- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
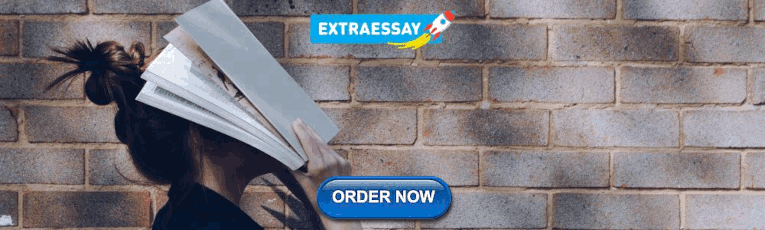
Assignment (=)
Baseline widely available.
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Basic assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript operators.
Javascript operators are used to perform different types of mathematical and logical computations.
The Assignment Operator = assigns values
The Addition Operator + adds values
The Multiplication Operator * multiplies values
The Comparison Operator > compares values
JavaScript Assignment
The Assignment Operator ( = ) assigns a value to a variable:
Assignment Examples
Javascript addition.
The Addition Operator ( + ) adds numbers:
JavaScript Multiplication
The Multiplication Operator ( * ) multiplies numbers:
Multiplying
Types of javascript operators.
There are different types of JavaScript operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- String Operators
- Logical Operators
- Bitwise Operators
- Ternary Operators
- Type Operators
JavaScript Arithmetic Operators
Arithmetic Operators are used to perform arithmetic on numbers:
Arithmetic Operators Example
Arithmetic operators are fully described in the JS Arithmetic chapter.
Advertisement
JavaScript Assignment Operators
Assignment operators assign values to JavaScript variables.
The Addition Assignment Operator ( += ) adds a value to a variable.
Assignment operators are fully described in the JS Assignment chapter.
JavaScript Comparison Operators
Comparison operators are fully described in the JS Comparisons chapter.
JavaScript String Comparison
All the comparison operators above can also be used on strings:
Note that strings are compared alphabetically:
JavaScript String Addition
The + can also be used to add (concatenate) strings:
The += assignment operator can also be used to add (concatenate) strings:
The result of text1 will be:
When used on strings, the + operator is called the concatenation operator.
Adding Strings and Numbers
Adding two numbers, will return the sum, but adding a number and a string will return a string:
The result of x , y , and z will be:
If you add a number and a string, the result will be a string!
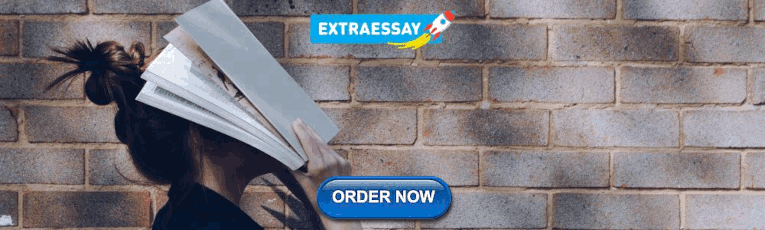
JavaScript Logical Operators
Logical operators are fully described in the JS Comparisons chapter.
JavaScript Type Operators
Type operators are fully described in the JS Type Conversion chapter.
JavaScript Bitwise Operators
Bit operators work on 32 bits numbers.
The examples above uses 4 bits unsigned examples. But JavaScript uses 32-bit signed numbers. Because of this, in JavaScript, ~ 5 will not return 10. It will return -6. ~00000000000000000000000000000101 will return 11111111111111111111111111111010
Bitwise operators are fully described in the JS Bitwise chapter.
Test Yourself With Exercises
Multiply 10 with 5 , and alert the result.
Start the Exercise
Test Yourself with Exercises!
Exercise 1 » Exercise 2 » Exercise 3 » Exercise 4 » Exercise 5 »

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript Operators
JavaScript Operators are symbols used to perform specific mathematical, comparison, assignment, and logical computations on operands. They are fundamental elements in JavaScript programming, allowing developers to manipulate data and control program flow efficiently. Understanding the different types of operators and how they work is important for writing effective and optimized JavaScript code.
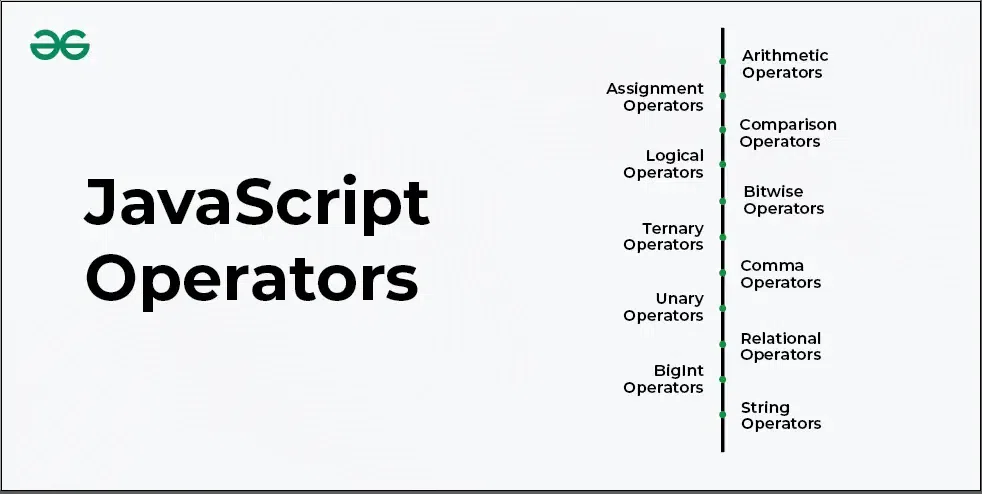
There are various operators supported by JavaScript.
Table of Content
JavaScript Arithmetic Operators
Javascript assignment operators, javascript comparison operators, javascript logical operators, javascript bitwise operators, javascript ternary operators, javascript comma operators, javascript unary operators, javascript relational operators, javascript bigint operators, javascript string operators.
JavaScript Arithmetic Operators perform arithmetic operations: addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
The assignment operation evaluates the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables
Comparison operators are mainly used to perform the logical operations that determine the equality or difference between the values.
JavaScript Logical Operators perform logical operations: AND (&&), OR (||), and NOT (!), evaluating expressions and returning boolean values.
The bitwise operator in JavaScript is used to convert the number to a 32-bit binary number and perform the bitwise operation. The number is converted back to the 64-bit number after the result.
The ternary operator has three operands. It is the simplified operator of if/else.
Comma Operator (,) mainly evaluates its operands from left to right sequentially and returns the value of the rightmost operand.
A unary operation is an operation with only one operand.
JavaScript Relational operators are used to compare its operands and determine the relationship between them. They return a Boolean value (true or false) based on the comparison result.
JavaScript BigInt operators support arithmetic operations on BigInt data type, including addition, subtraction, multiplication, division, and exponentiation. Most operators that can be used between numbers can be used between BigInt values as well.
JavaScript String Operators include concatenation (+) and concatenation assignment (+=), used to join strings or combine strings with other data types.
JavaScript operators are essential tools that empower developers to perform a wide array of operations on data, from simple arithmetic to complex logical decisions. By mastering these operators, you can write more efficient, readable, and maintainable code. Whether you’re performing calculations, making comparisons, assigning values, or manipulating data, understanding how to use JavaScript operators effectively is a fundamental skill for any JavaScript developer. As you continue to build your programming expertise, the versatility and power of operators will become increasingly apparent, enabling you to create more dynamic and robust applications.
JavaScript Operators – FAQs
What are javascript operators.
JavaScript operators are special symbols used to perform operations on operands, which can be values or variables. They allow you to manipulate data and perform computations.
What are the different types of JavaScript operators?
The main types of JavaScript operators include: Arithmetic Operators Assignment Operators Comparison Operators Logical Operators Bitwise Operators String Operators Conditional (Ternary) Operator Type Operators Comma Operator Unary Operators
Can you explain logical operators in JavaScript?
Logical operators are used to combine multiple Boolean expressions: && (Logical AND) || (Logical OR) ! (Logical NOT)
How does the ternary operator work in JavaScript?
The ternary operator is a shorthand for the if-else statement. It takes three operands: condition ? expressionIfTrue : expressionIfFalse;
What does the typeof operator do?
The typeof operator returns the type of a variable as a string. For example, typeof 42 returns “number”.
What is the purpose of the instanceof operator?
The instanceof operator checks if an object is an instance of a specific class or constructor. For example, let date = new Date(); date instanceof Date returns true.
We have a list of JavaScript Operators Reference where you can know more about these operators.
Similar Reads
- Web Technologies
- javascript-operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
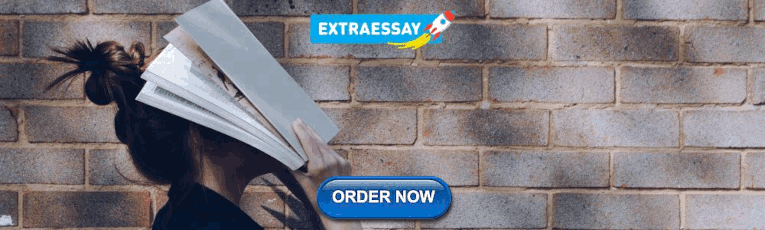
IMAGES
VIDEO