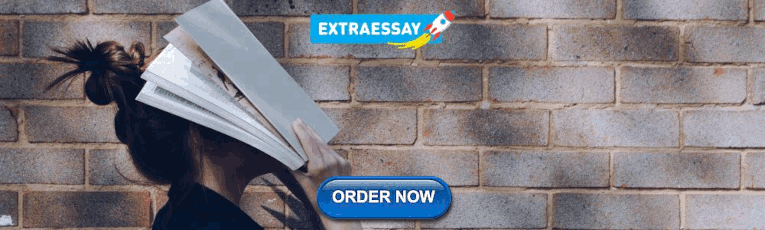
Make Random Student Groups
Make random student groups #.
This page demonstrates how to make a Python function called make_random_groups() that will take a list of student names and randomly create a desired number of groups from the list.
Import random module #
Make list of students #.
Here’s a list of student names. Yes, in this scenario, I’m teaching the NBA’s all-star basketball players.
Create make_random_groups() function #
Use make_random_groups() function #, make random student groups explained in more detail #, shuffle the order of the students #, extract a certain number of groups from the list of randomly shuffled students #.
all_groups = []
creates an empty list
range(number_of_groups)
creates a sequence of numbers from 0 to the desired number of groups
represents each number in that sequence
students[index::number_of_groups]
extracts a group of students from the list by selecting the student at whatever position in the list index represents, then jumping forward by number_of_groups spots to select a student at that position in the list, etc
all_groups.append(group)
adds each group to a master list
Let’s say we have 15 students and want 5 random groups. For each index aka number in range(5) — (0,1,2,3,4) — we make a group by selecting students[index::5] .
Group 1 students[0::5] We select the 0th student in the randomly shuffled list, then jump by 5 to select the 5th person in the list, and then jump by 5 to take the 10th person in the list.
Group 2 students[1::5] We select the 1st student in the randomly shuffled list, then jump by 5 to select the 6th person in the list, and then jump by 5 to take the 11th person in the list.
Group 3 students[2::5] We select the 2nd student in the randomly shuffled list, then jump by 5 to select the 7th person in the list, and then jump by 5 to take the 12th person in the list.
Group 4 students[3::5] We select the 3rd student in the randomly shuffled list, then jump by 5 to select the 8th person in the list, and then jump by 5 to take the 13th person in the list.
Group 5 students[4::5] We select the 4th student in the randomly shuffled list, then jump by 5 to select the 9th person in the list, and then jump by 5 to take the 14th person in the list.
Format and display each group #
- Python »
- 3.9.18 Documentation »
- The Python Standard Library »
- Numeric and Mathematical Modules »
- Theme Auto Light Dark |
random — Generate pseudo-random numbers ¶
Source code: Lib/random.py
This module implements pseudo-random number generators for various distributions.
For integers, there is uniform selection from a range. For sequences, there is uniform selection of a random element, a function to generate a random permutation of a list in-place, and a function for random sampling without replacement.
On the real line, there are functions to compute uniform, normal (Gaussian), lognormal, negative exponential, gamma, and beta distributions. For generating distributions of angles, the von Mises distribution is available.
Almost all module functions depend on the basic function random() , which generates a random float uniformly in the semi-open range [0.0, 1.0). Python uses the Mersenne Twister as the core generator. It produces 53-bit precision floats and has a period of 2**19937-1. The underlying implementation in C is both fast and threadsafe. The Mersenne Twister is one of the most extensively tested random number generators in existence. However, being completely deterministic, it is not suitable for all purposes, and is completely unsuitable for cryptographic purposes.
The functions supplied by this module are actually bound methods of a hidden instance of the random.Random class. You can instantiate your own instances of Random to get generators that don’t share state.
Class Random can also be subclassed if you want to use a different basic generator of your own devising: in that case, override the random() , seed() , getstate() , and setstate() methods. Optionally, a new generator can supply a getrandbits() method — this allows randrange() to produce selections over an arbitrarily large range.
The random module also provides the SystemRandom class which uses the system function os.urandom() to generate random numbers from sources provided by the operating system.
The pseudo-random generators of this module should not be used for security purposes. For security or cryptographic uses, see the secrets module.
M. Matsumoto and T. Nishimura, “Mersenne Twister: A 623-dimensionally equidistributed uniform pseudorandom number generator”, ACM Transactions on Modeling and Computer Simulation Vol. 8, No. 1, January pp.3–30 1998.
Complementary-Multiply-with-Carry recipe for a compatible alternative random number generator with a long period and comparatively simple update operations.
Bookkeeping functions ¶
Initialize the random number generator.
If a is omitted or None , the current system time is used. If randomness sources are provided by the operating system, they are used instead of the system time (see the os.urandom() function for details on availability).
If a is an int, it is used directly.
With version 2 (the default), a str , bytes , or bytearray object gets converted to an int and all of its bits are used.
With version 1 (provided for reproducing random sequences from older versions of Python), the algorithm for str and bytes generates a narrower range of seeds.
Changed in version 3.2: Moved to the version 2 scheme which uses all of the bits in a string seed.
Deprecated since version 3.9: In the future, the seed must be one of the following types: NoneType , int , float , str , bytes , or bytearray .
Return an object capturing the current internal state of the generator. This object can be passed to setstate() to restore the state.
state should have been obtained from a previous call to getstate() , and setstate() restores the internal state of the generator to what it was at the time getstate() was called.
Functions for bytes ¶
Generate n random bytes.
This method should not be used for generating security tokens. Use secrets.token_bytes() instead.
New in version 3.9.
Functions for integers ¶
Return a randomly selected element from range(start, stop, step) . This is equivalent to choice(range(start, stop, step)) , but doesn’t actually build a range object.
The positional argument pattern matches that of range() . Keyword arguments should not be used because the function may use them in unexpected ways.
Changed in version 3.2: randrange() is more sophisticated about producing equally distributed values. Formerly it used a style like int(random()*n) which could produce slightly uneven distributions.
Return a random integer N such that a <= N <= b . Alias for randrange(a, b+1) .
Returns a non-negative Python integer with k random bits. This method is supplied with the MersenneTwister generator and some other generators may also provide it as an optional part of the API. When available, getrandbits() enables randrange() to handle arbitrarily large ranges.
Changed in version 3.9: This method now accepts zero for k .
Functions for sequences ¶
Return a random element from the non-empty sequence seq . If seq is empty, raises IndexError .
Return a k sized list of elements chosen from the population with replacement. If the population is empty, raises IndexError .
If a weights sequence is specified, selections are made according to the relative weights. Alternatively, if a cum_weights sequence is given, the selections are made according to the cumulative weights (perhaps computed using itertools.accumulate() ). For example, the relative weights [10, 5, 30, 5] are equivalent to the cumulative weights [10, 15, 45, 50] . Internally, the relative weights are converted to cumulative weights before making selections, so supplying the cumulative weights saves work.
If neither weights nor cum_weights are specified, selections are made with equal probability. If a weights sequence is supplied, it must be the same length as the population sequence. It is a TypeError to specify both weights and cum_weights .
The weights or cum_weights can use any numeric type that interoperates with the float values returned by random() (that includes integers, floats, and fractions but excludes decimals). Behavior is undefined if any weight is negative. A ValueError is raised if all weights are zero.
For a given seed, the choices() function with equal weighting typically produces a different sequence than repeated calls to choice() . The algorithm used by choices() uses floating point arithmetic for internal consistency and speed. The algorithm used by choice() defaults to integer arithmetic with repeated selections to avoid small biases from round-off error.
New in version 3.6.
Changed in version 3.9: Raises a ValueError if all weights are zero.
Shuffle the sequence x in place.
The optional argument random is a 0-argument function returning a random float in [0.0, 1.0); by default, this is the function random() .
To shuffle an immutable sequence and return a new shuffled list, use sample(x, k=len(x)) instead.
Note that even for small len(x) , the total number of permutations of x can quickly grow larger than the period of most random number generators. This implies that most permutations of a long sequence can never be generated. For example, a sequence of length 2080 is the largest that can fit within the period of the Mersenne Twister random number generator.
Deprecated since version 3.9, will be removed in version 3.11: The optional parameter random .
Return a k length list of unique elements chosen from the population sequence or set. Used for random sampling without replacement.
Returns a new list containing elements from the population while leaving the original population unchanged. The resulting list is in selection order so that all sub-slices will also be valid random samples. This allows raffle winners (the sample) to be partitioned into grand prize and second place winners (the subslices).
Members of the population need not be hashable or unique. If the population contains repeats, then each occurrence is a possible selection in the sample.
Repeated elements can be specified one at a time or with the optional keyword-only counts parameter. For example, sample(['red', 'blue'], counts=[4, 2], k=5) is equivalent to sample(['red', 'red', 'red', 'red', 'blue', 'blue'], k=5) .
To choose a sample from a range of integers, use a range() object as an argument. This is especially fast and space efficient for sampling from a large population: sample(range(10000000), k=60) .
If the sample size is larger than the population size, a ValueError is raised.
Changed in version 3.9: Added the counts parameter.
Deprecated since version 3.9: In the future, the population must be a sequence. Instances of set are no longer supported. The set must first be converted to a list or tuple , preferably in a deterministic order so that the sample is reproducible.
Real-valued distributions ¶
The following functions generate specific real-valued distributions. Function parameters are named after the corresponding variables in the distribution’s equation, as used in common mathematical practice; most of these equations can be found in any statistics text.
Return the next random floating point number in the range [0.0, 1.0).
Return a random floating point number N such that a <= N <= b for a <= b and b <= N <= a for b < a .
The end-point value b may or may not be included in the range depending on floating-point rounding in the equation a + (b-a) * random() .
Return a random floating point number N such that low <= N <= high and with the specified mode between those bounds. The low and high bounds default to zero and one. The mode argument defaults to the midpoint between the bounds, giving a symmetric distribution.
Beta distribution. Conditions on the parameters are alpha > 0 and beta > 0 . Returned values range between 0 and 1.
Exponential distribution. lambd is 1.0 divided by the desired mean. It should be nonzero. (The parameter would be called “lambda”, but that is a reserved word in Python.) Returned values range from 0 to positive infinity if lambd is positive, and from negative infinity to 0 if lambd is negative.
Gamma distribution. ( Not the gamma function!) Conditions on the parameters are alpha > 0 and beta > 0 .
The probability distribution function is:
Gaussian distribution. mu is the mean, and sigma is the standard deviation. This is slightly faster than the normalvariate() function defined below.
Multithreading note: When two threads call this function simultaneously, it is possible that they will receive the same return value. This can be avoided in three ways. 1) Have each thread use a different instance of the random number generator. 2) Put locks around all calls. 3) Use the slower, but thread-safe normalvariate() function instead.
Log normal distribution. If you take the natural logarithm of this distribution, you’ll get a normal distribution with mean mu and standard deviation sigma . mu can have any value, and sigma must be greater than zero.
Normal distribution. mu is the mean, and sigma is the standard deviation.
mu is the mean angle, expressed in radians between 0 and 2* pi , and kappa is the concentration parameter, which must be greater than or equal to zero. If kappa is equal to zero, this distribution reduces to a uniform random angle over the range 0 to 2* pi .
Pareto distribution. alpha is the shape parameter.
Weibull distribution. alpha is the scale parameter and beta is the shape parameter.
Alternative Generator ¶
Class that implements the default pseudo-random number generator used by the random module.
Class that uses the os.urandom() function for generating random numbers from sources provided by the operating system. Not available on all systems. Does not rely on software state, and sequences are not reproducible. Accordingly, the seed() method has no effect and is ignored. The getstate() and setstate() methods raise NotImplementedError if called.
Notes on Reproducibility ¶
Sometimes it is useful to be able to reproduce the sequences given by a pseudo-random number generator. By re-using a seed value, the same sequence should be reproducible from run to run as long as multiple threads are not running.
Most of the random module’s algorithms and seeding functions are subject to change across Python versions, but two aspects are guaranteed not to change:
If a new seeding method is added, then a backward compatible seeder will be offered.
The generator’s random() method will continue to produce the same sequence when the compatible seeder is given the same seed.
Basic examples:
Simulations:
Example of statistical bootstrapping using resampling with replacement to estimate a confidence interval for the mean of a sample:
Example of a resampling permutation test to determine the statistical significance or p-value of an observed difference between the effects of a drug versus a placebo:
Simulation of arrival times and service deliveries for a multiserver queue:
Statistics for Hackers a video tutorial by Jake Vanderplas on statistical analysis using just a few fundamental concepts including simulation, sampling, shuffling, and cross-validation.
Economics Simulation a simulation of a marketplace by Peter Norvig that shows effective use of many of the tools and distributions provided by this module (gauss, uniform, sample, betavariate, choice, triangular, and randrange).
A Concrete Introduction to Probability (using Python) a tutorial by Peter Norvig covering the basics of probability theory, how to write simulations, and how to perform data analysis using Python.
The default random() returns multiples of 2⁻⁵³ in the range 0.0 ≤ x < 1.0 . All such numbers are evenly spaced and are exactly representable as Python floats. However, many other representable floats in that interval are not possible selections. For example, 0.05954861408025609 isn’t an integer multiple of 2⁻⁵³.
The following recipe takes a different approach. All floats in the interval are possible selections. The mantissa comes from a uniform distribution of integers in the range 2⁵² ≤ mantissa < 2⁵³ . The exponent comes from a geometric distribution where exponents smaller than -53 occur half as often as the next larger exponent.
All real valued distributions in the class will use the new method:
The recipe is conceptually equivalent to an algorithm that chooses from all the multiples of 2⁻¹⁰⁷⁴ in the range 0.0 ≤ x < 1.0 . All such numbers are evenly spaced, but most have to be rounded down to the nearest representable Python float. (The value 2⁻¹⁰⁷⁴ is the smallest positive unnormalized float and is equal to math.ulp(0.0) .)
Generating Pseudo-random Floating-Point Values a paper by Allen B. Downey describing ways to generate more fine-grained floats than normally generated by random() .
Table of Contents
- Bookkeeping functions
- Functions for bytes
- Functions for integers
- Functions for sequences
- Real-valued distributions
- Alternative Generator
- Notes on Reproducibility
Previous topic
fractions — Rational numbers
statistics — Mathematical statistics functions
- Report a Bug
- Show Source
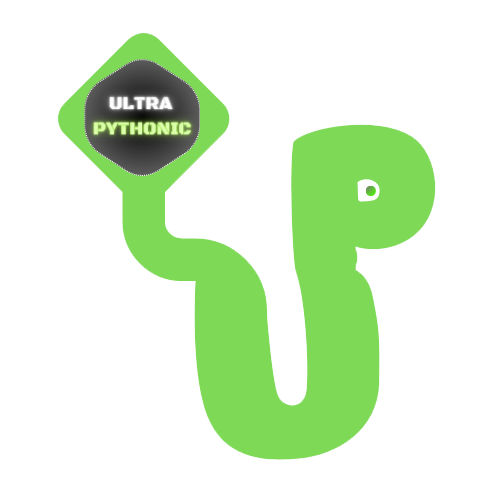
Buckle Up! Your Ultimate Python Random Module Tutorial Awaits
Hey there, awesome learners! Today, we’re diving into the exciting world of randomness in Python. Don’t worry; it’s not as random as it sounds! We’ll break it down step by step and have loads of fun while doing it.
What is randomness?
Randomness is like the spice of life for computers and programming. It’s all about introducing unpredictability and surprise into our code. Think of it like rolling a dice or shuffling a deck of cards – you never know what you’ll get, and that’s what makes things exciting!
Why is randomness important in programming?
Great question! Randomness adds a dose of realism and variety to our programs. It’s like giving your code a playful twist. We use randomness for games, simulations, and even cryptography! Plus, it keeps things fresh and prevents boring, repetitive results.
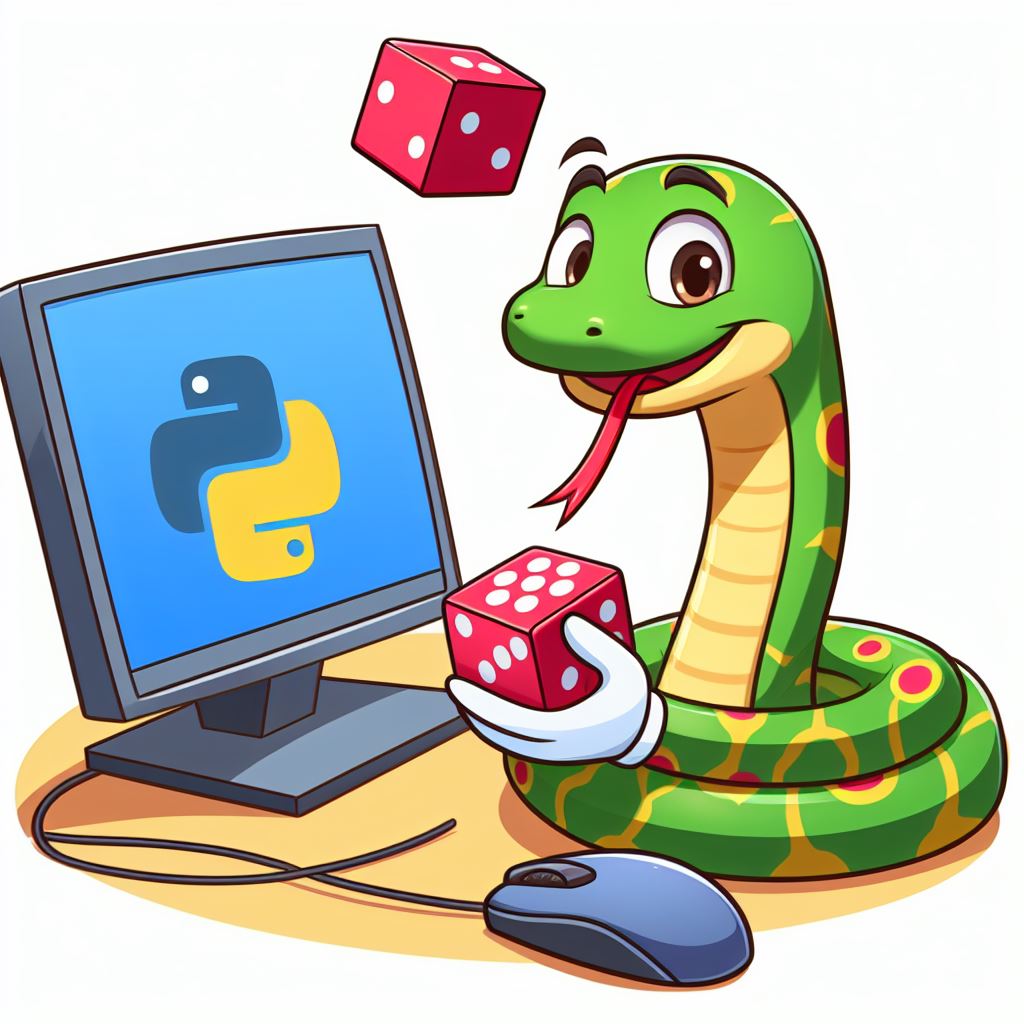
Python Random Module Introduction
Now, let’s meet our trusty sidekick for exploring randomness in Python – the Random module!
The Python Random module is like a magical toolbox that helps us work with randomness effortlessly. It’s a part of Python’s standard library, so you don’t need to install anything extra – it’s already there waiting for you to unleash its powers.
In the upcoming sections, we’ll get hands-on with this module, exploring how to generate random numbers, shuffle stuff, and even make decisions by the roll of a virtual dice. 🎲
Buckle up, because we’re about to embark on an exciting journey into the world of Python’s Random module! 🚀🐍
Next, we’ll dive into the nitty-gritty details of generating random numbers and having some fun with Python’s Random module.
Getting Started with the Random Module
Okay, so we’re about to unleash the magic of randomness in Python, but before we can do that, we need to make sure we have the Random module ready to roll. Here’s how you can import it:
That’s it! Now we’ve got all the randomness at our fingertips.
Generating random numbers using random()
Now that we have the Random module, let’s start by generating some random integers. We’ll use the random() function for this. Check it out:
When you run this code, you’ll get a random float between 0.0 and 1.0. Super cool, right? You can use this for all sorts of things, like creating random game levels or determining who goes first in a game.
Generating random floating-point numbers
But what if you need random floating-point numbers in a specific range? Python’s got you covered! You can use the uniform(a, b) function, where a is the minimum value and b is the maximum value. Here’s how you do it:
This code will give you a random float between 1.0 (inclusive) and 10.0 (exclusive).
And there you have it – the basics of getting started with the Python Random module! 🌟 Now you can add a dash of randomness to your programs, making them more exciting and unpredictable.
In the next section, we’ll explore more fun things you can do with the Random module, so stay tuned for even more randomness in action!
Generating Random Integers
Sometimes you need whole numbers, right? Python’s Random module has your back with the randint(a, b) function. It’s like having a magical number generator. Here’s how it works:
When you run this code, you’ll get a random integer between 1 and 10, including both 1 and 10. Pretty nifty for things like simulating dice rolls!
Specifying a range for random integers
But what if you want random integers within a specific range? No problem! You can use the randrange(start, stop, step) function. It’s like customizing your own randomness. Check it out:
In this example, you’ll get a random even integer between 10 (inclusive) and 21 (exclusive). You can change the step to control the spacing between numbers.
So there you have it, generating random integers like a pro! Python’s Random module makes it easy to add that extra spice of unpredictability to your programs.
In the next section, we’ll explore even more fun tricks with randomness.
Generating Random Sequences
Now that we’ve mastered random integers, let’s take it up a notch and create sequences of numbers! We can do this using the randrange(start, stop, step) function. It’s like having a magic wand for generating sequences. Check it out:
This code will give you a list of 5 random integers between 1 and 10, including both 1 and 10.
Generating random sequences of even or odd numbers
Want to be even more specific with your sequences? No problemo! You can use the randrange(start, stop, step) function to create sequences of even or odd numbers. Here’s how:
In the first example, you’ll get a list of 5 random even integers between 2 and 20. In the second example, you’ll get a list of 5 random odd integers between 1 and 19.
Selecting elements randomly from a list with choice()
Sometimes, you don’t want numbers; you want to randomly pick stuff from a list. Enter the choice(seq) function! It’s like grabbing a random treat from a jar. Here’s how it works:
This code will select a random fruit from the list. So, whether you’re deciding what to eat or adding variety to your programs, choice() has your back.
And there you have it – generating random sequences and making random selections with Python’s Random module! 🌈🐍
Shuffling and Sampling
Shuffling a list randomly with shuffle().
Ever wanted to mix things up randomly, like shuffling a deck of cards? Python’s got your back with the shuffle(seq) function. It’s like having a personal DJ for your list! Check it out:
When you run this code, your list my_deck will be shuffled into a random order. Perfect for card games or randomizing a playlist!
Randomly selecting multiple elements without repetition with sample()
Now, let’s say you need to pick a few items from a list without repeating any. The sample(seq, k) function is your go-to tool. It’s like reaching into a bag of goodies and getting exactly what you want! Here’s how it works:
In this example, you’ll get a list of 3 unique, randomly selected colors from the colors list. No duplicates allowed!
The Significance of Sampling in Statistics
So, what’s the big deal with sampling? Well, imagine you’re baking a huge batch of cookies, and you want to know if they taste good. Instead of eating all of them, you take a small, representative sample. Sampling in statistics is similar – it’s about studying a smaller group (sample) to draw conclusions about a larger group (population).
Random sampling is like the magician’s wand of statistics! 🎩✨ It ensures that each item in your population has an equal chance of being included in your sample. This randomness eliminates bias and makes your conclusions more trustworthy.
Resampling Techniques
Now, let’s talk about resampling techniques, like bootstrapping and jackknife. These methods are like having multiple chances to evaluate your data and make better decisions.
Bootstrapping
Bootstrapping is like creating mini replicas of your data by repeatedly sampling with replacement. It helps estimate the uncertainty in your data analysis.
With bootstrapping, you can calculate things like confidence intervals and standard errors.
Jackknife is like a systematic trial-and-error approach. You systematically leave out one data point at a time, creating multiple datasets, and evaluate how they impact your analysis.
Jackknife can help you assess the impact of individual data points on your analysis.
So, whether you’re reshuffling a playlist or picking random winners for a contest, Python’s Random module has you covered. These tools are like your trusty guides in the world of data analysis, helping you draw meaningful insights and make informed decisions.
Random Choices and Weighted Selection
Sometimes, you want to give certain options a higher chance of being picked – like picking your favorite candy more often than others. Python’s got you covered with the choices(seq, weights=None, k=1) function. It’s like customizing your own lucky draw! Check it out:
In this example, we have a list of options and corresponding weights. The higher the weight, the more likely that option is to be chosen. You can control the number of selections by changing the k parameter.
You can adjust the weights to match your preferences. Want more candy? Increase its weight! Want less fruit? Decrease its weight! It’s like being the chef of your own random menu.
So, whether you’re simulating choices in a game or creating a random menu, Python’s Random module gives you the power to control your selections.
Seed for Reproducibility: Making Randomness Feel Familiar
What is a random seed, anyway.
Sometimes, you want the random results to feel a bit more predictable, like when you’re sharing your cool code or running experiments. That’s where the concept of a “random seed” comes into play.
Think of a random seed as the secret ingredient that kickstarts the whole random number generation process. It’s like setting the stage for the randomness show.
Setting a random seed with seed() for that “Deja Vu” Feeling
Now, here’s where the magic happens. With Python’s Random module, you can actually set a specific seed value using the seed() function. 🌱
In this example, we’ve set the seed to the magical number 42 (because why not?). Now, here’s the cool part – no matter how many times you run this code, you’ll always get the same random numbers. It’s like having your own secret code for unlocking familiar randomness whenever you want!
So, whether you’re doing science-y stuff, creating a game, or just having some coding fun, Python’s Random module with a seed gives you the power to bring a touch of predictability to your randomness.
Random Distributions: Unleashing the Magic of Probability
Ever wonder how those random numbers in Python are born? It’s not all pure chaos; there’s a method to the madness, and it’s called probability distributions. Think of them as the blueprints for creating random numbers with different flavors.
Now, let’s roll up our sleeves and dive into some popular ones!
The Uniform Distribution: Where Everyone Gets a Fair Shot
Now, let’s chat about the Uniform Distribution. It’s like the fairest of them all when it comes to random numbers. You know why? Because in the uniform world, everyone gets an equal chance to shine!
What’s the deal with the Uniform Distribution?
Imagine you’re at a carnival, and there’s a game where you have to pop balloons with darts. If each balloon has an equal chance of being hit, you’re dealing with the Uniform Distribution. It’s all about providing a level playing field.
Now, let’s see how you can create some random numbers following the Uniform Distribution using Python:
Generating random numbers with uniform()
To create a random float within a specified range, you can use the uniform(a, b) function. It’s like throwing darts at a target, and every spot on that target has an equal shot at being hit:
With this code, you’ll get a random float between 1 and 10 (inclusive). It’s as if each number in that range has an equal chance of being picked.
So whether you’re simulating games, creating random test data, or just adding a touch of unpredictability to your code, the Uniform Distribution has got your back.
The Normal Distribution: Finding the Bell Curve in Randomness
Imagine you’re measuring the heights of a bunch of people. Most of them will cluster around the average height, with fewer people being extremely tall or extremely short. This clustering is the essence of the Normal Distribution. It’s all about the art of being typical!
Now, let’s see how you can create some random numbers that follow the Normal Distribution using Python:
Generating random numbers with gauss()
To create a random number from the Normal Distribution, you can use the gauss(mu, sigma) function. It’s like getting a random height for a person in a crowd, where mu is the mean (average) height, and sigma is the standard deviation (a measure of how spread out the heights are):
In this code, you’ll get a random float that follows the Normal Distribution with a mean (mu) of 0 and a standard deviation (sigma) of 1. The bell curve magic happens!
The Normal Distribution is a superstar in statistics and data science. It shows up when you least expect it, modeling everything from exam scores to physical measurements.
So, whether you’re analyzing data or just adding a touch of real-world randomness to your code, the Normal Distribution is your trusty companion.
The Exponential Distribution: Unveiling the Secrets of Random Timing
Now we’re going to explore the Exponential Distribution, a fascinating probability distribution that has a lot to say about the timing of random events. It’s like predicting when your favorite pizza delivery will arrive!
Imagine you’re waiting for a bus at a stop, and you’re curious about how long it’ll take for the next bus to show up. The Exponential Distribution can help you with that! It’s all about modeling the time between random events.
Now, let’s see how you can generate some random numbers following the Exponential Distribution using Python:
Generating random numbers with expovariate()
To create a random number from the Exponential Distribution, you can use the expovariate(lambd) function. It’s like predicting when the next bus will arrive, where lambd (lambda) represents the rate at which events occur:
In this code, you’ll get a random float that follows the Exponential Distribution with a rate parameter of 0.5. It’s as if you’re figuring out the timing of buses showing up at your stop.
The Exponential Distribution is super handy for modeling real-world events like arrival times, failure rates, or the time between customer arrivals at a store.
So, whether you’re simulating wait times, analyzing event data, or just adding a touch of time-based randomness to your code, the Exponential Distribution is your trusty companion.
The Triangular Distribution: Riding the Wave of Randomness
Now, let’s surf the waves of the Triangular Distribution, a cool and lesser-known probability distribution that adds an exciting twist to our random adventures. It’s like catching the perfect wave!
What’s the deal with the Triangular Distribution?
Imagine you’re trying to predict the score of a surprise test. You have a minimum and maximum possible score in mind, but you also think it’s more likely to fall somewhere in the middle. That’s where the Triangular Distribution comes into play. It’s all about modeling random events with a twist of “most likely” outcomes.
Now, let’s see how you can create some random numbers following the Triangular Distribution using Python:
Generating random numbers with triangular()
To create a random number from the Triangular Distribution, you can use the triangular(low, high, mode) function. It’s like predicting that your test score is most likely to be somewhere in the middle, but it could swing higher or lower:
In this code, you’re generating a random float that follows the Triangular Distribution. The low parameter is the minimum possible value, the high parameter is the maximum possible value, and the mode parameter is the most likely value.
The Triangular Distribution is super useful when you have an idea of the range of possible outcomes but also want to emphasize a “sweet spot” in the middle.
So, whether you’re making predictions, running simulations, or just adding a dash of uniqueness to your code, the Triangular Distribution is your go-to choice.
The Beta Distribution: Unraveling the Intricacies of Random Probability
Now let’s explore the Beta Distribution, a fascinating probability distribution that’s all about probabilities themselves. It’s like decoding the secrets of chance!
Imagine you’re conducting a survey, and you want to model the probability of people choosing “Option A” over “Option B.” The Beta Distribution can help you with that! It’s all about capturing the uncertainty of probabilities.
Now, let’s see how you can create some random numbers following the Beta Distribution using Python:
Generating random numbers with betavariate()
To create a random number from the Beta Distribution, you can use the betavariate(alpha, beta) function. It’s like simulating the probability of someone choosing “Option A” in your survey, where alpha and beta are parameters that control the shape of the distribution:
In this code, you’re generating a random float that follows the Beta Distribution with alpha set to 2 and beta set to 5. These parameters determine the shape of the distribution and how likely certain outcomes are.
The Beta Distribution is incredibly versatile and can be used in various scenarios, such as modeling probabilities, analyzing data, or even simulating random events.
So, whether you’re diving into statistics, conducting experiments, or just adding a pinch of probability to your code, the Beta Distribution is here to help.
The Gamma Distribution: Unveiling the Mysteries of Random Variables
Imagine you’re modeling the time it takes for customers to arrive at a store during different hours of the day. The Gamma Distribution can help you with that! It’s all about modeling continuous random events, like waiting times or durations.
Now, let’s see how you can create some random numbers following the Gamma Distribution using Python:
Generating random numbers with gammavariate()
To create a random number from the Gamma Distribution, you can use the gammavariate(alpha, beta) function. It’s like predicting the duration of a customer’s visit to your store, where alpha and beta are parameters that control the shape of the distribution:
In this code, you’re generating a random float that follows the Gamma Distribution with alpha set to 2 and beta set to 1. These parameters define the shape and scale of the distribution.
The Gamma Distribution is super versatile and can be used in various real-world scenarios, such as modeling waiting times, analyzing event durations, or even simulating complex random processes.
So, whether you’re dealing with time-based data, conducting experiments, or just adding a dose of randomness to your code, the Gamma Distribution is here to unravel the mysteries of random variables.
The Log-Normal Distribution: Unwrapping the Magic of Logarithmic Randomness
Imagine you’re tracking the growth of a population of organisms over time, and their growth rate follows a certain pattern. The Log-Normal Distribution can help you with that! It’s all about modeling data that has a natural logarithmic transformation.
Now, let’s see how you can create some random numbers following the Log-Normal Distribution using Python:
Generating random numbers with lognormvariate()
To create a random number from the Log-Normal Distribution, you can use the lognormvariate(mu, sigma) function. It’s like predicting the size of organisms in your population, where mu and sigma are parameters that control the shape and scale of the distribution:
In this code, you’re generating a random float that follows the Log-Normal Distribution with mu set to 0 and sigma set to 1. These parameters determine the shape and scale of the distribution, which is especially useful when dealing with data that has a skewed, rightward-sloping pattern.
The Log-Normal Distribution is a gem when it comes to modeling various real-world phenomena, from financial data to biological growth rates.
So, whether you’re analyzing financial markets, studying natural processes, or just adding a dash of logarithmic randomness to your code, the Log-Normal Distribution is here to unlock the magic of logarithmic numbers.
The Von Mises Distribution: Navigating Circular Randomness
Imagine you’re studying the direction in which migrating birds fly or analyzing daily temperature variations throughout the year. The Von Mises Distribution can help you with that! It’s tailor-made for data that revolves around a circle or repeats periodically.
Now, let’s see how you can create some random numbers following the Von Mises Distribution using Python:
Generating random numbers with vonmisesvariate()
To create a random number from the Von Mises Distribution, you can use the vonmisesvariate(mu, kappa) function. It’s like predicting the direction in which birds will migrate or the phase of the moon for a specific night. Here, mu represents the mean direction (in radians), and kappa controls the concentration of the distribution:
In this code, you’re generating a random float that follows the Von Mises Distribution with a mean direction of 0 radians and a concentration parameter (kappa) of 2. This distribution is often used for circular or directional data.
The Von Mises Distribution is a valuable tool when you’re dealing with data that repeats in a circular fashion, like angles, phases, or directions.
So, whether you’re studying natural phenomena, analyzing periodic trends, or simply adding a twist of circular randomness to your code, the Von Mises Distribution is here to guide you.
The Pareto Distribution: Revealing the Power of Extremes
Imagine you’re analyzing income data, and you notice that a small percentage of people earn most of the income. Or perhaps you’re studying the distribution of wealth in a population. The Pareto Distribution can help you with that! It’s all about modeling data where a small fraction of observations holds the lion’s share.
Now, let’s see how you can create some random numbers following the Pareto Distribution using Python:
Generating random numbers with paretovariate()
To create a random number from the Pareto Distribution, you can use the paretovariate(alpha) function. It’s like predicting the income of individuals in a population, where alpha is a shape parameter that determines the distribution’s tail:
In this code, you’re generating a random float that follows the Pareto Distribution with a shape parameter (alpha) set to 2. This distribution is often used to describe phenomena where a small number of elements have a disproportionately large effect.
The Pareto Distribution is incredibly valuable when you’re dealing with data that exhibits a “rich get richer” phenomenon, such as income distributions, wealth distributions, or even the popularity of online content.
So, whether you’re exploring economic inequality, modeling network traffic, or just adding a touch of extremeness to your code, the Pareto Distribution is here to help you uncover the outliers.
The Weibull Distribution: Unveiling the Secrets of Survival
Now, we’re diving into the Weibull Distribution, a fascinating probability distribution that’s all about understanding the probabilities of survival and failure over time. It’s like unraveling the mysteries of life itself!
Imagine you’re analyzing the lifespans of light bulbs, studying the time it takes for equipment to fail, or exploring the duration until a product needs replacement. The Weibull Distribution can help you with that! It’s all about modeling data where events occur over time, and the probability of survival changes as time goes on.
Now, let’s see how you can create some random numbers following the Weibull Distribution using Python:
Generating random numbers with weibullvariate()
To create a random number from the Weibull Distribution, you can use the weibullvariate(alpha, beta) function. It’s like predicting the lifespan of a product or the time until an event occurs. Here, alpha is the shape parameter that affects the distribution’s shape, and beta is the scale parameter that determines the scale of time:
In this code, you’re generating a random float that follows the Weibull Distribution with a shape parameter (alpha) of 2 and a scale parameter (beta) of 5. This distribution is often used to describe the distribution of failure times in reliability analysis.
The Weibull Distribution is a powerful tool when you’re dealing with data that involves survival or failure over time, whether it’s in engineering, healthcare, or other fields.
So, whether you’re conducting reliability studies, modeling lifespans, or just adding a hint of time-based randomness to your code, the Weibull Distribution is here to help you understand the dynamics of events over time.
Creating custom distributions using the random() function
Feeling creative? You can craft your very own probability distribution using the trusty random() function. It’s like being the chef of your own randomness recipe:
With this, you have complete control over your randomness! You can create any distribution you can dream of.
So, whether you’re counting events, designing your unique randomness, or just adding a sprinkle of unpredictability to your code, Python’s Random module has you covered.
Monte Carlo Simulations: Unveiling the Magic of Random Experiments
Now, we’re embarking on a journey into the realm of Monte Carlo simulations, a powerful tool used to tackle complex problems by conducting random experiments. It’s like having a crystal ball that predicts the future through the art of randomness!
What Are Monte Carlo Simulations, Anyway?
Imagine you have a complex problem that’s too tricky to solve directly. Monte Carlo simulations are your trusty sidekick in such situations. They’re a technique that uses random numbers to run thousands or even millions of experiments to find an approximate solution. Think of it as trying out different scenarios to get closer to the answer.
Using random numbers for simulations
Random numbers are the heart and soul of Monte Carlo simulations! 🎲 They help inject randomness into your experiments, making them as close to real-world scenarios as possible. Whether it’s modeling the movement of particles, predicting stock prices, or simulating game strategies, random numbers are the key to unlocking the magic.
Here’s a simple example of how you can use Python’s Random module for a Monte Carlo simulation:
With this code, you can simulate a coin toss – a fundamental experiment in the world of probability.
Applications in Finance, Physics, and Beyond
Monte Carlo simulations are like Swiss Army knives for problem-solving! They find applications in various fields:
- Predicting stock price movements.
- Assessing portfolio risk.
- Pricing complex financial derivatives.
- Simulating particle interactions.
- Studying quantum systems.
- Analyzing the behavior of gases and fluids.
Engineering
- Designing and testing new products.
- Evaluating the reliability of systems.
- Optimizing manufacturing processes.
- Testing game strategies.
- Simulating player behavior.
- Balancing in-game economies.
These are just a few examples of how Monte Carlo simulations revolutionize problem-solving across different domains. They’re like the magic wand of science and engineering!
So, whether you’re predicting the future of the stock market, exploring the behavior of particles in a particle accelerator, or fine-tuning your favorite video game, Monte Carlo simulations and random numbers are your partners in discovery.
Exploring Advanced Random Topics: From Cryptography to Gaming Fun
Now, we’re going to dive into some cool and practical uses of random numbers, from creating cryptographic secrets to adding the element of surprise in game development. Let’s unlock the secrets of randomness!
Cryptographically Secure Random Numbers with the Secrets Module
When it comes to cryptography, randomness is serious business! 🔐 The secrets module in Python is your go-to tool for generating cryptographically secure random numbers. These numbers are like fortresses, virtually impossible for anyone to predict.
Here’s a glimpse of how you can use the secrets module to generate a secure random integer:
These random numbers are essential for things like generating encryption keys or creating secure tokens for authentication.
Randomizing Elements in Game Development
Game developers know that randomness is the spice of gaming life! 🎮 Whether you’re creating loot drops in a role-playing game or determining enemy behavior in a shooter, random elements keep players on their toes.
Here’s an example of randomly shuffling a list of items in Python for game development:
This adds an element of surprise and excitement to your game.
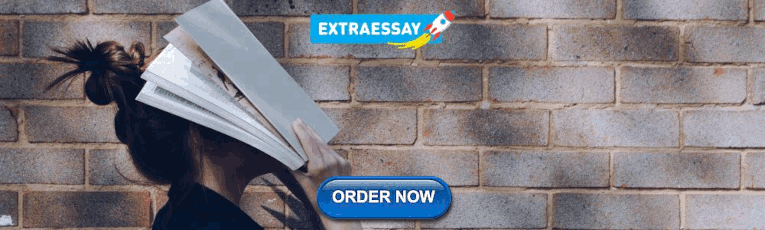
Generating Unique Identifiers and Tokens
In the digital world, unique identifiers and tokens are like fingerprints. They need to be truly one-of-a-kind. Randomness plays a crucial role in creating them.
For example, generating a random unique token in Python can be done like this:
These tokens are used in authentication, session management, and more.
Random Password Generation
Creating strong and unpredictable passwords is vital for cybersecurity. Python can be your trusty password generator!
Here’s how you can generate a random password with a mix of letters, numbers, and symbols:
Random passwords like these are excellent for protecting your online accounts.
So there you have it – a journey into the advanced applications of random numbers in cryptography, game development, unique identifiers, and password generation. These practical uses make randomness an invaluable tool in the digital age.
Best Practices and Considerations: Navigating the World of Randomness in Programming
Now, let’s dive into some best practices and important considerations when it comes to wielding the power of randomness in your code. It’s like becoming a master magician, knowing precisely when and how to pull the random rabbit out of the hat!
When and Where to Use Randomness in Programming
Randomness is like a versatile spice in your coding kitchen. 🌶️ It can add flavor and excitement to your programs, but knowing when and where to use it is key.
Here are some common scenarios:
- Simulations: Randomness is often used to mimic real-world situations, from predicting stock market fluctuations to modeling the behavior of particles in physics.
- Game Development: Games thrive on randomness! Whether it’s generating random maps, determining enemy behavior, or creating loot drops, randomness keeps players engaged.
- Security: Generating secure encryption keys and tokens relies on true randomness.
- Machine Learning: Randomness plays a role in techniques like random forest and dropout layers in neural networks.
- Testing: Random inputs can help you identify edge cases and unexpected behavior in your code.
The key is to use randomness purposefully and with a clear understanding of its implications.
Handling Randomization in Parallel Computing
Parallel computing is like juggling multiple balls at once, and introducing randomness can make it even more challenging! 🤹 When working with random code in parallel, consider using thread-safe random number generators or ensuring that each thread has its own independent random generator.
Dealing with Pseudo-Randomness Limitations
Pseudo-random number generators (PRNGs) are deterministic, which means they produce the same sequence of numbers if given the same seed. While this is often useful, it can be a limitation in some scenarios, especially when you need true randomness.
For cryptographic purposes or when absolute randomness is required, consider using a cryptographically secure random number generator like secrets in Python.
Performance Considerations for Large-Scale Simulations
When you’re dealing with large-scale simulations, the efficiency of your random code matters. Generating and managing a massive number of random numbers can be resource-intensive.
Consider using optimized libraries and data structures to speed up random operations. Parallelizing your simulations across multiple processors can also significantly boost performance.
In summary, randomness is a powerful tool in programming, but it’s essential to wield it wisely. Use randomness purposefully, ensure thread safety in parallel computing, address limitations when true randomness is required, and optimize your code for large-scale simulations.
Real-World Projects: Bringing Randomness to Life in Python
Now, let’s talk about some exciting real-world projects that will let you put your newfound knowledge of randomness into action. Let’s roll up our sleeves and get coding!
Building a Simple Random Number Generator Application
Ever wanted to create your own random number generator? It’s like having your own magic wand to conjure random numbers on demand!
Here’s a basic example in Python:
You can take this a step further by creating a user-friendly application with a graphical user interface (GUI) using libraries like Tkinter or PyQt.
Simulating Dice Rolls and Card Shuffling for Games
If you’re into game development, simulating dice rolls and card shuffling is a must. Whether you’re building a digital board game or a casino app, randomness adds the element of surprise.
Here’s an example of simulating a dice roll:
For card shuffling, you can create a deck of cards and use the random.shuffle() function to shuffle them.
Implementing a Monte Carlo Simulation for a Real-World Problem
Monte Carlo simulations are like Swiss Army knives for solving complex problems. Think of a real-world problem, and chances are, you can use Monte Carlo to tackle it.
For example, if you’re into finance, you can simulate the behavior of a portfolio of stocks to estimate its risk and potential returns. Or if you’re in logistics, you can use Monte Carlo to optimize supply chain routes.
The possibilities are endless, and Python is your trusty companion on this journey.
Conclusion: Embrace the Power of Randomness!
What a fantastic journey it has been exploring the world of randomness in Python! 🎲🔮 We’ve covered the basics, ventured into advanced topics, and even dived into real-world projects. Now, let’s recap what we’ve learned and encourage you to keep exploring this magical realm.
Recap of Key Concepts Learned
- We’ve explored random number generation in Python using the random module.
- We’ve ventured into probability distributions like Uniform, Normal, Exponential, etc.
- We’ve discussed resampling techniques, Monte Carlo simulations, and best practices.
- We’ve delved into real-world applications and performance considerations.
Encouragement for Further Exploration
The world of randomness is vast and ever-evolving. Python offers a wealth of tools and libraries for exploring it further. Whether you’re into data science, game development, cryptography, finance, or any other field, randomness will be your faithful companion.
Similar Posts
Tkinter spinbox.
Tkinter Spinbox Widget The Tkinter Spinbox widget is the advanced version of the Entry widget. In the Spinbox widget, a user can choose a value…
Hey Python Learner, In this tutorial, we’ll check out ttk buttons, which are like the fancier version of regular Tkinter buttons. They have cool features…
The Ultimate Reference for Tkinter Standard Options
Hey there! Welcome to our Python Tkinter tutorial series! In Tkinter, widgets are the building blocks of graphical user interfaces (GUIs). Each widget comes with…
Designing GUI Toolbars with Tkinter Menubutton
Hey there! Welcome to our Python Tkinter Menubutton Tutorial. What is a Tkinter Menubutton? In Tkinter, a Menubutton is a widget that displays a drop-down…
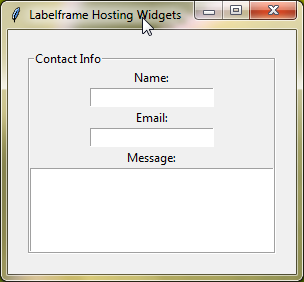
Design and Organize with Tkinter Labelframes
Hey there, fellow Python lovers! Welcome to this excellent Tkinter Labelframe tutorial. Buckle up because we’re diving deep into the fantastic world of Labelframes in…
ttk Notebook in Tkinter
ttk Notebook in Tkinter A ttk Notebook is a container widget that can contain multiple content windows inside it but display only one window at…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Privacy Overview
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Partial credit goes to the Programming Club at Normandale Community College This program tested and runs in python 3.2.5 Create/Modefy groups of people based on group size and without allowing even a single member to be in a new group with a member whos he/she has been in a group before. February 27 2015 Completed: + Creating new text file + Rea…
DenisProkopenko/-Python-Random-Group-Generator
Folders and files, repository files navigation, -python-random-group-generator.
Partial credit goes to the Programming Club at Normandale Community College
This program tested and runs in python 3.2.5
Create/Modefy groups of people based on group size and without allowing even a single member to be in a new group with a member whos he/she has been in a group before.
February 27 2015 Completed:
- Creating new text file
- Reading from text file
- Writing to text file
- Entering names
- Adding names
- Deleting names
- Printing names
- Group Algorithm (works but not yet impimented into this file)
Incomplete:
- Print Group Algorithm in a much clearer way
- Integrate students with the Group Algorithm
March 03 2015 Completed:
- Group Algorithm (algorithm changed to just grab name and pop name to remove name re-use)
- Integrate students with the Group Algorithm (removed this step and integrated it with Group Algorithm)
- Rearange groups if the user wants more or less # of groups (could also be done by recreating the groups)
- Python 100.0%
Random assignment of participants to groups
I am trying to set up a PsychoPy experiment, first time all by myself. Unfortunately, I am a total beginner when it comes to Python and programming. Good thing is, I already have a working experiment template from a previous pilot study which I now need to change to my needs.
Major thing which needs to be included is: Participants should randomly be assigned to one of four different groups. The experiment consists of two phases: training phase and generalisation phase. Thus, when a participant was assigned to group 1 in the training phase, they have to be assigned to the same group in the generalisation phase.
I’ve created the files I need (excel & folder with pictures) for each group and each phase: so I have for example the files for TrainingG1 (group 1) containing the TrainingG1 excel file and the TrainingG1 zip-folder with the PNG files. Same goes for TrainingG2-4 and GeneralisationG1-4.
I know how to set up the experiment with Training & Generalisation phase for one group, but how can I add a “junction” so to say where the participants are randomly assigned one way (either group1, group2, group3, group4)?
I would really appreciate your help!
Hello Lucia
Put this code at a “Begin Experiment”-tab. It loads four differtent stimulus-files depending on the participant number.
Hey Jens and LuciaIglhaut, two things: Do you know of a way to group participants with non-numeric IDs? I am using Prolific (for the first time) and I believe they don’t use a simple incrementing number as ID.
and for Lucial: once you used the Code of Jens, you need to use the variable (in this case stimfile) in the conditionsfile of a loop. like this: $stimfile it is necissary, that all of the variables in the .xlsx are called the same and is kind of prone to errors.
Regards, Fritz
Hello Becker,
sorry, I have not used Prolific, but you find suggestion on how to use Prolifc and Pavlovia in the forum Search results for 'Prolific' - PsychoPy
Hi @JensBoelte
I’m afraid that this code is incorrect. For four groups all of the statements should have %4 but then check for values 0, 1, 2 and 3.
You can use my VESPR study portal to assign participants to groups or assign numeric participant numbers.
https://moryscarter.com/vespr/portal
Hi @wakecarter
do you mind telling me what is wrong with this code? I checked it and it loads the simulus-files accordingly.
Best wishes Jens
In words, you’ve written: if divisible by 1 else if divisible by 3 else if divisible by 2 else.
Here are how the first 20 participants will be divided:
- 1; 5; 7; 11; 13; 17; 19 = 7 participants
- 2; 10; 14 = 3 participants
- 3; 6; 9; 15; 18 = 5 participants
- 4; 8; 12; 16; 20 = 5 participants
Please correct me if you think I’ve got that wrong. Participant 0 (if you have one) would be allocated to group 4.
My method is to divide by 4 and use the remainder which will equally be 0, 1, 2 or 3.
Hello @wakecarter
that is correct. My code does not result in an even distribution of participants to all groups. I knew that. Your code results in an even distribution of participants to groups which is probably advantageous in most cases.
Thanks for highlighting this.
Related Topics
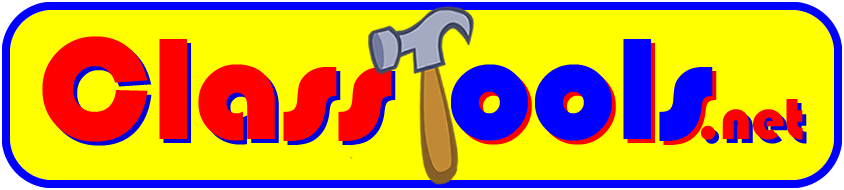
PREMIUM LOGIN
ClassTools Premium membership gives access to all templates, no advertisements, personal branding and other benefits!
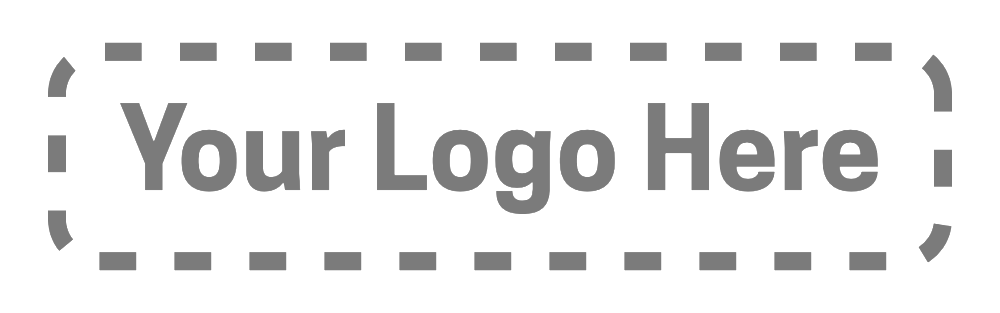
Random Group Generator
1. group title, 2. input names here.
Sandy Shaw Peg Baskett Sally Forth Pat Gently Sandy Beach Walter Melon Don Key Sonny Day Gene Pool
3. Number of groups required:
Video tutorial.
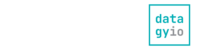
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Pandas GroupBy: Group, Summarize, and Aggregate Data in Python
- December 20, 2021 June 24, 2023
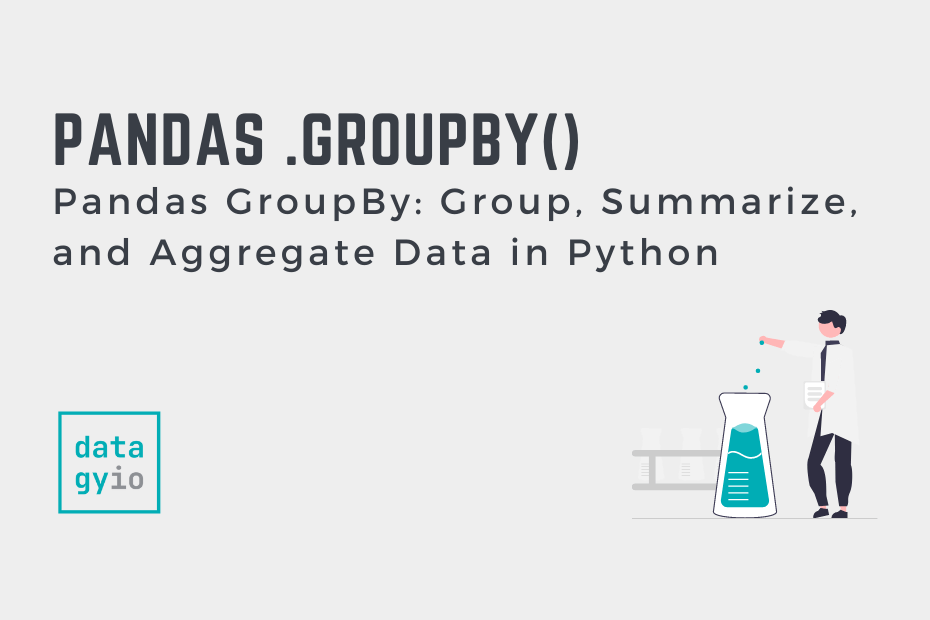
The Pandas groupby method is an incredibly powerful tool to help you gain effective and impactful insight into your dataset. In just a few, easy to understand lines of code, you can aggregate your data in incredibly straightforward and powerful ways.
By the end of this tutorial, you’ll have learned how the Pandas .groupby() method works by using split-apply-combine. This process efficiently handles large datasets to manipulate data in incredibly powerful ways. You’ll learn how to master the method from end to end, including accessing groups, transforming data, and generating derivative data.
Table of Contents
What is the Pandas GroupBy Method?
The Pandas .groupby() method works in a very similar way to the SQL GROUP BY statement. In fact, it’s designed to mirror its SQL counterpart leverage its efficiencies and intuitiveness. Similar to the SQL GROUP BY statement, the Pandas method works by splitting our data, aggregating it in a given way (or ways), and re-combining the data in a meaningful way.
Because the .groupby() method works by first splitting the data, we can actually work with the groups directly. Similarly, because any aggregations are done following the splitting, we have full reign over how we aggregate the data. Pandas then handles how the data are combined in order to present a meaningful DataFrame.
What’s great about this is that it allows us to use the method in a variety of ways, especially in creative ways. Because of this, the method is a cornerstone to understanding how Pandas can be used to manipulate and analyze data. This tutorial’s length reflects that complexity and importance!
Why Does Pandas Offer Multiple Ways to Aggregate Data?
Pandas seems to provide a myriad of options to help you analyze and aggregate our data. Why would there be, what often seem to be, overlapping method? The answer is that each method, such as using the .pivot() , .pivot_table() , .groupby() methods, provide a unique spin on how data are aggregated. They’re not simply repackaged, but rather represent helpful ways to accomplish different tasks.
Loading a Sample Pandas DataFrame
In order to follow along with this tutorial, let’s load a sample Pandas DataFrame. Let’s load in some imaginary sales data using a dataset hosted on the datagy Github page. If you want to follow along line by line, copy the code below to load the dataset using the .read_csv() method:
By printing out the first five rows using the .head() method, we can get a bit of insight into our data. We can see that we have a date column that contains the date of a transaction. We have string type columns covering the gender and the region of our salesperson. Finally, we have an integer column, sales , representing the total sales value.
Understanding Pandas GroupBy Objects
Let’s take a first look at the Pandas .groupby() method. We can create a GroupBy object by applying the method to our DataFrame and passing in either a column or a list of columns. Let’s see what this looks like – we’ll create a GroupBy object and print it out:
We can see that this returned an object of type DataFrameGroupBy . Because it’s an object, we can explore some of its attributes.
Pandas GroupBy Attributes
For example, these objects come with an attribute, .ngroups , which holds the number of groups available in that grouping:
We can see that our object has 3 groups. Similarly, we can use the .groups attribute to gain insight into the specifics of the resulting groups. The output of this attribute is a dictionary-like object, which contains our groups as keys. The values of these keys are actually the indices of the rows belonging to that group!
If we only wanted to see the group names of our GroupBy object, we could simply return only the keys of this dictionary.
We can see how useful this method already is! It allows us to group our data in a meaningful way
Selecting a Pandas GroupBy Group
We can also select particular all the records belonging to a particular group. This can be useful when you want to see the data of each group. In order to do this, we can apply the .get_group() method and passing in the group’s name that we want to select. Let’s try and select the 'South' region from our GroupBy object:
This can be quite helpful if you want to gain a bit of insight into the data. Similarly, it gives you insight into how the .groupby() method is actually used in terms of aggregating data. In the following section, you’ll learn how the Pandas groupby method works by using the split, apply, and combine methodology.
Understanding Pandas GroupBy Split-Apply-Combine
The Pandas groupby method uses a process known as split, apply, and combine to provide useful aggregations or modifications to your DataFrame. This process works as just as its called:
- Splitting the data into groups based on some criteria
- Applying a function to each group independently
- Combing the results into an appropriate data structure
In the section above, when you applied the .groupby() method and passed in a column, you already completed the first step! You were able to split the data into relevant groups, based on the criteria you passed in.
The reason for applying this method is to break a big data analysis problem into manageable parts. This allows you to perform operations on the individual parts and put them back together. While the apply and combine steps occur separately, Pandas abstracts this and makes it appear as though it was a single step.
Using Split-Apply-Combine Without GroupBy
Before we dive into how the .groupby() method works, lets take a look at how we can replicate it without the use of the function. The benefit of this approach is that we can easily understand each step of the process.
- Splitting the data: Let’s begin by splitting the data – we can loop over each unique value in the DataFrame, splitting the data by the 'region' column.
- Applying a aggregation function: From there, we can select the rows from the DataFrame that meet the condition and apply a function to it.
- Combining the Data: Finally, we can create a dictionary and add data to it and turn it back into a Pandas DataFrame.
This is a lot of code to write for a simple aggregation! Thankfully, the Pandas groupby method makes this much, much easier. In the next section, you’ll learn how to simplify this process tremendously.
Aggregating Data with Pandas GroupBy
In this section, you’ll learn how to use the Pandas groupby method to aggregate data in different ways. We’ll try and recreate the same result as you learned about above in order to see how much simpler the process actually is! Let’s take a look at what the code looks like and then break down how it works:
Take a look at the code! We were able to reduce six lines of code into a single line! Let’s break this down element by element:
- df.groupby('region') is familiar to you by now. It splits the data into different groups, based on the region column
- ['sales'] selects only that column from the groupings
- .mean() applies the mean method to the column in each group
- The data are combined into the resulting DataFrame, averages
Let’s take a look at the entire process a little more visually. In order to make it easier to understand visually, let’s only look at the first seven records of the DataFrame:
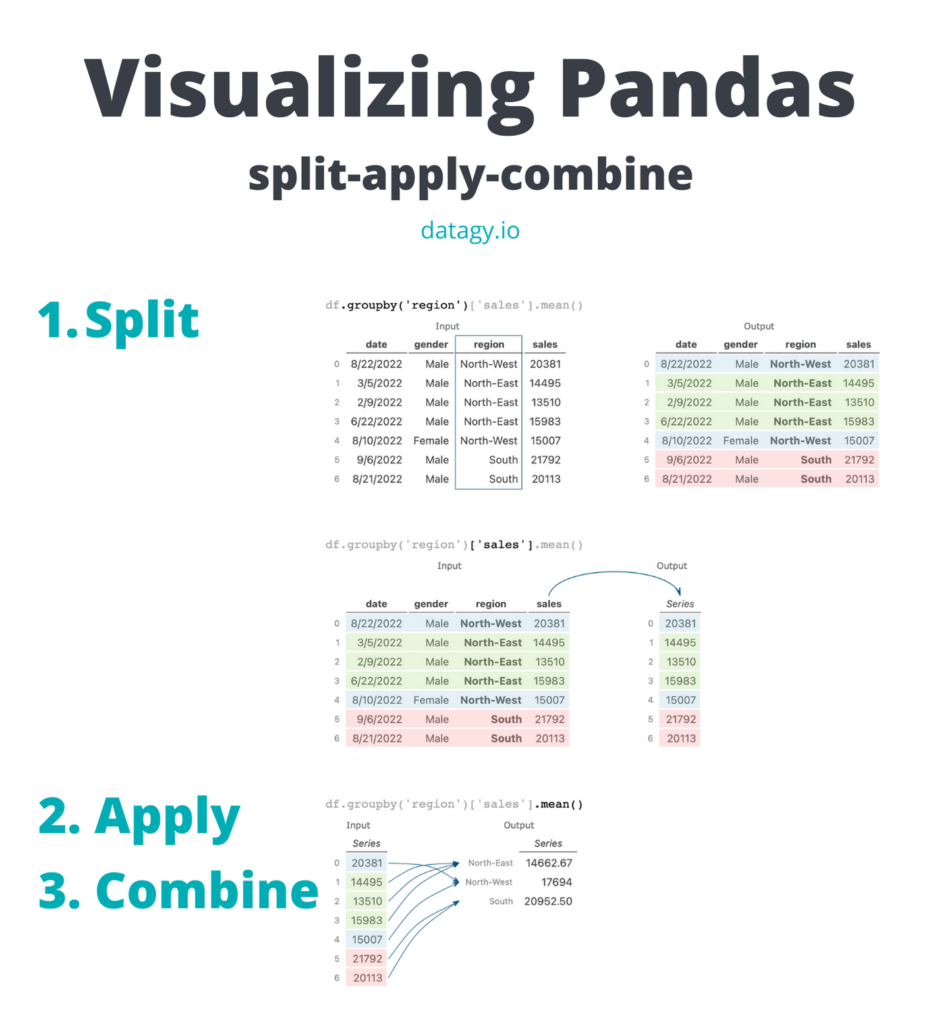
In the image above, you can see how the data is first split into groups and a column is selected, then an aggregation is applied and the resulting data are combined.
Other Aggregations with Pandas GroupBy
Now that you understand how the split-apply-combine procedure works, let’s take a look at some other aggregations work in Pandas. The table below provides an overview of the different aggregation functions that are available:
For example, if we wanted to calculate the standard deviation of each group, we could simply write:
Applying Multiple Aggregations Using Pandas GroupBy
Pandas also comes with an additional method, .agg() , which allows us to apply multiple aggregations in the .groupby() method. The method allows us to pass in a list of callables (i.e., the function part without the parentheses). Let’s see how we can apply some of the functions that come with the numpy library to aggregate our data.
Using the .agg() method allows us to easily generate summary statistics based on our different groups. Without this, we would need to apply the .groupby() method three times but here we were able tor reduce it down to a single method call!
Transforming Data with Pandas GroupBy
Another incredibly helpful way you can leverage the Pandas groupby method is to transform your data. What does this mean? By transforming your data, you perform some operation-specific to that group. This can include, for example, standardizing the data based only on that group using a z-score or dealing with missing data by imputing a value based on that group.
What makes the transformation operation different from both aggregation and filtering using .groupby() is that the resulting DataFrame will be the same dimensions as the original data. While this can be true for aggregating and filtering data, it is always true for transforming data.
The .transform() method will return a single value for each record in the original dataset. Because of this, the shape is guaranteed to result in the same size.
Using .transform In GroupBy
Let’s take a look at an example of transforming data in a Pandas DataFrame. In this example, we’ll calculate the percentage of each region’s total sales is represented by each sale. In order to do this, we can apply the .transform() method to the GroupBy object. We can pass in the 'sum' callable to return the sum for the entire group onto each row. Finally, we divide the original 'sales' column by that sum.
Let’s see what this code looks like:
In the resulting DataFrame, we can see how much each sale accounted for out of the region’s total.
Transforming Data without .transform
While in the previous section, you transformed the data using the .transform() function, we can also apply a function that will return a single value without aggregating. As an example, let’s apply the .rank() method to our grouping. This will allow us to, well, rank our values in each group. Rather than using the .transform() method, we’ll apply the .rank() method directly:
In this case, the .groupby() method returns a Pandas Series of the same length as the original DataFrame. Because of this, we can simply assign the Series to a new column.
Filtering Data with Pandas GroupBy
A great way to make use of the .groupby() method is to filter a DataFrame. This approach works quite differently from a normal filter since you can apply the filtering method based on some aggregation of a group’s values. For example, we can filter our DataFrame to remove rows where the group’s average sale price is less than 20,000.
Let’s break down how this works:
- We group our data by the 'region' column
- We apply the .filter() method to filter based on a lambda function that we pass in
- The lambda function evaluates whether the average value found in the group for the 'sales' column is less than 20,000
This approach saves us the trouble of first determining the average value for each group and then filtering these values out. In this example, the approach may seem a bit unnecessary. However, it opens up massive potential when working with smaller groups.
Grouping a Pandas DataFrame by Multiple Columns
We can extend the functionality of the Pandas .groupby() method even further by grouping our data by multiple columns. So far, you’ve grouped the DataFrame only by a single column, by passing in a string representing the column. However, you can also pass in a list of strings that represent the different columns. By doing this, we can split our data even further.
Let’s calculate the sum of all sales broken out by 'region' and by 'gender' by writing the code below:
What’s more, is that all the methods that we previously covered are possible in this regard as well. For example, we could apply the .rank() function here again and identify the top sales in each region-gender combination:
Using Custom Functions with Pandas GroupBy
Another excellent feature of the Pandas .groupby() method is that we can even apply our own functions. This allows us to define functions that are specific to the needs of our analysis. You’ve actually already seen this in the example to filter using the .groupby() method. We can either use an anonymous lambda function or we can first define a function and apply it.
Let’s take a look at how this can work. We can define a custom function that will return the range of a group by calculating the difference between the minimum and the maximum values. Let’s define this function and then apply it to our .groupby() method call:
The group_range() function takes a single parameter, which in this case is the Series of our 'sales' groupings. We find the largest and smallest values and return the difference between the two. This can be helpful to see how different groups’ ranges differ.
Useful Examples of Pandas GroupBy
In this section, you’ll learn some helpful use cases of the Pandas .groupby() method. The examples in this section are meant to represent more creative uses of the method. These examples are meant to spark creativity and open your eyes to different ways in which you can use the method.
Getting the First n Rows of a Pandas GroupBy
Let’s take a look at how you can return the five rows of each group into a resulting DataFrame. This can be particularly helpful when you want to get a sense of what the data might look like in each group. If it doesn’t matter how the data are sorted in the DataFrame, then you can simply pass in the .head() function to return any number of records from each group.
Let’s take a look at how to return two records from each group, where each group is defined by the region and gender:
Getting the nth Largest Row of a Pandas GroupBy
In this example, you’ll learn how to select the n th largest value in a given group. For this, we can use the .nlargest() method which will return the largest value of position n. For example, if we wanted to return the second largest value in each group, we could simply pass in the value 2. Let’s see what this looks like:
It’s time to check your learning! Use the exercises below to practice using the .groupby() method. The solutions are provided by toggling the section under each question.
Return a DataFrame containing the minimum value of each region’s dates.
Which is the smallest standard deviation of sales?
How would you return the last 2 rows of each group of region and gender?
Conclusion and Recap
In this tutorial, you learned about the Pandas .groupby() method. The method allows you to analyze, aggregate, filter, and transform your data in many useful ways. Below, you’ll find a quick recap of the Pandas .groupby() method:
- The Pandas .groupby() method allows you to aggregate, transform, and filter DataFrames
- The method works by using split, transform, and apply operations
- You can group data by multiple columns by passing in a list of columns
- You can easily apply multiple aggregations by applying the .agg() method
- You can use the method to transform your data in useful ways, such as calculating z-scores or ranking your data across different groups
The official documentation for the Pandas .groupby() method can be found here .
Additional Resources
To learn more about related topics, check out the tutorials below:
- Pandas: Count Unique Values in a GroupBy Object
- Python Defaultdict: Overview and Examples
- Calculate a Weighted Average in Pandas and Python
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
13 thoughts on “Pandas GroupBy: Group, Summarize, and Aggregate Data in Python”
Pingback: Creating Pivot Tables in Pandas with Python for Python and Pandas • datagy
Pingback: Pandas Value_counts to Count Unique Values • datagy
Pingback: Binning Data in Pandas with cut and qcut • datagy
thank you for your great explanation
Thanks Laurent!
That is wonderful explanation … really appreciated
Thanks Abdelghany!
Great tutorial like always! I would just add an example with firstly using sort_values, then groupby(), for example this line: df.sort_values(by=’sales’).groupby([‘region’, ‘gender’]).head(2)
That’s awesome! Thanks for adding this!
Hello, Question 2 is not formatted to copy/paste/run. It gives a SyntaxError: invalid character ‘‘’ (U+2018).
Thanks! I have fixed this issue.
sums = df.groupby([‘region’, ‘gender’]).sum() print(sums.head()) TypeError: datetime64 type does not support sum operations
maybe ? sums = df.groupby([‘region’, ‘gender’])[‘sales’].sum() print(sums.head())
Hi Konst, Thanks so much! I have updated the post.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Have a language expert improve your writing
Run a free plagiarism check in 10 minutes, generate accurate citations for free.
- Knowledge Base
Methodology
- Random Assignment in Experiments | Introduction & Examples
Random Assignment in Experiments | Introduction & Examples
Published on March 8, 2021 by Pritha Bhandari . Revised on June 22, 2023.
In experimental research, random assignment is a way of placing participants from your sample into different treatment groups using randomization.
With simple random assignment, every member of the sample has a known or equal chance of being placed in a control group or an experimental group. Studies that use simple random assignment are also called completely randomized designs .
Random assignment is a key part of experimental design . It helps you ensure that all groups are comparable at the start of a study: any differences between them are due to random factors, not research biases like sampling bias or selection bias .
Table of contents
Why does random assignment matter, random sampling vs random assignment, how do you use random assignment, when is random assignment not used, other interesting articles, frequently asked questions about random assignment.
Random assignment is an important part of control in experimental research, because it helps strengthen the internal validity of an experiment and avoid biases.
In experiments, researchers manipulate an independent variable to assess its effect on a dependent variable, while controlling for other variables. To do so, they often use different levels of an independent variable for different groups of participants.
This is called a between-groups or independent measures design.
You use three groups of participants that are each given a different level of the independent variable:
- a control group that’s given a placebo (no dosage, to control for a placebo effect ),
- an experimental group that’s given a low dosage,
- a second experimental group that’s given a high dosage.
Random assignment to helps you make sure that the treatment groups don’t differ in systematic ways at the start of the experiment, as this can seriously affect (and even invalidate) your work.
If you don’t use random assignment, you may not be able to rule out alternative explanations for your results.
- participants recruited from cafes are placed in the control group ,
- participants recruited from local community centers are placed in the low dosage experimental group,
- participants recruited from gyms are placed in the high dosage group.
With this type of assignment, it’s hard to tell whether the participant characteristics are the same across all groups at the start of the study. Gym-users may tend to engage in more healthy behaviors than people who frequent cafes or community centers, and this would introduce a healthy user bias in your study.
Although random assignment helps even out baseline differences between groups, it doesn’t always make them completely equivalent. There may still be extraneous variables that differ between groups, and there will always be some group differences that arise from chance.
Most of the time, the random variation between groups is low, and, therefore, it’s acceptable for further analysis. This is especially true when you have a large sample. In general, you should always use random assignment in experiments when it is ethically possible and makes sense for your study topic.
Receive feedback on language, structure, and formatting
Professional editors proofread and edit your paper by focusing on:
- Academic style
- Vague sentences
- Style consistency
See an example
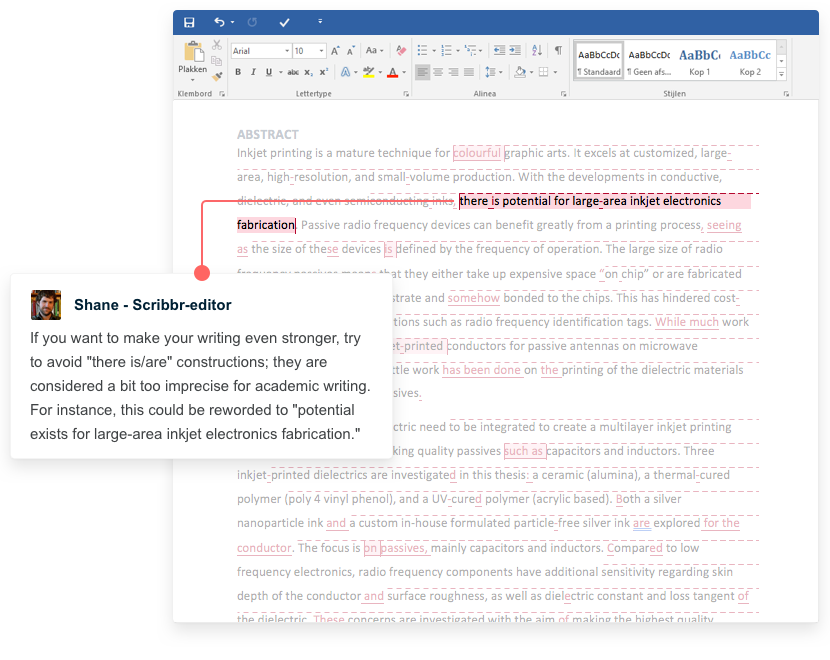
Random sampling and random assignment are both important concepts in research, but it’s important to understand the difference between them.
Random sampling (also called probability sampling or random selection) is a way of selecting members of a population to be included in your study. In contrast, random assignment is a way of sorting the sample participants into control and experimental groups.
While random sampling is used in many types of studies, random assignment is only used in between-subjects experimental designs.
Some studies use both random sampling and random assignment, while others use only one or the other.
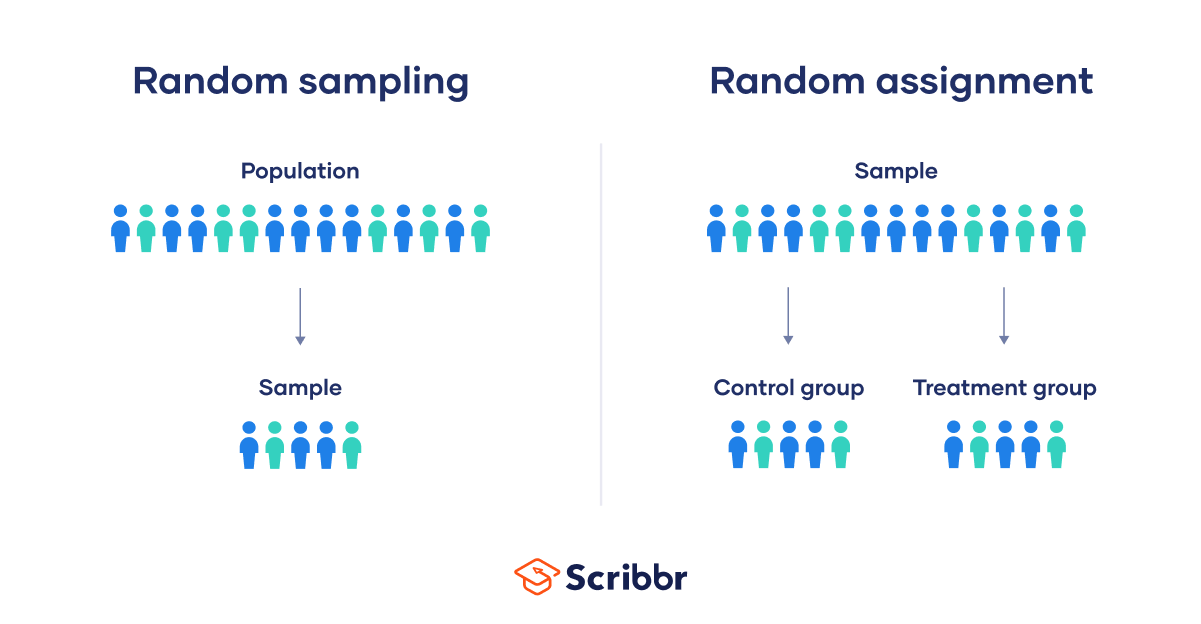
Random sampling enhances the external validity or generalizability of your results, because it helps ensure that your sample is unbiased and representative of the whole population. This allows you to make stronger statistical inferences .
You use a simple random sample to collect data. Because you have access to the whole population (all employees), you can assign all 8000 employees a number and use a random number generator to select 300 employees. These 300 employees are your full sample.
Random assignment enhances the internal validity of the study, because it ensures that there are no systematic differences between the participants in each group. This helps you conclude that the outcomes can be attributed to the independent variable .
- a control group that receives no intervention.
- an experimental group that has a remote team-building intervention every week for a month.
You use random assignment to place participants into the control or experimental group. To do so, you take your list of participants and assign each participant a number. Again, you use a random number generator to place each participant in one of the two groups.
To use simple random assignment, you start by giving every member of the sample a unique number. Then, you can use computer programs or manual methods to randomly assign each participant to a group.
- Random number generator: Use a computer program to generate random numbers from the list for each group.
- Lottery method: Place all numbers individually in a hat or a bucket, and draw numbers at random for each group.
- Flip a coin: When you only have two groups, for each number on the list, flip a coin to decide if they’ll be in the control or the experimental group.
- Use a dice: When you have three groups, for each number on the list, roll a dice to decide which of the groups they will be in. For example, assume that rolling 1 or 2 lands them in a control group; 3 or 4 in an experimental group; and 5 or 6 in a second control or experimental group.
This type of random assignment is the most powerful method of placing participants in conditions, because each individual has an equal chance of being placed in any one of your treatment groups.
Random assignment in block designs
In more complicated experimental designs, random assignment is only used after participants are grouped into blocks based on some characteristic (e.g., test score or demographic variable). These groupings mean that you need a larger sample to achieve high statistical power .
For example, a randomized block design involves placing participants into blocks based on a shared characteristic (e.g., college students versus graduates), and then using random assignment within each block to assign participants to every treatment condition. This helps you assess whether the characteristic affects the outcomes of your treatment.
In an experimental matched design , you use blocking and then match up individual participants from each block based on specific characteristics. Within each matched pair or group, you randomly assign each participant to one of the conditions in the experiment and compare their outcomes.
Sometimes, it’s not relevant or ethical to use simple random assignment, so groups are assigned in a different way.
When comparing different groups
Sometimes, differences between participants are the main focus of a study, for example, when comparing men and women or people with and without health conditions. Participants are not randomly assigned to different groups, but instead assigned based on their characteristics.
In this type of study, the characteristic of interest (e.g., gender) is an independent variable, and the groups differ based on the different levels (e.g., men, women, etc.). All participants are tested the same way, and then their group-level outcomes are compared.
When it’s not ethically permissible
When studying unhealthy or dangerous behaviors, it’s not possible to use random assignment. For example, if you’re studying heavy drinkers and social drinkers, it’s unethical to randomly assign participants to one of the two groups and ask them to drink large amounts of alcohol for your experiment.
When you can’t assign participants to groups, you can also conduct a quasi-experimental study . In a quasi-experiment, you study the outcomes of pre-existing groups who receive treatments that you may not have any control over (e.g., heavy drinkers and social drinkers). These groups aren’t randomly assigned, but may be considered comparable when some other variables (e.g., age or socioeconomic status) are controlled for.
Here's why students love Scribbr's proofreading services
Discover proofreading & editing
If you want to know more about statistics , methodology , or research bias , make sure to check out some of our other articles with explanations and examples.
- Student’s t -distribution
- Normal distribution
- Null and Alternative Hypotheses
- Chi square tests
- Confidence interval
- Quartiles & Quantiles
- Cluster sampling
- Stratified sampling
- Data cleansing
- Reproducibility vs Replicability
- Peer review
- Prospective cohort study
Research bias
- Implicit bias
- Cognitive bias
- Placebo effect
- Hawthorne effect
- Hindsight bias
- Affect heuristic
- Social desirability bias
In experimental research, random assignment is a way of placing participants from your sample into different groups using randomization. With this method, every member of the sample has a known or equal chance of being placed in a control group or an experimental group.
Random selection, or random sampling , is a way of selecting members of a population for your study’s sample.
In contrast, random assignment is a way of sorting the sample into control and experimental groups.
Random sampling enhances the external validity or generalizability of your results, while random assignment improves the internal validity of your study.
Random assignment is used in experiments with a between-groups or independent measures design. In this research design, there’s usually a control group and one or more experimental groups. Random assignment helps ensure that the groups are comparable.
In general, you should always use random assignment in this type of experimental design when it is ethically possible and makes sense for your study topic.
To implement random assignment , assign a unique number to every member of your study’s sample .
Then, you can use a random number generator or a lottery method to randomly assign each number to a control or experimental group. You can also do so manually, by flipping a coin or rolling a dice to randomly assign participants to groups.
Cite this Scribbr article
If you want to cite this source, you can copy and paste the citation or click the “Cite this Scribbr article” button to automatically add the citation to our free Citation Generator.
Bhandari, P. (2023, June 22). Random Assignment in Experiments | Introduction & Examples. Scribbr. Retrieved April 2, 2024, from https://www.scribbr.com/methodology/random-assignment/
Is this article helpful?
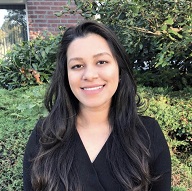
Pritha Bhandari
Other students also liked, guide to experimental design | overview, steps, & examples, confounding variables | definition, examples & controls, control groups and treatment groups | uses & examples, what is your plagiarism score.
Random Team Generator:
How to create randomized groups.
Enter each item on a new line, choose the amount of groups unders settings, and click the button to generate your randomized list. Don't like the first team? Just click again until you do.
Fairly pick teams without bias. No need to draw names out of a hat. No need to do a grade school style draft or put hours of thought into the most balanced teams. The most fair dividing method possible is random.
Mix up your to-do list by generating random groups out of them. For example, enter all your housecleaning activities and split them into seven groups, one for each day or one for each person.
Want something similar?
Use the list randomizer if you don't want separate groups or use the random name picker to pull a single name.
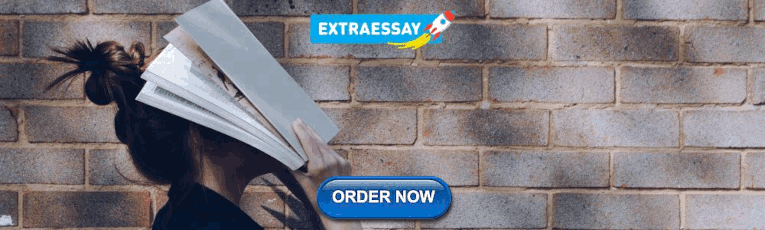
IMAGES
VIDEO
COMMENTS
I'm trying to make a random group generator splitting a list of students into n nearly-equal partitions (for example, if there are 11 students and three groups, we would want two groups of four and one group of 3) . We need to repeat this process for x number of assignments.
The problem is randomly picking a team for each player. As random.randint produces equally distributed values, each player has the same chance of being assigned to any given team, so you can end up with everyone in the same team.. Instead you should consider iterating over the teams and assigning a random player to it.
Let's say we have 15 students and want 5 random groups. For each index aka number in range(5) — (0,1,2,3,4) — we make a group by selecting students[index::5]. Group 1. students[0::5] We select the 0th student in the randomly shuffled list, then jump by 5 to select the 5th person in the list, and then jump by 5 to take the 10th person in ...
PRNGs in Python The random Module. Probably the most widely known tool for generating random data in Python is its random module, which uses the Mersenne Twister PRNG algorithm as its core generator. Earlier, you touched briefly on random.seed(), and now is a good time to see how it works. First, let's build some random data without seeding.
random.shuffle (x [, random]) ¶ Shuffle the sequence x in place.. The optional argument random is a 0-argument function returning a random float in [0.0, 1.0); by default, this is the function random().. To shuffle an immutable sequence and return a new shuffled list, use sample(x, k=len(x)) instead. Note that even for small len(x), the total number of permutations of x can quickly grow ...
PRNG is an acronym for pseudorandom number generator. As you know, using the Python random module, we can generate scalar random numbers and data. Use a NumPy module to generate a multidimensional array of random numbers. NumPy has the numpy.random package has multiple functions to generate the random n-dimensional array for various distributions.
Python Random Module Introduction. Now, let's meet our trusty sidekick for exploring randomness in Python - the Random module! ... to draw conclusions about a larger group (population). Random sampling is like the magician's wand of statistics! 🎩 It ensures that each item in your population has an equal chance of being included in your ...
-Python-Random-Group-Generator. Partial credit goes to the Programming Club at Normandale Community College. This program tested and runs in python 3.2.5. Create/Modefy groups of people based on group size and without allowing even a single member to be in a new group with a member whos he/she has been in a group before.
Use the random.choices () function to select multiple random items from a sequence with repetition. For example, You have a list of names, and you want to choose random four names from it, and it's okay for you if one of the names repeats. A random.choices() function introduced in Python 3.6.
import numpy import random array = numpy.zeros ( (6, 6)) while (array == 1).sum () != 18 or (array == 2).sum () != 18: for i in range (0, 6): for j in range (0, 6): array [i] [j] = random.randint (1, 2) print array. Sure, this works. But this seems to me to be extremely inefficient, as the code is literally having to insert new matrix values ...
events: an integer specifying how many group assignments should be created; group size: an integer specifying how big a group can be at maximum. The function should return a list of group assignments. The following shall apply to these: Each student must appear in a group only once per group assignment. A group assignment may not appear more ...
Major thing which needs to be included is: Participants should randomly be assigned to one of four different groups. The experiment consists of two phases: training phase and generalisation phase. Thus, when a participant was assigned to group 1 in the training phase, they have to be assigned to the same group in the generalisation phase.
Random Group Generator. 1. Group Title. 2. Input names here. Don Key Charity Case Gene Pool Celia Fate Minnie Driver Barb Dwyer Pat Gently Robyn Banks Peg Baskett. 3. Number of groups required:
Below, you'll find a quick recap of the Pandas .groupby() method: The Pandas .groupby() method allows you to aggregate, transform, and filter DataFrames. The method works by using split, transform, and apply operations. You can group data by multiple columns by passing in a list of columns.
To implement random assignment, assign a unique number to every member of your study's sample. Then, you can use a random number generator or a lottery method to randomly assign each number to a control or experimental group. You can also do so manually, by flipping a coin or rolling a dice to randomly assign participants to groups.
Random Assignment in Experiments | Introduction & Examples. Published on March 8, 2021 by Pritha Bhandari.Revised on June 22, 2023. In experimental research, random assignment is a way of placing participants from your sample into different treatment groups using randomization. With simple random assignment, every member of the sample has a known or equal chance of being placed in a control ...
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Group 4. Jesse. Jane. Hank. Holly. Brock Gale Gustavo Hank Hector Holly Jane Jesse Lydia Marie Mike Pete Saul Skyler Todd Walter. Paste your list and we'll randomly separate it into groups. You can specify as many groups as you need. Easily generate random teams or random groups.
Lists are a very nice method of being able to have random answers. You would first go into a ranged for loop: for i in range(3): myList.append(answers['Your question'][random.randint(0, 3)])