Python type hints: Mypy doesn’t allow variables to change type
Subscribe via RSS , Twitter , Mastodon , or email:
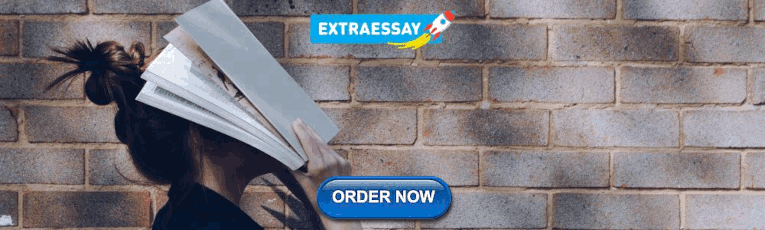
Improve typing, with ! to force not None value
Consider the following example
With mypy the errors out with
It would be great if ! could be used at the end to force the type checker that the value is not None like this
Does this do anything more than cast(str, foo("foo")) ? Not really a fan since cast does all the things it does and express the intent clearer.
Ok consider this exmaple:
It errors out with
It would require multiple cast to do so where as all can be done with adding a ! at the end @uranusjr
The exact syntax you propose is available in Hypertag ( http://hypertag.io/ ), a Python-inspired language for templating and document generation. There are two different postfix operators (“qualifiers”) that mark a subexpression as either “optional” (?) or “obligatory” (!). The latter enforces a true (non-empty, not-None) value of the expression, otherwise an exception is raised.
Obviously, this operator is applied to ordinary expressions (type declarations don’t exist in Hypertag), still it might do as a related implementation for what you propose. Details can be found here:
http://hypertag.io/#non-standard-postfix-operators
I would also see benefit in this:
It’s more or less shorter syntax:
While cast() is a super-set, it’s much more verbose.
Also, what if I rename SomeSlightlyLengthierClassName ? Fair enough, my IDE’s refactoring features should also rename the reference.
But what if I change get_it_somewhere() to return some other type? I need to manually iterate the code (let’s say I add a child class like SomeSlightlyLengthierChildClassName and return that instead).
If we don’t want to add new syntax to the language, how about a new function for this. It could be called something like unwrap() and would basically be sugar for the long form cast() .
Or maybe I’ve been looking at Rust code for too long.
I would be happy with Rust-like unwrap() or Swift-like ! . It really would be nice if there were some conventional way of doing this.
What does “forcing” a not-None value mean, though? Mypy and other type checkers all have reliable type narrowing behavior around if x is None and similar checks.
I think a more useful family of new syntax elements would be “null-short-circuiting”, such as func_or_none?(x, y, z) and a?.b and u ?+ v . PEP 505 attempted to specify something like this (albeit a limited subset) and was rejected.
I understand why PEP 505 was rejected. It introduces ugly syntax for a narrow set of cases. Using ! would be more general and cleaner. It would, for example, enable type narrowing of the sort we want in comprehensions and in function arguments where doing the whole
dance wouldn’t fit.
I suspect that the underlying disagreement in this discussion is about why some people (like me) really like the optionals of Rust and Swift (and also are into static type checking). I can elaborate on why I feel this would be a good thing and would not undermine anything Pythonic if asked.
I understand that Optional[T] is really just Union[T, None] and that the analogy to optionals in Rust or Swift is a limited analogy. But I want to point out why the kind of type narrowing I and others would like is distinct from other type narrowing.
Epoch Fails and the Problem with Zero
Let’s take a look at a kind of common bug that I like to call an “Epoch Fail”. This is where where some process tries to read a Unix time stamp and fails. Instead of handling that error, the buggy code treats the value as zero. But a zero Unix timestamp is the last second of 1969. We get results like

Using 0 to indicate a special case or the absence of a value leads to errors when 0 can also be a coherent value. So it is very useful to have a way to indicate that there is no value for something. None is perfect for that.
When None is not an error
Now in that example, failing to read a timestamp is almost certainly some error that should be handled as an error. But there are cases where having no value (aka None ) is not an error.
Suppose you have an object for some sort of game state or simulation that gets updated each round of the simulation. Some object variables will depend on things from the previous round. Some might even depend on the previous two rounds. Thus those variables cannot be set to anything meaningful during __init()__ . So during init, we’d want to set those variables to None.
During each round of our game or simulation there will be some methods called that need to behave is special ways. There will be a test if a variable is None . That case is fine and it doesn’t get helped by having an inline TypeGuard-like thing.
But there will be other functions that should assume that the parameters it is given are not not None. Suppose a probability gets updated, but doing so depends on certain search parameters and results from a previous round. Suppose we have something like
If updated_prob() is a fairly general function that really just takes float arguments then it should be declared with the type hints of float, float for its arguments. And while we could always add a
before the call to update_prob() , suppose we want to call update_prob() in a list comprehension or in an an argument to some function.
Being able to say things like
with the ! makes it much easier to write clean code and to communicate what we want to the type checker.
[Note. All of the code here is something I entered directly into the web interface for the discussion. It probably has numerous syntactic errors]
hello! Is there any advance on this suggestion? I believe it would very seriously improve the Python experience regarding typing, I cannot stop writing code where the variable has a possibility of None but we know at runtime, it would be filled.
Considering the following:
This is the dumbest example I can give for myself. To counter this problem, I just use raise keywords to ensure my variable is not None, but it gets very overwhelming, and I don’t believe that using typing.cast is something I’d like to use because I would have to include way too many typing.cast in my codebase.
Being able to indicate easily that my variable are not None is probably one of the greatest typing addition we could obtain.
It’s an issue when “None” as a value has a different meaning than “Not yet defined.” It would be great to have something like NotImplemented, but in case of an object that is REQUIRED to be set before the moment, it will be called for the read.
IMO this is something type checkers should be able to deduce that validate_requirements did not raise, so self.content is not None . So I’d start by raising an issue with mypy for this. It may be that it’s more complex than I’m assuming, though, so they may not be able to make this automatic. Even then, adding a simple assert self.content is not None should be enough to allow mypy to know that afterwards, self.content is definitely a str .
So I don’t think this example is a compelling argument for new syntax.
Apologize, I did not mean to send my post earlier.
I assume this is a decision made to optimize performance of mypy which can’t really be considered. I am actually editing a project containing a lot of code (Up to +5500 lines a file, if not more), and mypy is already taking a long time already to finish its analysis. Making mypy doing deeper analysis would probably be a huge issue if it needed to analyze all called functions to determine every variable’s types.
Sorry, but I’m quite confused by this. Both example (From me and @kumaraditya303 ) achieve the exact same goal, the modified object is the only difference. If this is accepted, I assume anything (Function calls, variables, etc.) will be affected by this new syntax.
Another applicable case is when properties are getting chained, but one property is None, and it would be cleaner to just indicate it’s not-None. For instance:
This example is a bit over the top, but it truly illustrates how checking all chained attributes can be more painful than practical and smart, most especially when we know None will never be present in our context.
This could be even more useful in event-based application. For example:
I wished to contact you personally through this platform, but I am not yet capable to, if you accept, could you please drop me an email at [email protected] so we can discuss this together? I’d like to hear more of your thought, for clarification, but this might get off-topic of this thread.
The ! suggestion isn’t just a Typing change, that would be a new feature in the language and require a PEP. It would be a postfix operator (the only one in the language).
And also, the unwrap function can already be defined that would allow basically exactly the correct behavior:
Works as far as I can tell. So the discussion question would be that value! is significantly better, so much so that it requires language support, compared to unwrap(value) . I haven’t seen an argument for that.
I think ? would actually be far more useful as far as postfix operators go, to allow things like foo?.bar returning None if foo is None , rather than having to write None if foo is None else foo.bar .
But neither ? nor ! really have that much to do with typing, they just make dealing with None easier, but excluding None from an optional type is far from the only type narrowing across method boundaries that will get missed by type checkers, it just happens to be a very common case, so you still will need to write assertions and TypeGuard functions anyways.
That being said I find it’s rare that I actually ever have to write things like assert foo is not None and foo.bar is not None and foo.bar.baz is not None and when I do it’s usually a sign I should refactor my code. assert foo is not None is quite common, but I personally am not that bothered by it.
That has been proposed, but it’s very VERY different from the current proposal, which is just a type-checker feature - equivalent to assert thing is not None (which is something all type checkers should already recognize).
(Side note: This wouldn’t be a “postfix” operator, since thing? wouldn’t really have any meaning on its own. It’s a variant form of attribute lookup ?. rather than being a separate operator that is handled first. Not that postfix operators are inherently bad or anything.)
It could be a postfix operator that turns None into a NoneProducer instance that returns None from all relevant magic methods. This could then be a single new magic method that has a default implemention for object that returns self and NoneType would overwrite that. But ofcourse, that isn’t the proposal in PEP 505 and I am not really sure if it has any real benefits.
Closing as this specific discussion died out 2 years ago, and new comments since it was resurrected are just going in circles with the same suggestions made here and elsewhere about coalescing, maybe, and other similar ideas. At this point, if you want to discuss it, probably best to go through the PEP process and get an official decision.
Related Topics

- Documentation
- System Status
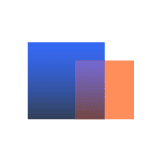
- Rollbar Academy
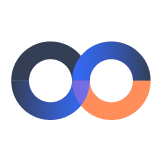
- Software Development
- Engineering Management
- Platform/Ops
- Customer Support
- Software Agency
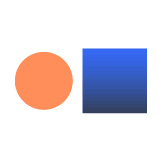
- Low-Risk Release
- Production Code Quality
- DevOps Bridge
- Effective Testing & QA
How to Handle the Incompatible Types Error in Java
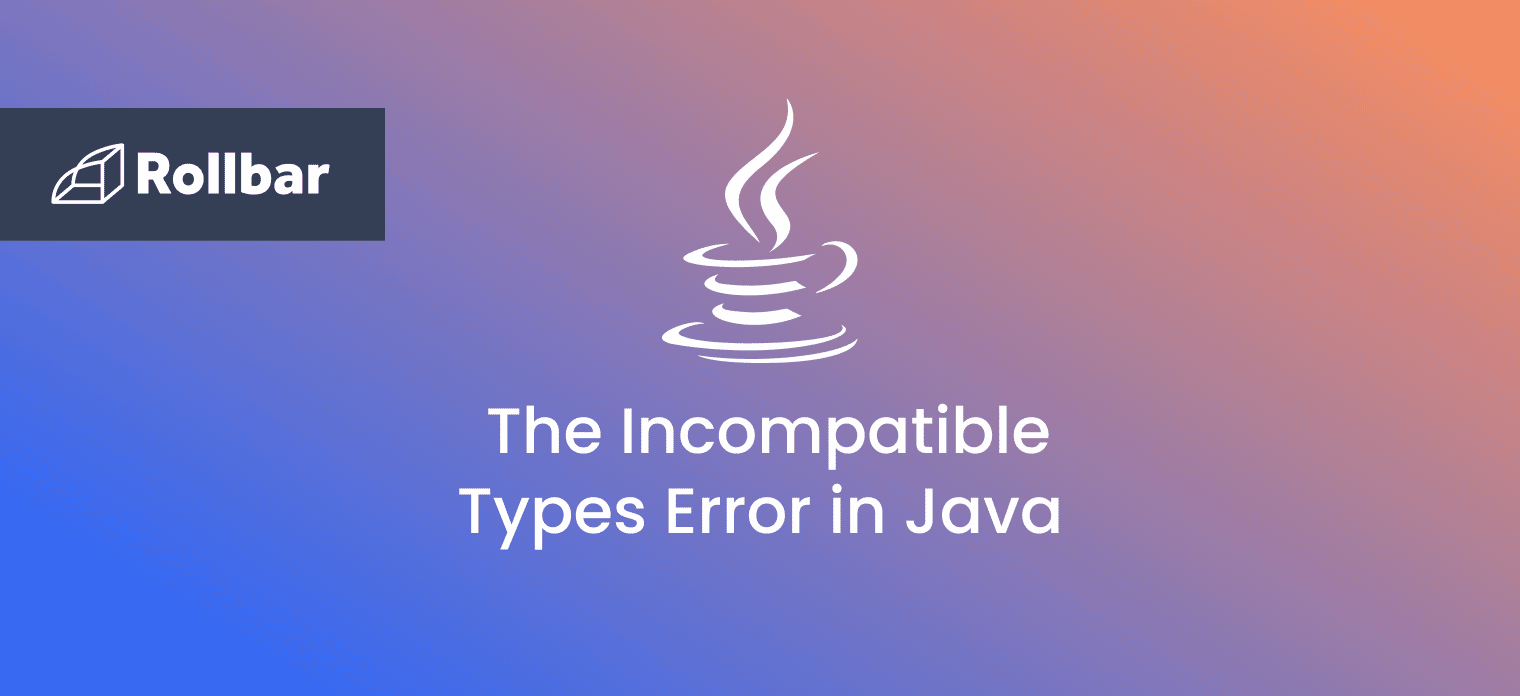
Table of Contents
Introduction to data types & type conversion.
Variables are memory containers used to store information. In Java, every variable has a data type and stores a value of that type. Data types, or types for short, are divided into two categories: primitive and non-primitive . There are eight primitive types in Java: byte , short , int , long , float , double , boolean and char . These built-in types describe variables that store single values of a predefined format and size. Non-primitive types, also known as reference types , hold references to objects stored somewhere in memory. The number of reference types is unlimited, as they are user-defined. A few reference types are already baked into the language and include String , as well as wrapper classes for all primitive types, like Integer for int and Boolean for boolean . All reference types are subclasses of java.lang.Object [ 1 ].
In programming, it is commonplace to convert certain data types to others in order to allow for the storing, processing, and exchanging of data between different modules, components, libraries, APIs, etc. Java is a statically typed language, and as such has certain rules and constraints in regard to working with types. While it is possible to convert to and from certain types with relative ease, like converting a char to an int and vice versa with type casting [ 2 ], it is not very straightforward to convert between other types, such as between certain primitive and reference types, like converting a String to an int , or one user-defined type to another. In fact, many of these cases would be indicative of a logical error and require careful consideration as to what is being converted and how, or whether the conversion is warranted in the first place. Aside from type casting, another common mechanism for performing type conversion is parsing [ 3 ], and Java has some predefined methods for performing this operation on built-in types.
Incompatible Types Error: What, Why & How?
The incompatible types error indicates a situation where there is some expression that yields a value of a certain data type different from the one expected. This error implies that the Java compiler is unable to resolve a value assigned to a variable or returned by a method, because its type is incompatible with the one declared on the variable or method in question . Incompatible, in this context, means that the source type is both different from and unconvertible (by means of automatic type casting) to the declared type.
This might seem like a syntax error, but it is a logical error discovered in the semantic phase of compilation. The error message generated by the compiler indicates the line and the position where the type mismatch has occurred and specifies the incompatible types it has detected. This error is a generalization of the method X in class Y cannot be applied to given types and the constructor X in class Y cannot be applied to given types errors discussed in [ 4 ].
The incompatible types error most often occurs when manual or explicit conversion between types is required, but it can also happen by accident when using an incorrect API, usually involving the use of an incorrect reference type or the invocation of an incorrect method with an identical or similar name.
Incompatible Types Error Examples
Explicit type casting.
Assigning a value of one primitive type to another can happen in one of two directions. Either from a type of a smaller size to one of a larger size (upcasting), or from a larger-sized type to a smaller-sized type (downcasting). In the case of the former, the data will take up more space but will be intact as the larger type can accommodate any value of the smaller type. So the conversion here is done automatically. However, converting from a larger-sized type to a smaller one necessitates explicit casting because some data may be lost in the process.
Fig. 1(a) shows how attempting to assign the values of the two double variables a and b to the int variables x and y results in the incompatible types error at compile-time. Prefixing the variables on the right-hand side of the assignment with the int data type in parenthesis (lines 10 & 11 in Fig. 1(b)) fixes the issue. Note how both variables lost their decimal part as a result of the conversion, but only one kept its original value—this is exactly why the error message reads possible lossy conversion from double to int and why the incompatible types error is raised in this scenario. By capturing this error, the compiler prevents accidental loss of data and forces the programmer to be deliberate about the conversion. The same principle applies to downcasting reference types, although the process is slightly different as polymorphism gets involved [ 5 ].
Explicit parsing
Parsing is a more complex process than type casting as it involves analyzing the underlying structure of a given data before converting it into a specific format or type. For instance, programmers often deal with incoming streams of characters, usually contained in a string that needs to be converted into specific types to make use of in the code. A common scenario is extracting numeric values from a string for further processing, which is where parsing is routinely used.
The main method in Fig. 2(a) declares the variable date which holds the current date as a String in the yyyy-MM-dd format. In order to get the year value into a separate variable it is necessary to “parse” the first 4 characters of the string, and this can be accomplished by splitting the string by specifying the “-” character as a delimiter and then accessing the first element (line 9 in Fig. 2(a)). With this, the year value has been successfully parsed and stored into a new variable. Attempting to increase the value of this new variable and store the resulting value in a separate int variable triggers the incompatible types error (line 10 in Fig. 2(a)). This is because even though the year has been isolated from the date and parsed into a new variable, it is impossible to perform arithmetic operations on a variable of type String . Therefore, it is necessary to represent this value as a numeric type. The best way to do this is to use Java’s built-in Integer::parseInt method which takes a String argument and converts it to an int (line 10 in Fig. 2(b)). (Note that if the given argument is not a valid integer value, this method will throw an exception.) Now that the year has been manually and explicitly parsed from the initial date string into an integer value that can be incremented, the program compiles and prints the expected message, as shown in Fig. 2(b).
Incorrect type assignments
Sometimes, the incompatible types error can occur due to basic negligence, where the only mistake is an accidental mis-declaration of a variable’s type (Fig. 3(a)). In these instances, the issue is quite obvious and simply correcting the type declaration solves the problem (Fig. 3(b)).
Incorrect method return types
A slightly less common but non-surprising occurence of the incompatible types error, especially when refactoring is involved, can be found in method return types. Namely, sometimes a method’s return statement ends up returning a value that doesn’t match the method’s declared return type (Fig. 4(a)). This issue has two possible remedies; either make the value returned match the return type (Fig. 4(b)), or change the method’s return type to match the actual value returned (Fig. 4(c)). And in the case of void methods (methods with no return type), one can simply get rid of the return statement if the return value is never used, as is the case with the example in Fig. 4.
Incorrect imports and similarly named reference types
It is not uncommon to come across classes or other reference types with the same or a similar name. In fact, this happens even within the standard Java API, and can baffle many programmers, beginners and experts alike. One such case is the List class which represents one of Java’s main collection data structures [ 6 ]. This reference type can easily come into collision with another type of the same name, but from a different package. Namely, that’s the java.awt.List class that is part of Java’s built-in AWT API for creating graphical user interfaces [ 7 ]. All it takes is accidentally importing the wrong package, and the compiler immediately complains about the type mismatch, raising the incompatible types error, as demonstrated in Fig. 5(a). Fixing the import on line 5, as shown in Fig. 5(b), sorts things out.
Popular external libraries are also prone to naming their reference types similarly, so whenever relying on such a library for a certain feature or functionality it is important to pick one, or be careful not to confuse one for another, if multiple libraries are already being used.
Fig. 6(a) shows an example of passing a JSON type object to a method as an argument. Here, the method printJson expects an argument of type JsonObject , but the calling method tries to pass in an instance of the similarly named JSONObject reference type, part of a different library altogether. This results in the incompatible types error being raised by the compiler, with the alert org.json.JSONObject cannot be converted to javax.json.JsonObject pointing to the erroneous method call. Swapping the inappropriate constructor call with an instance of the correct type solves the issue, as shown in Fig. 6(b).
Fig. 6 also serves as an example to show how the incompatible types error is, in fact, a generalization of the method X in class Y cannot be applied to given types error explored in [ 4 ]. Depending on the specific compiler that is being used and its configuration settings, either of these errors could be triggered in this kind of scenario.
As a strongly typed language, Java has strict rules regarding data types and how they interoperate. These rules affect variable assignment, method invocation, return values, etc. This makes Java code verbose, but at the same time quite secure, as it allows for many errors to be detected during compilation. One such error is the incompatible types error, which is directly tied to Java’s type system. This article provides some background into Java types and dives into the symptoms and causes behind the incompatible types error, by presenting a series of relevant examples tailored to bring clarity in understanding and successfully managing this error.
Track, Analyze and Manage Errors With Rollbar
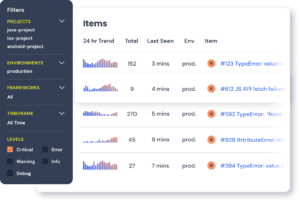
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Java errors easier than ever. Sign Up Today!
[1] R. Liguori and P. Liguori, 2017. Java Pocket Guide, 4th ed. Sebastopol, CA: O'Reilly Media, pp. 23-46.
[2] W3schools.com, 2021. Java Type Casting. Refsnes Data. [Online]. Available: https://www.w3schools.com/java/java_type_casting.asp . [Accessed: Dec. 18, 2021]
[3] D. Capka, 2021. Lesson 3 - Variables, type system and parsing in Java, Ictdemy.com. [Online]. Available: https://www.ictdemy.com/java/basics/variables-type-system-and-parsing-in-java . [Accessed: Dec. 19, 2021]
[4] Rollbar, 2021. How to Fix Method/Constructor X in Class Y Cannot be Applied to Given Types in Java, Rollbar Editorial Team. [Online]. Available: https://rollbar.com/blog/how-to-fix-method-constructor-in-class-cannot-be-applied-to-given-types-in-java/ . [Accessed: Dec. 21, 2021]
[5] W3schools.com, 2021. Java Type Casting. Refsnes Data. [Online]. Available: https://www.w3schools.com/java/java_type_casting.asp . [Accessed: Dec. 21, 2021]
[6] Oracle.com, 2021. Lesson: Implementations (The Java™ Tutorials > Collections). [Online]. Available: https://docs.oracle.com/javase/tutorial/collections/implementations/index.html . [Accessed: Dec. 21, 2021]
[7] Oracle.com, 2020. Package java.awt (Java SE 15 & JDK 15). Oracle and/or its affiliates [Online]. Available: https://docs.oracle.com/en/java/javase/15/docs/api/java.desktop/java/awt/package-summary.html . [Accessed: Dec. 21, 2021]
- error-handling
Related Resources
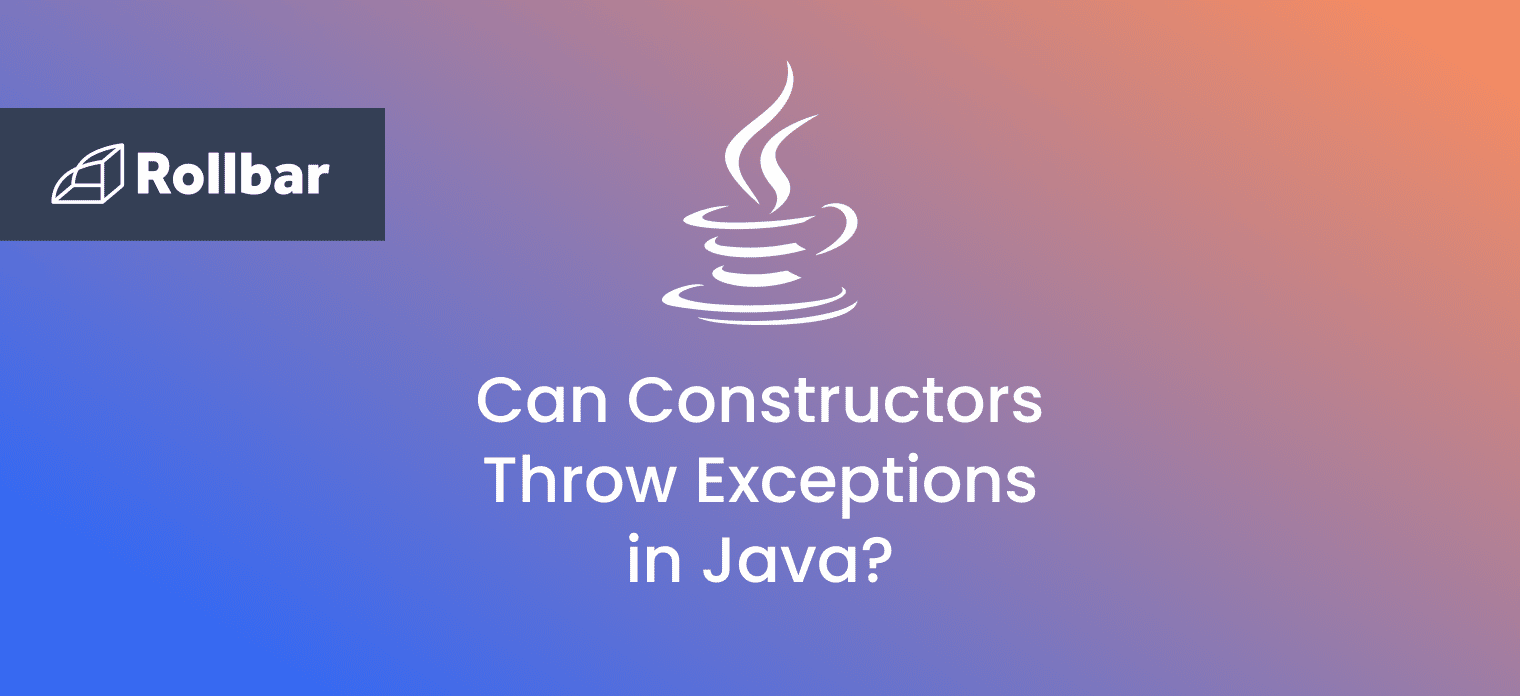
Can Constructors Throw Exceptions in Java
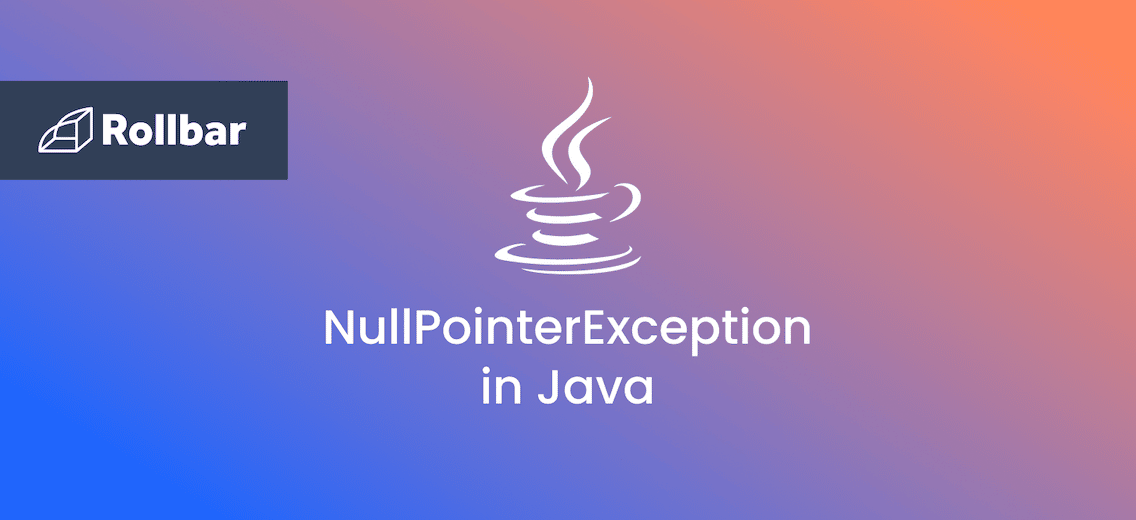
How to Fix and Avoid NullPointerException in Java
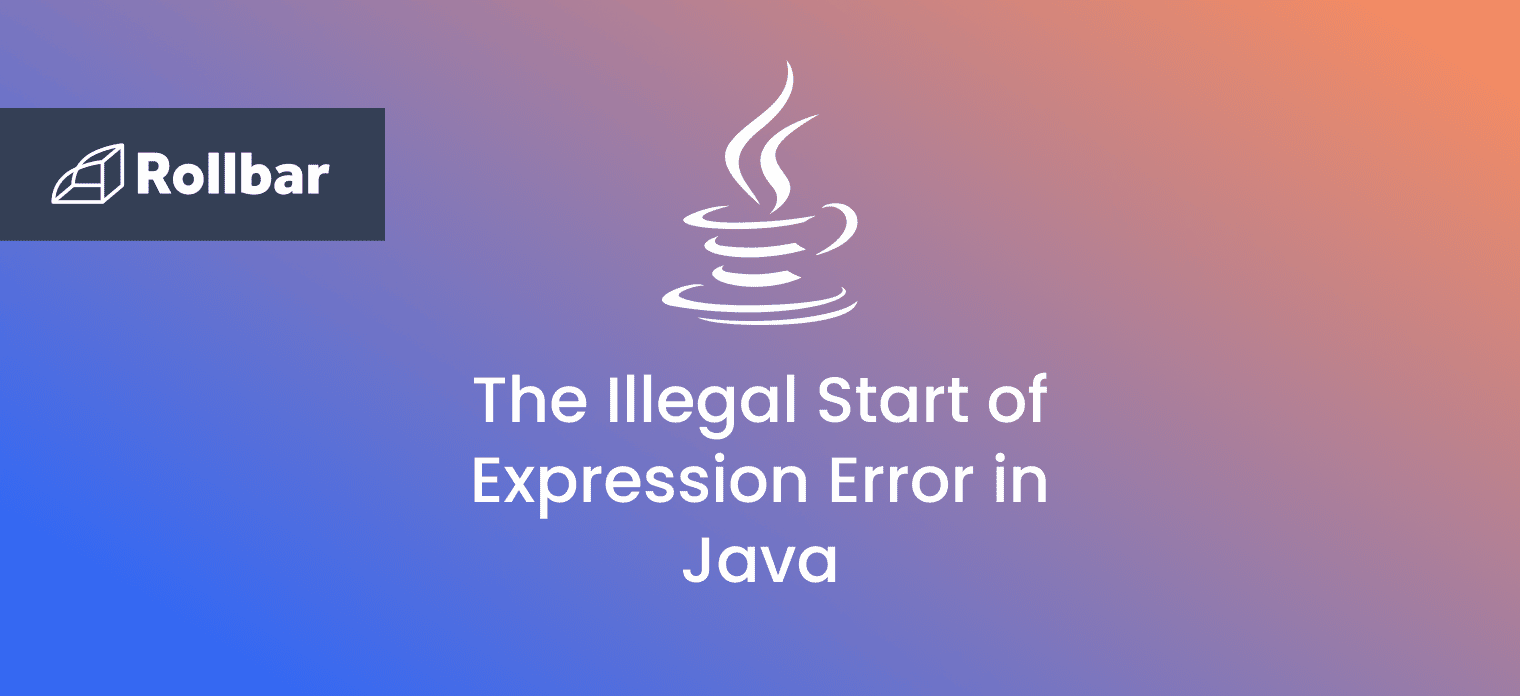
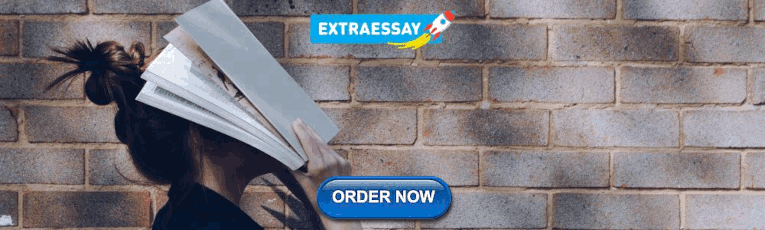
How to Fix "Illegal Start of Expression" in Java
"Rollbar allows us to go from alerting to impact analysis and resolution in a matter of minutes. Without it we would be flying blind."
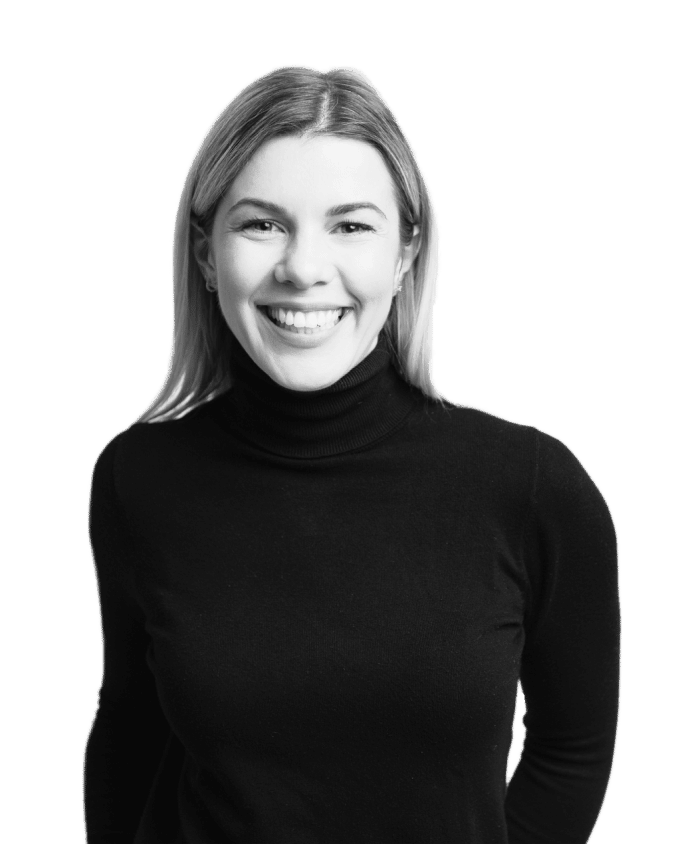
Start continuously improving your code today.
Resolving Error: Incompatible Type Assignment in Flutter/Dart using Bloc
Abstract: This article discusses a common error encountered when designing a Flutter/Dart application, where the Dart element type can't be assigned a list type Bloc . We will explore the root cause of this error and provide possible solutions.
When designing a new Flutter/Dart application, encountering errors is inevitable. One such error is the "Incompatible Type Assignment" error, which can occur when using the Bloc library. This article will provide a detailed explanation of this error, its causes, and solutions to help you resolve it.
Understanding the Bloc Library
The Bloc (Business Logic Component) library is a popular state management solution for Flutter applications. It simplifies app development by separating the UI, data, and business logic layers. The Bloc library uses events, states, and blocs to manage the app's state, making it easier to develop complex applications.
Incompatible Type Assignment Error
The "Incompatible Type Assignment" error occurs when you try to assign a value of a different type to a variable. This error can occur in various situations, such as assigning a string to an integer variable. In the context of the Bloc library, this error can occur when defining events, states, or blocs.
Example of Incompatible Type Assignment Error
Consider the following example:
In this example, the 'myString' variable is of type 'String', but the Bloc expects an event of type 'MyEvent'. This mismatch results in the "Incompatible Type Assignment" error.
To resolve the "Incompatible Type Assignment" error, follow these steps:
- Ensure that the event, state, and bloc types are correctly defined.
- Check that the event being dispatched matches the expected event type in the bloc.
- Verify that the 'mapEventToState' method returns the correct state type.
- Ensure that the 'yield' keyword is used correctly and returns the correct state type.
Preventing Incompatible Type Assignment Errors
To prevent the "Incompatible Type Assignment" error, follow these best practices:
- Define clear and concise types for events, states, and blocs.
- Use type annotations to ensure that variables are of the correct type.
- Consistently use the 'mapEventToState' method to handle events and return the correct state type.
- Test your application regularly to catch and fix errors early.
The "Incompatible Type Assignment" error in Flutter/Dart using the Bloc library can be frustrating, but it is usually easy to resolve. By understanding the Bloc library, identifying the causes of the error, and following the recommended solutions, you can quickly get your application back on track. Regular testing and following best practices can help prevent this error from occurring in the first place.
- Bloc Library
- Dart Variables
Tags: : Flutter Dart Bloc TypeError
Latest news
- Unexpected Resource Consumption by MySQL Service on Ubuntu VPS
- Connecting GoLang and Flutter on macOS
- Detecting Nmap Scans Traffic on Your Web Server
- Generating SIC/EuroSIC/SEPA/SWIFT PACS.008/PACS.009 messages using Java
- Helm Upgrade: 'false' Output Despite Successful Chart Update
- Self-Hosted Backup Management Solution for Multiple Shared Hosts and Various Providers: A Look at Akeeba Pro and Cove Data Protection
- Preventing AI Model from Generating Answers Outside Predefined Corpus in Python Chatbot
- Understanding Parallelism Behavior in Oracle 19c RAC Partitioned Environment with 50 CPUs
- Updated Matplotlib: Modules Won't Load in Python
- Implementing Voice Activity Detection in Twilio Voice Bot using Django
- Flattening Parent-Child Hierarchy Tables in Snowflake using Recursive CTE
- Issue Tripe Payment using Bank Account: A Step-by-Step Guide for Software Developers
- Adding a New Public Key to Your GitHub Account
- Skipping Build and Test Stages in GitLab: Conditions and Run Deploy Releases
- Connecting to Ubuntu GUI on EC2 Instance with AWS Session Manager
- Error Uploading Files to Laravel DigitalOceanspaces
- Why Isn't My Keras Model Training All Layers with Constant Validation Accuracy and Training Accuracy of 100%?
- PyAutoGUI Image Not Found: Resolving Directory Issues
- Python Error: Unable to Create Process using python - Access Denied
- Running Scenario Outline with Extent Reports using JUnit: Log feature WebdriverUniv
- Convert Unix File from Binary Text to Viewable Text
- Managing Multiple Connections in Production using Dialogflow and FastAPI
- Printing Five Elements of a Marie Assembly Array
- CSV, SQL, and Give ArgumentError: Query argument present
- Google Maps and Dynamic Lat-Lon in Angular: A Loading Issue
- Fixing CORS Policy Errors in ExpressJS Deployments
- Deploying Function Apps using Azure Release Pipelines: A How-To
- Minimum Permissions Required for Dataflow Usage by Datamodel Developers
- Next.js: Using SSR for a Global Navbar with useState()
- Choosing a Specific GPU Publisher for Running CLI Mode: A Software Development Perspective
- Comparing Unknown Class Objects in Java: A Solution
- Using KQL: Datetime Conversion with min/max functions
- Using zip() function with dict(), returns key-value pairs in Python
- Understanding Sequence Point Problem in C: An Example
- Creating a Multiplier with Carry Lookahead Adders: Consistent Output of 'zzz'
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Mypy reports incompatible types for an optional dataclass field with generic default factory #11923
sagittarian commented Jan 6, 2022
- 👍 10 reactions
yoann9344 commented Feb 24, 2023 • edited
Sorry, something went wrong.
Pycz commented Mar 2, 2023 • edited
Pycz commented mar 2, 2023.
lteich commented May 17, 2023
ilevkivskyi commented Oct 29, 2023
No branches or pull requests
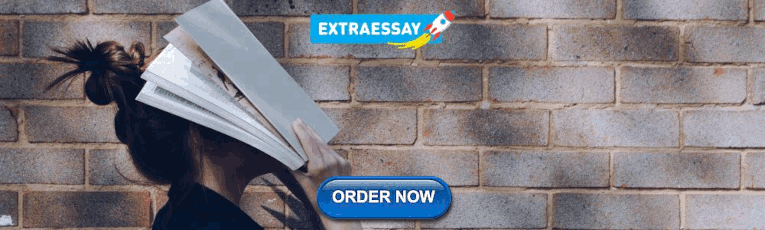
IMAGES
VIDEO
COMMENTS
Consequently, when you try and insert a str later, mypy (rightfully) complains. You have several options for fixing your code, which I'll list in order of least to most type-safe. Option 1 is to declare your dictionary such that its values are of type Any-- that is, your values will not be type-checked at all:
Incompatible types in assignment (expression has type "int", variable has type "Optional[str]") #8557 Closed waketzheng opened this issue Mar 18, 2020 · 5 comments
Saved searches Use saved searches to filter your results more quickly
This seems to be expected behavior, if the boolean something is false you reassign action to method2 which has an argument however in your code this creates a conflict because when you first typed action you said that it will never have any arguments but now it does, creating incompatible types in the assignment.
$ mypy example.py example.py:4: error: Incompatible types in assignment (expression has type "None", variable has type Module) ... Version 0.920 included a similar change, to allow a None assignment in an else block to affect a variable's inferred type. (See "Making a Variable Optional in an Else Block" in the release post.) But, at least ...
For return types, it's unsafe to override a method with a more general return type. Other incompatible signature changes in method overrides, such as adding an extra required parameter, or removing an optional parameter, will also generate errors. The signature of a method in a subclass should accept all valid calls to the base class method.
Incompatible types in assignment (expression has type "Optional[LinkedList]", variable has type "LinkedList") Why does this happen, and how can I resolve the problem? python; ... I think that the right solution is to do is to indicate that countries is a variable of type Optional[LinkedList], whenever declaring it:
After a quick Google search, the problem wasn't the second assignment but the first assignment. The first assignment was initialized with a four-item tuple, and mypy expected the second assignment to be a four-item tuple. I needed a type hint for the first assignment to set expectations for mypy regarding the second
The code fetches the size query parameter, which is a string, and tries to parse it as an int, or fall back to the default value 40.Python can run this code just fine, but if we check it with Mypy, we'll see some errors: $ mypy example.py example.py:7: error: Incompatible types in assignment (expression has type "int", variable has type "str") example.py:9: error: Incompatible types in ...
error: Incompatible types in assignment (expression has type "Tuple[int, int, int]", variable has type "List[int]") What is the behavior/output you expect? ... (For example --strict-optional) no flag. If mypy crashed with a traceback, please paste the full traceback below.
The latter enforces a true (non-empty, not-None) value of the expression, otherwise an exception is raised. Obviously, this operator is applied to ordinary expressions (type declarations don't exist in Hypertag), still it might do as a related implementation for what you propose.
error: Not overriding class variable previously declared in base class 'User' (misc) error: Incompatible types in assignment (expression has type "None", base class 'UserManager' has type 'UserManager[User]')
Introduction to Data Types & Type Conversion. Variables are memory containers used to store information. In Java, every variable has a data type and stores a value of that type. Data types, or types for short, are divided into two categories: primitive and non-primitive.There are eight primitive types in Java: byte, short, int, long, float, double, boolean and char.
gvanrossum / ilevkivskyi, thank you for your attention on this. I researched the suggested topics worked up a couple more examples and at first I couldn't see the problem.
incompatible types in assignment c; Share. Improve this question. Follow edited Jun 25, 2012 at 0:03. Darshan Rivka Whittle. 33.5k 7 7 gold badges 94 94 silver badges 112 112 bronze badges. asked Jun 24, 2012 at 23:33. Muaz Usmani Muaz Usmani.
ERROR VCP2852 "Incompatible types at assignment: .objSingle1 ← create(0)." "testbench.sv" 36 39 ERROR VCP2852 "Incompatible types at assignment: .objSingle2 ← create(0)." "testbench.sv" 37 39. Xiang_Li1 June 15, 2018, 7:09am 2. In reply to ASICverif: the function type of create should be singleton, so you can define the ...
Well you are probably right. It is the TODO comment for the case of ARG_STAR and ARG_STAR2 which is not handled as a context, and therefore falls back to the "no context" case, which does not handle the return type good enough for this case.
Ensure that the 'yield' keyword is used correctly and returns the correct state type. Preventing Incompatible Type Assignment Errors. To prevent the "Incompatible Type Assignment" error, follow these best practices: Define clear and concise types for events, states, and blocs. Use type annotations to ensure that variables are of the correct type.
In a dataclass with an optional field with a generic as the default factory, mypy reports that the types are incompatible when there should be no compatibility issue between them. from typing import List , Optional from dataclasses import dataclass , field @ dataclass class A : x : Optional [ List [ int ]] = field ( default_factory = list )
As others mentioned, you should be using std::string.But if you really want to assign a string literal to the array, you can do like below: struct message_text{ char text[1024]; template <int N> void assignText(const char (&other)[N]) { static_assert(N < 1024, "String contains more than 1024 chars"); for(int i =0 ; i < N ; ++i) { text[i] = other[i]; } } };