Home » PHP Tutorial » PHP Assignment Operators
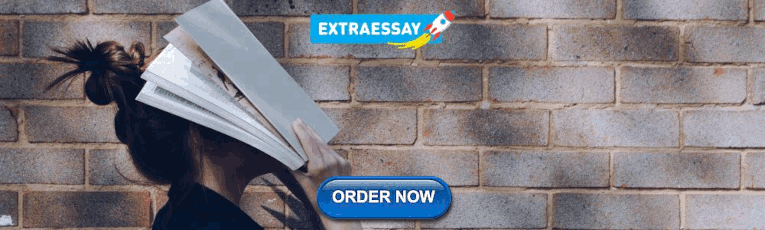
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- How to Concatenate Strings in PHP
Use the Concatenation Operator to Concatenation Strings in PHP
Use the concatenation assignment operator to concatenate strings in php, use the sprintf() function to concatenate strings in php.
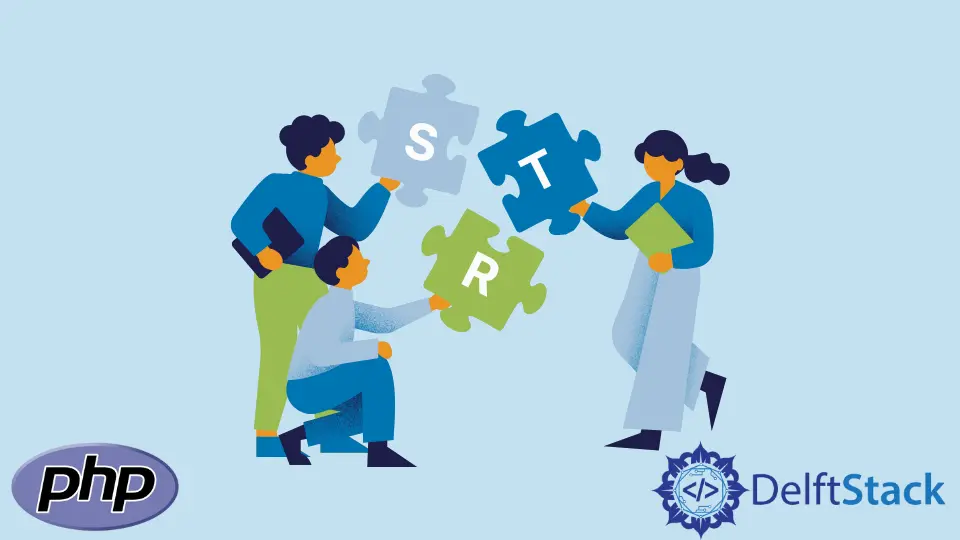
This article will introduce different methods to perform string concatenation in PHP.
The process of joining two strings together is called the concatenation process. In PHP, we can achieve this by using the concatenation operator. The concatenation operator is . . The correct syntax to use this operator is as follows.
The details of these variables are as follows.
The program below shows how we can use the concatenation operator to combine two strings.
Likewise, we can use this operator to combine multiple strings.
In PHP, we can also use the concatenation assignment operator to concatenate strings. The concatenation assignment operator is .= . The difference between .= and . is that the concatenation assignment operator .= appends the string on the right side. The correct syntax to use this operator is as follows.
The program below shows how we can use the concatenation assignment operator to combine two strings.
In PHP, we can also use the sprintf() function to concatenate strings. This function gives several formatting patterns to format strings. We can use this formatting to combine two strings. The correct syntax to use this function is as follows.
The function sprintf() accepts N+1 parameters. The detail of its parameters is as follows.
The function returns the formatted string. We will use the format %s %s to combine two strings. The program that combines two strings is as follows:
Related Article - PHP String
- How to Remove All Spaces Out of a String in PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert an Integer Into a String in PHP
- How to Convert an Array to a String in PHP
- How to Convert a String to a Number in PHP
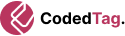
- PHP String Operators
In PHP, string operators, such as the concatenation operator (.) and its assignment variant (.=), are employed for manipulating and concatenating strings in PHP. This entails combining two or more strings. The concatenation assignment operator (.=) is particularly useful for appending the right operand to the left operand.
Let’s explore these operators in more detail:
Concatenation Operator (.)
The concatenation operator (.) is utilized to combine two strings. Here’s an example:
You can concatenate more than two strings by chaining multiple concatenation operations.
Let’s take a look at another pattern of the concatenation operator, specifically the concatenation assignment operator.
Concatenation Assignment Operator (.=)
The .= operator is a shorthand assignment operator that concatenates the right operand to the left operand and assigns the result to the left operand. This is particularly useful for building strings incrementally:
This is equivalent to $greeting = $greeting . " World!"; .
Let’s see some examples
Examples of Concatenating Strings in PHP
Here are some more advanced examples demonstrating the use of both the concatenation operator (.) and the concatenation assignment operator (.=) in PHP:
Concatenation Operator ( . ):
In this example, the . operator is used to concatenate multiple strings and variables into a single string.
Concatenation Assignment Operator ( .=) :
Here, the .= operator is used to append additional text to the existing string in the $paragraph variable. It is a convenient way to build up a string gradually.
Concatenation Within Iterations:
You can also use concatenation within iterations to build strings dynamically. Here’s an example using a loop to concatenate numbers from 1 to 5:
In this example, the .= operator is used within the for loop to concatenate the current number and a string to the existing $result string. The loop iterates from 1 to 5, building the final string. The rtrim function is then used to remove the trailing comma and space.
You can adapt this concept to various scenarios where you need to dynamically build strings within loops, such as constructing lists, sentences, or any other formatted output.
These examples showcase how you can use string concatenation operators in PHP to create more complex strings by combining variables, literals, iterations and other strings.
Let’s summarize it.
Wrapping Up
PHP provides powerful string operators that are essential for manipulating and concatenating strings. The primary concatenation operator (.) allows for the seamless combination of strings, while the concatenation assignment operator (.=) provides a convenient means of appending content to existing strings.
This versatility is demonstrated through various examples, including simple concatenation operations, the use of concatenation assignment for gradual string construction, and dynamic string building within iterations.
For more PHP tutorials, visit here or visit PHP Manual .
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Null Coalescing Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char
- Language Reference
Table of Contents
- Operator Precedence
- Increment and Decrement
- Error Control
An operator is something that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression).
Operators can be grouped according to the number of values they take. Unary operators take only one value, for example ! (the logical not operator ) or ++ (the increment operator ). Binary operators take two values, such as the familiar arithmetical operators + (plus) and - (minus), and the majority of PHP operators fall into this category. Finally, there is a single ternary operator , ? : , which takes three values; this is usually referred to simply as "the ternary operator" (although it could perhaps more properly be called the conditional operator).
A full list of PHP operators follows in the section Operator Precedence . The section also explains operator precedence and associativity, which govern exactly how expressions containing several different operators are evaluated.
Improve This Page
User contributed notes 4 notes.

Concatenate Strings in PHP
Php examples tutorial index.
Concatenating strings is a crucial concept in PHP that enables developers to merge two or more strings to create a single string. Understanding how to concatenate strings efficiently is vital in generating dynamic content, creating queries, and managing data output. In this tutorial, you will learn how to concatenate strings in PHP.
Understanding String Concatenation in PHP
String concatenation refers to joining two or more strings to create a new string. It is a typical and helpful operation in PHP, allowing developers to create customized and dynamic strings by combining different sources. The dot ( . ) operator performs this operation in PHP by joining the strings and creating a new string. This feature is simple and efficient and is commonly used in PHP scripting.
Basic Concatenation with the Dot Operator
The easiest and most common way to concatenate strings in PHP is to use the dot ( . ) operator. The dot operator takes two operands (the strings to be concatenated) and returns a new string as the result of concatenating them.
In the above example, we combine two variables ( $firstName and $lastName ) with a space between them to form a full name.
Concatenation with Assignment Operator
PHP makes string manipulation easier with the concatenation assignment operator ( .= ). This operator appends the right-side argument to the left-side argument, making it simple to expand an existing string.
The above method simplifies how to efficiently append text to an existing string variable, enhancing its content without redundancy.
Concatenating Multiple Variables and Strings
You can concatenate multiple variables and strings using multiple dot operators in a single statement. It is beneficial when you need to create a sentence or message from different data sources:
Dynamic Content Creation
String concatenation helps generate dynamic content and allows tailored user experiences based on specific inputs or conditions.
The above example illustrates how to create a personalized greeting message using concatenation dynamically.
In this tutorial, you have learned about the process of string concatenation in PHP, how to use the dot and assignment operators to join strings together, and some examples of string concatenation in PHP for different purposes. String concatenation is a crucial aspect of managing dynamic content and data output. Applying the best practices and techniques outlined in this tutorial, you can efficiently use string concatenation in your PHP projects.
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
Concatenate strings in PHP
This article demonstrates how to concatenate strings in PHP.
1. Using Concatenation Operator
Unlike other programming languages, which use the + operator for concatenating two strings, PHP uses the . concatenation operator for string concatenation. It returns the concatenation of its right and left arguments.
Download Run Code
Consider using the concatenating assignment operator ( .= ) to append one string to another. It appends the argument on the right side to the argument on the left side.
2. Using Variable Interpolation
The string concatenation using the . operator in PHP might result in performance overhead from constructing lots of intermediate strings along the way. For concatenating more than two strings, variable interpolation is a better choice. Using the curly braces syntax, you can interpolate a variable in a string literal. When a double-quoted string is processed by PHP, any interpolated variables will be parsed and replaced with their corresponding values.
It should be noted that the variable interpolation will only work in double-quoted strings. Interpolating a variable into a single-quoted string will result in the literal name of the supplied variable. For example, the following code tries to interpolate variables into a string literal enclosed in single quotes.
The above syntax encloses the variable name in curly braces for better visibility. However, it is also possible to interpolate variables within a string literal with double quotes without curly braces:
3. Using Heredoc syntax
Another option to concatenate strings in PHP is the Heredoc text. It behaves like a double-quoted string, without the double quotes. It has the syntax: <<< which is followed by an identifier, a newline, and the string itself. Finally, the same identifier is provided to close the quotation.
4. Using sprintf() function
If you want to apply some formatting options to the strings, you can use the sprintf() function for string concatenation. This is likely to run slower than all of the above proposed solutions. The printf() function can also be used if you want to output the result.
5. Using echo with commas
Finally, you can directly pass your strings to the echo statement, separated by commas ( , ). This will result in a minor speed improvement over the string concatenation operator ( . ), but will output the results and not assign them to a variable.
That’s all about concatenating strings in PHP.
Convert an integer or a float to a string in PHP
Rate this post
Average rating 5 /5. Vote count: 1
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
- How to Concatenate Strings in PHP ?
- How to Concatenate Strings in JavaScript ?
- How to Concatenate Two Arrays in PHP ?
- C++ program to concatenate a string given number of times
- PHP | is_string() Function
- How to append a string in PHP ?
- How to read each character of a string in PHP ?
- How to convert array to string in PHP ?
- How to get String Length in PHP ?
- How to convert a String to Lowercase in PHP?
- How to convert an Integer Into a String in PHP ?
- Concatenating Two Strings in C
- Python - Concatenation of two String Tuples
- String Concatenation in C++
- String concatenation in Scala
- String concatenation in Julia
- Python String Concatenation
- How to Optimize String Concatenation in Java?
- String Concatenation in R Programming
- How to Concatenate Multiple Strings in Java?
- How to Concatenate Strings in Swift?
- Shell Script to Concatenate Two Strings
- Python - Horizontal Concatenation of Multiline Strings
- Python - Triple quote String concatenation
- Batch Script - String Concatenation
- Concatenate Two Strings in R programming - paste() method
- How to Append or Concatenate Strings in Dart?
- How to Concatenate Multiple C++ Strings on One Line?
- Element-wise concatenation of two NumPy arrays of string
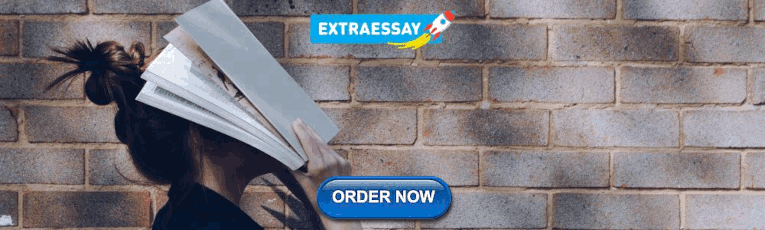
Concatenation of two strings in PHP
There are two string operators. The first is the concatenation operator (‘ . ‘), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (‘ .= ‘), which appends the argument on the right side to the argument on the left side.
Code #1:
Time complexity : O(n) Auxiliary Space : O(n)
Code #2 :
Code #3 :
Code #4 :
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples .
Please Login to comment...
Similar reads.
- Web Technologies

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Popular Articles
- Php Remove Last Character From String (Nov 08, 2023)
- Php Reset (Nov 08, 2023)
- Php Optional Parameters (Nov 08, 2023)
- Php Null (Nov 08, 2023)
- Php Join Array (Nov 08, 2023)
PHP Concat String
Switch to English
Table of Contents
Understanding String Concatenation in PHP
Concatenating multiple strings, the concatenation assignment operator, common pitfalls and how to avoid them.
Introduction When you're working with PHP, one of the fundamental tasks you'll encounter is manipulating strings. PHP is a server-side scripting language, and strings are used in virtually every application. A crucial facet of this string manipulation is 'string concatenation'. In the realm of programming, 'concatenation' essentially means the operation of joining two or more strings end-to-end.
- String concatenation in PHP is achieved using the '.' operator. This operator allows you to combine two or more strings to form a single string. The resulting string is a combination of the strings that were concatenated, in the order in which they were joined.
- More than two strings can be concatenated in a single statement. This is achieved by using the concatenation operator repeatedly.
- PHP also provides a concatenation assignment operator '.='. This operator appends the argument on the right side to the argument on the left side.
- One common mistake is to try to concatenate strings using the '+' operator. This works in some programming languages, but not in PHP. In PHP, the '+' operator is used for addition.
- Another common mistake is forgetting to include spaces when concatenating strings. If you don't include a space, the resulting string can be hard to read because the words run together.
String Operators in PHP : Tutorial
Concatenation operator, concatenation assignment operator (.=).
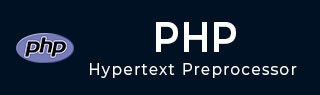
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP – String Operators
There are two operators in PHP for working with string data types: concatenation operator (".") and the concatenation assignment operator (".="). Read this chapter to learn how these operators work in PHP.
Concatenation Operator in PHP
The dot operator (".") is PHP's concatenation operator. It joins two string operands (characters of right hand string appended to left hand string) and returns a new string.
The following example shows how you can use the concatenation operator in PHP −
It will produce the following output −
Concatenation Assignment Operator in PHP
PHP also has the ".=" operator which can be termed as the concatenation assignment operator. It updates the string on its left by appending the characters of right hand operand.
The following example uses the concatenation assignment operator. Two string operands are concatenated returning the updated contents of string on the left side −
To Continue Learning Please Login
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php operators.
Operators are used to perform operations on variables and values.
PHP divides the operators in the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Advertisement
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
The PHP decrement operators are used to decrement a variable's value.
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
PHP String Operators
PHP has two operators that are specially designed for strings.
PHP Array Operators
The PHP array operators are used to compare arrays.
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:
PHP Exercises
Test yourself with exercises.
Multiply 10 with 5 , and output the result.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

PHP Tutorial
Control statement, php programs, php functions, php strings, php include, state management, upload download, php oops concepts, related tutorials.
Interview Questions
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

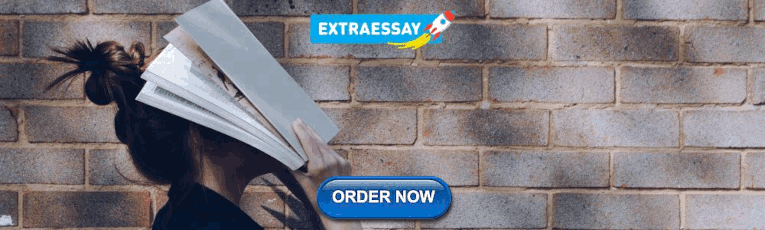
COMMENTS
There are two string operators. The first is the concatenation operator ('.'), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Please read Assignment Operators for more information.
PHP Compensation Operator is used to combine character strings. Operator. Description. . The PHP concatenation operator (.) is used to combine two string values to create one string. .=. Concatenation assignment.
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
String Concatenation. To concatenate, or combine, two strings you can use the . operator:
Use the Concatenation Assignment Operator to Concatenate Strings in PHP. In PHP, we can also use the concatenation assignment operator to concatenate strings. The concatenation assignment operator is .=. The difference between .= and . is that the concatenation assignment operator .= appends the string on the right side. The correct syntax to ...
In PHP, string operators, such as the concatenation operator (.) and its assignment variant (.=), are employed for manipulating and concatenating strings in PHP. This entails combining two or more strings. The concatenation assignment operator (.=) is particularly useful for appending the right operand to the left operand.
An operator is something that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression). Operators can be grouped according to the number of values they take. Unary operators take only one value, for example ! (the logical not operator) or ++ (the increment ...
String concatenation refers to joining two or more strings to create a new string. It is a typical and helpful operation in PHP, allowing developers to create customized and dynamic strings by combining different sources. The dot (.) operator performs this operation in PHP by joining the strings and creating a new string.
This article demonstrates how to concatenate strings in PHP. 1. Using Concatenation Operator. Unlike other programming languages, which use the + operator for concatenating two strings, PHP uses the . concatenation operator for string concatenation. It returns the concatenation of its right and left arguments. 1.
There are two string operators. The first is the concatenation operator ('. '), which returns the concatenation of its right and left arguments. The second is the concatenating assignment operator (' .= '), which appends the argument on the right side to the argument on the left side. Examples : string2 : World! Output : HelloWorld!
3. Because numbers with leading zeroes are treated as octals. if the concatenation would've included the leading zeroes, then 006 would've been interpretted as a string, which makes no sense, because it hasn't got quotes around it. If you want 006 to be treated as a string, write it as one: '006'. Leave it as is, and it'll be interpreted as an ...
Understanding String Concatenation in PHP. String concatenation in PHP is achieved using the '.' operator. This operator allows you to combine two or more strings to form a single string. The resulting string is a combination of the strings that were concatenated, in the order in which they were joined. For example, consider the following code ...
There are two String operators in PHP. 1. Concatenation Operator "." (dot) 2. Concatenation Assignment Operator ".=" (dot equals) Concatenation Operator Concatenation is the operation of joining two character strings/variables together. In PHP we use . (dot) to join two strings or variables. Below are some examples of string concatenation:
Two string operands are concatenated returning the updated contents of string on the left side −. It will produce the following output −. PHP - String Operators - There are two operators in PHP for working with string data types: concatenation operator (.) and the concatenation assignment operator (.=). Read this chapter to learn how ...
PHP Assignment Operators. The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
There are two types of string operators provided by PHP. 1. Concatenation Operator ("."): In PHP, this operator is used to combine the two string values and returns it as a new string. ... Let's take the various examples of how to use the Concatenation Assignment Operator (".=") to concatenate the strings in PHP. ...
PHP Concatenation assignment. Ask Question Asked 7 years, 10 months ago. Modified 7 years, 10 months ago. Viewed 455 times ... How do I use the .= operator within a loop, and any direction to tutorials to better understand this would be appreciated? php; Share. Improve this question.
With the "Concatenating Assignment Operator" I assigned in the loop two variables. I need to get each loop result separately. The problem is that I don't know why each next loop result is ... PHP 'foreach' array concatenate echo. 0. How to print multiple variables values using loop. 0. PHP Combine arrays in a loop. 1. Concatenate array on loop ...