Home » Python Basics » Python Ternary Operator
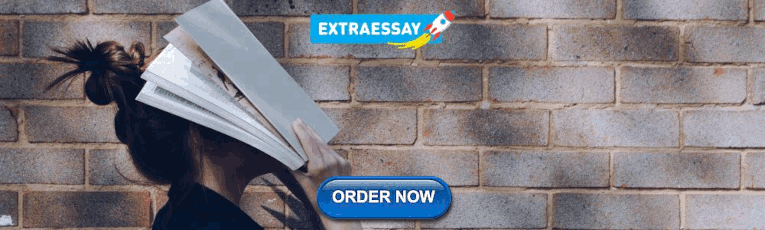
Python Ternary Operator
Summary : in this tutorial, you’ll learn about the Python ternary operator and how to use it to make your code more concise.
Introduction to Python Ternary Operator
The following program prompts you for your age and determines the ticket price based on it:
Here is the output when you enter 18:
In this example, the following if...else statement assigns 20 to the ticket_price if the age is greater than or equal to 18. Otherwise, it assigns the ticket_price 5:
To make it more concise, you can use an alternative syntax like this:
In this statement, the left side of the assignment operator ( = ) is the variable ticket_price .
The expression on the right side returns 20 if the age is greater than or equal to 18 or 5 otherwise.
The following syntax is called a ternary operator in Python:
The ternary operator evaluates the condition . If the result is True , it returns the value_if_true . Otherwise, it returns the value_if_false .
The ternary operator is equivalent to the following if...else statement:
Note that you have been programming languages such as C# or Java, and you’re familiar with the following ternary operator syntax:
However, Python doesn’t support this ternary operator syntax.
The following program uses the ternary operator instead of the if statement:
- The Python ternary operator is value_if_true if condition else value_if_false .
- Use the ternary operator to make your code more concise.

Python Ternary Operator - If Else One Line
In this tutorial, we will learn about the Python ternary operator. Moreover, we will look at its syntax, usage, and examples.
Table Of Contents - Python Ternary Operator
Ternary operator syntax
- python ternary operator example
- python ternary assignment
- Nested ternary operator
- python ternary operator multiple conditions
- python ternary operator without else
Ternary Operator In Python
The ternary operator in Python is a conditional operator that has three operands. The ternary operator is used to evaluate a condition and return either of the two values depending on the condition.
The ternary operator is just like a python if else statement but more clean, concise, and easy to understand.
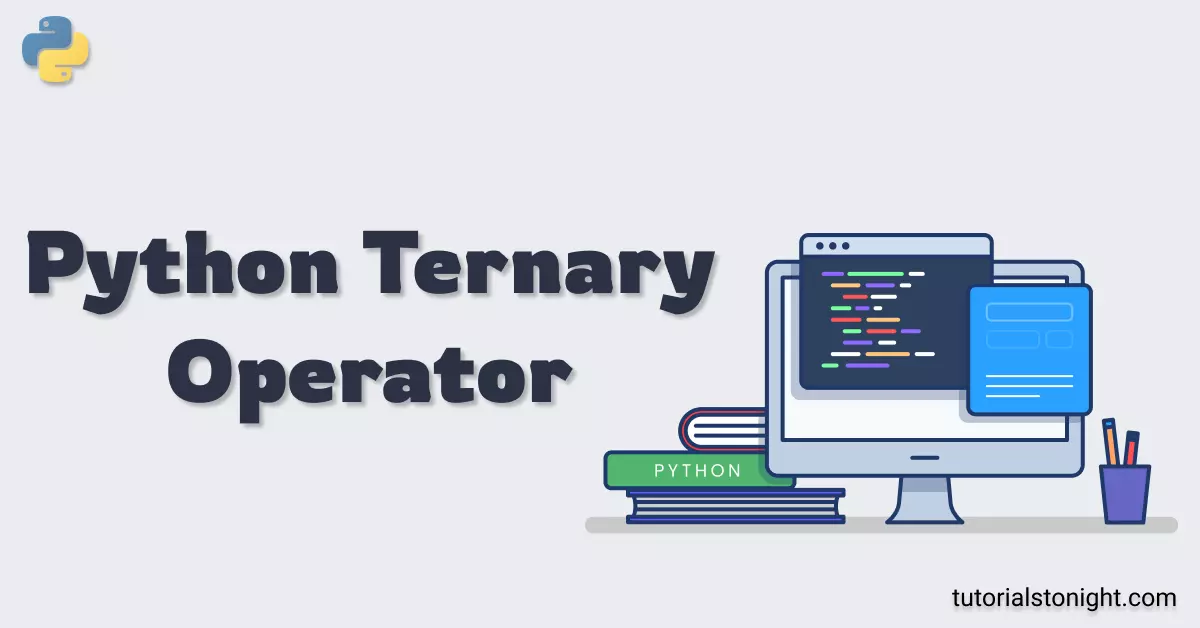
The syntax of Python ternary operator is as follows:
The 'condition' is the condition that is to be evaluated. The 'expression1' and 'expression2' are the expressions that are evaluated based on the condition.
The first condition is evaluated. If the condition is true, then the 'expression1' is evaluated and the value is returned. Otherwise, the 'expression2' is evaluated and the value is returned.
Note : Python ternary operator is the same as condition ? expression1 : expression2 in other programming languages like C, C++, JavaScript, etc.
Python Ternary Operator Example
The following example shows the usage of the ternary operator in python and also compares its code with the if-else statement.
- Ternary Operator
Here is another simple example of a ternary operator.
a is less than b
The above example compares the value of a and b, if a is greater than b then it prints "a is greater than b" otherwise it prints "a is less than b".
Python Ternary Assignment
The ternary operator is mostly used in assigning values to variables . When you have to decide different values of a variable based on the condition, then you can use the ternary operator.
Using a ternary operator for assigning values also makes the code more readable and concise.
Nested Ternary Operator
The ternary operator can also be nested. This means you can have a ternary operator inside another ternary operator.
A nested ternary operator is used to evaluate results based on multiple conditions.
Code explanation: Return the value of a if a is greater than b [ a if a > b else (b if a < b else a) ] , else return the value of b if a is less than b, else return the value of a [ b if a < b else a ].
Here is an example of 3 nested ternary operators.
Python Ternary Operator Multiple Conditions
You can also use multiple conditions in a ternary operator by using logical operator keywords like and , or , not
Separate as many conditions with logical operators like and , or , not and wrap them in parenthesis.
The above code checks if a and b are divisible by 2, 3, and 5 using multiple conditions. If all conditions are true then it prints "Yes" otherwise it prints "No".
Python Ternary Operator Without Else
Python does not allow using ternary operator without else.
But you can create similar functionality by using the and operator.
Here is an example of that:
Code explanation
- The above code has the format of True/False and string
- If the first statement is false then the and operator will return False and the second statement will not be executed.
- If the first statement is true then the and operator will return second statement.
Python Ternary Operator Conclusion
Ternary operator in python is an alternate way of writing if-else which is concise and simple.
It first evaluates the given condition, then executes expression based on the condition then returns the value.
The ternary operator is not always useful. It is helpful when you have only one statement to execute after the condition is evaluated.
- Python »
- 3.10.13 Documentation »
- The Python Language Reference »
- 6. Expressions
- Theme Auto Light Dark |
6. Expressions ¶
This chapter explains the meaning of the elements of expressions in Python.
Syntax Notes: In this and the following chapters, extended BNF notation will be used to describe syntax, not lexical analysis. When (one alternative of) a syntax rule has the form
and no semantics are given, the semantics of this form of name are the same as for othername .
6.1. Arithmetic conversions ¶
When a description of an arithmetic operator below uses the phrase “the numeric arguments are converted to a common type”, this means that the operator implementation for built-in types works as follows:
If either argument is a complex number, the other is converted to complex;
otherwise, if either argument is a floating point number, the other is converted to floating point;
otherwise, both must be integers and no conversion is necessary.
Some additional rules apply for certain operators (e.g., a string as a left argument to the ‘%’ operator). Extensions must define their own conversion behavior.
6.2. Atoms ¶
Atoms are the most basic elements of expressions. The simplest atoms are identifiers or literals. Forms enclosed in parentheses, brackets or braces are also categorized syntactically as atoms. The syntax for atoms is:
6.2.1. Identifiers (Names) ¶
An identifier occurring as an atom is a name. See section Identifiers and keywords for lexical definition and section Naming and binding for documentation of naming and binding.
When the name is bound to an object, evaluation of the atom yields that object. When a name is not bound, an attempt to evaluate it raises a NameError exception.
Private name mangling: When an identifier that textually occurs in a class definition begins with two or more underscore characters and does not end in two or more underscores, it is considered a private name of that class. Private names are transformed to a longer form before code is generated for them. The transformation inserts the class name, with leading underscores removed and a single underscore inserted, in front of the name. For example, the identifier __spam occurring in a class named Ham will be transformed to _Ham__spam . This transformation is independent of the syntactical context in which the identifier is used. If the transformed name is extremely long (longer than 255 characters), implementation defined truncation may happen. If the class name consists only of underscores, no transformation is done.
6.2.2. Literals ¶
Python supports string and bytes literals and various numeric literals:
Evaluation of a literal yields an object of the given type (string, bytes, integer, floating point number, complex number) with the given value. The value may be approximated in the case of floating point and imaginary (complex) literals. See section Literals for details.
All literals correspond to immutable data types, and hence the object’s identity is less important than its value. Multiple evaluations of literals with the same value (either the same occurrence in the program text or a different occurrence) may obtain the same object or a different object with the same value.
6.2.3. Parenthesized forms ¶
A parenthesized form is an optional expression list enclosed in parentheses:
A parenthesized expression list yields whatever that expression list yields: if the list contains at least one comma, it yields a tuple; otherwise, it yields the single expression that makes up the expression list.
An empty pair of parentheses yields an empty tuple object. Since tuples are immutable, the same rules as for literals apply (i.e., two occurrences of the empty tuple may or may not yield the same object).
Note that tuples are not formed by the parentheses, but rather by use of the comma operator. The exception is the empty tuple, for which parentheses are required — allowing unparenthesized “nothing” in expressions would cause ambiguities and allow common typos to pass uncaught.
6.2.4. Displays for lists, sets and dictionaries ¶
For constructing a list, a set or a dictionary Python provides special syntax called “displays”, each of them in two flavors:
either the container contents are listed explicitly, or
they are computed via a set of looping and filtering instructions, called a comprehension .
Common syntax elements for comprehensions are:
The comprehension consists of a single expression followed by at least one for clause and zero or more for or if clauses. In this case, the elements of the new container are those that would be produced by considering each of the for or if clauses a block, nesting from left to right, and evaluating the expression to produce an element each time the innermost block is reached.
However, aside from the iterable expression in the leftmost for clause, the comprehension is executed in a separate implicitly nested scope. This ensures that names assigned to in the target list don’t “leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated directly in the enclosing scope and then passed as an argument to the implicitly nested scope. Subsequent for clauses and any filter condition in the leftmost for clause cannot be evaluated in the enclosing scope as they may depend on the values obtained from the leftmost iterable. For example: [x*y for x in range(10) for y in range(x, x+10)] .
To ensure the comprehension always results in a container of the appropriate type, yield and yield from expressions are prohibited in the implicitly nested scope.
Since Python 3.6, in an async def function, an async for clause may be used to iterate over a asynchronous iterator . A comprehension in an async def function may consist of either a for or async for clause following the leading expression, may contain additional for or async for clauses, and may also use await expressions. If a comprehension contains either async for clauses or await expressions it is called an asynchronous comprehension . An asynchronous comprehension may suspend the execution of the coroutine function in which it appears. See also PEP 530 .
New in version 3.6: Asynchronous comprehensions were introduced.
Changed in version 3.8: yield and yield from prohibited in the implicitly nested scope.
6.2.5. List displays ¶
A list display is a possibly empty series of expressions enclosed in square brackets:
A list display yields a new list object, the contents being specified by either a list of expressions or a comprehension. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and placed into the list object in that order. When a comprehension is supplied, the list is constructed from the elements resulting from the comprehension.
6.2.6. Set displays ¶
A set display is denoted by curly braces and distinguishable from dictionary displays by the lack of colons separating keys and values:
A set display yields a new mutable set object, the contents being specified by either a sequence of expressions or a comprehension. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and added to the set object. When a comprehension is supplied, the set is constructed from the elements resulting from the comprehension.
An empty set cannot be constructed with {} ; this literal constructs an empty dictionary.
6.2.7. Dictionary displays ¶
A dictionary display is a possibly empty series of key/datum pairs enclosed in curly braces:
A dictionary display yields a new dictionary object.
If a comma-separated sequence of key/datum pairs is given, they are evaluated from left to right to define the entries of the dictionary: each key object is used as a key into the dictionary to store the corresponding datum. This means that you can specify the same key multiple times in the key/datum list, and the final dictionary’s value for that key will be the last one given.
A double asterisk ** denotes dictionary unpacking . Its operand must be a mapping . Each mapping item is added to the new dictionary. Later values replace values already set by earlier key/datum pairs and earlier dictionary unpackings.
New in version 3.5: Unpacking into dictionary displays, originally proposed by PEP 448 .
A dict comprehension, in contrast to list and set comprehensions, needs two expressions separated with a colon followed by the usual “for” and “if” clauses. When the comprehension is run, the resulting key and value elements are inserted in the new dictionary in the order they are produced.
Restrictions on the types of the key values are listed earlier in section The standard type hierarchy . (To summarize, the key type should be hashable , which excludes all mutable objects.) Clashes between duplicate keys are not detected; the last datum (textually rightmost in the display) stored for a given key value prevails.
Changed in version 3.8: Prior to Python 3.8, in dict comprehensions, the evaluation order of key and value was not well-defined. In CPython, the value was evaluated before the key. Starting with 3.8, the key is evaluated before the value, as proposed by PEP 572 .
6.2.8. Generator expressions ¶
A generator expression is a compact generator notation in parentheses:
A generator expression yields a new generator object. Its syntax is the same as for comprehensions, except that it is enclosed in parentheses instead of brackets or curly braces.
Variables used in the generator expression are evaluated lazily when the __next__() method is called for the generator object (in the same fashion as normal generators). However, the iterable expression in the leftmost for clause is immediately evaluated, so that an error produced by it will be emitted at the point where the generator expression is defined, rather than at the point where the first value is retrieved. Subsequent for clauses and any filter condition in the leftmost for clause cannot be evaluated in the enclosing scope as they may depend on the values obtained from the leftmost iterable. For example: (x*y for x in range(10) for y in range(x, x+10)) .
The parentheses can be omitted on calls with only one argument. See section Calls for details.
To avoid interfering with the expected operation of the generator expression itself, yield and yield from expressions are prohibited in the implicitly defined generator.
If a generator expression contains either async for clauses or await expressions it is called an asynchronous generator expression . An asynchronous generator expression returns a new asynchronous generator object, which is an asynchronous iterator (see Asynchronous Iterators ).
New in version 3.6: Asynchronous generator expressions were introduced.
Changed in version 3.7: Prior to Python 3.7, asynchronous generator expressions could only appear in async def coroutines. Starting with 3.7, any function can use asynchronous generator expressions.
6.2.9. Yield expressions ¶
The yield expression is used when defining a generator function or an asynchronous generator function and thus can only be used in the body of a function definition. Using a yield expression in a function’s body causes that function to be a generator function, and using it in an async def function’s body causes that coroutine function to be an asynchronous generator function. For example:
Due to their side effects on the containing scope, yield expressions are not permitted as part of the implicitly defined scopes used to implement comprehensions and generator expressions.
Changed in version 3.8: Yield expressions prohibited in the implicitly nested scopes used to implement comprehensions and generator expressions.
Generator functions are described below, while asynchronous generator functions are described separately in section Asynchronous generator functions .
When a generator function is called, it returns an iterator known as a generator. That generator then controls the execution of the generator function. The execution starts when one of the generator’s methods is called. At that time, the execution proceeds to the first yield expression, where it is suspended again, returning the value of expression_list to the generator’s caller, or None if expression_list is omitted. By suspended, we mean that all local state is retained, including the current bindings of local variables, the instruction pointer, the internal evaluation stack, and the state of any exception handling. When the execution is resumed by calling one of the generator’s methods, the function can proceed exactly as if the yield expression were just another external call. The value of the yield expression after resuming depends on the method which resumed the execution. If __next__() is used (typically via either a for or the next() builtin) then the result is None . Otherwise, if send() is used, then the result will be the value passed in to that method.
All of this makes generator functions quite similar to coroutines; they yield multiple times, they have more than one entry point and their execution can be suspended. The only difference is that a generator function cannot control where the execution should continue after it yields; the control is always transferred to the generator’s caller.
Yield expressions are allowed anywhere in a try construct. If the generator is not resumed before it is finalized (by reaching a zero reference count or by being garbage collected), the generator-iterator’s close() method will be called, allowing any pending finally clauses to execute.
When yield from <expr> is used, the supplied expression must be an iterable. The values produced by iterating that iterable are passed directly to the caller of the current generator’s methods. Any values passed in with send() and any exceptions passed in with throw() are passed to the underlying iterator if it has the appropriate methods. If this is not the case, then send() will raise AttributeError or TypeError , while throw() will just raise the passed in exception immediately.
When the underlying iterator is complete, the value attribute of the raised StopIteration instance becomes the value of the yield expression. It can be either set explicitly when raising StopIteration , or automatically when the subiterator is a generator (by returning a value from the subgenerator).
Changed in version 3.3: Added yield from <expr> to delegate control flow to a subiterator.
The parentheses may be omitted when the yield expression is the sole expression on the right hand side of an assignment statement.
The proposal for adding generators and the yield statement to Python.
The proposal to enhance the API and syntax of generators, making them usable as simple coroutines.
The proposal to introduce the yield_from syntax, making delegation to subgenerators easy.
The proposal that expanded on PEP 492 by adding generator capabilities to coroutine functions.
6.2.9.1. Generator-iterator methods ¶
This subsection describes the methods of a generator iterator. They can be used to control the execution of a generator function.
Note that calling any of the generator methods below when the generator is already executing raises a ValueError exception.
Starts the execution of a generator function or resumes it at the last executed yield expression. When a generator function is resumed with a __next__() method, the current yield expression always evaluates to None . The execution then continues to the next yield expression, where the generator is suspended again, and the value of the expression_list is returned to __next__() ’s caller. If the generator exits without yielding another value, a StopIteration exception is raised.
This method is normally called implicitly, e.g. by a for loop, or by the built-in next() function.
Resumes the execution and “sends” a value into the generator function. The value argument becomes the result of the current yield expression. The send() method returns the next value yielded by the generator, or raises StopIteration if the generator exits without yielding another value. When send() is called to start the generator, it must be called with None as the argument, because there is no yield expression that could receive the value.
Raises an exception at the point where the generator was paused, and returns the next value yielded by the generator function. If the generator exits without yielding another value, a StopIteration exception is raised. If the generator function does not catch the passed-in exception, or raises a different exception, then that exception propagates to the caller.
In typical use, this is called with a single exception instance similar to the way the raise keyword is used.
For backwards compatibility, however, the second signature is supported, following a convention from older versions of Python. The type argument should be an exception class, and value should be an exception instance. If the value is not provided, the type constructor is called to get an instance. If traceback is provided, it is set on the exception, otherwise any existing __traceback__ attribute stored in value may be cleared.
Raises a GeneratorExit at the point where the generator function was paused. If the generator function then exits gracefully, is already closed, or raises GeneratorExit (by not catching the exception), close returns to its caller. If the generator yields a value, a RuntimeError is raised. If the generator raises any other exception, it is propagated to the caller. close() does nothing if the generator has already exited due to an exception or normal exit.
6.2.9.2. Examples ¶
Here is a simple example that demonstrates the behavior of generators and generator functions:
For examples using yield from , see PEP 380: Syntax for Delegating to a Subgenerator in “What’s New in Python.”
6.2.9.3. Asynchronous generator functions ¶
The presence of a yield expression in a function or method defined using async def further defines the function as an asynchronous generator function.
When an asynchronous generator function is called, it returns an asynchronous iterator known as an asynchronous generator object. That object then controls the execution of the generator function. An asynchronous generator object is typically used in an async for statement in a coroutine function analogously to how a generator object would be used in a for statement.
Calling one of the asynchronous generator’s methods returns an awaitable object, and the execution starts when this object is awaited on. At that time, the execution proceeds to the first yield expression, where it is suspended again, returning the value of expression_list to the awaiting coroutine. As with a generator, suspension means that all local state is retained, including the current bindings of local variables, the instruction pointer, the internal evaluation stack, and the state of any exception handling. When the execution is resumed by awaiting on the next object returned by the asynchronous generator’s methods, the function can proceed exactly as if the yield expression were just another external call. The value of the yield expression after resuming depends on the method which resumed the execution. If __anext__() is used then the result is None . Otherwise, if asend() is used, then the result will be the value passed in to that method.
If an asynchronous generator happens to exit early by break , the caller task being cancelled, or other exceptions, the generator’s async cleanup code will run and possibly raise exceptions or access context variables in an unexpected context–perhaps after the lifetime of tasks it depends, or during the event loop shutdown when the async-generator garbage collection hook is called. To prevent this, the caller must explicitly close the async generator by calling aclose() method to finalize the generator and ultimately detach it from the event loop.
In an asynchronous generator function, yield expressions are allowed anywhere in a try construct. However, if an asynchronous generator is not resumed before it is finalized (by reaching a zero reference count or by being garbage collected), then a yield expression within a try construct could result in a failure to execute pending finally clauses. In this case, it is the responsibility of the event loop or scheduler running the asynchronous generator to call the asynchronous generator-iterator’s aclose() method and run the resulting coroutine object, thus allowing any pending finally clauses to execute.
To take care of finalization upon event loop termination, an event loop should define a finalizer function which takes an asynchronous generator-iterator and presumably calls aclose() and executes the coroutine. This finalizer may be registered by calling sys.set_asyncgen_hooks() . When first iterated over, an asynchronous generator-iterator will store the registered finalizer to be called upon finalization. For a reference example of a finalizer method see the implementation of asyncio.Loop.shutdown_asyncgens in Lib/asyncio/base_events.py .
The expression yield from <expr> is a syntax error when used in an asynchronous generator function.
6.2.9.4. Asynchronous generator-iterator methods ¶
This subsection describes the methods of an asynchronous generator iterator, which are used to control the execution of a generator function.
Returns an awaitable which when run starts to execute the asynchronous generator or resumes it at the last executed yield expression. When an asynchronous generator function is resumed with an __anext__() method, the current yield expression always evaluates to None in the returned awaitable, which when run will continue to the next yield expression. The value of the expression_list of the yield expression is the value of the StopIteration exception raised by the completing coroutine. If the asynchronous generator exits without yielding another value, the awaitable instead raises a StopAsyncIteration exception, signalling that the asynchronous iteration has completed.
This method is normally called implicitly by a async for loop.
Returns an awaitable which when run resumes the execution of the asynchronous generator. As with the send() method for a generator, this “sends” a value into the asynchronous generator function, and the value argument becomes the result of the current yield expression. The awaitable returned by the asend() method will return the next value yielded by the generator as the value of the raised StopIteration , or raises StopAsyncIteration if the asynchronous generator exits without yielding another value. When asend() is called to start the asynchronous generator, it must be called with None as the argument, because there is no yield expression that could receive the value.
Returns an awaitable that raises an exception of type type at the point where the asynchronous generator was paused, and returns the next value yielded by the generator function as the value of the raised StopIteration exception. If the asynchronous generator exits without yielding another value, a StopAsyncIteration exception is raised by the awaitable. If the generator function does not catch the passed-in exception, or raises a different exception, then when the awaitable is run that exception propagates to the caller of the awaitable.
Returns an awaitable that when run will throw a GeneratorExit into the asynchronous generator function at the point where it was paused. If the asynchronous generator function then exits gracefully, is already closed, or raises GeneratorExit (by not catching the exception), then the returned awaitable will raise a StopIteration exception. Any further awaitables returned by subsequent calls to the asynchronous generator will raise a StopAsyncIteration exception. If the asynchronous generator yields a value, a RuntimeError is raised by the awaitable. If the asynchronous generator raises any other exception, it is propagated to the caller of the awaitable. If the asynchronous generator has already exited due to an exception or normal exit, then further calls to aclose() will return an awaitable that does nothing.
6.3. Primaries ¶
Primaries represent the most tightly bound operations of the language. Their syntax is:
6.3.1. Attribute references ¶
An attribute reference is a primary followed by a period and a name:
The primary must evaluate to an object of a type that supports attribute references, which most objects do. This object is then asked to produce the attribute whose name is the identifier. This production can be customized by overriding the __getattr__() method. If this attribute is not available, the exception AttributeError is raised. Otherwise, the type and value of the object produced is determined by the object. Multiple evaluations of the same attribute reference may yield different objects.
6.3.2. Subscriptions ¶
The subscription of an instance of a container class will generally select an element from the container. The subscription of a generic class will generally return a GenericAlias object.
When an object is subscripted, the interpreter will evaluate the primary and the expression list.
The primary must evaluate to an object that supports subscription. An object may support subscription through defining one or both of __getitem__() and __class_getitem__() . When the primary is subscripted, the evaluated result of the expression list will be passed to one of these methods. For more details on when __class_getitem__ is called instead of __getitem__ , see __class_getitem__ versus __getitem__ .
If the expression list contains at least one comma, it will evaluate to a tuple containing the items of the expression list. Otherwise, the expression list will evaluate to the value of the list’s sole member.
For built-in objects, there are two types of objects that support subscription via __getitem__() :
Mappings. If the primary is a mapping , the expression list must evaluate to an object whose value is one of the keys of the mapping, and the subscription selects the value in the mapping that corresponds to that key. An example of a builtin mapping class is the dict class.
Sequences. If the primary is a sequence , the expression list must evaluate to an int or a slice (as discussed in the following section). Examples of builtin sequence classes include the str , list and tuple classes.
The formal syntax makes no special provision for negative indices in sequences . However, built-in sequences all provide a __getitem__() method that interprets negative indices by adding the length of the sequence to the index so that, for example, x[-1] selects the last item of x . The resulting value must be a nonnegative integer less than the number of items in the sequence, and the subscription selects the item whose index is that value (counting from zero). Since the support for negative indices and slicing occurs in the object’s __getitem__() method, subclasses overriding this method will need to explicitly add that support.
A string is a special kind of sequence whose items are characters . A character is not a separate data type but a string of exactly one character.
6.3.3. Slicings ¶
A slicing selects a range of items in a sequence object (e.g., a string, tuple or list). Slicings may be used as expressions or as targets in assignment or del statements. The syntax for a slicing:
There is ambiguity in the formal syntax here: anything that looks like an expression list also looks like a slice list, so any subscription can be interpreted as a slicing. Rather than further complicating the syntax, this is disambiguated by defining that in this case the interpretation as a subscription takes priority over the interpretation as a slicing (this is the case if the slice list contains no proper slice).
The semantics for a slicing are as follows. The primary is indexed (using the same __getitem__() method as normal subscription) with a key that is constructed from the slice list, as follows. If the slice list contains at least one comma, the key is a tuple containing the conversion of the slice items; otherwise, the conversion of the lone slice item is the key. The conversion of a slice item that is an expression is that expression. The conversion of a proper slice is a slice object (see section The standard type hierarchy ) whose start , stop and step attributes are the values of the expressions given as lower bound, upper bound and stride, respectively, substituting None for missing expressions.
6.3.4. Calls ¶
A call calls a callable object (e.g., a function ) with a possibly empty series of arguments :
An optional trailing comma may be present after the positional and keyword arguments but does not affect the semantics.
The primary must evaluate to a callable object (user-defined functions, built-in functions, methods of built-in objects, class objects, methods of class instances, and all objects having a __call__() method are callable). All argument expressions are evaluated before the call is attempted. Please refer to section Function definitions for the syntax of formal parameter lists.
If keyword arguments are present, they are first converted to positional arguments, as follows. First, a list of unfilled slots is created for the formal parameters. If there are N positional arguments, they are placed in the first N slots. Next, for each keyword argument, the identifier is used to determine the corresponding slot (if the identifier is the same as the first formal parameter name, the first slot is used, and so on). If the slot is already filled, a TypeError exception is raised. Otherwise, the value of the argument is placed in the slot, filling it (even if the expression is None , it fills the slot). When all arguments have been processed, the slots that are still unfilled are filled with the corresponding default value from the function definition. (Default values are calculated, once, when the function is defined; thus, a mutable object such as a list or dictionary used as default value will be shared by all calls that don’t specify an argument value for the corresponding slot; this should usually be avoided.) If there are any unfilled slots for which no default value is specified, a TypeError exception is raised. Otherwise, the list of filled slots is used as the argument list for the call.
CPython implementation detail: An implementation may provide built-in functions whose positional parameters do not have names, even if they are ‘named’ for the purpose of documentation, and which therefore cannot be supplied by keyword. In CPython, this is the case for functions implemented in C that use PyArg_ParseTuple() to parse their arguments.
If there are more positional arguments than there are formal parameter slots, a TypeError exception is raised, unless a formal parameter using the syntax *identifier is present; in this case, that formal parameter receives a tuple containing the excess positional arguments (or an empty tuple if there were no excess positional arguments).
If any keyword argument does not correspond to a formal parameter name, a TypeError exception is raised, unless a formal parameter using the syntax **identifier is present; in this case, that formal parameter receives a dictionary containing the excess keyword arguments (using the keywords as keys and the argument values as corresponding values), or a (new) empty dictionary if there were no excess keyword arguments.
If the syntax *expression appears in the function call, expression must evaluate to an iterable . Elements from these iterables are treated as if they were additional positional arguments. For the call f(x1, x2, *y, x3, x4) , if y evaluates to a sequence y1 , …, yM , this is equivalent to a call with M+4 positional arguments x1 , x2 , y1 , …, yM , x3 , x4 .
A consequence of this is that although the *expression syntax may appear after explicit keyword arguments, it is processed before the keyword arguments (and any **expression arguments – see below). So:
It is unusual for both keyword arguments and the *expression syntax to be used in the same call, so in practice this confusion does not arise.
If the syntax **expression appears in the function call, expression must evaluate to a mapping , the contents of which are treated as additional keyword arguments. If a parameter matching a key has already been given a value (by an explicit keyword argument, or from another unpacking), a TypeError exception is raised.
When **expression is used, each key in this mapping must be a string. Each value from the mapping is assigned to the first formal parameter eligible for keyword assignment whose name is equal to the key. A key need not be a Python identifier (e.g. "max-temp °F" is acceptable, although it will not match any formal parameter that could be declared). If there is no match to a formal parameter the key-value pair is collected by the ** parameter, if there is one, or if there is not, a TypeError exception is raised.
Formal parameters using the syntax *identifier or **identifier cannot be used as positional argument slots or as keyword argument names.
Changed in version 3.5: Function calls accept any number of * and ** unpackings, positional arguments may follow iterable unpackings ( * ), and keyword arguments may follow dictionary unpackings ( ** ). Originally proposed by PEP 448 .
A call always returns some value, possibly None , unless it raises an exception. How this value is computed depends on the type of the callable object.
The code block for the function is executed, passing it the argument list. The first thing the code block will do is bind the formal parameters to the arguments; this is described in section Function definitions . When the code block executes a return statement, this specifies the return value of the function call.
The result is up to the interpreter; see Built-in Functions for the descriptions of built-in functions and methods.
A new instance of that class is returned.
The corresponding user-defined function is called, with an argument list that is one longer than the argument list of the call: the instance becomes the first argument.
The class must define a __call__() method; the effect is then the same as if that method was called.
6.4. Await expression ¶
Suspend the execution of coroutine on an awaitable object. Can only be used inside a coroutine function .
New in version 3.5.
6.5. The power operator ¶
The power operator binds more tightly than unary operators on its left; it binds less tightly than unary operators on its right. The syntax is:
Thus, in an unparenthesized sequence of power and unary operators, the operators are evaluated from right to left (this does not constrain the evaluation order for the operands): -1**2 results in -1 .
The power operator has the same semantics as the built-in pow() function, when called with two arguments: it yields its left argument raised to the power of its right argument. The numeric arguments are first converted to a common type, and the result is of that type.
For int operands, the result has the same type as the operands unless the second argument is negative; in that case, all arguments are converted to float and a float result is delivered. For example, 10**2 returns 100 , but 10**-2 returns 0.01 .
Raising 0.0 to a negative power results in a ZeroDivisionError . Raising a negative number to a fractional power results in a complex number. (In earlier versions it raised a ValueError .)
This operation can be customized using the special __pow__() method.
6.6. Unary arithmetic and bitwise operations ¶
All unary arithmetic and bitwise operations have the same priority:
The unary - (minus) operator yields the negation of its numeric argument; the operation can be overridden with the __neg__() special method.
The unary + (plus) operator yields its numeric argument unchanged; the operation can be overridden with the __pos__() special method.
The unary ~ (invert) operator yields the bitwise inversion of its integer argument. The bitwise inversion of x is defined as -(x+1) . It only applies to integral numbers or to custom objects that override the __invert__() special method.
In all three cases, if the argument does not have the proper type, a TypeError exception is raised.
6.7. Binary arithmetic operations ¶
The binary arithmetic operations have the conventional priority levels. Note that some of these operations also apply to certain non-numeric types. Apart from the power operator, there are only two levels, one for multiplicative operators and one for additive operators:
The * (multiplication) operator yields the product of its arguments. The arguments must either both be numbers, or one argument must be an integer and the other must be a sequence. In the former case, the numbers are converted to a common type and then multiplied together. In the latter case, sequence repetition is performed; a negative repetition factor yields an empty sequence.
This operation can be customized using the special __mul__() and __rmul__() methods.
The @ (at) operator is intended to be used for matrix multiplication. No builtin Python types implement this operator.
The / (division) and // (floor division) operators yield the quotient of their arguments. The numeric arguments are first converted to a common type. Division of integers yields a float, while floor division of integers results in an integer; the result is that of mathematical division with the ‘floor’ function applied to the result. Division by zero raises the ZeroDivisionError exception.
This operation can be customized using the special __truediv__() and __floordiv__() methods.
The % (modulo) operator yields the remainder from the division of the first argument by the second. The numeric arguments are first converted to a common type. A zero right argument raises the ZeroDivisionError exception. The arguments may be floating point numbers, e.g., 3.14%0.7 equals 0.34 (since 3.14 equals 4*0.7 + 0.34 .) The modulo operator always yields a result with the same sign as its second operand (or zero); the absolute value of the result is strictly smaller than the absolute value of the second operand 1 .
The floor division and modulo operators are connected by the following identity: x == (x//y)*y + (x%y) . Floor division and modulo are also connected with the built-in function divmod() : divmod(x, y) == (x//y, x%y) . 2 .
In addition to performing the modulo operation on numbers, the % operator is also overloaded by string objects to perform old-style string formatting (also known as interpolation). The syntax for string formatting is described in the Python Library Reference, section printf-style String Formatting .
The modulo operation can be customized using the special __mod__() method.
The floor division operator, the modulo operator, and the divmod() function are not defined for complex numbers. Instead, convert to a floating point number using the abs() function if appropriate.
The + (addition) operator yields the sum of its arguments. The arguments must either both be numbers or both be sequences of the same type. In the former case, the numbers are converted to a common type and then added together. In the latter case, the sequences are concatenated.
This operation can be customized using the special __add__() and __radd__() methods.
The - (subtraction) operator yields the difference of its arguments. The numeric arguments are first converted to a common type.
This operation can be customized using the special __sub__() method.
6.8. Shifting operations ¶
The shifting operations have lower priority than the arithmetic operations:
These operators accept integers as arguments. They shift the first argument to the left or right by the number of bits given by the second argument.
This operation can be customized using the special __lshift__() and __rshift__() methods.
A right shift by n bits is defined as floor division by pow(2,n) . A left shift by n bits is defined as multiplication with pow(2,n) .
6.9. Binary bitwise operations ¶
Each of the three bitwise operations has a different priority level:
The & operator yields the bitwise AND of its arguments, which must be integers or one of them must be a custom object overriding __and__() or __rand__() special methods.
The ^ operator yields the bitwise XOR (exclusive OR) of its arguments, which must be integers or one of them must be a custom object overriding __xor__() or __rxor__() special methods.
The | operator yields the bitwise (inclusive) OR of its arguments, which must be integers or one of them must be a custom object overriding __or__() or __ror__() special methods.
6.10. Comparisons ¶
Unlike C, all comparison operations in Python have the same priority, which is lower than that of any arithmetic, shifting or bitwise operation. Also unlike C, expressions like a < b < c have the interpretation that is conventional in mathematics:
Comparisons yield boolean values: True or False . Custom rich comparison methods may return non-boolean values. In this case Python will call bool() on such value in boolean contexts.
Comparisons can be chained arbitrarily, e.g., x < y <= z is equivalent to x < y and y <= z , except that y is evaluated only once (but in both cases z is not evaluated at all when x < y is found to be false).
Formally, if a , b , c , …, y , z are expressions and op1 , op2 , …, opN are comparison operators, then a op1 b op2 c ... y opN z is equivalent to a op1 b and b op2 c and ... y opN z , except that each expression is evaluated at most once.
Note that a op1 b op2 c doesn’t imply any kind of comparison between a and c , so that, e.g., x < y > z is perfectly legal (though perhaps not pretty).
6.10.1. Value comparisons ¶
The operators < , > , == , >= , <= , and != compare the values of two objects. The objects do not need to have the same type.
Chapter Objects, values and types states that objects have a value (in addition to type and identity). The value of an object is a rather abstract notion in Python: For example, there is no canonical access method for an object’s value. Also, there is no requirement that the value of an object should be constructed in a particular way, e.g. comprised of all its data attributes. Comparison operators implement a particular notion of what the value of an object is. One can think of them as defining the value of an object indirectly, by means of their comparison implementation.
Because all types are (direct or indirect) subtypes of object , they inherit the default comparison behavior from object . Types can customize their comparison behavior by implementing rich comparison methods like __lt__() , described in Basic customization .
The default behavior for equality comparison ( == and != ) is based on the identity of the objects. Hence, equality comparison of instances with the same identity results in equality, and equality comparison of instances with different identities results in inequality. A motivation for this default behavior is the desire that all objects should be reflexive (i.e. x is y implies x == y ).
A default order comparison ( < , > , <= , and >= ) is not provided; an attempt raises TypeError . A motivation for this default behavior is the lack of a similar invariant as for equality.
The behavior of the default equality comparison, that instances with different identities are always unequal, may be in contrast to what types will need that have a sensible definition of object value and value-based equality. Such types will need to customize their comparison behavior, and in fact, a number of built-in types have done that.
The following list describes the comparison behavior of the most important built-in types.
Numbers of built-in numeric types ( Numeric Types — int, float, complex ) and of the standard library types fractions.Fraction and decimal.Decimal can be compared within and across their types, with the restriction that complex numbers do not support order comparison. Within the limits of the types involved, they compare mathematically (algorithmically) correct without loss of precision.
The not-a-number values float('NaN') and decimal.Decimal('NaN') are special. Any ordered comparison of a number to a not-a-number value is false. A counter-intuitive implication is that not-a-number values are not equal to themselves. For example, if x = float('NaN') , 3 < x , x < 3 and x == x are all false, while x != x is true. This behavior is compliant with IEEE 754.
None and NotImplemented are singletons. PEP 8 advises that comparisons for singletons should always be done with is or is not , never the equality operators.
Binary sequences (instances of bytes or bytearray ) can be compared within and across their types. They compare lexicographically using the numeric values of their elements.
Strings (instances of str ) compare lexicographically using the numerical Unicode code points (the result of the built-in function ord() ) of their characters. 3
Strings and binary sequences cannot be directly compared.
Sequences (instances of tuple , list , or range ) can be compared only within each of their types, with the restriction that ranges do not support order comparison. Equality comparison across these types results in inequality, and ordering comparison across these types raises TypeError .
Sequences compare lexicographically using comparison of corresponding elements. The built-in containers typically assume identical objects are equal to themselves. That lets them bypass equality tests for identical objects to improve performance and to maintain their internal invariants.
Lexicographical comparison between built-in collections works as follows:
For two collections to compare equal, they must be of the same type, have the same length, and each pair of corresponding elements must compare equal (for example, [1,2] == (1,2) is false because the type is not the same).
Collections that support order comparison are ordered the same as their first unequal elements (for example, [1,2,x] <= [1,2,y] has the same value as x <= y ). If a corresponding element does not exist, the shorter collection is ordered first (for example, [1,2] < [1,2,3] is true).
Mappings (instances of dict ) compare equal if and only if they have equal (key, value) pairs. Equality comparison of the keys and values enforces reflexivity.
Order comparisons ( < , > , <= , and >= ) raise TypeError .
Sets (instances of set or frozenset ) can be compared within and across their types.
They define order comparison operators to mean subset and superset tests. Those relations do not define total orderings (for example, the two sets {1,2} and {2,3} are not equal, nor subsets of one another, nor supersets of one another). Accordingly, sets are not appropriate arguments for functions which depend on total ordering (for example, min() , max() , and sorted() produce undefined results given a list of sets as inputs).
Comparison of sets enforces reflexivity of its elements.
Most other built-in types have no comparison methods implemented, so they inherit the default comparison behavior.
User-defined classes that customize their comparison behavior should follow some consistency rules, if possible:
Equality comparison should be reflexive. In other words, identical objects should compare equal:
x is y implies x == y
Comparison should be symmetric. In other words, the following expressions should have the same result:
x == y and y == x x != y and y != x x < y and y > x x <= y and y >= x
Comparison should be transitive. The following (non-exhaustive) examples illustrate that:
x > y and y > z implies x > z x < y and y <= z implies x < z
Inverse comparison should result in the boolean negation. In other words, the following expressions should have the same result:
x == y and not x != y x < y and not x >= y (for total ordering) x > y and not x <= y (for total ordering)
The last two expressions apply to totally ordered collections (e.g. to sequences, but not to sets or mappings). See also the total_ordering() decorator.
The hash() result should be consistent with equality. Objects that are equal should either have the same hash value, or be marked as unhashable.
Python does not enforce these consistency rules. In fact, the not-a-number values are an example for not following these rules.
6.10.2. Membership test operations ¶
The operators in and not in test for membership. x in s evaluates to True if x is a member of s , and False otherwise. x not in s returns the negation of x in s . All built-in sequences and set types support this as well as dictionary, for which in tests whether the dictionary has a given key. For container types such as list, tuple, set, frozenset, dict, or collections.deque, the expression x in y is equivalent to any(x is e or x == e for e in y) .
For the string and bytes types, x in y is True if and only if x is a substring of y . An equivalent test is y.find(x) != -1 . Empty strings are always considered to be a substring of any other string, so "" in "abc" will return True .
For user-defined classes which define the __contains__() method, x in y returns True if y.__contains__(x) returns a true value, and False otherwise.
For user-defined classes which do not define __contains__() but do define __iter__() , x in y is True if some value z , for which the expression x is z or x == z is true, is produced while iterating over y . If an exception is raised during the iteration, it is as if in raised that exception.
Lastly, the old-style iteration protocol is tried: if a class defines __getitem__() , x in y is True if and only if there is a non-negative integer index i such that x is y[i] or x == y[i] , and no lower integer index raises the IndexError exception. (If any other exception is raised, it is as if in raised that exception).
The operator not in is defined to have the inverse truth value of in .
6.10.3. Identity comparisons ¶
The operators is and is not test for an object’s identity: x is y is true if and only if x and y are the same object. An Object’s identity is determined using the id() function. x is not y yields the inverse truth value. 4
6.11. Boolean operations ¶
In the context of Boolean operations, and also when expressions are used by control flow statements, the following values are interpreted as false: False , None , numeric zero of all types, and empty strings and containers (including strings, tuples, lists, dictionaries, sets and frozensets). All other values are interpreted as true. User-defined objects can customize their truth value by providing a __bool__() method.
The operator not yields True if its argument is false, False otherwise.
The expression x and y first evaluates x ; if x is false, its value is returned; otherwise, y is evaluated and the resulting value is returned.
The expression x or y first evaluates x ; if x is true, its value is returned; otherwise, y is evaluated and the resulting value is returned.
Note that neither and nor or restrict the value and type they return to False and True , but rather return the last evaluated argument. This is sometimes useful, e.g., if s is a string that should be replaced by a default value if it is empty, the expression s or 'foo' yields the desired value. Because not has to create a new value, it returns a boolean value regardless of the type of its argument (for example, not 'foo' produces False rather than '' .)
6.12. Assignment expressions ¶
An assignment expression (sometimes also called a “named expression” or “walrus”) assigns an expression to an identifier , while also returning the value of the expression .
One common use case is when handling matched regular expressions:
Or, when processing a file stream in chunks:
Assignment expressions must be surrounded by parentheses when used as sub-expressions in slicing, conditional, lambda, keyword-argument, and comprehension-if expressions and in assert and with statements. In all other places where they can be used, parentheses are not required, including in if and while statements.
New in version 3.8: See PEP 572 for more details about assignment expressions.
6.13. Conditional expressions ¶
Conditional expressions (sometimes called a “ternary operator”) have the lowest priority of all Python operations.
The expression x if C else y first evaluates the condition, C rather than x . If C is true, x is evaluated and its value is returned; otherwise, y is evaluated and its value is returned.
See PEP 308 for more details about conditional expressions.
6.14. Lambdas ¶
Lambda expressions (sometimes called lambda forms) are used to create anonymous functions. The expression lambda parameters: expression yields a function object. The unnamed object behaves like a function object defined with:
See section Function definitions for the syntax of parameter lists. Note that functions created with lambda expressions cannot contain statements or annotations.
6.15. Expression lists ¶
Except when part of a list or set display, an expression list containing at least one comma yields a tuple. The length of the tuple is the number of expressions in the list. The expressions are evaluated from left to right.
An asterisk * denotes iterable unpacking . Its operand must be an iterable . The iterable is expanded into a sequence of items, which are included in the new tuple, list, or set, at the site of the unpacking.
New in version 3.5: Iterable unpacking in expression lists, originally proposed by PEP 448 .
The trailing comma is required only to create a single tuple (a.k.a. a singleton ); it is optional in all other cases. A single expression without a trailing comma doesn’t create a tuple, but rather yields the value of that expression. (To create an empty tuple, use an empty pair of parentheses: () .)
6.16. Evaluation order ¶
Python evaluates expressions from left to right. Notice that while evaluating an assignment, the right-hand side is evaluated before the left-hand side.
In the following lines, expressions will be evaluated in the arithmetic order of their suffixes:
6.17. Operator precedence ¶
The following table summarizes the operator precedence in Python, from highest precedence (most binding) to lowest precedence (least binding). Operators in the same box have the same precedence. Unless the syntax is explicitly given, operators are binary. Operators in the same box group left to right (except for exponentiation, which groups from right to left).
Note that comparisons, membership tests, and identity tests, all have the same precedence and have a left-to-right chaining feature as described in the Comparisons section.
While abs(x%y) < abs(y) is true mathematically, for floats it may not be true numerically due to roundoff. For example, and assuming a platform on which a Python float is an IEEE 754 double-precision number, in order that -1e-100 % 1e100 have the same sign as 1e100 , the computed result is -1e-100 + 1e100 , which is numerically exactly equal to 1e100 . The function math.fmod() returns a result whose sign matches the sign of the first argument instead, and so returns -1e-100 in this case. Which approach is more appropriate depends on the application.
If x is very close to an exact integer multiple of y, it’s possible for x//y to be one larger than (x-x%y)//y due to rounding. In such cases, Python returns the latter result, in order to preserve that divmod(x,y)[0] * y + x % y be very close to x .
The Unicode standard distinguishes between code points (e.g. U+0041) and abstract characters (e.g. “LATIN CAPITAL LETTER A”). While most abstract characters in Unicode are only represented using one code point, there is a number of abstract characters that can in addition be represented using a sequence of more than one code point. For example, the abstract character “LATIN CAPITAL LETTER C WITH CEDILLA” can be represented as a single precomposed character at code position U+00C7, or as a sequence of a base character at code position U+0043 (LATIN CAPITAL LETTER C), followed by a combining character at code position U+0327 (COMBINING CEDILLA).
The comparison operators on strings compare at the level of Unicode code points. This may be counter-intuitive to humans. For example, "\u00C7" == "\u0043\u0327" is False , even though both strings represent the same abstract character “LATIN CAPITAL LETTER C WITH CEDILLA”.
To compare strings at the level of abstract characters (that is, in a way intuitive to humans), use unicodedata.normalize() .
Due to automatic garbage-collection, free lists, and the dynamic nature of descriptors, you may notice seemingly unusual behaviour in certain uses of the is operator, like those involving comparisons between instance methods, or constants. Check their documentation for more info.
The power operator ** binds less tightly than an arithmetic or bitwise unary operator on its right, that is, 2**-1 is 0.5 .
The % operator is also used for string formatting; the same precedence applies.
Table of Contents
- 6.1. Arithmetic conversions
- 6.2.1. Identifiers (Names)
- 6.2.2. Literals
- 6.2.3. Parenthesized forms
- 6.2.4. Displays for lists, sets and dictionaries
- 6.2.5. List displays
- 6.2.6. Set displays
- 6.2.7. Dictionary displays
- 6.2.8. Generator expressions
- 6.2.9.1. Generator-iterator methods
- 6.2.9.2. Examples
- 6.2.9.3. Asynchronous generator functions
- 6.2.9.4. Asynchronous generator-iterator methods
- 6.3.1. Attribute references
- 6.3.2. Subscriptions
- 6.3.3. Slicings
- 6.3.4. Calls
- 6.4. Await expression
- 6.5. The power operator
- 6.6. Unary arithmetic and bitwise operations
- 6.7. Binary arithmetic operations
- 6.8. Shifting operations
- 6.9. Binary bitwise operations
- 6.10.1. Value comparisons
- 6.10.2. Membership test operations
- 6.10.3. Identity comparisons
- 6.11. Boolean operations
- 6.12. Assignment expressions
- 6.13. Conditional expressions
- 6.14. Lambdas
- 6.15. Expression lists
- 6.16. Evaluation order
- 6.17. Operator precedence
Previous topic
5. The import system
7. Simple statements
- Report a Bug
- Show Source
Conditional expression (ternary operator) in Python
Python has a conditional expression (sometimes called a "ternary operator"). You can write operations like if statements in one line with conditional expressions.
- 6. Expressions - Conditional expressions — Python 3.11.3 documentation
Basics of the conditional expression (ternary operator)
If ... elif ... else ... by conditional expressions, list comprehensions and conditional expressions, lambda expressions and conditional expressions.
See the following article for if statements in Python.
- Python if statements (if, elif, else)
In Python, the conditional expression is written as follows.
The condition is evaluated first. If condition is True , X is evaluated and its value is returned, and if condition is False , Y is evaluated and its value is returned.
If you want to switch the value based on a condition, simply use the desired values in the conditional expression.
If you want to switch between operations based on a condition, simply describe each corresponding expression in the conditional expression.
An expression that does not return a value (i.e., an expression that returns None ) is also acceptable in a conditional expression. Depending on the condition, either expression will be evaluated and executed.
The above example is equivalent to the following code written with an if statement.
You can also combine multiple conditions using logical operators such as and or or .
- Boolean operators in Python (and, or, not)
By combining conditional expressions, you can write an operation like if ... elif ... else ... in one line.
However, it is difficult to understand, so it may be better not to use it often.
The following two interpretations are possible, but the expression is processed as the first one.
In the sample code below, which includes three expressions, the first expression is interpreted like the second, rather than the third:
By using conditional expressions in list comprehensions, you can apply operations to the elements of the list based on the condition.
See the following article for details on list comprehensions.
- List comprehensions in Python
Conditional expressions are also useful when you want to apply an operation similar to an if statement within lambda expressions.
In the example above, the lambda expression is assigned to a variable for convenience, but this is not recommended by PEP8.
Refer to the following article for more details on lambda expressions.
- Lambda expressions in Python
Related Categories
Related articles.
- Shallow and deep copy in Python: copy(), deepcopy()
- Composite two images according to a mask image with Python, Pillow
- OpenCV, NumPy: Rotate and flip image
- pandas: Check if DataFrame/Series is empty
- Check pandas version: pd.show_versions
- Python if statement (if, elif, else)
- pandas: Find the quantile with quantile()
- Handle date and time with the datetime module in Python
- Get image size (width, height) with Python, OpenCV, Pillow (PIL)
- Convert between Unix time (Epoch time) and datetime in Python
- Convert BGR and RGB with Python, OpenCV (cvtColor)
- Matrix operations with NumPy in Python
- pandas: Replace values in DataFrame and Series with replace()
- Uppercase and lowercase strings in Python (conversion and checking)
- Calculate mean, median, mode, variance, standard deviation in Python
{{ activeMenu.name }}
- Python Courses
- JavaScript Courses
- Artificial Intelligence Courses
- Data Science Courses
- React Courses
- Ethical Hacking Courses
- View All Courses
Fresh Articles
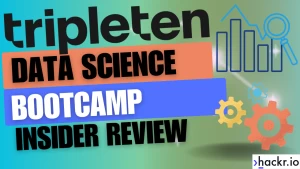
- Python Projects
- JavaScript Projects
- Java Projects
- HTML Projects
- C++ Projects
- PHP Projects
- View All Projects
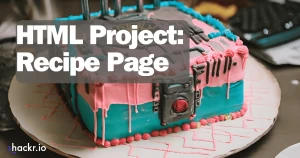
- Python Certifications
- JavaScript Certifications
- Linux Certifications
- Data Science Certifications
- Data Analytics Certifications
- Cybersecurity Certifications
- View All Certifications
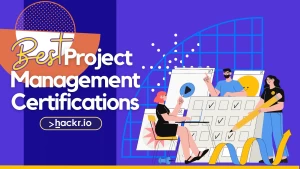
- IDEs & Editors
- Web Development
- Frameworks & Libraries
- View All Programming
- View All Development
- App Development
- Game Development
- Courses, Books, & Certifications
- Data Science
- Data Analytics
- Artificial Intelligence (AI)
- Machine Learning (ML)
- View All Data, Analysis, & AI
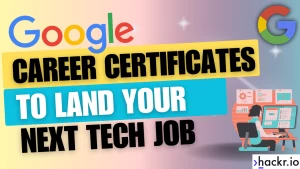
- Networking & Security
- Cloud, DevOps, & Systems
- Recommendations
- Crypto, Web3, & Blockchain
- User-Submitted Tutorials
- View All Blog Content
- JavaScript Online Compiler
- HTML & CSS Online Compiler
- Certifications
- Programming
- Development
- Data, Analysis, & AI
- Online JavaScript Compiler
- Online HTML Compiler
Don't have an account? Sign up
Forgot your password?
Already have an account? Login
Have you read our submission guidelines?
Go back to Sign In
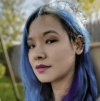
Python Ternary Operator: How and Why You Should Use It
Writing concise, practical, organized, and intelligible code should be a top priority for any Python developer. Enter, the Python ternary operator.
What is this? I hear you ask. Well, you can use the ternary operator to test a condition and then execute a conditional assignment with only one line of code. And why is this so great? Well, this means we can replace those ever-popular if-else statements with a quicker and more practical way to code conditional assignments.
Now don’t get me wrong, we still need if-else statements (check out our comprehensive guide and Python cheat sheet for the best way to use conditional statements). But, it’s always good practice to make your code more Pythonic when there’s a simpler way to get the same result, and that’s exactly what you get with the ternary operator.
- Why Use the Python Ternary Operator?
Ternary operators (also known as conditional operators) in Python perform evaluations and assignments after checking whether a given condition is true or false. This means that the ternary operator is a bit like a simplified, one-line if-else statement. Cool, right?
When used correctly, this operator reduces code size and improves readability .
Ternary Operator Syntax
A ternary operator takes three operands:
- condition: A Boolean expression that evaluates to true or false
- true_value: A value or expression to be assigned if the condition evaluates to true
- false_value: A value or expression to be assigned if the condition evaluates to false
This is how it should look when we put it all together:
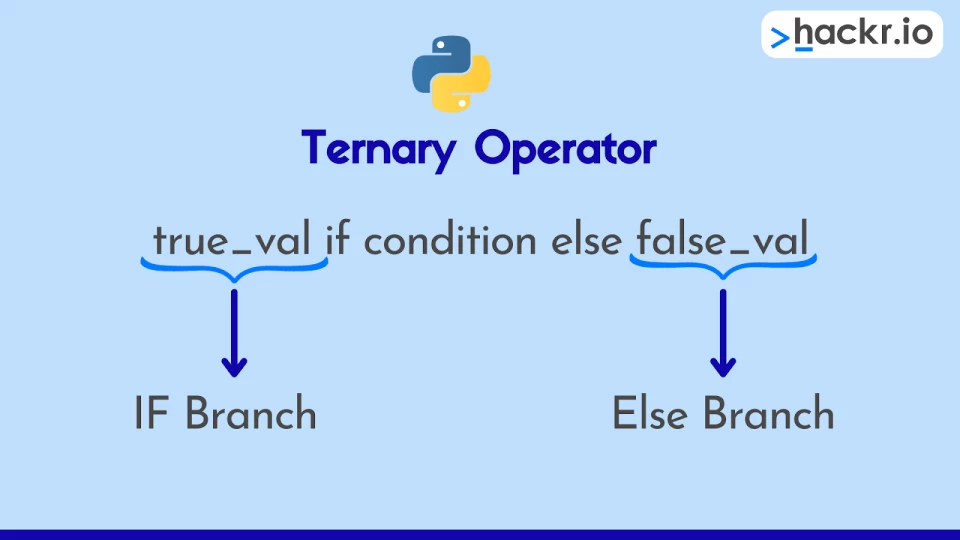
So, this means that the variable "var" on the left side of the assignment operator (=), will be assigned either:
- true_value if the Boolean expression evaluates to true
- false_value if the Boolean expression evaluates to false
- How to Use the Ternary Operator Instead of If-Else
To better understand how we replace an if-else statement with the ternary operator in Python, let’s write a simple program with each approach. In this first example, we’ll assume the user enters an integer number when prompted, and we’ll start with the familiar if-else.
Program Using an If-Else Statement
Here’s what we get when the user enters 22 at the input prompt:
In this example, the if-else statement assigns “Yes, you are old enough to watch this movie!” to the movie_acess variable if the user enters an age that is greater than or equal to 18, otherwise, it assigns "Sorry, you aren't old enough to watch this movie yet!”. Finally, we use an f-string to print out the result to the screen.
Now, let's use the ternary operator syntax to make the program much more concise.
Program Using a Ternary Operator
In this version of the program, the variable that receives the result of the conditional statement (movie_access) is to the left of the assignment operator (=).
The ternary operator evaluates the condition:
If the condition evaluates to true, the program assigns “Yes, you are old enough to watch this movie!” to movie_access, else it assigns “Sorry, you aren't old enough to watch this movie yet!”.
Let’s take a look at another example that uses the Python ternary operator and if-else to achieve the same goal. This time, we’ll write a simple Python code snippet to check whether a given integer is even or odd.
In this program, we’ve used a ternary operator and an if-else statement block to determine whether the given integer produces a zero remainder when using modulo divide by 2 (if you’re unfamiliar with this operator, take a look at our cheat sheet). If the remainder is zero, we have an even number, otherwise, we have an odd number.
Right away, we can tell what the if-else statement will do after evaluating the condition. With the ternary operator snippet, we can see the following:
- "Even" is assigned to msg if the condition (given_int % 2 == 0) is true
- "Odd" is assigned to msg if the condition (given_int % 2 == 0) is false
And, as we’ve set the given integer to an even number, the program will print “Even” to the screen.
So, we can see again that a ternary conditional statement is a much cleaner and more concise way to achieve the same outcome as an if-else statement. We simply evaluate the ternary expression from left to right, then assign the return value for the true or false condition.
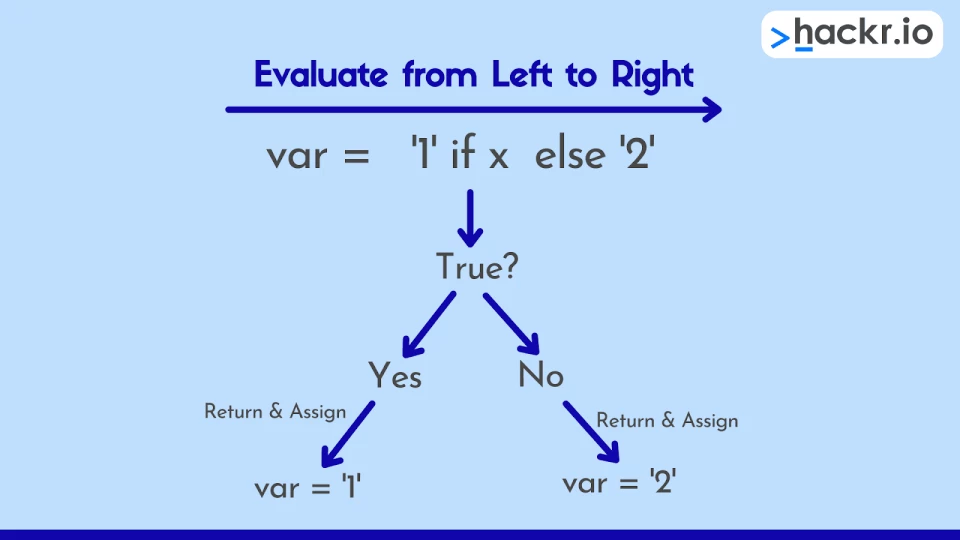
- Key Considerations for Python Ternary Statements
The ternary operator is not always suitable to replace if-else in your code.
If for example, we have more than two conditional branches with an if-elif-else statement, we can’t replace this with a single ternary operator. The clue here is in the name, as ternary refers to the number ‘three’, meaning that it expects three operands. But, if we try to replace an if-elif-else statement with a ternary operator, we’ll have four operands to handle (or possibly more if we have many ‘elif’ branches).
So what do we do here? Well, we need to chain together (or nest) multiple ternary operators (we cover this in the FAQs), but sometimes this can get a little messy, and it may be cleaner to use if-elif-else if the code becomes difficult to read.
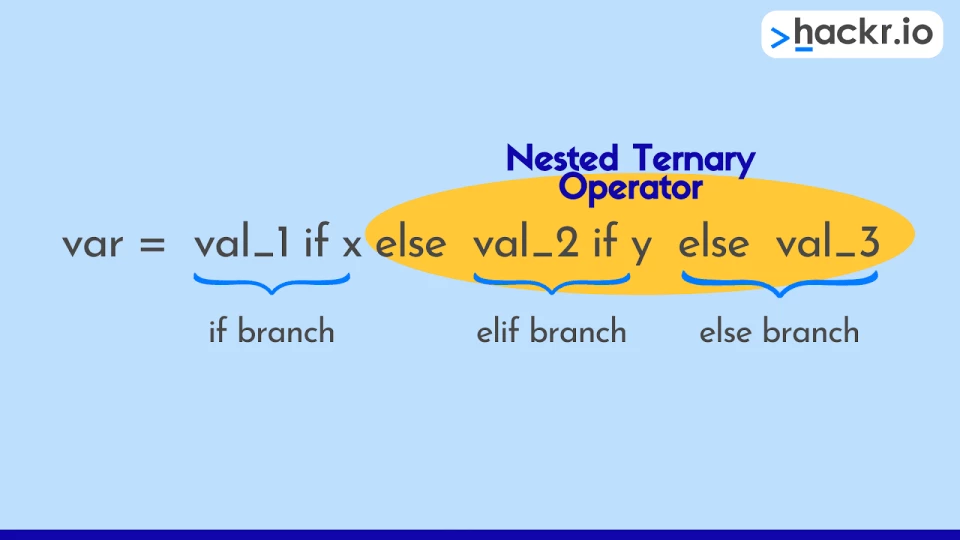
Another thing to keep in mind is that we’re meant to use the ternary operator for conditional assignment. What does this mean? Well, we can’t use the ternary approach if we want to execute blocks of code after evaluating a conditional expression: in these situations, it’s best to stick with if-else.
But, we can use the ternary operator if we are assigning something to a variable after checking against a condition. See, it’s easy if we remember that we’re supposed to be assigning something to something else.
Distinct Features of Python Ternary Statements
- Returns A or B depending on the Boolean result of the conditional expression (a < b)
- Compared with C-type languages(C/C++), it uses a different ordering of the provided arguments
- Conditional expressions have the lowest priority of all Python operations
That's all. We did our best to fill you in on everything there is to know about the Python ternary operator. We also covered how to use ternary statements in your Python code, and we compared them to their close relatives, the ever-popular if-else statement.
If you’re a beginner that’s looking for an easy start to your Python programming career, check out our learning guide along with our up-to-date list of Python courses where you can easily enroll online.
Lastly, don’t forget that you can also check out our comprehensive Python cheat sheet which covers various topics, including Python basics, flow control, Modules in Python, functions, exception handling, lists, dictionaries, and data structures, sets, the itertools module, comprehensions, lambda functions, string formatting, the ternary conditional operator, and much more.
- Frequently Asked Questions
1. What are Ternary Operators (including an example)?
Programmers like to use the concise ternary operator for conditional assignments instead of lengthy if-else statements.
The ternary operator takes three arguments:
- Firstly, the comparison argument
- Secondly, the value (or result of an expression) to assign if the comparison is true
- Thirdly, the value (or result of an expression) to assign if the comparison is false
Simple Ternary Operator Example:
If we run this simple program, the "(m < n)" condition evaluates to true, which means that "m" is assigned to "o" and the print statement outputs the integer 10.
The conditional return values of "m" and "n" must not be whole statements, but rather simple values or the result of an expression.
2. How Do You Write a 3-Condition Ternary Operator?
Say you have three conditions to check against and you want to use a ternary conditional statement. What do you do? The answer is actually pretty simple, chain together multiple ternary operators. The syntax below shows the general format for this:
If we break this syntax down, it is saying:
- If condition_1 is true, return value_1 and assign this to var
- Else, check if condition_2 is true. If it is, return value_2 and assign it to var
- If neither conditions are true, return value_3 and assign this to var
We’ve now created a one-line version of an if-elif-else statement using a chain of ternary operators.
The equivalent if-elif-else statement would be:
One thing to consider before chaining together ternary statements is whether it makes the code more difficult to read. If so, then it’s probably not very Pythonic and you’d be better off with an if-elif-else statement.
3. How Do You Code a Ternary Operator Statement?
We can easily write a ternary expression in Python if we follow the general form shown below:
So, we start by choosing a condition to evaluate against. Then, we either return the true_value or the false_value and then we assign this to results_var .
4. Is the Ternary Operator Faster Than If-Else?
If we consider that a ternary operator is a single-line statement and an if-else statement is a block of code, then it makes sense that if-else will take longer to complete, which means that yes, the ternary operator is faster.
But, if we think about speed in terms of Big-O notation (if I’ve lost you here, don’t worry, head on over to our Big-O cheat sheet ), then they are in fact equally fast. How can this be? This is because conditional statements are viewed as constant time operations when the size of our problem grows very large.
So, the answer is both yes and no depending on the type of question you’re asking.
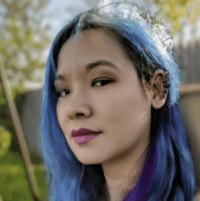
Jenna Inouye currently works at Google and has been a full-stack developer for two decades, specializing in web application design and development. She is a tech expert with a B.S. in Information & Computer Science and MCITP certification. For the last eight years, she has worked as a news and feature writer focusing on technology and finance, with bylines in Udemy, SVG, The Gamer, Productivity Spot, and Spreadsheet Point.
Subscribe to our Newsletter for Articles, News, & Jobs.
Disclosure: Hackr.io is supported by its audience. When you purchase through links on our site, we may earn an affiliate commission.
In this article
- 10 Vital Python Concepts for Data Science
- 10 Common Python Mistakes in 2024 | Are You Making Them? Python Data Science Programming Skills
- GitHub Copilot vs Amazon CodeWhisperer | Who's Best in 2024? Artificial Intelligence (AI) Code Editors IDEs AI Tools
Please login to leave comments
Always be in the loop.
Get news once a week, and don't worry — no spam.
- Help center
- We ❤️ Feedback
- Advertise / Partner
- Write for us
- Privacy Policy
- Cookie Policy
- Change Privacy Settings
- Disclosure Policy
- Terms and Conditions
- Refund Policy
Disclosure: This page may contain affliate links, meaning when you click the links and make a purchase, we receive a commission.
We Love Servers.
- WHY IOFLOOD?
- BARE METAL CLOUD
- DEDICATED SERVERS
Python Ternary Operator | Usage Guide with Examples
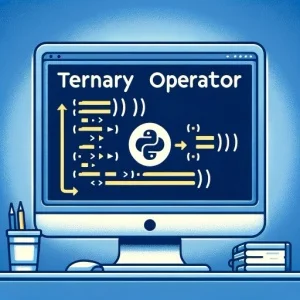
Struggling with Python’s ternary operator? Like a traffic light, the ternary operator can help control the flow of your code.
It’s a powerful tool that, when used correctly, can make your code more concise and easier to read. But, it can also be a bit tricky to master, especially if you’re new to Python or programming in general.
In this guide, we’ll demystify the ternary operator in Python. We’ll start with the basics, explaining what it is and how it works. Then, we’ll move on to more advanced topics, like how to use it effectively in your code and what pitfalls to avoid.
By the end of this article, you’ll have a solid understanding of the Python ternary operator and how to use it to write cleaner, more efficient code.
TL;DR: How Do I Use the Ternary Operator in Python?
The ternary operator in Python is a one-line shorthand for an if-else statement. It allows you to quickly test a condition and return a value based on whether that condition is true or false.
Here’s a simple example:
In this example, the condition x % 2 == 0 checks if the number x is even. If it is, the string ‘Even’ is printed. If not, the string ‘Odd’ is printed. The ternary operator allows us to do this in a single, concise line of code.
Keep reading for a more detailed explanation of how the ternary operator works in Python, as well as advanced usage scenarios and best practices.
Table of Contents
Unraveling the Python Ternary Operator
Exploring nested ternary operations in python, alternative ways to implement conditional logic in python, troubleshooting common issues with the python ternary operator, understanding operators in python, the ternary operator in real-world applications, exploring further, recap: mastering the python ternary operator.
The ternary operator in Python is a compact way of writing an if-else statement. It’s used when you want to return two different values based on the truth value of a condition. The syntax is:
Let’s take a look at a simple example:
In this example, the condition x % 2 == 0 checks if x is even. If it is, ‘Even’ is assigned to the variable message . If not, ‘Odd’ is assigned. Thus, the print statement outputs ‘Odd’ as x is 7, which is an odd number.
Advantages and Pitfalls of the Ternary Operator
The ternary operator can make your code more concise and easier to read when used appropriately. It’s great for simple, quick decisions in your code. However, it can become difficult to read and understand when overused or used for complex conditions. It’s best to use the ternary operator sparingly and for simple, clear conditions.
As you become more comfortable with the ternary operator, you might find yourself wondering if you can nest them. The answer is yes, you can, but with caution. Nested ternary operations can make your code compact, but they can also make it harder to read if overused.
Here’s an example of a nested ternary operation:
In this example, we first check if x is divisible by 5. If it is, we print ‘Divisible by 5’. If it’s not, we move on to the next condition, checking if x is even. If it is, we print ‘Even’. If it’s not even (and not divisible by 5), we print ‘Odd’.
Readability Concerns and Best Practices
While nested ternary operations can be useful, they can also make your code more complex and harder to read. As a best practice, avoid nesting ternary operations more than once. If your code requires more complex conditional logic, consider using traditional if-else statements for clarity. Remember, the goal of using the ternary operator should be to make your code more readable and efficient, not less.
While the ternary operator is a powerful tool, Python offers other ways to implement conditional logic. Understanding these alternatives can help you write code that’s easier to read and maintain, especially for complex conditions.
Traditional If-Else Statements
The most straightforward alternative to the ternary operator is a traditional if-else statement. Here’s how you’d write the earlier example using an if-else statement:
This code does exactly the same thing as the nested ternary operation example, but it’s arguably easier to read, especially for beginners.
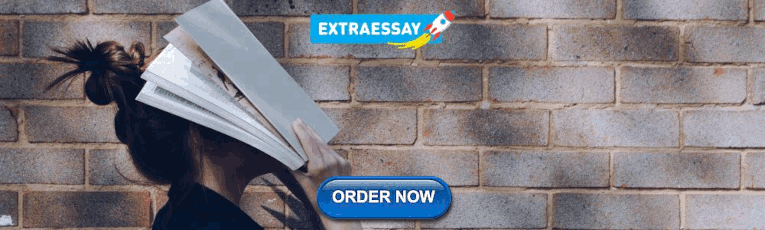
Switch-Case Statements Using Dictionaries
Python doesn’t have built-in switch-case statements like some other languages, but you can mimic them using dictionaries. Here’s an example:
In this example, we create a dictionary where each key-value pair corresponds to a case and its result. We use the get method to retrieve the value for the key x % 5 . If x % 5 isn’t a key in the dictionary, it returns ‘Not divisible by 5’.
While these alternatives might be more verbose than the ternary operator, they can make your code easier to read and understand, which is crucial when you’re working on complex projects or collaborating with other developers.
Like any feature in a programming language, the ternary operator has its quirks and pitfalls. Understanding these can help you avoid bugs and write more effective code. Let’s go over some common issues you might encounter when using the ternary operator in Python.
Operator Precedence Issues
One common issue arises from the fact that the ternary operator has lower precedence than most other operators in Python. This means that in an expression with multiple operators, the ternary operation will be performed last unless you use parentheses to change the order of operations.
Consider the following example:
You might expect this code to print ‘50.0’, but it actually prints ‘0’. That’s because x / y * 100 is evaluated first due to operator precedence, and then the ternary operation is performed.
To get the expected result, you need to use parentheses like so:
By using parentheses, you ensure that the division and multiplication are performed before the ternary operation, giving you the correct result.
Understanding operator precedence and using parentheses appropriately are key to avoiding bugs and confusion when using the ternary operator in Python.
Before we dive deeper into the ternary operator, let’s take a step back and understand what operators are in Python. Operators are special symbols in Python that carry out arithmetic or logical computation. The value or variable that the operator works on is called the operand.
For example, in the expression 5 + 3 , 5 and 3 are operands, and + is an operator.
Categories of Operators in Python
Python has several types of operators, including arithmetic operators ( + , - , * , etc.), comparison operators ( == , != , < , etc.), and logical operators ( and , or , not ).
The ternary operator is a type of conditional operator. It’s a shortcut for the if-else statement and is used to make decisions in your code.
The Role of the Ternary Operator in Python
The ternary operator in Python allows you to evaluate an expression based on a condition being true or false. It significantly shortens the amount of code you need to write and improves readability for simple conditions.
Here’s an example of how you might use it:
In this example, the ternary operator checks if x is greater than 0 . If it is, it assigns the string ‘Hello’ to message . If not, it assigns the string ‘Goodbye’. The ternary operator allows us to do this in a single line of code, making our code more concise and readable.
The ternary operator isn’t just a theoretical concept—it has practical applications in many areas of Python programming. Let’s explore a few of them.
Data Analysis
In data analysis, you often need to categorize or transform data based on certain conditions. The ternary operator makes this process much more streamlined. For instance, you might want to categorize a dataset of heights into ‘Tall’ and ‘Short’. The ternary operator can do this in a single line of code.
Web Development
In web development, the ternary operator can be used to control the flow of your application. For instance, you might want to display a different message to the user based on whether they’re logged in or not.
The ternary operator is part of a larger concept in Python—control flow. Control flow is all about making decisions and controlling the execution of your code. If you’re interested in learning more about control flow and decision making in Python, here are some online resources:
- Click Here for an in-depth overview on conditional statements in Python and how they enable dynamic code.
Using if-else in Python – A practical guide on structuring Python code with “if-else” for decision-making and branching.
Python’s “if not” Statements – Master the art of leveraging “if not” for advanced Python conditionals.
Python Booleans: The Definitive Guide – A guide by W3Schools that focuses on working with booleans in Python.
All About Conditional Execution in Python – A Medium article that delves deep into conditional execution in Python.
Python If-Else Statement – A FreeCodeCamp guide providing explanations on the use of conditional “if-else” statements in Python.
Mastering the ternary operator and other control flow tools will make you a more efficient Python programmer and open up new possibilities in your coding projects.
In this comprehensive guide, we’ve explored the ternary operator in Python, from its basic usage to advanced scenarios. We’ve learned that it’s a shorthand for the if-else statement, allowing us to write more concise and efficient code.
We’ve also delved into common issues, such as operator precedence, which can lead to unexpected results. Remember, using parentheses can help avoid these issues and ensure your ternary operations work as intended.
Additionally, we’ve looked at alternative approaches to conditional logic in Python, including traditional if-else statements and mimicking switch-case statements using dictionaries. While the ternary operator is a powerful tool, these alternatives can provide better readability and clarity in complex situations.
Here’s a quick comparison of the methods we’ve discussed:
As we’ve seen, the ternary operator is a versatile tool in Python, useful in various real-world applications like data analysis and web development. However, its power comes with the responsibility to use it wisely and appropriately. Happy coding!
About Author

Gabriel Ramuglia
Gabriel is the owner and founder of IOFLOOD.com , an unmanaged dedicated server hosting company operating since 2010.Gabriel loves all things servers, bandwidth, and computer programming and enjoys sharing his experience on these topics with readers of the IOFLOOD blog.
Related Posts
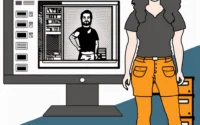

- Online Python Compiler
- Hello World
- Console Operations
- Conditional Statements
- Loop Statements
- Builtin Functions
- Type Conversion
Collections
- Classes and Objects
- File Operations
- Global Variables
- Regular Expressions
- Multi-threading
- phonenumbers
- Breadcrumbs
- ► Python Examples
- ► ► Conditional Statements
- ► ► ► Python Ternary Operator
- Python Datatypes
- Python - Conditional Statments
- Python - If
- Python - If else
- Python - Elif
- Python - If AND
- Python - If OR
- Python - If NOT
- Python - Ternary Operator
- Python Loops
Python Ternary Operator
Syntax of ternary operator.
- A simple example for Ternary Operator
- Print statements in ternary operator
- Nested Ternary Operator
Python Ternary operator is used to select one of the two values based on a condition. It is a miniature of if-else statement that assigns one of the two values to a variable.
In this tutorial, we will learn how to use Ternary Operator in Python, with the help of examples.
The syntax of Ternary Operator in Python is
value_1 is selected if expression evaluates to True . Or if the expression evaluates to False , value_2 is selected.
You can either provide a value, variable, expression, or statement, for the value_1 and value_2 .
In the following examples, we will see how to use Ternary Operator in selection one of the two values based on a condition, or executing one of the two statements based on a condition. We shall take a step further and look into nested Ternary Operator as well.
1. A simple example for Ternary Operator
In this example, we find out the maximum of given two numbers, using ternary operator.
The ternary operator in the following program selects a or b based on the condition a>b evaluating to True or False respectively.
Python Program
Run the program. As a>b returns False, b is selected.
You may swap the values of a and b , and run the program. The condition would evaluate to True and a would be selected.
2. Print statements in ternary operator
In this example, we will write print statements in the ternary operator. Based on the return value of condition, Python executes one of the print statements.
Run the program. As a>b returns False, second print statement is executed.
This example demonstrates that you can run any Python function inside a Ternary Operator.
3. Nested Ternary Operator
You can nest a ternary operator in another statement with ternary operator.
In the following example, we shall use nested ternary operator and find the maximum of three numbers.
After the first else keyword, that is another ternary operator.
Change the values for a, b and c, and try running the nested ternary operator.
In this tutorial of Python Examples , we learned what Ternary Operator is in Python, how to use it in programs in different scenarios like basic example; executing statements inside Ternary Operator; nested Ternary Operator; etc., with the help of well detailed Python programs.
Related Tutorials
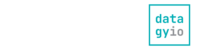
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Inline If in Python: The Ternary Operator in Python
- September 16, 2021 December 20, 2022
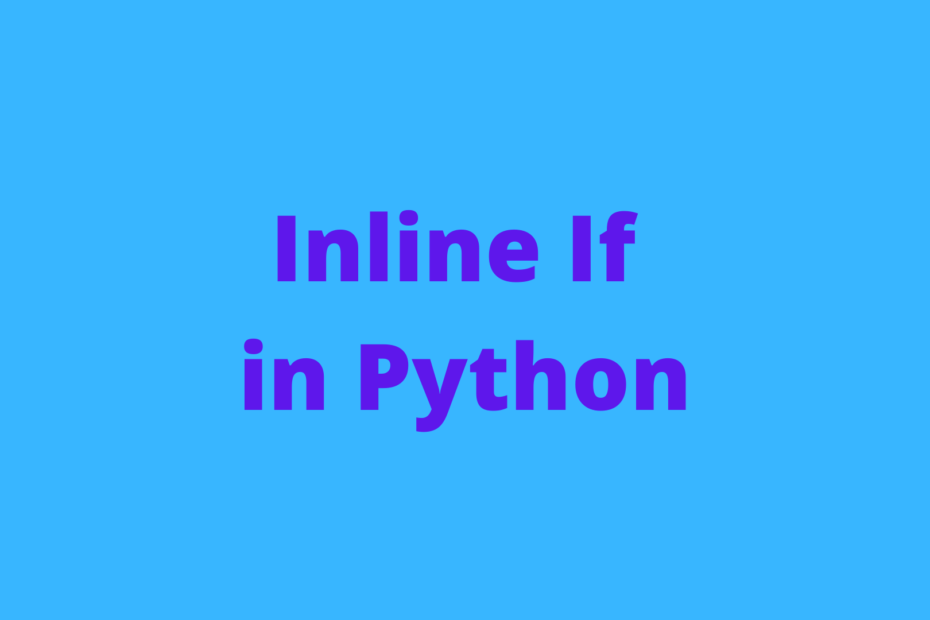
In this tutorial, you’ll learn how to create inline if statements in Python. This is often known as the Python ternary operator, which allows you to execute conditional if statements in a single line, allowing statements to take up less space and often be written in my easy-to-understand syntax! Let’s take a look at what you’ll learn.
The Quick Answer: Use the Python Ternary Operator
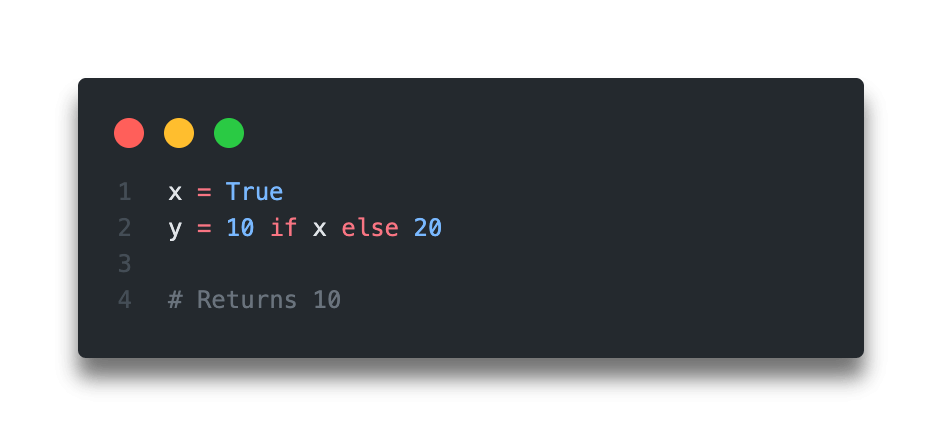
Table of Contents
What is the Python Ternary Operator?
A ternary operator is an inline statement that evaluates a condition and returns one of two outputs. It’s an operator that’s often used in many programming languages, including Python, as well as math. The Python ternary operator has been around since Python 2.5, despite being delayed multiple times.
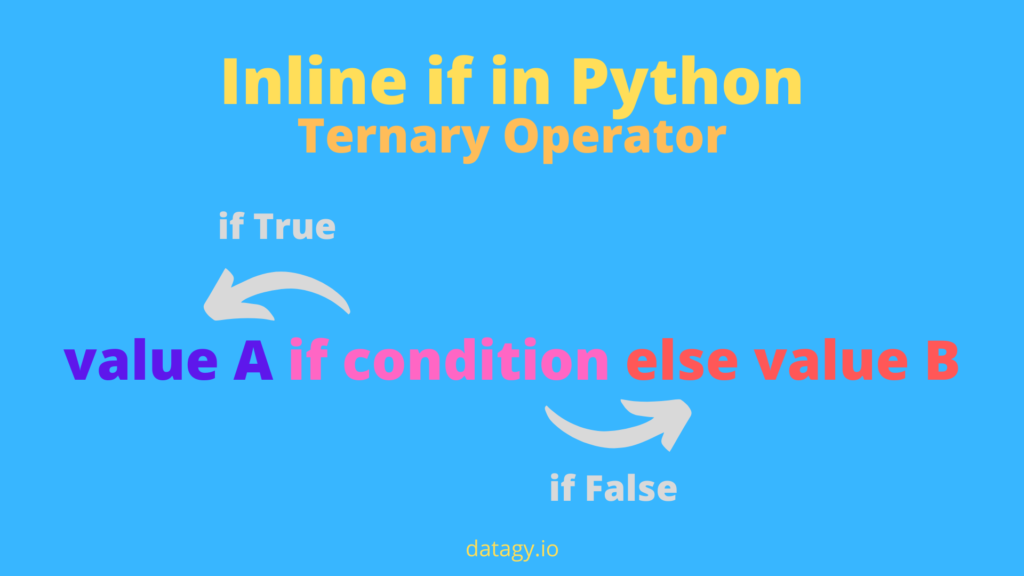
The syntax of the Python ternary operator is a little different than that of other languages. Let’s take a look at what it looks like:
Now let’s take a look at how you can actually write an inline if statement in Python.
How Do you Write an Inline If Statement in Python?
Before we dive into writing an inline if statement in Python, let’s take a look at how if statements actually work in Python. With an if statement you must include an if , but you can also choose to include an else statement, as well as one more of else-ifs, which in Python are written as elif .
The traditional Python if statement looks like this:
This can be a little cumbersome to write, especially if you conditions are very simple. Because of this, inline if statements in Python can be really helpful to help you write your code faster.
Let’s take a look at how we can accomplish this in Python:
This is significantly easier to write. Let’s break this down a little bit:
- We assign a value to x , which will be evaluated
- We declare a variable, y , which we assign to the value of 10, if x is True. Otherwise, we assign it a value of 20.
We can see how this is written out in a much more plain language than a for-loop that may require multiple lines, thereby wasting space.
Tip! This is quite similar to how you’d written a list comprehension. If you want to learn more about Python List Comprehensions, check out my in-depth tutorial here . If you want to learn more about Python for-loops, check out my in-depth guide here .
Now that you know how to write a basic inline if statement in Python, let’s see how you can simplify it even further by omitting the else statement.
How To Write an Inline If Statement Without an Else Statement
Now that you know how to write an inline if statement in Python with an else clause, let’s take a look at how we can do this in Python.
Before we do this, let’s see how we can do this with a traditional if statement in Python
You can see that this still requires you to write two lines. But we know better – we can easily cut this down to a single line. Let’s get started!
We can see here that really what this accomplishes is remove the line break between the if line and the code it executes.
Now let’s take a look at how we can even include an elif clause in our inline if statements in Python!
Check out some other Python tutorials on datagy.io, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas !
How to Write an Inline If Statement With an Elif Statement
Including an else-if, or elif , in your Python inline if statement is a little less intuitive. But it’s definitely doable! So let’s get started. Let’s imagine we want to write this if-statement:
Let’s see how we can easily turn this into an inline if statement in Python:
This is a bit different than what we’ve seen so far, so let’s break it down a bit:
- First, we evaluate is x == 1. If that’s true, the conditions end and y = 10.
- Otherwise, we create another condition in brackets
- First we check if x == 20, and if that’s true, then y = 20. Note that we did not repeated y= here.
- Finally, if neither of the other decisions are true, we assign 30 to y
This is definitely a bit more complex to read, so you may be better off creating a traditional if statement.
In this post, you learned how to create inline if statement in Python! You learned about the Python ternary operator and how it works. You also learned how to create inline if statements with else statements, without else statements, as well as with else if statements.
To learn more about Python ternary operators, check out the official documentation here .
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Master the Potential of Python Ternary Operator
Bashir Alam
January 9, 2024
Welcome to this comprehensive guide on Python ternary operator. Whether you're a beginner just starting out with Python, or an experienced developer looking to deepen your understanding, this article aims to provide you with the knowledge you need to make the most of this powerful language feature.
So, what exactly is a ternary operator? In simple terms, it's a concise way to perform conditional operations in Python . Instead of writing out a full if-else block, you can condense it into a single line of code using the ternary operator. Not only does this make your code shorter, but it can also make it more readable, and even slightly more efficient in some cases.
In the sections to follow, we will delve into the syntax, use-cases, advantages, and limitations of using the ternary operator in Python. We'll also discuss best practices, common pitfalls to avoid, and answer some frequently asked questions. Let's get started!
Definition of Python Ternary Operator
The ternary operator is a concise way of executing conditional statements in Python. It allows you to evaluate an expression and return a value based on whether the expression evaluates to True or False . Unlike conventional if-else statements that can span multiple lines, a ternary operator can accomplish the same in a single line of code. The basic syntax is:
Here's how it works:
- condition : This is the Boolean expression that the operator evaluates.
- value_if_true : This is the value that result will take if the condition is True .
- value_if_false : This is the value that result will take if the condition is False .
For example:
In this example, y will be assigned the value "Even" because x % 2 == 0 is True.
In Python, you may sometimes encounter the ternary operator used in combination with other Pythonic structures like tuple unpacking or even lambda functions , but the core syntax remains the same.
Simple Examples
Let's look at some simple, straightforward examples to help you get a better understanding of how the Python ternary operator works:
Checking if a number is positive, negative, or zero:
String formatting based on condition:
In list comprehensions:
Brief Comparison with Traditional If-Else Statements
Although both Python ternary operators and traditional if-else statements are used for conditional logic, there are key differences between them:
The ternary operator is more concise, allowing for shorter code. For example, consider this if-else block:
Using a Python ternary operator, the same logic can be condensed into a single line:
The ternary operator can make the code more readable when the conditional logic is simple. However, for more complex conditions, using a traditional if-else block might be more readable.
Traditional if-else statements are more versatile and can handle more complex logic with multiple conditions using elif. Ternary operators are best suited for straightforward conditions that result in a single outcome for True and False cases.
Use Cases for Beginners
Value Assignment
One of the most straightforward uses of the Python ternary operator is assigning a value to a variable based on a condition:
Simple Conditionals
The Python ternary operator shines in scenarios where you need to make a quick, simple decision within your code. For example, setting a flag based on user input:
It allows for more concise and often more readable code for simple conditional checks.
How Python Ternary Operator Works?
Once you've grasped the basics of the Python ternary operator, understanding its internals can offer you a deeper level of insight. This section aims to elucidate how the ternary operator works under the hood, focusing on evaluation order, return values, and the type of expressions allowed.
1. Evaluation Order
One of the key aspects to understand about the ternary operator is the order in which it evaluates its components. The general syntax, as a reminder, is:
Here's how the evaluation order works:
- First , the condition is evaluated.
- Next , based on whether the condition is True or False , either value_if_true or value_if_false is evaluated and returned. The other value is not evaluated at all, making the ternary operator a "short-circuit" operator.
In this example, because y != 0 evaluates to False , Python directly goes to the value_if_false , i.e., "Division by zero," without attempting to evaluate x / y , thus avoiding a runtime error.
2. Return Values
The return value of a Python ternary operation is the value that corresponds to the evaluated condition. Therefore, it could either be value_if_true or value_if_false , depending on the condition. This makes the Python ternary operator quite flexible in the types of operations it can perform and the types of data it can return.
3. Type of Expressions Allowed
The Python ternary operator is quite flexible when it comes to the types of expressions you can use for condition , value_if_true , and value_if_false . However, there are some considerations:
- Condition : Must evaluate to a Boolean value ( True or False ). It can be a comparison, logical operation, or any expression that returns a Boolean.
- value_if_true and value_if_false : Can be of any data type, even different types from each other. However, it's best practice to keep them of the same type for readability and predictability.
- Complex Expressions : You can use more complex expressions, like function calls or mathematical operations, but it may hamper readability.
Example with different types:
Example with function calls:
Advanced Usage
Here we'll look at chaining and nesting Python ternary operators, its use with functions and lambda expressions, as well as its application in data structures like lists, tuples, and dictionaries.
1. Using Ternary Operator in Function Calls
The Python ternary operator can be directly embedded in function calls to make the code more concise while still being readable. The key is to maintain the balance between brevity and readability.
Basic Example:
With Multiple Arguments:
You can use the Python ternary operator for one or more arguments in a function call.
Inline Decision Making:
The Python ternary operator can help you make inline decisions while calling a function, like choosing between different functions or methods to call.
2. Lambda Functions and Ternary Operator
Lambda functions in Python are anonymous functions defined using the lambda keyword. Since they are limited to a single expression, using the ternary operator within lambda functions can be very useful for simple conditional logic.
Basic Usage:
Here's a simple example that uses the Python ternary operator within a lambda function :
Multiple Conditions:
You can even chain Python ternary operations inside a lambda function for handling multiple conditions, although this can hurt readability if overused.
Use in Higher-Order Functions:
Lambda functions often find use in higher-order functions like map , filter , and sorted . The Python ternary operator can be quite useful in such cases.
In this example, the squared_or_cubed list will contain the squares of even numbers and cubes of odd numbers from the numbers list.
3. Chaining Ternary Operators
Chaining multiple Python ternary operators can help you represent more complex logic in a single line. While it provides brevity, be careful not to compromise readability.
Basic Chaining:
Extended Chaining:
4. Nested Ternary Operators
Nested ternary operators involve placing one or more ternary expressions inside another. While this can make your code more concise, it can also make it less readable and harder to debug if not used carefully.
Imagine you're choosing what to wear based on the weather. If it's sunny, you'll wear sunglasses. If it's not sunny but cloudy, you'll grab an umbrella just in case. If it's neither sunny nor cloudy, you decide to stay indoors. A Python ternary operator helps you make this decision in one go, and if you have to make another decision based on these conditions, you can "nest" another decision inside the first one. This is called a "nested ternary operator."
Example 1: Choosing a Drink
You go to a café. If they have orange juice, you'll take it. If they don't but have apple juice, you'll take that. If they have neither, you'll settle for water.
In Python, you could represent this decision like so:
Here, the decision about apple juice is "nested" inside the decision about orange juice. If drink_available is "orange", choice becomes "orange juice". Otherwise, another ternary operation is evaluated.
Example 2: Weather Example
You're deciding whether to go outside based on the weather. If it's sunny, you'll go to the beach. If it's cloudy but not raining, you'll go to a park. Otherwise, you'll stay home.
Here's how you could do that in Python:
If weather is "sunny", activity will be "beach". If weather is "cloudy", activity will be "park". For all other weather types, activity will be "home".
5. Ternary Operator with Lists, Tuples, and Dictionaries
You can use the ternary operator to conditionally construct or modify these data types.
List Comprehension:
Tuple Construction:
Dictionary Construction:
Performance Considerations
While the Python ternary operator provides a more compact way of writing conditionals, it's essential to consider its performance impact. This section will delve into the speed comparison with traditional if-else statements, its usage within class definitions, interoperability with Python's Walrus operator, and memory considerations.
1. Speed Comparison with If-Else
Generally speaking, the ternary operator tends to perform slightly faster than an if-else block for simple conditionals because it is optimized for such scenarios. However, the performance difference is often negligible and should not be the primary reason for choosing one over the other.
From the results, it appears that using_ternary is slightly faster than using_if_else . However, the difference is quite small (in the order of milliseconds for a million iterations), so in most real-world applications, you likely won't notice a performance difference between the two.
It's worth noting that while the ternary operator can be faster for simple conditions, the primary reason to use it is for code readability and brevity for straightforward conditions. For complex conditions or multi-step operations, a traditional if-else statement is usually more readable and should be preferred.
2. Ternary Operator in Class Definitions
Using the ternary operator within class definitions can lead to cleaner, more Pythonic code.
3. Using with Python’s Walrus Operator
Python 3.8 introduced the Walrus Operator ( := ), which allows assignment and evaluation in a single statement. You can use it in conjunction with the ternary operator to both evaluate and use a value conditionally.
4. Memory Usage
Memory usage generally isn't a major concern when using the ternary operator compared to traditional if-else statements for simple conditions. Both approaches are quite efficient in that regard. However, if the ternary operator's expressions involve creating large data structures or other memory-intensive operations, then memory usage could be a consideration.
Memory-Intensive Example:
In the above example, regardless of whether some_condition is True or False, a list with 1 million elements will be created, taking up a significant amount of memory.
Common Mistakes, Limitations, and Pitfalls
While the ternary operator in Python can make your code more concise, it's not without its drawbacks and potential for misuse. This section will highlight some common mistakes, limitations, and pitfalls you should be aware of.
When Not to Use Ternary Operators
- Complex Conditions : If the condition involves multiple and/or/nor logic, it's better to stick to if-else blocks for clarity.
- Multiple Actions : If you need to perform more than one action based on the condition, the ternary operator is not suitable.
- Long Expressions : If the expressions for value_if_true or value_if_false are long and complicated, they can make the ternary statement hard to read.
Overusing Ternary Operators
- Chaining : Excessive chaining of ternary operators can make your code difficult to understand and debug.
- Nesting : While nesting is possible, it often leads to unreadable code.
Type-related Mistakes
Type Inconsistency : Using different types for value_if_true and value_if_false can lead to unexpected behavior. For example:
In this example, x could either be an integer or a string, depending on some_condition . This can create issues later in the code.
Implicit Type Conversion : Python's dynamic typing can sometimes result in implicit type conversions, which might not be what you expect.
In this example, result could be either an integer or a float , which could lead to precision issues in calculations.
Error Handling
While the Python ternary operator simplifies conditional logic, it's not entirely immune to issues that can lead to errors or bugs. Understanding the kinds of errors that might occur and how to debug them is crucial. This section covers syntax errors, logical errors, and offers some debugging tips.
Syntax Errors
Syntax errors are mistakes in the language structure that the interpreter can catch before your program runs.
Incorrect Ordering : The ternary operator has a specific order: value_if_true if condition else value_if_false .
Missing Components : Omitting any part of the ternary operator will result in a syntax error.
Logical Errors
Logical errors occur when your program runs without crashing but doesn't produce the expected output.
Inverted Condition : Sometimes, you might accidentally invert the true and false parts of the ternary operator.
Chained Confusion : When chaining multiple ternary operators, keeping track of conditions can get confusing, leading to logical errors
Debugging Tips
Break It Down : If you're chaining or nesting ternary operators and encountering issues, break them down into separate if-else blocks for easier debugging.
Print Statements : Inserting print statements can help debug the flow of conditional logic. For example:
Code Formatting : Sometimes, simply formatting the code clearly can help identify errors in your ternary logic.
Comparison with Other Languages
The ternary conditional operator exists in many programming languages, although its syntax and capabilities can vary. Understanding these differences can be especially useful if you are transitioning from one language to another or working in a multi-language environment.
Ternary in C, C++, Java, etc.
The syntax for the ternary operator in languages like C, C++, and Java is usually in the form of condition ? value_if_true : value_if_false .
Example in C:
Example in Java:
Common Features:
- Type Safety : In statically typed languages like C++ and Java, the types of value_if_true and value_if_false usually must be compatible.
- Short-circuiting : Just like in Python, these languages also evaluate the condition and only one of the value_if_true or value_if_false , not both.
Uniqueness in Python
Python's syntax is somewhat more readable and fits better with its overall syntax style. Here's how the Python ternary operator is unique:
In Python, the ternary operator takes the form of value_if_true if condition else value_if_false .
Top 10 Frequently Asked Questions
What is the Python Ternary Operator?
The Python ternary operator is a shorthand way of writing an if-else statement. It allows you to return a value based on a condition, all in a single line.
How is the Ternary Operator Different from Traditional If-Else Statements?
The ternary operator is more concise than traditional if-else statements and is often used for simple, straightforward conditions. However, it is not suitable for complex conditions or multiple actions based on a condition.
Can I Nest Ternary Operators?
Yes, you can nest ternary operators, but it can make your code hard to read and understand. It's generally not recommended for complex conditions.
Is the Ternary Operator Faster Than If-Else Statements?
For simple conditions, the ternary operator can be slightly faster, but the performance difference is generally negligible for most applications.
Can I Use the Ternary Operator with Functions?
Yes, you can use the ternary operator within function calls or even within the definition of a function, as long as you adhere to its syntax and limitations.
What Types of Expressions Can I Use with the Ternary Operator?
You can use any expression that returns a value, including function calls, arithmetic operations, or even other ternary operations, as long as they fit within the syntax requirements.
Can I Use the Ternary Operator in List Comprehensions?
Yes, the ternary operator can be used in list comprehensions for conditional value assignment.
Are There Memory or Performance Concerns with the Ternary Operator?
Memory and performance are generally not major concerns for the ternary operator when compared to traditional if-else statements. However, be cautious when the expressions involved are memory-intensive or computationally heavy.
What Are Common Mistakes to Avoid?
Common mistakes include inverting the true and false parts of the operator, omitting parts of the syntax, or using it in situations where an if-else statement would be more appropriate due to complexity.
Can I Chain Multiple Ternary Operators Together?
Yes, you can chain multiple ternary operators, but doing so can make your code harder to read and debug. Use this feature sparingly and consider breaking down complex chains into simpler parts or using traditional if-else statements.
The Python ternary operator serves as a shorthand for conditional if-else statements , allowing for more concise and sometimes more readable code. While it offers various benefits, such as brevity and some performance advantages, it's essential to understand its limitations, syntax quirks, and best-use cases to leverage it effectively.
Key Takeaways
- Syntax is King : Ensure you understand the value_if_true if condition else value_if_false structure.
- Readability Over Brevity : Always prioritize code readability. If a ternary operator complicates understanding, consider using a traditional if-else block.
- Limited to Simple Cases : The ternary operator is best for simple, straightforward conditions and should not replace complex if-else statements.
- Dynamic and Flexible : Due to Python’s dynamic typing, you can use various types of expressions, but be cautious to maintain consistency.
- Debugging Challenges : Though concise, ternary operators can be tricky to debug, especially when nested or chained.
- Performance : While there can be slight performance benefits, they are often negligible for most real-world applications.
Additional Resources
For further reading and more in-depth understanding, you may consult the Python official documentation on conditional expressions .
He is a Computer Science graduate from the University of Central Asia, currently employed as a full-time Machine Learning Engineer at uExel. His expertise lies in OCR, text extraction, data preprocessing, and predictive models. You can reach out to him on his Linkedin or check his projects on GitHub page.
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
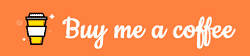
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
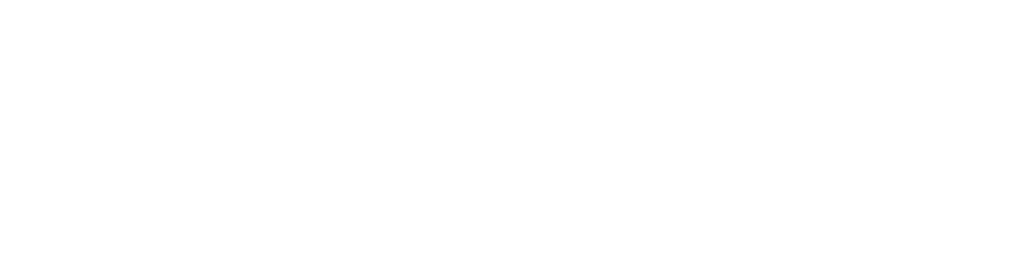
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Programming Languages
Exam certifications.
Certified Kubernetes Application Developer (CKAD)
CompTIA PenTest+ (PT0-002)
Red Hat EX407 (Ansible)
Hacker Rank Python
Privacy Policy
HTML Sitemap
Learn Python Ternary Operator With Examples
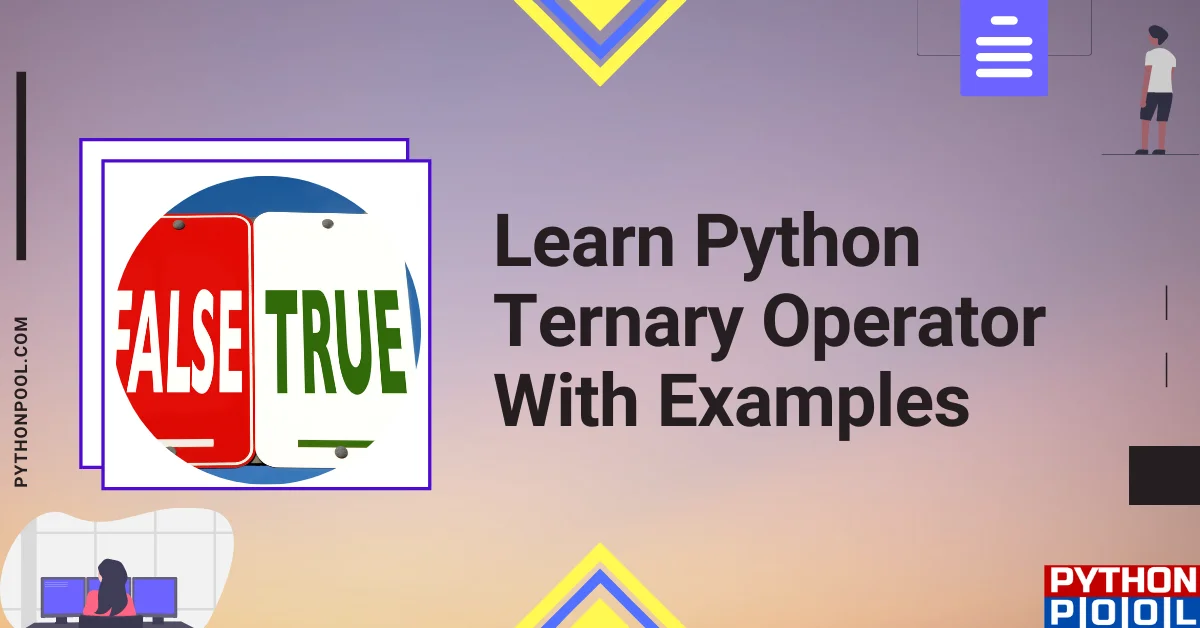
Python is notorious for one-liners. You can write an entire for loop in a single line using list comprehension, swap variables, and the list goes on. Moreover, in comparison to other high-level languages, python code is the most concise and readable. Python has another one-liner called the ternary operator for conditional computing statements.

Have a look at the image above. Does it feel familiar? If yes, you might have worked with it in other languages like C or Java or any different language. If not , then don’t worry. We will go through its working. However, first, we will look at traditional conditional statements.

Python’s Ternary Operator
As the name implies, ternary contains three operands. One is the condition that gets evaluated first. And then the condition short circuits to either true or false accordingly.

The ternary operator was non-existent before version 2.5. Besides, python’s ternary operator’s syntax is a little different from other languages. Python’s ternary operator doesn’t have ‘ ? ‘ and ‘ : ‘. Instead, it uses if-else keywords. Look at the syntax below:
Now let’s try to write down the if-else code in the introduction using the ternary operator.
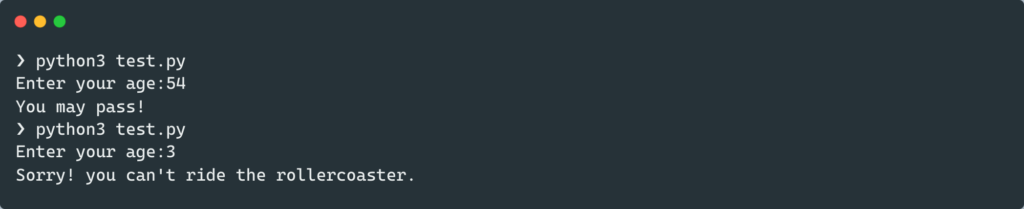
Examples of Python’s ternary operator
Let’s look at some examples of the ternary operator to consolidate our understanding.
Checking the greatest of two numbers.

Example 2: Nesting in ternary operator
I am checking the greatest of three numbers.

Alternative to ternary operators
Python’s tuple ternary operator.
Python has yet another way to use ternary operator-like functionality. Although, this method isn’t used much and has a disadvantage to it. Let’s discuss its syntax, work through some examples, and compare it to the regular python ternary operator.

As a matter of fact, the tuple operator feels similar to a regular python ternary operator. However, its inner working is quite the opposite. For instance, let’s take an example to clarify this distinction.
If you try to run the following code, the expected outcome should be ‘ 2 ‘. However, instead, we get a zero division error. This is because it evaluates both the expressions, unlike a regular python ternary operator, which short circuits to either expression.

Python’s List ternary operator
Like the tuple ternary operator, you can also use a list in place of a tuple. Let’s look at an example.
greet = False greet_person = [‘Hi’, ‘Bye’][greet] print(f'{greet_person}, Jiraya.’)

Python’s Dictionary ternary operator
Let’s look at an example of how can we can use it. For instance:

Ternary operator with lambda
Lambda or anonymous functions too can be used with the ternary operator in Python. We can apply conditions on the ternary operator, for instance:
The code above applies a discount to an item purchased if its price range is greater or less than 1000. When the price is more significant than 1000, a discount of 10% is applied, while for item prices less than 1000, only a 2% discount is added.

Ternary operator assignment multiple lines
You can assign logical lines into multiple physical lines using the parenthesis, square brackets, or even curly braces. Splitting expressions or lines of code into multiple physical lines is termed implicit line joining . Let’s look at an example:

Precedence of the ternary operator
Ternary operators or conditional expressions have low precedence. However, when used inside the parenthesis, square brackets, or curly braces, its precedence should increase.
Ternary operator without else in Python
While the regular ternary operator doesn’t support operation, moreover, it will throw an invalid syntax error if you try to do so. To demonstrate, we have commented on the else part of the ternary operator.

If you try to run the program, a syntax error will be thrown, as shown in the image above.
So what is the workaround to this problem? Long story short, the ternary operator doesn’t have such functionality. Therefore, we will use a logical operator and a condition. For instance:
We have to use the following syntax:
<condition> and <some operation>
So, and operator evaluates the proper side operation only if the condition is true. However, if the condition turned to be false, it won’t be operated.

FAQs on Python Ternary Operator
if_true if condition else if_false
The ternary operator was introduced in python version 2.5. However, its syntax is different from other programming languages.
Ternary operator automatically does short-circuiting. Ternary contains three operands. One is the condition that gets evaluated first. As a result, the condition short circuits to either true or false accordingly.
Yes, we can do a null ternary check using shorthand ternary . For instance: value = None msg = value or "Value is None" print(msg) Output: Value is None
The following article covered Python’s ternary operator. We looked at how it is different when compared to other programming languages’ ternary operators. Moreover, we also tried to understand its functioning using some examples.
Trending Python Articles
![python ternary operator with assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
Operator Associativity in Programming
- What are Operators in Programming?
- Types of Operators in Programming
- Difference between Operator Precedence and Operator Associativity
- Associative Property
- Associative Property of Multiplication
- Assignment Operators In C++
- Operators in Objective-C
- Operator Precedence and Associativity in C
- Precedence and Associativity of Operators in Python
- Floating Point Operations & Associativity in C, C++ and Java
- Assignment Operators in C
- Increment (++) and Decrement (--) Operator Overloading in C++
- Arithmetic Operators in C
- Operators in C
- Operators in C++
- Operators in Scala
- Operators Precedence in Scala
- Java Assignment Operators with Examples
- C | Operators | Question 23
- Program to print ASCII Value of a character
- Introduction of Programming Paradigms
- Program for Hexadecimal to Decimal
- Program for Decimal to Octal Conversion
- A Freshers Guide To Programming
- ASCII Vs UNICODE
- How to learn Pattern printing easily?
- Loop Unrolling
- How to Learn Programming?
- Program to Print the Trapezium Pattern
Operator associative refers to the order in which operators of the same precedence are used in a word. In a programming language, it is important to understand the interactions between operators to properly define and test expressions. In this article, we will discuss operator associativity in programming.
Table of Content
- Operator Associativity in Arithmetic Operators
- Operator Associativity in Relational Operators
- Operator Associativity in Logical Operators
- Operator Associativity in Assignment Operators
- Operator Associativity in Bitwise Operators
- Operator Associativity in Conditional (Ternary) Operator
- Operator Associativity in Unary Operators
- Operator Associativity in C
- Operator Associativity in C++
- Operator Associativity in Java
- Operator Associativity in Python
- Operator Associativity in C#
- Operator Associativity in Javascript
Here is a table illustrating the Operator Associativity in Programming:
Operator Associativity in Arithmetic Operators:
Mathematical operations such as addition (+), subtraction (-), multiplication (*), division (/), and parameter (%), are usually symmetrical from left to right. This means that if there are multiple preceding functions in an expression, the processing is done from left to right .
int result = 5 + 3 * 2; // result will be 11 (5 + (3 * 2))
In the expression `5 + 3 * 2`, multiplication (*) including left-right association, takes higher priority and is considered first. So, `3 * 2` is `6`. The addition (+) is then performed, resulting in `5 + 6`, which equals `11`. This arrangement ensures that actions flow from left to right, mirroring the natural reading pattern. Left to right associations remain consistent, ensuring terms are evaluated as predicted without the need for clear parentheses to indicate the order Overall, it ensures clarity and simplicity in mathematical terms by an implicit sequence of activities based on the location of operators in a word.
Operator Associativity in Relational Operators:
Relational operators, like equality (==), inequality (!=), greater than (>), and less than (<), usually have left-to-right associativity as well.
boolean condition = 2 < 5 == true; // condition will be true ((2 < 5) == true)
In the case of relational expressions such as `2 < 5 == true`, the left-to-right association implies a left-to-right evaluation. So, they first check `2 < 5`, get `true`, then evaluate `true == true`, resulting in `true`. This chart ensures accuracy when comparing values from left to right, and reflects a natural reading pattern. Left-to-right associations provide clarity by describing predictable behavioral patterns, conforming to observable expectations, and facilitating lexical analysis without the need for explicit parentheses and therefore `conditioning ` for `true`, which means that `2` is indeed less than `5`, and `true` is `true` It is equivalent.
Operator Associativity in Logical Operators:
Logical operators, including AND (&&) and OR (||), often exhibit left-to-right associativity.
bool_result = True or False and True # bool_result will be True (True or (False and True))
In logical terms such as `True or False and True`, a left-to-right association implies a left-to-right evaluation. Thus, `false and true` is the first analysis, which leads to `false`. A `true or false` evaluation is then performed, resulting in a `true` result. This process mirrors the natural reading process and ensures that logical positions are consistently explored from left to right. Left-to-right association provides clarity by defining a predictable order of execution, facilitates word analysis without the need for explicit parentheses. As a result, `bool_result` is assigned `True`, indicating that the first condition is true or the second condition evaluates to true.
Operator Associativity in Assignment Operators :
Assignment operators, like the simple assignment (=) and compound assignments (e.g., +=), typically have right-to-left associativity.
int a, b; a = b = 5; // Both a and b will be assigned the value 5 (b = 5, then a = b)
In applications like `a = b = 5`, the right-to-left association implies right-to-left analysis. As a result, the value `5` (`b = 5`) is first assigned to `b`, followed by the value `b` (`a = b`). This sequence ensures that `a` and `b` end up with the same value `5`. The right-to-left association simplifies the usage case by specifying an explicit usage pattern without the need for explicit parentheses, making the code readable and simple and thus providing `5` both `a` and `b`, making it a shorter and more efficient chaining function.
Operator Associativity in Bitwise Operators :
Bitwise operators, such as AND (&), OR (|), and XOR (^), usually have left-to-right associativity.
let result = 1 | 2 & 3; // result will be 3 (1 | (2 & 3))
`1 | 2 & 3`, left-to-right association versus left-to-right analysis. Thus, `2 & 3` are considered first, and `2` is the result. Then, `1 | 2` is evaluated, resulting in `3`. Left-to-right association ensures consistent analysis of bitwise functions while providing clarity in reference semantics. This association simplifies Bitwiz vocabulary by providing an explicit sequence of functions without the need for explicit parentheses. Consequently, a `result` is assigned to `3`, indicating a bitwise OR operation between `1` and `2`, after a bitwise AND operation between `2` and `3`.
Operator Associativity in Conditional (Ternary) Operator :
The conditional operator (?:) has right-to-left associativity.
let max = (a > b) ? a : b; // max will be the greater of a and b
The conditional function `(a > b) ? a : b`, right-to-left association versus right-to-left analysis. Consequently, if `a` is greater than `b`, then `a` is chosen; otherwise `b` is selected. Right-to-left association simplifies conditional terminology, and suggests a clear order of operation without the need for explicit parentheses. This association ensures that the conditional term is correctly evaluated, whereby the condition is first evaluated, followed by the selection of `a` or `b` depending on the condition and thus the `max` value of a higher between `a` and ` b`, in order to provide for shortened appointments Effective and conditional appointments.
Operator Associativity in Unary Operators :
Unary operators, like negation (-) and logical NOT (!), often have right-to-left associativity.
result = -(-5); # result will be 5 (-(-5))
In single expressions such as `-(-5)`, the right-to-left association implies right-to-left analysis. Initially, the internal negation `-5` is computed, resulting in `-(-5)` equal to `5`. Right-to-left association ensures that external negation acts on the consequences of internal negation. As a result, `-(-5)` evaluates to `5`, indicating that a negation is rejected. This association simplifies single terms and indicates a clear sequence of functions without the need for explicit parentheses. Thus, the resulting value is `5`, because the outer negation eventually negates the result, resulting in the original value
Operator Associativity in C:
Here are the implementation of Operator Associativity in C language:
Operator Associativity in C++:
Here are the implementation of Operator Associativity in C++ language:
Operator Associativity in Java:
Here are the implementation of Operator Associativity in java language:
Operator Associativity in Python:
Here are the implementation of Operator Associativity in python language:
Operator Associativity in C#:
Here are the implementation of Operator Associativity in C# language:
Operator Associativity in Javascript:
Here are the implementation of Operator Associativity in javascript language:
Conclusion:
Operator associativity determines the order in which operators of the same precedence are evaluated in an expression. In simple terms, it decides whether operators are evaluated from left to right or from right to left. This helps clarify the sequence of operations in complex expressions and ensures consistency in the behavior of operators.
Please Login to comment...
Similar reads.
- Programming
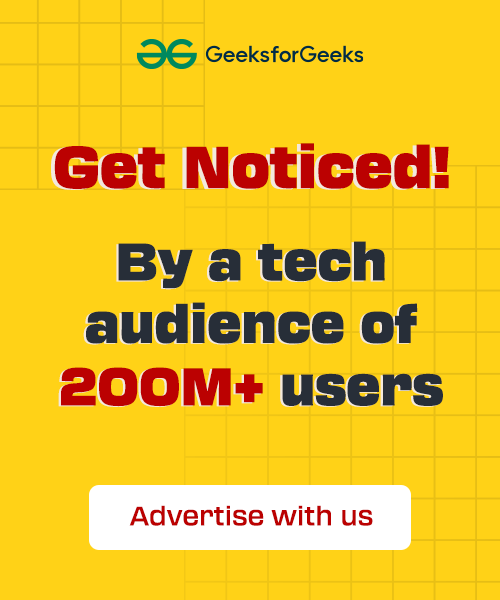
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
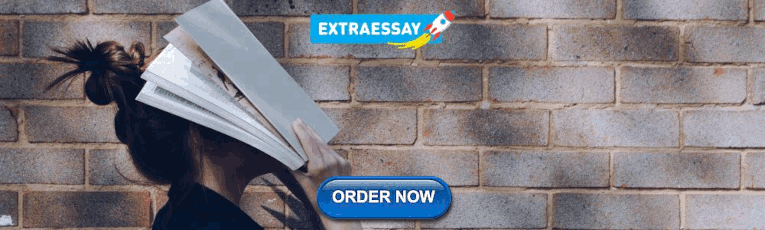
IMAGES
VIDEO
COMMENTS
1. For those interested in the ternary operator (also called a conditional expression ), here is a way to use it to accomplish half of the original goal: q = d[x] if x in d else {} The conditional expression, of the form x if C else y, will evaluate and return the value of either x or y depending on the condition C.
Note that each operand of the Python ternary operator is an expression, not a statement, meaning that we can't use assignment statements inside any of them. Otherwise, the program throws an error: ... This way of using the Python ternary operator isn't popular compared to its common syntax because, in this case, both elements of the tuple are ...
Syntax of Ternary Operator in Python. Syntax: [on_true] if [expression] else [on_false] expression: conditional_expression | lambda_expr. Simple Method to Use Ternary Operator. In this example, we are comparing and finding the minimum number by using the ternary operator.
The expression on the right side returns 20 if the age is greater than or equal to 18 or 5 otherwise. The following syntax is called a ternary operator in Python: value_if_true if condition else value_if_false Code language: Python (python) The ternary operator evaluates the condition. If the result is True, it returns the value_if_true.
Python Ternary Assignment. The ternary operator is mostly used in assigning values to variables. When you have to decide different values of a variable based on the condition, then you can use the ternary operator. Using a ternary operator for assigning values also makes the code more readable and concise. Example 3
Conditional Expressions (Python's Ternary Operator) ... A common use of the conditional expression is to select variable assignment. For example, suppose you want to find the larger of two numbers. Of course, there is a built-in function, max(), that does just this (and more) that you could use. But suppose you want to write your own code ...
comprehension::= assignment_expression comp_for comp_for ::= ["async"] ... operator is intended to be used for matrix multiplication. No builtin Python types implement this operator. New in version 3.5. The / ... (sometimes called a "ternary operator") have the lowest priority of all Python operations. ...
Here is a simple Python program where we find the greatest of 2 numbers using the ternary operator method. If [x>y] is true it returns 1, so the element with 1 index will be printed. Otherwise if [x>y] is false it will return 0, so the element with 0 index will be printed. Python ternary operator with Tuples.
Basics of the conditional expression (ternary operator) In Python, the conditional expression is written as follows. The condition is evaluated first. If condition is True, X is evaluated and its value is returned, and if condition is False, Y is evaluated and its value is returned. If you want to switch the value based on a condition, simply ...
The Python ternary operator provides a quick and easy way to build if-else sentences. It first analyses the supplied condition and then returns a value based on whether that condition is True or False. In the following post, we addressed Ternary Operator, one of Python's most effective tools, which has decreased code size by replacing typical ...
Programmers like to use the concise ternary operator for conditional assignments instead of lengthy if-else statements. The ternary operator takes three arguments: Firstly, the comparison argument. Secondly, the value (or result of an expression) to assign if the comparison is true.
The Role of the Ternary Operator in Python. The ternary operator in Python allows you to evaluate an expression based on a condition being true or false. It significantly shortens the amount of code you need to write and improves readability for simple conditions. Here's an example of how you might use it:
The syntax of Ternary Operator in Python is. [value_1] if [expression] else [value_2] value_1 is selected if expression evaluates to True. Or if the expression evaluates to False, value_2 is selected. You can either provide a value, variable, expression, or statement, for the value_1 and value_2.
A ternary operator is an inline statement that evaluates a condition and returns one of two outputs. It's an operator that's often used in many programming languages, including Python, as well as math. The Python ternary operator has been around since Python 2.5, despite being delayed multiple times.
The Python Ternary Operator is a type of condition expression in the Python programming language that allows the developers to evaluate statements. ... Python 3.8 introduced the Walrus Operator (:=), which allows assignment and evaluation in a single statement. You can use it in conjunction with the ternary operator to both evaluate and use a ...
6. Ternary Operators. Edit on GitHub. 6. Ternary Operators ¶. Ternary operators are more commonly known as conditional expressions in Python. These operators evaluate something based on a condition being true or not. They became a part of Python in version 2.4. Here is a blueprint and an example of using these conditional expressions.
The ternary operator was non-existent before version 2.5. Besides, python's ternary operator's syntax is a little different from other languages. Python's ternary operator doesn't have ' ? ' and ' : '. Instead, it uses if-else keywords. Look at the syntax below: if_true if condition else if_false
Ternary Operator. Ternary operators provide a way to quickly test a condition instead of using a multiline if statement. Thus, we can shorten our code significantly (yet maintain readability) by using ternary operators, which have the following format: x if C else y. As the name suggests (Ternary), Ternary operators consists of three operands ...
Ternary operator is very useful, why it does not work in this particular case: c="d" d={} d[c]+=1 if c in d else d[c]=1 It gives: d[c]+=1 if c in d else d[c]=1 ^ SyntaxError: invalid syntax I don't see nothing wrong here since the same thing without the ternary operator works:
Operator associative refers to the order in which operators of the same precedence are used in a word. In a programming language, it is important to understand the interactions between operators to properly define and test expressions. In this article, we will discuss operator associativity in programming.
python ternary operator with assignment. 10. Using statements on either side of a python ternary conditional. 0. if as a ternary operator python. 6. Assign two variables with ternary operator. 3. Conditional expression/ternary operator. 0. performing more that two actions in ternary conditional operator. 2.