Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
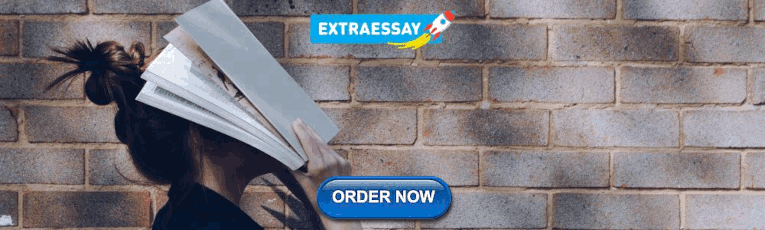
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, introduction.
- Getting Started with C
- Your First C Program
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
Programming Strings
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
Structure and Union
C structs and Pointers
C Structure and Function
Programming Files
- C File Handling
- C Files Examples
Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Struct Examples
- Add Two Complex Numbers by Passing Structure to a Function
- Add Two Distances (in inch-feet system) using Structures
In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name.
- Define Structures
Before you can create structure variables, you need to define its data type. To define a struct, the struct keyword is used.
Syntax of struct
For example,
Here, a derived type struct Person is defined. Now, you can create variables of this type.
Create struct Variables
When a struct type is declared, no storage or memory is allocated. To allocate memory of a given structure type and work with it, we need to create variables.
Here's how we create structure variables:
Another way of creating a struct variable is:
In both cases,
- person1 and person2 are struct Person variables
- p[] is a struct Person array of size 20.
Access Members of a Structure
There are two types of operators used for accessing members of a structure.
- . - Member operator
- -> - Structure pointer operator (will be discussed in the next tutorial)
Suppose, you want to access the salary of person2 . Here's how you can do it.
Example 1: C structs
In this program, we have created a struct named Person . We have also created a variable of Person named person1 .
In main() , we have assigned values to the variables defined in Person for the person1 object.
Notice that we have used strcpy() function to assign the value to person1.name .
This is because name is a char array ( C-string ) and we cannot use the assignment operator = with it after we have declared the string.
Finally, we printed the data of person1 .
- Keyword typedef
We use the typedef keyword to create an alias name for data types. It is commonly used with structures to simplify the syntax of declaring variables.
For example, let us look at the following code:
We can use typedef to write an equivalent code with a simplified syntax:
Example 2: C typedef
Here, we have used typedef with the Person structure to create an alias person .
Now, we can simply declare a Person variable using the person alias:
- Nested Structures
You can create structures within a structure in C programming. For example,
Suppose, you want to set imag of num2 variable to 11 . Here's how you can do it:
Example 3: C Nested Structures
Why structs in c.
Suppose you want to store information about a person: his/her name, citizenship number, and salary. You can create different variables name , citNo and salary to store this information.
What if you need to store information of more than one person? Now, you need to create different variables for each information per person: name1 , citNo1 , salary1 , name2 , citNo2 , salary2 , etc.
A better approach would be to have a collection of all related information under a single name Person structure and use it for every person.
More on struct
- Structures and pointers
- Passing structures to a function
Table of Contents
- C struct (Introduction)
- Create struct variables
- Access members of a structure
- Example 1: C++ structs
Sorry about that.
Related Tutorials
5.3. Structures
- Declaration and definition
- Stack And Heap
- new And delete
- Dot And Arrow
Programmers often use the term "structure" to name two related concepts. They form structure specifications with the struct keyword and create structure objects with a variable definition or the new operator. We typically call both specifications and objects structures, relying on context to clarify the the specific usage.
Structures are an aggregate data type whose contained data items are called fields or members . Once specified, the structure name becomes a new data type or type specifier in the program. After the program creates or instantiates a structure object, it accesses the fields with one of the selection operators . Referring to the basket analogy suggested previously, a structure object is a basket, and the fields or members are the items in the basket. Although structures are more complex than the fundamental types, programmers use the same pattern when defining variables of either type.
Structure Specifications: Fields And Members
A program must specify or declare a structure before using it. The specification determines the number, the type, the name, and the order of the data items contained in the structure.
Structure Definitions And Objects
We can use a blueprint as another analogy for a structure. Blueprints show essential details like the number and size of bedrooms, the location of the kitchen, etc. Similarly, structure specifications show the structure fields' number, type, name, and order. Nevertheless, a blueprint isn't a house; you can't live in it. However, given the blueprint, we can make as many identical houses as we wish, and while each one looks like the others, it has a different, distinct set of occupants and a distinct street address. Similarly, each object created from a structure specification looks like the others, but each can store distinct values and has a distinct memory address.
- C++ code that defines three new student structure variables or objects named s1 , s2 , and s3 . The program allocates the memory for the three objects on the stack.
- The pointer variables s4 , s5 , and s6 are defined and allocated automatically on the stack. However, the structure objects they point to are allocated dynamically on the heap.
- An abstract representation of three student objects on the stack. Each object has three fields.
- An abstract representation of three student pointer variables pointing to three student objects allocated dynamically on the heap with the new operator.
Initializing Structures And Fields
Figure 3 demonstrates that we are not required to initialize structure objects when we create them - perhaps the program will read the data from the console or a data file. However, we can initialize structures when convenient. C++ inherited an aggregate field initialization syntax from the C programming language, and the 2011 ANSI standard extends that syntax, making it conform to other major programming languages. More recently, the ANSI 2020 standard added a variety of default field initializations. The following figures illustrate a few of these options.
- The field order within the structure and the data order within the initialization list are significant and must match. The program saves the first list value in the first structure field until it saves the last list value in the last structure field. If there are fewer initializers (the elements inside the braces) than there are fields, the excess fields are initialized to their zero-equivalents . Having more initializers than fields is a compile-time error.
- Similar to (a), but newer standards no longer require the = operator.
- Historically, en block structure initialization was only allowed in the structure definition, but the ANSI 2015 standard removed that restriction, allowing en bloc assignment to previously defined variables.
- Abstract representations of the structure objects or variables created and initialized in (a), (b), and (c). Each object has three fields. Although every student must have these fields, the values stored in them may be different.
- Another way to conceptualize structures and variables is as the rows and columns of a simple database table. Each column represents one field, and each row represents one structure object. The database schema fixes the number of columns, but the program can add any number of rows to the table.
- Initializing a structure object when defining it. The = operator is now optional.
- Designated initializers are more flexible than the non-designated initializers illustrated in Figure 4, which are matched to structure fields by position (first initializer to the first field, second initializer to the second field, etc.). Designated initializers may skip a field - the program does not initialize name in this example.
- Programmers may save data to previously defined structure objects, possibly skipping some fields, with designators.
The next step is accessing the individual fields within a structure object.
Field Selection: The Dot And Arrow Operators
A basket is potentially beneficial, but so far, we have only seen how to put items into it, and then only en masse . To achieve its full potential, we need some way to put items into the basket one at a time, and there must be some way to retrieve them. C++ provides two selection operators that join a specific structure object with a named field. The combination of an object and a field form a variable whose type matches the field type in the structure specification.
The Dot Operator
The C++ dot operator looks and behaves just like Java's dot operator and performs the same tasks in both languages.
- The general dot operator syntax. The left-hand operand is a structure object, and the right-hand operator is the name of one of its fields.
- Examples illustrating the dot operator based on the student examples in Figure 3(a).
- The dot operator's left operand names a specific basket or object, and the right-hand operand names the field. If we view the structure as a table, the left-hand operand selects the row, and the right-hand operand selects the column. So, we can visualize s2 . name as the intersection of a row and column.
The Arrow Operator
Java only has one way of creating objects and only needs one operator to access its fields. C++ can create objects in two ways (see Figure 3 above) and needs an additional field access operator, the arrow: -> .
- The general arrow operator syntax. The left-hand operand is a pointer to a structure object, and the right-hand operator is the name of one of the object's fields.
- Examples illustrate the arrow operator based on the student examples in Figure 3(b).
Choosing the correct selection operator

- Choose the arrow operator if the left-hand operand is a pointer.
- Otherwise, choose the dot operator.
Moving Structures Within A Program
A basket is a convenient way to carry and move many items by holding its handle. Similarly, a structure object is convenient for a program to "hold" and move many data items with a single (variable) name. Specifically, a program can assign one structure to another (as long as they are instances of the same structure specification), pass them as arguments to functions, and return them from functions as the function's return value.
Assignment is a fundamental operation regardless of the kind of data involved. The C++ code to assign one structure to another is simple and should look familiar:
- C++ code defining a new structure object and copying an existing object to it by assignment.
- An abstract representation of a new, empty structure.
- An abstract representation of how a program copies the contents of an existing structure to another structure.
Function Argument
Once the program creates a structure object, passing it to a function looks and behaves like passing a fundamental-type variable. If we view the function argument as a new, empty structure object, we see that argument passing copies one structure object to another, behaving like an assignment operation.
- The first part of the C++ code fragment defines a function (similar to a Java method). The argument is a student structure. The last statement (highlighted) passes a previously created and initialized structure as a function argument.
- In this abstract representation, a program copies the data stored in s2 to the function argument temp . The braces forming the body of the print function create a new scope distinct from the calling scope where the program defines s2 . The italicized variable names label and distinguish the illustrated objects.
Function Return Value
Just as a program can pass a structure into a function as an argument, so it can return a structure as the function's return value, as demonstrated by the following example:
- C++ code defining a function named read that returns a structure. The program defines and initializes the structure object, temp , in local or function scope with a series of console read operations and returns it as the function return value. The assignment operator saves the returned structure in s3 .
- A graphical representation of the return process. Returning an object with the return operator copies the local object, temp , to the calling scope, where the program saves it in s1 .
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Assignment Operators
- 6 contributors
An assignment operation assigns the value of the right-hand operand to the storage location named by the left-hand operand. Therefore, the left-hand operand of an assignment operation must be a modifiable l-value. After the assignment, an assignment expression has the value of the left operand but isn't an l-value.
assignment-expression : conditional-expression unary-expression assignment-operator assignment-expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators:
In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions .
- Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default .
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The implicitly-declared copy assignment operator for class T is trivial if all of the following is true:
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std:: memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std:: memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
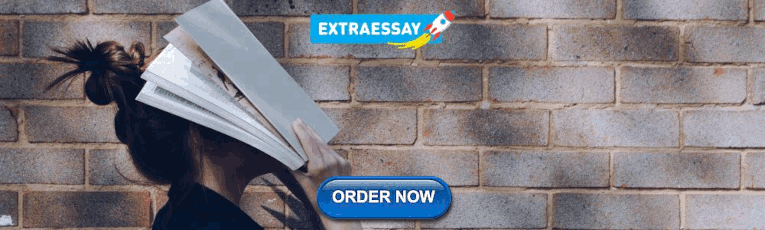
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std:: move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 15:13.
- This page has been accessed 1,333,785 times.
- Privacy policy
- About cppreference.com
- Disclaimers


21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- The operator is not user-provided (meaning, it is implicitly-defined or defaulted), and if it is defaulted, its signature is the same as implicitly-defined
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std::move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Assignment Operators In C++
- Move Assignment Operator in C++ 11
- JavaScript Assignment Operators
- Assignment Operators in Programming
- Is assignment operator inherited?
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- bitset operator[] in C++ STL
- C++ Assignment Operator Overloading
- Self assignment check in assignment operator
- Copy Constructor vs Assignment Operator in C++
- Operators in C++
- C++ Arithmetic Operators
- Bitwise Operators in C++
- Casting Operators in C++
- How to Create Custom Assignment Operator in C++?
- Default Assignment Operator and References in C++
- How to Implement Move Assignment Operator in C++?
- vector :: assign() in C++ STL
- Operators in LISP
- Assignment Operators in C
- Assignment Operators in Python
- Compound assignment operators in Java
- Arithmetic Operators in C
- Operators in C
- Basic Operators in Java
- Null-Coalescing Assignment Operator in C# 8.0
- JavaScript Logical OR assignment (||=) Operator
- Java Assignment Operators with Examples
- Parallel Assignment in Ruby
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Geeks Premier League

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
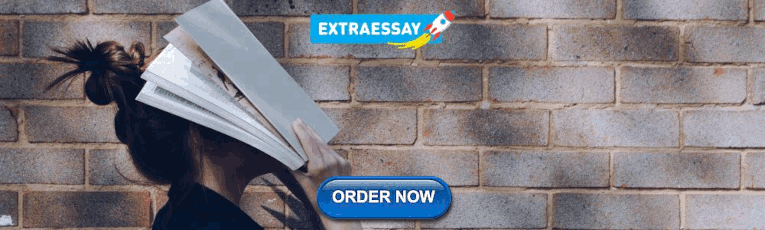
COMMENTS
Assignment operator - Called when one struct/class is assigned to another. This is the automatically generated method that's being called in the above case. Share. ... The assignment operator (operator=) is one of the implicitly generated functions for a struct or class in C++.
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
In this tutorial, you'll learn about struct types in C Programming. You will learn to define and use structures with the help of examples. In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name. ... and we cannot use the assignment operator = with it after we have declared the ...
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
The assignment operator works with structures. Used with structure operands, the assignment operator copies a block of bits from one memory address (the address of the right-hand object) to another (the address of the left-hand object). C++ code defining a new structure object and copying an existing object to it by assignment.
C Structures. The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: | =. In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place.
In those situations where copy assignment cannot benefit from resource reuse (it does not manage a heap-allocated array and does not have a (possibly transitive) member that does, such as a member std::vector or std::string), there is a popular convenient shorthand: the copy-and-swap assignment operator, which takes its parameter by value (thus working as both copy- and move-assignment ...
Code language:C++(cpp) The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand. Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
The assignment operator. The assignment operator. For any struct or class, we may sometimes need to use the assignment operator, as in "A = B". There is a default assignment operator provided by C++, but it is not always adequate. We may therefore sometimes need to provide our own assignment operator. Simple example of an assignment operator.
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
C17 6.5.16: An assignment operator stores a value in the object designated by the left operand. An assignment expression has the value of the left operand after the assignment, but is not an lvalue. The type of an assignment expression is the type the left operand would have after lvalue conversion. (Lvalue conversion in this case isn't ...
21.12 — Overloading the assignment operator. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
@gabeappleton Struct assignment always replace left side struct with the data from right side. On the right side of your sample is structure with designated initializer which missing fields will be initialized to zero by default. And then s1 will be replaced with content of new structure. So id field will be set to zero. -
Suppose I have a structure in C++ containing a name and a number, e.g. struct person { char name[20]; int ssn; }; ... The default assignment operator in C++ uses Memberwise Assignment to copy the values. That is it effectively assigns all members to each other. In this case that would cause b to have the same values as a.
Implicitly-declared copy assignment operator. If no user-defined copy assignment operators are provided for a class type (struct, class, or union), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T:: operator = (const T &) if all of the following is ...
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.