- Artificial Intelligence
- Generative AI
- Cloud Computing
- Data Management
- Emerging Technology
- Technology Industry
- Software Development
- Microsoft .NET
- Development Tools
- Open Source
- Programming Languages
- Enterprise Buyer’s Guides
- Newsletters
- Foundry Careers
- Terms of Service
- Privacy Policy
- Cookie Policy
- Copyright Notice
- Member Preferences
- About AdChoices
- E-commerce Affiliate Relationships
- Your California Privacy Rights
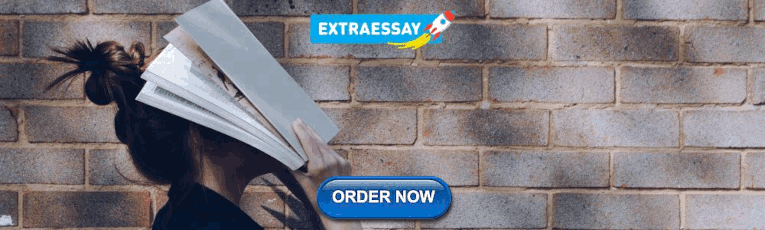
Our Network
- Computerworld
- Network World

Does Java pass by reference or pass by value?
You might know that java passes by value, but it helps to understand why. here's what happens when you pass mutable and immutable object references in java..

Many programming languages allow passing objects by reference or by value. In Java, we can only pass object parameters by value. This imposes limits and also raises questions. For instance, if the parameter value is changed in the method, what happens to the value following method execution? You may also wonder how Java manages object values in the memory heap. This article helps you resolve these and other common questions about object references in Java.
Passing object references in Java
In this article you’ll learn the difference between passing by reference and passing by value in Java and how to pass Java object references:
‘Pass by reference’ and ‘pass by value’ defined
Java object references are passed by value, passing primitive types in java, passing immutable object references in java, passing mutable object references in java.
- What to avoid when passing object references
- What to remember about passing object references
Pass by reference means we pass the location in memory where the variable’s value is stored and pass by value means we pass a copy of the variable’s actual value. It’s a bit more complicated than that, of course, but this definition is a good starting point.
- Passing by value means we pass a copy of the value.
- Passing by reference means we pass the real reference to the variable in memory.
All object references in Java are passed by value. This means that a copy of the value will be passed to a method. The tricky part is that passing a copy of the value changes the real value of the object. This example should help you understand why:
What do you think the simpson.name will be after the transformIntoHomer method is executed?
In this case, it will be Homer! The reason is that Java object variables are simply references that point to real objects in the memory heap. Therefore, even though Java passes parameters to methods by value, if the variable points to an object reference, the real object will also be changed.
If you’re still not sure how this works, consider the following diagram:
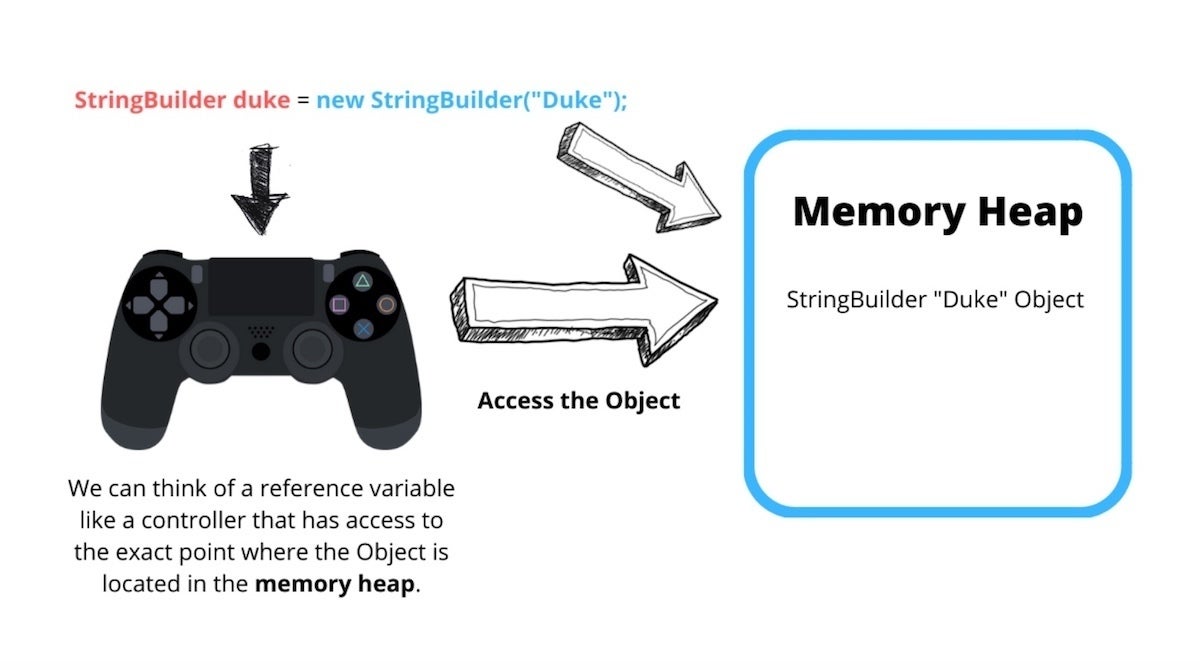
Like object types, primitive types are also passed by value. Can you guess what will happen to the primitive types in the following code example?
If you determined that the value would change to 30, you are correct. It’s 30 because (again) Java passes object parameters by value. The number 30 is just a copy of the value, not the real value. Primitive types are allocated in the stack memory, so only the local value will be changed. In this case, there is no object reference.
What if we did the same test with an immutable String object? Notice what happens when we change the value of a String :
What do you think the output will be? If you guessed “” then congratulations! That happens because a String object is immutable , which means that the fields inside the String are final and can’t be changed.
Making the String class immutable gives us better control over one of Java’s most used objects. If the value of a String could be changed, it would create many bugs. Note, also, that we are not changing an attribute of the String class; instead, we’re simply assigning a new String value to it. In this case, the “Homer” value will be passed to name in the changeToHomer method. The String “Homer” will be eligible to be garbage collected as soon as the changeToHomer method completes execution. Even though the object can’t be changed, the local variable will be.
Unlike String , most objects in the JDK are mutable, like the StringBuilder class. The example below is similar to the previous one, but features StringBuilder rather than String :
Can you guess the output for this example? In this case, because we’re working with a mutable object, the output will be “Homer Simpson.” You could expect the same behavior from any other mutable object in Java.
Summary of what you’ve learned
You’ve learned that Java objects are passed by value, meaning that a copy of the value is passed . Just remember that the copied value points to a real object in the Java memory heap. Also, remember that passing by value changes the value of the real object.
What to avoid when passing Java object references
- Don’t try to change an immutable value by reference.
- Don’t try to change a primitive variable by reference.
- Don’t expect the real object won’t change when you change a mutable object parameter in a method (it will change).
What to remember about passing Java object references
- Java always passes parameter variables by value.
- Object variables in Java always point to the real object in the memory heap.
- A mutable object’s value can be changed when it is passed to a method.
- An immutable object’s value cannot be changed, even if it is passed a new value.
Test what you’ve learned about object references
Now, let’s test what you’ve learned about object references. In the code example below, you see the immutable String and the mutable StringBuilder class. Each is being passed as a parameter to a method. Knowing that Java only passes by value, what do you believe will be the output once the main method from this class is executed?
Here are the options, check the end of the article for the answer.
A : Warrior=null Weapon=null B : Warrior=Dragon Weapon=Dragon C : Warrior=Dragon Knight Weapon=Dragon Sword D : Warrior=Dragon Knight Weapon=Sword
Solving the challenge
The first parameter in the above example is the warriorProfession variable, which is a mutable object. The second parameter, weapon, is an immutable String :
At the first line of this method, we append the Knight value to the warriorProfession variable. Remember that warriorProfession is a mutable object; therefore the real object will be changed, and the value from it will be “Dragon Knight.”
In the second instruction, the immutable local String variable will be changed to “Dragon Sword.” The real object will never be changed, however, since String is immutable and its attributes are final:
Finally, we pass null to the variables here, but not to the objects. The objects will remain the same as long as they are still accessible externally—in this case through the main method. And, although the local variables will be null, nothing will happen to the objects:
From this we can conclude that the final values from our mutable StringBuilder and immutable String will be:
The only value that changed in the changeWarriorClass method was warriorProfession , because it’s a mutable StringBuilder object. Note that warriorWeapon did not change because it’s an immutable String object.
The correct output from our Challenger code would be:
D : Warrior=Dragon Knight Weapon=Sword .
Video challenge! Debugging object references in Java
Debugging is one of the easiest ways to fully absorb programming concepts while also improving your code. In this video, you can follow along while I debug and explain object references in Java.
Learn more about Java
- Get more quick code tips: Read all of Rafael’s articles in the InfoWorld Java Challengers series.
- Check out more videos in Rafael’s Java Challengers video playlist .
- Find even more Java Challengers on Rafael’s Java Challengers blog and in his book, with more than 70 code challenges .
Related content
Syncfusion open-sources ui controls for .net maui, how developers can automate ‘computer use’ with anthropic’s new llm, the power of prime numbers in computing, why we get buggy software.

Rafael del Nero is a Java Champion and Oracle Ace, creator of the Java Challengers initiative, and a quiz master in the Oracle Dev Gym. Rafael is the author of "Java Challengers" and "Golden Lessons." He believes there are many techniques involved in creating high-quality software that developers are often unaware of. His purpose is to help Java developers use better programming practices to code quality software for stress-free projects with fewer bugs.
More from this author
How to use generics in your java programs, method overloading in the jvm, string comparisons in java, thread behavior in the jvm, polymorphism and inheritance in java, java inheritance vs. composition: how to choose, sorting java objects with comparable and comparator, comparing java objects with equals() and hashcode(), show me more, the best python libraries for parallel processing.

Developers embracing API-first development, survey says

JDK 24: The new features in Java 24

How to get better web requests in Python with httpx

How to better integrate Python/C with CFFI

How to create and work with zip archives using Python

Sponsored Links
- Get Cisco UCS X-Series Chassis and Fabric Interconnects offer.

Last Minute Java Object Assignment and Object Passing By Value or Reference Explained Tutorial
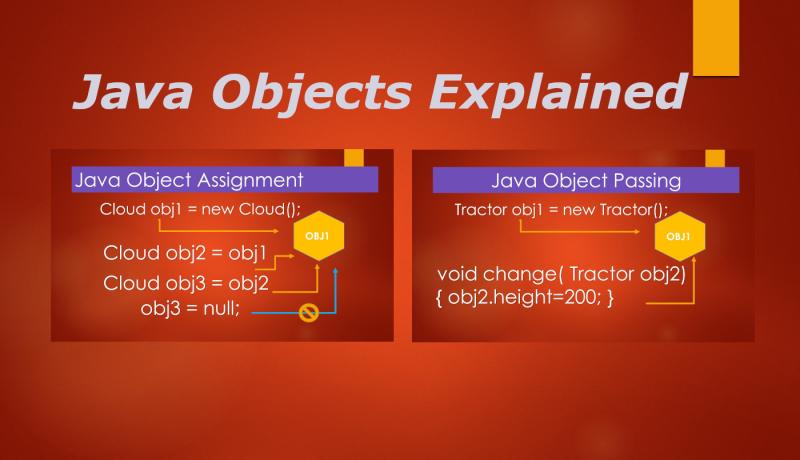
In Java, we create Classes. We create objects of the Classes. Let us know if it is possible to assign a Java Object from one variable to another variable by value or reference in this Last Minute Java Tutorial. We also try to know whether it is possible to pass Java Objects to another method by Value or Reference in this tutorial.
You may also read about Introduction to Java Class Structure .
Java Object Assignment by Value or Reference Explained
In Java, objects are always passed by Reference. Reference is nothing but the starting address of a memory location where the actual object lies. You can create any number of references to just One Object. Let us go by an example.
We create a Class "Cloud" with a single Property called "color". It is a String object. We create 3 objects of the Cloud type.
Notice that all three references obj1, obj2 and obj3 are pointing to the same Cloud object. Thus the above example outputs the same color-property value " RED ". Later, we made the references obj2 and obj3 to point to the null location. Still the original object pointed or referenced by obj1 holds the value. So the final PRINT statement outputs "RED" as usual.
Note : Reference is also called a Variable whether Primitive or Object.
This concludes that a Java object assignment happens by Reference . But the actual value of memory location pointed by References are copied by Value only. So we are actually copying memory locations from one Reference variable to another Reference variable by Value . Interviewers ask this famous Java question just to test your knowledge. If someone asks in C language point of view , say that it is by Reference . If they ask in Java point of view , say that it is by Value.
Java Object Passing by Value or Reference to a Method Explained
You already learnt that Object assignment in Java happens by Reference. Let us try to pass Objects to another method as a parameter or argument. We try to know whether Objects are passed by Value or Reference to another Method using the below example.
In the above example, we have created an object "obj1" of type Tractor. We assigned value of 100 to the property "height". We printed the value before passing the object to another method. Now, we passed the object to another method called "change" from the calling-method " main ". We modified the height property to 200 in the called-method . Now, we tried to print the value of the property in the Calling method. It shows that the Object is modified in the called-method.
Similarly, we can pass Java objects to the Constructor using Pass by Reference or simply Reference .
This concludes that Java Objects are passed to methods by Reference . Here also, we pass the memory location pointed by one Reference variable to another Reference variable of a Called method as a Value only. So, you are actually passing Values (memory locations represented by integer or long data type). But the actual passed-object can only be referenced by a Reference variable. Interviewers take advantage of this tricky logic to confuse you. If someone asks in C language point of view , say that it is by Reference . If they ask in Java point of view , say that it is by Value .
Let us know more about Java Classes with different Constructors and Methods in coming chapters.
Share this Last Minute Java Tutorial explaining Java Object Assignment using Reference and Java Object Passing with Pass by Reference with your friends and colleagues to encourage authors.
Java Class Structure Tutorial
Java Method Signature Rules Tutorial
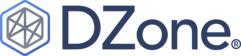
- Manage Email Subscriptions
- How to Post to DZone
- Article Submission Guidelines
- Manage My Drafts
How would you rate your organization's observability maturity? What are the main benefits and challenges of observability? Tell us!
Celebrate a decade of Kubernetes. Explore why K8s continues to be one of the most prolific open-source systems in the SDLC.
Threat Detection: Learn core practices for managing security risks and vulnerabilities in your organization — don't regret those threats !
[LAST CALL] What's in your tech stack? Tell us about it in our annual Community Survey, and help shape the future of DZone!
- Redefining Java Object Equality
- Addressing Memory Issues and Optimizing Code for Efficiency: Glide Case
- Singleton: 6 Ways To Write and Use in Java Programming
- Creating a Deep vs. Shallow Copy of an Object in Java
- Building Secure AI LLM APIs: A DevOps Approach to Preventing Data Breaches
- Four Essential Tips for Building a Robust REST API in Java
- Jenkins in the Age of Kubernetes: Strengths, Weaknesses, and Its Future in CI/CD
- Platform Engineering: A Strategic Response to the Growing Complexity of Modern Software Architectures
Passing by Value vs. Passing by Reference in Java
While many languages put information passing into the hands of developers, all data is passed by value in java. see how to turn that restriction to your advantage..
Join the DZone community and get the full member experience.
Understanding the technique used to pass information between variables and into methods can be a difficult task for a Java developer, especially those accustomed to a much more verbose programming language, such as C or C++. In these expressive languages, the developer is solely responsible for determining the technique used to pass information between different parts of the system. For example, C++ allows a developer to explicitly pass a piece of data either by value, by reference, or by pointer. The compiler simply ensures that the selected technique is properly implemented and that no invalid operation is performed.
In the case of Java, these low-level details are abstracted, which both reduces the onus on the developer to select a proper means of passing data and increases the security of the language (by inhibiting the manipulation of pointers and directly addressing memory). In addition, though, this level of abstraction hides the details of the technique performed, which can obfuscate a developer's understanding of how data is passed in a program. In this article, we will examine the various techniques used to pass data and deep-dive into the technique that the Java Virtual Machine (JVM) and the Java Programming Language use to pass data, as well as explore some examples that demonstrate in practical terms what these techniques mean for a developer.
Terminology
In general, there are two main techniques for passing data in a programming language: (1) passing by value and (2) passing by reference. While some languages consider passing by reference and passing by pointer two different techniques, in theory, one technique can be thought of as a specialization of the other, where a reference is simply an alias to an object, whose implementation is a pointer.
Passing by Value
The first technique, passing by value, is defined as follows:
Passing by value constitutes copying of data, where changes to the copied value are not reflected in the original value
For example, if we call a method that accepts a single integer argument and the method makes an assignment to this argument, the assignment is not preserved once the method returns. While one might expect that the assignment is preserved after the method returns, the assignment is lost because the value placed on the call stack was a copy of the value passed into the method, as illustrated in the snippet below:
If we execute this code, we obtain the following output:
We see that the change made to the argument passed into the process function was not preserved after we exited the scope of the function. This loss of data was due to the fact that a copy of the value held by the someValue variable was placed on the call stack prior to the execution of the process function. Once the process function exited, this copy was popped from the call stack and the changes made to it were lost, as illustrated in the figure below:
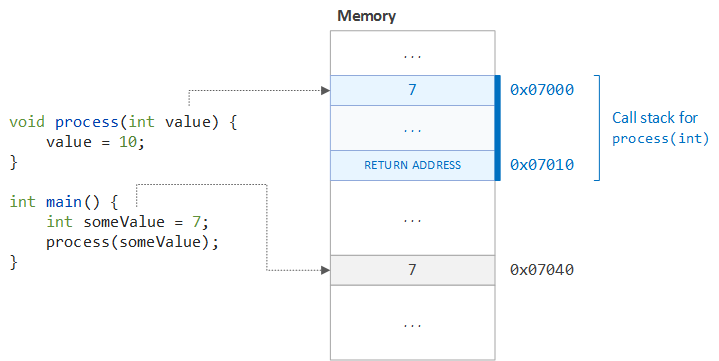
Additionally, the action of popping the call stack at the completion of the process method is illustrated in the figure below. Note that the value copied as the argument to the process method is lost (reclaimed) once the call stack is popped, and therefore, all changes made to that value are in turn lost during the reclamation step.
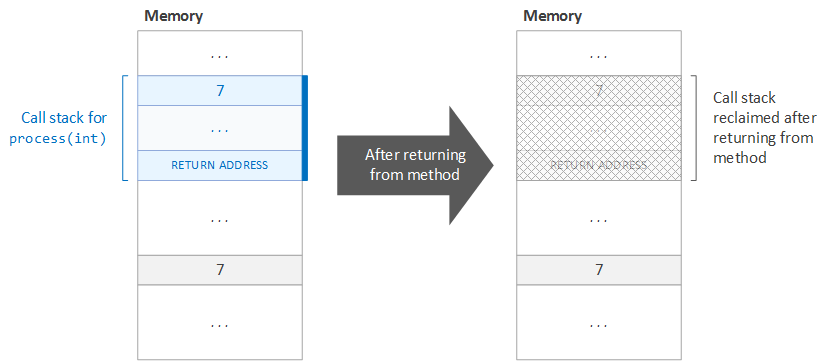
Passing by Reference
The alternative to passing by value is passing by reference, which is defined as follows:
Passing by reference consitutes the aliasing of data, where changes to the aliased value are reflected in the original value
Unlike passing by value, passing by reference ensures that a change made to a value in a different scope is preserved when that scope is exited. For example, if we pass a single argument into a method by reference, and the method makes an assignment to that value within its body, the assignment is preserved when the method exits. This can be demonstrated using the following snippet of C++ code:
If we run this code, we obtain the following output:
In this example, we can see that when we exit the function, the assignment we made to our argument that was passed by reference was preserved outside of the scope of the function. In the case of C++, we can see that under-the-hood, the compiler has passed a pointer into the function that points to the someValue variable. Thus, when this pointer is dereferenced (as happens during reassignment), we are making a change to the exact location in memory that stores the someValue variable. This principle is demonstrated in the illustrations below:
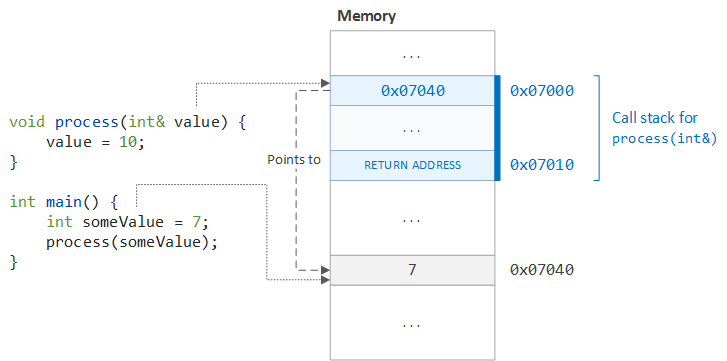
Passing Data in Java
Unlike in C++, Java does not have a means of explicitly differentiating between pass by reference and pass by value. Instead, the Java Language Specification ( Section 4.3 ) declares that the passing of all data, both object and primitive data, is defined by the following rule:
All data is passed by value
Although this rule may be simple on the surface, it requires some further explanation. In the case of primitive values, the value is simply the actual data associated with the primitive (.e.g 1 , 20.7 , true , etc.) and the value of the data is copied each time it is passed. For example, if we define an expression such as int x = 7 , the variable x holds the literal value of 7 . In the case of objects in Java, a more expanded rule is used:
The value associated with an object is actually a pointer, called a reference, to the object in memory
For example, if we define an expression such as Foo foo = new Foo() , the variable foo does not hold the Foo object created, but rather, a pointer value to the created Foo object. The value of this pointer to the object (what the Java specification calls an object reference , or simply reference) is copied each time it is passed. According to the Objects section (Section 4.3.1) of the Java Language Specification, only the following can be performed on an object reference:
- Field access, using either a qualified name or a field access expression
- Method invocation
- The cast operator
- The string concatenation operator + , which, when given a String operand and a reference, will convert the reference to a String by invoking the toString method of the referenced object (using "null" if either the reference or the result of toString is a null reference), and then will produce a newly created String that is the concatenation of the two strings
- The instanceof operator
- The reference equality operators == and !=
- The conditional operator ? :
In practice, this means that we can change the fields of the object passed into a method and invoke its methods, but we cannot change the object that the reference points to. Since the pointer is passed into the method by value, the original pointer is copied to the call stack when the method is invoked. When the method scope is exited, the copied pointer is lost, thus losing the change to the pointer value.
Although the pointer is lost, the changes to the fields are preserved because we are dereferencing the pointer to access the pointed-to object: The pointer passed into the method and the pointer copied to the call stack are identical (although independent) and thus point to the same object. Thus, when the pointer is dereferenced, the same object at the same location in memory is accessed. Therefore, when we make a change to the dereferenced object, we are changing a shared object. This concept is illustrated in the figure below:
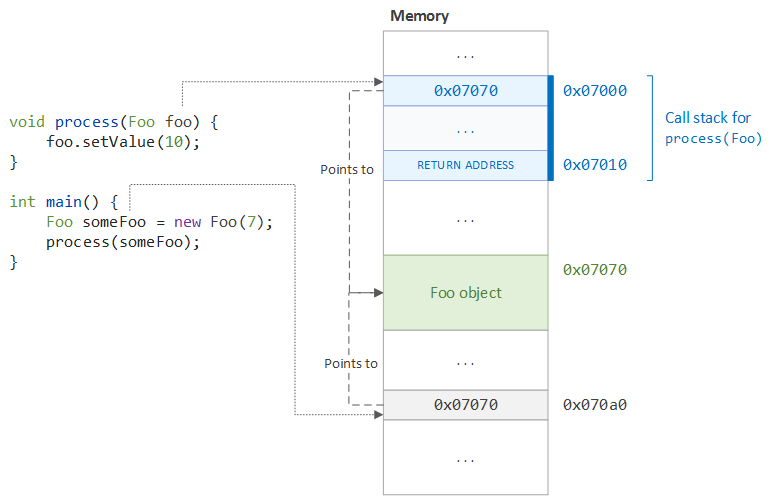
This should not be confused with passing by reference: If the pointer were passed by reference, the variable foo would be an alias to someFoo and changing the object that foo points to would also change the object that someFoo points to. In this case, though, a copied pointer is passed into the function, and thus, the change to the pointer value is lost once the call stack it popped.
While it is crucial to understand the concepts behind passing data in a programming language (Java in particular), many times it is difficult to solidify these theoretical ideas without concrete examples. In this section, we will cover four primary examples:
- Assigning primitive values to a variable
- Passing primitive values to a method
- Assigning object values to a variable
- Passing object values to a method
For each of these examples, we will explore a snippet of code accompanied by print statements that show the value of the primitive or object at each major step in the assignment or the argument-passing process.
Primitive Type Example
Since Java primitives are not objects, primitives and objects are treated as two separate cases with respect to data binding (assignment) and argument-passing. In this section, we will focus on binding primitive data to a variable and passing primitive data to a simple method.
Assigning Values to Variable
If we assign an existing primitive value, such as someValue , to a new variable, anotherValue , the primitive value is copied to the new variable. Since the value is copied, the two variables are not aliases of one another, and therefore, when the original variable, someValue , is changed, the change is not reflected in anotherValue :
If we execute this snippet, we receive the following output:
Passing Values to Method
Similar to making primitive assignments, the arguments for a method are bound by value, and thus, if a change is made to the argument within the scope of the method, the changes are not preserved when the method scope is exited:
If we run this code, we see that the original value of 7 is preserved when the scope of the process method is exited, even though that argument was assigned a value of 50 within the method scope:
Object Type Example
While all values, both primitive and object, are passed by value in Java, there are some nuances in passing objects by value that are made explicit when seen in an example. Just as with primitive types, we will explore both assignment and argument binding the following examples.
The variable binding semantics of for objects and primitives are nearly identical, but instead of binding a copy of the primitive value, we bind a copy of the object address. We can see this in action in the following snippet:
In this example, we expect that assigning a new Ball object to someBall (after assigning someBall to anotherBall ) does not change the value of anotherBall , since anotherBall holds a copy of the address for the original someBall . When the address stored at someBall changes, no change is made to anotherBall because the copied value in anotherBall is completely independent of the address value stored in someBall . If we execute this code, we see our expected results (note that the address of each Ball object will vary between executions, but the address in line 1 and line 3 should be identical, regardless of the specific address value):
Passing Values to Methods
The last case we must cover is that of passing an object into a method. In this case, we see that we are able to change the fields associated with the passed in object, but if we try to reassign a value to the argument itself, this reassignment is lost when the method scope is exited.
If we execute this code, we see the following output:
Although there is a large volume of output, if we take each line one at a time, we see that when we make a change to the fields of a Vehicle object passed into a method, the field changes are preserved, but when we try to reassign a new Vehicle object to the argument, the change is not preserved once we leave the scope of the method.
In the former case, the address of the Vehicle created outside the method is copied to the argument of the method, and thus both point to the same Vehicle object. If this pointer is dereferenced (which occurs when the fields of the object are accessed or changed), the same object is changed. In the latter case, when we try to reassign the argument with a new address, the change is lost because the argument is only a copy of the address of the original object, and thus, once the method scope is exited, the copy is lost.
A secondary principle can be formed from this mechanism in Java: Do not reassign arguments passed into a method (codified by Martin Fowler in the refactoring Remove Assignments to Parameters ). To ensure that no such reassignment of method arguments is made, the arguments can be marked as final in the method signature. Note that a new local variable can be used instead of the arguments if reassigned is required:
Although fundamental principles such as data binding schemes and data passing schemes can seem abstract in the realm of daily programming, these concepts are essential in avoiding subtle mistakes. Unlike other programming languages (such as C++), Java simplifies its data binding and passing scheme into a single rule: Data is always passed by value. Although this rule can be a harsh restriction, its simplicity, and understanding how to apply this simplicity, can be a major asset when accomplishing a slew of daily tasks.
Opinions expressed by DZone contributors are their own.
Partner Resources
- About DZone
- Support and feedback
- Community research
- Advertise with DZone
CONTRIBUTE ON DZONE
- Become a Contributor
- Core Program
- Visit the Writers' Zone
- Terms of Service
- Privacy Policy
- 3343 Perimeter Hill Drive
- Nashville, TN 37211
- [email protected]
Let's be friends:
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Assignment, Arithmetic, and Unary Operators
The simple assignment operator.
One of the most common operators that you'll encounter is the simple assignment operator " = ". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left:
This operator can also be used on objects to assign object references , as discussed in Creating Objects .
The Arithmetic Operators
The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics. The only symbol that might look new to you is " % ", which divides one operand by another and returns the remainder as its result.
The following program, ArithmeticDemo , tests the arithmetic operators.
This program prints the following:
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments . For example, x+=1; and x=x+1; both increment the value of x by 1.
The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
By the end of this program, the variable thirdString contains "This is a concatenated string.", which gets printed to standard output.
The Unary Operators
The unary operators require only one operand; they perform various operations such as incrementing/decrementing a value by one, negating an expression, or inverting the value of a boolean.
The following program, UnaryDemo , tests the unary operators:
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++; and ++result; will both end in result being incremented by one. The only difference is that the prefix version ( ++result ) evaluates to the incremented value, whereas the postfix version ( result++ ) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo , illustrates the prefix/postfix unary increment operator:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Java Program to Create an Object for Class and Assign Value in the Object Using Constructor
Java is one of the most popular programming languages. It is an object-oriented programming language which means that we can create classes, objects, and many more. It also supports inheritance, polymorphism, encapsulation, and many more. It is used in all applications starting from mobile applications to web-based applications. Classes are defined as the blue-print or template from which we can create objects and objects are the instances of the class which consist of states and behaviors.
- First, define a class with any name ‘SampleClass’ and define a constructor method.
- The constructor will always have the same name as the class name and it does not have a return type.
- Constructors are used to instantiating variables of the class.
- Now, using the constructors we can assign values.
- After the constructor method, implement a function that returns the value of any variables.
- Now in the main function create an object using the ‘new’ keyword. If there is no user-defined constructor of the class, then the default constructor of the class is called else when the object is created the user-defined constructor is called as per the type and number of parameters matched with the constructor of the class.
- We can call the above-declared function using the object.
- At last print the value from the function using the System.out.println() statement.
- Once you are done with the implementation run the program and will get the output.
Similar Reads
Please login to comment..., improve your coding skills with practice.
What kind of Experience do you want to share?

Java Tutorial
- What is Java
- History of Java
- Features of Java
- C++ vs Java
- Hello Java Program
- Program Internal
- How to set path?
- JDK, JRE and JVM
- JVM: Java Virtual Machine
- Java Variables
- Java Data Types
- Unicode System
Control Statements
- Java Control Statements
- Java If-else
- Java Switch
- Java For Loop
- Java While Loop
- Java Do While Loop
- Java Continue
- Java Comments
Java Programs
Java object class.
- Java OOPs Concepts
- Naming Convention
- Object and Class
- Constructor
- static keyword
- this keyword
Java Inheritance
- Inheritance(IS-A)
- Aggregation(HAS-A)
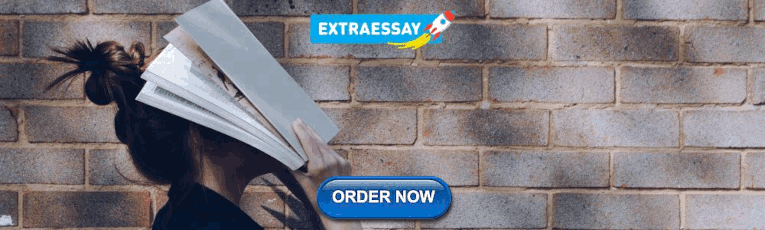
Java Polymorphism
- Method Overloading
- Method Overriding
- Covariant Return Type
- super keyword
- Instance Initializer block
- final keyword
- Runtime Polymorphism
- Dynamic Binding
- instanceof operator
Java Abstraction
- Abstract class
- Abstract vs Interface
Java Encapsulation
- Access Modifiers
- Encapsulation
Java OOPs Misc
- Object class
- Object Cloning
- Wrapper Class
- Java Recursion
- Call By Value
- strictfp keyword
- javadoc tool
- Command Line Arg
- Object vs Class
- Overloading vs Overriding
Java String
- What is String
- Immutable String
- String Comparison
- String Concatenation
- Methods of String class
- StringBuffer class
- StringBuilder class
- String vs StringBuffer
- StringBuffer vs Builder
- Creating Immutable class
- toString method
- StringTokenizer class
- Java String FAQs
Java String Methods
- String charAt()
- String compareTo()
- String concat()
- String contains()
- String endsWith()
- String equals()
- equalsIgnoreCase()
- String format()
- String getBytes()
- String getChars()
- String indexOf()
- String intern()
- String isEmpty()
- String join()
- String lastIndexOf()
- String length()
- String replace()
- String replaceAll()
- String split()
- String startsWith()
- String substring()
- String toCharArray()
- String toLowerCase()
- String toUpperCase()
- String trim()
Exception Handling
- Java Exceptions
- Java Try-catch block
- Java Multiple Catch Block
- Java Nested try
- Java Finally Block
- Java Throw Keyword
- Java Exception Propagation
- Java Throws Keyword
- Java Throw vs Throws
- Final vs Finally vs Finalize
- Exception Handling with Method Overriding
- Java Custom Exceptions
Java Inner Class
- What is inner class
- Member Inner class
- Anonymous Inner class
- Local Inner class
- static nested class
- Nested Interface
Java Multithreading
- What is Multithreading
- Life Cycle of a Thread
- How to Create Thread
- Thread Scheduler
- Sleeping a thread
- Start a thread twice
- Calling run() method
- Joining a thread
- Naming a thread
- Thread Priority
- Daemon Thread
- Thread Pool
- Thread Group
- ShutdownHook
- Performing multiple task
- Garbage Collection
- Runtime class
Java Synchronization
- Synchronization in java
- synchronized block
- static synchronization
- Deadlock in Java
- Inter-thread Comm
- Interrupting Thread
- Reentrant Monitor
Java Networking
- Networking Concepts
- Socket Programming
- URLConnection class
HttpURLConnection
- InetAddress class
Java Applet
- Applet Basics
- Graphics in Applet
- Displaying image in Applet
- Animation in Applet
- EventHandling in Applet
- JApplet class
- Painting in Applet
- Digital Clock in Applet
- Analog Clock in Applet
- Parameter in Applet
- Applet Communication
Java Reflection
- Reflection API
- newInstance() method
- creating javap tool
- creating appletviewer
- Call private method
Java Conversion
- Java String to int
- Java int to String
- Java String to long
- Java long to String
- Java String to float
- Java float to String
- Java String to double
- Java double to String
- Java String to Date
- Java Date to String
- Java String to char
- Java char to String
- Java String to Object
- Java Object to String
- Java int to long
- Java long to int
- Java int to double
- Java double to int
- Java char to int
- Java int to char
- Java String to boolean
- Java boolean to String
- Date to Timestamp
- Timestamp to Date
- Binary to Decimal
- Decimal to Binary
- Hex to Decimal
- Decimal to Hex
- Octal to Decimal
- Java Convert Decimal to Octal
- JDBC Introduction
- JDBC Driver
- DB Connectivity Steps
- Connectivity with Oracle
- Connectivity with MySQL
- Access without DSN
- DriverManager
- PreparedStatement
- ResultSetMetaData
- DatabaseMetaData
- Store image
- Retrieve image
- Retrieve file
- CallableStatement
- Transaction Management
- Batch Processing
- RowSet Interface
- Internationalization
- ResourceBundle class
- I18N with Date
- I18N with Time
- I18N with Number
- I18N with Currency
- Java Array Class
- getBoolean()
- getDouble()
- getLength()
- newInstance()
- setBoolean()
- setDouble()
- Java AtomicInteger Class
- addAndGet(int delta)
- compareAndSet(int expect, int update)
- decrementAndGet()
- doubleValue()
- floatValue()
- getAndAdd()
- getAndDecrement()
- getAndSet()
- incrementAndGet()
- getAndIncrement()
- lazySet(int newValue)
- longValue()
- set(int newValue)
- weakCompareAndSet(int expect,int newValue)
- Java AtomicLong Methods
- addAndGet()
- compareAndSet()
- weakCompareAndSet()
- Java Authenticator
- getPasswordAuthentication()
- getRequestingHost()
- getRequestingPort()
- getRequestingPrompt()
- getRequestingProtocol()
- getRequestingScheme()
- getRequestingSite()
- getRequestingURL()
- getRequestorType()
- setDefault()
- Java BigDecimal class
- intValueExact()
- movePointLeft()
- movePointRight()
- Big Integer Class
- bitLength()
- compareTo()
- divideAndRemainder()
- getLowestSetBit()
- isProbablePrime()
- modInverse()
- nextProbablePrime()
- probablePrime()
- shiftLeft()
- shiftRight()
- toByteArray()
- Java Boolean class
- booleanValue()
- logicalAnd()
- logicalOr()
- logicalXor()
- parseBoolean()
Java Byte Class
- byteValue()
- compareUnsigned()
- parseByte()
- shortValue()
- toUnsignedInt()
- toUnsignedLong()
- asSubclass()
- desiredAssertionStatus()
- getAnnotatedInterfaces()
- getAnnotatedSuperclass()
- getAnnotation()
- getAnnotationsByType()
- getAnnotations()
- getCanonicalName()
- getClasses()
- getClassLoader()
- getComponentType
- getConstructor()
- getConstructors()
- getDeclaredAnnotation()
- getDeclaredAnnotationsByType()
- getDeclaredAnnotations()
- getDeclaredConstructor()
- getDeclaredConstructors()
- getDeclaredField()
- getDeclaredFields()
- getDeclaredMethod()
- getDeclaredMethods()
- getDeclaringClass()
- getFields()
- getGenericInterfaces()
- getGenericSuperClass()
- getInterfaces()
- getMethod()
- getMethods()
- getModifiers()
- getPackage()
- getPackageName()
- getProtectionDomain()
- getResource()
- getSigners()
- getSimpleName()
- getSuperClass()
- isAnnotation()
- isAnnotationPresent()
- isAnonymousClass()
- isInstance()
- isInterface()
- isPrimitive()
- isSynthetic()
- Java Collections class
- asLifoQueue()
- binarySearch()
- checkedCollection()
- checkedList()
- checkedMap()
- checkedNavigableMap()
- checkedNavigableSet()
- checkedQueue()
- checkedSet()
- checkedSortedMap()
- checkedSortedSet()
- emptyEnumeration()
- emptyIterator()
- emptyList()
- emptyListIterator()
- emptyNavigableMap()
- emptyNavigableSet()
- emptySortedMap()
- emptySortedSet()
- enumeration()
- frequency()
- indexOfSubList()
- lastIndexOfSubList()
- newSetFromMap()
- replaceAll()
- reverseOrder()
- singleton()
- singletonList()
- singletonMap()
- synchronizedCollection()
- synchronizedList()
- synchronizedMap()
- synchronizedNavigableMap()
- synchronizedNavigableSet()
- synchronizedSet()
- synchronizedSortedMap()
- synchronizedSortedSet()
- unmodifiableCollection()
- unmodifiableList()
- unmodifiableMap()
- unmodifiableNavigableMap()
- unmodifiableNavigableSet()
- unmodifiableSet()
- unmodifiableSortedMap()
- unmodifiableSortedSet()
Java Compiler Class
- Java Compiler
- compileClass()
- compileClasses()
CopyOnWriteArrayList
- Java CopyOnWriteArrayList
- lastIndexOf()
Java Math Methods
- Math.round()
- Math.sqrt()
- Math.cbrt()
- Math.signum()
- Math.ceil()
- Math.copySign()
- Math.nextAfter()
- Math.nextUp()
- Math.nextDown()
- Math.floor()
- Math.floorDiv()
- Math.random()
- Math.rint()
- Math.hypot()
- Math.getExponent()
- Math.IEEEremainder()
- Math.addExact()
- Math.subtractExact()
- Math.multiplyExact()
- Math.incrementExact()
- Math.decrementExact()
- Math.negateExact()
- Math.toIntExact()
- Math.log10()
- Math.log1p()
- Math.expm1()
- Math.asin()
- Math.acos()
- Math.atan()
- Math.sinh()
- Math.cosh()
- Math.tanh()
- Math.toDegrees
- Math.toRadians
LinkedBlockingDeque
- Java LinkedBlockingDeque
- descendingIterator()
- offerFirst()
- offerLast()
- peekFirst()
- pollFirst()
- Java Long class
LinkedTransferQueue
- Java LinkedTransferQueue
- spliterator()
- Difference between Array and ArrayList
- When to use ArrayList and LinkedList in Java
- Difference between ArrayList and Vector
- How to Compare Two ArrayList in Java
- How to reverse ArrayList in Java
- How to make ArrayList Read Only
- Difference between length of array and size() of ArrayList in Java
- How to Synchronize ArrayList in Java
- How to convert ArrayList to Array and Array to ArrayList in java
- Array vs ArrayList in Java
- How to Sort Java ArrayList in Descending Order
- How to remove duplicates from ArrayList in Java
- Java MulticastSocket
- getInterface()
- getLoopbackMode()
- getNetworkInterface()
- getTimeToLive()
- joinGroup()
- leaveGroup()
- setInterface()
- setLoopbackMode()
- setNetworkInterface()
- setTimeToLive()
- Java Number Class
Java Phaser Class
- Java Phaser
- arriveAndAwaitAdvance()
- arriveAndDeregister()
- getParent()
- awaitAdvanceInterruptibly()
- awaitAdvance()
- bulkRegister()
- forceTermination()
- getArrivedParties()
- getRegisteredParties()
- getUnarrivedParties()
- isTerminated()
ArrayList Methods
- listIterator()
- removeRange
Java Thread Methods
- currentThread()
- getPriority()
- setPriority()
- setDaemon()
- interrupt()
- isinterrupted()
- interrupted()
- activeCount()
- checkAccess()
- dumpStack()
- getStackTrace()
- enumerate()
- getThreadGroup()
- notifyAll()
- setContextClassLoader()
- getContextClassLoader()
- getDefaultUncaughtExceptionHandler()
- setDefaultUncaughtExceptionHandler()
Java Projects
- Free Java Projects
- Payment Bill(JSP)
- Transport (JSP)
- Connect Globe (JSP)
- Online Banking (JSP)
- Online Quiz (JSP)
- Classified (JSP)
- Mailcasting (JSP)
- Online Library (JSP)
- Pharmacy (JSP)
- Mailer (Servlet)
- Baby Care (Servlet)
- Chat Server (Core)
- Library (Core)
- Exam System (Core)
- Java Apps (Core)
- Fee Report (Core)
- Fee (Servlet)
- eLibrary (Servlet)
- Fire Detection
- Attendance System
- Fibonacci Series in Java
- Prime Number Program in Java
- Palindrome Program in Java
- Factorial Program in Java
- Armstrong Number in Java
- How to Generate Random Number in Java
- How to Print Pattern in Java
- How to Compare Two Objects in Java
- How to Create Object in Java
- How to Print ASCII Value in Java
- How to Reverse a Number in Java
- Java Program to convert Number to Word
- Automorphic Number Program in Java
- Peterson Number in Java
- Sunny Number in Java
- Tech Number in Java
- Fascinating Number in Java
- Keith Number in Java
- Neon Number in Java
- Spy Number in Java
- ATM program Java
- Autobiographical Number in Java
- Emirp Number in Java
- Sphenic Number in Java
- Buzz Number Java
- Duck Number Java
- Evil Number Java
- ISBN Number Java
- Krishnamurthy Number Java
- Bouncy Number in Java
- Mystery Number in Java
- Smith Number in Java
- Strontio Number in Java
- Xylem and Phloem Number in Java
- nth Prime Number Java
- Java Program to Display Alternate Prime Numbers
- Java Program to Find Square Root of a Number Without sqrt Method
- Java Program to Swap Two Numbers Using Bitwise Operator
- Java Program to Find GCD of Two Numbers
- Java Program to Find Largest of Three Numbers
- Java Program to Find Smallest of Three Numbers Using Ternary Operator
- Java Program to Check if a Number is Positive or Negative
- Java Program to Check if a Given Number is Perfect Square
- Java Program to Display Even Numbers From 1 to 100
- Java Program to Display Odd Numbers From 1 to 100
- Java Program to Find Sum of Natural Numbers
- Java Program to copy all elements of one array into another array
- Java Program to find the frequency of each element in the array
- Java Program to left rotate the elements of an array
- Java Program to print the duplicate elements of an array
- Java Program to print the elements of an array
- Java Program to print the elements of an array in reverse order
- Java Program to print the elements of an array present on even position
- Java Program to print the elements of an array present on odd position
- Java Program to print the largest element in an array
- Java Program to print the smallest element in an array
- Java Program to print the number of elements present in an array
- Java Program to print the sum of all the items of the array
- Java Program to right rotate the elements of an array
- Java Program to sort the elements of an array in ascending order
- Java Program to sort the elements of an array in descending order
- Java Program to Find 3rd Largest Number in an array
- Java Program to Find 2nd Largest Number in an array
- Java Program to Find Largest Number in an array
- Java to Program Find 2nd Smallest Number in an array
- Java Program to Find Smallest Number in an array
- Java Program to Remove Duplicate Element in an array
- Java Program to Print Odd and Even Numbers from an array
- How to Sort an Array in Java
- Java Matrix Programs
- Java Program to Add Two Matrices
- Java Program to Multiply Two Matrices
- Java Program to subtract the two matrices
- Java Program to determine whether two matrices are equal
- Java Program to display the lower triangular matrix
- Java Program to display the upper triangular matrix
- Java Program to find the frequency of odd & even numbers in the given matrix
- Java Program to find the product of two matrices
- Java Program to find the sum of each row and each column of a matrix
- Java Program to find the transpose of a given matrix
- Java Program to determine whether a given matrix is an identity matrix
- Java Program to determine whether a given matrix is a sparse matrix
- Java Program to Transpose matrix
- Java Program to count the total number of characters in a string
- Java Program to count the total number of characters in a string 2
- Java Program to count the total number of punctuation characters exists in a String
- Java Program to count the total number of vowels and consonants in a string
- Java Program to determine whether two strings are the anagram
- Java Program to divide a string in 'N' equal parts.
- Java Program to find all subsets of a string
- Java Program to find the longest repeating sequence in a string
- Java Program to find all the permutations of a string
- Java Program to remove all the white spaces from a string
- Java Program to replace lower-case characters with upper-case and vice-versa
- Java Program to replace the spaces of a string with a specific character
- Java Program to determine whether a given string is palindrome
- Java Program to determine whether one string is a rotation of another
- Java Program to find maximum and minimum occurring character in a string
- Java Program to find Reverse of the string
- Java program to find the duplicate characters in a string
- Java program to find the duplicate words in a string
- Java Program to find the frequency of characters
- Java Program to find the largest and smallest word in a string
- Java Program to find the most repeated word in a text file
- Java Program to find the number of the words in the given text file
- Java Program to separate the Individual Characters from a String
- Java Program to swap two string variables without using third or temp variable.
- Java Program to print smallest and biggest possible palindrome word in a given string
- Reverse String in Java Word by Word
- Reserve String without reverse() function
- Linear Search in Java
- Binary Search in Java
- Bubble Sort in Java
- Selection Sort in Java
- Insertion Sort in Java
- How to convert String to int in Java
- How to convert int to String in Java
- How to convert String to long in Java
- How to convert long to String in Java
- How to convert String to float in Java
- How to convert float to String in Java
- How to convert String to double in Java
- How to convert double to String in Java
- How to convert String to Date in Java
- How to convert Date to String in Java
- How to convert String to char in Java
- How to convert char to String in Java
- How to convert String to Object in Java
- How to convert Object to String in Java
- How to convert int to long in Java
- How to convert long to int in Java
- How to convert int to double in Java
- How to convert double to int in Java
- How to convert char to int in Java
- How to convert int to char in Java
- How to convert String to boolean in Java
- How to convert boolean to String in Java
- How to convert Date to Timestamp in Java
- How to convert Timestamp to Date in Java
- How to convert Binary to Decimal in Java
- How to convert Decimal to Binary in Java
- How to convert Hex to Decimal in Java
- How to convert Decimal to Hex in Java
- How to convert Octal to Decimal in Java
- How to convert Decimal to Octal in Java
- Java program to print the following spiral pattern on the console
- Java program to print the following pattern
- Java program to print the following pattern 2
- Java program to print the following pattern 3
- Java program to print the following pattern 4
- Java program to print the following pattern 5
- Java program to print the following pattern on the console
- Java program to print the following pattern on the console 2
- Java program to print the following pattern on the console 3
- Java program to print the following pattern on the console 4
- Java program to print the following pattern on the console 5
- Java program to print the following pattern on the console 6
- Java program to print the following pattern on the console 7
- Java program to print the following pattern on the console 8
- Java program to print the following pattern on the console 9
- Java program to print the following pattern on the console 10
- Java program to print the following pattern on the console 11
- Java program to print the following pattern on the console 12
- Singly linked list Examples in Java
- Java Program to create and display a singly linked list
- Java program to create a singly linked list of n nodes and count the number of nodes
- Java program to create a singly linked list of n nodes and display it in reverse order
- Java program to delete a node from the beginning of the singly linked list
- Java program to delete a node from the middle of the singly linked list
- Java program to delete a node from the end of the singly linked list
- Java program to determine whether a singly linked list is the palindrome
- Java program to find the maximum and minimum value node from a linked list
- Java Program to insert a new node at the middle of the singly linked list
- Java program to insert a new node at the beginning of the singly linked list
- Java program to insert a new node at the end of the singly linked list
- Java program to remove duplicate elements from a singly linked list
- Java Program to search an element in a singly linked list
- Java program to create and display a Circular Linked List
- Java program to create a Circular Linked List of N nodes and count the number of nodes
- Java program to create a Circular Linked List of n nodes and display it in reverse order
- Java program to delete a node from the beginning of the Circular Linked List
- Java program to delete a node from the end of the Circular Linked List
- Java program to delete a node from the middle of the Circular Linked List
- Java program to find the maximum and minimum value node from a circular linked list
- Java program to insert a new node at the beginning of the Circular Linked List
- Java program to insert a new node at the end of the Circular Linked List
- Java program to insert a new node at the middle of the Circular Linked List
- Java program to remove duplicate elements from a Circular Linked List
- Java program to search an element in a Circular Linked List
- Java program to sort the elements of the Circular Linked List
- Java program to convert a given binary tree to doubly linked list
- Java program to create a doubly linked list from a ternary tree
- Java program to create a doubly linked list of n nodes and count the number of nodes
- Java program to create a doubly linked list of n nodes and display it in reverse order
- Java program to create and display a doubly linked list
- Java program to delete a new node from the beginning of the doubly linked list
- Java program to delete a new node from the end of the doubly linked list
- Java program to delete a new node from the middle of the doubly linked list
- Java program to find the maximum and minimum value node from a doubly linked list
- Java program to insert a new node at the beginning of the Doubly Linked list
- Java program to insert a new node at the end of the Doubly Linked List
- Java program to insert a new node at the middle of the Doubly Linked List
- Java program to remove duplicate elements from a Doubly Linked List
- Java program to rotate doubly linked list by N nodes
- Java program to search an element in a doubly linked list
- Java program to sort the elements of the doubly linked list
- Java Program to calculate the Difference between the Sum of the Odd Level and the Even Level Nodes of a Binary Tree
- Java program to construct a Binary Search Tree and perform deletion and In-order traversal
- Java program to convert Binary Tree to Binary Search Tree
- Java program to determine whether all leaves are at same level
- Java program to determine whether two trees are identical
- Java program to find maximum width of a binary tree
- Java program to find the largest element in a Binary Tree
- Java program to find the maximum depth or height of a tree
- Java program to find the nodes which are at the maximum distance in a Binary Tree
- Java program to find the smallest element in a tree
- Java program to find the sum of all the nodes of a binary tree
- Java program to find the total number of possible Binary Search Trees with N keys
- Java program to implement Binary Tree using the Linked List
- Java program to search a node in a Binary Tree
- Candy Distribution Problem in Java
- Find Rectangle in a Matrix with Corner as 1 in Java
- Minimum Number of Taps to Open to Water a Garden in Java
- Seven Segment Display Problem in Java
- Split Array Largest Sum in Java
- Find Original Array from a Double Array in Java
- Minimum Lights to Activate Problem in Java
- Rotate List in Java
- Count of Range Sum Problem in Java
- Create A Tree of Coprime in Java
- Convert Integer to Roman Numerals in Java
- Check if n and its Double Exist or not in Java
- Array of Doubled Pair Problem in Java
- Tag Content Extractor Problem in Java
- Convert Roman to Integer in Java
- Minimum Number of Flips to Convert Binary Matrix into Zero Matrix in Java
- XOR of Array Elements Except Itself in Java
- Check If the Given Array is Mirror Inverse in Java
- How to Create a Mirror Image of A 2D Array in Java
- Add Numbers Represented by Linked Lists in Java
- Majority Element In an Array in Java
- Block Swap Algorithm or Array Rotation in Java
- Minimum Difference Among Group Size Two in Java
- Missing Number in An Arithmetic Progression in Java
- Peak Index of Mountain Array Problem in Java
- Minimum Number of Meeting Room Required Problem in Java
- Move Zeros to End in Java
- Count Inversions in an array in Java
- Count Smaller Elements on The Right Side in Java
- Interleaving String Problem in Java
- Bulb Chain Problem in Java
- Java Main Method
- System.out.println()
- Java Memory Management
- Java ClassLoader
- Java Decompiler
- Java vs. JavaScript
- Java vs. Kotlin
- Java vs. Python
- Java Absolute Value
- How to Create File
- Delete a File in Java
- Open a File in Java
- Sort a List in Java
- Convert byte Array to String
- Java Basics
- How to Compile & Run Java Program
- How to Run Java Program in Eclipse
- How to Verify Java Version
- Ways to Create an Object in Java
- How to Run a Java program in Windows 10
- Runnable Interface in Java
- Java Keystore
- Get input from user in Java
- Read file line by line in Java
- Take String input in Java
- How to Read Excel File in Java
- Read XML File in Java
- CompletableFuture in Java
- Java ExecutorService
- How to iterate Map in Java
- How to Return an Array in Java
- How to Sort HashMap by Value
- How to Sort HashMap in Java
- Load Factor in HashMap
- Array vs ArrayList
- HashMap vs TreeMap
- HashSet vs HashMap class
- Compare Two ArrayList in Java
- Merge Two Arrays in Java
- Print Array in Java
- Read CSV File in Java
- Remove Special Characters from String
- ArrayIndexOutOfBoundsException
- ConcurrentModificationException
- NoSuchElementException
- NumberFormatException
- How to Sort ArrayList in Java
- How to Download Java
- How to Call a Method in Java
- How to Create Singleton Class in Java
- How to Find Array Length in Java
- How to Read Character in Java
- Can We Overload main() Method in Java
- How to Convert Char Array to String in Java
- How to Run Java Program in CMD Using Notepad
- How to Sort String Array in Java
- How to Compare Dates in Java
- How to Take Multiple String Input in Java Using Scanner
- How to Remove Last Character from String in Java
- How TreeMap Works Internally in Java
- Java Program to Break Integer into Digits
- Java Program to Calculate Area and Circumference of Circle
- What is Diamond Problem in Java
- Java Program to Read Number from Standard Input
- How to Download Minecraft Java Edition
- Can We Override Static Method in Java
- How to Avoid Deadlock in Java
- How to Achieve Abstraction in Java
- How Garbage Collection Works in Java
- How to Take Array Input in Java
- How to Create Array of Objects in Java
- How to Create Package in Java
- How to Print in Java
- What is Framework in Java
- Why Java is Secure
- How to Iterate List in Java
- How to Use Eclipse for Java
- Which Package is Imported by Default in Java
- Could Not Find or Load Main Class in Java
- How to Compare Two Arrays in Java
- How to Convert String to JSON Object in Java
- Which is Better Java or Python
- How to Update Java
- How to Get Value from JSON Object in Java Example
- How to Split a String in Java with Delimiter
- Structure of Java Program
- Why We Use Constructor in Java
- Java Create Excel File
- Java Interpreter
- javac is not Recognized
- Dynamic Array in Java
- Shunting yard algorithm
- Java Destructor
- Custom ArrayList in Java
- ArrayList vs HashMap
- Java Constant
- Java Tokens
- How to Enable Java in Chrome
- Java Semaphore
- Array to List in Java
- JIT in Java
- How to Clear Screen in Java
- Java Logger
- Reverse a String Using Recursion in Java
- Java Path Vs File
- Float Vs Double Java
- Stack vs Heap Java
- Abstraction vs Encapsulation
- Top 10 Java Books
- Public vs Private
- What is Java Used For
- Bitwise Operator in Java
- SOLID Principles Java
- Type Casting in Java
- Conditional Operator in Java
- Ternary Operator Java
- Java Architecture
- REPL in Java
- Types of Exception in Java
- Why String is Immutable or Final in Java
- Java vs Kotlin
- Set in Java
- Why non-static variable cannot be referenced from a static context in Java
- Java Developer Roles and Responsibilities
- Types of Classes in Java
- Marker Interface in Java
- Static Function in Java
- Unary Operators in Java
- What is Advance Java
- ArrayList Implementation
- Convert ArrayList to String Array
- Hashmap vs ConcurrentHashMap
- List vs ArrayList
- Map vs HashMap
- HashSet vs LinkedHashSet
- How TreeSet Works Internally
- LinkedHashMap vs HashMap
- Java Program to Solve Quadratic Equation
- Scope Resolution Operator in Java
- Composition in Java
- File Operations in Java
- NoClassDefFoundError in Java
- Thread Concept in Java
- Upcasting and Downcasting in Java
- Dynamic Polymorphism in Java
- String Pool in Java
- What is constructor chaining in Java
- Add elements to Array in Java
- Advantages and disadvantages of Java
- Advantages of JavaBeans
- AWS SDK for Java with Apache Maven
- AWT and Swing in Java
- AWT Program in Java
- Boolean values in Java
- ByteStream Classes in Java
- CharacterStream Classes in Java
- Class and Interface in Java
- ClassCast Exception in Java
- Cloneable in Java
- Constructor overloading in Java
- Control Flow in Java
- Convert Java Object to Json using GSON
- Convert XML to JSON in Java
- How to avoid null pointer exception in Java
- Java constructor returns a value, but what
- Singleton Class in Java
- Doubly Linked List Program in Java
- Association in Java
- Big data Java vs Python
- Branching Statements in Java
- Collections Sort in Java 8
- List vs Set in Java
- How many days required to learn Java
- Implicitly Typecasting in Java
- Legacy Class in Java
- Character Array in Java
- Equals() and Hashcode() in Java
- Externalization in Java
- Identifiers in Java
- InvocationTargetException
- Java Pass by Value
- Mutable and Immutable in Java
- Power Function in Java
- Primitive Data Types in Java
- String Array in Java
- Virtual Function in Java
- C vs C++ vs Java
- Java String Max Size
- Convert Java object to JSON
- How to Calculate Date Difference in Java
- How to Improve Coding Skills in Java
- Java Email Validation
- Java Testing Tools
- Permutation and Combination in Java
- Unique Number in Java Program
- Java Code for DES
- Pig Latin Program in Java
- Array Rotation in Java
- Equilibrium Index of an Array in Java
- Different Ways to Print Exception Message in Java
- Java Copy Constructor Example
- Why We Use Static Class in Java
- What is Core Java
- Set vs Map in Java
- How to Create a New Folder in Java
- Remove an Element from ArrayList in Java
- How to Create Test Cases for Exceptions in Java
- How to Convert JSON Array to ArrayList in Java
- How to Create a Class File in Java
- Java Spring Pros & Cons
- Java Stack Trace
- Array Slicing in Java
- Flutter vs Java
- Permutation of Numbers in Java
- Magic Number in Java
- Reference Data Types in Java
- Counter variable in Java
- How to take Character Input in Java using BufferedReader Class
- Java employee details program
- Java is case sensitive explain
- Ramanujan Number or Taxicab Number in Java
- Advanced Java Books in 2021
- Fail Fast and Fail Safe Iterator in Java
- How to build a Web Application Using Java
- Is Java Interpreted or Compiled
- Java Big Data Frameworks
- Java Get Data From URL
- No Main Manifest Attribute
- Java missing return statement
- Java program to remove duplicate characters from a string
- JUnit test case example in Java
- List of logical programs in Java
- PermGen space Java
- Unsigned Right Shift Operator in Java
- Infix to Postfix Java
- Memory Leak in Java
- How To Write Test Cases In Java
- Java 32-Bit Download For Windows 10
- FizzBuzz Program in Java
- A Java Runtime Environment JRE Or JDK Must Be Available
- Java Does Not Open
- No Java Virtual Machine was Found
- Java Program Number to Word
- Types of Garbage Collector in Java
- No Suitable Driver Found For JDBC
- AVL Tree program in Java
- Fail-fast and Fail-safe in Java
- Find unique elements in array Java
- Highest precedence in Java
- Java Closure
- Java String Encoding
- Prim's algorithm Java
- Quartz scheduler java
- Red Black Tree Java
- GC Overhead Limit Exceeded
- Generating QR Code in Java
- Delegation Event Model in Java
- Java Profilers
- Java Flight Recorder
- Bucket Sort in Java
- Java Atomic
- Wait vs Sleep in Java
- Executor Framework Java
- Gregorian calendar Java
- int vs Integer Java
- What is truncation in Java
- Java HTTP Proxy Server
- Java Static Constructor
- How to prepare for Java Interview
- Java callback function
- Java 8 vs Java 11
- Login Form Java
- Vaadin Framework Java
- EJB vs. Spring
- Types of Applets in Java
- Visitor Design Pattern Java
- Advantages of Python over Java
- Design Principles in Java
- JSON Validator Java
- Pseudocode Java
- Windows Programming Using Java
- Vert.x Java
- Complex Java Programs
- ORE Number Java
- PalPrime Number Java
- Twin Prime Numbers
- Twisted Prime Number Java
- Ugly number Java
- Achilles Number in Java
- Amicable Pair Number in Java
- Playfair Cipher Program in Java
- Java.lang.outofmemoryerror: java heap space
- Banker's Algorithm Java
- Kruskal Algorithm Java
- Longest Common Subsequence
- Travelling Salesman Problem
- & vs && in Java
- Jumping Number in Java
- Lead Number in Java
- Lucky Number in Java
- Middle Digit Number in Java
- Special Number in Java
- Passing Array to Function In Java
- Lexicographical Order Java
- Adam Number in Java
- Bell Number in Java
- Reduce Java
- LRU Cache Implementation
- Goldbach Number in Java
- How to Find Number of Objects Created in Java
- Multiply Two Numbers Without Using Arithmetic Operator in Java
- Sum of Digits of a Number in Java
- Sum of Numbers in Java
- Power of a Number in Java
- Sum of Prime Numbers in Java
- Cullen Number in Java
- Mobile Number Validation in Java
- Fermat Number in Java
- Instantiation in Java
- Exception Vs Error in Java
- flatMap() Method in Java 8
- How to Print Table in Java
- Java Create PDF
- Mersenne Number in Java
- Pandigital Number in Java
- Pell Number in Java
- Java Get Post
- Fork Join in Java
- Java Callable Example
- Blockchain Java
- Design of JDBC
- Java Anon Proxy
- Knapsack Problem Java
- Session Tracking in Java
- What is Object-Oriented Programming
- Literals in Java
- Square Free Number in Java
- What is an anagram in Java
- What is programming
- Iterate JSON Array Java
- Java Date Add Days
- Javac Command Not Found
- Factorial Program in Java Using while Loop
- Frugal Number in Java
- Java Digital Signature
- Catalan Number in Java
- Partition Number in Java
- Powerful Number in Java
- Practical Number in Java
- Chromatic Number in Java
- Sublime Number in Java
- Advanced Java Viva Questions
- Getter and Setter Method in Java Example
- How to convert String to String array in Java
- How to Encrypt Password in Java
- Instance Variable in Java
- Java File Extension
- Types of Inheritance in Java
- Untouchable Number in Java
- AES 256 Encryption in Java
- Applications of Array in Java
- Example of Static Import in Java
- Hill Cipher Program in Java
- Lazy Loading in Java
- Rectangular Number in Java
- How to Print Table in Java Using Formatter
- IdentityHashMap Class in Java
- Undulating Number in Java
- Java Obfuscator
- Java Switch String
- Applet Life Cycle in Java
- Banking Application in Java
- Duodecimal in Java
- Economical Number in Java
- Figurate Number in Java
- How to resolve IllegalStateException in Java
- Java Coding Software
- Java Create Jar Files
- Java Framework List
- Java Initialize array
- java lang exception no runnable methods
- Nonagonal Number in Java
- SexagesimalFormatter in Java
- Sierpinski Number in Java
- Vigesimal in Java
- Java Color Codes
- JDoodle Java
- Online Java Compiler
- Pyramidal Number in Java
- Relatively Prime in Java
- Java Modulo
- Repdigit Numbers in Java
- Abstract Method in Java
- Convert Text-to-Speech in Java
- Java Editors
- MVC Architecture in Java
- Narcissistic Number in Java
- Hashing Algorithm in Java
- Java Escape Characters
- Java Operator Precedence
- Private Constructor in Java
- Scope of Variables in Java
- Groovy vs Java
- Java File Upload to a Folder
- Java Full Stack
- Java Developer
- Thread States in Java
- Java EE vs Node.js
- Loose Coupling in Java
- Java Top 10 Libraries
- Method Hiding in Java
- Dijkstra Algorithm Java
- Extravagant Number in Java
- Java Unicode
- New Line in Java
- Return Statement in Java
- Order of Execution of Constructors in Java Inheritance
- Cardinal Number in Java
- Hyperfactorial in Java
- Identifier Expected Error in Java
- Java Generate UUID
- Labeled Loop in Java
- Lombok Java
- Ordinal Number in Java
- Tetrahedral Number in Java
- Cosmic Superclass in Java
- Shallow Copy Java
- BiFunction Java 8
- Equidigital Number in Java
- Fall Through in Java
- Java Reserved Keywords
- Parking Lot Design Java
- Boyer Moore Java
- Java Security Framework
- Tetranacci Number in Java
- BFS Algorithm in Java
- CountDownLatch in Java
- Counting sort in Java
- CRC Program in Java
- FileNotFoundException in Java
- InputMismatchException in Java
- Java ASCII Table
- Lock in Java
- Segment Tree in Java
- Why main() method is always static in Java
- Bellman-Ford Algorithm Java
- BigDecimal toString() in Java
- .NET vs Java
- Java ZipFile
- Lazy Propagation in Segment Tree in Java
- Magnanimous Number in Java
- Binary Tree Java
- How to Create Zip File in Java
- Java Dot Operator
- Associativity of Operators in Java
- Fenwick Tree in Java
- How annotation works in Java
- How to Find Length of Integer in Java
- Java 8 filters
- List All Files in a Directory in Java
- How to Get Day Name from Date in Java
- Zigzag Array in Java
- Class Definition in Java
- Find Saddle Point of a Matrix in Java
- Non-primitive data types in Java
- Pancake Number in Java
- Pancake Sorting in Java
- Print Matrix Diagonally in Java
- Sort Dates in Java
- Carmichael Numbers in Java
- Contextual Keywords in Java
- How to Open Java Control Panel
- How to Reverse Linked List in Java
- Interchange Diagonal Elements Java Program
- Java Set to List
- Level Order Traversal of a Binary Tree in Java
- Bully algorithm in Java
- Convert JSON File to String in Java
- Convert Milliseconds to Date in Java
- Copy Content/ Data From One File to Another in Java
- Constructor vs Method in Java
- Access Specifiers vs Modifiers
- Java vs PHP
- replace() vs replaceAll() in Java
- this vs super in Java
- Heap implementation in Java
- How to Check null in Java
- Java Arrays Fill
- Rotate Matrix by 90 Degrees in Java
- Exception Class in Java
- Transient variable in Java
- Web crawler Java
- Zigzag Traversal of a Binary Tree in Java
- Java Get File Size
- Internal Working of ArrayList in Java
- Java Program to Print Matrix in Z Form
- Vertical Order Traversal of a Binary Tree in Java
- Group By in Java 8
- Hashing Techniques in Java
- Implement Queue Using Array in Java
- Java 13 Features
- Package Program in Java
- Canonical Name Java
- Method Chaining in Java
- Orphaned Case Java
- Bottom View of a Binary Tree in Java
- Coercion in Java
- Dictionary Class in Java
- Left View of a Binary Tree in Java
- Pangram Program in Java
- Top View of a Binary Tree in Java
- Tribonacci Series in Java
- Hollow Diamond Pattern in Java
- Normal and Trace of a Matrix in Java
- Right View of a Binary Tree in Java
- Dining Philosophers Problem and Solution in Java
- Shallow Copy vs Deep Copy in Java
- Java Password Generator
- Java Program for Shopping Bill
- Lock Interface in Java
- Convert JSON to Map in Java
- Convert JSON to XML in Java
- Middle Node of a Linked List in Java
- Pernicious Number in Java
- Cohesion in Java
- How to get UTC time in Java
- Jacobsthal Number in Java
- Java Calculate Age
- Tribonacci Number Java
- Bernoulli number in Java
- Cake Number in Java
- Compare time in Java
- Compare Two Sets in Java
- Crown Pattern in Java
- Convert List to Array in Java
- Aggregation vs Composition
- Morris Traversal for Inorder in Java
- Morris Traversal for Preorder in Java
- Package Naming Conversion in Java
- India Map Pattern in Java
- Ladder Pattern in Java
- ORM Tools in Java
- Odious Number in Java
- Rat in a Maze Problem in Java
- Sudoku in Java
- Christmas Tree Pattern in Java
- Double Hashing in Java
- Magic Square in Java
- Possible Paths from Top Left to Bottom Right of a Matrix in Java
- Palindrome Partitioning Problem in Java
- Rehashing in Java
- Round Robin Scheduling Program in Java
- Types of Statements in Java
- Compound Assignment Operator in Java
- Prime Points in Java
- Butterfly Pattern in Java
- Fish Pattern in Java
- Flag Pattern in Java
- Kite pattern in Java
- Swastika Pattern in Java
- Tug of War in Java
- Clone HashMap in Java
- Fibodiv Number in Java
- Heart Pattern in Java
- How to check data type in Java
- Java Array Clone
- Use of final Keyword in Java
- Factorial of a Large Number in Java
- Race Condition in Java
- Static Array in Java
- Water Jug Problem in Java
- Electricity Bill Program in Java
- Facts about null in Java
- Maximizing Profit in Stock Buy Sell in Java
- Permutation Coefficient in Java
- Convert List to String in Java
- List of Constants in Java
- MOOD Factors to Assess a Java Program
- Computing Digit Sum of All Numbers From 1 to n in Java
- Read PDF File in Java
- Finding Odd Occurrence of a Number in Java
- Java Indentation
- Zig Zag Star and Number Pattern in Java
- Check Whether a Number is a Power of 4 or not in Java
- Kth Smallest in an Unsorted Array in Java
- BlockingQueue in Java
- Next Greater Element in Java
- Star Numbers in Java
- 3N+1 Problem in Java
- Java Program to Find Local Minima in An Array
- Processing Speech in Java
- Java Output Formatting
- House Numbers in Java
- Java Program to Generate Binary Numbers
- Longest Odd-Even Subsequence in Java
- Java Subtract Days from Current Date
- Java Future Example
- Minimum Cost Path Problem in Java
- Diffie-Hellman Algorithm in Java
- Ganesha's Pattern in Java
- Hamming Code in Java
- Map of Map in Java
- Print Pencil Shape Pattern in Java
- Zebra Puzzle in Java
- Display Unique Rows in a Binary Matrix in Java
- Rotate A Matrix By 180 Degree in Java
- Dangling Else Problem in Java
- Java Application vs Java Applet
- Dutch National Flag Problem in Java
- Java Calculate Average of List
- compareToIgnoreCase Java
- Trimorphic Numbers in Java
- Arithmetic Exception in Java
- Java instanceof operator
- Java Localization
- Minimum XOR Value Pair in Java
- Iccanobif Numbers in Java
- Java Program to Count the Occurrences of Each Character
- Java Technologies List
- Java Program to Find the Minimum Number of Platforms Required for a Railway Station
- Shift Operators in Java
- Final Object in Java
- Object Definition in Java
- Shadowing in Java
- Zipping and Unzipping Files in Java
- Display the Odd Levels Nodes of a Binary Tree in Java
- Java Variable Declaration
- Nude Numbers in Java
- Java Programming Challenges
- Java URL Encoder
- anyMatch() in Java 8
- Sealed Class in Java
- Camel case in Java
- Career Options for Java Developers to Aim in 2022
- Java Progress Bar
- Maximum Rectangular Area in a Histogram in Java
- Polygonal Number in Java
- Two Sorted LinkedList Intersection in Java
- Set Matrix Zeros in Java
- Find Number of Island in Java
- Balanced Prime Number in Java
- Minecraft Bedrock vs Java Minecraft
- arr.length vs arr[0].length vs arr[1].length in Java
- Future in Java 8
- How to Set Timer in Java
- Construct the Largest Number from the Given Array in Java
- Minimum Coins for Making a Given Value in Java
- Eclipse Shortcuts Java
- Empty Statement in Java
- Java Program to Implement Two Stacks in an Array
- Java Snippet
- Longest Arithmetic Progression Sequence in Java
- Types of Sockets in Java
- Java Program to Add Digits Until the Number Becomes a Single Digit Number
- Next Greater Number with Same Set of Digits in Java
- Split the Number String into Primes in Java
- Java Cron Expression
- Huffman Coding Java
- Java Snippet Class
- Why Java is So Popular
- Java Project idea
- Java Web Development
- Brilliant Numbers in Java
- Sort Elements by Frequency in Java
- Beautiful Array in Java
- Moran Numbers in Java
- Intersection Point of Two Linked List in Java
- Sparse Number in Java
- How to Check JRE Version
- Java Programming Certification
- Two Decimal Places Java
- Eclipse Change Theme
- Java how to Convert Bytes to Hex
- Decagonal Numbers in Java
- Java Binary to Hexadecimal Conversion
- Java Hexadecimal to Binary Conversion
- How to Capitalize the First Letter of a String in Java
- Java &0XFF Example
- Stream findFirst() Method in Java
- Balanced Parentheses in Java
- Caesar Cipher Program in Java
- next() vs nextLine()
- Java Split String by Comma
- Spliterator in java 8
- Tree Model Nodes in Jackson
- Types of events in Java
- Callable and Future in Java
- How to Check Current JDK Version installed in Your System Using CMD
- How to Round Double and Float up to Two Decimal Places in Java
- Java 8 Multimap
- Parallel Stream in Java
- Java Convert Bytes to Unsigned Bytes
- Display List of TimeZone with GMT and UTC in Java
- Binary Strings Without Consecutive Ones in Java
- Convert IP to Binary in Java
- Returning Multiple Values in Java
- Centered Square Numbers in Java
- ProcessBuilder in Java
- How to Clear Java Cache
- IntSummaryStatistics Class in Java
- Java ProcessBuilder Example
- Java Program to Delete a Directory
- Java Program to Print Even Odd Using Two Threads
- Java Variant
- MessageDigest in Java
- Alphabet Pattern in Java
- Java Linter
- Java Mod Example
- Stone Game in Java
- TypeErasure in Java
- How to Remove substring from String in Java
- Program to print a string in vertical in Java
- How to Split a String between Numbers and Letters
- String Handling in Java
- Isomorphic String in Java
- Java ImageIO Class
- Minimum Difference Subarrays in Java
- Plus One to Array Problem in Java
- Unequal Adjacent Elements in Java
- Java Parallel Stream Example
- SHA Hashing in Java
- How to make Java projects
- Java Fibers
- Java MD5 Hashing Example
- Hogben Numbers in Java
- Self-Descriptive Numbers in Java
- Hybrid Inheritance in Java
- Java IP Address (IPv4) Regex Examples
- Converting Long to Date in JAVA
- Java 17 new features
- GCD of Different SubSequences in Java
- Sylvester Sequence in Java
- Console in Java
- Asynchronous Call in Java
- Minimum Window Substring in Java
- Nth Term of Geometric Progression in Java
- Coding Guidelines in Java
- Couple Holding Hands Problem in Java
- Count Ones in a Sorted binary array in Java
- Ordered Pair in Java
- Tetris Game in Java
- Factorial Trailing Zeroes in Java
- Java Assert Examples
- Minimum Insertion To Form A Palindrome in Java
- Wiggle Sort in Java
- Java Exit Code 13
- Java JFileChooser
- What is LINQ
- NZEC in Java
- Box Stacking Problem
- K Most Frequent Elements in Java
- Parallel Programming in Java
- How to Generate JVM Heap Memory Dump
- Java Program to use Finally Block for Catching Exceptions
- Count Login Attempts Java
- Largest Independent Set in Java
- Longest Subarray With All Even or Odd Elements in Java
- Open and Closed Hashing in Java
- DAO Class in Java
- Kynea Numbers in Java
- UTF in Java
- Zygodromes in Java
- ElasticSearch Java API
- Form Feed in Java
- Java Clone Examples
- Payment Gateway Integration in Java
- What is PMD
- RegionMatches() Method in Java
- Repaint() Method in Java
- Serial Communication in Java
- Count Double Increasing Series in A Range in Java
- Longest Consecutive Subsequence in Java
- Smallest Subarray With K Distinct Numbers in Java
- String Sort Custom in Java
- Count Number of Distinct Substrings in a String in Java
- Display All Subsets of An Integer Array in Java
- Digit Count in a Factorial Of a Number in Java
- Valid Parentheses Problem in Java
- Median Of Stream Of Running Integers in Java
- Arrow Operator in Java
- Java Learning app
- Create Preorder Using Postorder and Leaf Nodes Array
- Display Leaf nodes from Preorder of a BST in Java
- Unicodes for Operators in Java
- XOR and XNOR operators in Java
- AWS Lambda in Java
- AWS Polly in Java
- SAML in Java
- SonarQube in Java
- UniRest in Java
- Override equals method in Java
- Undo and Redo Operations in Java
- Size of longest Divisible Subset in an Array in Java
- Sort An Array According To The Set Bits Count in Java
- Two constructors in one class in Java
- Union in Java
- What is New in Java 15
- ART in Java
- Definite Assignment in Java
- Cast Operator in Java
- Diamond operator in Java
- Java Singleton Enum
- Three-way operator | Ternary operator in Java
- GoF Design Pattern Java
- Shorthand Operator in Java
- What is new in Java 17
- How to Find the Java Version in Linux
- What is New in Java 12
- Exception in Thread Main java.util.NoSuchElementException no line Found
- How to reverse a string using recursion in Java
- Java Program to Reverse a String Using Stack
- Java Program to Reverse a String Using the Stack Data Structure
- Reverse Middle Words of a String in Java
- Sastry Numbers in Java
- Sum of LCM in Java
- Tilde Operator in Java
- 8 Puzzle problems in Java
- Maximum Sum Such That No Two Elements Are Adjacent in Java
- Reverse a String in Place in Java
- Reverse a string Using a Byte array in Java
- Reverse a String Using Java Collections
- Reverse String with Special Characters in Java
- get timestamp in java
- How to convert file to hex in java
- AbstractSet in java
- List vs Set vs Map in Java
- Birthday Problem in Java
- How to Calculate the Time Difference Between Two Dates in Java
- Number of Mismatching Bits in Java
- Palindrome Permutation of a String in Java
- Grepcode java.util.Date
- How to add 24 hrs to date in Java
- How to Change the Day in The Date Using Java
- Java ByteBuffer Size
- java.lang.NoSuchMethodError
- Maximum XOR Value in Java
- How to Add Hours to The Date Object in Java
- How to Increment and Decrement Date Using Java
- Multithreading Scenarios in Java
- Switch case with enum in Java
- Longest Harmonious Subsequence in Java
- Count OR Pairs in Java
- Merge Two Sorted Arrays Without Extra Space in Java
- How to call a concrete method of abstract class in Java
- How to create an instance of abstract class in Java
- Java Console Error
- 503 error handling retry code snippets Java
- Implementation Of Abstraction In Java
- How to avoid thread deadlock in Java
- Number of Squareful Arrays in Java
- One-Time Password Generator Code In Java
- Real-Time Face Recognition In Java
- Converting Integer Data Type to Byte Data Type Using Typecasting in Java
- How to Generate File checksum Value
- Index Mapping (or Trivial Hashing) With Negatives allowed in Java
- Shortest Path in a Binary Maze in Java
- customized exception in Java
- Difference between error and exception in Java
- How to solve deprecated error in Java
- Jagged Array in Java
- CloneNotSupportedException in Java with Examples
- Difference Between Function and Method in Java
- Immutable List in Java
- Nesting Of Methods in Java
- How to Convert Date into Character Month and Year Java
- How to Mock Lambda Expression in Java
- How to Return Value from Lambda Expression Java
- if Condition in Lambda Expression Java
- Chained Exceptions in Java
- Final static variable in Java
- Java File Watcher
- Various Operations on HashSet in Java
- Word Ladder Problem in Java
- Various Operations on Queue in Java
- Various Operations on Queue Using Linked List in Java
- Various Operations on Queue Using Stack in Java
- Get Yesterday's Date from Localdate Java
- Get Yesterday's Date by No of Days in Java
- Advantages of Lambda Expression in Java 8
- Cast Generic Type to Specific type Java
- ConcurrentSkipListSet in Java
- Fail Fast Vs. Fail-Safe in Java
- Get Yesterday's Date in Milliseconds Java
- Get Yesterday's Date Using Date Class Java
- Getting First Date of Month in Java
- Gregorian Calendar Java Current Date
- How to Calculate Time Difference Between Two Dates in Java
- How to Calculate Week Number from Current Date in Java
- Keystore vs Truststore
- Leap Year Program in Java
- Online Java Compiler GDB
- Operators in Java MCQ
- Separators In Java
- StringIndexOutOfBoundsException in Java
- Anonymous Function in Java
- Default Parameter in Java
- Group by Date Code in Java
- How to add 6 months to Current Date in Java
- How to Reverse A String in Java Letter by Letter
- Java 8 Object Null Check
- Java Synchronized
- Types of Arithmetic Operators in Java
- Types of JDBC Drivers in Java
- Unmarshalling in Java
- Write a Program to Print Reverse of a Vowels String in Java
- ClassNotFound Exception in Java
- Null Pointer Exception in Java
- Why Does BufferedReader Throw IOException in Java
- Java Program to Add two Complex Numbers
- Read and Print All Files From a Zip File in Java
- Reverse an Array in Java
- Right Shift Zero Fill Operator in Java
- Static Block in Java
- Accessor and Mutator in Java
- Array of Class Objects in Java
- Benefits of Generics in Java
- Can Abstract Classes Have Static Methods in Java
- ClassNotFoundException Java
- Creating a Custom Generic Class in Java
- Generic Queue Java
- Getting Total Hours From 2 Dates in Java
- How to add 2 dates in Java
- How to Break a Date and Time in Java
- How to Call Generic Method in Java
- How to Increment and Decrement Date using Java
- Java Class Methods List
- Java Full Stack Developer
- Java.lang.NullPointerException
- Least Operator to Express Number in Java
- Shunting Yard Algorithm in Java
- Switch Case Java
- Treeset Java Operations
- Types of Logical Operators in Java
- What is Cast Operator in Java
- What is Jersey in Java
- Alternative to Java Serialization
- API Development in Java
- Disadvantage of Multithreading in Java
- Find the row with the maximum number of 1s
- Generic Comparator in Java
- Generic LinkedList in Java
- Generic Programming in Java Example
- How Can I Give the Default Date in The Array Java
- How to Accept Date in Java
- How to add 4 years to Date in Java
- How to Check Date Equality in Java
- How to Modify HTML File Using Java
- Java 8 Multithreading Features
- Java Abstract Class and Methods
- Java Thread Dump Analyser
- Process vs. Thread in Java
- Reverse String Using Array in Java
- Types of Assignment Operators in Java
- Types of Bitwise Operators in Java
- Union and Intersection Of Two Sorted Arrays In Java
- Vector Operations Java
- Java Books Multithreading
- Advantages of Generics in Java
- Arrow Operator Java
- Generic Code in Java
- Generic Method in Java Example
- Getting a Range of Dates in Java
- Getting the Day from a Date in Java
- How Counter Work with Date Using Java
- How to Add Date in Arraylist Java
- How to Create a Generic List in Java
- Java Extend Multiple Classes
- Java Function
- Java Generics Design Patterns
- Why Are Generics Used in Java
- XOR Binary Operator in Java
- Check if the given string contains all the digits in Java
- Constructor in Abstract Class in Java
- Count number of a class objects created in Java
- Difference Between Byte Code and Machine Code in Java
- Java Program to Append a String in an Existing File
- Main thread in Java
- Store Two Numbers in One Byte Using Bit Manipulation in Java
- The Knight's Tour Problem in Java
- Business Board Problem in Java
- Business Consumer Problem in Java
- Buy as Much Candles as Possible Java Problem
- Get Year from Date in Java
- How to Assign Static Value to Date in Java
- Java List Node
- Java List Sort Lambda
- Java Program to Get the Size of a Directory
- Misc Operators in Java
- Reverse A String and Reverse Every Alternative String in Java
- Reverse a String in Java Using StringBuilder
- Reverse Alternate Words in A String Java
- Size of Empty Class in Java
- Titniry Operation in Java
- Triple Shift Operator in Java
- Types of Conditional Operators in Java
- View Operation in Java
- What is Linked list Operation in Java
- What is Short Circuit && And or Operator in Java
- What is the & Operator in Java
- Why to use enum in Java
- XOR Bitwise Operator in Java
- XOR Logical Operator Java
- Compile-Time Polymorphism in Java
- Convert JSON to Java Object Online
- Difference between comparing String using == and .equals() method in Java
- Difference Between Singleton Pattern and Static Class in Java
- Difference Between Static and Non-Static Nested Class in Java
- Getting Date from Calendar in Java
- How to Swap or Exchange Objects in Java
- Java Get Class of Generic Parameter
- Java Interface Generic Parameter
- Java Map Generic
- Java Program for Maximum Product Subarray
- Java Program To Print Even Length Words in a String
- Logger Class in Java
- Manacher's Algorithm in Java
- Mutable Class in Java
- Online Java IDE
- Package getImplementationVersion() method in Java with Examples
- Set Default Close Operation in Java
- Sorting a Java Vector in Descending Order Using Comparator
- Types of Interfaces in Java
- Understanding String Comparison Operator in Java
- User-Defined Packages in Java
- Valid variants of main() in Java
- What is a Reference Variable in Java
- What is an Instance in Java
- What is Retrieval Operation in ArrayList Java
- When to Use the Static Method in Java
- XOR Operations in Java
- 7th Sep - Array Declaration in Java
- 7th Sep - Bad Operand Types Error in Java
- 7th Sep - Data Structures in Java
- 7th Sep - Generic Type Casting In Java
- 7th Sep - Multiple Inheritance in Java
- 7th Sep - Nested Initialization for Singleton Class in Java
- 7th Sep - Object in Java
- 7th Sep - Recursive Constructor Invocation in Java
- 7th Sep - Java Language / What is Java
- 7th Sep - Why is Java Platform Independent
- 7th Sep - Card Flipping Game in Java
- 7th Sep - Create Generic Method in Java
- 7th Sep - Difference between super and super() in Java with Examples
- 7th Sep - for loop enum Java
- 7th Sep - How to Convert a String to Enum in Java
- 7th Sep - Illustrate Class Loading and Static Blocks in Java Inheritance
- 7th Sep - Introduction To Java
- 7th Sep - Java Lambda foreach
- 7th Sep - Java Latest Version
- 7th Sep - Java Method Signature
- 7th Sep - Java Practice Programs
- 7th Sep - Java SwingWorker Class
- 7th Sep - java.util.concurrent.RecursiveAction class in Java With Examples
- 7th Sep - Largest Palindrome by Changing at Most K-digits in Java
- 7th Sep - Parameter Passing Techniques in Java with Examples
- 7th Sep - Reverse a String in Java Using a While Loop
- 7th Sep - Reverse a String Using a For Loop in Java
- 7th Sep - Short Circuit Operator in Java
- 7th Sep - Java 8 Stream API
- 7th Sep - XOR Operation on Integers in Java
- 7th Sep - XOR Operation on Long in Java
- Array Programs in Java
- Concrete Class in Java
- Difference between Character Stream and Byte Stream in Java
- Difference Between Static and non-static in Java
- Different Ways to Convert java.util.Date to java.time.LocalDate in Java
- Find the Good Matrix Problem in Java
- How Streams Work in Java
- How to Accept Different Formats of Date in Java
- How to Add Date in MySQL from Java
- How to Find the Size of int in Java
- How to Make a Field Serializable in Java
- How to Pass an Array to Function in Java
- How to Pass an ArrayList to a Method in Java
- Implementing the Java Queue Interface
- Initialization of local variable in a conditional block in Java
- isnull() Method in Java
- Java Array Generic
- Java Program to Demonstrate the Lazy Initialization Non-Thread-Safe
- Java Program to Demonstrate the Non-Lazy Initialization Thread-Safe
- Java Static Field Initialization
- Machine Learning Using Java
- Mars Rover Problem in Java
- Model Class in Java
- Nested Exception Handling in Java
- Program to Convert List to Stream in Java
- Static Polymorphism in Java
- Static Reference Variables in Java
- Sum of Two Arrays in Java
- What is Is-A-Relationship in Java
- When to Use Vector in Java
- Which Class cannot be subclassed in Java
- Word Search Problem in Java
- XOR Operation Between Sets in Java
- Burger Problem in Java Game
- Convert Set to List in Java
- Floyd Triangle in Java
- How to Call Static Blocks in Java
- Interface Attributes in Java
- Java Applications in the Real World
- Java Concurrent Array
- Java Detect Date Format
- Java Interface Without Methods
- Java Iterator Performance
- Java Packet
- Java Static Instance of Class
- Java TreeMap Sort by Value
- Length of List in Java
- List of Checked Exceptions in Java
- Message Passing in Java
- Product Maximization Problem in Java
- Terminal Operations in Java 8
- Understanding Base Class in Java
- Difference between Early Binding and Late Binding in Java
- Collectors toCollection() in Java
- Difference between ExecutorService execute() and submit() method in Java
- Difference between Java and Core Java
- Different Types of Recursions in Java
- Initialize a static map in Java with Examples
- Merge Sort Using Multithreading in Java
- Why Thread.stop(), Thread.suspend(), and Thread.resume() Methods are Deprecated After JDK 1.1 Version
- Circular Primes in Java
- Difference Between poll() and remove() Method of a Queue
- EvalEx Java: Expression Evaluation in Java
- Exeter Caption Contest Java Program
- FileInputStream finalize() Method in Java
- Find the Losers of the Circular Game problem in Java
- Finding the Differences Between Two Lists in Java
- Finding the Maximum Points on a Line in Java
- Get Local IP Address in Java
- Handling "Handler dispatch failed" Nested Exception: java.lang.StackOverflowError in Java
- Harmonic Number in Java
- How to Find the Percentage of Uppercase Letters, Lowercase Letters, Digits, and Special Characters in a String Using Java
- Interface Variables in Java
- Java 8 Interface Features
- Java Class Notation
- Java Exception Messages Examples and Explanations
- Java Package Annotation
- Java Program to Find First Non-Repeating Character in String
- Java Static Type Vs. Dynamic Type
- Kaprekar Number in Java
- Multitasking in Java
- Niven Number in Java
- Rhombus Pattern in Java
- Shuffle an Array in Java
- Static Object in Java
- The Scope of Variables in Java
- Toggle String in Java
- Use of Singleton Class in Java
- What is the Difference Between Future and Callable Interfaces in Java
- Aggregate Operation in Java 8
- Bounded Types in Java
- Calculating Batting Average in Java
- Compare Two LinkedList in Java
- Comparison of Autoboxed Integer objects in Java
- Count Tokens in Java
- Cyclomatic Complexity in Java
- Deprecated Meaning in Java
- Double Brace Initialization in Java
- Functional Interface in Java
- How to prevent objects of a class from Garbage Collection in Java
- Java Cast Object to Class
- Java isAlive() Method
- Java Line Feed Character
- java.net.MulticastSocket class in Java
- Keytool Error java.io.FileNotFoundException
- Matrix Diagonal Sum in Java
- Number of Boomerangs Problem in Java
- Sieve of Eratosthenes Algorithm in Java
- Similarities Between Bastar and Java
- Spring vs. Struts in Java
- Switch Case in Java 12
- The Pig Game in Java
- Unreachable Code Error in Java
- Who Were the Kalangs of Java
- 2048 Game in Java
- Abundant Number in Java
- Advantages of Applet in Java
- Alpha-Beta Pruning Java
- ArgoUML Reverse Engineering Java
- Can Constructor be Static in Java
- Can we create object of interface in Java
- Chatbot Application in Java
- Difference Between Component and Container in Java
- Difference Between Java.sql and Javax.sql
- Find A Pair with Maximum Product in Array of Integers
- Goal Stack Planning Program in Java
- Half Diamond Pattern in Java
- How to find trigonometric values of an angle in Java
- How to Override tostring() method in Java
- Inserting a Node in a Doubly Linked List in Java
- Java 9 Immutable Collections
- Java 9 Interface Private Methods
- Java Convert Array to Collection
- Java Transaction API
- Methods to Take Input in Java
- Parallelogram Pattern in Java
- Reminder Program in Java
- Sliding Window Protocol in Java
- Static Method in Java
- String Reverse in Java 8 Using Lambdas
- Types of Threads in Java
- What is thread safety in Java? How do you achieve it?
- xxwxx.dll Virus Java 9
- Java 8 Merge Two Maps with Same Keys
- Java 8 StringJoiner, String.join(), and Collectors.joining()
- Java 9 @SafeVarargs Annotation Changes
- Java 9 Stream API Improvements
- Java 11 var in Lambda Expressions
- Sequential Search Java
- Thread Group in Java
- User Thread Vs. Daemon Thread in Java
- Collections Vs. Streams in Java
- Import statement in Java
- init() Method in Java
- Java Generics Jenkov
- Ambiguity in Java
- Benefits of Learning Java
- Designing a Vending Machine in Java
- Monolithic Applications in Java
- Name Two Types of Java Program
- Random Access Interface in Java
- Rust Vs. Java
- Types of Constants in Java
- Execute the Main Method Multiple Times in Java
- Find the element at specified index in a Spiral Matrix in Java
- Find The Index of An Array Element in Java
- Mark-and-Sweep Garbage Collection Algorithm in Java
- Shadowing of Static Functions in Java
- Straight Line Numbers in Java
- Zumkeller Numbers in Java
- Types of Layout Manager in Java
- Virtual Threads in Java 21
- Add Two Numbers Without Using Operator in Java
- Automatic Type Promotion in Java
- ContentPane Java
- Difference Between findElement() and findElements() in Java
- Difference Between Inheritance and Interfaces in Java
- Difference Between Jdeps and Jdeprscan tools in Java
- Find Length of String in Java Without Using Function
- InvocationTargetException in Java
- Java Maps to JSON
- Key Encapsulation Mechanism API in Java 21
- Placeholder Java
- String Templates in Java 21
- Why Java is Robust Language
- Collecting in Java 8
- containsIgnoreCase() Method in Java
- Convert String to Biginteger In Java
- Convert String to Map in Java
- Define Macro in Java
- Difference Between Lock and Monitor in Java Concurrency
- Difference Between the start() and run() Methods in Java
- Generalization and Specialization in Java
- getChannel() Method in Java
- How to Check Whether an Integer Exists in a Range with Java
- HttpEntity in Java
- Lock Framework Vs. Thread Synchronization in Java
- Niven Number Program in Java
- Passing Object to Method in Java
- Pattern Matching for Switch in Java 21
- Swap First and Last Digit of a Number in Java
- Adapter Design Pattern in Java
- Best Automation Frameworks for Java
- Building a Search Engine in Java
- Bytecode Verifier in Java
- Caching Mechanism in Java
- Comparing Two HashMap in Java
- Cryptosystem Project in Java
- Farthest from Zero Program in Java
- How to Clear Linked List in Java
- Primitive Data Type Vs. Object Data Type in Java
- setBounds() Method in Java
- Unreachable Code or Statement in Java
- What is Architecture Neutral in Java
- Difference between wait and notify in Java
- Dyck Path in Java
- Find the last two digits of the Factorial of a given Number in Java
- How to Get an Environment Variable in Java
- Java Program to open the command prompt and insert commands
- JVM Shutdown Hook in Java
- Semiprimes Numbers in Java
- 12 Tips to Improve Java Code Performance
- Ad-hoc Polymorphism in Java
- Array to String Conversion in Java
- CloudWatch API in Java
- Essentials of Java Programming Language
- Extends Vs. Implements in Java
- 2d Array Sorting in Java
- Aliquot Sequence in Java
- Authentication and Authorization in Java
- Cannot Find Symbol Error in Java
- Compare Two Excel Files in Java
- Consecutive Prime Sum Program in Java
- Count distinct XOR values among pairs using numbers in range 1 to N
- Difference Between Two Tier and Three Tier Architecture in Java
- Different Ways of Reading a Text File in Java
- Empty Array in Java
- FCFS Program in Java with Arrival Time
- Immutable Map in Java
- K-4 City Program in Java
- Kahn's algorithm for Topological Sorting in Java
- Most Popular Java Backend Tools
- Recursive Binary Search in Java
- Set Intersection in Java
- String Reverse Preserving White Spaces in Java
- The Deprecated Annotation in Java
- What is JNDI in Java
- Backtracking in Java
- Comparing Doubles in Java
- Consecutive Prime Sum in Java
- Finding Missing Numbers in an Array Using Java
- Good Number Program in Java
- How to Compress Image in Java Source Code
- How to Download a File from a URL in Java
- Passing an Object to The Method in Java
- Permutation program in Java
- Profile Annotation in Java
- Scenario Based Questions in Java
- Understanding Static Synchronization in Java
- Types of Errors in Java
- Abstract Factory Design Pattern in Java
- Advantages of Kotlin Over Java
- Advantages of Methods in Java
- Applet Program in Java to Draw House with Output
- Atomic Boolean in Java
- Bitset Class in Java
- Bouncy Castle Java
- Chained Exception in Java
- Colossal Numbers in Java
- Compact Profiles Java 8
- Convert Byte to Image in Java
- Convert Set to Array in Java
- Copy ArrayList to another ArrayList Java
- Copy Data from One File to Another in Java
- Dead Code in Java
- Driver Class Java
- EnumMap in Java
- Farthest Distance of a 0 From the Centre of a 2-D Matrix in Java
- How to Terminate a Program in Java
- Instance Block in Java
- Iterative Constructs in Java
- Java 10 var Keyword
- Nested ArrayList in Java
- Square Pattern in Java
- String Interpolation in Java
- Unnamed Classes and Instance Main Method in Java 21
- What is difference between cacerts and Keystore in Java
- Agile Principles Patterns and Practices in Java
- Color Method in Java
- Concurrent Collections in Java
- Create JSON Node in Java
- Difference Between Checkbox and Radio Button in Java
- Difference Between Jdeps and Jdeprscan Tools in Java
- Difference Between Static and Dynamic Dispatch in Java
- Difference Between Static and Non-Static Members in Java
- Error Java Invalid Target Release 9
- Filedialog Java
- String Permutation in Java
- Structured Concurrency in Java
- Uncaught Exception in Java
- ValueOf() Method in Java
- Virtual Thread in Java
- Difference Between Constructor Overloading and Method Overloading in Java
- Difference Between for loop and for-each Loop in Java
- Difference Between Fork/Join Framework and ExecutorService in Java
- Difference Between Local, Instance, and Static Variables in Java
- Difference Between Multithreading and Multiprocessing in Java
- Difference Between Serialization and Deserialization in Java
- Difference Between Socket and Server Socket in Java
- Advantages of Immutable Classes in Java
- BMI Calculator Java
- Code Coverage Tools in Java
- How to Declare an Empty Array in Java
- How To Resolve Java.lang.ExceptionInInitializerError in Java
- Java 18 Snippet Tag with Example
- Object Life Cycle in Java
- print() Vs. println() in Java
- @SuppressWarnings Annotation in Java
- Types of Cloning in Java
- What is portable in Java
- What is the use of an interpreter in Java
- Abstract Syntax Tree (AST) in Java
- Aliasing in Java
- CRUD Operations in Java
- Euclid-Mullin Sequence in Java
- Frame Class in Java
- Initializing a List in Java
- Number Guessing Game in Java
- Number of digits in N factorial to the power N in Java
- Rencontres Number in Java
- Skewed Binary Tree in Java
- Vertical zig-zag traversal of a tree in Java
- Wap to Reverse a String in Java using Lambda Expression
- Concept of Stream in Java
- Constraints in Java
- Context Switching in Java
- Dart Vs. Java
- Dependency Inversion Principle in Java
- Difference Between Containers and Components in Java
- Difference Between CyclicBarrier and CountDownLatch in Java
- Difference Between Shallow and Deep Cloning in Java
- Dots and Boxes Game Java Source code
- DRY Principle Java
- How to get File type in Java
- IllegalArgumentException in Java example
- Is the main() method compulsory in Java
- Java Paradigm
- Lower Bound in Java
- Method Binding in Java
- Overflow and Underflow in Java
- Padding in Java
- Passing and Returning Objects in Java
- Single Responsibility Principle in Java
- ClosedChannelException in Java with Examples
- How to Fix java.net.ConnectException Connection refused connect in Java
- java.io.UnsupportedEncodingException in java with Examples
- Selection Statement in Java
- Difference Between Java 8 and Java 9
- Difference Between Nested Class and Inner Class in Java
- Difference Between OOP and POP in Java
- Difference Between Static and Dynamic in Java
- Difference Between Static Binding and Dynamic Binding in Java
- Difference Between Variable and Constant in Java
- Alternate Pattern Program in Java
- Architecture Neutral in Java
- AutoCloseable Interface in Java
- BitSet Class in Java
- Border Layout Manager in Java
- Digit Extraction in Java
- Dynamic Method Dispatch Java
- Dynamic Variable in Java
- How to Convert Double to string in Java
- How to Convert Meter to Kilometre in Java
- How to Install SSL Certificate in Java
- How to Protect Java Source Code
- How to Use Random Object in Java
- Java Backward Compatibility
- Java New String Class Methods from Java 8 to Java 17
- Mono in Java
- Object to int in Java
- Predefined Streams in Java
- Prime Factor Program in Java
- Transfer Statements in Java
- What is Interceptor in Java
- Java Array Methods
- java.lang.Class class in Java
- Reverse Level Order Traversal in Java
- Working with JAR and Manifest files In Java
- Alphabet Board Path Problem in Java
- Composite Design Pattern Java
- Default and Static Methods in Interface Java 8
- Difference Between Constraints and Annotations in Java
- Difference Between fromJson() and toJson() Methods of GSON in Java
- Difference Between Java 8 and Java 11
- Difference Between map() and flatmap() Method in Java 8
- Difference Between next() and nextLine() Methods in Java
- Difference Between orTimeout() and completeOnTimeOut() Methods in Java 9
- Disadvantages of Array in Java
- How Synchronized works in Java
- How to Create a Table in Java
- ID Card Generator Using Java
- Introspection in JavaBeans
- Java 15 Features
- Java Object Model
- Java Tools and Command-List
- Next Permutation Java
- Object as Parameter in Java
- Optimizing Java Code Performance
- Pervasive Shallowness in Java
- Sequenced Collections in Java 21
- Stdin and Stdout in Java
- Stream count() Function in Java
- String.strip() Method in Java
- Vertical Flip Matrix Problem in Java
- Calling Object in Java
- Characteristics of Constructor in Java
- Counting Problem in Multithreading in Java
- Creating Multiple Pools of Objects of Variable Size in Java
- Default Exception in Java
- How to Install Multiple JDK's in Windows
- Differences Between Vectors and Arrays in Java
- Duplicate Class Errors in Java
- Example of Data Hiding in Java
- Foreign Function and Memory APIs in Java 21
- Generic Tree Implementation in Java
- getSource() Method in Java
- Giuga numbers in Java
- Hessian Java
- How to Connect Login Page to Database in Java
- Difference between BlueJ and JDK 1.3
- How to Solve Incompatible Types Error in Java
- Java 8 Method References
- Java 9 Try with Resources Improvements
- Menu-Driven Program in Java
- Mono Class in Java
- Multithreading Vs. Asynchronous in Java
- Nested HashMap in Java
- Number Series Program in Java
- Object Slicing in Java
- Oracle Java
- Print 1 to 100 Without Loop in Java
- Remove elements from a List that satisfy given predicate in Java
- Replace Element in Arraylist Java
- Sliding Puzzle Game in Java
- Strobogrammatic Number in Java
- Web Methods in Java
- Web Scraping Java
- Window Event in Java
- @Builder Annotation in Java
- Advantages of Abstraction in Java
- Advantages of Packages in Java
- Bounce Tales Java Game Download
- Breaking Singleton Class Pattern in Java
- Building a Brick Breaker Game in Java
- Building a Scientific Calculator in Java
- Circle Program in Java
- Class Memory in Java
- Convert Byte to an Image in Java
- Count Paths in Given Matrix in Java
- Difference Between Iterator and ListIterator in Java with Example
- Distinct Character Count Java Stream
- EOFException in Java
- ExecutionException Java 8
- Generic Object in Java
- How to Create an Unmodifiable List in Java
- How to Create Dynamic SQL Query in Java
- How to Return a 2D Array in Java
- Java 8 Stream.distinct() Method
- Java setPriority() Method
- Mutator Methods in Java
- Predicate Consumer Supplier Java 8
- Program to Generate CAPTCHA and Verify User Using Java
- Random Flip Matrix in Java
- System Class in Java
- Vigenere Cipher Program in Java
- Behavior-Driven Development (BDD) in Java
- CI/ CD Tools for Java
- cint in Java
- Command Pattern in Java
- CSV to List Java
- Difference Between Java Servlets and CGI
- Difference Between Multithreading Multitasking, and Multiprocessing in Java
- Encoding Three Strings in Java
- How to Import Jar File in Eclipse
- Meta Class Vs. Class in Java
- Meta Class Vs. Super Class in Java
- Print Odd and Even Numbers by Two Threads in Java
- Scoped value in Java
- Upper-Bounded Wildcards in Java
- Wildcards in Java
- Zero Matrix Problem in Java
- All Possible Combinations of a String in Java
- Atomic Reference in Java
- Final Method Overloading in Java| Can We Overload Final Methods
- Constructor in Inheritance in Java
- Design Your Custom Connection Pool in Java
- How Microservices Communicate with Each Other in Java
- How to Convert String to Timestamp in Java
- Java 10 Collectors Methods
- Java and Apache OpenNLP
- Java Deep Learning
- Java Iterator Vs. Listiterator Vs. Spliterator
- Pure Functions in Java
- Use of Constructor in Java | Purpose of Constructor in Java
- Implement Quintet Class with Quartet Class in Java using JavaTuples
- Java Best Practices
- Efficiently Reading Input For Competitive Programming using Java 8
- Length of the longest substring without repeating characters in Java
- Advantages of Inner Class in Java
- AES GCM Encryption Java
- Array Default Values in Java
- Copy File in Java from one Location to Another
- Creating Templates in Java
- Different Packages in Java
- How to Add Elements to an Arraylist in Java Dynamically
- How to Add Splash Screen in Java
- How to Calculate Average Star Rating in Java
- Immutable Class with Mutable Object in Java
- Java instanceOf() Generics
- Set Precision in Java
- Snake Game in Java
- Tower of Hanoi Program in Java
- Two Types of Streams Offered by Java
- Uses of Collections in Java
- Additive Numbers in Java
- Association Vs. Aggregation Vs. Composition in Java
- Covariant and Contravariant Java
- Creating Immutable Custom Classes in Java
- mapToInt() in Java
- Methods of Gson in Java
- Server Socket in Java
- Check String Are Permutation of Each Other in Java
- Containerization in Java
- Difference Between Multithreading and Multiprogramming in Java
- Flyweight Design Pattern
- HMAC Encryption in Java
- How to Clear Error in Java Program
- 5 Types of Java
- Design a Job Scheduler in Java
- Elements of Java Programming
- Generational ZCG in Java 21
- How to Print Arraylist Without Brackets Java
- Interface Vs. Abstract Class After Java 8
- Java 9 Optional Class Improvements
- Number of GP sequence Problem in Java
- Pattern Matching for Switch
- Range Addition Problem in Java
- Swap Corner Words and Reverse Middle Characters in Java
- Kadane's Algorithm in Java
- Capture the Pawns Problem in Java
- Find Pair With Smallest Difference in Java
- How to pad a String in Java
- When to use Serialization and Externalizable Interface
- Which Component is responsible to run Java Program
- Difference Between Java and Bastar
- Difference Between Static and Instance Methods in Java
- Difference Between While and Do While loop in Java
- Future Interface in Java
- Invert a Binary tree in Java
- Java Template Engine
- KeyValue Class in JavaTuples
- Quantifiers in Java
- Swapping Pairs of Characters in a String in Java
- Version Enhancements in Exception Handling introduced in Java SE 7
- Find all Palindromic Sub-Strings of a given String in Java
- Find if String is K-Palindrome or not in Java
- Count Pairs from an Array with Even Product of Count of Distinct Prime Factor in Java
- Find if an Array of Strings can be Chained to form a Circle in Java
- Find largest factor of N such that NF is less than K in Java
- Lexicographically First Palindromic String in Java
- LinkedTransferQueue removeAll() method in Java with Examples
- Next Smallest Palindrome problem in Java
- Why Java is not a Purely Object-Oriented Language
Java Date Class
- java.util.Date
- toInstant()
- getMinutes()
- setMinutes()
- getSeconds()
- getTimezoneOffset()
- toGMTString()
- toLocaleString()
Java ListIterator Class
- Java ListIterator
- hasPrevious()
- nextIndex()
- previousIndex()
Java NIO Tutorial
- NIO Components
- NIO Package
- NIO Channels
- NIO Buffers
- NIO Scatter/Gather
- NIO Data Transfer
- NIO Selector
- NIO SocketChannel
- NIO ServerSocketChannel
- NIO CharSet
- NIO Encode/Decode
- NIO Channels FileLock
- Java HttpURLConnection
- disconnect()
- getErrorStream()
- getFollowRedirects()
- getHeaderField()
- getResponseCode()
- getResponseMessage()
- setAuthenticator()
- setFollowRedirects()
- removeFirst()
- removeLast()
- removeFirstOccurrence()
- removeLastOccurrence()
Java HttpCookie Class
- Java HttpCookie
- domainMatches()
- getComment()
- getCommentURL()
- getDiscard()
- getDomain()
- getMaxAge()
- getPortList()
- getSecure()
- setComment()
- setCommentURL()
- setDiscard()
- setDomain()
- setHttpOnly()
- setMaxAge()
Java URL class
- class getDefaultPort()
- getAuthority()
- getContent()
- getProtocol()
- getUserInfo()
- openConnection()
- toExternalfile()
Java List Methods
- containsAll()
Java Vector class
- addElement()
- ensureCapacity()
- firstElement()
- elementAt()
- insertElementAt()
- lastElement()
- removeAllElements()
- removeAll()
- removeElementAt()
- removeElement()
- retainAll()
- removeRange()
- setElementAt()
- trimToSize()
Java Collection Methods
Java socket class.
- Socket Class
- get00BInline()
- getChannel()
- getInetAddress()
- getKeepAlive()
- getLocalPort()
- getLocalSocketAddress()
- getReceiveBufferSize()
- getRemoteSocketAddress()
- getReuseAddress()
- getSendBufferSize()
- getSoLinger()
- getSoTimeout()
- getTcpNoDelay()
- getTrafficClass()
- isConnected()
- isInputShutdown()
- isOutputShutdown()
- sendUrgentData()
- setKeepAlive()
- setOOBInline()
- setTrafficClass()
- shutdownInput()
- shutdownOutput()
- setSoLinger()
- setSoTimeout()
- setTcpNoDelay()
- getInputStream()
- getOutputStream()
- setReceiveBufferSize()
- setReuseAddress()
- setSendBufferSize()
- Java Executors
- defaultThreadFactory()
- newCachedThreadPool()
- newFixedThreadPool()
- newScheduledThreadPool()
- newSingleThreadExecutor()
- newWorkStealingPool()
- privilegedThreadFactory()
Java ConcurrentHashMap
Java concurrentlinkedqueue, latest courses.

We provides tutorials and interview questions of all technology like java tutorial, android, java frameworks
Contact info
G-13, 2nd Floor, Sec-3, Noida, UP, 201301, India
[email protected] .

Latest Post
PRIVACY POLICY
Interview Questions
Online compiler.
Filter a List by Any Matching Field
Last updated: October 18, 2024
- Java Streams
- Java Search

Retrieval-Augmented Generation (RAG) is a powerful approach in Artificial Intelligence that's very useful in a variety of tasks like Q&A systems, customer support, market research, personalized recommendations, and more.
A key component of RAG applications is the vector database , which helps manage and retrieve data based on semantic meaning and context.
Learn how to build a gen AI RAG application with Spring AI and the MongoDB vector database through a practical example:
>> Building a RAG App Using MongoDB and Spring AI
Mocking is an essential part of unit testing, and the Mockito library makes it easy to write clean and intuitive unit tests for your Java code.
Get started with mocking and improve your application tests using our Mockito guide :
Download the eBook
Baeldung Pro comes with both absolutely No-Ads as well as finally with Dark Mode , for a clean learning experience:
>> Explore a clean Baeldung
Once the early-adopter seats are all used, the price will go up and stay at $33/year.
Azure Container Apps is a fully managed serverless container service that enables you to build and deploy modern, cloud-native Java applications and microservices at scale. It offers a simplified developer experience while providing the flexibility and portability of containers.
Of course, Azure Container Apps has really solid support for our ecosystem, from a number of build options, managed Java components, native metrics, dynamic logger, and quite a bit more.
To learn more about Java features on Azure Container Apps, visit the documentation page .
You can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Modern software architecture is often broken. Slow delivery leads to missed opportunities, innovation is stalled due to architectural complexities, and engineering resources are exceedingly expensive.
Orkes is the leading workflow orchestration platform built to enable teams to transform the way they develop, connect, and deploy applications, microservices, AI agents, and more.
With Orkes Conductor managed through Orkes Cloud, developers can focus on building mission critical applications without worrying about infrastructure maintenance to meet goals and, simply put, taking new products live faster and reducing total cost of ownership.
Try a 14-Day Free Trial of Orkes Conductor today.
To learn more about Java features on Azure Container Apps, you can get started over on the documentation page .
And, you can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Whether you're just starting out or have years of experience, Spring Boot is obviously a great choice for building a web application.
Jmix builds on this highly powerful and mature Boot stack, allowing devs to build and deliver full-stack web applications without having to code the frontend. Quite flexibly as well, from simple web GUI CRUD applications to complex enterprise solutions.
Concretely, The Jmix Platform includes a framework built on top of Spring Boot, JPA, and Vaadin , and comes with Jmix Studio, an IntelliJ IDEA plugin equipped with a suite of developer productivity tools.
The platform comes with interconnected out-of-the-box add-ons for report generation, BPM, maps, instant web app generation from a DB, and quite a bit more:
>> Become an efficient full-stack developer with Jmix
Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push.
Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding.
Write code that works the way you meant it to:
>> CodiumAI. Meaningful Code Tests for Busy Devs
The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI .
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick.
Simplify Your Coding Journey with Machinet AI :
>> Install Machinet AI in your IntelliJ
Handling concurrency in an application can be a tricky process with many potential pitfalls . A solid grasp of the fundamentals will go a long way to help minimize these issues.
Get started with understanding multi-threaded applications with our Java Concurrency guide:
>> Download the eBook
Spring 5 added support for reactive programming with the Spring WebFlux module, which has been improved upon ever since. Get started with the Reactor project basics and reactive programming in Spring Boot:
>> Download the E-book
Let's get started with a Microservice Architecture with Spring Cloud:
Download the Guide
Since its introduction in Java 8, the Stream API has become a staple of Java development. The basic operations like iterating, filtering, mapping sequences of elements are deceptively simple to use.
But these can also be overused and fall into some common pitfalls.
To get a better understanding on how Streams work and how to combine them with other language features, check out our guide to Java Streams:
Download the E-book
Do JSON right with Jackson
Get the most out of the Apache HTTP Client
Get Started with Apache Maven:
Working on getting your persistence layer right with Spring?
Explore the eBook
Building a REST API with Spring?
Get started with Spring and Spring Boot, through the Learn Spring course:
Explore Spring Boot 3 and Spring 6 in-depth through building a full REST API with the framework:
>> The New “REST With Spring Boot”
Get started with Spring and Spring Boot, through the reference Learn Spring course:
>> LEARN SPRING
Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework.
I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project .
You can explore the course here:
>> Learn Spring Security
Spring Data JPA is a great way to handle the complexity of JPA with the powerful simplicity of Spring Boot .
Get started with Spring Data JPA through the guided reference course:
>> CHECK OUT THE COURSE
1. Overview
We often need to filter a List of objects by checking if any of their fields match a given String in many real-world Java applications. In other words, we want to search for a String and filter the objects that match in any of their properties.
In this tutorial, we’ll walk through different approaches to filtering a List by any matching fields in Java.
2. Introduction to the Problem
As usual, let’s understand the problem through examples.
Let’s say we have a Book class with fields like title , tags , intro, and pages :
Next, let’s create four Book instances using the defined constructor and put them into a List<Book> :
Now, we want to perform a filter operation on BOOKS to find all Book objects that contain a keyword String in the title , tags , or intro . In other words, we would like to execute a full-text search on BOOKS .
For example, if we want to search for “Java”, the JAVA and KOTLIN instances should be in the result. This is because JAVA.title contains “Java” and KOTLIN.tags contains “Java”.
Similarly, if we search for “Art “, we expect JAVA and GUITAR to be found since JAVA.title and GUITAR.tags contain the word “Art. ” When “ Let’s “ is the keyword, KOTLIN and GUITAR should be in the result as KOTLIN.title and GUITAR.intro contain the keyword.
Next, let’s explore how to solve this problem and use these three keyword examples to check our solutions.
For simplicity, we assume all Book ‘s properties are not null , and we’ll leverage unit test assertions to verify whether our solutions work as expected.
Next, let’s dive into the code.
3. Using the Stream.filter() Method
Stream API provides the convenient filter() method, which allows us to filter objects in a Stream through a lambda expression easily .
Next, let’s solve the full-text search problem using this approach:
As we can see, the above implementation is pretty straightforward. In the lambda expression that we pass to filter() , we check whether any property contains the keyword.
It’s worth mentioning that as Book.tags is a List<String> , we leverage Stream.anyMatch() to check if any tag in the List contains the keyword.
Next, let’s test whether this approach works correctly:
The test passes if we give it a run.
In this example, we only need to check three properties in the Book class. However, a full-text search might check a class’s dozen properties in an actual application. In this case, the lambda expression would be pretty long and can make the Stream pipeline difficult to read and maintain.
Of course, we can extract the lambda expression as a method to solve it. Alternatively, we can create a function to generate the String representation for full-text search.
Next, let’s take a closer look at this approach.
4. Creating a String Representation For Filtering
We know toString() returns a String representation of an object. Similarly, we can create a method to provide an object’s String representation for full-text search :
As the code above shows, the strForFiltering() function joins all full-text search required String values to a linebreak-separated String. If we take KOTLIN as an example, this method returns the following String :
In this example, we use Java text block to present the multiline String .
Then, a full-text search would be an easy task for us. We just check if book.strForFilter()’s result contains keyword :
Next, let’s check if this solution works as expected:
The test passes. Therefore, this approach does the job.
5. Creating a General Full-Text Search Method
In this section, let’s try to create a general method to perform a full-text search on any object:
The fullTextSearchOnObject() method accepts three parameters: the object we want to perform the search, the keyword, and the excluded field names.
The implementation uses reflection to retrieve all fields of the object . Then, we loop through the fields, skip excludedFields using Stream.nonMatch() , and obtain the field’s value by field.get(obj) . Since we aim to perform a String -based search, we convert the value to a String using toString() and check if the field’s value contains the search term.
Our object may contain nested objects. Therefore, we recursively check the fields of nested objects . If any field is an object (other than a primitive or String ), it calls fullTextSearchOnObject() on that field, enabling us to search through deeply nested structures.
Now, we can make use of fullTextSearchOnObject() to create a method to full-text filter a List of Book objects:
Next, let’s run the same test to verify if this approach works as expected:
As we can see, we passed “ pages ” as the excluded fields in the test above. If it’s required, we can conveniently extend excluded fields for a custom full-text search:
This example showcases how to perform a full-text search only on title and intro fields. As GUITAR only contains the search keyword “ Art ” in tags , it gets filtered out.
Using reflection and recursion, we can implement a full-text search on a Java object that checks all fields, including nested fields, for a given String keyword. This approach allows us to dynamically search through an object’s fields without explicitly knowing the structure of the class.
6. Conclusion
In this article, we’ve explored different solutions to filtering a List by any field matching a String in Java.
These techniques will help us write cleaner, more maintainable code while providing a powerful way to search and filter Java objects.
As always, the complete source code for the examples is available over on GitHub .
Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE:
Basically, write code that works the way you meant it to.
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code.
>>Download the E-book
The Apache HTTP Client is a very robust library, suitable for both simple and advanced use cases when testing HTTP endpoints . Check out our guide covering basic request and response handling, as well as security, cookies, timeouts, and more:
Get started with Spring Boot and with core Spring, through the Learn Spring course:
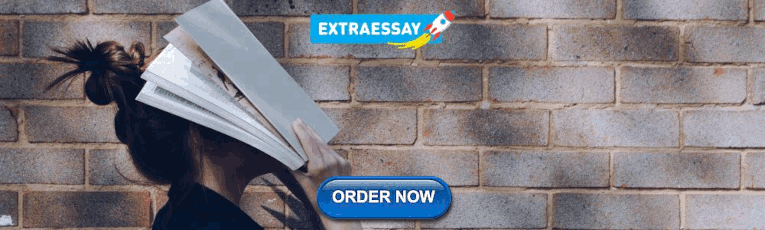
IMAGES
VIDEO
COMMENTS
a = b makes a new assignment to the reference a, not f, of the object whose attribute is "b". ... In general, Java has primitive types (int, bool, char, double, etc) that are passed directly by value. Then Java has objects (everything that derives from java.lang.Object). Objects are actually always handled through a reference (a reference being ...
Because java uses the concept of string literal. Suppose there are 5 reference variables, all refers to one object "0".If one reference variable changes the value of the object, it will be affected to all the reference variables. That is why string objects are immutable in java.
Java always passes parameter variables by value. Object variables in Java always point to the real object in the memory heap. A mutable object's value can be changed when it is passed to a method.
When we assign an integer value to an Integer object, the value is autoboxed into an Integer object. For example the statement "Integer x = 10" creates an object 'x' with value 10. Following are some interesting output questions based on comparison of Autoboxed Integer objects. Predict the output of following Java Program // file name: Main.java pu
Pass-by-Reference. When a parameter is pass-by-reference, the caller and the callee operate on the same object. It means that when a variable is pass-by-reference, the unique identifier of the object is sent to the method. Any changes to the parameter's instance members will result in that change being made to the original value.
In case of call by reference original value is changed if we made changes in the called method. If we pass object in place of any primitive value, original value will be changed. In this example we are passing object as a value. Let's take a simple example: download this example. Output:before change 50.
The first statement invokes rectOne's getArea() method and displays the results. The second line moves rectTwo because the move() method assigns new values to the object's origin.x and origin.y.. As with instance fields, objectReference must be a reference to an object. You can use a variable name, but you also can use any expression that returns an object reference.
This concludes that Java Objects are passed to methods by Reference. Here also, we pass the memory location pointed by one Reference variable to another Reference variable of a Called method as a Value only. So, you are actually passing Values (memory locations represented by integer or long data type). But the actual passed-object can only be ...
Technically, Java is always pass by value, because even though a variable might hold a reference to an object, that object reference is a value that represents the object's location in memory. Object references are therefore passed by value. Both reference data types and primitive data types are passed by value.
Unlike in C++, Java does not have a means of explicitly differentiating between pass by reference and pass by value. Instead, the Java Language Specification (Section 4.3) declares that the ...
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases.
This beginner Java tutorial describes fundamentals of programming in the Java programming language ... it assigns the value on its right to the operand on its left: int cadence = 0; int speed = 0; int gear = 1; This operator can also be used on objects to assign object references, as discussed in Creating Objects. The Arithmetic Operators.
The constructor class provides information about a single constructor for a class and it also provides access to that constructor. The equals() method of java.lang.reflect.Constructor is used to compare this Constructor against the passed object. If the objects are the same then the method returns true. In java Two Constructor objects are the same
About the Parameters Passed in Java. The fundamental concept for passing the parameters in modern programming languages is passing by value and passing by reference. But, in Java, the pass by reference concept is degraded. It supports only the pass by value concept. The primitive variables hold the actual values, whereas the non-primitive ...
Assigning one object to another just assigns the object reference (a pointer more or less). It does NOT copy member variables etc. You need to read about cloning.From the first paragraph of the wiki for Java clone():. In Java, objects are manipulated through reference variables, and there is no operator for copying an object—the assignment operator duplicates the reference, not the object.
Using reflection and recursion, we can implement a full-text search on a Java object that checks all fields, including nested fields, for a given String keyword. This approach allows us to dynamically search through an object's fields without explicitly knowing the structure of the class. 6. Conclusion