- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
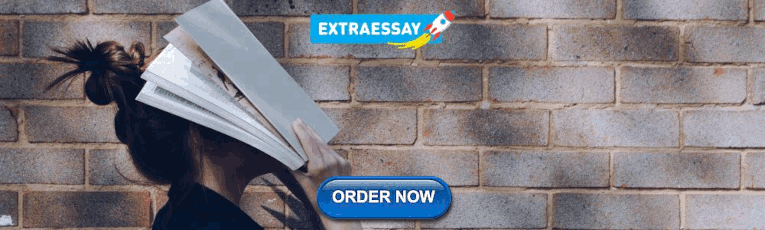
Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python Exercise: Practice makes you perfect in everything. This proverb always proves itself correct. Just like this, if you are a Python learner, then regular practice of Python exercises makes you more confident and sharpens your skills. So, to test your skills, go through these Python exercises with solutions.
Python is a widely used general-purpose high-level language that can be used for many purposes like creating GUI, web Scraping, web development, etc. You might have seen various Python tutorials that explain the concepts in detail but that might not be enough to get hold of this language. The best way to learn is by practising it more and more.
The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains. So if you are at any stage like beginner, intermediate or advanced this Python practice set will help you to boost your programming skills in Python.
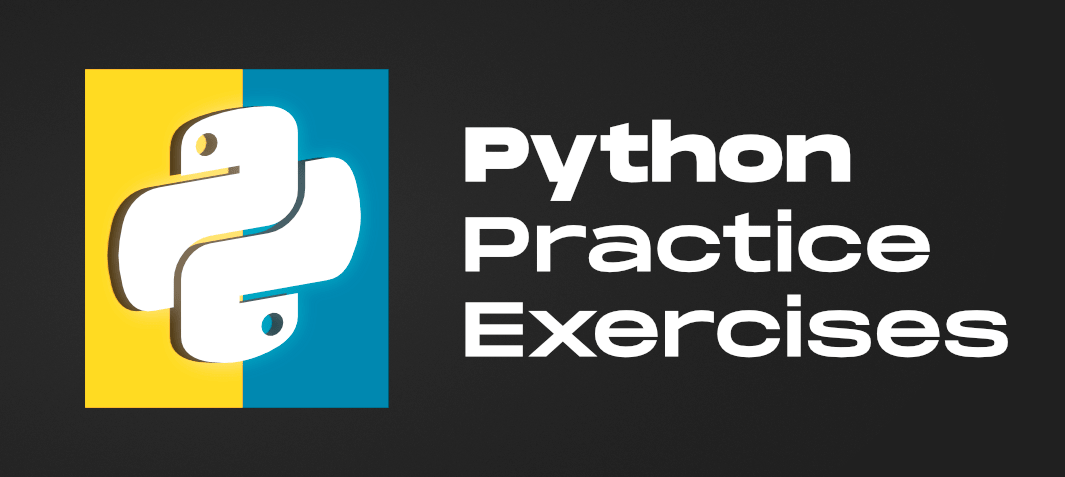
List of Python Programming Exercises
In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set.
Python List Exercises
- Python program to interchange first and last elements in a list
- Python program to swap two elements in a list
- Python | Ways to find length of list
- Maximum of two numbers in Python
- Minimum of two numbers in Python
>> More Programs on List
Python String Exercises
- Python program to check whether the string is Symmetrical or Palindrome
- Reverse words in a given String in Python
- Ways to remove i’th character from string in Python
- Find length of a string in python (4 ways)
- Python program to print even length words in a string
>> More Programs on String
Python Tuple Exercises
- Python program to Find the size of a Tuple
- Python – Maximum and Minimum K elements in Tuple
- Python – Sum of tuple elements
- Python – Row-wise element Addition in Tuple Matrix
- Create a list of tuples from given list having number and its cube in each tuple
>> More Programs on Tuple
Python Dictionary Exercises
- Python | Sort Python Dictionaries by Key or Value
- Handling missing keys in Python dictionaries
- Python dictionary with keys having multiple inputs
- Python program to find the sum of all items in a dictionary
- Python program to find the size of a Dictionary
>> More Programs on Dictionary
Python Set Exercises
- Find the size of a Set in Python
- Iterate over a set in Python
- Python – Maximum and Minimum in a Set
- Python – Remove items from Set
- Python – Check if two lists have atleast one element common
>> More Programs on Sets
- Python – Assigning Subsequent Rows to Matrix first row elements
- Python – Group similar elements into Matrix
>> More Programs on Matrices
>> More Programs on Functions
- Python | Find the Number Occurring Odd Number of Times using Lambda expression and reduce function
>> More Programs on Lambda
- Programs for printing pyramid patterns in Python
>> More Programs on Python Pattern Printing
- Python program to get Current Time
- Get Yesterday’s date using Python
- Python program to print current year, month and day
- Python – Convert day number to date in particular year
- Get Current Time in different Timezone using Python
>> More Programs on DateTime
>> More Programs on Python OOPS
- Python – Check if String Contain Only Defined Characters using Regex
>> More Programs on Python Regex
>> More Programs on Linked Lists
>> More Programs on Python Searching
- Python Program for Bubble Sort
- Python Program for QuickSort
- Python Program for Insertion Sort
- Python Program for Selection Sort
- Python Program for Heap Sort
>> More Programs on Python Sorting
- Program to Calculate the Edge Cover of a Graph
- Python Program for N Queen Problem
>> More Programs on Python DSA
- Read content from one file and write it into another file
- Write a dictionary to a file in Python
- How to check file size in Python?
- Find the most repeated word in a text file
- How to read specific lines from a File in Python?
>> More Programs on Python File Handling
- Update column value of CSV in Python
- How to add a header to a CSV file in Python?
- Get column names from CSV using Python
- Writing data from a Python List to CSV row-wise
>> More Programs on Python CSV
>> More Programs on Python JSON
- Python Script to change name of a file to its timestamp
>> More Programs on OS Module
- Python | Create a GUI Marksheet using Tkinter
- Python | ToDo GUI Application using Tkinter
- Python | GUI Calendar using Tkinter
- File Explorer in Python using Tkinter
- Visiting Card Scanner GUI Application using Python
>> More Programs on Python Tkinter
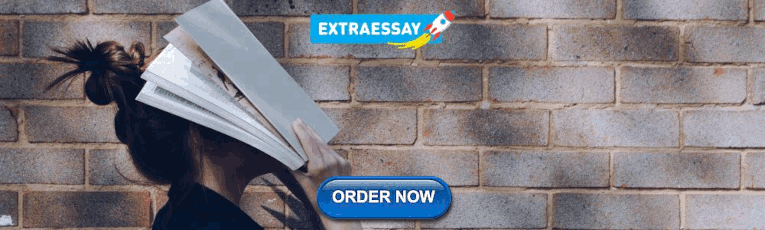
NumPy Exercises
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Replace NumPy array elements that doesn’t satisfy the given condition
- Get the maximum value from given matrix
>> More Programs on NumPy
Pandas Exercises
- Make a Pandas DataFrame with two-dimensional list | Python
- How to iterate over rows in Pandas Dataframe
- Create a pandas column using for loop
- Create a Pandas Series from array
- Pandas | Basic of Time Series Manipulation
>> More Programs on Python Pandas
>> More Programs on Web Scraping
- Download File in Selenium Using Python
- Bulk Posting on Facebook Pages using Selenium
- Google Maps Selenium automation using Python
- Count total number of Links In Webpage Using Selenium In Python
- Extract Data From JustDial using Selenium
>> More Programs on Python Selenium
- Number guessing game in Python
- 2048 Game in Python
- Get Live Weather Desktop Notifications Using Python
- 8-bit game using pygame
- Tic Tac Toe GUI In Python using PyGame
>> More Projects in Python
In closing, we just want to say that the practice or solving Python problems always helps to clear your core concepts and programming logic. Hence, we have designed this Python exercises after deep research so that one can easily enhance their skills and logic abilities.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
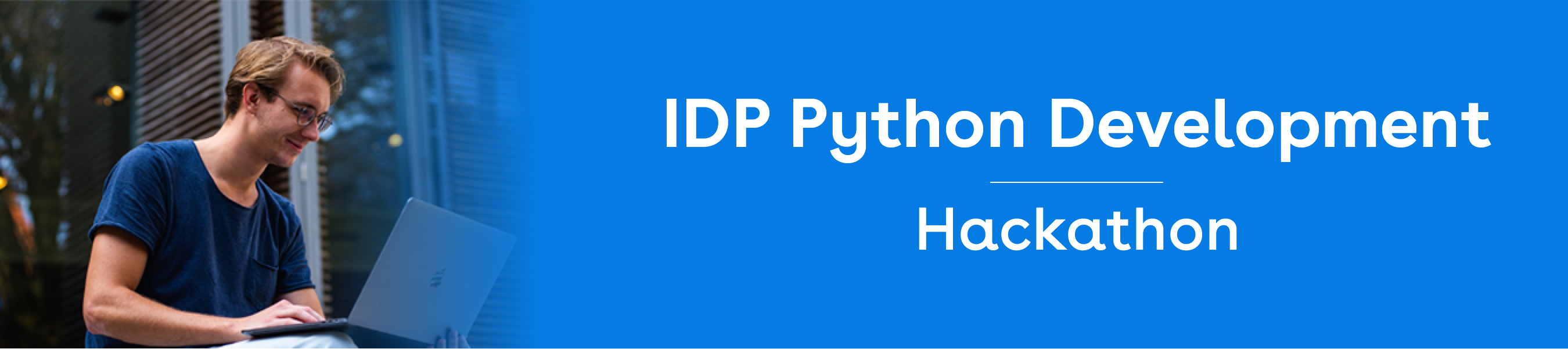
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
python-practice-questions
Here are 14 public repositories matching this topic..., laibanasir / python-assignments.
Mini python programs for practice and better understanding
- Updated Jul 16, 2019
sagargoswami2001 / Python-Conditional-Statement-Programs
This is a Python Basic Conditional Statement Programs.
- Updated Jan 21, 2023
JRudransh / Python_Bits
Python basic project for practice. It contains lots of python programme, best for beginners.
- Updated Sep 29, 2022
atchyutn / hackerrank-python-solutions
My Hacker Rank python solutions
- Updated Aug 18, 2018
atharvaagrawal / Python-Challenges
This is Python Practice repository for Python Challenges Solver
- Updated Apr 2, 2020
ProjectWorst / Practice-Exam-with-MySQL-Database
While studying for my PMP exam, I wanted to create a practice exam with Python. This program is for any practice exam and not just the PMP. Your practice exam would depend on the database/API you use within the program. I made this program to pull data from a MySQL database. With a few changes, you could include any database/API as you wish.
- Updated Jun 21, 2023
prak112 / python_practice
Solutions for DailyCodingProblems & HackerRank
- Updated Oct 12, 2023
laibanasir / code-fest
10 python tasks
- Updated Jul 15, 2019
Noorain-Raza-coder / Python-ControlFlow-Problems
In this file, I've tackled 70 problems across various difficulty levels, including basic, medium, advanced, and challenging. Through solving these problems, I've significantly enhanced my proficiency in control flow techniques.
- Updated Mar 29, 2024
- Jupyter Notebook
Noorain-Raza-coder / Python-ForLoop-ListComprehension
In this file, I have solved 40 Python problems, with 10 on each level: Basic, Intermediate, Hard, and Challenge. After solving these problems, I understood some of the concepts of list comprehension in depth, such as how to write nested loops, if-else conditions, and nested if conditions in list comprehension.
- Updated Apr 23, 2024
Noorain-Raza-coder / Python-Map-Reduce-Filter-Recursion
In this assignment, I solved 106 Questions on some of the concept of python such as map, reduce, filter and recursion with proper step by step explanation. I have also give the answer of some theoretical questions.
- Updated Apr 2, 2024
Noorain-Raza-coder / Python-Data-Types-Assignment
In this assignment, I solved 200 python problems on Python data types i.e List, Tuple, String and Set. After Solving these problems I have cleared all fundamentals of python data types.
- Updated Mar 15, 2024
sci-c0 / exercism-learning
Learning and Problem Solving from exercism.io
- Updated Oct 5, 2021
Sanushi-Salgado / HackerRank-Python-Coding-Challenges
- Updated Jul 1, 2020
Improve this page
Add a description, image, and links to the python-practice-questions topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the python-practice-questions topic, visit your repo's landing page and select "manage topics."
NxtWave Help Center
If you still have questions or prefer to get help directly from an agent, please contact Nxtwave learner support(help) here They’ll get back to you as soon as possible.
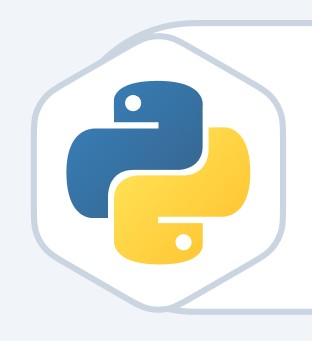
- Chat with us
- Python IDP Exam
- Exam Details
How to clear/crack Python the IDP Test?
To prepare for the Python IDP, we suggest the following steps:
Review all the concepts in the Python Programming Foundations course up to Foundation Exam 4. Solve the Python IDP Prep Series to improve your problem-solving skills even further. Check out the "Cracking IDP" course in Tech Foundation and attend problem-solving sessions we conducted to get a better understanding of the types of problems you might encounter. You can also practice previous Python IDP questions available here: https://bit.ly/40qMzvC. We hope these resources will help you succeed in the Python IDP. Good luck!
Related Articles
- How many times can I attempt the test?
- Will the coming IDP tests have the same questions?
- What is the importance of Python IDP Mock Practice Test?
- Is it possible to take Python IDP Tests during pause?
- How it works
- Homework answers
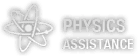
Answer to Question #255529 in Python for GANESH
How to reverse a string in a given sentence. a. Example: Given string is “ Ram is learning in CCBP Intensive course”. Reverse the word “learning” in the above sentence
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. import numpy as npdef matrix(m,n): list1 = [] list2 = [] for i in range(m*n): inputs = int(input(
- 2. Recreate the pokemon table using Colum by column on the documentation:Column by columnyou can add da
- 3. Recreate the pokemon table using All rows at once on the documentation:All rows at onceWhen you have
- 4. Create a program to develop a Regex that will find all website URLS that begin with http://www or ht
- 5. Given an M x N matrix find all anti-diagonal elements of the matrix..input :the first input will con
- 6. The Airplane always needs to check with the Airport to see if it has anavailable runway before it
- 7. MT_ACT1: Object BasicsINSTRUCTIONS1. Define a class bicycle2. Properties: brand, model, year, descri
- Programming
- Engineering
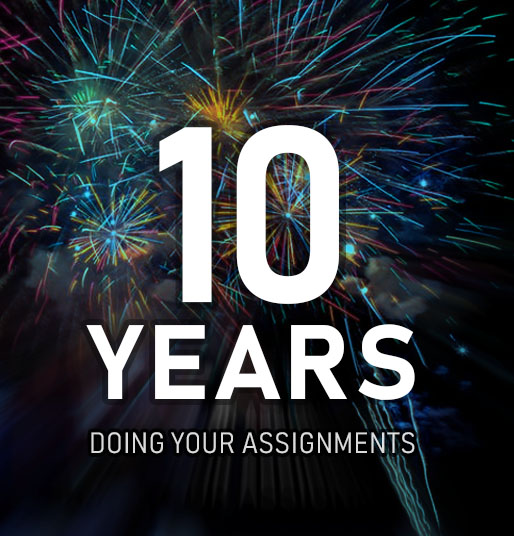
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
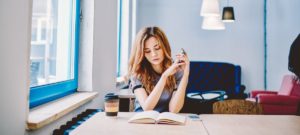
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
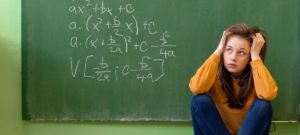
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
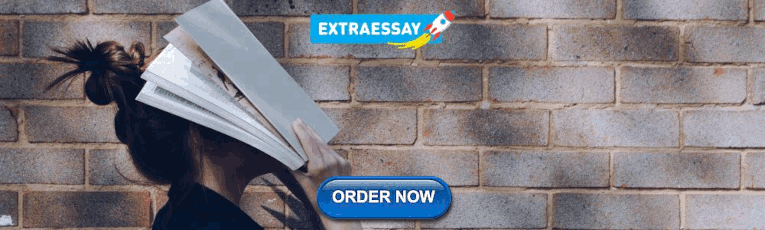
IMAGES
VIDEO
COMMENTS
Test Match 1 : Dhoni : 56 , Balaji : 94. Test Match 2 : Balaji : 80 , Dravid : 105. Calculate the highest number of runs scored by an individual cricketer in both of the matches. Create a python function Max_Score (M) that reads a dictionary M that recognizes the player with the highest total score. This function will return ( Top player ...
How many questions will be there in Python IDP Level - 1? My Python IDP test is not opening. What should I do? Is it okay to use a pen and paper or rough sheets during the test? How can I see my score? When will you conduct the Python IDP exam again? Is it mandatory to clear the Python IDP level 1 and 2 exams?
Instantly Download or Run the code at https://codegive.com title: a comprehensive guide to python idp test questions with assignment expertpython idp (inter...
The input is 5. In the first row, we need to print N+1 underscores i.e.,6. After the row with underscores, we need to print the 5 rows. For every row from 2nd row to Nth row i.e; 5 starts with | and ends with /. can you provide python code for this ? thanks in advance. for i in range(N+1): print ( '_', end = '' )
The Python IDP test plays an important role in testing the candidate ' s programming skills. Here you will have Python IDP Level - 1 and Level - 2 tests. To qualify for the Python IDP Level - 1 test, you must finish growth cycle 1 and foundation exam 4, while for the Level - 2 test, you must pass the Python IDP level - 1 test ( score at least ...
Packages. No packages published. Next wave python idp series 1 answers. Contribute to SantoshNarasimha/idp-set-1 development by creating an account on GitHub.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
The Python IDP Level - 1 test details will be displayed on the placement preparation page. Whereas, Level - 2 test details will be shared with you on Announcements section or to your email id once you clear Python IDP Level - 1(Score atleast 75). Note: 1. The tests will be accessible only during the scheduled times. 2. Attempting this Mock ...
#idp #nxtwave #nxtwaveccbp #exam #techcareer #ccbp #pass #howto @tejabhaiupdates @NxtWaveTech @youtubecreators @YouTube @YouTubeViewers డిజిటల్ మీడియా రంగంల...
Our Teligram group Link 🔗https://t.me/shivatechcareerhub Instagram id : https://instagram.com/mr_chiva?igshid=YmMyMTA2M2Y=Cal me on Calme4 app:id: shivapra...
Python. Question #334308. SNAKY CONVERSION. you are given a string S print the string in N rows containing a snaky pattern represenation as described below. INPUT:the first line should be a string. explanation: s=AWESOMENESS N=4. consider the following snaky representation of the word. A E.
Here, you will have the opportunity to take the Python IDP Level 1 and Level 2 tests. To qualify for the Python IDP Level 1 test, you must complete the following: Growth Cycle 1; Foundation Exam 4; IDP Prep Series Level 1; To qualify for the Python IDP Level 2 test, you must pass the Python IDP Level 1 test with a score of at least 75.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
#idp #nxtwave #nxtwaveccbp #exam #techcareer #ccbp #pass #howto Build Logic in Programing Video link :- https://youtu.be/EiD8XI3lyRs@tejabhaiupdates @YouTube...
About US : An ASX100 business part that is owned by Australian universities, IDP is a pioneer in international education services. Our core business lines include student placement to Australia, US, UK, Canada and New Zealand institutions, English-language testing and training. IDP Digital Campus in Chennai is the engine room that drives innovation and customer-led product design.
11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle. 11.2 Create an instance of the triangle class and call all the defined methods. 11.3 Create three child classes of triangle class - isosceles_triangle, right_triangle and equilateral_triangle. 11.4 Define methods which check for their properties.
To associate your repository with the python-practice-questions topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
Here you will have Python IDP Level - 1 and Level - 2 tests. The pattern of the Python IDP Level 1 test is as follows: Eligibility: Finish growth cycle 1 and foundation exam 4 and python IDP practice series; No. Of questions: 4; Duration: 75 min; Total marks: 100; Pass marks: 75; The pattern of the Python IDP Level 2 test is as follows:
Create a program in Python to calculate grade point averages for college students. Grade point avera; 2. For this problem, the prefilled code will contain a list of tuples. Write a program to replace the l; 3. Consider the following Python function.def mystery(l): if l == []: return(l) else: 4.
How many questions will be there in Python IDP Level - 2? Does a laptop mandatory to take Python IDP Tests? Do we have any specific classes or preparation guides to clear/crack the Python IDP Test? Why is my previous Python IDP score showing as Python IDP level 1? test.
Check out the "Cracking IDP" course in Tech Foundation and attend problem-solving sessions we conducted to get a better understanding of the types of problems you might encounter. You can also practice previous Python IDP questions available here: https://bit.ly/40qMzvC. We hope these resources will help you succeed in the Python IDP. Good luck!
Cricket Stadium | Find Available Spots for N Friends to Sit Together | CCBP Python IDP Exam Question AnswerA group of N friends are going to see a cricket ma...
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter… How to Finish Assignments When You Can't. Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.… How to Effectively Study for a Math Test