Go Tutorial
Go exercises, go assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

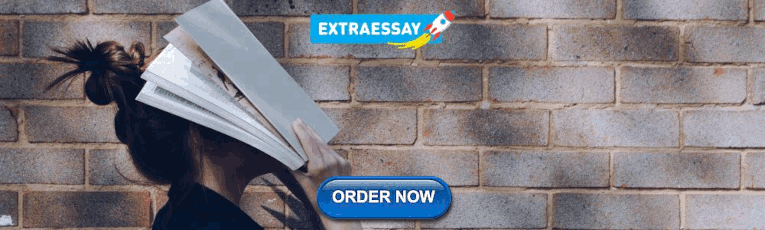
COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Popular Tutorials
Popular examples, learn python interactively, go introduction.
- Golang Getting Started
- Go Variables
- Go Data Types
- Go Print Statement
- Go Take Input
- Go Comments
Go Operators
- Go Type Casting
Go Flow Control
- Go Boolean Expression
- Go if...else
- Go for Loop
- Go while Loop
- Go break and continue
Go Data Structures
- Go Functions
- Go Variable Scope
- Go Recursion
- Go Anonymous Function
- Go Packages
Go Pointers & Interface
Go Pointers
- Go Pointers and Functions
- Go Pointers to Struct
- Go Interface
- Go Empty Interface
- Go Type Assertions
Go Additional Topics
Go defer, panic, and recover
Go Tutorials
Go Booleans (Relational and Logical Operators)
- Go Pointers to Structs
In Computer Programming, an operator is a symbol that performs operations on a value or a variable.
For example, + is an operator that is used to add two numbers.
Go programming provides wide range of operators that are categorized into following major categories:
- Arithmetic operators
- Assignment operator
- Relational operators
- Logical operators
- Arithmetic Operator
We use arithmetic operators to perform arithmetic operations like addition, subtraction, multiplication, and division.
Here's a list of various arithmetic operators available in Go.
Example 1: Addition, Subtraction and Multiplication Operators
Example 2: golang division operator.
In the above example, we have used the / operator to divide two numbers: 11 and 4 . Here, we get the output 2 .
However, in normal calculation, 11 / 4 gives 2.75 . This is because when we use the / operator with integer values, we get the quotients instead of the actual result.
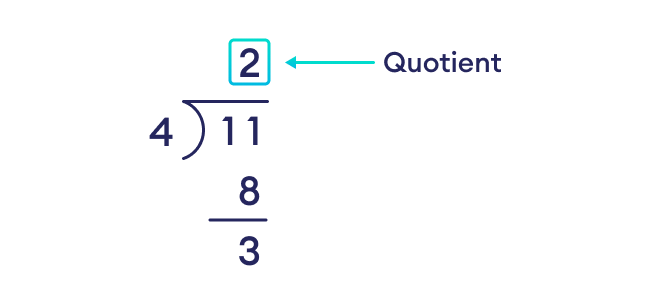
If we want the actual result we should always use the / operator with floating point numbers. For example,
Here, we get the actual result after division.
Example 3: Modulus Operator in Go
In the above example, we have used the modulo operator with numbers: 11 and 4 . Here, we get the result 3 .
This is because in programming, the modulo operator always returns the remainder after division.
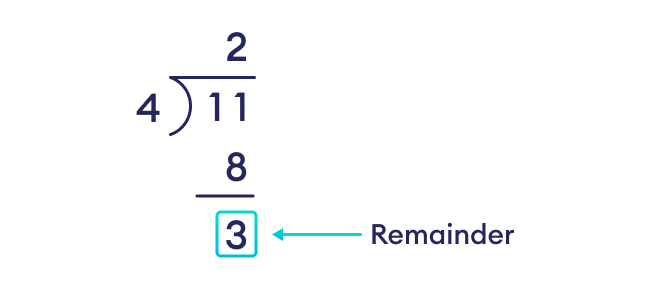
Note: The modulo operator is always used with integer values.
- Increment and Decrement Operator in Go
In Golang, we use ++ (increment) and -- (decrement) operators to increase and decrease the value of a variable by 1 respectively. For example,
In the above example,
- num++ - increases the value of num by 1 , from 5 to 6
- num-- - decreases the value of num by 1 , from 5 to 4
Note: We have used ++ and -- as prefixes (before variable). However, we can also use them as postfixes ( num++ and num-- ).
There is a slight difference between using increment and decrement operators as prefixes and postfixes. To learn the difference, visit Increment and Decrement Operator as Prefix and Postfix .
- Go Assignment Operators
We use the assignment operator to assign values to a variable. For example,
Here, the = operator assigns the value on right ( 34 ) to the variable on left ( number ).
Example: Assignment Operator in Go
In the above example, we have used the assignment operator to assign the value of the num variable to the result variable.
- Compound Assignment Operators
In Go, we can also use an assignment operator together with an arithmetic operator. For example,
Here, += is additional assignment operator. It first adds 6 to the value of number ( 2 ) and assigns the final result ( 8 ) to number .
Here's a list of various compound assignment operators available in Golang.
- Relational Operators in Golang
We use the relational operators to compare two values or variables. For example,
Here, == is a relational operator that checks if 5 is equal to 6 .
A relational operator returns
- true if the comparison between two values is correct
- false if the comparison is wrong
Here's a list of various relational operators available in Go:
To learn more, visit Go relational operators .
- Logical Operators in Go
We use the logical operators to perform logical operations. A logical operator returns either true or false depending upon the conditions.
To learn more, visit Go logical operators .
More on Go Operators
The right shift operator shifts all bits towards the right by a certain number of specified bits.
Suppose we want to right shift a number 212 by some bits then,
For example,
The left shift operator shifts all bits towards the left by a certain number of specified bits. The bit positions that have been vacated by the left shift operator are filled with 0 .
Suppose we want to left shift a number 212 by some bits then,
In Go, & is the address operator that is used for pointers. It holds the memory address of a variable. For example,
Here, we have used the * operator to declare the pointer variable. To learn more, visit Go Pointers .
In Go, * is the dereferencing operator used to declare a pointer variable. A pointer variable stores the memory address. For example,
In Go, we use the concept called operator precedence which determines which operator is executed first if multiple operators are used together. For example,
Here, the / operator is executed first followed by the * operator. The + and - operators are respectively executed at last.
This is because operators with the higher precedence are executed first and operators with lower precedence are executed last.
Table of Contents
- Introduction
- Example: Addition, Subtraction and Multiplication
- Golang Division Operator
Sorry about that.
Related Tutorials
Programming
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Go Programming Language (Introduction)
- How to Install Go on Windows?
- How to Install Golang on MacOS?
- Hello World in Golang
Fundamentals
- Identifiers in Go Language
- Go Keywords
- Data Types in Go
- Go Variables
- Constants- Go Language
Go Operators
Control statements.
- Go Decision Making (if, if-else, Nested-if, if-else-if)
- Loops in Go Language
- Switch Statement in Go
Functions & Methods
- Functions in Go Language
- Variadic Functions in Go
- Anonymous function in Go Language
- main and init function in Golang
- What is Blank Identifier(underscore) in Golang?
- Defer Keyword in Golang
- Methods in Golang
- Structures in Golang
- Nested Structure in Golang
- Anonymous Structure and Field in Golang
- Arrays in Go
- How to Copy an Array into Another Array in Golang?
- How to pass an Array to a Function in Golang?
- Slices in Golang
- Slice Composite Literal in Go
- How to sort a slice of ints in Golang?
- How to trim a slice of bytes in Golang?
- How to split a slice of bytes in Golang?
- Strings in Golang
- How to Trim a String in Golang?
- How to Split a String in Golang?
- Different ways to compare Strings in Golang
- Pointers in Golang
- Passing Pointers to a Function in Go
- Pointer to a Struct in Golang
- Go Pointer to Pointer (Double Pointer)
- Comparing Pointers in Golang
Concurrency
- Goroutines - Concurrency in Golang
- Select Statement in Go Language
- Multiple Goroutines
- Channel in Golang
- Unidirectional Channel in Golang
Operators are the foundation of any programming language. Thus the functionality of the Go language is incomplete without the use of operators. Operators allow us to perform different kinds of operations on operands. In the Go language , operators Can be categorized based on their different functionality:
Arithmetic Operators
Relational operators, logical operators, bitwise operators, assignment operators, misc operators.
These are used to perform arithmetic/mathematical operations on operands in Go language:
- Addition: The ‘+’ operator adds two operands. For example, x+y.
- Subtraction: The ‘-‘ operator subtracts two operands. For example, x-y.
- Multiplication: The ‘*’ operator multiplies two operands. For example, x*y.
- Division: The ‘/’ operator divides the first operand by the second. For example, x/y.
- Modulus: The ‘%’ operator returns the remainder when the first operand is divided by the second. For example, x%y.
Note: -, +, !, &, *, <-, and ^ are also known as unary operators and the precedence of unary operators is higher. ++ and — operators are from statements they are not expressions, so they are out from the operator hierarchy.
Example:
Output:
Relational operators are used for the comparison of two values. Let’s see them one by one:
- ‘=='(Equal To) operator checks whether the two given operands are equal or not. If so, it returns true. Otherwise, it returns false. For example, 5==5 will return true.
- ‘!='(Not Equal To) operator checks whether the two given operands are equal or not. If not, it returns true. Otherwise, it returns false. It is the exact boolean complement of the ‘==’ operator. For example, 5!=5 will return false.
- ‘>'(Greater Than) operator checks whether the first operand is greater than the second operand. If so, it returns true. Otherwise, it returns false. For example, 6>5 will return true.
- ‘<‘(Less Than) operator checks whether the first operand is lesser than the second operand. If so, it returns true. Otherwise, it returns false. For example, 6<5 will return false.
- ‘>='(Greater Than Equal To) operator checks whether the first operand is greater than or equal to the second operand. If so, it returns true. Otherwise, it returns false. For example, 5>=5 will return true.
- ‘<='(Less Than Equal To) operator checks whether the first operand is lesser than or equal to the second operand. If so, it returns true. Otherwise, it returns false. For example, 5<=5 will also return true.
They are used to combine two or more conditions/constraints or to complement the evaluation of the original condition in consideration.
- Logical AND: The ‘&&’ operator returns true when both the conditions in consideration are satisfied. Otherwise it returns false. For example, a && b returns true when both a and b are true (i.e. non-zero).
- Logical OR: The ‘||’ operator returns true when one (or both) of the conditions in consideration is satisfied. Otherwise it returns false. For example, a || b returns true if one of a or b is true (i.e. non-zero). Of course, it returns true when both a and b are true.
- Logical NOT: The ‘!’ operator returns true the condition in consideration is not satisfied. Otherwise it returns false. For example, !a returns true if a is false, i.e. when a=0.
In Go language, there are 6 bitwise operators which work at bit level or used to perform bit by bit operations. Following are the bitwise operators :
- & (bitwise AND): Takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
- | (bitwise OR): Takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 any of the two bits is 1.
- ^ (bitwise XOR): Takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
- << (left shift): Takes two numbers, left shifts the bits of the first operand, the second operand decides the number of places to shift.
- >> (right shift): Takes two numbers, right shifts the bits of the first operand, the second operand decides the number of places to shift.
- &^ (AND NOT): This is a bit clear operator.
Assignment operators are used to assigning a value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error. Different types of assignment operators are shown below:
- “=”(Simple Assignment): This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left.
- “+=”(Add Assignment): This operator is a combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
- “-=”(Subtract Assignment): This operator is a combination of ‘-‘ and ‘=’ operators. This operator first subtracts the current value of the variable on left from the value on the right and then assigns the result to the variable on the left.
- “*=”(Multiply Assignment): This operator is a combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
- “/=”(Division Assignment): This operator is a combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “%=”(Modulus Assignment): This operator is a combination of ‘%’ and ‘=’ operators. This operator first modulo the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “&=”(Bitwise AND Assignment): This operator is a combination of ‘&’ and ‘=’ operators. This operator first “Bitwise AND” the current value of the variable on the left by the value on the right and then assigns the result to the variable on the left.
- “^=”(Bitwise Exclusive OR): This operator is a combination of ‘^’ and ‘=’ operators. This operator first “Bitwise Exclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “|=”(Bitwise Inclusive OR): This operator is a combination of ‘|’ and ‘=’ operators. This operator first “Bitwise Inclusive OR” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “<<=”(Left shift AND assignment operator): This operator is a combination of ‘<<’ and ‘=’ operators. This operator first “Left shift AND” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- “>>=”(Right shift AND assignment operator): This operator is a combination of ‘>>’ and ‘=’ operators. This operator first “Right shift AND” the current value of the variable on left by the value on the right and then assigns the result to the variable on the left.
- &: This operator returns the address of the variable.
- *: This operator provides pointer to a variable.
- <-: The name of this operator is receive. It is used to receive a value from the channel.
Please Login to comment...
Similar reads.
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
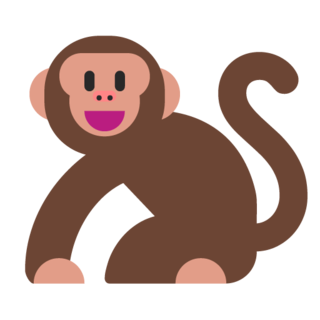
Go Assignment Operators
What are assignment operators.
Assignment operators are used to assign variables to values.
The Assignment Operator ( = )
The equal sign that we are all familiar with is called the assignment operator, because it is the most important out of all the assignment operators. All the other assignment operators are built off of it. For example, the below code assigns the variable a the value of 3, the constant pi the value of 3.14, and the variable website the value of Learnmonkey:
Notice that we can use the assignment operator to make constants and variables. We can also use the assignment operator to set variables (not constants) once they are already created:
Other Assignment Operators
The other assignment operators were created so that as developers, our lives would be easier. They are all equivalent to using the assignment operator in some way:
- Introduction to Go
- Environment Setup
- Your First Go Program
- More on Printing
- PyQt5 ebook
- Tkinter ebook
- SQLite Python
- wxPython ebook
- Windows API ebook
- Java Swing ebook
- Java games ebook
- MySQL Java ebook
Go operators
last modified April 11, 2024
In this article we cover Go operators. We show how to use operators to create expressions.
We use Go version 1.22.2.
An operator is a special symbol which indicates a certain process is carried out. Operators in programming languages are taken from mathematics. Programmers work with data. The operators are used to process data. An operand is one of the inputs (arguments) of an operator.
Expressions are constructed from operands and operators. The operators of an expression indicate which operations to apply to the operands. The order of evaluation of operators in an expression is determined by the precedence and associativity of the operators.
An operator usually has one or two operands. Those operators that work with only one operand are called unary operators . Those who work with two operands are called binary operators .
Certain operators may be used in different contexts. For instance the + operator can be used in different cases: it adds numbers, concatenates strings, or indicates the sign of a number. We say that the operator is overloaded .
Go sign operators
There are two sign operators: + and - . They are used to indicate or change the sign of a value.
The + and - signs indicate the sign of a value. The plus sign can be used to signal that we have a positive number. It can be omitted and it is in most cases done so.
The minus sign changes the sign of a value.
Go assignment operator
The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one.
Here we assign a number to the x variable.
This expression does not make sense in mathematics, but it is legal in programming. The expression adds 1 to the x variable. The right side is equal to 2 and 2 is assigned to x .
This code line leads to a syntax error. We cannot assign a value to a literal.
Go has a short variable declaration operator := ; it declares a variable and assigns a value in one step. The x := 2 is equal to var x = 2 .
Go increment and decrement operators
We often increment or decrement a value by one in programming. Go has two convenient operators for this: ++ and -- .
In the above example, we demonstrate the usage of both operators.
We initiate the x variable to 6. Then we increment x two times. Now the variable equals to 8.
We use the decrement operator. Now the variable equals to 7.
Go compound assignment operators
The compound assignment operators consist of two operators. They are shorthand operators.
The += compound operator is one of these shorthand operators. The above two expressions are equal. Value 3 is added to the a variable.
Other compound operators include:
In the code example, we use two compound operators.
The a variable is initiated to one. 1 is added to the variable using the non-shorthand notation.
Using a += compound operator, we add 5 to the a variable. The statement is equal to a = a + 5 .
Using the *= operator, the a is multiplied by 3. The statement is equal to a = a * 3 .
Go arithmetic operators
The following is a table of arithmetic operators in Go.
The following example shows arithmetic operations.
In the preceding example, we use addition, subtraction, multiplication, division, and remainder operations. This is all familiar from the mathematics.
The % operator is called the remainder or the modulo operator. It finds the remainder of division of one number by another. For example, 9 % 4 , 9 modulo 4 is 1, because 4 goes into 9 twice with a remainder of 1.
Next we will show the distinction between integer and floating point division.
In the preceding example, we divide two numbers.
In this code, we have done integer division. The returned value of the division operation is an integer. When we divide two integers the result is an integer.
If one of the values is a double or a float, we perform a floating point division. In our case, the second operand is a double so the result is a double.
Go Boolean operators
In Go we have three logical operators.
Boolean operators are also called logical.
Many expressions result in a boolean value. For instance, boolean values are used in conditional statements.
Relational operators always result in a boolean value. These two lines print false and true.
The body of the if statement is executed only if the condition inside the parentheses is met. The y > x returns true, so the message "y is greater than x" is printed to the terminal.
The true and false keywords represent boolean literals in Go.
The code example shows the logical and (&&) operator. It evaluates to true only if both operands are true.
Only one expression results in true.
The logical or ( || ) operator evaluates to true if either of the operands is true.
If one of the sides of the operator is true, the outcome of the operation is true.
Three of four expressions result in true.
The negation operator ! makes true false and false true.
The example shows the negation operator in action.
Go comparison operators
Comparison operators are used to compare values. These operators always result in a boolean value.
comparison operators are also called relational operators.
In the code example, we have four expressions. These expressions compare integer values. The result of each of the expressions is either true or false. In Go we use the == to compare numbers. (Some languages like Ada, Visual Basic, or Pascal use = for comparing numbers.)
Go bitwise operators
Decimal numbers are natural to humans. Binary numbers are native to computers. Binary, octal, decimal, or hexadecimal symbols are only notations of the same number. Bitwise operators work with bits of a binary number.
The bitwise and operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 only if both corresponding bits in the operands are 1.
The first number is a binary notation of 6, the second is 3, and the result is 2.
The bitwise or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if either of the corresponding bits in the operands is 1.
The result is 00110 or decimal 7.
The bitwise exclusive or operator performs bit-by-bit comparison between two numbers. The result for a bit position is 1 if one or the other (but not both) of the corresponding bits in the operands is 1.
The result is 00101 or decimal 5.
Go pointer operators
In Go, the & is an address of operator and the * is a pointer indirection operator.
In the code example, we demonstrate the two operators.
An integer variable is defined.
We get the address of the count variable; we create a pointer to the variable.
Via the pointer dereference, we modify the value of count.
Again, via pointer dereference, we print the value to which the pointer refers.
Go channel operator
A channels is a typed conduit through which we can send and receive values with the channel operator <- .
The example presents the channel operator.
We send a value to the channel.
We receive a value from the channel.
Go operator precedence
The operator precedence tells us which operators are evaluated first. The precedence level is necessary to avoid ambiguity in expressions.
What is the outcome of the following expression, 28 or 40?
Like in mathematics, the multiplication operator has a higher precedence than addition operator. So the outcome is 28.
To change the order of evaluation, we can use parentheses. Expressions inside parentheses are always evaluated first. The result of the above expression is 40.
In this code example, we show a few expressions. The outcome of each expression is dependent on the precedence level.
This line prints 28. The multiplication operator has a higher precedence than addition. First, the product of 5 * 5 is calculated, then 3 is added.
The evaluation of the expression can be altered by using round brackets. In this case, the 3 + 5 is evaluated and later the value is multiplied by 5. This line prints 40.
In this case, the negation operator has a higher precedence than the bitwise or. First, the initial true value is negated to false, then the | operator combines false and true, which gives true in the end.
Associativity rule
Sometimes the precedence is not satisfactory to determine the outcome of an expression. There is another rule called associativity . The associativity of operators determines the order of evaluation of operators with the same precedence level.
What is the outcome of this expression, 9 or 1? The multiplication, deletion, and the modulo operator are left to right associated. So the expression is evaluated this way: (9 / 3) * 3 and the result is 9.
Arithmetic, boolean and relational operators are left to right associated. The ternary operator, increment, decrement, unary plus and minus, negation, bitwise not, type cast, object creation operators are right to left associated.
In the code example, we the associativity rule determines the outcome of the expression.
The compound assignment operators are right to left associated. We might expect the result to be 1. But the actual result is 0. Because of the associativity. The expression on the right is evaluated first and then the compound assignment operator is applied.
The Go Programming Language Specification
In this article we have covered Go operators.
My name is Jan Bodnar and I am a passionate programmer with many years of programming experience. I have been writing programming articles since 2007. So far, I have written over 1400 articles and 8 e-books. I have over eight years of experience in teaching programming.
List all Go tutorials .

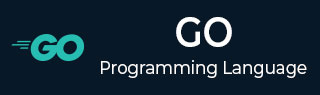
- Go Tutorial
- Go - Overview
- Go - Environment Setup
- Go - Program Structure
- Go - Basic Syntax
- Go - Data Types
- Go - Variables
- Go - Constants
- Go - Operators
- Go - Decision Making
- Go - Functions
- Go - Scope Rules
- Go - Strings
- Go - Arrays
- Go - Pointers
- Go - Structures
- Go - Recursion
- Go - Type Casting
- Go - Interfaces
- Go - Error Handling
- Go Useful Resources
- Go - Questions and Answers
- Go - Quick Guide
- Go - Useful Resources
- Go - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Go - Assignment Operators
The following table lists all the assignment operators supported by Go language −
Try the following example to understand all the assignment operators available in Go programming language −
When you compile and execute the above program it produces the following result −
To Continue Learning Please Login
- Getting started with Go
- Awesome Book
- Awesome Community
- Awesome Course
- Awesome Tutorial
- Awesome YouTube
- Base64 Encoding
- Best practices on project structure
- Build Constraints
- Concurrency
- Console I/O
- Cross Compilation
- Cryptography
- Developing for Multiple Platforms with Conditional Compiling
- Error Handling
- Executing Commands
- Getting Started With Go Using Atom
- HTTP Client
- HTTP Server
- Inline Expansion
- Installation
- JWT Authorization in Go
- Memory pooling
- Object Oriented Programming
- Panic and Recover
- Parsing Command Line Arguments And Flags
- Parsing CSV files
- Profiling using go tool pprof
- Protobuf in Go
- Select and Channels
- Send/receive emails
- Text + HTML Templating
- The Go Command
- Type conversions
- Basic Variable Declaration
- Blank Identifier
- Checking a variable's type
- Multiple Variable Assignment
- Worker Pools
- Zero values
Go Variables Multiple Variable Assignment
Fastest entity framework extensions.
In Go, you can declare multiple variables at the same time.
If a function returns multiple values, you can also assign values to variables based on the function's return values.
Got any Go Question?

- Advertise with us
- Cookie Policy
- Privacy Policy
Get monthly updates about new articles, cheatsheets, and tricks.
Let's start a new assignment project together, Get Exclusive Free Assistance Now!

Need help? Click to chat :
- Assignment Writing Service
- Assignment Editing Service
- Assignment Masters
- Assignment Provider
- Buy Assignment Online
- Do My Assignment
- Assignment Writers
- College Assignment Help
- Assignment Help Canberra
- Instant Assignment Help
Assignment Help Sydney
Assignment help melbourne.
- All Assignment Help
Assignment Help Perth
- Assignment Help Adelaide
- Make My Assignment
Assignment Help Brisbane
- Assignment Help UAE
- My Assignment Help
- Essay Writing Service
- Online Essay Help
- Do My Essay
- Write My Essay
- Essay Assignment Help
- Essay Writer
- Essay Typer
- College Essay Writing Service
- Essay Editor
- Types Of Essays
- Expository Essays
- Types Of Expository Essays
- Narrative Essays
- Narrative Essay Examples
- Narrative Essay Hooks
- Narrative Essay Childhood Memory
- Descriptive Essay About An Event
- Types Of Essays In Ielts
- Application Essay Writing
- Argumentative Essay
- Global Warming Essay
- Critical Analysis Essay
- Academic Essay
- University Essay
- Education Essay
- 2000 Word Essay
- Marketing Essay
- Economics Essay
- Literature Essay
- Discursive Essay
- Reflective Essay
- Analytical Essay
- Comparative Essay
- Persuasive Essay
- English Essay
- Discussion Essay
- Expository Essay Examples
- 1000 Word Essay
- Personal Essay
- 500 Word Essay
- Animal Farm Essay
- Technology Essay
- Scholarship Essay
- Philosophy Essay
- Creative Essay
- Scientific Essay
- Descriptive Essay
- History Essay
- Business Essay
- Expository Essay Topics
- Response Essay
- Illustration Essay
- 3000 Word Essay
- Evaluative Essay
- Argumentative Essay Help
- Criminal Law Essay
- Paper Writing Service
- Research Paper Help
- Term Paper Help
- Write My paper
- Paper Editor
- Research Proposal Help
- Thesis Writing Help
- Thesis Statement Help
- Homework Help
- Do My Homework
- Statistics Homework Help
- Physics Homework Help
- Word Problem Solver
- Accounting Homework Help
- Math Homework Help
- Solve my Math Problem
- College Homework Help
- Online Tutoring Service
- Algebra Homework Help
- CPM Homework Help
- Homework Answers
- Lab Report Help
- Book Review Help
- Book Report Help
- Business Report Writing
- University Assignment Help
- Monash University Assignment Help
- University Of Southern Queensland Assignment Help
- Edith Cowan University Ecu Assignment Help
- University Of New South Wales Unsw Assignment Help
- Federation University Feduni Assignment Help
- Charles Darwin University Cdu Assignment Help
- University Of Notre Dame Unda Assignment Help
- Holmes Institute Assignment Help
- James Cook University Jcu Assignment Help
- Australian National University Anu Assignment Help
- Flinders University Assignment Help
- Deakin University Assignment Help
- Australian Catholic University Acu Assignment Help
- Swinburne University Of Technology Assignment Help
- Victoria University Vu Assignment Help
- University Of Queensland Uq Assignment Help
- International College Of Management Sydney Assignment Help
- Southern Cross University Scu Assignment Help
- Queensland University Of Technology Qut Assignment Help
- University Of Adelaide Assignment Help
- Griffith University Assignment Help
- University Of Newcastle Assignment Help
- Bond University Assignment Help
- University Of South Australia Unisa Assignment Help
- Rmit Assignment Help
- Curtin University Assignment Help
- Central Queensland University Cqu Assignment Help
- Charles Sturt University Csu Assignment Help
- Resume Writing Services
- Annotated Bibliography
- Ghostwriter
- Personal Statement Help
- Speech Writer
- Proofreading Services
- Capstone Project Help
- computer science assignment help
- computation assignment help
- dbms assignment help
- microprocessor assignment help
- oracle assignment help
- pascal assignment help
- perl assignment help
- ruby assignment help
- sql assignment help
- uml assignment help
- web designing assignment help
- Network Security Assignment Help
- Operating System Assignment Help
- Cloud Computing Assignment Help
- Linux Assignment Help
- Assembly Language Assignment Help
- Computer Network Assignment Help
- Software Engineering Assignment Help
- Visual Basic Assignment Help
- medical assignment help
- epidemiology assignment help
- nursing assignment help
- pharmacology assignment help
- psychology assignment help
- management assignment help
- brand management assignment help
- construction management assignment help
- customer relationship management
- healthcare management assignment help
- mba assignment help
- myob assignment help
- recruitment assignment help
- strategy analysis assignment help
- Leadership Assignment Help
- Change Management Assignment Help
- Strategic Management Assignment Help
- Human Resource Management Assignment Help
- It Management Assignment Help
- Operation Research Assignment Help
- Hospitality Management Assignment Help
- Project Management Assignment Help
- Product Development Assignment Help
- Operation Management Assignment Help
- Risk Management Assignment Help
- economics assignment help
- pricing strategy assignment help
- Macroeconomics Assignment Help
- Microeconomics Assignment Help
- Managerial Economics Assignment Help
- business assignment help
- business analytics assignment help
- business communication assignment help
- Business Plan Assignment Help
- business statistics assignment help
- business decision making assignment help
- business environment assignment help
- business development assignment help
- business ethics assignment help
- business intelligence assignment help
- marketing assignment help
- e commerce assignment help
- Marketing Plan Assignment Help
- Digital Marketing Assignment Help
- Marketing Mix Assignment Help
- Marketing Research Assignment Help
- Communication Assignment Help
- finance assignment help
- international finance assignment help
- quantitative analysis assignment help
- Bookkeeping Assignment Help
- Accounting Assignment Help
- Capital Budgeting Assignment Help
- Corporate Accounting Assignment Help
- Corporate Finance Assignment Help
- Cost Accounting Assignment Help
- Managerial Accounting Assignment Help
- Personal Finance Assignment Help
- Financial Statement Analysis Assignment Help
- perdisco assignment help
- auditing assignment help
- engineering assignment help
- engineering mathematics assignment help
- civil engineering assignment help
- transportation assignment
- electronics assignment help
- geotechnical engineering assignment help
- telecommunication assignment help
- biomedical engineering assignment help
- Fluid Mechanics Assignment Help
- Environmental Engineering Assignment Help
- mechanical engineering assignment help
- system analysis and design assignment help
- data mining assignment help
- data structure assignment help
- autocad assignment help
- aerospace engineering assignment help
- artificial intelligence assignment help
- data analysis assignment help
- electrical assignment help
- chemical engineering assignment help
- humanities assignment help
- rationalism assignment help
- philosophy assignment help
- religion assignment help
- arts assignment help
- geography assignment help
- history assignment help
- science assignment help
- physics assignment help
- biology assignment help
- botany assignment help
- bioinformatics assignment help
- biochemistry assignment help
- biotechnology assignment help
- chemistry assignment help
- microbiology assignment help
- mathematics assignment help
- eviews assignment help
- linear programming assignment help
- minitab assignment help
- probability assignment help
- spss assignment help
- stata assignment help
- algebra assignment help
- geometry assignment help
- calculus assignment help
- trigonometry assignment help
- statistics assignment help
- programming assignment help
- android assignment help
- c programing assignment help
- c sharp assignment help
- c plus plus assignment help
- fortran assignment help
- haskell assignment help
- html assignment help
- java assignment help
- python programming assignment help
- sap assignment help
- web programming assignment help
- excel assignment help
- PHP assignment help
- matlab assignment help
- javascript assignment help
- R assignment help
- Law Assignment Help
- Taxation Law Aassignment Help
- Constitutional Law Assignment help
- contract law assignment help
- civil law assignment help
- company law assignment help
- property law assignment help
- international law assignment help
- human rights law assignment help
- business law assignment help
- administrative law assignment help
- corporate law assignment help
- criminal law assignment help
- employment law assignment help
- commercial law assignment help
- agriculture assignment help
- anthropology assignment help
- childcare assignment help
- english assignment help
- fashion assignment help
- music assignment help
- tourism assignment help
- sociology assignment help
- astronomy assignment help
- biostatistics assignment help
- media assignment help
- real estate assignment help
- linguistics assignment help
- cryptography assignment help
- hnd assignment help
- Kaplan Assignment Answer Help
- nursing assignment help brisbane
- cookery assignment help
- entrepreneurship assignment help
- game theory assignment help
- genetics assignment help
- mass communication assignment help
- public relations assignment help
- research assignment help
- anatomy assignment help
- political science assignment help
- How It Works
Struggling with Your Assignment? Get FREE Consultation!
Australia’s #1 assignment help service.
Find expert academic writers for your assignments. Get 100% plagiarism-free work. GoAssignmentHelp offers 24/7 study help at affordable prices! We feature:
- Free plagiarism check
- 100% satisfaction guarantee
- 100% confidentiality
- Quick turnaround times
- Work in any citation style you choose
- High-quality papers
Trusted By 35,000+ Students Of :
The Best Assignment Help in Australia by PhD Experts!
Say goodbye to all your assignment woes with the best assignment help experts in Australia! We help you get your assignments done in no time. Thousands of happy students recommend us!
Do you need to submit your assignment tomorrow? We are the best academic support providers in Australia and can write essays, papers, or do any other kind of academic writing task for you.
Get Expert Help with Your Assignments!
Get the study help right when you need it. We have a team of academic experts who are always ready to help you with your studies. Whether it's 3 am or 3 pm, Anzac Day or Boxing Day, we are here to help you.
Boost your academic grades with native subject experts pan-Australia. Connect with a tutor in Brisbane, Sydney, Melbourne, Perth or anywhere else in Australia now!
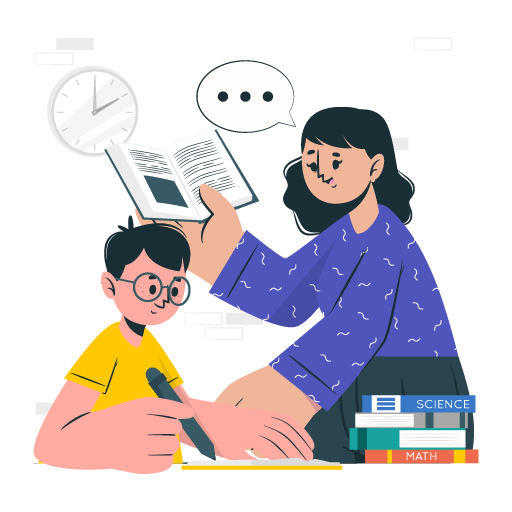
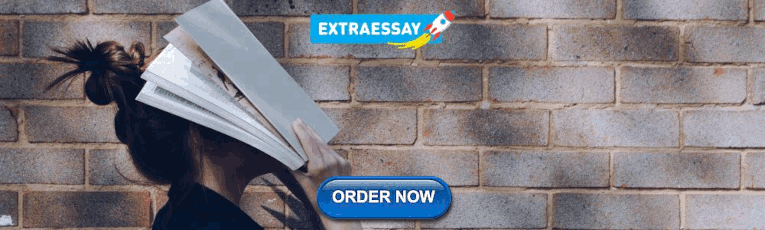
3-STEP ASSIGNMENT HELP PROCESS WE FOLLOW!
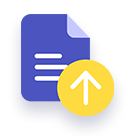
Ask Questions
We're ready for you! Share your assignment topic, submission deadline and other requirements with us. Find out what we have in store for you now! Quick, simple and secure - all within 15 minutes.
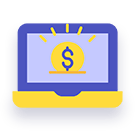
Pay Us Online
We accept PayPal, Debit Cards or Credit Card transactions. Our payment channels are safe, secure and encrypted. We consider your payment as confirmation of the order.
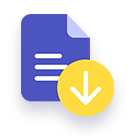
Get Answers
We take PayPal, Debit Cards or Credit Card payments. Our payment channels are safe and secure! We never share your information with any other company - ever!
Most Comprehensive and Best-Value Online Assignment Services!
- Assignment Writing Services
- Homework Help Service
- Resume Writing Service
Looking for assignment help Australia service? You've come to the right place! At GoAssignmentHelp, we offer professional assignment writing services to students of all levels. Whether you're struggling with a complex assignment or just need a little extra help, our expert writers are here to assist you.
We understand the challenges that students face, and we're committed to providing the best possible service. With our assignment help, you can be confident that your assignment will be well-written and free of errors. So what are you waiting for? Contact us today and let us help you get the grade you deserve!
Are you struggling to write your essays? If you're finding it difficult to get your ideas down on paper, or if you're worried about meeting the word count, then you may be considering using an essay writing service. Our Australian assignment help experts understand that not everyone is a natural-born writer. That's why we offer a range of online assignment help services to suit your needs.
Whether you need help with planning and structuring your essay, or you're looking for someone to proofread and edit your work, we can provide the support you need to get the best possible results. And because we offer our services online, we can easily accommodate students from all over Australia. So if you're looking for assignment help in Australia, don't hesitate to get in touch. We'll be happy to discuss your needs and provide a no-obligation quote.
Assignment help services in Australia are a boon for students across the country. GoAssignmentHelp offers the best assignment help in Australia if you need professional assistance with writing papers. Our assignment help Au experts include paper writers who offer the best paper writing services at very affordable rates so that students can focus on their studies and score well in their exams.
These service providers can assist you with essay writing, term paper writing, research paper writing, and thesis writing. Hence, students can rely on these service providers for all their academic needs. Students can avail of these services by submitting their requirements online and receiving assignment help within the specified deadline.
Australian students are some of the busiest in the world. They have a lot of responsibilities, and homework is just one of them. Unfortunately, not all students have the time or the ability to complete their homework on their own. That's where our Australian assignment writing service comes in.
We provide online assignment help to school students who need it. We have a team of experienced writers who can take care of any assignment, no matter how difficult it may be. So if you're struggling with your homework, don't hesitate to contact us. We'll be happy to help you get the grades you deserve.
When it comes to university assignment help, there are many online resources available to students. Our assignment help online experts are highly qualified and experienced. They can provide valuable assistance with researching and writing essays, term papers, and other types of assignments. Our assignment helpers can also offer tips and advice on how to improve your grades and performance in class.
Our Australia assignment help service is an excellent resource for students who need assistance with their studies. We offer customised assignment solutions that meet university guidelines and the grading criteria used to assess them. These online services can provide you with the support you need to succeed in your studies and get the most out of your university experience.
Looking for a reliable resume writing service? GoAssignmentHelp's online assignment help Australia service offers you the best resume writing services at an affordable price. Our team of expert writers will work with you to create a personalized resume that highlights your skills and experience. We know what employers are looking for, so we'll make sure your resume stands out from the rest.
We have a team of professional writers who are experts in resume writing and have years of experience in the field. Though they are part of our cheap assignment help Australia team, they can create a resume that is tailored to your specific needs and will get you the scholarship, internship or job you want. Contact us today to get started.
Bonus Features We Offer for Free @GoAssignmentHelp!
Get a 30% discount on your first order, find australian assignment help experts for 270+ subjects.

Physics assignment helpers on our platform are quite popular with students of the University of Sydney, UNSW Sydney, University of Melbourne, the University of Queensland, RMIT University and more because they are functionally competent, have the best resources available, and can help with research designing, performing a literature review, writing a project proposal, solving numerical, using related software, and more.

Information Technology
If you're stuck with an IT project or need help understanding a concept, our IT assignment writers will be able to provide the best possible guidance and support. We have over 100K satisfied Information Technology students from all over Australia including Monash University, University of Sydney, University of Melbourne, Queensland University of Technology and more.

- Engineering
Our engineering assignment writing experts possess in-depth knowledge of various concepts and can provide expert guidance on topics such as electrical engineering, mechanical engineering, civil engineering, computer science and more. We have helped students from universities such as the University of Queensland, Griffith University, Monash University, RMIT University, University of Melbourne and more.

Programming Assignment Help
Avail our Programming Assignment Help in Australia now and forget all your worries. We offer a 100% error-free solutions at extremely affordable prices for all programming languages such as C, C++, C#, JAVA, Python, AI and Machine Learning etc. Submit your assignments queries today and find the best programming solutions by qualified experts. We assure top quality programming assignment help service with the shortest turn-around time for delivering solutions.

Health Studies
We have a team of experienced and certified health professionals who can provide expert guidance on a wide range of topics such as nursing, medicine, nutrition, dietetics, exercise science, and more. We have helped students from the top medical and nursing schools across Australia, such as the University of Sydney, Monash University, Deakin University, La Trobe University and more.

Management assignment writers on our team include experienced business professionals who can guide topics such as marketing, finance, human resources, project management, and more. We have helped students from the top business schools in Australia, including the University of Melbourne, Monash University, The University of Queensland Business School, and more.

Food & Hospitality
Our food and hospitality assignment helpers are experienced professionals who can provide guidance on topics such as catering, event management, food science, tourism, and more. We have helped students from the top hospitality and tourism schools in Australia, such as the Blue Mountains International Hotel Management School, William Angliss Institute, the William Blue College of Hospitality Management, Le Cordon Bleu Australia, and more.

Nursing Assignment Help
Now you are just a click away from your dream grades in Nursing by getting help with nursing assignments. Avail Australia’s best nursing assignment help services and reduce your academic burden of submitting assignments within the deadline. Our Nursing assignment writing service in Australia is considered the best among all!
Find Instant Assignment Help Wherever You Are By Native Experts!
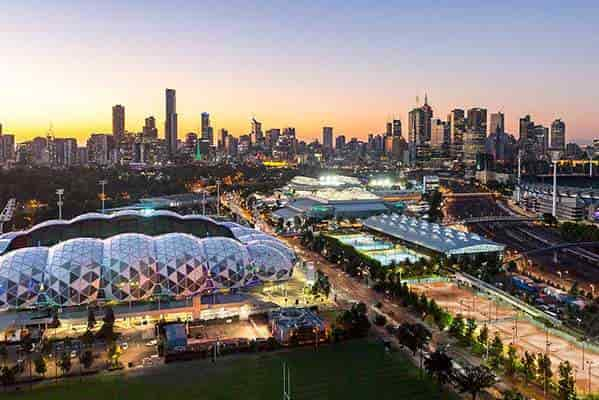
Melbourne is one of the most popular study destinations in Australia and is home to some of the top universities such as the University of Melbourne, Monash University, RMIT University and more. Our assignment help experts in Melbourne include experts from different communities, different industries and different academic disciplines. Connect with a tutor of your choice and get the best help now!
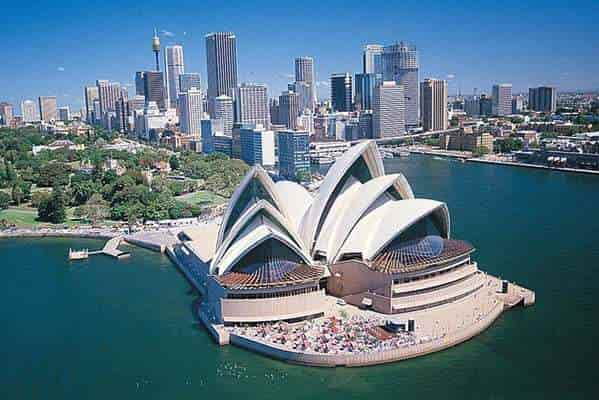
Sydney is home to many top universities in Australia, including the University of Sydney, UNSW Sydney, Macquarie University, University of Technology Sydney and more. Our assignment help experts in Sydney are well-versed with the curriculum followed in these universities and can provide top-quality assistance with assignments, essays, dissertations, research papers, and more.
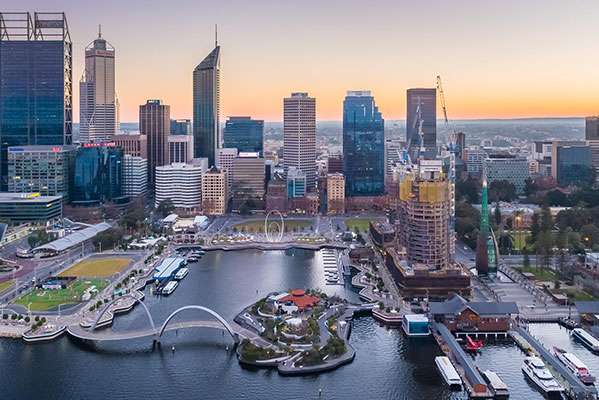
Perth is home to many top universities such as Curtin University, Murdoch University, Edith Cowan University and more. Our assignment help experts in Perth help students by offering them top-quality assignment assistance that addresses their specific requirements. So if you're stuck with an assignment or need help understanding a concept, connect with us today and get the best help now!
Brisbane is one of the most popular study destinations in Australia and is home to some of the top universities, such as the University of Queensland, Griffith University, Queensland University of Technology, James Cook University and more. If you are in Brisbane and need help with your assignments, connect with us today and get the best help from our native assignment writers.
4 Reasons to Choose GoAssignemntHelp for Assignment Help
We are affordable and light on your pocket. All our experts hold Master's or PhD degrees in their subjects with 5+ years of industry experience. But the real deal is our glorious track record. We have:
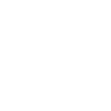
Delivered Order
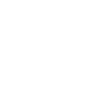
Happy Clients
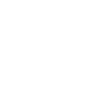
Clients Rating
Hire our assignment writing experts to get solutions that help you understand why and what of your topic. To complete the most challenging writing tasks in the shortest possible time, GoAssignmentHelp is your best choice!
GOASSIGNMENTHELP GUARANTEES
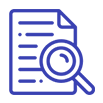
100% Original Assignments
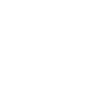
We ensure the originality of all assignments delivered to you. Each paper is thoroughly checked for plagiarism manually and with advanced software. You receive assignments that are 100% original, unique, and error-free.
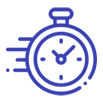
24/7 Customer Support
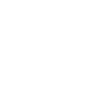
If you need assistance, you can always reach us via Live Chat or WhatsApp. Our advisors can suggest you the right expert, send a price quote, guide you to the best deal, and seek answers from experts who are working on your project or have already delivered it to you recently.
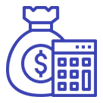
Easy Ordering Process
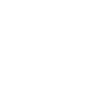
With our lightning-fast and convenient payment options, we make the ordering process simple. Through the Order Form, you can ask us questions and include information like the date for submission and your academic standing. Debit/credit cards, as well as PayPal, are accepted for payments.
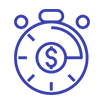
Quick Delivery
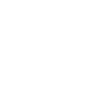
We provide papers as quickly as we can, usually well before the deadline you shared with us when placing your request. Let us know if you require a paper immediately, and we'll get it done. To prevent any last-minute deadline stress, we advise placing an order in advance.
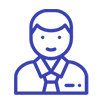
Professional Academic Writers
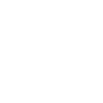
Our assignment writers hold advanced degrees (MA, MSc, MAW, or PhD) and have extensive work experience. They have written hundreds of research papers, assignments, term papers, and reports for students across Australia. Their background in academic writing and competencies enable them to produce the best assignment for you.
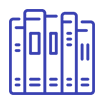
Qualified Subject Experts
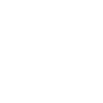
Our subject matter specialists hold the best degrees in their fields, as well as the necessary accreditations. Many of them are native English speakers from Australia. They enjoy assisting students in need and providing clear, straightforward explanations of things. They don't just follow directions; they also provide you advice on how to do well in the subject.
Can I Trust GoAssignmentHelp?
Of course, you can! We are a leading academic help provider in Australia and have been helping students with their studies for years. We offer unmatched quality at the most competitive prices. We are 100% confidential, so you can be sure your personal information is safe with us.
How Much Does It Cost?
The cost of your assignment depends on the level of study, the number of words, the academic level, and the deadline. You can get a free quote from us by submitting your requirements through the Order Form.
Deals & Discounts
Yes, we do! We offer the best deals and discounts on all our services. You can save up to 50% on your first order with us. We offer attractive discounts on bulk orders and also run referral programs. We also have seasonal discounts and special offers that you can avail of.
What if I am Not Satisfied with the Order?
We offer unlimited revisions on all orders. If you are not satisfied with your order, simply contact our customer support and they will help you resolve the issue.
You can also request a refund if you are not satisfied with the final deliverable. We offer a 100% money-back guarantee on all our services.
5 Reasons Why Students Seek Assignment Help Services
They want a bonzer assignment & don't have time for it.
Let's face it, no student wants to write an assignment. It's tedious, time-consuming, and sometimes even boring. But it has to be done if you want to get good grades. This is where our professional writers come in. They can help you write a top-quality assignment within the shortest time possible.
Get a Good Night's Sleep
Most students are juggling a lot of things at once - studies, extracurricular activities, part-time jobs, etc. This leaves them with little to no time for themselves. As a result, they are often sleep-deprived and exhausted. Our academic help services can take some of the burdens off your shoulders so that you can get some well-deserved rest.
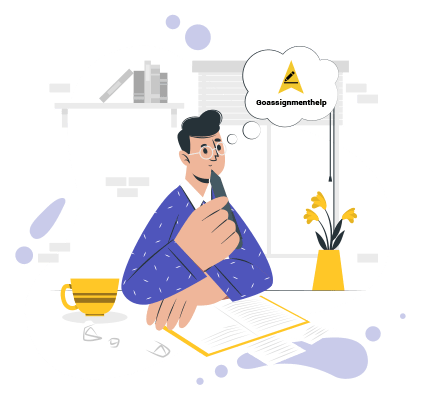
To Ace the Class with Grace
Every student wants to get good grades and perform well in their exams. But not everyone has the time or energy to study for hours on end. This is where our academic help services come in handy. We can help you in preparing excellent study notes to ace every class with ease.
Get Help from Bruce
Many Aussie students only want Australians and there's nothing wrong with that. Native experts know the education system and curriculum well and can offer insights that international experts might not be able to provide. On our platform, you can find thousands of native and experienced assignment writers who can help you with all your academic needs.
Where can I get help with My Assignment?
You are at the right place. GoAssignmentHelp is Australia's leading assignment writing service with the best online tutors. We have helped over 35,000 students succeed in their academic careers by providing quality assignments within set deadlines.
Why GoAssignmentHelp is the Right Choice:
- Professional Service with a Personal Touch: Our team of experts will take care of everything for you. They guide you on how to choose a topic, format the paper and reference it correctly. All you need to do is sit back and relax!
- Engaging and Easy-to-Follow Assignment Solutions: We make sure that you understand what you're doing, and why. When we answer a question for you or solve a Math problem, we include a step-by-step explanation so you can follow along. We also add a touch of humour to make learning fun!
- Get More Out of Your Education: Achieve your academic goals and get ahead in your studies with the best study guides online. At GoAssignmentHelp, we don't just provide solutions - we help you understand the concepts so you can apply them in your future studies.
- We're Here to Help - 24/7: We know that students have a lot on their plate, and sometimes things come up at the last minute. That's why we offer 24/7 customer support, so you can get the help you need when you need it. We are available on all days - be it Christmas or New Year - and at all hours!
- Achieve Your Dreams: Get the grades you need to get into the college of your dreams. We'll help you every step of the way, from choosing a topic to writing a killer essay. All you need to do is ask!
Still Not Convinced?
Reach out to us now and see how we can help you achieve your academic goals! You can also ask for assignment samples from our student advisors to assess the quality of our work. Read through our student clients' reviews and testimonials to know what they think of us!
Frequently Asked Questions
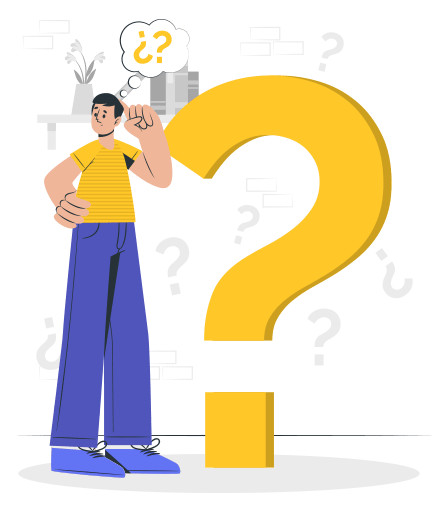
Where can I get help with my assignment?
Where can i ask for help with homework, what is the best assignment help website, can someone do my assignment for me, can i get caught if i buy an essay from you online, what if i don't like my assignment, testimonial - what they say.

Sarah, Sydney
Thank you so much for all the help that you have provided me with. I was struggling with my marketing assignment and your team helped me a lot. The paper was delivered on time and it was of very good quality." - Sarah, Sydney

Melinda, Sydney
It was quite difficult to complete my statistics assignment on time. During every submission time, it was a struggle for me. After I found Go Assignment Help, I am able to submit my assignments on time. They have been very helpful every time I needed them for assignments.
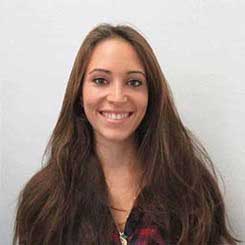
Sandra, Brisbane
Assignment submission always gave me nervous breakdown because of tight deadlines. Then one of my batchmates told me about Go Assignment Help and now assignment submission has become a cakewalk for me. Thanks to them and their efforts.
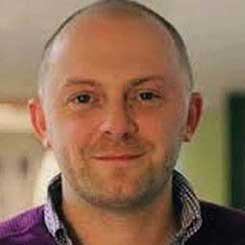
Alex, Melbourne
I am doing a part-time job to support my study fees. So it was a tough time for me during assignment submission. Go Assignment Help has made my assignment submission task easier. With their help, I am able to complete my physics assignments on time and even submit them without delay.
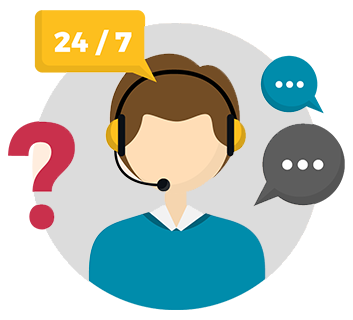
Want to Know More?
Share your contact details & get a call back from our experts..
Fill up this 1-sec form to get a call from our student counsellors. We will hear you out, answer your queries, analyze what you need, and offer you solutions and best deals.

[email protected] (+1)617-933-5480 -->
C-1198 Toorak Road Camberwell, Vic 3124
100% Secure Payment
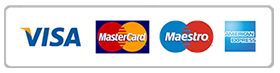
- Speech Writing Service
- Programming
- Scholarship
- Privacy Policy
- Terms & Condition
We offer assignment writing services in :
- Los Angeles
Disclaimer: Any material such as academic assignments, essays, articles, term and research papers, dissertations, coursework, case studies, PowerPoint presentations, reviews, etc. is solely for referential purposes. We do not encourage plagiarism in any form. We trust that our clients will use the provided material purely as a reference point in their own writing efforts.
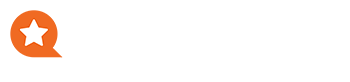
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
filer.backup fails with panic: assignment to entry in nil map #5571
stibi commented May 8, 2024
chrislusf commented May 9, 2024
Sorry, something went wrong.
stibi commented May 9, 2024
No branches or pull requests
Contribute to the Teams forum! Click here to learn more 💡
April 9, 2024
Contribute to the Teams forum!
Click here to learn more 💡
- Search the community and support articles
- Microsoft Teams
- Teams for education
- Search Community member
Ask a new question
TEAMS - Reading Progress Not Working
Good Morning,
I have tried to set an assignment with Reading Progress and from my end (as the teacher) it looks fine. When my students go to access the assignment, it is fine until it goes to display the text they are to read. The page to display the text is completely blank. This has previously been working with no issues.
Is someone able to provide some advice/support?
- Subscribe to RSS feed
Report abuse
Reported content has been submitted
Replies (4)
- Independent Advisor
Hello Joshua, I am a Microsoft user like you, providing solutions to community members; I am NOT a Microsoft employee. It can be frustrating when Reading Progress isn't working as expected in Microsoft Teams. Here are some troubleshooting steps you can try to address the blank page issue your students are encountering: Check Browser Compatibility: Ensure that your students are using a supported browser to access Microsoft Teams. Microsoft recommends using the latest versions of Microsoft Edge, Google Chrome, or Mozilla Firefox for the best experience. Clear Browser Cache: Instruct your students to clear their browser cache and cookies, as outdated or corrupted cache files can sometimes interfere with the proper display of content. Try a Different Device or Browser: Encourage your students to access the assignment from a different device or browser to see if the issue persists. This can help determine if the problem is specific to their current device or browser configuration. Update Teams App: If your students are accessing Teams via the desktop or mobile app, make sure they have the latest version installed. Updates often include bug fixes and improvements that may address issues with Reading Progress. Verify Assignment Settings: Double-check the settings of the assignment to ensure that Reading Progress is enabled and configured correctly. Make sure that the text to be read is properly uploaded or linked to the assignment. Hope this helps. -Stephen N.
Was this reply helpful? Yes No
Sorry this didn't help.
Great! Thanks for your feedback.
How satisfied are you with this reply?
Thanks for your feedback, it helps us improve the site.
Thanks for your feedback.
Hi Stephen,
Thanks for your tips. All of these tips have been followed and my students are still unable to see the text to read and record it.
Since none of the above solutions work, consider reporting the issue to Microsoft. Provide details about the error, troubleshooting steps taken, and any specific error messages encountered by your students. You can report the issue through the Microsoft Teams UserVoice forum: https://feedbackportal.microsoft.com/feedback/forum/ad198462-1c1c-ec11-b6e7-0022481f8472
Question Info
- Reading coach/ Reading progress
- Norsk Bokmål
- Ελληνικά
- Русский
- עברית
- العربية
- ไทย
- 한국어
- 中文(简体)
- 中文(繁體)
- 日本語
CMU Baseball Pitching aces first round assignment against CSU Pueblo
GRAND JUNCTION, Colo. (KJCT) - The Colorado Mesa Mavericks opened up Rocky Mountain Athletic Conference Tournament play in Grand Junction as the one-seed, and showed why they are atop the conference, powering past CSU Pueblo in the first round 5-3.
A two-run lead may not seem like a lot for a Mavericks team that was putting up an average of over twenty runs a game in their last series against Adams State , but make no mistake, the Mavericks were in control of this rivalry matchup seemingly all game long.
This time around it wasn’t a wild day at the plate from Maverick Hitters that got things done, it was a pair of power pitching performances. Junior Pitcher Caleb Ruter got things rolling. The Maverick starter tossed 5.2 innings with only two earned runs and two walks, while striking out eight on just over one hundred pitchers. Ruter also made a heads up play to get a Thunderwolf runner at home plate on a bunt attempt for the out and deny them a run.
After Ruter, Junior Pitcher Ethan Voss came in and was just effective, pitching the remainder of the game, striking out five and only allowing one more run.
At the plate the Mavericks did what they needed to do, when they needed to do it, and came up in the clutch time and time again. Including Junior Outfielder Paul Schoenfeld picking up an RBI base hit where he collided with the CSU Pueblo Pitcher at first. Shoenfeld and the pitcher, Tyler Curtis would both be ok and remain in the game.
The Mavericks advance in the Winner’s Bracket and will face off Adams State at 3:00p.m. Thursday.
Copyright 2024 KJCT. All rights reserved.
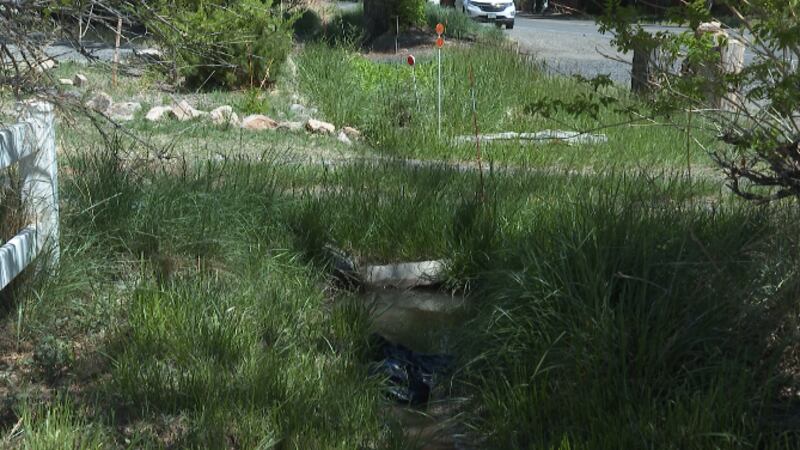
Unknown body found in ditch at Grand Junction home

Matthew Dunham set to go to court Thursday
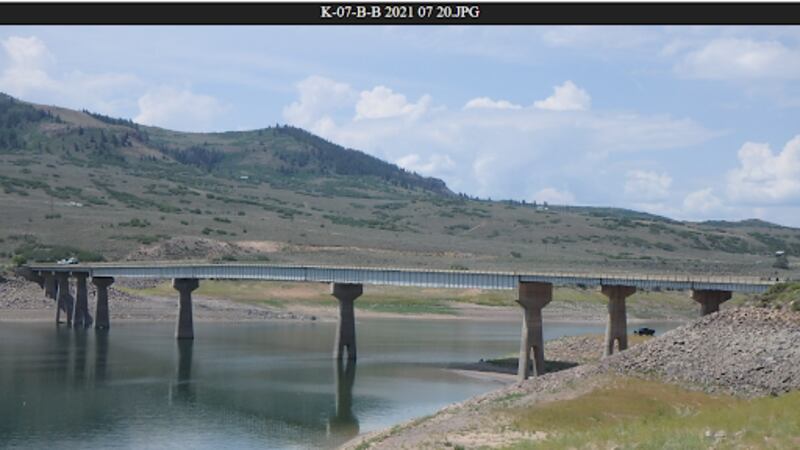
CDOT holds meeting to give public update on Highway 50 bridge closure

Mail delays: Nearly 80,000 pieces of undelivered mail found at single post office, audit finds
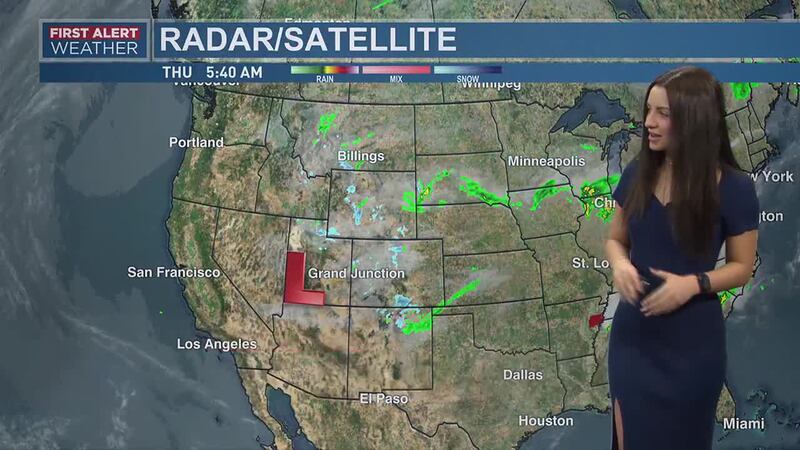
Heavy Cloud Coverage Today, Leading to Rain This Weekend
Latest news.

CMU Baseball powers past Adams State in RMAC Tournament, Softball falls in Regionals

Mesa County unhoused resource center reaches new heights

CPW urges people to leave wildlife alone this Spring
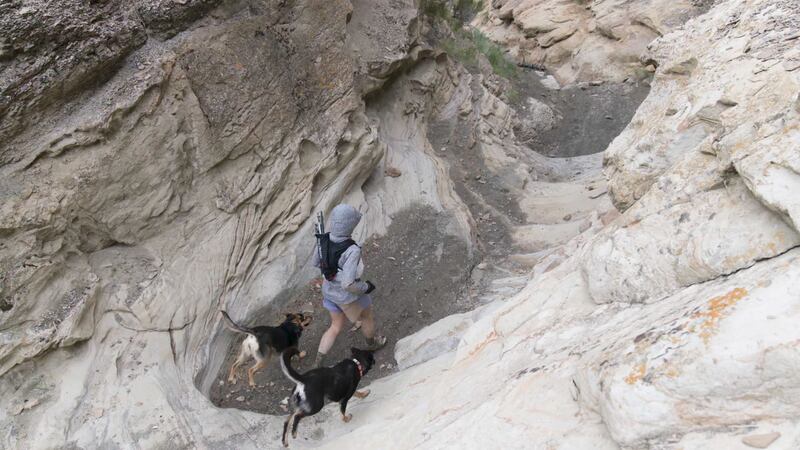
Grand Junction woman completes run around the Grand Valley
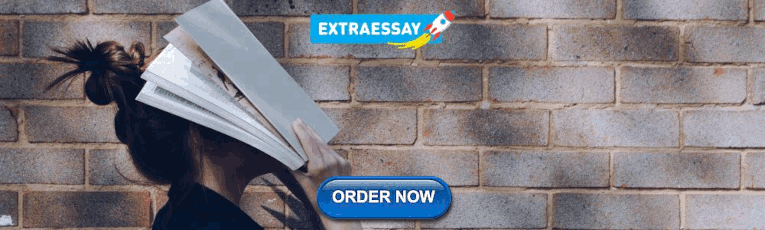
COMMENTS
Go Assignment Operators Previous Next Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example. package main import ("fmt") func main() { var x = 10
Inside a function, the := short assignment statement can be used in place of a var declaration with implicit type. Outside a function, every statement begins with a keyword ( var, func, and so on) and so the := construct is not available. < 10/17 >. short-variable-declarations.go Syntax Imports.
Change a variable's value, use: =. There are some rules and the TLDR is not always true. As others have explained already, := is for both declaration, assignment, and also for redeclaration; and it guesses ( infers) the variable's type automatically. For example, foo := 32 is a short-hand form of: var foo int.
The pre-Go1.18 version, without generics, can be found here . For more information and other documents, see go.dev . Go is a general-purpose language designed with systems programming in mind. It is strongly typed and garbage-collected and has explicit support for concurrent programming.
Go is a new language. Although it borrows ideas from existing languages, it has unusual properties that make effective Go programs different in character from programs written in its relatives. ... In this declaration, the assignment involving a conversion of a *RawMessage to a Marshaler requires that *RawMessage implements Marshaler, and that ...
In Go, we can also use an assignment operator together with an arithmetic operator. For example, number := 2 number += 6. Here, += is additional assignment operator. It first adds 6 to the value of number (2) and assigns the final result (8) to number. Here's a list of various compound assignment operators available in Golang.
Different types of assignment operators are shown below: "="(Simple Assignment): This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. "+="(Add Assignment): This operator is a combination of '+' and '=' operators. This operator first adds the current value ...
Go has a compound assignment operator for each of the arithmetic operators discussed in this tutorial. To add then assign the value: y += 1. To subtract then assign the value: y -= 1. To multiply then assign then value: y *= 2. To divide then assign the value: y /= 3. To return the remainder then assign the value: y %= 3
All the other assignment operators are built off of it. For example, the below code assigns the variable a the value of 3, the constant pi the value of 3.14, and the variable website the value of Learnmonkey: var a = 3 const pi = 3.14 var website = "Learnmonkey". Notice that we can use the assignment operator to make constants and variables.
Go assignment operator. The assignment operator = assigns a value to a variable. A variable is a placeholder for a value. In mathematics, the = operator has a different meaning. In an equation, the = operator is an equality operator. The left side of the equation is equal to the right one. var x = 1 Here we assign a number to the x variable. x ...
Variables in Go are created by first using the var keyword, then specifying the variable name (x), ... The x = x + y form is so common in programming that Go has a special assignment statement: +=. We could have written x = x + "second" as x += "second" and it would have done the same thing. (Other operators can be used the same way)
The following table lists all the assignment operators supported by Go language −. Operator. Description. Example. =. Simple assignment operator, Assigns values from right side operands to left side operand. C = A + B will assign value of A + B into C. +=. Add AND assignment operator, It adds right operand to the left operand and assign the ...
Output. 1032048535. In this example, Go does the math for us, subtracting 813 from the variable i to return the sum 1032048535. Speaking of math, variables can be set equal to the result of a math equation. You can also add two numbers together and store the value of the sum into the variable x: x := 76 + 145.
The USG routinely intercepts and monitors communications on this IS for purposes including, but not limited to, penetration testing, COMSEC monitoring, network operations and defense, personnel misconduct (PM), law enforcement (LE), and counterintelligence (CI) investigations. At any time, the USG may inspect and seize data stored on this IS.
Learn Go - Multiple Variable Assignment. Example. In Go, you can declare multiple variables at the same time. // You can declare multiple variables of the same type in one line var a, b, c string var d, e string = "Hello", "world!"
If Statements. We will start with the if statement, which will evaluate whether a statement is true or false, and run code only in the case that the statement is true. In a plain text editor, open a file and write the following code: import "fmt" func main() {. grade := 70 if grade >= 65 {.
Google Classroom is a great app for assignments and the classroom in general, but there's some problems on the go for mobile users. I have a Google Pixel 8 (amazing phone), and when I'm on the go, no matter the network, albeit 5G, 5G UC (T-Mobile), or my Gigabit wifi at home, the app still opens really slowly and takes around 15-30 seconds to load a class page.
Australia's #1 Assignment Help Service! Find expert academic writers for your assignments. Get 100% plagiarism-free work. GoAssignmentHelp offers 24/7 study help at affordable prices! We feature: Free plagiarism check. 100% satisfaction guarantee. 100% confidentiality. Quick turnaround times.
To resolve this error, the External tool URL for the GoReact assignment will need to be edited by following the steps below. Login to Canvas, click courses, choose a desired course and visit the Assignments (A) tab. Click the three-dot kabob (B) by the assignment name. then Edit (C). In the dialog box, choose More Options.
Pointers. Go has pointers. A pointer holds the memory address of a value. The type *T is a pointer to a T value. Its zero value is nil.. var p *int. The & operator generates a pointer to its operand.. i := 42 p = &i. The * operator denotes the pointer's underlying value.. fmt.Println(*p) // read i through the pointer p *p = 21 // set i through the pointer p
12. One possible way to do this in just one line by using a map, simple I am checking whether a > b if it is true I am assigning c to a otherwise b. c := map[bool]int{true: a, false: b}[a > b] However, this looks amazing but in some cases it might NOT be the perfect solution because of evaluation order.
Welcome back to Masters, your go-to channel for high-quality educational content! In today's video, we're diving into the sta301 assignment 1 solution for Vi...
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Join us at 6 PM (WAT) this Thursday May 9, 2024, as our distinguish guest will be discussing the topic: GEN-Z ACCOUNTANTS: Redefining Traditional...
Medicare.gov Care Compare is a new tool that helps you find and compare the quality of Medicare-approved providers near you. You can search for nursing homes, doctors, hospitals, hospice centers, and more. Learn how to use Care Compare and make informed decisions about your health care. Official Medicare site.
Go provides a familiar syntax for working with maps. This statement sets the key "route" to the value 66: m["route"] = 66. This statement retrieves the value stored under the key "route" and assigns it to a new variable i: i := m["route"] If the requested key doesn't exist, we get the value type's zero value .
Updates often include bug fixes and improvements that may address issues with Reading Progress. Verify Assignment Settings: Double-check the settings of the assignment to ensure that Reading Progress is enabled and configured correctly. Make sure that the text to be read is properly uploaded or linked to the assignment. Hope this helps. -Stephen N.
The Colorado Mesa Mavericks opened up Tournament play in Grand Junction as the one-seed, and showed why they are atop the conference, powering past CSU Pueblo in the first round
If with a short statement. Like for, the if statement can start with a short statement to execute before the condition.. Variables declared by the statement are only in scope until the end of the if. (Try using v in the last return statement.) < 6/14 >