Vending Machine Python Program With Code
Last updated April 25, 2023 by Jarvis Silva
Want to know how to create a vending machine python program, then you are at the right place. Today in this tutorial I will show you step by step on how to create a simple vending machine program using python.
A vending machine is an automated machine which provides food items to the consumers. The consumer has to click the item they want and enter the money or cash into the machine to get the item.
We will create a similar vending machine program which will allow users to get the food item they want so let’s see how to do it.
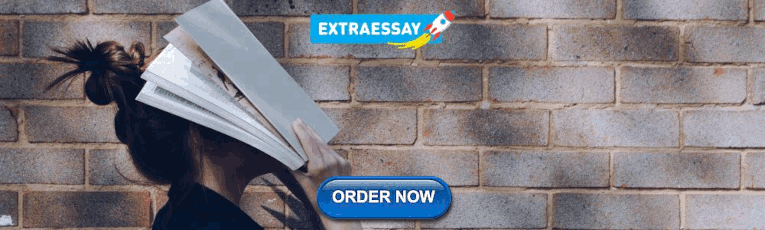
Creating Vending Machine Python Program
This program will be command line based and it will have no GUI. It will be a very simple and easy to use program.
Here’s how this vending machine program will work:
- It will show user a list of food items along with their prices.
- It will ask user to enter the code of the food that they want.
- It will do user input and other validation.
- It will ask the user to enter the price of the food they want.
- Then it will give the user the food, just programmatically.
Above are some of the things about this program. I will show you step by step how to create this program, so let’s start.
1. Download and Install Python
Skip this step if you have done it, First you need to download python from the official website and after downloading run the setup it will install python on your computer.
To check if python is installed you can open command prompt and type python, it should not give any errors.
2. Project Setup
Now you have python installed, create a new folder for this program, open it in a code editor if you don’t have a code editor you can download and use vs code.
After opening the folder in vs code you can create a python file and go to the next step of copy and pasting the vending machine python code.
3. Vending Machine Python Code
Above is the python vending machine code, copy it and paste it in your python file. I have not used any libraries, so you don’t have to install any.
Now to run the program, open a command prompt or terminal at the project folder location and paste the below command to run the program or else you can use this online python compiler.
This will start the program and you should see a welcome to vending machine message. It will show you a list of food items, you can add more if you want.
It will ask you to enter the code number of the food item which is given, then it will ask you to enter the price of the item, then you will get the food item. Below is an example output of this program.
As you can see, we have successfully created a vending machine program and it works similar to a vending machine.
Python Vending Machine Program Explanation
Now let’s see and understand how each line of this program works. If you are an experienced programmer then you don’t have to read this, but if you are a beginner then read this part.
First we have the items list which has nested python dictionaries. As you can see, each item has 3 keys: name, code and price. You can add more food items to this list if you want but remember to add the code value linearly.
Then we have the while loop which runs till the variable is_quit becomes true, this allows the program to run as long as the user wants to quit.
Now inside the while loop we have a print statement which welcomes and a for loop which iterates over the food items and prints the name, code and price in order format.
Now this line of code asks the user to enter the code number of the food item they want, after that it finds the food item with the code number and set’s the food item into an item variable.
If they enter any wrong or invalid code number, then it prints an INVALID CODE. If it enters correctly and matches the food item, then it goes in the else statement.
Inside else statement it prints the food details they want with price then it asks the user to enter the price amount to get the food and if it matches the price of the food they want they get the food, if not then they don’t get.
Lastly it asks the user if they want to continue or quit the program, so to continue they can enter anything except q because that is for quitting.
This was the tutorial on creating a vending machine program in python. I hope you found this program useful and helpful. Do share this with your friends who might be interested in this program.
Here are more python programs you might find interesting:
- Even odd numbers check program in python.
- NxNxN Matrix Python 3 program.
- Atm machine program in python.
I hope you found what you were looking for from this python tutorial and if you want more python tutorials like this then do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂
Related content
Our website is undergoing major changes. You may experience unexpected issues while navigating. I appreciate your patience.🙏
< BACK TO PYTHON PROJECTS
Create Your Own Vending Machine with Python (Source Code)
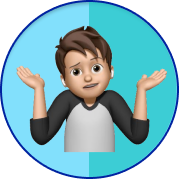
By Faraz - May 16, 2023
Learn how to build a vending machine using Python. This comprehensive guide will walk you through the process from start to finish.
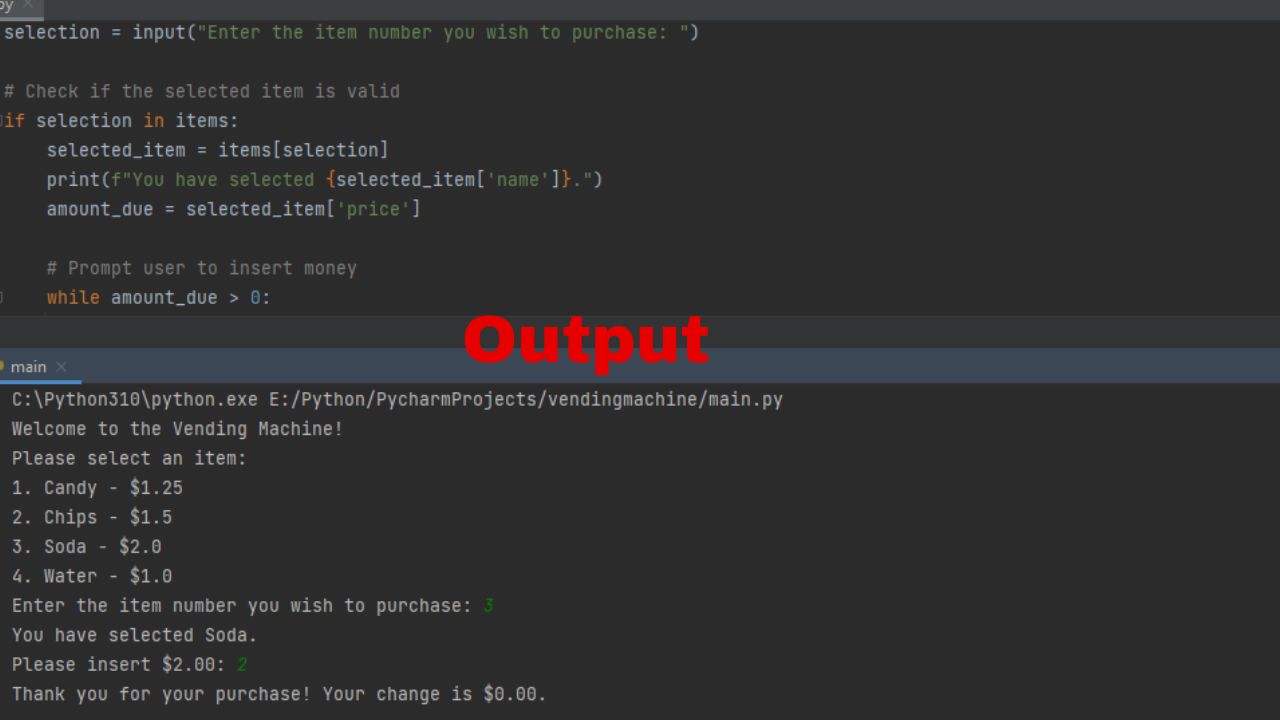
Vending machines have become an integral part of our modern world. You can find them in various locations, from schools and offices to train stations and shopping malls. These automated machines provide a convenient way for people to purchase products or access services without the need for human assistance.
Why create your own vending machine?
Building your own vending machine is not only a fun and engaging project, but it also offers several benefits. Here are a few reasons why you might consider creating your own vending machine:
- Learn and apply programming skills:
- Customize products and functionality:
- Gain insights into automation and robotics:
- Showcase your creativity:
Overview of the project
In this tutorial, we will guide you through the process of creating your own vending machine using the Python programming language.The project will cover all essential aspects, from setting up the necessary software and hardware to designing the vending machine's layout and implementing the logic behind product selection, inventory management, and dispensing.
Whether you are a beginner looking to explore the world of hardware programming or an intermediate programmer seeking a challenging project, this tutorial will provide you with the knowledge and guidance to embark on your vending machine creation journey. So, let's dive in and discover how to bring your own vending machine to life with Python!
Writing the code structure
Explanation.
Let's go through the code step by step:
- The code defines a dictionary called items which represents the available items in the vending machine. Each item is assigned a unique key (e.g., '1', '2', '3', '4'), and the corresponding value is another dictionary containing the item's name and price.
- The code then displays a welcome message and prompts the user to select an item.
- It loops through each item in the items dictionary and prints the item's key, name, and price.
- After displaying the available items and prices, the code prompts the user to enter the item number they wish to purchase.
- The code checks if the selected item is valid by verifying if the entered item number exists as a key in the items dictionary.
- If the selected item is valid, it retrieves the item's details (name and price) from the items dictionary and stores them in the selected_item variable.
- The code then sets the amount_due variable to the price of the selected item.
- Next, it enters a loop where it prompts the user to insert money until the amount_due is paid in full.
- Within the loop, it attempts to convert the user's input to a float (assuming the user enters a valid number for payment). If the conversion is successful, it compares the payment amount with the amount_due .
- If the payment is greater than or equal to the amount_due , it calculates the change by subtracting the amount_due from the payment and prints a thank you message along with the calculated change.
- If the payment is less than the amount_due , it informs the user that the payment is insufficient and prompts for more money. The amount_due is updated by subtracting the payment from it.
- If the user enters an invalid payment amount (e.g., non-numeric input), it catches the ValueError exception and displays an error message.
- If the selected item is not valid (i.e., the entered item number does not exist in the items dictionary), it prints an " Invalid selection " message.
That's the overall flow of the code. It provides a simple vending machine experience by allowing the user to select an item, insert money, and receive change if necessary.
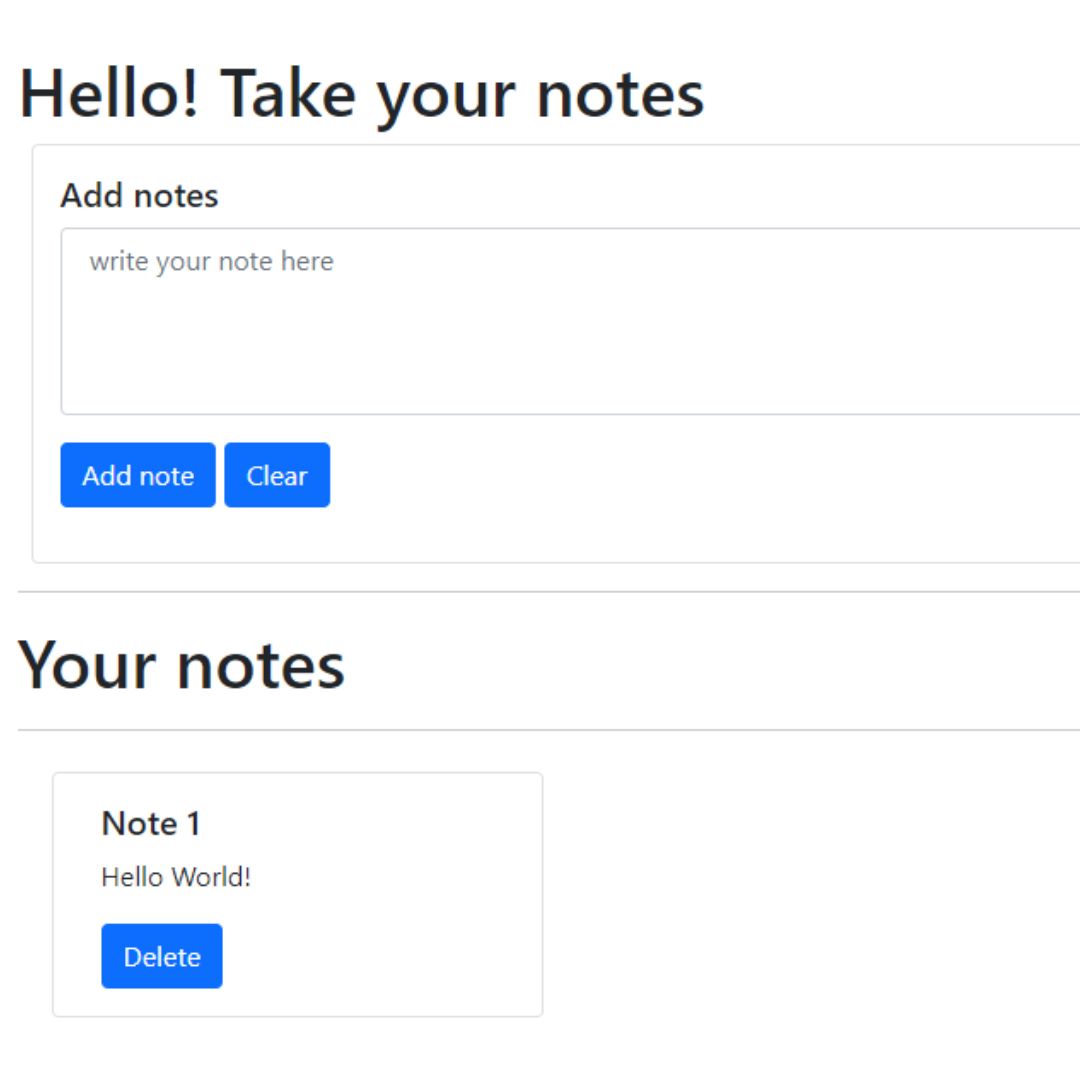
That’s a wrap!
I hope you enjoyed this article
Did you like it? Let me know in the comments below 🔥 and you can support me by buying me a coffee.
And don’t forget to sign up to our email newsletter so you can get useful content like this sent right to your inbox!
Thanks! Faraz 😊
Subscribe to my Newsletter
Get the latest posts delivered right to your inbox, latest post.
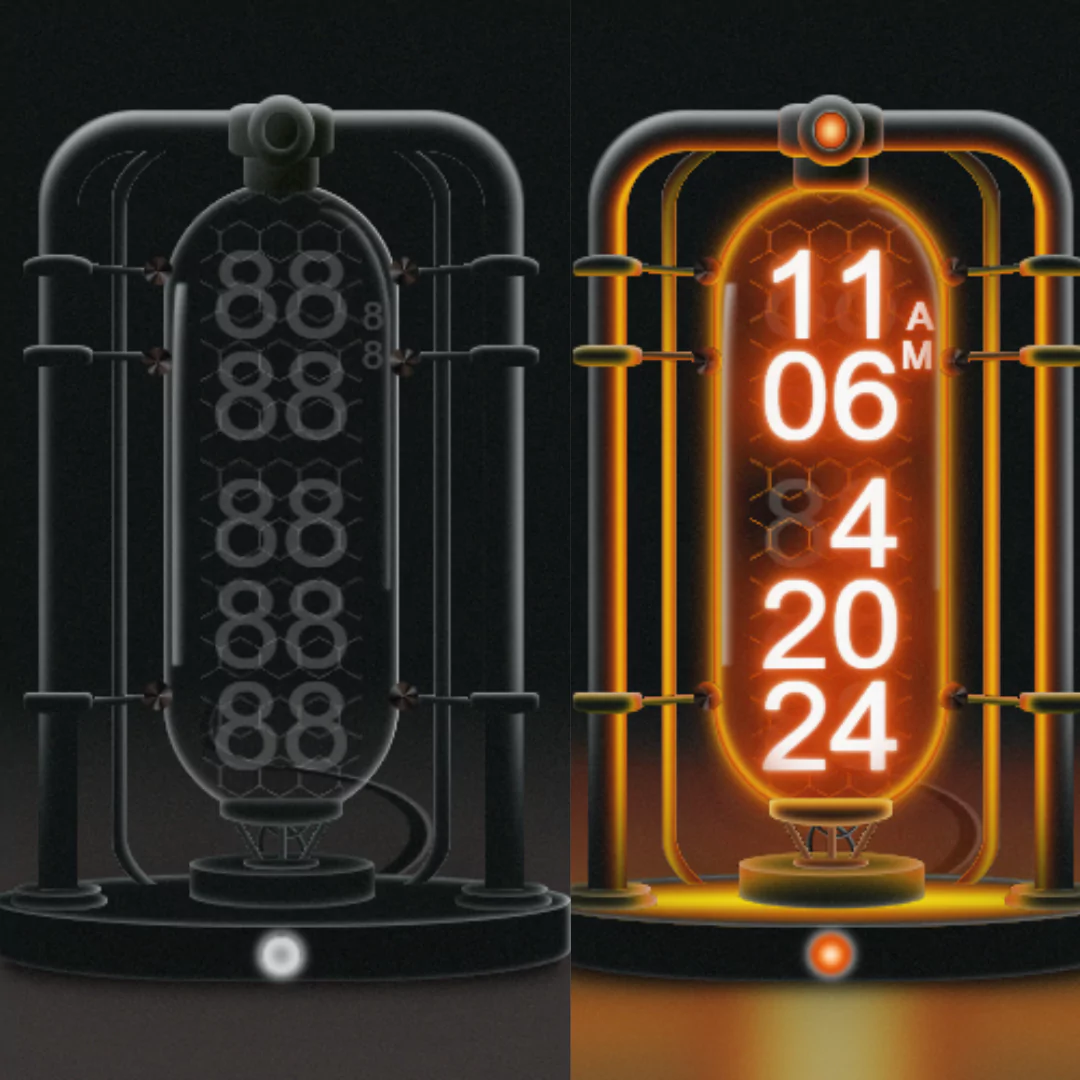
Build Your Own Nixie Tube Clock using HTML, CSS, and JavaScript (Source Code)
Learn how to create a stunning Nixie Tube Clock using HTML, CSS, and JavaScript. Get the source code and step-by-step instructions today!
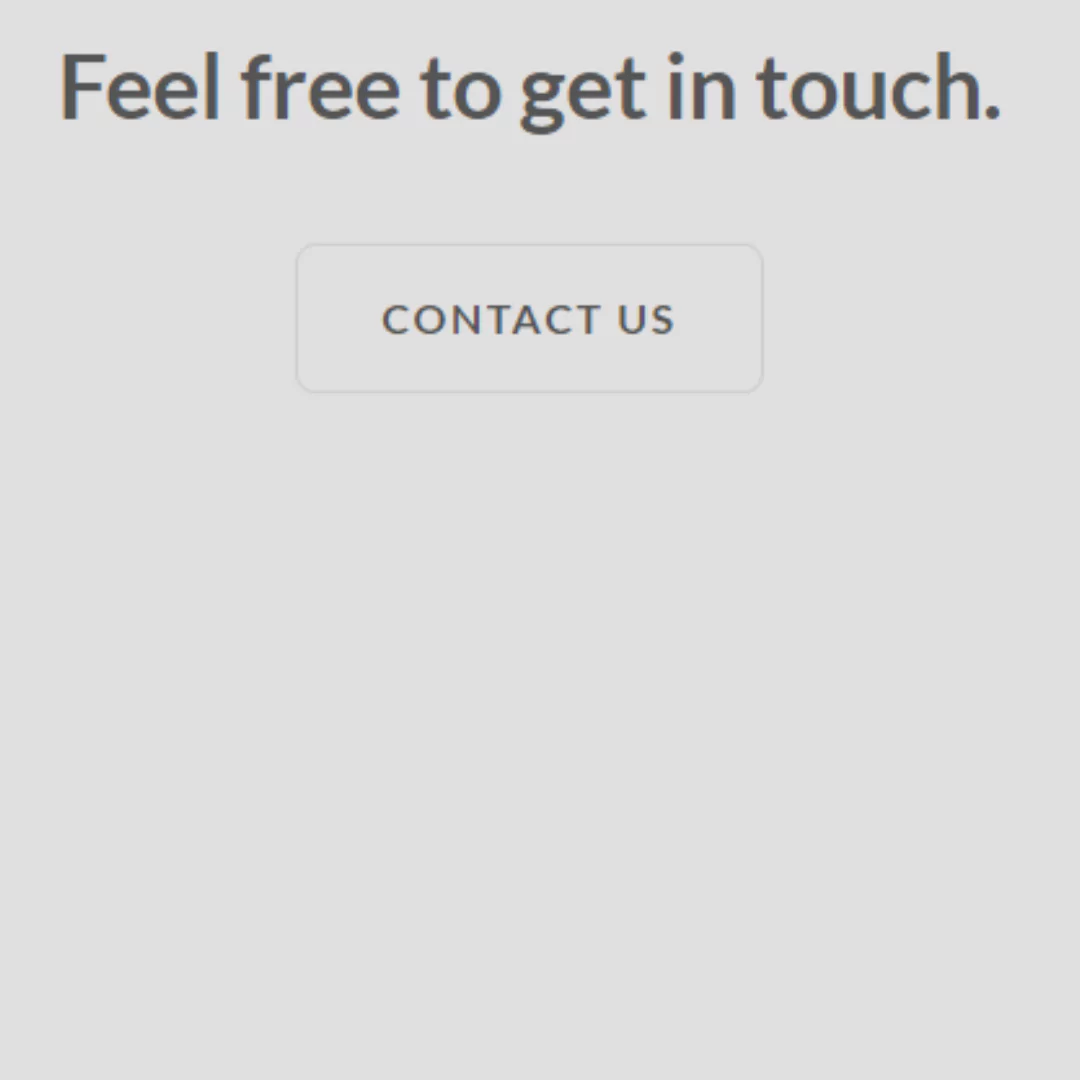
Create a Responsive Popup Contact Form: HTML, CSS, JavaScript Tutorial
April 17, 2024
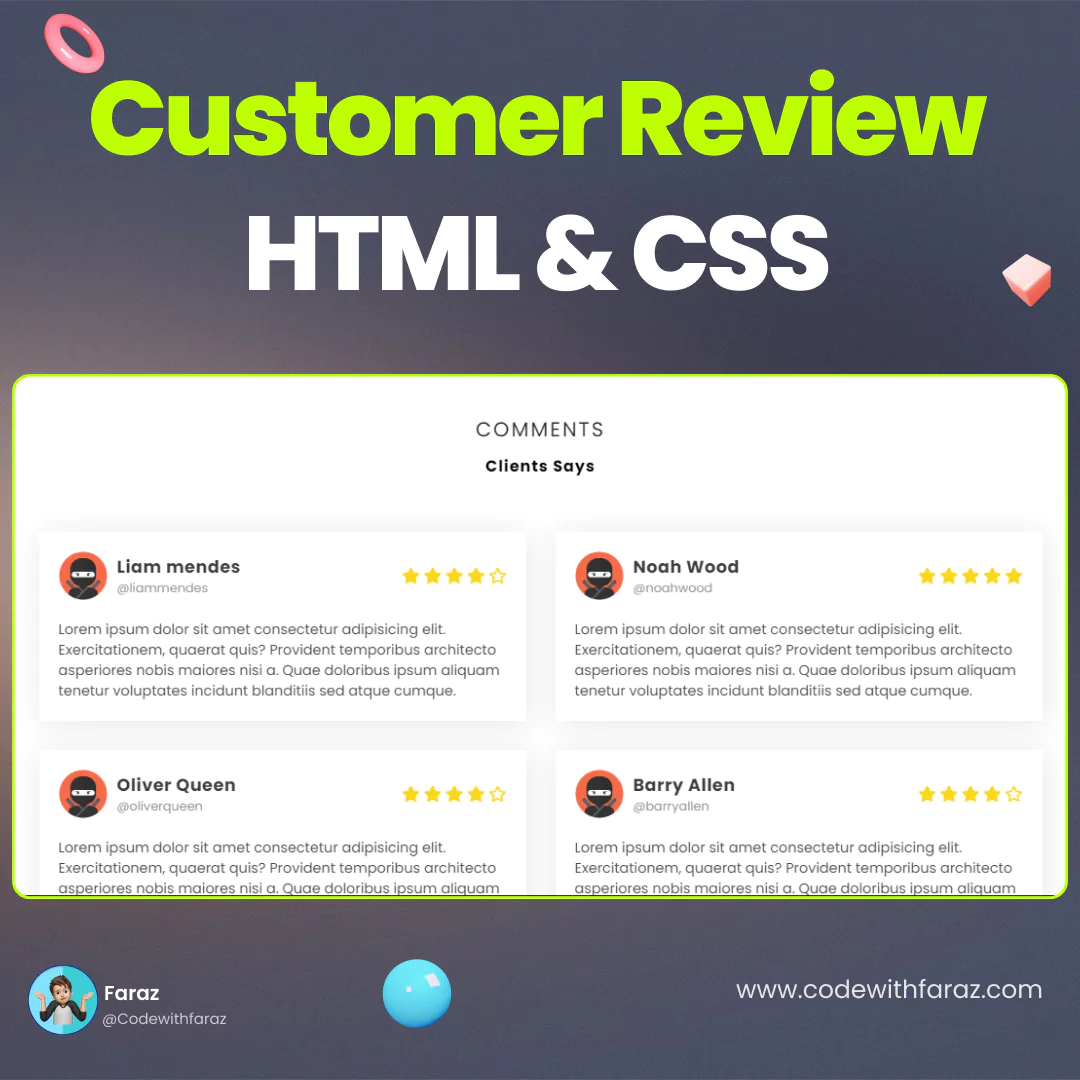
Create a Responsive Customer Review Using HTML and CSS
April 14, 2024
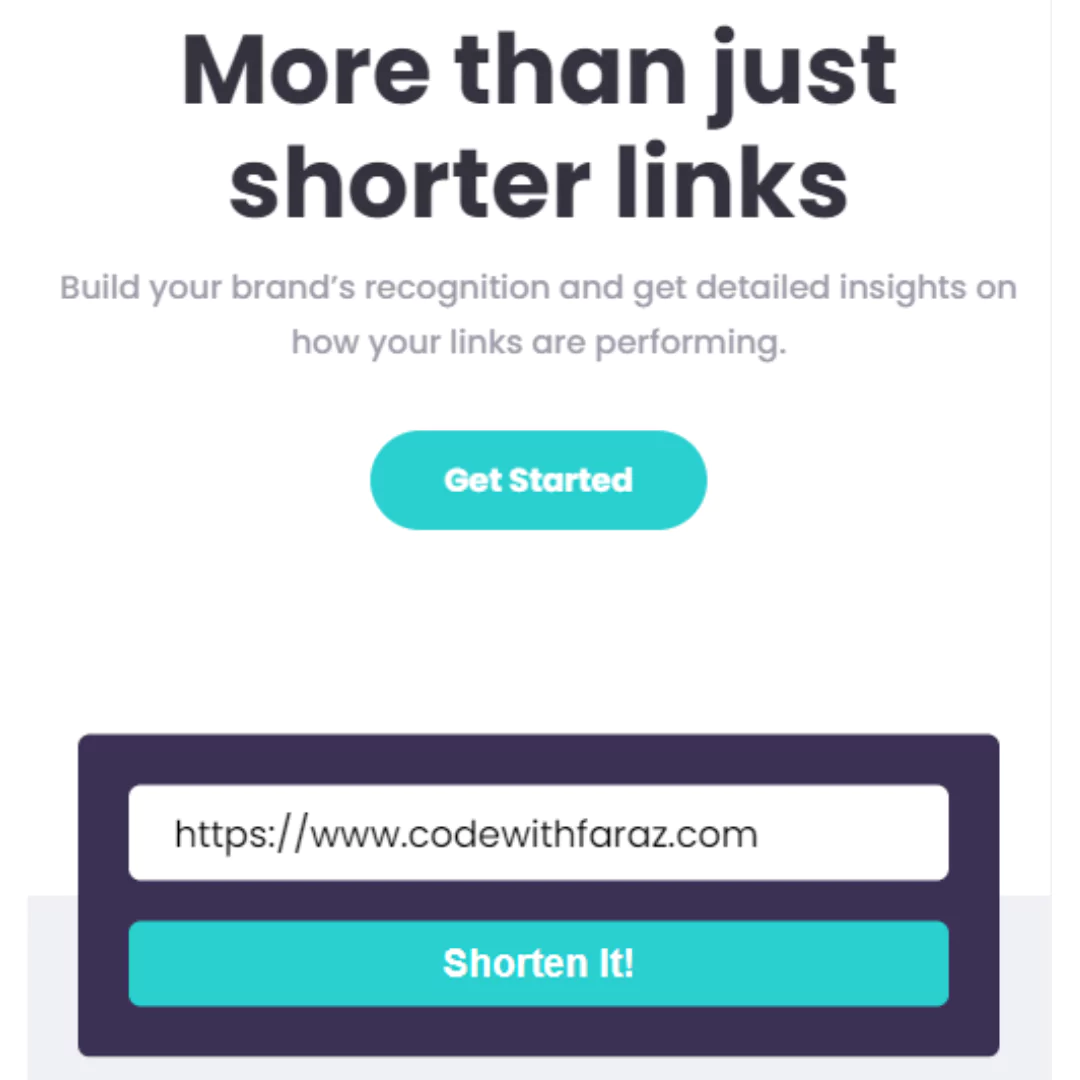
Create a URL Shortening Landing Page using HTML, CSS, and JavaScript
April 08, 2024
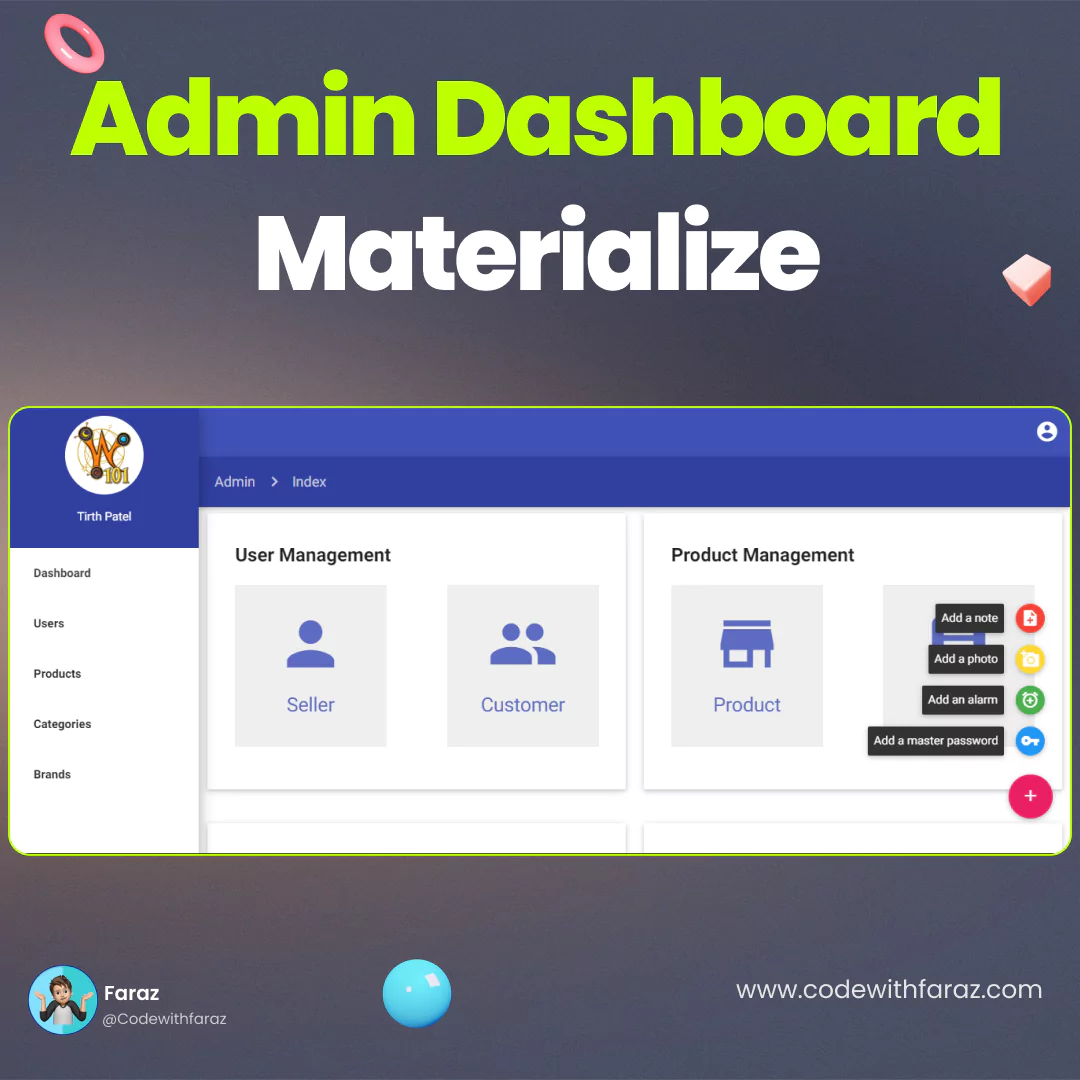
Develop Responsive Admin Dashboard with HTML, Materialize CSS, and JavaScript
April 05, 2024
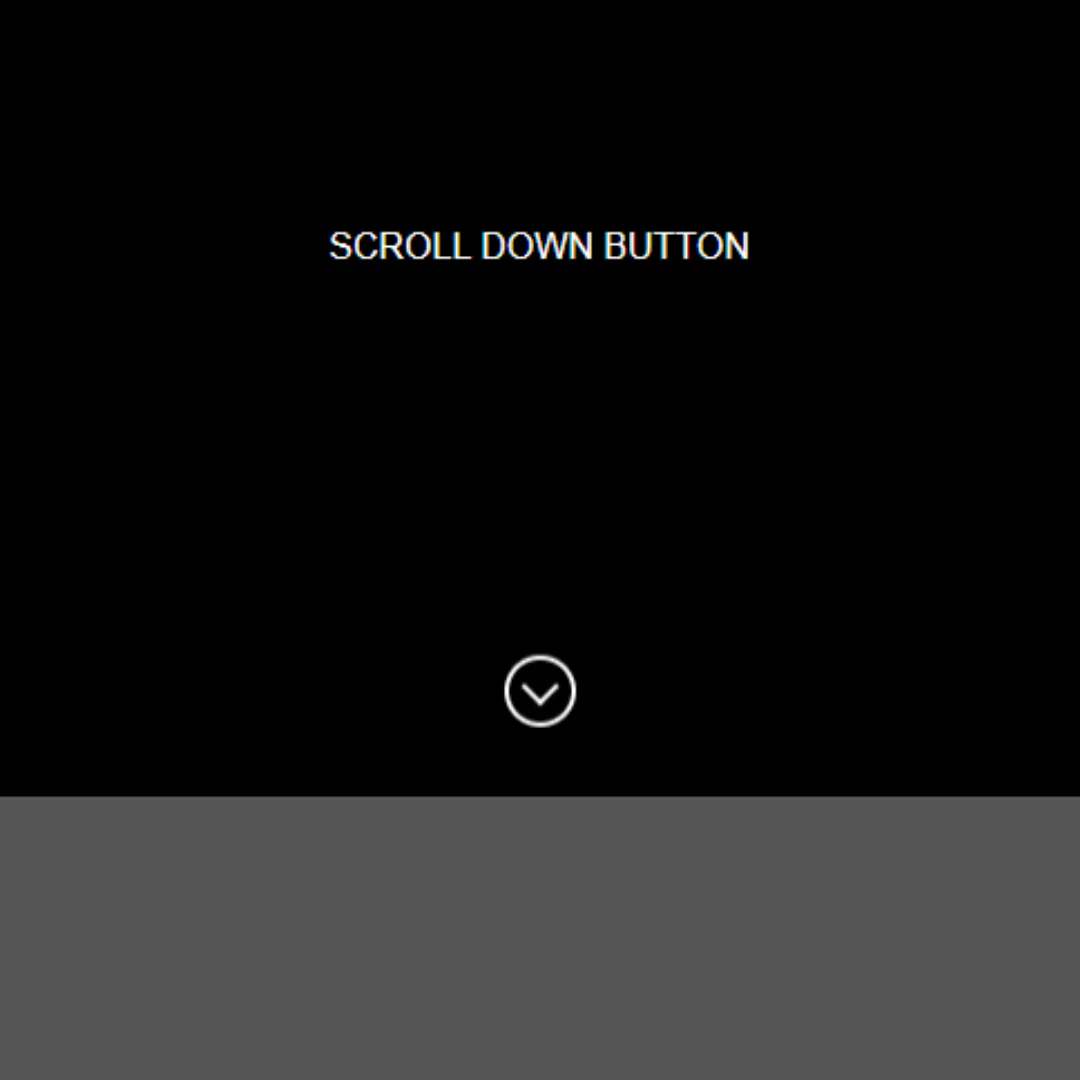
How to Create a Scroll Down Button: HTML, CSS, JavaScript Tutorial
Learn to add a sleek scroll down button to your website using HTML, CSS, and JavaScript. Step-by-step guide with code examples.
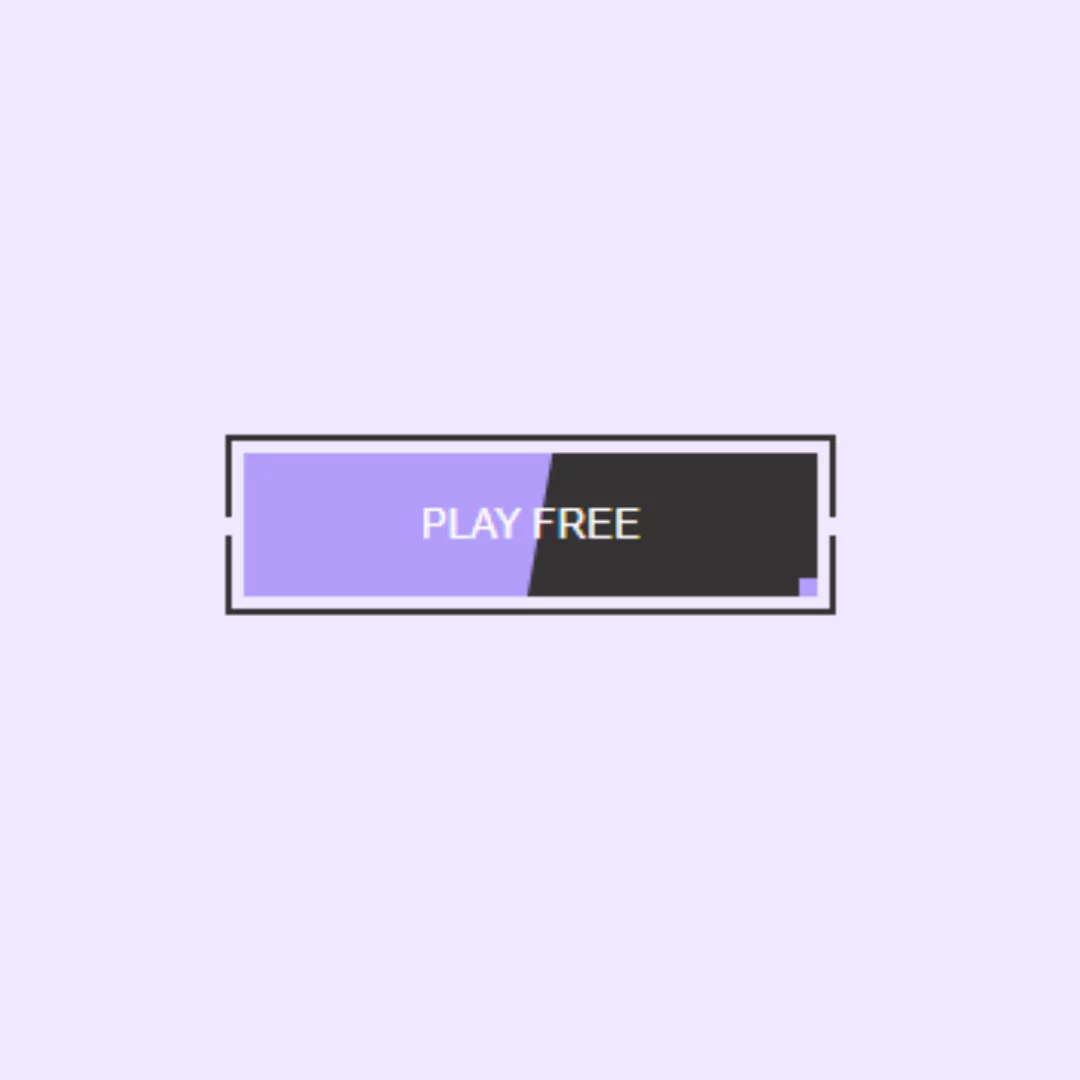
How to Create a Trending Animated Button Using HTML and CSS
March 15, 2024
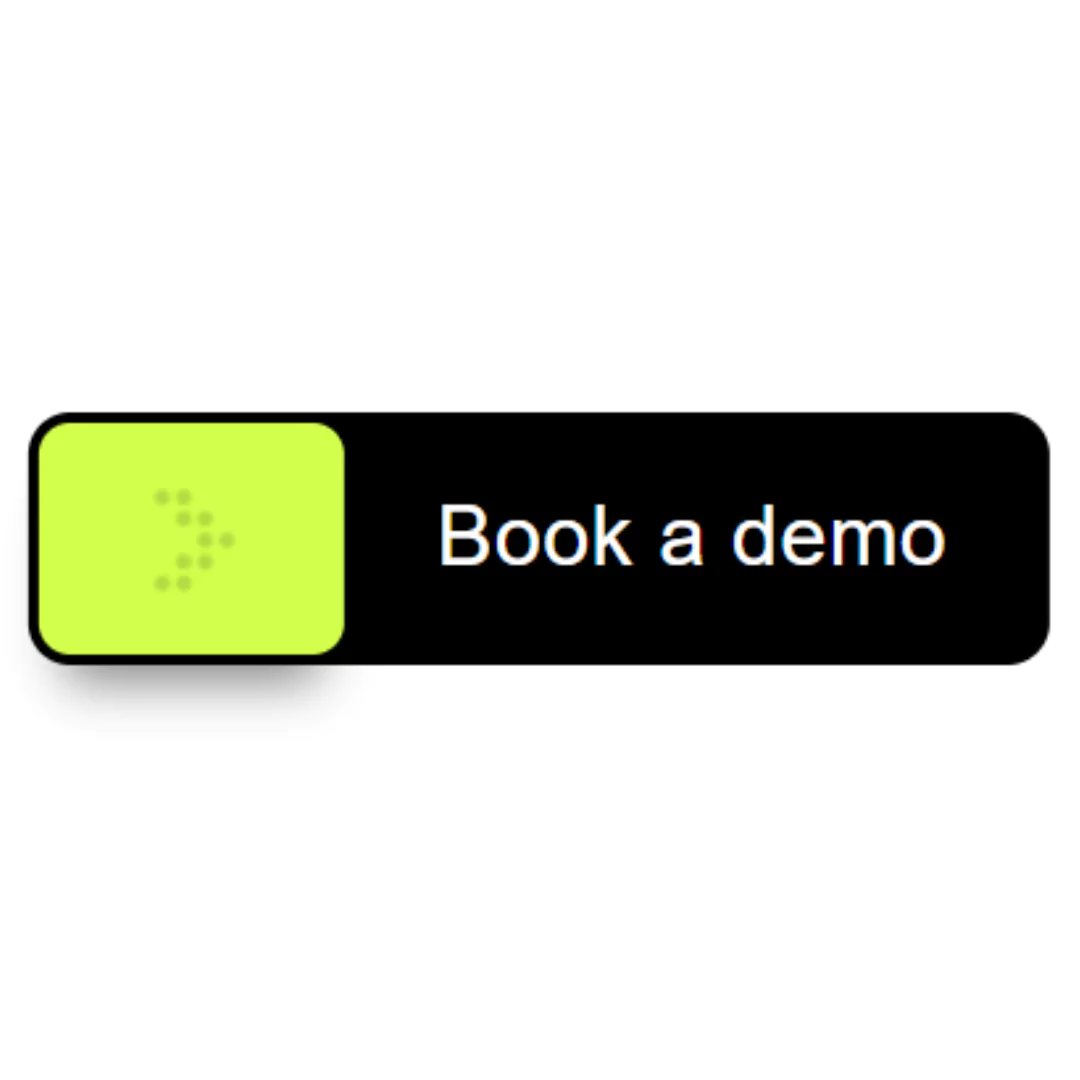
Create Interactive Booking Button with mask-image using HTML and CSS (Source Code)
March 10, 2024
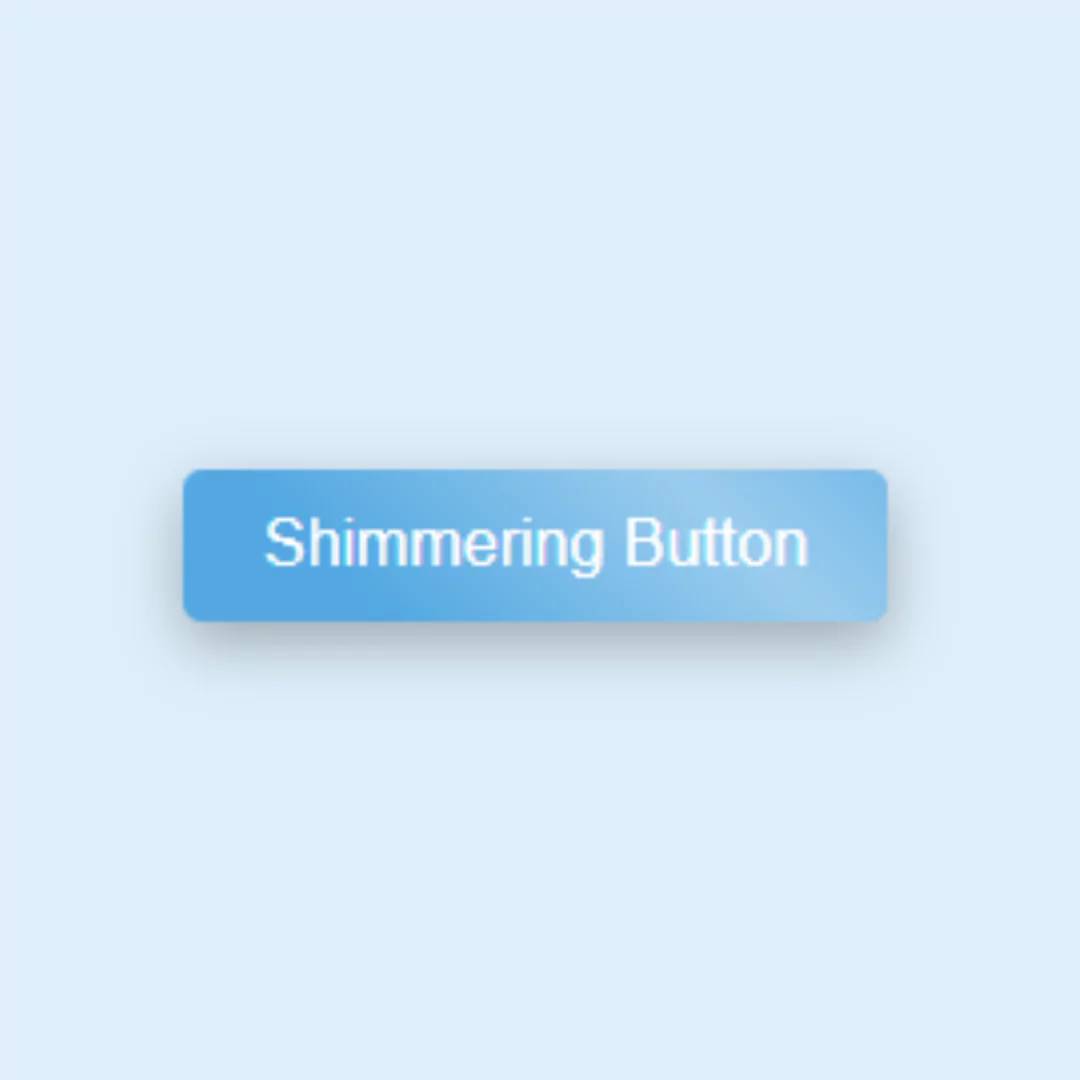
Create Shimmering Effect Button: HTML & CSS Tutorial (Source Code)
March 07, 2024
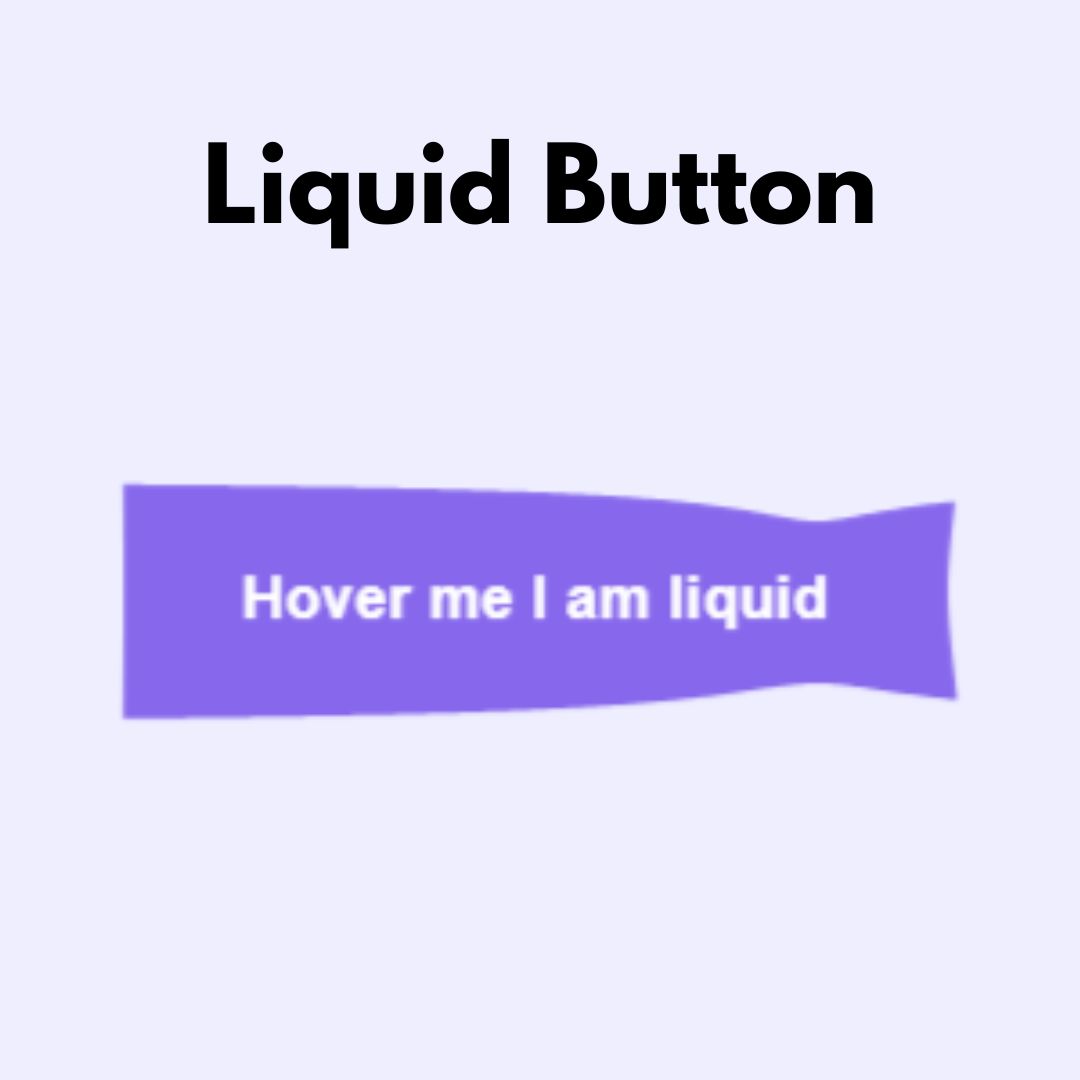
How to Create a Liquid Button with HTML, CSS, and JavaScript (Source Code)
March 01, 2024
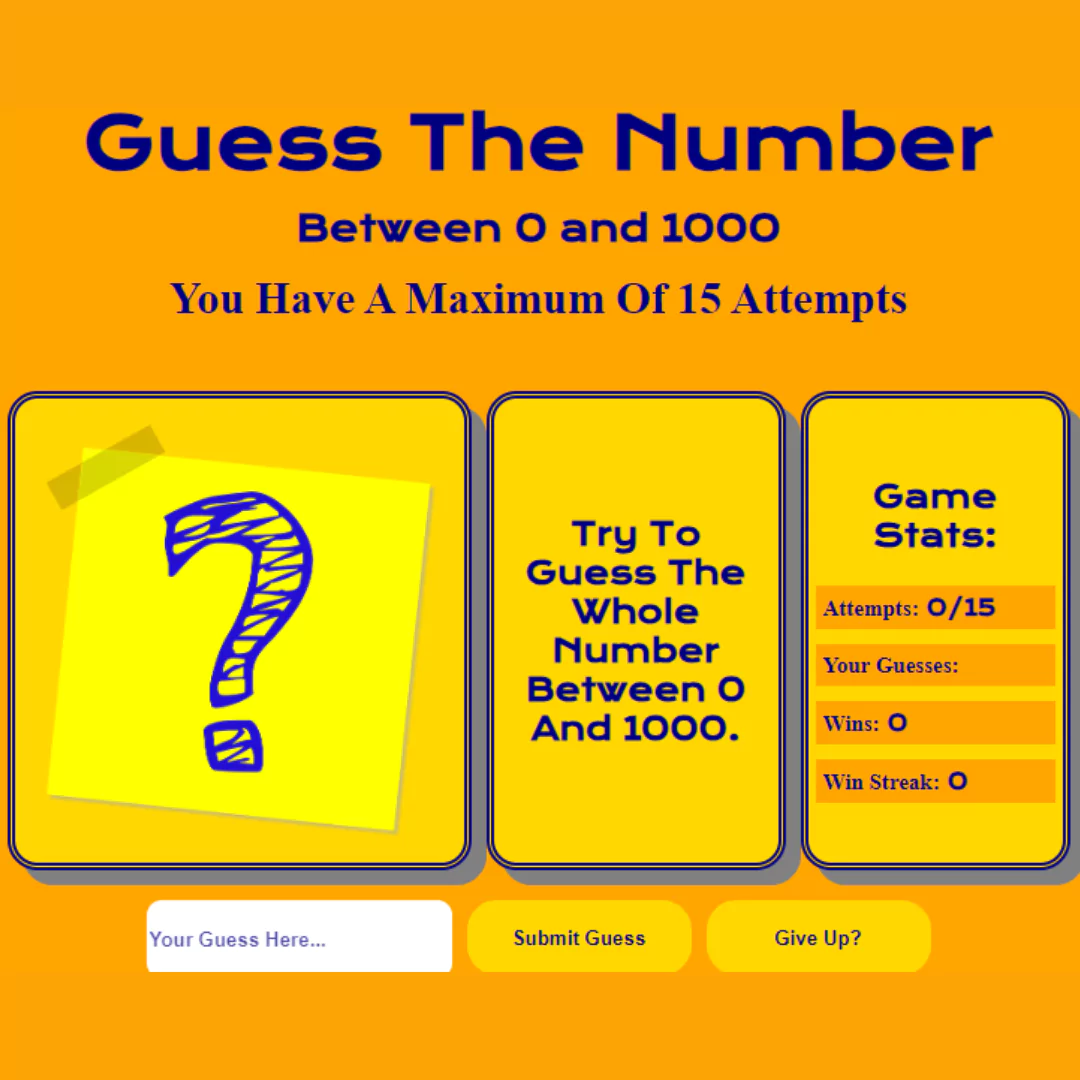
Build a Number Guessing Game using HTML, CSS, and JavaScript | Source Code
Learn how to create an interactive Number Guessing Game from scratch using HTML, CSS, and JavaScript with this beginner-friendly tutorial.
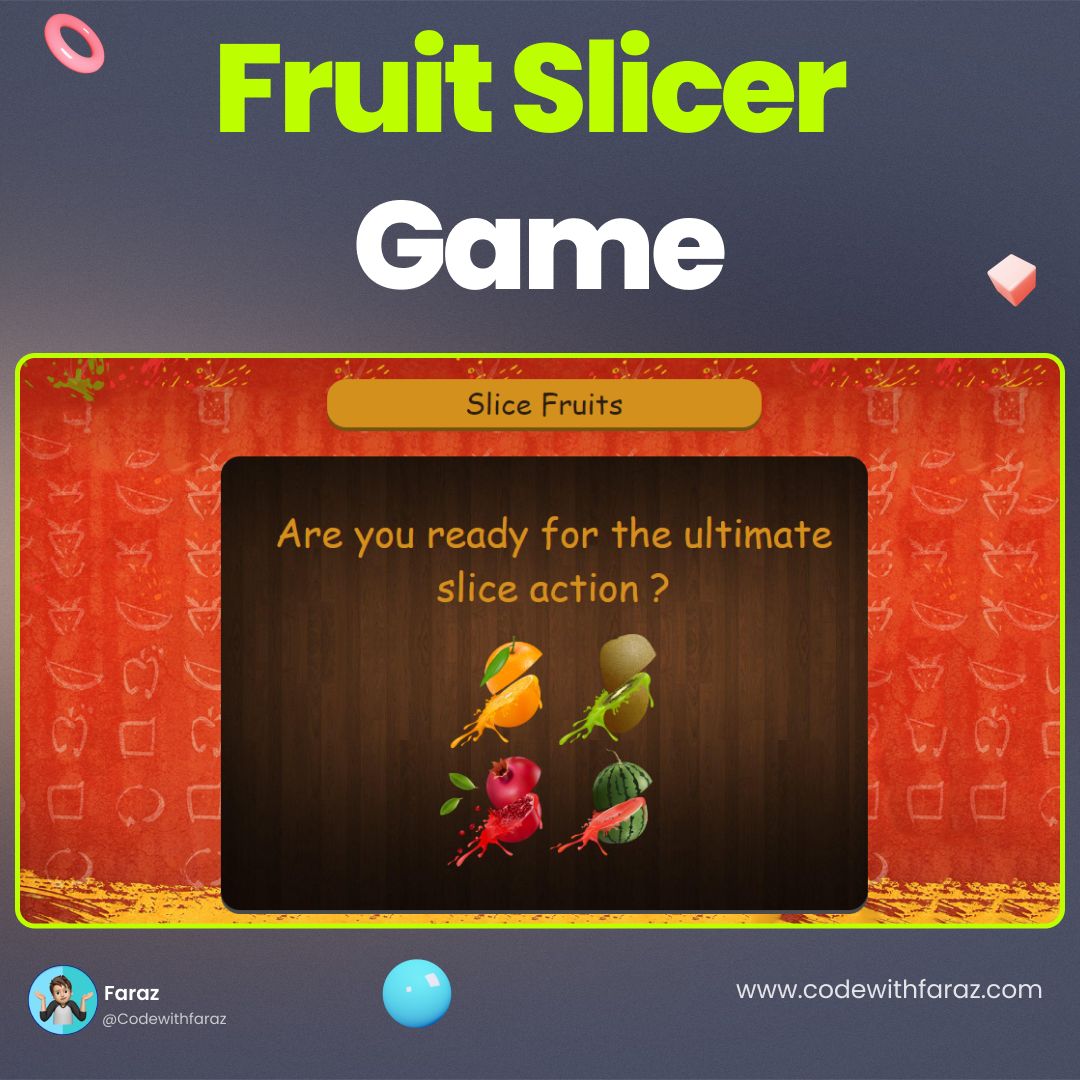
Building a Fruit Slicer Game with HTML, CSS, and JavaScript (Source Code)
December 25, 2023
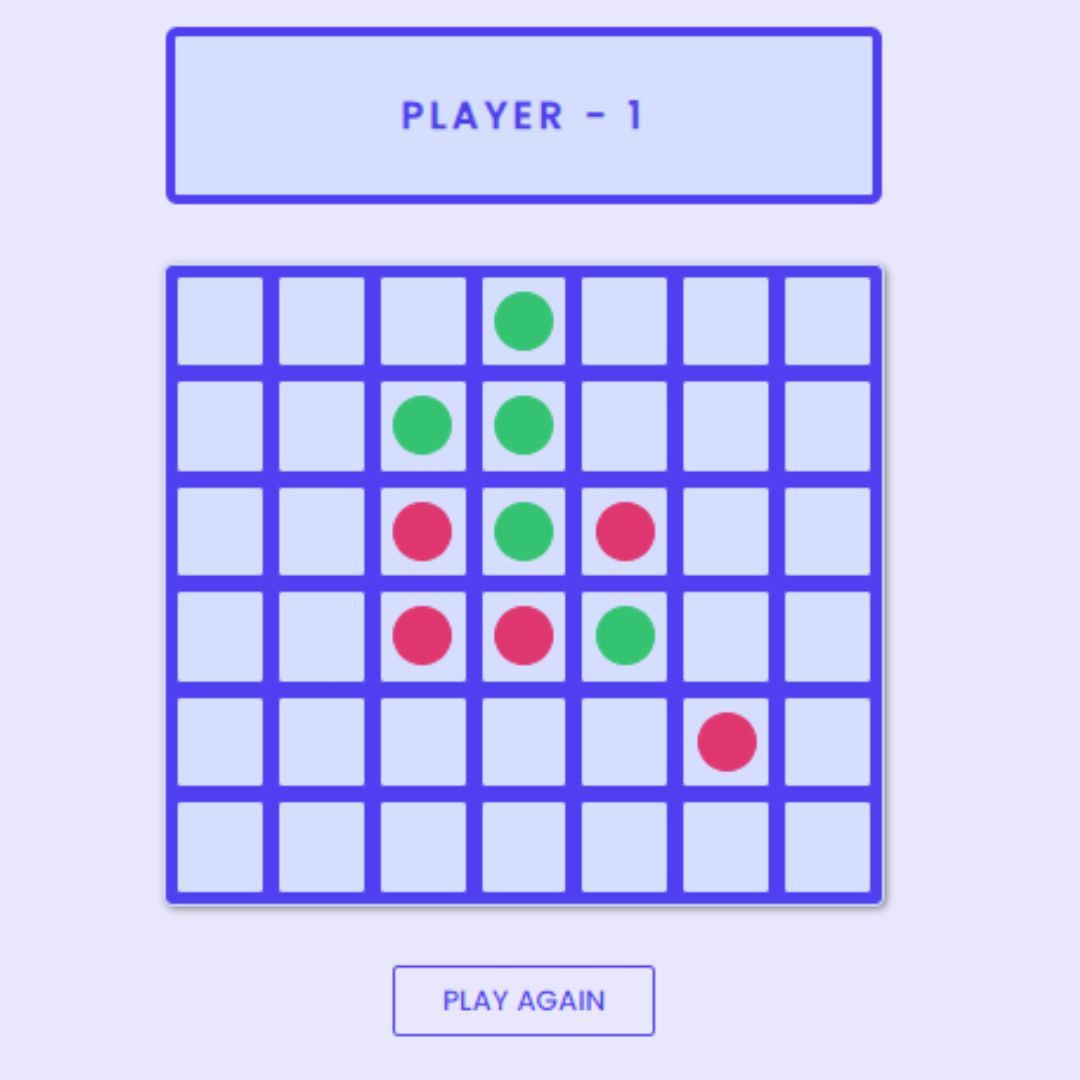
Create Connect Four Game Using HTML, CSS, and JavaScript (Source Code)
December 07, 2023
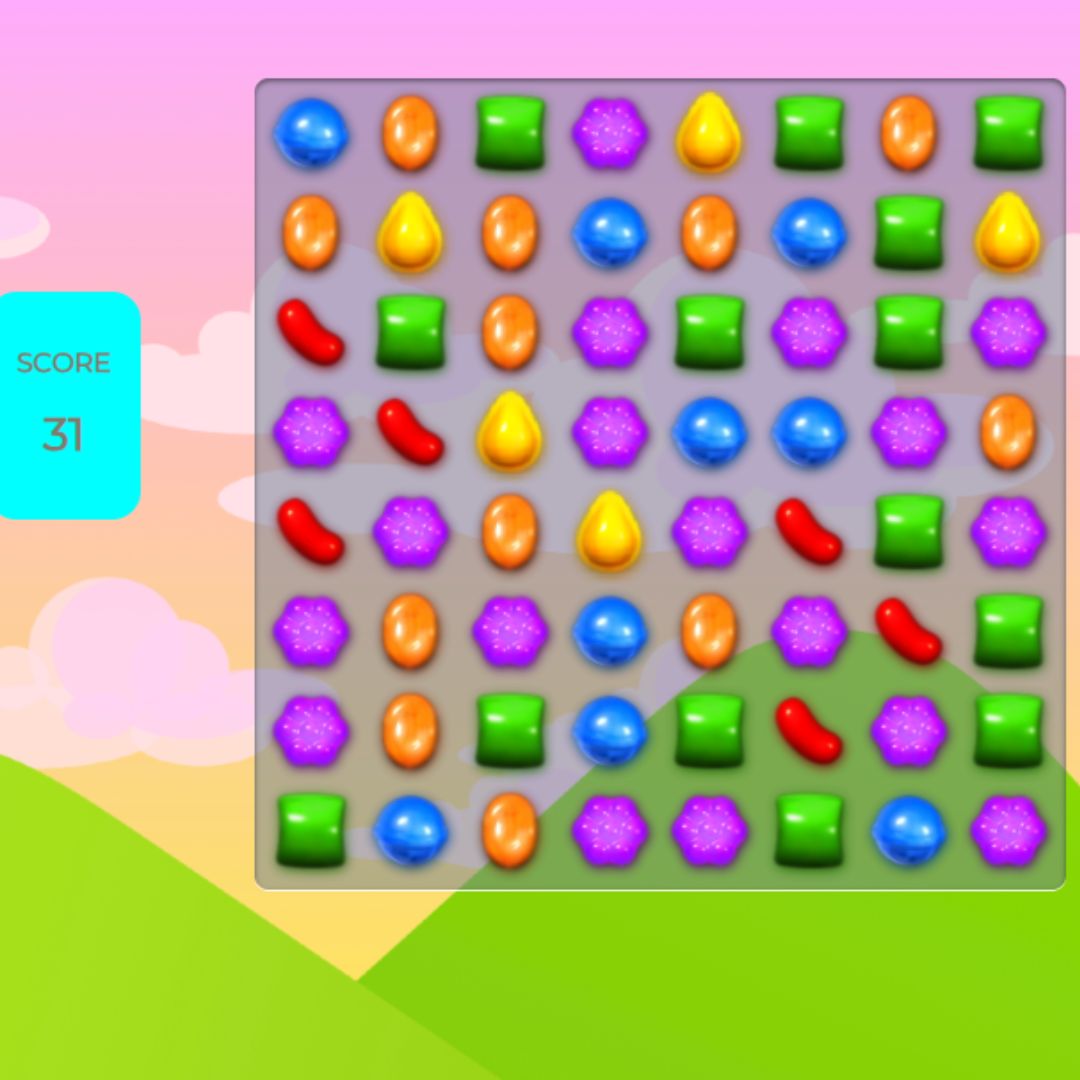
Creating a Candy Crush Clone: HTML, CSS, and JavaScript Tutorial (Source Code)
November 17, 2023
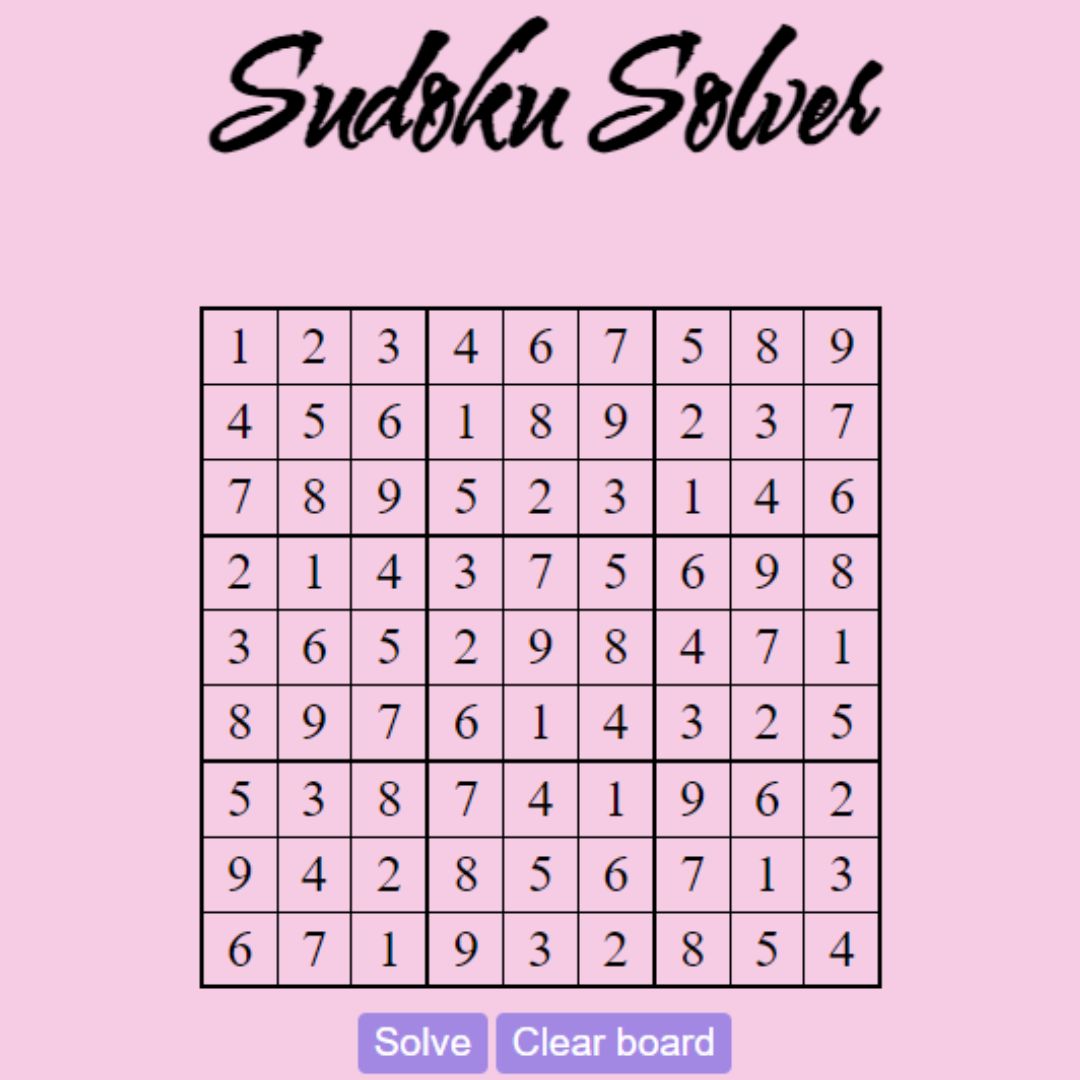
Sudoku Solver with HTML, CSS, and JavaScript
October 16, 2023
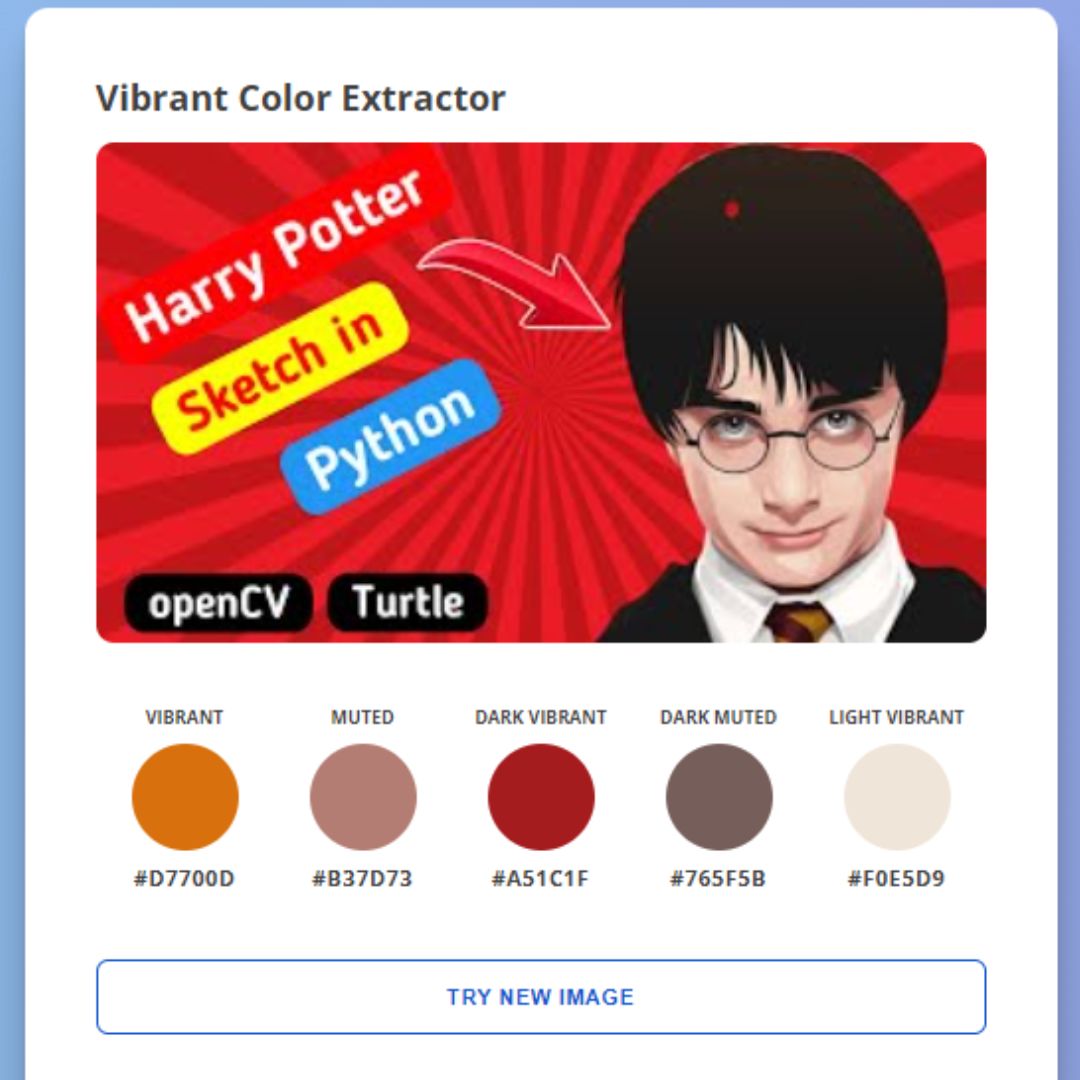
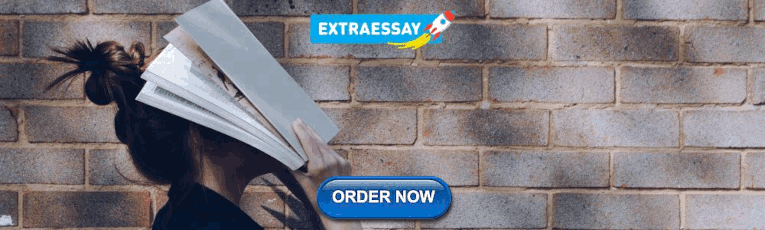
Create Image Color Extractor Tool using HTML, CSS, JavaScript, and Vibrant.js
Master the art of color picking with Vibrant.js. This tutorial guides you through building a custom color extractor tool using HTML, CSS, and JavaScript.
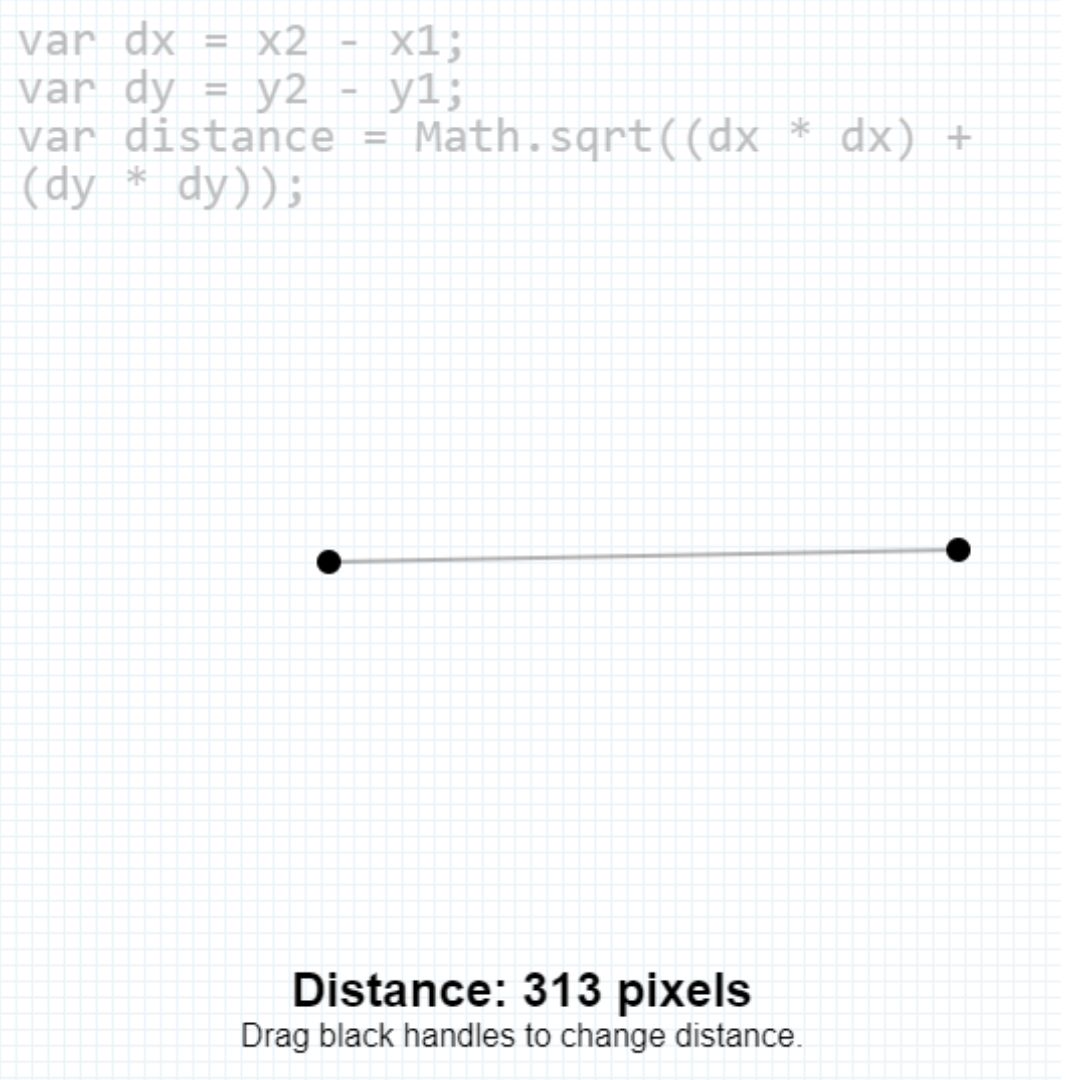
Build a Responsive Screen Distance Measure with HTML, CSS, and JavaScript
January 04, 2024
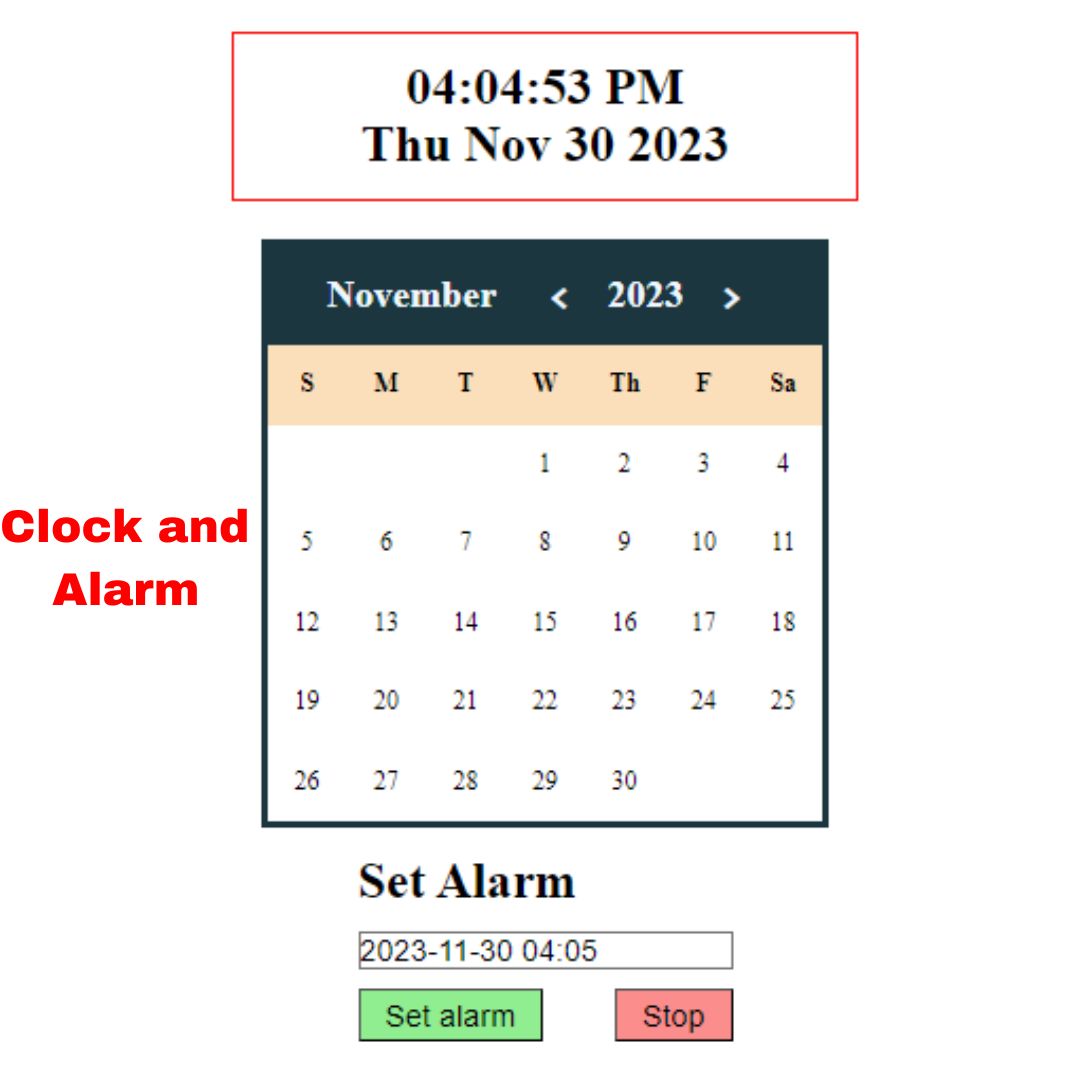
Crafting Custom Alarm and Clock Interfaces using HTML, CSS, and JavaScript
November 30, 2023
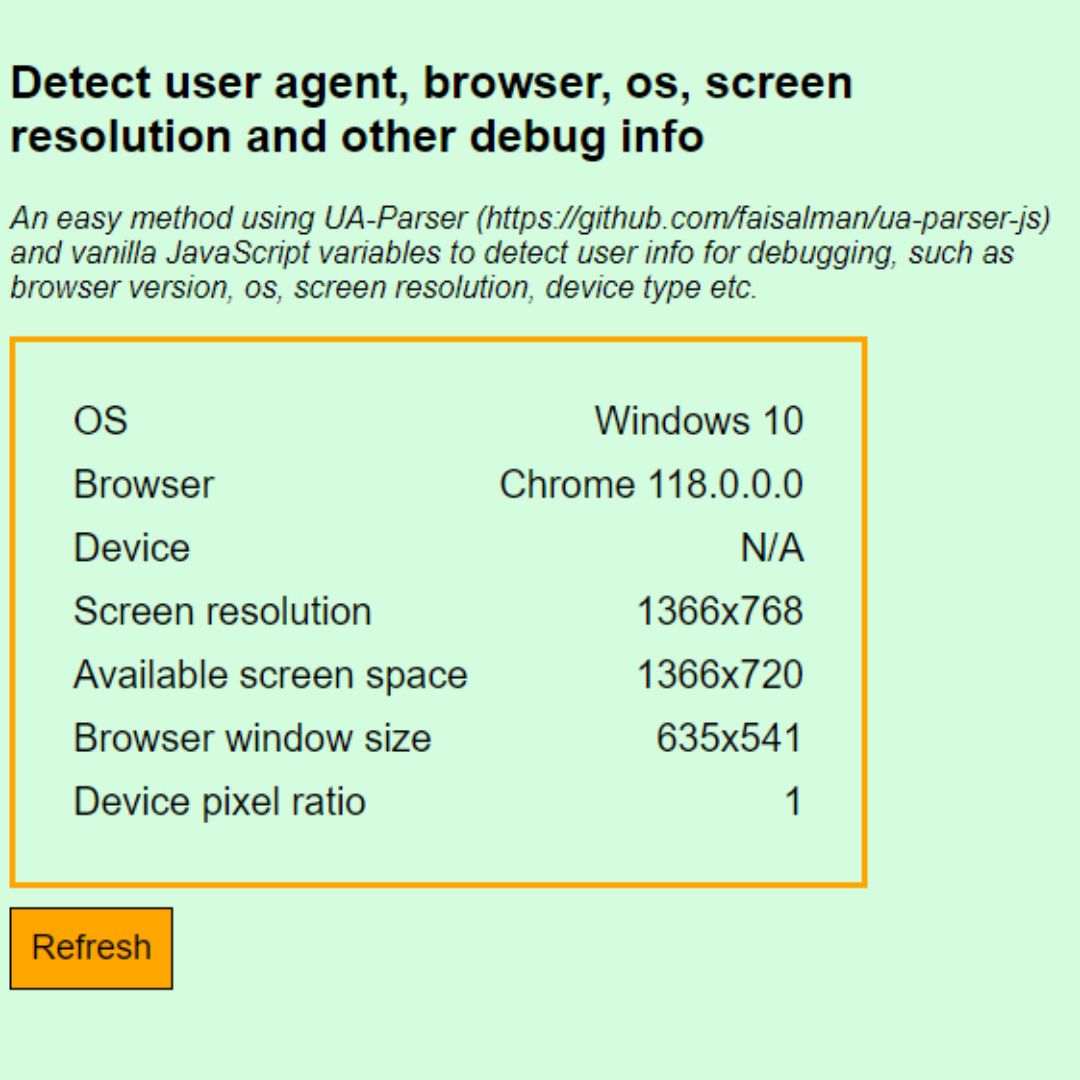
Detect User's Browser, Screen Resolution, OS, and More with JavaScript using UAParser.js Library
October 30, 2023
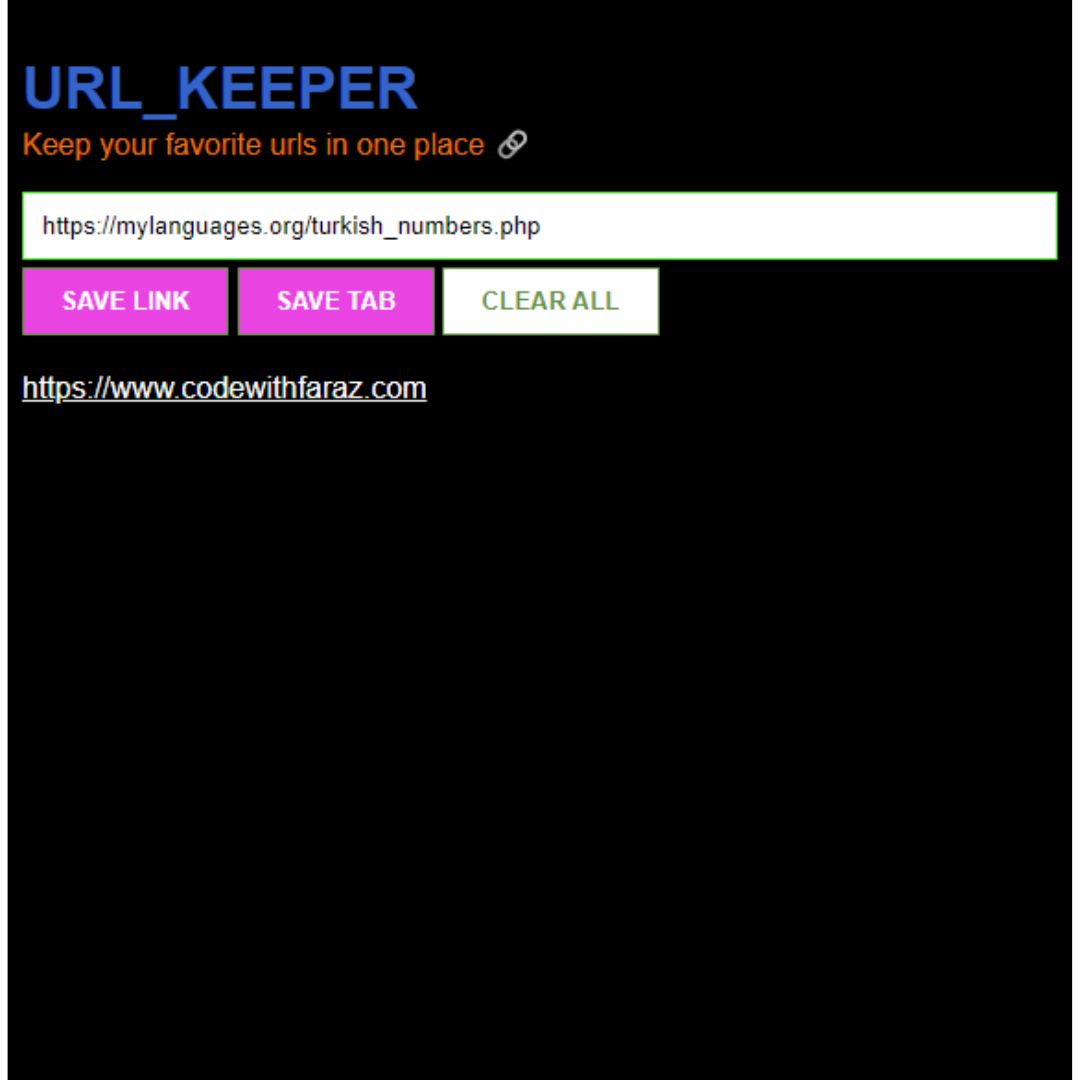
URL Keeper with HTML, CSS, and JavaScript (Source Code)
October 26, 2023
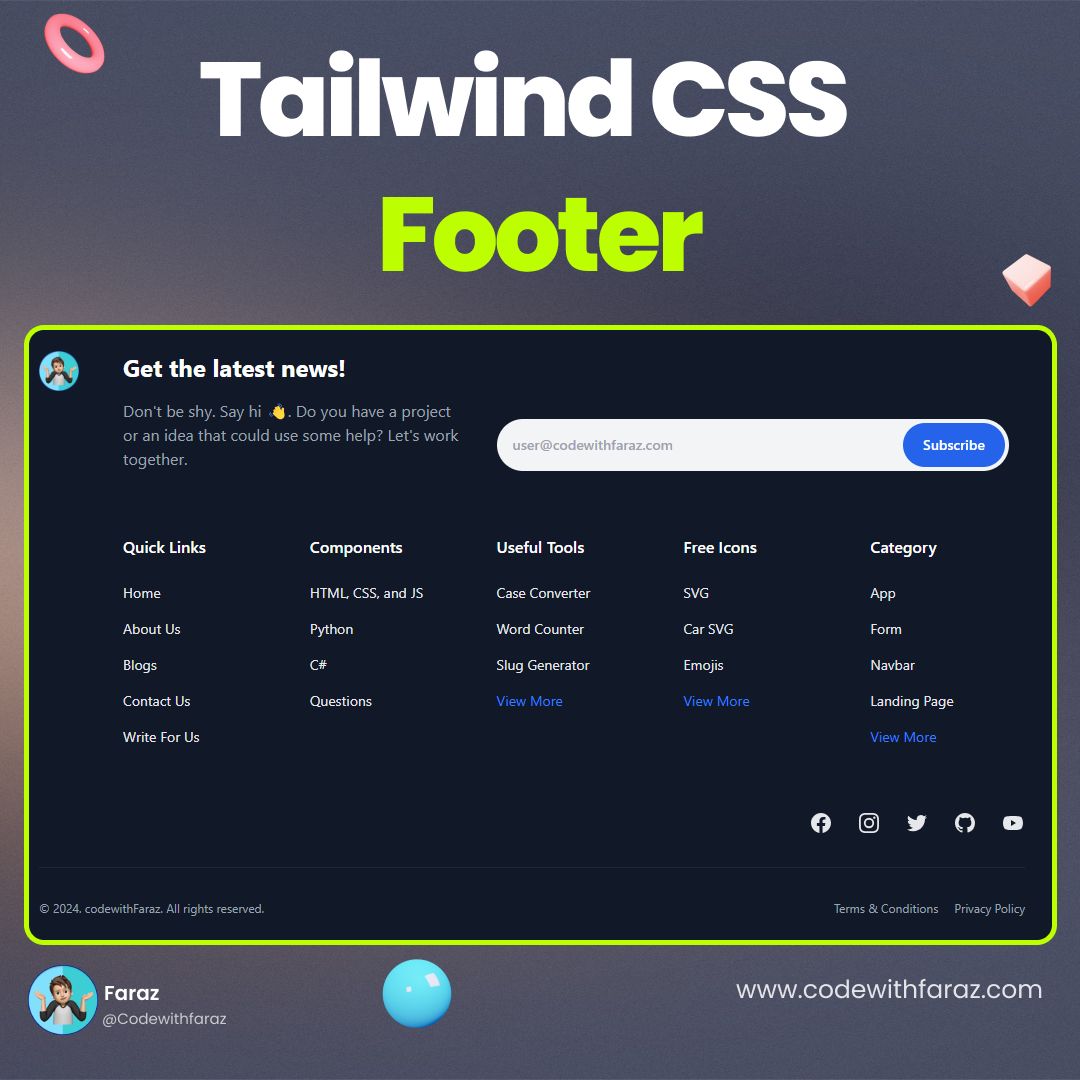
Creating a Responsive Footer with Tailwind CSS (Source Code)
Learn how to design a modern footer for your website using Tailwind CSS with our detailed tutorial. Perfect for beginners in web development.
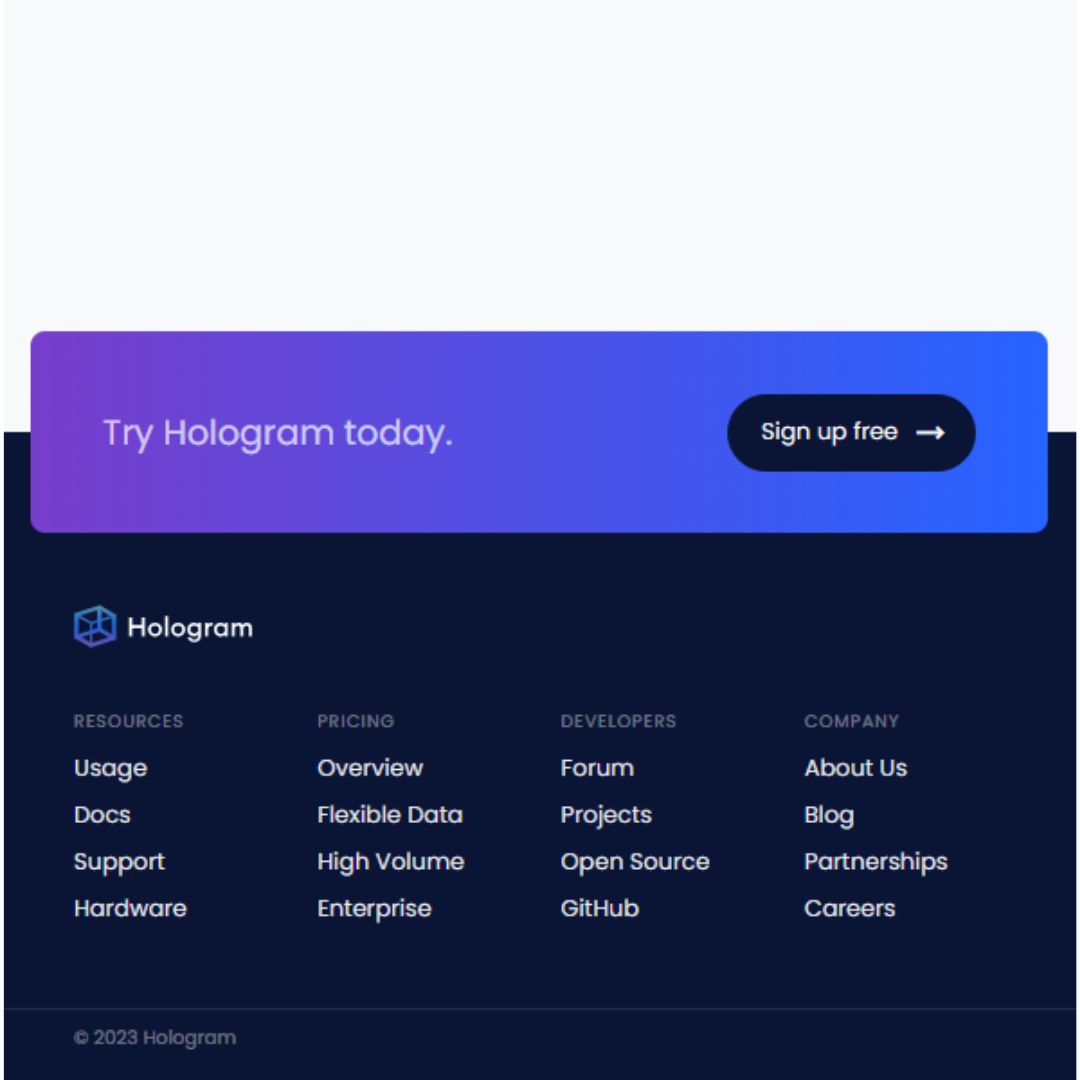
Crafting a Responsive HTML and CSS Footer (Source Code)
November 11, 2023
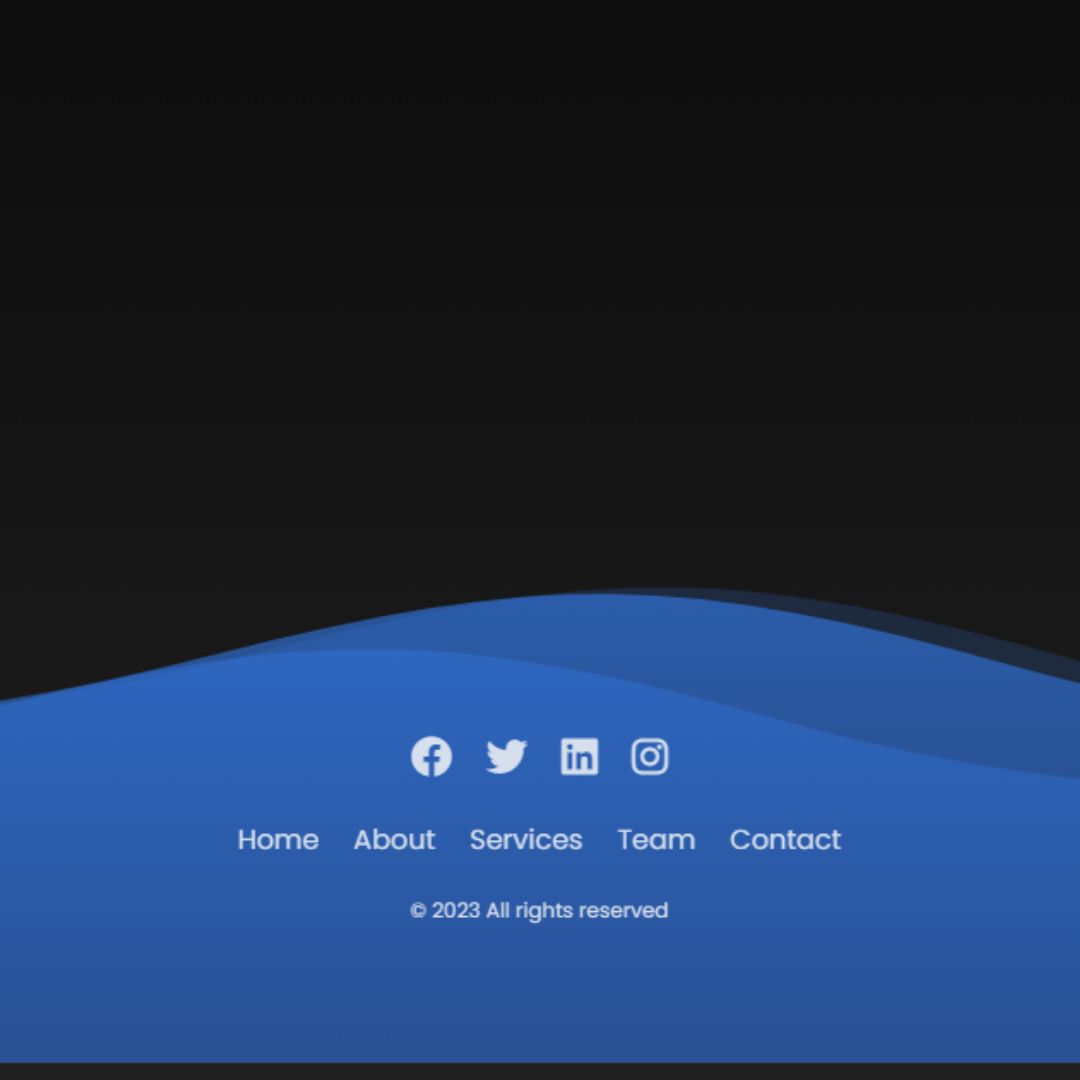
Create an Animated Footer with HTML and CSS (Source Code)
October 17, 2023
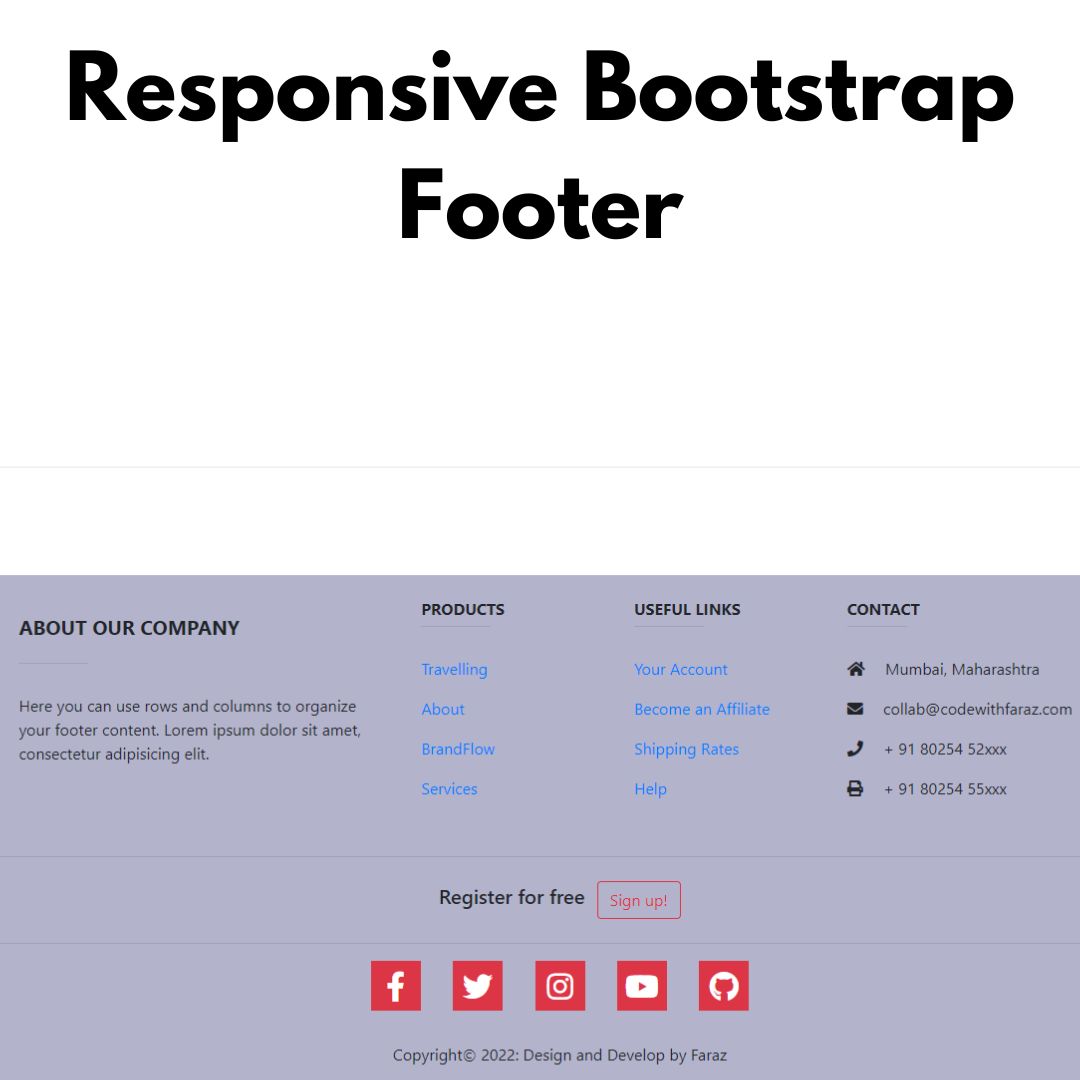
Bootstrap Footer Template for Every Website Style
March 08, 2023
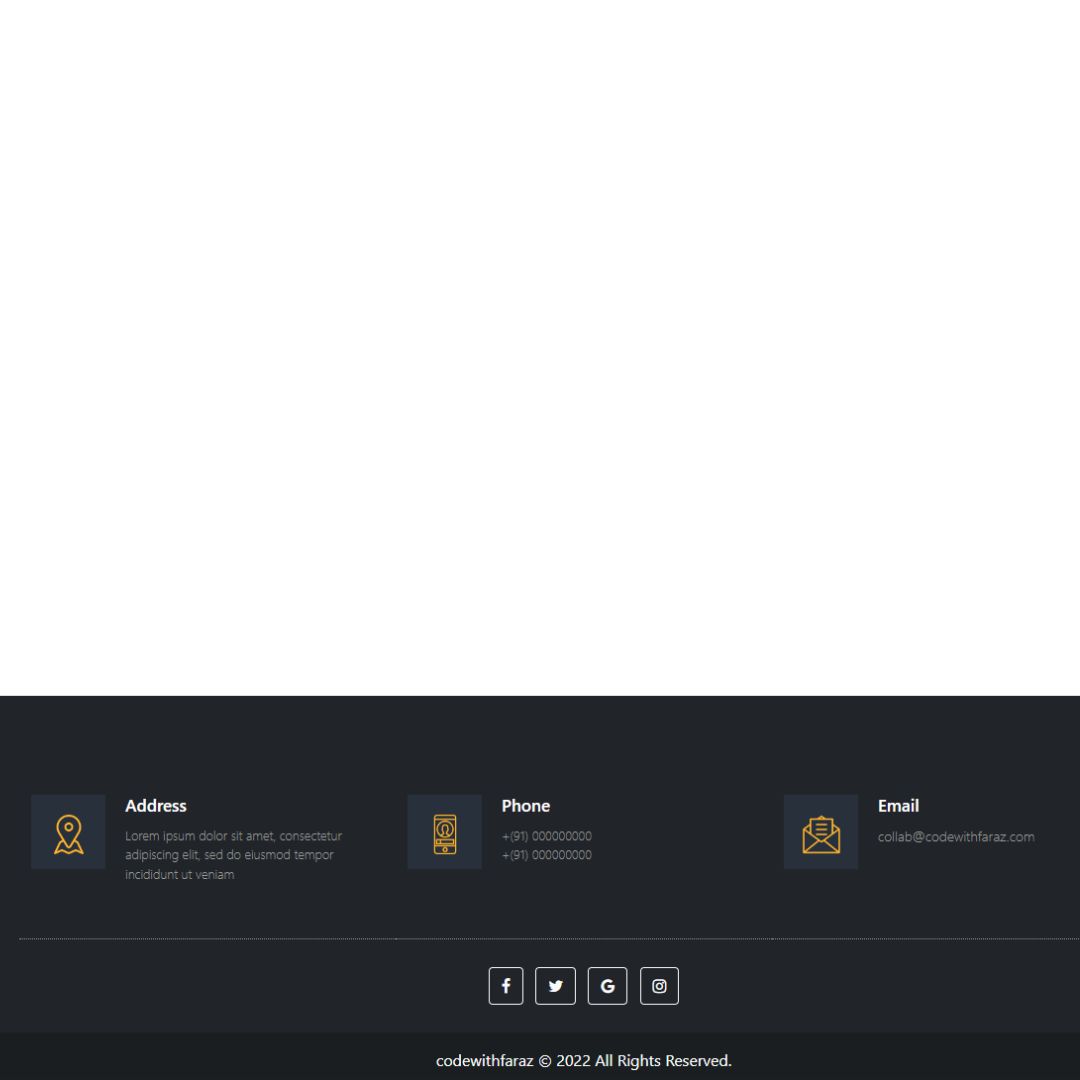
How to Create a Responsive Footer for Your Website with Bootstrap 5
August 19, 2022

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
How do you code a vending machine in Python?
In this article, we will learn to code a vending machine in Python.
Vending Machine with Python
Each item will have its product id, product name, and product cost properties stored in a dictionary. a list that is currently empty but will later be filled with all the selected items.
The value of a "run" variable is True up until the point at which the user decides they are satisfied and do not wish to buy any more products; at that point, the value is changed to False, and the loop ends.
We will now try to understand the Python code for the vending machine.
Printing Menu
A simple and direct loop is written to print the menu for the vending machine along with each item's necessary properties
Calculating Total Price
A function called sum() is created, and it iterates over the list of all the chosen items for purchase. After executing a loop over the list and adding the property of product_cost to the total, the function will then return the total amount.
The logic of Vending Machine
Machine(), the primary function of the Python program, is written in the vending machine. The three parameters this function will accept are the items_data dictionary, the run variable with a boolean value, and the item list, which includes all the items the user desires. A while loop is used, however, it only functions when the run variable's value is True.
The product id of the desired item must be entered here. If the product id is less than the total length of items_data dictionary, the entire set of id properties must be added to the list of the item; otherwise, the message "Wrong Product ID" will be printed. If the user declines, the run variable will change to False and they will be prompted to add more items. A prompt will ask if you want to print the entire bill or just the total sum.
Function for Creating Bill
The creation of a bill display on the console is another feature of Vending Machine with Python. The function create_bill() will accept two arguments −
the item list of the chosen products
the bill , which is a string of boilerplate menus, and that has been chosen.
The item's name and price are selected as it iterates over the item list, and the necessary information is printed. Finally, this code will once again output the entire cost using the previous sum () function.
Remember that this create_bill () method was created independently outside of the sum () function.
Complete Code of Python Vending Machine
Below is the complete code of above all the steps of creating a vending machine with python −
We studied how to create a vending machine program in Python and how the primary logic works in detail in this article.

Related Articles
- How do you make code reusable in C#?
- What do you mean by machine?
- How will you Convert MATLAB Code into Python Code?
- How do you write a Javascript code on the HTML page?
- How do I execute a string containing Python code in Python?
- Auto Machine Learning Python Equivalent code explained
- How do you Loop Through a Dictionary in Python?
- How do you round up a float number in Python?
- How do you remove duplicates from a list in Python?
- How do you make a higher order function in Python?
- How do you properly ignore Exceptions in Python?
- How do you make an array in Python?
- How do you implement persistent objects in Python?
- How do you append to a file with Python?
- How do you remove multiple items from a list in Python?
Kickstart Your Career
Get certified by completing the course
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
TheRakib/assignment-1-vending-machine-api-python
Folders and files, repository files navigation, vending machine api.
This API has been built for the following exercise:
Introduction
Design an API for a vending machine, allowing users with a “seller” role to add, update or remove products, while users with a “buyer” role can deposit coins into the machine and make purchases. Your vending machine should only accept 5, 10, 20, 50 and 100 cent coins
- REST API should be implemented consuming and producing “application/json”
- Implement product model with amountAvailable, cost (should be in multiples of 5), productName and sellerId fields
- Implement user model with username, password, deposit and role fields
- Implement an authentication method (basic, oAuth, JWT or something else, the choice is yours)
- All of the endpoints should be authenticated unless stated otherwise
- Implement CRUD for users (POST /user should not require authentication to allow new user registration)
- Implement CRUD for a product model (GET can be called by anyone, while POST, PUT and DELETE can be called only by the seller user who created the product)
- Implement /deposit endpoint so users with a “buyer” role can deposit only 5, 10, 20, 50 and 100 cent coins into their vending machine account (one coin at the time)
- Implement /buy endpoint (accepts productId, amount of products) so users with a “buyer” role can buy a product (shouldn't be able to buy multiple different products at the same time) with the money they’ve deposited. API should return total they’ve spent, the product they’ve purchased and their change if there’s any (in an array of 5, 10, 20, 50 and 100 cent coins)
- Implement /reset endpoint so users with a “buyer” role can reset their deposit back to 0
- Take time to think about possible edge cases and access issues that should be solved
Evaluation criteria:
- Language/Framework of choice best practices
- Edge cases covered
- Write tests for /deposit, /buy and one CRUD endpoint of your choice
- Code readability and optimization
- If somebody is already logged in with the same credentials, the user should be given a message "There is already an active session using your account". In this case the user should be able to terminate all the active sessions on their account via an endpoint i.e. /logout/all
- Attention to security
Deliverables
A Github repository with public access. Please have the solution running and a Postman / Swagger collection ready on your computer so the domain expert can tell you which tests to run on the API.
Instructions
In order to run the API, follow the following steps:
- Create a conda environment: "conda create -n vending_machine python=3.8"
- Activate the conda environment: "conda activate vending_machine"
- Pip install all requirements: "pip install -r requirements.txt"
- Navigate to the root folder of the project
- Run the following command: "python api.py"
- The command will open a link at http://0.0.0.0:8000
- Swagger endpoint can be accessed using http://0.0.0.0:8000/docs
- Python 100.0%
- Vending Machine Model Assignment Solutions
Implement a class that models a vending machine.
The vending machines we model shall be quite simple. They hold a single kind of drink, assume a bottle of water. They can accept money (the US, coins, but not pennies) and can dispense drinks and return change.
Get Free Quote
Vending machine model assignment solutions submit your homework, attached files.
- How it works
- Homework answers
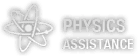
Answer to Question #306569 in Python for babu
question: Cards Game
There are M people from 1 to M . Andy has N cards with her. Starting with person Z , he gives the cards one by one to the people in the numbering order. Z, Z+1, Z+2,.....,M, 1, 2,....Z-1. Your task is to output the number of the person who will get the last card.
input 1:- 3 3 2
output 1:- 1
input 2 :- 1 100 1
output 2:- 1
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. question: Difficult AdditionArjun is trying to add two numbers. since he has learned addition recent
- 2. Question 3Rules:1. Play a simple Black Jack game.2. The player will get two cards first.3. If the pl
- 3. Machine Problem 2Create a Python script that will generate random n integers from a given start and
- 4. Machine problem 3In the game Rock Paper Scissors, two players simultaneously choose one of the three
- 5. Machine Problem 1Number Guessing GameIn the number guessing game, the computer will randomly select
- 6. Using Python class Person, write a program that will generate the output below:Hello, my name is Bob
- 7. input:The cat is on the mat. output: ehT tac si no eht .tamehT tac si no eht .tamusing split revers
- Programming
- Engineering
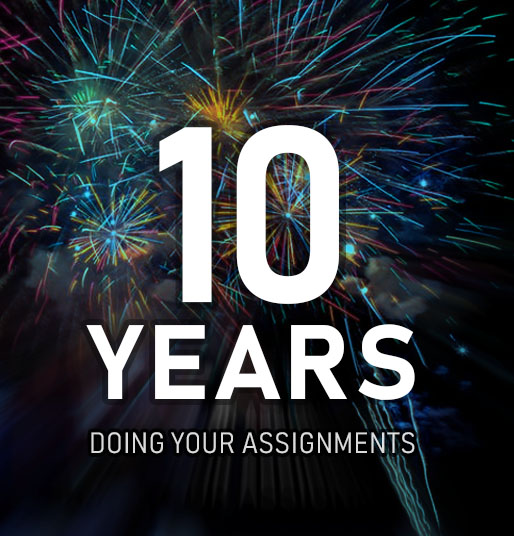
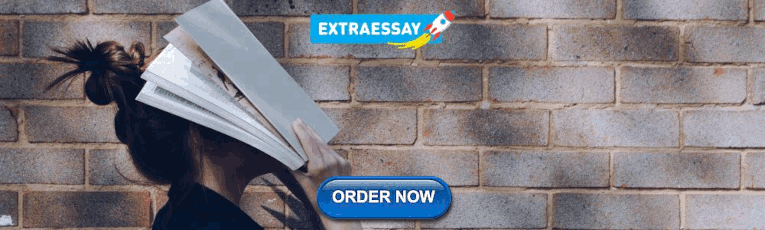
IMAGES
VIDEO
COMMENTS
2. Project Setup. Now you have python installed, create a new folder for this program, open it in a code editor if you don't have a code editor you can download and use vs code. After opening the folder in vs code you can create a python file and go to the next step of copy and pasting the vending machine python code. 3.
Answer to Question #334302 in Python for sandhya. There is a chocolate vending machine.intially it contains a total of K chocolates N people are standing in a queue from left to rigth.Each person wants to withdraw chocolates.The i-i-th person wants to take Ai units of chocolates.People come in and try to withdraw chocolates one by one,in the ...
Learn and apply programming skills: By building a vending machine with Python, you can enhance your programming knowledge and gain hands-on experience in hardware programming. It's an excellent opportunity to bridge the gap between software and hardware. Customize products and functionality: When you create your own vending machine, you have ...
This is probably better suited for the code review board, but anyways...Really great for a first project! That being said, you've already identified the major shortcoming of your code - you shouldn't have to use all those if/elifs.I also don't think you can justify wrapping most of your code into classes, like you've done.
The logic of Vending Machine. Machine(), the primary function of the Python program, is written in the vending machine. The three parameters this function will accept are the items_data dictionary, the run variable with a boolean value, and the item list, which includes all the items the user desires.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Computer Science. Computer Science questions and answers. Assignment Overview: You will create a vending machine program in Python that sells at least 5 items of your choice. You will write a program that prompts a user to select items from your vending machine with a menu. The user can select as many items as they would like and your program ...
Assignment Specification Write a Python program that includes a main function. (You must define a main function and call it). Your main function should also call the following functions: get_user_input() o Asks the user for the cost of their item. o Returns the cost of the item. calculate_correct_amount_of_change(cost_of_item)
Contribute to S-Rafhanah/Vending-Machine-Python- development by creating an account on GitHub. ... Python Assignment (214107Y) - Sri Rafhanah Bte Rudi ... View all files. Repository files navigation. README; Vending-Machine-Python-Year 1 Sem 1 Project Programming Essentials Module. 2 parts of assignment. One is based off vendor's perspective ...
Programming Project: Vending Machine Project This assignment is worth 120 points (15% of your course grade) and must be completed and turned in by Sunday, April 25, 2021 before 11:59pm. Assignment Overview: You will create a vending machine program in Python that sells at least 5 items of your choice. This assignment will give you more experience on the use of: 1. integers (int) 2. floats ...
And handle all exceptions of the system. Use Python to write a program of vending machine with GUI. Must include stock record on a txt. file and also include payment method and shopping cart. It should start running ( server & client) from CMD. And handle all exceptions of the system. Try focusing on one step at a time.
The biggest thing you can do is to use string formatting to remove redundancy. Now instead of having to repeatedly type "1 for Coke 2 for Tango Cherry 3 for Dr Pepper:, you factor out the common parts, put the foods in a list, and use that list to populate the string.I also removed your first assignment to item, (ie item = "Gum") for each, because they immediately get removed.
Contribute to TheRakib/assignment-1-vending-machine-api-python development by creating an account on GitHub.
Implement a class that models a vending machine. The vending machines we model shall be quite simple. They hold a single kind of drink, assume a bottle of water. They can accept money (the US, coins, but not pennies) and can dispense drinks and return change. Solution: # File: VendingMachine.py # # Create a class that models a vending machine.
a vending machine program in Python that sells at least 5 items of your choice. This assignment will give you more experience on the use of: integers (int) floats (float) Strings, output formatting (str) conditionals; iteration; data structures (lists) functions a program that prompts a user to select items from your vending machine with a menu.
Vending machine assignment. My assignment is to create a vending machine, I am a lost on where to start I have created the main function and assume i should begin creating functions on the coin and transaction criteria but I don't know where to begin. Any help is appreciated :) #List of products and price. products = {"Coffee":300,"Tea":250 ...
Python. Question #334308. SNAKY CONVERSION. you are given a string S print the string in N rows containing a snaky pattern represenation as described below. INPUT:the first line should be a string. explanation: s=AWESOMENESS N=4. consider the following snaky representation of the word. A E.
Please help me with my assignment for python programming class.... I create a vending machine with PYTHON. I need to do the following..... 1.Create a Program That Allows Displays and Allows Someone to Purchase Items from a Vending Machine… 2.You Should Have a Least 5 Items in the Vending Machine, Each With Varying Prices…
Programming Project 03: Vending Machine This assignment is worth 30 points (3.0% of the course grade) and must be completed and turned in before 11:59 on Monday, January 28, 2013. Assignment Overview This assignment will give you more experience on the use of: 1. integers (int) 2. floats (float) 3. strings (str) 4. conditionals 5. iteration
Machine problem 3In the game Rock Paper Scissors, two players simultaneously choose one of the three; 5. Machine Problem 1Number Guessing GameIn the number guessing game, the computer will randomly select ; 6. Using Python class Person, write a program that will generate the output below:Hello, my name is Bob; 7. input:The cat is on the mat ...
Question: Please help me with my assignment for python programming class.... I create a vending machine with PYTHON. I need to do the following..... 1.Create a Program That Allows Displays and Allows Someone to Purchase Items from a Vending Machine… 2.You Should Have a Least 5 Items in the Vending Machine, Each With Varying Prices… 3.You Need to Display the Item and