Understanding Python's slice assignment
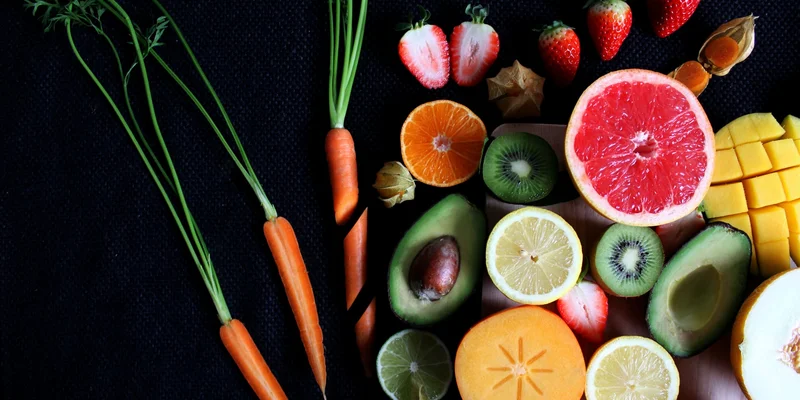
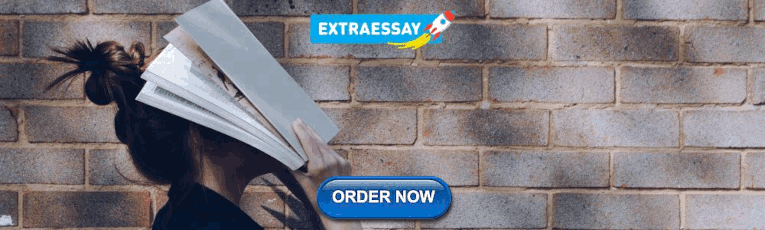
Python slice notation
Understanding python's slice notation.
- Understanding Python's slice assignment (this blog post)
Basic syntax
In order to understand Python's slice assignment, you should at least have a decent grasp of how slicing works. Here's a quick recap:
Where start_at is the index of the first item to be returned (included), stop_before is the index of the element before which to stop (not included) and step is the stride between any two items.
Slice assignment has the same syntax as slicing a list with the only exception that it's used on the left-hand side of an expression instead of the right-hand side. Since slicing returns a list, slice assignment requires a list (or other iterable). And, as the name implies, the right-hand side should be the value to assign to the slice on the left-hand side of the expression. For example:
Changing length
The part of the list returned by the slice on the left-hand side of the expression is the part of the list that's going to be changed by slice assignment. This means that you can use slice assignment to replace part of the list with a different list whose length is also different from the returned slice. For example:
If you take empty slices into account, you can also insert elements into a list without replacing anything in it. For example:
Using steps
Last but not least, step is also applicable in slice assignment and you can use it to replace elements that match the iteration after each stride. The only difference is that if step is not 1 , the inserted list must have the exact same length as that of the returned list slice. For example:
More like this
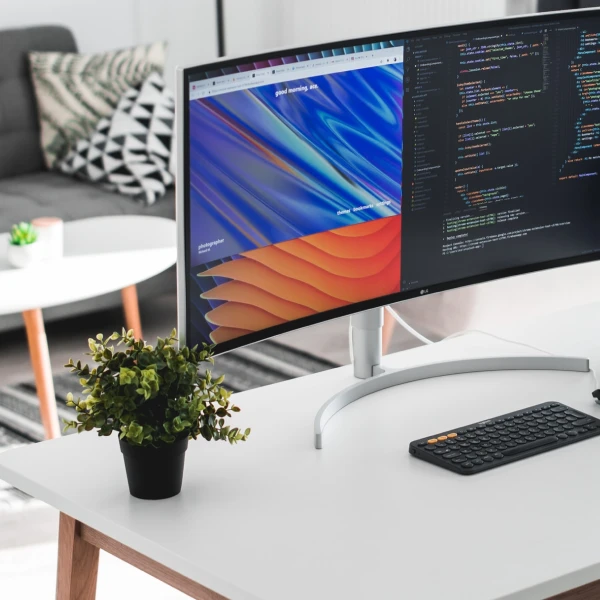
Python Lists
A snippet collection of list helpers and tips for Python 3.6.
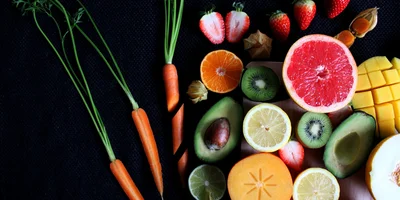
Learn everything you need to know about Python's slice notation with this handy guide.
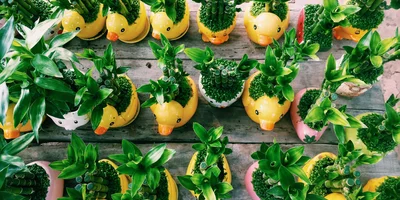
What is the difference between list.sort() and sorted() in Python?
Learn the difference between Python's built-in list sorting methods and when one is preferred over the other.
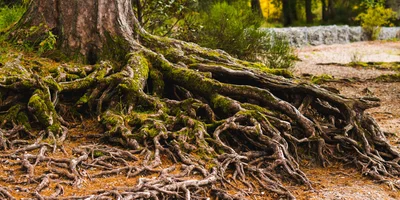
Find matches in a list or dictionary
Learn how to find the matching values, indexes or keys in a list or dictionary.
Start typing a keyphrase to see matching snippets.
CodeFatherTech
Learn to Code. Shape Your Future
Python List Slicing: How to Use It [With Simple Examples]
When writing a Python program you might want to access multiple elements in a list. In this scenario, Python list slicing can be very useful.
With Python’s list slicing notation you can select a subset of a list, for example, the beginning of a list up to a specific element or the end of a list starting from a given element. The slicing notation allows specifying the start index, stop index, and interval between elements (step) to select.
Slicing is a topic that can be a bit confusing for Python beginners and in this article, I will help you understand slicing based on my personal experience of using it in several Python applications.
Let’s see some examples of list slicing!
What Is List Slicing in Python?
In Python, you can use the colon character( : ) within square brackets to print part of a list (this is called slicing).
The first step to using slicing with a Python list is to understand the syntax for slicing:
The first important concept to know is that when you apply the slicing operator to a list you get back another list.
The syntax of slicing in Python supports the following arguments:
- start : the start index (inclusive)
- stop : the stop index (exclusive)
- step : the interval between elements returned in the slice
Based on my experience, one of the confusing aspects of slicing can be the fact that the start index is inclusive and the stop index is exclusive.
This is something you will have to remember and get used to to make sure you select the correct subset of a list.
6 Examples of List Slicing in Python
Let’s go through a few examples to explain how you can use the start , stop , and step arguments in the slicing notation .
Let’s take a list that contains the first 10 numbers of the Fibonacci sequence:
In the following sections, you will see the lists returned by the slicing operator when using different combinations of the start, stop, and step arguments.
1. Slice with Start and Stop Arguments
In this example, we specify where the slice starts and stops.
Start is equal to 2 and stop is equal to 5. The result is a slice of the original list that goes from index 2 (inclusive) to index 5 (exclusive).
2. Slice with Start Argument Only
When you only specify the start argument, the slice goes from the start index to the end of the list.
Considering that start is equal to 3 the slice goes from index 3 to the end of the original list.
3. Slice with Stop Argument Only
When you only specify the stop argument the slice goes from the beginning of the list to the stop index (exclusive).
Given that stop is equal to 4 the slice goes from the beginning of the original list to index 4 exclusive.
4. Slice with Start, Stop, and Step Arguments
You can combine the start, stop, and step arguments to have more control over the slice of the original list.
This is the first time we use the step argument in this tutorial, here is what it does:
In this example, start is equal to 1, stop is equal to 8 and step is equal to 2. This means that the slice goes from index 1 (inclusive) to index 8 (exclusive).
The fact that step is 2 means that the slice includes every other element of the list within the limits defined by start and stop.
5. Slicing with Positive Step Argument Only (without Start and Stop)
Let’s see what happens if you only use a positive step value with the list slicing operator.
Notice that the slice notation contains two colons followed by the value 2. That’s because there are no values for start/stop and the step is equal to 2.
The result is a slice that contains every other element in the original list.
6. Slice with Negative Step Argument Only
You can use an empty start, stop, and a negative value for the step argument (-1) to reverse the elements of a list.
This is similar to the result you can get using Python’s built-in reversed() function .
In this tutorial, you have learned what list slicing is in Python and how you can use different combinations of start, stop, and step values to obtain different slices.
How are you using slicing in your Python programs? Let me know in the comments below!
Related article : go through the following CodeFatherTech tutorial to learn about methods provided by Python lists .
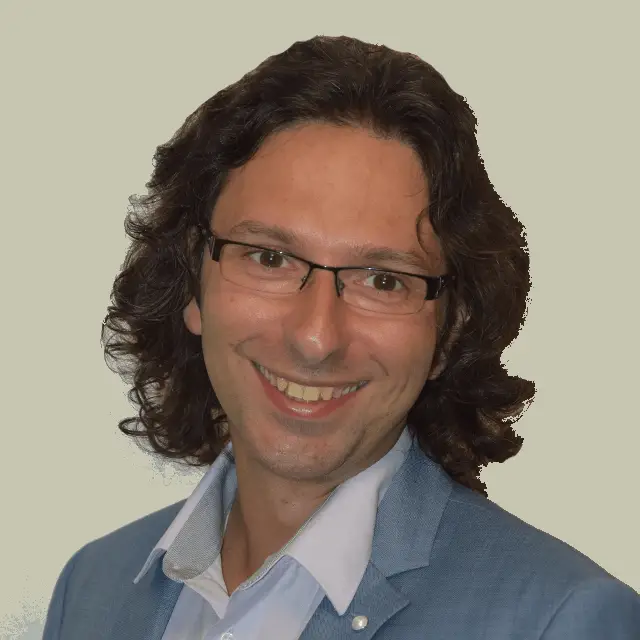
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute .
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
Related posts:
- 5 Ways to Copy a List in Python: Let’s Discover Them
- How Do You Get Every Other Element From a Python List?
- Unpack List in Python: A Beginner-Friendly Tutorial
- How to Create a List of Random Numbers in Python
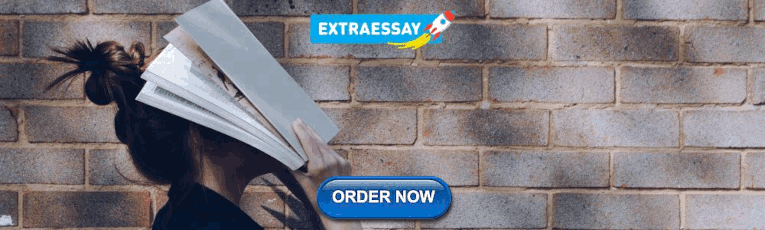
1 thought on “Python List Slicing: How to Use It [With Simple Examples]”
Thank you for sharing your knowledge!
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.

- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.
Python List Slicing
Imagine having a long loaf of bread. Sometimes, you want the whole thing, but other times you only need a few slices. List slicing in Python works in a similar way—it lets you extract specific portions of a list rather than working with the entire list at once. This is incredibly useful when dealing with large datasets, manipulating specific ranges of data, or simply extracting the information you need.
To perform slicing, you use square brackets [] along with a special syntax. This syntax involves specifying the starting index (where your slice begins), the stopping index (where it ends), and the step size (how many elements you skip between each included element). With this simple technique, you can access elements from the beginning, middle, or end of a list, modify, remove, or insert elements in a list, and even create copies of entire lists.
Throughout this tutorial, we’ll learn how this syntax works and explore examples of different slicing techniques. Let’s get started!
The basic syntax for slicing a list is:
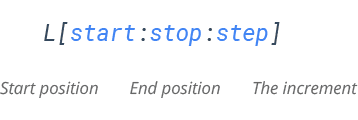
- start : The index at which the slice begins (inclusive). If omitted, the slice starts from the beginning of the list.
- stop : The index at which the slice ends (exclusive). If omitted, the slice goes up to the end of the list.
- step : The interval between elements in the slice. If omitted, the default step is 1.
So, if you have a list named L, writing L[start:stop:step] gives you a new list containing the elements from L starting at the start index, going up to (but not including) the stop index, and taking elements in steps of step .
Basic Slicing
Let’s look at a simple example of list slicing. Imagine you have a list of letters named L:
Now, let’s say you want to extract a portion of this list, specifically the elements from ‘c’ to ‘g’. You can do this using slicing:
In this example, we start at index 2, which corresponds to the letter ‘c’. Then we slice up to, but not including, index 7. This means we stop just before the letter ‘h’ at index 7. The result is a new list that contains the elements at indices 2 through 6, giving us the desired sequence of letters from ‘c’ to ‘g’.
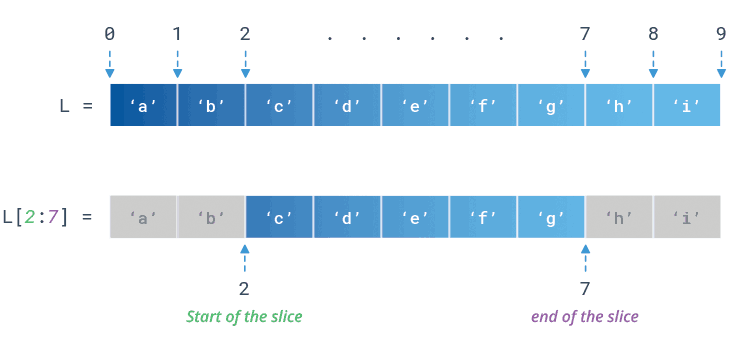
Slicing with Negative Indices
When slicing lists in Python, you can use negative indices as well. This allows you to reference elements from the end of the list. For example, the index -1 represents the last element, -2 represents the second-to-last element, and so on.
Consider the same list of letters. If you wanted to extract the elements from ‘c’ to ‘g’ using negative indices, you could write:
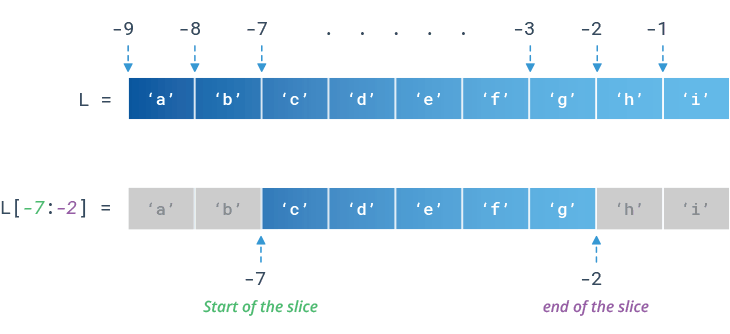
Slicing with Positive and Negative Indices
Python allows you to mix positive and negative indices within the same slice. This can be particularly useful when you want to select elements relative to both the beginning and the end of your list.
Slicing with a Step
In addition to specifying start and stop indices, slicing also allows you to introduce a step value, enabling you to extract elements at regular intervals.
Let’s take our familiar list of letters as an example. If you want to get every other element starting from index 2 (the letter ‘c’) and going up to, but not including, index 7, you can use a step value of 2:
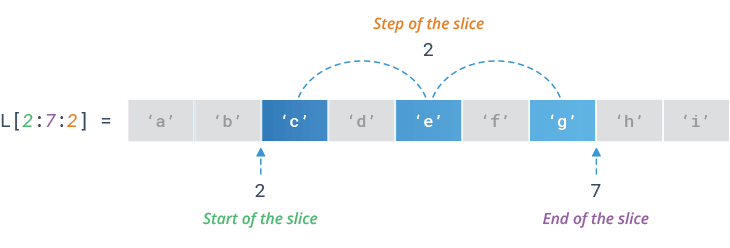
Negative Step
A negative step reverses the order of elements. In the example below, the slice starts at index 6 and ends before index 1, taking every second element in reverse order.
When using a negative step , ensure the start index is greater than the stop index.
Slice at the Beginning and to the End
When you omit the start index, the slice begins at the start of the list. So, L[:stop] is equivalent to L[0:stop] .
For example, to get the first three items of a list named L, you would use L[:3] .
On the other hand, when you omit the stop index, the slice goes up to the end of the list. So, L[start:] is equivalent to L[start:len(L)] .
For example, to obtain the last three items, you’d use L[6:] .
Reversing a List
Omitting both the start and stop indices while specifying a negative step value of -1 reverses the order of elements in the slice.
Replacing a Slice
Slicing can also be used to modify lists. You can replace elements within a list by assigning a new list to a slice of the original list.
For example, if you want to replace the elements from index 1 to 3 (inclusive) with the values 1, 2, and 3, you can do:
Interestingly, you can replace a single element with multiple elements using this technique.
Inserting Elements
You can efficiently insert new elements into a list without replacing existing ones by specifying a zero-length slice.
To insert elements at the beginning of a list, you can use a slice starting from the beginning ( L[:0] ) and assign the new elements to it.
If you want to insert elements at the end, you can use a slice that starts at the current length of the list ( L[len(L):] ) and assign the new elements there.
Inserting in the middle of the list is also possible. By using a slice with the same start and stop index, you can insert elements at the desired position within the list without overwriting any existing elements.
Removing a Slice
You can remove multiple elements from the middle of a list using slicing techniques. One way to do this is by assigning an empty list to the desired slice.
Alternatively, you can use the del statement to achieve the same result.
Copying the Entire List
In Python, when you assign one list to another (e.g., new_list = old_list ), you’re not creating a true copy. Instead, you’re creating a new reference that points to the same underlying list object. Any changes made to either new_list or old_list will affect both, as they share the same data.
To create an actual copy of the list, you can use the slicing operator. By omitting both the start and stop indices ( L1[:] ), you create a copy of the entire list L1. This means L2 is a new list object containing the same elements as L1, but changes to one list won’t affect the other.
It’s important to note that this slicing technique creates a shallow copy. This means a new list is made, but if the original list contains mutable objects (like nested lists or dictionaries), those objects are not duplicated. Both lists share references to the same mutable objects.
For deeper copies where nested mutable objects are also duplicated, you can use the list.copy() method or the copy.deepcopy() function (if you need to copy nested objects recursively).
Important Considerations
In list slicing, certain considerations are important to avoid unexpected behavior.
Out of Range Indices
When you use indices that go beyond the boundaries of a list, Python adjusts the indices to the nearest valid values instead of causing an error. For example:
Here, L[3:100] starts at index 3 and attempts to go up to index 100. Since the list ends before index 100, the slice includes all elements from index 3 to the end.
Empty Slice
If you try to create a slice where the starting index is the same as or greater than the stopping index (and the step is positive), you’ll get an empty list:
Step Size of 0
Specifying a step size of 0 is not allowed and will result in a ValueError :
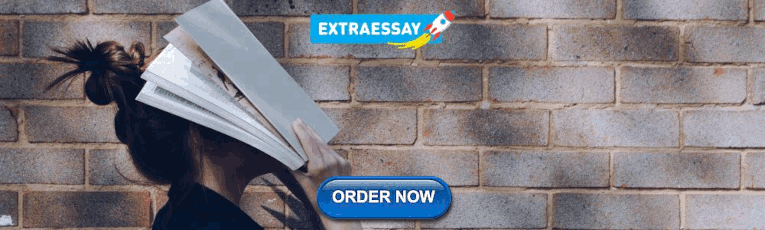