Declaration and Reassignment
Declaring single variables.
In Python, a variable is created during assignment. Python is type inferred — therefore there is no need to explicitly define a variable’s type. It can simply be created by naming it and then assigning any value irrespective of the type to it.
For instance, let’s name a variable moving_average . To save or assign a value to it, we will use the assignment operator (=) followed by the said value.
Here, the variables are moving_average , name_of_language , and main_languages , and each variable has a different type of value. 10.2, “Python”, and [‘Python’, ‘JavaScript’, ‘C++’, ‘Java’, ‘GO’] are literals. In Python, literals refer to a specific and fixed value of a variable at any instant.
Literals , in Python, refer to a specific and fixed value at any instant.
Note : We can not use reserved keywords to name variables. The following table shows the list of reserved keywords found in Python 3.12.0.
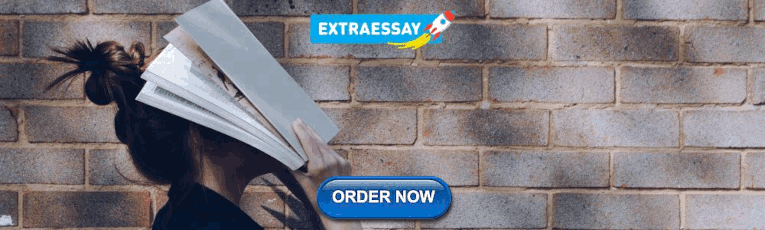
Reassigning Variables
When there is a need to update a variable, they can be reassigned values easily as well
Suppose the moving_average changes to 10.5, Python allows changing the value of a variable by simply reassigning it again.
Declaring Multiple Variables
We can define multiple variables in one line by following a comma separated syntax. Python also provides for the ease of saving the same value in more than one variable.
For example, let’s create two variables, called mean and median , and save 5 and 7 in them, respectively. Let’s also create sorted_list and sorted_list2 and save [‘1’, ‘2’, ‘3’, ‘4’] in both of these variables.
Last modified: Sep 20, 2023 By Alexander Williams
Python: Change Variable Value [Examples]
Example 1: reassigning a variable, example 2: incrementing a numeric variable, example 3: decrementing a numeric variable, example 4: concatenating strings, example 5: updating lists, example 6: modifying dictionary values, example 7: multiplying numeric variables, example 8: updating variables conditionally, example 9: swapping variable values, example 10: updating variables using a function, example 11: appending to a list, example 12: removing an item from a list, example 13: updating dictionary values, example 14: clearing a list, example 15: deleting a variable, related tutorials:.
- Python: Print Variable Name and Value (Examples)
Recent Tutorials:
- Python Pretty Print JSON Guide
- Python Logging DictConfig with JSON: A Complete Guide
- Python JSON dumps: A Complete Guide
- Convert Python Dict to JSON
- Python JSON Parsing Guide
- How to Index JSON in Python: A Complete Guide
- Python PyTZ: Time Zone Handling Made Easy
- Python process_time(): Measuring CPU Time for Code Performance
6.1 Variable Reassignment, Revisited
So far, we have largely treated values and variables in Python as being constant over time. Once a variable is initialized, its value does not change as the program runs. This principle has made it easier to reason about our code: once we assign a variable to a value, we can easily look up the variable’s value at any later point in the program. Indeed, this is a fact that we take for granted in mathematics: if we say “let \(x\) = 10” in a calculation or proof, we expect \(x\) to keep that same value from start to finish!
However, in programs it is sometimes useful to have variables change value over time. We saw one example of this last week when we studied for loops, in which both the loop variable and accumulator are reassigned to different values as the loop iterates. In this chapter, we’ll go deeper and investigate two related but distinct ways that a variable can change over time in a program: variable reassignment and object mutation . Let’s begin by revisiting variable reassigment.
Variable reassignment
Recall that a Python statement of the form ___ = ___ is called an assignment statement , which takes a variable name on the left-hand side and an expression on the right-hand side, and assigns the value of the expression to the variable.
A variable reassignment is a Python action that assigns a value to a variable when that variable already refers to a value. The most common kind of variable reassignment is with an assignment statement:
The for loops that we studied in Chapter 5 all used variable reassignment to update the accumulator variable inside the loop. For example, here is our my_sum function from 5.4 Repeated Execution: For Loops .
At each loop iteration, the statement sum_so_far = sum_so_far + number did two things:
- Evaluate the right-hand side ( sum_so_far + number ) using the current value of sum_so_far , obtaining a new value.
- Reassign sum_so_far to refer to that new value.
This is the Python mechanism that causes sum_so_far to refer to the total sum at the end of the loop, which of course was the whole point of the loop! Indeed, updating loop accumulators is one of the most natural uses of variable reassignment.
This for loop actually illustrates another common form of variable reassignment: reassigning the loop variable to a different value at each loop iteration of a for loop. For example, when we call my_sum([10, 20, 30]) , the loop variable number gets assigned to the value 10 , then reassigned to the value 20 , and then reassigned to the value 30 .
Variable reassignment never affects other variables
Consider the following Python code:
Here, the variable x is reassigned to 7 on line 3. But what happens to y ? Does it now also get “reassigned” to 9 (which is 7 + 2 ), or does it stay at its original value 3 ?
We can state Python’s behaviour here with one simple rule: variable reassignment only changes the immediate variable being reassigned, and does not change any other variables or values, even ones that were defined using the variable being reassigned . And so in the above example, y still refers to the value 3 , even after x is reassigned to 7 .
This rule might seem a bit strange at first, but is actually the simplest way that Python could execute variable reassignment: it allows programmers to reason about these assignment statements in a top-down order, without worrying that future assignment statements could affect previous ones. If we’re tracing through our code carefully and read y = x + 2 , I can safely predict the value of y based on the current value of x , without worrying about how x might be reassigned later in the program. While this reasoning is correct for variable reassignment, the story will get more complicated when we discuss the other form of “value change” in the next section.
Augmented assignment statements
Our my_sum code from earlier this section uses a very common case of variable reassignment: updating a numeric variable by adding a given value.
Because this type of reassignment is so common, Python (and many other programming languages) defines a special syntax to represent this operation called the augmented assignment statement (often shortened to augmented assignment ). There are a few different forms of augmented assignment, supporting different “update” operations. Here is the syntax for an addition-based augmented assignment:
When the Python interpreter executes the statement for numeric data , it does the following:
- Check that <variable> already exists (i.e., is assigned a value). If the variable doesn’t exist, a NameError is raised. Try it in the Python console!
- Evaluate <expression> .
- Add the value of <variable> and the value of <expression> from Step 2.
- Reassign <variable> to the new sum value produced in Step 3.
So for example, we could replace the line of code sum_so_far = sum_so_far + number with the augmented assignment statement to achieve the same effect:
Pretty cool! The Python programming language defines other forms of augmented assignment for different arithmetic operations: -= , *= , //= , %= , /= , and **= , although of these -= and *= are most common. We encourage you to try each of these out in the Python console, and keep them in mind when you are performing variable reassignment with numeric values.
Looking ahead: augmented assignment for other data types
You might have wondered about two subtleties in our introduction of augmented assignment. Why do we call this type of statement “augmented assignment” and not “augmented re assignment”? And why did we restrict our attention to only numeric data, when we know Python operators like + support other kinds of data types, like strings and lists?
It turns out that these augmented assignment operators like += behave differently for different data types, and in some cases do not actually reassign a variable. This might sound confusing at first, but leads into the next section, where we’ll discuss the other way of “changing a variable’s value”: object mutation . Let’s keep going!
Programming in Python
Variables and assignment.
Variables are one of the most important things in programming languages. They allow us to assign a name to an object so we can store it for use later on in our program.
1. Assignment
We assign an object to a variable using the assignment operator = . For example,
assigns 3.14 to the variable named pi .
We can then use the variable in our programs. Consider the following program,
this will print the value of the circumference of the circle with radius 6.
The point is that the line circumference = 2 * pi * radius now used the variables pi and radius to calculate the value of the circumference .
The following image illustrates the binding of the variables to the objects in memory. Note it isn't really how Python works internally, but it is good enough as a mental picture.

2. Reassignment
Python lets you reassign a variable to another object.
The following code,
will output,
because we reassigned the variable radius to the value 0 , but we did not recalculate the circumference .

3. Objects in Memory
Python stores objects in memory and when you assign a variable it binds that variable to the location of the object in memory.
You can find the unique id of an object by using the id() function. This refers to the object's memory address and will be different each time you run the program.
Input the following two lines into the terminal, remember to press enter after each line:
For now the id() function is a bit of a novelty. We will see later on that it can prove essential in debugging programs when we are editing mutable (changeable) types like lists.
Note that two objects can have the same id if one object has been removed from memory (Python automatically cleans up objects that are no longer used). Essentially the unique id is being re-used.
=== TASK ===
This should be relatively simple, consider it a freebie.
Copy the following code into a new Python file.
- Reassign radius to the value 15
- Reassign circumference to the circumference of the circule with radius 15
- In addition to the existing print statement, print out the new circumference.
The output of the program should be:
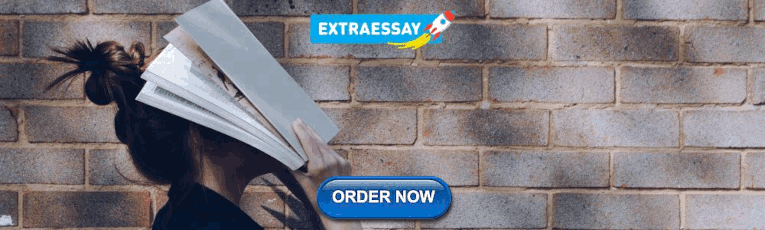
Can variables be reassigned to another data type?
After a variable is assigned to an initial value, can the variable later be reassigned to another value that is of a different data type than its initial value?
Yes, variables in Python can be reassigned to a new value that is a different data type from its current value. In fact, variables can be reassigned to any valid value in Python, regardless of its current value.
Variables are essentially like an empty box, that can contain something like a string, number, or other value. When you assign it a value, the box will contain that value, and when you reassign it, it will empty out the old value, and the new value will be placed inside of it.
Example code
Do we place a divider or How do we know that the value of the second variable will be taken?
The assignment operator, = (it is not an “equals sign”), does the work. Each time the Python interpreter sees this symbol, three things happen:
- the expression on the right side of the symbol is evaluated to obtain a value.
- that value is placed in a memory location
- the address of that location is assigned to the variable on the left of the symbol.
Once this is accomplished, the value is bound to the variable.
so can we define a variable a specific type? like var should be an integer type throughout the program. var = 10 And if any other type value is assigned to var it should throw an error stating type mismatch? var = ‘sleep’.
Not in Python! Python types variables at the time of assignment. Some people call it “duck typing,” as in, “if it walks like a duck…” etc.
You can reassign any variable at any time without error.
Declaration and definition are done at the same time?
Not explicitly. In Python, we do not declare variables. The assignment statement does everything: it tells the interpreter to evaluate the expression on the right, place its value in memory, and assign the memory address to the variable on the left.
The interpreter keeps track of the number of references to the address, and when that number is zero, the memory is released (actually, tagged for “garbage collection”). Any type of object can go in any address. Again, there is no explicit typing or variable declaration; it’s all “under the hood.”
- What is the life span of a variable in python?
- Is there any concept of variable that is not recently used is GC’d or something like that?
hi Patrickd , thank you for your answers
Yes, Python uses garbage collection. The life span is as long as it is referenced and then once it isn’t anymore however long it takes before the garbage collection gets it - as a side note, it is possible to run the GC manually.
One interesting life span is for default values of functions, they are evaluated once and not each time the function is called, so if you used something mutable you could have some weird side effects, like so:
Can you please clarify the concept of duck typing & duck test?
Jephos249 this was really helpful. Thank you.
A post was split to a new topic: What is that supposed to mean?
How can we ensure that we don’t accidentally perform operations on a variable after reassigning it to another data type? Are there any best practices for handling reassignment of variables in Python?
my question is - for example in the lesson there is meal = “english muffin” but later on it used again and provides next data with different wards so do you have to keep repeating it in that case if u gotta use it multiple times or u can say meal1 and for next one meal2 and meal 3? or it doesn’t matter because it knows the pattern/can tell what goes first what second? Is it better to lable them diffentet like “meal1” and so on , right?
Basic understanding would say that is only necessary when you want to keep the original value as well as a new one. So for instance, lets say you wanted to use multiple variables within a single sentence. You would then define each variable with a new command. Here is an article that will better explain it: Why change a variable rather than just create a new one? - Get Help / Python - Codecademy Forums
@swapnilb007 it’s colloquial, it means that Python looks at the value and determines the type of the variable based on what it notices as attributes - like the saying ‘If it walks like a duck and it quacks like a duck, then it must be a duck’.
I.e.: If the variable is ‘XXX’. Python looks at it and goes, well it has quotes around it, by all conventions it should be a string. Another example, if the variable is 42. Python looks at it and determines that without any other information based on the fact it’s numeric in absence of anything else it is probably numeric.
There’s a wikipedia artiicle that explicitly explains this in the context of Python 3:
P.S: Duck test is also in there in the wikipedia article.
@ajax3916882621 Python doesn’t declare variable types nor track variable types as other have said. The onus is really on you the programmer to make sure you keep track of the variables you created and how you use them. If you are reassigning variables often, it would be good to have a great name for it so that it’s not confusing when you reassign them in a program.
It’s not the biggest deal in the world. The worst that happens is that you run into an error and you go back and fix the variable.
Related topics

- Coding Fundamentals and Patterns
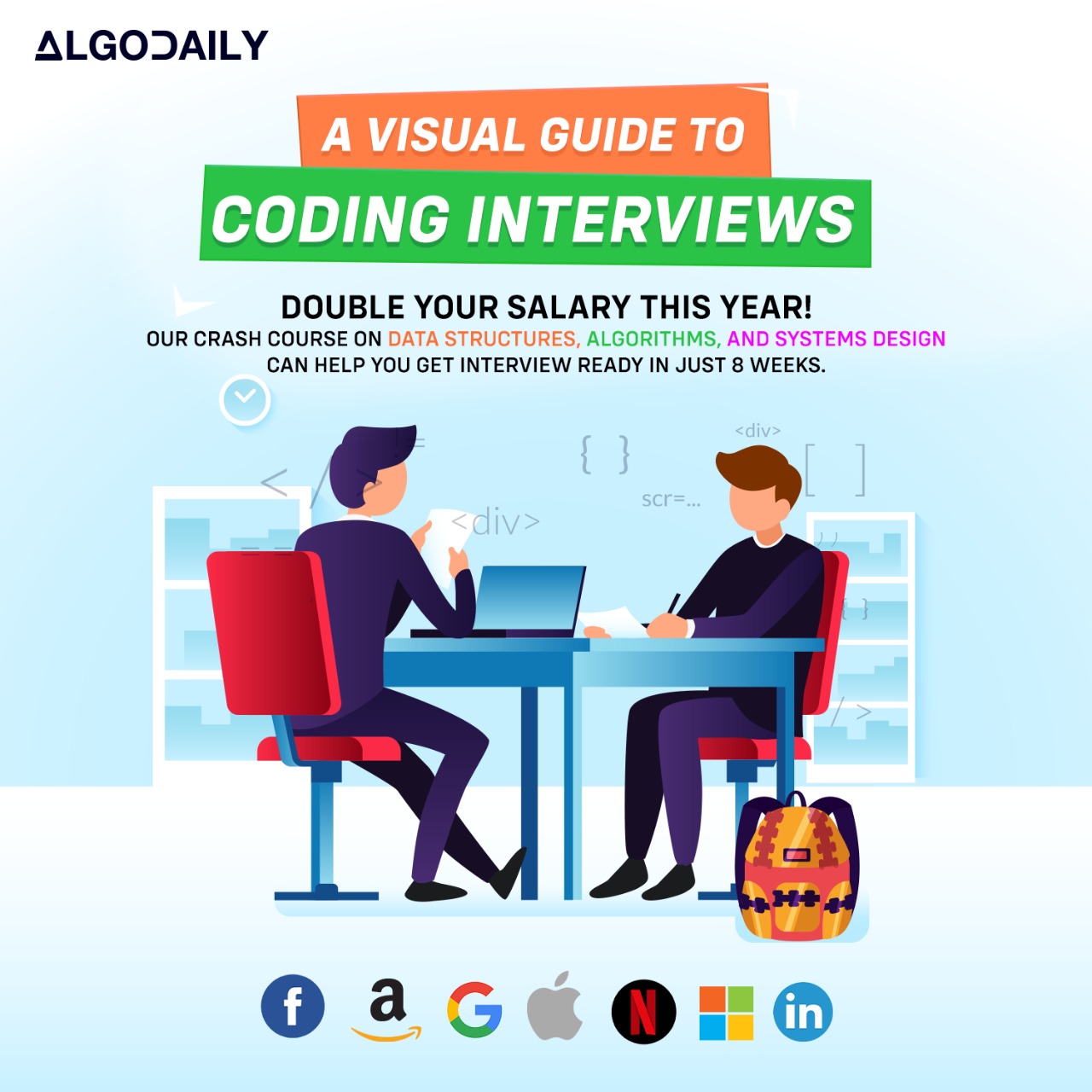
In this lesson, we will discuss the use of variables and assignment operators in Python, with a focus on the following key points:
- What are variables and the assignment of variables in the Python programming language?
- Working with variables and assignment of variables in an introductory programming language.
This is a general purpose tutorial for multiple languages. For the Javascript-specific version of this lesson that focuses on fundamentals frontend engineers need to know, please click here .
Throughout the day, we often need to store a piece of information somewhere and refer to it later.
We encounter these situations while writing a computer program as well, where we often need to store some information or data temporarily. For this specific purpose, variables are used. These variables can store almost any type of data, whether they are numbers, strings, or lists. Data is stored in variables by assign -ing data values to them. This lesson will expand on this topic and discuss variables and their assignment in detail.
As discussed above, variable s can store data. Data values can be assigned to a variable by using the assignment operator , which is the equal sign (=).
Once a value is assigned to the variable, it corresponds to that value unless it is re-assigned. Suppose we assign a value 5 to a variable a . This concept can be visualized as below.

This ensures that whenever we use the variable a in our program, we will get a value of 5. Let's understand this with code examples in Python.
Variables in Python
In Python, variables can be directly created by providing a variable name and assigning it some corresponding value. The code block below shows the creation of the variable number , which has the value 10 .
If we execute this code in a Python interpreter, it will give no output. Why?
Because this is a statement , and this statement did not tell the programming language to display the value of variable number . For that, we'd need to either print() it, or log it out in some other way.
However, it did create a variable number in memory and assigned it the value 10 . To check if the value was properly assigned, type either the variable name on the console, or use the print() function. It will display the variable name on the console.
The value that you stored earlier in number is successfully displayed, and we can see that it correctly assigned the value.
Important Rules while Creating Variables
Programming languages understand instructions only if they are given in a specified format (or syntax). When creating variables, we need to be careful of these rules, or the variables will not be created properly, and the program may give errors.
- Variable names can be created using alphabetical letters (a-z and A-Z), digits (0-9), or underscore symbol (_). Special symbols are not allowed. For example, abc&def is an invalid variable name.
- Variable names can begin with the underscore symbol or alphabetical letters only, not digits. For example, 123abc is an invalid variable name and will give you an error.
- Variables in Python are case-sensitive . This means that if we declare two variables score and Score with different values assigned to them, then they are two different variables (not the same variable!).
- Every programming language has certain keywords , that define in-built functionality in the language. These should not be used as variable names. Since it has a different meaning in the language, declaring variables with already existing keyword names will cause an error. These keywords will be highlighted with a different color whenever you type them, so it is easy for you to distinguish. For example, and is a keyword in Python, hence declaring a variable with that name would raise an error.
- In Python, it is important to assign a value to a variable. If you only define the variable without assigning it a value, it will produce an error.
Now that we know how to create variables, let's see if we understood them properly.
Let's test your knowledge. Is this statement true or false?
Is the following code snippet a valid way to declare a variable?
Press true if you believe the statement is correct, or false otherwise.
Are you sure you're getting this? Is this statement true or false?
Is _name a valid variable name?
Reassigning variables
Values can be reassigned to variables in Python. When variables are reassigned, their value changes to that of the newer value specified, and the previous value is lost.

Let's look at an example. We can reassign variable values in Python as follows.
The reassignment works similarly to how we create the variable. We only provide a different value than what was stored before. Here, the initial value of a was 10 , which was reassigned to 20 from the second line in the above code block.
Operations on Variables
Using variables in the program makes it easy for us to perform various operations on data. Different types of variables support different types of operations. For simplicity, let's consider the type integer. On this type of variable, all mathematical operations are valid.
Let's understand this with a code example.
This code block shows that if we have two integer variables, we can add them and get the result. Similarly, other mathematical operations such as subtraction, multiplication, and division are also supported.
Now let's consider if we have a variable of type string. In this case, the operations are defined differently. Here, a + symbol between two variables will join the two strings together.
Here, the two strings are joined together to produce a new string. This operation is called concatenation . There are several other operations that we can perform on strings, integers, and other types of variables, but we will discuss them in later lessons.
Time for some practice questions!
Build your intuition. Fill in the missing part by typing it in.
What will be the final value of variable a below?
Write the missing line below.
Build your intuition. Click the correct answer from the options.
What will the following program output?
Click the option that best answers the question.
- Will give an error
In this lesson, we talked about a basic concept in programming, about the creation of variables and assigning values to them. A useful tip for creating variables is to use meaningful names while creating them. If you have a lot of variables in your program and you don't name them properly, there is a high chance that you'll get confused about which variables were supposed to store what value!
One Pager Cheat Sheet
- This lesson will discuss variables and assignment of data values in Python for storing data temporarily.
- Variables are placeholders for data values that can be assigned using the assignment operator (=) and re-assigned when needed.
- Python can create variables directly by providing a name and assigning it a value, as shown in the code blocks, and then use the print() or variable name to display the stored value.
- We need to be careful to follow the specific syntax rules when creating variables, or they may not be created correctly and cause errors.
- Creating a variable in Python is done by having a variable name followed by an assignment operator (=) and a value , as is the case with the code snippet words = "Hello World!" , which is a valid way to declare the variable words .
- Yes, using an underscore (_) is a valid way to name variables in Python.
- Variables can be reassigned in Python, replacing their previous value with a newer one .
- We can perform different operations on each type of variable, such as mathematical operations on integers or concatenation of strings.
- The final value of a is "World" .
- The program prints the result of concatenating the values in the first_name and last_name variables, which is AnnaGreen .
- Creating meaningful variable names is key to being able to keep track of values stored in a program .

Programming Categories
- Basic Arrays Interview Questions
- Binary Search Trees Interview Questions
- Dynamic Programming Interview Questions
- Easy Strings Interview Questions
- Frontend Interview Questions
- Graphs Interview Questions
- Hard Arrays Interview Questions
- Hard Strings Interview Questions
- Hash Maps Interview Questions
- Linked Lists Interview Questions
- Medium Arrays Interview Questions
- Queues Interview Questions
- Recursion Interview Questions
- Sorting Interview Questions
- Stacks Interview Questions
- Systems Design Interview Questions
- Trees Interview Questions
Popular Lessons
- All Courses, Lessons, and Challenges
- Data Structures Cheat Sheet
- Free Coding Videos
- Bit Manipulation Interview Questions
- Javascript Interview Questions
- Python Interview Questions
- Java Interview Questions
- SQL Interview Questions
- QA and Testing Interview Questions
- Data Engineering Interview Questions
- Data Science Interview Questions
- Blockchain Interview Questions
- Introduction to C++ Projects
- Best Programming Projects for Resume
- C++ in Practice: Building a Finance Application
- Expanding Data Structure Capabilities
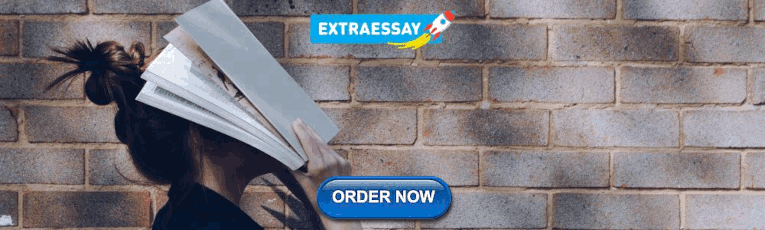
IMAGES
VIDEO
COMMENTS
Ok, you can think of variables in Python as references. When you do: a1 = a Both a1 and a are references to the same object, so if you modify the object pointed by a you will see the changes in a1, because - surprise - they are the same object (and the list.insert method mutates the list in place).. But when you do: a2 = a[:] Then a2 is a new list instance, and when you modify a you are not ...
Here is what reassignment looks like in a reference diagram: It is important to note that in mathematics, a statement of equality is always true. If a is equal to b now, then a will always equal to b. In Python, an assignment statement can make two variables refer to the same object and therefore have the same value. They appear to be equal.
Declaring Multiple Variables. We can define multiple variables in one line by following a comma separated syntax. Python also provides for the ease of saving the same value in more than one variable. Example. For example, let's create two variables, called mean and median, and save 5 and 7 in them, respectively.
Example 1: Reassigning a Variable # Initial value x = 5 # Reassigning the variable x = 10 # Print the updated value print(x) Output: 10 Example 2: Incrementing a Numeric Variable
Here is what reassignment looks like in a reference diagram: It is important to note that in mathematics, a statement of equality is always true. If a is equal to b now, then a will always equal to b. In Python, an assignment statement can make two variables refer to the same object and therefore have the same value. They appear to be equal.
6.1 Variable Reassignment, Revisited. So far, we have largely treated values and variables in Python as being constant over time. Once a variable is initialized, its value does not change as the program runs. ... A variable reassignment is a Python action that assigns a value to a variable when that variable already refers to a value. The most ...
The following depicts the reassignment of the variable radius. Image reproduced from Chapter 2: Introduction to Computation and Programming Using Python. 3. Objects in Memory. Python stores objects in memory and when you assign a variable it binds that variable to the location of the object in memory.
Not explicitly. In Python, we do not declare variables. The assignment statement does everything: it tells the interpreter to evaluate the expression on the right, place its value in memory, and assign the memory address to the variable on the left.. The interpreter keeps track of the number of references to the address, and when that number is zero, the memory is released (actually, tagged ...
Creating a variable in Python is done by having a variable name followed by an assignment operator (=) and a value, as is the case with the code snippet words = "Hello World!", which is a valid way to declare the variable words. Yes, using an underscore (_) is a valid way to name variables in Python.
One of the most common forms of reassignment is an update where the new value of the variable depends on the old. For example, x = x + 1. ... Remember that variables in Python are different from variables in math in that they (temporarily) hold values, but can be reassigned.