, we had to add explicit checks:
We first declare a new type, nullableUndefinedString , which represents a string that might also be null or undefined . Then, we implement a function that returns the same string passed in as a parameter, if it’s defined, and the string "default" otherwise.
This approach works just fine, but it leads to complex code. Also, it’s difficult to concatenate calls where different parts of the call chain might be either null or undefined :
In the example above, we defined a class Person using the type nullableUndefinedString we previously introduced. Then, we implemented a method to return the full name of the person, in uppercase. As the field we access might be null or undefined , we have to continuously check for the actual value. For example, in Person::getUppercaseFullName() we return undefined if the full name is not defined.
This way of implementing things is quite cumbersome and difficult to both read and maintain. Hence, since version 3.7, TypeScript has introduced optional chaining and nullish coalescing.
Optional chaining
As we saw in the introduction, the core of optional chaining is the ?. operator, allowing us to stop running expressions when the runtime encounters a null or undefined . In this section we’ll see three possible uses of such an operator.
Using ?. , we can rewrite Person::getUppercaseFullName() in a much simpler way:
The implementation of Person::getUppercaseFullName() is now a one-liner. If this.fullName is defined, then this.fullName.toUpperCase() will be computed. Otherwise, undefined will be returned, as before.
Still, the expression returned by the first invocation of ?. might be null or undefined . Hence, the optional property access operator can be chained:
In the example above, we first created an array, people , containing two objects of type Person . Then, using Array::find() , we search for a person whose uppercase name is SOMEONE ELSE . As Array.find() returns undefined if no element in the array satisfies the constraint, we have to account for that when printing the length of the name. In the example, we did that by chaining ?. calls.
Furthermore, r?.getUppercaseFullName() represents the second use of ?. , optional call. This way, we can conditionally call expressions if they are not null or undefined .
If we didn’t use ?. , we would have to use a more complex if statement:
Nonetheless, there’s an important difference between the if and the chain of ?. calls. The former is short-circuited while the latter is not. This is intentional, as the new operator does not short-circuit on valid data, such as 0 or empty strings.
The last use of ?. is optional element access, allowing us to access non-identifier properties, if defined:
In the example above we wrote a function that returns the first element of a list (assuming the list is modeled as an array) if defined, undefined otherwise. list is represented as an optional parameter, via the question mark after its name. Hence, its type is T[] | undefined .
The two calls of head will print, respectively, 1 and undefined .
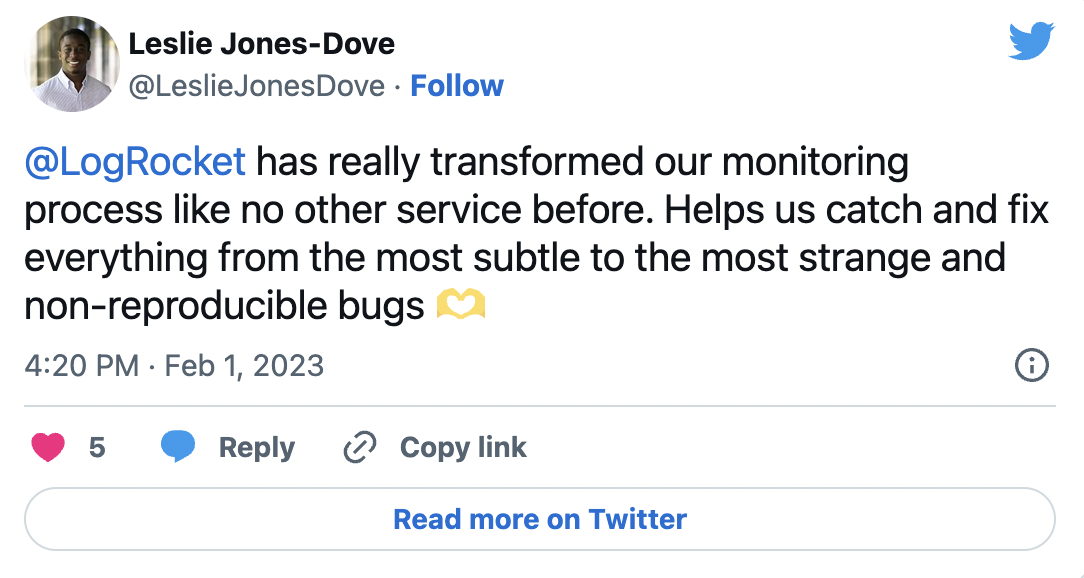
Over 200k developers use LogRocket to create better digital experiences

When optional chaining is involved in larger expressions, the short-circuiting it provides does not expand further than the three cases we saw above:
The example above shows a function computing the percentage on some numeric field. Nonetheless, as foo?.bar might be undefined , we might end up dividing undefined by 100. This is why, with strictNullChecks enabled, the expression above does not type check: “Object is possibly undefined.”
Nullish coalescing allows us to specify a kind of a default value to be used in place of another expression, which is evaluated to null or undefined . Strictly speaking, the expression let x = foo ?? bar(); is the same as let x = foo !== null && foo !== undefined ? foo : bar(); . Using it, as well as optional property access, we can rewrite Person::getFullNameLength() as follows:
This new version is much more readable than before. Similarly to ?. , ?? only operates on null and undefined . Hence, for instance, if this.fullName was an empty string, the returned value would be 0 , not -1 .
In this article, we analyzed two features added in TypeScript 3.7: optional chaining and nullish coalescing. We saw how we can use both of them together to write simple and readable code, without bothering with never-ending conditionals. We also compared them with the code we could write in TypeScript 3.6, which is much more verbose.
In any case, it is always recommended to enable strictNullChecks . Such a flag comes with a number of additional type checks, which help us write sounder and safer code, by construction.
LogRocket : Full visibility into your web and mobile apps
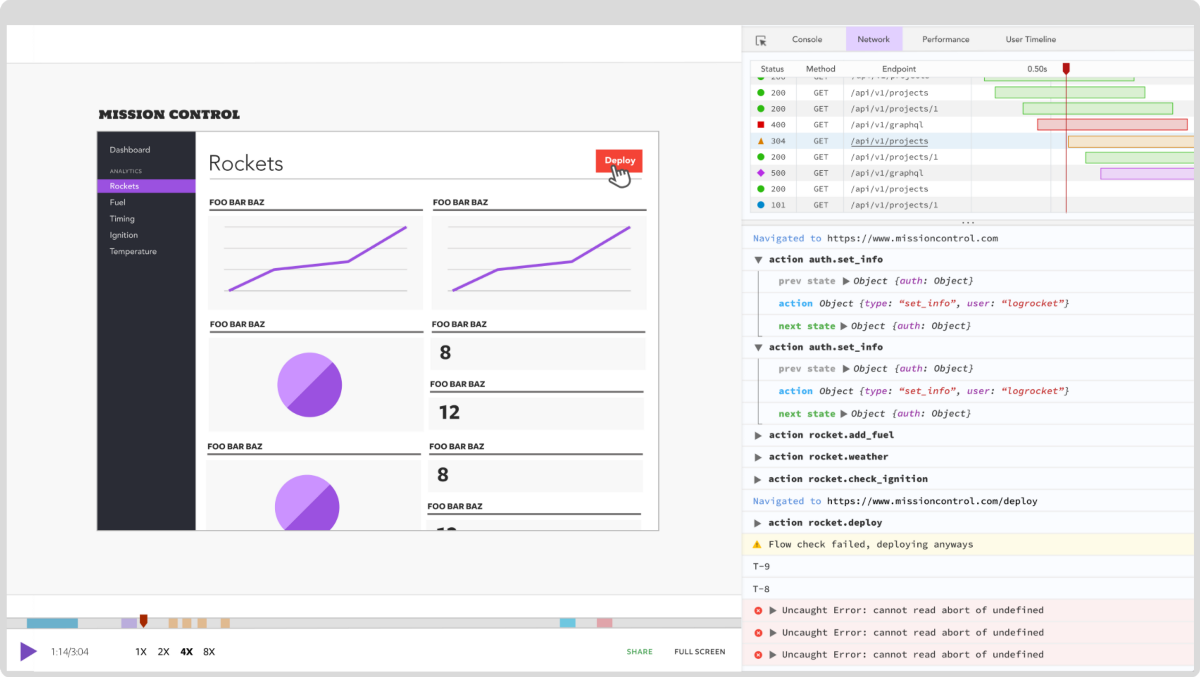
LogRocket is a frontend application monitoring solution that lets you replay problems as if they happened in your own browser. Instead of guessing why errors happen or asking users for screenshots and log dumps, LogRocket lets you replay the session to quickly understand what went wrong. It works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store.
In addition to logging Redux actions and state, LogRocket records console logs, JavaScript errors, stacktraces, network requests/responses with headers + bodies, browser metadata, and custom logs. It also instruments the DOM to record the HTML and CSS on the page, recreating pixel-perfect videos of even the most complex single-page and mobile apps.
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #typescript
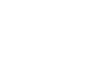
Stop guessing about your digital experience with LogRocket
Recent posts:.
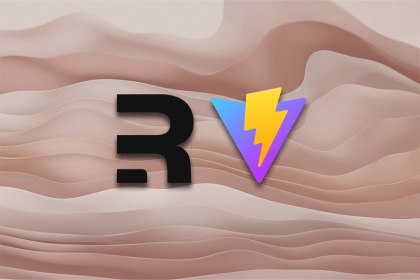
Exploring Remix Vite support and other v2.20 and v7 changes
The Remix Vite integration helps speeds up development via features like HDR and HMR. Read about Remix Vite and other breaking changes.
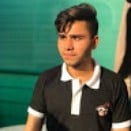
Caching in Next.js with unstable_cache
In this article, we explore the different caching strategies in Next.js, focusing on the `unstable_cache` function.
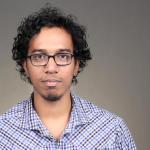
ReScript vs. TypeScript: Overview and comparison
Many modern web developers are embracing emerging technologies that improve the speed of development as well as the quality of […]
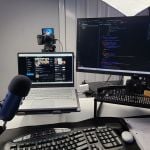
Using Pavex for Rust web development
The Pavex Rust web framework is an exciting project that provides high performance, great usability, and speed.
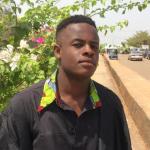
2 Replies to "Optional chaining and nullish coalescing in TypeScript"
I think this article could potentially “misteach” people to create types like `nullableUndefinedString` when you could mostly use `argOrProp?: string | null`.
Also, there’s no need to use strict types checks for null and undefined when you could check for both like `value == null`.
Regarding nullableUndefinedString, you’re right and, as a matter of fact, it was just an easy way to define a single type used throughout the entire article without repeating it meaning every time.
strictNullChecks, on the other hand, is recommended by the documentation itself. Hence, even if there are other ways in the language to achieve the same result, to me, the pros of that flag outweigh the cons.
Leave a Reply Cancel reply
Demystifying TypeScript's Double Question Mark: Nullish Coalescing
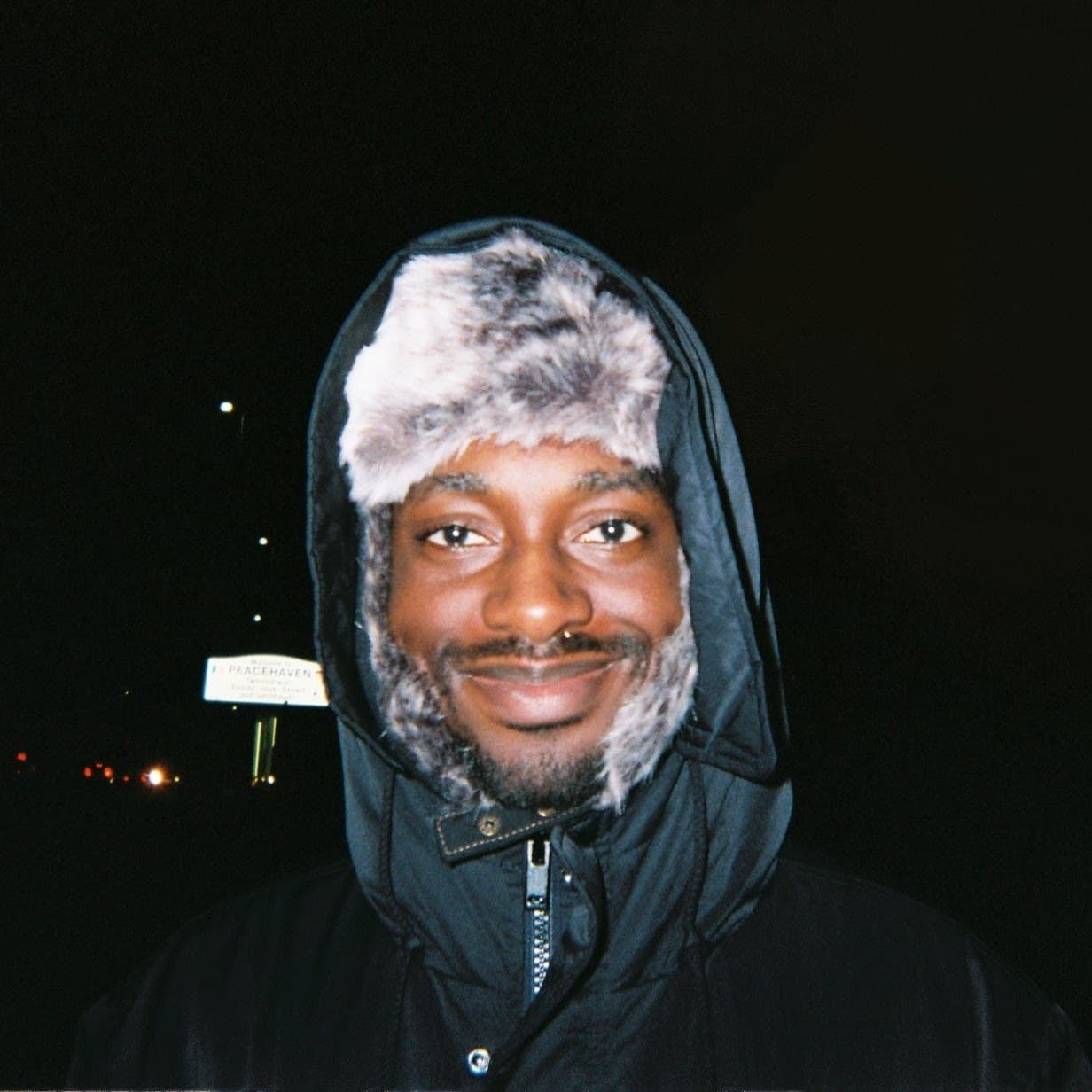
In your TypeScript travels, you might have come across many strange, possibly lesser-used operators. One of these is TypeScript's double question mark. In this article I'll explore what that operator does, why to use it, and when.
Nullish Coalescing Operator .
The double question marks you're seeing are the nullish coalescing operator - a logical operator that returns the right operand when the operand is null or undefined (hence the null-ish), and returns the left operand in other cases.
It looks a little like this:
If "left" is null or undefined, then the result will be whatever is in "right".
You can also chain as many nullish coalescing operators as you like:
Example - Environment Variables .
A common use-case for these double question marks is assigning default values to variables - in particular variables coming from some external source, such as environment variables.
Imagine some code like this:
A simple API call to fetch some users. If you're running this imaginary program locally on your machine, you might feel confident that those environment variables will always exist, but TypeScript won't.
Type-safety aside, if we could have a default value for these - that avoids having to provide some of the environment variables altogether, unless you want to override them.
This is where the nullish coalescing operator, those two question marks you've been seeing in TypeScript, come in handy:
With one operator, we've given a default value to "API_URL" and "API_USERS_PATH" - pretty handy! You might not want to store a secret API token in code though, so sometimes a default value isn't the best choice, so I've chosen to throw an error if there is no token instead.
The OR Operator .
Let's think through the logic of the nullish coalescing operator. We pick the left side if it isn't null or undefined, otherwise we pick the right-hand side.
If we take a look at the result through a table:
It looks pretty similar to another operator you might be familiar with. We can just change out those double question marks for double vertical bars:
The nullish coalescing operator is nearly identical to the logical OR operator, but "||" returns the right-hand side if the value is any falsy value, not just null or undefined. For a lot of cases this means the difference doesn't matter. One of the cases you do need to watch for are the number 0, which is falsy:
In this scenario if the player has no lives left, 0 is falsy, so it will resolve to the value on the right.
The other case is empty strings:
In this function, if you try to join an array with an empty string then that value is falsy, so the result will be the default join character.
The main thing here is to be aware of when the operators are different, and make sure to know if you want to handle values that are just null and undefined, or all falsy values.
Nullish coalescing assignment .
As well as the null coalescing operator, you might also find the less commonly used Nullish coalescing assignment operator used in TypeScript - double question marks followed by equals. As the name implies, it's nullish coalescing combined with assignment:
In the example above, "var1" is null. The first nullish coalescing assignment does store the string in "var1", since "var1" is null. Then the next assignment is skipped, since "var1" is not null.
This can be used to assign a value only if the variable is null. It’s less commonly seen, but you might still run into it.
TypeScript .
The nullish coalescing operator is actually not unique to TypeScript. The double question marks come from JavaScript, but it has some implications for TypeScript. The main addition is type narrowing. Let's take a function that can return a nullish value:
The return type for this is "foo" | null. If you want to use that value somewhere, usually you'd need to check if the return type is null first:
Or you can use the nullish coalescing operator to remove null from the type union:
Conclusion .
Thanks for reading! Hopefully, with this article, you now know what those double question marks mean in TypeScript, and you're also able to use the nullish coalescing operator in your own code. It's a very useful tool for creating more concise, safer TypeScript code, and dealing with potential null or undefined values in a quick and easy way. For a quick summary of everything:
- Double question marks in TypeScript means the nullish coalescing operator. If the left-hand side operand is undefined or null, it returns the left-hand side, otherwise it returns the right-hand side operand
- It's identical to the logical OR operator, but the logical OR operator handles all falsy values, not just null or undefined
- The main case where this matters is for 0 or empty strings, which are falsy
- Use the operand when you want to check for null or undefined and provide default values in those cases
Thanks for reading! If you liked this article, feel free to share it!
read more .
- How to Create Objects with Dynamic Keys in TypeScript
- ECMAScript vs JavaScript vs TypeScript
- A Comprehensive Guide to the typeOf Operator in Typescript
- How to Detect Null and Undefined in Your TypeScript Code
- TypeScript Enums Made Simple
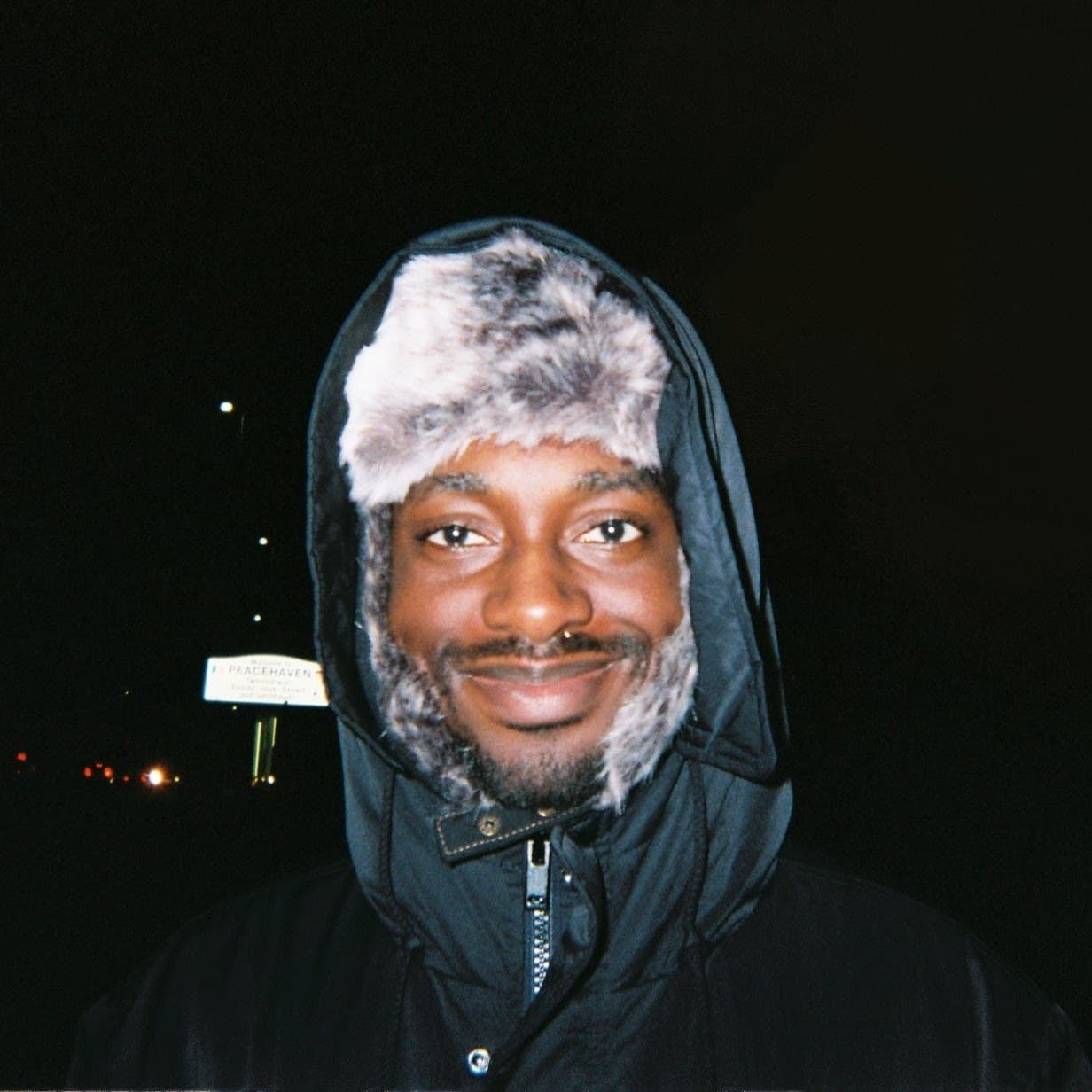
I'm a full-stack developer from the UK. I'm currently looking for graduate and freelance software engineering roles, so if you liked this article, feel free to reach out.
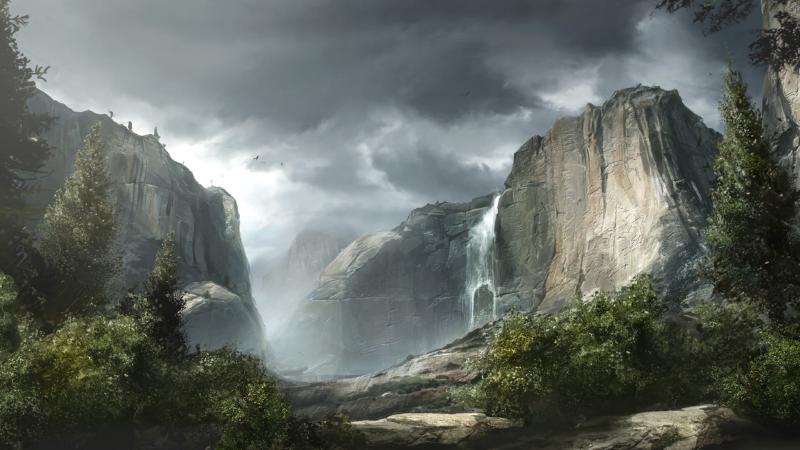
Nullish Coalescing: The ?? Operator in TypeScript
TypeScript 3.7 added support for the ?? operator, which is known as the nullish coalescing operator . We can use this operator to provide a fallback value for a value that might be null or undefined .
Truthy and Falsy Values in JavaScript
Before we dive into the ?? operator, let’s recall { 回想 } that JavaScript values can either be truthy or falsy : when coerced { 强制,强迫 } to a Boolean, a value can either produce the value true or false . In JavaScript, the following values are considered to be falsy:
- ""
All other JavaScript values will produce the value true when coerced to a Boolean and are thus considered truthy.
Providing Fallback Values with the ?? Operator
The ?? operator can be used to provide a fallback value in case another value is null or undefined . It takes two operands and is written like this:
If the left operand is null or undefined , the ?? expression evaluates to the right operand:
Otherwise, the ?? expression evaluates to the left operand:
Notice that all left operands above are falsy values. If we had used the || operator instead of the ?? operator, all of these expressions would’ve evaluated to their respective right operands:
This behavior is why you shouldn’t use the || operator to provide a fallback value for a nullable value . For falsy values, the result might not be the one you wanted or expected. Consider this example:
The expression options.prettyPrint ?? true lets us provide the default value true in case that the prettyPrint property contains the value null or undefined . If prettyPrint contains the value false , the expression false ?? true still evaluates to false , which is exactly the behavior we want here.
Note that using the || operator here would lead to incorrect results. options.prettyPrint || true would evaluate to true for the values null and undefined , but also for the value false . This would clearly not be intended. I’ve seen this happen in practice a handful of { 一把;少量的 } times, so make sure to keep this case in mind and use towards the ?? operator instead.
Compiled Output: ES2020 and Newer
The nullish coalescing operator has reached Stage 4 (“Finished”) of the TC39 process and is now officially part of ES2020 . Therefore, the TypeScript compiler will emit the ?? operator as is without any downleveling when you’re targeting "ES2020" (or a newer language version) or "ESNext" in your tsconfig.json file:
So, this simple expression will be emitted unchanged:
If you’re planning on using the ?? operator while targeting "ES2020" or a newer language version, head over to caniuse.com and node.green and make sure that all the JavaScript engines you need to support have implemented the operator.
Compiled JavaScript Output: ES2019 and Older
If you’re targeting "ES2019" or an older language version in your tsconfig.json file, the TypeScript compiler will rewrite the nullish coalescing operator into a conditional expression. That way, we can start using the ?? operator in our code today and still have the compiled code successfully parse and execute in older JavaScript engines.
Let’s look at the same simple ?? expression again:
Assuming we’re targeting "ES2019" or a lower language version, the TypeScript compiler will emit the following JavaScript code:
The value variable is compared against both null and undefined ( the result of the expression void 0 ). If both comparisons produce the value false , the entire expression evaluates to value ; otherwise, it evaluates to fallbackValue .
Now, let’s look at a slightly more complex example. Instead of a simple value variable, we’re going to use a getValue() call expression as the left operand of the ?? operator:
In this case, the compiler will emit the following JavaScript code (modulo whitespace differences):
You can see that the compiler generated an intermediate variable _a to store the return value of the getValue() call. The _a variable is then compared against null and void 0 and (potentially) used as the resulting value of the entire expression. This intermediate variable is necessary so that the getValue function is only called once.
Compiled Output: Checking for null and undefined
You might be wondering why the compiler emits the following expression to check the value variable against null and undefined :
Couldn’t the compiler emit the following shorter check instead?
Unfortunately, it can’t do that without sacrificing { 牺牲 } correctness. For almost all values in JavaScript, the comparison value == null is equivalent to value === null || value === undefined . For those values, the negation value != null is equivalent to value !== null && value !== undefined . However, there is one value for which these two checks aren’t equivalent, and that value is document.all :
The value document.all is not considered to be strictly equal to either null or undefined , but it is considered to be loosely equal to both null and undefined . Because of this anomaly { 异常事物,反常现象 } , the TypeScript compiler can’t emit value != null as a check because it would produce incorrect results for document.all .
You can read more about this curious behavior in an answer to the Why is document.all falsy? question on Stack Overflow. Oh, the things we do for web compatibility.
- Nullish Coalescing The Operator in TypeScript — Marius Schulz
Emerson Souza's Blog
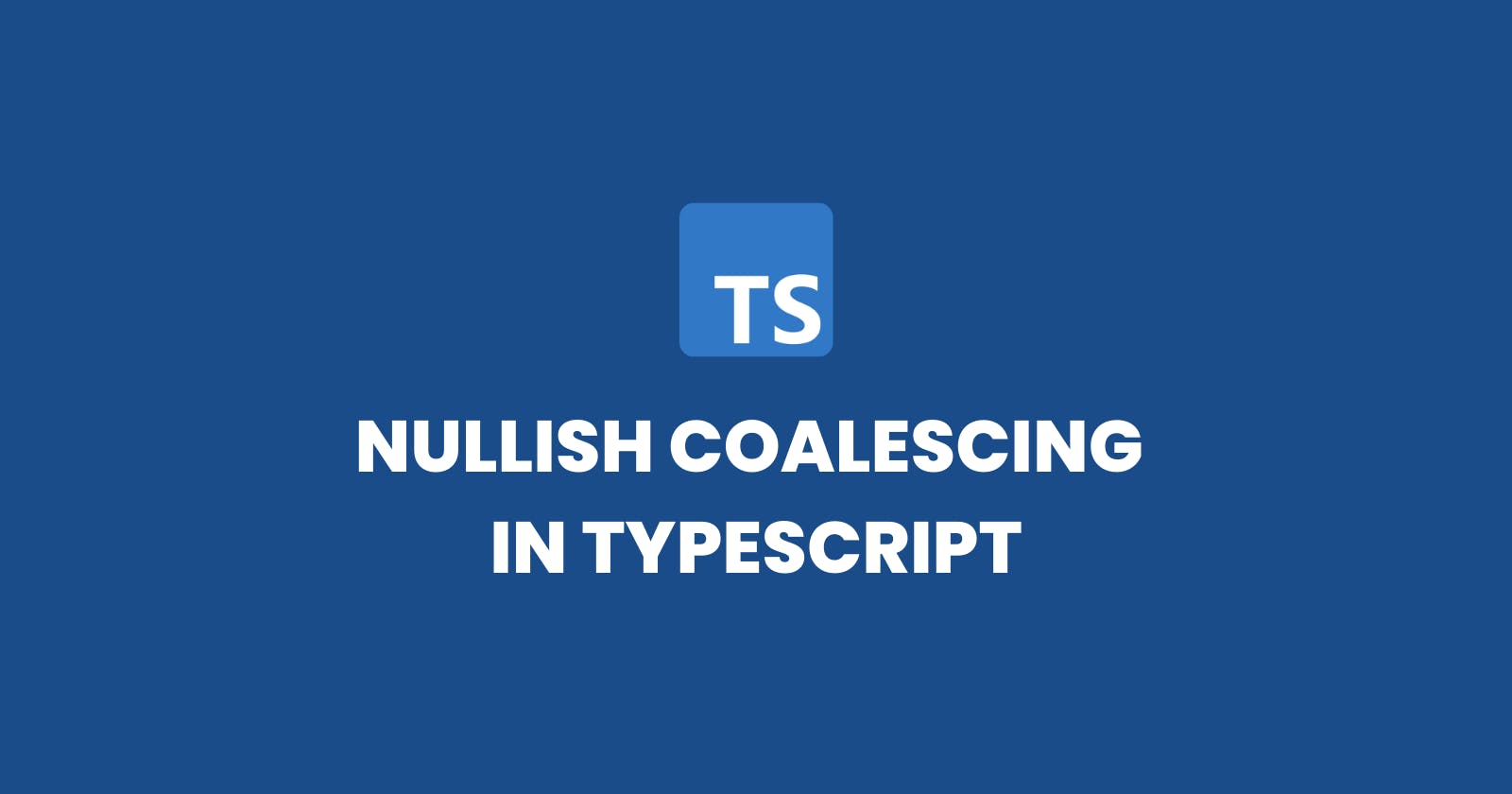
Streamlining Nullish Value Handling in TypeScript
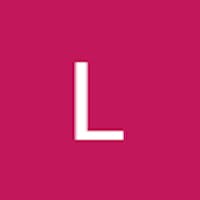
The Nullish Coalescing Operator (??) is a feature introduced in TypeScript to handle the case of null or undefined values more effectively. It provides a concise way to check if a value is nullish and fallback to a default value if it is.
Consider a scenario where you have an API response that may contain a nullable property. Traditionally, you would use conditional statements or the logical OR operator (||) to assign a default value when the property is null or undefined. However, this approach falls short when the property has a falsy value like an empty string or zero, as these values would be treated as nullish and the default value would be assigned incorrectly.
With the Nullish Coalescing Operator, you can succinctly handle this scenario. Let's say you have an API response object with a nullable name property. Instead of using conditional statements, you can use the nullish coalescing operator to assign a default name when it is null or undefined:
In this example, if apiResponse.name is null or undefined, the name variable will be assigned the value "Anonymous". If apiResponse.name has any other falsy value, the default value will not be applied.
The Nullish Coalescing Operator is a powerful addition to TypeScript, providing a concise and reliable way to handle nullish values. It simplifies the code and avoids unexpected behavior caused by falsy values. By leveraging this operator, you can write cleaner and more robust code.
TypeScript 3.7
Optional chaining.
Optional chaining is issue #16 on our issue tracker. For context, there have been over 23,000 issues on the TypeScript issue tracker since then.
At its core, optional chaining lets us write code where TypeScript can immediately stop running some expressions if we run into a null or undefined . The star of the show in optional chaining is the new ?. operator for optional property accesses . When we write code like
this is a way of saying that when foo is defined, foo.bar.baz() will be computed; but when foo is null or undefined , stop what we’re doing and just return undefined .”
More plainly, that code snippet is the same as writing the following.
Note that if bar is null or undefined , our code will still hit an error accessing baz . Likewise, if baz is null or undefined , we’ll hit an error at the call site. ?. only checks for whether the value on the left of it is null or undefined - not any of the subsequent properties.
You might find yourself using ?. to replace a lot of code that performs repetitive nullish checks using the && operator.
Keep in mind that ?. acts differently than those && operations since && will act specially on “falsy” values (e.g. the empty string, 0 , NaN , and, well, false ), but this is an intentional feature of the construct. It doesn’t short-circuit on valid data like 0 or empty strings.
Optional chaining also includes two other operations. First there’s the optional element access which acts similarly to optional property accesses, but allows us to access non-identifier properties (e.g. arbitrary strings, numbers, and symbols):
There’s also optional call , which allows us to conditionally call expressions if they’re not null or undefined .
The “short-circuiting” behavior that optional chains have is limited property accesses, calls, element accesses - it doesn’t expand any further out from these expressions. In other words,
doesn’t stop the division or someComputation() call from occurring. It’s equivalent to
That might result in dividing undefined , which is why in strictNullChecks , the following is an error.
More more details, you can read up on the proposal and view the original pull request .
Nullish Coalescing
The nullish coalescing operator is another upcoming ECMAScript feature that goes hand-in-hand with optional chaining, and which our team has been involved with championing in TC39.
You can think of this feature - the ?? operator - as a way to “fall back” to a default value when dealing with null or undefined . When we write code like
this is a new way to say that the value foo will be used when it’s “present”; but when it’s null or undefined , calculate bar() in its place.
Again, the above code is equivalent to the following.
The ?? operator can replace uses of || when trying to use a default value. For example, the following code snippet tries to fetch the volume that was last saved in localStorage (if it ever was); however, it has a bug because it uses || .
When localStorage.volume is set to 0 , the page will set the volume to 0.5 which is unintended. ?? avoids some unintended behavior from 0 , NaN and "" being treated as falsy values.
We owe a large thanks to community members Wenlu Wang and Titian Cernicova Dragomir for implementing this feature! For more details, check out their pull request and the nullish coalescing proposal repository .
Assertion Functions
There’s a specific set of functions that throw an error if something unexpected happened. They’re called “assertion” functions. As an example, Node.js has a dedicated function for this called assert .
In this example if someValue isn’t equal to 42 , then assert will throw an AssertionError .
Assertions in JavaScript are often used to guard against improper types being passed in. For example,
Unfortunately in TypeScript these checks could never be properly encoded. For loosely-typed code this meant TypeScript was checking less, and for slightly conservative code it often forced users to use type assertions.
The alternative was to instead rewrite the code so that the language could analyze it, but this isn’t convenient.
Ultimately the goal of TypeScript is to type existing JavaScript constructs in the least disruptive way. For that reason, TypeScript 3.7 introduces a new concept called “assertion signatures” which model these assertion functions.
The first type of assertion signature models the way that Node’s assert function works. It ensures that whatever condition is being checked must be true for the remainder of the containing scope.
asserts condition says that whatever gets passed into the condition parameter must be true if the assert returns (because otherwise it would throw an error). That means that for the rest of the scope, that condition must be truthy. As an example, using this assertion function means we do catch our original yell example.
The other type of assertion signature doesn’t check for a condition, but instead tells TypeScript that a specific variable or property has a different type.
Here asserts val is string ensures that after any call to assertIsString , any variable passed in will be known to be a string .
These assertion signatures are very similar to writing type predicate signatures:
And just like type predicate signatures, these assertion signatures are incredibly expressive. We can express some fairly sophisticated ideas with these.
To read up more about assertion signatures, check out the original pull request .
Better Support for never -Returning Functions
As part of the work for assertion signatures, TypeScript needed to encode more about where and which functions were being called. This gave us the opportunity to expand support for another class of functions: functions that return never .
The intent of any function that returns never is that it never returns. It indicates that an exception was thrown, a halting error condition occurred, or that the program exited. For example, process.exit(...) in @types/node is specified to return never .
In order to ensure that a function never potentially returned undefined or effectively returned from all code paths, TypeScript needed some syntactic signal - either a return or throw at the end of a function. So users found themselves return -ing their failure functions.
Now when these never -returning functions are called, TypeScript recognizes that they affect the control flow graph and accounts for them.
As with assertion functions, you can read up more at the same pull request .
(More) Recursive Type Aliases
Type aliases have always had a limitation in how they could be “recursively” referenced. The reason is that any use of a type alias needs to be able to substitute itself with whatever it aliases. In some cases, that’s not possible, so the compiler rejects certain recursive aliases like the following:
This is a reasonable restriction because any use of Foo would need to be replaced with Foo which would need to be replaced with Foo which would need to be replaced with Foo which… well, hopefully you get the idea! In the end, there isn’t a type that makes sense in place of Foo .
This is fairly consistent with how other languages treat type aliases , but it does give rise to some slightly surprising scenarios for how users leverage the feature. For example, in TypeScript 3.6 and prior, the following causes an error.
This is strange because there is technically nothing wrong with any use users could always write what was effectively the same code by introducing an interface.
Because interfaces (and other object types) introduce a level of indirection and their full structure doesn’t need to be eagerly built out, TypeScript has no problem working with this structure.
But workaround of introducing the interface wasn’t intuitive for users. And in principle there really wasn’t anything wrong with the original version of ValueOrArray that used Array directly. If the compiler was a little bit “lazier” and only calculated the type arguments to Array when necessary, then TypeScript could express these correctly.
That’s exactly what TypeScript 3.7 introduces. At the “top level” of a type alias, TypeScript will defer resolving type arguments to permit these patterns.
This means that code like the following that was trying to represent JSON…
can finally be rewritten without helper interfaces.
This new relaxation also lets us recursively reference type aliases in tuples as well. The following code which used to error is now valid TypeScript code.
For more information, you can read up on the original pull request .
--declaration and --allowJs
The declaration flag in TypeScript allows us to generate .d.ts files (declaration files) from TypeScript source files (i.e. .ts and .tsx files). These .d.ts files are important for a couple of reasons.
First of all, they’re important because they allow TypeScript to type-check against other projects without re-checking the original source code. They’re also important because they allow TypeScript to interoperate with existing JavaScript libraries that weren’t built with TypeScript in mind. Finally, a benefit that is often underappreciated: both TypeScript and JavaScript users can benefit from these files when using editors powered by TypeScript to get things like better auto-completion.
Unfortunately, declaration didn’t work with the allowJs flag which allows mixing TypeScript and JavaScript input files. This was a frustrating limitation because it meant users couldn’t use the declaration flag when migrating codebases, even if they were JSDoc-annotated. TypeScript 3.7 changes that, and allows the two options to be used together!
The most impactful outcome of this feature might a bit subtle: with TypeScript 3.7, users can write libraries in JSDoc annotated JavaScript and support TypeScript users.
The way that this works is that when using allowJs , TypeScript has some best-effort analyses to understand common JavaScript patterns; however, the way that some patterns are expressed in JavaScript don’t necessarily look like their equivalents in TypeScript. When declaration emit is turned on, TypeScript figures out the best way to transform JSDoc comments and CommonJS exports into valid type declarations and the like in the output .d.ts files.
As an example, the following code snippet
Will produce a .d.ts file like
This can go beyond basic functions with @param tags too, where the following example:
will be transformed into the following .d.ts file:
Note that when using these flags together, TypeScript doesn’t necessarily have to downlevel .js files. If you simply want TypeScript to create .d.ts files, you can use the emitDeclarationOnly compiler option.
For more details, you can check out the original pull request .
The useDefineForClassFields Flag and The declare Property Modifier
Back when TypeScript implemented public class fields, we assumed to the best of our abilities that the following code
would be equivalent to a similar assignment within a constructor body.
Unfortunately, while this seemed to be the direction that the proposal moved towards in its earlier days, there is an extremely strong chance that public class fields will be standardized differently. Instead, the original code sample might need to de-sugar to something closer to the following:
While TypeScript 3.7 isn’t changing any existing emit by default, we’ve been rolling out changes incrementally to help users mitigate potential future breakage. We’ve provided a new flag called useDefineForClassFields to enable this emit mode with some new checking logic.
The two biggest changes are the following:
- Declarations are initialized with Object.defineProperty .
- Declarations are always initialized to undefined , even if they have no initializer.
This can cause quite a bit of fallout for existing code that use inheritance. First of all, set accessors from base classes won’t get triggered - they’ll be completely overwritten.
Secondly, using class fields to specialize properties from base classes also won’t work.
What these two boil down to is that mixing properties with accessors is going to cause issues, and so will re-declaring properties with no initializers.
To detect the issue around accessors, TypeScript 3.7 will now emit get / set accessors in .d.ts files so that in TypeScript can check for overridden accessors.
Code that’s impacted by the class fields change can get around the issue by converting field initializers to assignments in constructor bodies.
To help mitigate the second issue, you can either add an explicit initializer or add a declare modifier to indicate that a property should have no emit.
Currently useDefineForClassFields is only available when targeting ES5 and upwards, since Object.defineProperty doesn’t exist in ES3. To achieve similar checking for issues, you can create a separate project that targets ES5 and uses noEmit to avoid a full build.
For more information, you can take a look at the original pull request for these changes .
We strongly encourage users to try the useDefineForClassFields flag and report back on our issue tracker or in the comments below. This includes feedback on difficulty of adopting the flag so we can understand how we can make migration easier.
Build-Free Editing with Project References
TypeScript’s project references provide us with an easy way to break codebases up to give us faster compiles. Unfortunately, editing a project whose dependencies hadn’t been built (or whose output was out of date) meant that the editing experience wouldn’t work well.
In TypeScript 3.7, when opening a project with dependencies, TypeScript will automatically use the source .ts / .tsx files instead. This means projects using project references will now see an improved editing experience where semantic operations are up-to-date and “just work”. You can disable this behavior with the compiler option disableSourceOfProjectReferenceRedirect which may be appropriate when working in very large projects where this change may impact editing performance.
You can read up more about this change by reading up on its pull request .
Uncalled Function Checks
A common and dangerous error is to forget to invoke a function, especially if the function has zero arguments or is named in a way that implies it might be a property rather than a function.
Here, we forgot to call isAdministrator , and the code incorrectly allows non-administrator users to edit the configuration!
In TypeScript 3.7, this is identified as a likely error:
This check is a breaking change, but for that reason the checks are very conservative. This error is only issued in if conditions, and it is not issued on optional properties, if strictNullChecks is off, or if the function is later called within the body of the if :
If you intended to test the function without calling it, you can correct the definition of it to include undefined / null , or use !! to write something like if (!!user.isAdministrator) to indicate that the coercion is intentional.
We owe a big thanks to GitHub user @jwbay who took the initiative to create a proof-of-concept and iterated to provide us with the current version .
// @ts-nocheck in TypeScript Files
TypeScript 3.7 allows us to add // @ts-nocheck comments to the top of TypeScript files to disable semantic checks. Historically this comment was only respected in JavaScript source files in the presence of checkJs , but we’ve expanded support to TypeScript files to make migrations easier for all users.
Semicolon Formatter Option
TypeScript’s built-in formatter now supports semicolon insertion and removal at locations where a trailing semicolon is optional due to JavaScript’s automatic semicolon insertion (ASI) rules. The setting is available now in Visual Studio Code Insiders , and will be available in Visual Studio 16.4 Preview 2 in the Tools Options menu.
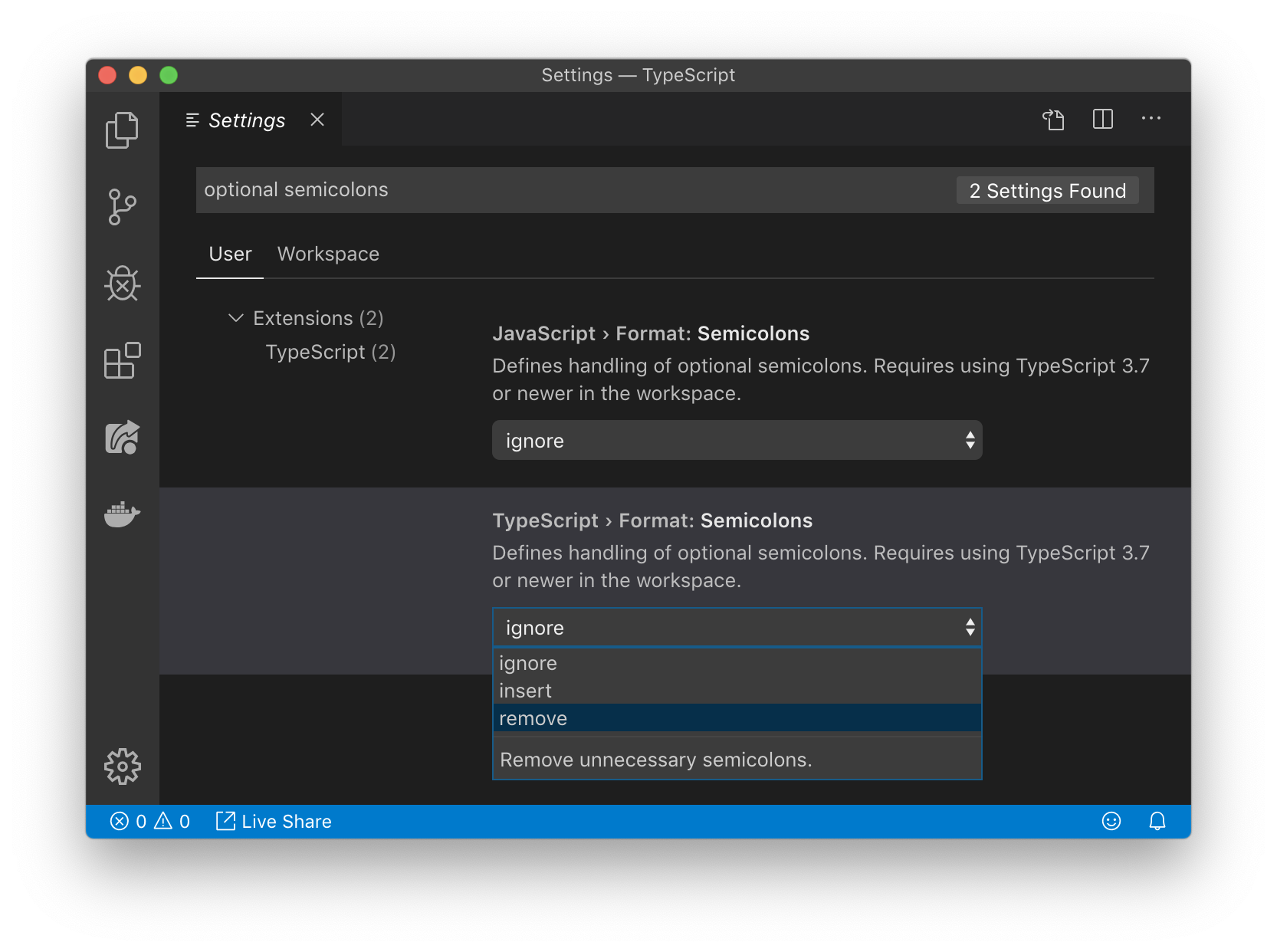
Choosing a value of “insert” or “remove” also affects the format of auto-imports, extracted types, and other generated code provided by TypeScript services. Leaving the setting on its default value of “ignore” makes generated code match the semicolon preference detected in the current file.
3.7 Breaking Changes
Dom changes.
Types in lib.dom.d.ts have been updated . These changes are largely correctness changes related to nullability, but impact will ultimately depend on your codebase.
Class Field Mitigations
As mentioned above , TypeScript 3.7 emits get / set accessors in .d.ts files which can cause breaking changes for consumers on older versions of TypeScript like 3.5 and prior. TypeScript 3.6 users will not be impacted, since that version was future-proofed for this feature.
While not a breakage per se, opting in to the useDefineForClassFields flag can cause breakage when:
- overriding an accessor in a derived class with a property declaration
- re-declaring a property declaration with no initializer
To understand the full impact, read the section above on the useDefineForClassFields flag .
Function Truthy Checks
As mentioned above, TypeScript now errors when functions appear to be uncalled within if statement conditions. An error is issued when a function type is checked in if conditions unless any of the following apply:
- the checked value comes from an optional property
- strictNullChecks is disabled
- the function is later called within the body of the if
Local and Imported Type Declarations Now Conflict
Due to a bug, the following construct was previously allowed in TypeScript:
Here, SomeType appears to originate in both the import declaration and the local interface declaration. Perhaps surprisingly, inside the module, SomeType refers exclusively to the import ed definition, and the local declaration SomeType is only usable when imported from another file. This is very confusing and our review of the very small number of cases of code like this in the wild showed that developers usually thought something different was happening.
In TypeScript 3.7, this is now correctly identified as a duplicate identifier error . The correct fix depends on the original intent of the author and should be addressed on a case-by-case basis. Usually, the naming conflict is unintentional and the best fix is to rename the imported type. If the intent was to augment the imported type, a proper module augmentation should be written instead.
3.7 API Changes
To enable the recursive type alias patterns described above, the typeArguments property has been removed from the TypeReference interface. Users should instead use the getTypeArguments function on TypeChecker instances.
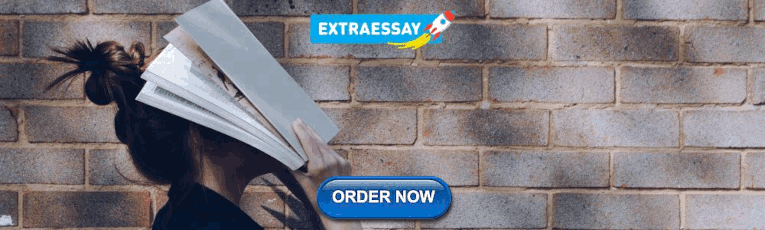
Nightly Builds
How to use a nightly build of TypeScript
The TypeScript docs are an open source project. Help us improve these pages by sending a Pull Request ❤
Last updated: May 13, 2024
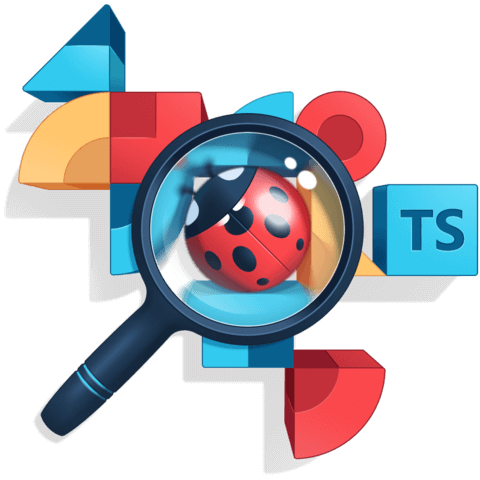
Advanced TypeScript Fundamentals
- 1 Use the Optional Chaining Operator in TypeScript 7m 13s
- 2 Use the Nullish Coalescing Operator in TypeScript 5m 7s
- 3 Statically Type Unknown Values with TypeScript's unknown Type 7m 12s
- 4 Narrow the unknown Type with TypeScript's Assertion Functions 4m 31s
- 5 Perform null Checks with Assertion Functions 3m 58s
- 6 Create Private Class Fields Using TypeScript's private Modifier 2m 56s
- 7 Create Truly Private Class Fields Using the #fieldName Syntax 2m 35s
- 8 Encapsulate Truly Private Variables in a Closure 1m 3s
- 9 Ensure Initialization of Class Instance Properties in TypeScript 6m 2s
- 10 Declare Read-Only Array Types in TypeScript 2m 44s
- 11 Declare Read-Only Tuple Types in TypeScript 2m 32s
- 12 Prevent Type Widening of Object Literals with TypeScript's const Assertions 2m 29s
- 13 Prevent Type Widening of Array Literals with TypeScript's const Assertions 2m 14s
- 14 Implement and Type Enum-Style Objects in TypeScript 3m 54s
- 15 Implement a NonNullish<T> Conditional Type in TypeScript 6m 7s
- 16 Implement a ReturnTypeOf<T> Conditional Type in TypeScript 3m 23s
- 17 Statically Type String Literals with Template Literal Types in TypeScript 4m 6s
- 18 Use TypeScript's Mapped Types and Template Literal Types Together 6m 12s
Use the Nullish Coalescing Operator in TypeScript

Social Share Links
This lesson introduces the ?? operator which is known as nullish coalescing . The ?? operator produces the value on the right-hand side if (and only if) the value on the left-hand side is null or undefined , making it a useful operator for providing fallback values.
We'll contrast the ?? operator with the || logical OR operator which produces the value on the right-hand side if the value on the left-hand side is any falsy value (such as null , undefined , false , "" , 0 , …).
Additional Reading
- Nullish Coalescing: The ?? Operator in TypeScript
- The && and || Operators in JavaScript
Instructor: [0:00] In the previous lesson, we looked at the optional chaining operator and how to use it in TypeScript. In this lesson, I want to talk about the nullish coalescing operator, which is written as two question marks. It's oftentimes used in conjunction with optional chaining.
[0:15] Before we jump into the nullish coalescing operator, I want to motivate why it's useful. We can parse in the indentation level when we call the serializeJSON() function. In this case, I'm parsing the indentation level two. If we don't specify the indent property, the default indentation level is zero, and we can verify this by running this program.
[0:37] In the output, we can see that the JSON is not indented at all, so the indentation level is zero. Now, let's say that we wanted the default indentation level to be two rather than zero. If we don't parse an indent property, we should use the value two.
[0:54] We can do this by using the logical OR operator and parsing two as the right operand. If we run the program again, we can now see that the resulting JSON is indented with two spaces. Of course, we can also still specify a different indentation. If I come back down here and I specify an indentation level of four, and if I run this one more time, we can see that the output is indented with four spaces.
[1:21] Let's see what happens when I set the indent property to zero. We would expect the resulting JSON to not be indented at all. However, this is not what's happening. We still get an indentation level of two. This is because of how we're using the OR operator here.
[1:38] The logical OR operator has a left operand and a right operand. It evaluates to the right operand if, and only if the left operand is falsy. If we have the expression null or two, the resulting value is two because null is a falsy value, so the operator produces the right operand. Similarly, if we have the expression undefined or two, we also get the value two because undefined is also a falsy value.
[2:07] However, we shouldn't forget that the number zero is also considered a falsy value. If we have the expression zero or two, the result is two, because the left operand is a falsy value. This applies to other falsy values as well, for example, the values false, Not-a-Number or the empty string.
[2:29] In all cases, the logical OR operator produces the right operand because the left-hand side is falsy. This is where it gets tricky sometimes if you're trying to mix nullable values with a logical OR operator. We want to provide a fallback value, but we only want to provide it for null and undefined, not for any other falsy values.
[2:49] This is where we should be using the nullish coalescing operator instead. The nullish coalescing operator is written as two question marks. It also takes two operands. It produces the value on the right-hand side if the value on the left-hand is null or undefined. Differently, we only fallback to the right-hand side if we have a nullish value on the left-hand side.
[3:13] Nullish in this case means null or undefined. That's why it's called nullish coalescing. Here is where the operator comes in useful. If we have a falsy value on the left-hand side that is not null or undefined, we keep the left-hand side, so we can write, 0'2. That expression will evaluate to the value of zero. This is exactly the behavior that we want in our serialized JSON function.
[3:40] For the sake of completeness, let's go through the other examples we've seen before. We also tried the values false, not a number, and the empty string. You can see that in all cases, even though these are falsy values, we keep the left-hand side when using the nullish coalescing operator.
[4:00] Let's jump back into our example. Let's fix our code. Let's see if this works. We can see that we get the JSON serialized string with no indentation because we passed the value of zero. Let's now set the indentation level to four. Let's give this another run. That's looking fine as well. Finally, if we don't specify any indentation level, we now use the fallback value of two.
[4:33] You'll oftentimes see the nullish coalescing operator used in conjunction with the optional chaining operator. Optional chaining can produce the value undefined. Nullish coalescing is a great way to provide a fallback value. Be careful when using the logical OR operator. It might not have the behavior that you want.
[4:52] In a lot of cases, the nullish coalescing operator should be preferred over the logical OR operator. To know which one you should be using, ask yourself whether you want to provide a fallback value for falsy values or for nullish values only.

Member comments are a way for members to communicate, interact, and ask questions about a lesson.
The instructor or someone from the community might respond to your question Here are a few basic guidelines to commenting on egghead.io
Be on-Topic
Comments are for discussing a lesson. If you're having a general issue with the website functionality, please contact us at [email protected].
Avoid meta-discussion
- This was great!
- This was horrible!
- I didn't like this because it didn't match my skill level.
- +1 It will likely be deleted as spam.
Code Problems?
Should be accompanied by code! Codesandbox or Stackblitz provide a way to share code and discuss it in context
Details and Context
Vague question? Vague answer. Any details and context you can provide will lure more interesting answers!

The Ultimate Guide to the ES2020 Nullish Coalescing Operator

Faraz Kelhini
Bits and Pieces
Perhaps the most interesting aspect of logical operators in JavaScript is their ability to execute code conditionally. JavaScript evaluates logical operators from left to right. If the first argument is sufficient to determine the outcome of the operator, JavaScript skips evaluating the second argument. This behavior is called short-circuiting and provides developers with the opportunity to write a more concise and readable code.
Up until recently, JavaScript had only two short-circuiting operators: && and || . The nullish coalescing ( ?? ) operator is an ES2020 feature that brings a new short-circuiting operator to JavaScript, and it works differently from its counterparts.
In this article, we’ll first look at how short-circuiting in JavaScript works. Then we’ll see why the existing short-circuiting operators aren’t effective for specific use cases, and how the new nullish coalescing operator helps developers write less error-prone and more concise code.
Tip: Use Bit ( Github ) to share, document, and manage reusable components from different projects. It’s a great way to increase code reuse, speed up development, and build apps that scale.
The shared component cloud
Bit is a scalable and collaborative way to build and reuse components. it's everything you need from local development…, why is short-circuiting useful.
When we use logical operators (also known as short-circuiting operators) with boolean values, they return a boolean value. The logical AND ( && ) operator, for example, returns true if both of its operands evaluate to true :
If the left-hand operand of the && operator evaluates to false , the JavaScript interpreter is smart enough to know that the result of the operator will be false , so it doesn’t see any point in wasting resources to evaluate the right operand. This triggers what is known as short-circuiting.
JavaScript developers often use short-circuiting as a shortcut for the if…else statement. Consider the following example:
This code checks whether the result of a < b is true . If so, it multiplies a by b and assigns the result to c . If not, it assigns the result of a < b to c , which is false . This code is roughly equivalent to:
Similarly, the || operator executes the right operand only if the left one is falsy:
This code uses the || operator to check whether user.job has a falsy value. Since null is falsy, the operator returns the result of its right operand, which acts as a default value for userJob .
&& and || are known as short-circuit operators because they determine the outcome of the overall expression by evaluating as fewest operands as possible.
What are truthy and falsy values?
The outcome of JavaScript short-circuiting operators depends on whether their first argument is truthy or falsy. But what exactly does that mean? In JavaScript, any value is considered truthy except for undefined , null , false , 0 , NaN , and the empty string '' .
When we use a falsy value in a context where a Boolean is expected, such as conditionals and loops, JavaScript automatically converts the value into false . Conversely, when we use a truthy value in a context where a Boolean is expected, JavaScript converts it into true .
The problem with the existing short-circuiting operators
The && and || operators work perfectly for many programming tasks. But, they can also be a source of bugs if we’re not careful. Suppose we want to give a default value to a variable, so when we pass no value to a function, the variable will have a value of true . A wrong approach is to use the || operator, as shown in this example:
Here, darkMode is supposed to be an optional property with a default value of true that can be changed by passing {darkMode: false} to the function. But no matter what value we pass, the operator will always return true .
The bug in this code is the result of misunderstanding how the || operator works. One way to fix this is to use the destructuring assignment syntax:
This code defines a default value for the destructured variable, which is used only if the expected property is missing or explicitly set to undefined .
However, this could cause another problem. What if the value passed to the function isn’t even an object to perform destructing on? Some developers take advantage of the strict inequality operator ( !== ) to clearly define the value they expect:
Programming tasks like this are very prevalent. That’s why the Ecma Technical Committee has decided to introduce a dedicated operator to streamline the process.
Introducing the nullish coalescing operator
The nullish coalescing ( ?? ) operator is JavaScript’s third short-circuiting operator that acts very similar to the logical OR ( || ) operator, except that it checks whether the value is nullish rather than falsy. That means only null and undefined values will cause the operator to return its right-hand side operand. In the expression x ?? Y , for example, the resulting value is y only if x has a value of undefined or null . Compare:
As you can see, any falsy value except for null and undefined forces the ?? operator to return its left operand. This is very useful when providing a default value for a property or variable. Using the ?? operator, we can rewrite the example in the previous section like this:
The ?? operator is also particularly useful when dealing with DOM APIs. For instance, we can use it when selecting an HTML element that may not exist:
In this code, the querySelector() method attempts to return the element that matches elem . But if there’s no such an element in the document, it returns null , causing the ?? operator to execute its right operand.
Combining the nullish coalescing operator with the optional chaining operator
Another interesting ES2020 feature is the optional chaining operator ( ?. ), which allows us to access a nested property without having to explicitly check that each object in the chain exists.
By combining the nullish coalescing operator with the optional chaining operator, we can safely provide a value other than undefined for a missing property. Here’s an example:
This code assigns a default value for a nested property that may not exist or have a nullish value. As a result, it automatically falls back to the value Europe .
Operator precedence
Often, JavaScript expressions are made of just one or two operands and a single operator. But in more complex expressions, where there are two or more operators, JavaScript determines the order in which it evaluates the operators based on operator precedence.
Consider the expression 2+4*3 . The result of this expression depends on the order in which we perform the calculations. If we perform the addition first and then the multiplication, the result will be 18 . But if we calculate from right to left, the result will be 14 . Therefore, operator precedence is crucial to eliminate this ambiguity.
When mixing the && and || operators, && has higher precedence, so it’s evaluated first:
But what if we want the || operator to be evaluated before && ? To change the order of the evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first:
The new ?? operator is different from its counterparts in that we must explicitly denote the order of evaluation; otherwise, we’ll get a syntax error:
The ?? operator always requires parentheses when mixing it with other short-circuiting operators. The reason for this design is to eliminate any ambiguity when interpreting the developer’s intention and to improve the readability of the code. Here are some more examples:
The nullish coalescing ( ?? ) operator is a welcome addition to the JavaScript language that complements the existing short-circuiting operators. Unlike the current operators, the nullish coalescing operator only checks whether the left operand is null or undefined , which is extremely useful for many programming tasks.
Although this new operator is still at the proposal stage, we can use it today. All modern browsers, including Chrome 80+, Firefox 72+, Safari 13.1+, have already implemented the feature, and we can add support to older browsers by using a transpiler.
If you have any questions feel free to ask in the comments, I’m also on twitter .
Code Principles Every Programmer Should Follow
Yagni, law of demeter, single responsibility and other useful principles for better coding..
blog.bitsrc.io
Understanding WeakMaps in JavaScript
Learn how to use this underrated data structure from es6, npm tips and tricks, 12 useful npm tips and tricks for more productive javascript coding..

Written by Faraz Kelhini
Faraz is a professional JavaScript developer who is passionate about promoting patterns and ideas that make web development more productive. Website: eloux.com
More from Faraz Kelhini and Bits and Pieces
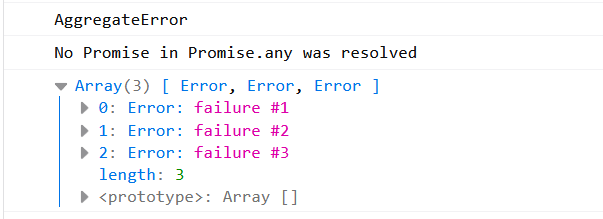
The Pragmatic Programmers
Using AggregateError in JavaScript
See multiple errors in one error.
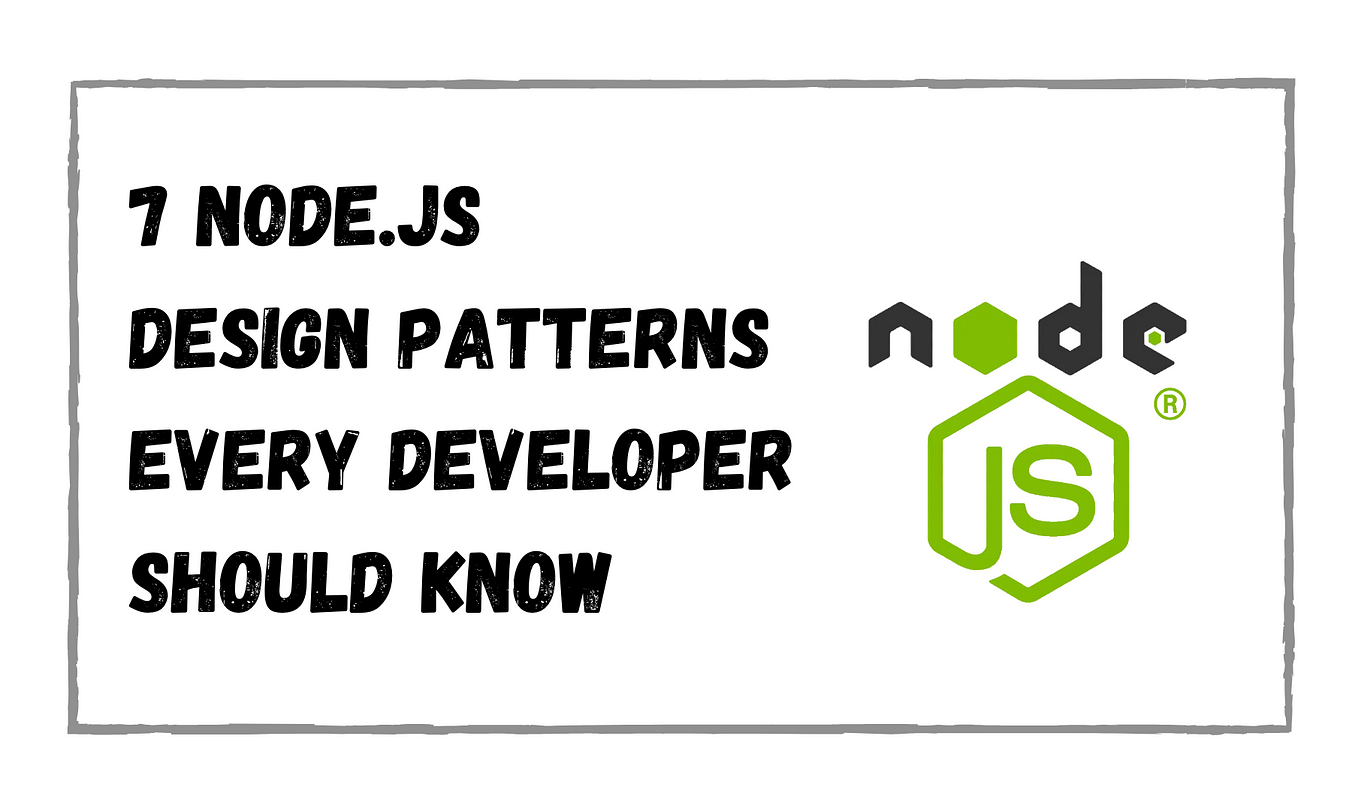
Danusha Navod
7 Node.js Design Patterns Every Developer Should Know
Explore the facade, adapter, singleton, prototype, builder, proxy and factory for modern software design..
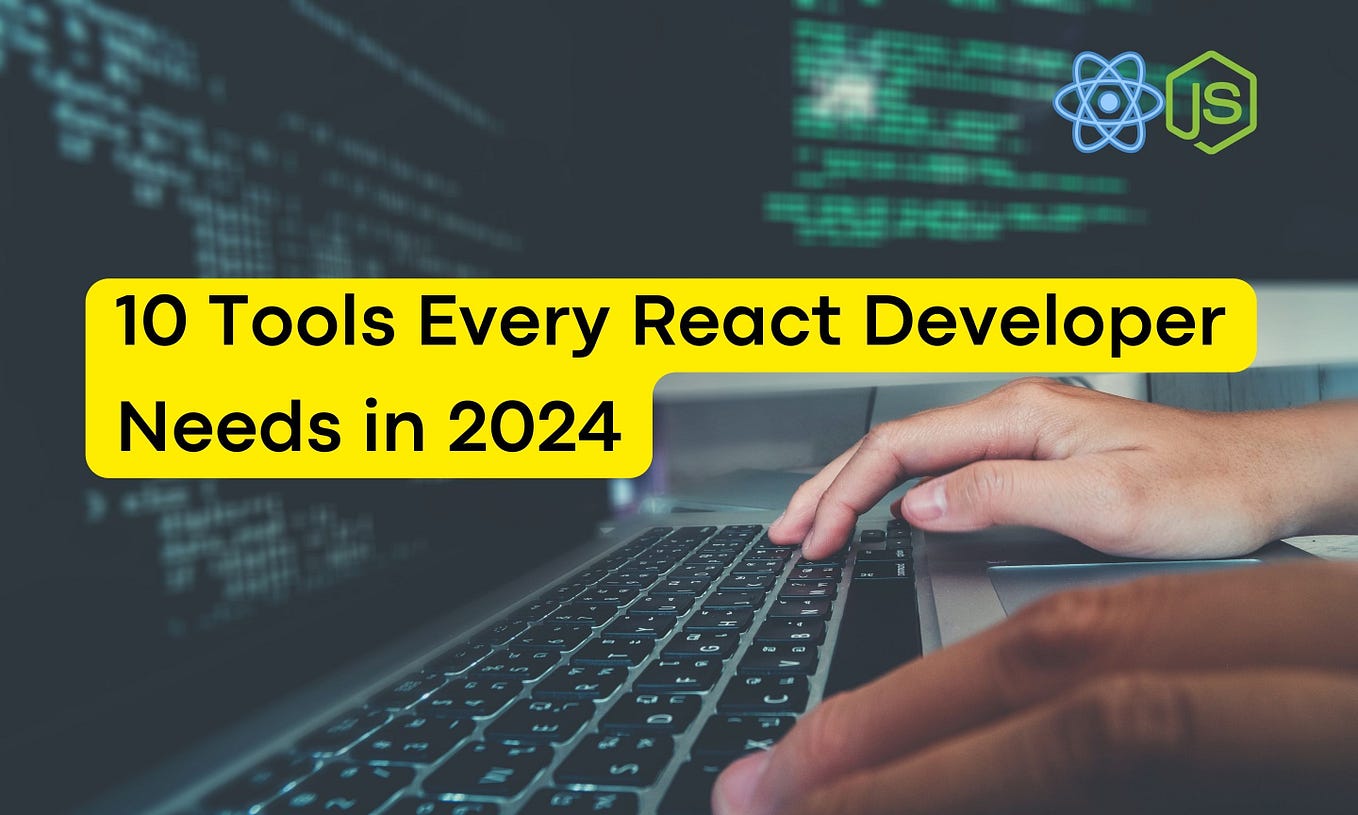
Ashan Fernando
Top 10 Tools Every React Developer Needs in 2024
Enhancing efficiency and creativity in the react ecosystem using these highly effective tools.

Automatic Dark Mode with JavaScript
Detecting users’ os dark mode preference using matchmedia(), recommended from medium.
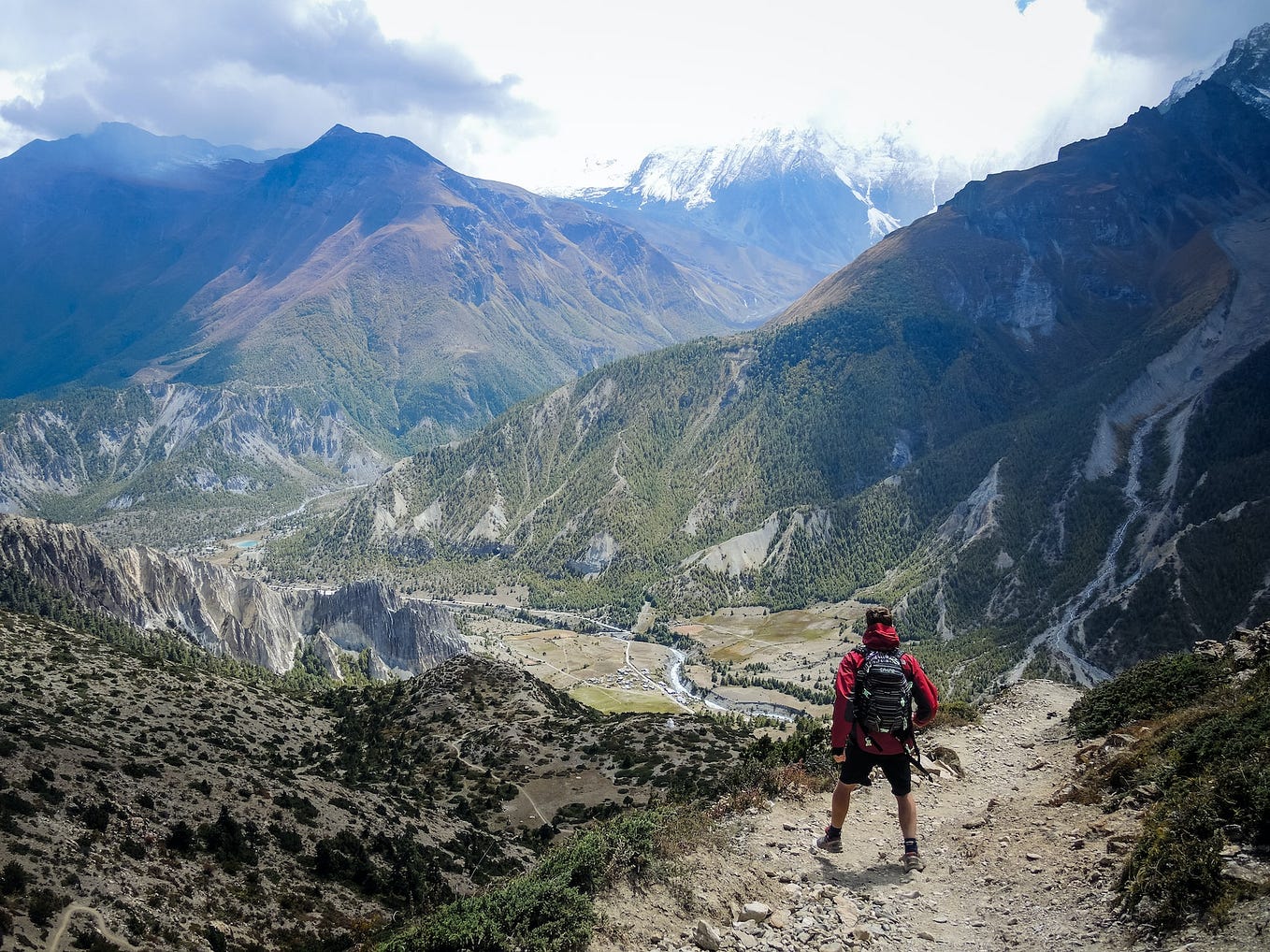
Benoit Ruiz
Better Programming
Advice From a Software Engineer With 8 Years of Experience
Practical tips for those who want to advance in their careers.
Somnath Singh
Level Up Coding
The Era of High-Paying Tech Jobs is Over
The death of tech jobs..

Stories to Help You Grow as a Software Developer

General Coding Knowledge
Coding & Development

Tech & Tools
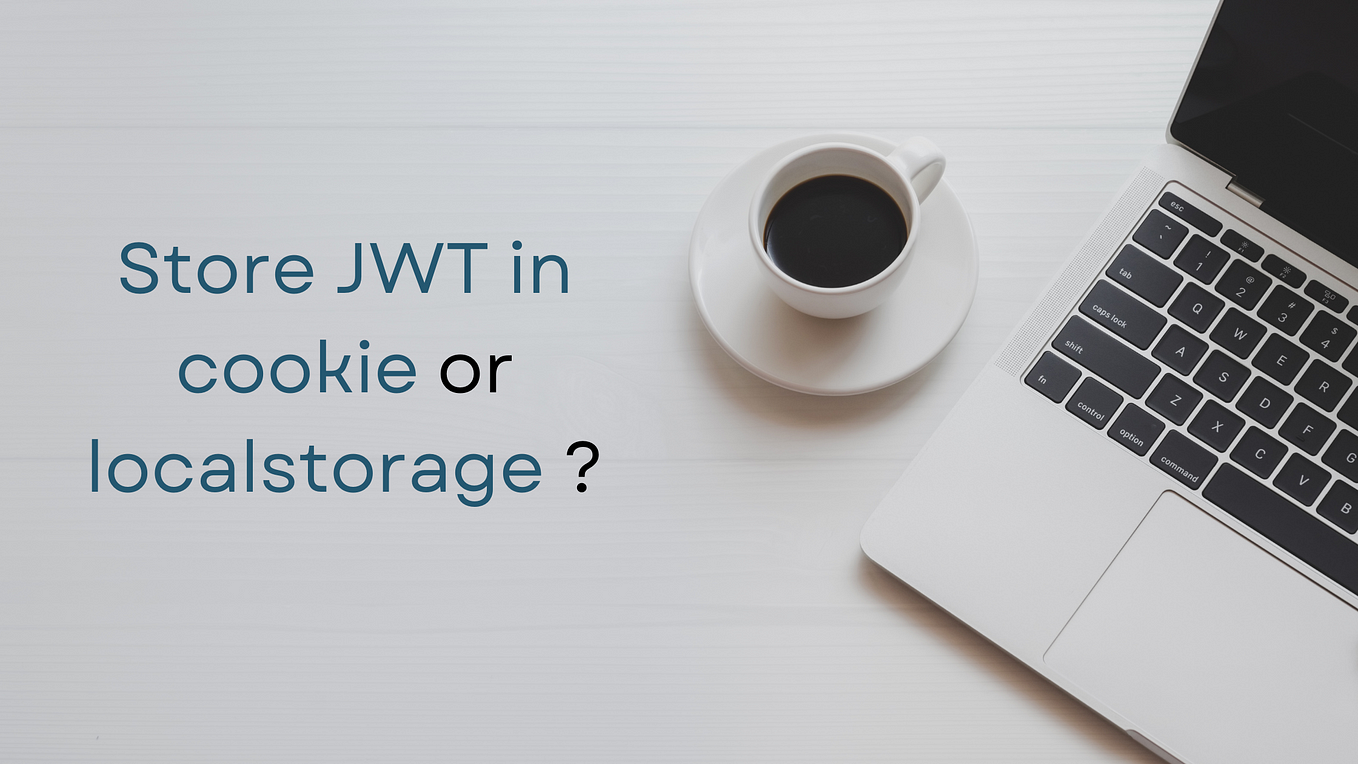
Store JWT in cookie or localstorage ?
Source:- store jwt in cookie or localstorage.
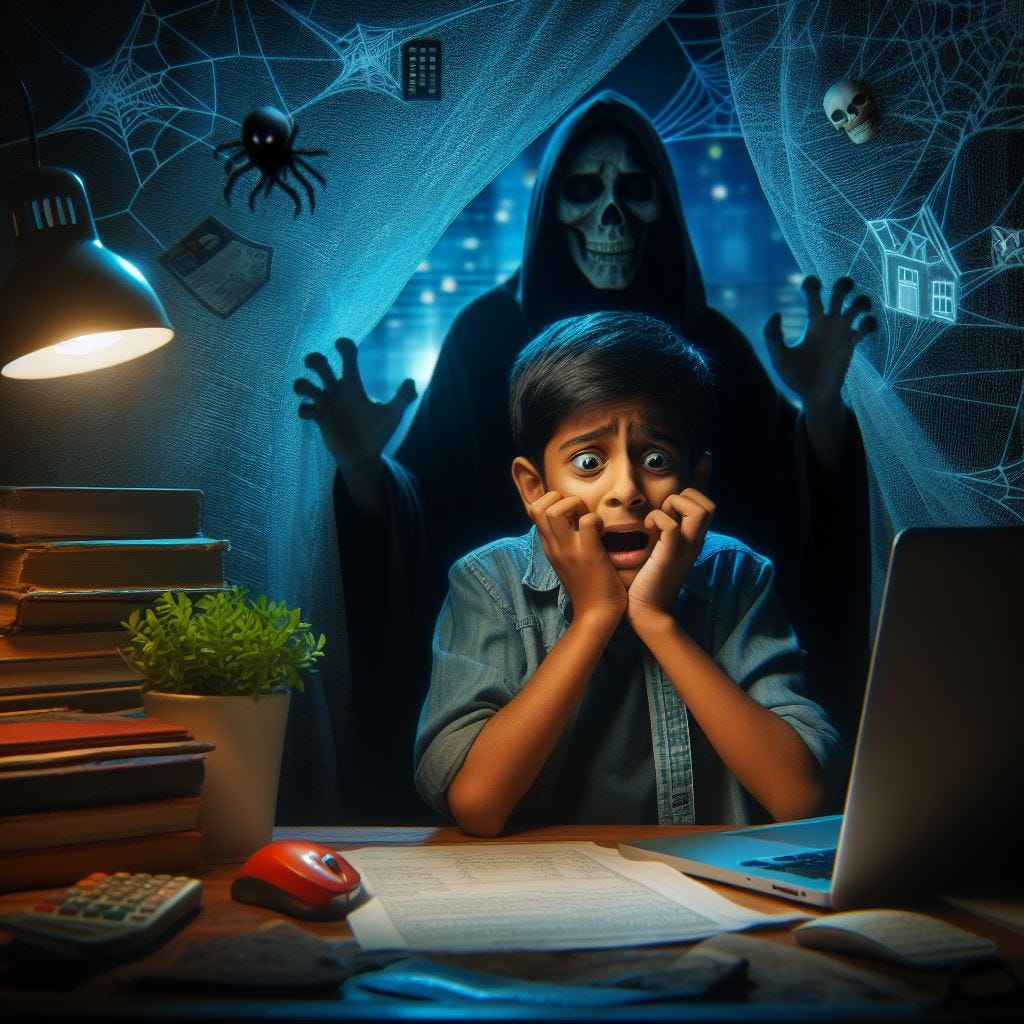
Kallol Mazumdar
ILLUMINATION
I Went on the Dark Web and Instantly Regretted It
Accessing the forbidden parts of the world wide web, only to realize the depravity of humanity.
Hazel Paradise
How I Create Passive Income With No Money
Many ways to start a passive income today.
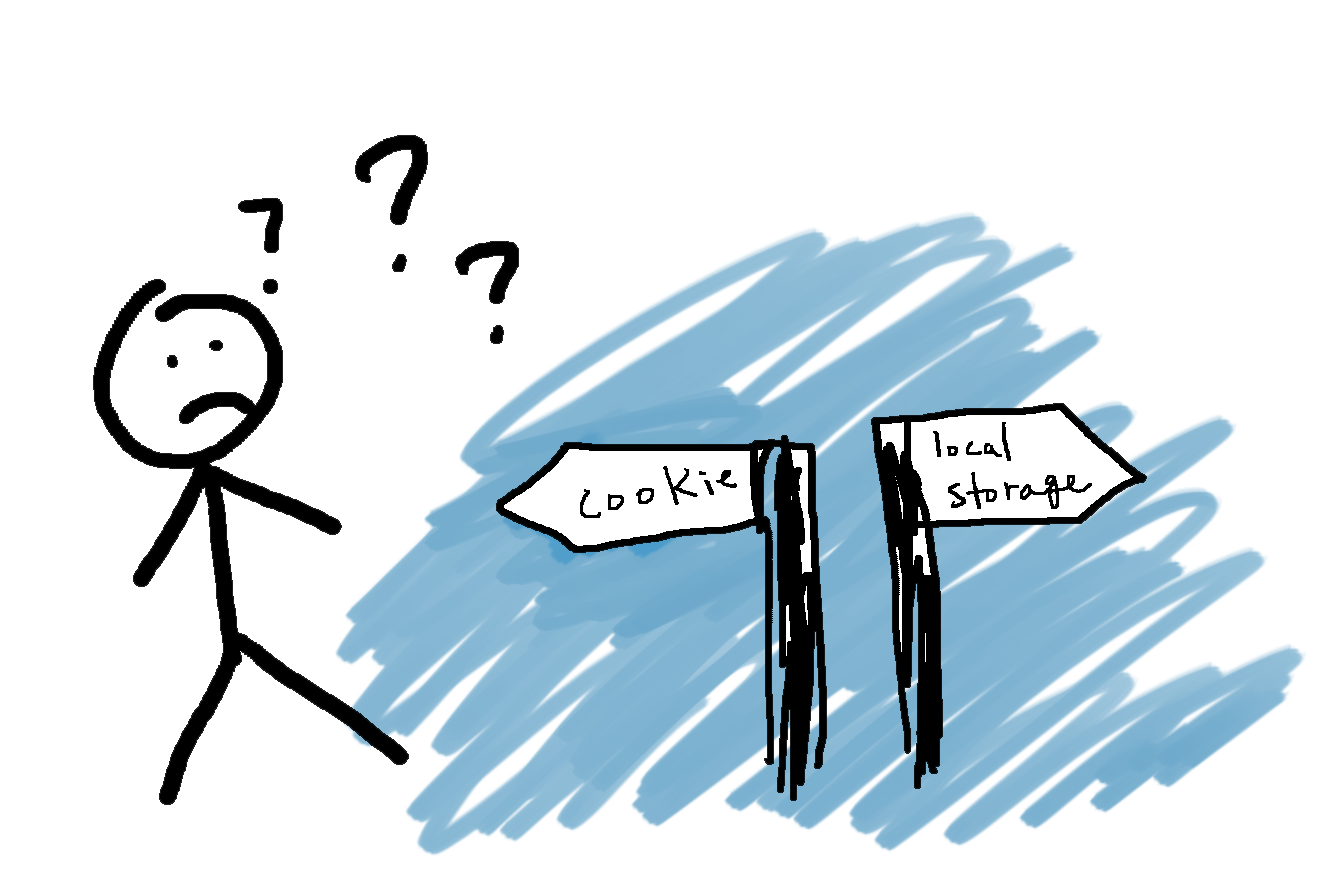
Julien Etienne
Stop Using localStorage
Bid farewell to localstorage embrace indexeddb for speed, type-safe storage, and non-blocking data transactions. #indexeddb #webdev.
Text to speech
- Web Development
What Is the JavaScript Logical Nullish Assignment Operator?
- Daniyal Hamid
- 24 Dec, 2021
Introduced in ES12, you can use the logical nullish assignment operator ( ??= ) to assign a value to a variable if it hasn't been set already. Consider the following examples, which are all equivalent:
The null coalescing assignment operator only assigns the default value if x is a nullish value (i.e. undefined or null ). For example:
In the following example, x already has a value, therefore, the logical nullish assignment operator would not set a default (i.e. it won't return the value on the right side of the assignment operator):
In case, you use the nullish assignment operator on an undeclared variable, the following ReferenceError is thrown:
This post was published 24 Dec, 2021 by Daniyal Hamid . Daniyal currently works as the Head of Engineering in Germany and has 20+ years of experience in software engineering, design and marketing. Please show your love and support by sharing this post .
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Nullish Coalescing and Logical Compound Assignments ( ??= , ||= , &&= ) #37255
danielrentz commented Mar 6, 2020
- 👍 33 reactions
IllusionMH commented Mar 6, 2020
- 👍 2 reactions
Sorry, something went wrong.
danielrentz commented Mar 6, 2020 • edited
DanielRosenwasser commented Mar 6, 2020
- 👍 4 reactions
Kingwl commented Mar 31, 2020
- 👍 7 reactions
- 😄 1 reaction
- ❤️ 5 reactions
DanielRosenwasser commented Mar 31, 2020 • edited
- 👀 2 reactions
DanielRosenwasser commented Mar 31, 2020
- 🎉 29 reactions
- ❤️ 1 reaction
- 🚀 7 reactions
Kingwl commented Apr 1, 2020
aminpaks commented Jun 16, 2020 • edited
Kingwl commented jun 16, 2020.
kolpav commented Feb 16, 2021
Danielrentz commented feb 16, 2021 • edited.
- 😕 1 reaction
- 👍 1 reaction
gustavopch commented Jun 24, 2021
Kolpav commented jun 27, 2021 • edited.
Successfully merging a pull request may close this issue.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JS Arithmetic Operators
- Addition(+) Arithmetic Operator in JavaScript
- Subtraction(-) Arithmetic Operator in JavaScript
- Multiplication(*) Arithmetic Operator in JavaScript
- Division(/) Arithmetic Operator in JavaScript
- Modulus(%) Arithmetic Operator in JavaScript
- Exponentiation(**) Arithmetic Operator in JavaScript
- Increment(+ +) Arithmetic Operator in JavaScript
- Decrement(--) Arithmetic Operator in JavaScript
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Arithmetic Unary Negation(-) Operator
JS Assignment Operators
- Addition Assignment (+=) Operator in Javascript
- Subtraction Assignment( -=) Operator in Javascript
- Multiplication Assignment(*=) Operator in JavaScript
- Division Assignment(/=) Operator in JavaScript
- JavaScript Remainder Assignment(%=) Operator
- Exponentiation Assignment(**=) Operator in JavaScript
- Left Shift Assignment (<<=) Operator in JavaScript
- Right Shift Assignment(>>=) Operator in JavaScript
- Bitwise AND Assignment (&=) Operator in JavaScript
- Bitwise OR Assignment (|=) Operator in JavaScript
- Bitwise XOR Assignment (^=) Operator in JavaScript
- JavaScript Logical AND assignment (&&=) Operator
- JavaScript Logical OR assignment (||=) Operator
Nullish Coalescing Assignment (??=) Operator in JavaScript
Js comparison operators.
- Equality(==) Comparison Operator in JavaScript
- Inequality(!=) Comparison Operator in JavaScript
- Strict Equality(===) Comparison Operator in JavaScript
- Strict Inequality(!==) Comparison Operator in JavaScript
- Greater than(>) Comparison Operator in JavaScript
- Greater Than or Equal(>=) Comparison Operator in JavaScript
- Less Than or Equal(
JS Logical Operators
- NOT(!) Logical Operator inJavaScript
- AND(&&) Logical Operator in JavaScript
- OR(||) Logical Operator in JavaScript
JS Bitwise Operators
- AND(&) Bitwise Operator in JavaScript
- OR(|) Bitwise Operator in JavaScript
- XOR(^) Bitwise Operator in JavaScript
- NOT(~) Bitwise Operator in JavaScript
- Left Shift (
- Right Shift (>>) Bitwise Operator in JavaScript
- Zero Fill Right Shift (>>>) Bitwise Operator in JavaScript
JS Unary Operators
- JavaScript typeof Operator
- JavaScript delete Operator
JS Relational Operators
- JavaScript in Operator
- JavaScript Instanceof Operator
JS Other Operators
- JavaScript String Operators
- JavaScript yield Operator
- JavaScript Pipeline Operator
- JavaScript Grouping Operator
This is a new operator introduced by javascript. This operator is represented by x ??= y and it is called Logical nullish assignment operator. Only if the value of x is nullish then the value of y will be assigned to x that means if the value of x is null or undefined then the value of y will be assigned to x.
Let’s discuss how this logical nullish assignment operator works. Firstly we all know that logical nullish assignment is represented as x ??= y , this is derived by two operators nullish coalescing operator and assignment operator we can also write it as x ?? (x = y) . Now javascript checks the x first, if it is nullish then the value of y will be assigned to x .
Example 1 :
Example 2 :
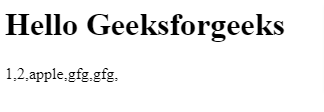
Supported browsers
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
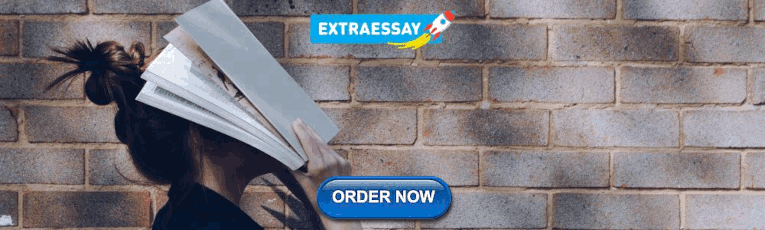
IMAGES
VIDEO
COMMENTS
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
Nullish Coalescing: The ?? Operator in TypeScript August 6, 2020. TypeScript 3.7 added support for the ?? operator, which is known as the nullish coalescing operator.We can use this operator to provide a fallback value for a value that might be null or undefined. #Truthy and Falsy Values in JavaScript Before we dive into the ?? operator, let's recall that JavaScript values can either be truthy ...
Nullish Coalescing. The nullish coalescing operator is an alternative to || which returns the right-side expression if the left-side is null or undefined. In contrast, || uses falsy checks, meaning an empty string or the number 0 would be considered false. A good example for this feature is dealing with partial objects which have defaults when ...
The OR operator || uses the right value if left is falsy, while the nullish coalescing operator ?? uses the right value if left is null or undefined. These operators are often used to provide a default value if the first one is missing. But the OR operator || can be problematic if your left value might contain "" or 0 or false (because these ...
In TypeScript, optional chaining is defined as the ability to immediately stop running an expression if a part of it evaluates to either null or undefined.It was introduced in TypeScript 3.7 with the ?. operator.. Optional chaining is often used together with nullish coalescing, which is the ability to fall back to a default value when the primary expression evaluates to null or undefined.
Then the next assignment is skipped, since "var1" is not null. This can be used to assign a value only if the variable is null. It's less commonly seen, but you might still run into it. TypeScript. The nullish coalescing operator is actually not unique to TypeScript.
Overview TypeScript 3.7 added support for the ?? operator, which is known as the nullish coalescing operator. We can use this operator to provide a fallback value for a value that might be null or undefined. Truthy and Falsy Values in JavaScript Before we dive into the ?? operator, let's recall { 回想 } that JavaScript values can either be truthy or falsy: when coerced { 强制,
The Nullish Coalescing Operator (??) is a feature introduced in TypeScript to handle the case of null or undefined values more effectively. It provides a concise way to check if a value is nullish and fallback to a default value if it is. Example. Consider a scenario where you have an API response that may contain a nullable property.
In TypeScript 3.7, when opening a project with dependencies, TypeScript will automatically use the source .ts / .tsx files instead. This means projects using project references will now see an improved editing experience where semantic operations are up-to-date and "just work".
The nullish coalescing operator is written as two question marks. It also takes two operands. It produces the value on the right-hand side if the value on the left-hand is null or undefined. Differently, we only fallback to the right-hand side if we have a nullish value on the left-hand side. [3:13] Nullish in this case means null or undefined.
The Ultimate Guide to the ES2020 Nullish Coalescing Operator. Perhaps the most interesting aspect of logical operators in JavaScript is their ability to execute code conditionally. JavaScript evaluates logical operators from left to right. If the first argument is sufficient to determine the outcome of the operator, JavaScript skips evaluating ...
In the following example, x already has a value, therefore, the logical nullish assignment operator would not set a default (i.e. it won't return the value on the right side of the assignment operator):
Saved searches Use saved searches to filter your results more quickly
Do you have accesss to your webpack.config.js file? Usually you need to add a loader (like tslint-loader), a tsconfig file and a rule in webpack config in order to let webpack transpile the TS code into JS.. If CRA doesn't let you modify that file without ejecting, there must be a way in which you can update the loader to the latest typescript specifications
The nullish coalescing operator ( ?? or typescript double question mark) is a logical operator that takes two arguments. It returns the right-hand side operand when its left-hand side operand is null or undefined. otherwise, it returns its left-hand side operand. Table of Contents.
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
I experimented a bit further and found it is not only an issue isolated to the nullish coalescing operator, but couldn't bend my mind around when typescript is able to use soemthing as a typeguard and when not (below you find some examples) The two last line were confusing me most. It seems to me both expressions that are assigned to x7 and x8 ...
TypeScript Tutorial. An assignment operator requires two operands. The value of the right operand is assigned to the left operand. The sign = denotes the simple assignment operator. The typescript also has several compound assignment operators, which is actually shorthand for other operators. List of all such operators are listed below.
Stack Overflow Jobs powered by Indeed: A job site that puts thousands of tech jobs at your fingertips (U.S. only).Search jobs
59. Nullish coalescing operator allows assigning a variable if it's not null or undefined, or an expression otherwise. It is an improvement over previously used || because || will also assign other if b is empty string or other falsy, but not nullish value. However, sometimes, we also use && for value assignment, for example.