- Collections
- Dart Interview Questions
- Kotlin Android
- Android with Java
- Android Studio
- Dart Tutorial
- Introduction to Dart Programming Language
- Dart SDK Installation
- Dart - Comments
- Dart - Variables
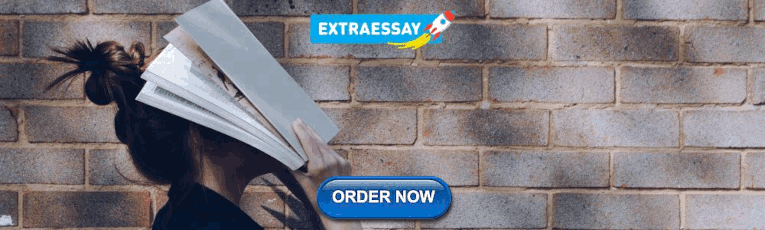
Operators in Dart
- Dart - Standard Input Output
- Dart - Data Types
- Basics of Numbers in Dart
- Strings in Dart
- Dart - Sets
- Dart Programming - Map
- Queues in Dart
- Data Enumeration in Dart
Control Flow
- Switch Case in Dart
- Dart - Loops
- Dart - Loop Control Statements (Break and Continue)
- Labels in Dart
Key Functions
- Dart - Anonymous Functions
- Dart - main() Function
- Dart - Common Collection Methods
- How to Exit a Dart Application Unconditionally?
- Dart - Getters and Setters
- Dart - Classes And Objects
Object-Oriented Programming
- Dart - this keyword
- Dart - Static Keyword
- Dart - Super and This keyword
- Dart - Concept of Inheritance
- Instance and class methods in Dart
- Method Overriding in Dart
- Getter and Setter Methods in Dart
- Abstract Classes in Dart
- Dart - Builder Class
- Concept of Callable Classes in Dart
- Interface in Dart
- Dart - extends Vs with Vs implements
- Dart - Date and Time
- Using await async in Dart
Dart Utilities
- How to Combine Lists in Dart?
- Dart - Finding Minimum and Maximum Value in a List
- Dart - Splitting of String
Dart Programs
- Dart - Sort a List
- Dart - String toUpperCase() Function with Examples
- Dart - Convert All Characters of a String in Lowercase
- How to Replace a Substring of a String in Dart?
- How to Check String is Empty or Not in Dart (Null Safety)?
- Exception Handling in Dart
- Assert Statements in Dart
- Fallthrough Condition in Dart
- Concept of Isolates in Dart
Advance Concepts
- Dart - Collections
- Dart - Basics of Packages
- Dart - String codeUnits Property
- HTML Document Object Model and Dart Programming
The operators are special symbols that are used to carry out certain operations on the operands. The Dart has numerous built-in operators which can be used to carry out different functions, for example, ‘+’ is used to add two operands. Operators are meant to carry operations on one or two operands.Ā
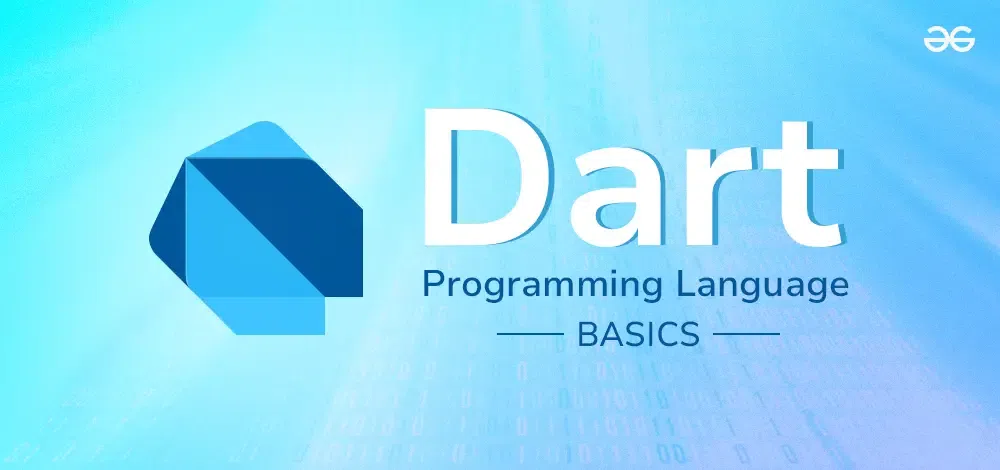
Precedence Table of Operators in Dart
Different types of operators in dart.
The following are the various types of operators in Dart:
- Arithmetic Operators
- Relational Operators
- Type Test Operators
- Bitwise Operators
- Assignment Operators
- Logical Operators
- Conditional Operators
- Cascade Notation Operators:
1. Arithmetic Operators
This class of operators contain those operators which are used to perform arithmetic operation on the operands. They are binary operators i.e they act on two operands. They go like this:Ā
Example showing the use of all Arithmetic Operator:
2. relational operators.
This class of operators contain those operators which are used to perform relational operation on the operands. It goes like this:Ā
Example showing the use of Relational Operators: Ā
Note: == operator can’t be used to check if the object is same. So, to check if the object are same we use identical() function.
3. Type Test Operators
This class of operators contain those operators which are used to perform comparison on the operands. It goes like this:Ā
Example: Using Type Test Operators in the program Ā
as Operator
as Operator is used for Typecasting. It performs a cast at runtime if the cast is valid else, it throws an error. It is of two types Downcasting and Type Assertion.
Below is the implementation of as operator:
4. Bitwise Operators
This class of operators contain those operators which are used to perform bitwise operation on the operands. It goes like this:Ā
Example: Using Bitwise Operators in the program Ā
5. Assignment Operators
This class of operators contain those operators which are used to assign value to the operands. It goes like this:Ā
Example: Using Assignment Operators in the program Ā
Compound Assignment Operator
Apart from there is another way where we can use a operator that is compound assignment operator where we combine an operator with an assignment operatorso to shorten the steps and make code more effective.
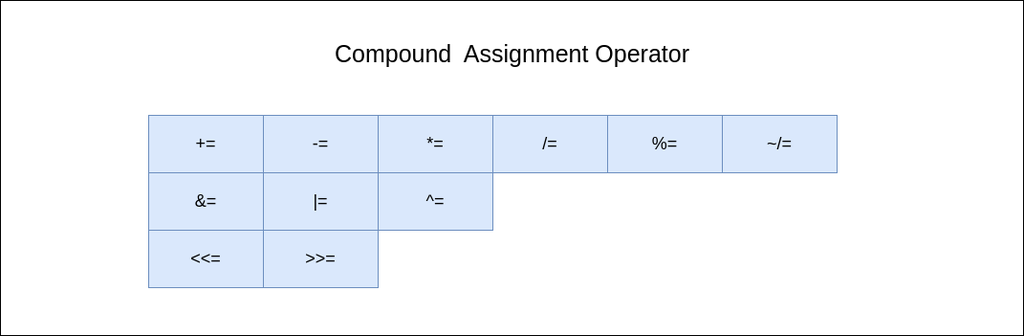
6. Logical Operators
This class of operators contain those operators which are used to logically combine two or more conditions of the operands. It goes like this:Ā
Example 1: Using Logical Operators in the program Ā
Note: Logical operator can only be application to boolean expression and in dart, non-zero numbers are not considered as true and zero as false
Example 2: (Incorrect Way)
Example 3: (correct way), 7. conditional operators, example: using conditional operators in the program Ā .
Note: Also In The Above Code,You May Notice That The Variable ‘n’ is Declared As “int? n”.By declaring n as int?, you are indicating that the variable n can hold an integer value or a null value. The ? denotes that the variable is nullable, meaning it can be assigned a null value in addition to integer values.
8. Cascade Notation Operators:
This class of operators allows you to perform a sequence of operation on the same element. It allows you to perform multiple methods on the same object. It goes like this:Ā
Example: Using Cascade Notation Operators in the program Ā
To know more about dart please check dart tutorial, please login to comment..., similar reads.
- Dart-basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- React Native
Conditional (Ternary) Operator in Dart and Flutter
( 91 Articles )

March 6, 2024
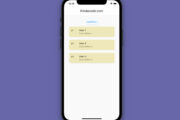
September 15, 2023

February 14, 2023

February 3, 2023

April 19, 2023

February 15, 2023

April 27, 2023
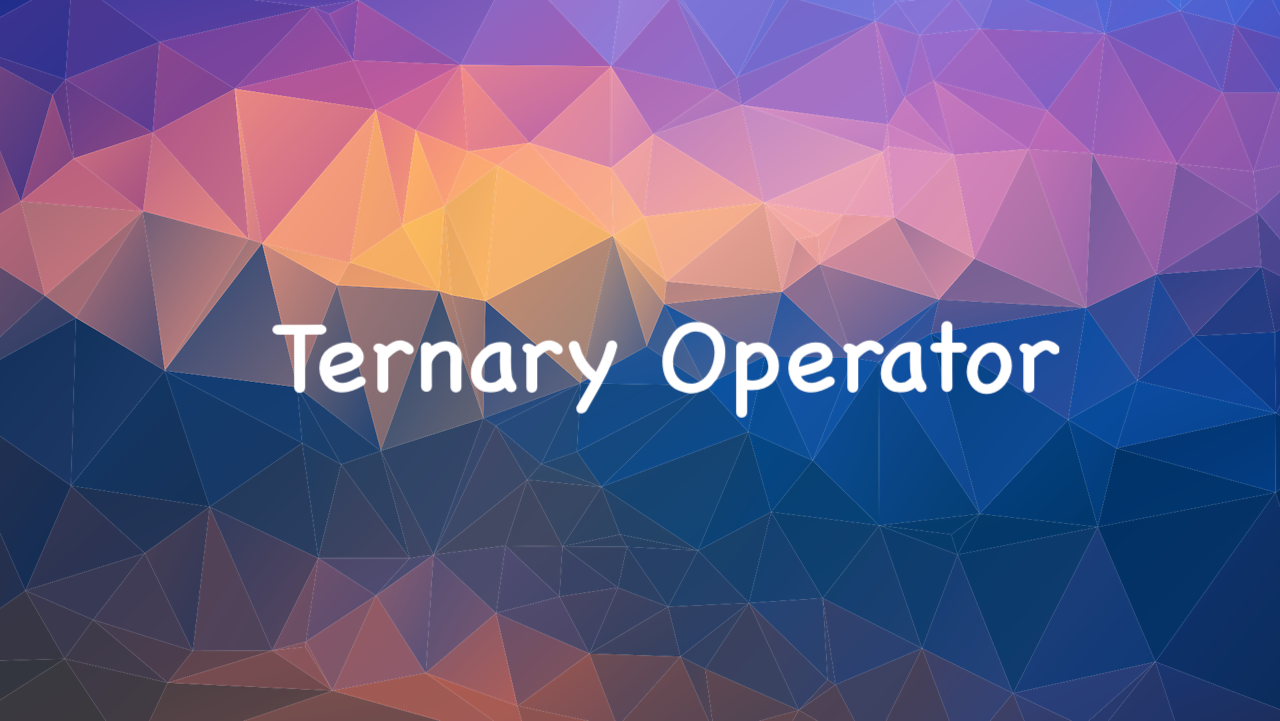
This article is a deep dive into conditional (ternary) operators in Dart and Flutter.
The conditional (ternary) operator is just a Dart operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy .
The syntax:
- condition : An expression whose value is used as a condition.
- exprIfTrue : An expression that is evaluated if the condition evaluates to a truthy value (one which equals or can be converted to true ).
- exprIfFalse : An expression that is executed if the condition is falsy (that is, has a value that can be converted to false ).
Conditional chains
The ternary operator is right-associative, which means it can be āchainedā to check multiple conditions in turn.
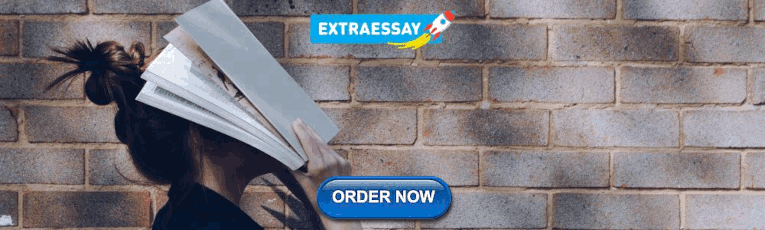
Null Checking Expression
This expression uses a double-question mark and can be used to test for null .
If expr1 is non-null, returns its value ; otherwise, evaluates and returns the value of expr2 .
Ternary operator vs If-else statement
The conditional expression condition ? expr1: expr2 has a value of expr1 or expr2 while an if-else statement has no value. A statement often contains one or more expressions, but an expression canāt directly contain a statement.
There are some things that you can do with the ternary operator but canāt do with if-else. For example, if you need to initialize a constant or reference:
Writing Concise Code
In the vast majority of cases, you can do the same thing with a ternary operator and an if-else statement. However, using ? : helps us avoid redundantly repeating other parts of the same statements/function-calls, for example:
Compare with:
Youāve learned the fundamentals of using conditional expressions when programming in Dart. Continue exploring more new and interesting stuff by taking a look at the following articles:
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter and Firestore Database: CRUD example
- Flutter & Dart: Get File Name and Extension from Path/URL
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- How to Create a Countdown Timer in Flutter
- Flutter: Making a Tic Tac Toe Game from Scratch
You can also take a tour around our Flutter topic page , or Dart topic page for the latest tutorials and examples.
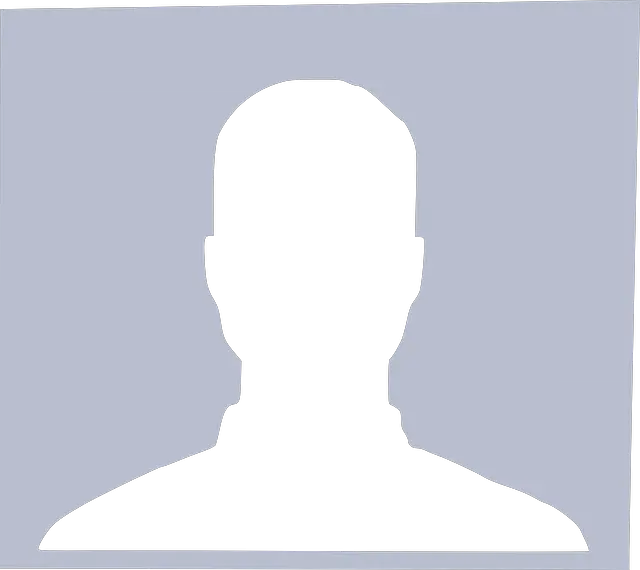
Related Articles

Dart: 2 Ways to Convert an Object to JSON
September 17, 2023

Dart Map.putIfAbsent() method (explained with examples)
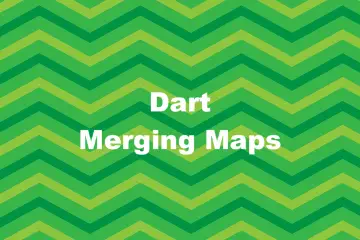
How to Merge 2 Maps in Dart (3 Approaches)
September 16, 2023

Dart: 2 Ways to Find Common Elements of 2 Lists

Dart: Remove Consecutive White Spaces from a String (3 Ways)
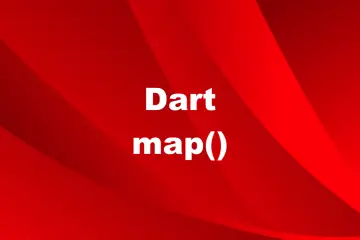
Dart map() Method: Explained with Examples

Dart: How to Convert a List/Map into Bytes
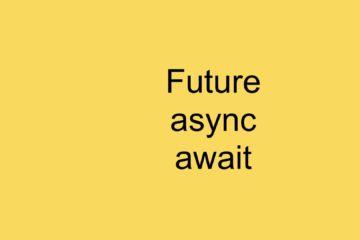
Examples of using Future, async, await in Flutter
September 14, 2023
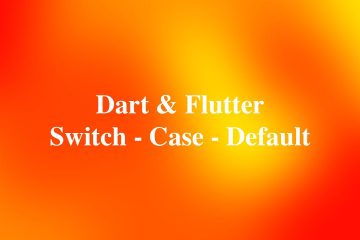
Using Switch-Case-Default in Dart & Flutter (4 Examples)
September 13, 2023
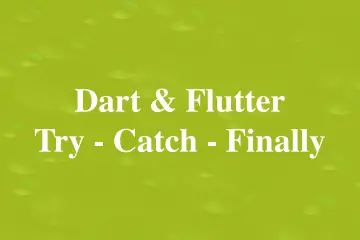
Using Try-Catch-Finally in Dart (3 examples)

6 Ways to iterate through a list in Dart and Flutter
September 11, 2023
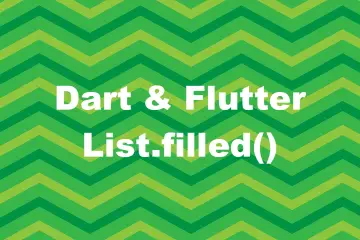
Using List.filled() in Dart and Flutter (with examples)
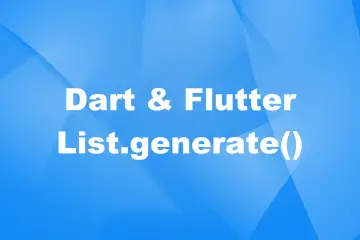
Using List.generate() in Flutter and Dart (4 examples)
September 10, 2023

Flutter: How to Fully Print a Long String/List/Map
September 1, 2023
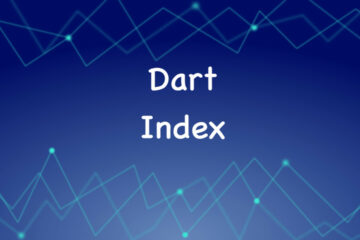
Dart & Flutter: Get the Index of a Specific Element in a List
August 24, 2023

Dart & Flutter: 2 Ways to Count Words in a String
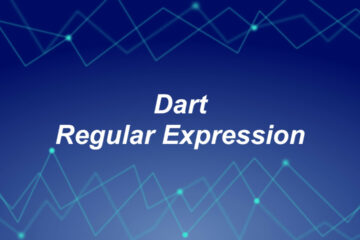
Flutter & Dart: Regular Expression Examples
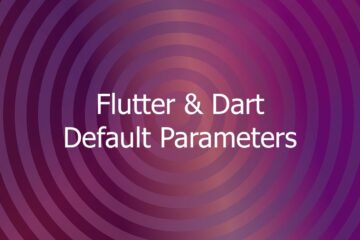
Set Default Parameter Values for a Function in Flutter
August 19, 2023


Episode 3: Exploring Dart: Operators
Season 2: exploring dart #dartforbeginners.

Dart, a powerful programming language, brings to the table a plethora of operators that serve different purposes. This article aims to explore these operators, delineating their associativity and precedence, and providing practical examples of their use.
Dart's operators can be grouped into various categories including arithmetic, relational, logical, bitwise, shift, conditional, cascade, and more. Let's delve into each of these categories to understand their functionality.
Thanks for reading Flutter Explained! Subscribe for free to receive new posts and support my work.
1. Unary Operators
These are operators that act on a single operand. They can be divided into:
a. Unary Postfix:
Associativity: None
b. Unary Prefix:
2. arithmetic operators.
These operators are used for mathematical operations. They include:
a. Multiplicative:
Associativity: Left
b. Additive:
Here's an example of arithmetic operators in action:
3. Shift Operators
These operators shift the bits of their operand.
4. Bitwise Operators
Used to manipulate individual bits:
5. Relational and Type Test Operators
For comparison and type checks:
6. Equality Operators
These are for equality checks:
7. Logical Operators
Logical AND and OR:
8. Conditional Operators
These include the standard ternary operator:
Associativity: Right
9. Cascade Notation
A way to make a sequence of operations on the same object:
10. Assignment Operators
These include standard and compound assignments:
Special Considerations
The above table should be used as a guide, but for authoritative behavior, consult Dart's language specification.
Precedence Example
In Dart, higher-precedence operators execute before lower-precedence ones:
Type Test Operators
as , is , and is! operators are handy for checking types:
Cascade Notation
Cascades allow a sequence of operations on the same object:
Other Operators
Other operators like function application () , subscript access [] , conditional subscript access ?[] , and member access . are essential as well.
Dart offers an extensive set of operators, each with a distinct purpose. The above guide is intended to serve as a comprehensive reference for Dart developers seeking to understand and implement these operators effectively.
By familiarizing themselves with the syntax and usage of these operators, developers can write concise and efficient code. The Dart language specification should be referred to for the most authoritative and detailed information on this subject.

Ready for more?
Google uses cookies to deliver its services, to personalize ads, and to analyze traffic. You can adjust your privacy controls anytime in your Google settings . Learn more .
Dart 3.4 is live! Check out the blog post .
- List of Dart codelabs
- Language cheatsheet
- Iterable collections
- Asynchronous programming
- Introduction
- Libraries & imports
- Built-in types
- Collections
- Type system
- Overview & usage
- Pattern types
- Error handling
- Constructors
- Extend a class
- Extension methods
- Extension types
- Callable objects
- Class modifiers for API maintainers
- Asynchronous support
- Sound null safety
- Migrating to null safety
- Understanding null safety
- Unsound null safety
- dart:convert
- Using streams
- Creating streams
- Futures and error handling
- Documentation
- How to use packages
- Commonly used packages
- Creating packages
- Publishing packages
- Writing package pages
- Dependencies
- Package layout conventions
- Pub environment variables
- Pubspec file
- Troubleshooting pub
- Verified publishers
- Security advisories
- Futures, async, await
- Number representation
- Google APIs
- Multi-platform apps
- Get started
- Write command-line apps
- Fetch data from the internet
- Write HTTP servers
- Libraries & packages
- Google Cloud
- Wasm compilation
- Environment declarations
- Objective-C & Swift interop
- Java & Kotlin interop
- Past JS interop
- Web interop
- IntelliJ & Android Studio
- Dart DevTools
- Troubleshooting DartPad
- dart analyze
- dart compile
- dart create
- dart format
- dartaotruntime
- Experiment flags
- build_runner
- Customizing static analysis
- Fixing common type problems
- Fixing type promotion failures
- Linter rules
- Diagnostic messages
- Debugging web apps
- What not to commit
- Breaking changes
- Language evolution
- Language specification
- Dart 3 migration guide
- JavaScript to Dart
- Swift to Dart
- API reference
- DartPad (online editor)
- Package site
Hello World
Control flow statements, inheritance, interfaces and abstract classes, important concepts, additional resources, introduction to dart.
This page provides a brief introduction to the Dart language through samples of its main features.
To learn more about the Dart language, visit the in-depth, individual topic pages listed under Language in the left side menu.
For coverage of Dart's core libraries, check out the core library documentation . You can also try the Dart cheatsheet codelab , for a more hands-on introduction.
Every app requires the top-level main() function, where execution starts. Functions that don't explicitly return a value have the void return type. To display text on the console, you can use the top-level print() function:
Read more about the main() function in Dart, including optional parameters for command-line arguments.
Even in type-safe Dart code, you can declare most variables without explicitly specifying their type using var . Thanks to type inference, these variables' types are determined by their initial values:
Read more about variables in Dart, including default values, the final and const keywords, and static types.
Dart supports the usual control flow statements:
Read more about control flow statements in Dart, including break and continue , switch and case , and assert .
We recommend specifying the types of each function's arguments and return value:
A shorthand => ( arrow ) syntax is handy for functions that contain a single statement. This syntax is especially useful when passing anonymous functions as arguments:
Besides showing an anonymous function (the argument to where() ), this code shows that you can use a function as an argument: the top-level print() function is an argument to forEach() .
Read more about functions in Dart, including optional parameters, default parameter values, and lexical scope.
Dart comments usually start with // .
Read more about comments in Dart, including how the documentation tooling works.
To access APIs defined in other libraries, use import .
Read more about libraries and visibility in Dart, including library prefixes, show and hide , and lazy loading through the deferred keyword.
Here's an example of a class with three properties, two constructors, and a method. One of the properties can't be set directly, so it's defined using a getter method (instead of a variable). The method uses string interpolation to print variables' string equivalents inside of string literals.
Read more about strings, including string interpolation, literals, expressions, and the toString() method.
You might use the Spacecraft class like this:
Read more about classes in Dart, including initializer lists, optional new and const , redirecting constructors, factory constructors, getters, setters, and much more.
Enums are a way of enumerating a predefined set of values or instances in a way which ensures that there cannot be any other instances of that type.
Here is an example of a simple enum that defines a simple list of predefined planet types:
Here is an example of an enhanced enum declaration of a class describing planets, with a defined set of constant instances, namely the planets of our own solar system.
You might use the Planet enum like this:
Read more about enums in Dart, including enhanced enum requirements, automatically introduced properties, accessing enumerated value names, switch statement support, and much more.
Dart has single inheritance.
Read more about extending classes, the optional @override annotation, and more.
Mixins are a way of reusing code in multiple class hierarchies. The following is a mixin declaration:
To add a mixin's capabilities to a class, just extend the class with the mixin.
PilotedCraft now has the astronauts field as well as the describeCrew() method.
Read more about mixins.
All classes implicitly define an interface. Therefore, you can implement any class.
Read more about implicit interfaces , or about the explicit interface keyword .
You can create an abstract class to be extended (or implemented) by a concrete class. Abstract classes can contain abstract methods (with empty bodies).
Any class extending Describable has the describeWithEmphasis() method, which calls the extender's implementation of describe() .
Read more about abstract classes and methods.
Avoid callback hell and make your code much more readable by using async and await .
The method above is equivalent to:
As the next example shows, async and await help make asynchronous code easy to read.
You can also use async* , which gives you a nice, readable way to build streams.
Read more about asynchrony support, including async functions, Future , Stream , and the asynchronous loop ( await for ).
To raise an exception, use throw :
To catch an exception, use a try statement with on or catch (or both):
Note that the code above is asynchronous; try works for both synchronous code and code in an async function.
Read more about exceptions, including stack traces, rethrow , and the difference between Error and Exception .
As you continue to learn about the Dart language, keep these facts and concepts in mind:
Everything you can place in a variable is an object , and every object is an instance of a class . Even numbers, functions, and null are objects. With the exception of null (if you enable sound null safety ), all objects inherit from the Object class.
Although Dart is strongly typed, type annotations are optional because Dart can infer types. In var number = 101 , number is inferred to be of type int .
If you enable null safety , variables can't contain null unless you say they can. You can make a variable nullable by putting a question mark ( ? ) at the end of its type. For example, a variable of type int? might be an integer, or it might be null . If you know that an expression never evaluates to null but Dart disagrees, you can add ! to assert that it isn't null (and to throw an exception if it is). An example: int x = nullableButNotNullInt!
When you want to explicitly say that any type is allowed, use the type Object? (if you've enabled null safety), Object , orāif you must defer type checking until runtimeāthe special type dynamic .
Dart supports generic types, like List<int> (a list of integers) or List<Object> (a list of objects of any type).
Dart supports top-level functions (such as main() ), as well as functions tied to a class or object ( static and instance methods , respectively). You can also create functions within functions ( nested or local functions ).
Similarly, Dart supports top-level variables , as well as variables tied to a class or object (static and instance variables). Instance variables are sometimes known as fields or properties .
Unlike Java, Dart doesn't have the keywords public , protected , and private . If an identifier starts with an underscore ( _ ), it's private to its library. For details, see Libraries and imports .
Identifiers can start with a letter or underscore ( _ ), followed by any combination of those characters plus digits.
Dart has both expressions (which have runtime values) and statements (which don't). For example, the conditional expression condition ? expr1 : expr2 has a value of expr1 or expr2 . Compare that to an if-else statement , which has no value. A statement often contains one or more expressions, but an expression can't directly contain a statement.
Dart tools can report two kinds of problems: warnings and errors . Warnings are just indications that your code might not work, but they don't prevent your program from executing. Errors can be either compile-time or run-time. A compile-time error prevents the code from executing at all; a run-time error results in an exception being raised while the code executes.
You can find more documentation and code samples in the core library documentation and the Dart API reference . This site's code follows the conventions in the Dart style guide .
An Introduction to Operators
Letās overview the operators provided by Dart.
Types of operators
Operators and expressions.
Operators are symbols that perform operations used for modifying or manipulating data. Manipulating data is an essential part of any programming language, and Dart is no different, providing a rich set of operators for its basic types.
In this chapter, we are going to cover the following built-in operators in Dart:
- Arithmetic Operators
- Equality and Relational Operators
- Type Test Operators
- Assignment Operators
- Logical Operators
- Bitwise and Shift Operators
An Expression is a special type of statement that evaluates to some value. For instance, in mathematics, 1 + 1 is an expression because it evaluates to 2 . Expressions are composed of two things; operands and operators .
Operators usually follow an infix notation. The infix notation is where the operator is situated between two operands.
Create a free account to access the full course.
By signing up, you agree to Educative's Terms of Service and Privacy Policy
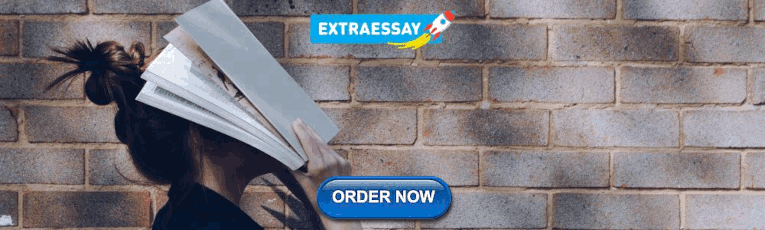
IMAGES
VIDEO
COMMENTS
Learn about the operators Dart supports. To test whether two objects x and y represent the same thing, use the == operator. (In the rare case where you need to know whether two objects are the exact same object, use the identical() function instead.) Here's how the == operator works:. If x or y is null, return true if both are null, and false if only one is null.
8. Assignment Operators in Dart 8.1 Simple Assignment (=) The simple assignment operator (=) assigns a value to a variable. Example: int a = 5; int b; b = a; print(b); // Output: 5 8.2 Compound Assignment (+=, *=, etc.) Compound assignment operators combine an operation with an assignment. They offer a concise way to perform an operation and ...
Compound Assignment Operator. Apart from there is another way where we can use a operator that is compound assignment operator where we combine an operator with an assignment operatorso to shorten the steps and make code more effective. Example: a+=1; // The above statement is same as // the statement mentioned below a=a+1; 6. Logical Operators
Get introduced to assignment operators in Dart. We'll cover the following. Overview. Compound assignment operators. Syntax. Examples. The += operator. The &= operator. The ~/= operator.
š Welcome to our latest tutorial in the Complete Flutter Development with AI Course series! In this session, we delve into the fundamental concept of the "A...
what's the meaning of this assignment operator? example in Flutter source code. flutter; dart; operators; Share. Improve this question. Follow edited Nov 2, 2020 at 9:48. Hashem Aboonajmi. asked Nov 2, 2020 at 9:04. Hashem Aboonajmi Hashem Aboonajmi.
In this video, you'll learn everything you need to know about arithmetic and assignment operators in Flutter.We'll cover the basics of how to perform arithme...
Unit 02 | Lesson 04 | Compound Assignment Operators | FlutteršNotes for this tutorialYou can find all the Codes that we discuss in the lessons with the Ques...
7. ~/. Dart also has a few operators for speeding up arithmetic operations. The ~/ operator divides and returns the floored (integer part) of the result. These were a few operators which simplify ...
The Basic. The conditional (ternary) operator is just a Dart operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. The syntax: condition ? exprIfTrue : exprIfFalse. Where:
Dart offers an extensive set of operators, each with a distinct purpose. The above guide is intended to serve as a comprehensive reference for Dart developers seeking to understand and implement these operators effectively. By familiarizing themselves with the syntax and usage of these operators, developers can write concise and efficient code.
This page provides a brief introduction to the Dart language through samples of its main features. To learn more about the Dart language, visit the in-depth, individual topic pages listed under Language in the left side menu.. For coverage of Dart's core libraries, check out the core library documentation.You can also try the Dart cheatsheet codelab, for a more hands-on introduction.
An Expression is a special type of statement that evaluates to some value. For instance, in mathematics, 1 + 1 is an expression because it evaluates to 2 . Expressions are composed of two things; operands and operators. Operators usually follow an infix notation. The infix notation is where the operator is situated between two operands.
Dart, like many programming languages, supports a variety of operators that perform operations on variables and values. Here are some of the common operators in Dart: 1. Arithmetic Operators
The reason for this is that these operators still have a specific operator precedence. This caused the code above to first execute two * 0.5 and then execute the plus operation. Adding parentheses ...
I've seen this code and need an explanation for "??". I know ternary operators like "?" and then the true-condition and after ":" the false/else condition. But what me...
this operator is useful when you need to compare actual values of two objects/classses, because flutter by default Compares instances of objects and that case two objects will never be same even their actual values are same, For Example, ~just run following examples on DartPad.dev. Flutter default case: void main() {.
1. Like other languages, Flutter Dart also provides null-aware operators to make us deal with nullable variables. Let's start understanding them with this piece of code: Safe Navigation Operator ...