Optional chaining '?.'
The optional chaining ?. is a safe way to access nested object properties, even if an intermediate property doesn’t exist.
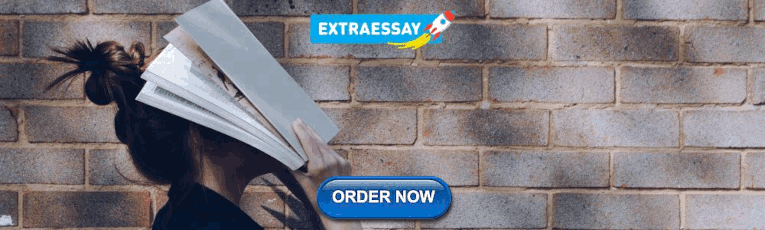
The “non-existing property” problem
If you’ve just started to read the tutorial and learn JavaScript, maybe the problem hasn’t touched you yet, but it’s quite common.
As an example, let’s say we have user objects that hold the information about our users.
Most of our users have addresses in user.address property, with the street user.address.street , but some did not provide them.
In such case, when we attempt to get user.address.street , and the user happens to be without an address, we get an error:
That’s the expected result. JavaScript works like this. As user.address is undefined , an attempt to get user.address.street fails with an error.
In many practical cases we’d prefer to get undefined instead of an error here (meaning “no street”).
…and another example. In Web development, we can get an object that corresponds to a web page element using a special method call, such as document.querySelector('.elem') , and it returns null when there’s no such element.
Once again, if the element doesn’t exist, we’ll get an error accessing .innerHTML property of null . And in some cases, when the absence of the element is normal, we’d like to avoid the error and just accept html = null as the result.
How can we do this?
The obvious solution would be to check the value using if or the conditional operator ? , before accessing its property, like this:
It works, there’s no error… But it’s quite inelegant. As you can see, the "user.address" appears twice in the code.
Here’s how the same would look for document.querySelector :
We can see that the element search document.querySelector('.elem') is actually called twice here. Not good.
For more deeply nested properties, it becomes even uglier, as more repetitions are required.
E.g. let’s get user.address.street.name in a similar fashion.
That’s just awful, one may even have problems understanding such code.
There’s a little better way to write it, using the && operator:
AND’ing the whole path to the property ensures that all components exist (if not, the evaluation stops), but also isn’t ideal.
As you can see, property names are still duplicated in the code. E.g. in the code above, user.address appears three times.
That’s why the optional chaining ?. was added to the language. To solve this problem once and for all!
Optional chaining
The optional chaining ?. stops the evaluation if the value before ?. is undefined or null and returns undefined .
Further in this article, for brevity, we’ll be saying that something “exists” if it’s not null and not undefined .
In other words, value?.prop :
- works as value.prop , if value exists,
- otherwise (when value is undefined/null ) it returns undefined .
Here’s the safe way to access user.address.street using ?. :
The code is short and clean, there’s no duplication at all.
Here’s an example with document.querySelector :
Reading the address with user?.address works even if user object doesn’t exist:
Please note: the ?. syntax makes optional the value before it, but not any further.
E.g. in user?.address.street.name the ?. allows user to safely be null/undefined (and returns undefined in that case), but that’s only for user . Further properties are accessed in a regular way. If we want some of them to be optional, then we’ll need to replace more . with ?. .
We should use ?. only where it’s ok that something doesn’t exist.
For example, if according to our code logic user object must exist, but address is optional, then we should write user.address?.street , but not user?.address?.street .
Then, if user happens to be undefined, we’ll see a programming error about it and fix it. Otherwise, if we overuse ?. , coding errors can be silenced where not appropriate, and become more difficult to debug.
If there’s no variable user at all, then user?.anything triggers an error:
The variable must be declared (e.g. let/const/var user or as a function parameter). The optional chaining works only for declared variables.
Short-circuiting
As it was said before, the ?. immediately stops (“short-circuits”) the evaluation if the left part doesn’t exist.
So, if there are any further function calls or operations to the right of ?. , they won’t be made.
For instance:
Other variants: ?.(), ?.[]
The optional chaining ?. is not an operator, but a special syntax construct, that also works with functions and square brackets.
For example, ?.() is used to call a function that may not exist.
In the code below, some of our users have admin method, and some don’t:
Here, in both lines we first use the dot ( userAdmin.admin ) to get admin property, because we assume that the user object exists, so it’s safe read from it.
Then ?.() checks the left part: if the admin function exists, then it runs (that’s so for userAdmin ). Otherwise (for userGuest ) the evaluation stops without errors.
The ?.[] syntax also works, if we’d like to use brackets [] to access properties instead of dot . . Similar to previous cases, it allows to safely read a property from an object that may not exist.
Also we can use ?. with delete :
The optional chaining ?. has no use on the left side of an assignment.
For example:
The optional chaining ?. syntax has three forms:
- obj?.prop – returns obj.prop if obj exists, otherwise undefined .
- obj?.[prop] – returns obj[prop] if obj exists, otherwise undefined .
- obj.method?.() – calls obj.method() if obj.method exists, otherwise returns undefined .
As we can see, all of them are straightforward and simple to use. The ?. checks the left part for null/undefined and allows the evaluation to proceed if it’s not so.
A chain of ?. allows to safely access nested properties.
Still, we should apply ?. carefully, only where it’s acceptable, according to our code logic, that the left part doesn’t exist. So that it won’t hide programming errors from us, if they occur.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Home » JavaScript Tutorial » JavaScript Logical Assignment Operators
JavaScript Logical Assignment Operators
Summary : in this tutorial, you’ll learn about JavaScript logical assignment operators, including the logical OR assignment operator ( ||= ), the logical AND assignment operator ( &&= ), and the nullish assignment operator ( ??= ).
ES2021 introduces three logical assignment operators including:
- Logical OR assignment operator ( ||= )
- Logical AND assignment operator ( &&= )
- Nullish coalescing assignment operator ( ??= )
The following table shows the equivalent of the logical assignments operator:
The Logical OR assignment operator
The logical OR assignment operator ( ||= ) accepts two operands and assigns the right operand to the left operand if the left operand is falsy:
In this syntax, the ||= operator only assigns y to x if x is falsy. For example:
In this example, the title variable is undefined , therefore, it’s falsy. Since the title is falsy, the operator ||= assigns the 'untitled' to the title . The output shows the untitled as expected.
See another example:
In this example, the title is 'JavaScript Awesome' so it is truthy. Therefore, the logical OR assignment operator ( ||= ) doesn’t assign the string 'untitled' to the title variable.
The logical OR assignment operator:
is equivalent to the following statement that uses the logical OR operator :
Like the logical OR operator, the logical OR assignment also short-circuits. It means that the logical OR assignment operator only performs an assignment when the x is falsy.
The following example uses the logical assignment operator to display a default message if the search result element is empty:
The Logical AND assignment operator
The logical AND assignment operator only assigns y to x if x is truthy:
The logical AND assignment operator also short-circuits. It means that
is equivalent to:
The following example uses the logical AND assignment operator to change the last name of a person object if the last name is truthy:
The nullish coalescing assignment operator
The nullish coalescing assignment operator only assigns y to x if x is null or undefined :
It’s equivalent to the following statement that uses the nullish coalescing operator :
The following example uses the nullish coalescing assignment operator to add a missing property to an object:
In this example, the user.nickname is undefined , therefore, it’s nullish. The nullish coalescing assignment operator assigns the string 'anonymous' to the user.nickname property.
The following table illustrates how the logical assignment operators work:
- The logical OR assignment ( x ||= y ) operator only assigns y to x if x is falsy.
- The logical AND assignment ( x &&= y ) operator only assigns y to x if x is truthy.
- The nullish coalescing assignment ( x ??= y ) operator only assigns y to x if x is nullish.
Optional Chaining in JavaScript – Explained with Examples
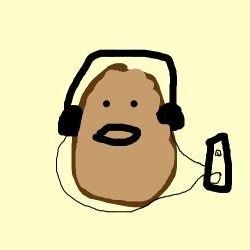
JavaScript development often involves navigating through nested objects, which can be cumbersome and error-prone, especially when dealing with null or undefined values. Enter optional chaining—a game-changer in modern JavaScript syntax.
In this article, we'll explore optional chaining through practical examples, demonstrating how it streamlines code and makes development more efficient.
You can get all the source code from here .
Table of Contents
- What is Optional Chaining ?
How to Access Nested Properties
How to call nested methods, dynamic property access, what is optional chaining ( . ).
Optional chaining, introduced in ECMAScript 2020, is a feature that simplifies the process of accessing properties and methods of nested objects or arrays when intermediate properties may be null or undefined .
The optional chaining operator ( ?. ) allows you to access properties or methods without the need for explicit null or undefined checks. If any intermediate property in the chain is null or undefined , the expression short-circuits, and the result is set to undefined .
In programming, "short-circuiting" refers to the behavior where the evaluation of an expression stops as soon as a null or undefined value is encountered along the chain of properties or methods being accessed. Instead of continuing to evaluate the expression, the result is immediately set to undefined , and any subsequent property or method access is skipped.
Now, let's dive into practical examples to see how optional chaining works in real-world scenarios.
In this example, we have a JavaScript object representing a user with nested address information.
Traditional Approach
The traditional way to access the city property within the user's address involves multiple checks to ensure that the properties exist and are not null or undefined .
This code checks if the user object exists, if it has an address property, and if the address property has a city property. If any of these conditions fail, the city variable is set to "Unknown".
Optional Chaining Approach
With optional chaining, accessing the nested city property becomes much simpler:
The ?. operator is used to access the city property of the user's address. If any intermediate property ( user or address ) is null or undefined , the expression short-circuits, and the result is immediately set to "Unknown".
In this example, we have a user object with a method getAddress() that returns the user's address.
The traditional way to call the getAddress() method and access the city property involves additional checks to ensure that the method exists and returns a non-null value.
This code first checks if the user object exists and if it has a getAddress method. Then it calls the method and checks if the returned address object exists before accessing its city property.
With optional chaining, calling the nested method and accessing the city property can be done in a more concise manner:
Here, the optional chaining operator is used to call the getAddress() method and access its city property. If getAddress method or any intermediate property in the chain is null or undefined , the expression short-circuits, and the result is immediately set to "Unknown".
In this example, we have an array of users, where each user may or may not have a profile.
The traditional way to access the profile name dynamically involves multiple checks for each user object in the array.
This code uses map() to iterate over each user object in the array and checks if it has a profile with a name property. If the name property exists, it is returned – otherwise, "Unknown" is returned.
With optional chaining, dynamic property access becomes more straightforward:
Here, the optional chaining operator is used to access the name property of each user's profile. If any intermediate property in the chain is null or undefined , the expression short-circuits, and "Unknown" is returned as the default value.
By applying optional chaining, you can streamline your code and make it more readable and maintainable.
As JavaScript continues to evolve, features like optional chaining play a crucial role in enhancing developer productivity and code quality.
Read more posts .
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Currently Reading :
Currently reading:
How can I declare optional function parameters in JavaScript?
How to declare optional function parameters in javascript.
Pooja Hariharan
Software Developer
Published on Thu Mar 10 2022
Declaring optional function parameters in JavaScript enhances flexibility and usability. There are several methods to achieve this. One common approach is to assign a default value to the parameter. This ensures that if the parameter is not provided, the function will still execute using the default value. Another method involves checking the arguments . length property within the function to determine if a parameter has been passed or not. Additionally, the logical OR operator ( || ) can be used to set default values for parameters if they are not explicitly provided. These techniques offer different ways to handle optional parameters in JavaScript functions, catering to various programming needs and styles.
Declaring optional function parameters in JavaScript provides flexibility and adaptability to your code. There are various methods to achieve this, each with its own advantages and use cases.
Using the default value to the parameter
One method to declare optional parameters is by assigning a default value to the parameter within the function signature. This ensures that if the parameter is not provided when calling the function, it will default to a predetermined value.
Using the arguments.length Property
Another approach involves checking the arguments . length property within the function. This property returns the number of arguments passed to the function, allowing you to determine whether a parameter has been provided or not.
Using the logical OR operator (‘||’)
The logical OR operator ( || ) can also be utilized to declare optional parameters. By using this operator along with the parameter and a default value, you can set the parameter to the provided value or to a default value if none is provided.
How to Use Optional Function Parameters in JavaScript ES6
Using optional function parameters in JavaScript ES6 provides developers with powerful tools to enhance code flexibility and readability. ES6 introduced several features that make working with optional parameters more convenient and concise.
One of the most commonly used methods is utilizing default parameter values. By assigning a default value to a parameter in the function signature, you can ensure that the parameter is optional and falls back to the default value if not provided.
Another approach involves leveraging destructuring assignment in function parameters. With destructuring, you can specify default values directly within the parameter list, allowing for more concise code.
These techniques, among others available in ES6, empower developers to handle optional parameters efficiently and effectively in their JavaScript functions.
Passing undefined vs. other falsy values
When working with optional function parameters in JavaScript, it's important to understand the distinction between passing undefined and other falsy values. While both undefined , null , 0 , false , NaN , and an empty string ( '' ) are falsy values in JavaScript, they behave differently when used as arguments for optional parameters.
Passing undefined explicitly indicates that the parameter was not provided when calling the function. In contrast, passing other falsy values might lead to unexpected behavior, as these values can be valid inputs for the function.
Therefore, when designing functions with optional parameters, it's crucial to consider how different falsy values may affect the behavior of the function and handle them accordingly to ensure desired functionality.
How Optional Parameters work?
Optional parameters in JavaScript provide flexibility in function declarations by allowing certain parameters to be omitted when calling the function. When a parameter is marked as optional, it means that it may or may not be provided when invoking the function.
If an optional parameter is not provided, its value defaults to undefined . Developers can utilize default parameter values or other techniques to handle scenarios where optional parameters are not passed. Optional parameters enhance the versatility of functions and simplify their usage in various contexts.
About the author
Pooja Hariharan is an experienced data scientist with a strong focus on predictive analytics and machine learning. She excels in turning data insights into strategic solutions for business improvements.
Related Blogs
Fill an array using JavaScript
Vinayaka Tadepalli
JavaScript String Length property explained
Ancil Eric D'Silva
JavaScript appendchild(): What it is and when to use it

Varun Omprakash
How to Get Last Element in an Array in JavaScript?
How to disable or enable buttons using javascript and jquery
Various methods for a Javascript new line
Browse Flexiple's talent pool
Explore our network of top tech talent. Find the perfect match for your project.
- Programmers
- React Native
- Ruby on Rails
Mastering JavaScript: optional chaining and nullish coalescing
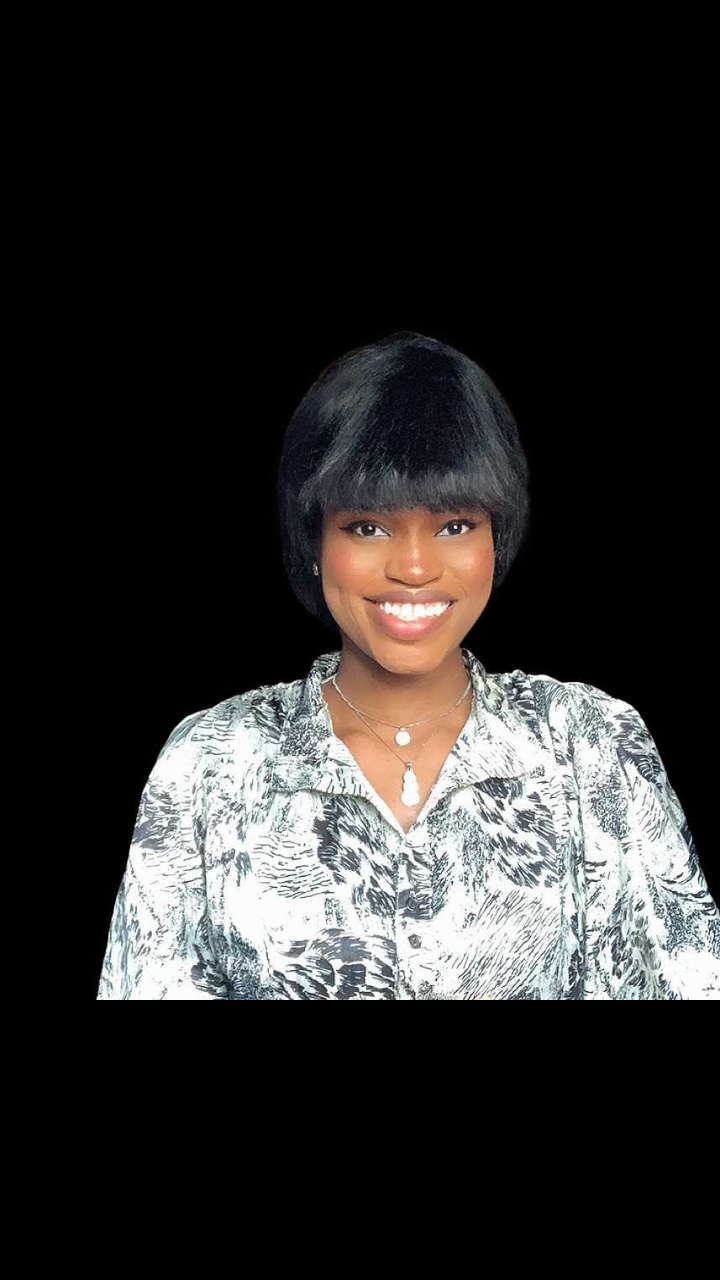
Mar 13, 2023 · 8 min read
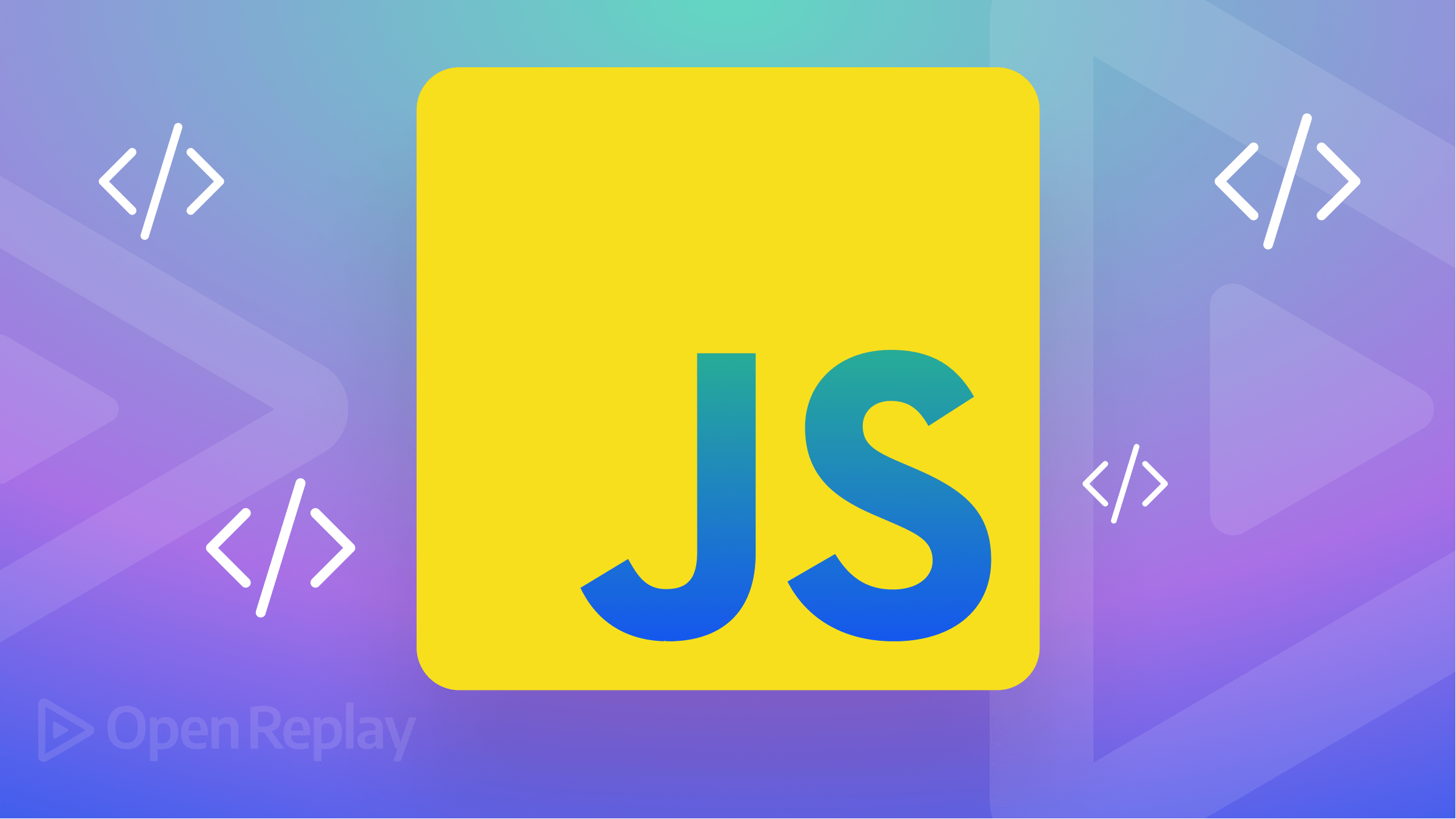
JavaScript is a popular programming language widely used in web development, and it empowers developers to build complex and interactive applications. As with any language, there are always new techniques and features that developers can learn and master to improve their skills and productivity.
Among these advanced JavaScript techniques are Optional Chaining and Nullish Coalescing . These features were introduced in ECMAScript 2020 (ES2020) and they provide a clear and concise way to access and manipulate values within objects and arrays .
They also help to minimize the amount of code required to handle null and undefined values, which can often lead to errors and bugs in JavaScript applications.
In this article, we will explore Optional Chaining and Nullish Coalescing in detail and learn how to use these techniques to write more efficient and reliable code in JavaScript. We will also look at some practical examples of how these features can be used in real-world scenarios.
Optional Chaining: what it is and how it works
Optional Chaining is a feature in JavaScript that allows developers to safely access the properties of an object without having to manually check if the object is null or undefined . This can be particularly useful when working with complex, nested objects.
The symbol used to denote Optional Chaining in JavaScript is the ?. (question mark followed by a period).
Prior to Optional Chaining, developers often had to use the && (logical AND) operator to check if an object was null or undefined before accessing its properties. For example, consider the following code:
This code checks if obj is defined and not null , and if it is, it checks if obj.a is defined and not null, and so on. If any of these checks fail, the value variable will not be assigned a value.
With Optional Chaining, we can rewrite the above code as follows:
This syntax works by first checking if obj is defined and not null . If it is, it accesses the a property of obj . If obj.a is defined and not null , it accesses the b property of obj.a . If obj.a.b is defined and not null , it accesses the c property of obj.a.b . If any of these checks fail, the whole expression returns undefined without throwing an error.
Optional Chaining can also be used to call functions. For example:
In this example, the person object has a car property, an object with a getInfo method that returns a string with the car’s make and model. We can use the Optional Chaining operator (?.) to access the car property and then call the getInfo method. If the person object or car property is undefined , the expression will return undefined , and no error will be thrown.
Optional Chaining is a handy feature that can help simplify and improve the readability of your code. It is supported in modern browsers and can also be used with transpilers such as Babel]( https://babeljs.io/ ) to provide support in older browsers.
Understanding nullish coalescing in JavaScript
JavaScript has two types of null values: null and undefined . null represents an intentional absence of a value, while undefined represents an uninitialized or missing value. Sometimes, you might want to use a default value if a variable is either null or undefined . This is where ‘Nullish Coalescing’ comes in.
Nullish Coalescing is a feature in JavaScript that allows you to check if a value is null or undefined ; if it is, use a default value instead.
The symbol used to denote Nullish Coalescing in JavaScript is the ?? (double question mark).
This operator checks if the value on the left side is null or undefined . If it is, it returns the value on the right side; otherwise, it returns the value on the left side. It is very useful when you want to check if a variable has a value and provide a default value. It also helps eliminate the need for multiple lines of code that would have been used to check for null or undefined values, thereby making it more readable and clean.
Let’s take a look at a little example:
In this example, the displayName variable is assigned the value of defaultName because the name variable is null . If the name variable had been undefined , the displayName variable would also have been assigned the value of defaultName .
One thing to note is that the Nullish Coalescing operator only considers null and undefined as falsy values. All other values, including empty strings and the number 0 , will be considered truthy. Here’s an example to illustrate this:
In this example, the displayCount variable is assigned the value of count , 0 , because the Nullish Coalescing operator( ?? ) only considers null and undefined as falsy values.
The displayCount variable would have been assigned the value of defaultCount if we had used the logical OR operator (||) , this is because when using this operator, 0 is always considered as a falsy value.
The Nullish Coalescing operator also can be helpful in situations where you want to use a default value if a variable is null or undefined , but you don’t want to use the default value if the variable is an empty string or the number 0 .
Here’s an example of how you can use the Nullish Coalescing operator with a function argument:
In this example, the getUserName function takes an object as an argument and returns the name property of the object if it exists. If the name property is null or undefined , the Optional Chaining operator( ?. ) will return null or undefined , and the Nullish Coalescing operator ( ?? ) will use the default value of ‘John Doe’.
The getUserName function is then called with four different arguments:
- user1 , which has a name property with a value of ‘Jane Doe’
- user2 , which is an empty object and therefore does not have a name property
- user3 , which is null
- user4 , which is undefined
For user1 , the function returns the value of the name property, ‘Jane Doe’. For user2 , the function returns the default value of ‘John Doe’ because the name property is not defined. For user3 and user4 , the function also returns the default value of ‘John Doe’ because the user argument is null or undefined .
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay suite for developers. It can be self-hosted in minutes, giving you complete control over your customer data
Happy debugging! Try using OpenReplay today.
Use Cases for Optional Chaining and Nullish Coalescing
Let us dive deeply into a few practical applications of Optional Chaining and Nullish Coalescing in the real world and see how they can solve problems and improve code efficiency across various industries.
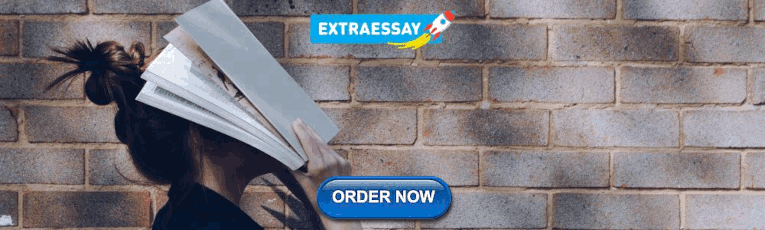
Use Case 1: Deal with missing values
Let’s say we have an e-commerce website that displays a user’s order history.
The feature would allow users to view their past orders, including information about the products they purchased, the date of purchase, and the order total.
The data for the orders are stored in a JavaScript object that looks something like this:
To display the order history, we can loop through the orders array and use optional chaining to access the properties of each order. For example, to display the name of the product and its quantity, we can use the following code:
If the item object does not have a product property, item.product?.name will return undefined , and the code will not throw an error.
Also, to display the shipping address of an order, we can use the following code:
If the order object does not have a shipping property, order.shipping?.address?.street will return undefined and ?? 'N/A' will assign the value N/A to the shippingAddress variable, thus preventing the code from throwing an error.
This way, we can use Optional Chaining and Nullish Coalescing to provide default values if a property is null or undefined .
Use case 2: Access deeply nested object properties
Suppose you have an object representing a customer record with nested properties for the customer’s name, address, and phone number. The object might look like this:
If you want to access the customer’s phone number, you can simply use dot notation:
But if you want to access a nested property that might be null or undefined , you can use Optional Chaining to safely access the property without causing an error:
Without Optional Chaining, you would have to check whether the address property is truthy before attempting to access the zip property:
Use case 3: Provide default values
Another example of using Optional Chaining and Nullish Coalescing in a JavaScript application could be a social media platform feature allowing users to view and edit their profile information.
The data for the user’s profile is stored in a JavaScript object that looks something like this:
To display the user’s social media links on their profile, we can use Optional Chaining to access the properties of the social object. For example, to display the user’s Facebook and Twitter links, we can use the following code:
Here, if the user object does not have a social property or if it doesn’t have a Facebook or Twitter property, user.social?.facebook or user.social?.twitter will return undefined and ?? "Add Facebook link" or ?? "Add Twitter link" will assign the default value to the variable, thus preventing the code from throwing an error.
To allow users to edit their profile information, we can use Nullish Coalescing to provide default values when updating the user’s preferences. For example, to update the user’s theme and notifications preferences, we can use the following code:
Here, if the theme or notifications values passed to the function are null or undefined , ?? user.preferences.theme or ?? user.preferences.notifications will keep the previous value of the theme or notifications and prevent the code from assigning null or undefined to the user’s preferences.
In this way, we can use Optional Chaining to safely access properties of nested objects and Nullish Coalescing to provide default values when updating data in the application.
Tips for effective use of optional chaining and nullish coalescing
Optional Chaining and Nullish Coalescing can be very useful when working with complex nested objects and data structures. Still, they also have their own quirks and edge cases of which we should be aware before using them. Let’s take a look at some:
- Be aware that the Optional Chaining operator (?.) only works with objects and will not work with primitive values such as numbers , strings , or booleans . If you try to use it with a primitive value, you will get a TypeError . Here is an example:
In the above example, using the Optional Chaining operator to access the name property of the person object works as expected, but trying to use it to access the age property of a string variable throws a TypeError .
- You can also use optional chaining in combination with the destructuring assignment to extract multiple properties from an object in a concise and readable way. For example, instead of writing
You could use the following:
- When using the Optional Chaining operator on a function call, be aware that if the function returns null or undefined , the entire expression will also evaluate to null or undefined . So it’s important to check the return value of the function.
In the first example, the function getAge returns the value of user.age , so the Optional Chaining operator works as expected. But in the second example, the function getAddress returns null , so the Optional Chaining operator also evaluates to null .
- Understand that when using the Nullish Coalescing operator (??) with falsy values. The operator only returns the default value when the variable is null or undefined , not when it’s “falsy” (false, 0, "" , etc.). In such cases, you should use a Conditional Ternary Operator ? : instead. For example:
In this example, the age is falsy but not null or undefined , so the ?? operator will not work and will return the value of user.age . But in the case of user.address , either null or undefined , the ?? operator will return the default value Not specified .
- When using the Nullish Coalescing operator with objects, be careful when the default value is also an object because JavaScript objects are compared by reference, not by value.
In the first example, the default value is assigned to user.address , but in the second example, the default value is not assigned because it’s the same object as the one in user.address , so the ?? operator returns the same object.
- Use Nullish Coalescing when you want to provide a default value for a variable if it is null or undefined . This allows you to avoid having to use the ternary operator ? : or a default parameter in a function. For example:
- Always be mindful of the order of precedence of the operators. In javascript, the ?. operator has higher precedence than the ?? operator. So let name = person?.name ?? 'John Doe' will check for person.name first, and if it’s null or undefined , it will check for the default value.
So that your code behaves as expected and avoids any potential errors, It’s very important to be mindful of these when using optional chaining and nullish coalescing in JavaScript because they can lead to unexpected results if not used correctly.
In conclusion, mastering advanced JavaScript techniques such as Optional Chaining and Nullish Coalescing can greatly improve the clarity and simplicity of your code. Optional Chaining allows for concise handling of potentially null or undefined values, while nullish coalescing allows for a default value to be assigned in the case of null or undefined . These techniques have various use cases and can be effectively utilized with a bit of practice and careful consideration. Using Optional Chaining and Nullish Coalescing, developers can write more efficient, clean, and maintainable code.
Also, as these are both relatively new features in JavaScript, they may not be fully supported in all browsers. It’s always a good idea to check the current browser support for new features before using them in production code. You can use a tool like caniuse.com or check the browser’s developer documentation for more information.
I hope this article brought clear light to these topics, and as always, Happy Coding.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs and track user frustrations. Get complete visibility into your frontend with OpenReplay, the most advanced open-source session replay tool for developers.
Check our GitHub Repo
More articles from OpenReplay Blog
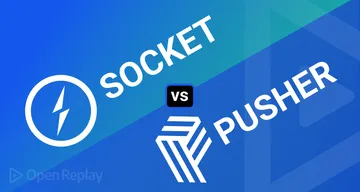
Jan 19, 2023, 11 min read
Socket.io vs. Pusher -- A Comparison
{post.frontmatter.excerpt}
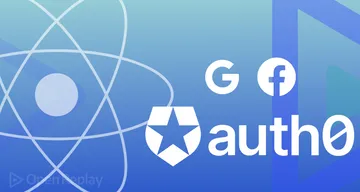
Jan 9, 2023, 8 min read
Social Media Authentication in React Native using Auth0
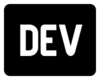
DEV Community

Posted on Feb 5, 2023 • Updated on Mar 28, 2023
JavaScript's Optional Chaining (?.) Operator
JavaScript is one of the most popular and widely used programming languages for web development. With its vast array of features and capabilities, JavaScript developers are continuously pushing the limits of what's possible on the web.
In this article, we'll be exploring an exciting feature in JavaScript known as the Optional Chaining Operator (?.). This operator has been introduced in ECMAScript 2020 and has since become a staple in modern JavaScript development. The Optional Chaining Operator allows developers to access properties of an object without worrying about TypeError, making the code more concise and less prone to bugs. We'll learn how you can leverage its power to simplify your code and improve the overall readability of your applications. So buckle up and get ready to unleash the power of JavaScript's Optional Chaining (?.) Operator!
Optional chaining (?.)
The Optional Chaining ?. operator is a powerful tool for accessing object properties or calling functions in JavaScript. Unlike traditional methods, which can result in a TypeError when the object is undefined or null , the Optional Chaining operator short circuits and simply returns undefined . This makes the code more concise and reduces the risk of encountering bugs, making it an essential tool for modern JavaScript development.
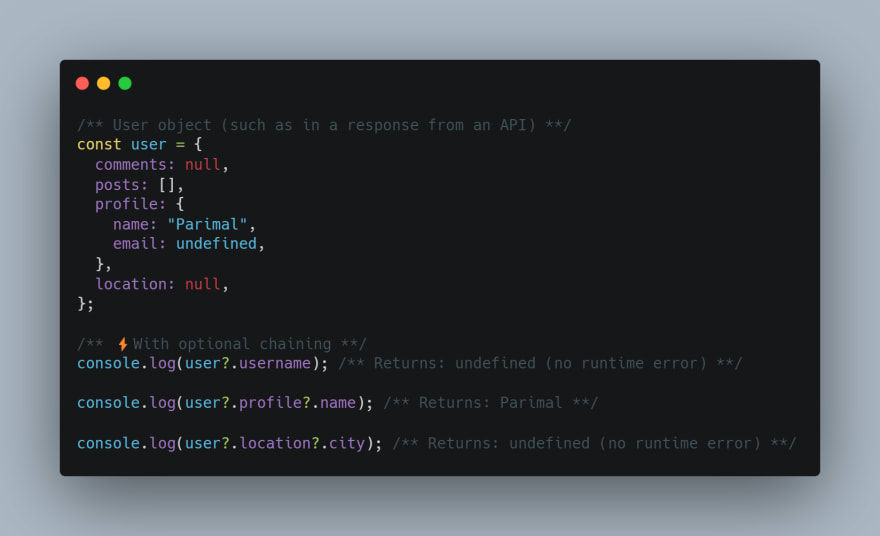
The syntax is straightforward and easy to understand. The operator is placed after the object you wish to access and before the property, array expression, or function call you want to make.
Here's how it works:
obj.val?.prop - Accesses the prop property of the obj.val object, only if obj.val exists and is not null or undefined.
obj.val?.[expr] - Accesses a property or an array element of obj.val using an expression expr, only if obj.val exists and is not null or undefined .
obj.func?.(args) - Calls the function obj.func with the given arguments args , only if obj.func exists and is not null or undefined .
Optional chaining ?. is used to access object properties and methods in a safe way , by handling the possibility of encountering null or undefined values. In React.js or Next.js, it can be used to access properties in state or props objects and to call methods on components, without risking runtime errors from trying to access a property or method on a null or undefined value.
Let's say, instead of writing:
you can write:
This can simplify your code and make it more concise. Useful in cases where you are not sure if the object exists or not.
It's important to note that the Optional Chaining operator cannot be used on a non-declared root object. Attempting to do so will result in a ReferenceError being thrown, indicating that the root object is not defined. However, the operator can be used on a root object that has a value of undefined .

The "non-existing property" problem
If you've just started to read the tutorial and learn JavaScript, maybe the problem hasn't touched you yet, but it's quite common.
As an example, let's say we have user objects that hold the information about our users.
Most of our users have addresses in user.address property, with the street user.address.street , but some did not provide them.
In such case, when we attempt to get user.address.street , and the user happens to be without an address, we get an runtime error:

Function Calls with Optional Chaining
The Optional Chaining operator is not just useful for accessing object properties, but it can also be applied to function calls. This makes it an ideal tool for handling scenarios in which the method you are trying to call may not exist. This is especially useful when working with APIs that have varying levels of support, or when dealing with features that may not be available on the user's device.

In this case, if the customMethod is not found on the someInterface object, the expression will return undefined instead of throwing an error. This allows your code to gracefully handle such scenarios and avoid the risk of unintended exceptions. With the Optional Chaining operator, you can write cleaner and more robust code that is better equipped to handle the complexities of real-world development.
Optional chaining with expressions
The Optional Chaining operator can also be combined with bracket notation to access properties with dynamic names. This is done by passing an expression as the property name, inside square brackets [] .
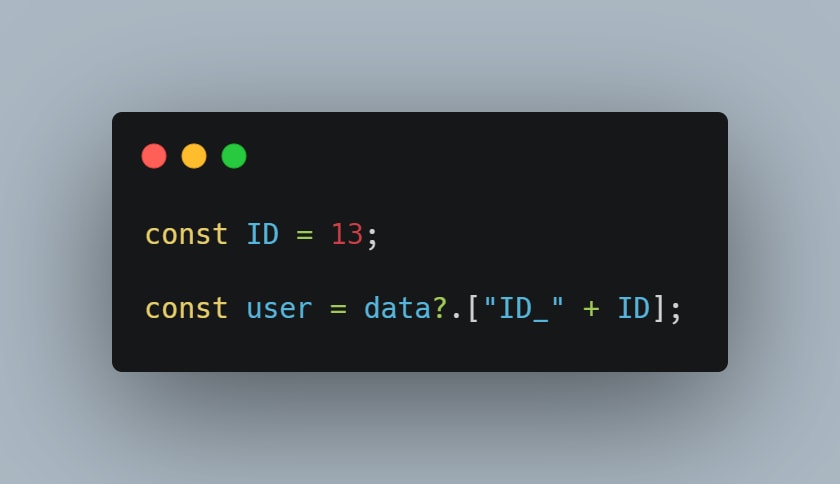
Optional chaining not valid on the left-hand side of an assignment
It is not possible to use the Optional Chaining operator for assigning values to object properties. Attempting to do so will result in a SyntaxError .
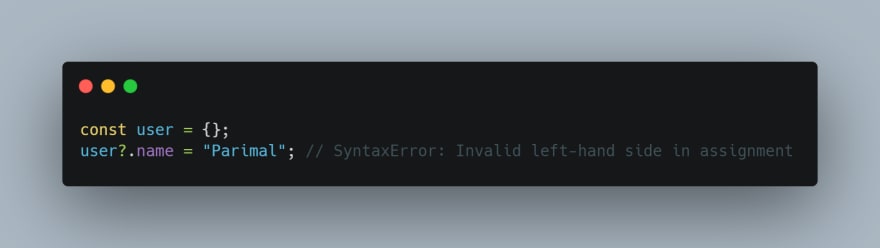
What are the downsides of optional chaining?
Optional chaining is easy to misuse and can potentially confuse future readers of your code in certain scenarios. This operator makes it easier for devs to express chained nullable references in a much concise manner.
Don't overuse the optional chaining
We should use ?. only where it's ok that something doesn't exist.
Let's say, if according to our code logic user object must exist, but address is optional, then we should write user.address?.street , but not user?.address?.street .
Then, if user happens to be undefined , we'll see a programming error about it and fix it. Otherwise, if we overuse ?. , coding errors can be silenced where not appropriate, and become more difficult to debug.
🍀Conclusion
The Optional Chaining (?.) operator is a powerful and elegant feature in JavaScript that can simplify our code and prevent runtime errors. By allowing us to safely access properties and call methods without having to check for undefined values, it allows us to write more concise and readable code. It's important to understand when and how to use this operator effectively, as overusing it can hide potential errors and make debugging more difficult. When used strategically, the Optional Chaining operator can be a valuable tool in our JavaScript toolkit.
Please consider following and supporting us by subscribing to our channel. Your support is greatly appreciated and will help us continue creating content for you to enjoy. Thank you in advance for your support!
YouTube Discord GitHub
Top comments (2)
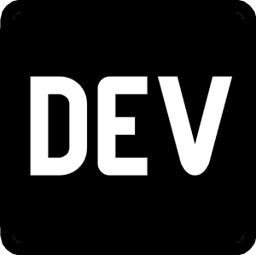
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Dec 11, 2022
I've been seeing this syntax and wondering about its purpose. Thanks for posting!

- Email [email protected]
- Work Full Stack Developer
- Joined Dec 23, 2022
You're welcome sir, I'm glad I could help clarify the purpose of the optional chaining.. if you have any further questions or need more information, please feel free to ask..
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
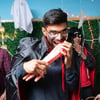
Whitelisting URL Paths Using Regular Expressions
Afraz Khan - Apr 28

Gustavo H. J. - Apr 28
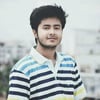
Why NVM is Tremendously Helpful for Web Developers
Mohammad Faisal - May 11
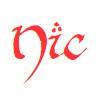
Earth Day Celebration Landing Page
Nic - Apr 27
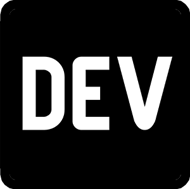
We're a place where coders share, stay up-to-date and grow their careers.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript | Optional chaining
- JavaScript Promise Chaining
- Method Chaining in JavaScript
- JavaScript Symbol hasInstance Property
- Chaining of Array Methods in JavaScript
- Javascript Short Circuiting Operators
- Shadowing Properties in JavaScript
- AND(&&) Logical Operator in JavaScript
- What is () => in JavaScript ?
- JavaScript Nullish Coalescing(??) Operator
- Understanding the Prototype Chain in JavaScript
- Variadic Functions in JavaScript
- What are Ternary Operator in JavaScript?
- JavaScript Unary Operators
- JavaScript Check the existence of variable
- JavaScript isNaN() Function
- JavaScript Set has() Method
- OR(||) Logical Operator in JavaScript
- Conditional Statements in JavaScript
- Optional Chaining in Swift
The optional chaining ‘?.’ is an error-proof way to access nested object properties, even if an intermediate property doesn’t exist. It was recently introduced by ECMA International, Technical Committee 39 – ECMAScript which was authored by Claude Pache, Gabriel Isenberg, Daniel Rosenwasser, Dustin Savery. It works similar to Chaining ‘.’ except that it does not report the error, instead it returns a value which is undefined. It also works with function call when we try to make a call to a method which may not exist.
When we want to check a value of the property which is deep inside a tree-like structure, we often have to perform check whether intermediate nodes exist.
The Optional Chaining Operator allows a developer to handle many of those cases without repeating themselves and/or assigning intermediate results in temporary variables:
Syntax:
Note: If this code gives any error try to run it on online JavaScript editor.
Example: Optional Chaining with Object
Example: Optional Chaining with Function Call
Please Login to comment...
Similar reads.
- JavaScript-Misc
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Optional chaining
Introducing the optional chaining operator #, additional syntax forms: calls and dynamic properties #, properties of the optional chaining operator #, support for optional chaining #.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
The yield operator is used to pause and resume a generator function .
The value to yield from the generator function via the iterator protocol . If omitted, undefined is yielded.
Return value
Returns the optional value passed to the generator's next() method to resume its execution.
Note: This means next() is asymmetric: it always sends a value to the currently suspended yield , but returns the operand of the next yield . The argument passed to the first next() call cannot be retrieved because there's no currently suspended yield .
Description
The yield keyword pauses generator function execution and the value of the expression following the yield keyword is returned to the generator's caller. It can be thought of as a generator-based version of the return keyword.
yield can only be used directly within the generator function that contains it. It cannot be used within nested functions.
Calling a generator function constructs a Generator object. Each time the generator's next() method is called, the generator resumes execution, and runs until it reaches one of the following:
- A yield expression. In this case, the generator pauses, and the next() method return an iterator result object with two properties: value and done . The value property is the value of the expression after the yield operator, and done is false , indicating that the generator function has not fully completed.
- The end of the generator function. In this case, execution of the generator ends, and the next() method returns an iterator result object where the value is undefined and done is true .
- A return statement. In this case, execution of the generator ends, and the next() method returns an iterator result object where the value is the specified return value and done is true .
- A throw statement. In this case, execution of the generator halts entirely, and the next() method throws the specified exception.
Once paused on a yield expression, the generator's code execution remains paused until the generator's next() method is called again. If an optional value is passed to the generator's next() method, that value becomes the value returned by the generator's current yield operation. The first next() call does not have a corresponding suspended yield operation, so there's no way to get the argument passed to the first next() call.
If the generator's return() or throw() method is called, it acts as if a return or throw statement was executed at the paused yield expression. You can use try...catch...finally within the generator function body to handle these early completions. If the return() or throw() method is called but there's no suspended yield expression (because next() has not been called yet, or because the generator has already completed), then the early completions cannot be handled and always terminate the generator.
Using yield
The following code is the declaration of an example generator function.
Once a generator function is defined, it can be used by constructing an iterator as shown.
You can also send a value with next(value) into the generator. step evaluates as a return value of the yield expression — although the value passed to the generator's next() method the first time next() is called is ignored.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Iteration protocols
- function* expression
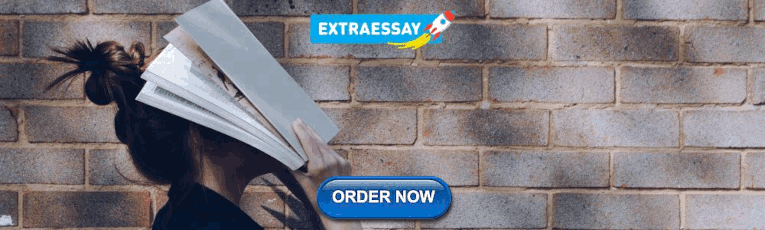
IMAGES
VIDEO
COMMENTS
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
In this article, I'm going to teach you how to use three advanced JavaScript operators: the Nullish Coalescing, Optional Chaining, and Destructuring Assignment operators. These three operators will help you write clearer and less error-prone code. The Nullish Coalescing Operator When you're inspecting JavaScript code, you may.
Summary. The optional chaining ?. syntax has three forms:. obj?.prop - returns obj.prop if obj exists, otherwise undefined.; obj?.[prop] - returns obj[prop] if obj exists, otherwise undefined. obj.method?.() - calls obj.method() if obj.method exists, otherwise returns undefined. As we can see, all of them are straightforward and simple to use. The ?. checks the left part for null ...
The logical OR assignment operator ( ||=) accepts two operands and assigns the right operand to the left operand if the left operand is falsy: x ||= y. In this syntax, the ||= operator only assigns y to x if x is falsy. For example: let title; title ||= 'untitled'; console.log (title);Code language:JavaScript(javascript) Output: untitled.
JavaScript development often involves navigating through nested objects, which can be cumbersome and error-prone, especially when dealing with null or undefined values. Enter optional chaining—a game-changer in modern JavaScript syntax. In this article, we'll explore optional chaining through practical examples, demonstrating how it streamlines code and makes development more
When working with optional function parameters in JavaScript, it's important to understand the distinction between passing undefined and other falsy values. While both undefined, null, 0, false, NaN, and an empty string ('') are falsy values in JavaScript, they behave differently when used as arguments for optional parameters.
Optional Chaining allows for concise handling of potentially null or undefined values, while nullish coalescing allows for a default value to be assigned in the case of null or undefined. These techniques have various use cases and can be effectively utilized with a bit of practice and careful consideration. Using Optional Chaining and Nullish ...
JavaScript is one of the most popular and widely used programming languages for web development. With its vast array of features and capabilities, JavaScript developers are continuously pushing the limits of what's possible on the web. In this article, we'll be exploring an exciting feature in JavaScript known as the Optional Chaining Operator
What is a good way of assigning a default value to an optional parameter? Background. I'm playing around with optional parameters in JavaScript, and the idea of assigning a default value if a parameter is not specified. My method in question accepts two parameters, the latter of which I deem to be optional, and if unspecified should default to ...
Using the Assignment operator ("=") In this approach the optional variable is assigned the default value in the declaration statement itself. Note: The optional parameters should always come at the end of the parameter list. Syntax: function myFunc(a, b = 0) {. // function body. } Example: In the following program the optional parameter is ...
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
You can use optional chaining when attempting to call a method which may not exist. This can be helpful, for example, when using an API in which a method might be unavailable, either due to the age of the implementation or because of a feature which isn't available on the user's device. Using optional chaining with function calls causes the ...
The nullish coalescing operator can be seen as a special case of the logical OR ( ||) operator. The latter returns the right-hand side operand if the left operand is any falsy value, not only null or undefined. In other words, if you use || to provide some default value to another variable foo, you may encounter unexpected behaviors if you ...
Last Updated : 10 May, 2021. The optional chaining '?.' is an error-proof way to access nested object properties, even if an intermediate property doesn't exist. It was recently introduced by ECMA International, Technical Committee 39 - ECMAScript which was authored by Claude Pache, Gabriel Isenberg, Daniel Rosenwasser, Dustin Savery.
8. I was looking myself into this and regretfully, as the other commenters already stated, an assignment via optional chaining appears to not be possible in typescript as of the time of writing and will indeed yield you the parser warning: The left-hand side of an assignment expression may not be an optional property access. When attempting ...
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.
The optional chaining operator has a few interesting properties: short-circuiting, stacking, and optional deletion. Let's walk through each of these with an example. Short-circuiting means not evaluating the rest of the expression if an optional chaining operator returns early: // `age` is incremented only if `db` and `user` are defined.
I have a TypeScript interface with some optional fields and a variable of that type: interface Foo { config?: { longFieldName?: string; } } declare let f: Foo; I'd like to put longFieldName in a variable of the same name. If config weren't optional, I'd use destructuring assignment to do this without repeating longFieldName. But it is, so I get ...
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
It was raised in the tc39 proposal that brought us optional chaining under the discussion should we include "optional property assignment" a?.b = c, but ultimately not supported. However, this is supported by CoffeeScript's existential operator which given the following example: el?.href = "b".
You can't assign to an optionally chained expression; they can't appear on the "left-hand side" of an assignment. This would require something like this to work: a?.foo = 1 How is this supposed to work when a is null? You'd effectively be producing the statement undefined = 1. In your case you're expecting this to work:
The yield keyword pauses generator function execution and the value of the expression following the yield keyword is returned to the generator's caller. It can be thought of as a generator-based version of the return keyword. yield can only be used directly within the generator function that contains it. It cannot be used within nested functions.