- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
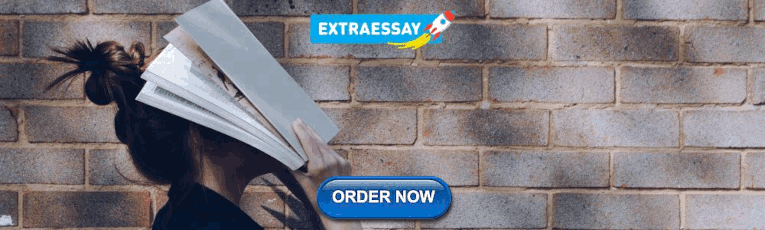
JavaScript Assignment Operators
A ssignment operators.
Assignment operators are used to assign values to variables in JavaScript.
Assignment Operators List
There are so many assignment operators as shown in the table with the description.
Below we have described each operator with an example code:
Addition assignment operator(+=).
The Addition assignment operator adds the value to the right operand to a variable and assigns the result to the variable. Addition or concatenation is possible. In case of concatenation then we use the string as an operand.
Subtraction Assignment Operator(-=)
The Substraction Assignment Operator subtracts the value of the right operand from a variable and assigns the result to the variable.
Multiplication Assignment Operator(*=)
The Multiplication Assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable.
Division Assignment Operator(/=)
The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable.
Remainder Assignment Operator(%=)
The Remainder Assignment Operator divides a variable by the value of the right operand and assigns the remainder to the variable.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator raises the value of a variable to the power of the right operand.
Left Shift Assignment Operator(<<=)
This Left Shift Assignment O perator moves the specified amount of bits to the left and assigns the result to the variable.
Right Shift Assignment O perator(>>=)
The Right Shift Assignment Operator moves the specified amount of bits to the right and assigns the result to the variable.
Bitwise AND Assignment Operator(&=)
The Bitwise AND Assignment Operator uses the binary representation of both operands, does a bitwise AND operation on them, and assigns the result to the variable.
Btwise OR Assignment Operator(|=)
The Btwise OR Assignment Operator uses the binary representation of both operands, does a bitwise OR operation on them, and assigns the result to the variable.
Bitwise XOR Assignment Operator(^=)
The Bitwise XOR Assignment Operator uses the binary representation of both operands, does a bitwise XOR operation on them, and assigns the result to the variable.
Logical AND Assignment Operator(&&=)
The Logical AND Assignment assigns the value of y into x only if x is a truthy value.
Logical OR Assignment Operator( ||= )
The Logical OR Assignment Operator is used to assign the value of y to x if the value of x is falsy.
Nullish coalescing Assignment Operator(??=)
The Nullish coalescing Assignment Operator assigns the value of y to x if the value of x is null.
Supported Browsers: The browsers supported by all JavaScript Assignment operators are listed below:
- Google Chrome
- Microsoft Edge
JavaScript Assignment Operators – FAQs
What are assignment operators in javascript.
Assignment operators in JavaScript are used to assign values to variables. The most common assignment operator is the equals sign (=), but there are several other assignment operators that perform an operation and assign the result to a variable in a single step.
What is the basic assignment operator?
The basic assignment operator is =. It assigns the value on the right to the variable on the left.
What are compound assignment operators?
Compound assignment operators combine a basic arithmetic or bitwise operation with assignment. For example, += combines addition and assignment.
What does the += operator do?
The += operator adds the value on the right to the variable on the left and then assigns the result to the variable.
How does the *= operator work?
The *= operator multiplies the variable by the value on the right and assigns the result to the variable.
Can you use assignment operators with strings?
Yes, you can use the += operator to concatenate strings.
What is the difference between = and ==?
The = operator is the assignment operator, used to assign a value to a variable. The == operator is the equality operator, used to compare two values for equality, performing type conversion if necessary.
What does the **= operator do?
The **= operator performs exponentiation (raising to a power) and assigns the result to the variable.
Similar Reads
- Web Technologies
- javascript-operators
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?
Home » JavaScript Tutorial » JavaScript Assignment Operators
JavaScript Assignment Operators
Summary : in this tutorial, you will learn how to use JavaScript assignment operators to assign a value to a variable.
Introduction to JavaScript assignment operators
An assignment operator ( = ) assigns a value to a variable. The syntax of the assignment operator is as follows:
In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a .
The following example declares the counter variable and initializes its value to zero:
The following example increases the counter variable by one and assigns the result to the counter variable:
When evaluating the second statement, JavaScript evaluates the expression on the right-hand first ( counter + 1 ) and assigns the result to the counter variable. After the second assignment, the counter variable is 1 .
To make the code more concise, you can use the += operator like this:
In this syntax, you don’t have to repeat the counter variable twice in the assignment.
The following table illustrates assignment operators that are shorthand for another operator and the assignment:
Chaining JavaScript assignment operators
If you want to assign a single value to multiple variables, you can chain the assignment operators. For example:
In this example, JavaScript evaluates from right to left. Therefore, it does the following:
- Use the assignment operator ( = ) to assign a value to a variable.
- Chain the assignment operators if you want to assign a single value to multiple variables.
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Ternary Operator
JavaScript Booleans
JavaScript Bitwise Operators
- JavaScript Object.is()
- JavaScript typeof Operator
JavaScript operators are special symbols that perform operations on one or more operands (values). For example,
Here, we used the + operator to add the operands 2 and 3 .
JavaScript Operator Types
Here is a list of different JavaScript operators you will learn in this tutorial:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Miscellaneous Operators
1. JavaScript Arithmetic Operators
We use arithmetic operators to perform arithmetic calculations like addition, subtraction, etc. For example,
Here, we used the - operator to subtract 3 from 5 .
Commonly Used Arithmetic Operators
Example 1: arithmetic operators in javascript.
Note: The increment operator ++ adds 1 to the operand. And, the decrement operator -- decreases the value of the operand by 1 .
To learn more, visit Increment ++ and Decrement -- Operators .
2. JavaScript Assignment Operators
We use assignment operators to assign values to variables. For example,
Here, we used the = operator to assign the value 5 to the variable x .
Commonly Used Assignment Operators
Example 2: assignment operators in javascript, 3. javascript comparison operators.
We use comparison operators to compare two values and return a boolean value ( true or false ). For example,
Here, we have used the > comparison operator to check whether a (whose value is 3 ) is greater than b (whose value is 2 ).
Since 3 is greater than 2 , we get true as output.
Note: In the above example, a > b is called a boolean expression since evaluating it results in a boolean value.
Commonly Used Comparison Operators
Example 3: comparison operators in javascript.
The equality operators ( == and != ) convert both operands to the same type before comparing their values. For example,
Here, we used the == operator to compare the number 3 and the string 3 .
By default, JavaScript converts string 3 to number 3 and compares the values.
However, the strict equality operators ( === and !== ) do not convert operand types before comparing their values. For example,
Here, JavaScript didn't convert string 4 to number 4 before comparing their values.
Thus, the result is false , as number 4 isn't equal to string 4 .
4. JavaScript Logical Operators
We use logical operators to perform logical operations on boolean expressions. For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the combined result is true .
Commonly Used Logical Operators
Example 4: logical operators in javascript.
Note: We use comparison and logical operators in decision-making and loops. You will learn about them in detail in later tutorials.
More on JavaScript Operators
We use bitwise operators to perform binary operations on integers.
Note: We rarely use bitwise operators in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
In JavaScript, you can also use the + operator to concatenate (join) two strings. For example,
Here, we used the + operator to concatenate str1 and str2 .
JavaScript has many more operators besides the ones we listed above. You will learn about them in detail in later tutorials.
Table of Contents
- Introduction
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
Video: JavaScript Operators
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
JavaScript Tutorial

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
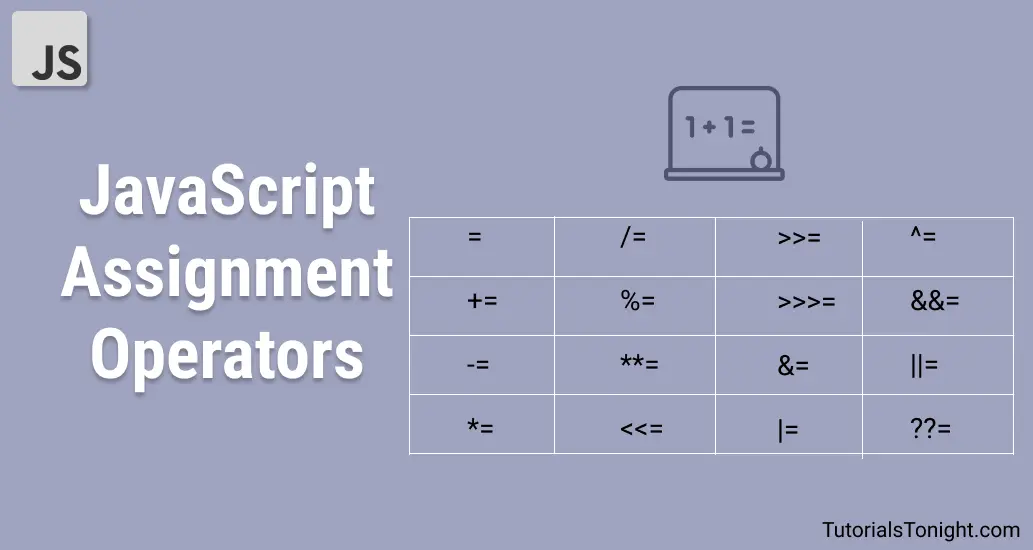
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).

25+ JavaScript Coding Interview Questions (SOLVED with CODE)
Having a JavaScript Coding Interview Session on this week? Fear not, we got your covered! Check that ultimate list of 25 advanced and tricky JavaScript Coding Interview Questions and Challenges to crack on your next senior web developer interview and got your next six-figure job offer in no time!
Q1 : Explain what a callback function is and provide a simple example
A callback function is a function that is passed to another function as an argument and is executed after some operation has been completed. Below is an example of a simple callback function that logs to the console after some operations have been completed.
Q2 : Given a string, reverse each word in the sentence
For example Welcome to this Javascript Guide! should be become emocleW ot siht tpircsavaJ !ediuG
Q3 : How to check if an object is an array or not? Provide some code.
The best way to find whether an object is instance of a particular class or not using toString method from Object.prototype
One of the best use cases of type checking of an object is when we do method overloading in JavaScript. For understanding this let say we have a method called greet which take one single string and also a list of string, so making our greet method workable in both situation we need to know what kind of parameter is being passed, is it single value or list of value?
However, in above implementation it might not necessary to check type for array, we can check for single value string and put array logic code in else block, let see below code for the same.
Now it's fine we can go with above two implementations, but when we have a situation like a parameter can be single value , array , and object type then we will be in trouble.
Coming back to checking type of object, As we mentioned that we can use Object.prototype.toString
If you are using jQuery then you can also used jQuery isArray method:
FYI jQuery uses Object.prototype.toString.call internally to check whether an object is an array or not.
In modern browser, you can also use:
Array.isArray is supported by Chrome 5, Firefox 4.0, IE 9, Opera 10.5 and Safari 5
Q4 : How to empty an array in JavaScript?
How could we empty the array above?
Above code will set the variable arrayList to a new empty array. This is recommended if you don't have references to the original array arrayList anywhere else because It will actually create a new empty array. You should be careful with this way of empty the array, because if you have referenced this array from another variable, then the original reference array will remain unchanged, Only use this way if you have only referenced the array by its original variable arrayList .
For Instance:
Above code will clear the existing array by setting its length to 0. This way of empty the array also update all the reference variable which pointing to the original array. This way of empty the array is useful when you want to update all the another reference variable which pointing to arrayList .
Above implementation will also work perfectly. This way of empty the array will also update all the references of the original array.
Above implementation can also empty the array. But not recommended to use often.
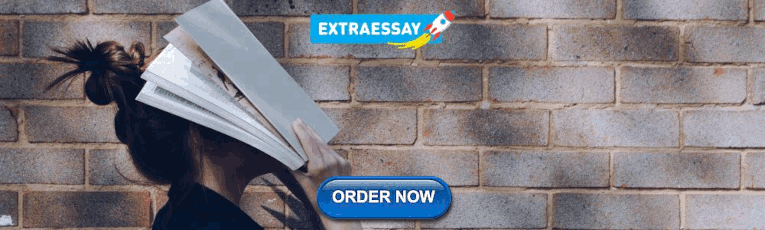
Q5 : How would you check if a number is an integer?
A very simply way to check if a number is a decimal or integer is to see if there is a remainder left when you divide by 1.
Q6 : Implement enqueue and dequeue using only two stacks
Enqueue means to add an element, dequeue to remove an element.
Q7 : Make this work
Q8 : write a "mul" function which will properly when invoked as below syntax.
Here mul function accept the first argument and return anonymous function which take the second parameter and return anonymous function which take the third parameter and return multiplication of arguments which is being passed in successive
In JavaScript function defined inside has access to outer function variable and function is the first class object so it can be returned by function as well and passed as argument in another function.
- A function is an instance of the Object type
- A function can have properties and has a link back to its constructor method
- Function can be stored as variable
- Function can be pass as a parameter to another function
- Function can be returned from function
Q9 : Write a function that would allow you to do this?
You can create a closure to keep the value passed to the function createBase even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable baseNumber .
Q10 : FizzBuzz Challenge
Create a for loop that iterates up to 100 while outputting "fizz" at multiples of 3 , "buzz" at multiples of 5 and "fizzbuzz" at multiples of 3 and 5 .
Check out this version of FizzBuzz:
Q11 : Given two strings, return true if they are anagrams of one another
For example: Mary is an anagram of Army
Q12 : How would you use a closure to create a private counter?
You can create a function within an outer function (a closure) that allows you to update a private variable but the variable wouldn't be accessible from outside the function without the use of a helper function.
Q13 : Provide some examples of non-bulean value coercion to a boolean one
The question is when a non-boolean value is coerced to a boolean, does it become true or false , respectively?
The specific list of "falsy" values in JavaScript is as follows:
- "" (empty string)
- 0 , -0 , NaN (invalid number)
- null , undefined
Any value that's not on this "falsy" list is "truthy." Here are some examples of those:
- [ ] , [ 1, "2", 3 ] (arrays)
- { } , { a: 42 } (objects)
- function foo() { .. } (functions)
Q14 : What will be the output of the following code?
Above code would give output 1undefined . If condition statement evaluate using eval so eval(function f() {}) which return function f() {} which is true so inside if statement code execute. typeof f return undefined because if statement code execute at run time, so statement inside if condition evaluated at run time.
Above code will also output 1undefined .
Q15 : What will the following code output?
The code above will output 5 even though it seems as if the variable was declared within a function and can't be accessed outside of it. This is because
is interpreted the following way:
But b is not declared anywhere in the function with var so it is set equal to 5 in the global scope .
Q16 : Write a function that would allow you to do this
You can create a closure to keep the value of a even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable a .
Q17 : How does the this keyword work? Provide some code examples
In JavaScript this always refers to the “owner” of the function we're executing, or rather, to the object that a function is a method of.
Q18 : How would you create a private variable in JavaScript?
To create a private variable in JavaScript that cannot be changed you need to create it as a local variable within a function. Even if the function is executed the variable cannot be accessed outside of the function. For example:
To access the variable, a helper function would need to be created that returns the private variable.
Q19 : What is Closure in JavaScript? Provide an example
A closure is a function defined inside another function (called parent function) and has access to the variable which is declared and defined in parent function scope.
The closure has access to variable in three scopes:
- Variable declared in his own scope
- Variable declared in parent function scope
- Variable declared in global namespace
innerFunction is closure which is defined inside outerFunction and has access to all variable which is declared and defined in outerFunction scope. In addition to this function defined inside function as closure has access to variable which is declared in global namespace .
Output of above code would be:
Q20 : What will be the output of the following code?
Above code will output 0 as output. delete operator is used to delete a property from an object. Here x is not an object it's local variable . delete operator doesn't affect local variable.
Q21 : What will be the output of the following code?
Above code will output xyz as output. Here emp1 object got company as prototype property. delete operator doesn't delete prototype property.
emp1 object doesn't have company as its own property. You can test it like:
However, we can delete company property directly from Employee object using delete Employee.company or we can also delete from emp1 object using __proto__ property delete emp1.__proto__.company .
Q22 : What will the following code output?
This will surprisingly output false because of floating point errors in internally representing certain numbers. 0.1 + 0.2 does not nicely come out to 0.3 but instead the result is actually 0.30000000000000004 because the computer cannot internally represent the correct number. One solution to get around this problem is to round the results when doing arithmetic with decimal numbers.
Q23 : When would you use the bind function?
The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
A good use of the bind function is when you have a particular function that you want to call with a specific this value. You can then use bind to pass a specific object to a function that uses a this reference.
Q24 : Write a recursive function that performs a binary search
Q25 : describe the revealing module pattern design pattern.
A variation of the module pattern is called the Revealing Module Pattern . The purpose is to maintain encapsulation and reveal certain variables and methods returned in an object literal. The direct implementation looks like this:
An obvious disadvantage of it is unable to reference the private methods
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

40 JavaScript Projects for Beginners – Easy Ideas to Get Started Coding JS
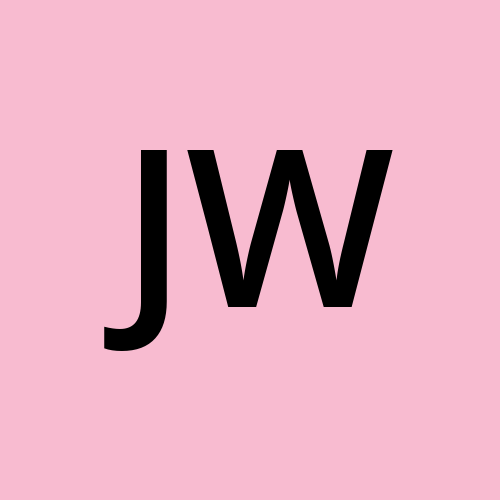
The best way to learn a new programming language is to build projects.
I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
Vanilla JavaScript Projects
How to create a color flipper.
- How to create a counter
- How to create a review carousel
- How to create a responsive navbar
- How to create a sidebar
- How to create a modal
How to create a FAQ page
How to create a restaurant menu page, how to create a video background, how to create a navigation bar on scroll, how to create tabs that display different content, how to create a countdown clock, how to create your own lorem ipsum, how to create a grocery list, how to create an image slider, how to create a rock paper scissors game, how to create a simon game, how to create a platformer game.
- How to create Doodle Jump
- How to create Flappy Bird
- How to create a Memory game
- How to create a Whack-a-mole game
- How to create Connect Four game
- How to create a Snake game
- How to create a Space Invaders game
- How to create a Frogger game
- How to create a Tetris game
React Projects
How to build a tic-tac-toe game using react hooks, how to build a tetris game using react hooks, how to create a birthday reminder app.
- How to create a tours page
How to create an accordion menu
How to create tabs for a portfolio page, how to create a review slider, how to create a color generator, how to create a stripe payment menu page, how to create a shopping cart page, how to create a cocktail search page, typescript projects, how to build a quiz app with react and typescript, how to create an arkanoid game with typescript.
If you have not learned JavaScript fundamentals, then I would suggest watching this course before proceeding with the projects.
Many of the screenshots below are from here .

In this John Smilga tutorial , you will learn how to create a random background color changer. This is a good project to get you started working with the DOM.
In Leonardo Maldonado's article on why it is important to learn about the DOM, he states:
By manipulating the DOM, you have infinite possibilities. You can create applications that update the data of the page without needing a refresh. Also, you can create applications that are customizable by the user and then change the layout of the page without a refresh.
Key concepts covered:
- document.getElementById()
- document.querySelector()
- addEventListener()
- document.body.style.backgroundColor
- Math.floor()
- Math.random()
- array.length
Before you get started, I would suggest watching the introduction where John goes over how to access the setup files for all of his projects.
How to create a Counter
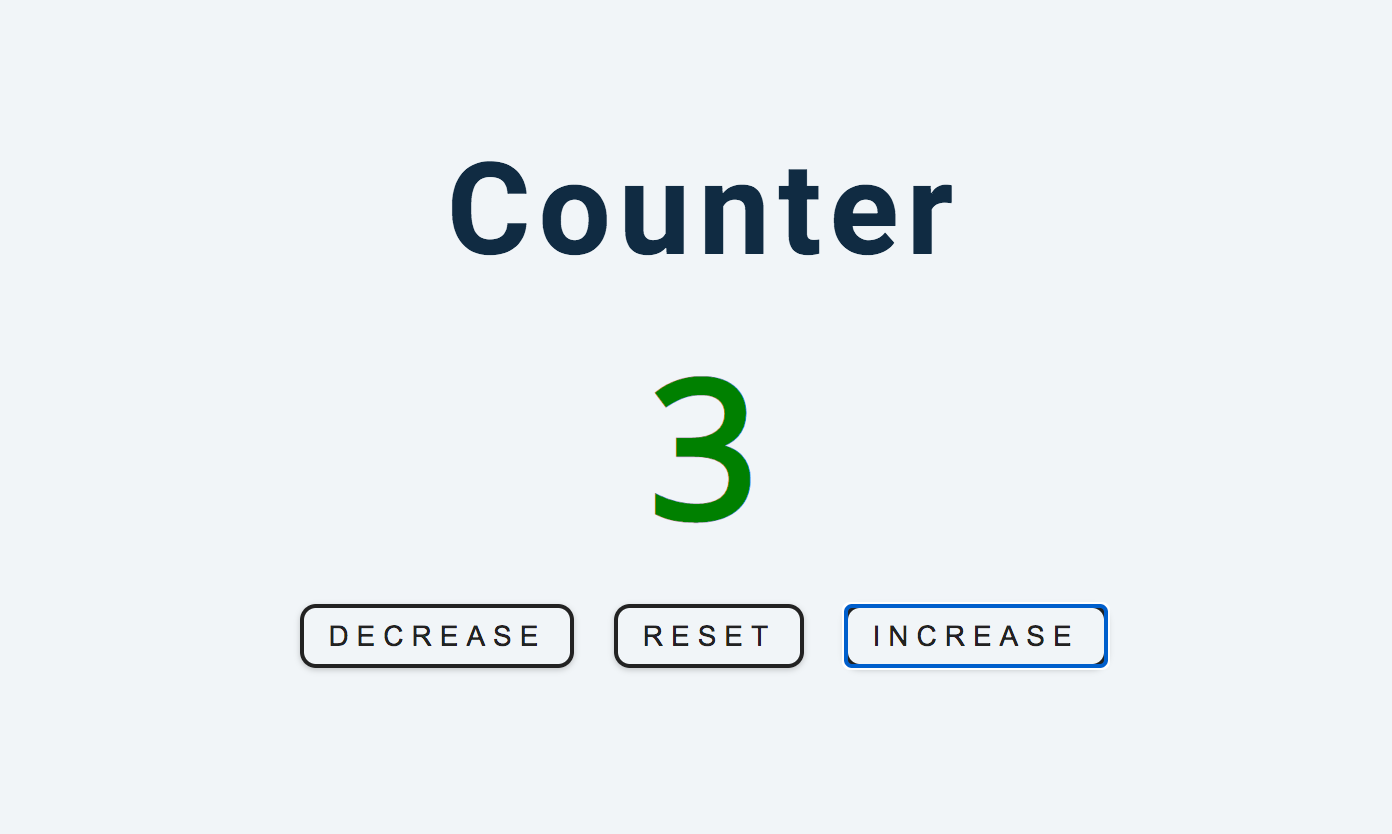
In this John Smilga tutorial , you will learn how to create a counter and write conditions that change the color based on positive or negative numbers displayed.
This project will give you more practice working with the DOM and you can use this simple counter in other projects like a pomodoro clock.
- document.querySelectorAll()
- currentTarget property
- textContent
How to create a Review carousel

In this tutorial , you will learn how to create a carousel of reviews with a button that generates random reviews.
This is a good feature to have on an ecommerce site to display customer reviews or a personal portfolio to display client reviews.
- DOMContentLoaded
How to create a responsive Navbar
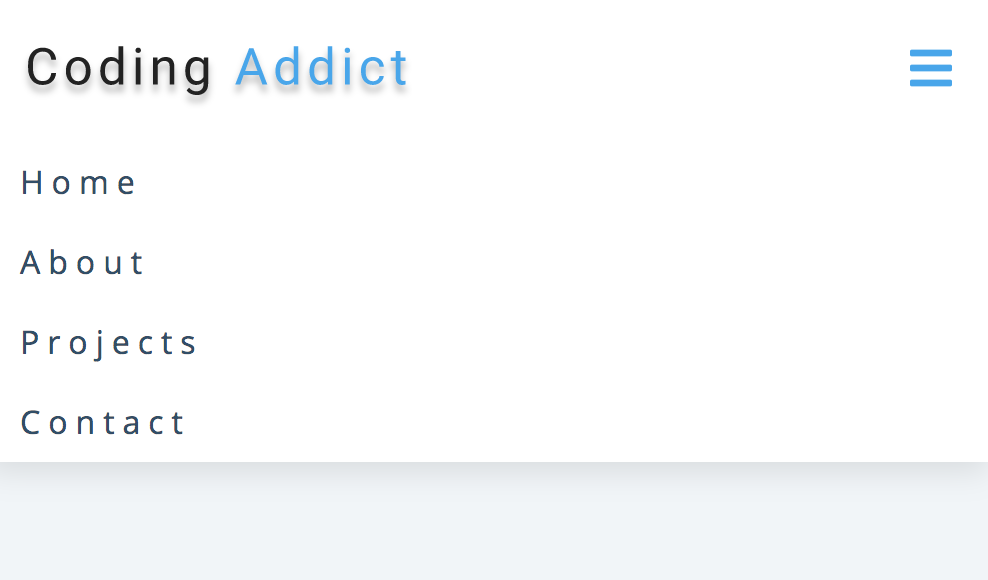
In this tutorial , you will learn how to create a responsive navbar that will show the hamburger menu for smaller devices.
Learning how to develop responsive websites is an important part of being a web developer. This is a popular feature used on a lot of websites.
- classList.toggle()
How to create a Sidebar

In this tutorial , you will learn how to create a sidebar with animation.
This is a cool feature that you can add to your personal website.
- classList.remove()
How to create a Modal
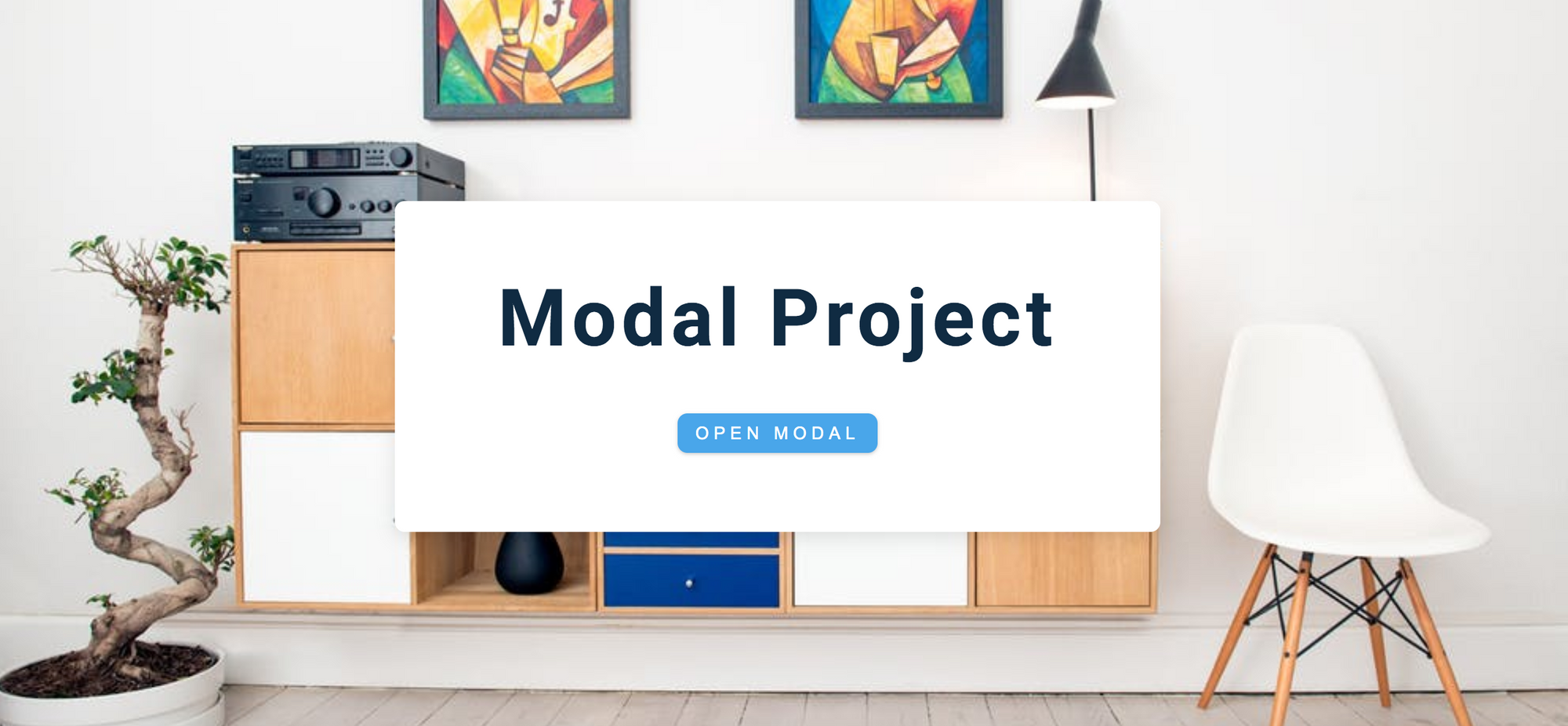
In this tutorial , you will learn how to create a modal window which is used on websites to get users to do or see something specific.
A good example of a modal window would be if a user made changes in a site without saving them and tried to go to another page. You can create a modal window that warns them to save their changes or else that information will be lost.
- classList.add()
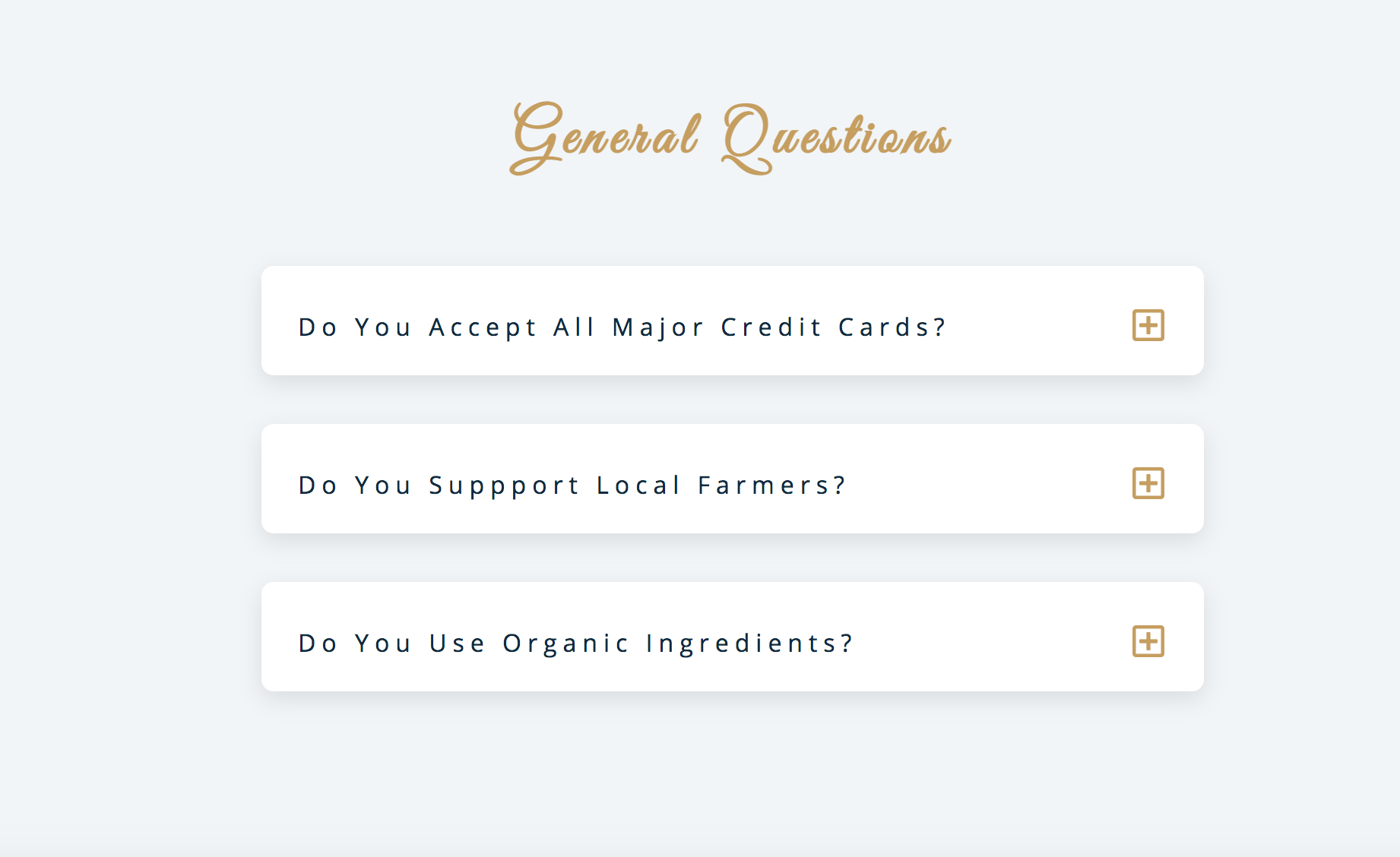
In this tutorial , you will learn how to create a frequently asked questions page which educates users about a business and drives traffic to the website through organic search results.

In this tutorial , you will learn how to make a restaurant menu page that filters through the different food menus. This is a fun project that will teach you higher order functions like map, reduce, and filter.
In Yazeed Bzadough's article on higher order functions, he states:
the greatest benefit of HOFs is greater reusability.
- map, reduce, and filter
- includes method

In this tutorial , you will learn how to make a video background with a play and pause feature. This is a common feature found in a lot of websites.
- classList.contains()
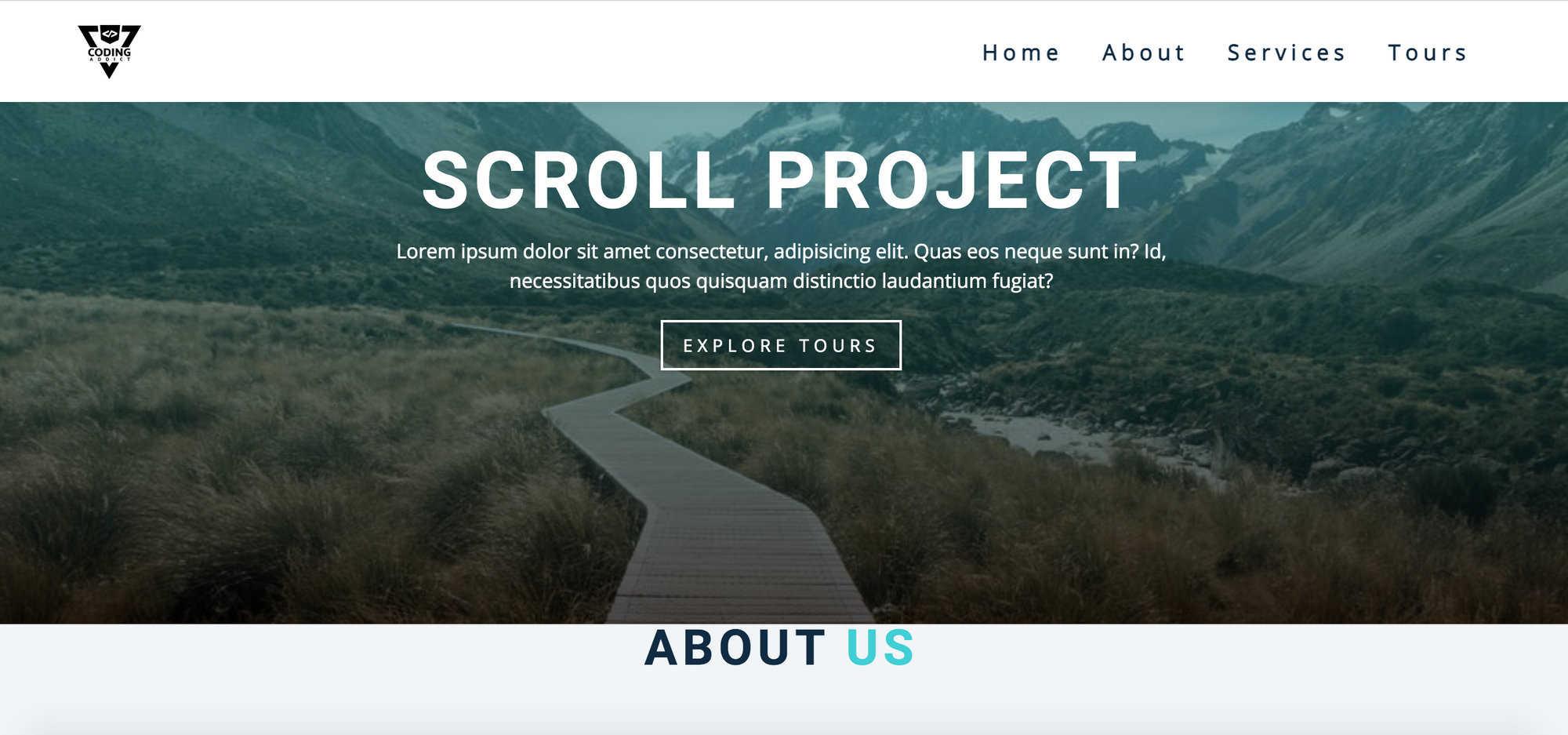
In this tutorial , you will learn how to create a navbar that slides down when scrolling and then stays at a fixed position at a certain height.
This is a popular feature found on many professional websites.
- getFullYear()
- getBoundingClientRect()
- slice method
- window.scrollTo()

In this tutorial , you will learn how to create tabs that will display different content which is useful when creating single page applications.
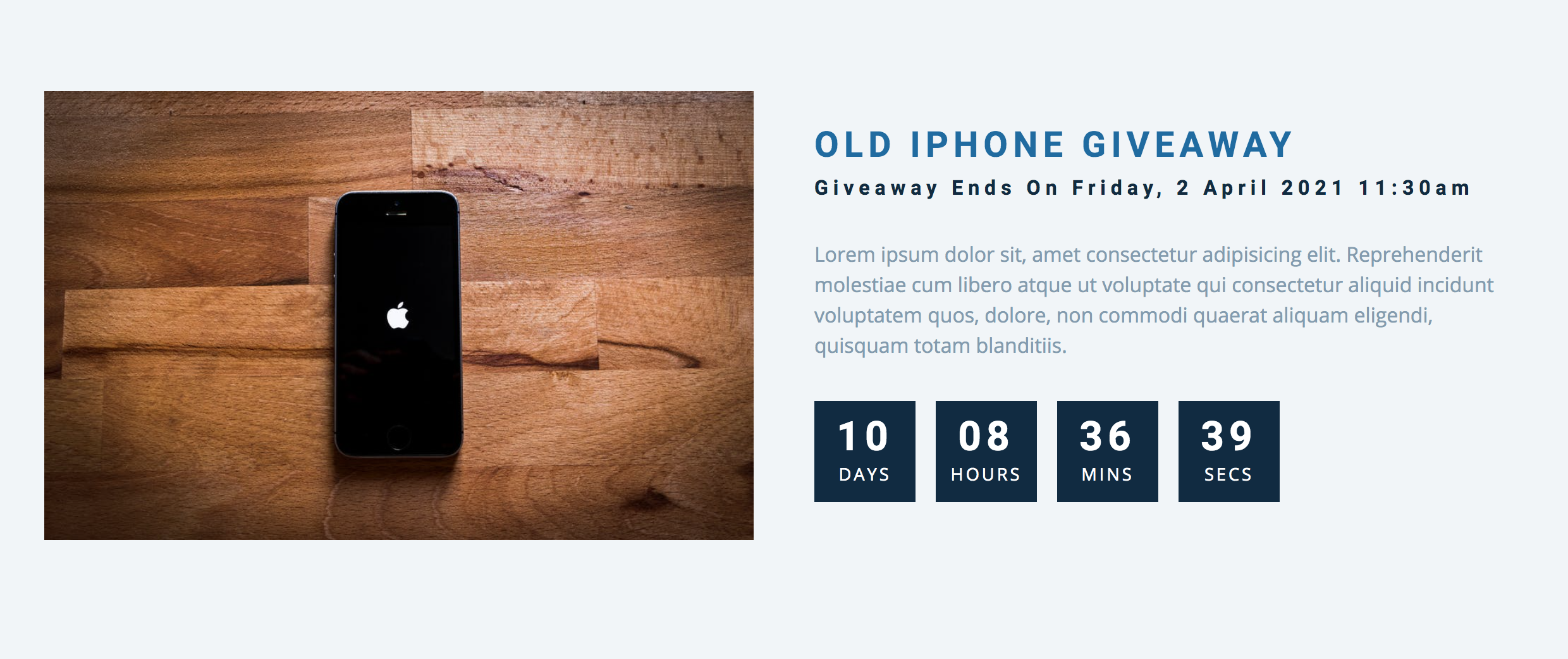
In this tutorial , you will learn how to make a countdown clock which can be used when a new product is coming out or a sale is about to end on an ecommerce site.
- setInterval()
- clearInterval()
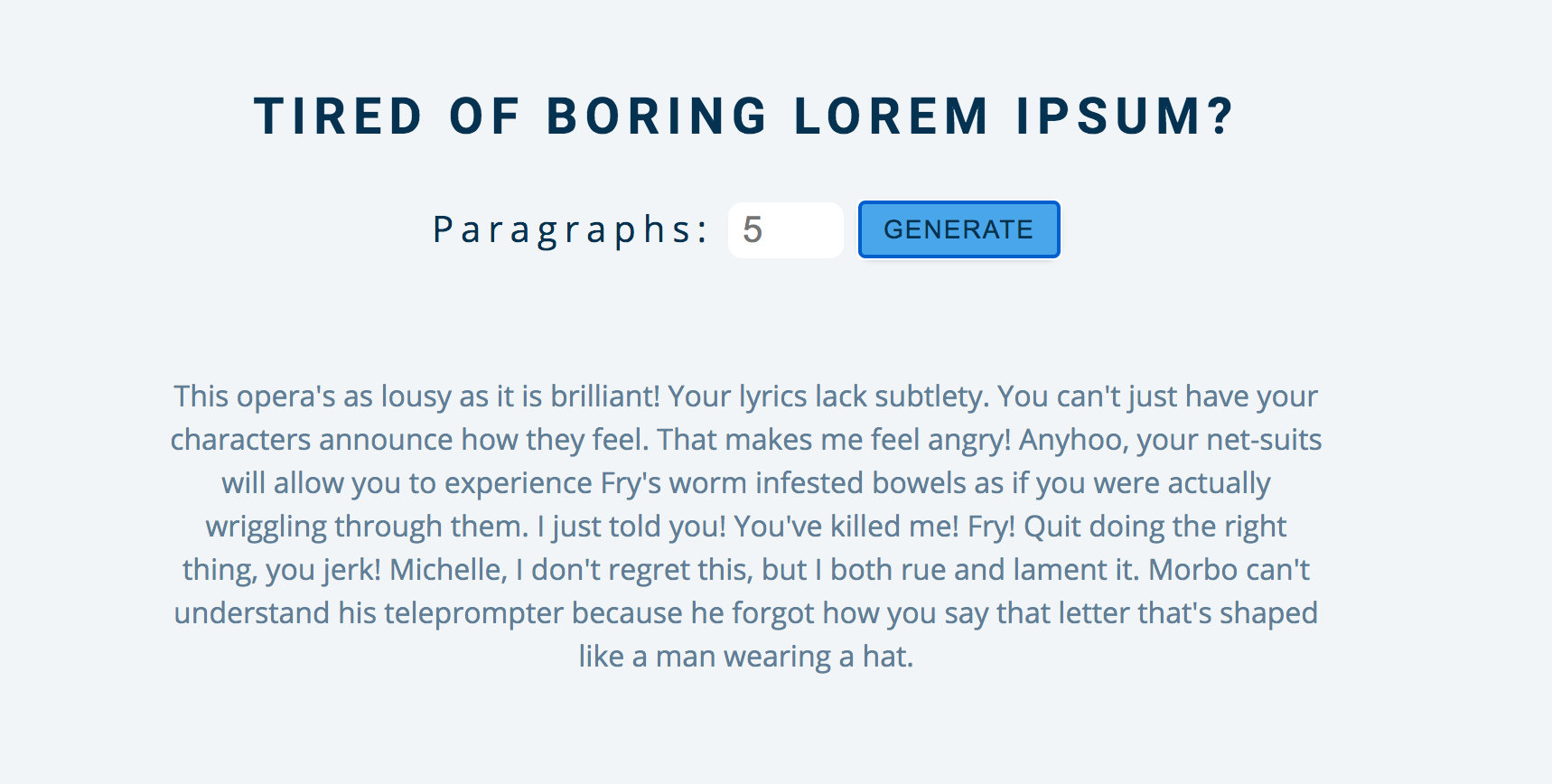
In this tutorial , you will learn how to create your own Lorem ipsum generator.
Lorem ipsum is the go to placeholder text for websites. This is a fun project to show off your creativity and create your own text.
- event.preventDefault()

In this tutorial , you will learn how to update and delete items from a grocery list and create a simple CRUD (Create, Read, Update, and Delete) application.
CRUD plays a very important role in developing full stack applications. Without it, you wouldn't be able to do things like edit or delete posts on your favorite social media platform.
- createAttribute()
- setAttributeNode()
- appendChild()
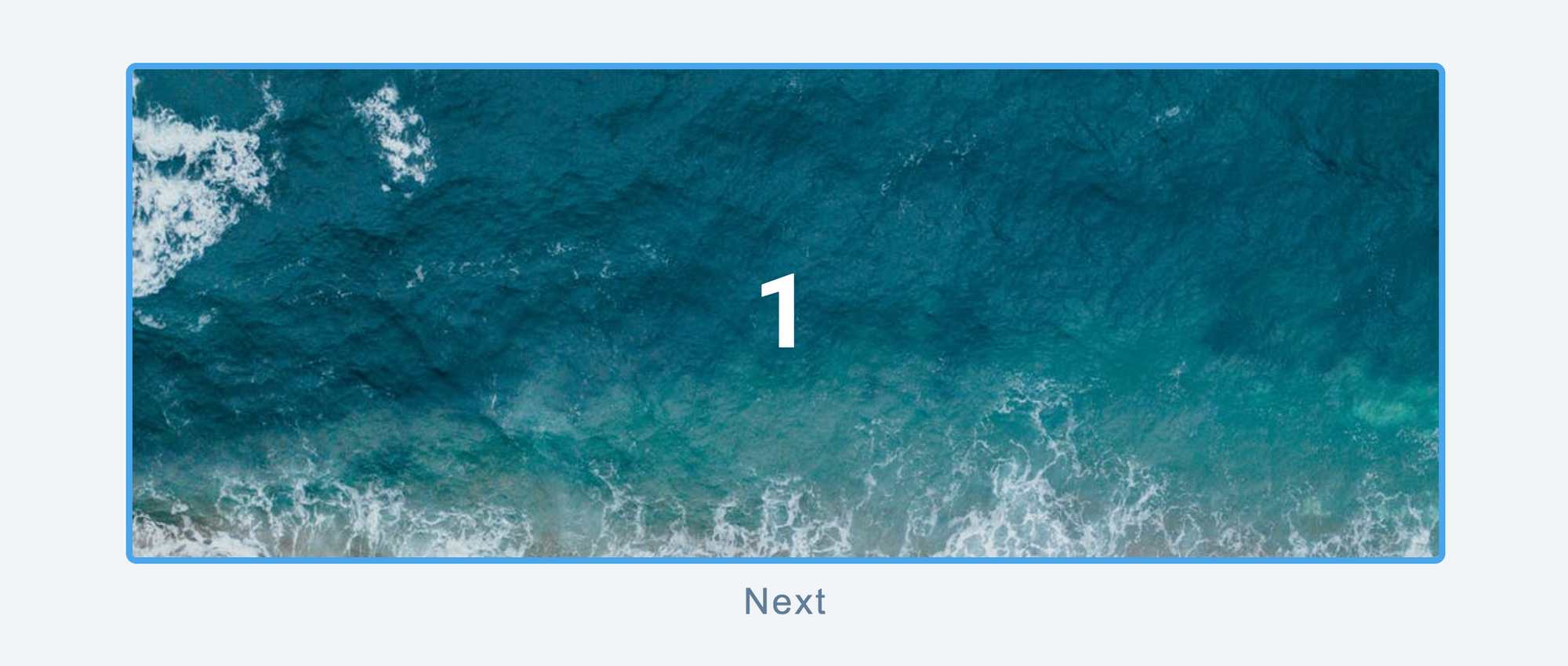
In this tutorial , you will learn how to build an image slider that you can add to any website.
- querySelectorAll()
- if/else statements
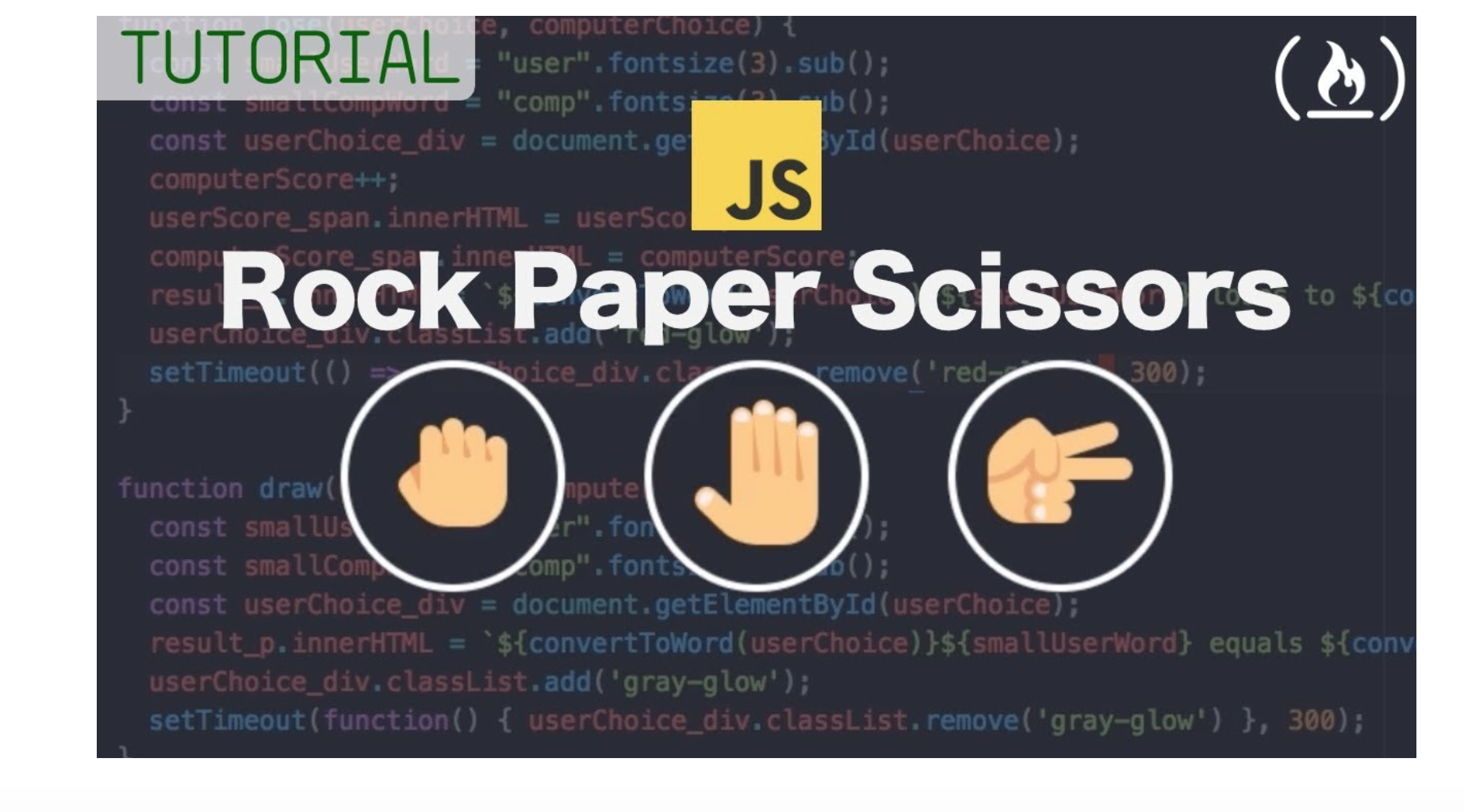
In this tutorial , Tenzin will teach you how to create a Rock Paper Scissors game. This is a fun project that will give more practice working with the DOM.
- switch statements

In this tutorial , Beau Carnes will teach you how to create the classic Simon Game. This is a good project that will get you thinking about the different components behind the game and how you would build out each of those functionalities.
- querySelector()
- setTimeout()
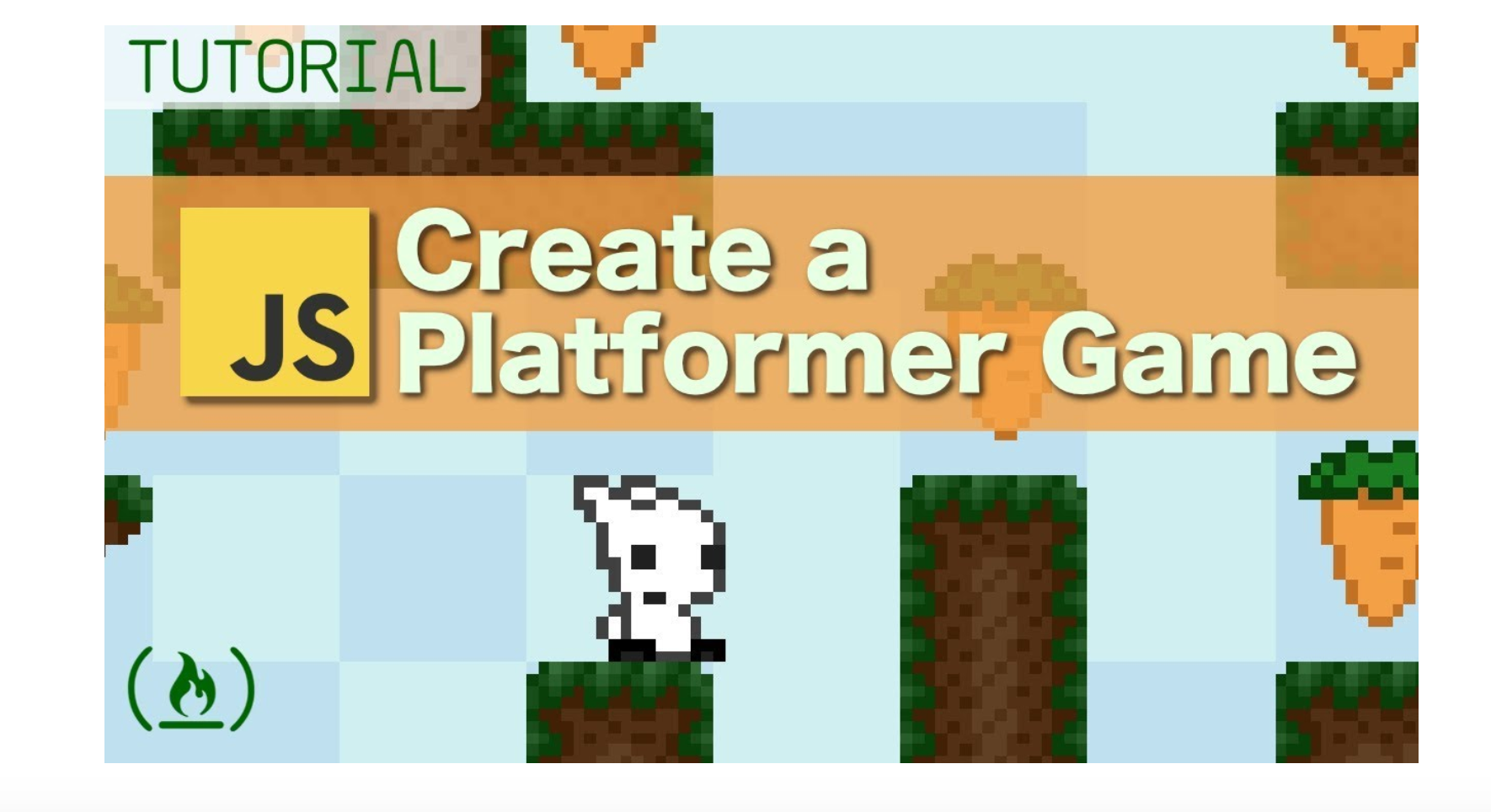
In this tutorial , Frank Poth will teach you how to build a platformer game. This project will introduce you to Object Oriented Programming principles and the Model, View, Controller software pattern.
- this keyword
- OOP principles
- MVC pattern
How to create Doodle Jump and Flappy Bird

In this video series , Ania Kubow will teach you how to build Doodle Jump and Flappy Bird .
Building games are a fun way to learn more about JavaScript and will cover many popular JavaScript methods.
- createElement()
- removeChild()
- removeEventListener()
How to create seven classic games with Ania Kubow

You will have a lot of fun creating seven games in this course by Ania Kubow:
- Memory Game
- Whack-a-mole
- Connect Four
- Space Invaders
- onclick event
- arrow functions
If you are not familiar with React fundamentals, then I would suggest taking this course before proceeding with the projects.
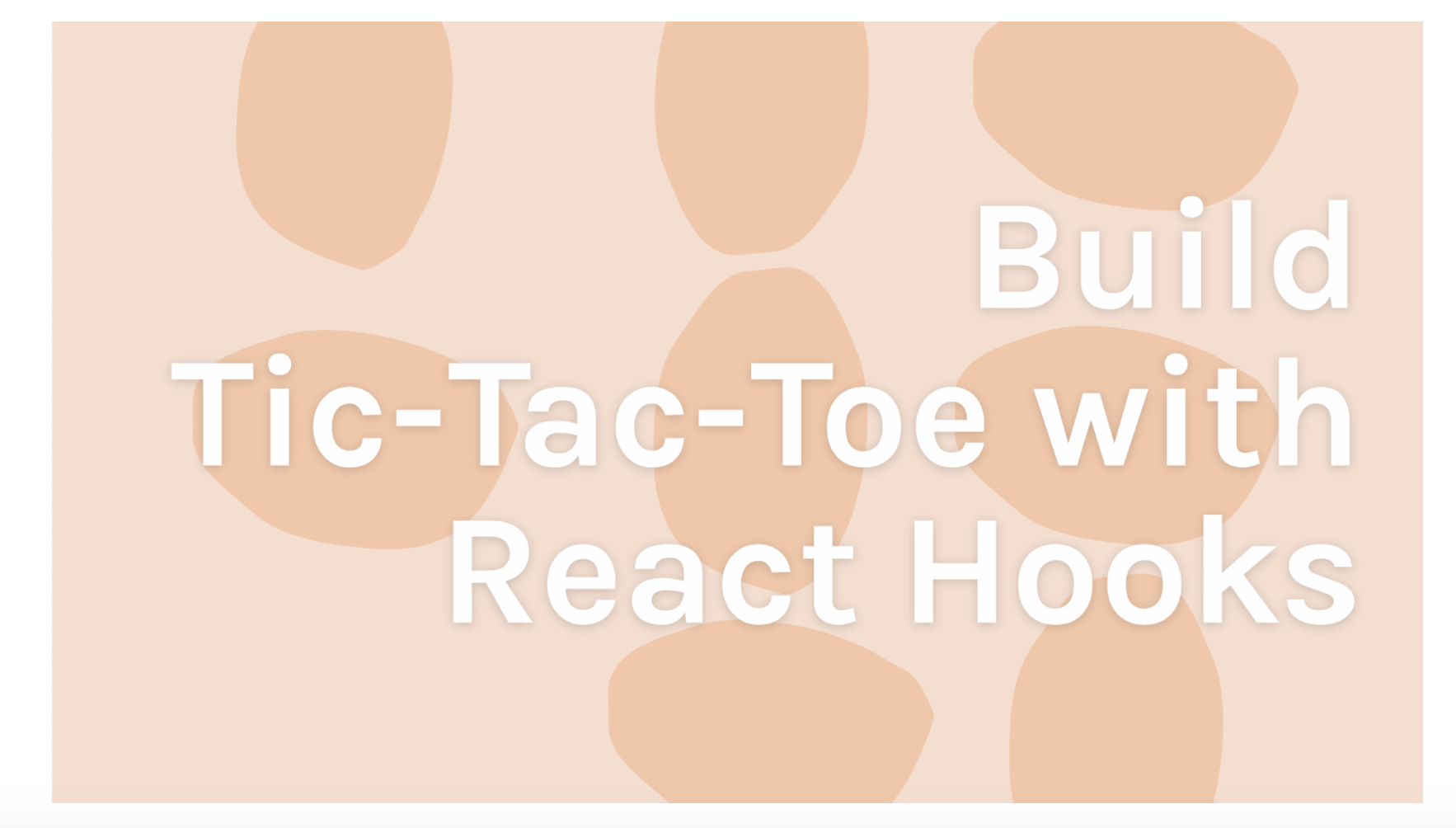
In this freeCodeCamp article , Per Harald Borgen talks about Scrimba's Tic-Tac-Toe game tutorial led by Thomas Weibenfalk. You can view the video course on Scimba's YouTube Channel.
This is a good project to start getting comfortable with React basics and working with hooks.
- import / export
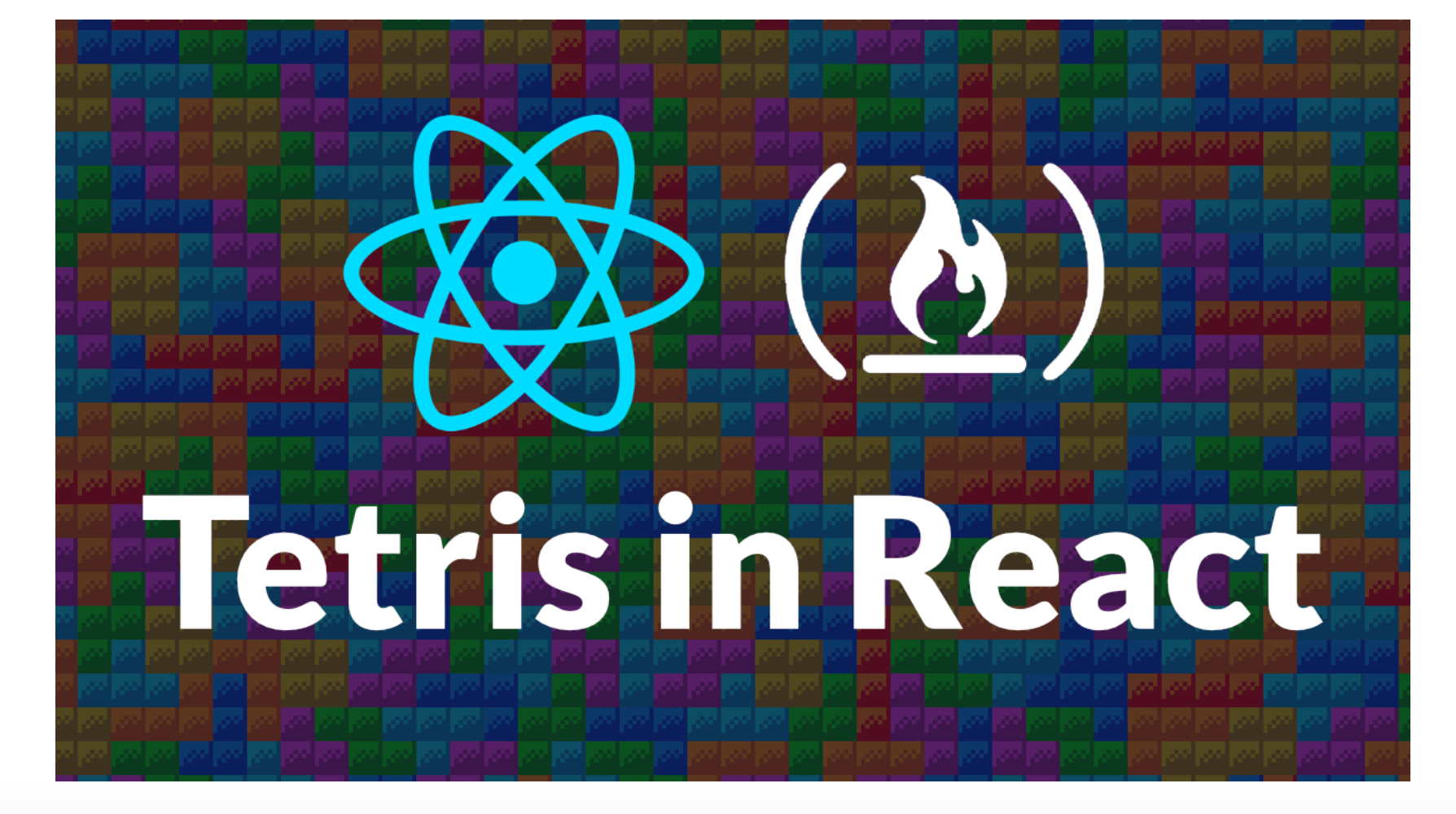
In this tutorial , Thomas Weibenfalk will teach you how to build a Tetris game using React Hooks and styled components.
- useEffect()
- useCallback()
- styled components
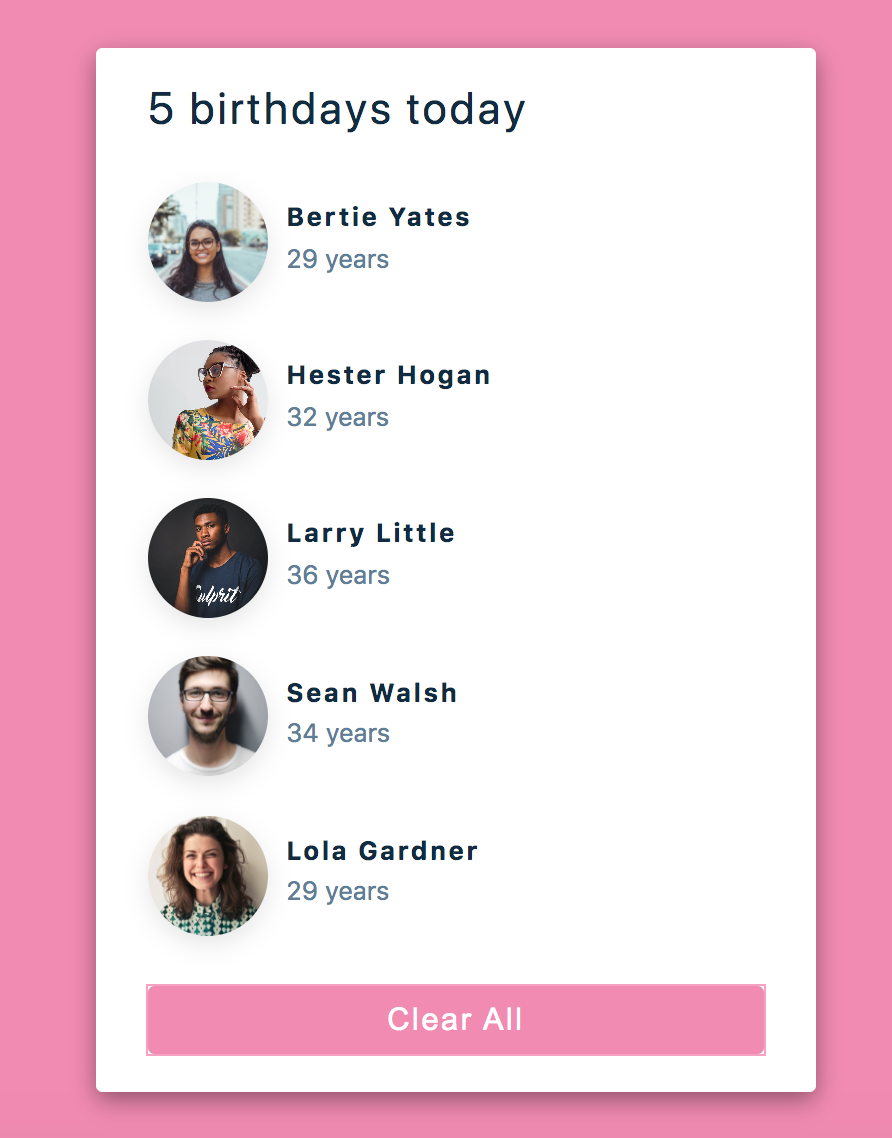
In this John Smilga course, you will learn how to create a birthday reminder app. This is a good project to start getting comfortable with React basics and working with hooks.
I would also suggest watching John's video on the startup files for this project.
How to create a Tours Page

In this tutorial , you will learn how to create a tours page where the user can delete which tours they are not interested in.
This will give you practice with React hooks and the async/await pattern.
- try...catch statement
- async/await pattern

In this tutorial , you will learn how to create a questions and answers accordion menu. These menus can be helpful in revealing content to users in a progressive way.
- React icons

In this tutorial , you will learn how to create tabs for a mock portfolio page. Tabs are useful when you want to display different content in single page applications.

In this tutorial , you will learn how to create a review slider that changes to a new review every few seconds.
This is a cool feature that you can incorporate into an ecommerce site or portfolio.

In this tutorial , you will learn how to create a color generator. This is a good project to continue practicing working with hooks and setTimeout.
- clearTimeout()

In this tutorial , you will learn how to create a Stripe payment menu page. This project will give you good practice on how to design a product landing page using React components.
- useContext()

In this tutorial , you will learn how to create a shopping cart page that updates and deletes items. This project will also be a good introduction to the useReducer hook.
- <svg> elements
- useReducer()
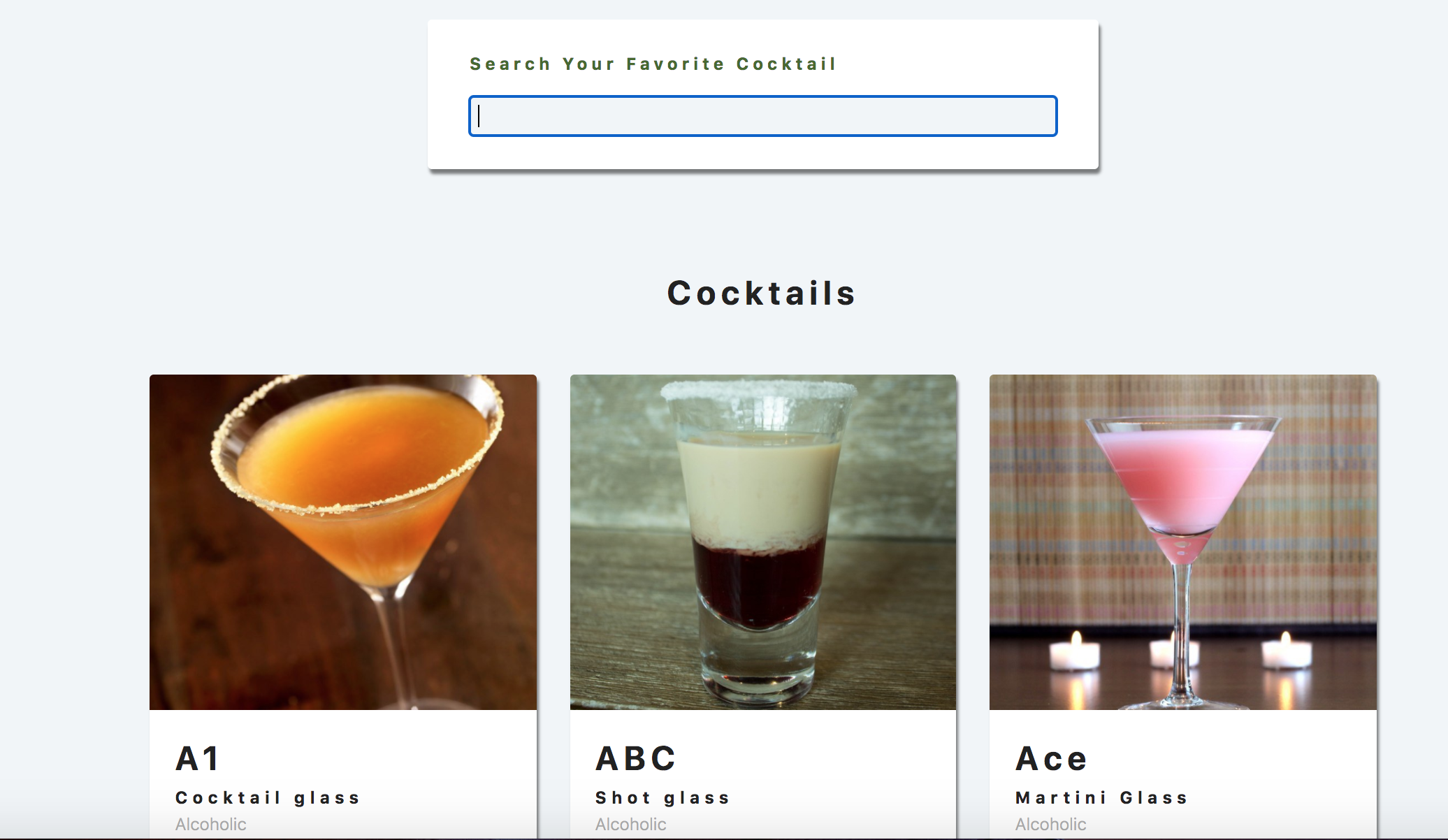
In this tutorial , you will learn how to create a cocktail search page. This project will give you an introduction to how to use React router.
React router gives you the ability to create a navigation on your website and change views to different components like an about or contact page.
- <Router>
- <Switch>
If you are unfamiliar with TypeScript, then I would suggest watching this course before proceeding with this project.
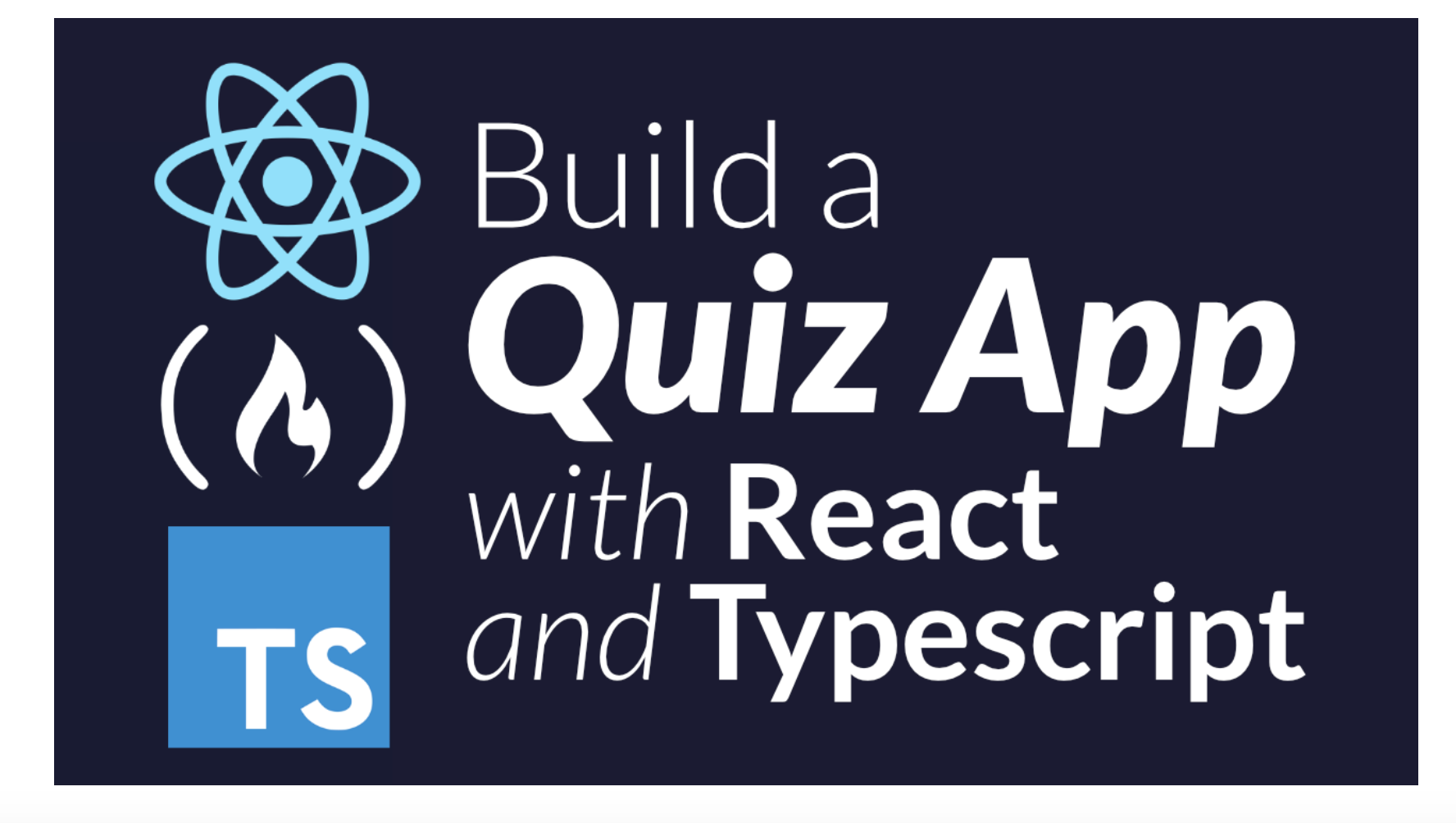
In this tutorial , Thomas Weibenfalk will teach you how to build a quiz app with React and TypeScript. This is a good opportunity to practice the basics of TypeScript.
- dangerouslySetInnerHTML

In this tutorial , Thomas Weibenfalk will teach you how to build the classic Arkanoid game in TypeScript. This is a good project that will give you practice working with the basic concepts for TypeScript.
- HTMLCanvasElement
I hope you enjoy this list of 40 project tutorials in Vanilla JavaScript, React and TypeScript.
Happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
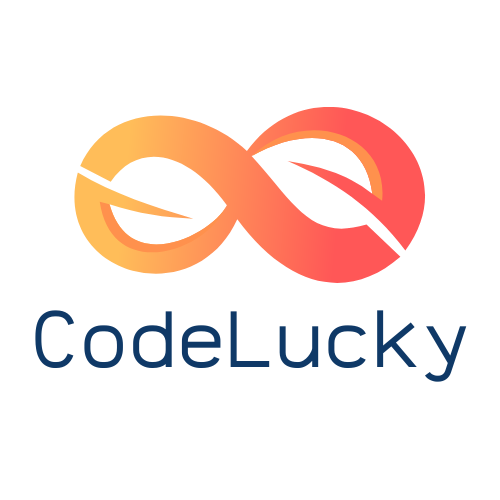
JavaScript Assignment: Variable Assignment Techniques
August 20, 2024
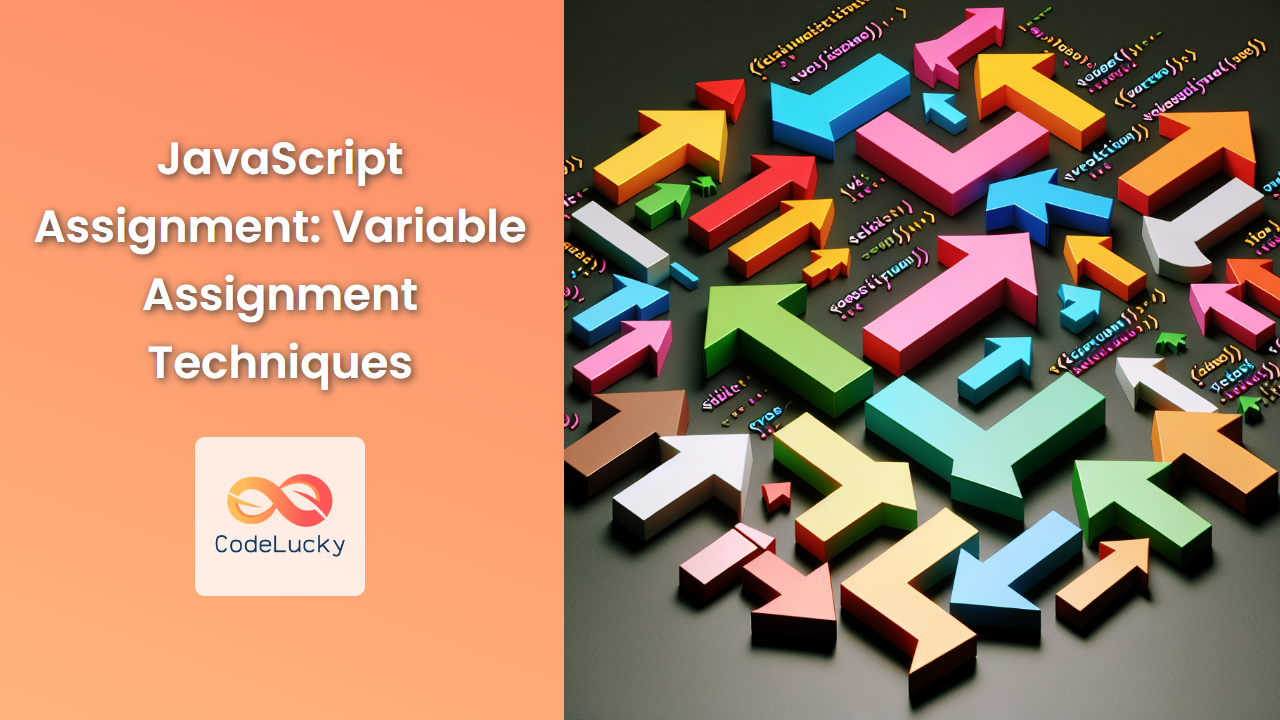
JavaScript, as a versatile and dynamic programming language, offers various ways to assign values to variables. Understanding these assignment techniques is crucial for writing efficient and clean code. In this comprehensive guide, we'll explore different methods of variable assignment in JavaScript, from basic to advanced techniques.
Table of Contents
- Basic Variable Assignment
Let's start with the fundamentals. In JavaScript, we use the = operator for basic variable assignment.
In this example, we're using three different keywords to declare variables: let , const , and var . Each has its own characteristics:
- let : Allows reassignment and is block-scoped.
- const : Creates a constant that can't be reassigned and is also block-scoped.
- var : Function-scoped or globally-scoped, and can be reassigned.
🔑 Key Point: Always use const by default, and only use let if you know the variable will be reassigned. Avoid var in modern JavaScript development.
- Multiple Variable Assignment
JavaScript allows you to assign multiple variables in a single line, which can make your code more concise.
This technique is particularly useful when initializing related variables. However, be cautious not to overuse this method, as it can make your code less readable if overdone.
Destructuring Assignment
Destructuring is a powerful feature in JavaScript that allows you to extract values from arrays or properties from objects and assign them to variables in a more convenient way.
- Array Destructuring
In this example, we're extracting the first two elements of the array into separate variables, and the rest into an array using the spread operator ( ... ).
- Object Destructuring
Here, we're extracting properties from the person object. Note how we can rename variables during destructuring (e.g., city to residence ).
🚀 Pro Tip: Destructuring is incredibly useful when working with complex data structures or when you need to extract specific values from function returns.
Assignment with Operators
JavaScript provides several operators that combine assignment with other operations. These can make your code more concise and efficient.
- Addition Assignment (+=)
- Subtraction Assignment (-=)
- Multiplication Assignment (*=)
- Division Assignment (/=)
- Remainder Assignment (%=)
These compound assignment operators are not just syntactic sugar; they can lead to more efficient code execution in certain scenarios.
Logical Assignment Operators
Introduced in ECMAScript 2021, logical assignment operators combine logical operations with assignment.
- Logical AND Assignment (&&=)
- Logical OR Assignment (||=)
- Nullish Coalescing Assignment (??=)
These logical assignment operators are particularly useful for providing default values or conditionally updating variables.
- Dynamic Property Assignment
JavaScript's flexibility allows for dynamic property assignment in objects.
This technique is powerful when you need to assign properties to an object based on variables or dynamic conditions.
- Assignment in Conditional Statements
You can perform assignments within conditional statements, although this practice should be used judiciously to maintain code readability.
In this example, someFunction() is called and its return value is assigned to result . The if statement then evaluates result .
⚠️ Warning: While this technique can be useful, it can also lead to confusion and bugs if not used carefully. Always prioritize code clarity.
- Chained Assignment
Chained assignment allows you to assign the same value to multiple variables in one line.
This works because the assignment operator ( = ) returns the assigned value. However, be cautious with this technique, especially with complex values, as it can lead to unexpected behavior with reference types.
- Assignment with Computed Property Names
ECMAScript 6 introduced computed property names, allowing you to use expressions for property names in object literals.
This feature is particularly useful when you need to create property names dynamically.
Mastering these variable assignment techniques in JavaScript will significantly enhance your coding skills. From basic assignments to advanced destructuring and logical operators, each method has its place in writing efficient, readable, and maintainable code.
Remember, the key to becoming a proficient JavaScript developer is not just knowing these techniques, but understanding when and how to apply them effectively. Practice these methods in your projects, and you'll find yourself writing more elegant and powerful JavaScript code.
🌟 Pro Tip: Always consider the readability and maintainability of your code when choosing an assignment technique. Sometimes, a simple approach is the best approach.
As you continue your JavaScript journey, keep exploring and experimenting with these techniques. The more you practice, the more natural and intuitive they'll become, allowing you to write JavaScript code that's not just functional, but also clean, efficient, and expressive.
Continue Reading
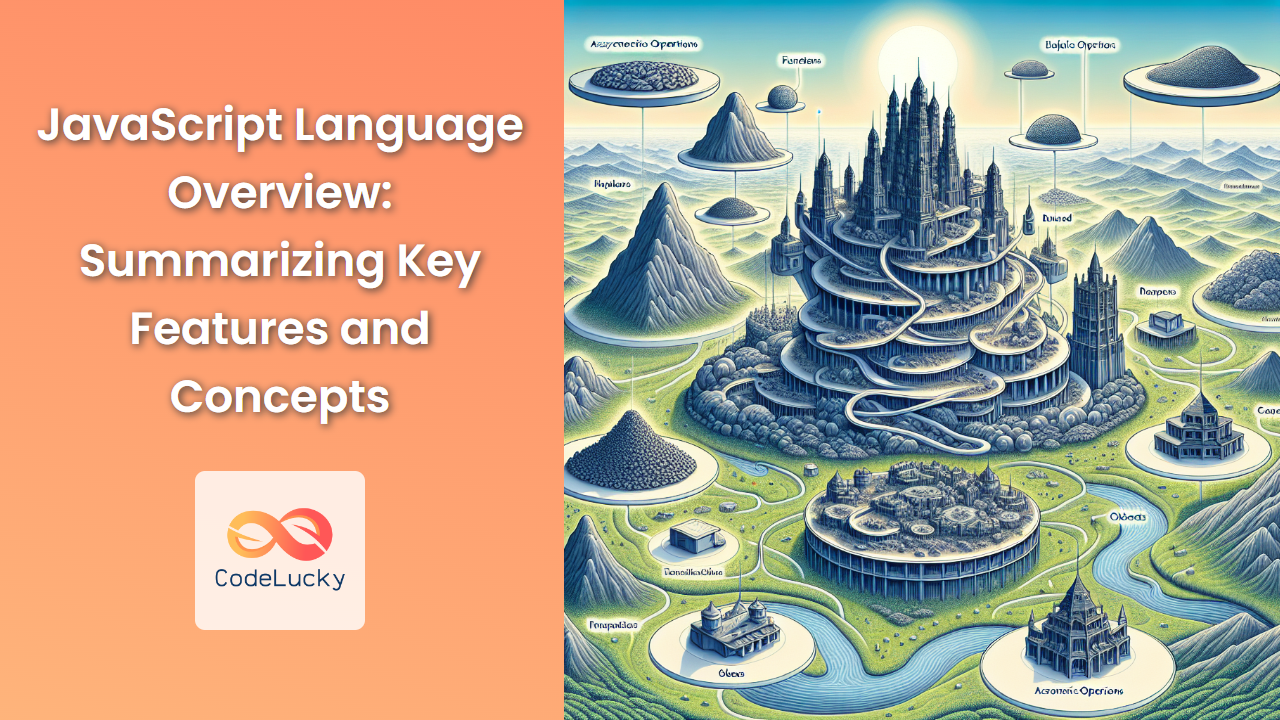
JavaScript Language Overview: Summarizing Key Features and Concepts
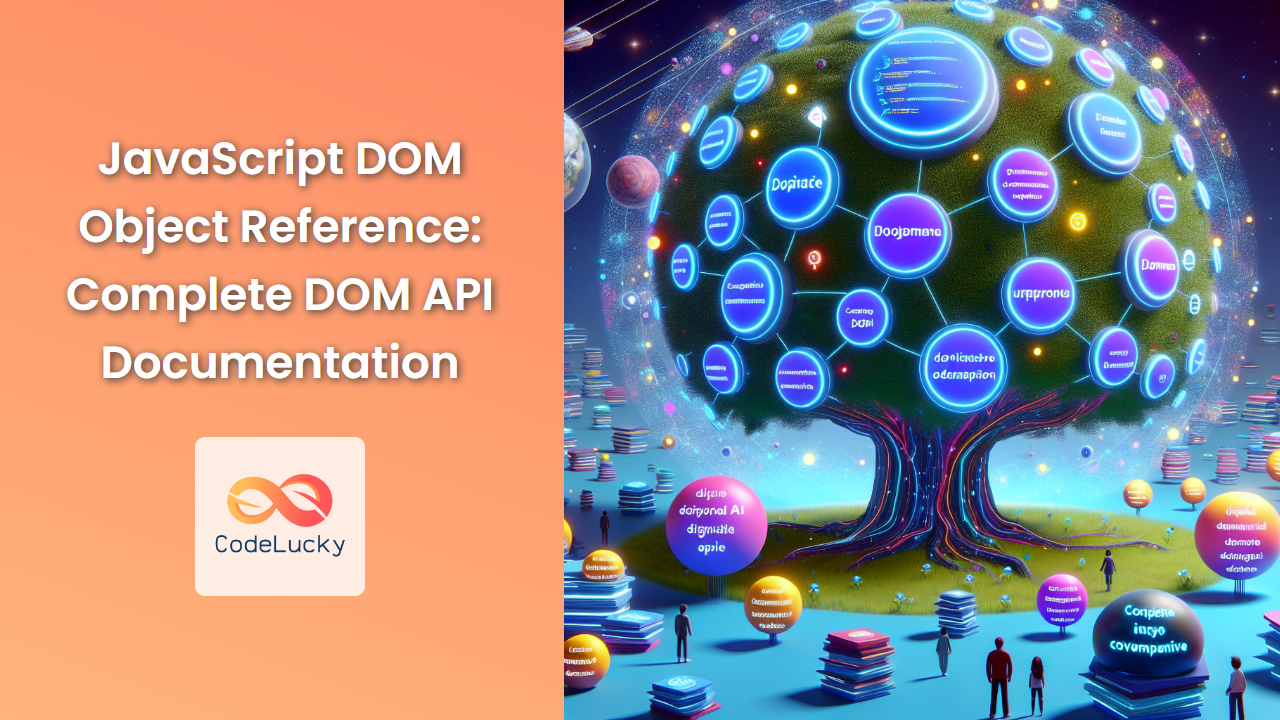
JavaScript DOM Object Reference: Complete DOM API Documentation
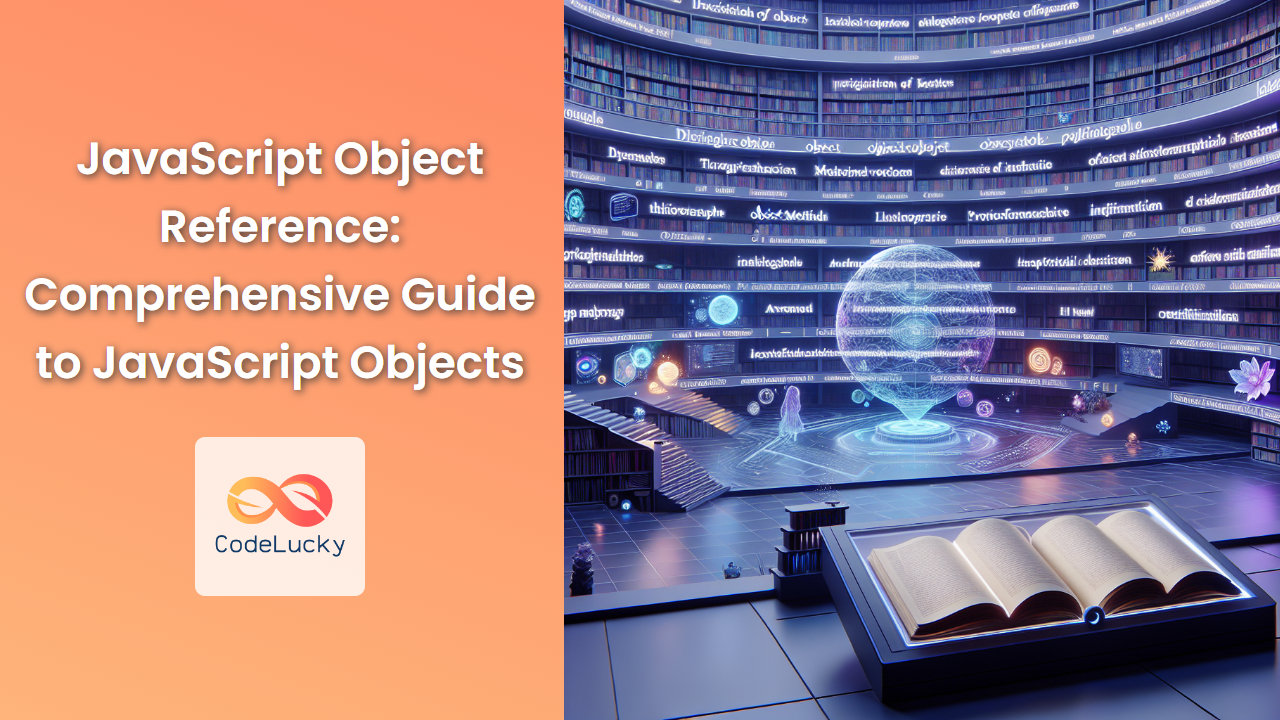
JavaScript Object Reference: Comprehensive Guide to JavaScript Objects
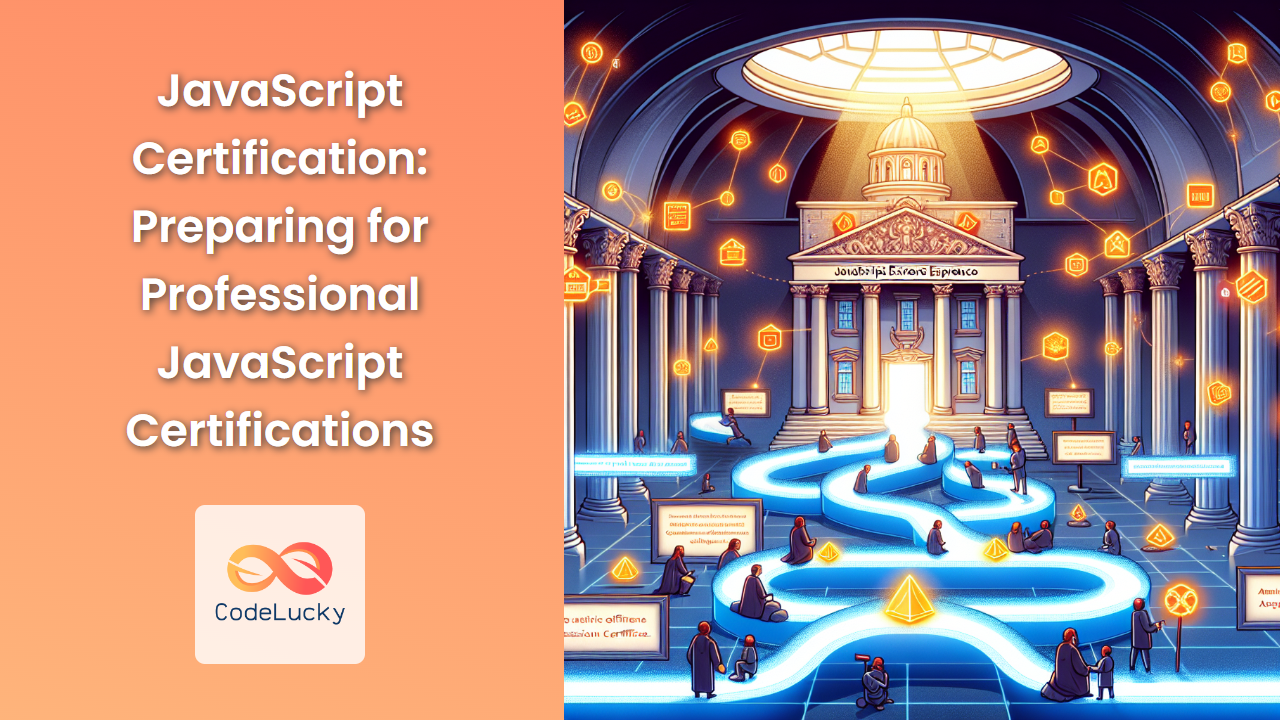
JavaScript Certification: Preparing for Professional JavaScript Certifications
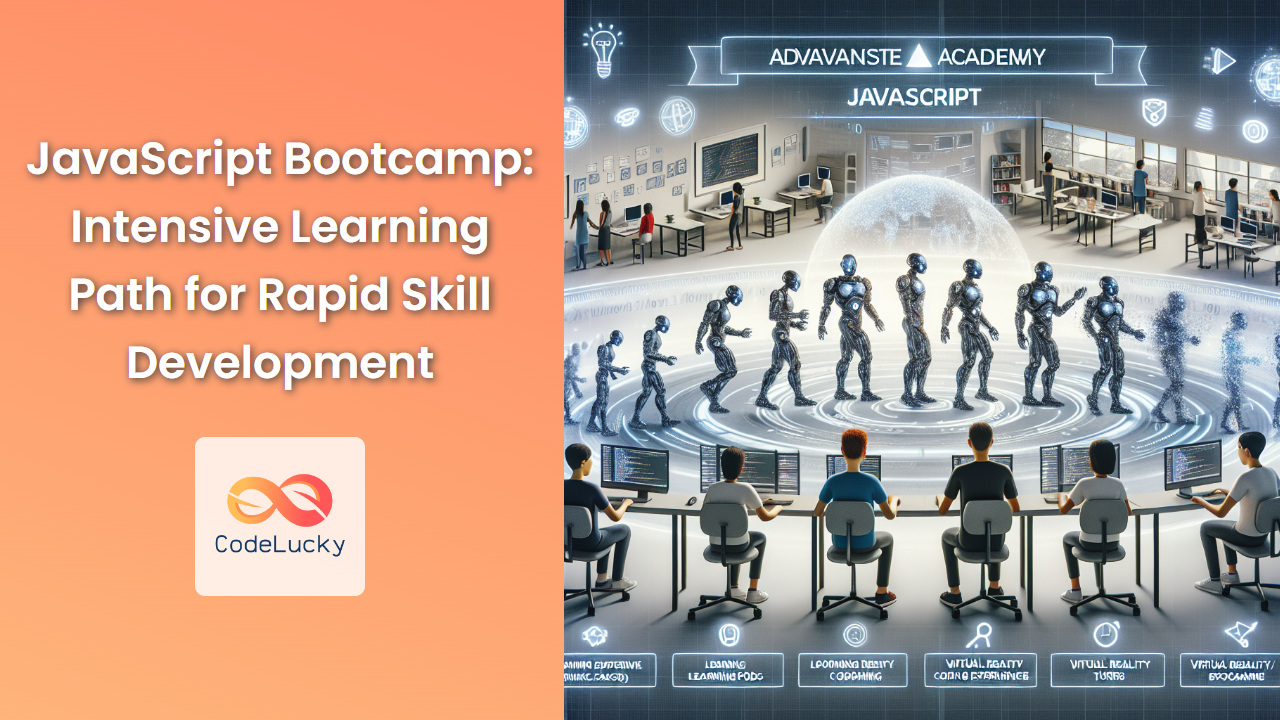
JavaScript Bootcamp: Intensive Learning Path for Rapid Skill Development
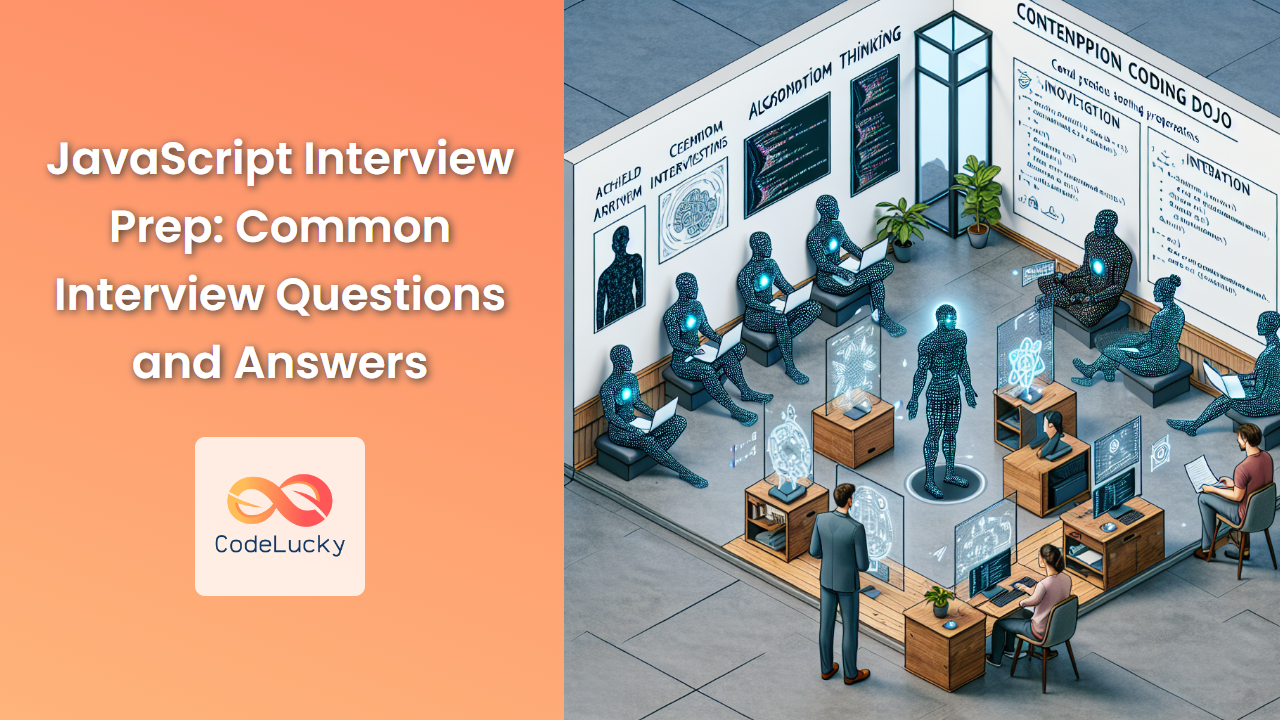
JavaScript Interview Prep: Common Interview Questions and Answers
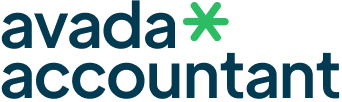
Innovative IT and Ed-Tech Solutions to Empower Your Business and Shape the Future.
© 2024 • CodeLucky IT Services
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
Logical OR assignment (||=)
The logical OR assignment ( ||= ) operator only evaluates the right operand and assigns to the left if the left operand is falsy .
Description
Logical OR assignment short-circuits , meaning that x ||= y is equivalent to x || (x = y) , except that the expression x is only evaluated once.
No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const :
Neither would the following trigger the setter:
In fact, if x is not falsy, y is not evaluated at all.
Setting default content
If the "lyrics" element is empty, display a default value:
Here the short-circuit is especially beneficial, since the element will not be updated unnecessarily and won't cause unwanted side-effects such as additional parsing or rendering work, or loss of focus, etc.
Note: Pay attention to the value returned by the API you're checking against. If an empty string is returned (a falsy value), ||= must be used, so that "No lyrics." is displayed instead of a blank space. However, if the API returns null or undefined in case of blank content, ??= should be used instead.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Logical OR ( || )
- Nullish coalescing operator ( ?? )
- Bitwise OR assignment ( |= )
Biotech & Health
Cloud Computing
Fundraising
Government & Policy
Media & Entertainment
Transportation
More from TechCrunch
Startup Battlefield
Newsletters
Partner Content
TechCrunch Brand Studio
Crunchboard

Anthropic’s AI can now run and write code
Anthropic’s Claude chatbot can now write and run JavaScript code.
Today, Anthropic launched a new analysis tool that helps Claude respond with what the company describes as “mathematically precise and reproducible answers.” With the tool enabled — it’s currently in preview — Claude can perform calculations and analyze data from files like spreadsheets and PDFs, rendering the results as interactive visualizations.
“Think of the analysis tool as a built-in code sandbox, where Claude can do complex math, analyze data, and iterate on different ideas before sharing an answer,” Anthropic wrote in a blog post. “Instead of relying on abstract analysis alone, it can systematically process your data — cleaning, exploring, and analyzing it step-by-step until it reaches the correct result.”
Anthropic gives a few examples of where this might be useful. For instance, a product manager could upload sales data and ask Claude for country-specific performance analysis, while an engineer could give Claude monthly financial data and have it create a dashboard highlighting key trends.
Claude could attempt these tasks before. But, because it lacked a mechanism to mathematically verify the results, the answers weren’t always incredibly accurate.
Google offers a comparable feature for its Gemini models called Code Execution, which lets the models generate and run Python code to learn iteratively from the results. OpenAI’s flagship models similarly can write and execute code via a capability that the company calls Advanced Data Analysis .
Anthropic’s analysis tool is available for all Claude users on the web as of Thursday. To turn it on, log into Claude.ai and click here.
Most Popular
Unitedhealth says change healthcare hack affects over 100 million, the largest-ever us healthcare data breach.
- Zack Whittaker
Here is Notion’s email client
- Frederic Lardinois
Notion’s email product is nearing launch
- Sarah Perez
X’s India revenue falls 90%, filing shows
- Manish Singh
Tesla Cybertruck pushes past Ford Mach-E to become third best-selling EV in America
- Sean O'Kane
Bookcase can turn an iPhone into an e-reader
- Brian Heater
Amazon adds gasoline discounts for Prime members, EV charging savings to follow
- Lauren Forristal
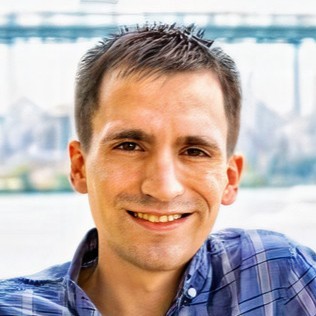
Senior Reporter, Enterprise
Subscribe for the industry’s biggest tech news
TechCrunch Daily News
Every weekday and Sunday, you can get the best of TechCrunch’s coverage.
TechCrunch AI
TechCrunch's AI experts cover the latest news in the fast-moving field.
TechCrunch Space
Every Monday, gets you up to speed on the latest advances in aerospace.
Startups Weekly
Startups are the core of TechCrunch, so get our best coverage delivered weekly.
By submitting your email, you agree to our Terms and Privacy Notice .

Latest in AI

‘They wish this technology didn’t exist’: Perplexity responds to News Corp’s lawsuit
- Maxwell Zeff

Apple will pay security researchers up to $1 million to hack its private AI cloud

Google adds new disclosures for AI photos, but it’s still not obvious at first glance
JS Reference
Html events, html objects, other references, javascript object.assign(), description.
The Object.assign() method copies properties from one or more source objects to a target object.
Related Methods:
Object.assign() copies properties from a source object to a target object.
Object.create() creates an object from an existing object.
Object.fromEntries() creates an object from a list of keys/values.
Return Value
Advertisement
Browser Support
Object.assign() is an ECMAScript6 (ES6) feature.
ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
Object.assign() is not supported in Internet Explorer.
Object Tutorials
JavaScript Objects
JavaScript Object Definition
JavaScript Object Methods
JavaScript Object Properties

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
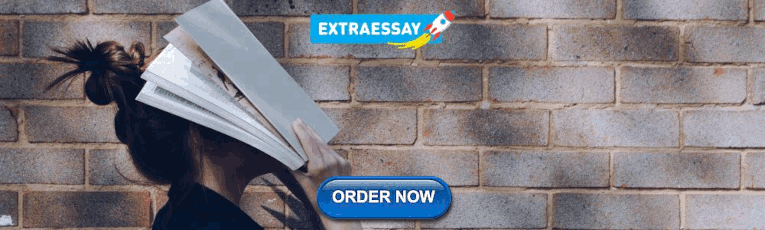
IMAGES
VIDEO
COMMENTS
With our online code editor, you can edit code and view the result in your browser. Videos. Learn the basics of HTML in a fun and engaging video tutorial. ... JavaScript Assignment Operators. Assignment operators assign values to JavaScript variables. Operator Example Same As = x = y: x = y += x += y: x = x + y-= x -= y: x = x - y *= x *= y: x ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
The assignment (=) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. ... (globalThis, "String")), you can use the following code: js. function foo() { String("s"); // The function `String` is globally available } ... Note that the implication of the above is ...
JavaScript is a cross-platform, object-oriented scripting language. It is a small and lightweight language. Inside a host environment ( a web browser), JavaScript can be connected to the objects of its environment to provide programmatic control over them. JavaScript contains a standard library of objects, such as Array, Date, and Math, and a ...
Benefits of Practice JavaScript. Master JavaScript through engaging exercises that offer: Hands-on learning with interactive quizzes. Progress tracking to monitor your improvement. Effective skill enhancement at your own pace. Immediate feedback for quick learning. Access anytime, anywhere for convenient learning.
A student learning JavaScript was trying to make a function. His code should concatenate a passed string name with string "Edabit" and stores it in a variable called result. He needs your help to fix this code. Examples nameString("Mubashir") "MubashirEdabit" nameString("Matt") "MattEdabit" nameString("javaScript") …
The Destructuring assignment is the important technique introduced in ECMAScript 2015 (ES6) version of JavaScript that provides a shorthand syntax to extract or unpack array elements or properties of an object into distinct variables using a single line of code.
An assignment operator (=) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
JavaScript Booleans. Display the value of Boolean (10 > 9) Display the value of 10 > 9 Everything with a real value is true The Boolean value of zero is false The Boolean value of minus zero is false The Boolean value of an empty string is false The Boolean value of undefined is false The Boolean value of null is false The Boolean value of ...
Learn to code solving problems and writing code with our hands-on JavaScript course. Learn to code solving problems with our hands-on JavaScript course! Try Programiz PRO today. Sale ends in . ... JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5;
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript. Assignment Operators. In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
Get certified by completing the JavaScript course. Track your progress - it's free! Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Answer. Method 1. arrayList = []; Above code will set the variable arrayList to a new empty array. This is recommended if you don't have references to the original array arrayList anywhere else because It will actually create a new empty array.
40 JavaScript Projects for Beginners - Easy Ideas to Get Started Coding JS. The best way to learn a new programming language is to build projects. I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript. My advice for tutorials would be to watch the video, build the project, break it apart ...
JavaScript, as a versatile and dynamic programming language, offers various ways to assign values to variables. Understanding these assignment techniques is crucial for writing efficient and clean code. In this comprehensive guide, we'll explore different methods of variable assignment in JavaScript, from basic to advanced techniques.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. ... = 1; console.log(a, toFixed); // undefined ƒ toFixed() { [native code] } Same as accessing properties, ...
The var keyword was used in all JavaScript code from 1995 to 2015. The let and const keywords were added to JavaScript in 2015. ... The Assignment Operator. In JavaScript, the equal sign (=) is an "assignment" operator, not an "equal to" operator. This is different from algebra. The following does not make sense in algebra:
The logical OR assignment (||=) operator only evaluates the right operand and assigns to the left if the left operand is falsy. Try it. Syntax. js. x ||= y. Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once.
Code Editor (Try it) ... Large collection of code snippets for HTML, CSS and JavaScript. CSS Framework. Build fast and responsive sites using our free W3.CSS framework Browser Statistics. Read long term trends of browser usage ... Destructuring Assignment Syntax.
Anthropic's Claude chatbot can now write and run JavaScript code. Today, Anthropic launched a new analysis tool that helps Claude respond with what the company describes as "mathematically ...
Related Methods: Object.assign () copies properties from a source object to a target object. Object.create () creates an object from an existing object. Object.fromEntries () creates an object from a list of keys/values.