- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
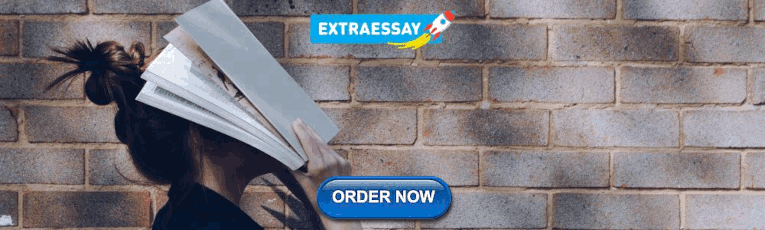
Assignment Operators in Programming
- Operator Associativity in Programming
- C++ Assignment Operator Overloading
- What are Operators in Programming?
- Assignment Operators In C++
- Types of Operators in Programming
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- JavaScript Assignment Operators
- How to Create Custom Assignment Operator in C++?
- Assignment Operators in Python
- Assignment Operators in C
- Compound assignment operators in Java
- Java Assignment Operators with Examples
- When should we write our own assignment operator in C++?
- Parallel Assignment in Ruby
- Self assignment check in assignment operator
- Different Forms of Assignment Statements in Python
- What is the difference between = (Assignment) and == (Equal to) operators
- Rules for operator overloading
- Program to print ASCII Value of a character
- Introduction of Programming Paradigms
- Program for Hexadecimal to Decimal
- Program for Decimal to Octal Conversion
- A Freshers Guide To Programming
- ASCII Vs UNICODE
- How to learn Pattern printing easily?
- Loop Unrolling
- How to Learn Programming?
- Program to Print the Trapezium Pattern
Assignment operators in programming are symbols used to assign values to variables. They offer shorthand notations for performing arithmetic operations and updating variable values in a single step. These operators are fundamental in most programming languages and help streamline code while improving readability.
Table of Content
What are Assignment Operators?
- Types of Assignment Operators
- Assignment Operators in C++
- Assignment Operators in Java
- Assignment Operators in C#
- Assignment Operators in Javascript
- Application of Assignment Operators
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign ( = ), which assigns the value on the right side of the operator to the variable on the left side.
Types of Assignment Operators:
- Simple Assignment Operator ( = )
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
Below is a table summarizing common assignment operators along with their symbols, description, and examples:
Assignment Operators in C:
Here are the implementation of Assignment Operator in C language:
Assignment Operators in C++:
Here are the implementation of Assignment Operator in C++ language:
Assignment Operators in Java:
Here are the implementation of Assignment Operator in java language:
Assignment Operators in Python:
Here are the implementation of Assignment Operator in python language:
Assignment Operators in C#:
Here are the implementation of Assignment Operator in C# language:
Assignment Operators in Javascript:
Here are the implementation of Assignment Operator in javascript language:
Application of Assignment Operators:
- Variable Initialization : Setting initial values to variables during declaration.
- Mathematical Operations : Combining arithmetic operations with assignment to update variable values.
- Loop Control : Updating loop variables to control loop iterations.
- Conditional Statements : Assigning different values based on conditions in conditional statements.
- Function Return Values : Storing the return values of functions in variables.
- Data Manipulation : Assigning values received from user input or retrieved from databases to variables.
Conclusion:
In conclusion, assignment operators in programming are essential tools for assigning values to variables and performing operations in a concise and efficient manner. They allow programmers to manipulate data and control the flow of their programs effectively. Understanding and using assignment operators correctly is fundamental to writing clear, efficient, and maintainable code in various programming languages.
Please Login to comment...
Similar reads.
- Programming
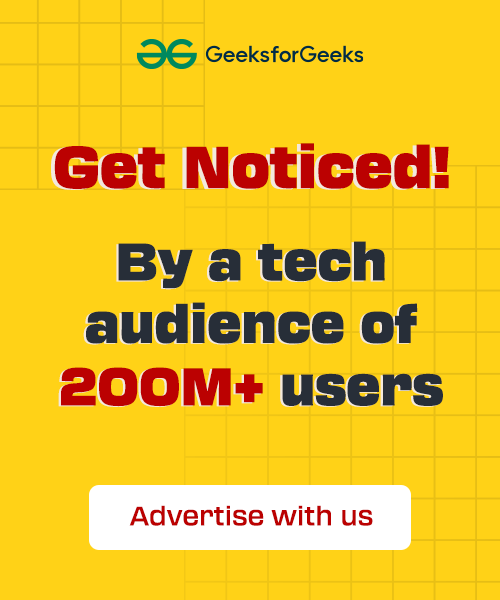
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

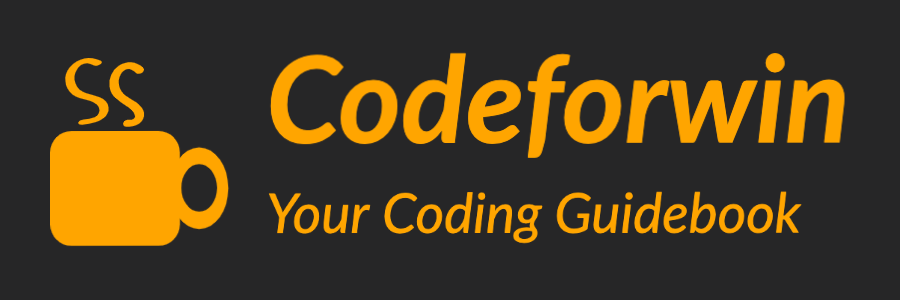
Assignment and shorthand assignment operator in C
Quick links.
- Shorthand assignment
Assignment operator is used to assign value to a variable (memory location). There is a single assignment operator = in C. It evaluates expression on right side of = symbol and assigns evaluated value to left side the variable.
For example consider the below assignment table.
The RHS of assignment operator must be a constant, expression or variable. Whereas LHS must be a variable (valid memory location).
Shorthand assignment operator
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator.
For example, consider following C statements.
The above expression a = a + 2 is equivalent to a += 2 .
Similarly, there are many shorthand assignment operators. Below is a list of shorthand assignment operators in C.

21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
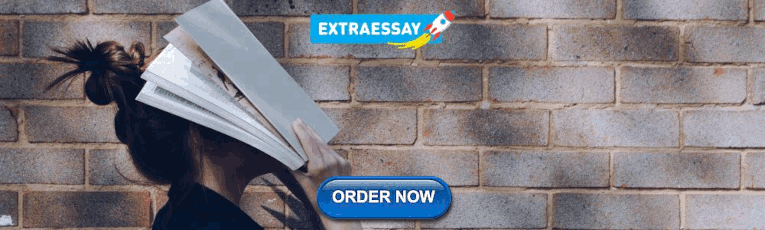
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
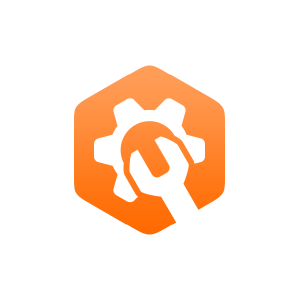
4.4: Arithmetic Assignment Operators
- Last updated
- Save as PDF
- Page ID 11256
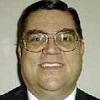
- Kenneth Leroy Busbee
- Houston Community College via OpenStax CNX
Overview of Arithmetic Assignment
The five arithmetic assignment operators are a form of short hand. Various textbooks call them "compound assignment operators" or "combined assignment operators". Their usage can be explaned in terms of the assignment operator and the arithmetic operators. In the table we will use the variable age and you can assume that it is of integer data type.
Demonstration Program in C++
Creating a folder of sub-folder for source code files.
Depending on your compiler/IDE, you should decide where to download and store source code files for processing. Prudence dictates that you create these folders as needed prior to downloading source code files. A suggested sub-folder for the Bloodshed Dev-C++ 5 compiler/IDE might be named:
- Demo_Programs
If you have not done so, please create the folder(s) and/or sub-folder(s) as appropriate.
Download the Demo Program
Download and store the following file(s) to your storage device in the appropriate folder(s). Following the methods of your compiler/IDE, compile and run the program(s). Study the soruce code file(s) in conjunction with other learning materials.
Download from Connexions: Demo_Arithmetic_Assignment.cpp
C++ Tutorial
C++ functions, c++ classes, c++ reference, c++ examples, c++ assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
A Comprehensive Guide to Augmented Assignment Operators in Python
Augmented assignment operators are a vital part of the Python programming language. These operators provide a shortcut for assigning the result of an operation back to a variable in an expressive and efficient manner.
In this comprehensive guide, we will cover the following topics related to augmented assignment operators in Python:
Table of Contents
What are augmented assignment operators, arithmetic augmented assignments, bitwise augmented assignments, sequence augmented assignments, advantages of augmented assignment operators, augmented assignment with mutable types, operator precedence and order of evaluation, updating multiple references, augmented assignment vs normal assignment, comparisons to other languages, best practices and style guide.
Augmented assignment operators are a shorthand technique that combines an arithmetic or bitwise operation with an assignment.
The augmented assignment operator performs the operation on the current value of the variable and assigns the result back to the same variable in a compact syntax.
For example:
Here x += 3 is equivalent to x = x + 3 . The += augmented assignment operator adds the right operand 3 to the current value of x , which is 2 . It then assigns the result 5 back to x .
This shorthand allows you to reduce multiple lines of code into a concise single line expression.
Some key properties of augmented assignment operators in Python:
- Operators act inplace directly modifying the variable’s value
- Work on mutable types like lists, sets, dicts unlike normal operators
- Generally have equivalent compound statement forms using standard operators
- Have right-associative evaluation order unlike arithmetic operators
- Available for arithmetic, bitwise, and sequence operations
Now let’s look at the various augmented assignment operators available in Python.
Augmented Assignment Operators List
Python supports augmented versions of all the arithmetic, bitwise, and sequence assignment operators.
These perform the standard arithmetic operations like addition or exponentiation and assign the result back to the variable.
These allow you to perform bitwise AND, OR, XOR, right shift, and left shift operations combined with assignment.
The += operator can also be used to concatenate sequences like lists, tuples, and strings.
These operators provide a shorthand for sequence concatenation.
Some key advantages of using augmented assignment operators:
Conciseness : Performs an operation and assignment in one concise expression rather than multiple lines or steps.
Readability : The operator itself makes the code’s intention very clear. x += 3 is more readable than x = x + 3 .
Efficiency : Saves executing multiple operations and creates less intermediate objects compared to chaining or sequencing the operations. The variable is modified in-place.
For these reasons, augmented assignments should be preferred over explicit expansion into longer compound statements in most cases.
Some examples of effective usage:
- Incrementing/decrementing variables: index += 1
- Accumulating sums: total += price
- Appending to sequences: names += ["Sarah", "John"]
- Bit masking tasks: bits |= 0b100
So whenever you need to assign the result of some operation back into a variable, consider using the augmented version.
Common Mistakes to Avoid
While augmented assignment operators are very handy, some common mistakes can occur:
Augmented assignments act inplace and modify the existing object. This can be problematic with mutable types like lists:
In contrast, normal operators with immutable types create a new object:
So be careful when using augmented assignments with mutable types, as they modify the object in-place rather than creating a new object.
Augmented assignment operators have right-associativity. This can cause unexpected results:
The right-most operation y += 1 is evaluated first updating y. Then x += y uses the new value of y.
To avoid this, use parenthesis to control order of evaluation:
When you augmented assign to a variable, it updates all references to that object:
y also reflects the change since it points to the same mutable list as x .
To avoid this, reassign the variable rather than using augmented assignment:
While the augmented assignment operators provide a shorthand, they differ from standard assignment in some key ways:
Inplace modification : Augmented assignment acts inplace and modifies the existing variable rather than creating a new object.
Mutable types : Works directly on mutable types like lists, sets, and dicts unlike normal assignment.
Order of evaluation : Has right-associativity unlike left-associativity of normal assignment.
Multiple references : Affects all references to a mutable object unlike normal assignment.
In summary, augmented assignment operators combine both an operation and assignment but evaluate differently than standard operators.
Augmented assignments exist in many other languages like C/C++, Java, JavaScript, Go, Rust, etc. Some key differences to Python:
In C/C++ augmented assignments return the assigned value allowing usage in expressions unlike Python which returns None .
Java and JavaScript don’t allow augmented assignment with strings unlike Python which supports += for concatenation.
Go doesn’t have an increment/decrement operator like ++ and -- . Python’s += 1 and -= 1 serves a similar purpose.
Rust doesn’t allow built-in types like integers to be reassigned with augmented assignment and requires mutable variables be defined with mut .
So while augmented assignment is common across languages, Python provides some unique behaviors to be aware of.
Here are some best practices when using augmented assignments in Python:
Use whitespace around the operators: x += 1 rather than x+=1 for readability.
Limit chaining augmented assignments like x = y = 0 . Use temporary variables if needed for clarity.
Don’t overuse augmented assignment especially with mutable types. Reassignment may be better if the original object shouldn’t be changed.
Watch the order of evaluation with multiple augmented assignments on one line due to right-associativity.
Consider parentheses for explicit order of evaluation: x += (y + z) rather than relying on precedence.
For increments/decrements, prefer += 1 and -= 1 rather than x = x + 1 and x = x - 1 .
Use normal assignment for updating multiple references to avoid accidental mutation.
Following PEP 8 style, augmented assignments should have the same spacing and syntax as normal assignment operators. Just be mindful of potential pitfalls.
Augmented assignment operators provide a compact yet expressive shorthand for modifying variables in Python. They combine an operation and assignment into one atomic expression.
Key takeaways:
Augmented operators perform inplace modification and behave differently than standard operators in some cases.
Know the full list of arithmetic, bitwise, and sequence augmented assignment operators.
Use augmented assignment to write concise and efficient updates to variables and sequences.
Be mindful of right-associativity order of evaluation and behavior with mutable types to avoid bugs.
I hope this guide gives you a comprehensive understanding of augmented assignment in Python. Use these operators appropriately to write clean, idiomatic Python code.
- python
- variables-and-operators
ADVERTISEMENT
Coding Essentials Guidebook for Developers
This book covers core coding concepts and tools. It contains chapters on computer architecture, the Internet, Command Line, HTML, CSS, JavaScript, Python, Java, SQL, Git, and more.
Learn more!
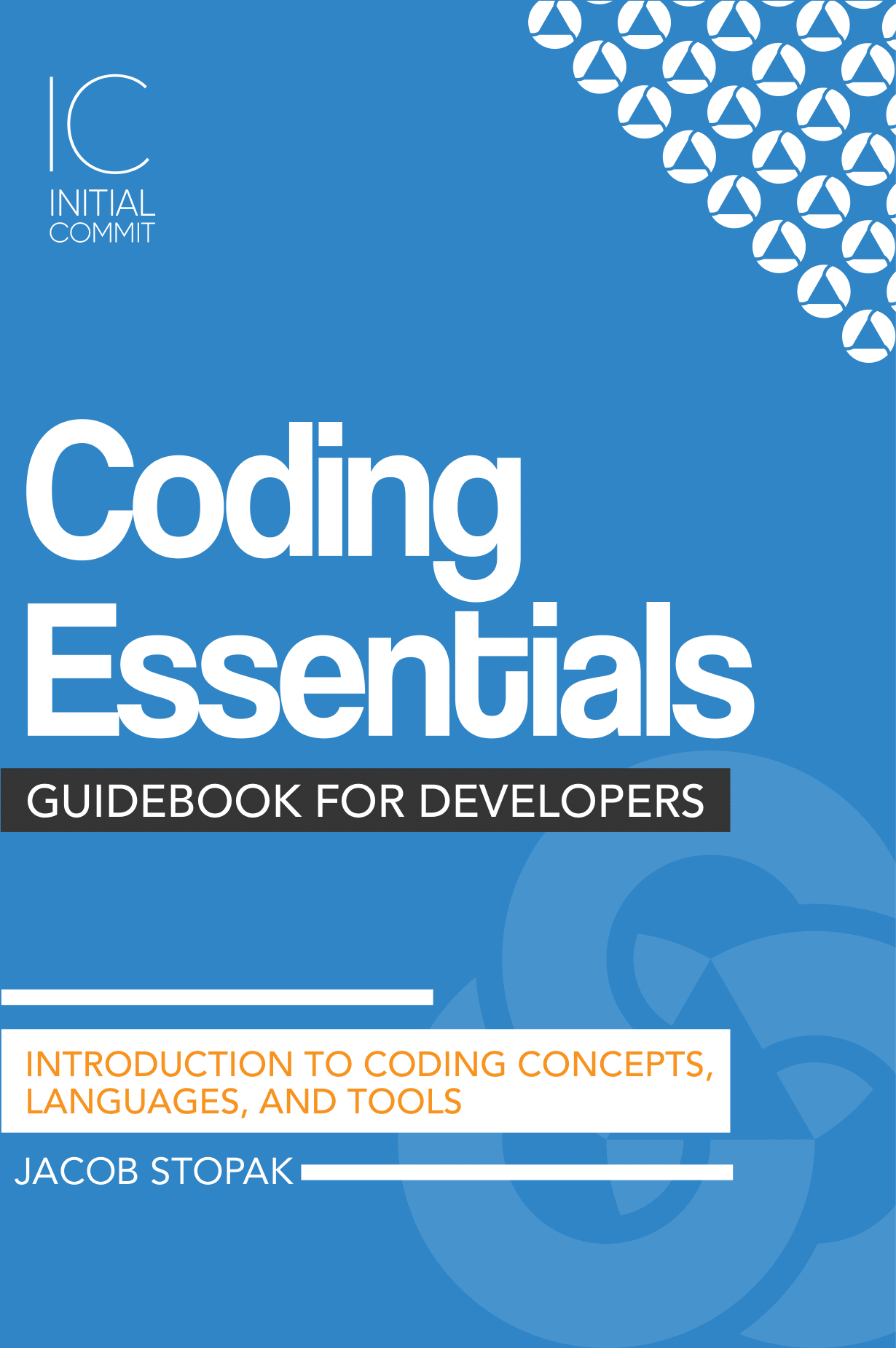
Decoding Git Guidebook for Developers
This book dives into the initial commit of Git's C code in detail to help developers learn what makes Git tick. If you're curious how Git works under the hood, you'll enjoy this.
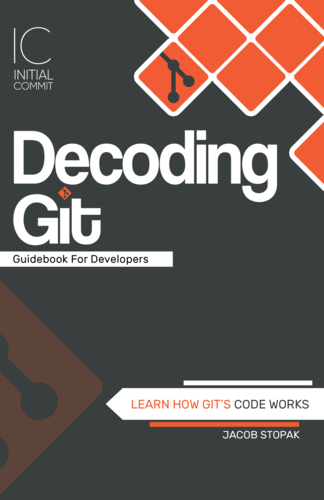
Decoding Bitcoin Guidebook for Developers
This book dives into the initial commit of Bitcoin's C++ code. The book strives to unearth and simplify the concepts that underpin the Bitcoin software system, so that beginner and intermediate developers can understand how it works.
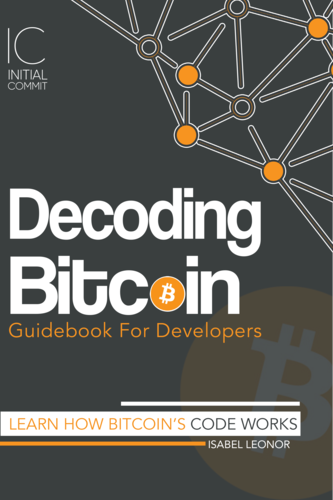
Subscribe to be notified when we release new content and features!
Follow us on your favorite channels!
Assignment Operators in Python
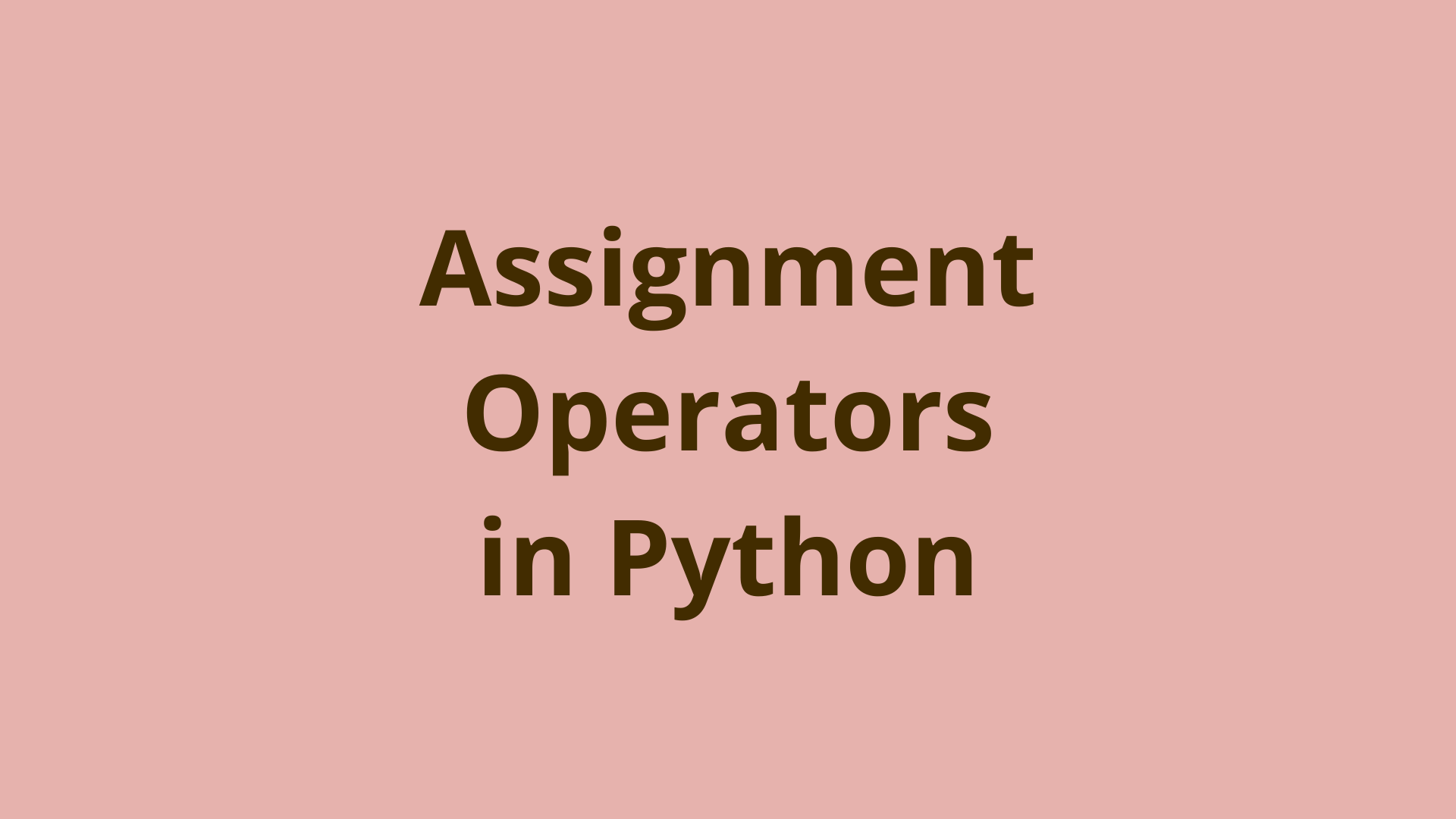
Table of Contents
Introduction, what are the assignment operators and why would you use them in python, how do you use the assignment operators in python and what are the benefits of doing so, what are the benefits of using the assignment operators in python, what are the potential drawbacks of using the assignment operators in python, when is it best to use the assignment operators in python, when is it not recommended to use the assignments operators in python, is there ++ in python, why isn't there ++ and -- in python.
Whether you are an old hand or you are just learning to program , assignment operators are a useful tool to add to your programming arsenal. Assignment operators are often used when you want to make a variable change by a specific amount in shorthand.
In this blog post, we will discuss what the assignment operators are, how to use them in Python, and the benefits and drawbacks of doing so. We will also explore when it is best to use assignment operators in Python, and when it may not be the best option. Finally, we will answer the question of whether or not the incrementing operator ++ is available in Python.
An assignment operator is a symbol that you use in Python to indicate that you want to perform some action and then assign the value to a . You would use an addition or subtraction operator in Python when you want to calculate the result of adding or subtracting two numbers. There are two types of addition operators: the plus sign + and the asterisk * . There are also two types of subtraction operators: the minus sign - and the forward slash / .
The plus sign + is used to indicate that you want to add two numbers together. The asterisk * is used to indicate that you want to multiply two numbers together. The minus sign - is used to indicate that you want to subtract two numbers. The forward slash / is used to indicate that you want to divide two numbers.
Here are some examples of how to use the addition and subtraction operators in Python:
The += operator is used to add two numbers together. The result of the addition will be stored in the variable that is on the left side of the += symbol. In this example, the result of adding y (15) to x (25) is stored in the variable x .
The -= operator is used to subtract one number from another. The result of the subtraction will be stored in the variable that is on the left side of the -= symbol. In this example, the result of subtracting y (15) from x (25) is stored in the variable x.
These operators are convenient ways to add or subtract values from a variable without having to use an intermediate variable. For example, if you want to add two numbers together and store the result in a new variable, you would write:
first_number = first_number + second_number
However, if you want to use the += operator instead, you can simply write:
first_number += second_number
This will save you a few keystrokes and also make your code more readable.
The -= operator is similarly useful for subtracting values from a variable. For example:
first_number -= second_number
This will subtract the second_number from the variable first_number .
One potential drawback to using the += and -= assignment operators in Python is that they can be confusing for beginners.
Another potential drawback is that they can lead to code that is difficult to read and understand.
However, these drawbacks are outweighed by the benefits of using these operators, which include increased readability and brevity of code. For these reasons, I recommend using the += , -= , *= , and /= assignment operators in Python whenever possible.
The increment operator is best used when you want to increment or decrement a variable by a number and you want to eliminate some keystrokes or create some brevity in your code.
In addition, it can also be used with other operators, such as the multiplication operator * . The following example shows how to use the increment operator with the multiplication operator:
This will print out the value of x as "120".
You may consider not using the assignment operators when you are dealing with a team of new programmers who may find the syntax confusing.
There are ways to mitigate this though, with training, lunch and learns, and more you can bring up the developers to the place where using some of the intricacies of Python.
No, the ++ (and -- ) operators available in C and other languages were not brought over to Python.
If you attempt to use ++ and -- the way they are used in C you will not get the results you are expecting. Use the += and -= operators.
Python doesn't have a ++ operator because it can be easily replaced with the += operator. Having both was probably thought to create more confusion.
There's a certain amount of ambiguity in the operator to the language parser. It could interpret the ++ in Python as two unary operators (+ and +) or it could be one unary operator (++).
Lastly, it can cause confusing side effects and Python likes to eliminate edge cases when interpreting the code. Look up C precedence issues of pre and post incrementation if you want to know more. It's not fun.
The += and -= assignment operators in Python are convenient ways to add or subtract values from a variable without having to use an intermediate variable. They are also more readable than traditional methods of adding or subtracting values. For these reasons, I recommend using the += and -= assignment operators whenever possible. You can learn more in the Python documentation . Start using these powerful tools today!
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers , where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to [email protected] .
Final Notes
Recommended product: Coding Essentials Guidebook for Developers
Related Articles
Python zfill method.
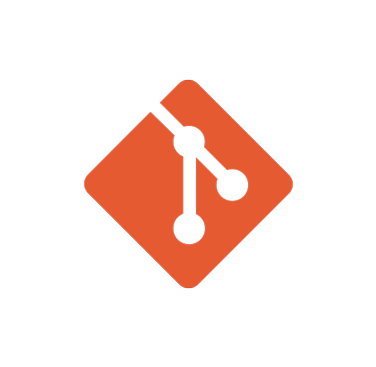
Python Yield vs Return
Python zip two lists, using raw_input() in python 3, python list print, numpy around() in python, get the first 5 chapters of our coding essentials guidebook for developers free , the programming guide i wish i had when i started learning to code... 🚀👨💻📚.
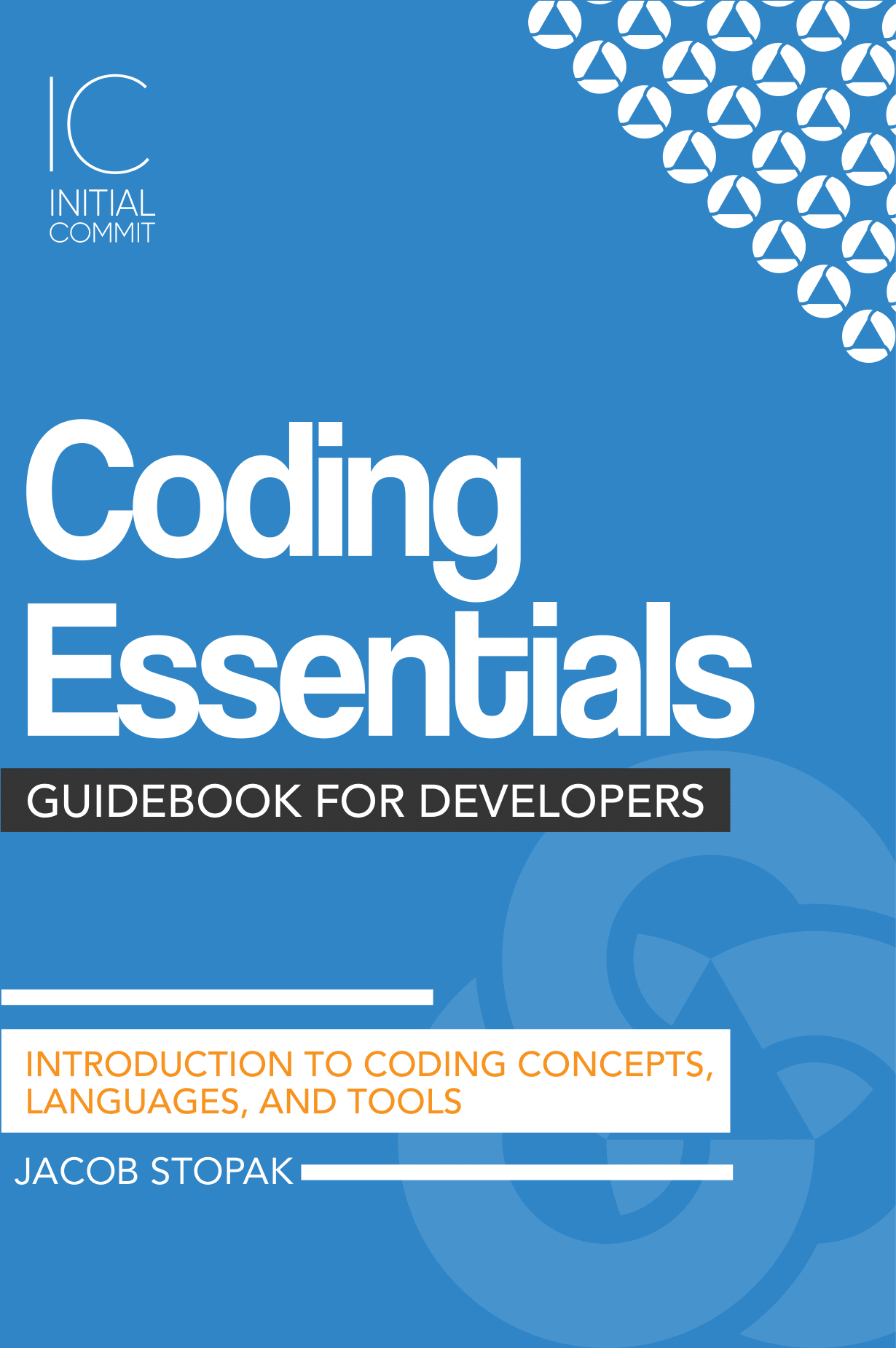
Check out our Coding Essentials Guidebook for Developers
- C Programming
- Java Programming
- Data Structures
- Web Development
- Tech Interview
Java's Basic and Shorthand Assignment Operators
Reference assignment, primitive assignment, primitive casting, shorthand assignment operators, basic assignment operator.
Java's basic assignment operator is a single equals-to (=) sign that assigns the right-hand side value to the left-hand side operand. On left side of the assignment operator there must be a variable that can hold the value assigned to it. Assignment operator operates on both primitive and reference types. It has the following syntax: var = expression; Note that the type of var must be compatible with the type of expression .
Chaining of assignment operator is also possible where we can create a chain of assignments in order to assign a single value to multiple variables. For example,
The above piece of code sets the variables x, y, and z to 100 using a single statement. This works because the = is an operator that yields the value of the right-hand expression. Thus, the value of z = 100 is 100, which is then assigned to y , which in turn is assigned to x . Using a "chain of assignment" is an easy way to set a group of variables to a common value.
A variable referring to an object is a reference variable not an object. We can assign a newly created object to an object reference variable as follows:
The above line of code performs three tasks
- Creates a reference variable named st1 , of type Student .
- Creates a new Student object on the heap.
- Assigns the newly created Student object to the reference variable st1
We can also assign null to an object reference variable, which simply means the variable is not referring to any object:
Above line of code creates space for the Student reference variable st2 but doesn't create an actual Student object.
Note that, we can also use a reference variable to refer to any object that is a subclass of the declared reference variable type.
Primitive variables can be assigned either by using a literal or the result of an expression. For example,
The most important point that we should keep in mind, while assigning primitive types is if we are going to assign a bigger value to a smaller type, we have to typecast it properly. In Above piece of code the literal integer 130 is implicitly an int but it is acceptable here because the variable x is of type int . It gets weird if you try 130 to assign to a byte variable. For example, The below piece of code will not compile because literal 130 is implicitly an integer and it does not fit into a smaller type byte x . Likewise, byte c = a + b will also not compile because the result of an expression involving anything int-sized or smaller is always an int .
Casting lets us convert primitive values from one type to another (specially from bigger to smaller types). Casts can be implicit or explicit. An implicit cast means we don't have to write code for the cast; the conversion happens automatically. Typically, an implicit cast happens when we do a widening conversion. In other words, putting a smaller thing (say, a byte ) into a bigger container (like an int ). Remember those "possible loss of precision" compiler errors we saw during assignments. Those happened when we tried to put a larger thing (say, an int ) into a smaller container (like a byte ). The large-value-into-small-container conversion is referred to as narrowing and requires an explicit cast, where we tell the compiler that we are aware of the danger and accept full responsibility.
As a final note on casting, it is very important to note that the shorthand assignment operators let us perform addition, subtraction, multiplication or division without putting in an explicit cast. In fact, +=, -=, *=, and /= will all put in an implicit cast. Below is an example:
In addition to the basic assignment operator, Java also defines 12 shorthand assignment operators that combine assignment with the 5 arithmetic operators ( += , -= , *= , /= , %= ) and the 6 bitwise and shift operators ( &= , |= , ^= , <<= , >>= , >>>= ). For example, the += operator reads the value of the left variable, adds the value of the right operand to it, stores the sum back into the left variable as a side effect, and returns the sum as the value of the expression. Thus, the expression x += 2 is almost the same x = x + 2 .
The difference between these two expressions is that when we use the += operator, the left operand is evaluated only once. This makes a difference when that operand has a side effect. Consider the following two expressions a[i++] += 2; and a[i++] = a[i++] + 2; , which are not equivalent:
In this tutorial we discussed basic and shorthand (compound) assignment operators of Java. Hope you have enjoyed reading this tutorial. Please do write us if you have any suggestion/comment or come across any error on this page. Thanks for reading!
- Core Java Volume I - Fundamentals
- Java: The Complete Reference, Seventh Edition
- Operators: Sun tutorial
- Bit Twiddling Hacks
- C Programming Tutorials
- Java Programming Tutorials
- Data Structures Tutorials
- All Tech Interview Questions
- C Interview Question Answers
- Java Interview Question Answers
- DSA Interview Question Answers
Get Free Tutorials by Email
About the Author
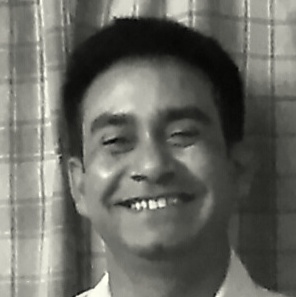
Krishan Kumar is the founder and main contributor for cs-fundamentals.com. He is a software professional (post graduated from BITS-Pilani) and loves writing technical articles on programming and data structures.
Today's Tech News
- Write For Us
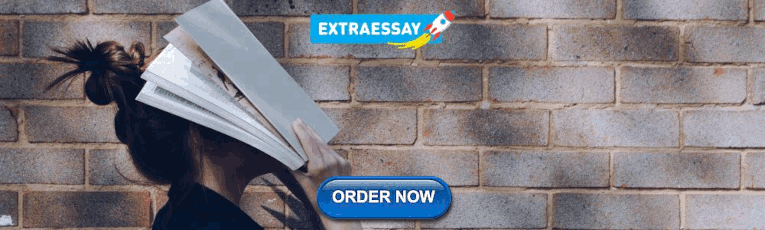
IMAGES
VIDEO
COMMENTS
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
@MSharathHegde If the compound assignment operator were spelled "=*", there might be some ambiguity here, but since it is in fact spelled "*=", ... Advantage of using compound assignment. There is a disadvantage too. Consider the effect of types. long long exp1 = 20; int b=INT_MAX; // All additions use `long long` math exp1 = exp1 + 10 + b; ...
C supports a short variant of assignment operator called compound assignment or shorthand assignment. Shorthand assignment operator combines one of the arithmetic or bitwise operators with assignment operator. For example, consider following C statements. The above expression a = a + 2 is equivalent to a += 2.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Discussion. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
Assignment Operator. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly ...
The five arithmetic assignment operators are a form of short hand. Various textbooks call them "compound assignment operators" or "combined assignment operators". Their usage can be explaned in terms of the assignment operator and the arithmetic operators. In the table we will use the variable age and you can assume that it is of integer data type.
Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator ( =) to assign the value 10 to a variable called x:
Advantages of Augmented Assignment Operators. Some key advantages of using augmented assignment operators: Conciseness: Performs an operation and assignment in one concise expression rather than multiple lines or steps. Readability: The operator itself makes the code's intention very clear. x += 3 is more readable than x = x + 3.
An assignment operator is a symbol that you use in Python to indicate that you want to perform some action and then assign the value to a . You would use an addition or subtraction operator in Python when you want to calculate the result of adding or subtracting two numbers. There are two types of addition operators: the plus sign + and the ...
The above piece of code sets the variables x, y, and z to 100 using a single statement. This works because the = is an operator that yields the value of the right-hand expression. Thus, the value of z = 100 is 100, which is then assigned to y, which in turn is assigned to x.Using a "chain of assignment" is an easy way to set a group of variables to a common value.
These operators are a convenient way to make your code more concise and readable. Compound assignment operators of Java are particularly useful when you want to modify a variable's value by a specific amount or using a specific operation. Suggested Java Concepts:-Java Constants Operators in Java. Java Arithmetic Operators. Bitwise Operators in Java
For a += 4 javac can produce IIC instruction which does Increment local variable by constant. It is theoretically more efficient then IADD. IADD does Add int with popping up two values from stack then pushing back the result. IIC does nothing on stack but increments local variable. So if you may work on a very limited and primitive JVM like you ...
When looking at some code on one or another git, sometimes I can see that devs use bitwise inclusive OR compound assignment operator (|=) where simple assignment would be enough. Unfortunately, I don't have any code with this solution at hand, so I'll try to describe it as best I can.