Constructors and member initializer lists
Constructor is a special non-static member function of a class that is used to initialize objects of its class type.
In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual base subobjects and non-static data members. ( Not to be confused with std::initializer_list )
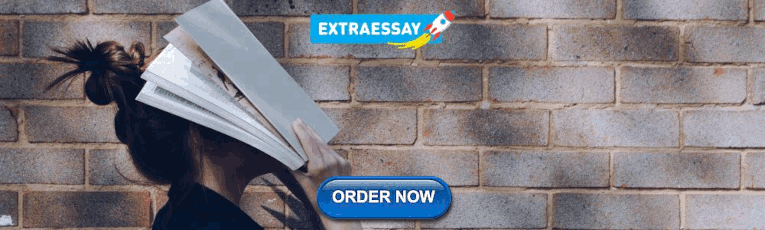
[ edit ] Syntax
Constructors are declared using member function declarators of the following form:
Where class-name must name the current class (or current instantiation of a class template), or, when declared at namespace scope or in a friend declaration, it must be a qualified class name.
The only specifiers allowed in the decl-specifier-seq of a constructor declaration are friend , inline , explicit and constexpr (in particular, no return type is allowed). Note that cv- and ref-qualifiers are not allowed either; const and volatile semantics of an object under construction don't kick in until the most-derived constructor completes.
The body of a function definition of any constructor, before the opening brace of the compound statement, may include the member initializer list , whose syntax is the colon character : , followed by the comma-separated list of one or more member-initializers , each of which has the following syntax
[ edit ] Explanation
Constructors have no names and cannot be called directly. They are invoked when initialization takes place, and they are selected according to the rules of initialization. The constructors without explicit specifier are converting constructors . The constructors with a constexpr specifier make their type a LiteralType . Constructors that may be called without any argument are default constructors . Constructors that take another object of the same type as the argument are copy constructors and move constructors .
Before the compound statement that forms the function body of the constructor begins executing, initialization of all direct bases, virtual bases, and non-static data members is finished. Member initializer list is the place where non-default initialization of these objects can be specified. For members that cannot be default-initialized, such as members of reference and const-qualified types, member initializers must be specified.
The initializers where class-or-identifier names a virtual base class are ignored during execution of constructors of any class that is not the most derived class of the object that's being constructed.
Names that appear in expression-list or brace-init-list are evaluated in scope of the constructor:
Exceptions that are thrown from member initializers may be handled by function-try-block
Member functions (including virtual member functions) can be called from member initializers, but the behavior is undefined if not all direct bases are initialized at that point.
For virtual calls (if the bases are initialized), the same rules apply as the rules for the virtual calls from constructors and destructors: virtual member functions behave as if the dynamic type of * this is the class that's being constructed (dynamic dispatch does not propagate down the inheritance hierarchy) and virtual calls (but not static calls) to pure virtual member functions are undefined behavior.
[ edit ] Initialization order
The order of member initializers in the list is irrelevant: the actual order of initialization is as follows:
(Note: if initialization order was controlled by the appearance in the member initializer lists of different constructors, then the destructor wouldn't be able to ensure that the order of destruction is the reverse of the order of construction)
[ edit ] Example
[ edit ] references.
- C++11 standard (ISO/IEC 14882:2011):
- 12.1 Constructors [class.ctor]
- 12.6.2 Initializing bases and members [class.base.init]
- C++98 standard (ISO/IEC 14882:1998):
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- C++ default constructor | Built-in types for int(), float, double()
- Templates and Default Arguments
- Integer literal in C/C++ (Prefixes and Suffixes)
- Convert C/C++ program to Preprocessor code
- How does 'void*' differ in C and C++?
- const_cast in C++ | Type Casting operators
- Hiding of all Overloaded Methods with Same Name in Base Class in C++
- When should we write our own assignment operator in C++?
- Conversion Operators in C++
- Incompatibilities between C and C++ codes
- Socket Programming in C/C++: Handling multiple clients on server without multi threading
- When are Constructors Called?
- Amazing stuff with system() in C / C++
- Assertions in C/C++
- Exception Handling and Object Destruction in C++
- Quine - A self-reproducing program
- Program to print last 10 lines
- Use of explicit keyword in C++
- Default Assignment Operator and References in C++
When do we use Initializer List in C++?
Initializer List is used in initializing the data members of a class. The list of members to be initialized is indicated with constructor as a comma-separated list followed by a colon. Following is an example that uses the initializer list to initialize x and y of Point class.
The above code is just an example for syntax of the Initializer list. In the above code, x and y can also be easily initialed inside the constructor. But there are situations where initialization of data members inside constructor doesn’t work and Initializer List must be used. The following are such cases:
1. For Initialization of Non-Static const Data Members
const data members must be initialized using Initializer List. In the following example, “t” is a const data member of Test class and is initialized using Initializer List. Reason for initializing the const data member in the initializer list is because no memory is allocated separately for const data member, it is folded in the symbol table due to which we need to initialize it in the initializer list.
Also, it is a Parameterized constructor and we don’t need to call the assignment operator which means we are avoiding one extra operation.
2. For Initialization of Reference Members
Reference members must be initialized using the Initializer List. In the following example, “t” is a reference member of the Test class and is initialized using the Initializer List.
3. For Initialization of Member Objects that do not have a Default Constructor
In the following example, an object “a” of class “A” is a data member of class “B”, and “A” doesn’t have a default constructor. Initializer List must be used to initialize “a”.
If class A had both default and parameterized constructors, then Initializer List is not a must if we want to initialize “a” using the default constructor, but it is must to initialize “a” using the parameterized constructor.
4. For Initialization of Base Class Members
Like point 3, the parameterized constructor of the base class can only be called using the Initializer List.
5. When the Constructor’s Parameter Name is the Same as Data Member
If the constructor’s parameter name is the same as the data member name then the data member must be initialized either using this pointer or Initializer List. In the following example, both the member name and parameter name for A() is “i”.
6. For Performance Reasons
It is better to initialize all class variables in the Initializer List instead of assigning values inside the body. Consider the following example:
Here compiler follows following steps to create an object of type MyClass
1. Type’s constructor is called first for “a”.
2. Default construct “variable”
3. The assignment operator of “Type” is called inside body of MyClass() constructor to assign
4. And then finally destructor of “Type” is called for “a” since it goes out of scope.
Now consider the same code with MyClass() constructor with Initializer List
With the Initializer List, the following steps are followed by compiler:
1. Type’s constructor is called first for “a”. 2. Parameterized constructor of “Type” class is called to initialize: variable(a). The arguments in the initializer list are used to copy construct “variable” directly. 3. The destructor of “Type” is called for “a” since it goes out of scope.
As we can see from this example if we use assignment inside constructor body there are three function calls: constructor + destructor + one addition assignment operator call. And if we use Initializer List there are only two function calls: copy constructor + destructor call. See this post for a running example on this point.
This assignment penalty will be much more in “real” applications where there will be many such variables. Thanks to ptr for adding this point.
Parameter vs Uniform Initialization in C++
It is better to use an initialization list with uniform initialization {} rather than parameter initialization () to avoid the issue of narrowing conversions and unexpected behavior. It provides stricter type-checking during initialization and prevents potential narrowing conversions
Code using parameter initialization ()
In the above code, the value 300 is out of the valid range for char, which may lead to undefined behavior and potentially incorrect results. The compiler might generate a warning or error for this situation, depending on the compilation settings.
Code using uniform initialization {}
By using uniform initialization with {} and initializing x with the provided value a, the compiler will perform stricter type-checking and issue a warning or error during compilation, indicating the narrowing conversion from int to char. Here is code with uniform initialization {} , which results in a warning and thus better to use
Please Login to comment...
Similar reads.
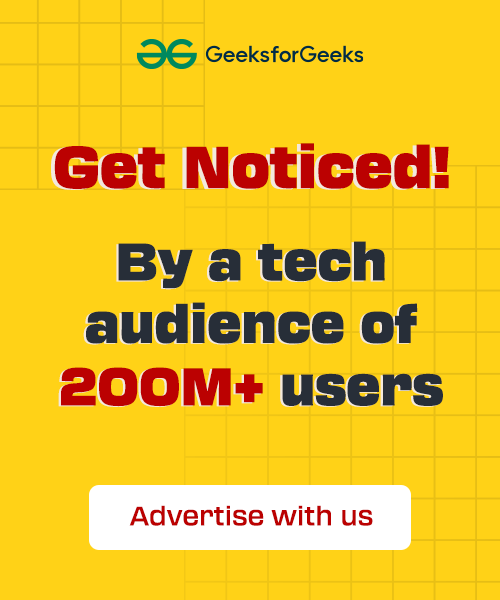
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Defined in: <initializer_list>
std::initializer_list is a light container that holds objects from list initialization or braced initialization.
This section requires improvement. You can help by editing this doc page.
Technical details
An object of type std::initializer_list<T> is a lightweight proxy object that provides access to an array of objects of type const T .
A std::initializer_list<T> object is automatically constructed when:
- A braced-init-list is used to list-initialize an object, where the corresponding constructor accepts an std::initializer_list<T> parameter
- A braced-init-list is used as the right operand of assignment or as a function call argument, and the corresponding assignment operator/function accepts an std::initializer_list<T> parameter
- A braced-init-list is bound to auto, including in a ranged for loop
Initializer lists may be implemented as a pair of pointers or pointer and length.
Copying a std::initializer_list does not copy the underlying objects.
The underlying array is a temporary array of type const T[N] , in which each element is copy-initialized (except that narrowing conversions are invalid) from the corresponding element of the original initializer list. The lifetime of the underlying array is the same as any other temporary object, except that initializing an initializer list object from the array extends the lifetime of the array exactly like binding a reference to a temporary (with the same exceptions, such as for initializing a non-static class member). The underlying array may be allocated in read-only memory.
The program is ill-formed
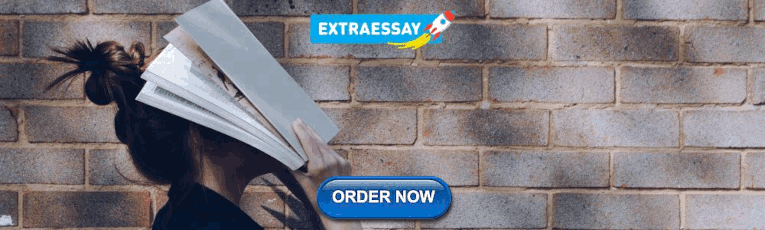
std :: initializer_list
Template parameters , type names , member functions , iterators , non-member functions , free function templates overloaded for std::initializer_list .
- Technical details
- Template parameters
- Non-member functions
- Free function templates overloaded for std::initializer_list

14.10 — Constructor member initializer lists
This lesson continues our introduction of constructors from lesson 14.9 -- Introduction to constructors .
Member initialization via a member initialization list
To have a constructor initialize members, we do so using a member initializer list (often called a “member initialization list”). Do not confuse this with the similarly named “initializer list” that is used to initialize aggregates with a list of values.
Member initialization lists are something that is best learned by example. In the following example, our Foo(int, int) constructor has been updated to use a member initializer list to initialize m_x , and m_y :
The member initializer list is defined after the constructor parameters. It begins with a colon (:), and then lists each member to initialize along with the initialization value for that variable, separated by a comma. You must use a direct form of initialization here (preferably using braces, but parentheses works as well) -- using copy initialization (with an equals) does not work here. Also note that the member initializer list does not end in a semicolon.
This program produces the following output:
When foo is instantiated, the members in the initialization list are initialized with the specified initialization values. In this case, the member initializer list initializes m_x to the value of x (which is 6 ), and m_y to the value of y (which is 7 ). Then the body of the constructor runs.
When the print() member function is called, you can see that m_x still has value 6 and m_y still has value 7 .
Member initializer list formatting
C++ provides a lot of freedom to format your member initializer lists as you prefer, as it doesn’t care where you put your colon, commas, or whitespace.
The following styles are all valid (and you’re likely to see all three in practice):
Our recommendation is to use the third style above:
- Put the colon on the line after the constructor name, as this cleanly separates the member initializer list from the function prototype.
- Indent your member initializer list, to make it easier to see the function names.
If the member initialization list is short/trivial, all initializers can go on one line:
Otherwise (or if you prefer), each member and initializer pair can be placed on a separate line (starting with a comma to maintain alignment):
Member initialization order
Because the C++ standard says so, the members in a member initializer list are always initialized in the order in which they are defined inside the class (not in the order they are defined in the member initializer list).
In the above example, because m_x is defined before m_y in the class definition, m_x will be initialized first (even if it is not listed first in the member initializer list).
Because we intuitively expect variables to be initialized left to right, this can cause subtle errors to occur. Consider the following example:
In the above example, our intent is to calculate the larger of the initialization values passed in (via std::max(x, y) and then use this value to initialize both m_x and m_y . However, on the author’s machine, the following result is printed:
What happened? Even though m_y is listed first in the member initialization list, because m_x is defined first in the class, m_x gets initialized first. And m_x gets initialized to the value of m_y , which hasn’t been initialized yet. Finally, m_y gets initialized to the greater of the initialization values.
To help prevent such errors, members in the member initializer list should be listed in the order in which they are defined in the class. Some compilers will issue a warning if members are initialized out of order.
Best practice
Member variables in a member initializer list should be listed in order that they are defined in the class.
It’s also a good idea to avoid initializing members using the value of other members (if possible). That way, even if you do make a mistake in the initialization order, it shouldn’t matter because there are no dependencies between initialization values.
Member initializer list vs default member initializers
Members can be initialized in a few different ways:
- If a member is listed in the member initializer list, that initialization value is used
- Otherwise, if the member has a default member initializer, that initialization value is used
- Otherwise, the member is default initialized.
This means that if a member has both a default member initializer and is listed in the member initializer list for the constructor, the member initializer list value takes precedence.
Here’s an example showing all three initialization methods:
On the author’s machine, this output:
Here’s what’s happening. When foo is constructed, only m_x appears in the member initializer list, so m_x is first initialized to 6 . m_y is not in the member initialization list, but it does have a default member initializer, so it is initialized to 2 . m_z is neither in the member initialization list, nor does it have a default member initializer, so it is default initialized (which for fundamental types, means it is left uninitialized). Thus, when we print the value of m_z , we get undefined behavior.
Constructor function bodies
The bodies of constructors functions are most often left empty. This is because we primarily use constructor for initialization, which is done via the member initializer list. If that is all we need to do, then we don’t need any statements in the body of the constructor.
However, because the statements in the body of the constructor execute after the member initializer list has executed, we can add statements to do any other setup tasks required. In the above examples, we print something to the console to show that the constructor executed, but we could do other things like open a file or database, allocate memory, etc…
New programmers sometimes use the body of the constructor to assign values to members:
Although in this simple case this will produce the expected result, in case where members are required to be initialized (such as for data members that are const or references) assignment will not work.
Prefer using the member initializer list to initialize your members over assigning values in the body of the constructor.
Question #1
Write a class named Ball. Ball should have two private member variables, one to hold a color, and one to hold a radius. Also write a function to print out the color and radius of the ball.
The following sample program should compile:
and produce the result:
Show Solution
Question #2
Why did we make print() a non-member function instead of a member function?
The rationale for this is given in lesson 14.8 -- The benefits of data hiding (encapsulation) .
Question #3
Why did we make m_color a std::string instead of a std::string_view ?
In this particular example, it doesn’t matter (because our color arguments are C-style string literals, which doesn’t go out of scope).
But conceptually, we want our Ball class to be an owner of the color passed in. If m_color were a std::string_view , passing in a temporary object for the color argument (e.g. a std::string returned from a function) would leave m_color dangling when the temporary color argument was destroyed.
We discuss this case in more detail (and show an example) in lesson 13.11 -- Struct miscellany .
std:: initializer_list
An object of type std::initializer_list<T> is a lightweight proxy object that provides access to an array of objects of type T .
A std::initializer_list object is automatically constructed when:
- a braced-init-list is used in list-initialization , including function-call list initialization and assignment expressions (not to be confused with constructor initializer lists )
- a braced-init-list is bound to auto , including in a ranged for loop
Initializer lists may be implemented as a pair of pointers or pointer and length. Copying a std::initializer_list does not copy the underlying objects. The underlying array is not guaranteed to exist after the lifetime of the original initializer list object has ended. The storage for std::initializer_list is unspecified (i.e. it could be automatic, temporary, or static read-only memory, depending on the situation).
[ edit ] Member types
[ edit ] member functions, [ edit ] non-member functions, [ edit ] example.
Constructors and member initializer lists
Constructor is a special non-static member function of a class that is used to initialize objects of its class type.
In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual base subobjects and non-static data members. ( Not to be confused with std::initializer_list )
Constructors are declared using member function declarators of the following form:
Where class-name must name the current class (or current instantiation of a class template), or, when declared at namespace scope or in a friend declaration, it must be a qualified class name.
The only specifiers allowed in the decl-specifier-seq of a constructor declaration are friend , inline , explicit and constexpr (in particular, no return type is allowed). Note that cv- and ref-qualifiers are not allowed either; const and volatile semantics of an object under construction don't kick in until the most-derived constructor completes.
The body of a function definition of any constructor, before the opening brace of the compound statement, may include the member initializer list , whose syntax is the colon character : , followed by the comma-separated list of one or more member-initializers , each of which has the following syntax
Explanation
Constructors have no names and cannot be called directly. They are invoked when initialization takes place, and they are selected according to the rules of initialization. The constructors without explicit specifier are converting constructors . The constructors with a constexpr specifier make their type a LiteralType . Constructors that may be called without any argument are default constructors . Constructors that take another object of the same type as the argument are copy constructors and move constructors .
Before the compound statement that forms the function body of the constructor begins executing, initialization of all direct bases, virtual bases, and non-static data members is finished. Member initializer list is the place where non-default initialization of these objects can be specified. For members that cannot be default-initialized, such as members of reference and const-qualified types, member initializers must be specified. No initialization is performed for anonymous unions or variant members that do not have a member initializer.
The initializers where class-or-identifier names a virtual base class are ignored during execution of constructors of any class that is not the most derived class of the object that's being constructed.
Names that appear in expression-list or brace-init-list are evaluated in scope of the constructor:
Exceptions that are thrown from member initializers may be handled by function-try-block
Member functions (including virtual member functions) can be called from member initializers, but the behavior is undefined if not all direct bases are initialized at that point.
For virtual calls (if the bases are initialized), the same rules apply as the rules for the virtual calls from constructors and destructors: virtual member functions behave as if the dynamic type of * this is the class that's being constructed (dynamic dispatch does not propagate down the inheritance hierarchy) and virtual calls (but not static calls) to pure virtual member functions are undefined behavior.
Initialization order
The order of member initializers in the list is irrelevant: the actual order of initialization is as follows:
(Note: if initialization order was controlled by the appearance in the member initializer lists of different constructors, then the destructor wouldn't be able to ensure that the order of destruction is the reverse of the order of construction)
Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
- C++11 standard (ISO/IEC 14882:2011):
- 12.1 Constructors [class.ctor]
- 12.6.2 Initializing bases and members [class.base.init]
- C++98 standard (ISO/IEC 14882:1998):
cppreference.com
Std::list<t,allocator>:: operator=.
Replaces the contents of the container.
[ edit ] Parameters
[ edit ] return value, [ edit ] complexity, [ edit ] exceptions, [ edit ] notes.
After container move assignment (overload (2) ), unless element-wise move assignment is forced by incompatible allocators, references, pointers, and iterators (other than the end iterator) to other remain valid, but refer to elements that are now in * this . The current standard makes this guarantee via the blanket statement in [container.reqmts]/67 , and a more direct guarantee is under consideration via LWG issue 2321 .
[ edit ] Example
The following code uses operator = to assign one std::list to another:
[ edit ] See also
- conditionally noexcept
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 13 November 2021, at 08:24.
- This page has been accessed 56,380 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Member initializer list
In the definition of a constructor of a class, specifies initializers for direct and virtual base subobjects and non-static data members. ( Not to be confused with std::initializer_list )
[ edit ] Syntax
Appears as part of function definition syntax, for class constructors only, as the first part of the function body (before the opening brace of the compound statement)
The first character is the colon : , followed by the comma-separated list of one or more member-initializers , each of which has the following syntax
[ edit ] Explanation
Before the compound statement that forms the function body of the constructor begins executing, initialization of all direct bases, virtual bases, and non-static data members is finished. Member initializer list is the place where non-default initialization of these objects can be specified. For members that cannot be default-initialized, such as members of reference and const-qualified types, member initializers must be specified.
The initializers where class-or-identifier names a virtual base class are ignored during execution of constructors of any class that is not the most derived class of the object that's being constructed.
Names that appear in expression-list or brace-init-list are evaluated in scope of the constructor:
Member functions (including virtual member functions) can be called from member initializers, but the behavior is undefined if not all direct bases are initialized at that point.
For virtual calls (if the bases are initialized), the same rules apply as the rules for the virtual calls from constructors and destructors: virtual member functions behave as if the dynamic type of * this is the class that's being constructed (dynamic dispatch does not propagate down the inheritance hierarchy) and virtual calls (but not static calls) to pure virtual member functions are undefined behavior.
[ edit ] Initialization order
The order of member initializers in the list is irrelevant: the actual order of initialization is as follows:
(Note: if initialization order was controlled by the appearance in the member initializer lists of different constructors, then the destructor wouldn't be able to ensure that the order of destruction is the reverse of the order of construction)
[ edit ] Example
[ edit ] references.
- C++11 standard (ISO/IEC 14882:2011):
- 12.6.2 Initializing bases and members [class.base.init]
- C++98 standard (ISO/IEC 14882:1998):
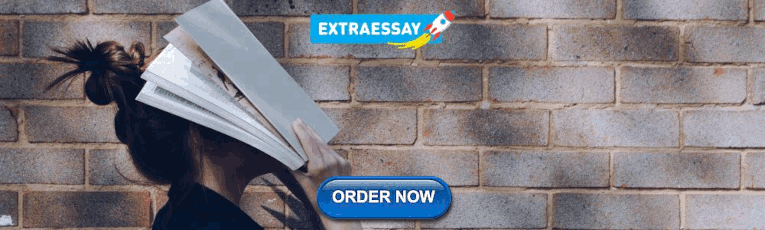
IMAGES
VIDEO
COMMENTS
An object of type std::initializer_list<T> is a lightweight proxy object that provides access to an array of objects of type const T (that may be allocated in read-only memory). a brace-enclosed initializer list is bound to auto, including in a ranged for loop . std::initializer_list may be implemented as a pair of pointers or pointer and length.
Unlike auto, where a braced-init-list is deduced as an initializer_list, template argument deduction considers it to be a non-deduced context, unless there exists a corresponding parameter of type initializer_list<T>, in which case the T can be deduced.. From §14.8.2.1/1 [temp.deduct.call] (emphasis added). Template argument deduction is done by comparing each function template parameter type ...
Class assignment using std::initializer_list. You can also use std::initializer_list to assign new values to a class by overloading the assignment operator to take a std::initializer_list parameter. This works analogously to the above. We'll show an example of how to do this in the quiz solution below.
Constructors and member initializer lists. Constructor is a special non-static member function of a class that is used to initialize objects of its class type. In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual base subobjects and non-static data members.
The initializer list is the place where initialization of the object should occur, there is where the constructors for base classes and members are called. Members are initialized in the same order as they are declared, not as they appear in the initializer list. If a parameter in the constructor has the same name as one of the members, the ...
Explanation. List initialization is performed in the following situations: 1) initialization of a named variable with a brace-enclosed list of expressions or nested lists (braced-init-list) 2) initialization of an unnamed temporary with a braced-init-list. 3) initialization of an object with dynamic storage duration with a new-expression, where ...
List-initializing std::initializer_list. An object of type std::initializer_list<E> is constructed from an initializer list as if the compiler generated and materialized (since C++17) a prvalue of type "array of Nconst E ", where N is the number of elements in the initializer list; this is called the initializer list's backing array .
Initializer List is used in initializing the data members of a class. The list of members to be initialized is indicated with constructor as a comma-separated list followed by a colon. Following is an example that uses the initializer list to initialize x and y of Point class. Example. C++.
A braced-init-list is used to list-initialize an object, where the corresponding constructor accepts an std::initializer_list<T> parameter; A braced-init-list is used as the right operand of assignment or as a function call argument, and the corresponding assignment operator/function accepts an std::initializer_list<T> parameter
This program produces the following output: Foo(6, 7) constructed Foo(6, 7) When foo is instantiated, the members in the initialization list are initialized with the specified initialization values. In this case, the member initializer list initializes m_x to the value of x (which is 6), and m_y to the value of y (which is 7).Then the body of the constructor runs.
An object of type std::initializer_list<T> is a lightweight proxy object that provides access to an array of objects of type T.. A std::initializer_list object is automatically constructed when: . a braced-init-list is used in list-initialization, including function-call list initialization and assignment expressions (not to be confused with constructor initializer lists)
Constructor is a special non-static member function of a class that is used to initialize objects of its class type.. In the definition of a constructor of a class, member initializer list specifies the initializers for direct and virtual base subobjects and non-static data members. ( Not to be confused with std::initializer_list) . Syntax. Constructors are declared using member function ...
It is trivial to allow initializer lists as the right-hand operand of binary operators, in subscripts, and similar isolated parts of the grammar. The real problem is to allow ;a={1,2}+b; as an assignment-statement without also allowing ;{1,2}+b;. We suspect that allowing initializer lists as right-hand, but nor [sic] as left-hand arguments to ...
1) Copy assignment operator. Replaces the contents with a copy of the contents of other . If std::allocator_traits<allocator_type>::propagate_on_container_copy_assignment::value is true, the allocator of *this is replaced by a copy of other. If the allocator of *this after assignment would compare unequal to its old value, the old allocator is ...
Before the compound statement that forms the function body of the constructor begins executing, initialization of all direct bases, virtual bases, and non-static data members is finished. Member initializer list is the place where non-default initialization of these objects can be specified. For members that cannot be default-initialized, such ...