Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
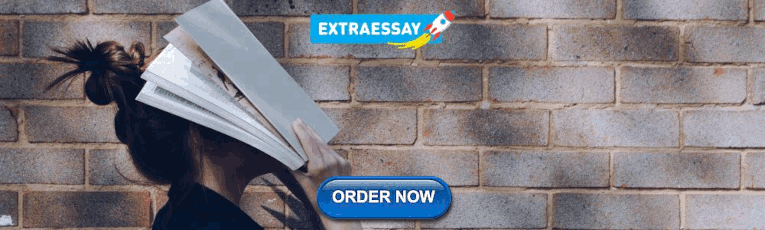
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Source code for the 2022-23 AP Computer Science A course on Project Stem.
ricky8k/APCSA-ProjectStem
Folders and files, repository files navigation.

APCSA Project Stem
Source code for the 2022-23 AP Computer Science A course on Project Stem. Browse the source code »
This repository contains the source code to various problems on Project Stem. Organized by unit, you will find the necessary activity files to be compiled by the Java environment, as well as runner files provided by Project Stem to test execution (when available).
The provided source code is intended to work with the 2023 AP CS A course. These solutions may grow out-of-date as new changes are made to the course every year.
The user-friendly website front-end found here is built using Retype .
Table of Contents
- Unit 1: Primitive Types
- Unit 2: Using Objects
- Unit 3: Boolean Expressions and If Statements
- Unit 4: Iteration
- Unit 5: Writing Classes
- Unit 6: Array
- Unit 7: ArrayList
- Unit 8: 2D Array
- Unit 9: Inheritance
- Unit 10: Recursion
📝 Contributing
Notice a typo or error? Feel free to create an issue !
Please note that support will not be provided for code that does not work in newer lessons. As such, the source code will not be updated to reflect newer curricula.
This repository is licensed under GPL 3.0 .
Contributors 2
- Java 100.0%
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
Intro to computer science - Python
This course is under construction, unit 1: computational thinking with variables, unit 2: designing algorithms with conditionals, unit 3: simulating phenomena with loops, unit 4: playing games with functions.
Interested in a verified certificate or a professional certificate ?
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and “debug” it. Designed for students with or without prior programming experience who’d like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals and Boolean expressions; and loops. Learn how to handle exceptions, find and fix bugs, and write unit tests; use third-party libraries; validate and extract data with regular expressions; model real-world entities with classes, objects, methods, and properties; and read and write files. Hands-on opportunities for lots of practice. Exercises inspired by real-world programming problems. No software required except for a web browser, or you can write code on your own PC or Mac.
Whereas CS50x itself focuses on computer science more generally as well as programming with C, Python, SQL, and JavaScript, this course, aka CS50P, is entirely focused on programming with Python. You can take CS50P before CS50x, during CS50x, or after CS50x. But for an introduction to computer science itself, you should still take CS50x!
How to Take this Course
Even if you are not a student at Harvard, you are welcome to “take” this course for free via this OpenCourseWare by working your way through the course’s ten weeks of material. If you’d like to submit the course’s problem sets and final project for feedback, be sure to create an edX account , if you haven’t already. Ask questions along the way via any of the course’s communities !
- If interested in a verified certificate from edX , enroll at cs50.edx.org/python instead.
- If interested in a professional certificate from edX , enroll at cs50.edx.org/programs/python (for Python) or cs50.edx.org/programs/data (for Data Science) instead.
How to Teach this Course
If you are a teacher, you are welcome to adopt or adapt these materials for your own course, per the license .

CS-IP-Learning-Hub
Important Questions and Notes
100 Important Python Fundamentals Practice Questions
Python fundamentals practice questions.

Table of Contents
- Python Fundamentals Practice Questions – Test 1
Python Fundamentals Practice Questions – Test 2
Python fundamentals practice questions – test 3, python fundamentals practice questions – test 4.
- Python Fundamentals Practice Questions – Test 5
- Python Fundamentals Practice Questions – Test 6
- Python Fundamentals Practice Questions – Test 7
- Python Fundamentals Practice Questions – Test 8
- Python Fundamentals Practice Questions – Test 9
- Python Fundamentals Practice Questions – Test 10
Python Fundamentals Practice Questions – Test 1
Q. Name two modes of Python.
Show Answer Ans. Interactive Mode and Script Mode
Q2. Write Full Form of IDLE
Show Answer Ans. Integrated Development Learning Environment
Q3. Interface mode of python is also known as _______________.
Show Answer Ans. Python Shell
Q4. In which mode we get result immediately after executing the command?
Show Answer Ans. Interactive mode
Q5. Write the output of the following.
Show Answer Ans
Q6. Write one drawback of interactive mode.
Show Answer Ans. We can not save our commands
Q7. Ananya purchased 5 pencils and 2 erasers at the cost of Rs 7 and Rs 5 respectively. Write the program to calculate & display the total amount paid by ananya.
Show Answer Ans.
Q8. What do you mean by comments in Python?
Show Answer Ans. Non executable lines are called Comments
Q9. Which symbols are used for single line comments and multiple line comments?
Show Answer Ans. # symbol
Q10. What do you mean by variable?
Show Answer Ans. Named storage location of value is called variable
Q1. What is the purpose of creating variables? Show Answer Ans. Variables are used to store values which we can use later in our programs. Q2. Write code to find the address of variable. Show Answer Ans. id command is used to find the address of variable for example, to find the address of variable ‘x’ code is >>>id(x) Q3. What do you mean by data type? Show Answer Ans. Data type refers to the type of value used for example integer, float string etc Q4. Write three numeric data type in python. Show Answer Ans. Integer, Floating Point and Complex Q5. Name three sequential data types in python. Show Answer Ans. List, tuple and String Q6. >>> x = 200 >>> y = 10.5 Write the data type of variable x and y. Show Answer Ans. a. Integer b. Floating point Q7. Data type of variable is according to the value it holds.(T/F) Show Answer Ans. True Q8. Which data type store the combination of real and imaginary numbers? Show Answer Ans. Complex Q9. Write the output of the following: >>> 4.7e7 >>> 3.9e2 Show Answer Ans. 47000000 390 Q10. Which data type return value True or False? Show Answer Ans. Boolean
Q1. Write the output of the following :
- >>> (75 > 2 **5)
- >>> (25 != 5 *2)
Q2. Which operator can be changed in above part (2) so that it returns True?
Show Answer Ans. == in place of !=
Q3. Dictionary is enclosed in ___________ brackets.
Show Answer Ans. Curly braces {}
Q4. What do you mean by keywords in python?
Show Answer Ans. Keywords are reserved words. We can not use keywords for variable name or any other identifier.
Q5. Keywords can be used as variable names (T/F)
Show Answer Ans. False
Q6. Write the code to display all keywords in python.
Show Answer Ans import keyword print(keyword.kwlist)
Q7. Write two keywords which start with capital letters.
Show Answer Ans. True, False
Q8. Write the output of the following
Show Answer Ans. Type Error
Q9. Write the output of the following:
Show Answer Ans. [6, 2, 3 ]
Q10. Name three types of operators in python.
Show Answer Ans. 1. Mathematical Operator 2. Comparison Operator 3. Logical Operator
Python Fundamentals Practice Questions Test 5
Python fundamentals practice questions test 6, python fundamentals practice questions test 7, python fundamentals practice questions test 8, python fundamentals practice questions test 9, python fundamentals practice questions test 10.

120+ MySQL Practice Questions

90+ Practice Questions on List

50+ Output based Practice Questions

100 Practice Questions on String

70 Practice Questions on Loops

120 Practice Questions of Computer Network in Python

70 Practice Questions on if-else

40 Practice Questions on Data Structure
Class 12 Computer Science Sample Paper 2020-2021 .
Class 12 Computer Science Sample Paper Marking Scheme
Class 12 Computer Science Test Series
Leave a Reply Cancel reply
Python Fundamentals
Class 11 - computer science with python sumita arora, multiple choice questions.
Special meaning words of Pythons, fixed for specific functionality are called .......... .
- Identifiers
Names given to different parts of a Python program are .......... .
- Identifiers ✓
Data items having fixed value are called .......... .
Which of the following is/are correct ways of creating strings ?
- name = Jiya
- name = 'Jiya' ✓
- name = "Jiya" ✓
- name = (Jiya)
Which of the following are keyword(s) ?
Which of the following are valid identifiers ?
Which of the following are literals ?
Escape sequences are treated as .......... .
- characters ✓
- none of these
Which of the following is an escape sequence for a tab character ?
Question 10
Which of the following is an escape sequence for a newline character ?
Question 11
Which of the following is not a legal integer type value in Python ?
- Hexadecimal
Question 12
Which of the following symbols are not legal in an octal value ?
Question 13
Value 17.25 is equivalent to
- 0.1725E+2 ✓
Question 14
Value 0.000615 is equivalent to
Question 15
Which of the following is/are expression(s) ?
Question 16
The lines beginning with a certain character, and which are ignored by a compiler and not executed, are called ..........
Question 17
Which of the following can be used to create comments ?
- ''' . . . ''' ✓
Question 18
Which of the following functions print the output to the console ?
Question 19
Select the reserved keyword in Python.
- all of these ✓
Question 20
The input( ) returns the value as .......... type.
- floating point
Question 21
To convert the read value through input( ) into integer type, ..........( ) is used.
Question 22
To convert the read value through input( ) into a floating point number, ..........( ) is used.
Question 23
To print a line a text without ending it with a newline, .......... argument is used with print( )
Question 24
The default separator character of print( ) is ..........
Question 25
To give a different separator with print( ) .......... argument is used.
Fill in the Blanks
A keyword is a reserved word carrying special meaning and purpose.
Identifiers are the user defined names for different parts of a program.
Literals are the fixed values.
Operators are the symbols that trigger some computation or action.
An expression is a legal combination of symbols that represents a value.
Non-executable, additional lines added to a program, are known as comments .
In Python, the comments begin with # character.
Python is a case sensitive language.
The print( ) function prints the value of a variable/expression.
The input( ) function gets the input from the user.
The input( ) function returns the read value as of string type.
To convert an input( )'s value in integer type, int( ) function is used.
To convert an input( )'s value in floating-point type, float( ) function is used.
Strings can be created with single quotes, double quotes and triple quotes.
True/False Questions
Keywords can be used as identifier names. False
The identifiers in Python can begin with an underscore. True
0128 is a legal literal value in Python. False
0x12EFG is a legal literal value in Python. False
0123 is a legal literal value in Python. True
Variables once assigned a value can be given any other value. True
Variables are created when they are first assigned their value. True
Python variables support dynamic typing. True
You can rename a keyword. False
String values in Python can be single line strings, and multi-line strings. True
A variable can contain values of different types at different times. True
Expressions contain values/variables along with operators. True
Type A : Short Answer Questions/Conceptual Questions
What are tokens in Python ? How many types of tokens are allowed in Python ? Examplify your answer.
The smallest individual unit in a program is known as a Token. Python has following tokens:
- Keywords — Examples are import, for, in, while, etc.
- Identifiers — Examples are MyFile, _DS, DATE_9_7_77, etc.
- Literals — Examples are "abc", 5, 28.5, etc.
- Operators — Examples are +, -, >, or, etc.
- Punctuators — ' " # () etc.
How are keywords different from identifiers ?
Keywords are reserved words carrying special meaning and purpose to the language compiler/interpreter. For example, if, elif, etc. are keywords. Identifiers are user defined names for different parts of the program like variables, objects, classes, functions, etc. Identifiers are not reserved. They can have letters, digits and underscore. They must begin with either a letter or underscore. For example, _chk, chess, trail, etc.
What are literals in Python ? How many types of literals are allowed in Python ?
Literals are data items that have a fixed value. The different types of literals allowed in Python are:
- String literals
- Numeric literals
- Boolean literals
- Special literal None
- Literal collections
Can nongraphic characters be used in Python ? How ? Give examples to support your answer.
Yes, nongraphic characters can be used in Python with the help of escape sequences. For example, backspace is represented as \b, tab is represented as \t, carriage return is represented as \r.
How are floating constants represented in Python ? Give examples to support your answer.
Floating constants are represented in Python in two forms — Fractional Form and Exponent form. Examples:
- Fractional Form — 2.0, 17.5, -13.0, -0.00625
- Exponent form — 152E05, 1.52E07, 0.152E08, -0.172E-3
How are string-literals represented and implemented in Python ?
A string-literal is represented as a sequence of characters surrounded by quotes (single, double or triple quotes). String-literals in Python are implemented using Unicode.
Which of these is not a legal numeric type in Python ? (a) int (b) float (c) decimal.
decimal is not a legal numeric type in Python.
Which argument of print( ) would you set for:
(i) changing the default separator (space) ? (ii) printing the following line in current line ?
(i) sep (ii) end
What are operators ? What is their function ? Give examples of some unary and binary operators.
Operators are tokens that trigger some computation/action when applied to variables and other objects in an expression. Unary plus (+), Unary minus (-), Bitwise complement (~), Logical negation (not) are a few examples of unary operators. Examples of binary operators are Addition (+), Subtraction (-), Multiplication (*), Division (/).
What is an expression and a statement ?
An expression is any legal combination of symbols that represents a value. For example, 2.9, a + 5, (3 + 5) / 4. A statement is a programming instruction that does something i.e. some action takes place. For example: print("Hello") a = 15 b = a - 10
What all components can a Python program contain ?
A Python program can contain various components like expressions, statements, comments, functions, blocks and indentation.
What do you understand by block/code block/suite in Python ?
A block/code block/suite is a group of statements that are part of another statement. For example:
What is the role of indentation in Python ?
Python uses indentation to create blocks of code. Statements at same indentation level are part of same block/suite.
What are variables ? How are they important for a program ?
Variables are named labels whose values can be used and processed during program run. Variables are important for a program because they enable a program to process different sets of data.
What do you understand by undefined variable in Python ?
In Python, a variable is not created until some value is assigned to it. A variable is created when a value is assigned to it for the first time. If we try to use a variable before assigning a value to it then it will result in an undefined variable. For example:
The first line of the above code snippet will cause an undefined variable error as we are trying to use x before assigning a value to it.
What is Dynamic Typing feature of Python ?
A variable pointing to a value of a certain type can be made to point to a value/object of different type.This is called Dynamic Typing. For example:
What would the following code do : X = Y = 7 ?
It will assign a value of 7 to the variables X and Y.
What is the error in following code : X, Y = 7 ?
The error in the above code is that we have mentioned two variables X, Y as Lvalues but only give a single numeric literal 7 as the Rvalue. We need to specify one more value like this to correct the error:
Following variable definition is creating problem X = 0281, find reasons.
Python doesn't allow decimal numbers to have leading zeros. That is the reason why this line is creating problem.
"Comments are useful and easy way to enhance readability and understandability of a program." Elaborate with examples.
Comments can be used to explain the purpose of the program, document the logic of a piece of code, describe the behaviour of a program, etc. This enhances the readability and understandability of a program. For example:
Type B: Application Based Questions
From the following, find out which assignment statement will produce an error. State reason(s) too.
(a) x = 55 (b) y = 037 (c) z = 0o98 (d) 56thnumber = 3300 (e) length = 450.17 (f) !Taylor = 'Instant' (g) this variable = 87.E02 (h) float = .17E - 03 (i) FLOAT = 0.17E - 03
- y = 037 ( option b ) will give an error as decimal integer literal cannot start with a 0.
- z = 0o98 ( option c ) will give an error as 0o98 is an octal integer literal due to the 0o prefix and 8 & 9 are invalid digits in an octal number.
- 56thnumber = 3300 ( option d ) will give an error as 56thnumber is an invalid identifier because it starts with a digit.
- !Taylor = 'Instant' ( option f ) will give an error as !Taylor is an invalid identifier because it contains the special character !.
- this variable = 87.E02 ( option g ) will give an error due to the space present between this and variable. Identifiers cannot contain any space.
- float = .17E - 03 ( option h ) will give an error due to the spaces present in exponent part (E - 03). A very important point to note here is that float is NOT a KEYWORD in Python. The statement float = .17E-03 will execute successfully without any errors in Python.
- FLOAT = 0.17E - 03 ( option i ) will give an error due to the spaces present in exponent part (E - 03).
How will Python evaluate the following expression ?
(i) 20 + 30 * 40
20 + 30 * 40 ⇒ 20 + 1200 ⇒ 1220
(ii) 20 - 30 + 40
20 - 30 + 40 ⇒ -10 + 40 ⇒ 30
(iii) (20 + 30) * 40
(20 + 30) * 40 ⇒ 50 * 40 ⇒ 2000
(iv) 15.0 / 4 + (8 + 3.0)
15.0 / 4 + (8 + 3.0) ⇒ 15.0 / 4 + 11.0 ⇒ 3.75 + 11.0 ⇒ 14.75
Find out the error(s) in following code fragments:
The call to print function is missing parenthesis. The correct way to call print function is this: print(temperature)
There are two errors in this code fragment:
- In the statement b=a+b variable b is undefined.
- In the statement print (a And b) , And should be written as and.
In the statement print (a ; b ; c) use of semicolon will give error. In place of semicolon, we must use comma like this print (a, b, c)
The statement 4 = X is incorrect as 4 cannot be a Lvalue. It is a Rvalue.
- Variable X is undefined
- "X =" and X should be separated by a comma like this print("X =", X)
else is a keyword in Python so it can't be used as a variable name.
What will be the output produced by following code fragment (s) ?
Explanation
- X = 10 ⇒ assigns an initial value of 10 to X.
- X = X + 10 ⇒ X = 10 + 10 = 20. So value of X is now 20.
- X = X - 5 ⇒ X = 20 - 5 = 15. X is now 15.
- print (X) ⇒ print the value of X which is 15.
- X, Y = X - 2, 22 ⇒ X, Y = 13, 22.
- print (X, Y) ⇒ prints the value of X which is 13 and Y which is 22.
- first = 2 ⇒ assigns an initial value of 2 to first.
- second = 3 ⇒ assigns an initial value of 3 to second.
- third = first * second ⇒ third = 2 * 3 = 6. So variable third is initialized with a value of 6.
- print (first, second, third) ⇒ prints the value of first, second, third as 2, 3 and 6 respectively.
- first = first + second + third ⇒ first = 2 + 3 + 6 = 11
- third = second * first ⇒ third = 3 * 11 = 33
- print (first, second, third) ⇒ prints the value of first, second, third as 11, 3 and 33 respectively.
- side = int(input('side') ) ⇒ This statements asks the user to enter the side. We enter 7 as the value of side.
- area = side * side ⇒ area = 7 * 7 = 49.
- print (side, area) ⇒ prints the value of side and area as 7 and 49 respectively.
What is the problem with the following code fragments ?
The problem with the above code is inconsistent indentation. The statements print(a) , print(b) , print(s) are indented but they are not inside a suite. In Python, we cannot indent a statement unless it is inside a suite and we can indent only as much is required.
In the print statement we are trying to add name which is a string to age which is an integer. This is an invalid operation in Python.
The statement a = "New" converts a to string type from numeric type due to dynamic typing. The statement q = a / 10 is trying to divide a string with a number which is an invalid operation in Python.
Predict the output:
- x = 40 ⇒ assigns an initial value of 40 to x.
- y = x + 1 ⇒ y = 40 + 1 = 41. So y becomes 41.
- x = 20, y + x ⇒ x = 20, 41 + 40 ⇒ x = 20, 81. This makes x a Tuple of 2 elements (20, 81) .
- print (x, y) ⇒ prints the tuple x and the integer variable y as (20, 81) and 41 respectively.
- x, y = 20, 60 ⇒ assigns an initial value of 20 to x and 60 to y.
- y, x, y = x, y - 10, x + 10 ⇒ y, x, y = 20, 60 - 10, 20 + 10 ⇒ y, x, y = 20, 50, 30 First RHS value 20 is assigned to first LHS variable y. After that second RHS value 50 is assigned to second LHS variable x. Finally third RHS value 30 is assigned to third LHS variable which is again y. After this assignment, x becomes 50 and y becomes 30.
- print (x, y) ⇒ prints the value of x and y as 50 and 30 respectively.
- a, b = 12, 13 ⇒ assigns an initial value of 12 to a and 13 to b.
- c, b = a*2, a/2 ⇒ c, b = 12*2, 12/2 ⇒ c, b = 24, 6.0. So c has a value of 24 and b has a value of 6.0.
- print (a, b, c) ⇒ prints the value of a, b, c as 12, 6.0 and 24 respectively.
- print (print(a + b)) ⇒ First print(a + b) function is called which prints 25. After that, the outer print statement prints the value returned by print(a + b) function call. As print function does not return any value so outer print function prints None.
Predict the output
- a, b, c = 10, 20, 30 ⇒ assigns initial value of 10 to a, 20 to b and 30 to c.
- p, q, r = c - 5, a + 3, b - 4 ⇒ p, q, r = 30 - 5, 10 + 3, 20 - 4 ⇒ p, q, r = 25, 13, 16. So p is 25, q is 13 and r is 16 .
- print ('a, b, c :', a, b, c, end = '') ⇒ This statement prints a, b, c : 10 20 30 . As we have given end = '' so output of next print statement will start in the same line.
- print ('p, q, r :', p, q, r) ⇒ This statement prints p, q, r : 25 13 16
Find the errors in following code fragment
- x is undefined in the statement y = x + 5
- Y is undefined in the statement print (x, Y) . As Python is case-sensitive hence y and Y will be treated as two different variables.
Python doesn't allow assignment of variables while they are getting printed.
The input( ) function always returns a value of String type so variable a is a string. This statement b = a/2 is trying to divide a string with an integer which is invalid operation in Python.
Find the errors in following code fragment : (The input entered is XI)
The input value XI is not int type compatible.
Consider the following code :
was intended to print output as
But it is printing the output as :
What could be the problem ? Can you suggest the solution for the same ?
The print() function appends a newline character at the end of the line unless we give our own end argument. Due to this behaviour of print() function, the statement print ('Hi', name, ',1) is printing a newline at the end. Hence "How are you doing?" is getting printed on the next line. To fix this we can add the end argument to the first print() function like this:
Find the errors in following code fragment :
- c - 1 is outside the parenthesis of print function. It should be specified as one of the arguments of print function.
- c is a string as input function returns a string. With c - 1 , we are trying to subtract a integer from a string which is an invalid operation in Python.
The corrected program is like this:
What will be returned by Python as result of following statements?
(a) >>> type(0)
<class 'int'>
(b) >>> type(int(0))
(c) >>>.type(int('0')
SyntaxError: invalid syntax
(d) >>> type('0')
<class 'str'>
(e) >>> type(1.0)
<class 'float'>
(f) >>> type(int(1.0))
(g) >>>type(float(0))
(h) >>> type(float(1.0))
(i) >>> type( 3/2)
What will be the output produced by following code ?
(a) >>> str(print())+"One"
print() function doesn't return any value so its return value is None. Hence, str(print()) becomes str(None) . str(None) converts None into string 'None' and addition operator joins 'None' and 'One' to give the final output as 'NoneOne'.
(b) >>> str(print("hello"))+"One"
hello NoneOne
First, print("hello") function is executed which prints the first line of the output as hello. The return value of print() function is None i.e. nothing. str() function converts it into string and addition operator joins 'None' and 'One' to give the second line of the output as 'NoneOne'.
(c) >>> print(print("Hola"))
First, print("Hola") function is executed which prints the first line of the output as Hola. The return value of print() function is None i.e. nothing. This is passed as argument to the outer print function which converts it into string and prints the second line of output as None.
(d) >>> print (print ("Hola", end = " "))
First, print ("Hola", end = " ") function is executed which prints Hola. As end argument is specified as " " so newline is not printed after Hola. The next output starts from the same line. The return value of print() function is None i.e. nothing. This is passed as argument to the outer print function which converts it into string and prints None in the same line after Hola.
Carefully look at the following code and its execution on Python shell. Why is the last assignment giving error ?
Due to the prefix 0o, the number is treated as an octal number by Python but digit 8 is invalid in Octal number system hence we are getting this error.
- a, b, c = 2, 3, 4 ⇒ assigns initial value of 2 to a, 3 to b and 4 to c.
- a, b, c = a*a, a*b, a*c ⇒ a, b, c = 2*2, 2*3, 2*4 ⇒ a, b, c = 4, 6, 8
- print(a, b, c) ⇒ prints values of a, b, c as 4, 6 and 8 respectively.
The id( ) can be used to get the memory address of a variable. Consider the adjacent code and tell if the id( ) functions will return the same value or not(as the value to be printed via print() ) ? Why ? [There are four print() function statements that are printing id of variable num in the code shown on the right.
For the print statements commented as print 1 and print 3 above, the id() function will return the same value. For print 2 and print 4, the value returned by id() function will be different.
The reason is that for both print 1 and print 3 statements the value of num is the same which is 13. So id(num) gives the address of the memory location which contains 13 in the front-loaded dataspace.
Consider below given two sets of codes, which are nearly identical, along with their execution in Python shell. Notice that first code-fragment after taking input gives error, while second code-fragment does not produce error. Can you tell why ?
In part a, the value entered by the user is converted to a float type and passed to the print function by assigning it to a variable named num. It means that we are passing an argument named num to the print function. But print function doesn't accept any argument named num. Hence, we get this error telling us that num is an invalid argument for print function.
In part b, we are converting the value entered by the user to a float type and directly passing it to the print function. Hence, it works correctly and the value gets printed.
Predict the output of the following code :
If the input given is in this order : 1, 2, 3, 4
What will the following code result into ?
The code will result into an error as in the statement n4 = n4 + 2 , variable n4 is undefined.
Correct the following program so that it displays 33 when 30 is input.
Below is the corrected program:
Type C : Programming Practice/Knowledge based Questions
Write a program that displays a joke. But display the punchline only when the user presses enter key. (Hint. You may use input( ))
Write a program to read today's date (only del part) from user. Then display how many days are left in the current month.
Write a program that generates the following output : 5 10 9 Assign value 5 to a variable using assignment operator (=) Multiply it with 2 to generate 10 and subtract 1 to generate 9.
Modify above program so as to print output as 5@10@9.
Write the program with maximum three lines of code and that assigns first 5 multiples of a number to 5 variables and then print them.
Write a Python program that accepts radius of a circle and prints its area.
Write Python program that accepts marks in 5 subjects and outputs average marks.
Write a short program that asks for your height in centimetres and then converts your height to feet and inches. (1 foot = 12 inches, 1 inch = 2.54 cm).
Write a program to read a number n and print n 2 , n 3 and n 4 .
Write a program to find area of a triangle.
Write a program to compute simple interest and compound interest.
Write a program to input a number and print its first five multiples.
Write a program to read details like name, class, age of a student and then print the details firstly in same line and then in separate lines. Make sure to have two blank lines in these two different types of prints.
Write a program to input a single digit(n) and print a 3 digit number created as <n(n + 1)(n + 2)> e.g., if you input 7, then it should print 789. Assume that the input digit is in range 1-7.
Write a program to read three numbers in three variables and swap first two variables with the sums of first and second, second and third numbers respectively.
ICSE/ISC Textbook Solutions Class - 6 Concise Biology Selina Solutions Class - 6 Veena Bhargava Geography Solutions Class - 6 Effective History & Civics Solutions Class - 6 APC Understanding Computers Solutions Class - 7 Concise Physics Selina Solutions Class - 7 Concise Chemistry Selina Solutions Class - 7 Dalal Simplified Middle School Chemistry Solutions Class - 7 Concise Biology Selina Solutions Class - 7 Living Science Biology Ratna Sagar Solutions Class - 7 Around the World Geography Solutions Class - 7 Veena Bhargava Geography Solutions Class - 7 Effective History & Civics Solutions Class - 7 APC Understanding Computers Solutions Class - 8 Concise Physics Selina Solutions Class - 8 Concise Chemistry Selina Solutions Class - 8 Dalal Simplified Middle School Chemistry Solutions Class - 8 Concise Biology Selina Solutions Class - 8 Living Science Biology Ratna Sagar Solutions Class - 8 Around the World Geography Solutions Class - 8 Veena Bhargava Geography Solutions Class - 8 Effective History & Civics Solutions Class - 8 APC Understanding Computers Solutions Class - 8 Kips Logix Computers Solutions Class - 9 Concise Physics Selina Solutions Class - 9 Concise Chemistry Selina Solutions Class - 9 Dalal Simplified ICSE Chemistry Solutions Class - 9 Concise Biology Selina Solutions Class - 9 Total Geography Morning Star Solutions Class - 9 Veena Bhargava Geography Solutions Class - 9 Total History & Civics Solutions Class - 9 APC Understanding Computers Solutions Class - 9 Kips Logix Computers Solutions Class - 10 ML Aggarwal Mathematics Solutions Class - 10 Concise Physics Selina Solutions Class - 10 Concise Chemistry Selina Solutions Class - 10 Dalal Simplified ICSE Chemistry Solutions Class - 10 Concise Biology Selina Solutions Class - 10 Total Geography Morning Star Solutions Class - 10 Veena Bhargava Geography Solutions Class - 10 Total History & Civics Solutions Class - 10 APC Modern History & Civics Solutions Class - 10 APC Understanding Computers Solutions Class - 10 Sumita Arora ICSE Computers Solutions Class - 10 Kips Logix Computers Solutions Class - 11 APC Understanding Computers Solutions Class - 12 APC Understanding Computers Solutions ICSE/ISC SOLVED QUESTION PAPERS ICSE Class 10 Computers Solved 10 Yrs Question Papers Sample Papers ICSE Class 10 Computer Applications ICSE Class 10 Physics Solved 10 Yrs Question Papers Sample Papers ICSE Class 10 Physics ICSE Class 10 Chemistry Solved 10 Yrs Question Papers Sample Papers ICSE Class 10 Chemistry ICSE Class 10 Biology Solved 10 Yrs Question Papers Sample Papers ICSE Class 10 Biology Class - 12 ISC Computer Science Solved Practical Papers Class - 10 CBSE Computer Applications Solved Question Papers Class - 10 CBSE Computer Applications Solved Sample Papers Class - 10 CBSE Science Solved Question Papers Class - 12 CBSE Computer Science Solved Question Papers STUDYLIST Java Pattern Programs Java Series Programs Java Number Programs (ICSE Classes 9 / 10) Java Number Programs (ISC Classes 11 / 12) Output Questions for Class 10 ICSE Computer Applications Algorithms & Flowcharts for ICSE Computers ICSE Class 8 Computers Differentiate Between the Following CBSE Textbook Solutions Class - 8 NCERT Science Solutions Class - 9 NCERT Science Solutions Class - 9 NCERT Geography Contemporary India 1 Solutions Class - 9 Sumita Arora Computer Code 165 Solutions Class - 9 Kips Cyber Beans Computer Code 165 Solutions Class - 10 NCERT Mathematics Solutions Class - 10 NCERT Science Solutions Class - 10 NCERT Geography Contemporary India 2 Solutions Class - 10 NCERT History India & Contemporary World 2 Solutions Class - 10 Sumita Arora Computer Code 165 Solutions Class - 10 Kips Cyber Beans Computer Code 165 Solutions Class - 11 CBSE Sumita Arora Python Solutions Class - 12 CBSE Sumita Arora Python Solutions Class - 12 NCERT Computer Science Solutions Company About Us Contact Us Privacy Policy Terms of Service Copyright © KnowledgeBoat 2024
- New Sandbox Program
Click on one of our programs below to get started coding in the sandbox!
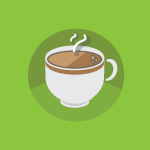
Introduction to Computer Science in Python
This course teaches the fundamentals of computer programming as well as some advanced features of the Python 3 language. By the end of this course, students build a simple console-based game and learn material equivalent to a semester college introductory Python course.

Overview & Highlights
Course overview.
To view the entire syllabus, click here or click to explore the full course .
Demo Programs
Explore programs that your students will build throughout this course!
- Python Console Exercise: Librarian
- Project: Guess the Word
- Supplemental Tracy Challenge: Pizza
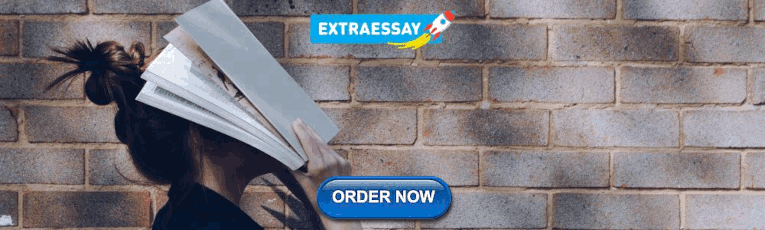
Course Resources
Here are a few examples of teacher resources and materials to use in the Introduction to Computer Science in Python course
- Sample Handout (teacher): Lesson 2.4
- Sample Handout (student): Lesson 2.4
- Lesson Plan: 2.1
Industry-Relevant Certifications for High School Computer Science
The CodeHS Python Level 1 Certification offers high school students the opportunity to validate their mastery of Python, giving them a competitive advantage when entering college or the workforce.
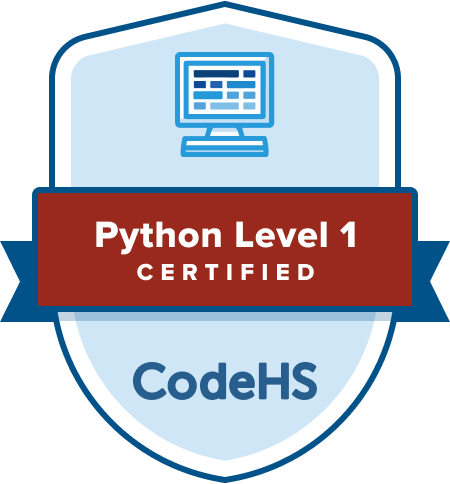
- Professional Development
Teaching Intro to Computer Science in Python 3
Introduction to Computer Science in Python is aligned with the following standards
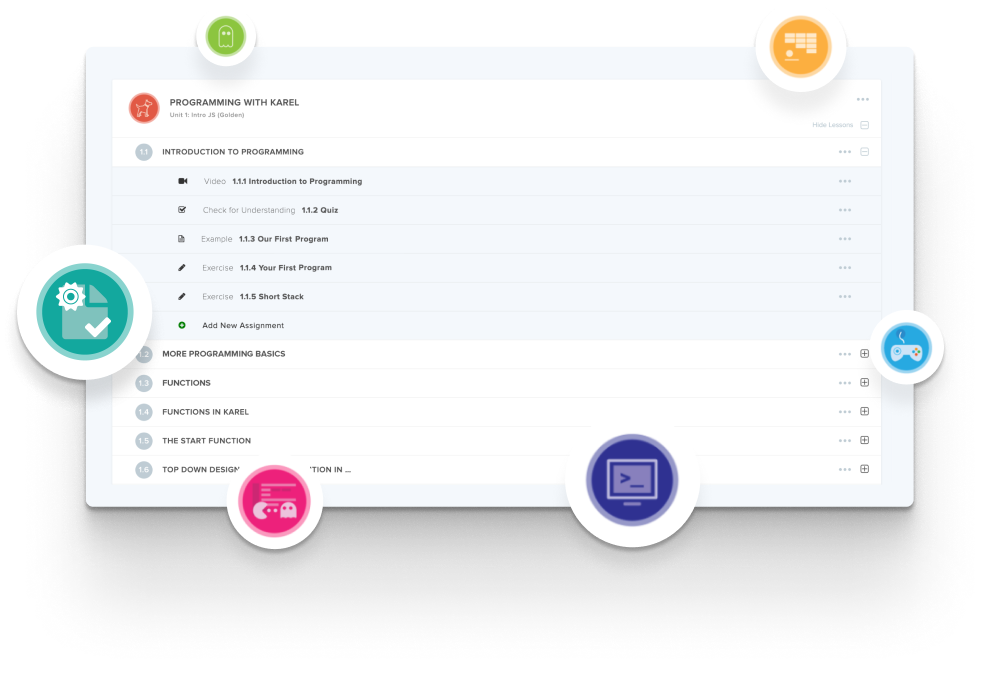
Customizable Assignments
Create and organize Assignments in any CodeHS course that you're teaching. You can even add custom assignments to pre-existing CodeHS courses.
Actions for Teachers
Didn’t find what you were looking for? Here are a few links that might be useful to you.
- Explore Course
- Video Tutorials (Youtube)
You also might like
Related Courses
Which python course should I use? Click here
Web Design (Picasso) [2022]
Semester course that covers the fundamentals including the Internet, HTML, CSS, and Bootstrap concepts.
- Level High School
- Contact Hours 140
- Timeframe Year
Introduction to Python Programming
Introductory course covering the fundamentals of the Python language
- Contact Hours 120
- Contact Hours 175
Interested in teaching with CodeHS?
Get in touch to learn how to bring codehs to your school.
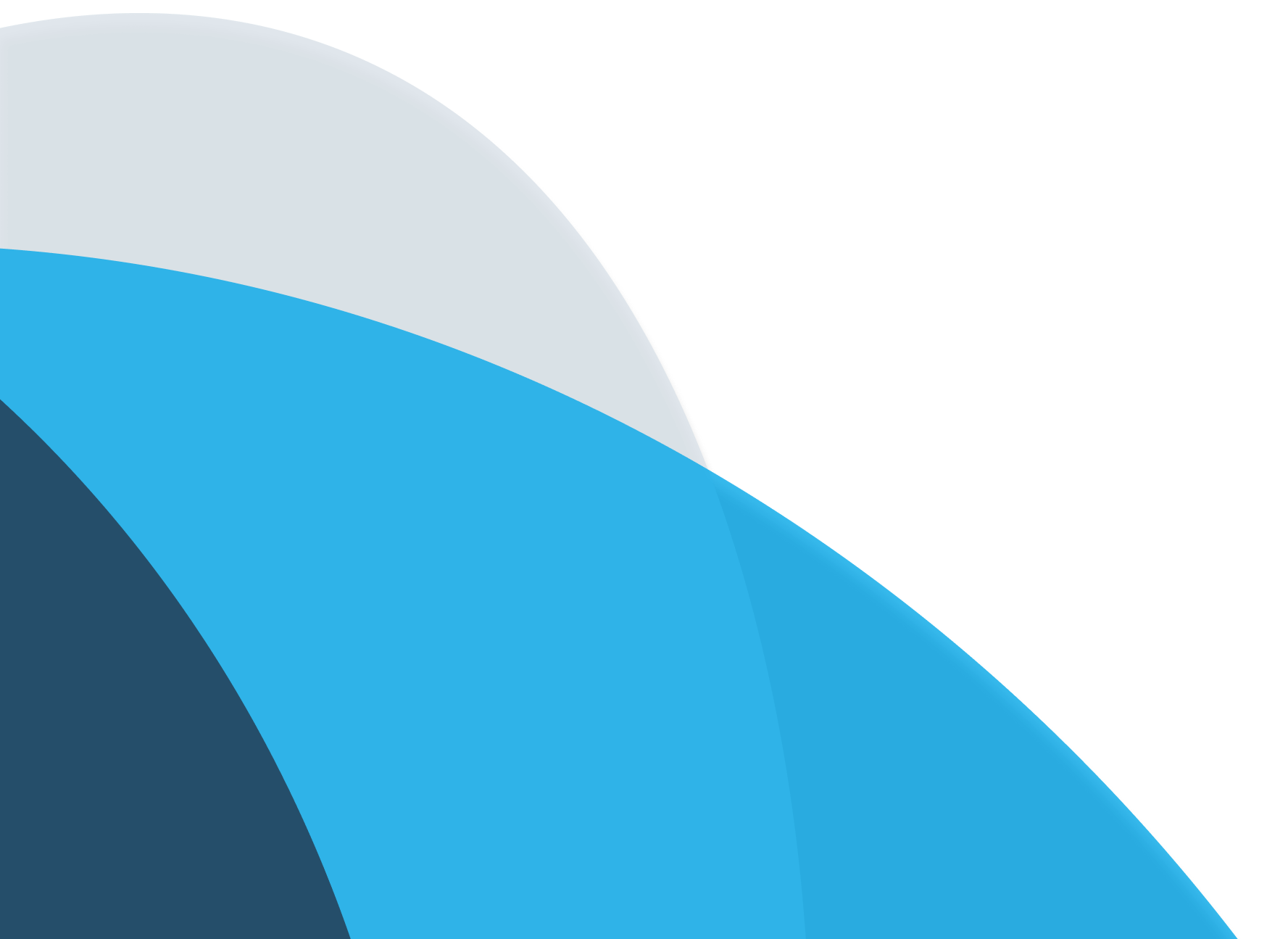
- Computer Science Curriculum
- Certifications
- Assignments
- Classroom Management
- Integrations
- Course Catalog
- Project Catalog
- K-12 Pathways
- State Courses
- Spanish Courses
- Hour of Code
- Digital Textbooks
- Online PD Courses
- In-Person PD Workshops
- Virtual PD Workshops
- Free PD Workshops
- Teacher Certification Prep
- Microcredentials
- PD Membership
Programming Languages
- Case Studies
- Testimonials
- Read Write Code Blog
- Read Write Code Book
- Knowledge Base
- Student Projects
- Career Center
- Privacy Center
- Privacy Policy
- Accessibility

Welcome to Python Programming Fundamentals Second Edition by Kent D. Lee. This text, available from Springer , is an introductory computer programming text. The text is oriented towards students who have not taken any other programming course previously. However, it can be used by students with some programming skills in another language. If you would like to begin learning Python programming on your own, you can begin by installing Python and Wing IDE 101 on your computer. Then buy my book and begin working through the many practice exercises and examples in the text. The links to the two YouTube videos below show you how you can install Python and Wing IDE 101 on your computer.
Installing Python and Wing IDE 101
I hope you enjoy reading the text and learning to program a computer using Python! It’s creative, fun, and the skills you will learn could provide you with access to a very fullfilling and rewarding career in Computer Science! Give it a try!
Errata and Suggestions ¶
I hope both professors and students enjoy using the text! I hope to get constructive feedback from folks so if you find an error or have a suggestion don’t hesitate to email me. My email is kentdlee at luther.edu. Please contact me via email if you have errata or suggestions and I will post them here.
On page 22. The sentence containing “Dividing 83/2 yields 41.5 if it is written 81/2” should be “… if it is written 83/2”.
Instructor Support Materials ¶
If you are an instructor using or considering using the text to teach a course and would like lecture slides, solutions to exercises, and answers to review questions I would be happy to provide them. Detailed lecture slides are available with all examples and practice problems from the text as well as solutions to all the exercises and review questions from the text. Please email me and provide me with information about where you are teaching and a web site where I can verify that you are a teacher at your institution and I will direct you to these support materials.
Support Files for Individual Chapters ¶
The following sections contain support files, instructional videos, and additional information for individual chapters. The support files may be downloaded by students or faculty to complete projects from each of the chapters below.
The sections below also contain videos for the various topics presented in the text. These videos help to reinforce the topics discussed in the text. I would recommend that you watch the video and then read the section of the text that goes along with the video for a more detailed explanation of the concepts in that section.
In addition, there are practice exercises in each section of the text (with answers at the end of the chapter) that you can use to test your understanding material. Use these exercises to motivate your reading of the text.
Chapter 1 ¶
The first chapter is about 30 pages long and contains a variety of learning objectives. This is perhaps the most broadly focused of the chapters in the text. Its goal is to give you some basis for talking about computer programming and to give you a gentle introduction to the process of writing programs. Specifically, the learning objectives of the first chapter are outlined below.
Section 1.3 - In this section of the text you use the Wing IDE to write a simple program. You learn the steps that you must take to open the Wing IDE, enter a program, and run it. Section 1.4 - In this section you learn a little about the architecture of a computer and some general terms to use when talking about computer programs. Sections 1.5 to 1.7 Part 1 - Covers conversion to binary. Sections 1.5 to 1.7 Part 2 - Signed and unsigned binary number representation is discussed. Sections 1.5 to 1.7 Part 3 - A first algorithm is presented and discussed. Sections 1.5 to 1.8 Part 1 - In these sections of the text, you learn about the importance of types in our programs and ASCII representation of characters. Sections 1.5 to 1.8 Part 2 - In this video you learn to use an assignment statement in Python to store a value in RAM and retrieve it later. Sections 1.9 and 1.17 - In section 1.9 and 1.17 you learn what a syntax error is, how to recognize it in your program, and how to fix it. Sections 1.10 to 1.14 - In this part of the text you learn about references, types, and type conversion. Sections 1.15 & 1.16 - Here you practice with strings and getting input from the user and printing output.
Chapter 2 ¶
In chapter 2 you learn how to make decisions in your programs using if statements. An if statements alters the sequence of executed statements depending on a condition. You also learn about the guess and check pattern of computation. This pattern is important to learn and memorize because you use it over and over in programs you write. Learning objectives for this chapter are outlined below.
Sections 2.1 & 2.2 - Here you learn about the guess and check pattern of computation. You can watch this video that goes along with these chapters. Sections 2.3, 2.4, & 2.5 - In these sections you learn about choosing from a list of alternatives, Boolean values, and short-circuit logic. The video below helps to further explain these concepts. Section 2.6 - In this section we learn that floating point numbers are only approximations of real numbers and therefore we must take some care when comparing floats for equality. It is likely that two floats will be close to the same value, but not exactly the same value, even when we would like them to be equal. The video below helps to illustrate this problem and the solution to it.
Chapter 2 Support Files ¶
In exercise 2 of chapter 3 the problem asks you to write a menu driven address book program. You can use this text file as the data file for your address book or you can create one yourself. Just be sure to use the same format as is presented in the book for your records within the file.
Chapter 3 ¶
In chapter 3 you learn how to repeatedly execute code in programs so you can process repetitive data. This is where computers really are important, since people don’t like to do the same thing over and over again. Computers are good at doing the same thing over and over. Again, there are video lectures below that complement the text.
Sections 3.1 & 3.2 - You should read the introduction to chapter 3 as well. In these two sections you learn about sequences and how to iterate over them. Section 3.3 - In this section you learn about nested for loops. Nested for loops are when you have one loop inside another loop. Section 3.4 - In this section of the text you learn how to use the guess and check pattern when a list is involved. Sections 3.5 & 3.6 - In these sections of the text you learn about the accumulator pattern and how to use it to count or add together a list of numbers.
Chapter 4 ¶
In chapter 4 you learn about objects and how to create objects and call methods on them. The chapter uses turtle graphics and XML parsing as two examples where many objects are created and methods are called.
Sections 4.1 to 4.3 - The introduction and these sections of the text show you how to use modules in Python and how to create some objects. This video complements these sections of the text. Plotting Data - In this video you learn how to use turtle graphics to plot data that you read from a file. In this video I read the file called djia-100.txt . This file is provided here for you to download if you want to try out my example. Section 4.8 - In this section of the text you learn about dictionaries in Python. It shows you how to create a dictionary, put a key/value pair in dictionary, and how to lookup a key in a dictionary to get its value. The video below demonstrates these concepts as well. Sections 4.6 to 4.9 - In these sections of the text you learn how to read and parse through the information in an XML file. XML documents are used in many different applications these days and understanding what an XML document is and how to read it in a program is a valuable skill. The video below helps to illustrate these ideas. This video use an XML file called kml.kml as an example. That file can be downloaded here if you want to try my example. Section 4.7 - In this section of the text I show you how to get at the attributes in an XML element. The attributes are recorded as a dictionary so you’ll want to know what a dictionary is first (see section 4.8 and its video above). In the video that goes along with this section I read a couple of XML files called biking3-15-2012.tcx and workouts.tcx . You can download these files here if you want to try my examples, but these files are large and a little unwieldy. You might start with some smaller XML files first (see the exercises at the end of the chapter in the text).
Support Files ¶
In chapter 4 there are several programming problems that must read data from a file. In a few cases, some code is provided to get you started. Each exercise’s extra files are provided below.
Exercises 2, 3, and 4 require you to read an XML file containing a picture. The XML file can be generated by using this drawing program , written in Python. You can download this program and run it with Python 3 to be able to draw a picture and save it to an XML file. The XML file for the picture in the text (Fig. 4.4 page 99) can be found here as flowerandbg.xml . Exercises 5 and 6 use mileage and fuel data from a 2007 Toyota 4Runner, a 2007 Nissan Versa, and a 2007 Suzuki S40 motorcycle. These two programs require you to read one of these data files below. This data was exported from a program called Gas Cubby that is available as an iPhone/iPod Touch App. Toyota4Runner.csv - Data for gas mileage for a 2007 Toyota 4Runner. NissanVersa.csv - Data for gas mileage for a 2009 Nissan Versa. SuzukiS40.csv - Data for gas mileage for a 2007 Suzuki S40 Motorcycle.
Chapter 5 ¶
Chapter 5 introduces you to defining and calling functions of your own. Functions offer us a means of abstracting away details and concentrating on what a function does instead of how it does it.
Defining and calling functions is discussed in this video and in sections 5.1 and 5.2 of the text. Functions and Scope are described in section 5.3 of the text and this video lecture. The Run-time Stack is described in this video lecture and section 5.4 of the text. Bottom-up Design is described in section 5.8 of the text and this video lecture. The Main Function is described at the end of chapter 5 and in this video lecture.
Chapter 6 ¶
Chapter 6 introduces Tkinter programming through an example Reminder application. Documentation for the Tkinter API can be found here. You can download the Reminder.py code from the example in that chapter here. Exercise 4 requires you to create an XML file to hold the reminder notes when the program is terminated and for the program to read when the application is started. You can use the format found below for your XML file. Name the file “Reminders.xml” and place the xml file in the same directory or folder as your program. You can begin this exercise by downloading the Reminder.py program from the link above and then modifying the code to read from an XML file using the minidom parser described in chapter 4. You can write to a file using Python’s file write method like the code in Reminder.py already does. Of course, you need to modify the code to write the file in this XML format so it can be read by the minidom parser.
One thing to be aware of: When using double quotes (i.e. “) in strings, you can you single quotes (i.e. ‘) to delimit those strings. Python lets you use either double or single quotes to delimit strings and this is one instance where using single quotes will come in handy.
One other thing to be aware of: Sometimes exception handling code (i.e. try except statements) get in the way of debugging your code. You may want to remove (or just comment out) the try except statements in the Reminders.py program before making modifications to it so you can see what errors occur when you make the modifications for this assignment. You should put the try except statements back in once you have debugged your code.
There is one video lecture for chapter 6 that goes along with the material in all of chapter 6 and teaches you about tkinter programming.
You can find the Reminder Application here as it is described in chapter 6 of the text.
Chapter 7 ¶
In chapter 7 there are two sample programs that are developed to demonstrate object-oriented programming and inheritance using Turtle graphics.
There are two video lectures that go along with this chapter.
Object-Oriented Programming and creating and using classes in described in this video. Inheritance is described in this lecture and the reuse of code through inheritance.
The first sample program is a drawing application. The source code for the drawing program can be downloaded here . The second example is a bouncing ball program and that code can be downloaded here. The bouncing ball program needs the soccerball.gif file to run. You might also like to make kitties bounce around, so kitty.gif is also provided. Chapter 7 includes an exercise on developing an asteroids application. There are five lessons that will take you through developing this application. The five lessons below can be followed to implement the basic asteroids application. As part of the assignment in the text, you are to implement one more level to the application when level 1 is completed. Lesson 1 Lesson 2 Lesson 3 Lesson 4 Lesson 5 In addition to the exercises at the end of chapter 7 I have had students implement a minesweeper game . This web page provides a sample as a compiled Python program and provides students with directions for completing the application from the starter code that is provided to them.
Table of Contents
Browse Course Material
Course info, instructors.
- Dr. Ana Bell
- Prof. Eric Grimson
- Prof. John Guttag
Departments
- Electrical Engineering and Computer Science
As Taught In
- Algorithms and Data Structures
- Programming Languages
Learning Resource Types
Introduction to computer science and programming in python, course description.
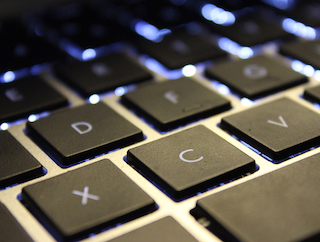
You are leaving MIT OpenCourseWare
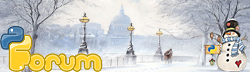
- View Active Threads
- View Today's Posts
- View New Posts
- My Discussions
- Unanswered Posts
- Unread Posts
- Active Threads
- Mark all forums read
- Member List
- Interpreter
Calendar program
- Python Forum
- Python Coding
- 0 Vote(s) - 0 Average
- View a Printable Version
User Panel Messages
Announcements.
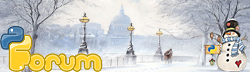
Login to Python Forum
Learn the fundamentals of Computer Science and Python in this free virtual club
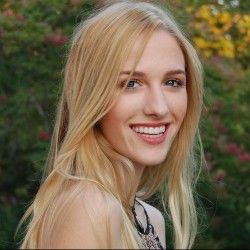
Are you learning how to code in 2020?
Or are you already working as a developer but want to learn computer science fundamentals?
I'd like to invite you to join the CodeBookClub, a free virtual meetup for new and intermediate programmers. The club is gearing up to read a new book together--an intro to computer science book that focuses on teaching the basics of computer science and the programming language Python.
You may think of a book club as a group of people just reading and discussing a book together. However, our reading will include ongoing activities, challenges and community events that will keep us actively learning and creating.
Read on for more details!
Who is this meetup for?
- People with no previous coding experience who’d like to get started with coding.
- Self-taught developers who want to learn computer science fundamentals.
- JavaScript developers interested in learning Python (and Scheme).
Why join this group?
freeCodeCamp founder Quincy Larson has said, “ the worst way to learn to program is to learn alone. ”
When you’re learning alone, it’s easier to give up when you get stuck on a coding problem. Or you lose motivation because you had no one keeping you accountable to keep learning. This is where the club comes in!
Whether you're learning to code for the first time or already a developer, in this club we'll learn computer science fundamentals together, work through problems when we get stuck, and hold each other accountable to keep learning.
I currently work as a software developer, but 3 years ago I used online resources like freeCodeCamp to teach myself how to code.

The one thing that helped me most was finding communities of people who were learning with me. Our book club is for developers of any experience level who would like to join us!
What will we be learning?
Our group will be reading Intro To Computing, an intro to computer science textbook written by Professor David Evans of Computer Science at the University of Virginia.
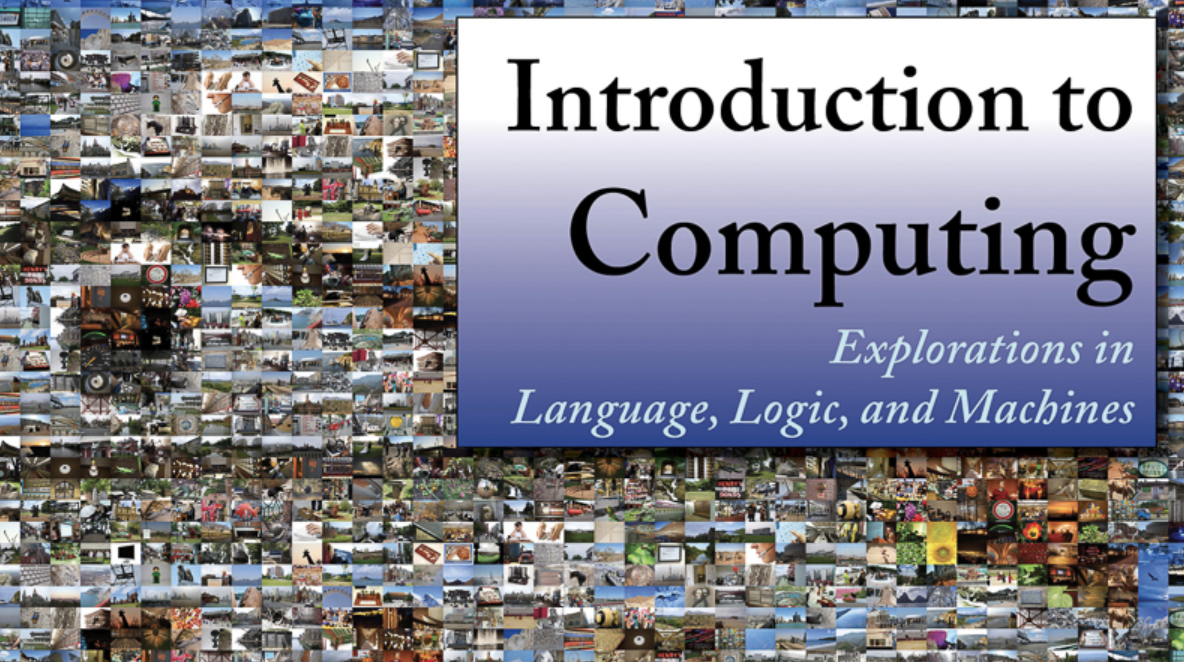
The book is an introduction to several fundamentals in computer science that you've perhaps heard of but want to learn more about: algorithms, coding languages, data, interpreters, measuring cost, and more. If you'd like to view the table of contents of the book, you can do so here .
The book also introduces us to Python and (some Scheme) with coding exercises and homework assignments--so it’s not just a book focused on theory, but has many exercises throughout.
How do members participate?
Actively learning with other developers is what the club is all about. If you join our reading, you can choose to complete various activities each week such as completing coding exercises from the book with other members of the club, answering discussion questions, or blogging or Tweeting about what you're learning. For more details on how you can participate:
We'll also set up some live Zoom calls where we meet on a video call to discuss the book and our coding exercises.
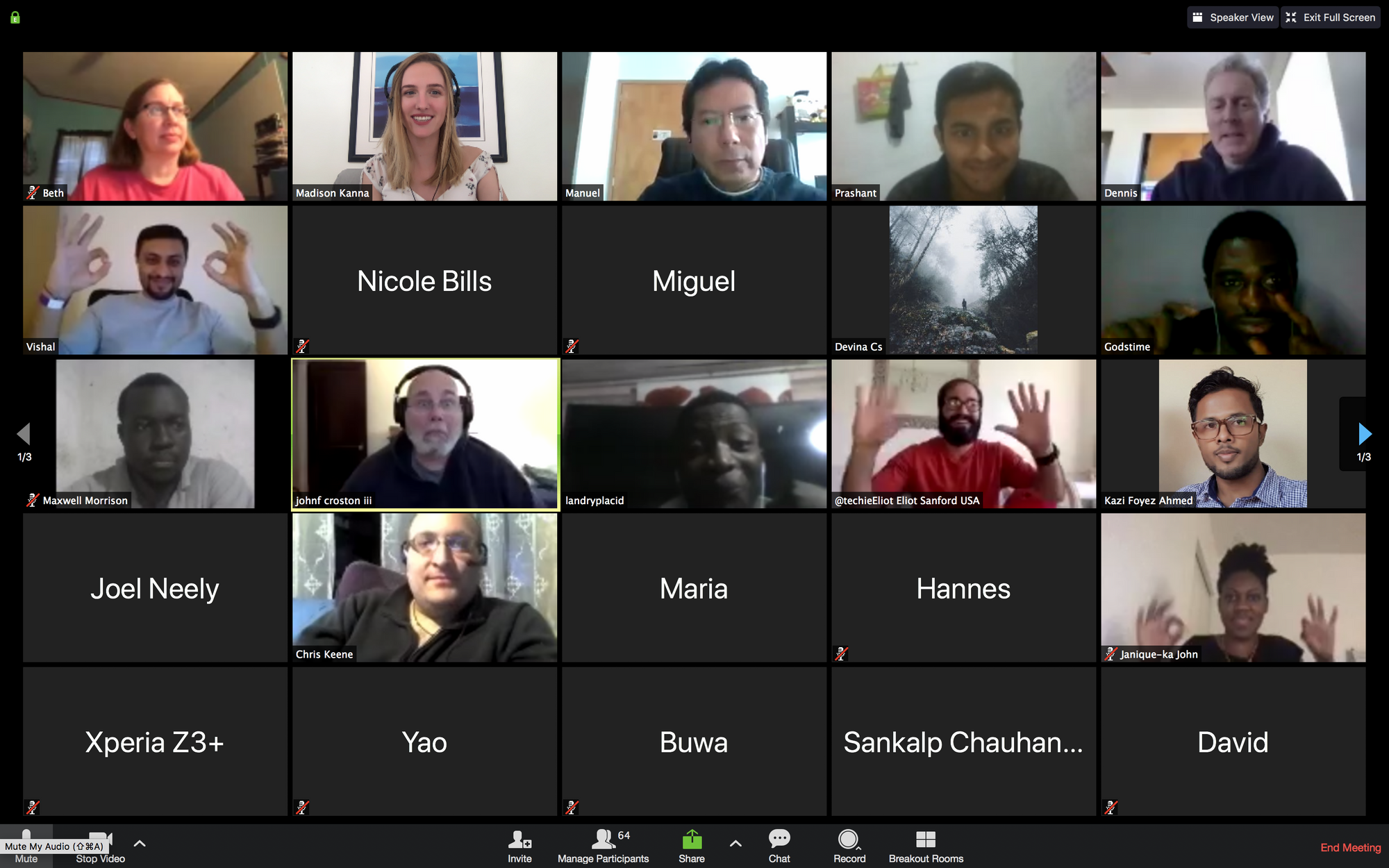
What is the cost of the book and club?
This book is 100% free and can be found here . The club is also free.
When does the reading start?
You should have read chapter 1 of the book by April 12th.
Here are more details on the club:
If yo u 'd like to join the club, sign up for our email list to be invited, get instructions on how to join the club and join us within 5 minutes. you'll also get updates on future readings and community events., the club is 100% free, always. if you have feedback or questions on this post, feel free to tweet me @madisonkanna or follow the @codebookclub..
Read more posts .
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Study Guides
- Homework Questions
CS 1101-01 - AY2024-T3 - Programming Assignment Unit 1 Rina Webster
- Computer Science
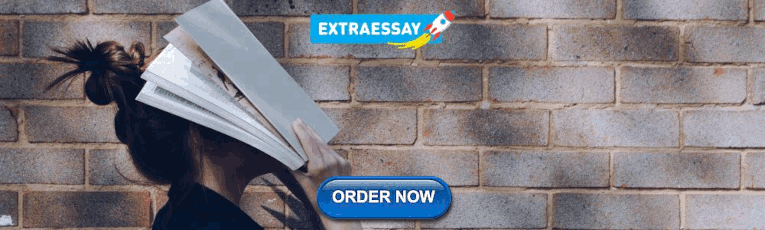
IMAGES
VIDEO
COMMENTS
Intro to CS - Unit 7 Calendar Assignment . hey to any active members of this subreddit, if you can provide any sort of help on this assignment I'd appreciate it. I literally could not explain how confused I am over it. thanks in advance lmao Share Sort by: Best. Open comment sort options. Best. Top. New ...
The provided source code is intended to work with the 2023 AP CS A course. These solutions may grow out-of-date as new changes are made to the course every year. The user-friendly website front-end found here is built using Retype. Table of Contents. Unit 1: Primitive Types;
Hello, I need help with this assignment please!! It'd mean a lot if I could see how you'd do this :(( Instructions. You should see a large block of code in the programming environment below. First, run this code to see what it does.
Unit 4: Playing games with functions. In this course, you'll use programming as a tool to measure environmental footprints, model infectious diseases, design game levels, and more. Starting with Python fundamentals like variables, conditionals, loops, and functions, you'll grow your programming toolkit as you build a portfolio of projects ...
In this assignment, you will learn how to search for the index of multiple instances of a subset in a string.
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and "debug" it. Designed for students with or without prior programming experience who'd like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals ...
Q5. Keywords can be used as variable names (T/F) Q6. Write the code to display all keywords in python. Q7. Write two keywords which start with capital letters. Q8. Write the output of the following.
Study with Quizlet and memorize flashcards containing terms like A command that displays text and numbers on the screen., A computer component that carries out a program's instructions., Information sent to the computer by the user, in the form of letters, numbers, or symbols. and more.
Question 7. Variables are created when they are first assigned their value. True. Question 8. Python variables support dynamic typing. True. Question 9. You can rename a keyword. False. Question 10. String values in Python can be single line strings, and multi-line strings. True. Question 11. A variable can contain values of different types at ...
Introduction to Computer Science in Python. This course teaches the fundamentals of computer programming as well as some advanced features of the Python 3 language. By the end of this course, students build a simple console-based game and learn material equivalent to a semester college introductory Python course. View Syllabus Explore Course.
The five lessons below can be followed to implement the basic asteroids application. As part of the assignment in the text, you are to implement one more level to the application when level 1 is completed. Lesson 1. Lesson 2. Lesson 3. Lesson 4. Lesson 5. In addition to the exercises at the end of chapter 7 I have had students implement a ...
_6.0001 Introduction to Computer Science and Programming in Python_ is intended for students with little or no programming experience. It aims to provide students with an understanding of the role computation can play in solving problems and to help students, regardless of their major, feel justifiably confident of their ability to write small programs that allow them to accomplish useful goals.
Module 2 • 5 hours to complete. This module will introduce you the concept, Variables, and primitive data types. You will also learn the fundamental operations in Python: Assignment, Arithmetic, Relational, and Logical operations. With these tools unlocked, you can create a more useful computational program.
1.7 CS Fundamentals- Python. Flashcards; Learn; Test; ... CS-2365 Quiz 2. 8 terms. RhettKDavis. Preview. Terms in this set (8) int() A function that translates strings to integer numbers. str() A function that tells Python to handle the value in the parentheses as a string, not as a number. string. Can be made up of letters, numbers, and/or ...
The official dedicated python forum. This is my assignment calendar program that allows the user to enter a day, month, and year in three separate variables as shown below. Please enter a date Day: Month: Year: Then, ask the user to select from a menu of choices using this formatting: Menu: 1) Calculate the number of days in the given month. 2) Calculate the number of days left in the given ...
The club is gearing up to read a new book together--an intro to computer science book that focuses on teaching the basics of computer science and the programming language Python. You may think of a book club as a group of people just reading and discussing a book together. However, our reading will include ongoing activities, challenges and ...
Which of the following is NOT an example of a Python built-in function? for. What is the primary use of functions? To organize longer programs. 7.2 Lesson Practice. We call the central part of the program _____. Main. To create the body of a function, we _____ the code.
Programming Assignment tutorial for University of the People CS1101 Programming Fundamentals Unit 7 .
Solution to the week's task and assignment for the material of study for the week. programming assignment unit fundamentals of programming (cs 1101) university
2. To find the number of days in a month, you make a function that contains if statements. If your variable "month" is 1, 3, 5, 7, 8, 10, or 12, it will return 31, as there are 31 days in each of those months. In your if statement for month 2, February, you will add leap_year (y) to 28. If it is not a leap year, it will add 0 to the number of ...
CS1101 Programming Assignment Unit 7. unit 7. Course. Programming Fundamentals (CS 1101) 999+ Documents. Students shared 1074 documents in this course. University ... (2015). Think Python: How to think like a computer scientist. Needham, Massachusetts: Green Tree Press. def histogram(s): d = dict() for c in s: if c not in d: d[c] = 1 else: d[c ...
CS Python Fundamentals Test 1. Analog has ___ sound waves. Click the card to flip 👆. round, different lengths. Click the card to flip 👆. 1 / 19.
Computer-science document from University of the People, 6 pages, 1 Programming Assignment Unit 1 Rina Webster Computer Science Program, University of the People CS 1101: Programming Fundamentals - AY2024-T3 Rupali Memane February 8, 2024 2 Part 1 Learn From Your Mistakes! A. If you are trying to print your name, what
Project stem CS python fundamentals UNIT 1 SV. Get a hint. 1.1 lesson practice question 1. 1. Natural language __________. Click the card to flip 👆. is what humans use to communicate with each other. Click the card to flip 👆. 1 / 58.