- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
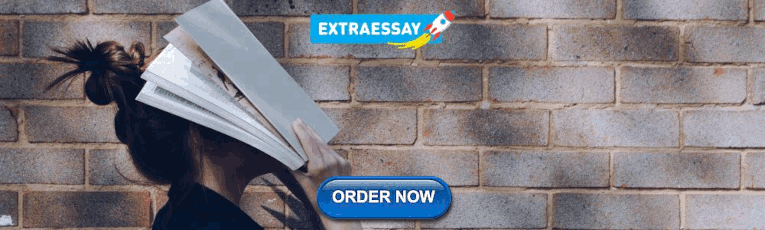
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
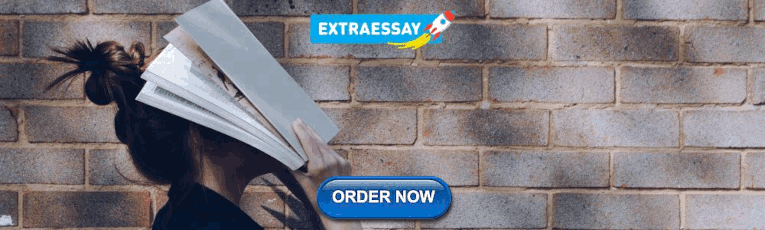
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
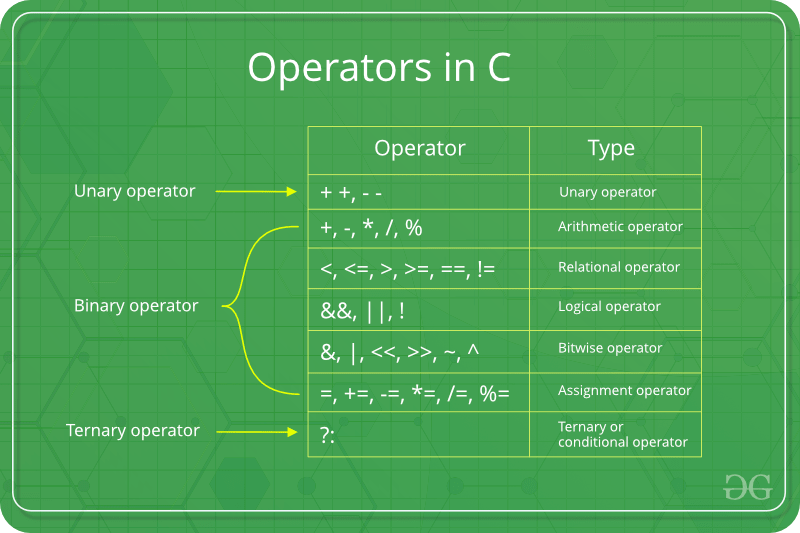
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
Conditional branching: if, '?'
Sometimes, we need to perform different actions based on different conditions.
To do that, we can use the if statement and the conditional operator ? , that’s also called a “question mark” operator.
The “if” statement
The if(...) statement evaluates a condition in parentheses and, if the result is true , executes a block of code.
For example:
In the example above, the condition is a simple equality check ( year == 2015 ), but it can be much more complex.
If we want to execute more than one statement, we have to wrap our code block inside curly braces:
We recommend wrapping your code block with curly braces {} every time you use an if statement, even if there is only one statement to execute. Doing so improves readability.
Boolean conversion
The if (…) statement evaluates the expression in its parentheses and converts the result to a boolean.
Let’s recall the conversion rules from the chapter Type Conversions :
- A number 0 , an empty string "" , null , undefined , and NaN all become false . Because of that they are called “falsy” values.
- Other values become true , so they are called “truthy”.
So, the code under this condition would never execute:
…and inside this condition – it always will:
We can also pass a pre-evaluated boolean value to if , like this:
The “else” clause
The if statement may contain an optional else block. It executes when the condition is falsy.
Several conditions: “else if”
Sometimes, we’d like to test several variants of a condition. The else if clause lets us do that.
In the code above, JavaScript first checks year < 2015 . If that is falsy, it goes to the next condition year > 2015 . If that is also falsy, it shows the last alert .
There can be more else if blocks. The final else is optional.
Conditional operator ‘?’
Sometimes, we need to assign a variable depending on a condition.
For instance:
The so-called “conditional” or “question mark” operator lets us do that in a shorter and simpler way.
The operator is represented by a question mark ? . Sometimes it’s called “ternary”, because the operator has three operands. It is actually the one and only operator in JavaScript which has that many.
The syntax is:
The condition is evaluated: if it’s truthy then value1 is returned, otherwise – value2 .
Technically, we can omit the parentheses around age > 18 . The question mark operator has a low precedence, so it executes after the comparison > .
This example will do the same thing as the previous one:
But parentheses make the code more readable, so we recommend using them.
In the example above, you can avoid using the question mark operator because the comparison itself returns true/false :
Multiple ‘?’
A sequence of question mark operators ? can return a value that depends on more than one condition.
It may be difficult at first to grasp what’s going on. But after a closer look, we can see that it’s just an ordinary sequence of tests:
- The first question mark checks whether age < 3 .
- If true – it returns 'Hi, baby!' . Otherwise, it continues to the expression after the colon “:”, checking age < 18 .
- If that’s true – it returns 'Hello!' . Otherwise, it continues to the expression after the next colon “:”, checking age < 100 .
- If that’s true – it returns 'Greetings!' . Otherwise, it continues to the expression after the last colon “:”, returning 'What an unusual age!' .
Here’s how this looks using if..else :
Non-traditional use of ‘?’
Sometimes the question mark ? is used as a replacement for if :
Depending on the condition company == 'Netscape' , either the first or the second expression after the ? gets executed and shows an alert.
We don’t assign a result to a variable here. Instead, we execute different code depending on the condition.
It’s not recommended to use the question mark operator in this way.
The notation is shorter than the equivalent if statement, which appeals to some programmers. But it is less readable.
Here is the same code using if for comparison:
Our eyes scan the code vertically. Code blocks which span several lines are easier to understand than a long, horizontal instruction set.
The purpose of the question mark operator ? is to return one value or another depending on its condition. Please use it for exactly that. Use if when you need to execute different branches of code.
if (a string with zero)
Will alert be shown?
Yes, it will.
Any string except an empty one (and "0" is not empty) becomes true in the logical context.
We can run and check:
The name of JavaScript
Using the if..else construct, write the code which asks: ‘What is the “official” name of JavaScript?’
If the visitor enters “ECMAScript”, then output “Right!”, otherwise – output: “You don’t know? ECMAScript!”
Demo in new window
Show the sign
Using if..else , write the code which gets a number via prompt and then shows in alert :
- 1 , if the value is greater than zero,
- -1 , if less than zero,
- 0 , if equals zero.
In this task we assume that the input is always a number.
Rewrite 'if' into '?'
Rewrite this if using the conditional operator '?' :
Rewrite 'if..else' into '?'
Rewrite if..else using multiple ternary operators '?' .
For readability, it’s recommended to split the code into multiple lines.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
AP®︎/College Computer Science Principles
Course: ap®︎/college computer science principles > unit 3, conditionals with if, else, and booleans.
- Nested conditionals
- Compound Booleans with logical operators
A simple conditional
Comparison operators, the else statement, conditionals in pseudocode, want to join the conversation.
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

Conditional Assignment Operator in Python
- Python How-To's
- Conditional Assignment Operator in …
Meaning of ||= Operator in Ruby
Implement ruby’s ||= conditional assignment operator in python using the try...except statement, implement ruby’s ||= conditional assignment operator in python using local and global variables.
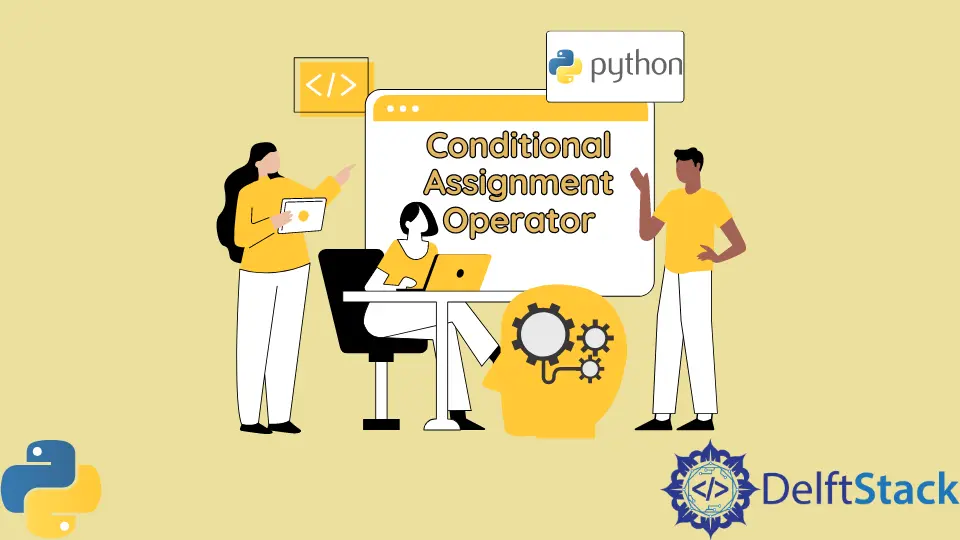
There isn’t any exact equivalent of Ruby’s ||= operator in Python. However, we can use the try...except method and concepts of local and global variables to emulate Ruby’s conditional assignment operator ||= in Python.
The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed.
But this operator is much more complex and confusing than other simpler conditional operators like += , -= because whenever any variable is encountered as undefined, the console throws out NameError .
a+=b evaluates to a=a+b .
a||=b looks as a=a||b but actually behaves as a||a=b .
We use try...except to catch and handle errors. Whenever the try except block runs, at first, the code lying within the try block executes. If the block of code within the try block successfully executes, then the except block is ignored; otherwise, the except block code will be executed, and the error is handled. Ruby’s ||= operator can roughly be translated in Python’s try-catch method as :
Here, if the variable x is defined, the try block will execute smoothly with no NameError exception. Hence, no assignment operation is performed. If x is not defined, the try block will generate NameError , then the except block gets executed, and variable x is assigned to 10 .
The scope of local variables is confined within a specific code scope, whereas global variables have their scope defined in the entire code space.
All the local variables in a particular scope are available as keys of the locals dictionary in that particular scope. All the global variables are stored as keys of the globals dictionary. We can access those variables whenever necessary using the locals and the globals dictionary.
We can check if a variable exists in any of the dictionaries and set its value only if it does not exist to translate Ruby’s ||= conditional assignment operator in Python.
Here, if the variable x is present in either global or local scope, we don’t perform any assignment operation; otherwise, we assign the value of x to 10 . It is similar to x||=10 in Ruby.
Related Article - Python Operator
- Python Bitwise NOT
- How to Unpack Operator ** in Python
- How to Overload Operator in Python
- Python Annotation ->
- The Walrus Operator := in Python

Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! 😊
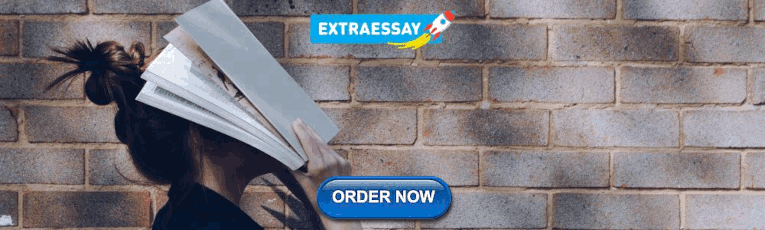
IMAGES
VIDEO
COMMENTS
"To avoid accidental misuse of a variable, it is usually a good idea to introduce the variable into the smallest scope possible. In particular, it is usually best to delay the definition of a variable until one can give it an initial value ... One of the most elegant applications of these two principles is to declare a variable in a conditional."
13 Answers. Answer. + 8. Yes you can put assignment operator (=) inside if conditional statement (C/C++) and its boolean type will be always evaluated to true since it will generate side effect to variables inside in it. And if you use equality relational operator (==) then its boolean type will be evaluated to true or false depending upon ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
condition. An expression that is considered to be either truthy or falsy.. statement1. Statement that is executed if condition is truthy.Can be any statement, including further nested if statements. To execute multiple statements, use a block statement ({ /* ... */ }) to group those statements.To execute no statements, use an empty statement.. statement2
Can assignment operator be used in if statements. As if statements are used Boolean expression [duplicate] Ask Question Asked 3 ... It is valid C to make an assignment inside of an if condition. The assignment evaluates to the assigned value. Everything else than an assigned value of 0 evaluates to a true condition. - RobertS supports Monica ...
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
Depending on the condition company == 'Netscape', either the first or the second expression after the ? gets executed and shows an alert. We don't assign a result to a variable here. Instead, we execute different code depending on the condition. It's not recommended to use the question mark operator in this way.
Conditionals with if, else, and Booleans. As humans, we make decisions every day, like what to eat for lunch or whether to wear a raincoat. When we make those decisions, we consider many conditions of our world, like the contents of our fridge for the lunch decision, or the weather outside for the raincoat decision.
Meaning of ||= Operator in Ruby. x ||= y. The basic meaning of this operator is to assign the value of the variable y to variable x if variable x is undefined or is falsy value, otherwise no assignment operation is performed. But this operator is much more complex and confusing than other simpler conditional operators like +=, -= because ...
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator. In this tutorial, we will look at different ways to assign values to a variable based on some condition. 1.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (? ), then an expression to execute if the condition is truthy followed by a colon (: ), and finally the expression to execute if the condition is falsy . This operator is frequently used as an alternative to an ...
I am aware that when a non zero value is provided as a condition in an if statement, the condition is evaluated to be true. Yet, I'm wondering how an assignment(=) is evaluated to be true here, instead of a comparison(==), and runs without any errors in the below C program.
A common use of the conditional expression is to select variable assignment. For example, suppose you want to find the larger of two numbers. Of course, there is a built-in function, max(), that does just this (and more) that you could use. But suppose you want to write your own code from scratch.
In the next section, we'll explore using assignment expressions inside of while loops.. Using Assignment Expressions in while Loops. Assignment expressions often work well in while loops because they allow us to fold more context into the loop condition.. Consider the following example that embeds a user input function inside the while loop condition:. while (directive := input ("Enter text ...
However, if we use some falsy value then the result will be entirely different. In that case a will contain that falsy value and b will remain untouched. Share. Improve this answer. ... Understanding conditional operator and assignment in condition in code snippet. 1. Assigning values inside conditional operator.
Can we use bash's conditional operator with assignment operators after colon? Bash reference manual explains the arithmetic operators as follows. ... The importance of this type-checking lies in the operator's most common use—in conditional assignment statements. In this usage it appears as an expression on the right side of an assignment ...
Costfoto/NurPhoto/AP. "Geomagnetic storms can impact infrastructure in near-Earth orbit and on Earth's surface, potentially disrupting communications, the electric power grid, navigation ...
The rule is not that "assignment can't be used in an if statement", but that "the condition in an if statement must be of type boolean". An assignment expression produces a value of the type being assigned, so Java only permits assignment in an if statement if you're assigning a boolean value.